Chapter 4 ClientSide Programming the Java Script Language
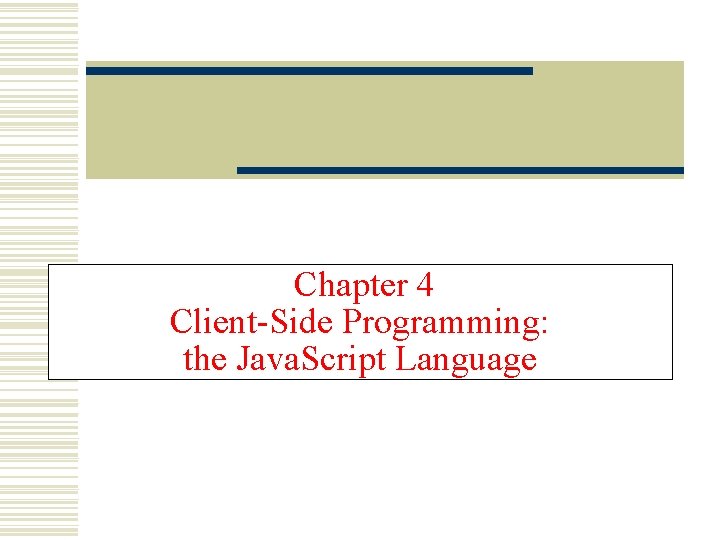
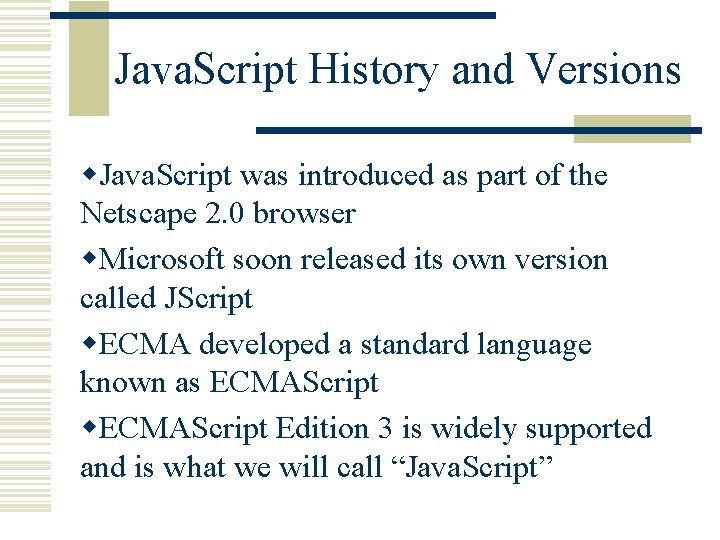
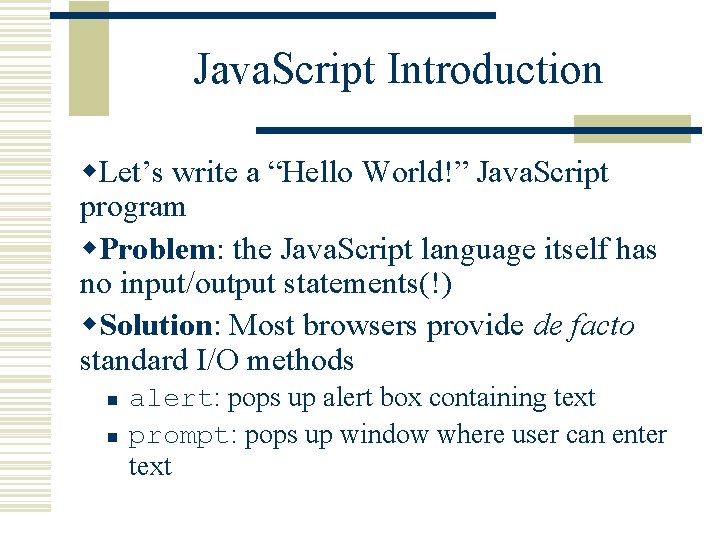
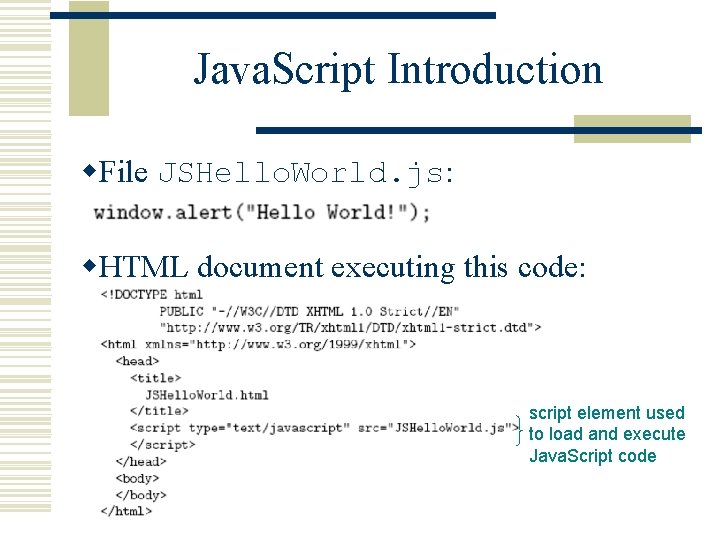
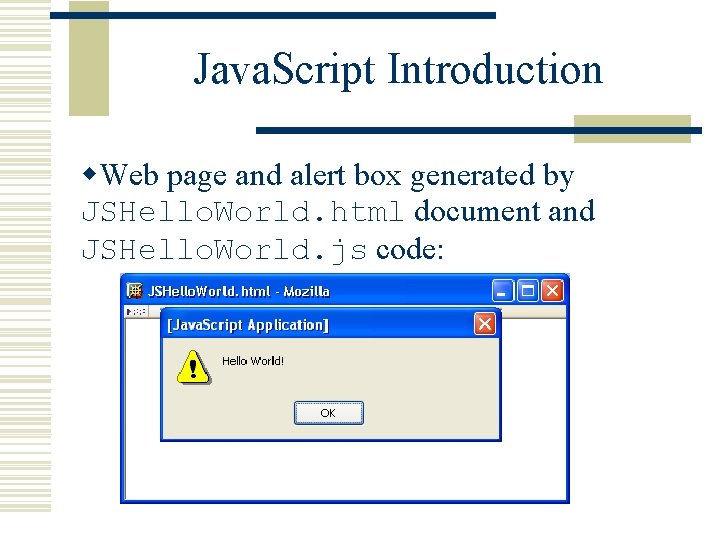
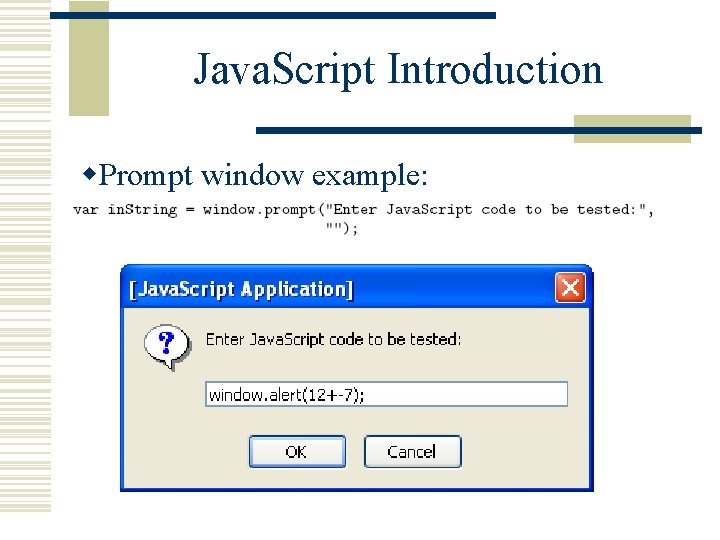
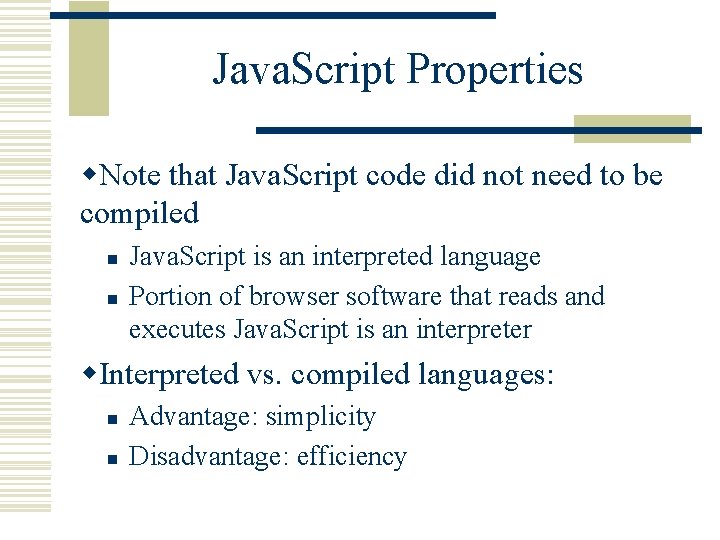
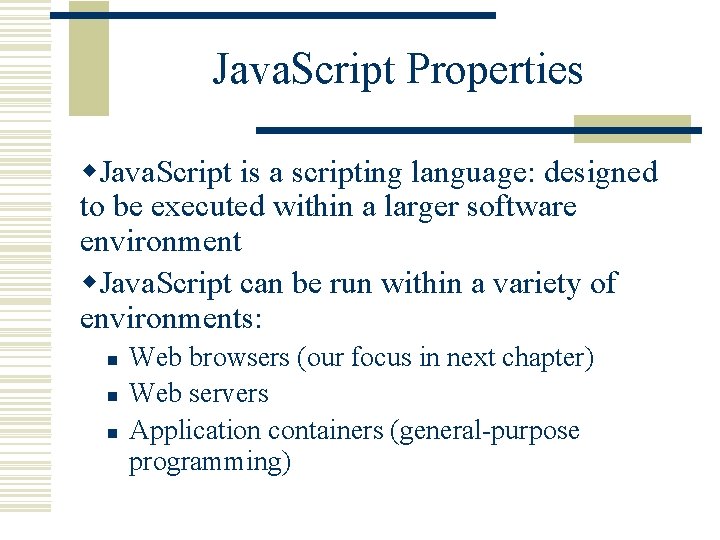
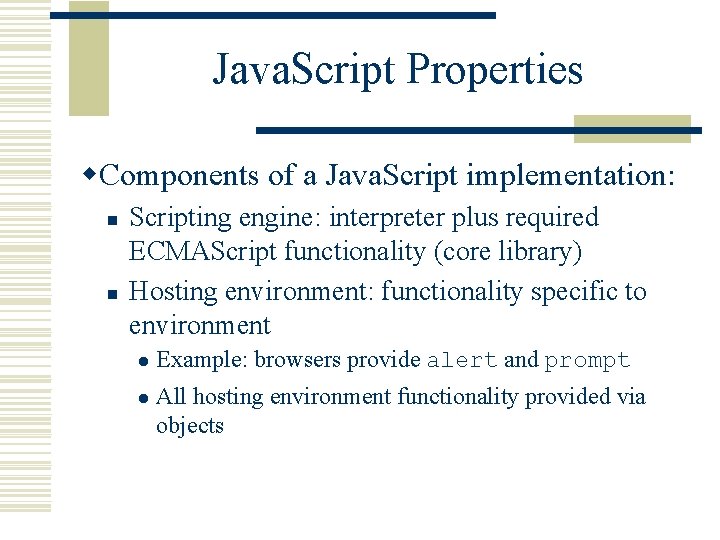
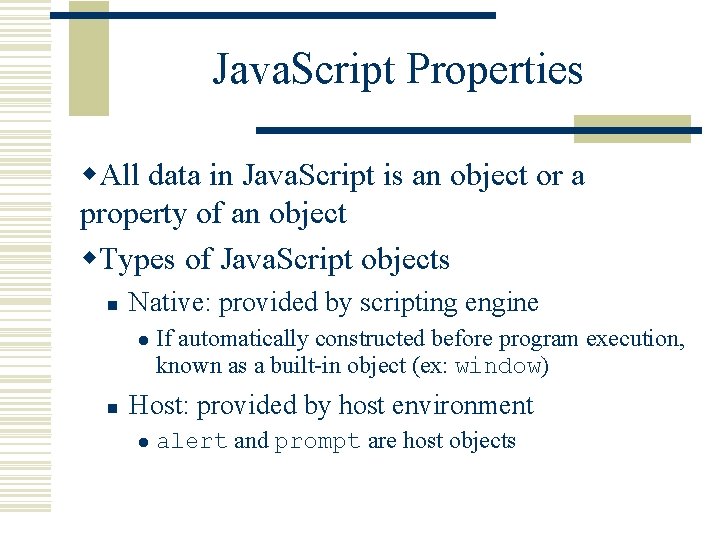
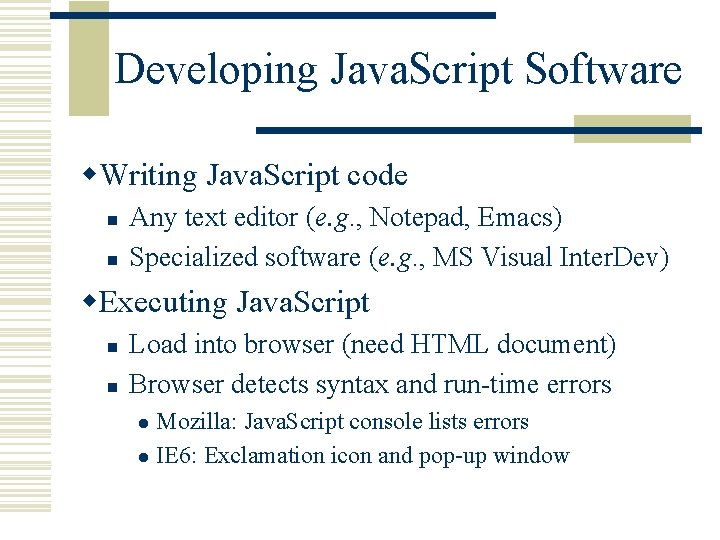
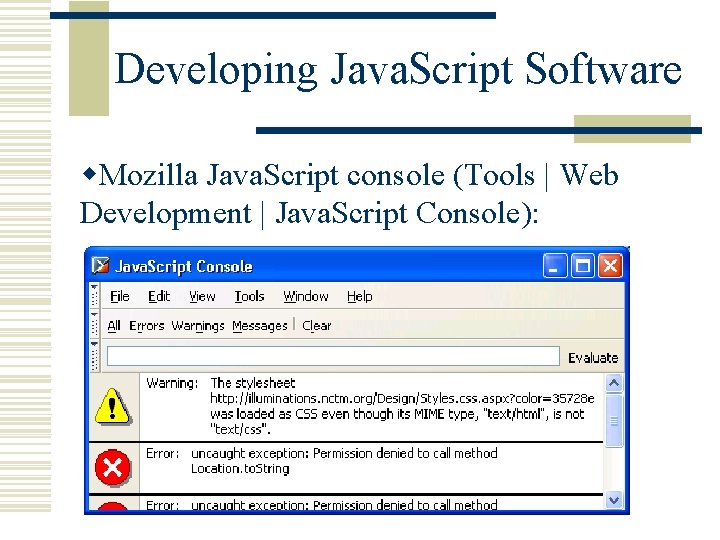
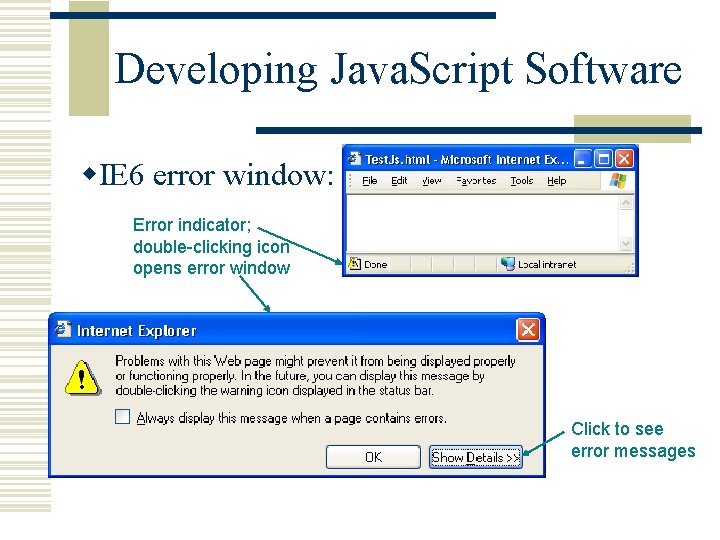
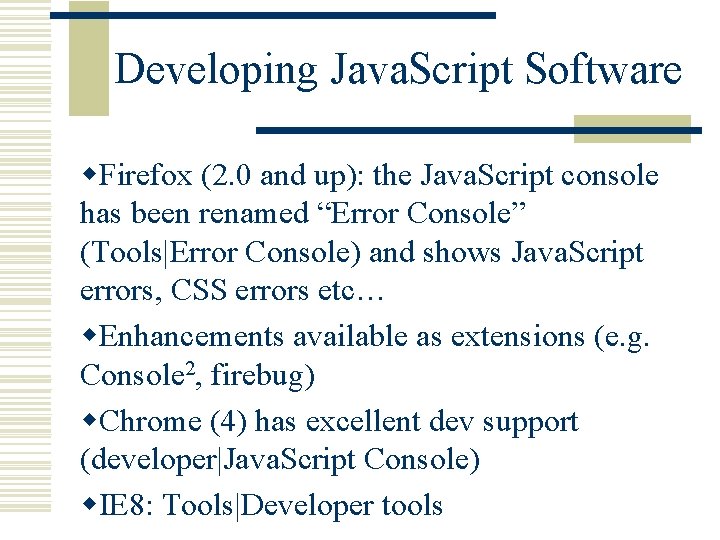
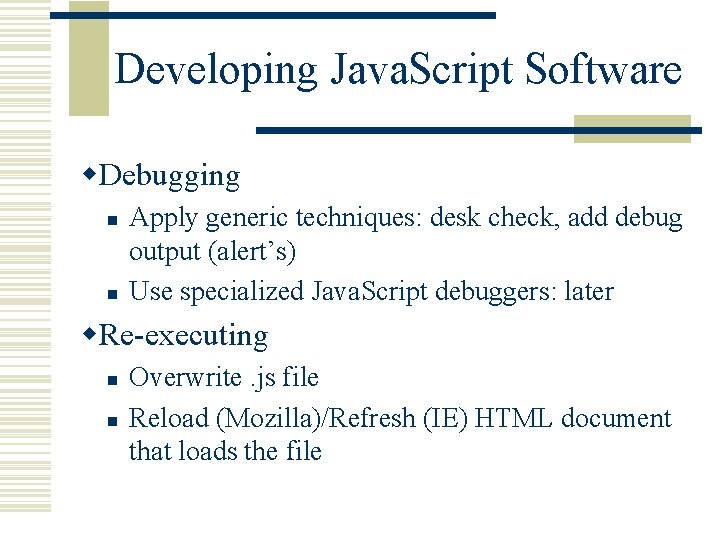
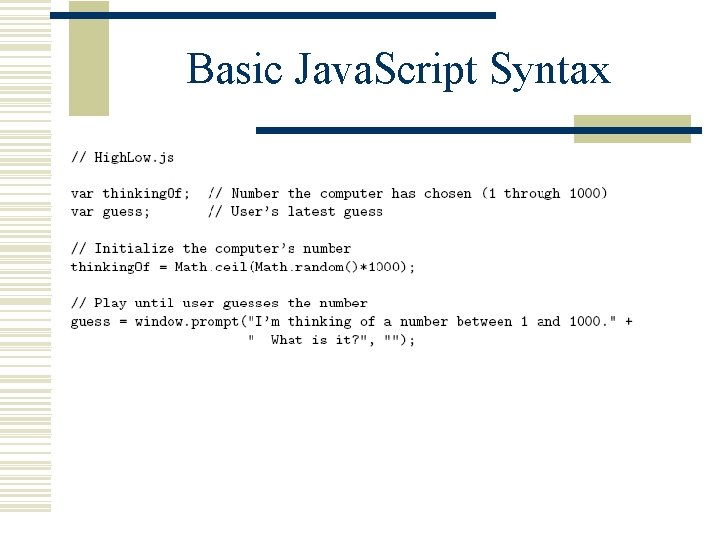
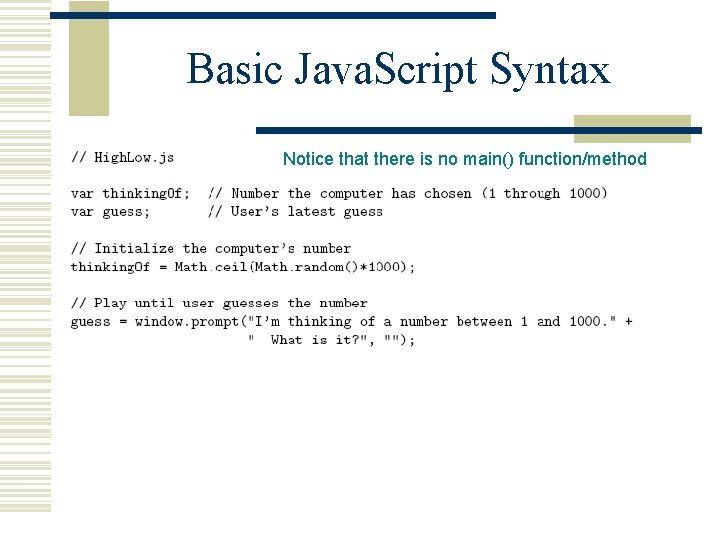
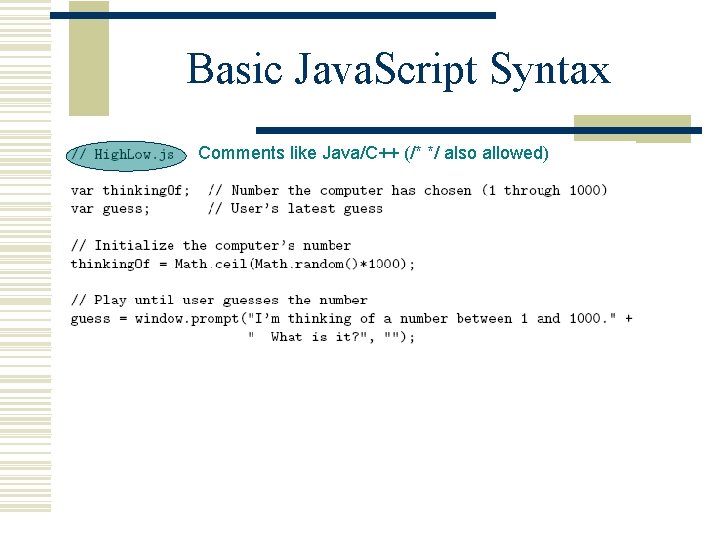
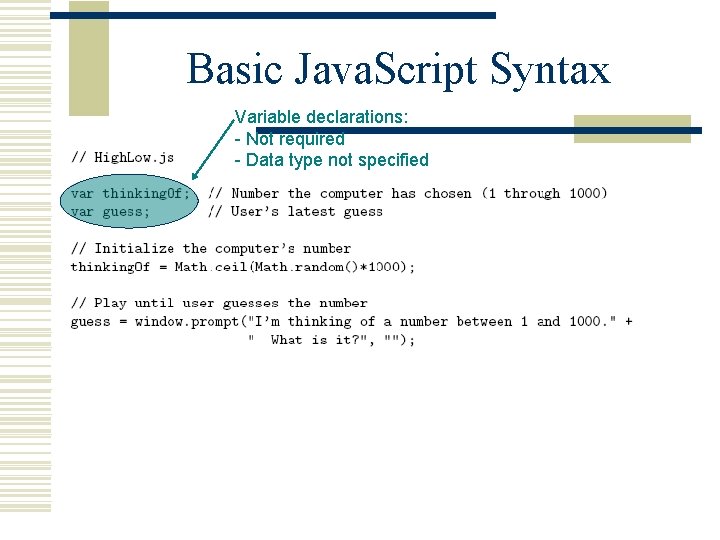
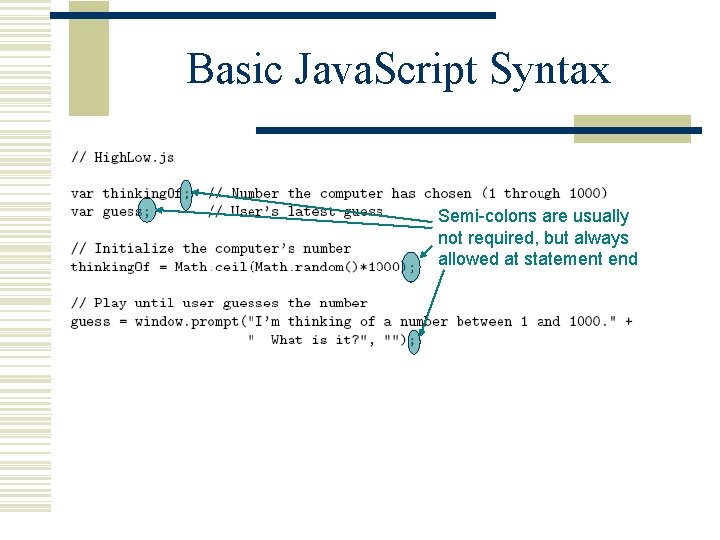
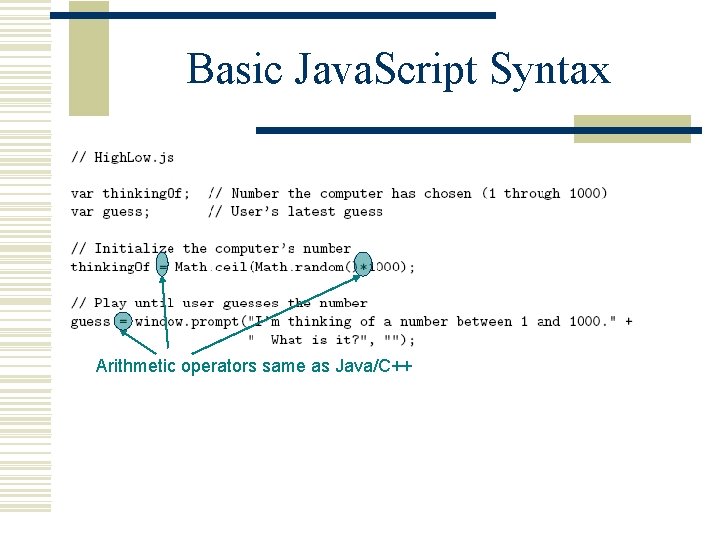
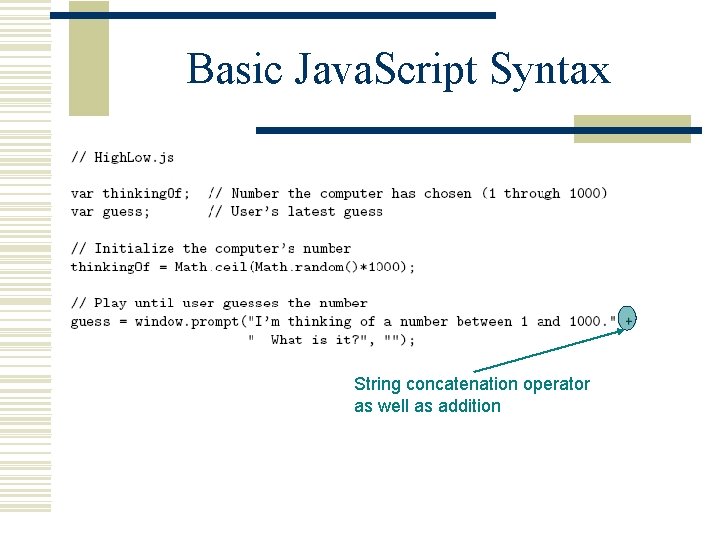
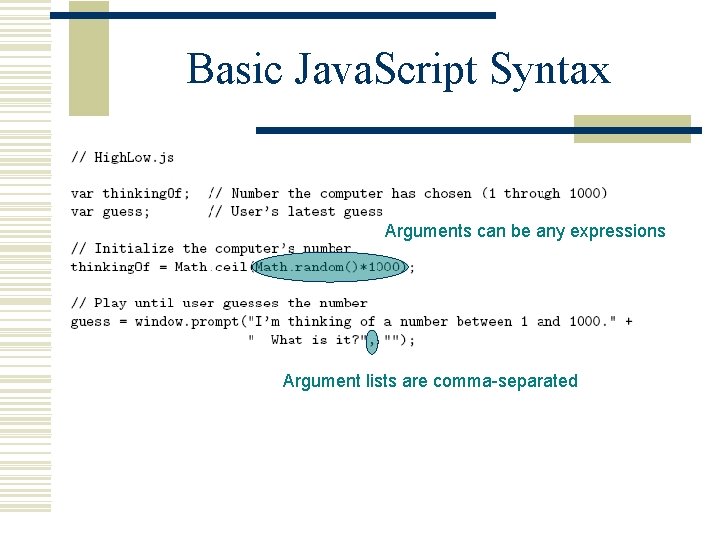
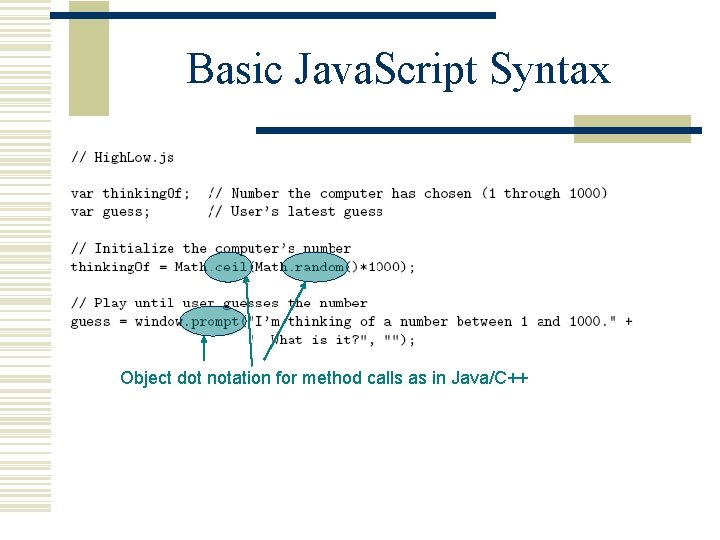
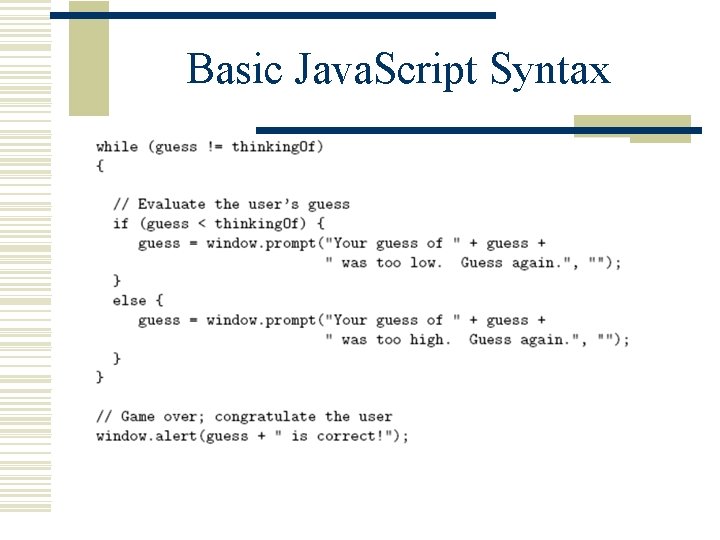
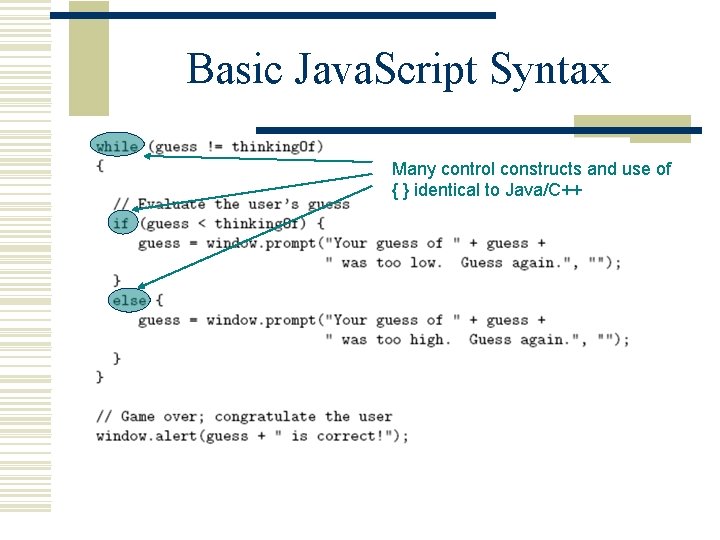
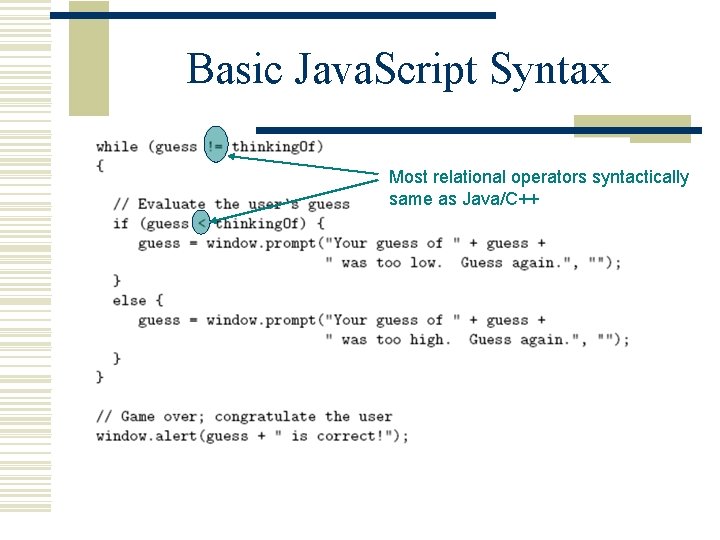
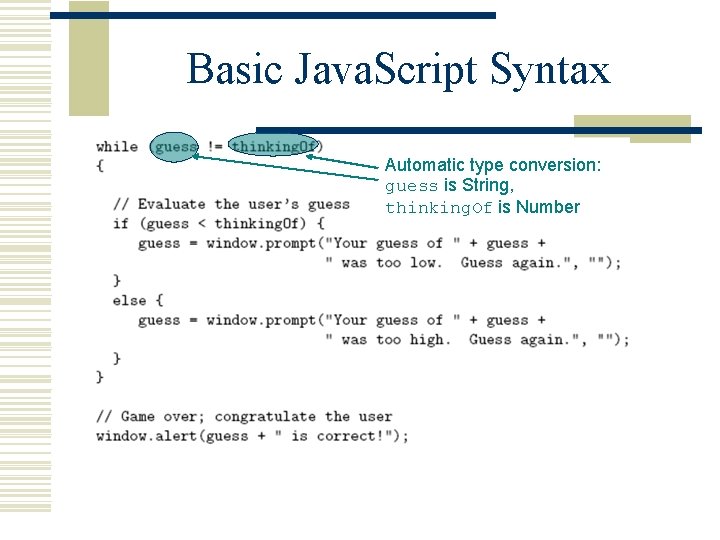
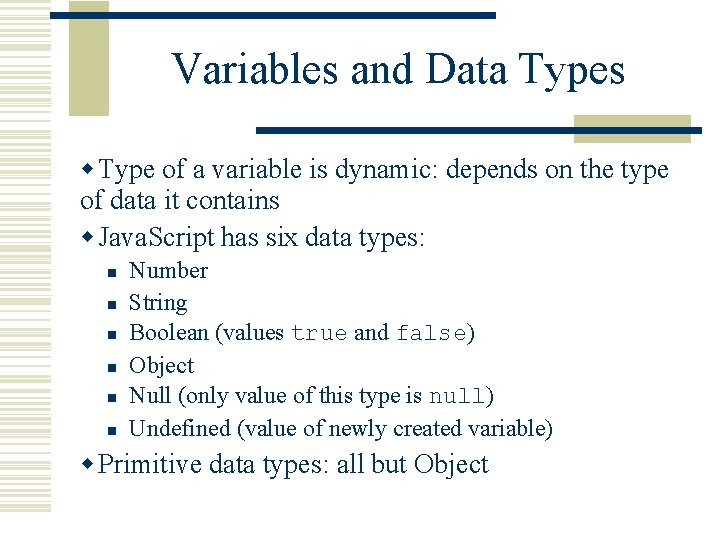
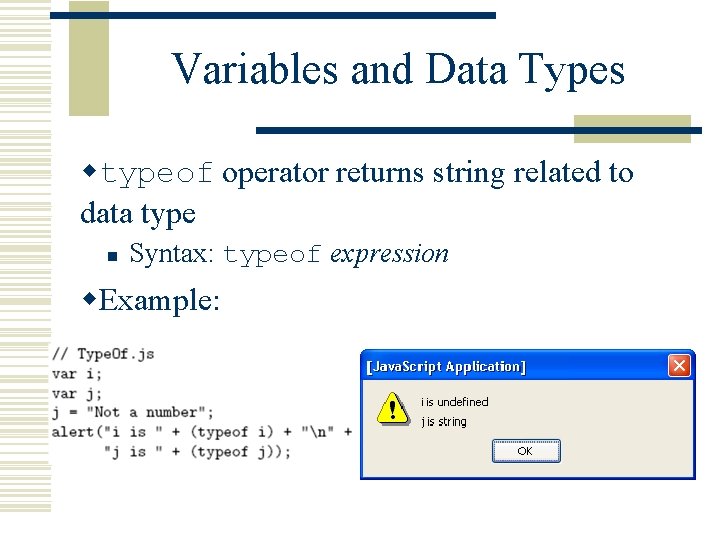
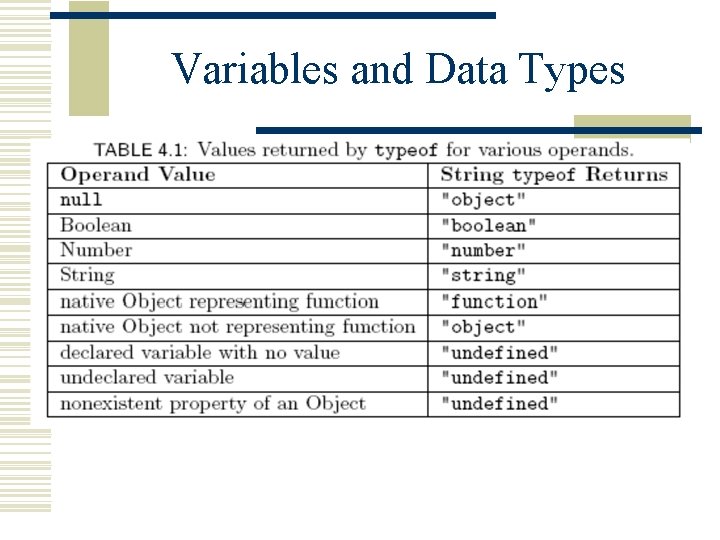
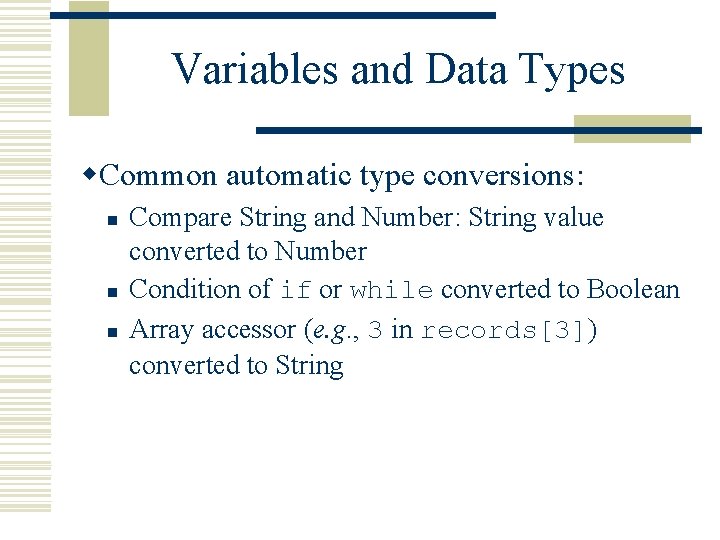
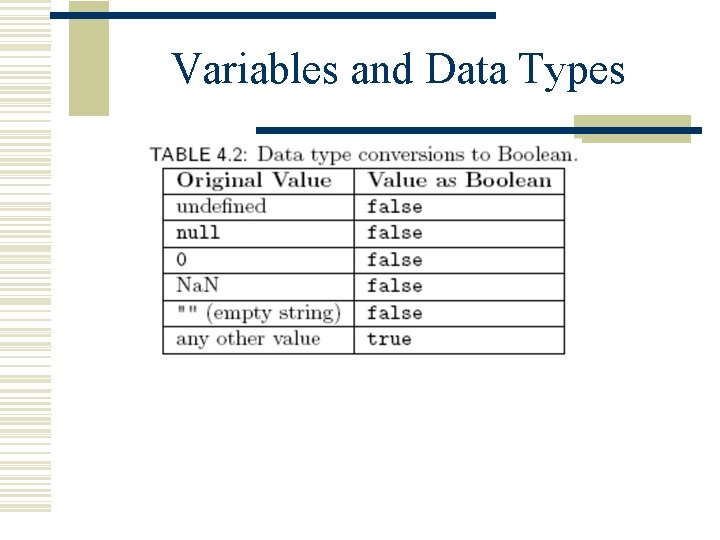
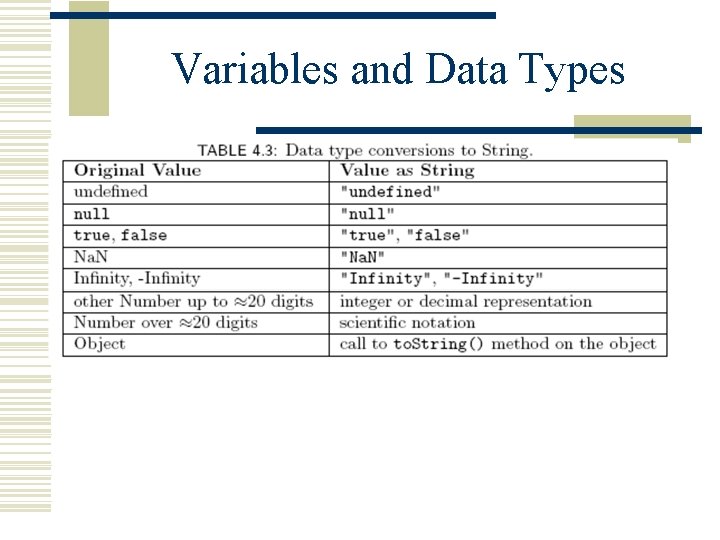
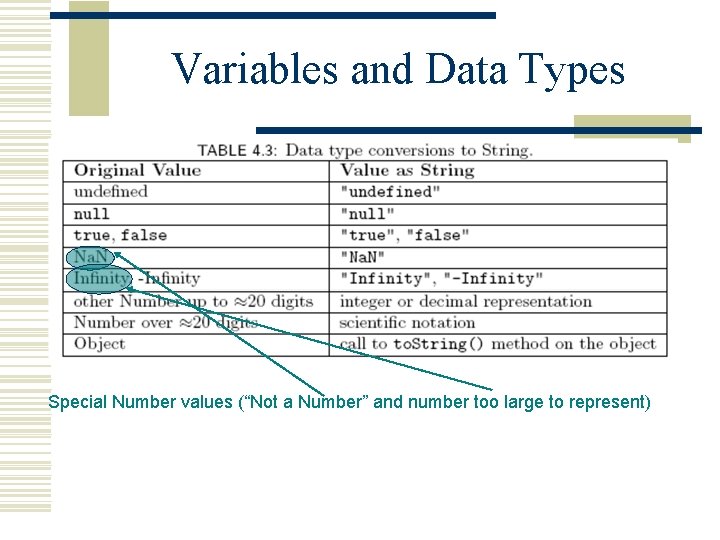
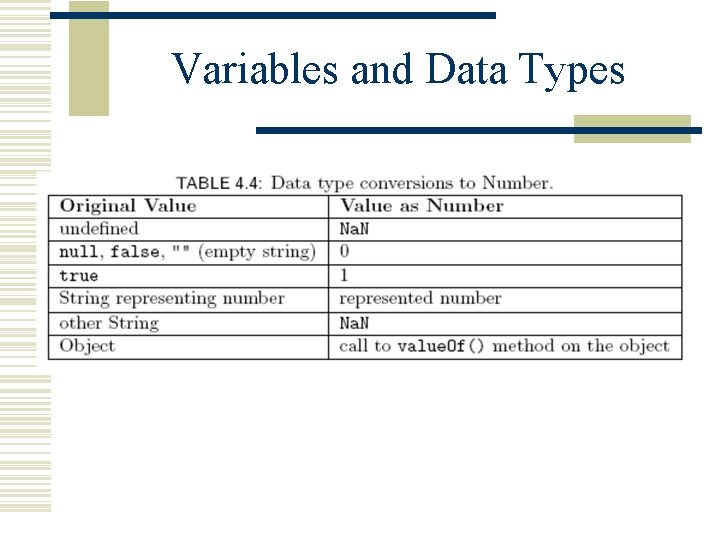
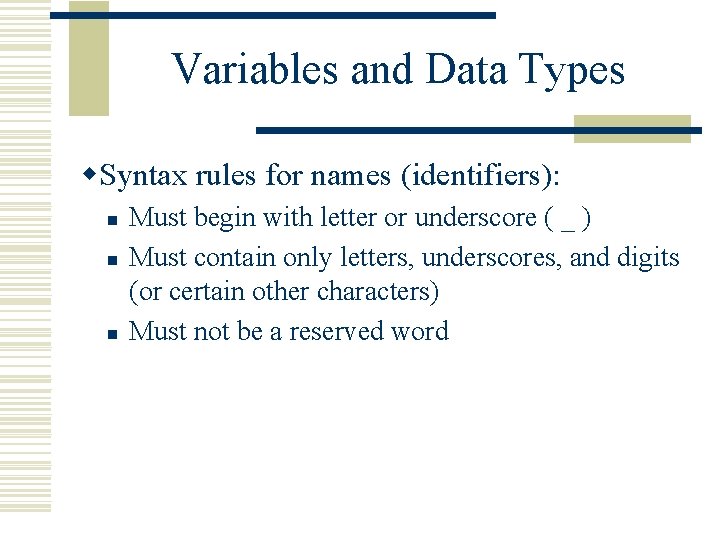
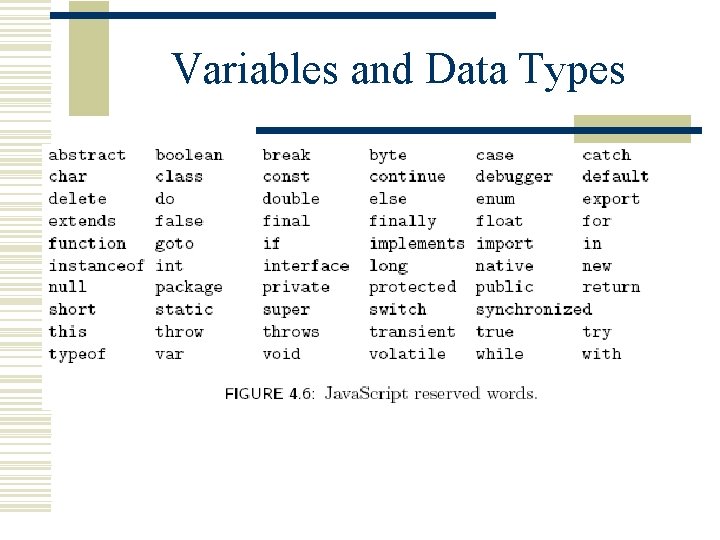
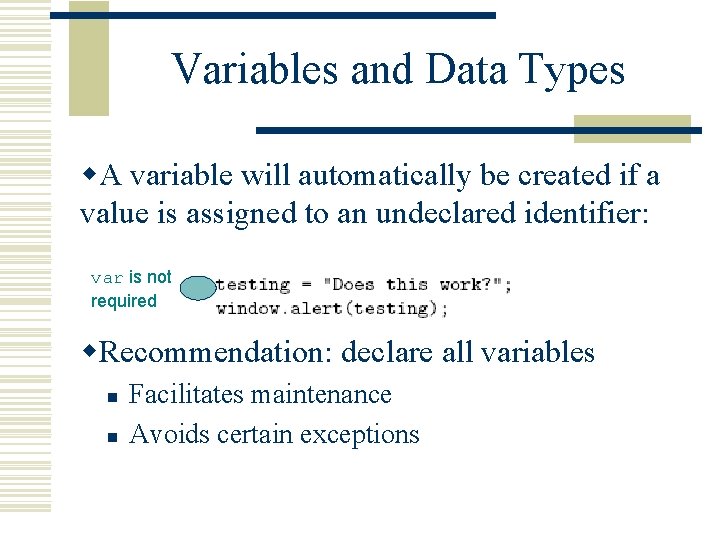
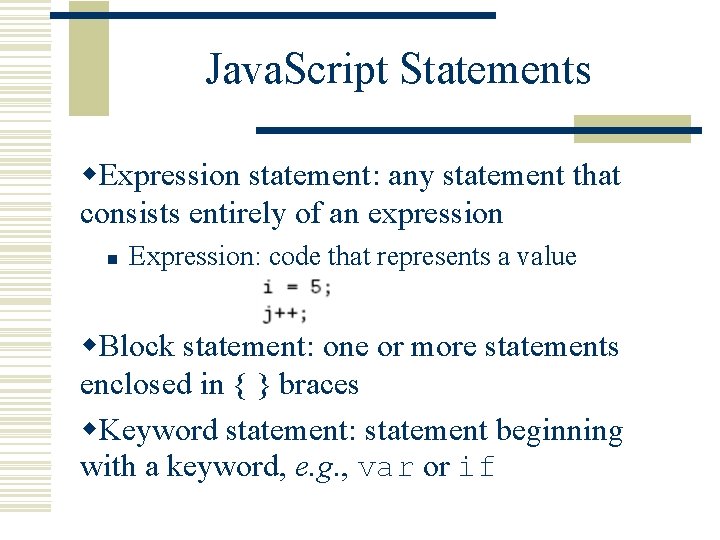
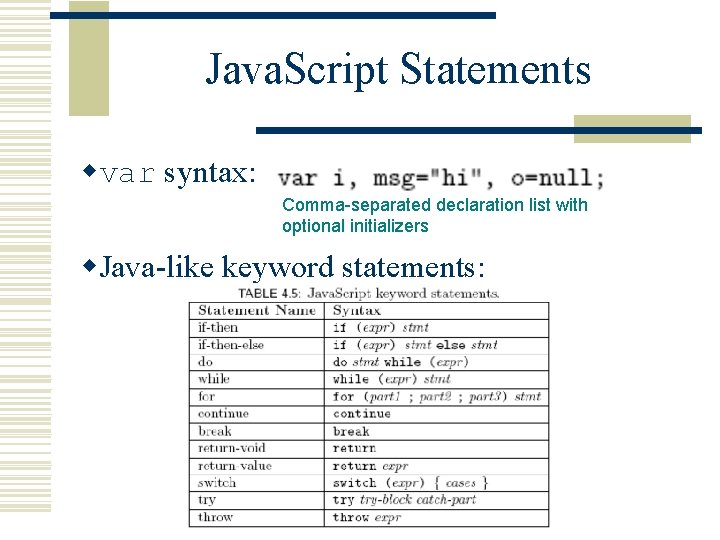
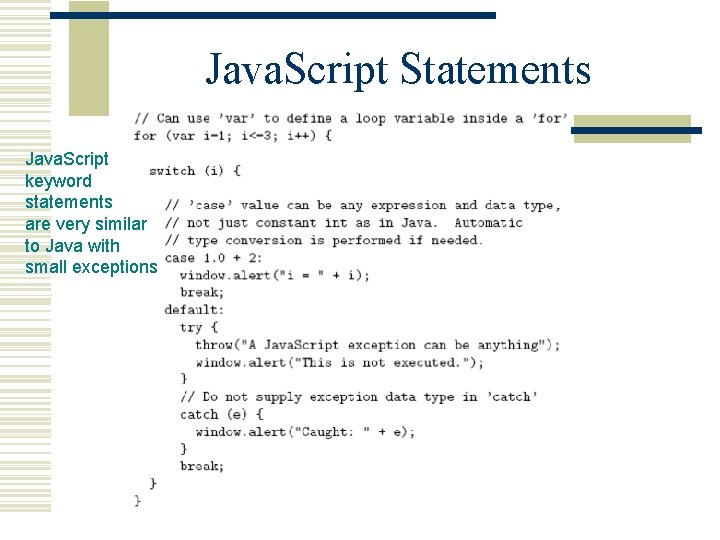
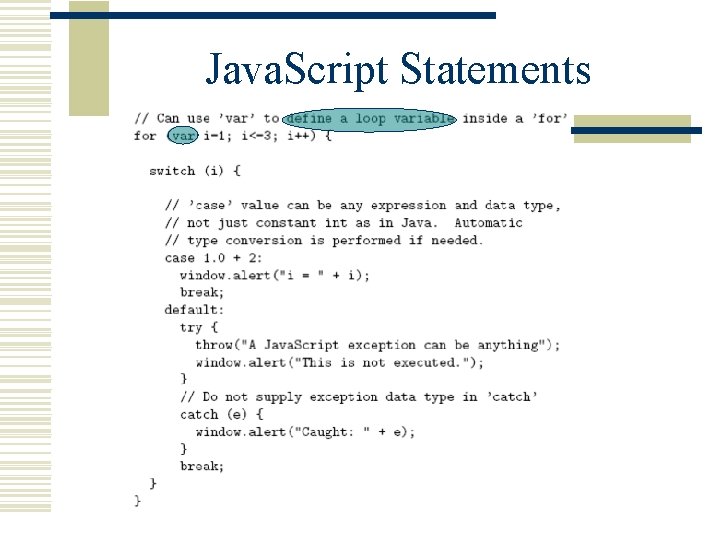
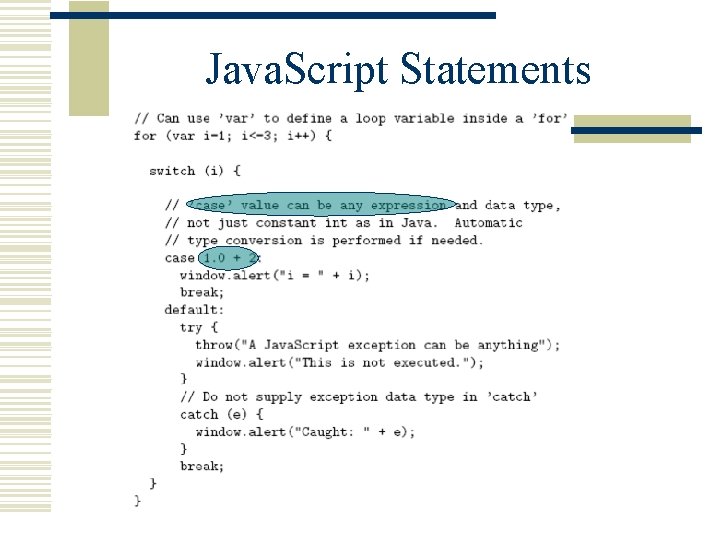
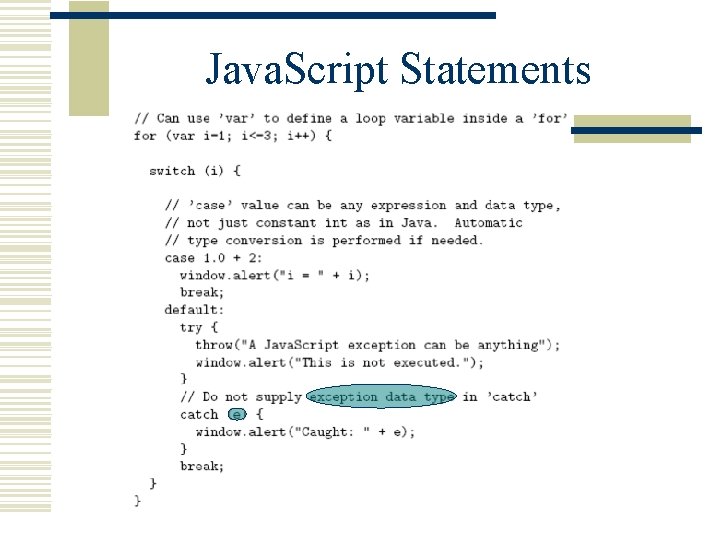
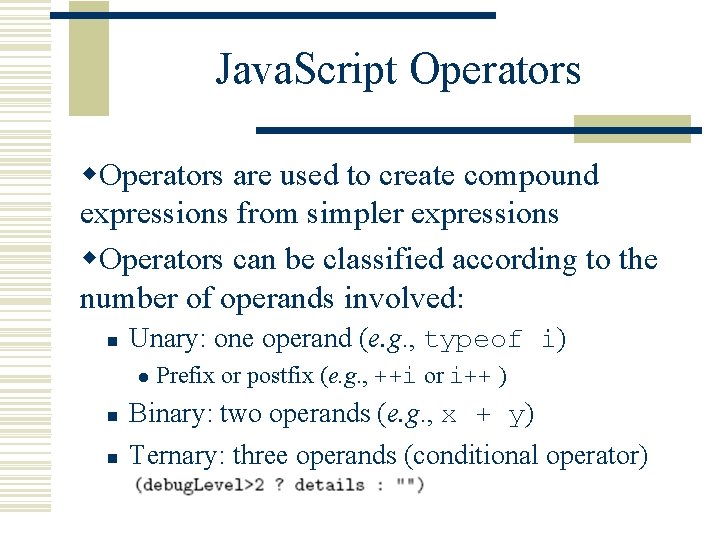
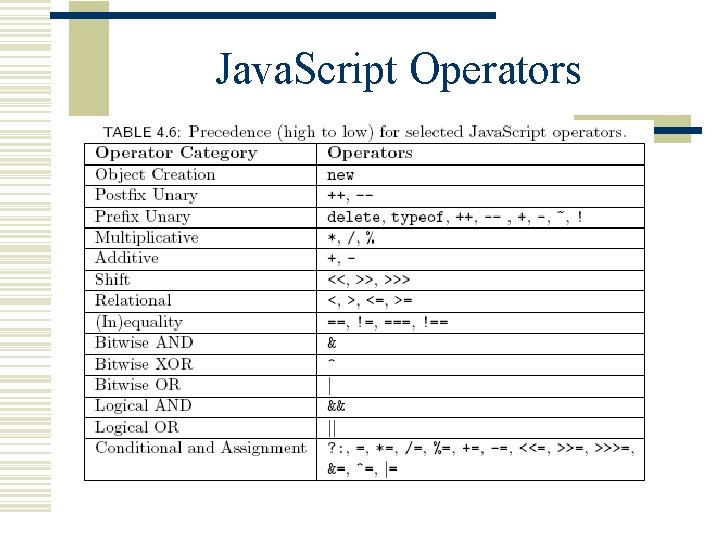
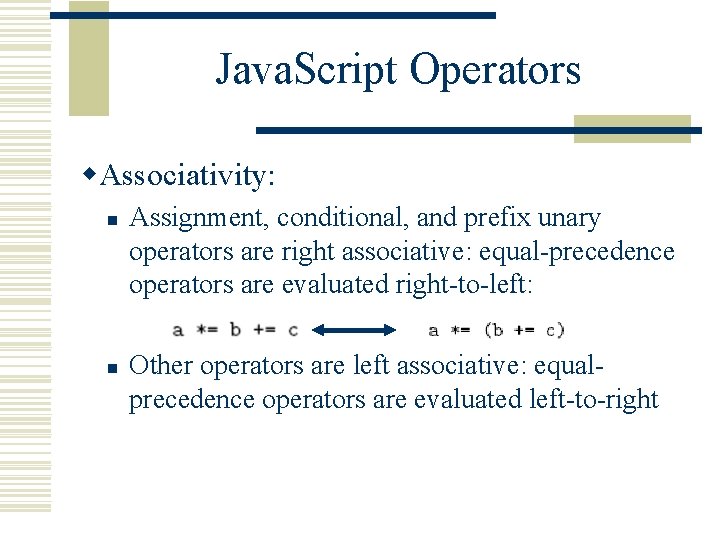
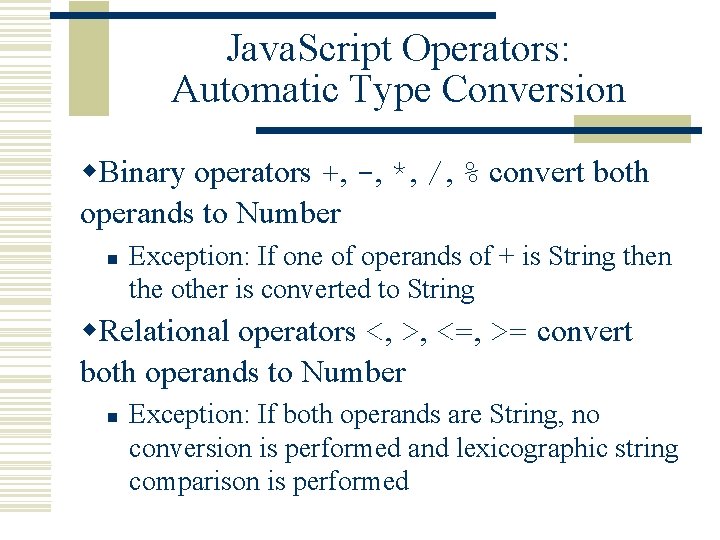
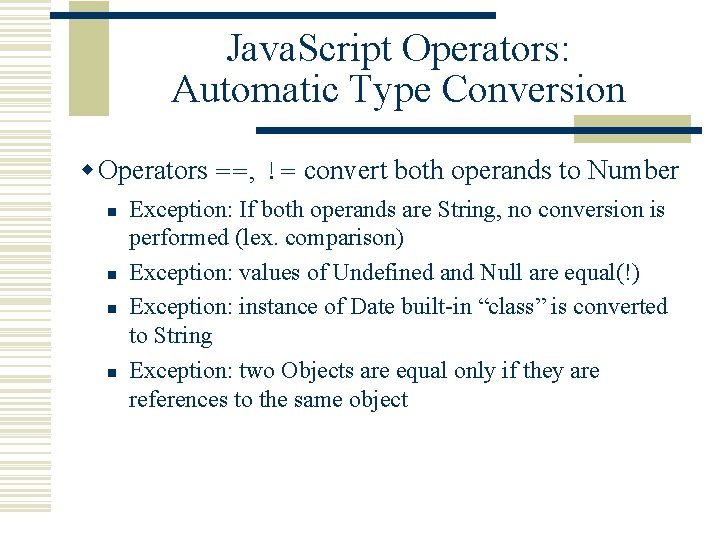
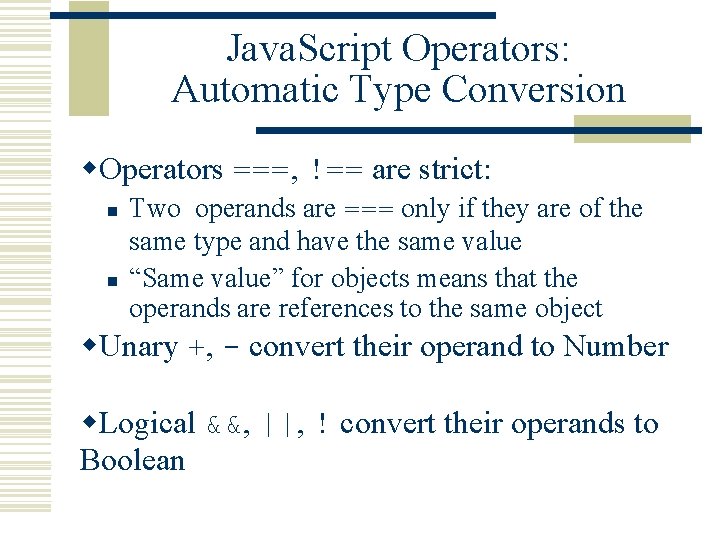
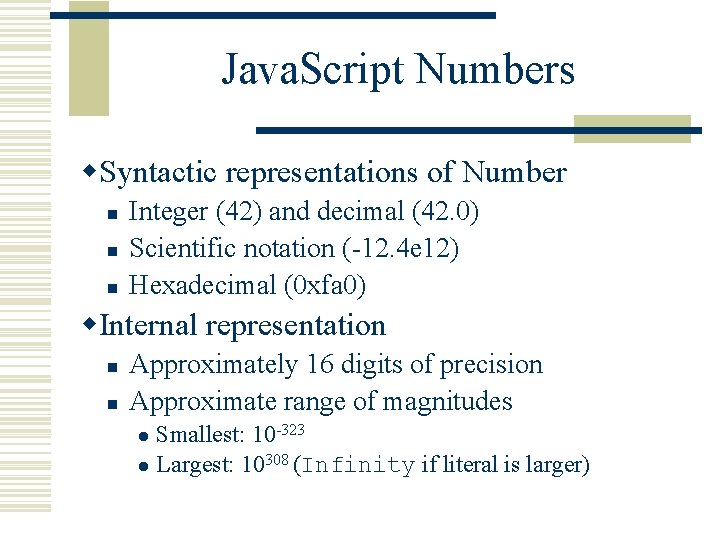
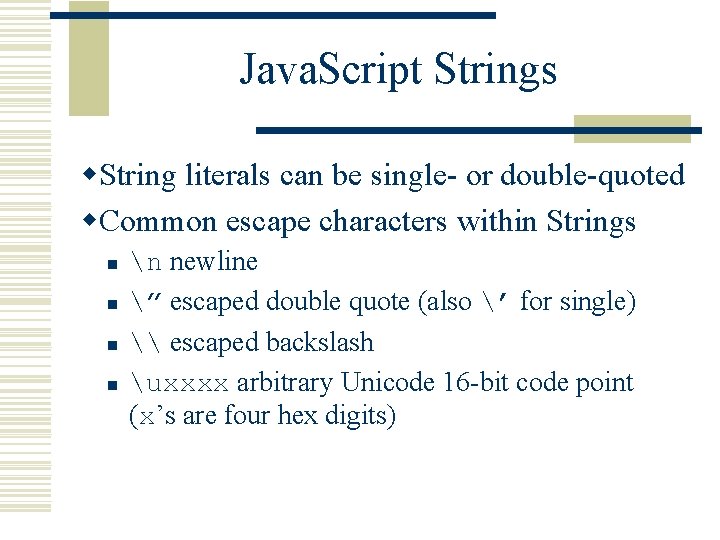
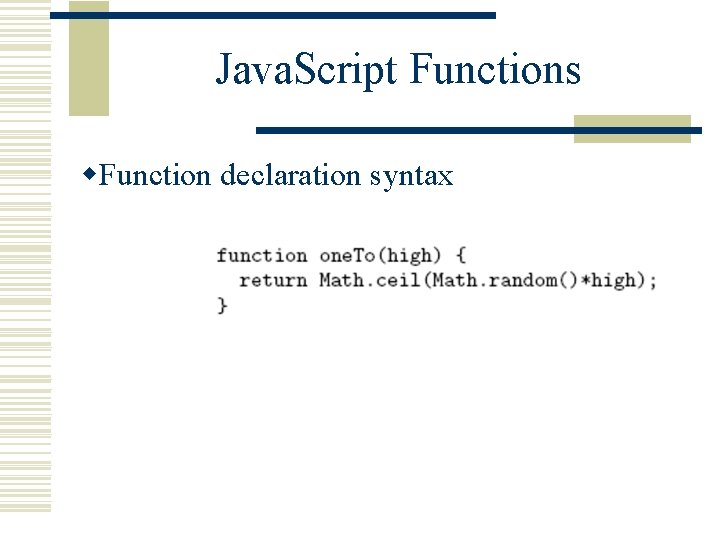
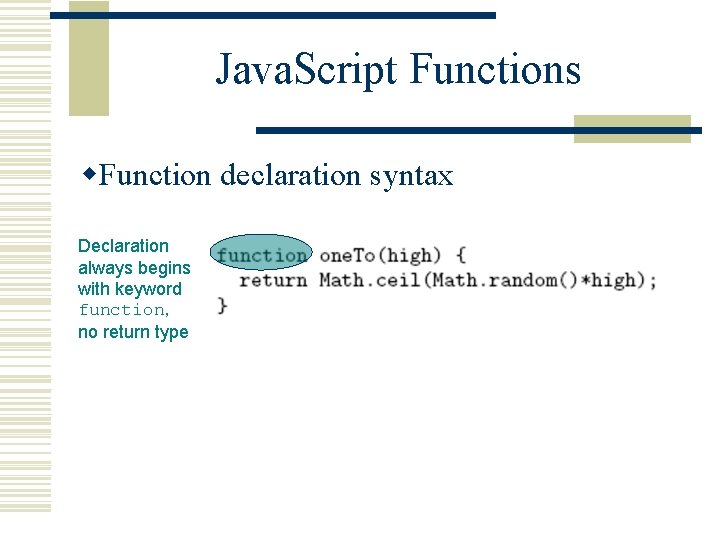
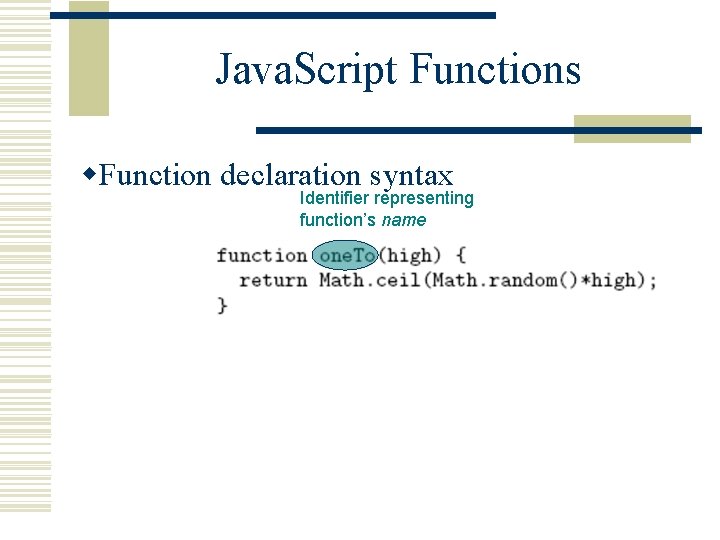
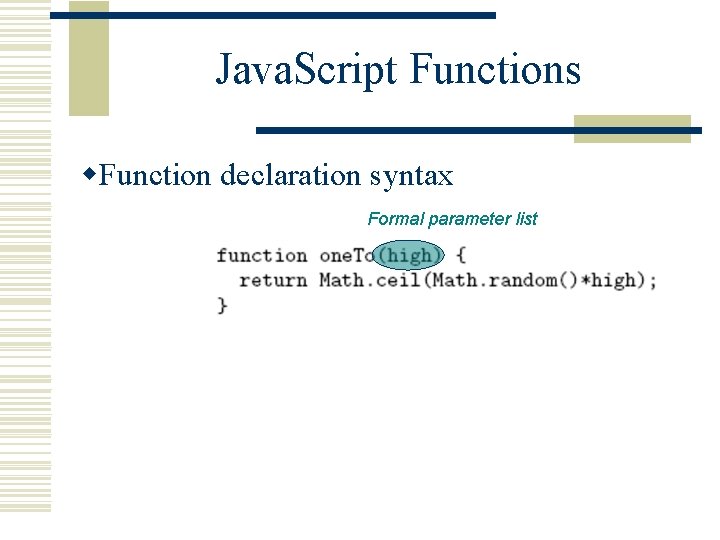
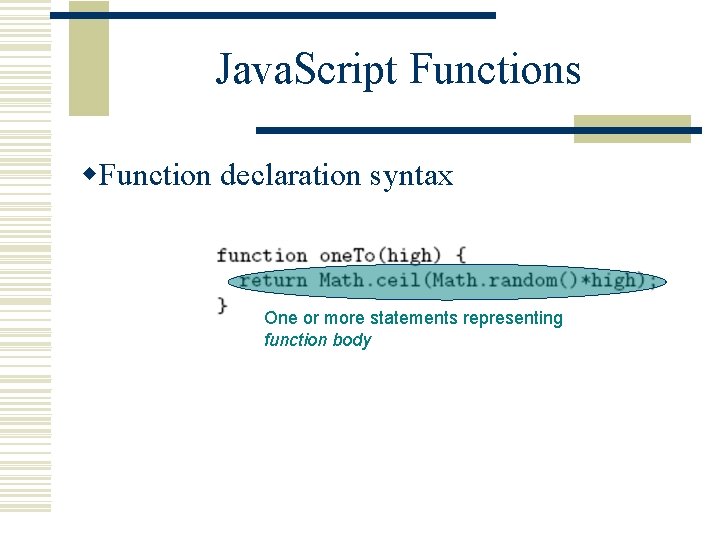
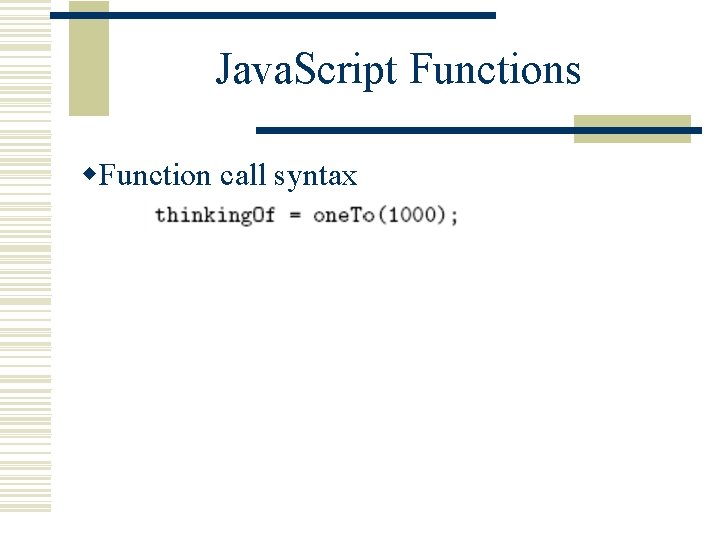
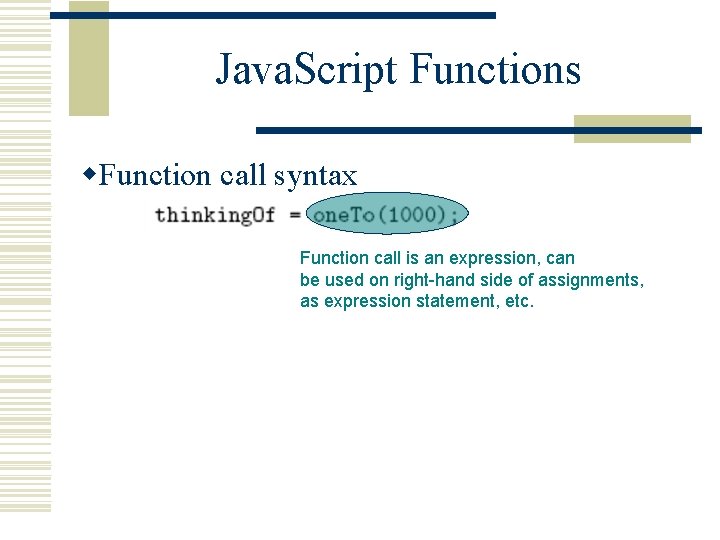
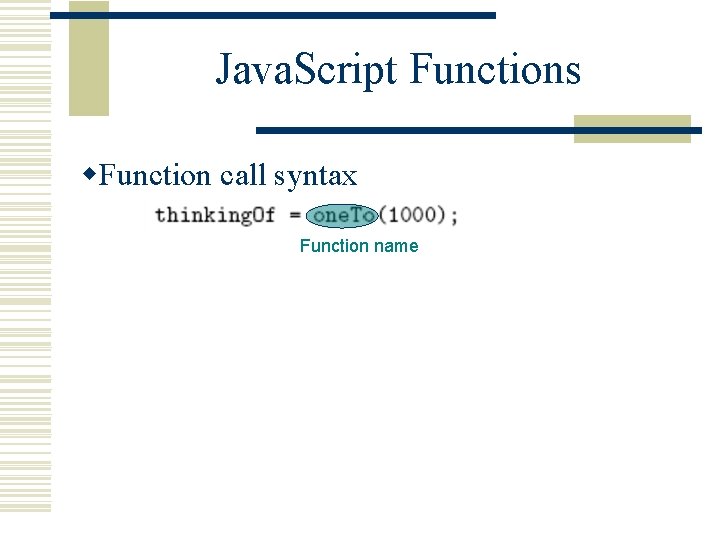
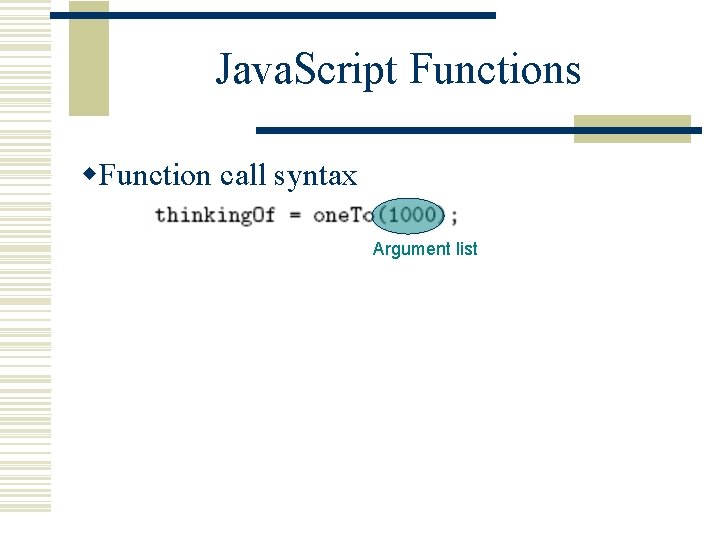
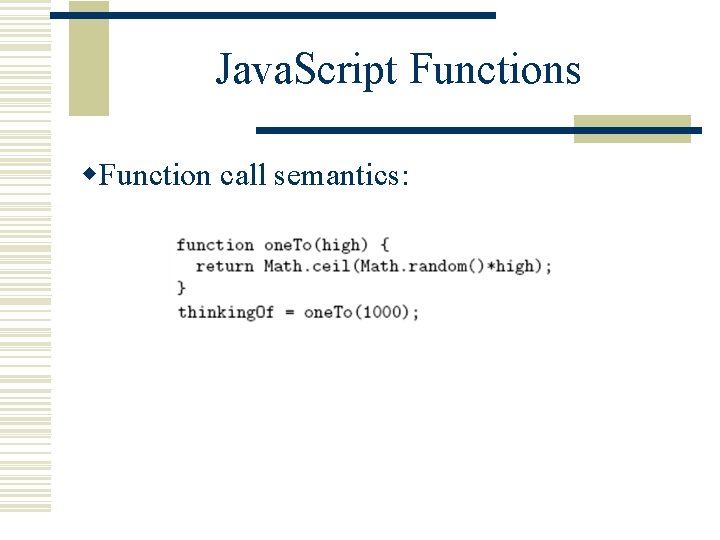
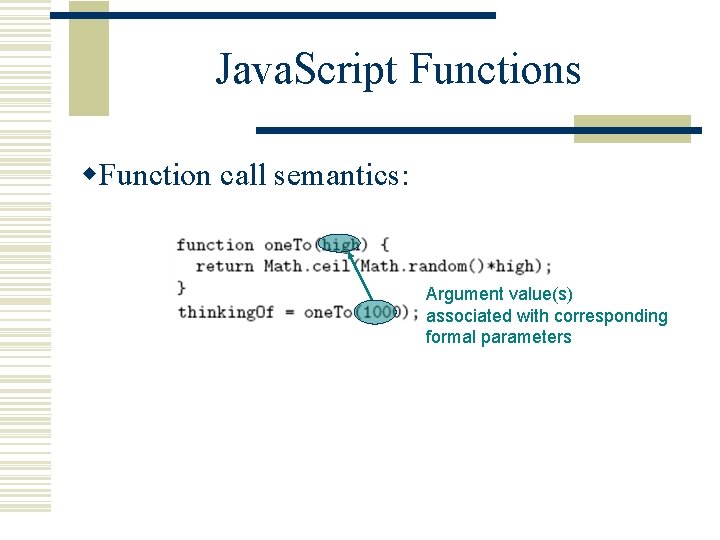
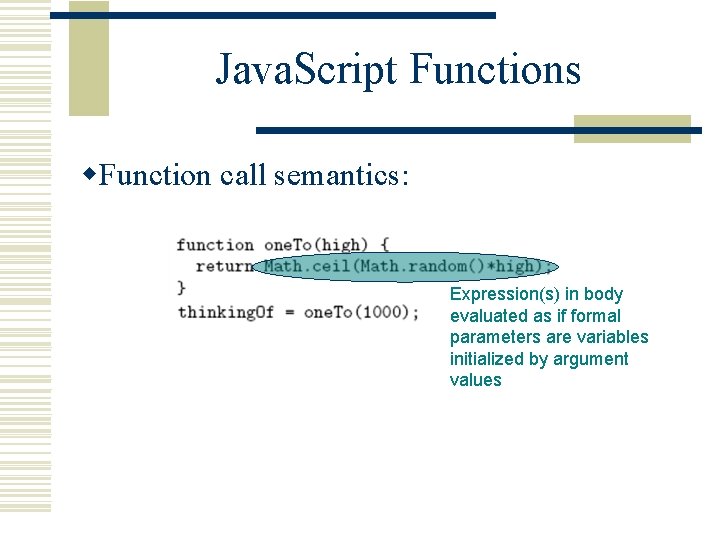
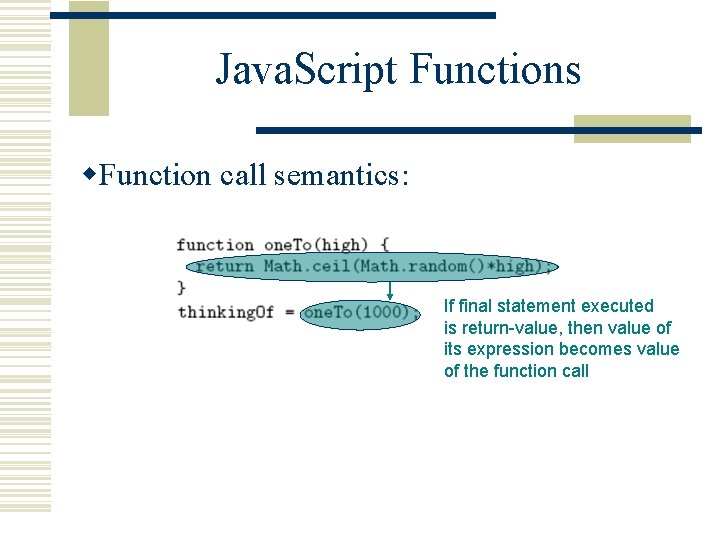
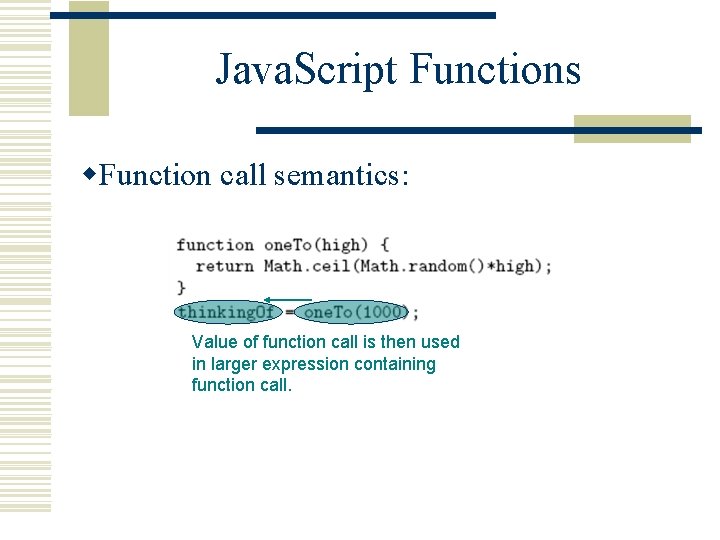
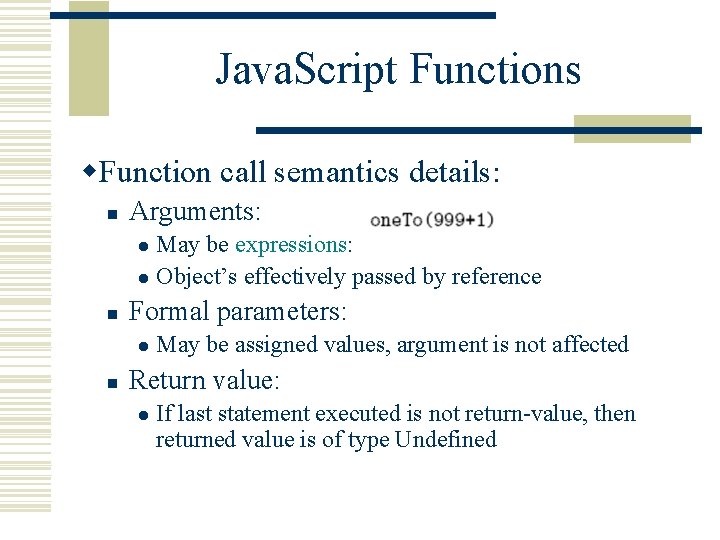
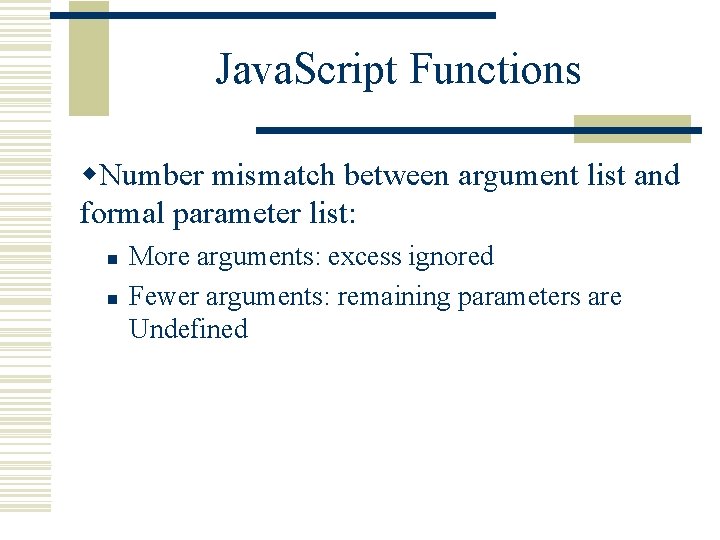
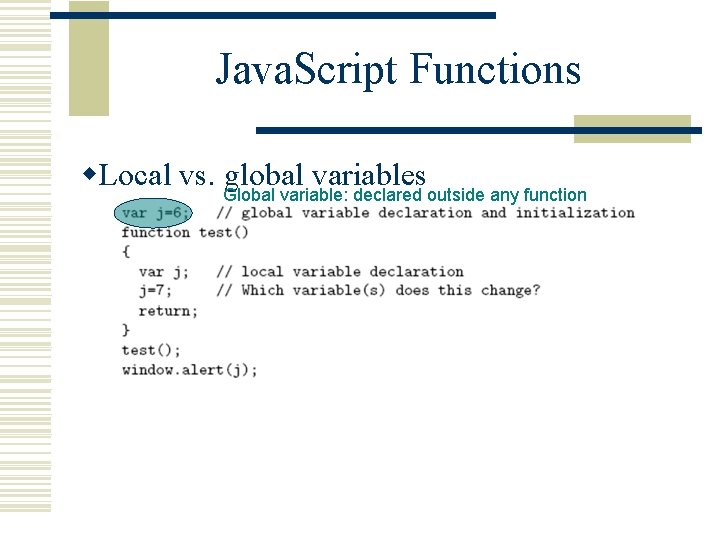
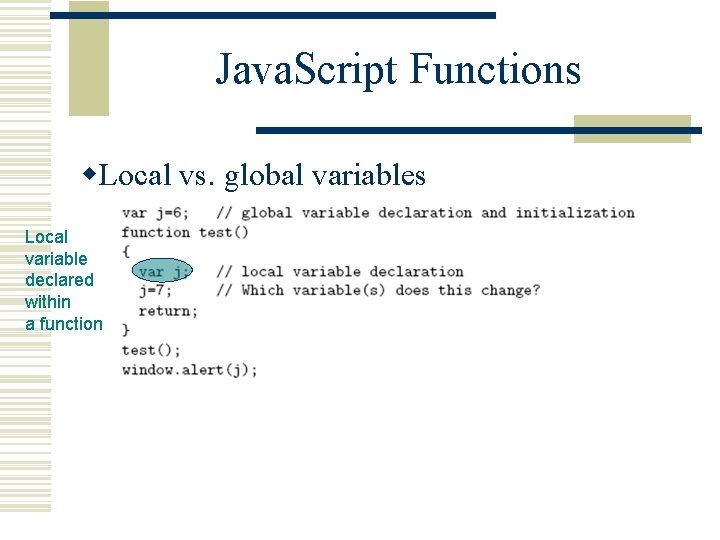
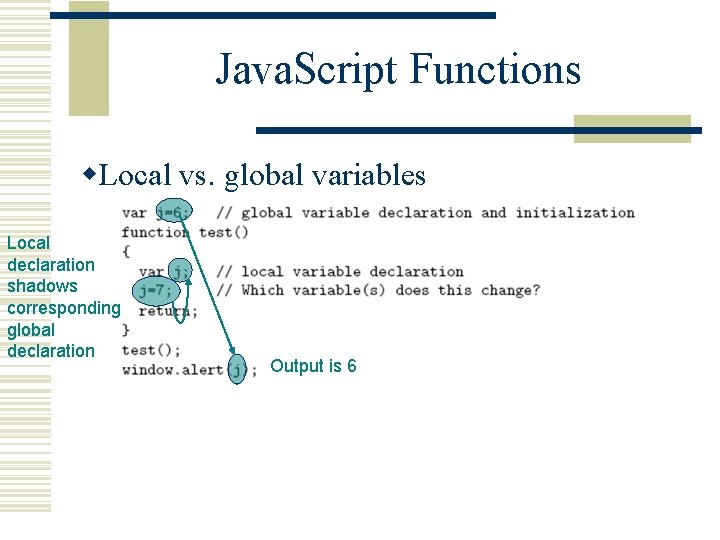
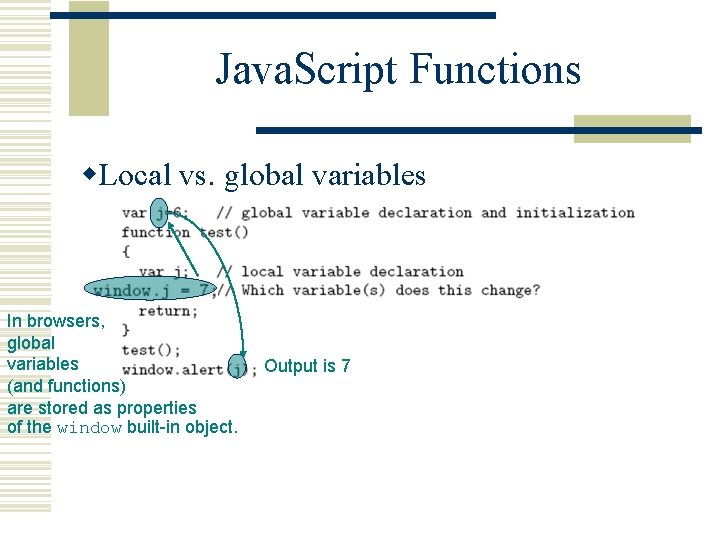
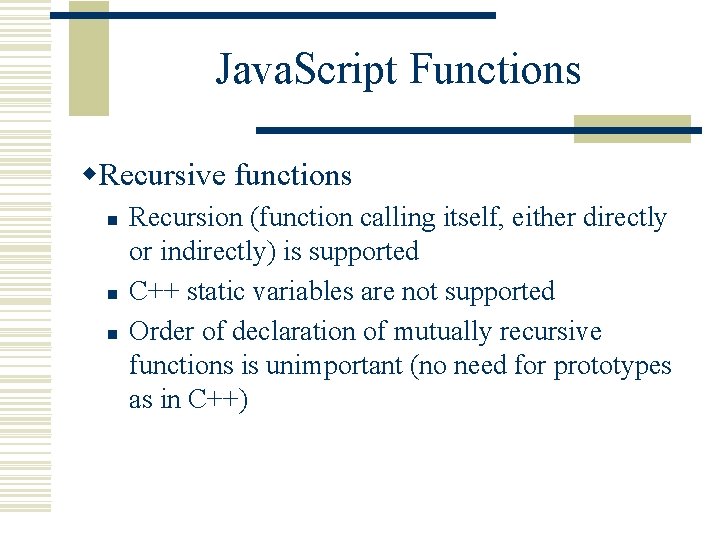
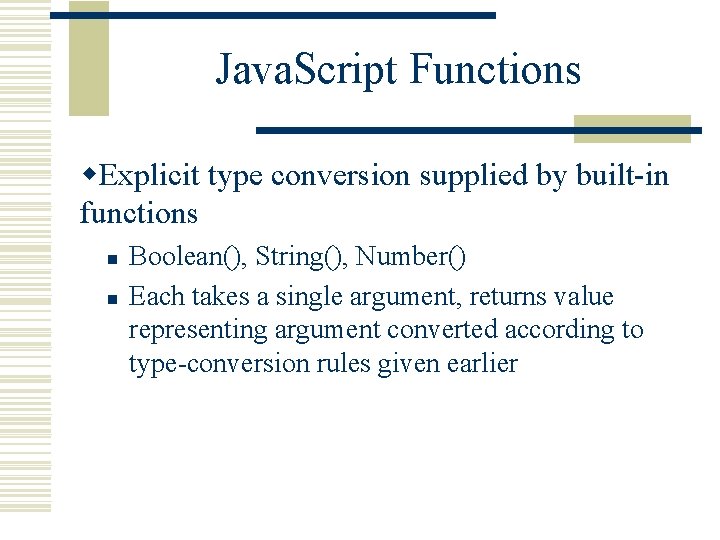
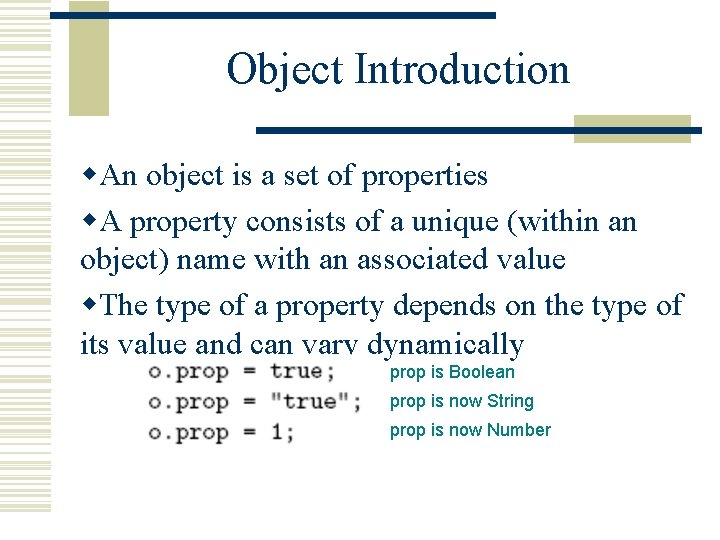
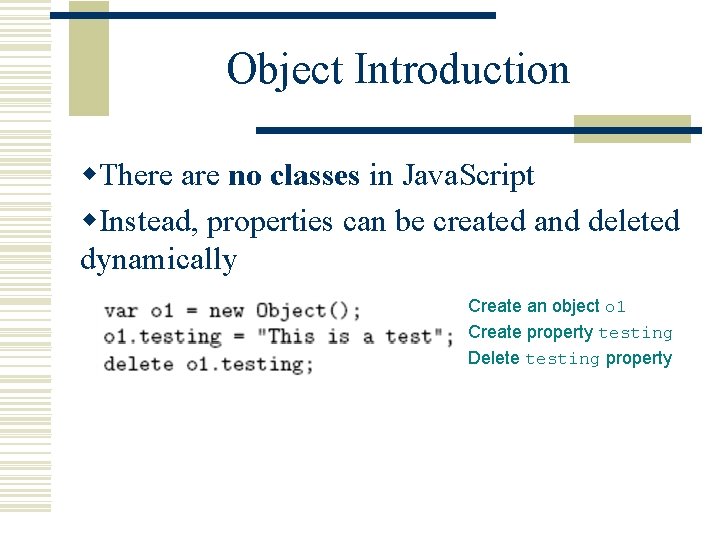
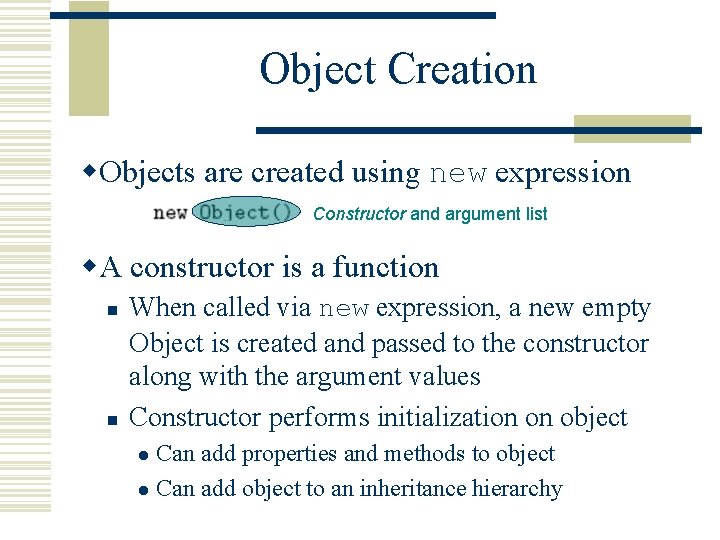
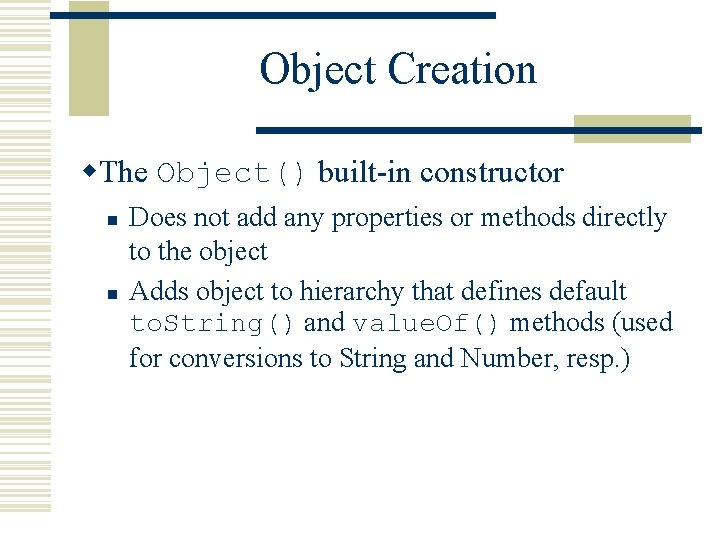
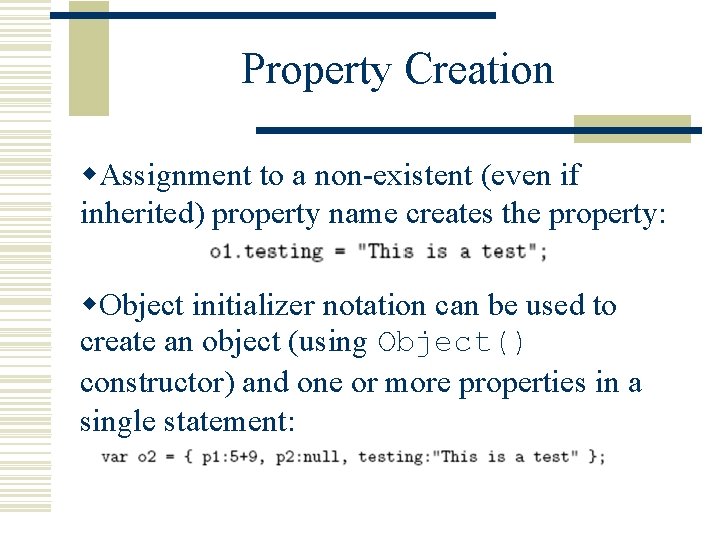
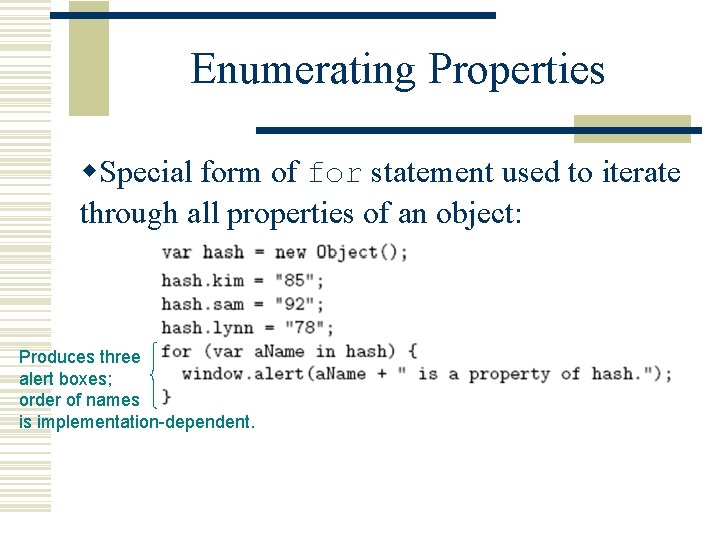
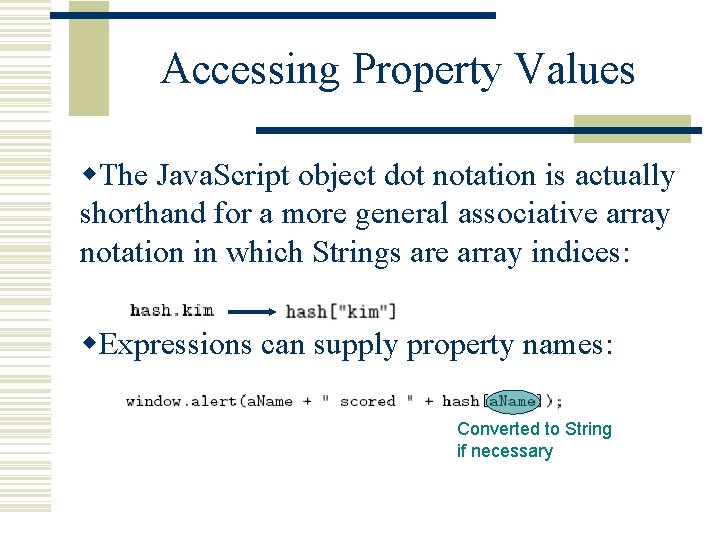
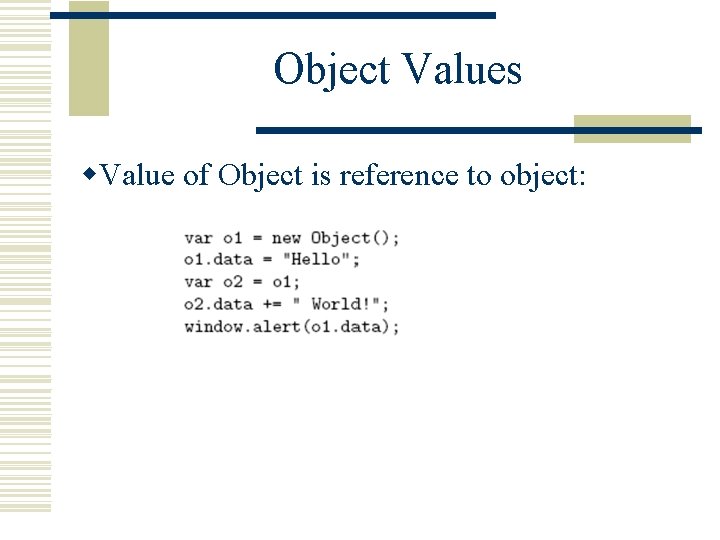
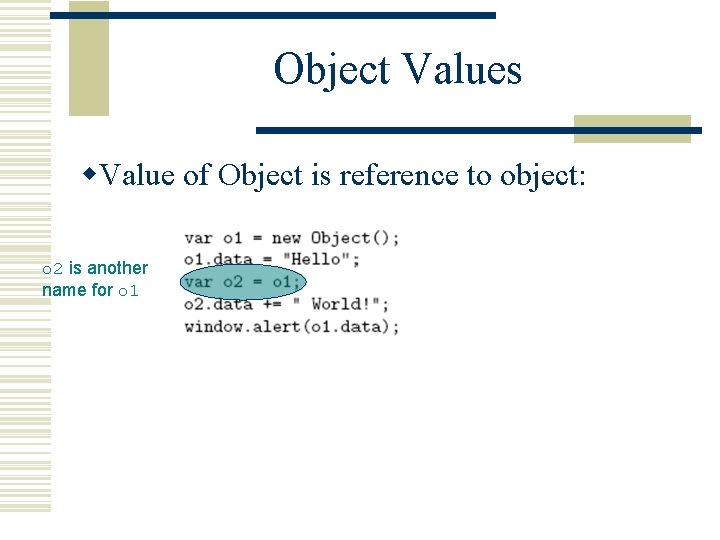
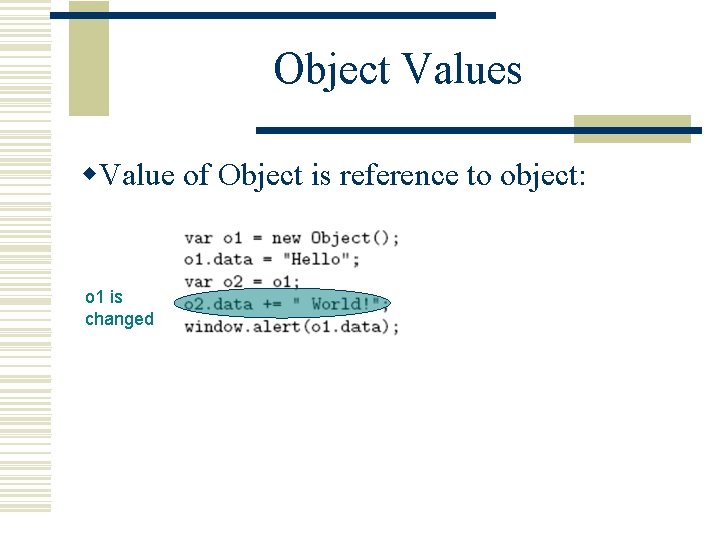
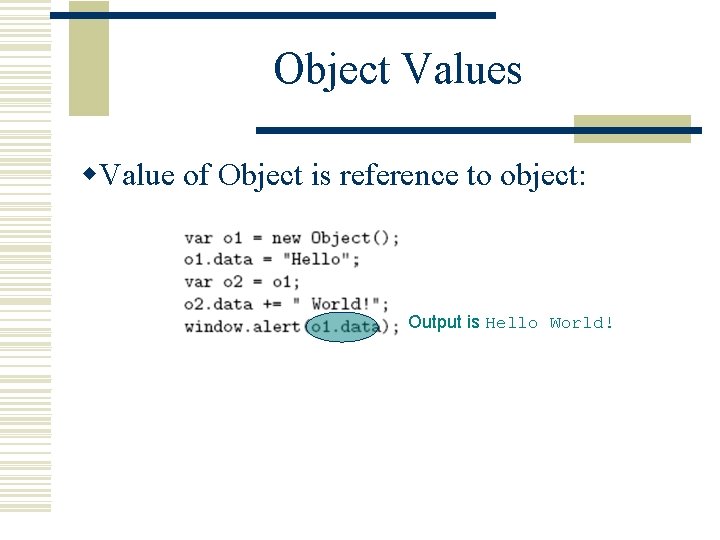
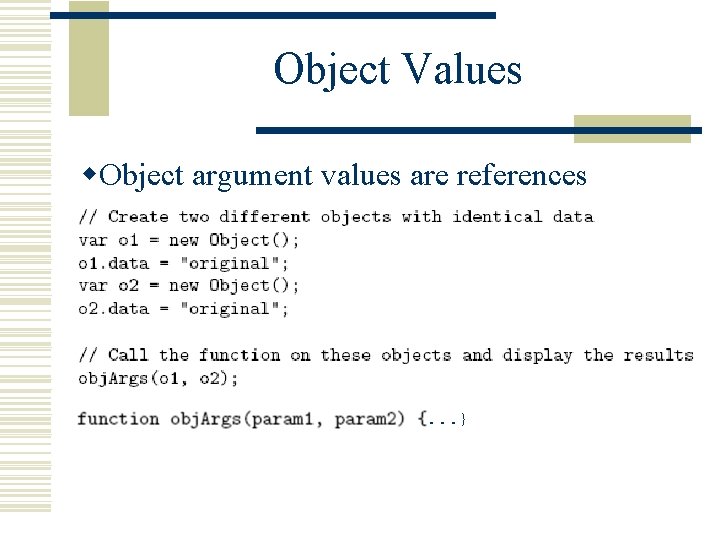
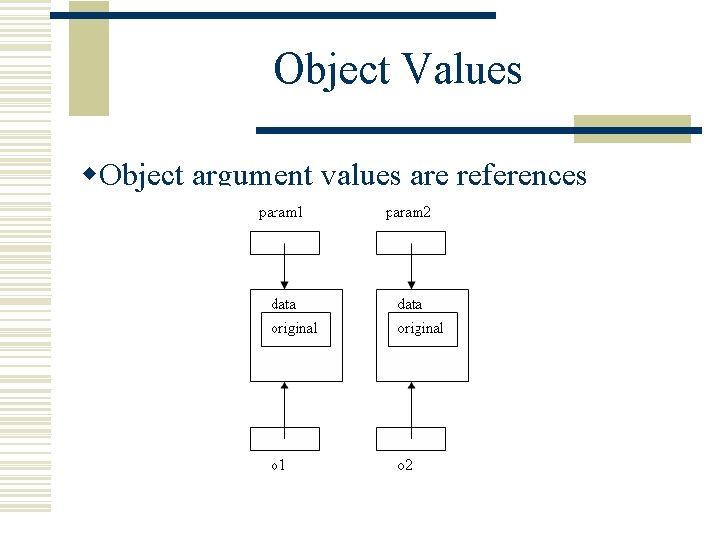
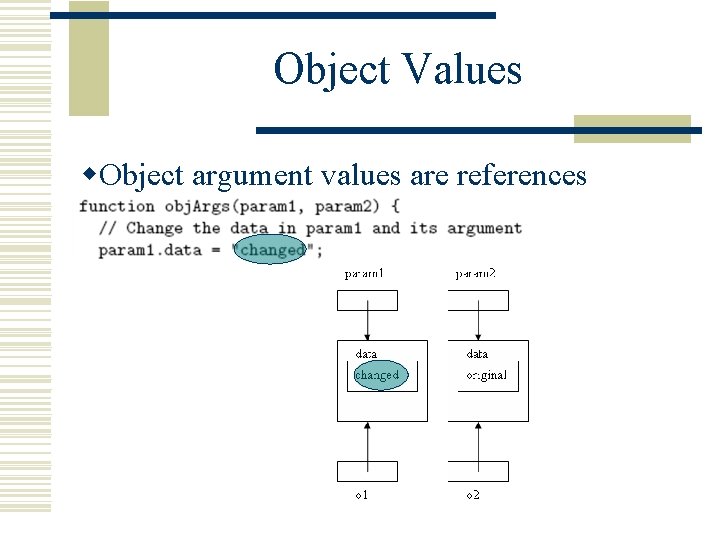
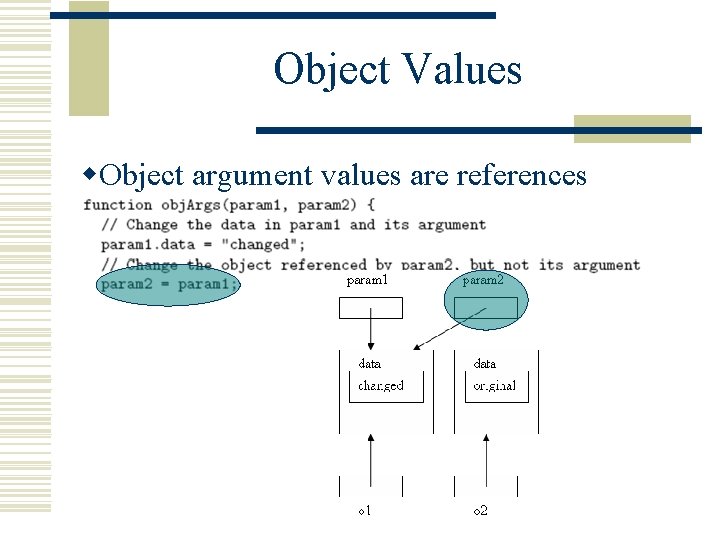
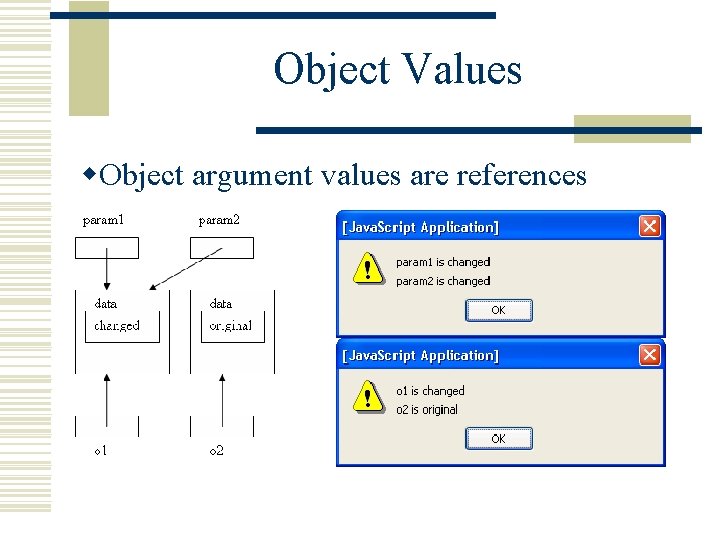
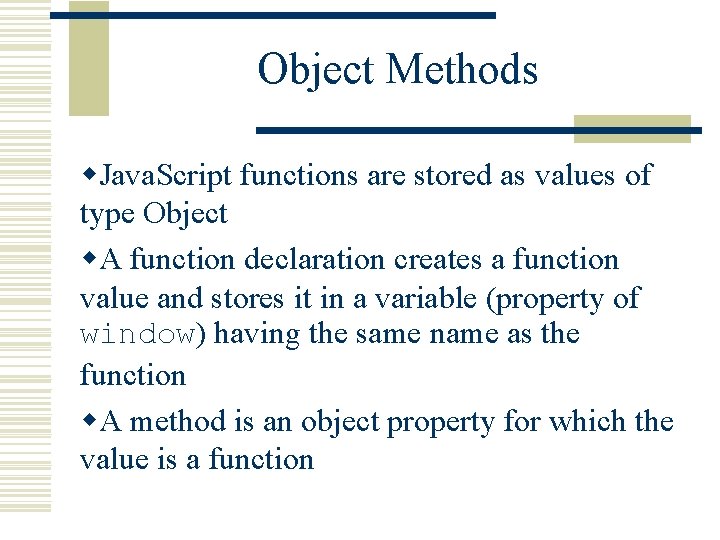
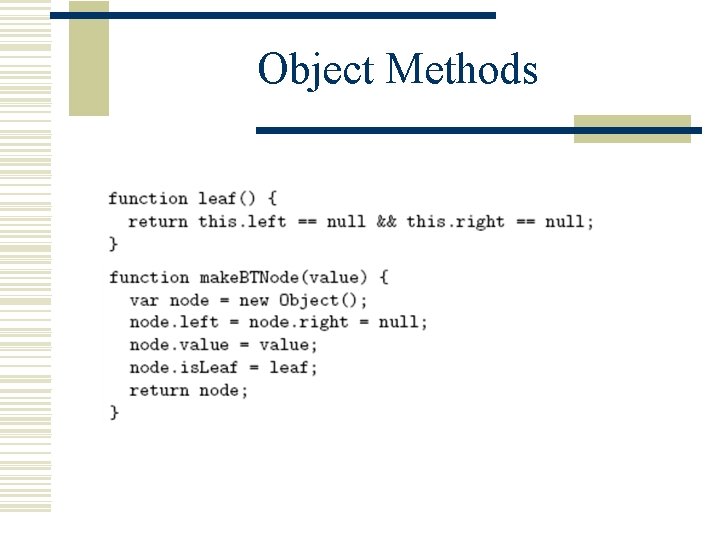
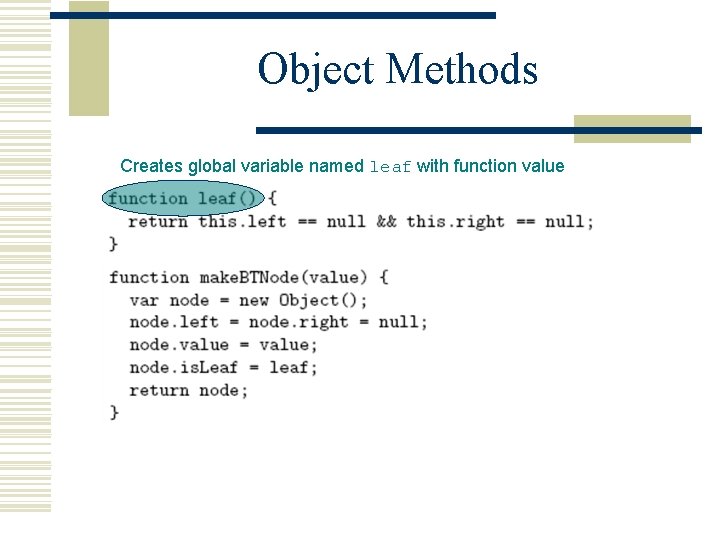
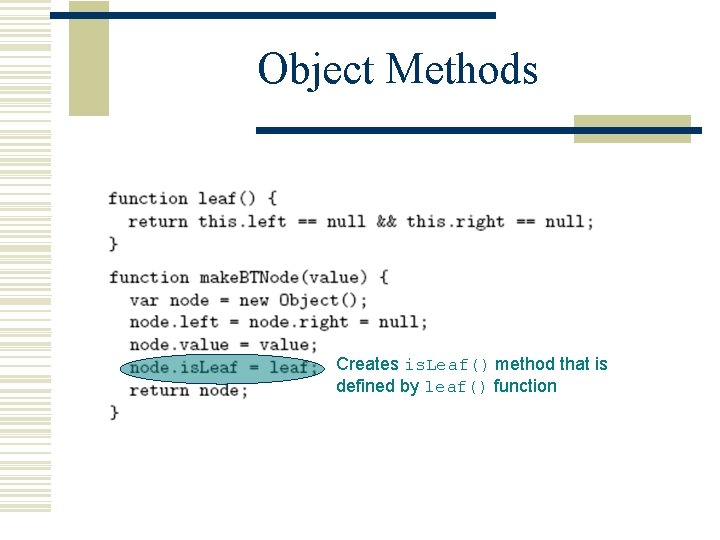
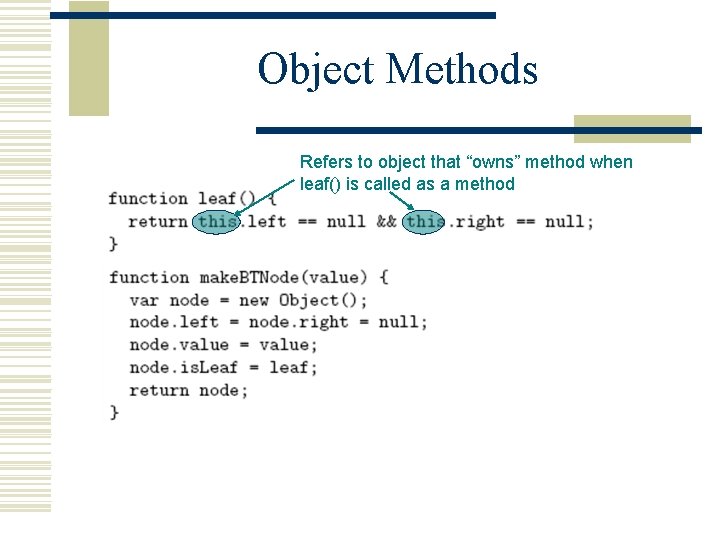
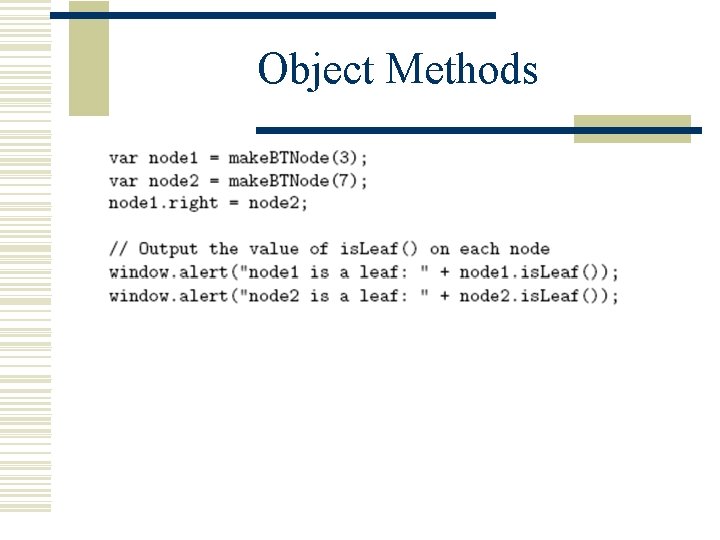
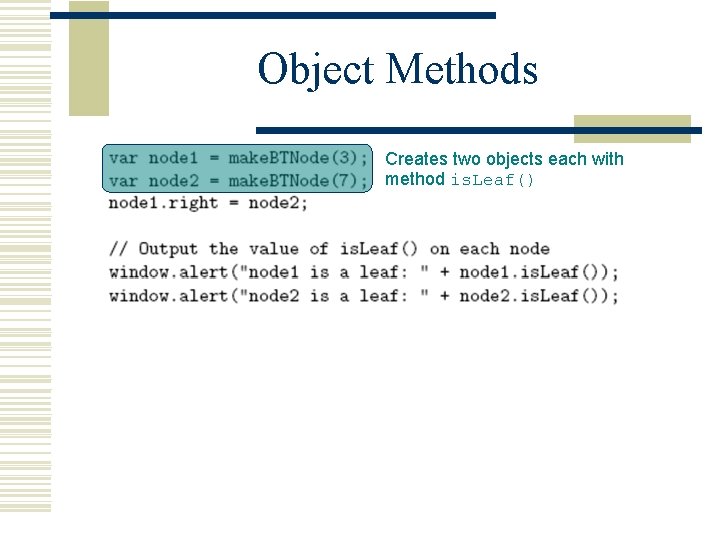
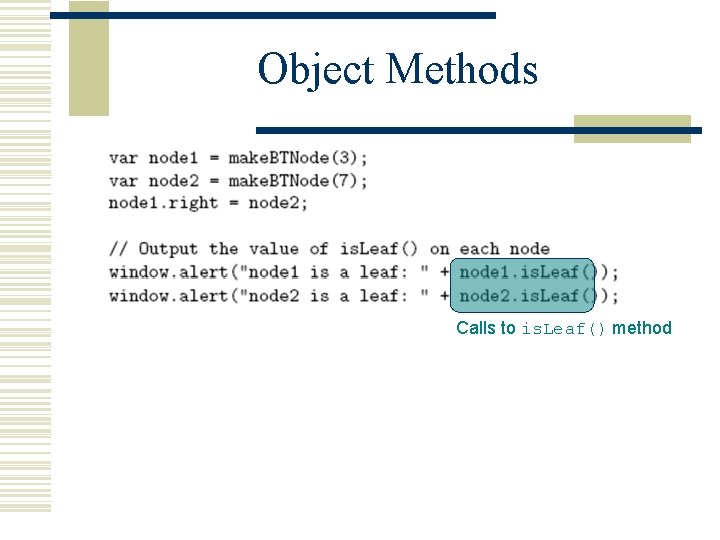
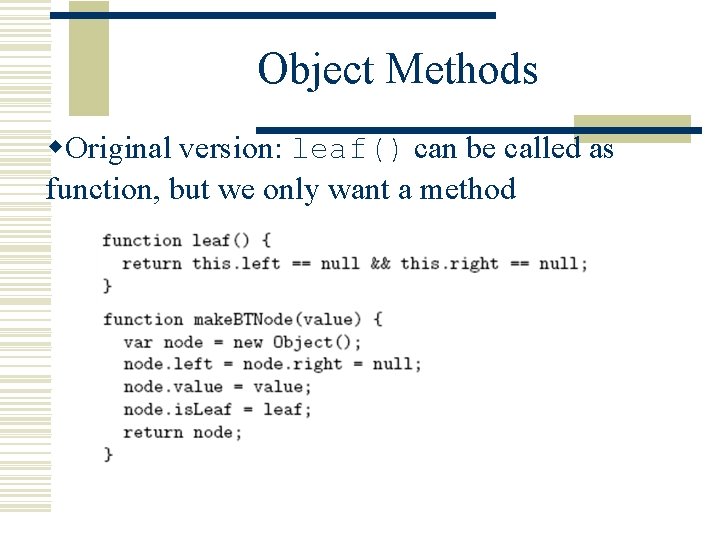
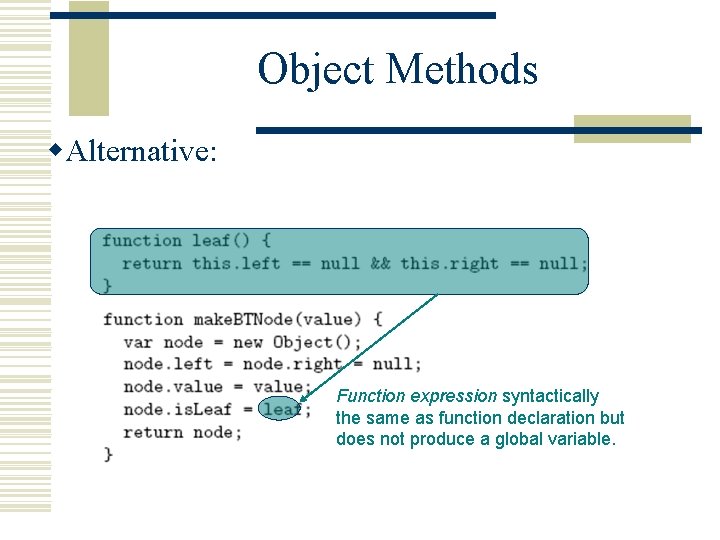
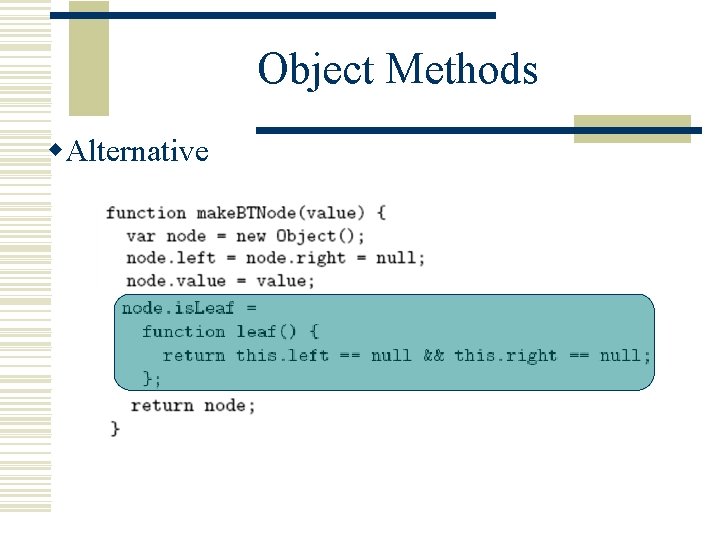
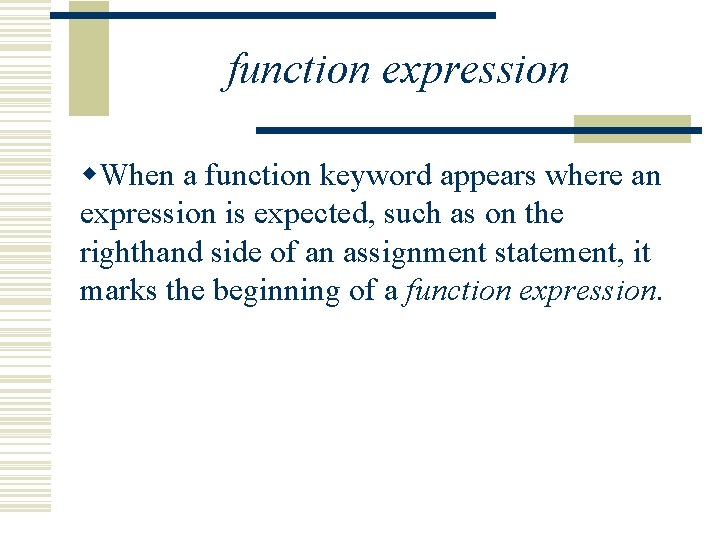
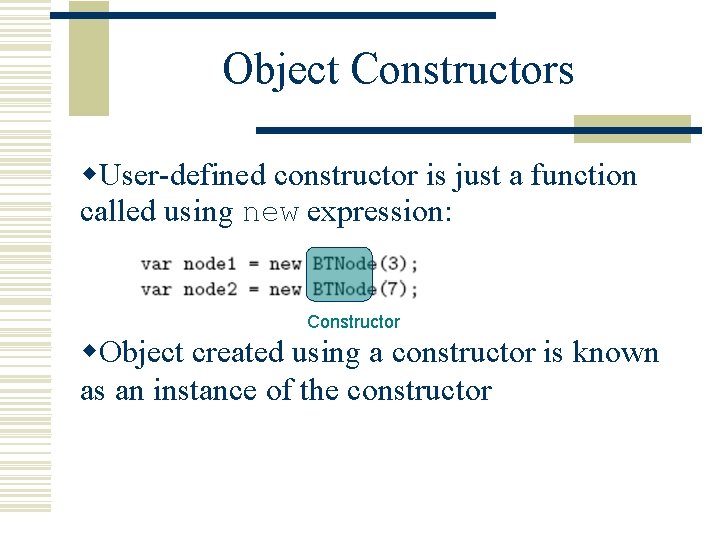
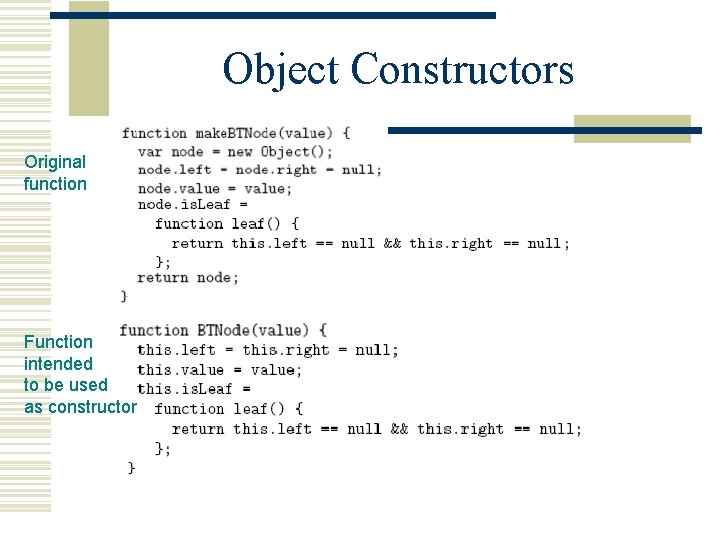
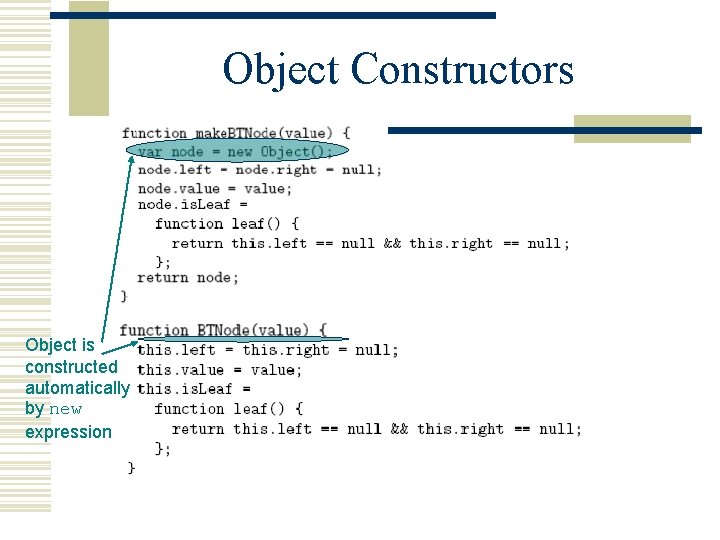
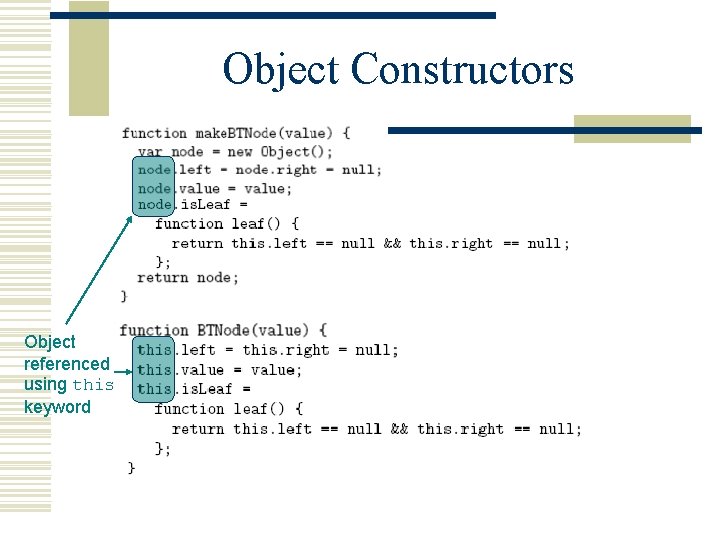
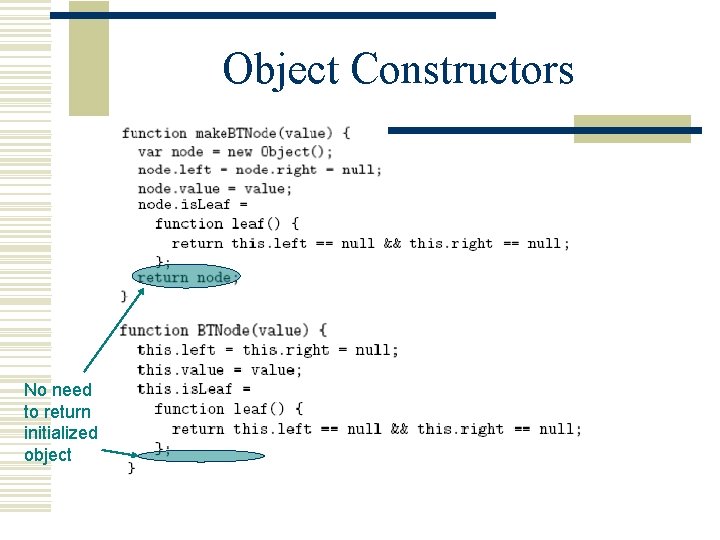
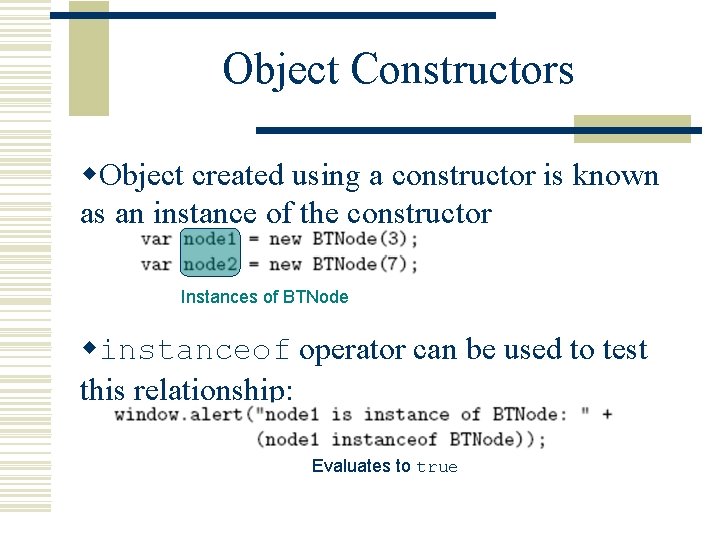
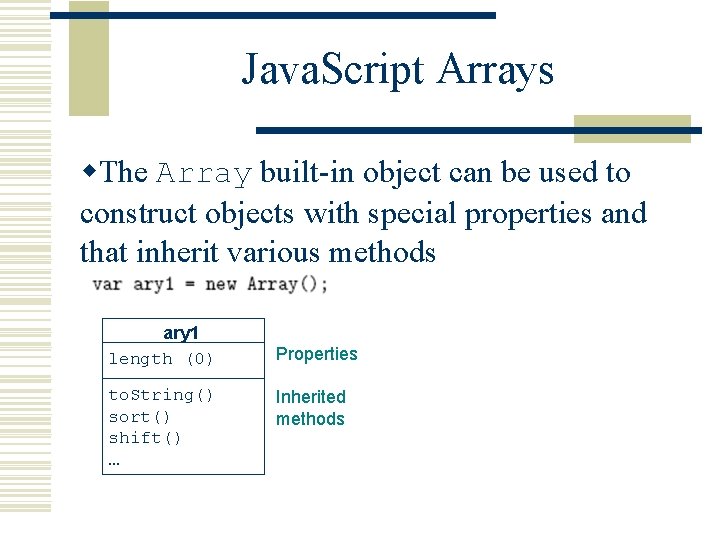
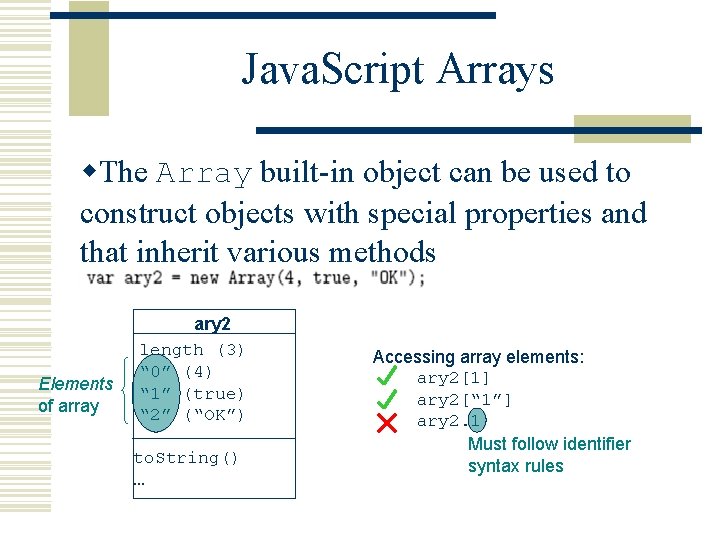
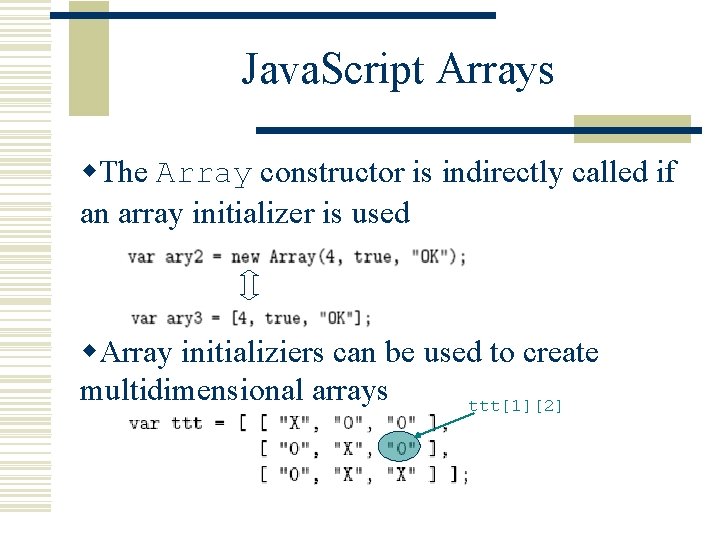
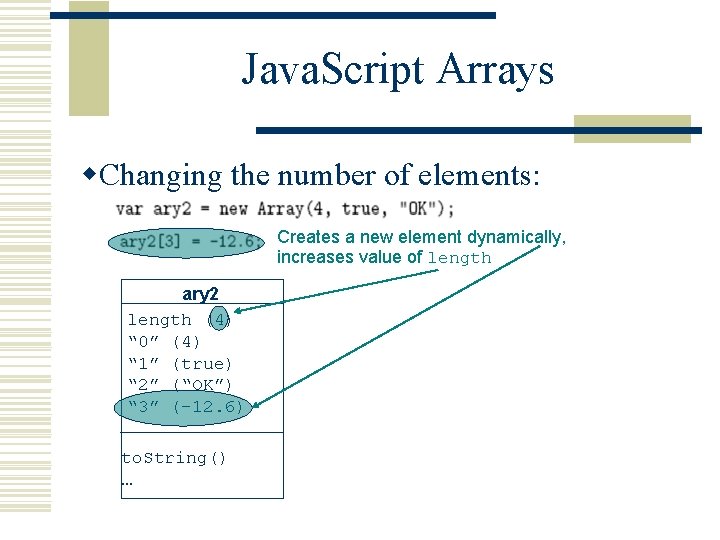
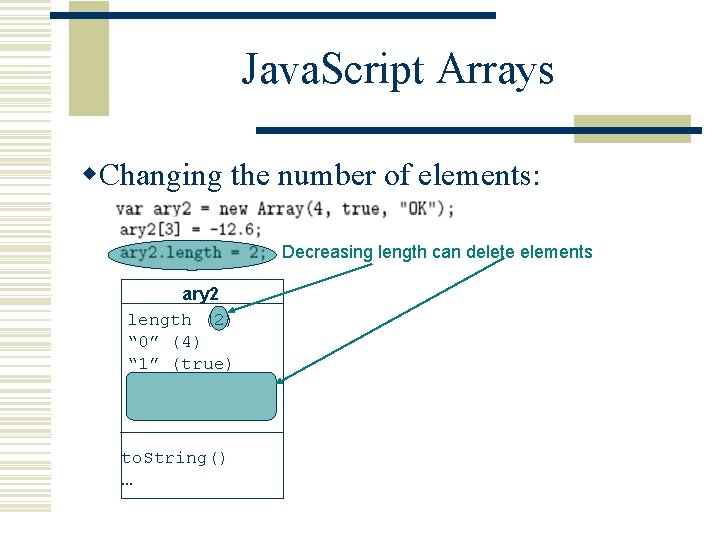
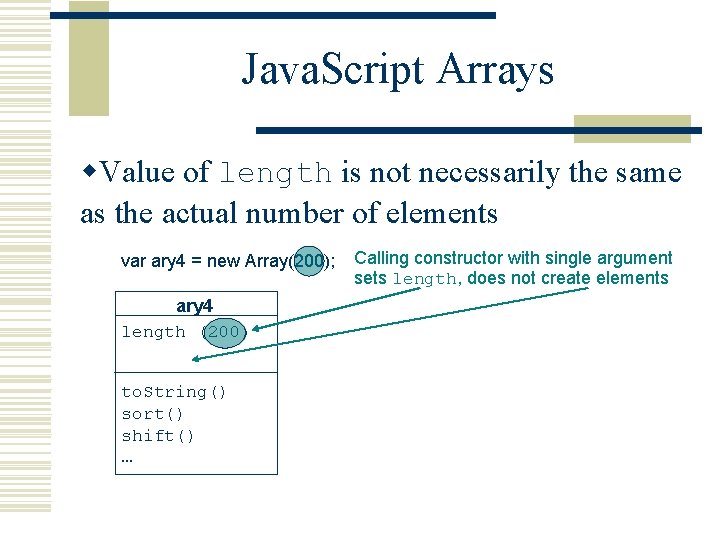
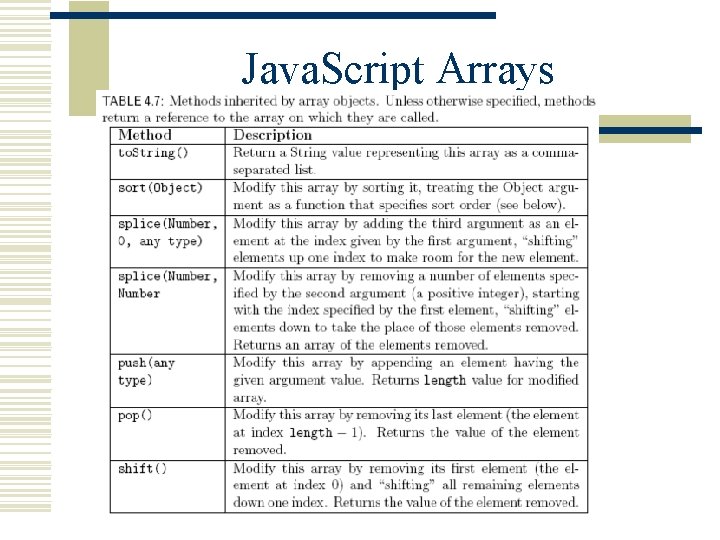
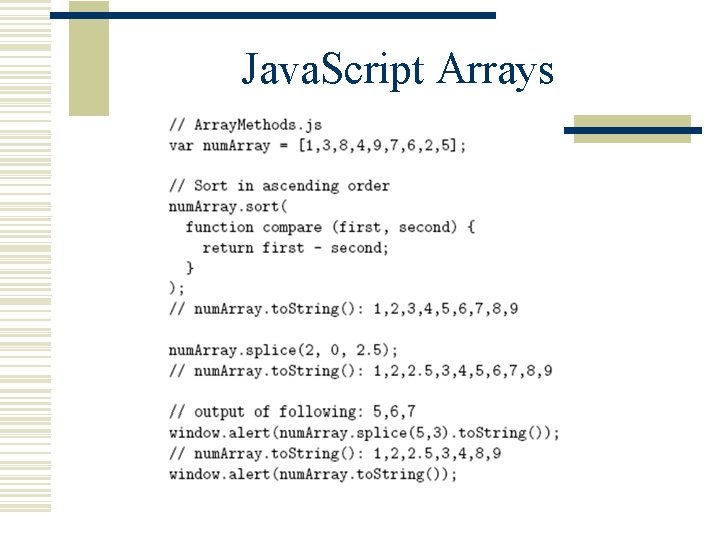
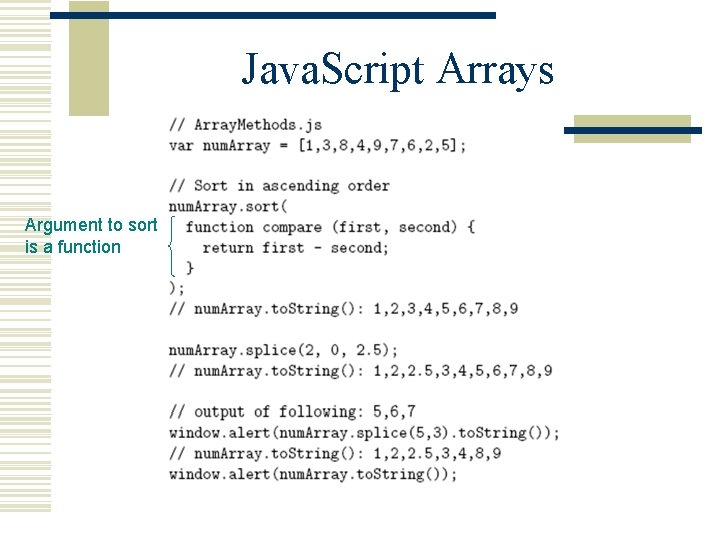
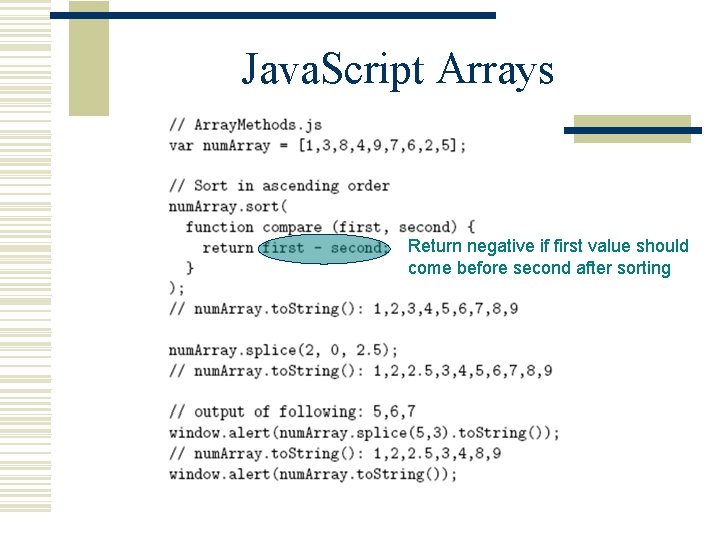
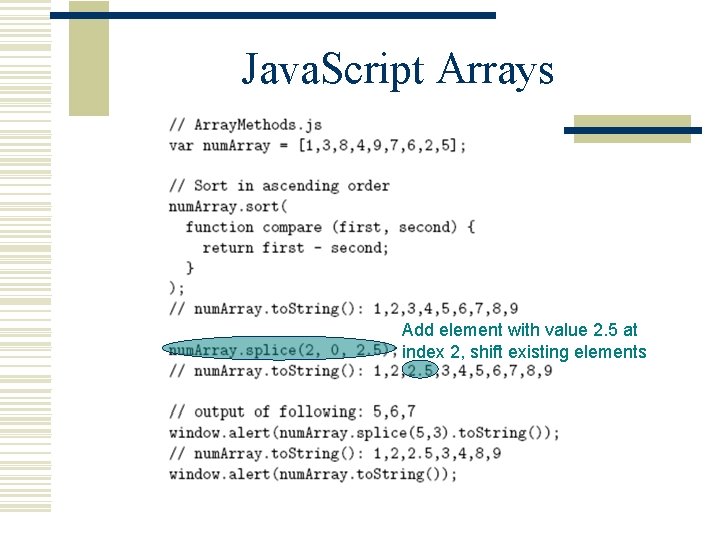
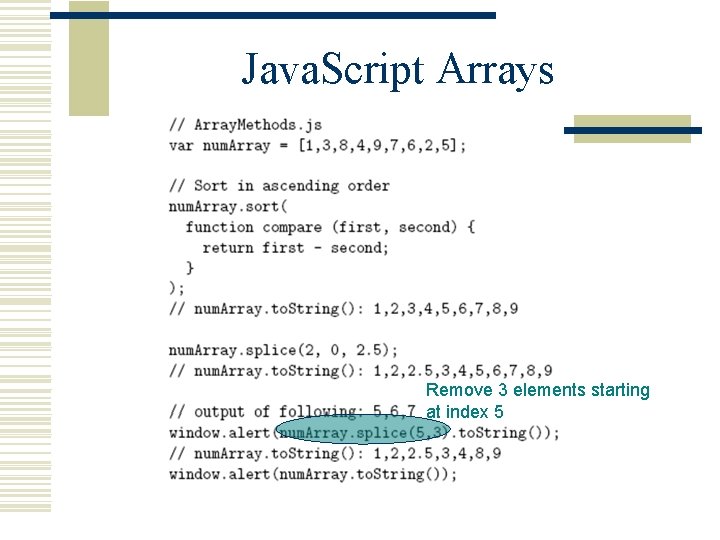
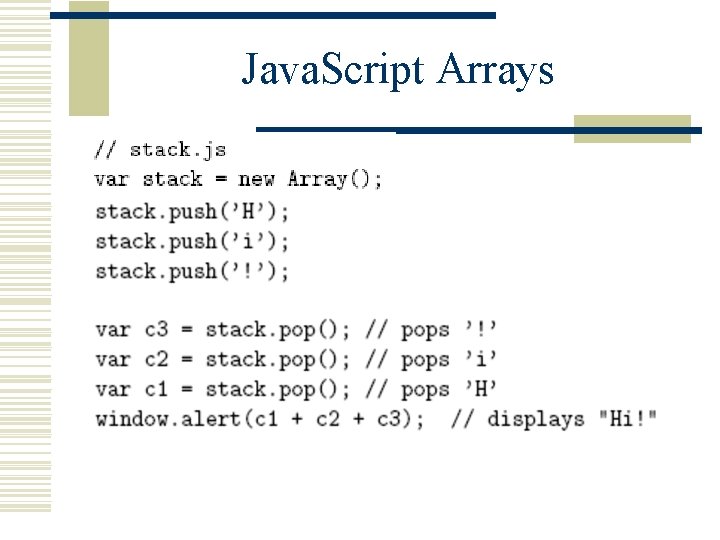
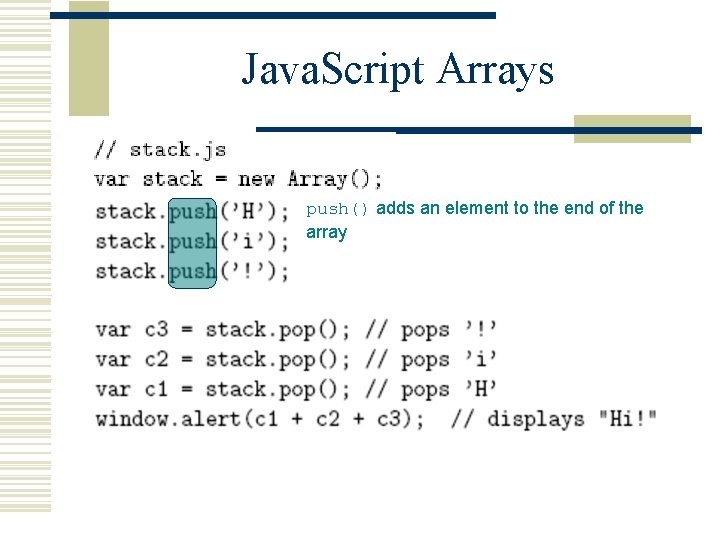
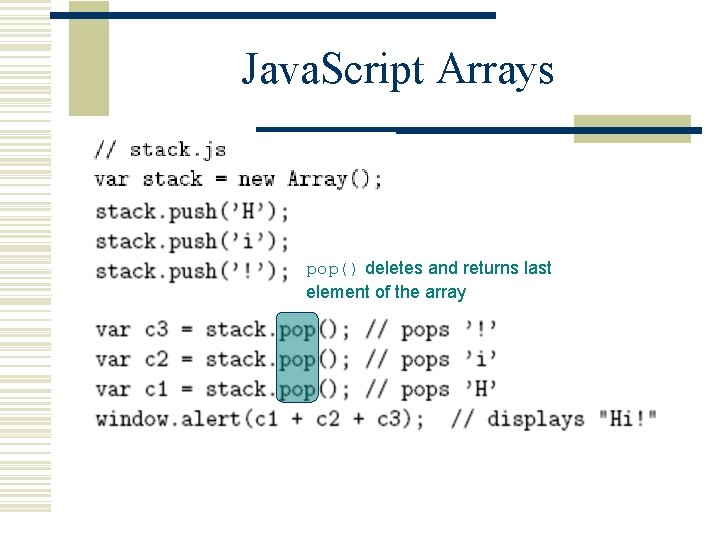
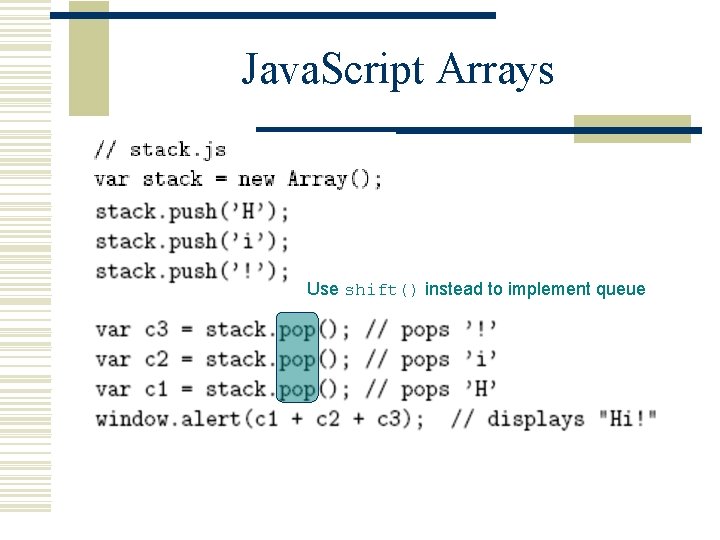
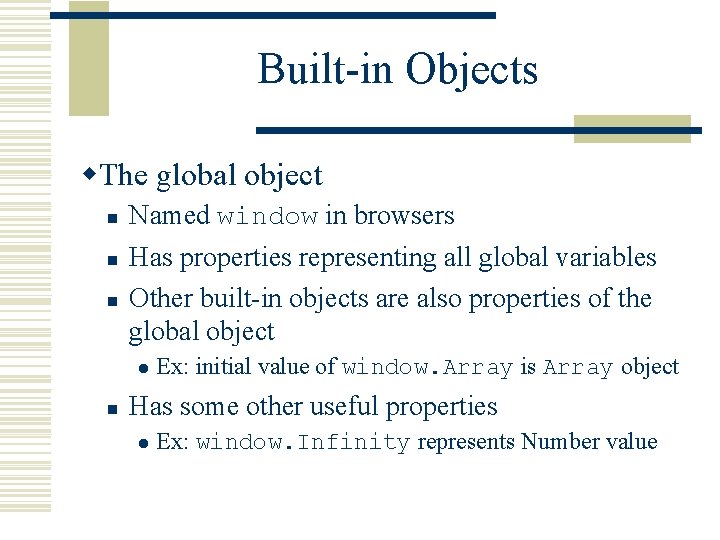
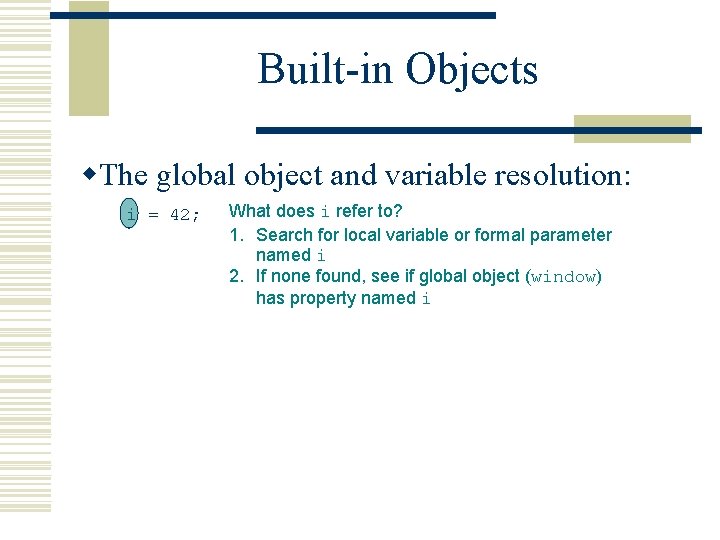
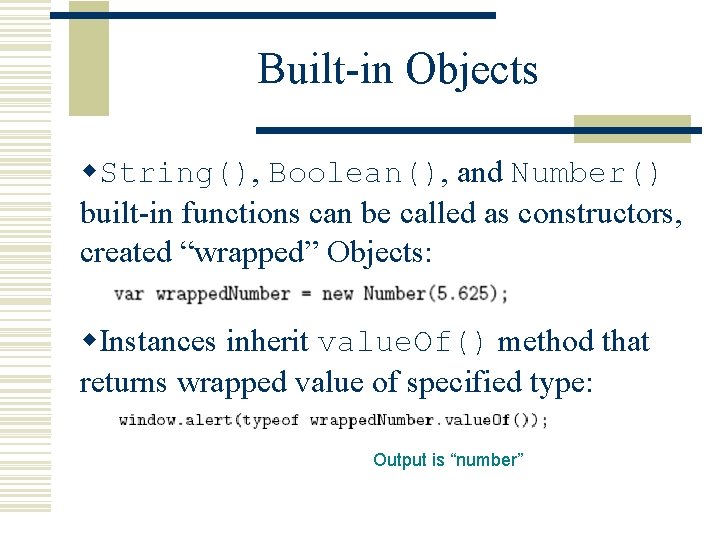
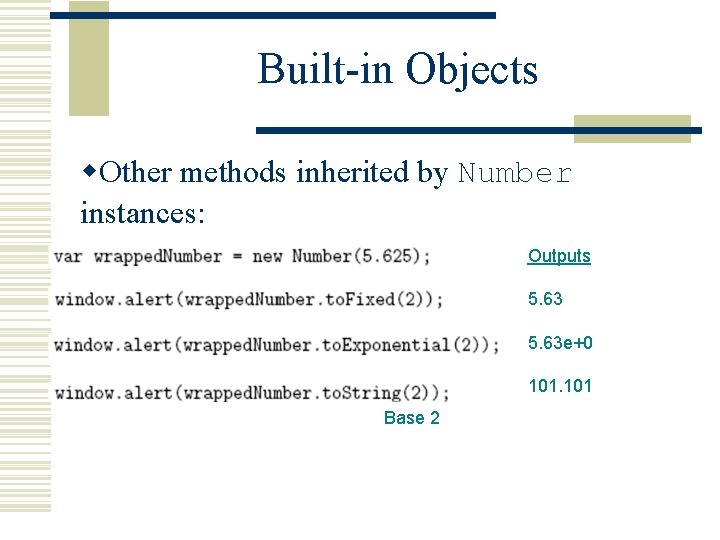
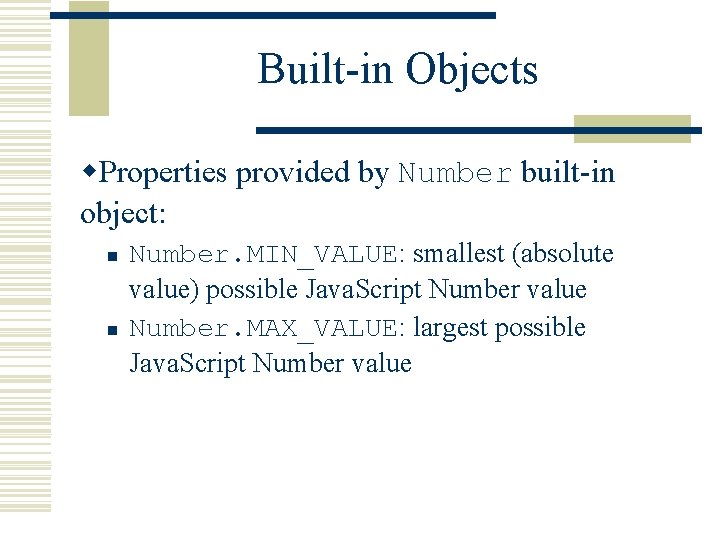
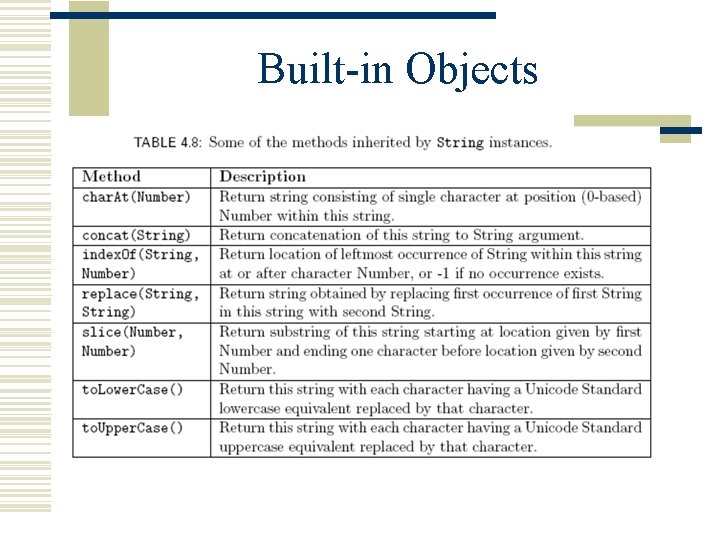
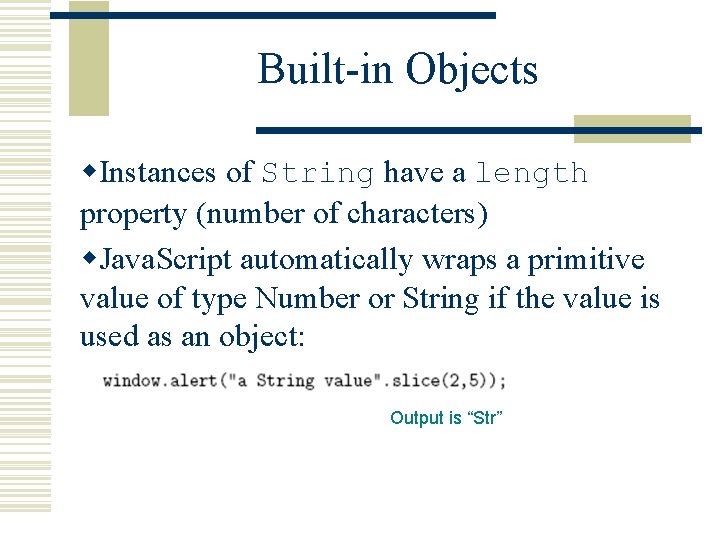
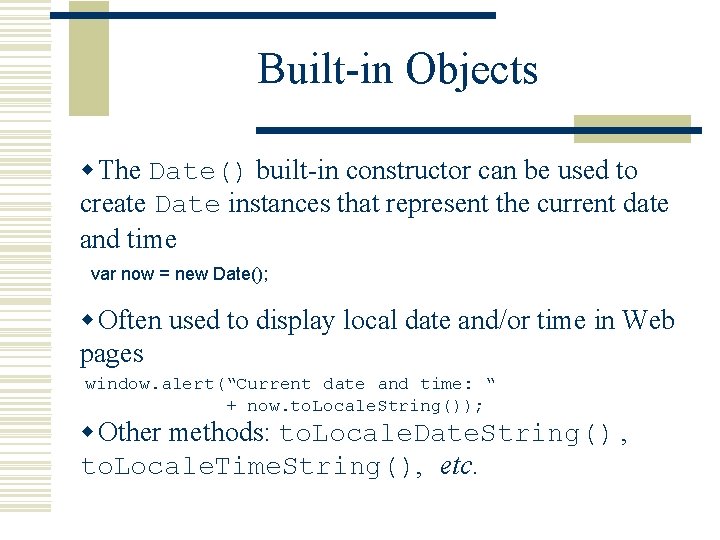
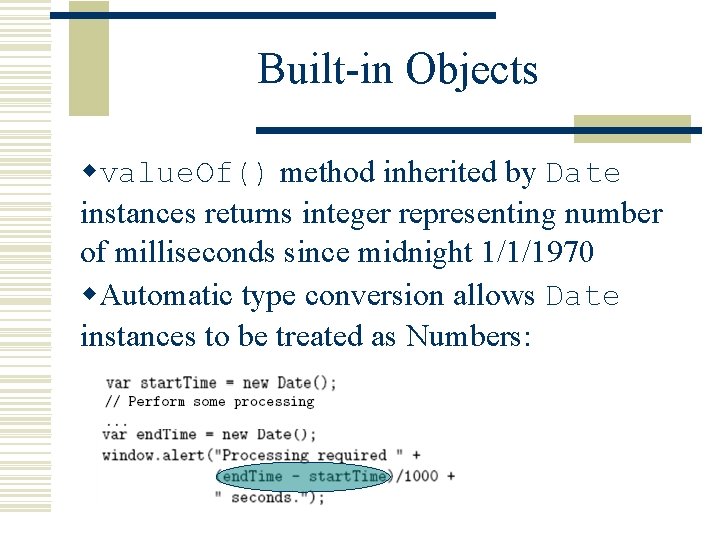
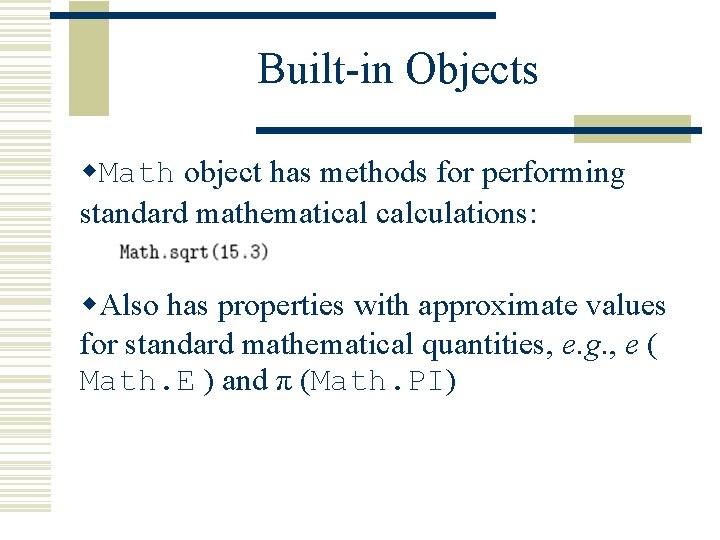
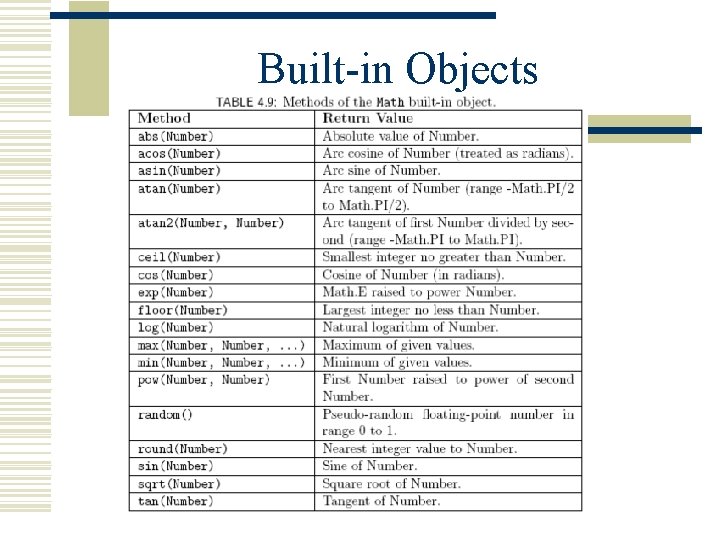
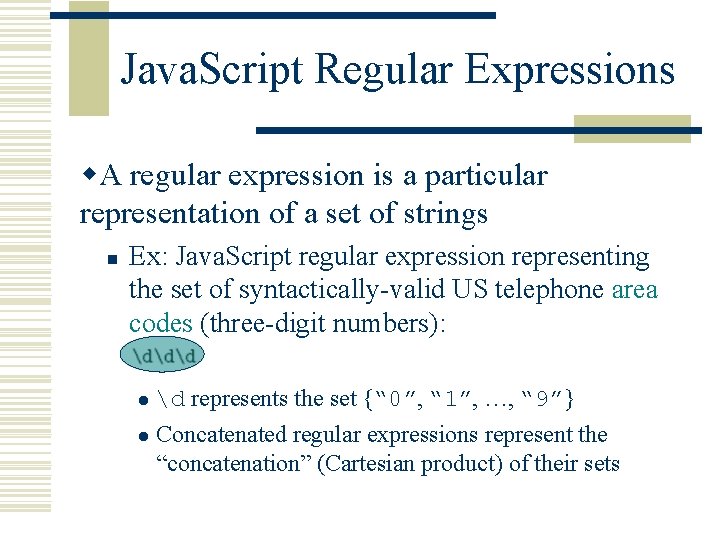
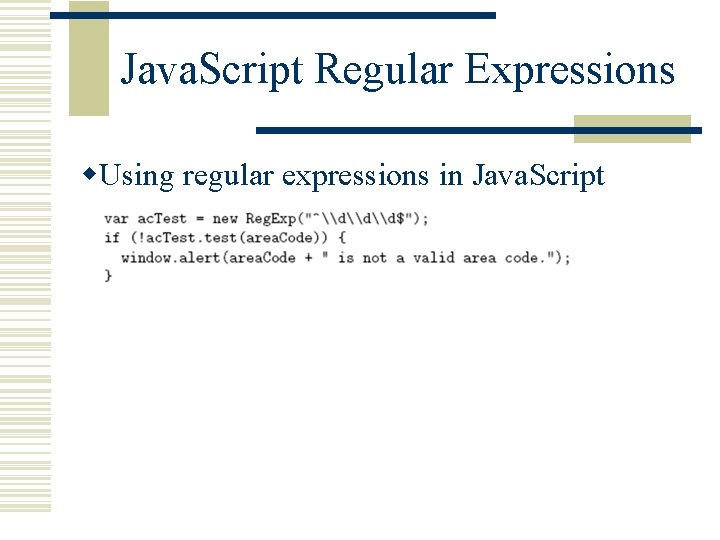
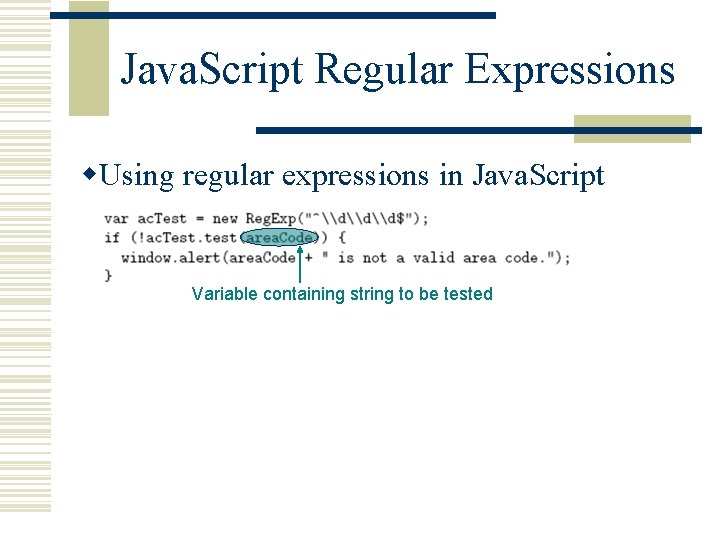
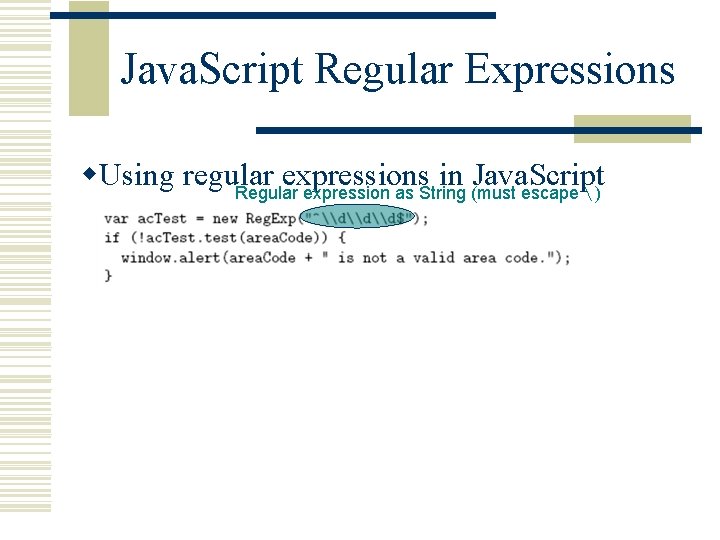
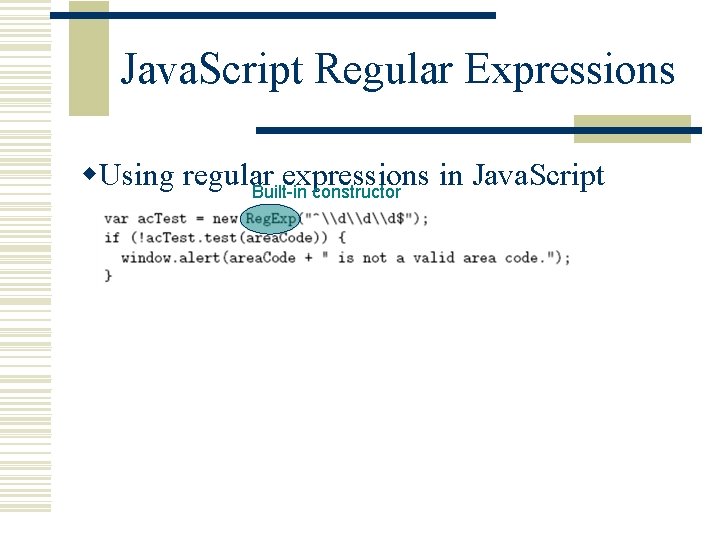
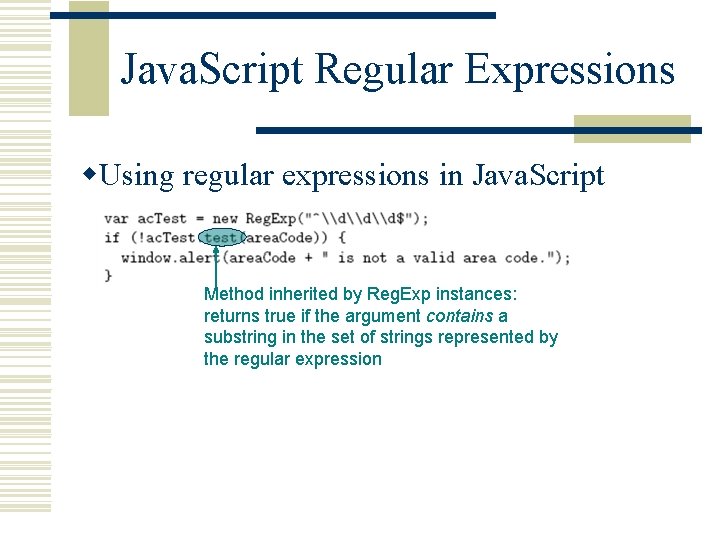
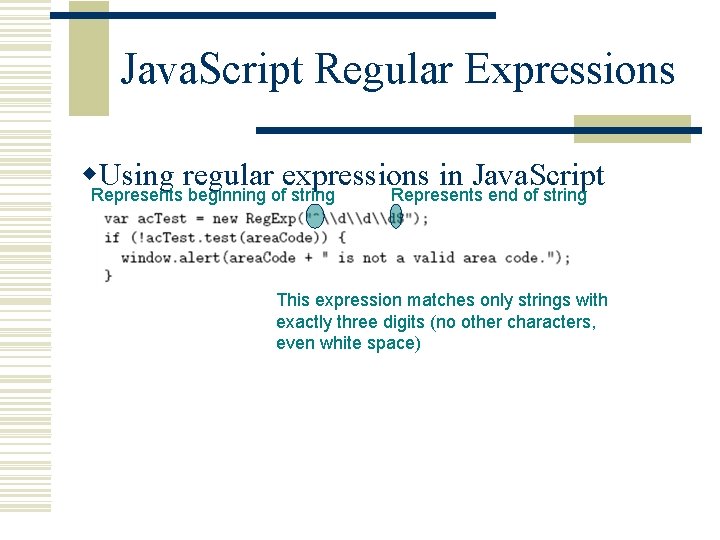
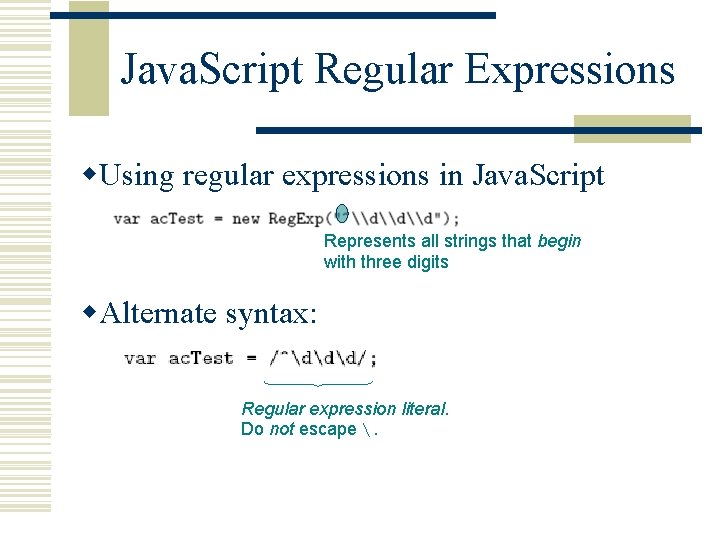
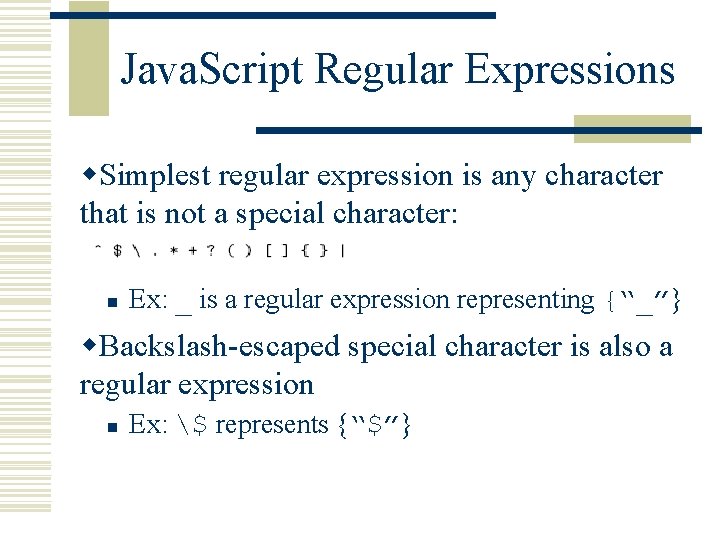
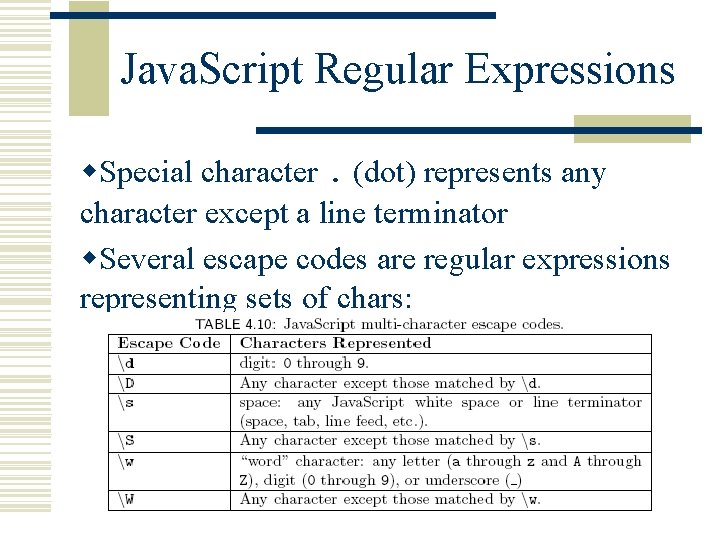
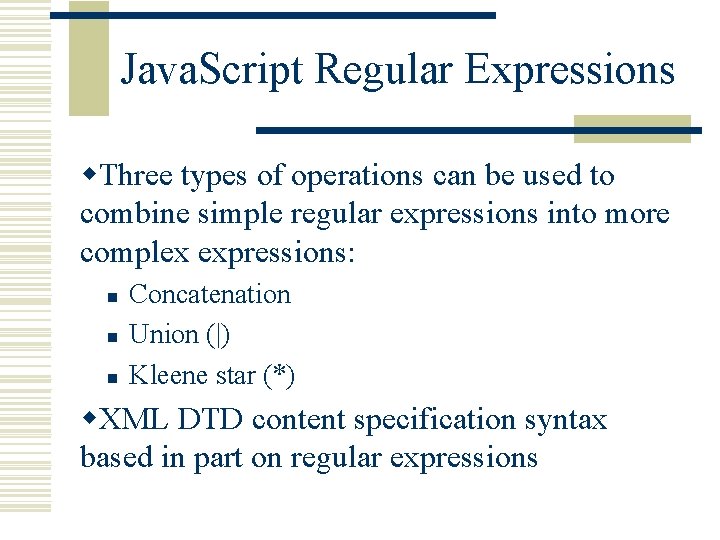
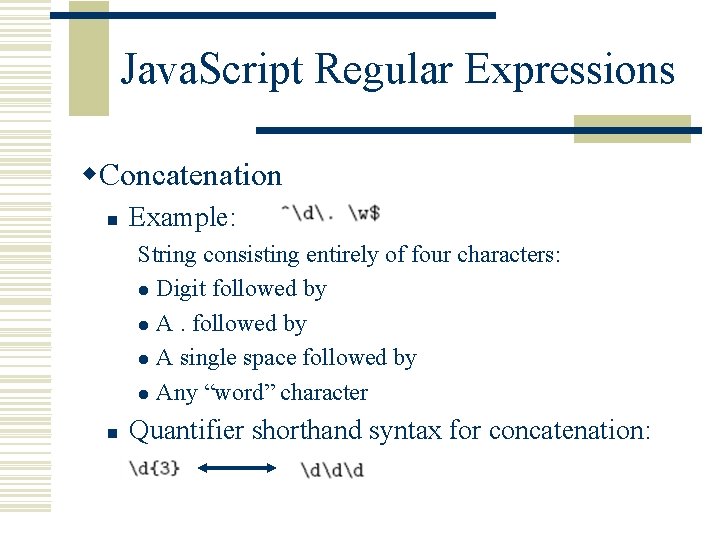
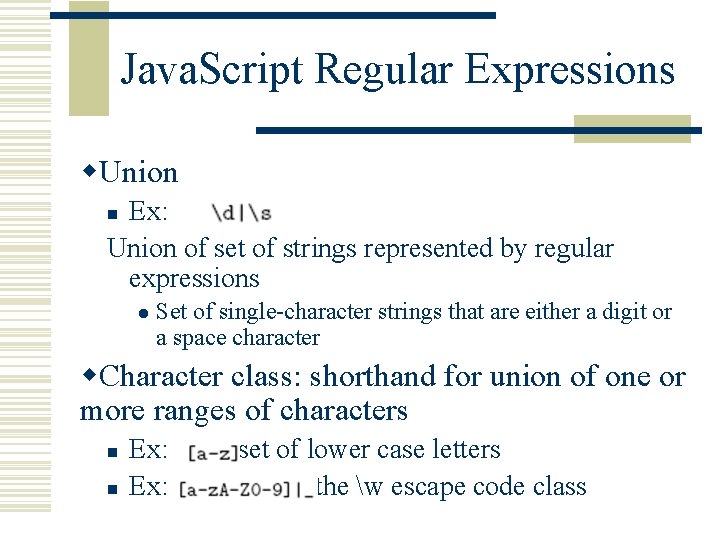
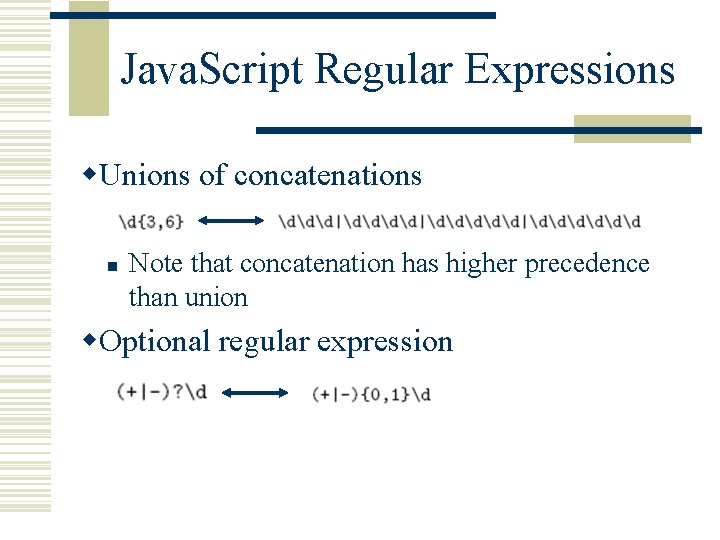
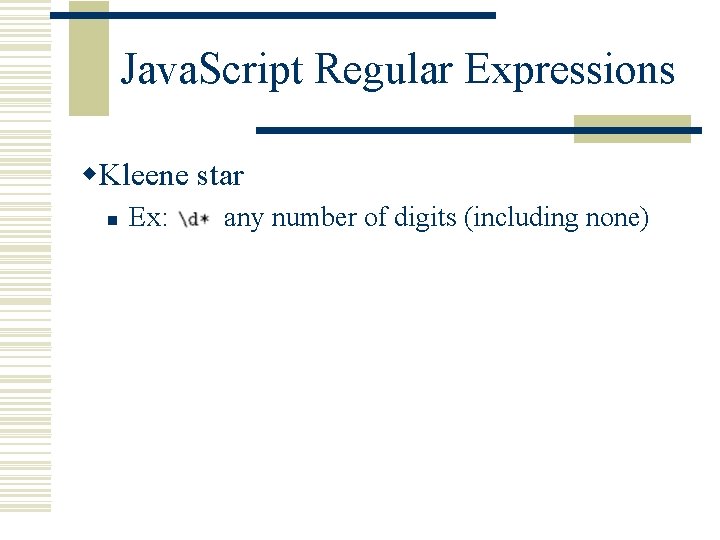
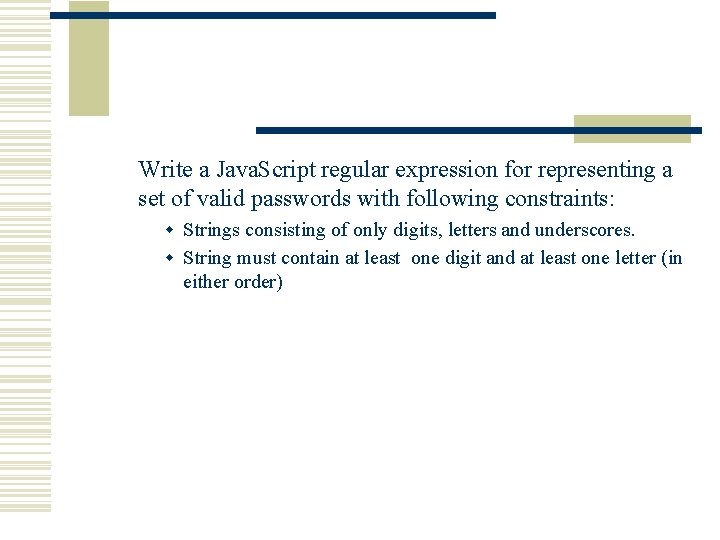
![ww*(dw*[a-z. A-Z]|[a-z. A-Z]w*d)w* ww*(dw*[a-z. A-Z]|[a-z. A-Z]w*d)w*](https://slidetodoc.com/presentation_image_h/181b3a96c0123f868ad0582743dbd6cd/image-153.jpg)
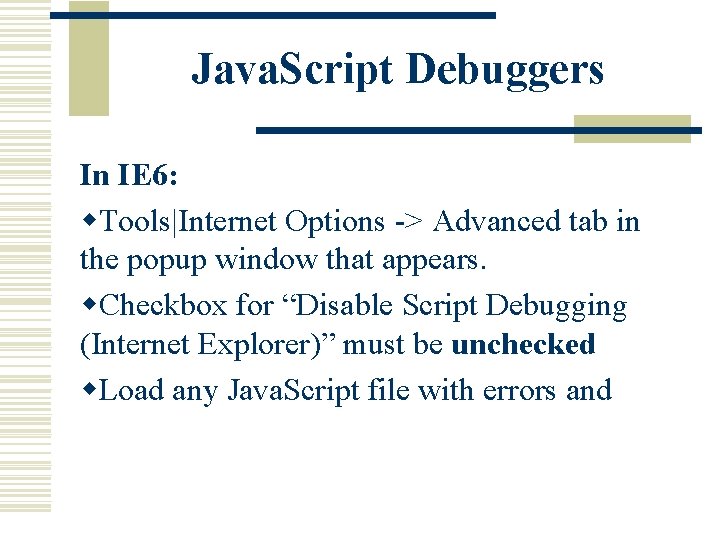
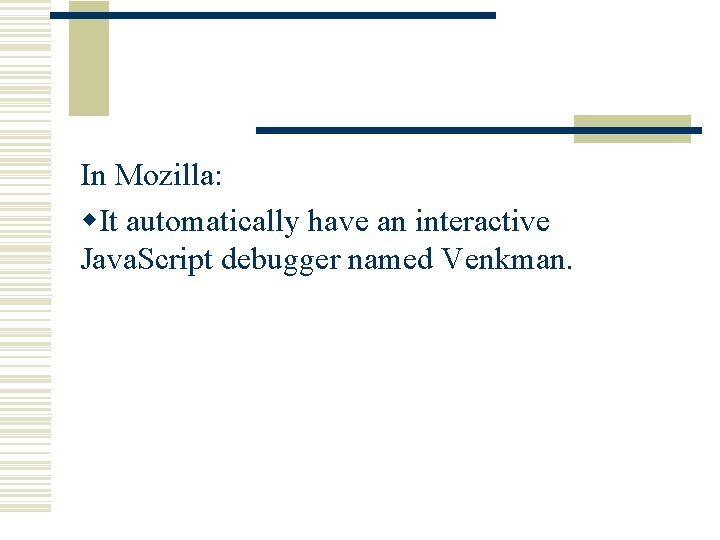
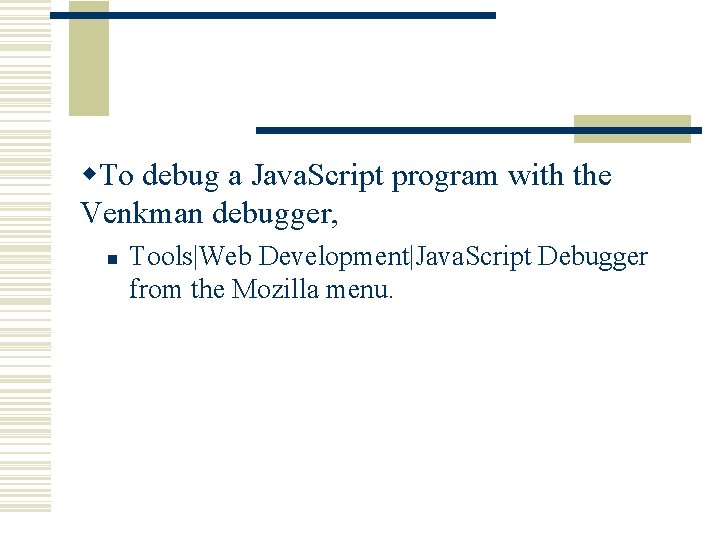
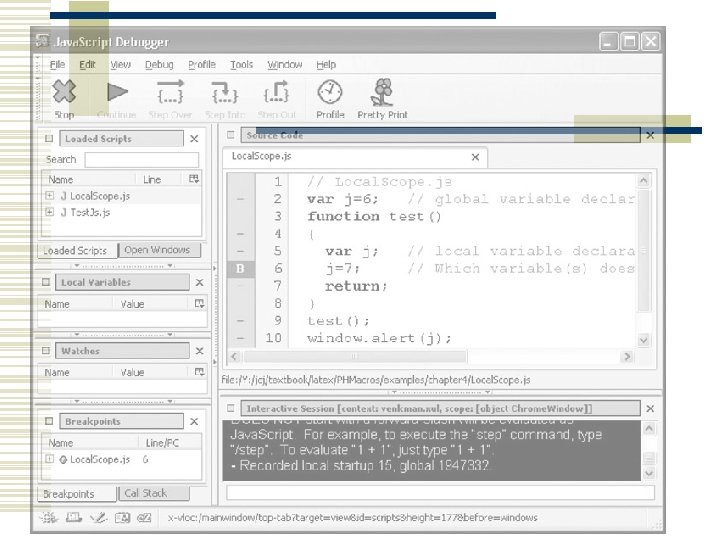
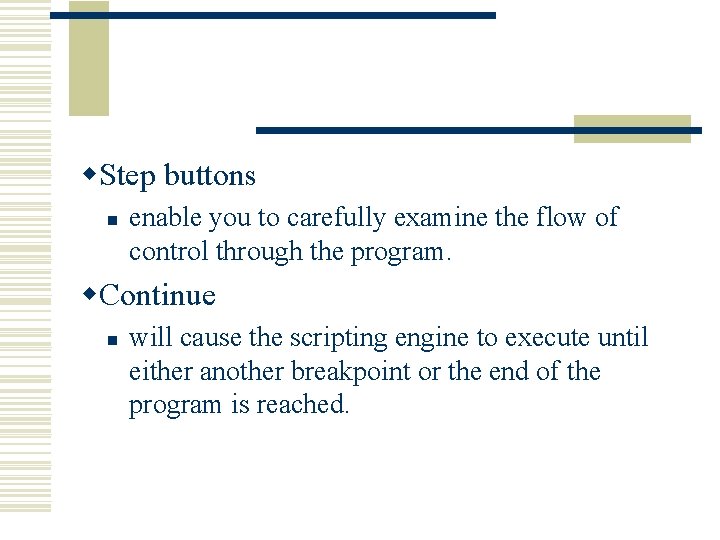
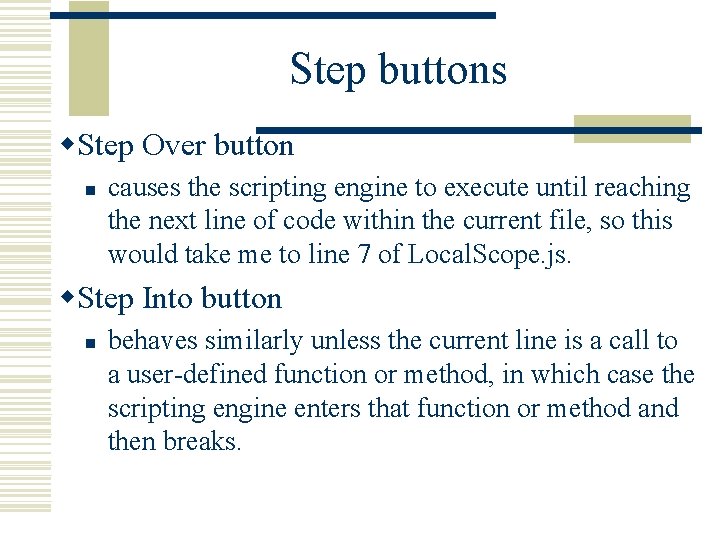
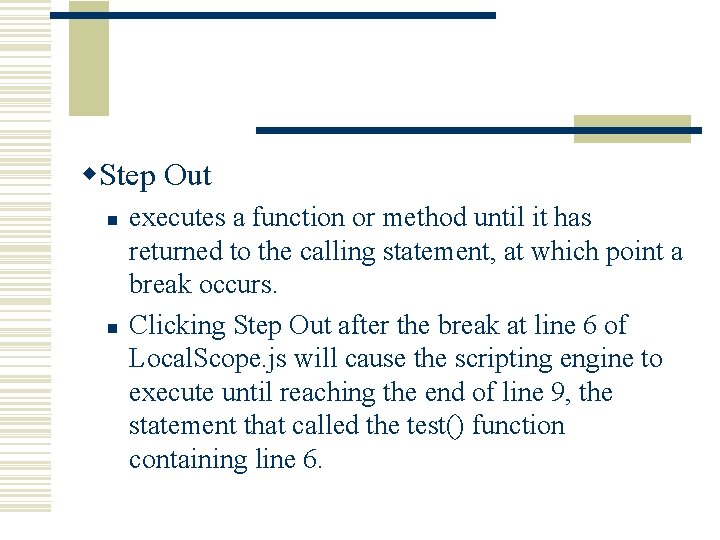
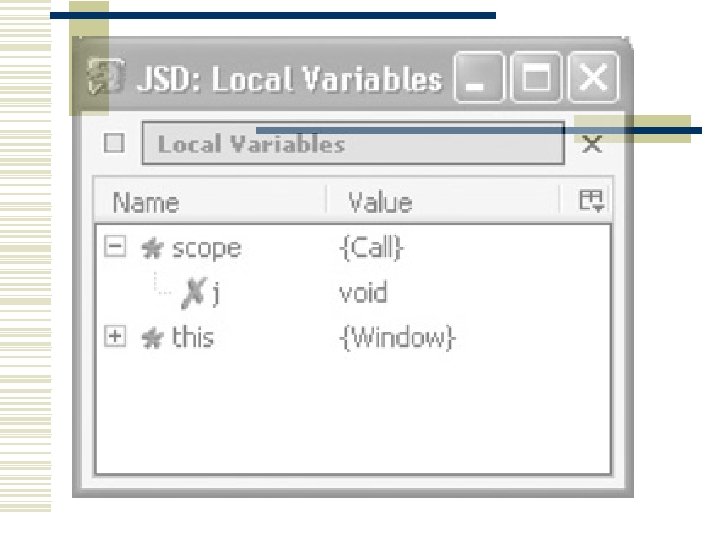
- Slides: 161
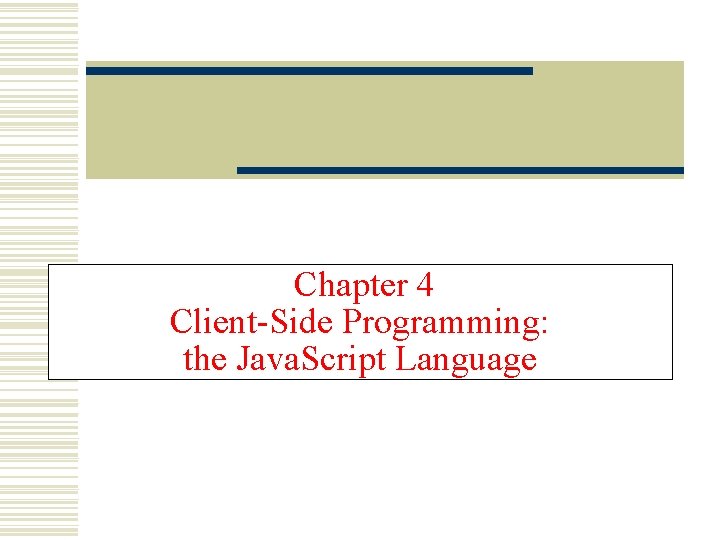
Chapter 4 Client-Side Programming: the Java. Script Language
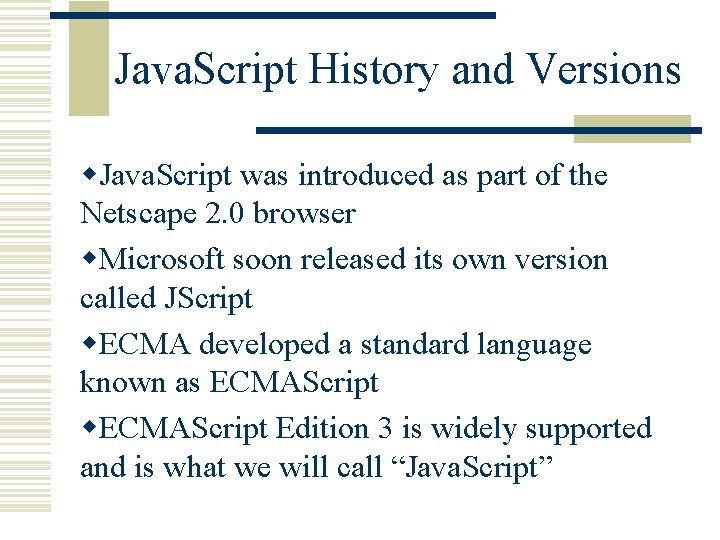
Java. Script History and Versions w. Java. Script was introduced as part of the Netscape 2. 0 browser w. Microsoft soon released its own version called JScript w. ECMA developed a standard language known as ECMAScript w. ECMAScript Edition 3 is widely supported and is what we will call “Java. Script”
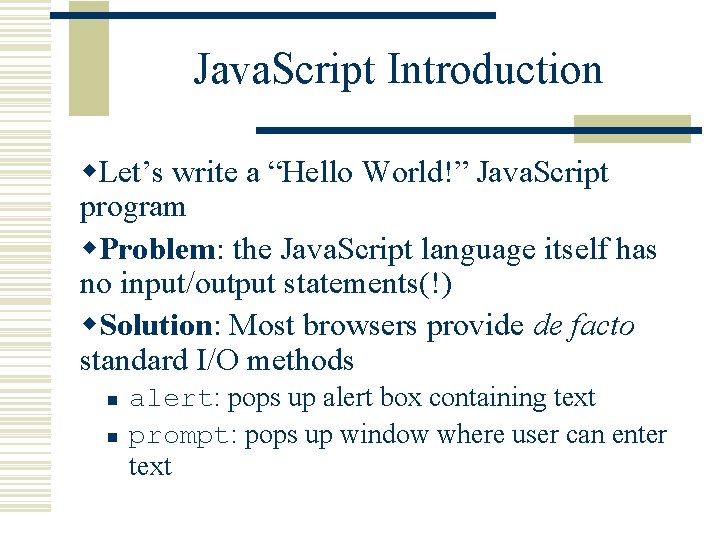
Java. Script Introduction w. Let’s write a “Hello World!” Java. Script program w. Problem: the Java. Script language itself has no input/output statements(!) w. Solution: Most browsers provide de facto standard I/O methods n n alert: pops up alert box containing text prompt: pops up window where user can enter text
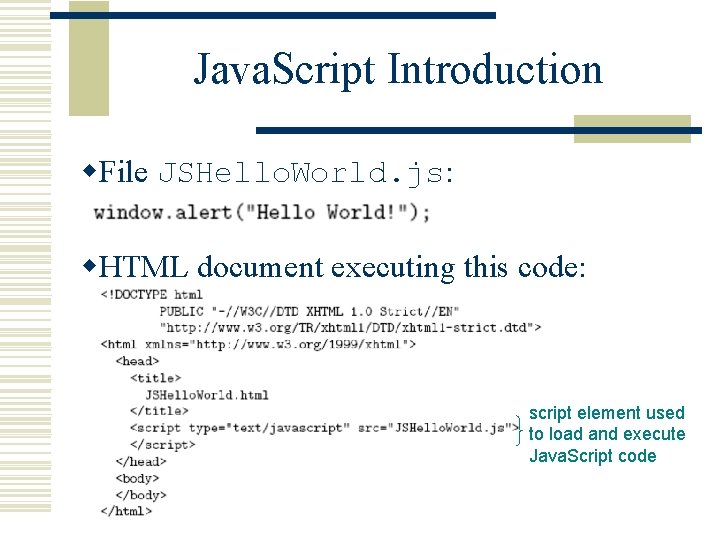
Java. Script Introduction w. File JSHello. World. js: w. HTML document executing this code: script element used to load and execute Java. Script code
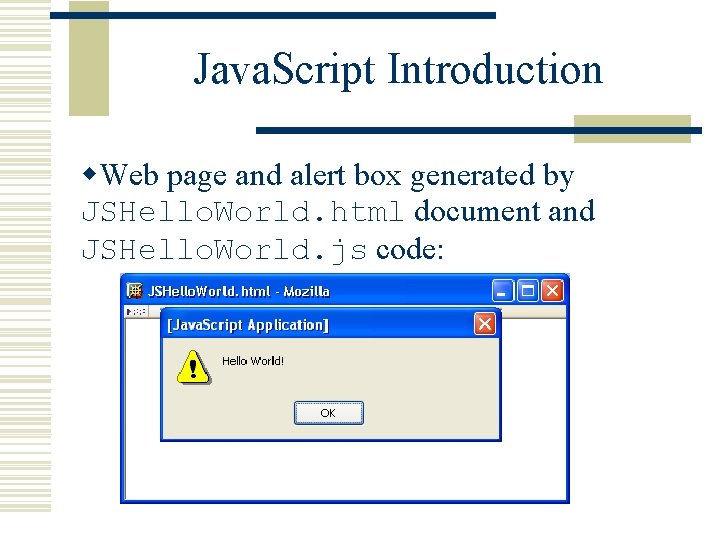
Java. Script Introduction w. Web page and alert box generated by JSHello. World. html document and JSHello. World. js code:
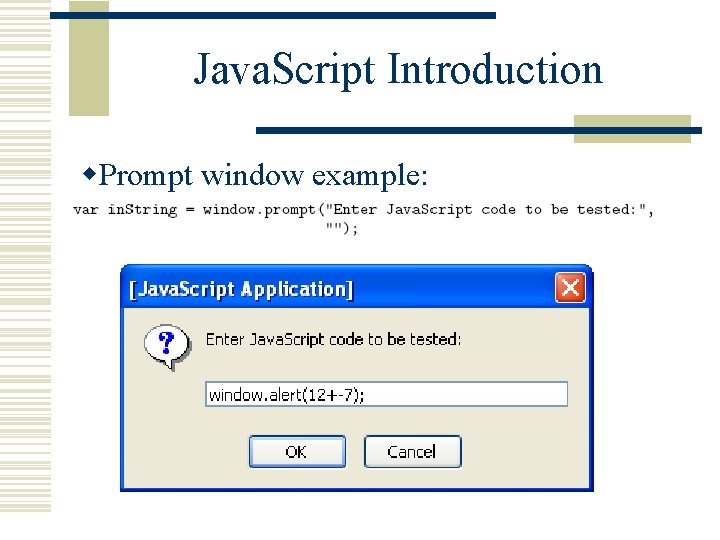
Java. Script Introduction w. Prompt window example:
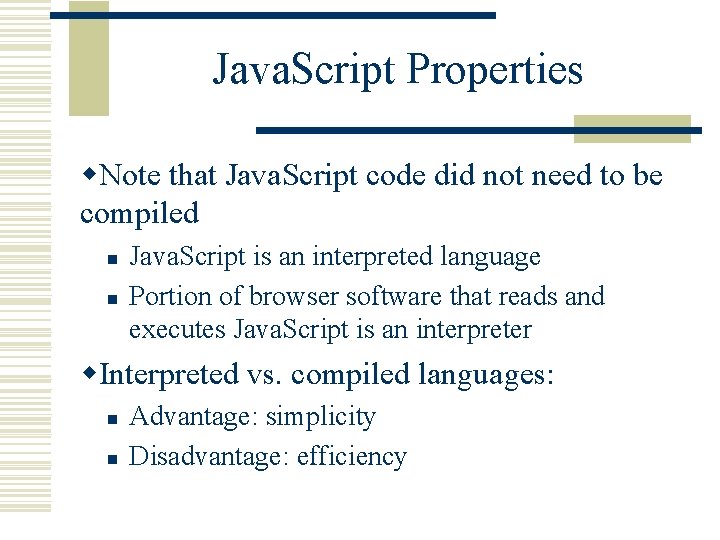
Java. Script Properties w. Note that Java. Script code did not need to be compiled n n Java. Script is an interpreted language Portion of browser software that reads and executes Java. Script is an interpreter w. Interpreted vs. compiled languages: n n Advantage: simplicity Disadvantage: efficiency
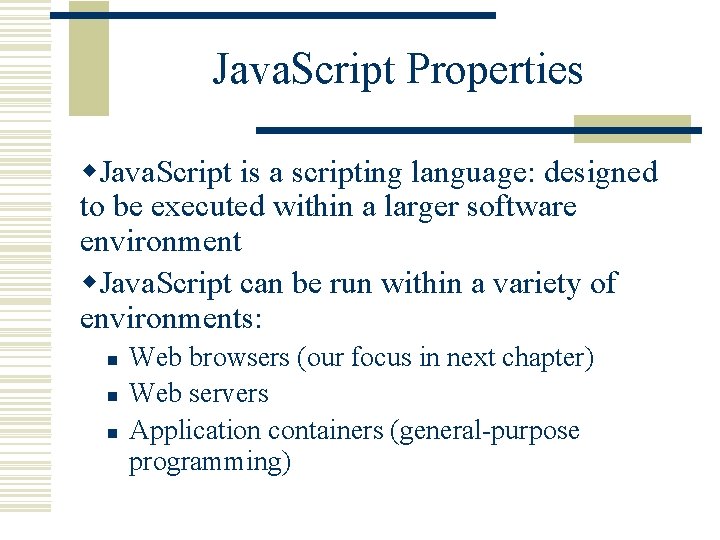
Java. Script Properties w. Java. Script is a scripting language: designed to be executed within a larger software environment w. Java. Script can be run within a variety of environments: n n n Web browsers (our focus in next chapter) Web servers Application containers (general-purpose programming)
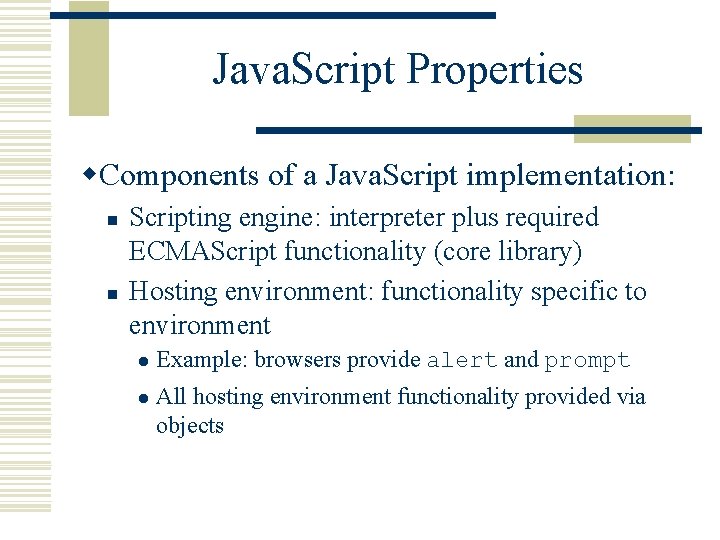
Java. Script Properties w. Components of a Java. Script implementation: n n Scripting engine: interpreter plus required ECMAScript functionality (core library) Hosting environment: functionality specific to environment l Example: browsers provide alert and prompt l All hosting environment functionality provided via objects
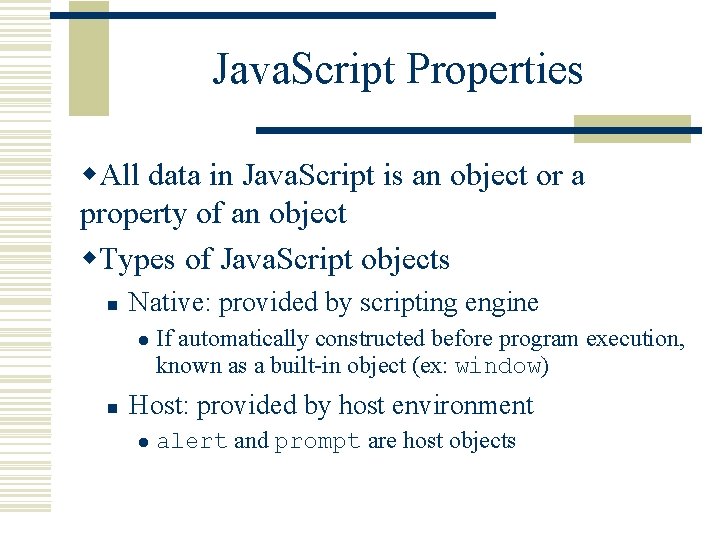
Java. Script Properties w. All data in Java. Script is an object or a property of an object w. Types of Java. Script objects n Native: provided by scripting engine l n If automatically constructed before program execution, known as a built-in object (ex: window) Host: provided by host environment l alert and prompt are host objects
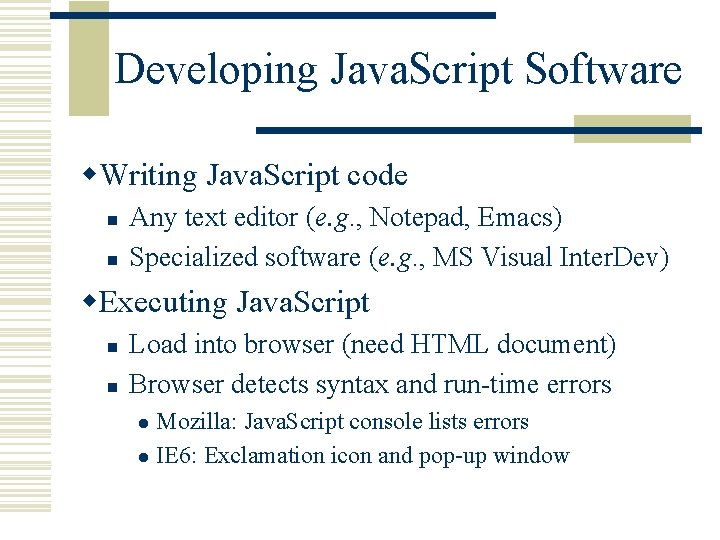
Developing Java. Script Software w. Writing Java. Script code n n Any text editor (e. g. , Notepad, Emacs) Specialized software (e. g. , MS Visual Inter. Dev) w. Executing Java. Script n n Load into browser (need HTML document) Browser detects syntax and run-time errors Mozilla: Java. Script console lists errors l IE 6: Exclamation icon and pop-up window l
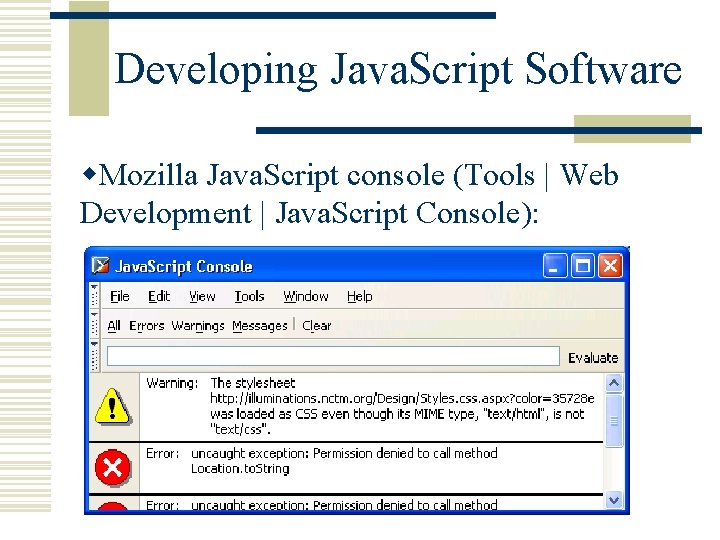
Developing Java. Script Software w. Mozilla Java. Script console (Tools | Web Development | Java. Script Console):
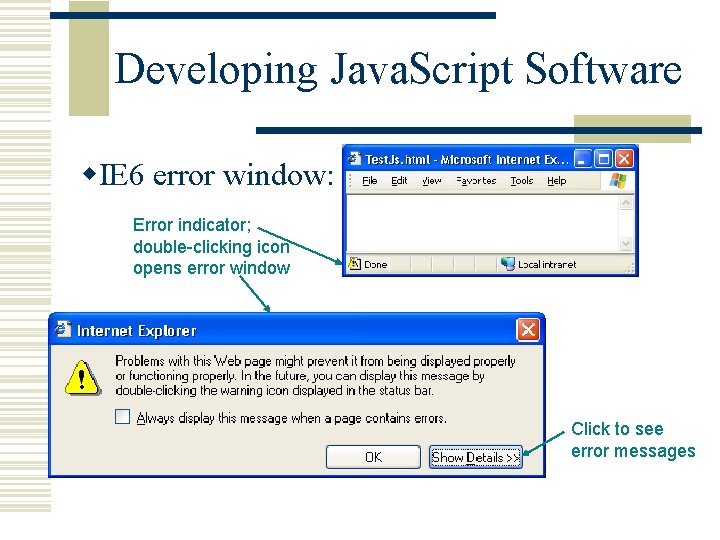
Developing Java. Script Software w. IE 6 error window: Error indicator; double-clicking icon opens error window Click to see error messages
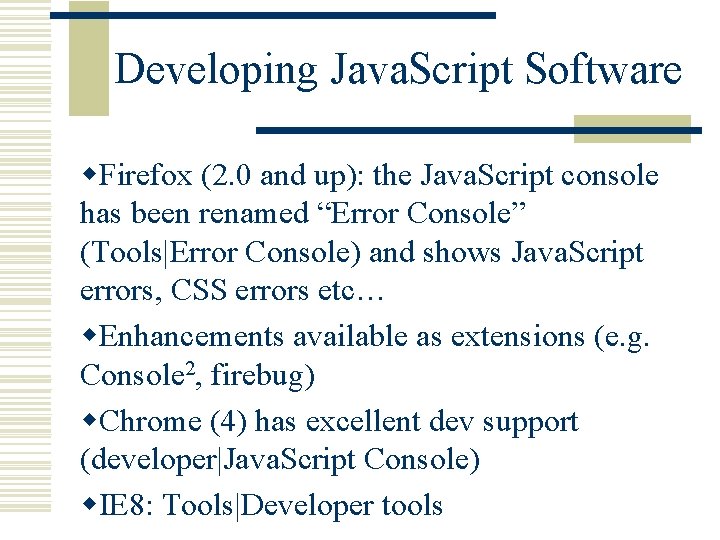
Developing Java. Script Software w. Firefox (2. 0 and up): the Java. Script console has been renamed “Error Console” (Tools|Error Console) and shows Java. Script errors, CSS errors etc… w. Enhancements available as extensions (e. g. Console 2, firebug) w. Chrome (4) has excellent dev support (developer|Java. Script Console) w. IE 8: Tools|Developer tools
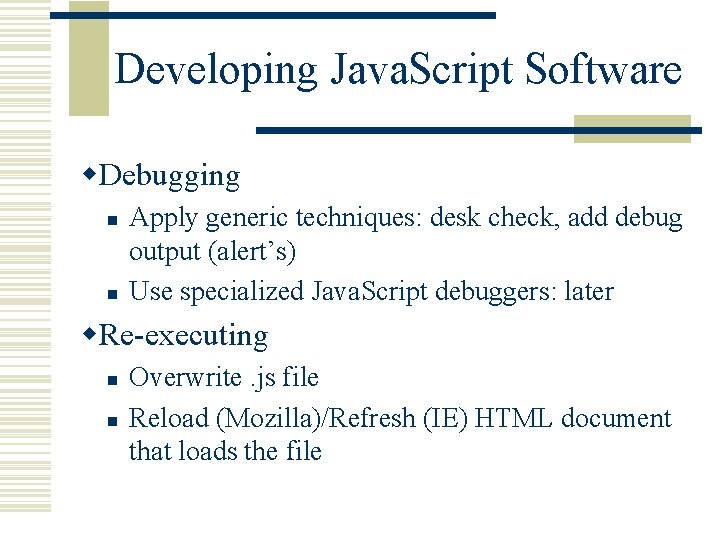
Developing Java. Script Software w. Debugging n n Apply generic techniques: desk check, add debug output (alert’s) Use specialized Java. Script debuggers: later w. Re-executing n n Overwrite. js file Reload (Mozilla)/Refresh (IE) HTML document that loads the file
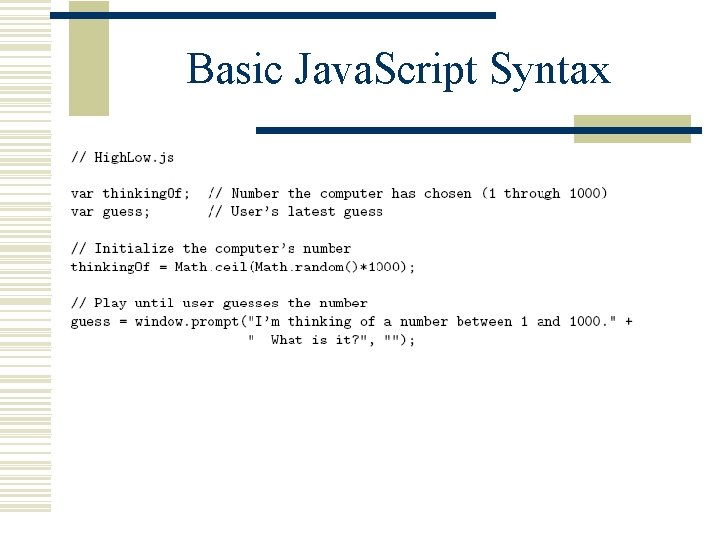
Basic Java. Script Syntax
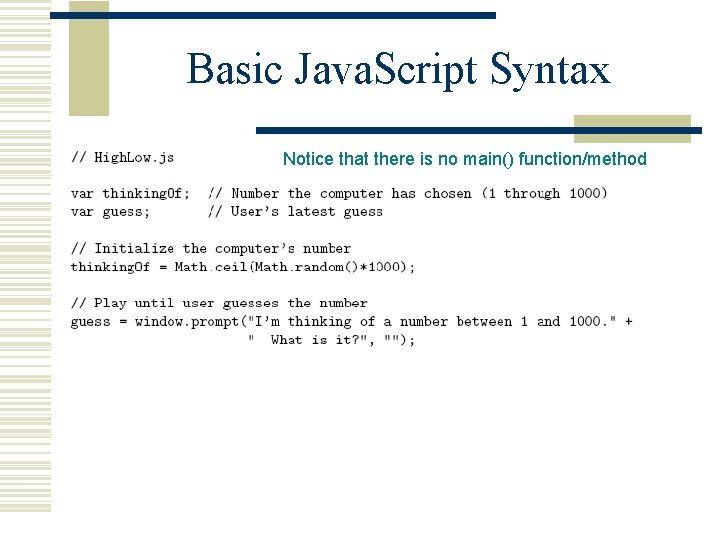
Basic Java. Script Syntax Notice that there is no main() function/method
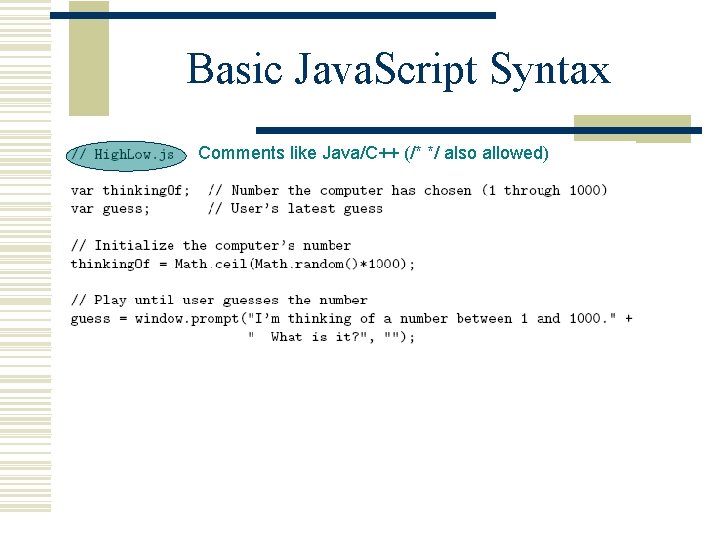
Basic Java. Script Syntax Comments like Java/C++ (/* */ also allowed)
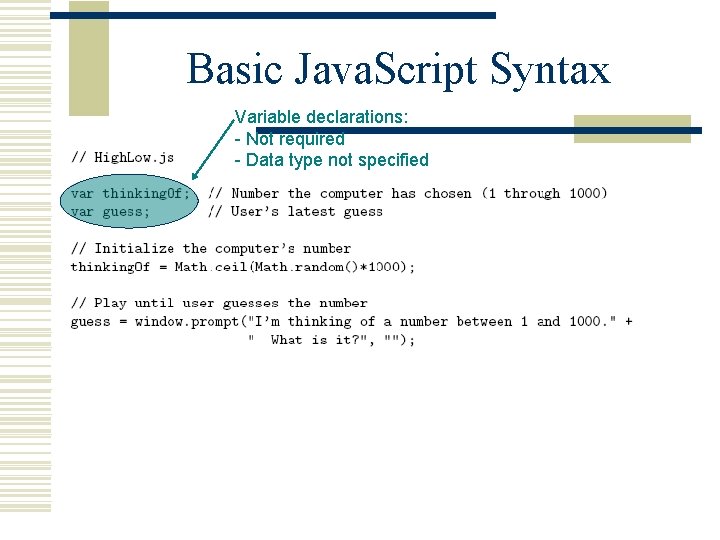
Basic Java. Script Syntax Variable declarations: - Not required - Data type not specified
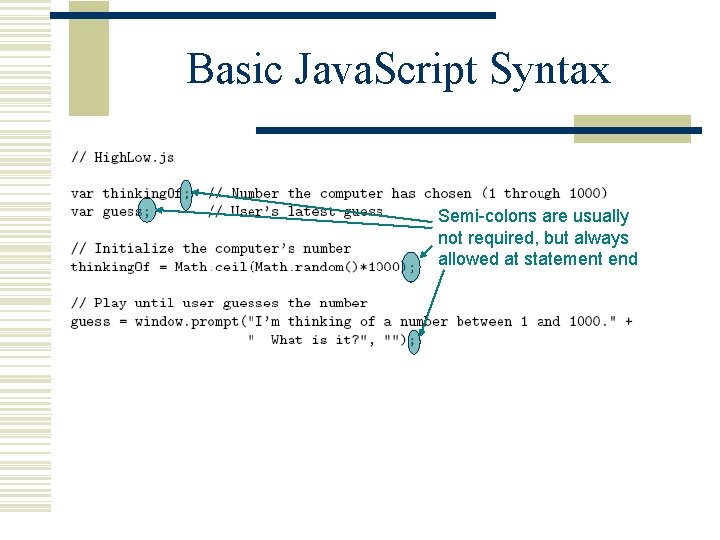
Basic Java. Script Syntax Semi-colons are usually not required, but always allowed at statement end
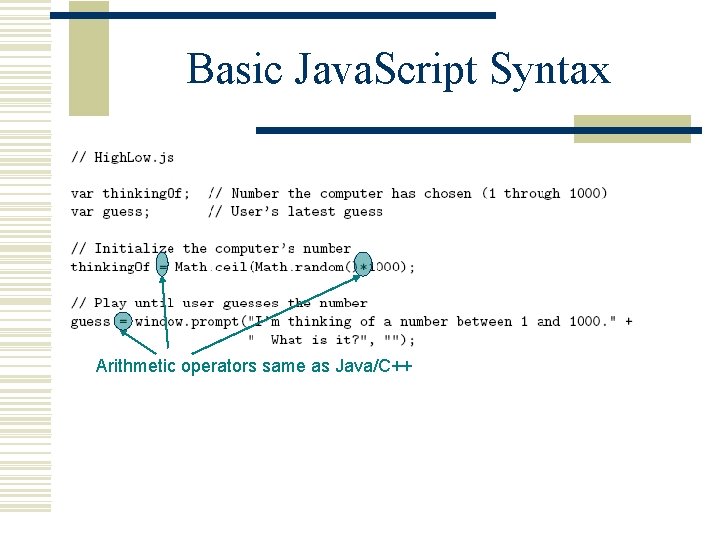
Basic Java. Script Syntax Arithmetic operators same as Java/C++
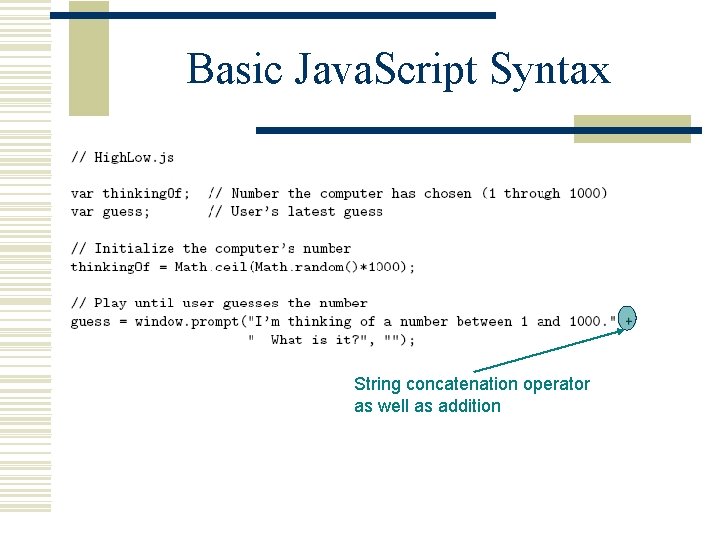
Basic Java. Script Syntax String concatenation operator as well as addition
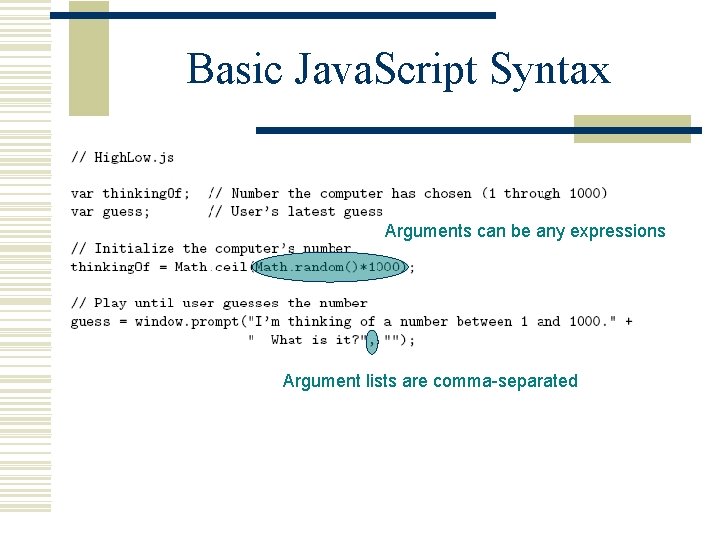
Basic Java. Script Syntax Arguments can be any expressions Argument lists are comma-separated
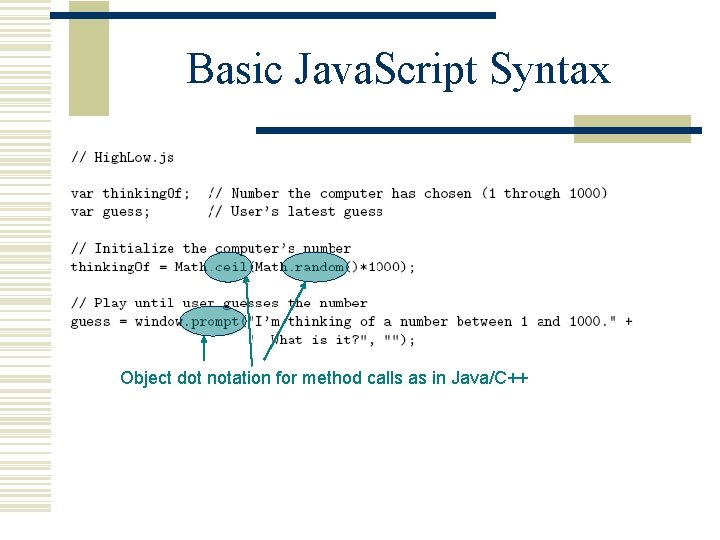
Basic Java. Script Syntax Object dot notation for method calls as in Java/C++
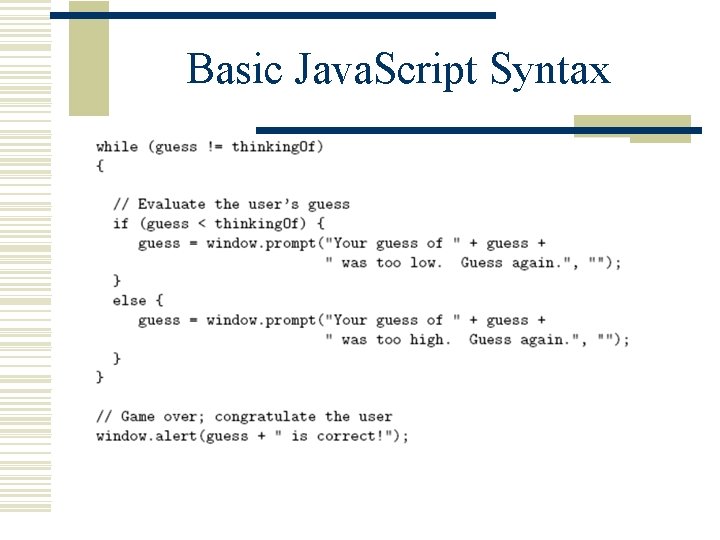
Basic Java. Script Syntax
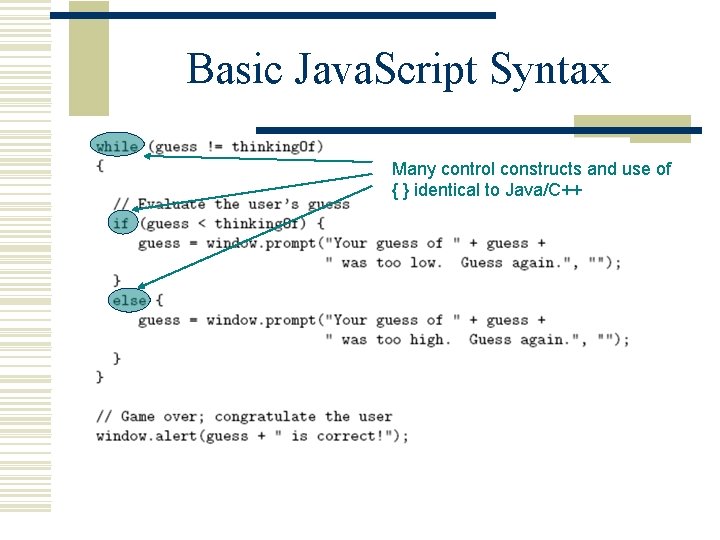
Basic Java. Script Syntax Many control constructs and use of { } identical to Java/C++
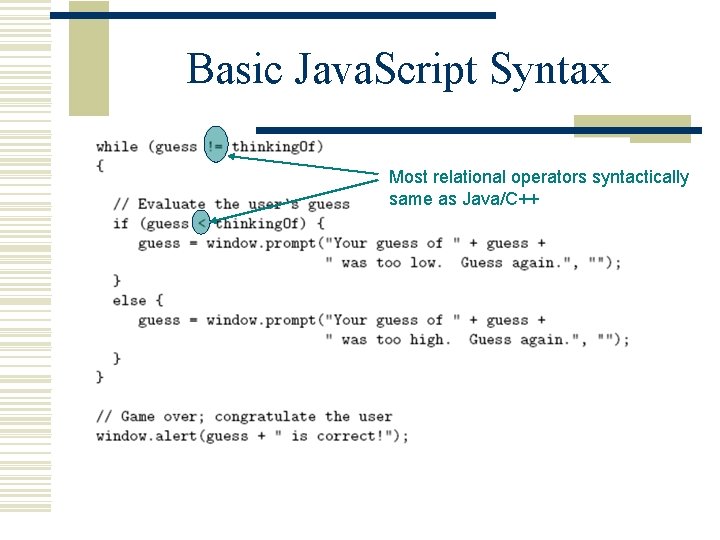
Basic Java. Script Syntax Most relational operators syntactically same as Java/C++
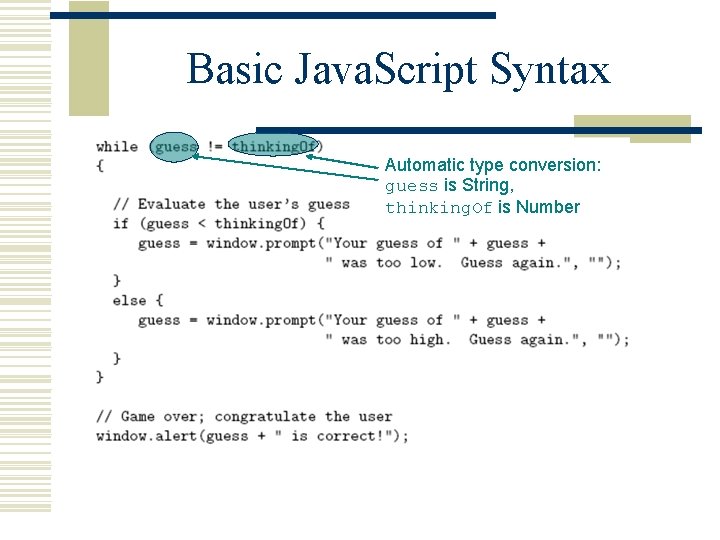
Basic Java. Script Syntax Automatic type conversion: guess is String, thinking. Of is Number
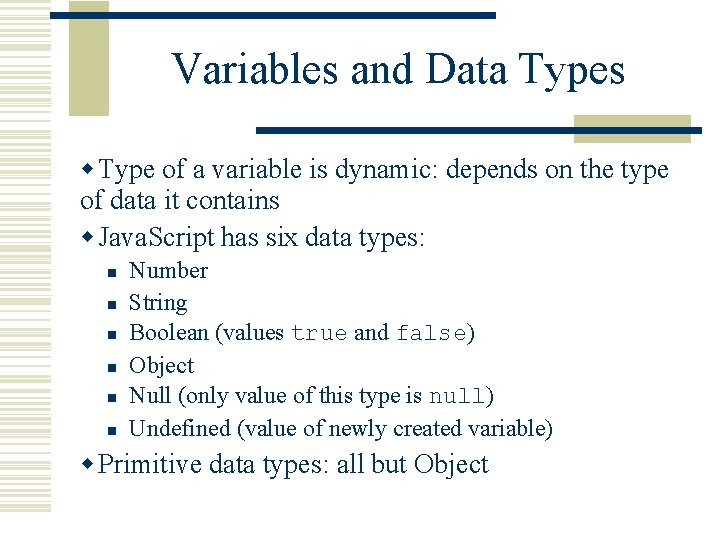
Variables and Data Types w Type of a variable is dynamic: depends on the type of data it contains w Java. Script has six data types: n n n Number String Boolean (values true and false) Object Null (only value of this type is null) Undefined (value of newly created variable) w Primitive data types: all but Object
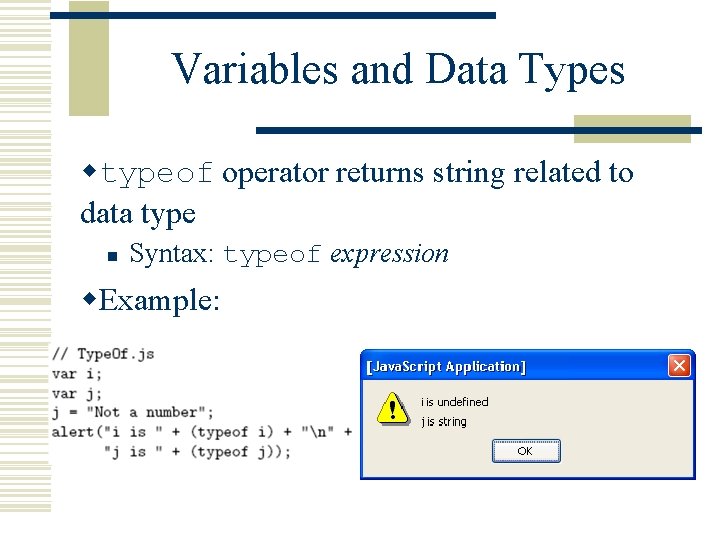
Variables and Data Types wtypeof operator returns string related to data type n Syntax: typeof expression w. Example:
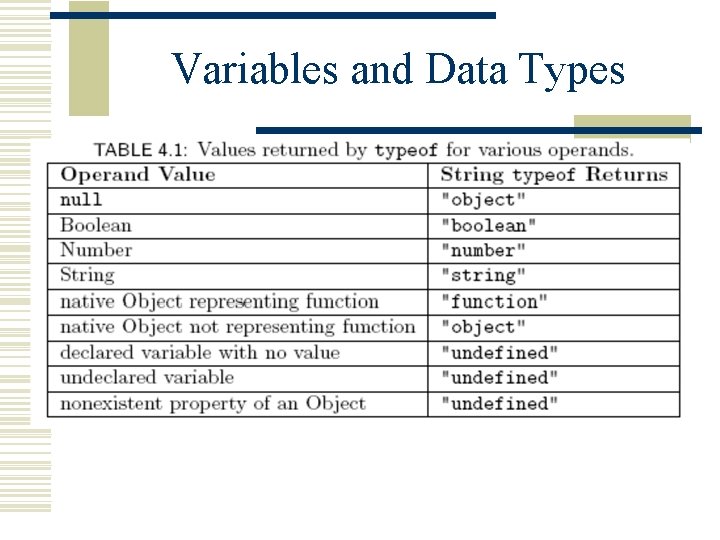
Variables and Data Types
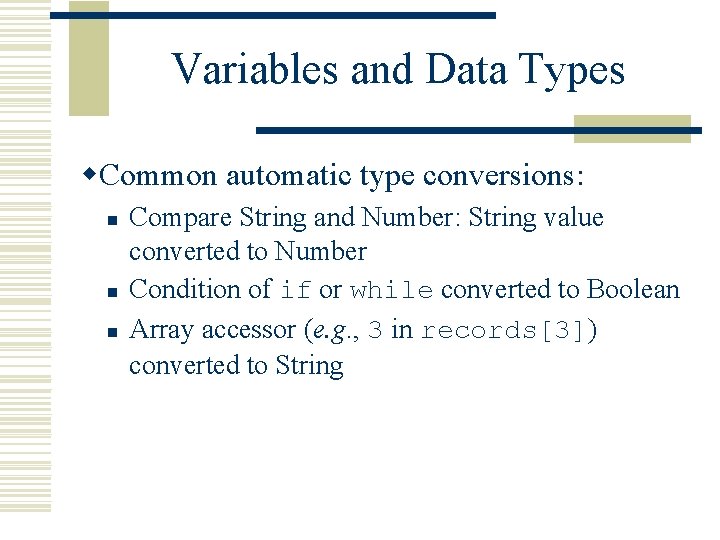
Variables and Data Types w. Common automatic type conversions: n n n Compare String and Number: String value converted to Number Condition of if or while converted to Boolean Array accessor (e. g. , 3 in records[3]) converted to String
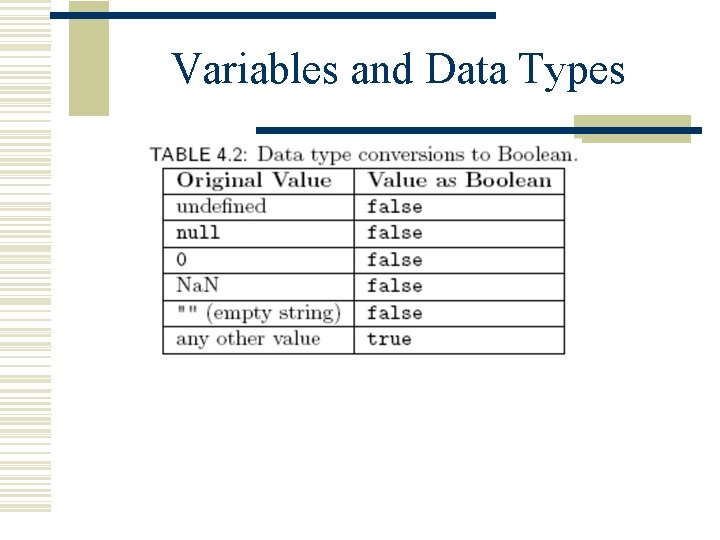
Variables and Data Types
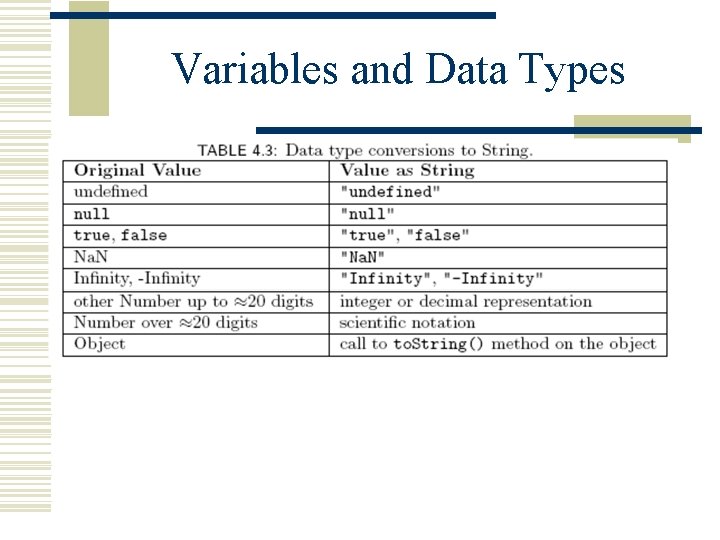
Variables and Data Types
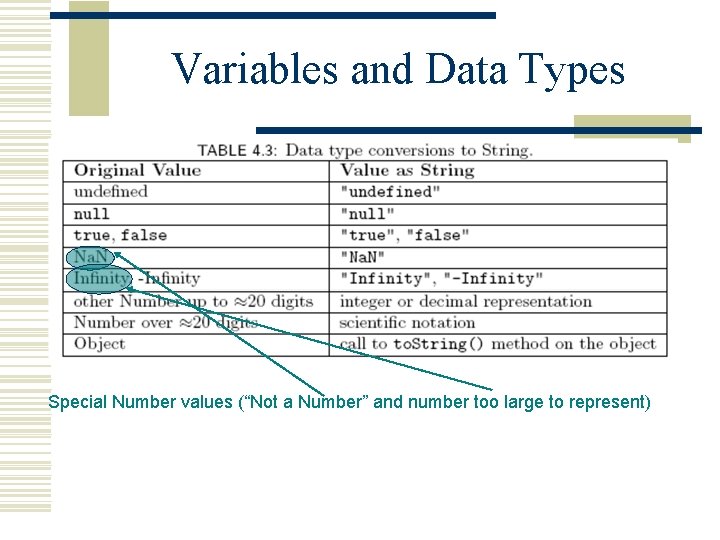
Variables and Data Types Special Number values (“Not a Number” and number too large to represent)
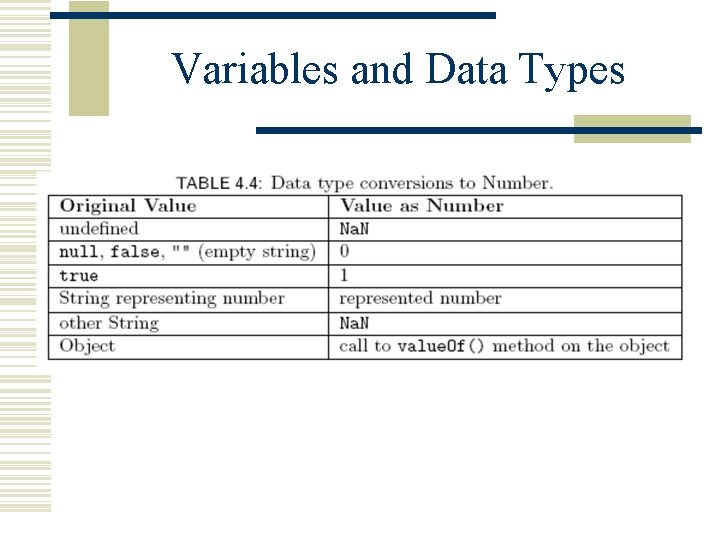
Variables and Data Types
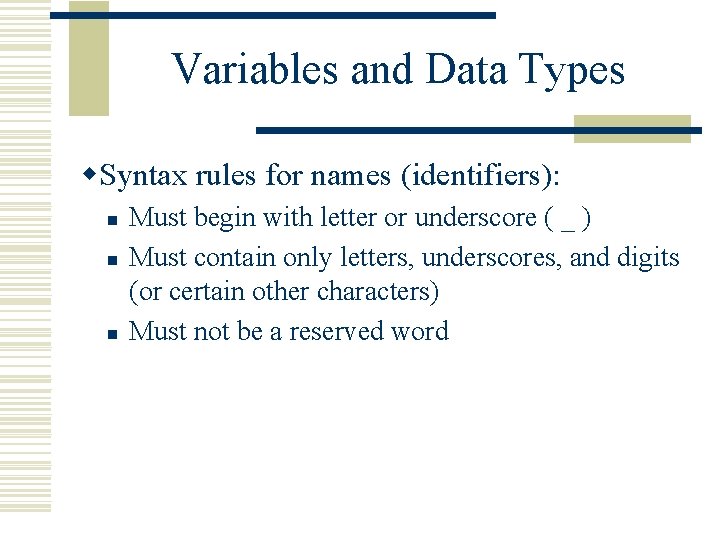
Variables and Data Types w. Syntax rules for names (identifiers): n n n Must begin with letter or underscore ( _ ) Must contain only letters, underscores, and digits (or certain other characters) Must not be a reserved word
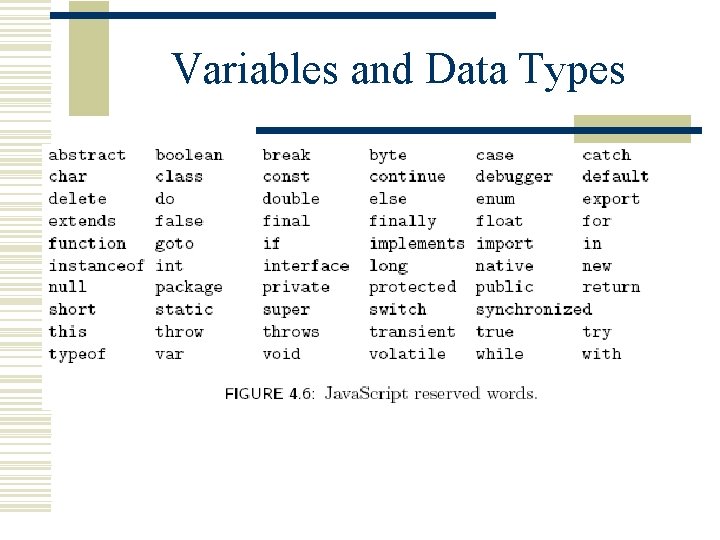
Variables and Data Types
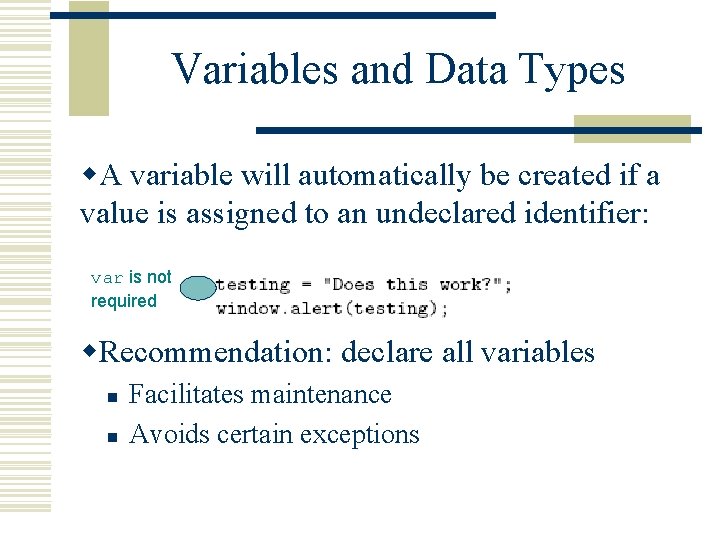
Variables and Data Types w. A variable will automatically be created if a value is assigned to an undeclared identifier: var is not required w. Recommendation: declare all variables n n Facilitates maintenance Avoids certain exceptions
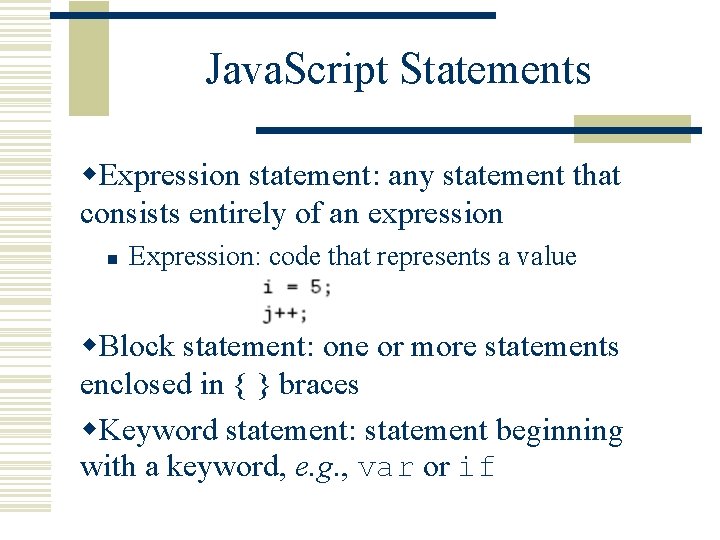
Java. Script Statements w. Expression statement: any statement that consists entirely of an expression n Expression: code that represents a value w. Block statement: one or more statements enclosed in { } braces w. Keyword statement: statement beginning with a keyword, e. g. , var or if
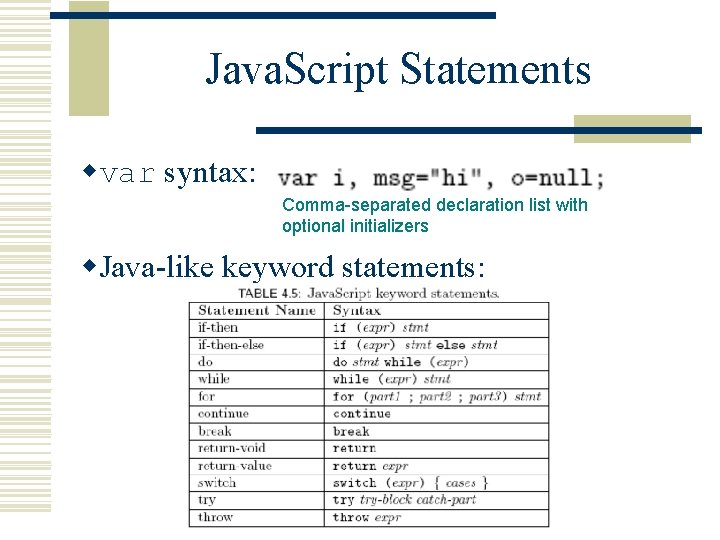
Java. Script Statements wvar syntax: Comma-separated declaration list with optional initializers w. Java-like keyword statements:
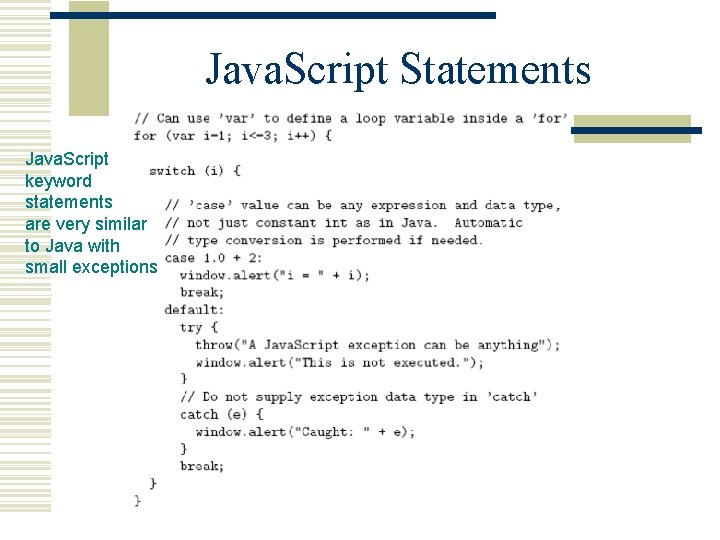
Java. Script Statements Java. Script keyword statements are very similar to Java with small exceptions
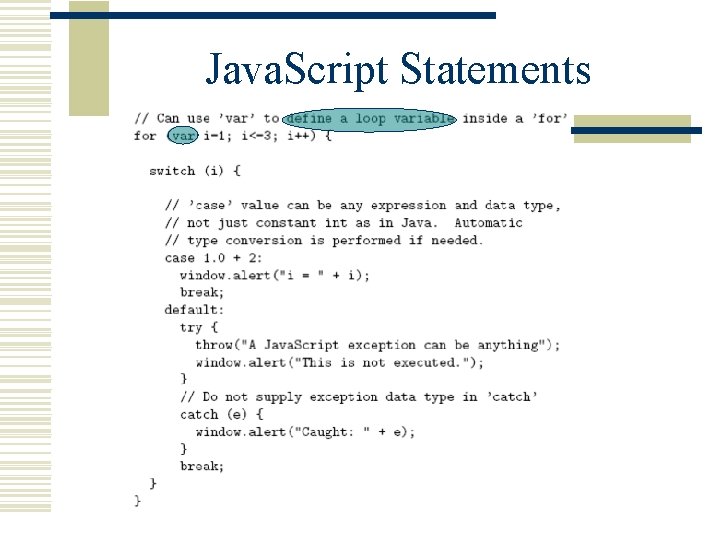
Java. Script Statements
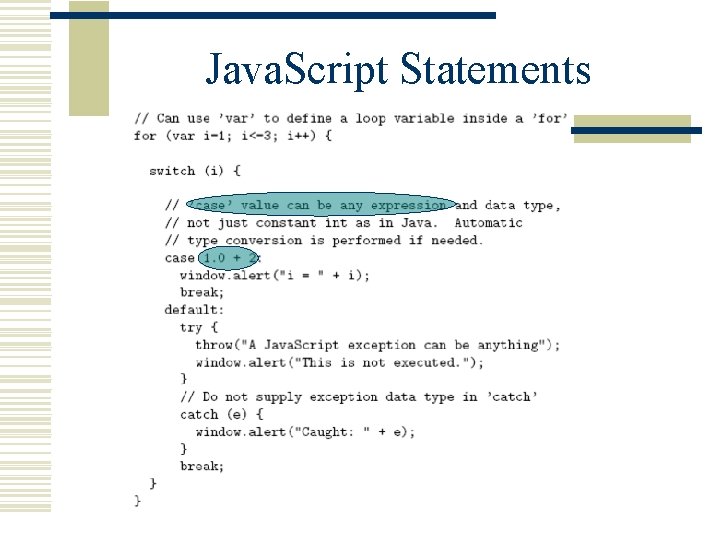
Java. Script Statements
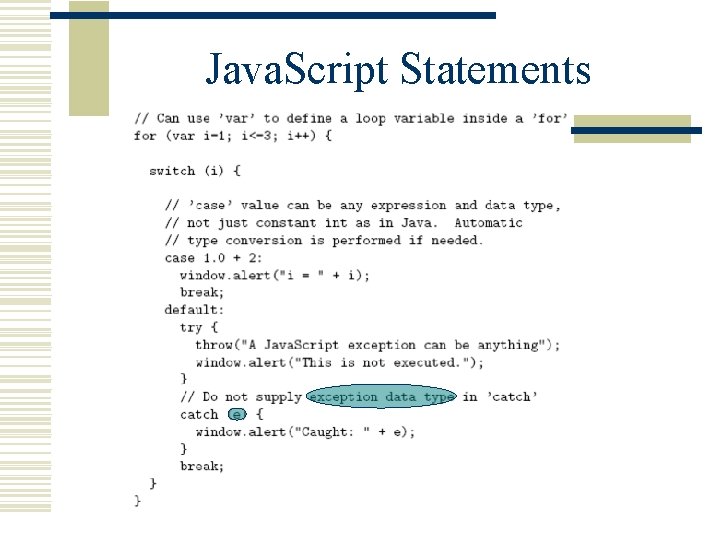
Java. Script Statements
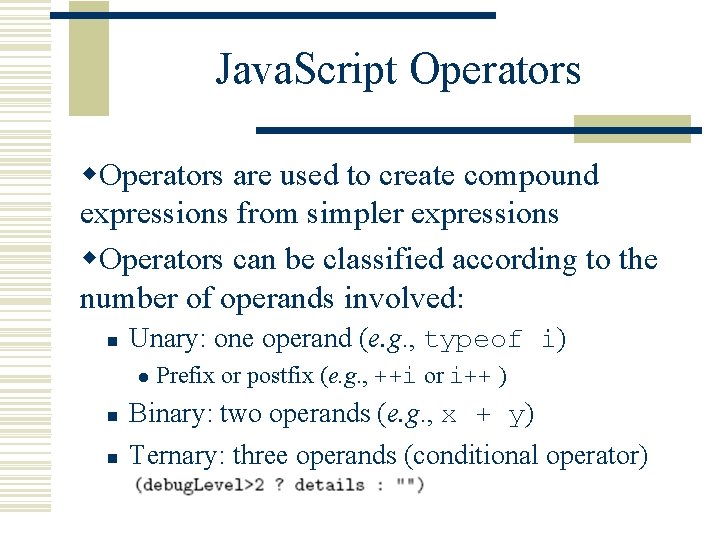
Java. Script Operators w. Operators are used to create compound expressions from simpler expressions w. Operators can be classified according to the number of operands involved: n Unary: one operand (e. g. , typeof i) l Prefix or postfix (e. g. , ++i or i++ ) n Binary: two operands (e. g. , x + y) n Ternary: three operands (conditional operator)
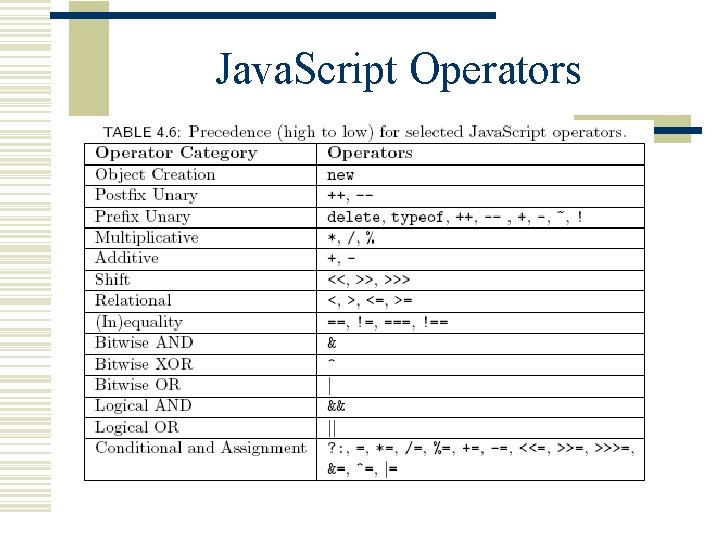
Java. Script Operators
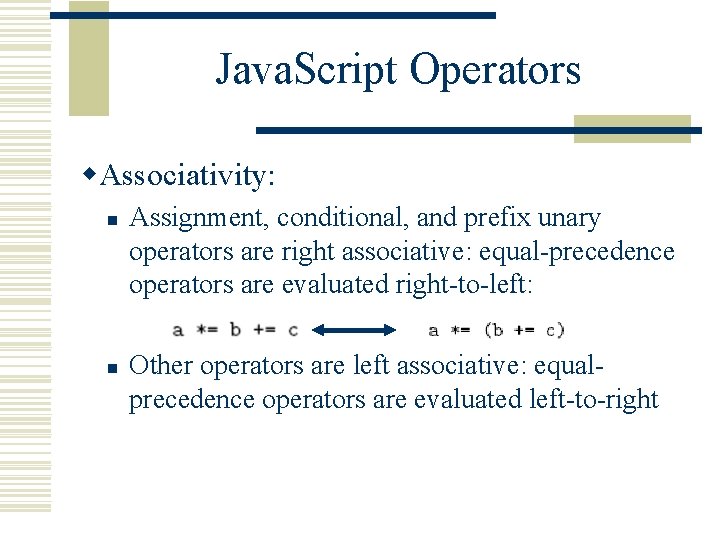
Java. Script Operators w. Associativity: n n Assignment, conditional, and prefix unary operators are right associative: equal-precedence operators are evaluated right-to-left: Other operators are left associative: equalprecedence operators are evaluated left-to-right
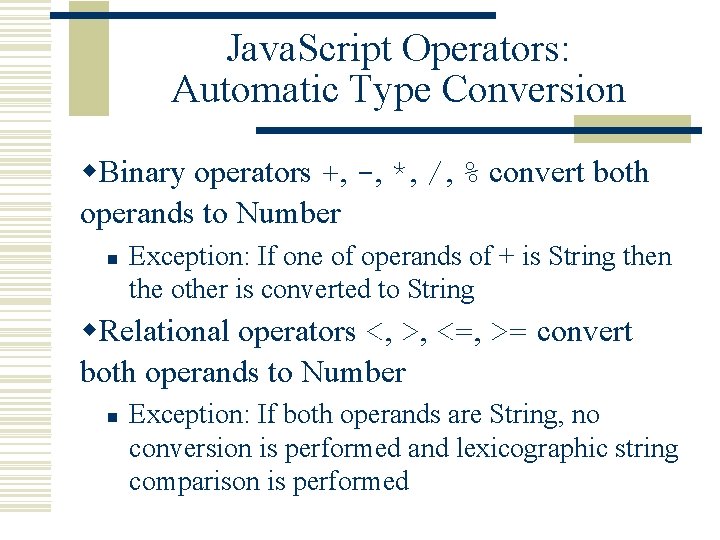
Java. Script Operators: Automatic Type Conversion w. Binary operators +, -, *, /, % convert both operands to Number n Exception: If one of operands of + is String then the other is converted to String w. Relational operators <, >, <=, >= convert both operands to Number n Exception: If both operands are String, no conversion is performed and lexicographic string comparison is performed
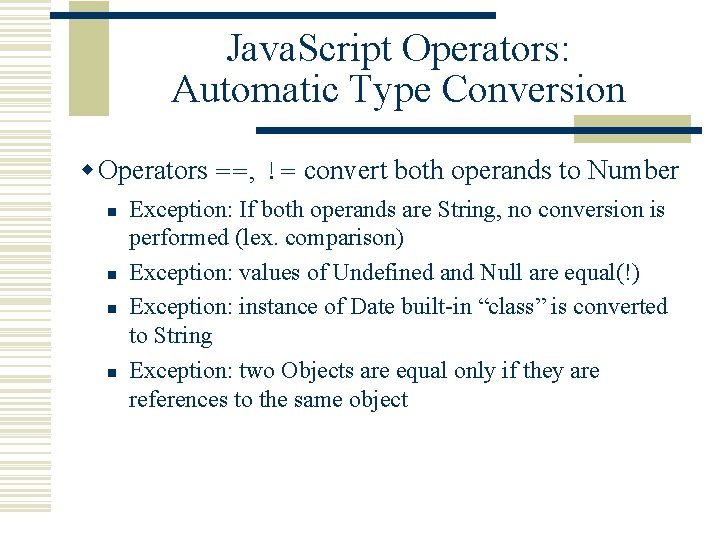
Java. Script Operators: Automatic Type Conversion w Operators ==, != convert both operands to Number n n Exception: If both operands are String, no conversion is performed (lex. comparison) Exception: values of Undefined and Null are equal(!) Exception: instance of Date built-in “class” is converted to String Exception: two Objects are equal only if they are references to the same object
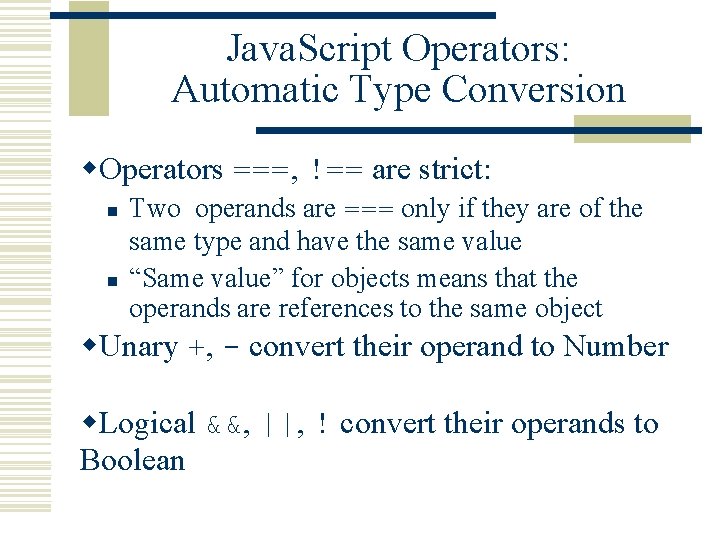
Java. Script Operators: Automatic Type Conversion w. Operators ===, !== are strict: n n Two operands are === only if they are of the same type and have the same value “Same value” for objects means that the operands are references to the same object w. Unary +, - convert their operand to Number w. Logical &&, ||, ! convert their operands to Boolean
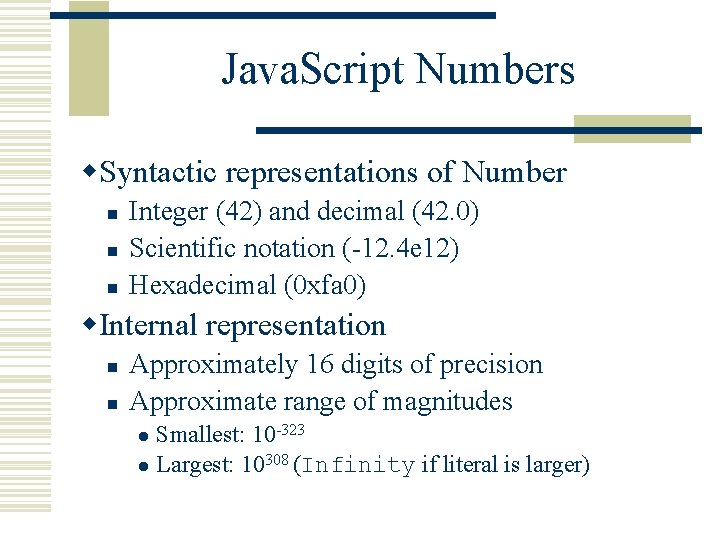
Java. Script Numbers w. Syntactic representations of Number n n n Integer (42) and decimal (42. 0) Scientific notation (-12. 4 e 12) Hexadecimal (0 xfa 0) w. Internal representation n n Approximately 16 digits of precision Approximate range of magnitudes Smallest: 10 -323 l Largest: 10308 (Infinity if literal is larger) l
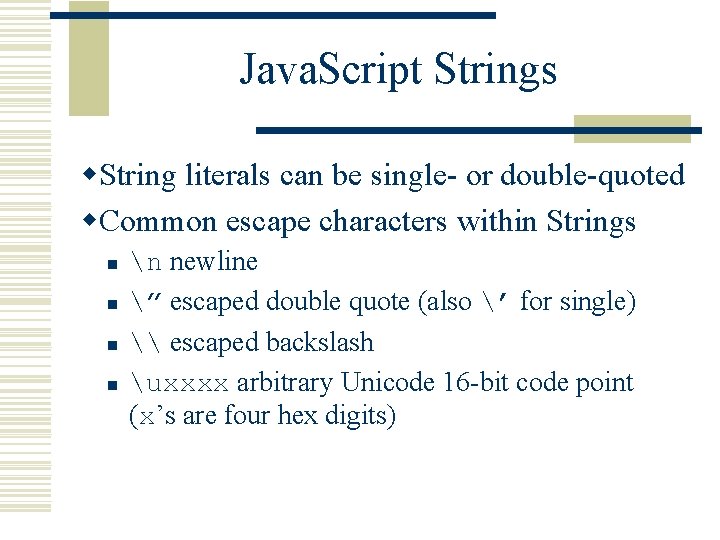
Java. Script Strings w. String literals can be single- or double-quoted w. Common escape characters within Strings n n n newline ” escaped double quote (also ’ for single) \ escaped backslash uxxxx arbitrary Unicode 16 -bit code point (x’s are four hex digits)
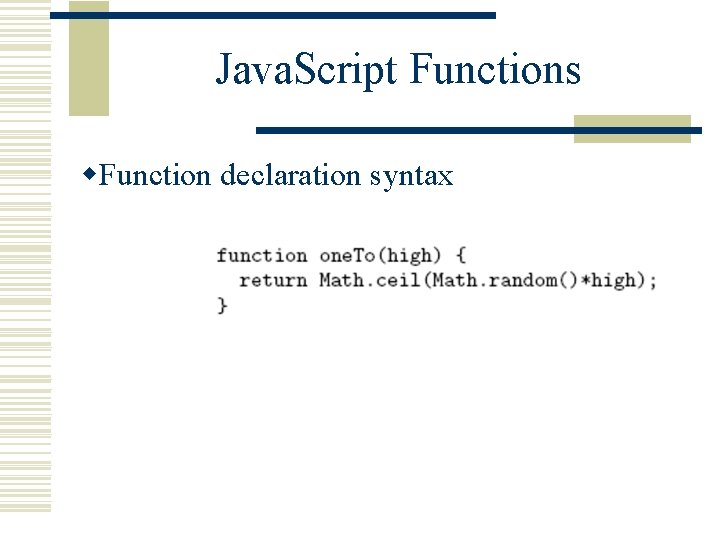
Java. Script Functions w. Function declaration syntax
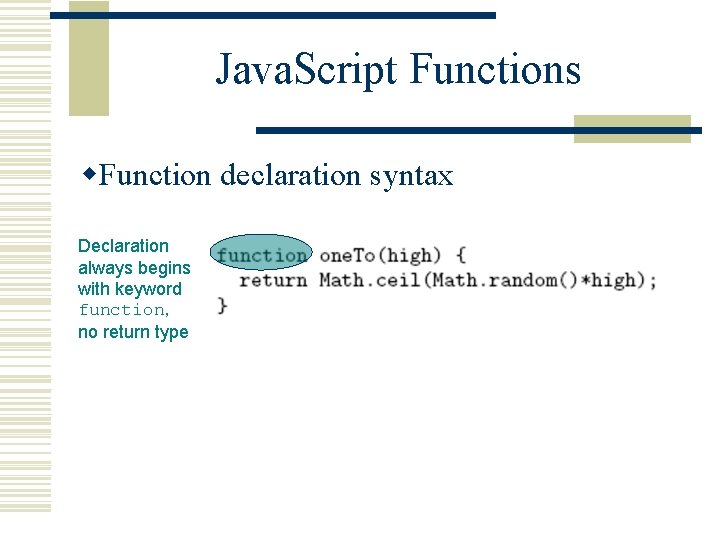
Java. Script Functions w. Function declaration syntax Declaration always begins with keyword function, no return type
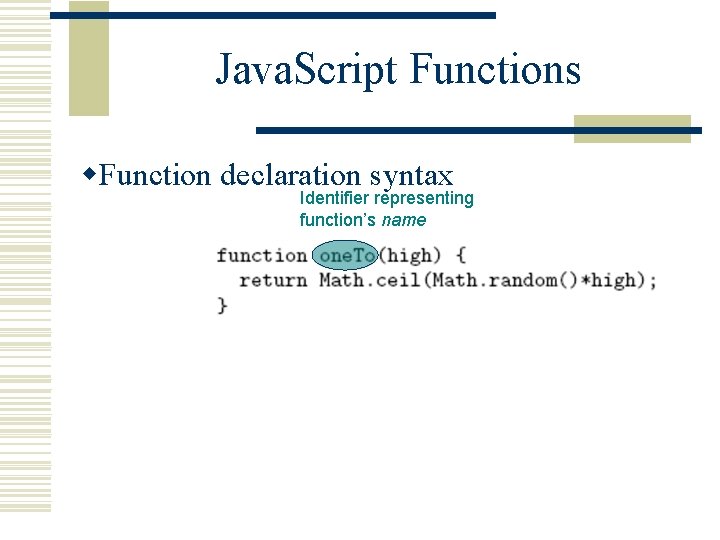
Java. Script Functions w. Function declaration syntax Identifier representing function’s name
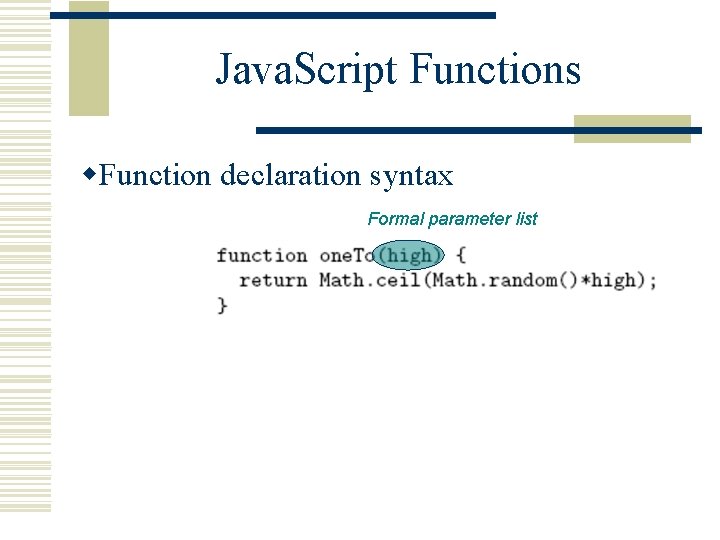
Java. Script Functions w. Function declaration syntax Formal parameter list
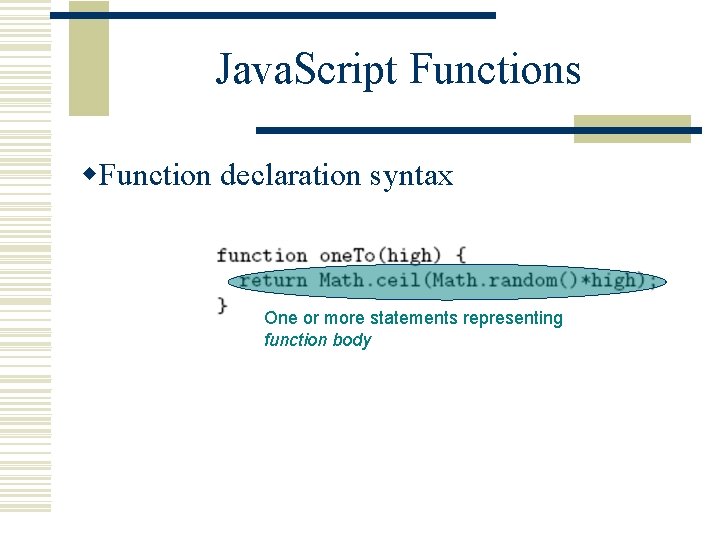
Java. Script Functions w. Function declaration syntax One or more statements representing function body
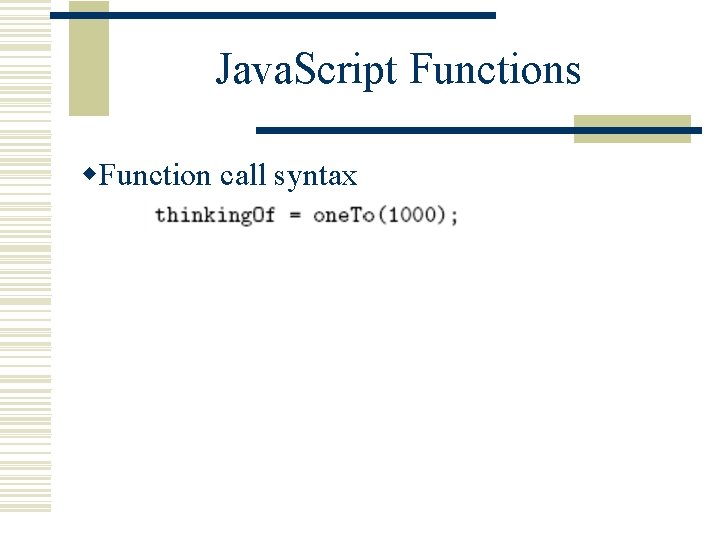
Java. Script Functions w. Function call syntax
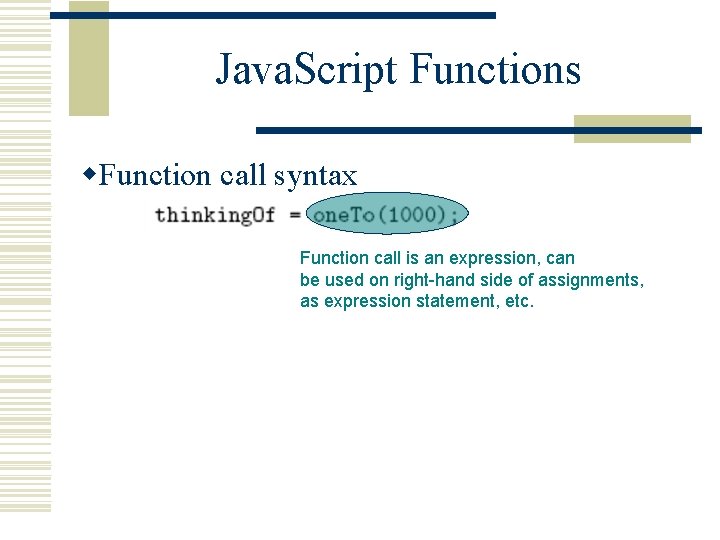
Java. Script Functions w. Function call syntax Function call is an expression, can be used on right-hand side of assignments, as expression statement, etc.
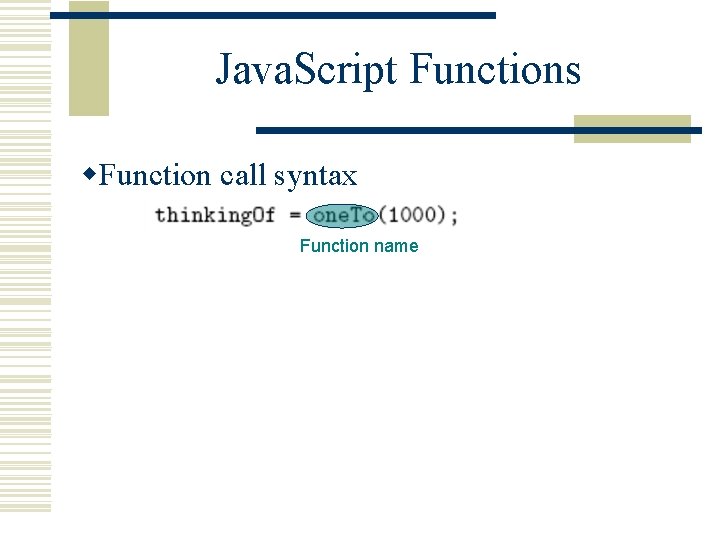
Java. Script Functions w. Function call syntax Function name
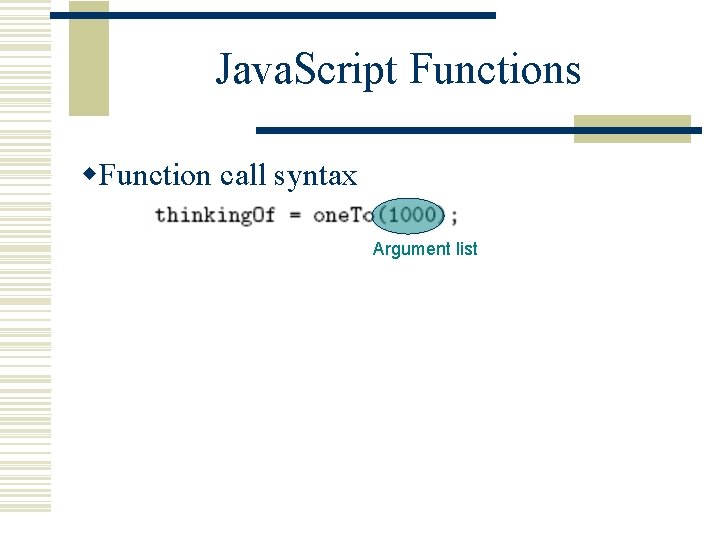
Java. Script Functions w. Function call syntax Argument list
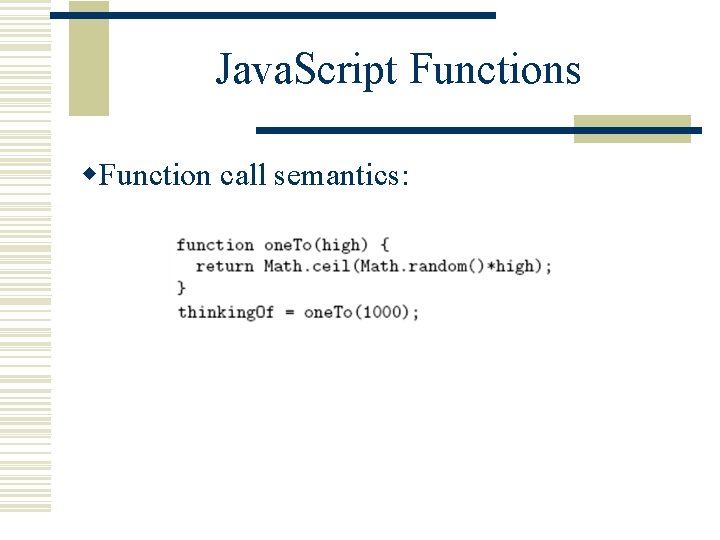
Java. Script Functions w. Function call semantics:
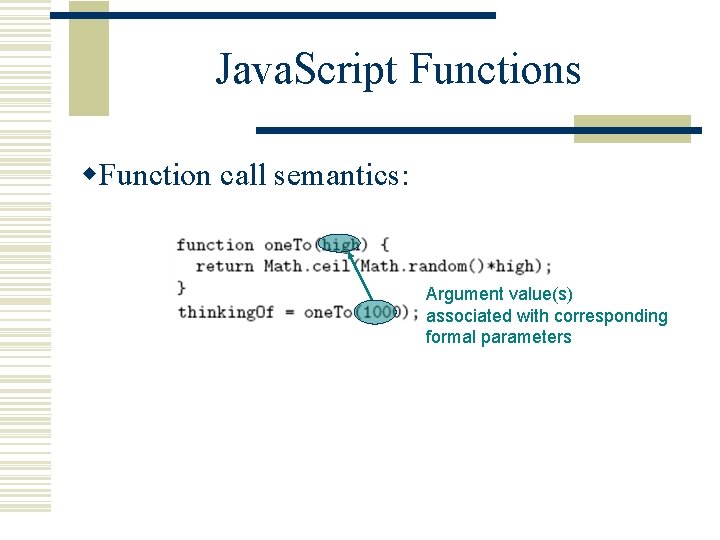
Java. Script Functions w. Function call semantics: Argument value(s) associated with corresponding formal parameters
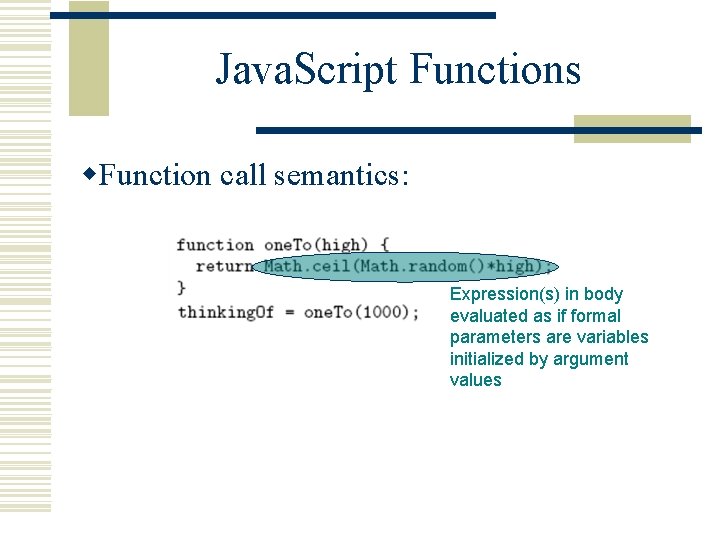
Java. Script Functions w. Function call semantics: Expression(s) in body evaluated as if formal parameters are variables initialized by argument values
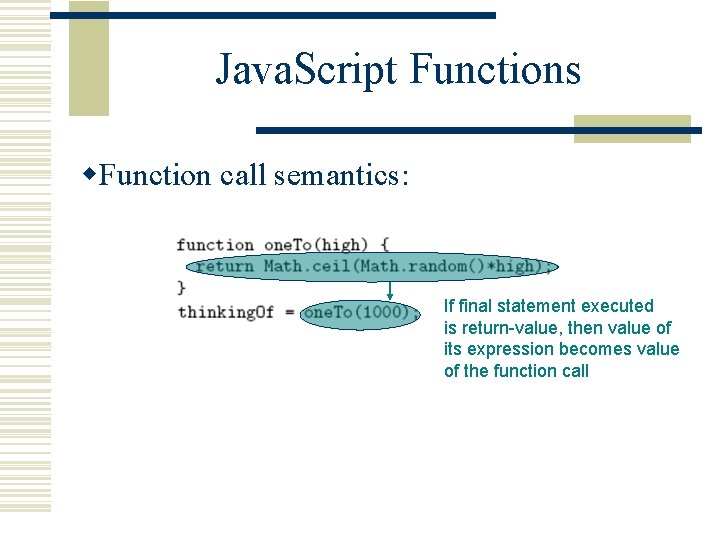
Java. Script Functions w. Function call semantics: If final statement executed is return-value, then value of its expression becomes value of the function call
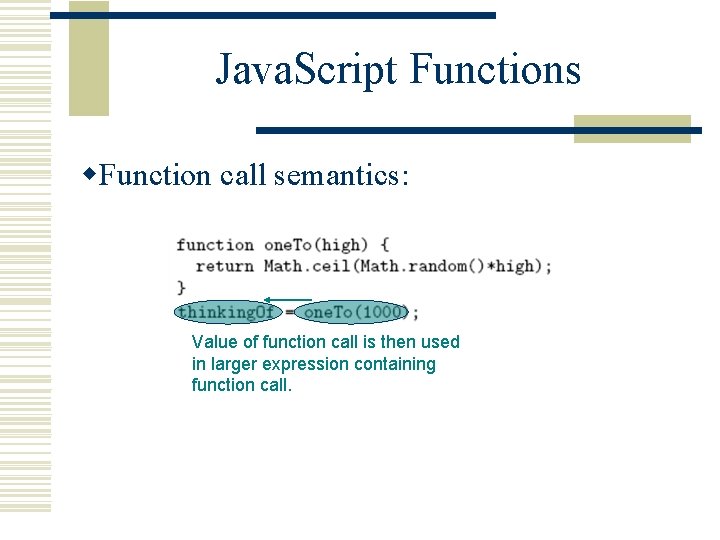
Java. Script Functions w. Function call semantics: Value of function call is then used in larger expression containing function call.
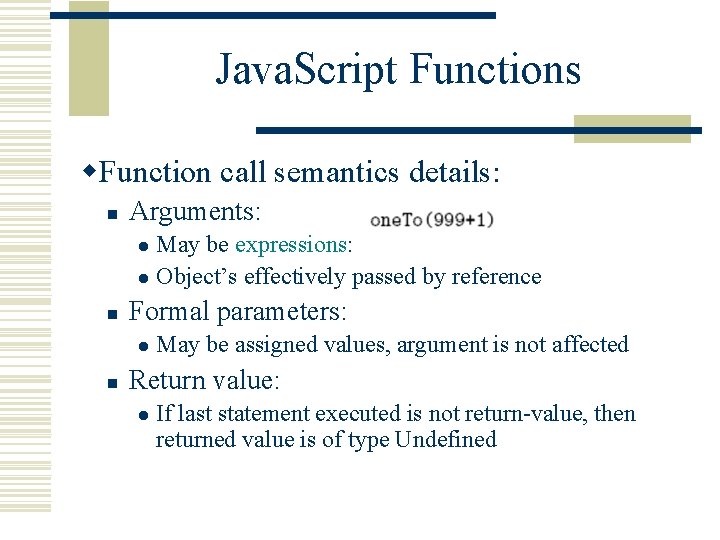
Java. Script Functions w. Function call semantics details: n Arguments: May be expressions: l Object’s effectively passed by reference l n Formal parameters: l n May be assigned values, argument is not affected Return value: l If last statement executed is not return-value, then returned value is of type Undefined
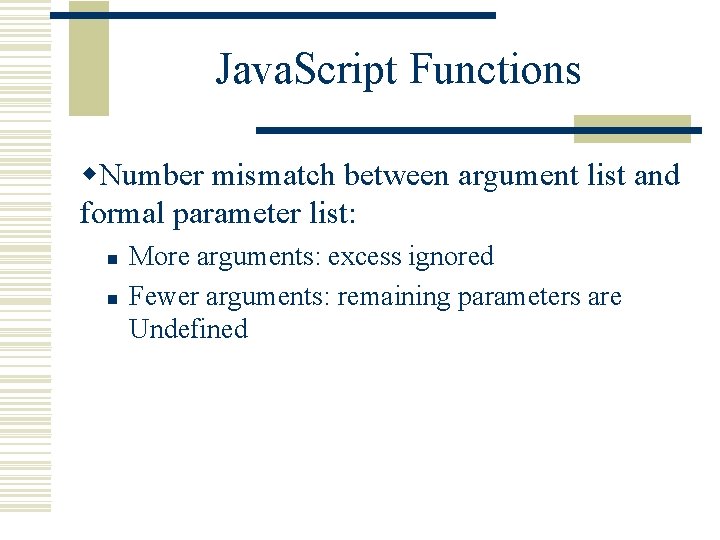
Java. Script Functions w. Number mismatch between argument list and formal parameter list: n n More arguments: excess ignored Fewer arguments: remaining parameters are Undefined
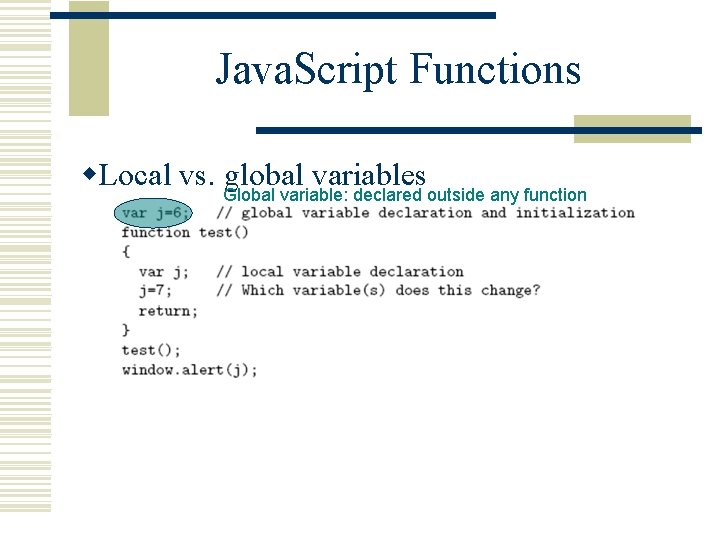
Java. Script Functions w. Local vs. Global global variables variable: declared outside any function
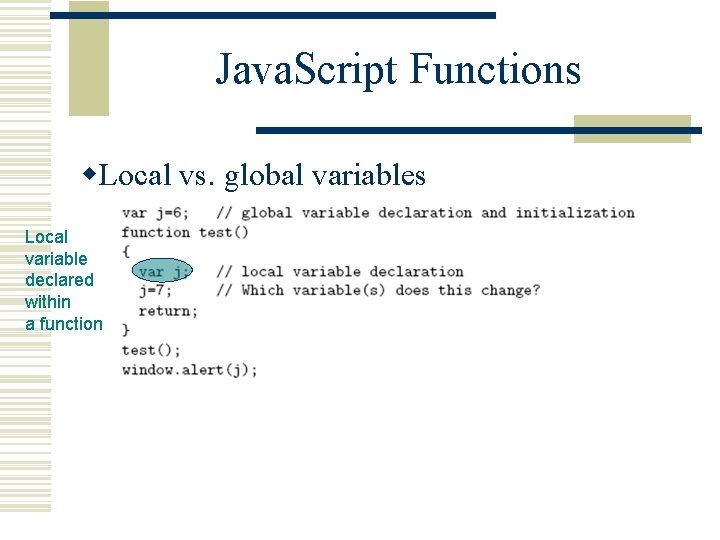
Java. Script Functions w. Local vs. global variables Local variable declared within a function
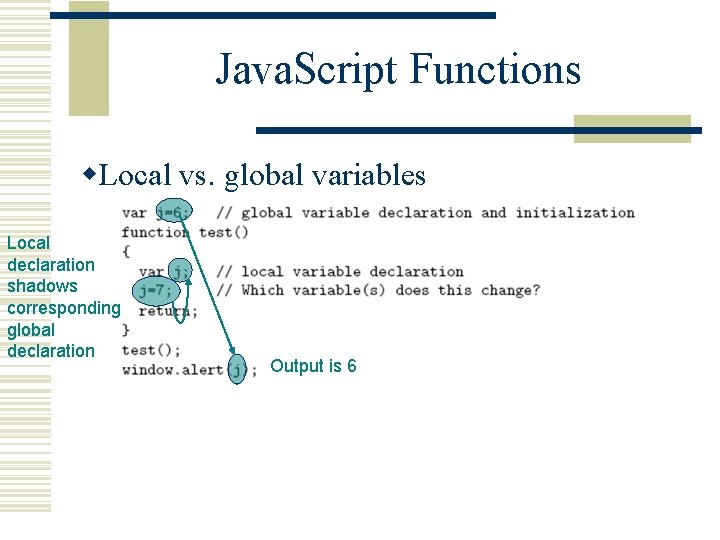
Java. Script Functions w. Local vs. global variables Local declaration shadows corresponding global declaration Output is 6
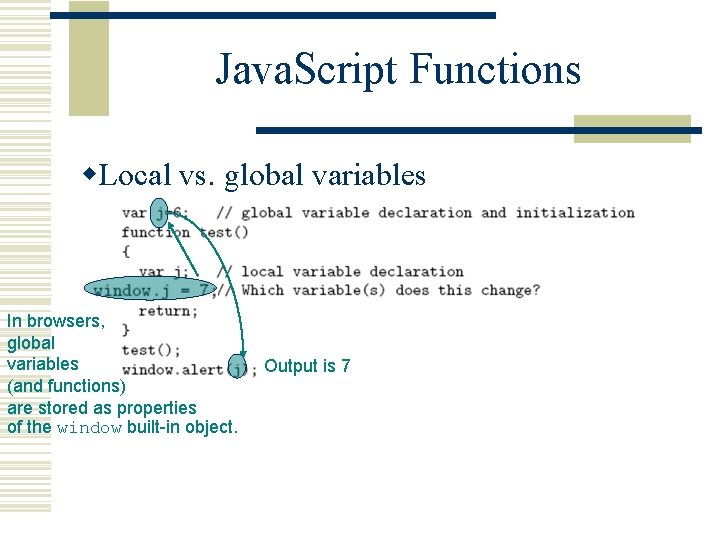
Java. Script Functions w. Local vs. global variables In browsers, global variables (and functions) are stored as properties of the window built-in object. Output is 7
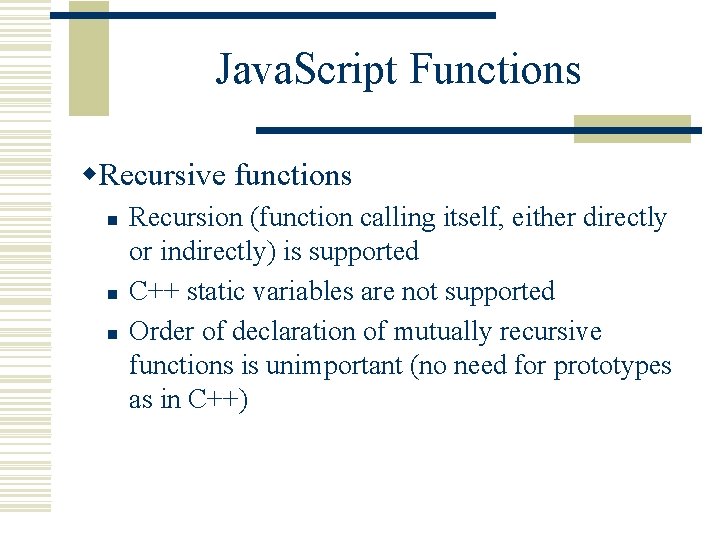
Java. Script Functions w. Recursive functions n n n Recursion (function calling itself, either directly or indirectly) is supported C++ static variables are not supported Order of declaration of mutually recursive functions is unimportant (no need for prototypes as in C++)
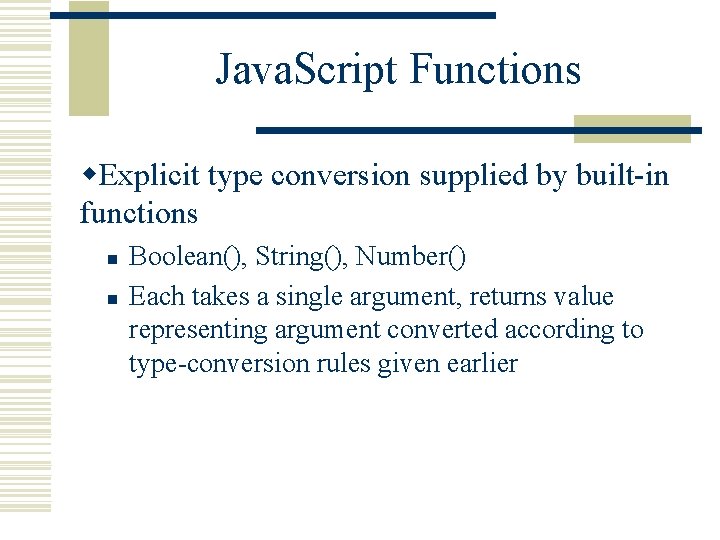
Java. Script Functions w. Explicit type conversion supplied by built-in functions n n Boolean(), String(), Number() Each takes a single argument, returns value representing argument converted according to type-conversion rules given earlier
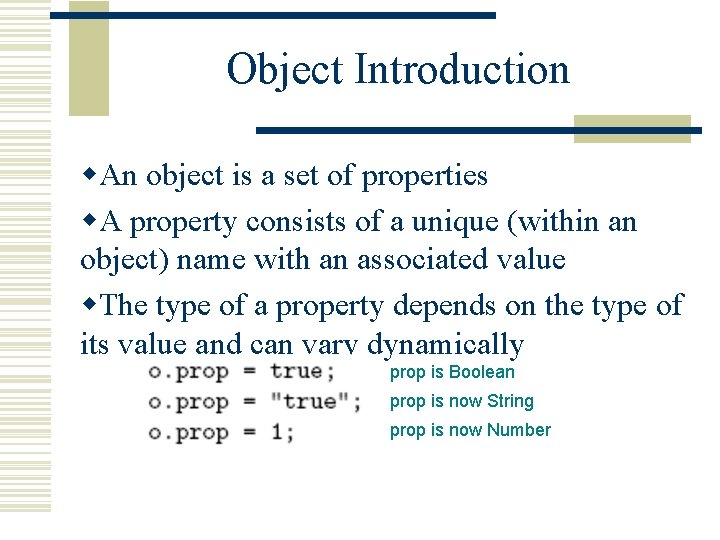
Object Introduction w. An object is a set of properties w. A property consists of a unique (within an object) name with an associated value w. The type of a property depends on the type of its value and can vary dynamically prop is Boolean prop is now String prop is now Number
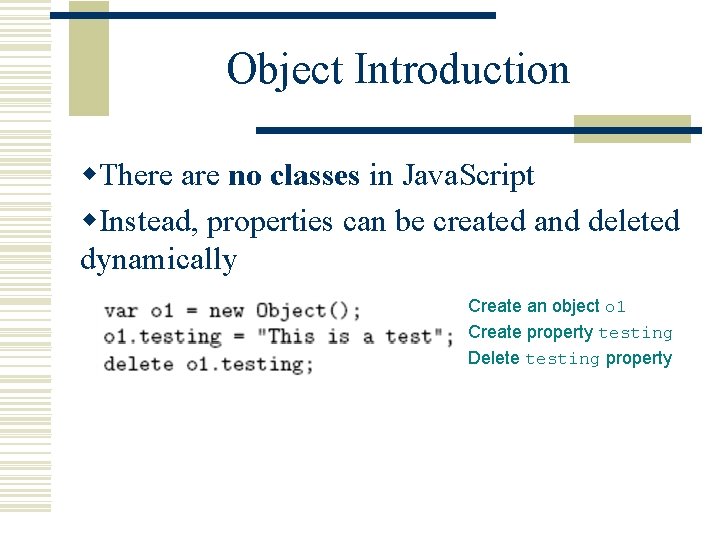
Object Introduction w. There are no classes in Java. Script w. Instead, properties can be created and deleted dynamically Create an object o 1 Create property testing Delete testing property
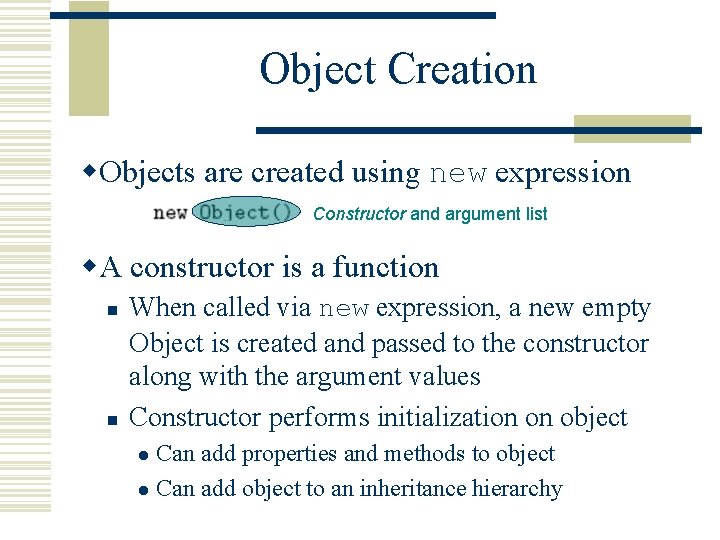
Object Creation w. Objects are created using new expression Constructor and argument list w. A constructor is a function n n When called via new expression, a new empty Object is created and passed to the constructor along with the argument values Constructor performs initialization on object Can add properties and methods to object l Can add object to an inheritance hierarchy l
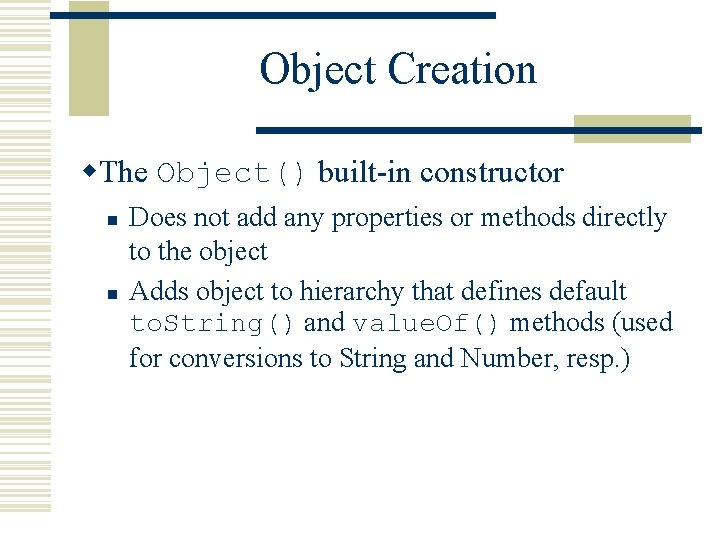
Object Creation w. The Object() built-in constructor n n Does not add any properties or methods directly to the object Adds object to hierarchy that defines default to. String() and value. Of() methods (used for conversions to String and Number, resp. )
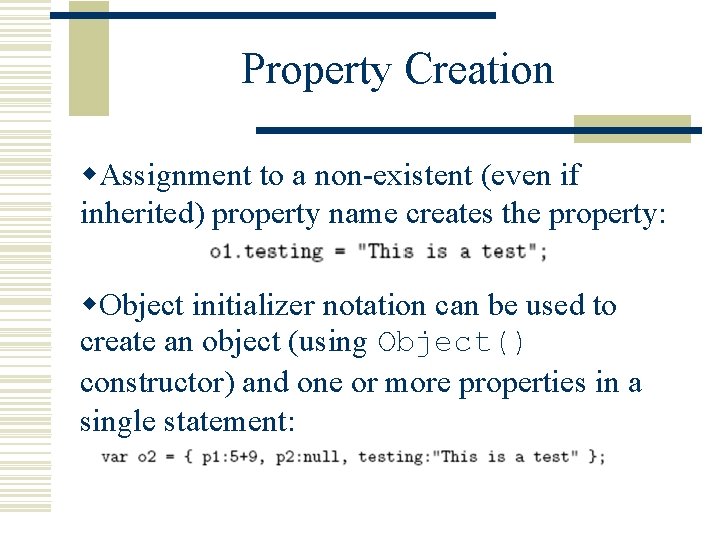
Property Creation w. Assignment to a non-existent (even if inherited) property name creates the property: w. Object initializer notation can be used to create an object (using Object() constructor) and one or more properties in a single statement:
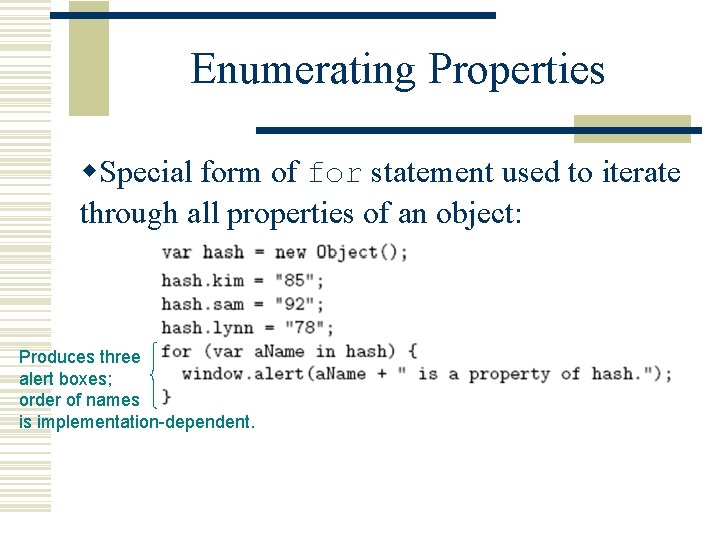
Enumerating Properties w. Special form of for statement used to iterate through all properties of an object: Produces three alert boxes; order of names is implementation-dependent.
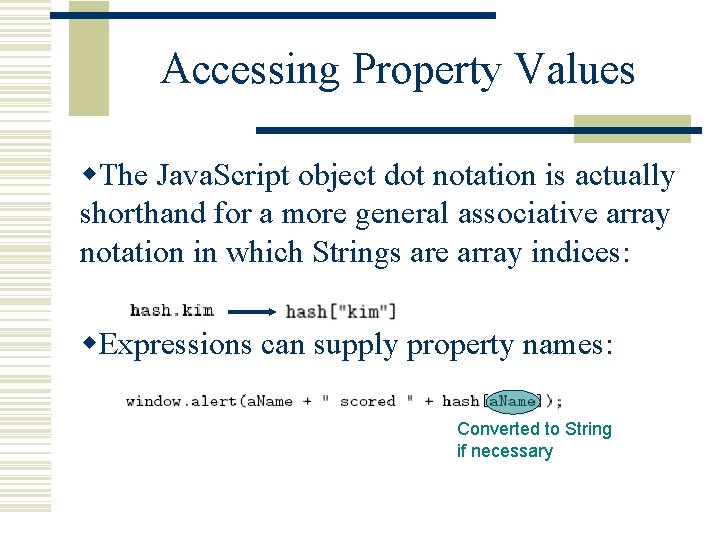
Accessing Property Values w. The Java. Script object dot notation is actually shorthand for a more general associative array notation in which Strings are array indices: w. Expressions can supply property names: Converted to String if necessary
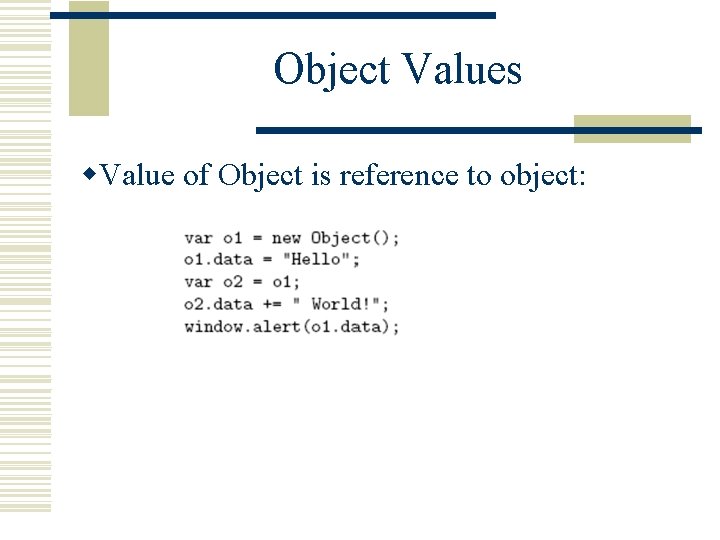
Object Values w. Value of Object is reference to object:
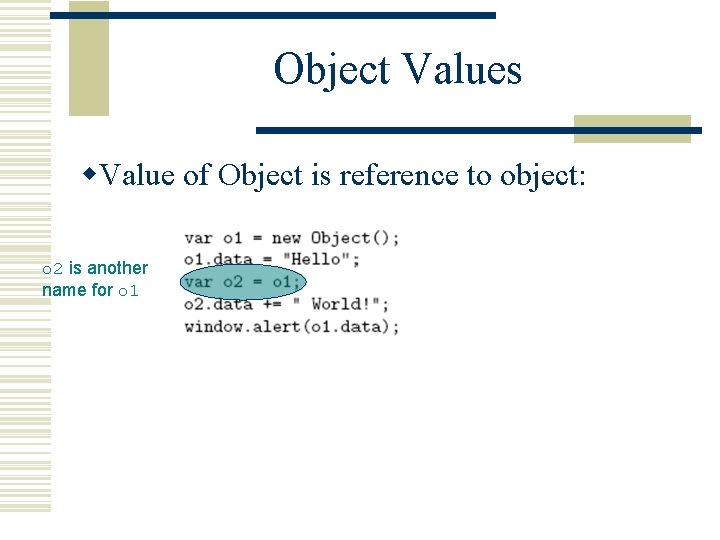
Object Values w. Value of Object is reference to object: o 2 is another name for o 1
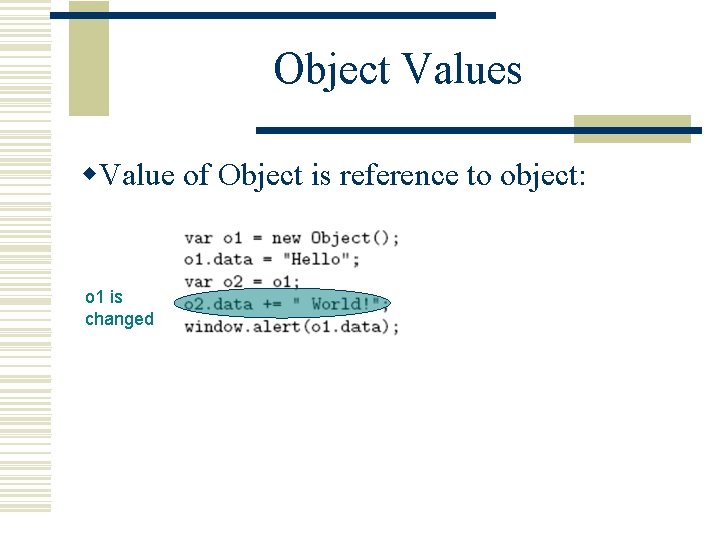
Object Values w. Value of Object is reference to object: o 1 is changed
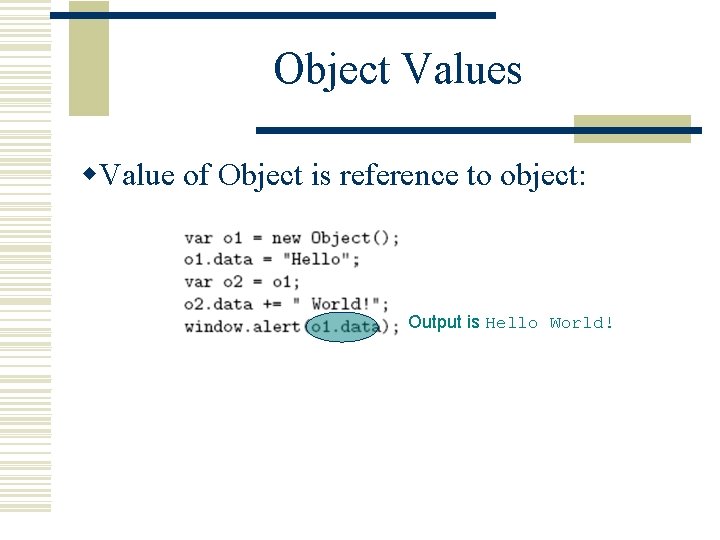
Object Values w. Value of Object is reference to object: Output is Hello World!
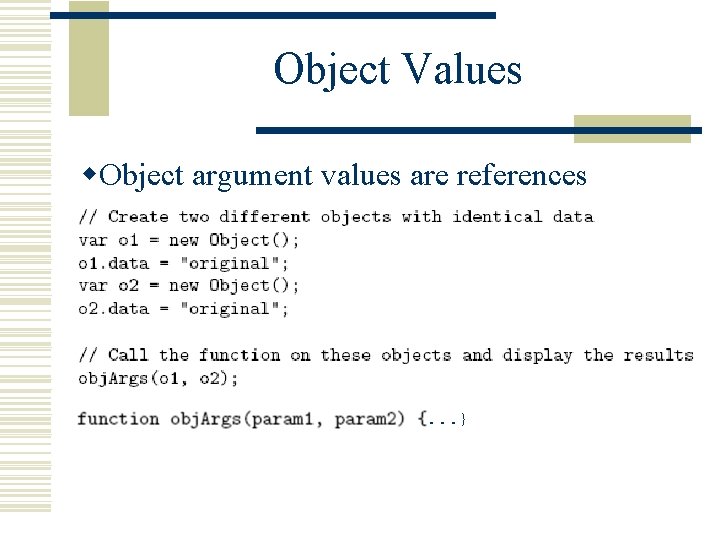
Object Values w. Object argument values are references . . . }
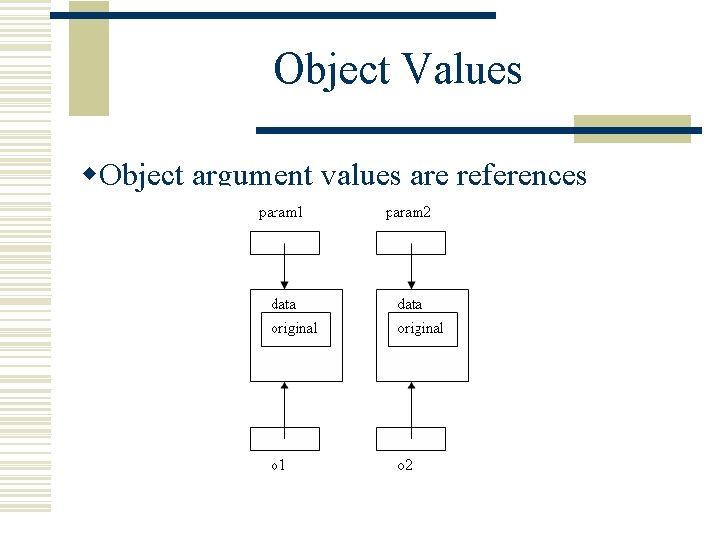
Object Values w. Object argument values are references
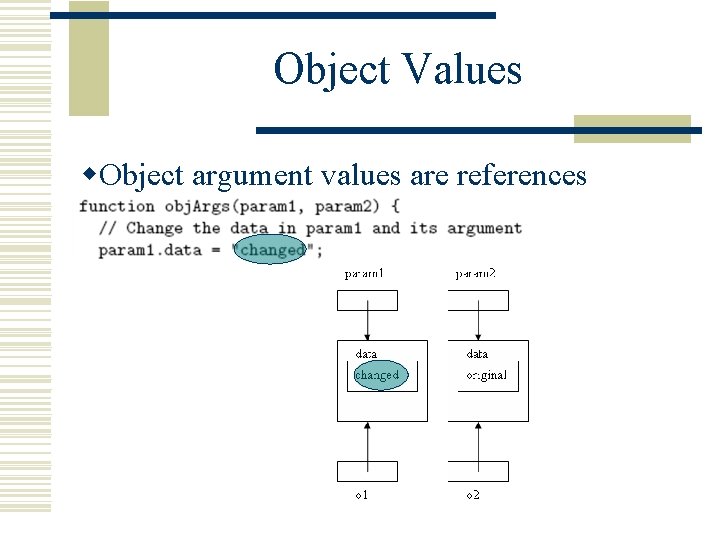
Object Values w. Object argument values are references
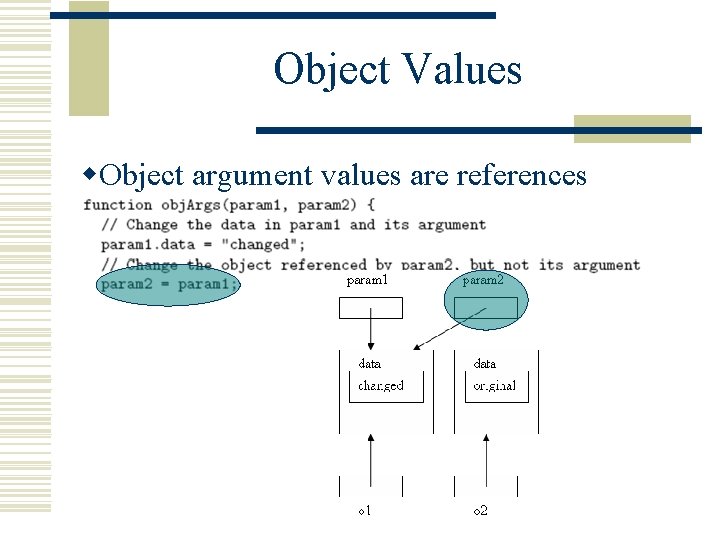
Object Values w. Object argument values are references
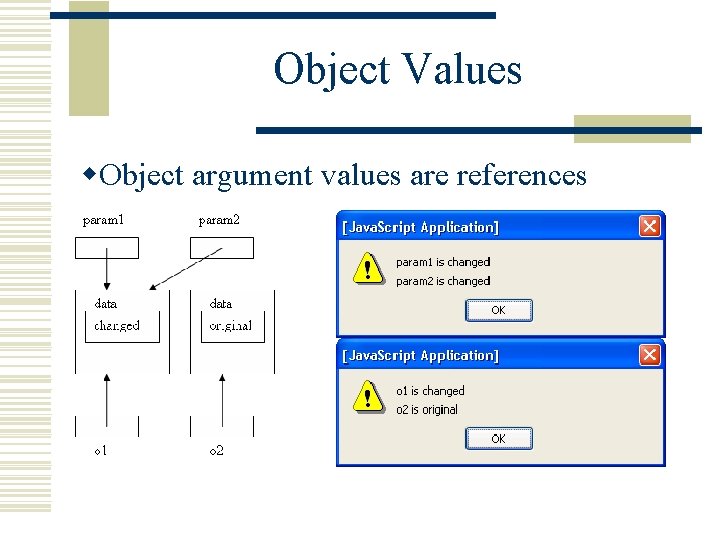
Object Values w. Object argument values are references
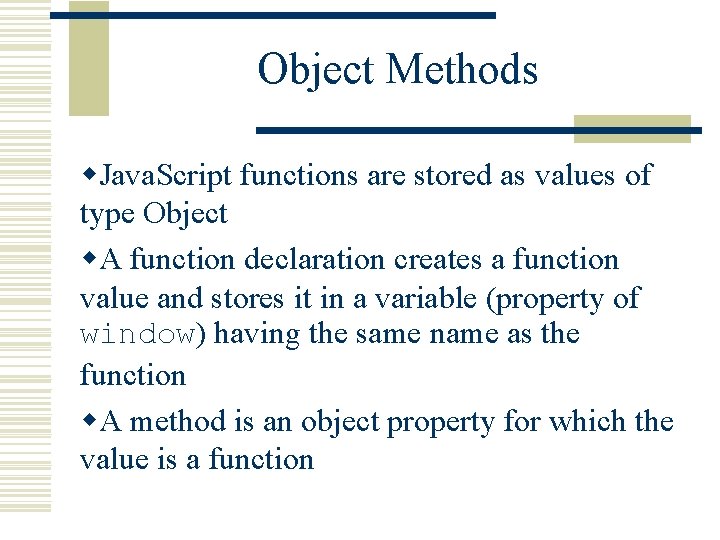
Object Methods w. Java. Script functions are stored as values of type Object w. A function declaration creates a function value and stores it in a variable (property of window) having the same name as the function w. A method is an object property for which the value is a function
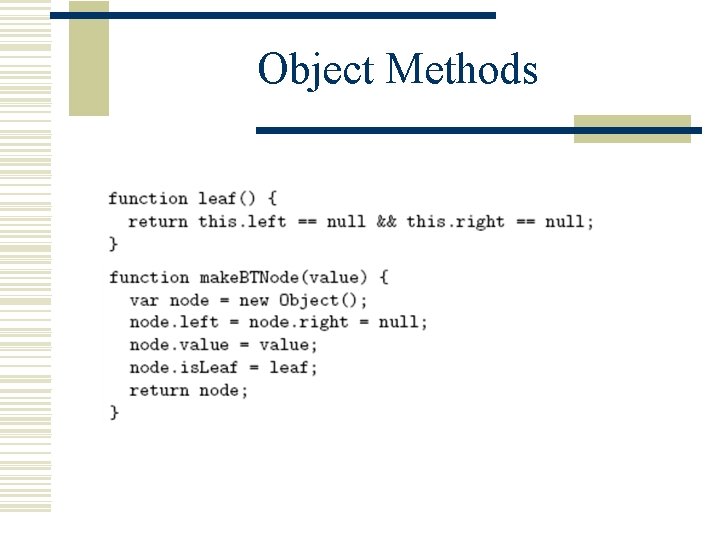
Object Methods
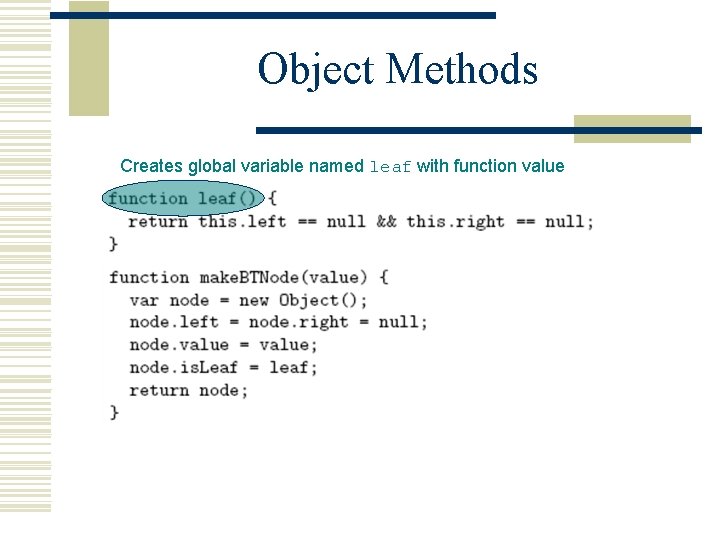
Object Methods Creates global variable named leaf with function value
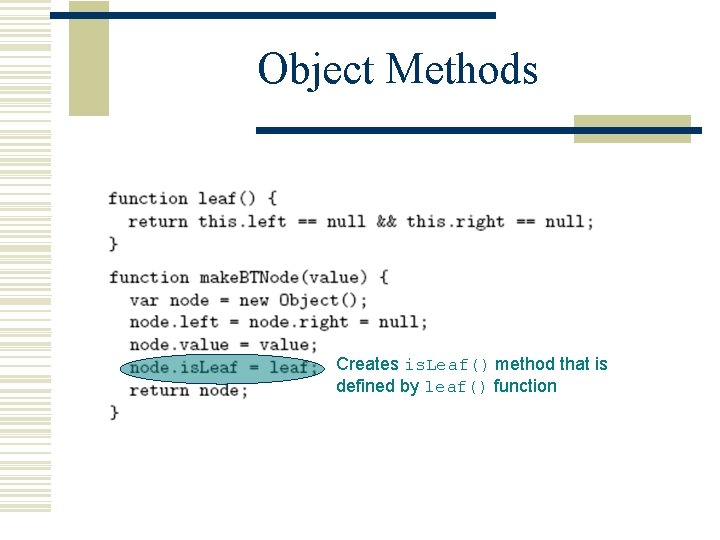
Object Methods Creates is. Leaf() method that is defined by leaf() function
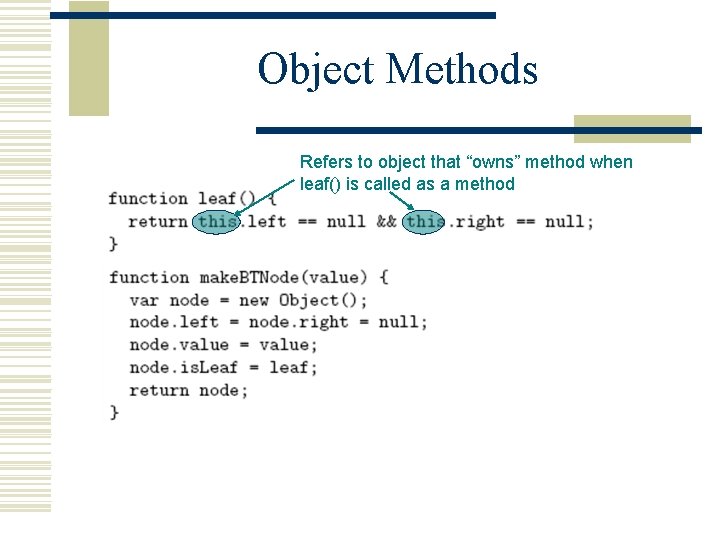
Object Methods Refers to object that “owns” method when leaf() is called as a method
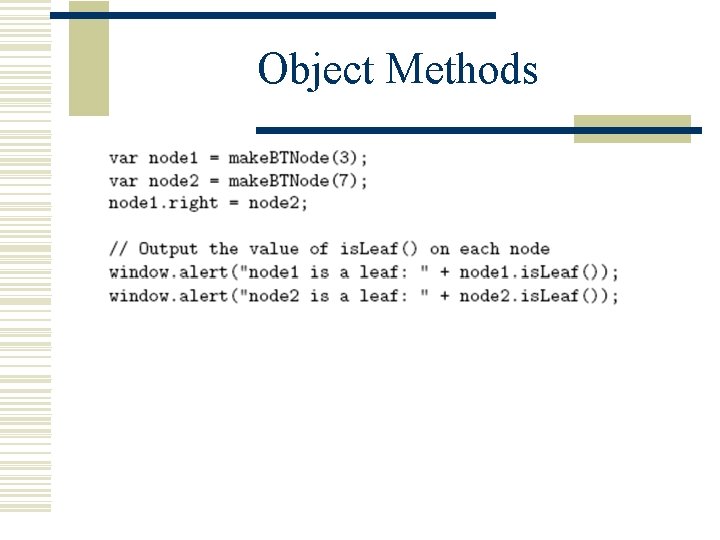
Object Methods
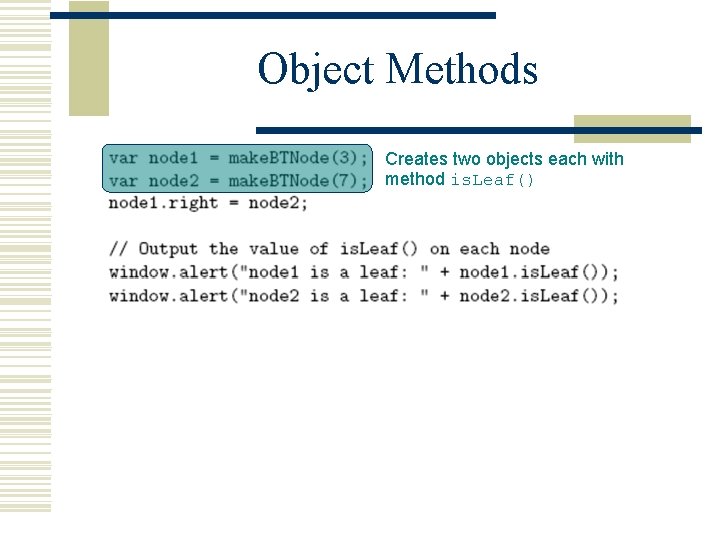
Object Methods Creates two objects each with method is. Leaf()
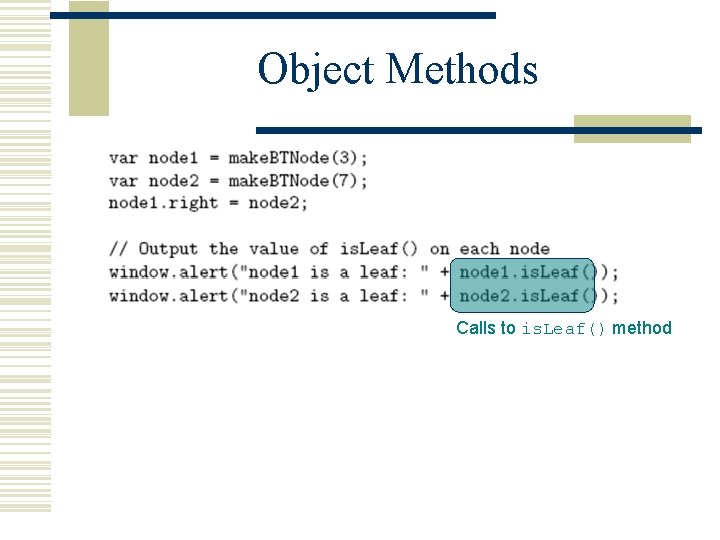
Object Methods Calls to is. Leaf() method
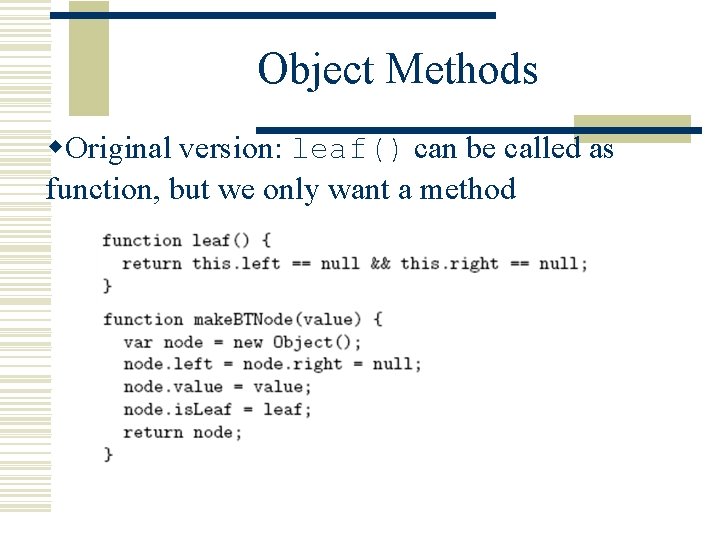
Object Methods w. Original version: leaf() can be called as function, but we only want a method
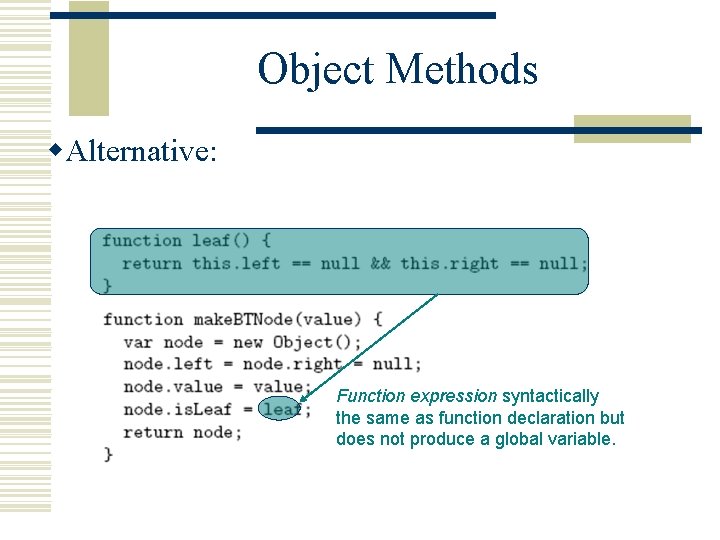
Object Methods w. Alternative: Function expression syntactically the same as function declaration but does not produce a global variable.
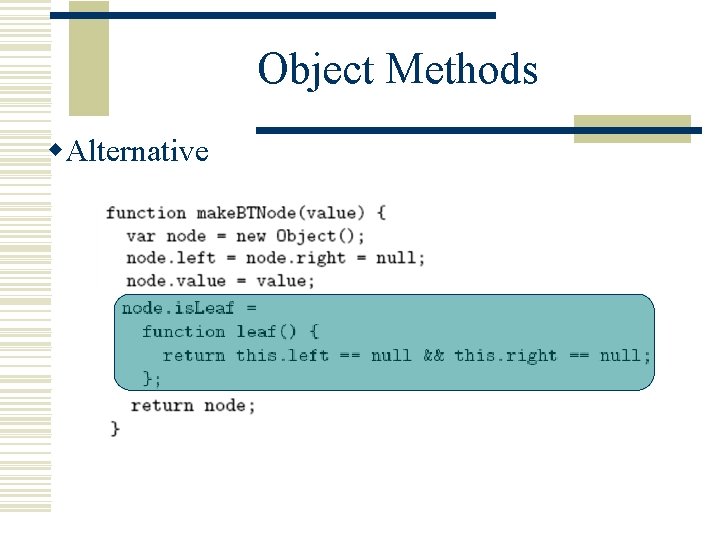
Object Methods w. Alternative
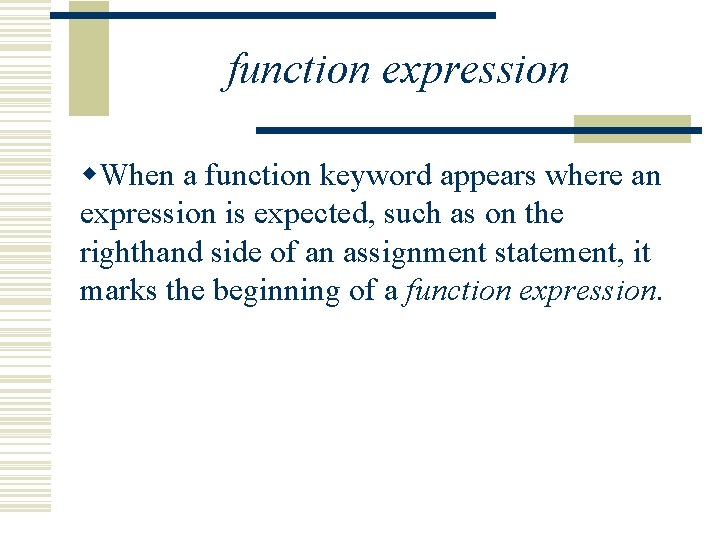
function expression w. When a function keyword appears where an expression is expected, such as on the righthand side of an assignment statement, it marks the beginning of a function expression.
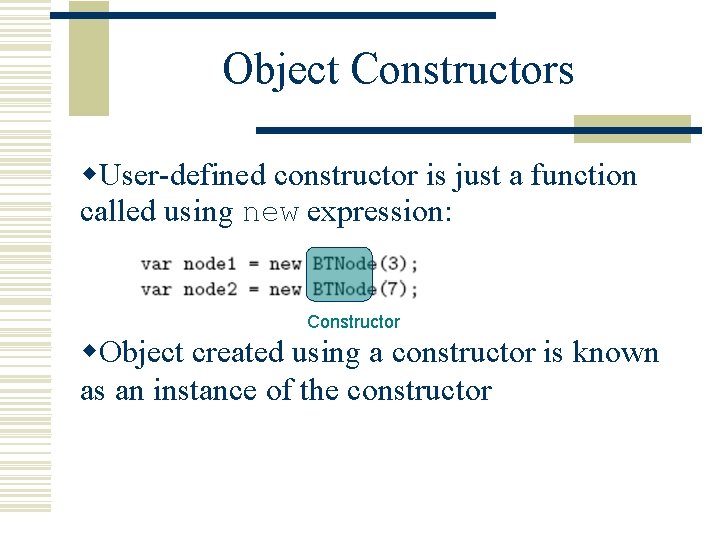
Object Constructors w. User-defined constructor is just a function called using new expression: Constructor w. Object created using a constructor is known as an instance of the constructor
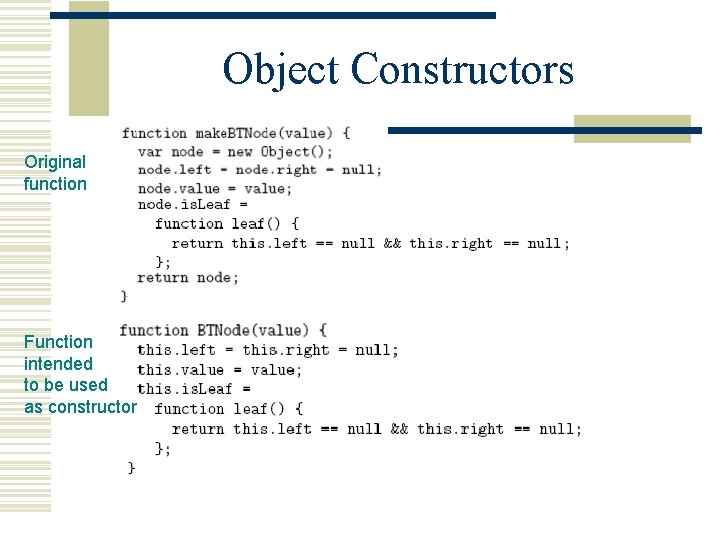
Object Constructors Original function Function intended to be used as constructor
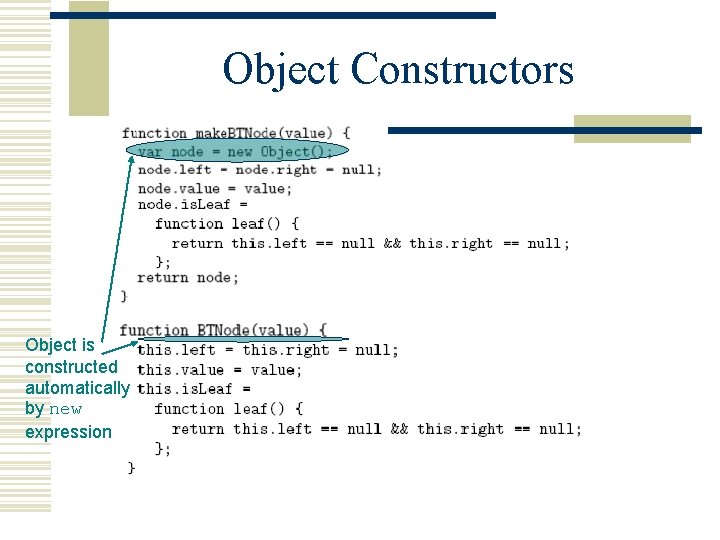
Object Constructors Object is constructed automatically by new expression
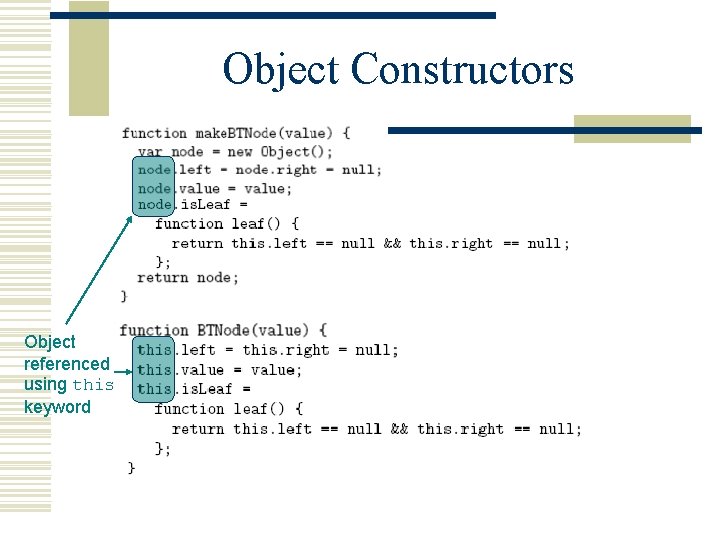
Object Constructors Object referenced using this keyword
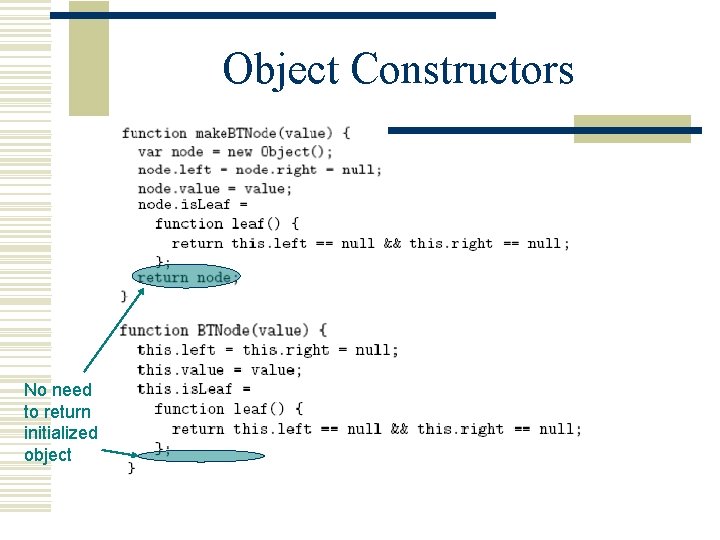
Object Constructors No need to return initialized object
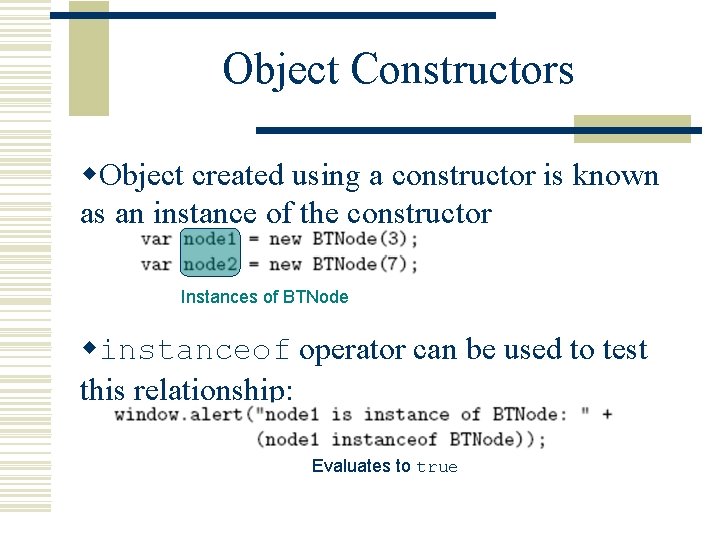
Object Constructors w. Object created using a constructor is known as an instance of the constructor Instances of BTNode winstanceof operator can be used to test this relationship: Evaluates to true
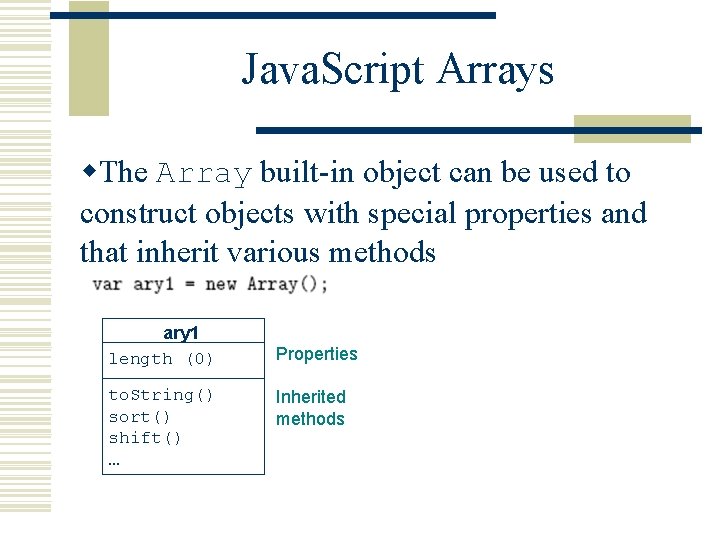
Java. Script Arrays w. The Array built-in object can be used to construct objects with special properties and that inherit various methods ary 1 length (0) to. String() sort() shift() … Properties Inherited methods
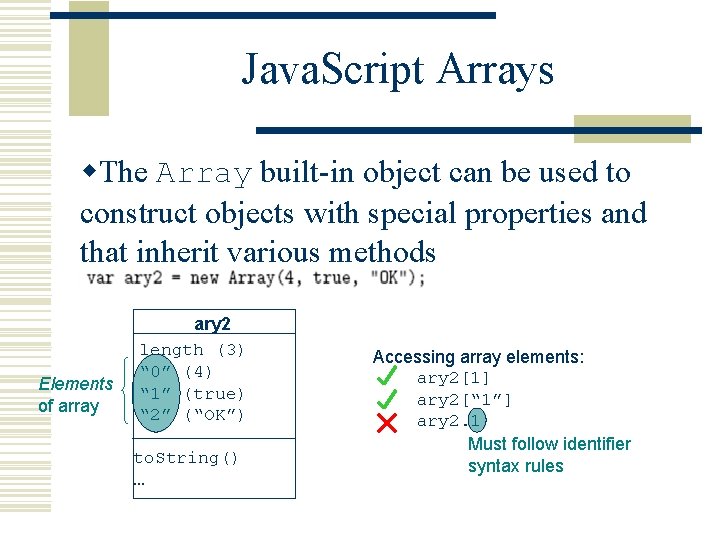
Java. Script Arrays w. The Array built-in object can be used to construct objects with special properties and that inherit various methods Elements of array ary 2 length (3) “ 0” (4) “ 1” (true) “ 2” (“OK”) to. String() … Accessing array elements: ary 2[1] ary 2[“ 1”] ary 2. 1 Must follow identifier syntax rules
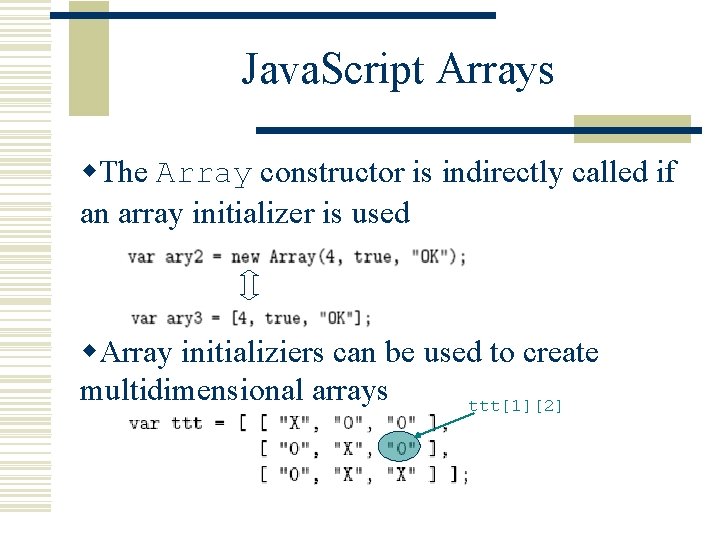
Java. Script Arrays w. The Array constructor is indirectly called if an array initializer is used w. Array initializiers can be used to create multidimensional arrays ttt[1][2]
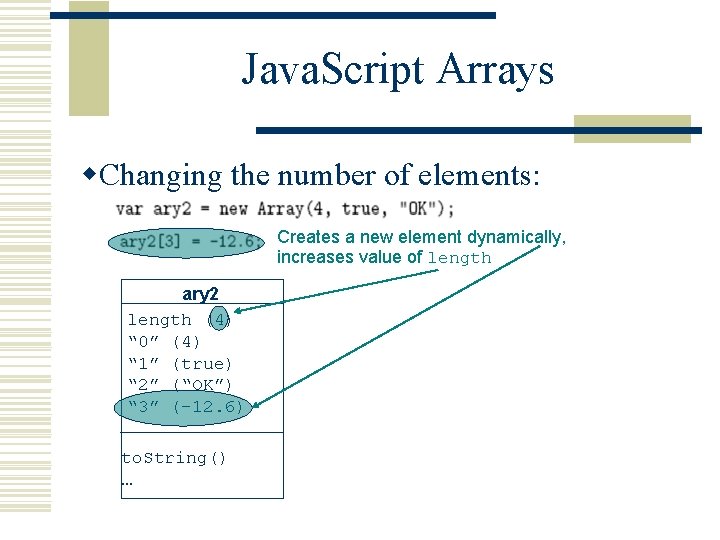
Java. Script Arrays w. Changing the number of elements: Creates a new element dynamically, increases value of length ary 2 length (4) “ 0” (4) “ 1” (true) “ 2” (“OK”) “ 3” (-12. 6) to. String() …
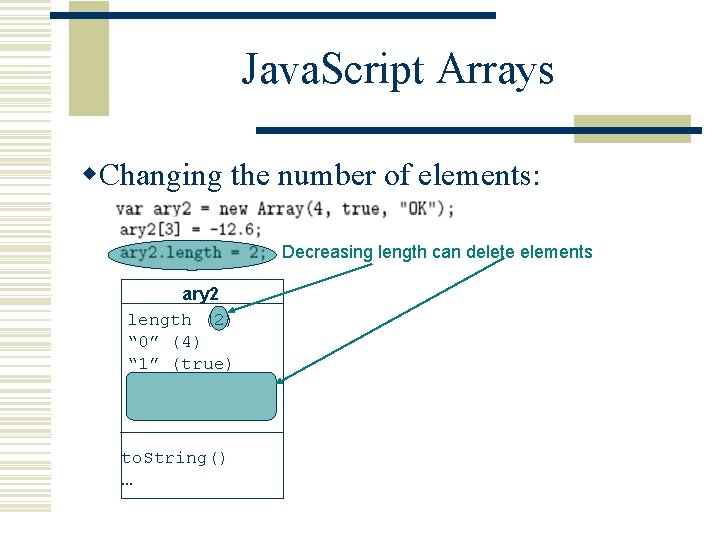
Java. Script Arrays w. Changing the number of elements: Decreasing length can delete elements ary 2 length (2) “ 0” (4) “ 1” (true) to. String() …
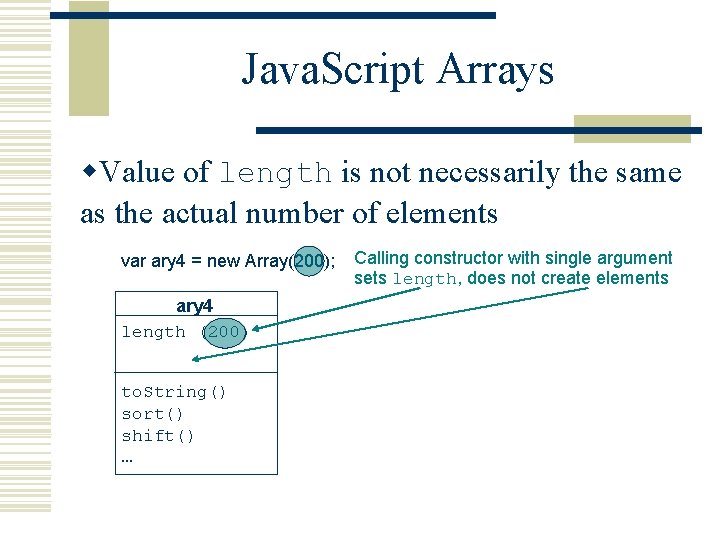
Java. Script Arrays w. Value of length is not necessarily the same as the actual number of elements var ary 4 = new Array(200); ary 4 length (200) to. String() sort() shift() … Calling constructor with single argument sets length, does not create elements
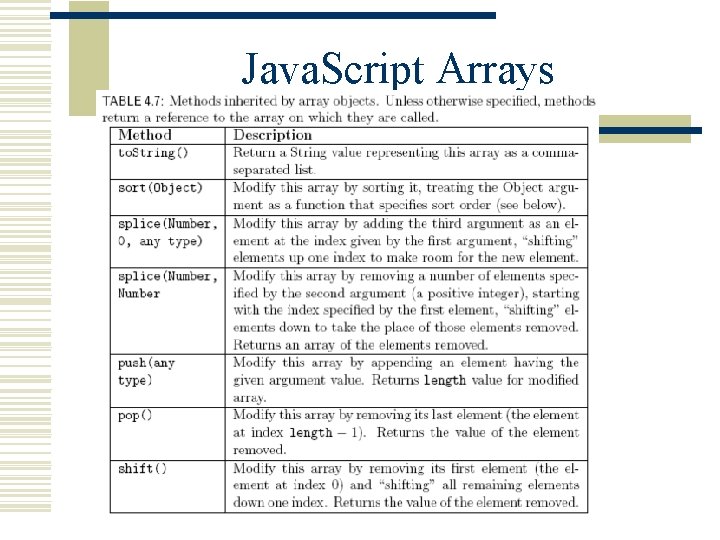
Java. Script Arrays
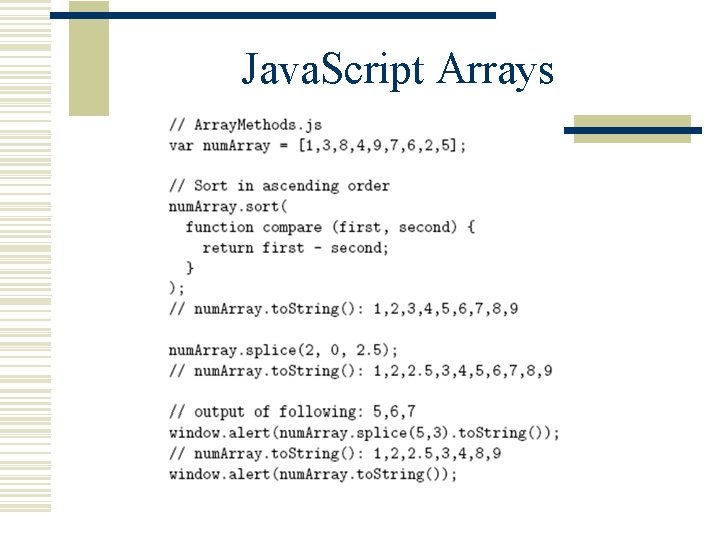
Java. Script Arrays
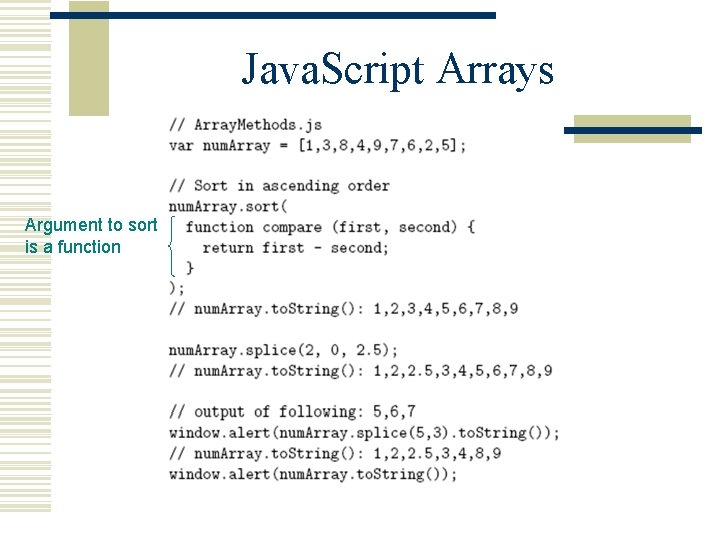
Java. Script Arrays Argument to sort is a function
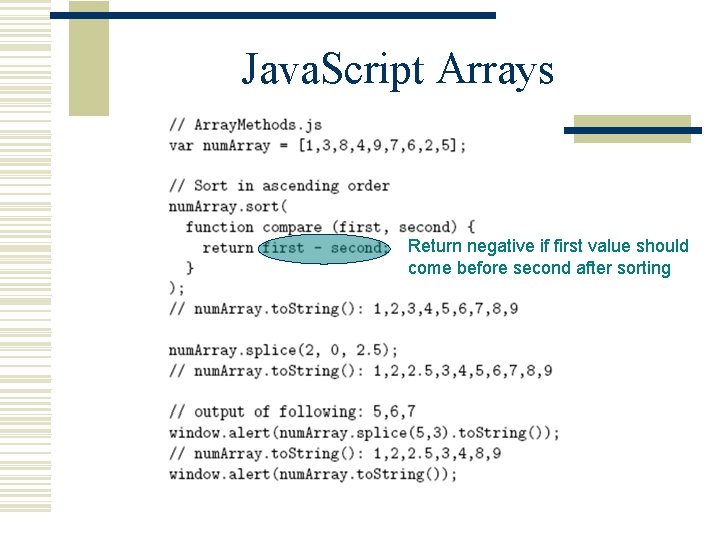
Java. Script Arrays Return negative if first value should come before second after sorting
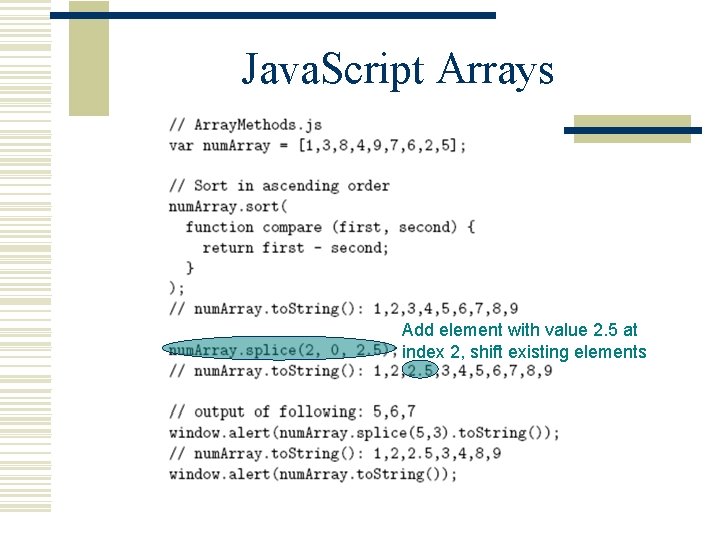
Java. Script Arrays Add element with value 2. 5 at index 2, shift existing elements
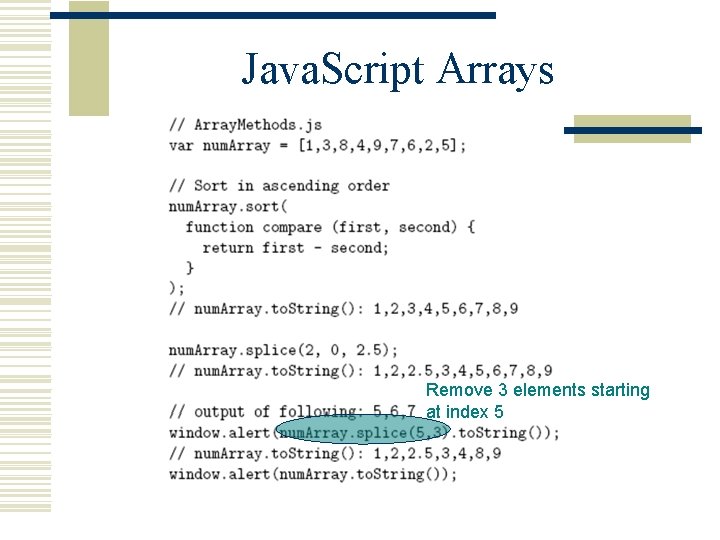
Java. Script Arrays Remove 3 elements starting at index 5
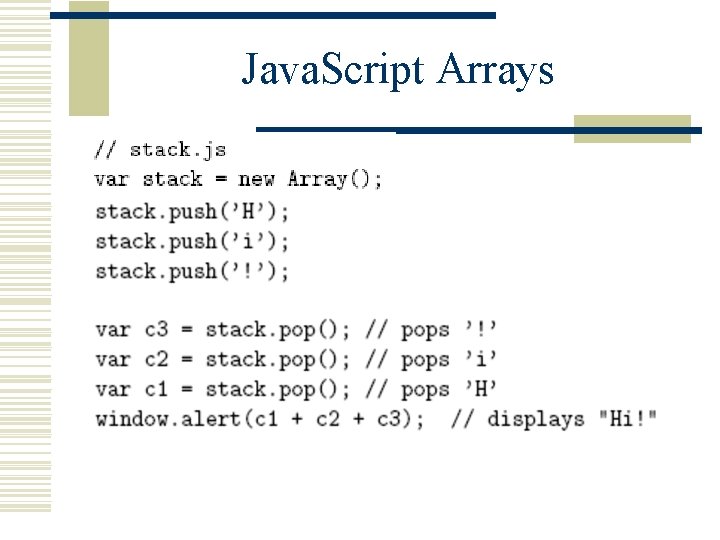
Java. Script Arrays
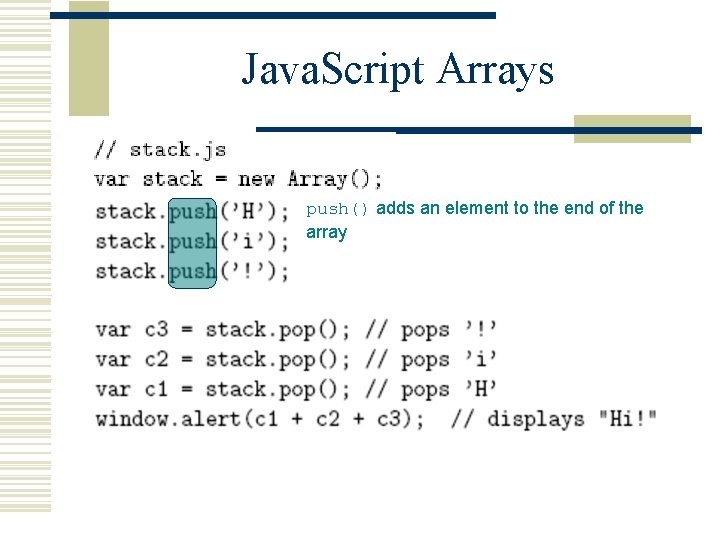
Java. Script Arrays push() adds an element to the end of the array
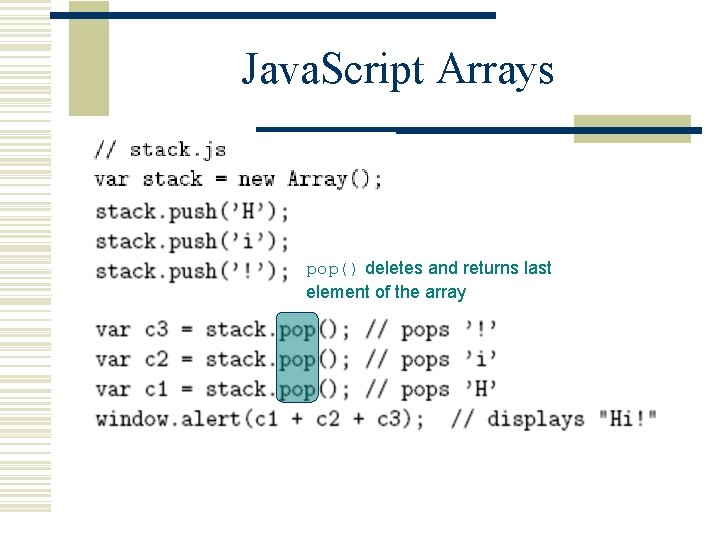
Java. Script Arrays pop() deletes and returns last element of the array
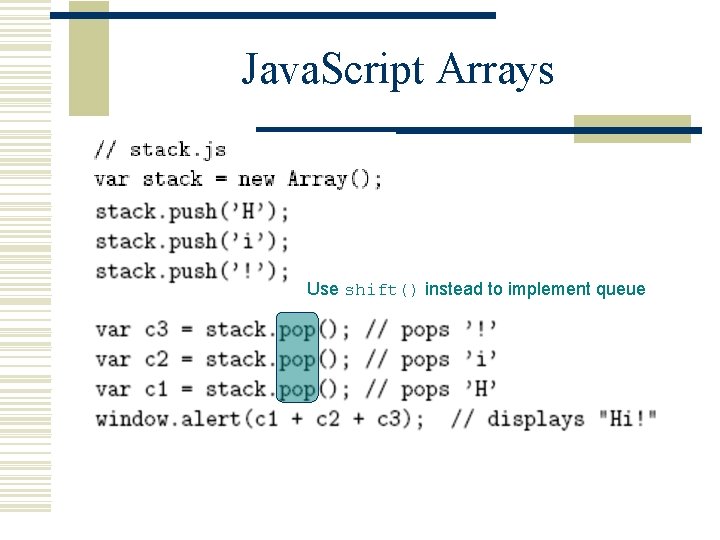
Java. Script Arrays Use shift() instead to implement queue
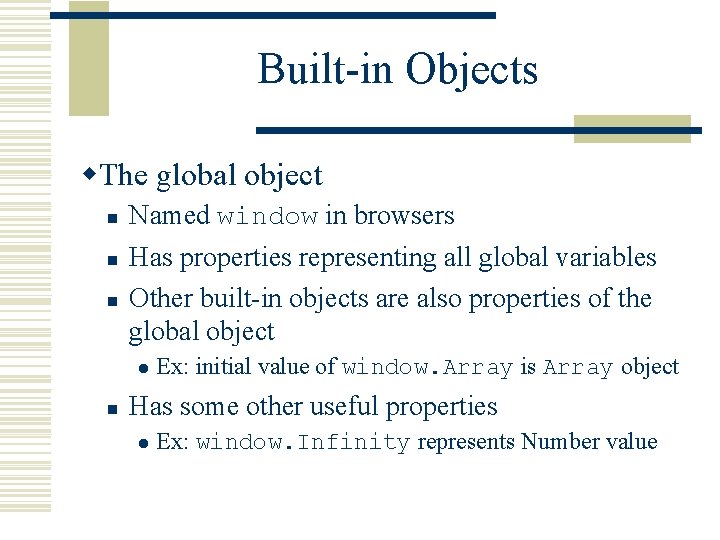
Built-in Objects w. The global object n n n Named window in browsers Has properties representing all global variables Other built-in objects are also properties of the global object l n Ex: initial value of window. Array is Array object Has some other useful properties l Ex: window. Infinity represents Number value
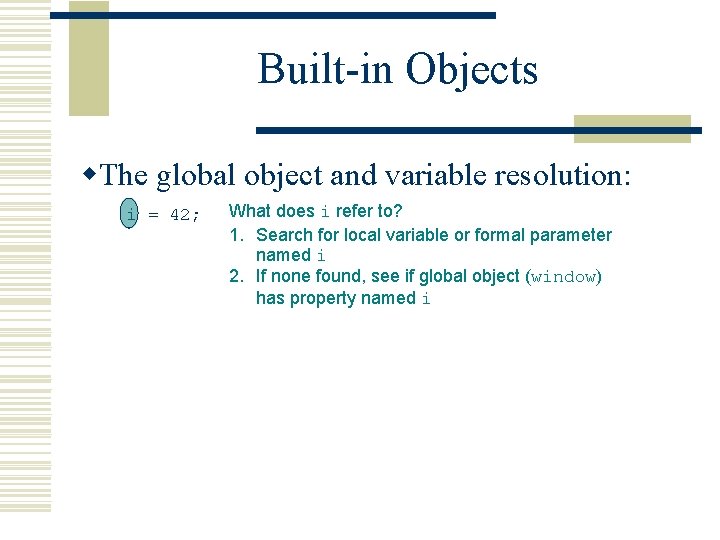
Built-in Objects w. The global object and variable resolution: i = 42; What does i refer to? 1. Search for local variable or formal parameter named i 2. If none found, see if global object (window) has property named i
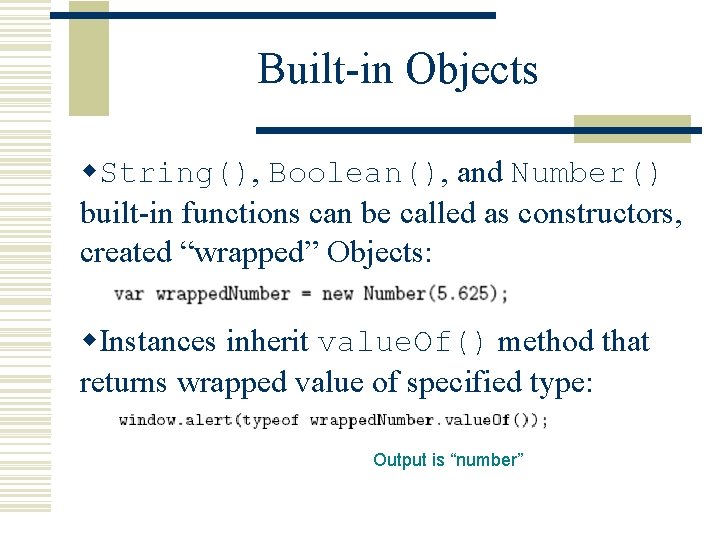
Built-in Objects w. String(), Boolean(), and Number() built-in functions can be called as constructors, created “wrapped” Objects: w. Instances inherit value. Of() method that returns wrapped value of specified type: Output is “number”
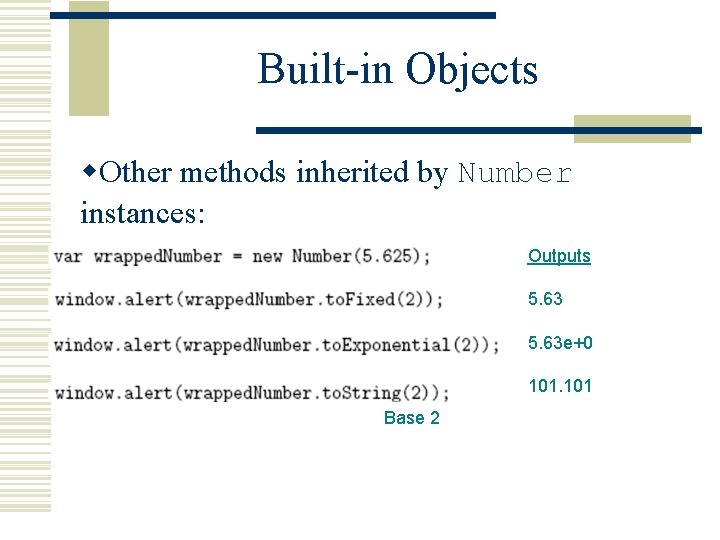
Built-in Objects w. Other methods inherited by Number instances: Outputs 5. 63 e+0 101 Base 2
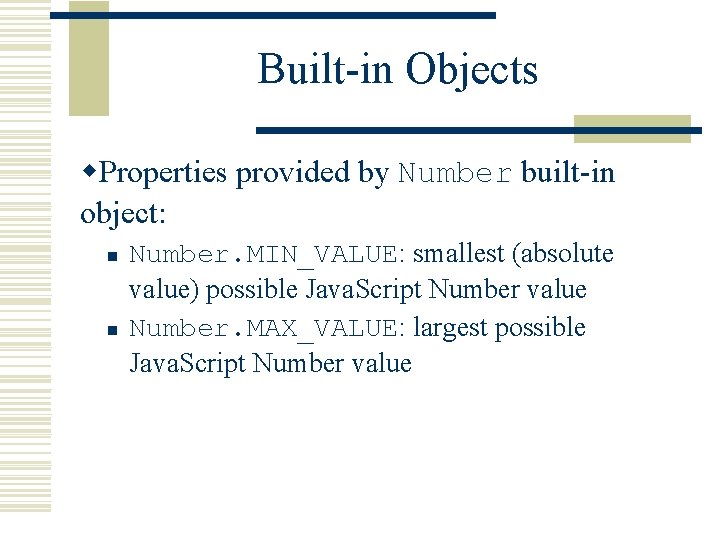
Built-in Objects w. Properties provided by Number built-in object: n n Number. MIN_VALUE: smallest (absolute value) possible Java. Script Number value Number. MAX_VALUE: largest possible Java. Script Number value
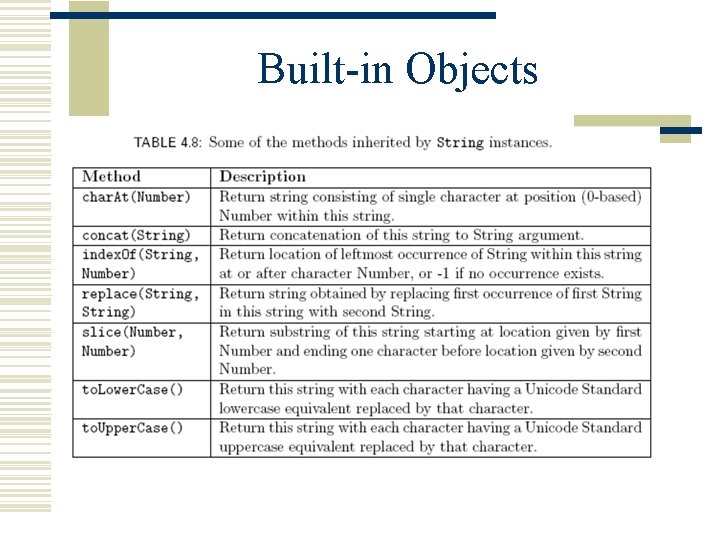
Built-in Objects
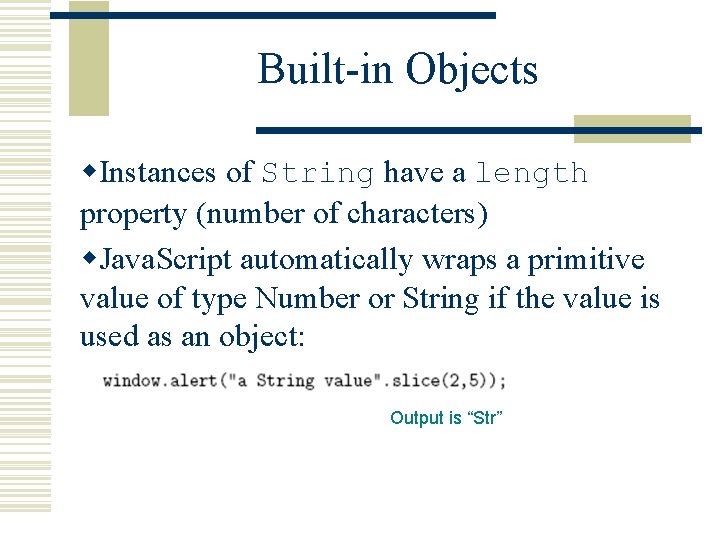
Built-in Objects w. Instances of String have a length property (number of characters) w. Java. Script automatically wraps a primitive value of type Number or String if the value is used as an object: Output is “Str”
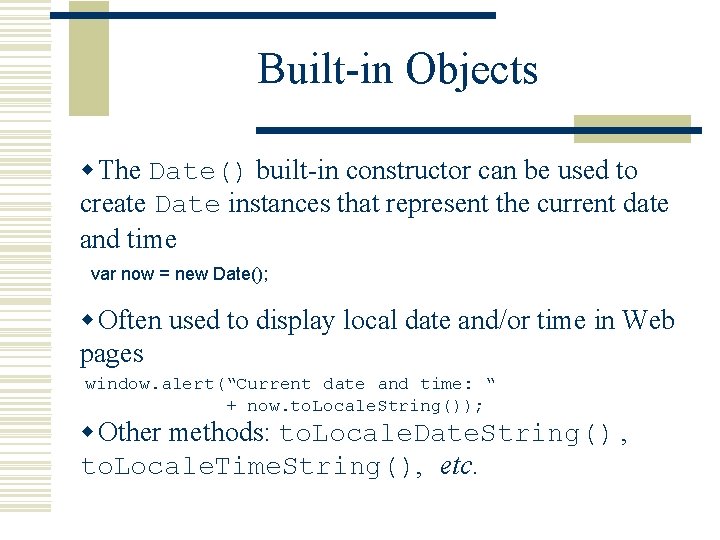
Built-in Objects w The Date() built-in constructor can be used to create Date instances that represent the current date and time var now = new Date(); w Often used to display local date and/or time in Web pages window. alert(“Current date and time: “ + now. to. Locale. String()); w Other methods: to. Locale. Date. String() , to. Locale. Time. String(), etc.
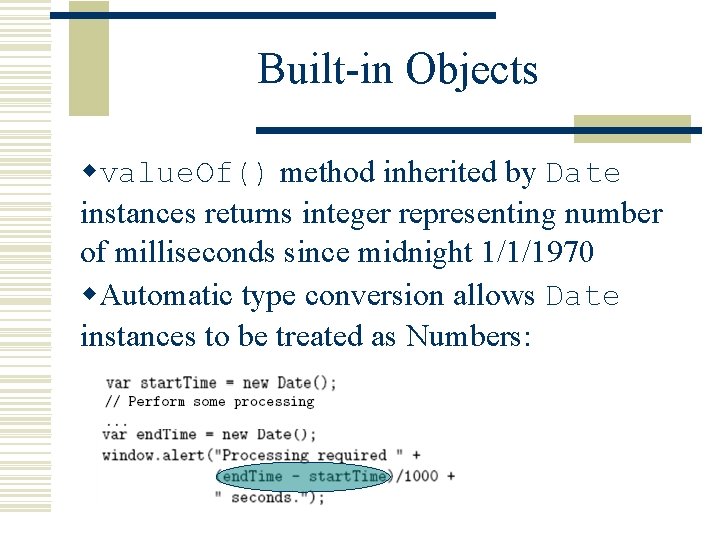
Built-in Objects wvalue. Of() method inherited by Date instances returns integer representing number of milliseconds since midnight 1/1/1970 w. Automatic type conversion allows Date instances to be treated as Numbers:
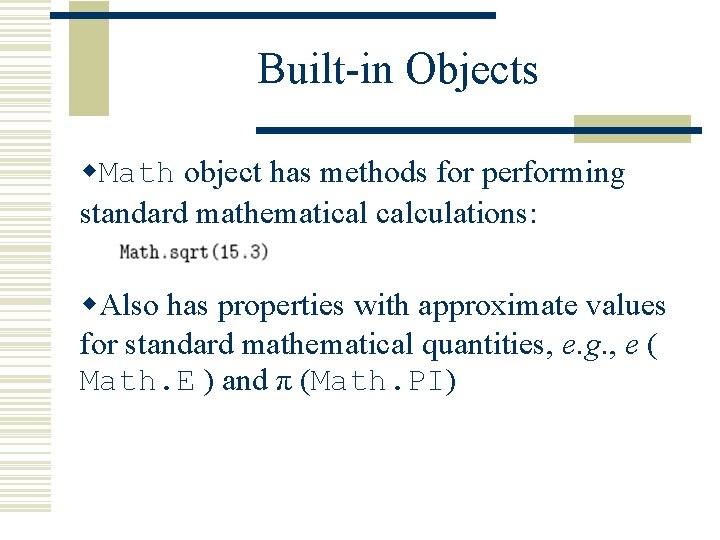
Built-in Objects w. Math object has methods for performing standard mathematical calculations: w. Also has properties with approximate values for standard mathematical quantities, e. g. , e ( Math. E ) and π (Math. PI)
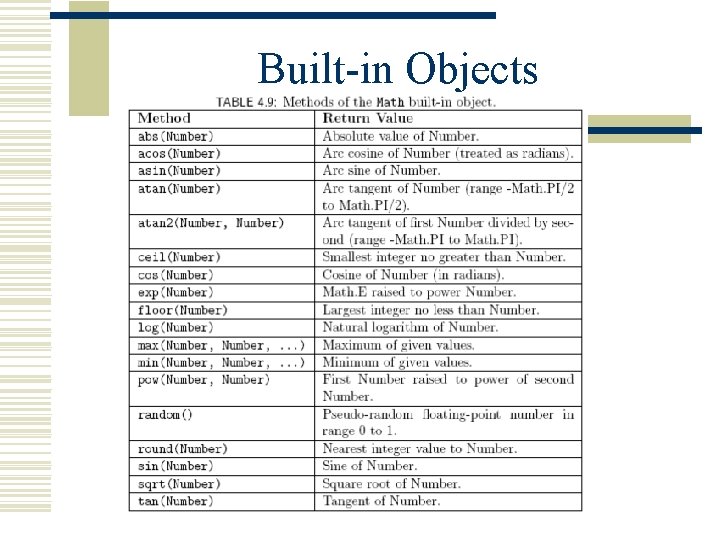
Built-in Objects
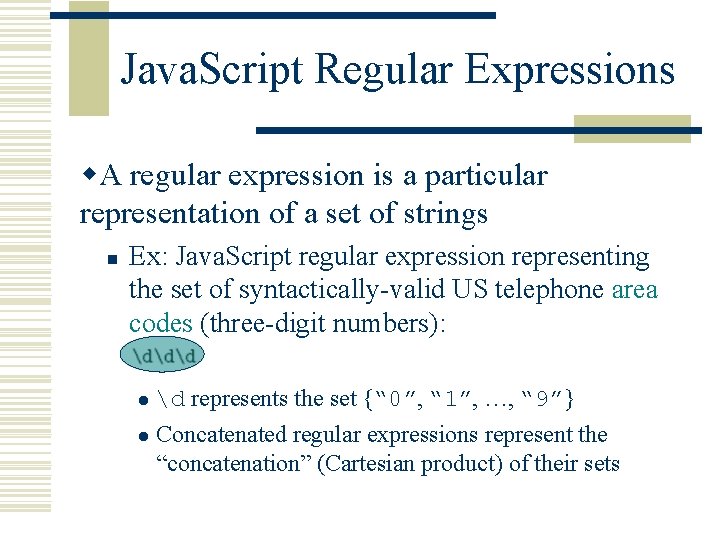
Java. Script Regular Expressions w. A regular expression is a particular representation of a set of strings n Ex: Java. Script regular expression representing the set of syntactically-valid US telephone area codes (three-digit numbers): l d represents the set {“ 0”, “ 1”, …, “ 9”} l Concatenated regular expressions represent the “concatenation” (Cartesian product) of their sets
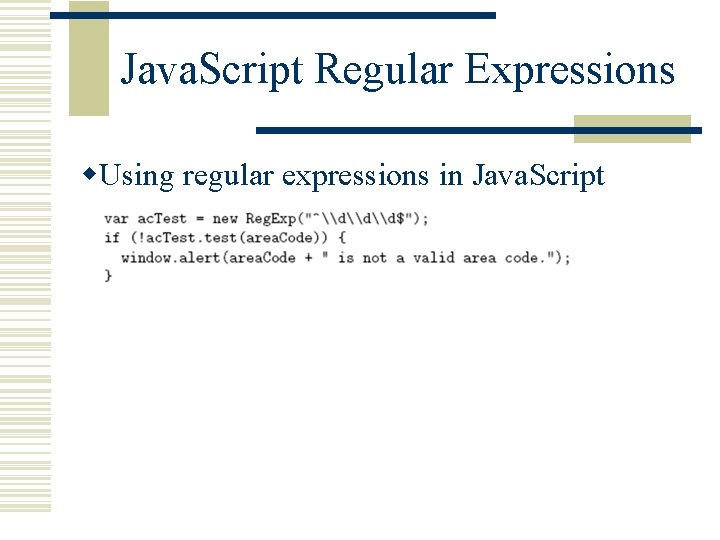
Java. Script Regular Expressions w. Using regular expressions in Java. Script
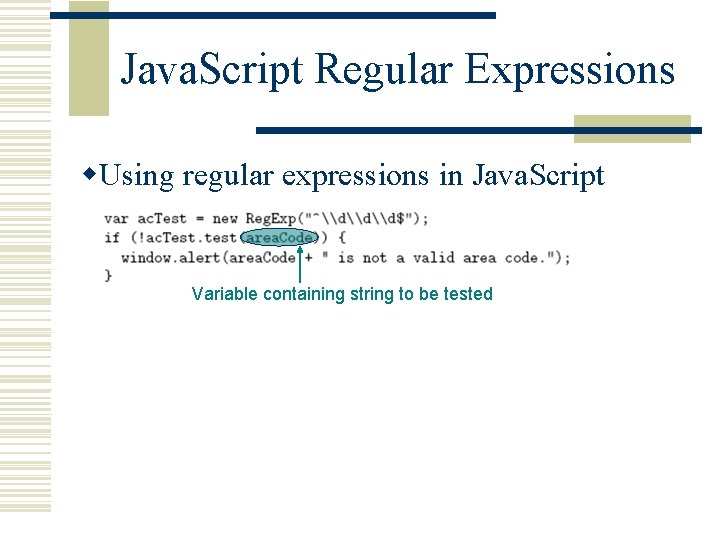
Java. Script Regular Expressions w. Using regular expressions in Java. Script Variable containing string to be tested
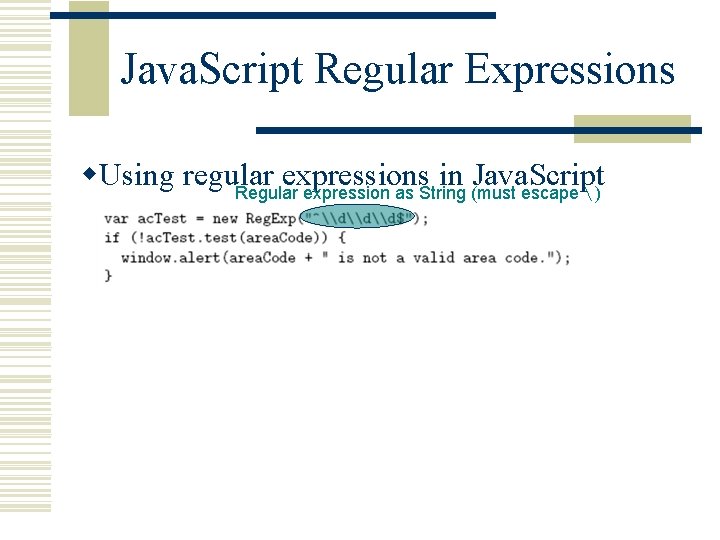
Java. Script Regular Expressions w. Using regular expressions in Java. Script Regular expression as String (must escape )
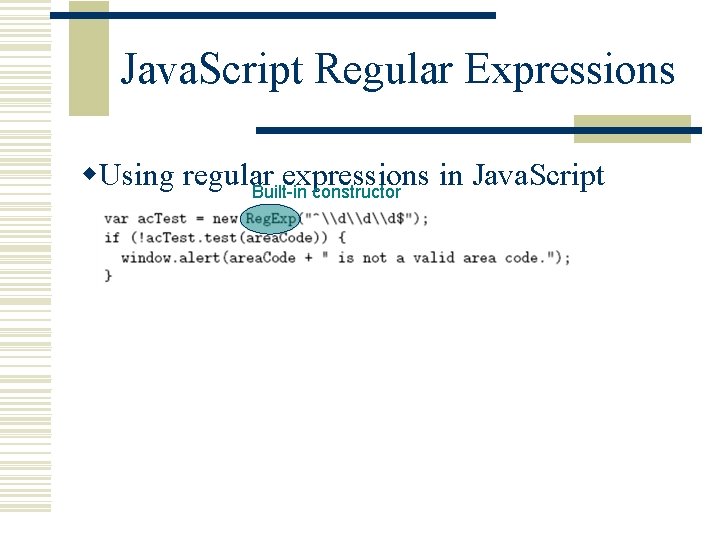
Java. Script Regular Expressions w. Using regular expressions in Java. Script Built-in constructor
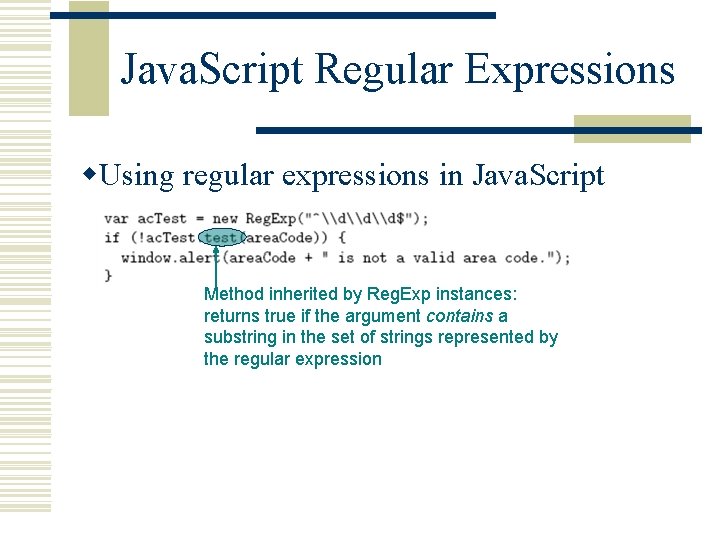
Java. Script Regular Expressions w. Using regular expressions in Java. Script Method inherited by Reg. Exp instances: returns true if the argument contains a substring in the set of strings represented by the regular expression
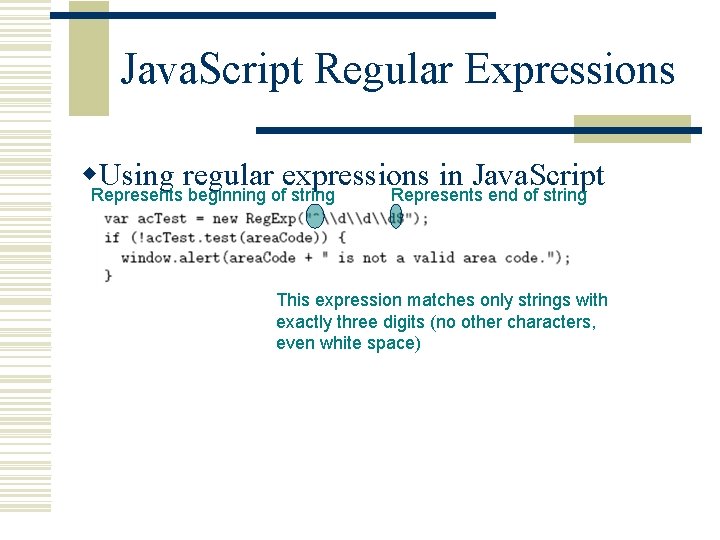
Java. Script Regular Expressions w. Represents Using regular expressions in Java. Script beginning of string Represents end of string This expression matches only strings with exactly three digits (no other characters, even white space)
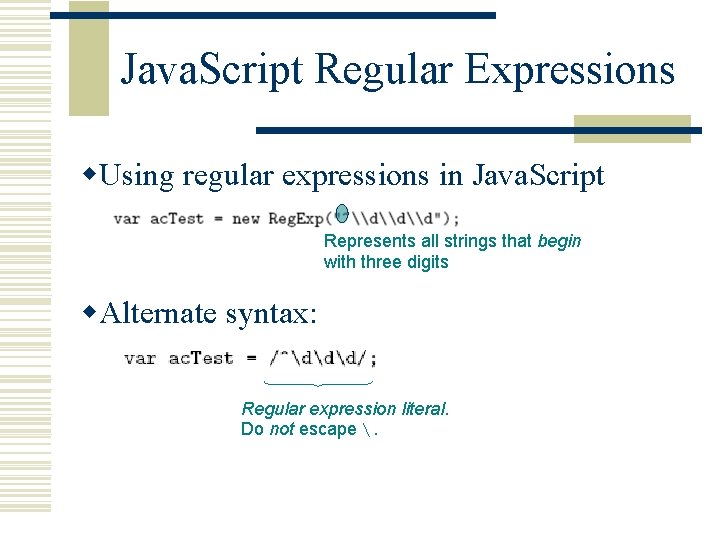
Java. Script Regular Expressions w. Using regular expressions in Java. Script Represents all strings that begin with three digits w. Alternate syntax: Regular expression literal. Do not escape .
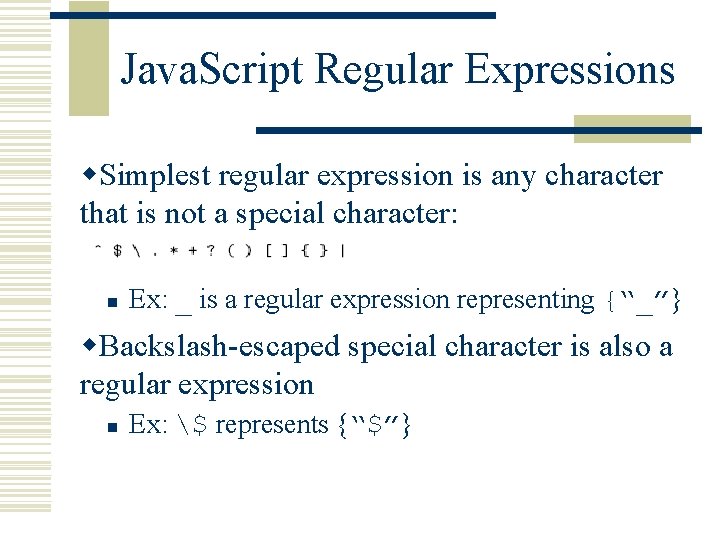
Java. Script Regular Expressions w. Simplest regular expression is any character that is not a special character: n Ex: _ is a regular expression representing {“_”} w. Backslash-escaped special character is also a regular expression n Ex: $ represents {“$”}
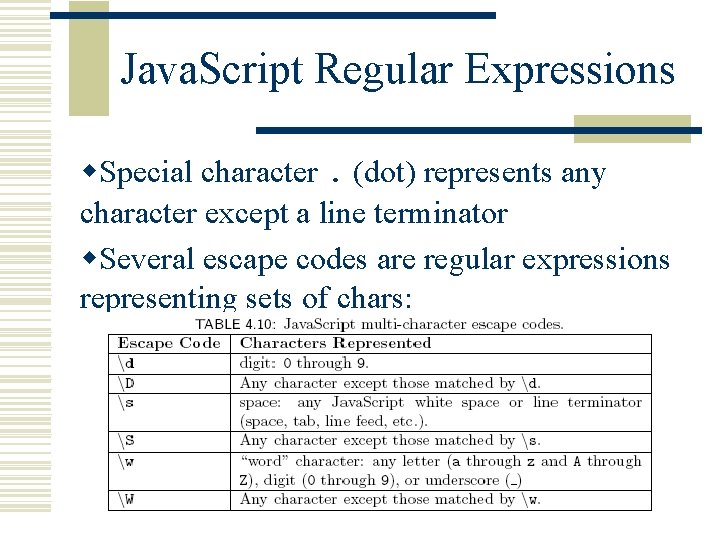
Java. Script Regular Expressions w. Special character. (dot) represents any character except a line terminator w. Several escape codes are regular expressions representing sets of chars:
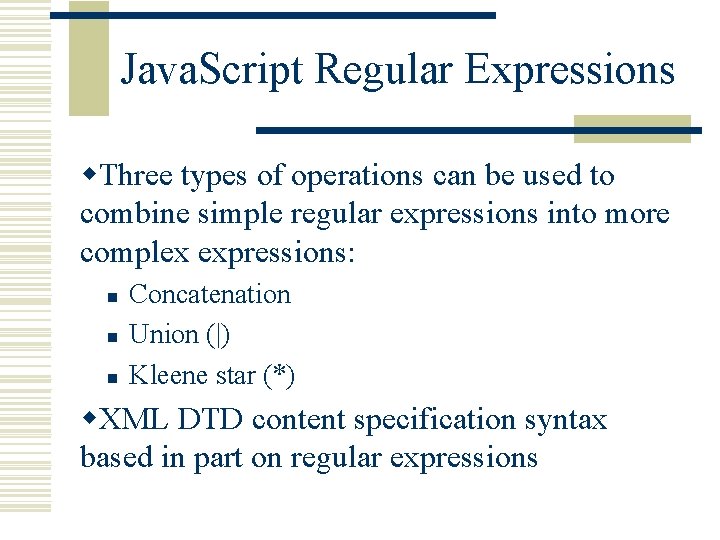
Java. Script Regular Expressions w. Three types of operations can be used to combine simple regular expressions into more complex expressions: n n n Concatenation Union (|) Kleene star (*) w. XML DTD content specification syntax based in part on regular expressions
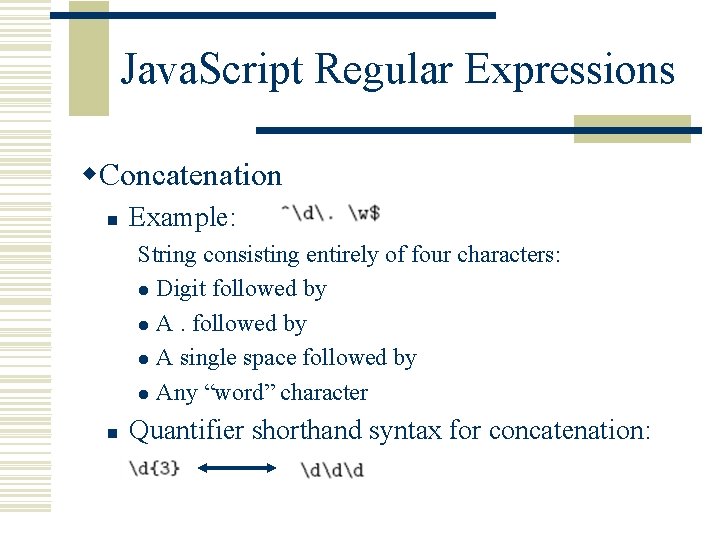
Java. Script Regular Expressions w. Concatenation n Example: String consisting entirely of four characters: l Digit followed by l A single space followed by l Any “word” character n Quantifier shorthand syntax for concatenation:
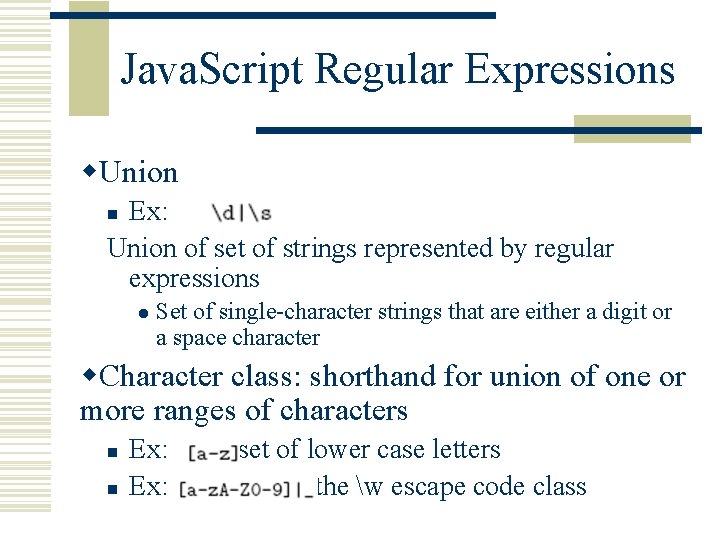
Java. Script Regular Expressions w. Union Ex: Union of set of strings represented by regular expressions n l Set of single-character strings that are either a digit or a space character w. Character class: shorthand for union of one or more ranges of characters n n Ex: set of lower case letters the w escape code class
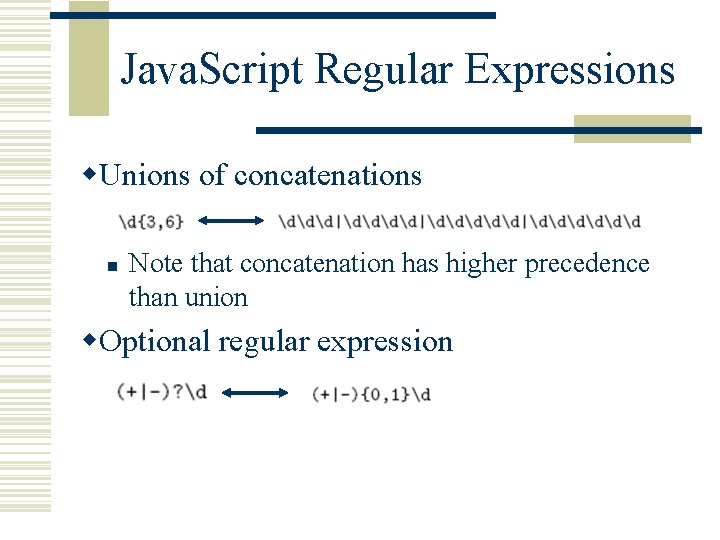
Java. Script Regular Expressions w. Unions of concatenations n Note that concatenation has higher precedence than union w. Optional regular expression
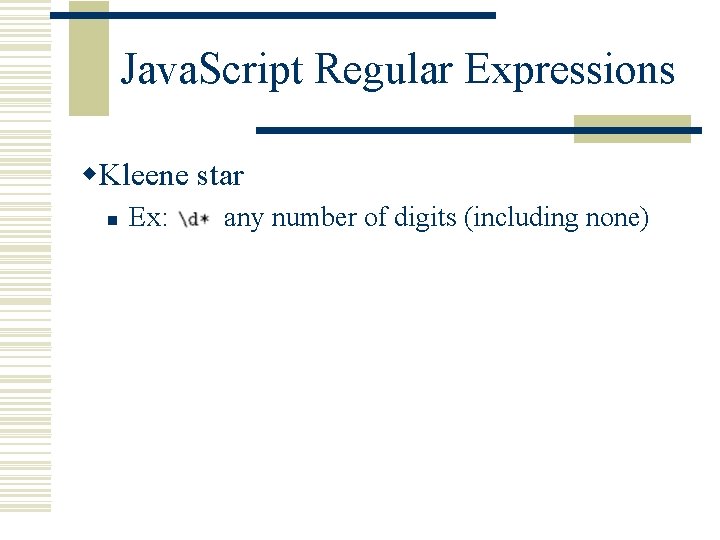
Java. Script Regular Expressions w. Kleene star n Ex: any number of digits (including none)
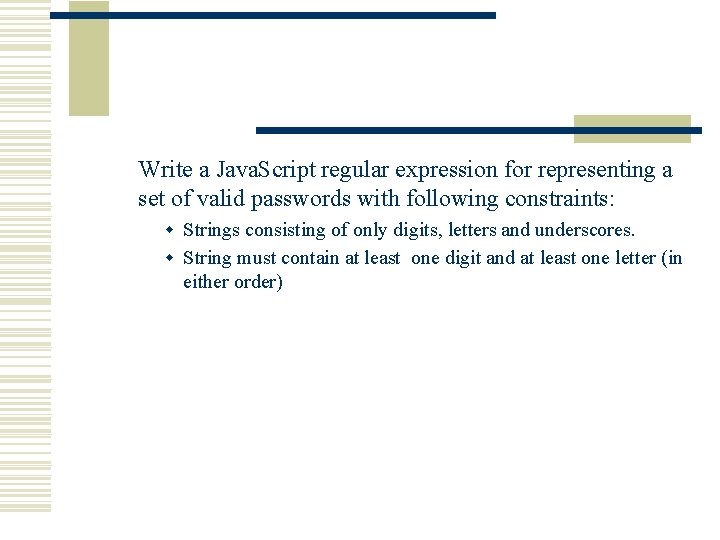
Write a Java. Script regular expression for representing a set of valid passwords with following constraints: w Strings consisting of only digits, letters and underscores. w String must contain at least one digit and at least one letter (in either order)
![wwdwaz AZaz AZwdw ww*(dw*[a-z. A-Z]|[a-z. A-Z]w*d)w*](https://slidetodoc.com/presentation_image_h/181b3a96c0123f868ad0582743dbd6cd/image-153.jpg)
ww*(dw*[a-z. A-Z]|[a-z. A-Z]w*d)w*
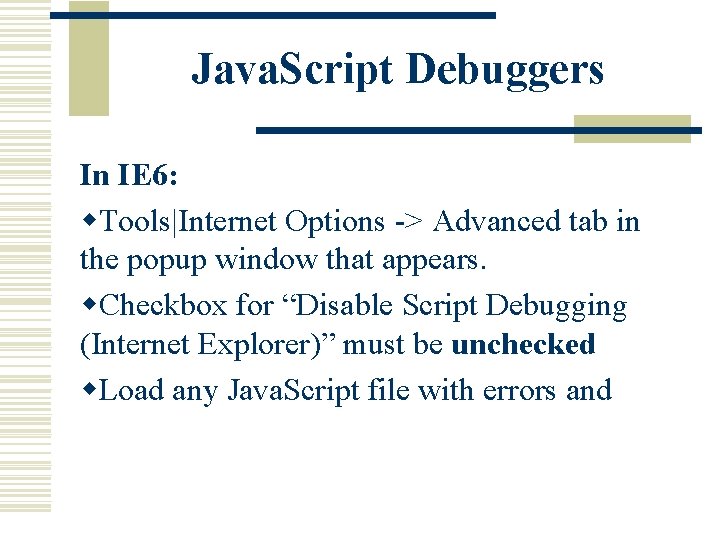
Java. Script Debuggers In IE 6: w. Tools|Internet Options -> Advanced tab in the popup window that appears. w. Checkbox for “Disable Script Debugging (Internet Explorer)” must be unchecked w. Load any Java. Script file with errors and
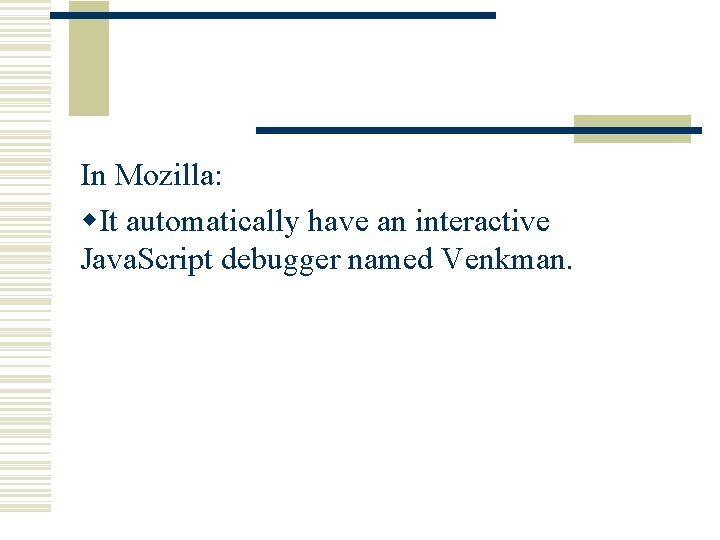
In Mozilla: w. It automatically have an interactive Java. Script debugger named Venkman.
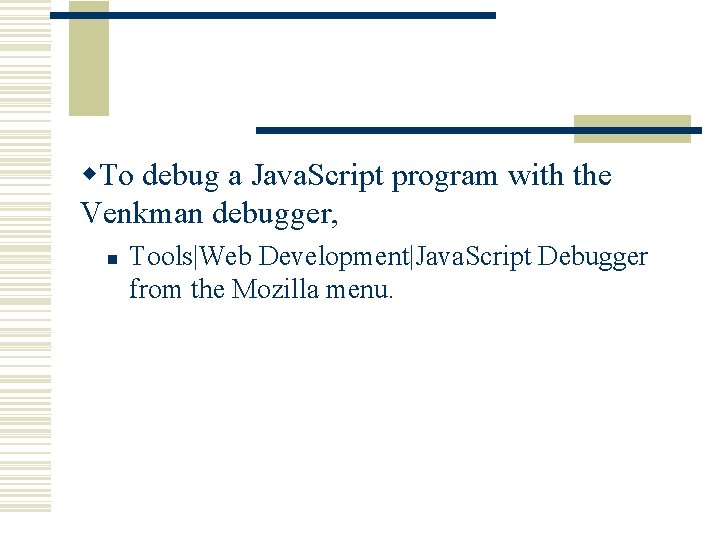
w. To debug a Java. Script program with the Venkman debugger, n Tools|Web Development|Java. Script Debugger from the Mozilla menu.
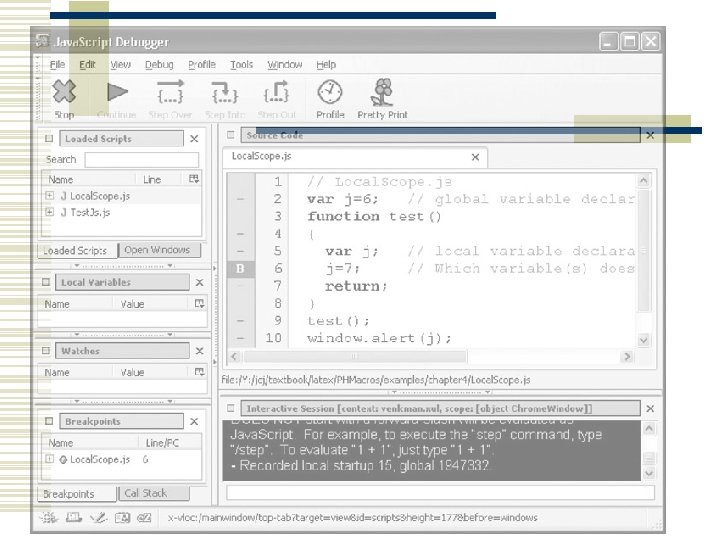
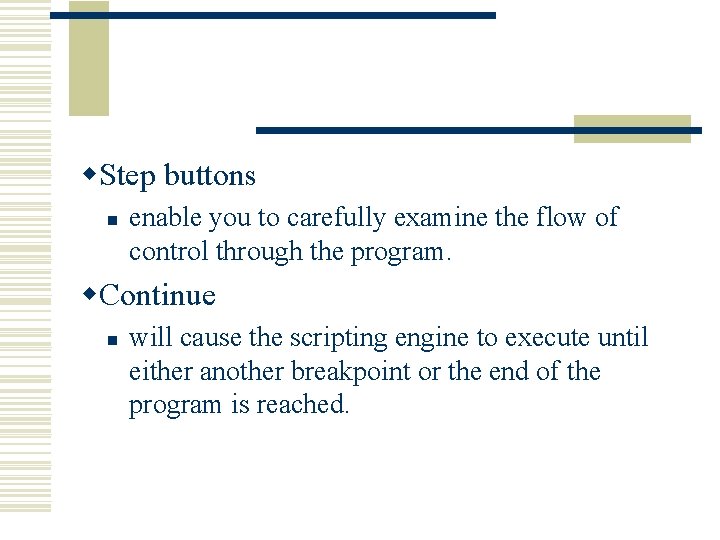
w. Step buttons n enable you to carefully examine the flow of control through the program. w. Continue n will cause the scripting engine to execute until either another breakpoint or the end of the program is reached.
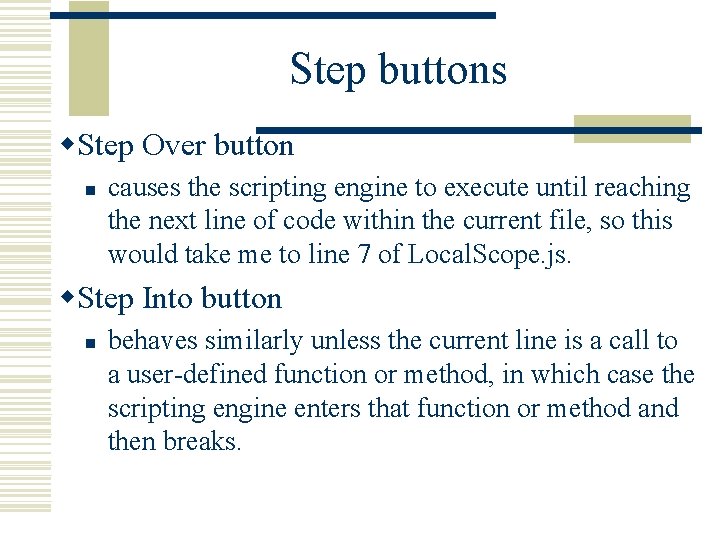
Step buttons w. Step Over button n causes the scripting engine to execute until reaching the next line of code within the current file, so this would take me to line 7 of Local. Scope. js. w. Step Into button n behaves similarly unless the current line is a call to a user-defined function or method, in which case the scripting engine enters that function or method and then breaks.
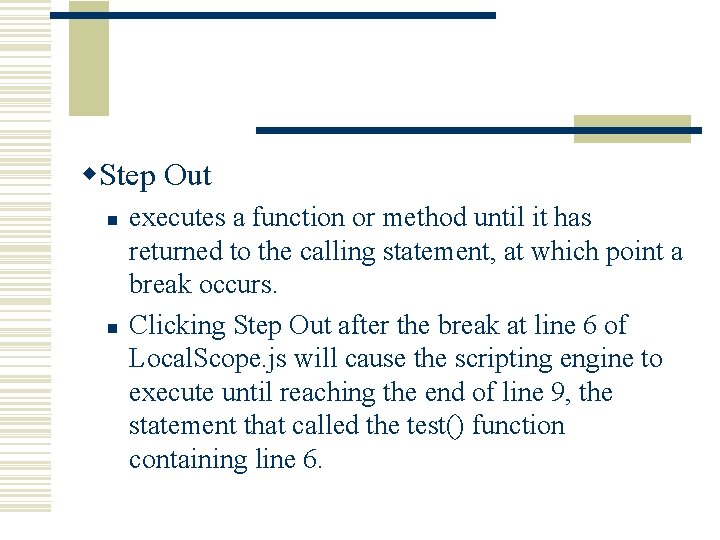
w. Step Out n n executes a function or method until it has returned to the calling statement, at which point a break occurs. Clicking Step Out after the break at line 6 of Local. Scope. js will cause the scripting engine to execute until reaching the end of line 9, the statement that called the test() function containing line 6.
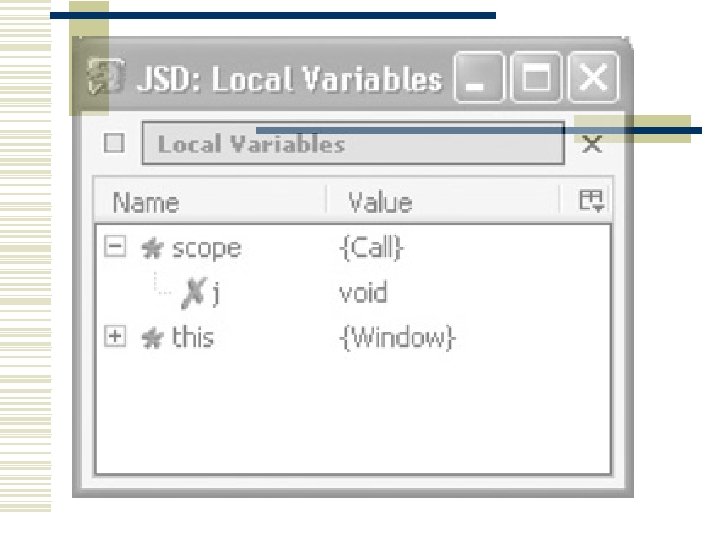
Java programming language
Vhdl hardware description language
Script de java
Data types in javascript
Java script wikipedia
"java script"
"java script"
"java script"
Java script course
Java script
"java script"
Khan academy object oriented programming
Java script examples
Nside which html element do we put the javascript?
Java script email
Js ide
"language fundamentals"
"language fundamentals"
Java script classes
Chapter 16 programming language
Chapter 16 programming language
Chapter 16 programming language
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
System programming
Integer programming vs linear programming
Programing adalah
Create socket java
What is parallel programming in java
Java object oriented exercises
Java introduction to problem solving and programming
Java event driven programming example
Elementary programming in java
Java asynchronous programming
Java structured programming
Importance of java programming
Event driven programming in java
Defensive programming java
Java programming refresher
Open source java games
Java code symbols
Java introduction to problem solving and programming
Programming c
Java database programming
Java asynchronous programming
Conclusion of java
Java programming
Elementary programming in java
Basic elements of java
Advanced programming in java
Java programming enterprise edition course
Dangling else java
Java introduction to problem solving and programming
Introduction to java programming 10th edition quizzes
Language script
Language script
Hát kết hợp bộ gõ cơ thể
Bổ thể
Tỉ lệ cơ thể trẻ em
Voi kéo gỗ như thế nào
Glasgow thang điểm
Hát lên người ơi alleluia
Môn thể thao bắt đầu bằng chữ f
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công của trọng lực
Trời xanh đây là của chúng ta thể thơ
Cách giải mật thư tọa độ
Làm thế nào để 102-1=99
độ dài liên kết
Các châu lục và đại dương trên thế giới
Thể thơ truyền thống
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Cái miệng nó xinh thế chỉ nói điều hay thôi
Vẽ hình chiếu vuông góc của vật thể sau
Nguyên nhân của sự mỏi cơ sinh 8
đặc điểm cơ thể của người tối cổ
V. c c
Vẽ hình chiếu đứng bằng cạnh của vật thể
Vẽ hình chiếu vuông góc của vật thể sau
Thẻ vin
đại từ thay thế
điện thế nghỉ
Tư thế ngồi viết
Diễn thế sinh thái là
Dạng đột biến một nhiễm là
Số nguyên tố là số gì
Tư thế ngồi viết
Lời thề hippocrates
Thiếu nhi thế giới liên hoan
ưu thế lai là gì
Hươu thường đẻ mỗi lứa mấy con
Sự nuôi và dạy con của hươu
Hệ hô hấp
Từ ngữ thể hiện lòng nhân hậu
Thế nào là mạng điện lắp đặt kiểu nổi
Import java util scanner
Java import java.util.*
Import java.applet.applet
Import java.util.scanner;
Import java.io.*
Import java.util.scanner;
Java import java.util.*
Import java.io.* in java
Import java
Java thread import
Perbedaan java swing dan awt
Import java.awt.event
Language
Ejb javatpoint
Pros and cons of virtualization
Assembler
Pic language
Cern programming language
Beta language
Microcontroller assembly language programming
Lll programming language
Language level
Repeat 3 fd 100 rt 120
Programming language logo
Disadvantage of assembly language
Jess expert system
Small talk programming language
Visual studio flowchart
Language
Fundamentals of functional programming language
Cyclone programming language
Blitz programming language
Elements of assembly language programming
Plx programming language
Introduction to assembly language ppt
Assembly language programming examples
Programming language hierarchy
Loop instruction in 8086
Language
Language
Example of fourth generation programming language
Acs programming language
Assembly language programming 8051
What is structured programming language
Rb programming language
Openstack programming language
Arc (programming language)
Manfaat pemrograman terstruktur
Language
Lua rust
Language
Syntactic criteria
Jess shell
8051 interrupts
Eviews programming language
What is structured programming language
Common business oriented language
Flow programming
Fundamentals of functional programming language
Coding hierarchy chart
Programming languages flowchart
Ror programming language
Cem programming language
Second life rotation script