import import java util Random class Import Example
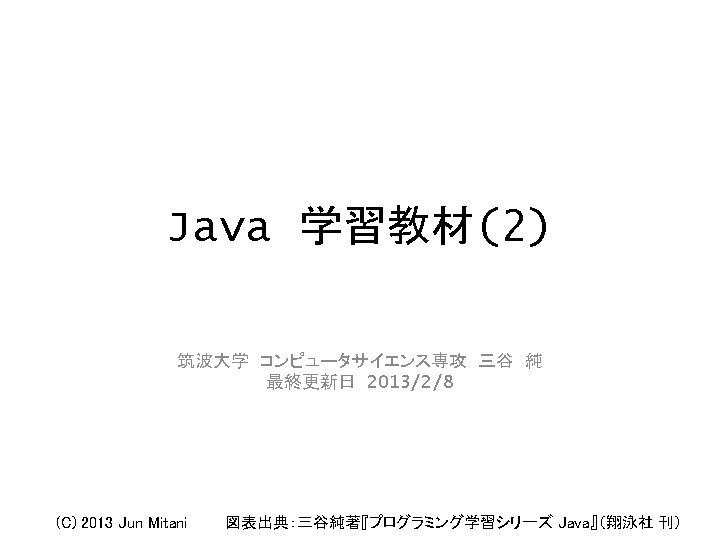
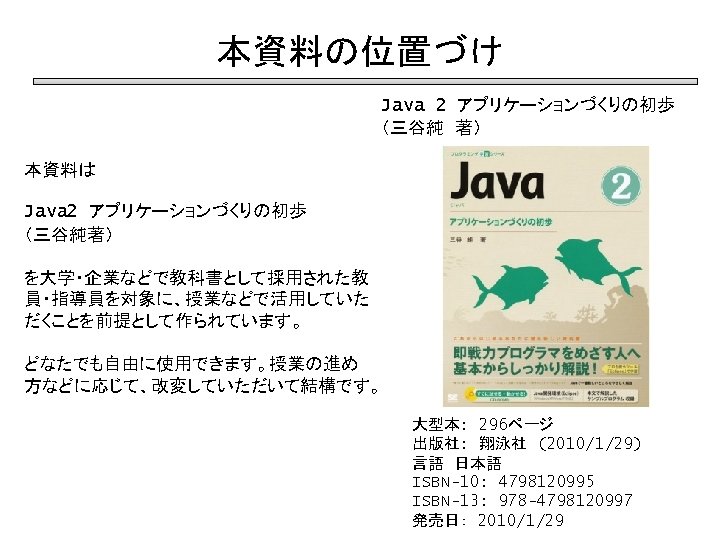
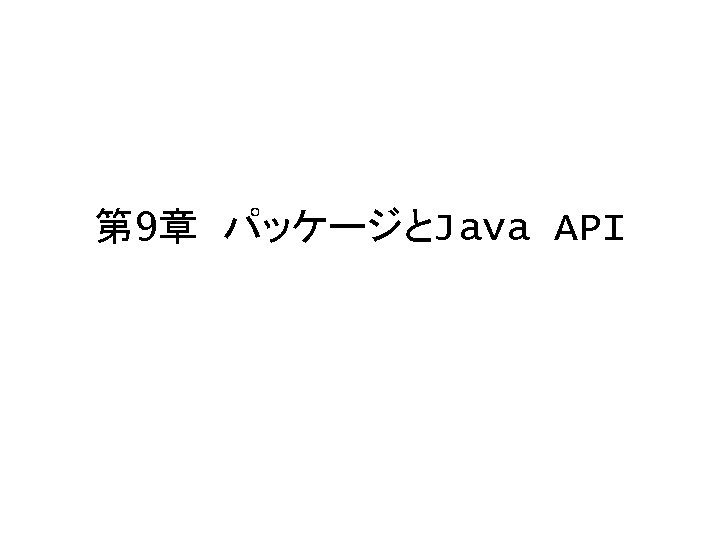
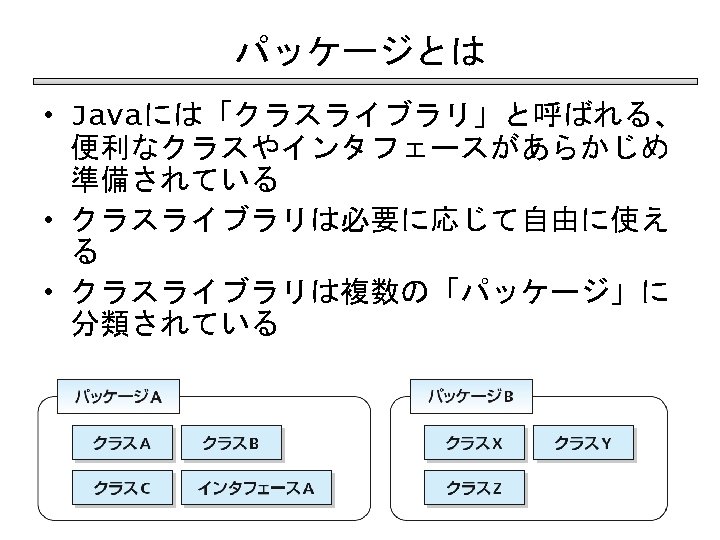
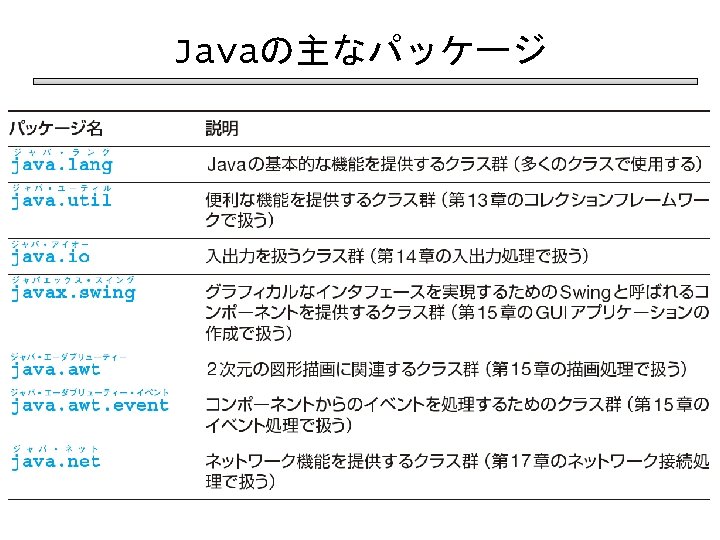
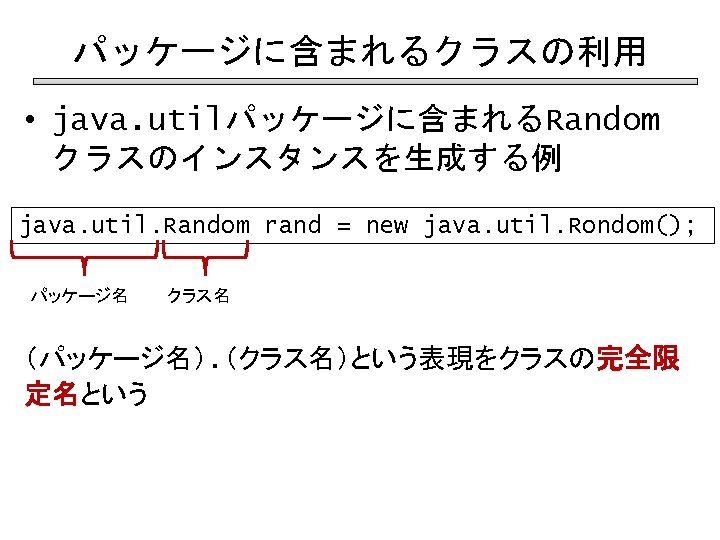
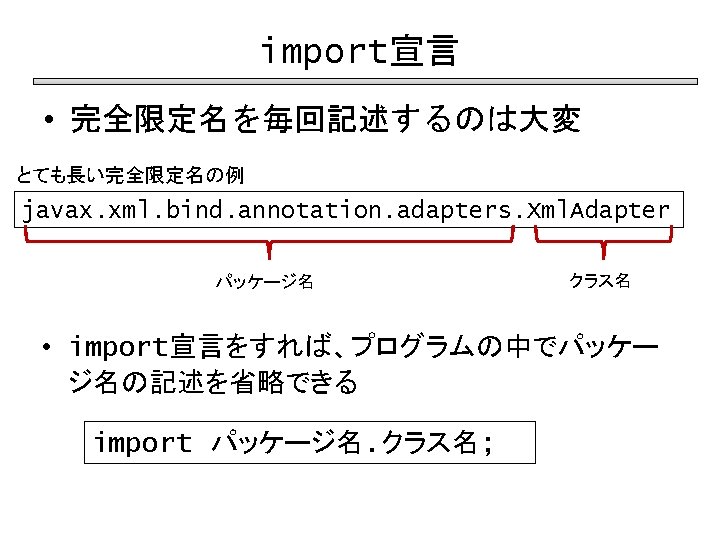
![import宣言の使用例 import java. util. Random; class Import. Example { public static void main(String[] args) import宣言の使用例 import java. util. Random; class Import. Example { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-8.jpg)
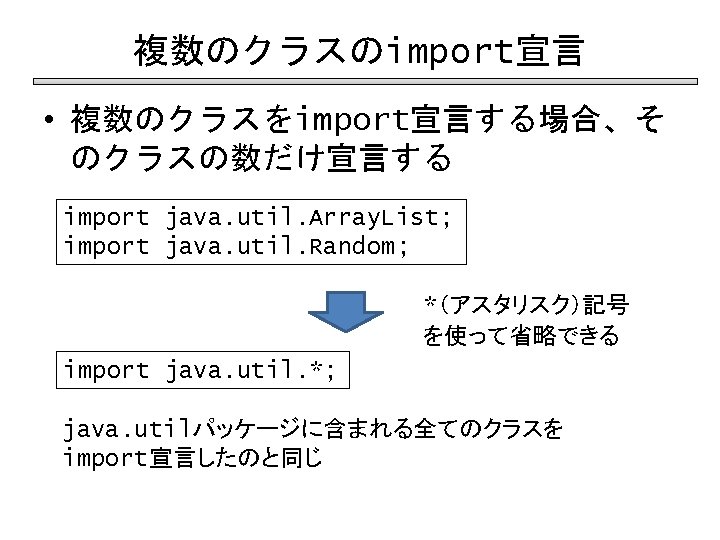
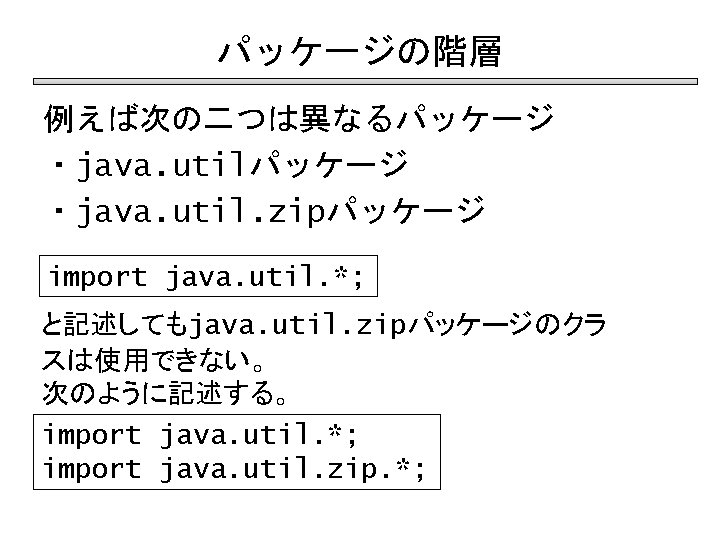
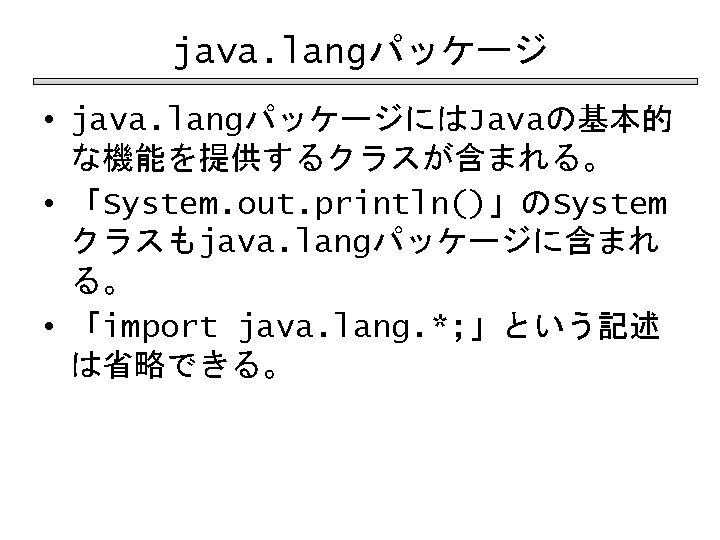
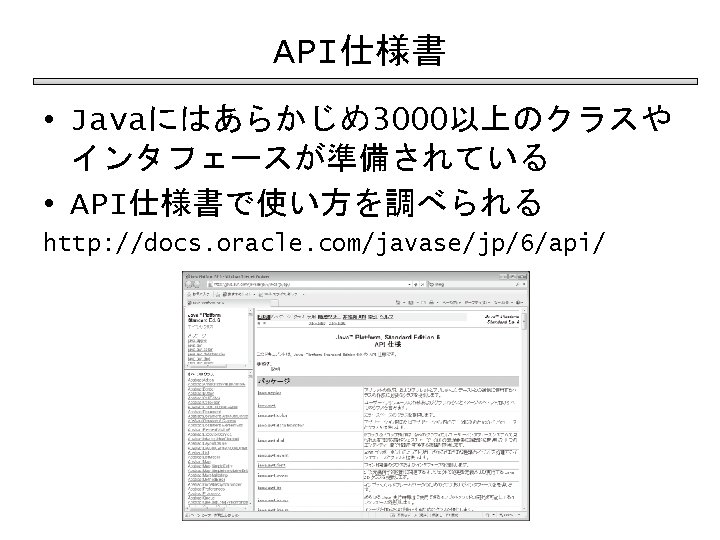
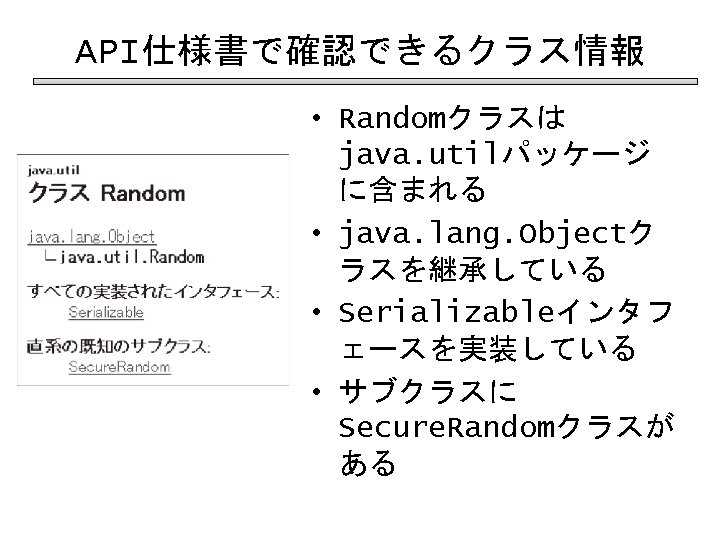
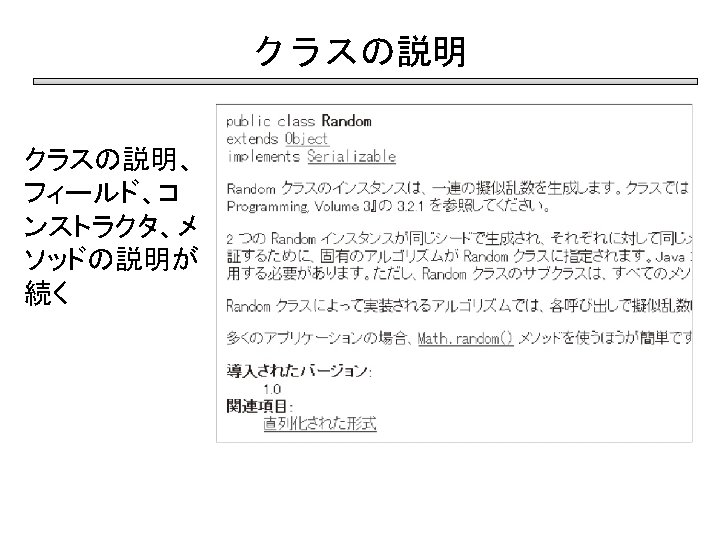
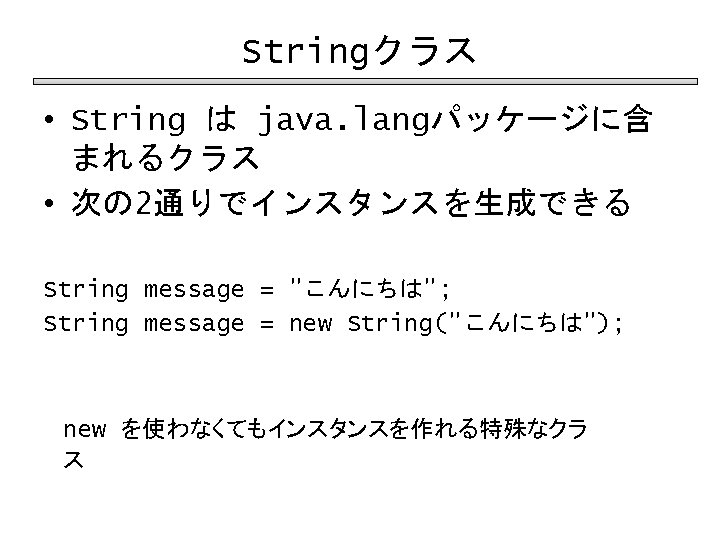
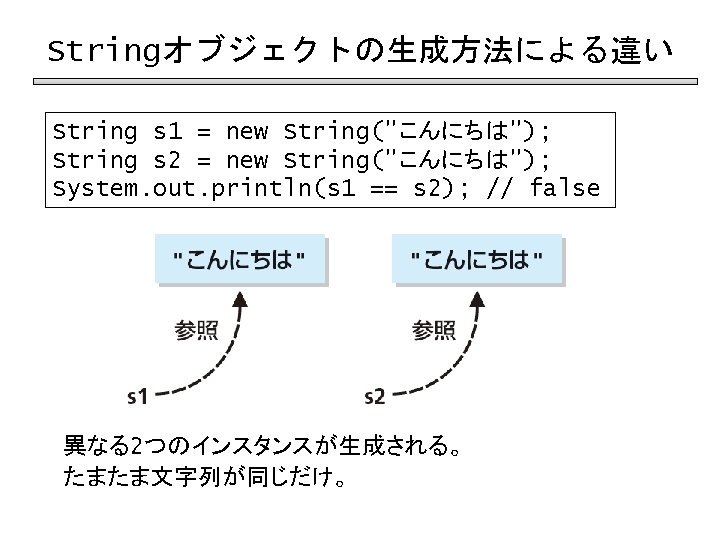
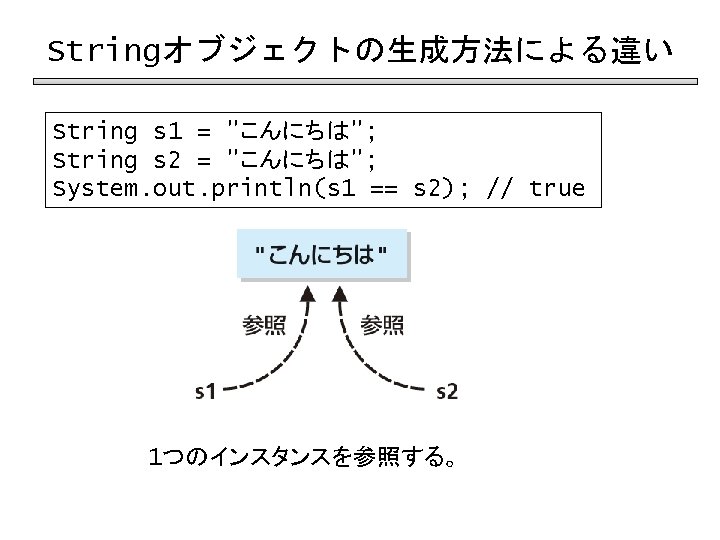
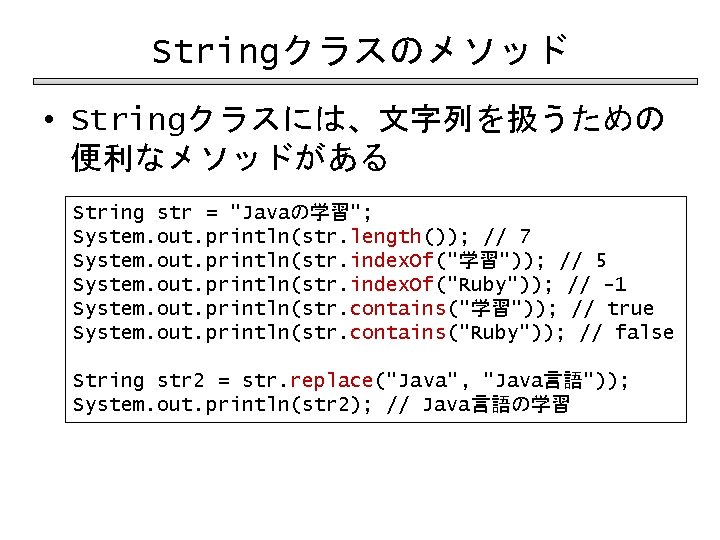
![Stringクラスのメソッド 文字列を区切り記号で分割する例 String str = "2012/12/31"; String[] items = str. split("/"); for(int i = Stringクラスのメソッド 文字列を区切り記号で分割する例 String str = "2012/12/31"; String[] items = str. split("/"); for(int i =](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-19.jpg)
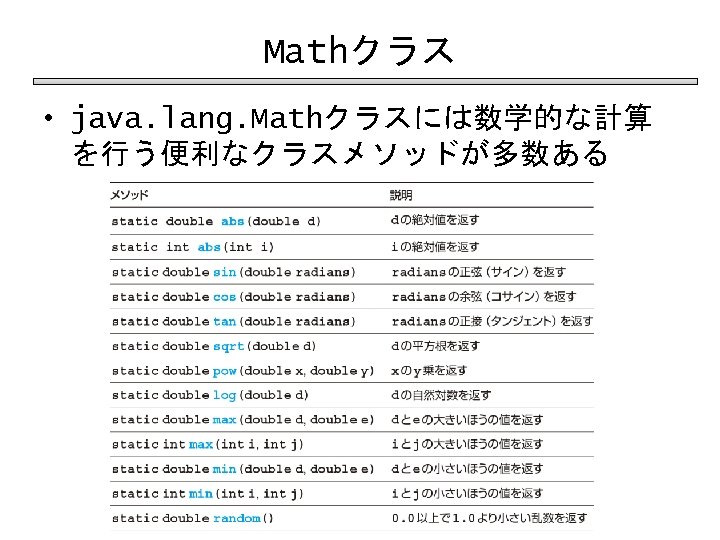
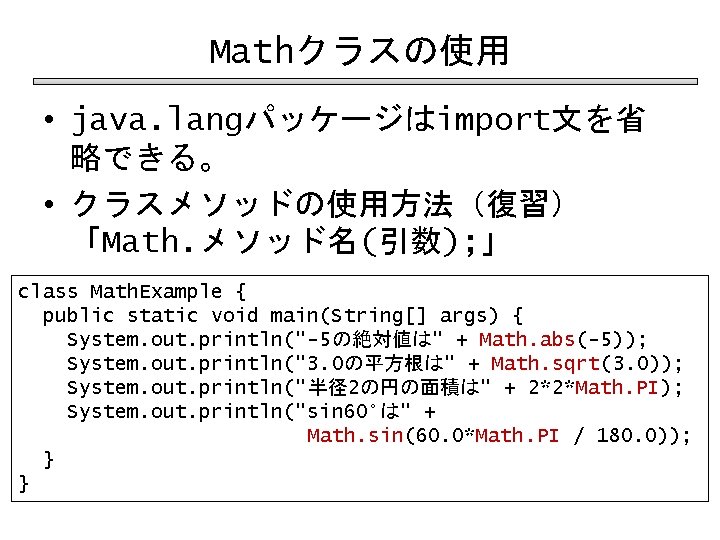
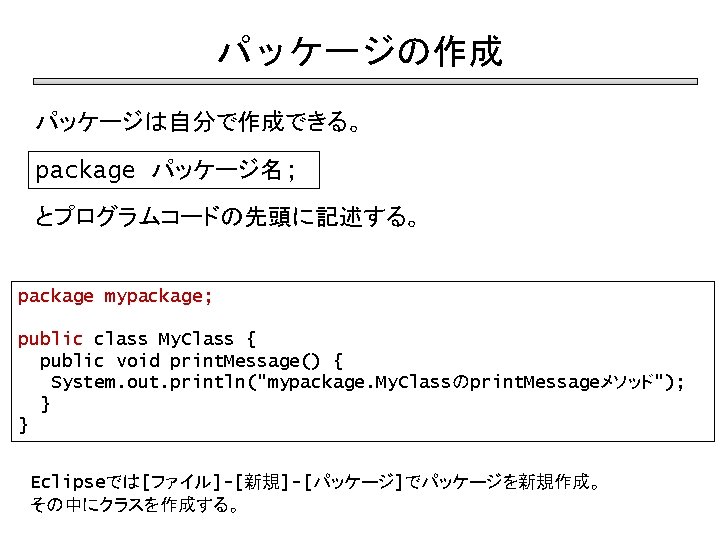
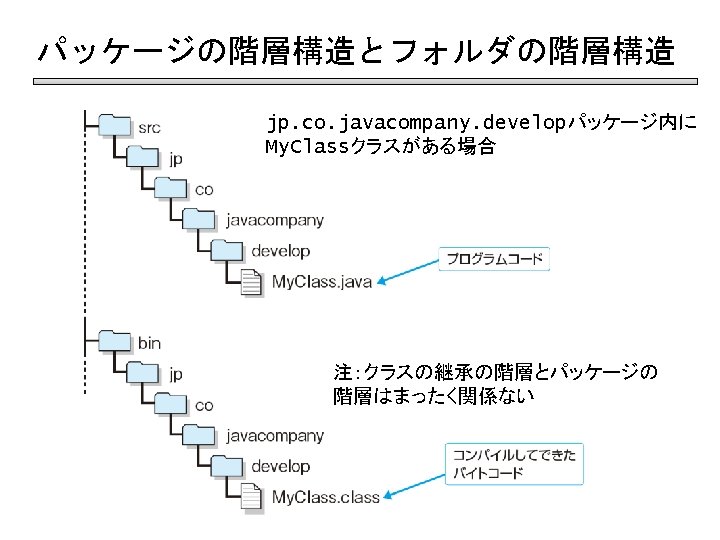
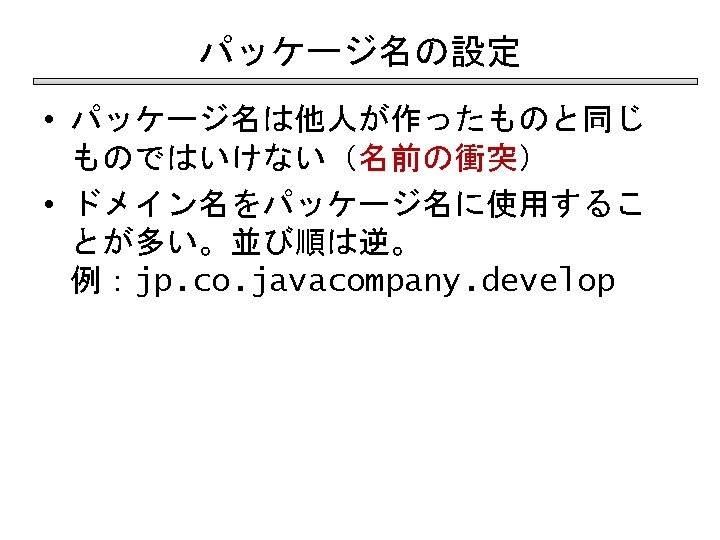
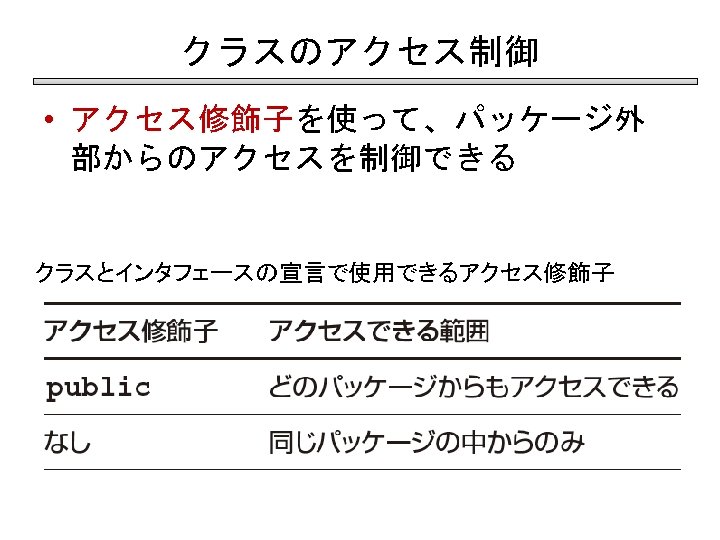
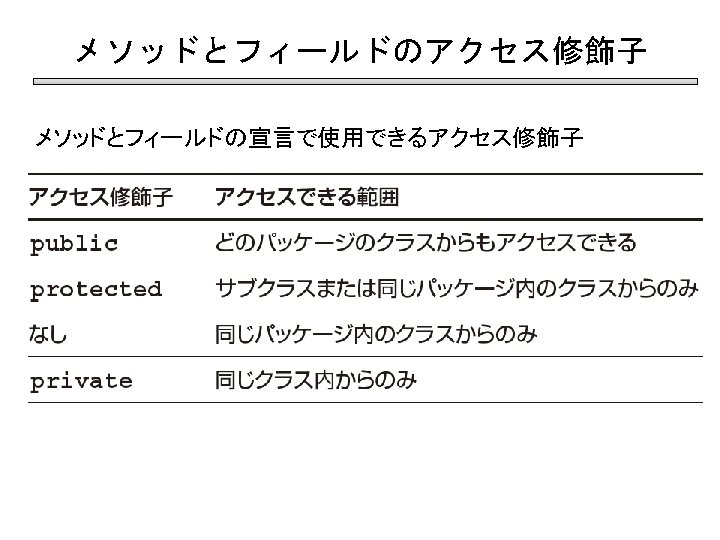
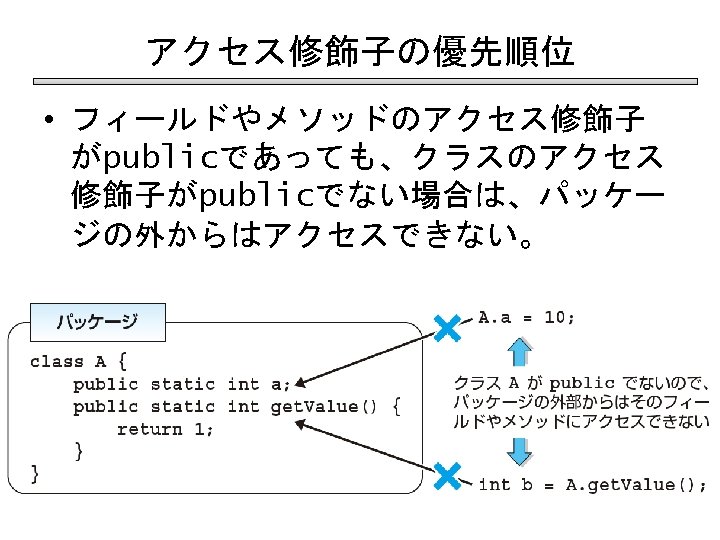
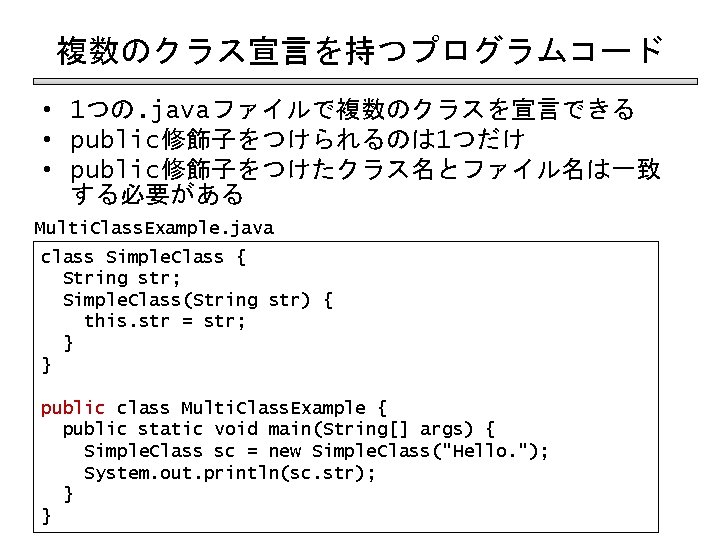
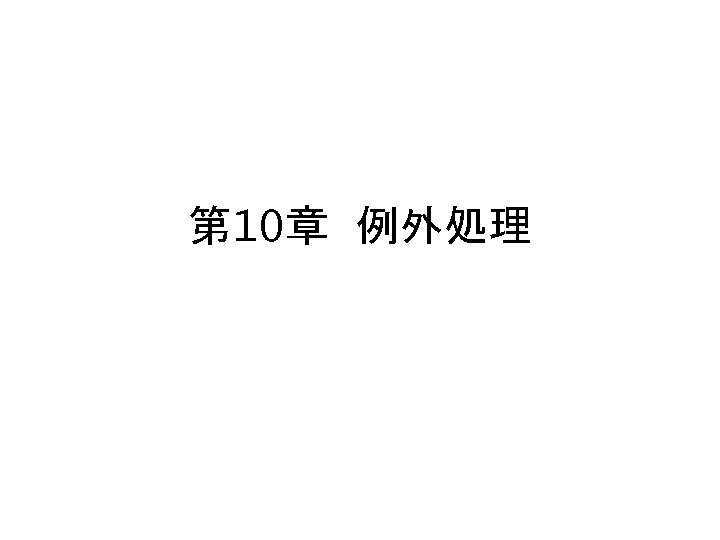
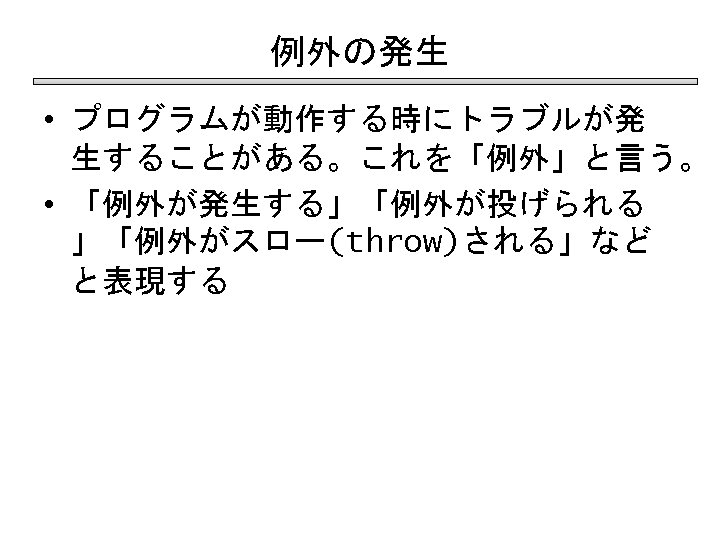
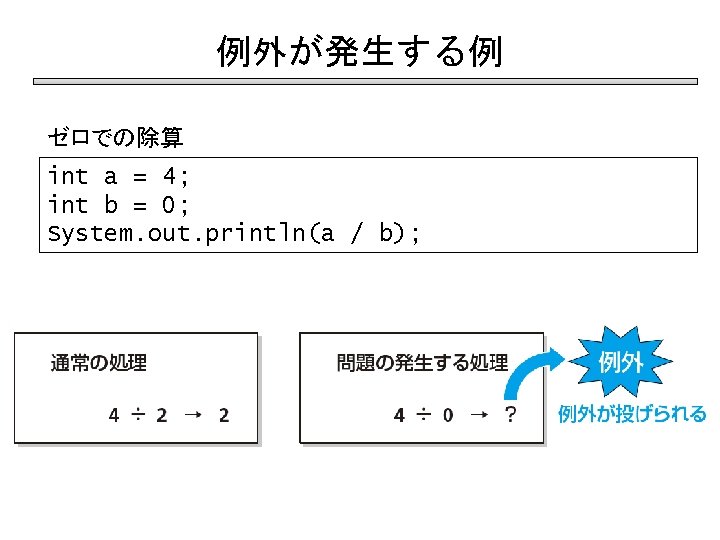
![例外が発生する例 範囲を超えたインデックスの参照 int[] scores = new int[3]; scores[0] = 50; scores[1] = 55; scores[2] 例外が発生する例 範囲を超えたインデックスの参照 int[] scores = new int[3]; scores[0] = 50; scores[1] = 55; scores[2]](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-32.jpg)
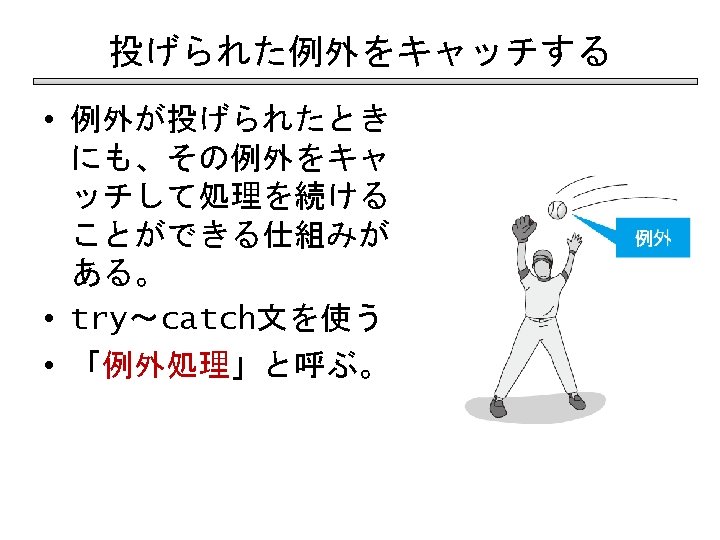
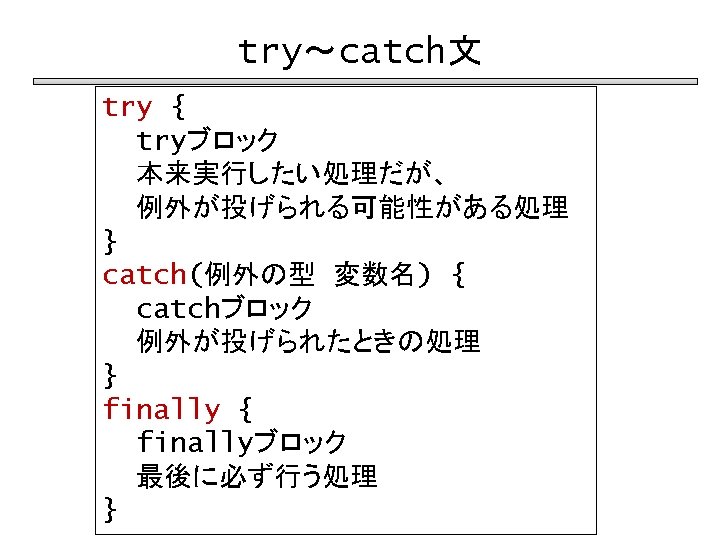
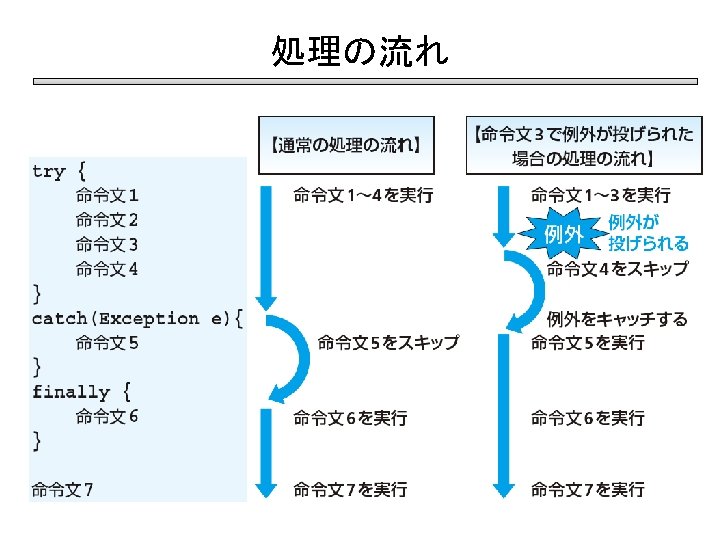
![例外処理の例 public class Exception. Example 3 { public static void main(String[] args) { int 例外処理の例 public class Exception. Example 3 { public static void main(String[] args) { int](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-36.jpg)
![finallyの処理 public static void main(String[] args) { int a = 4; int b = finallyの処理 public static void main(String[] args) { int a = 4; int b =](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-37.jpg)
![catchブロックの検索 class Simple. Class { void do. Something() { int array[] = new int[3]; catchブロックの検索 class Simple. Class { void do. Something() { int array[] = new int[3];](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-38.jpg)
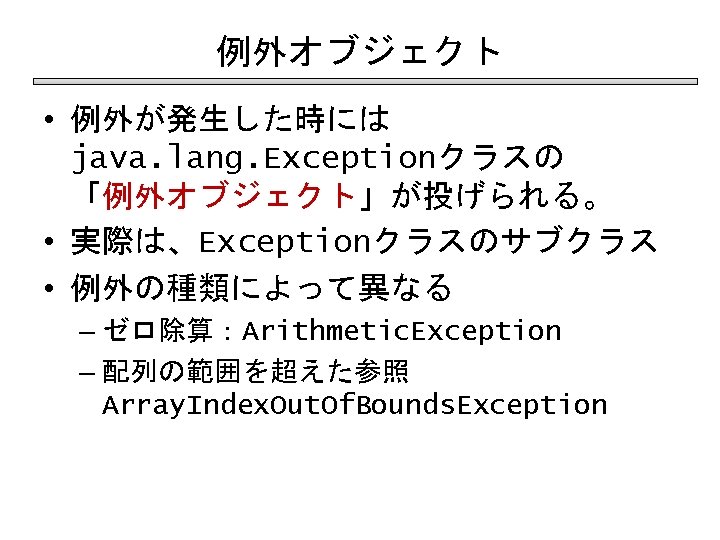
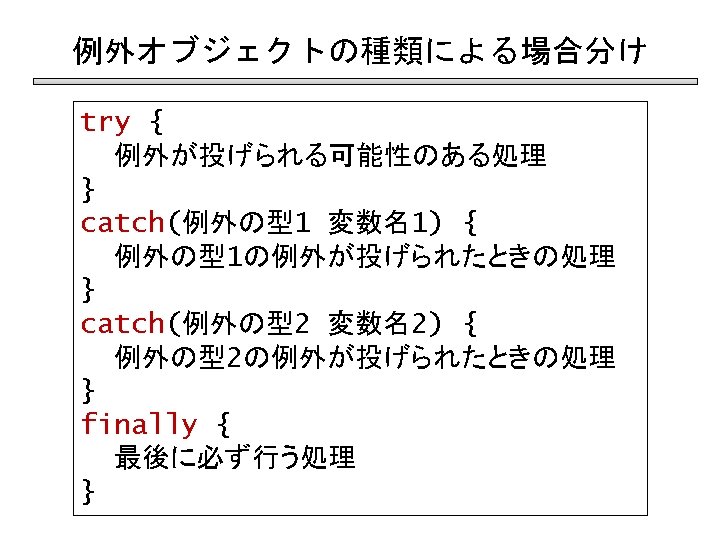
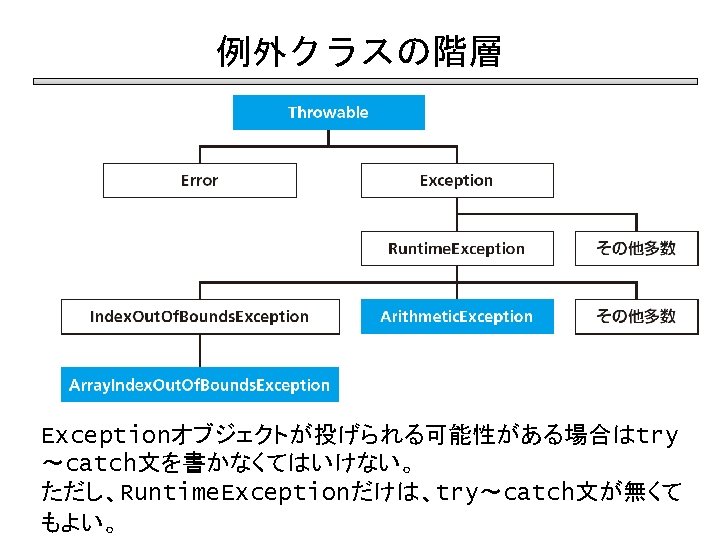
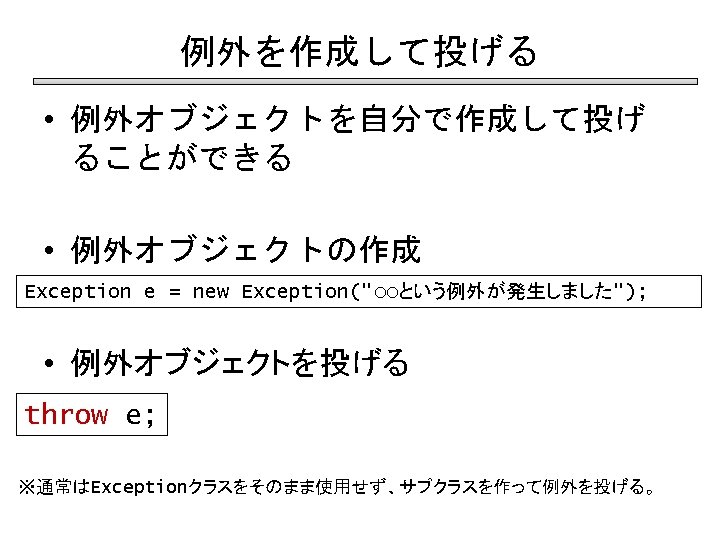
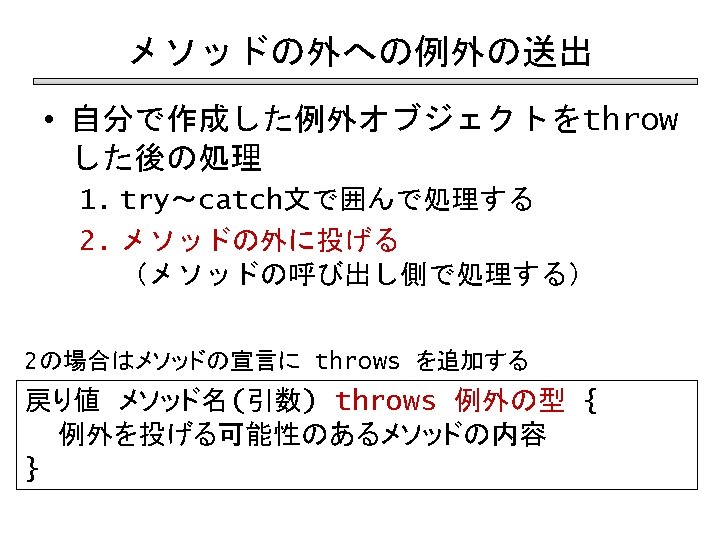
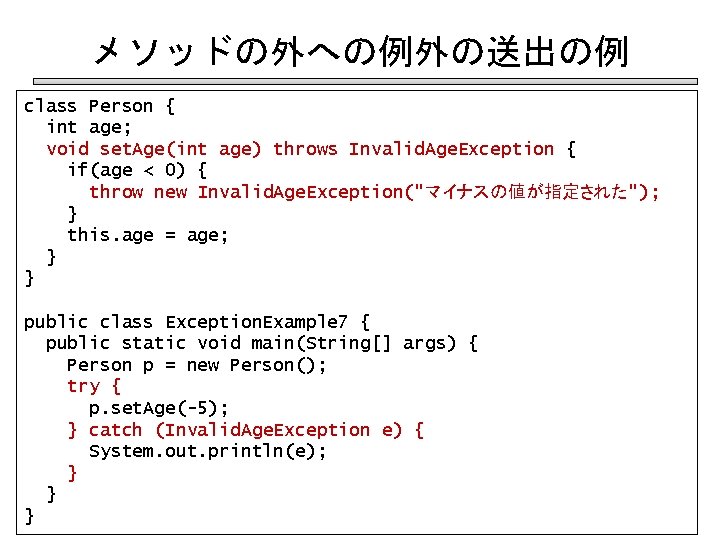
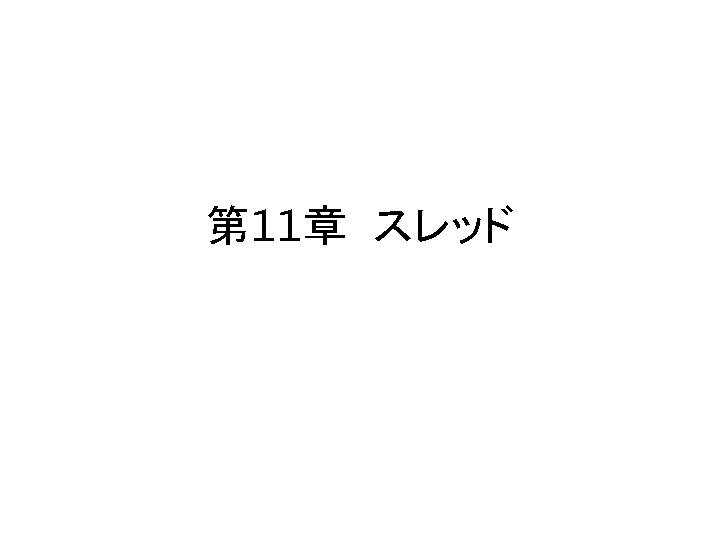
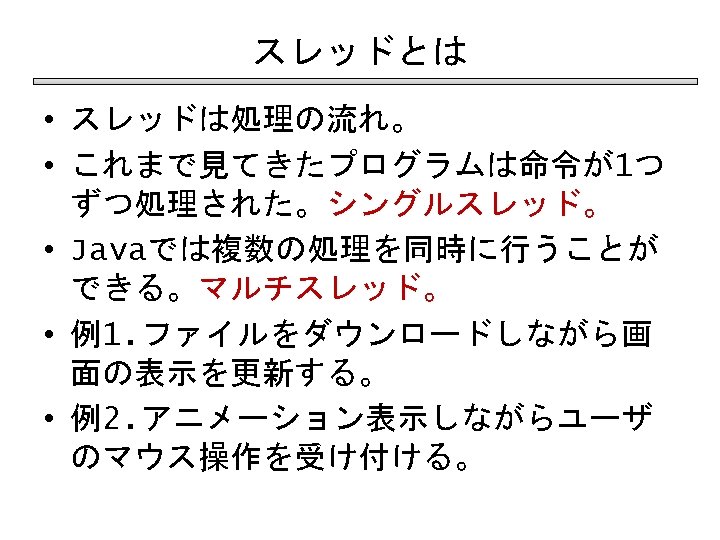
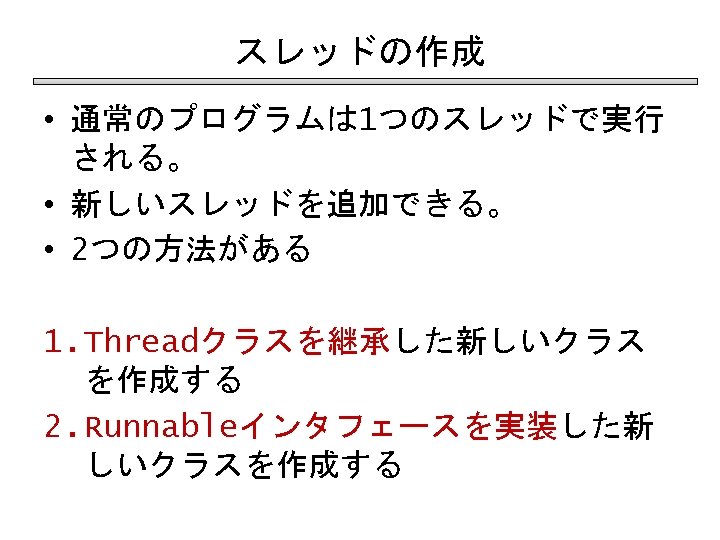
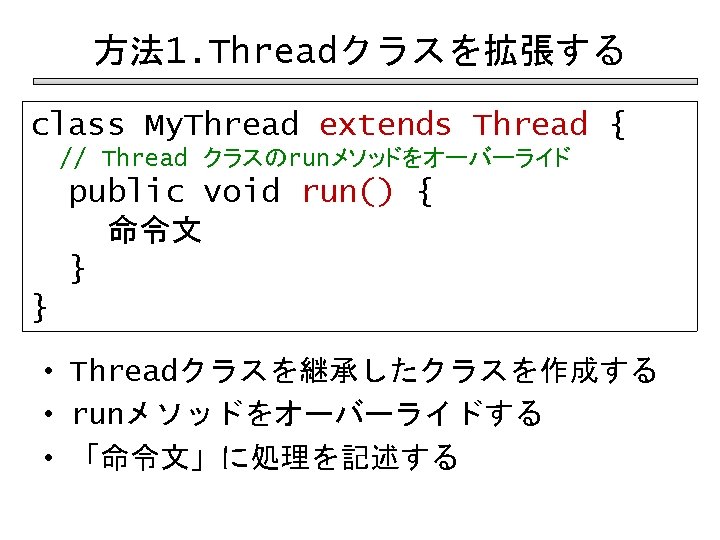
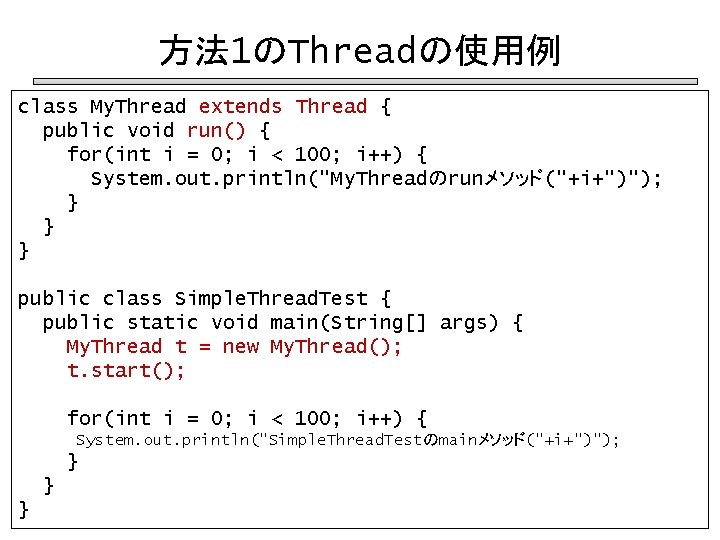
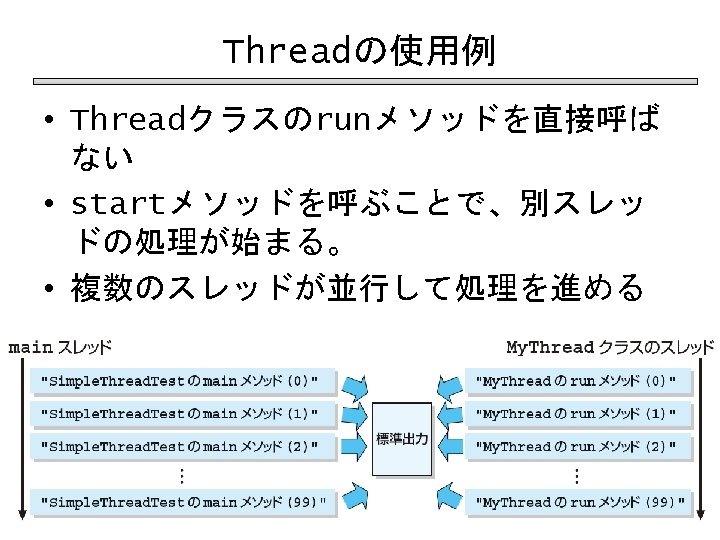
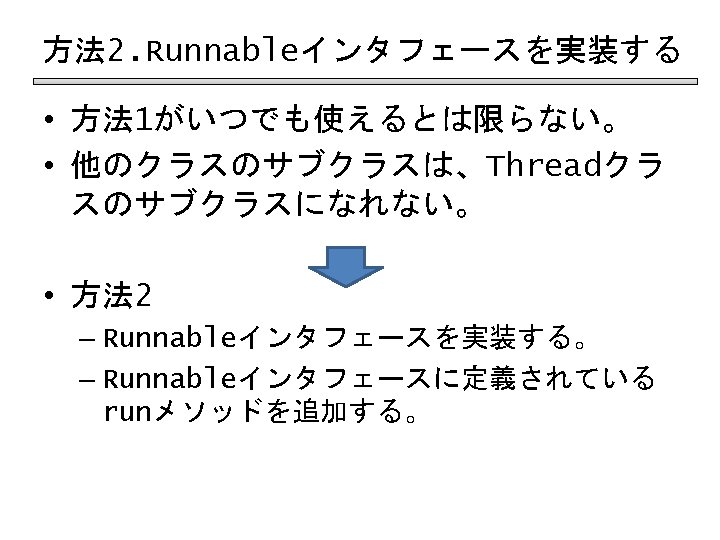
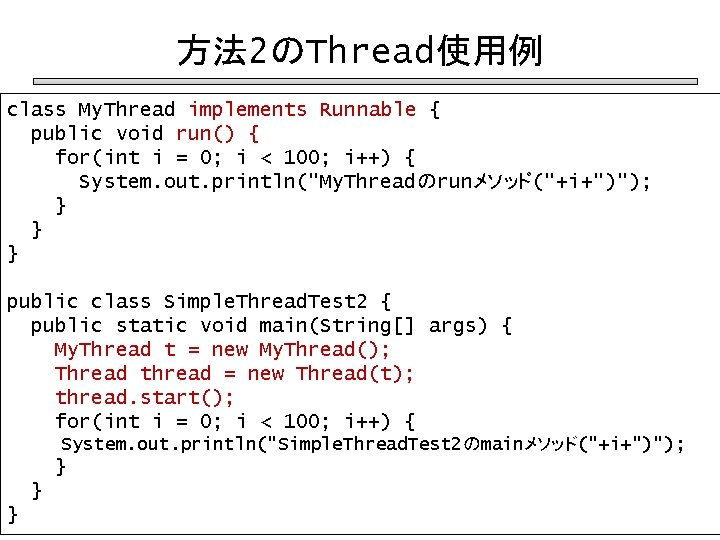
![スレッドを一定時間停止させる Thread. sleep(停止時間(ミリ秒)); public class Sleep. Example { public static void main(String[] args) { スレッドを一定時間停止させる Thread. sleep(停止時間(ミリ秒)); public class Sleep. Example { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-53.jpg)
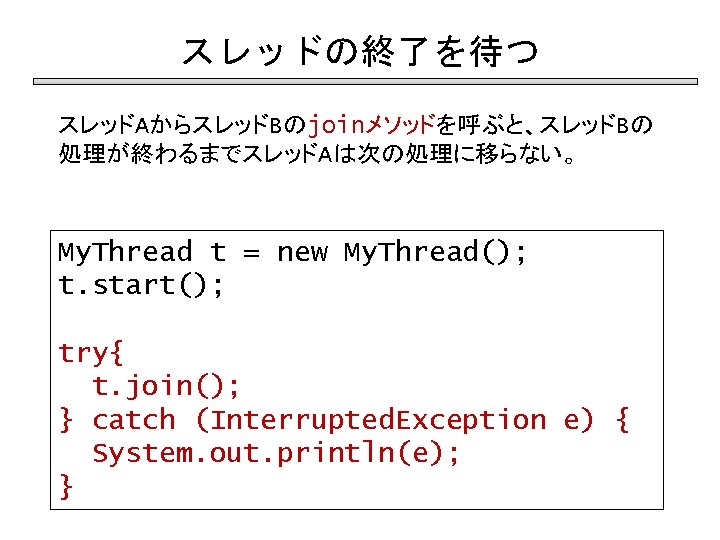
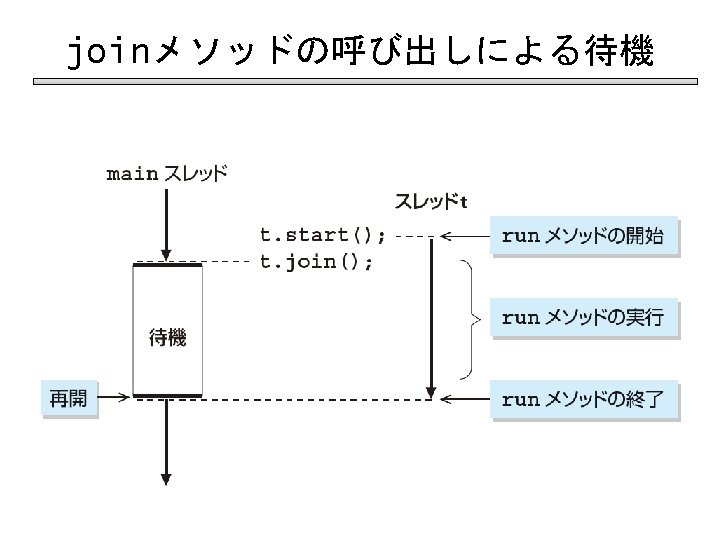
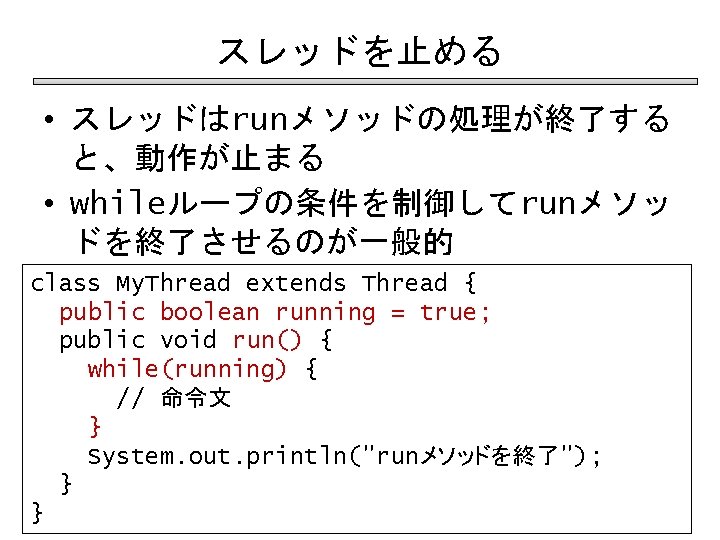
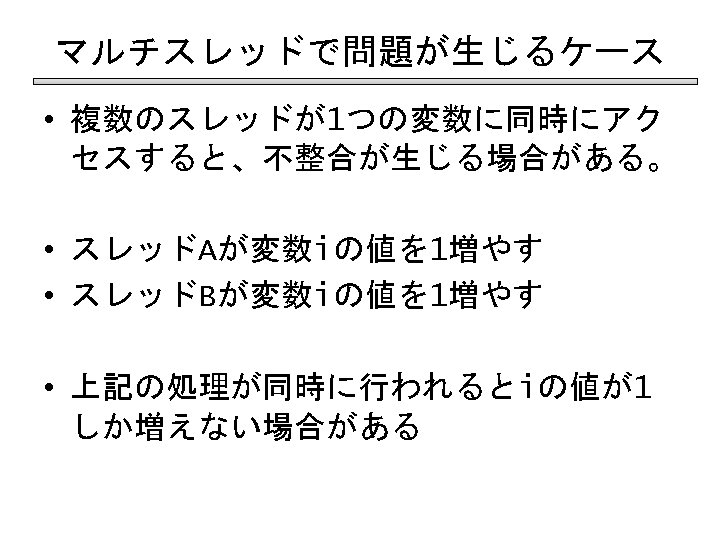
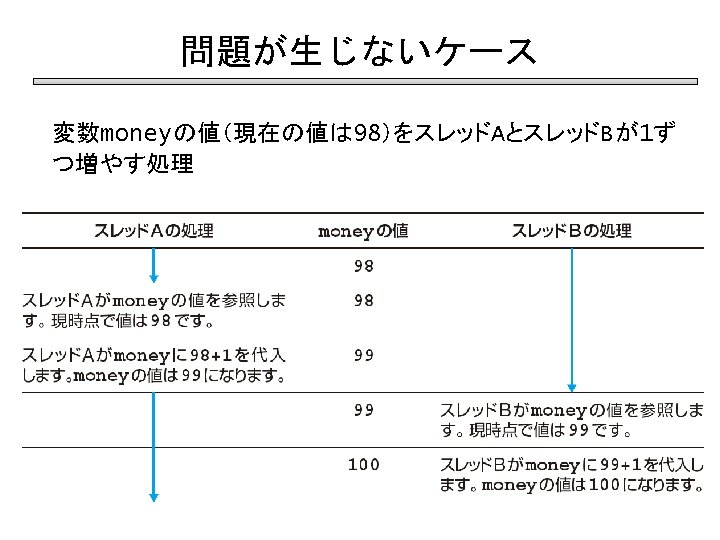
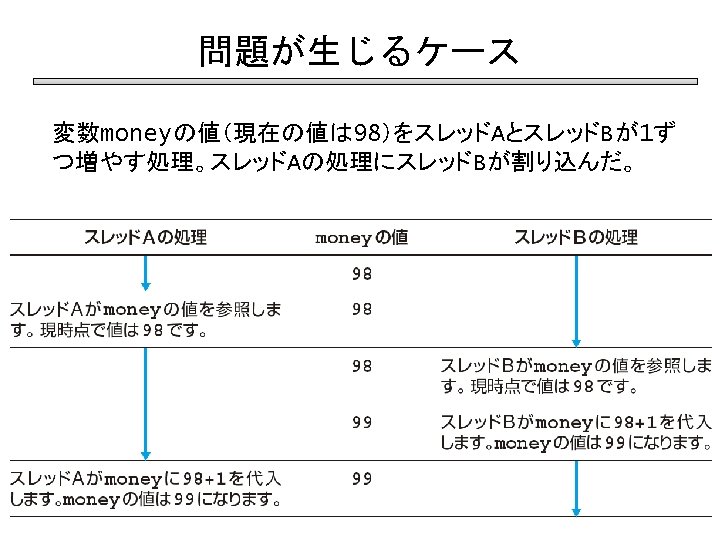
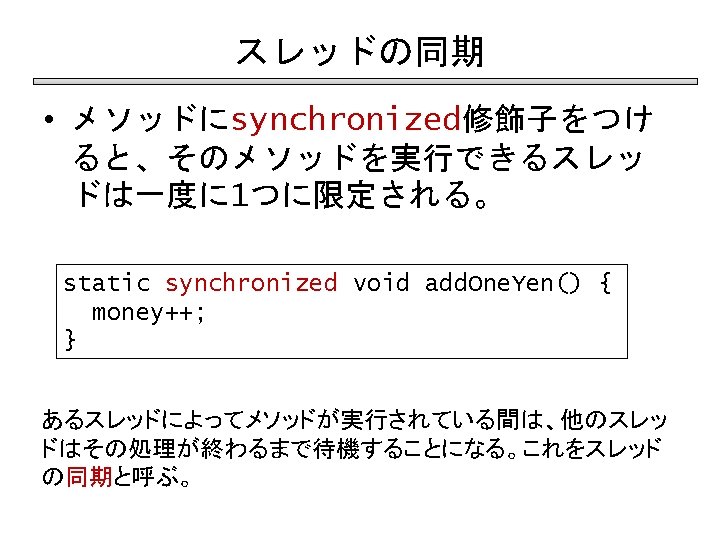
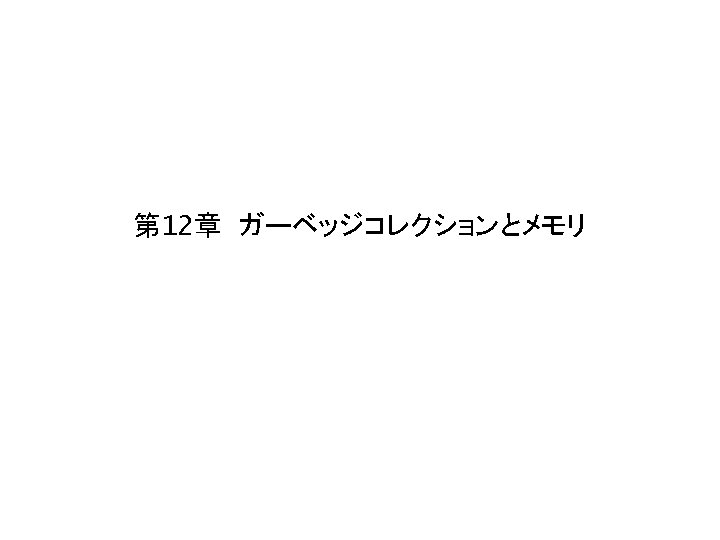
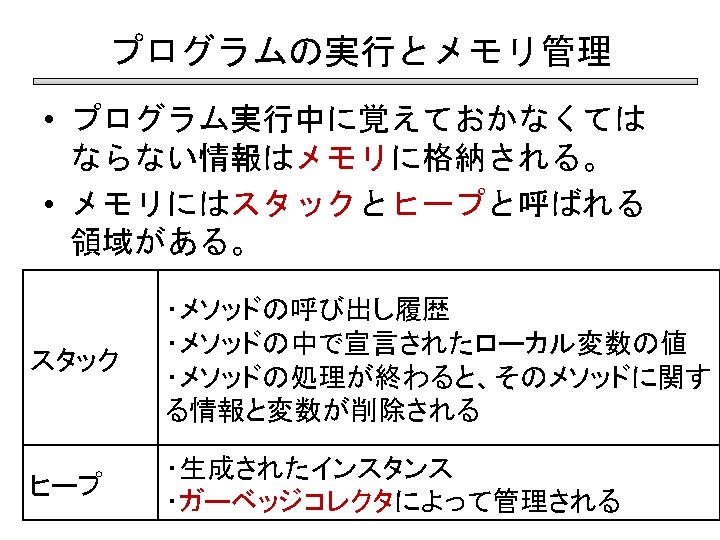
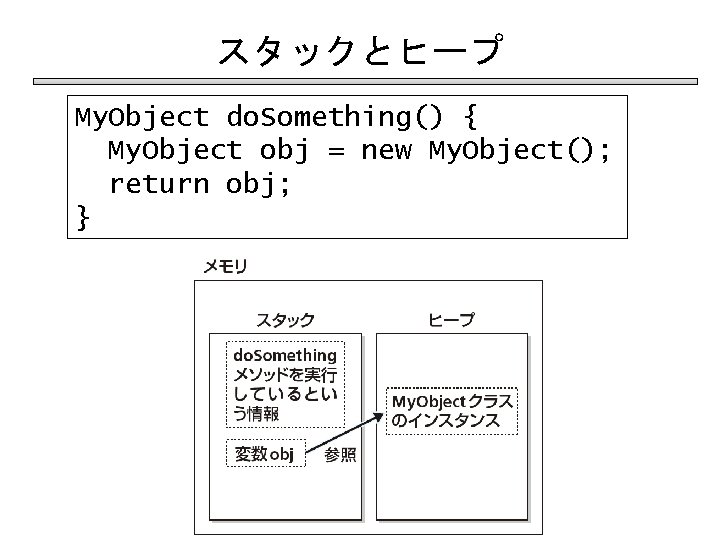
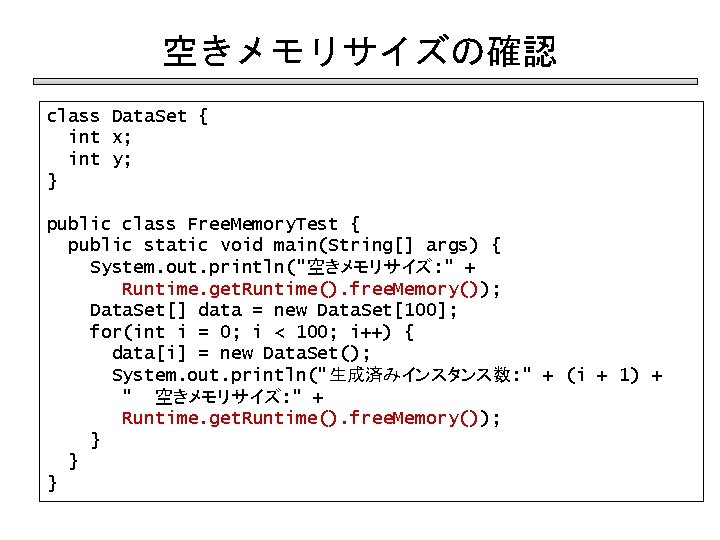
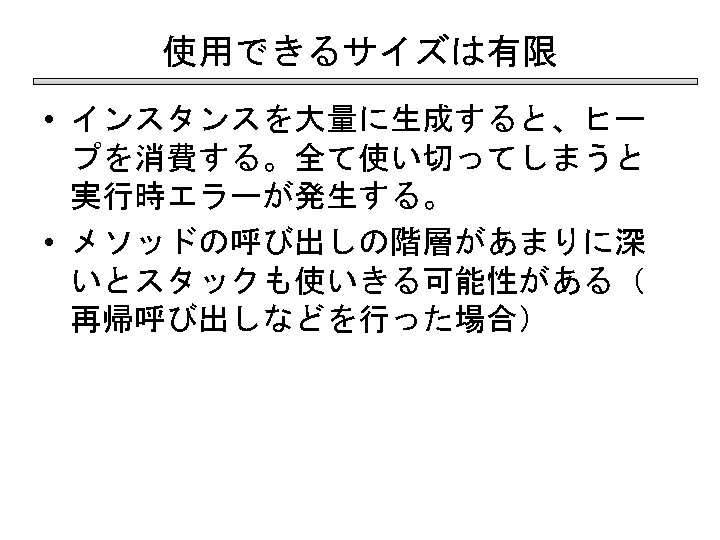
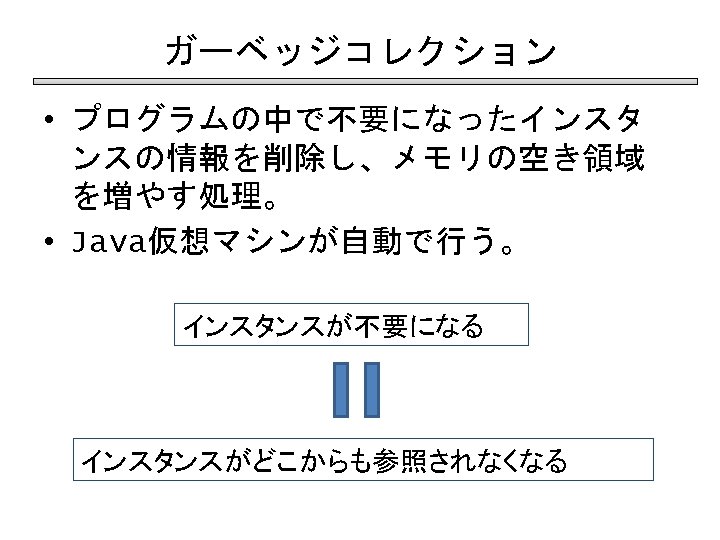
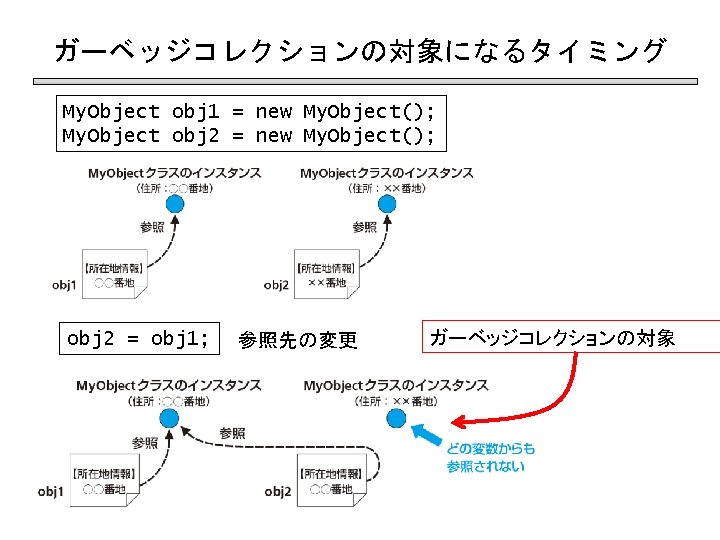
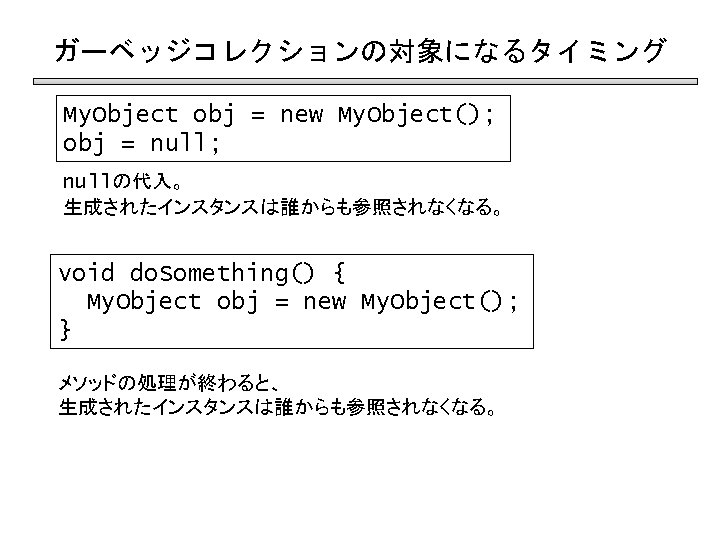
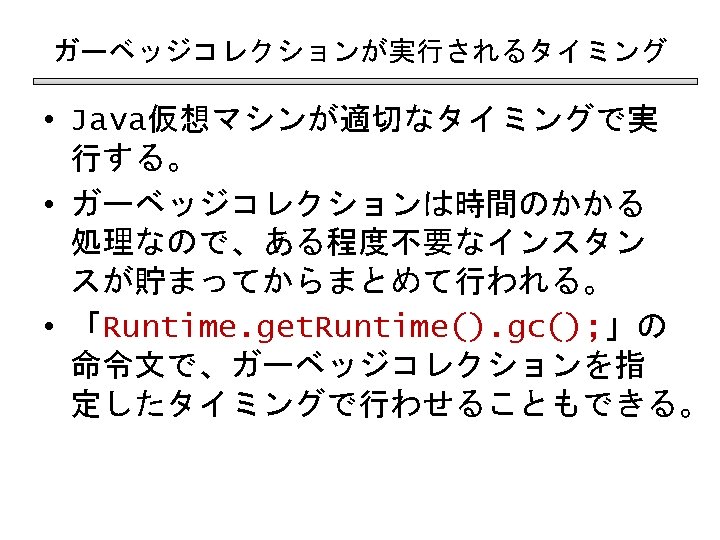
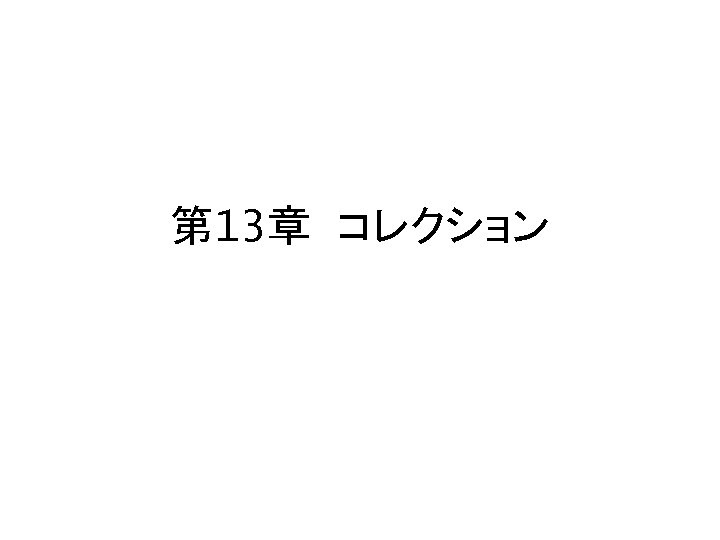
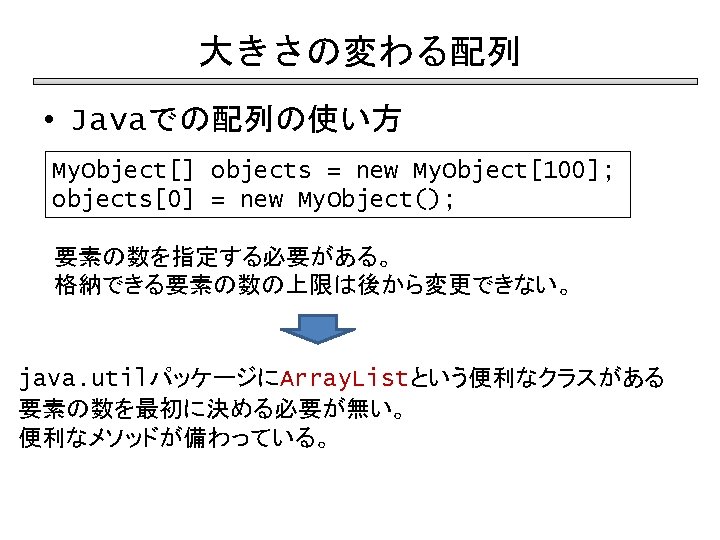
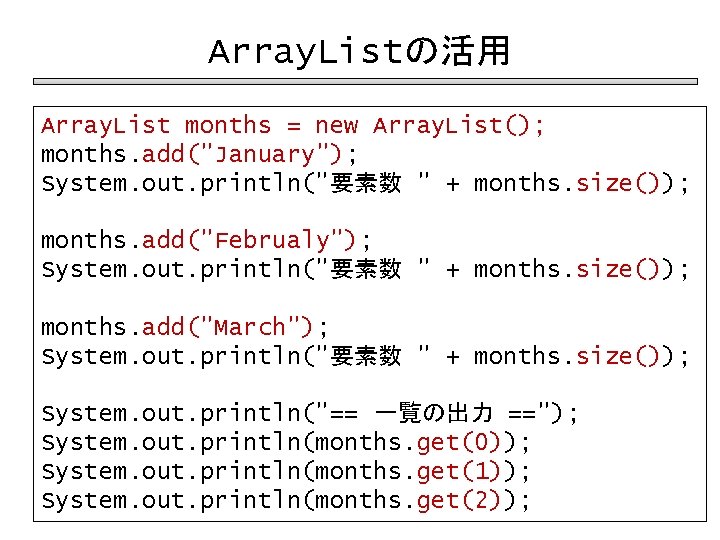
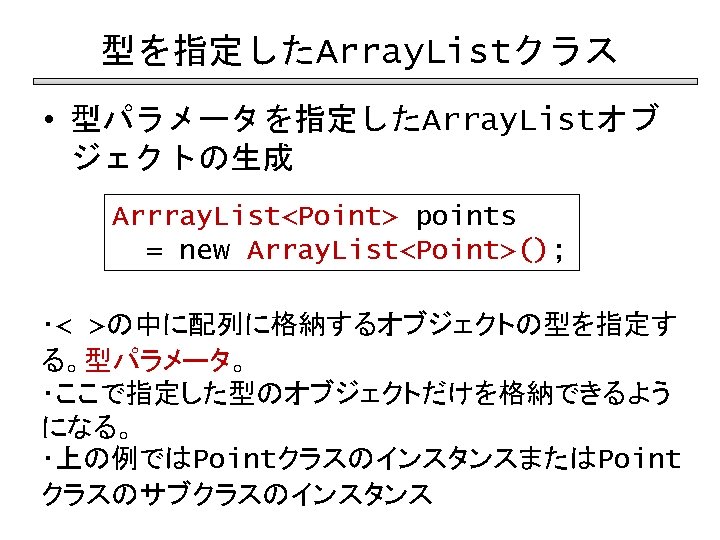
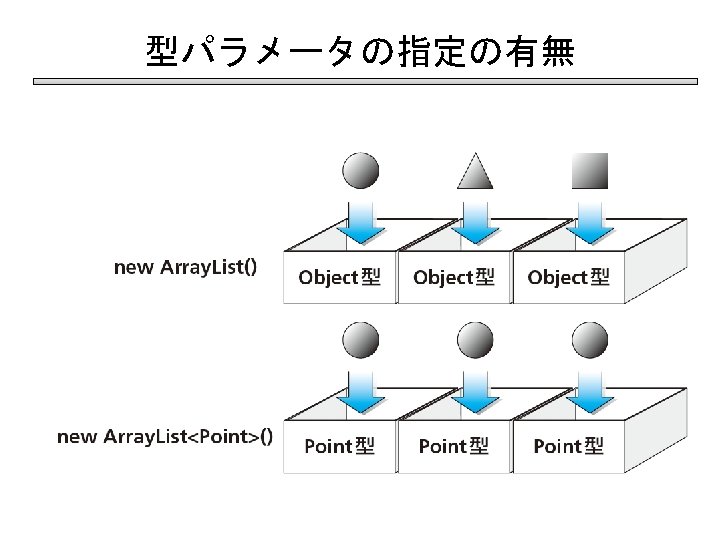
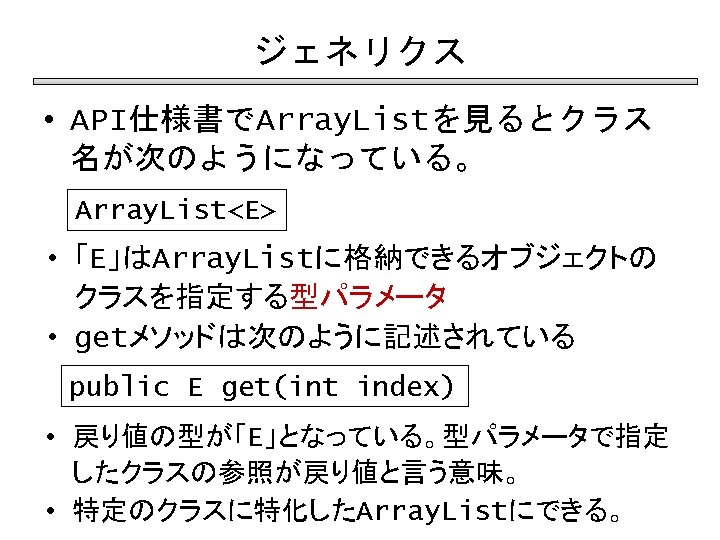
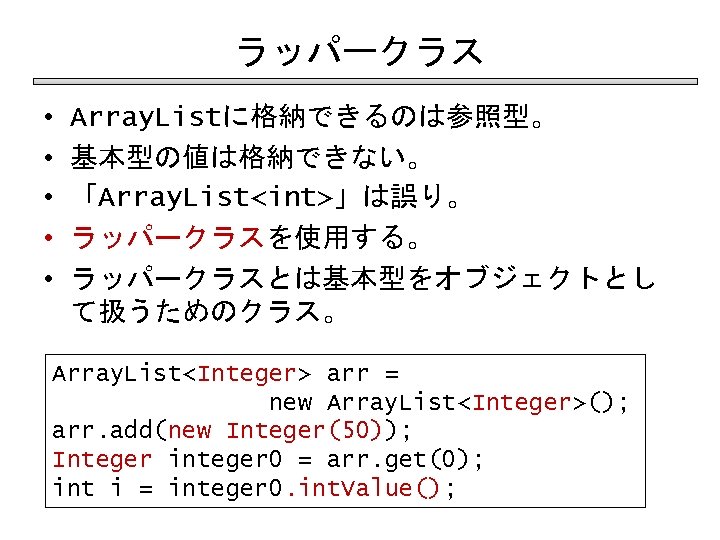
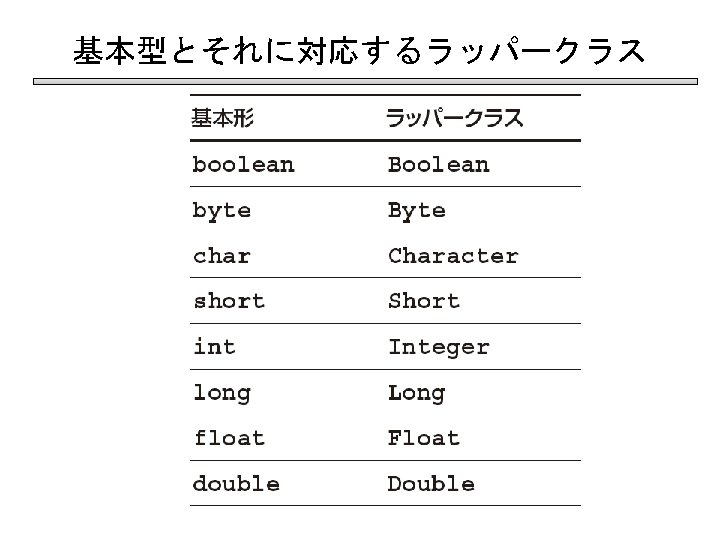
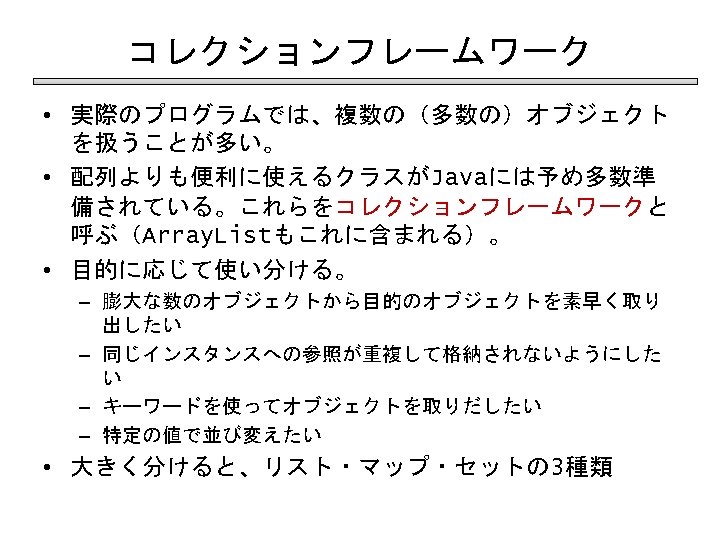
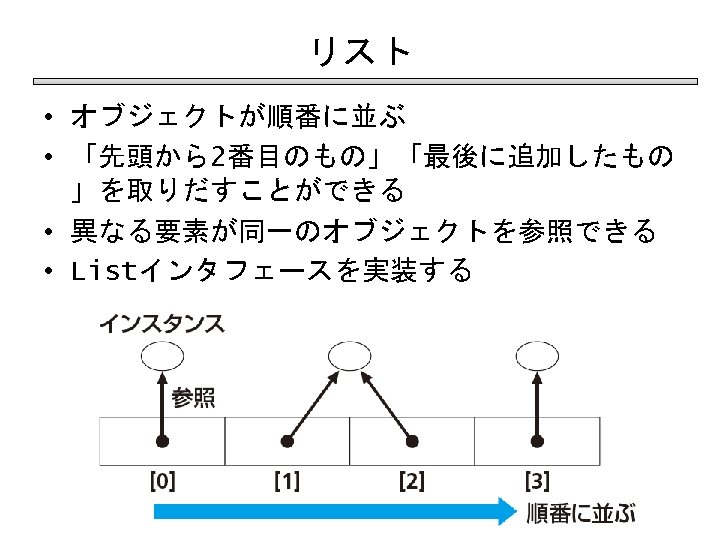
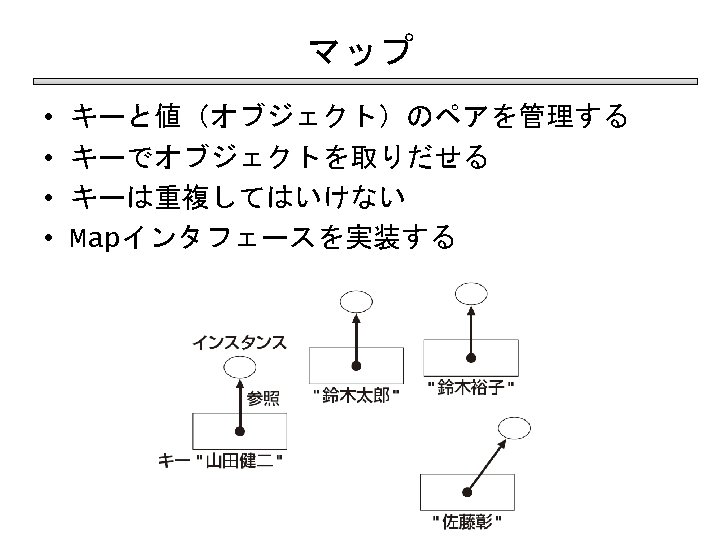
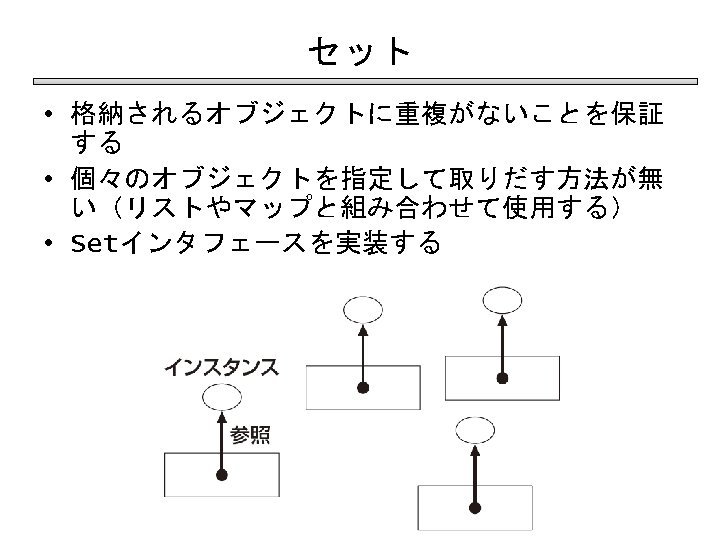
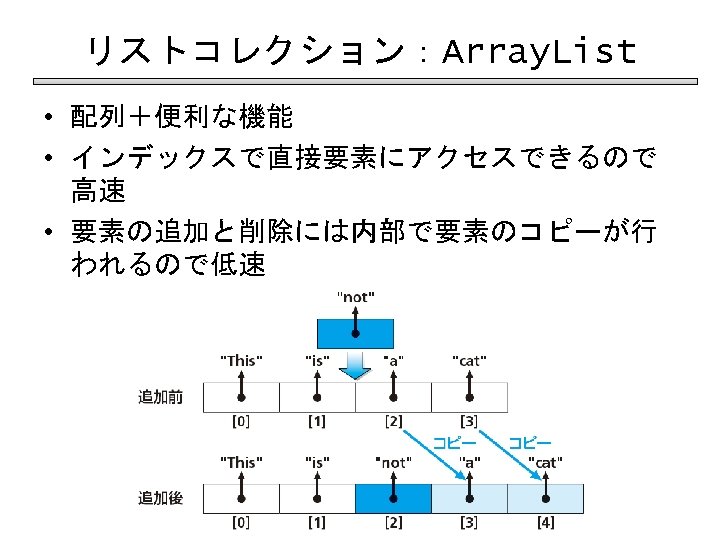
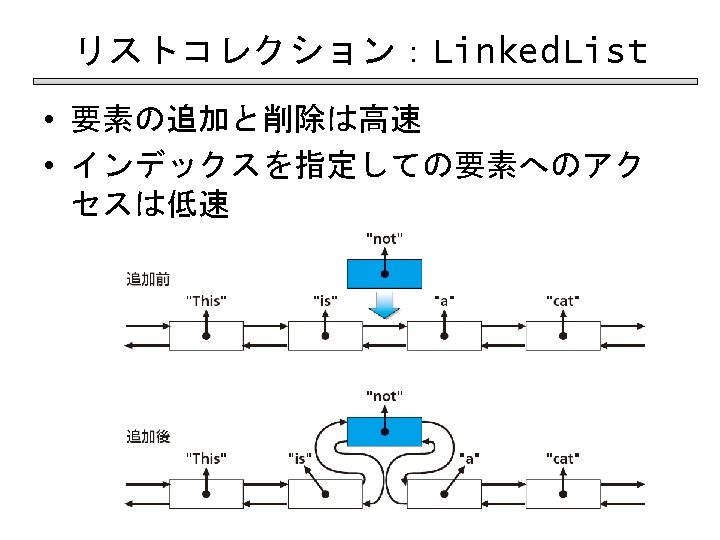
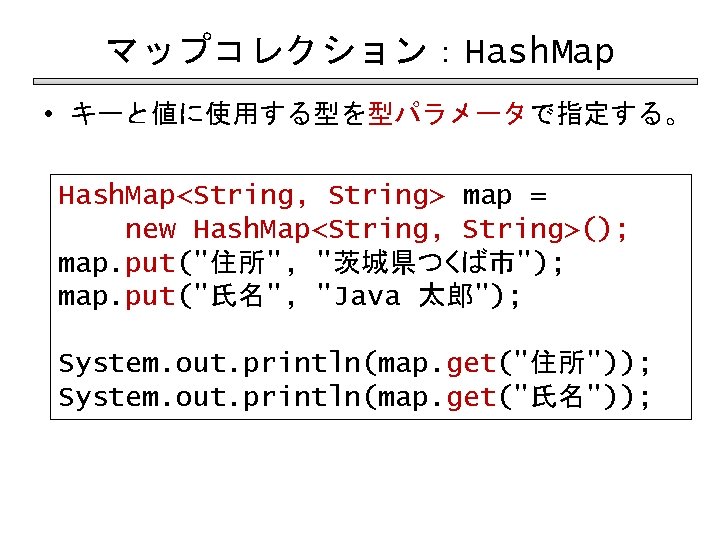
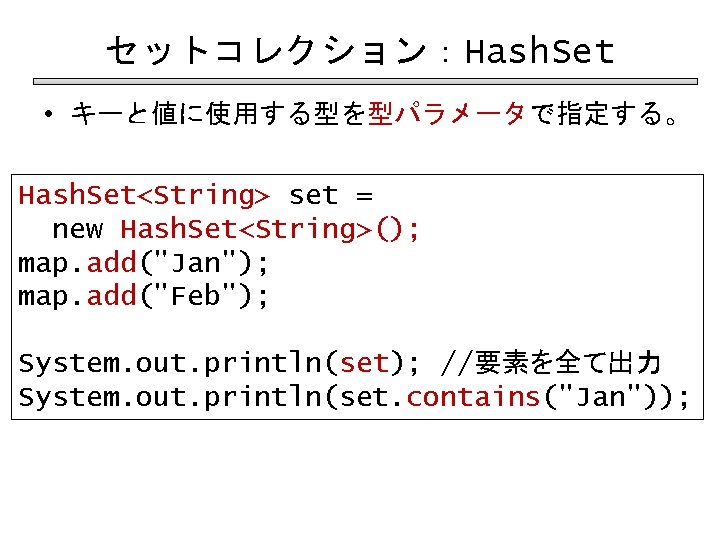
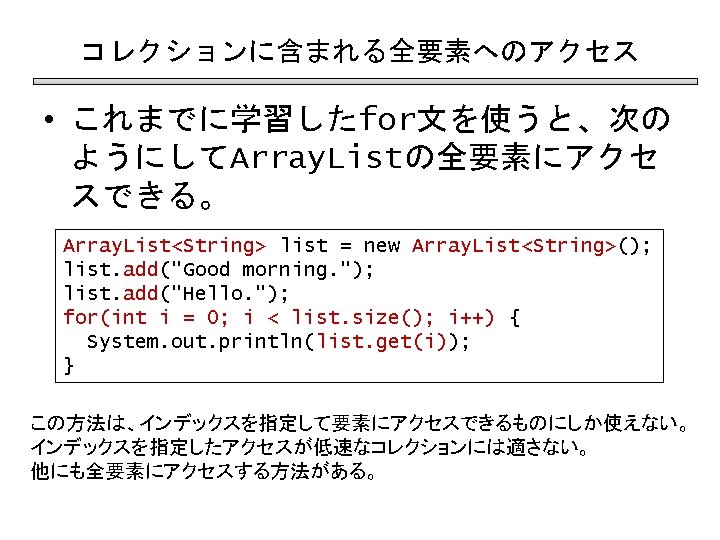
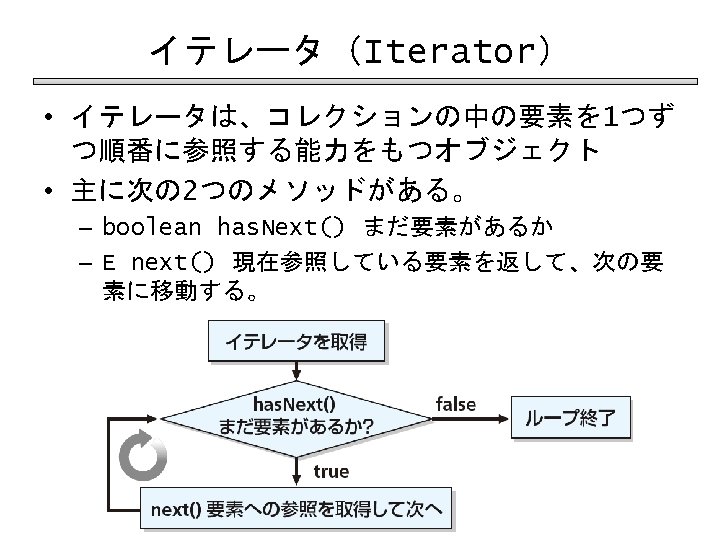
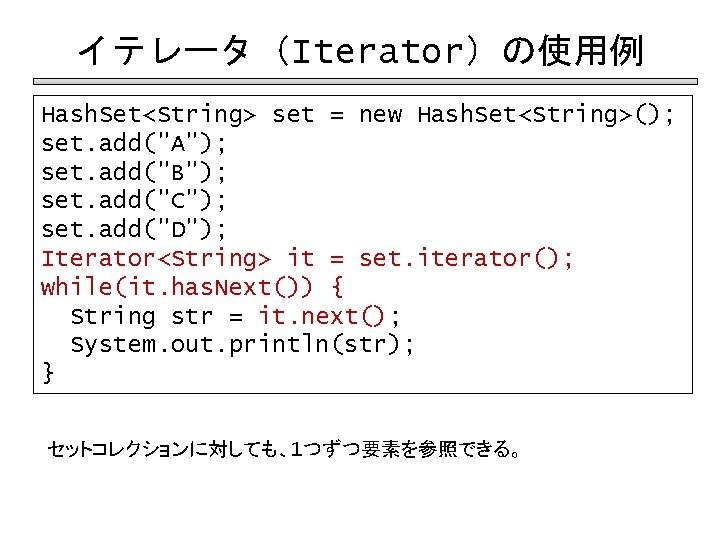
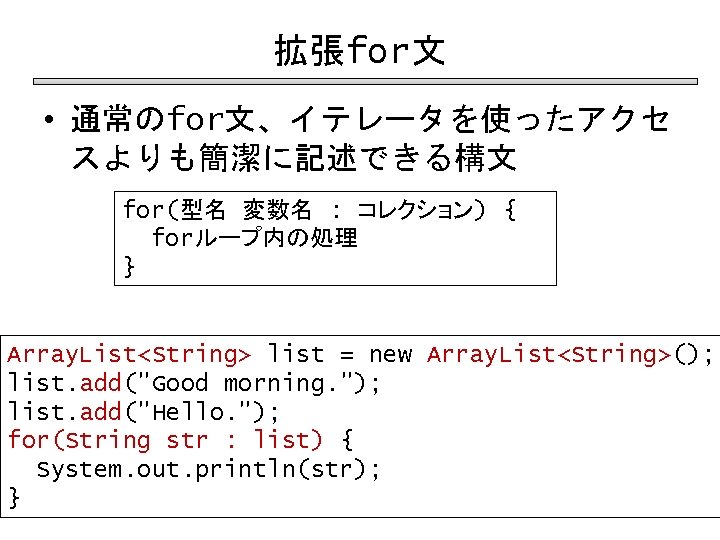
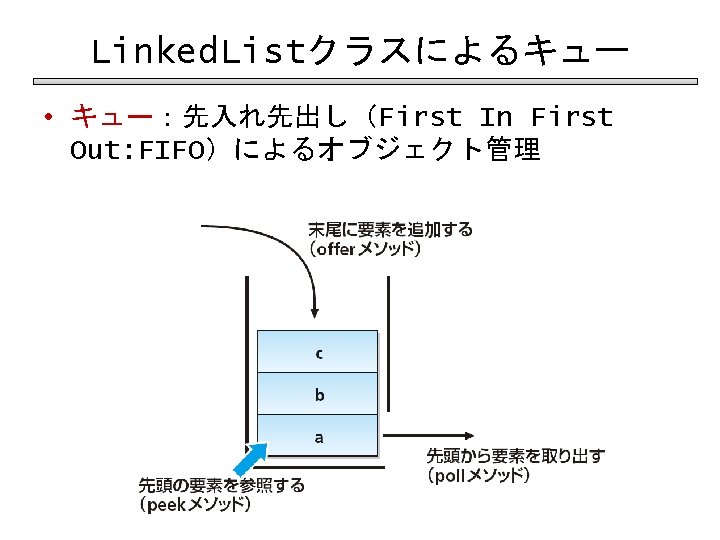
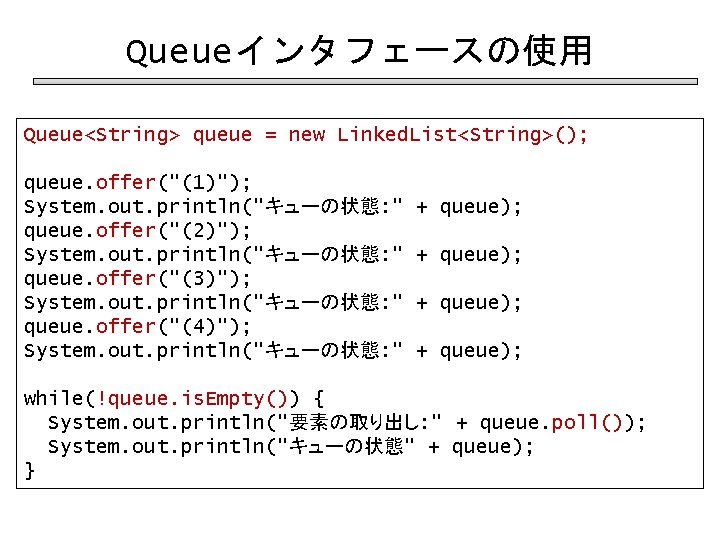
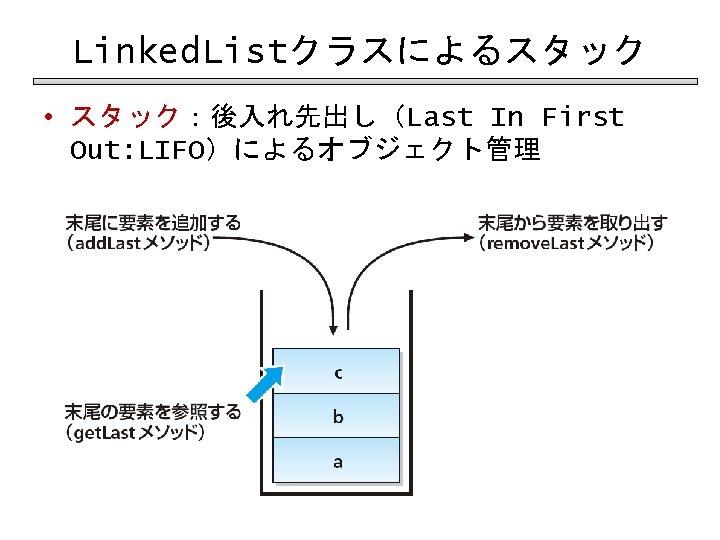
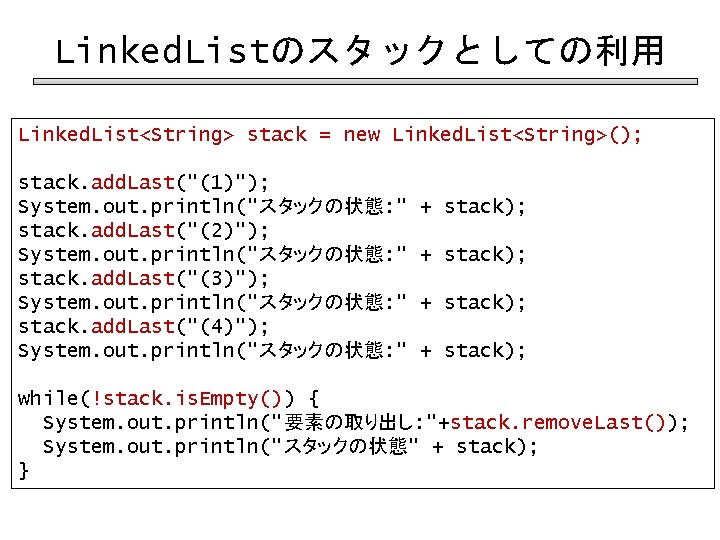
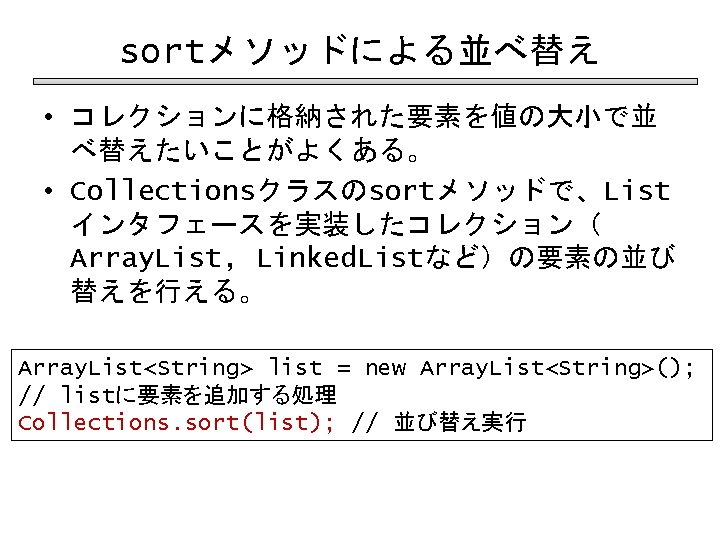
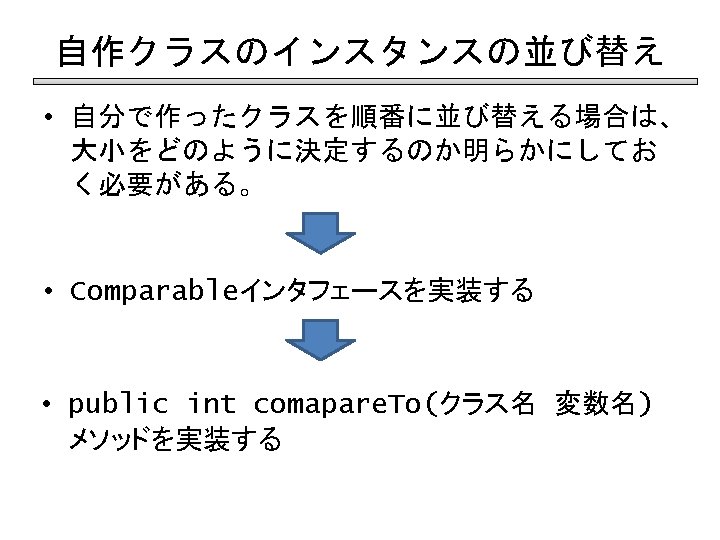
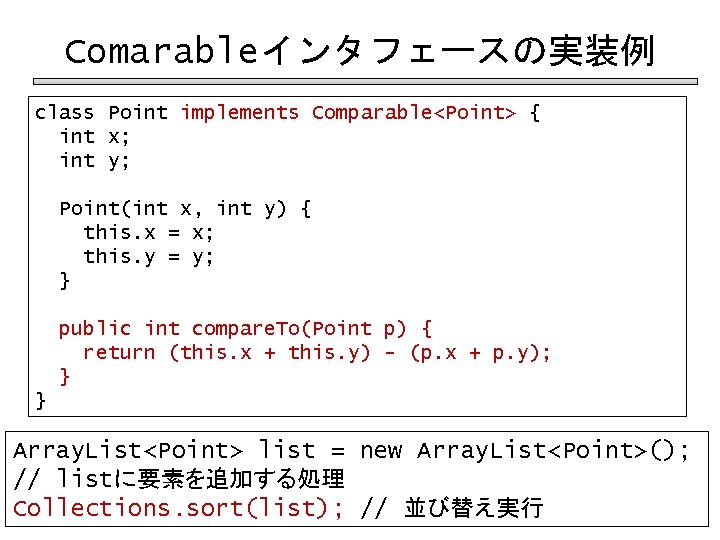
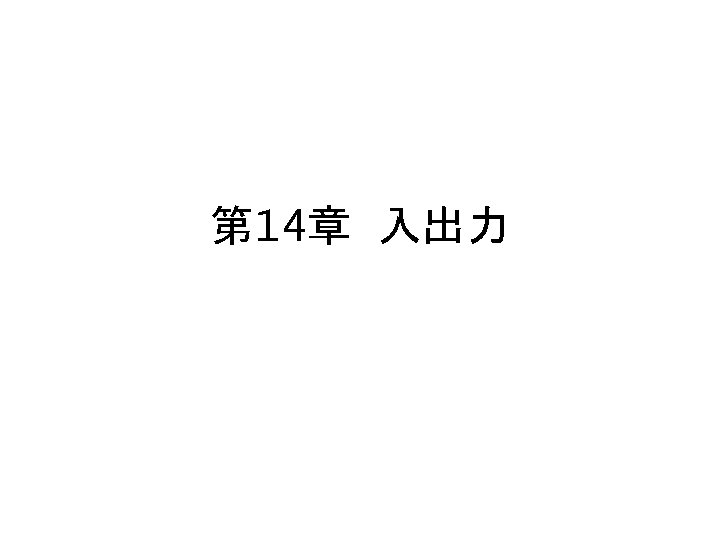
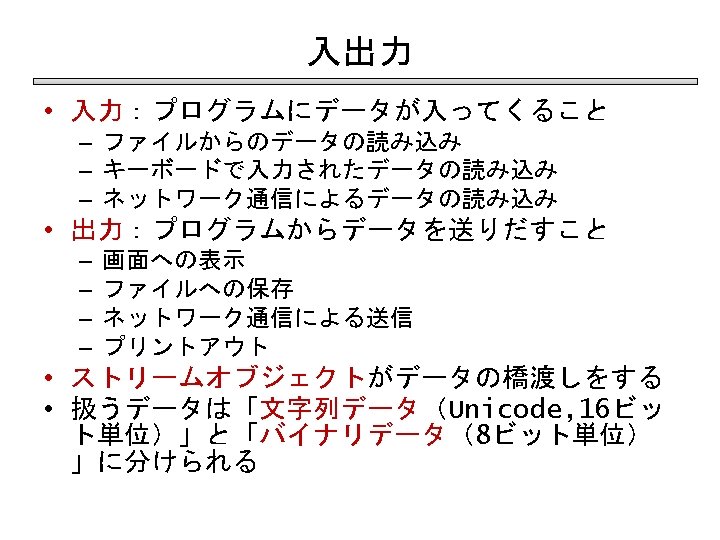
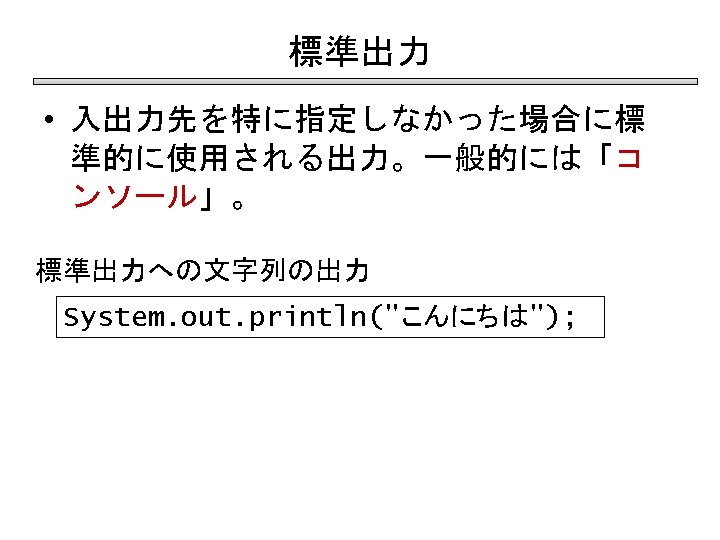
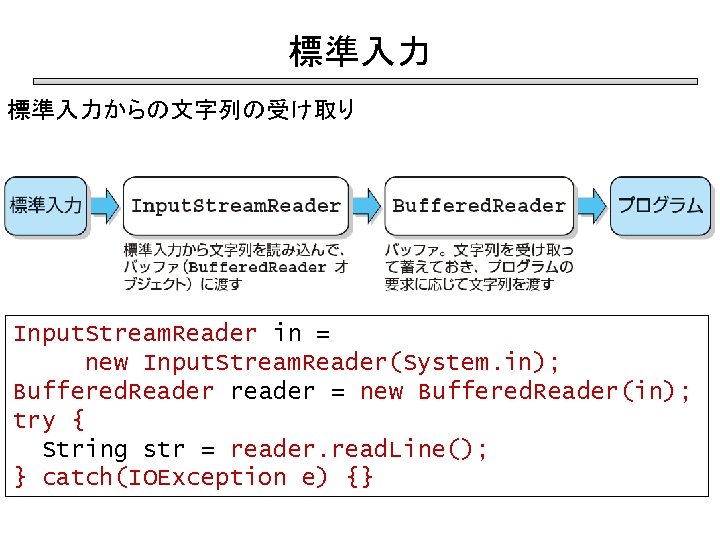
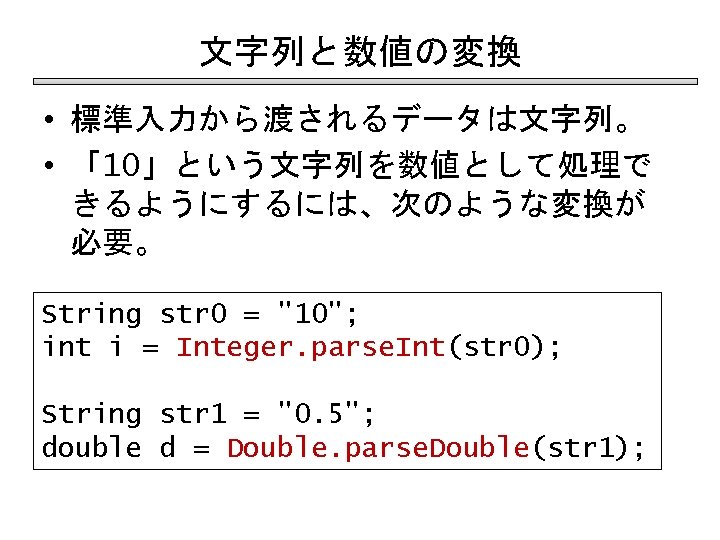
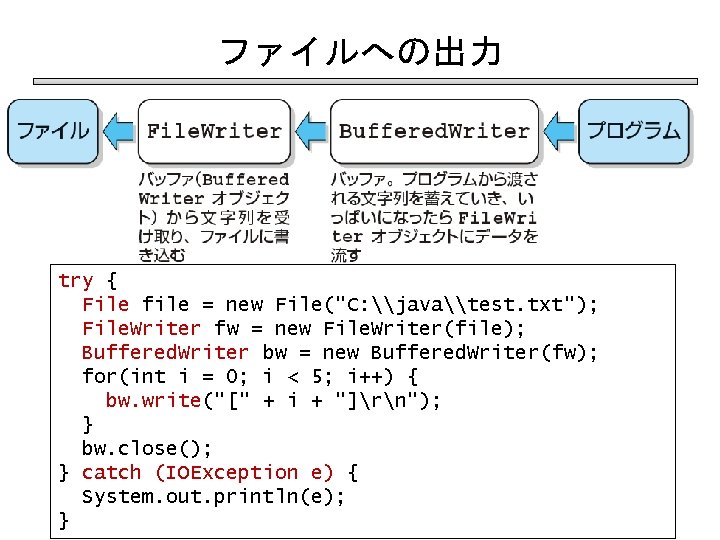
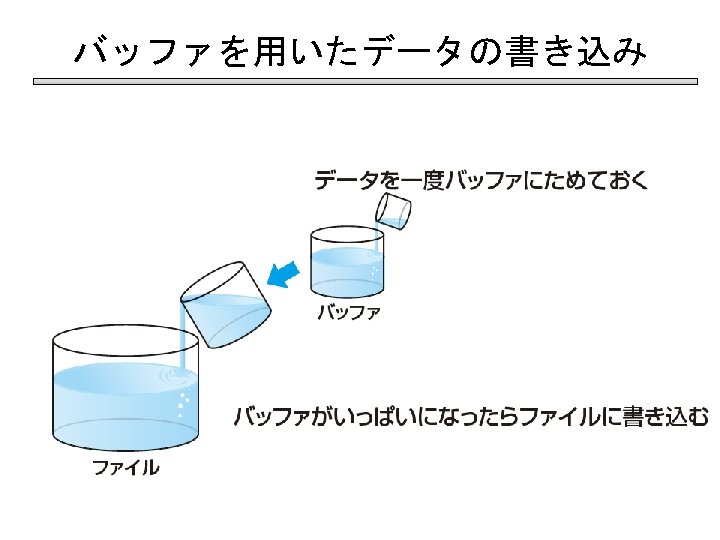
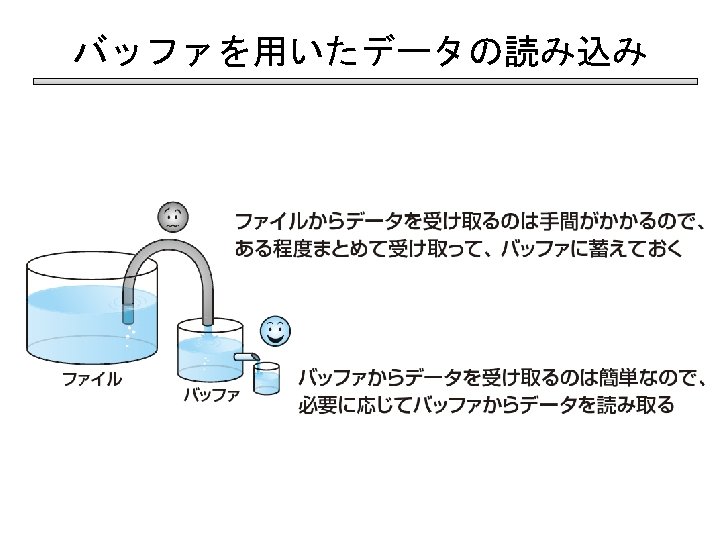
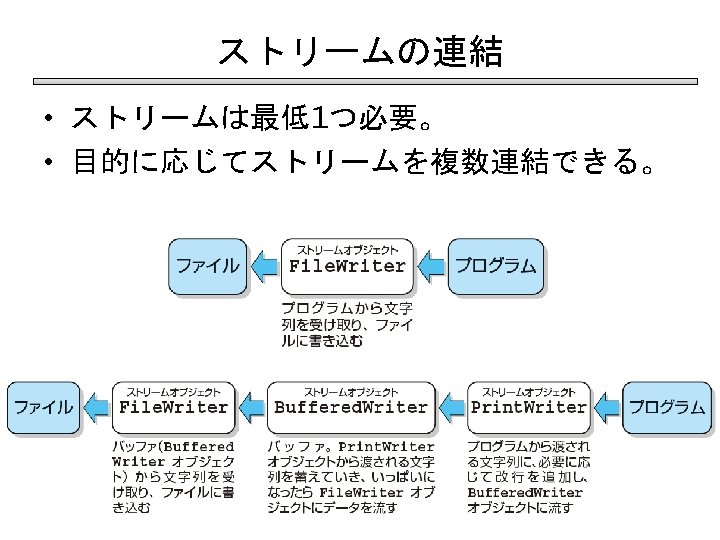
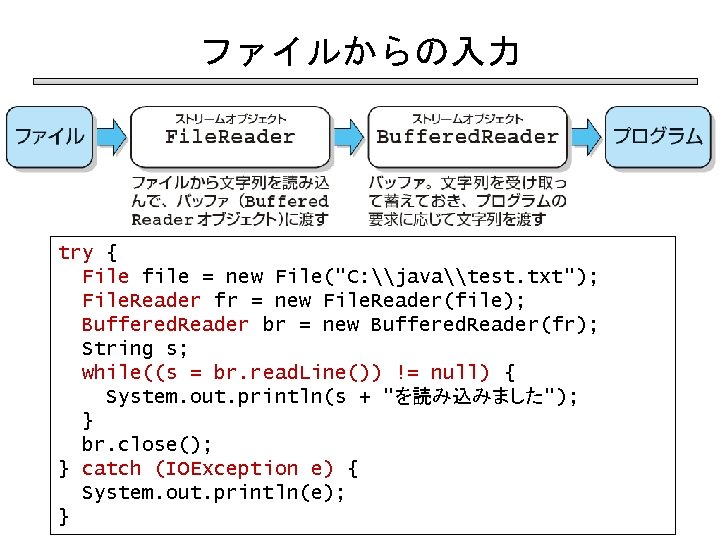
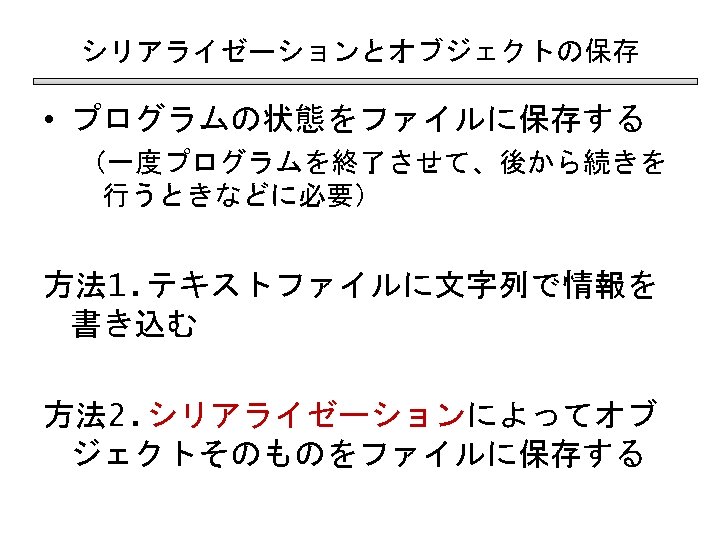
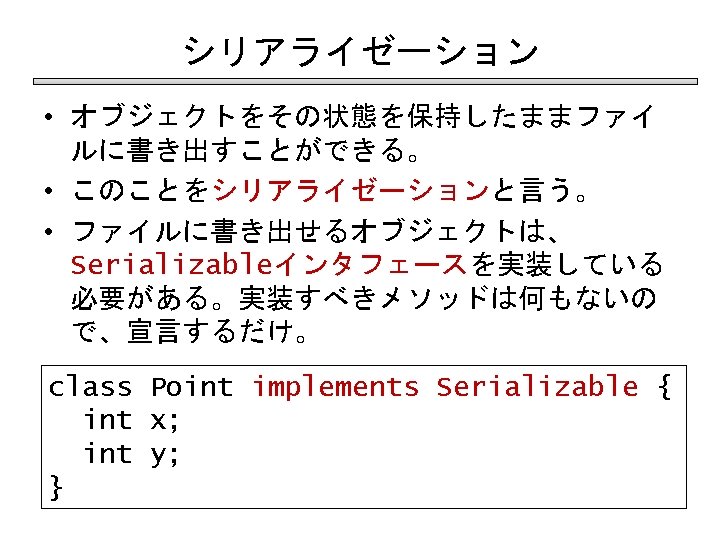
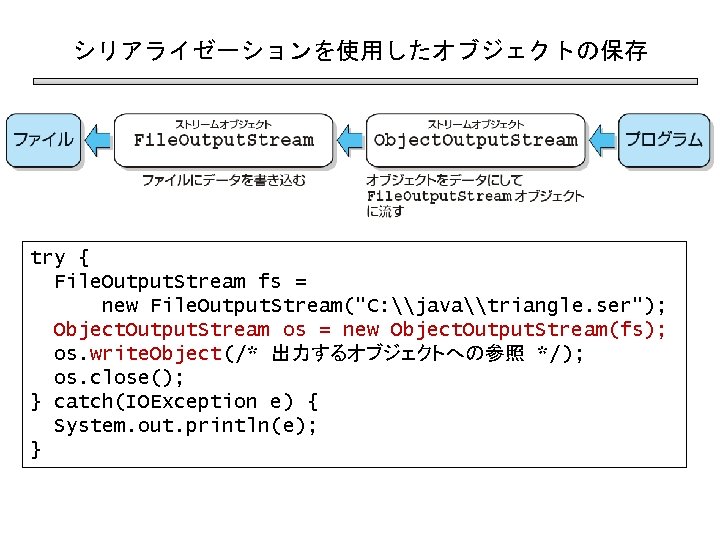
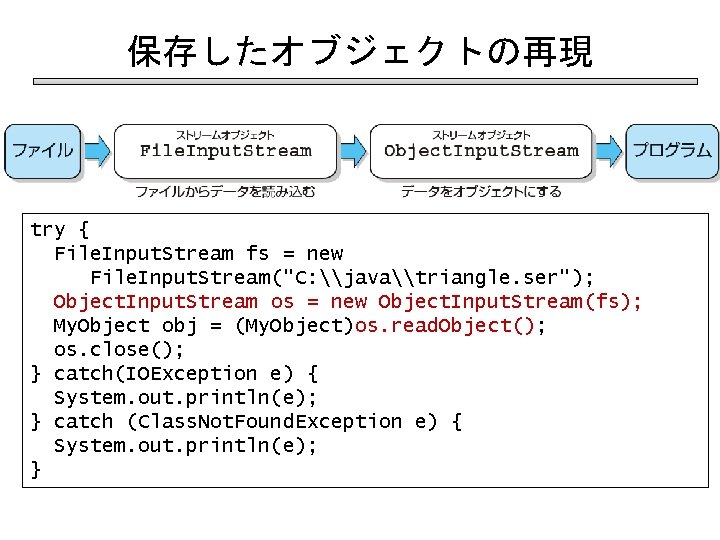
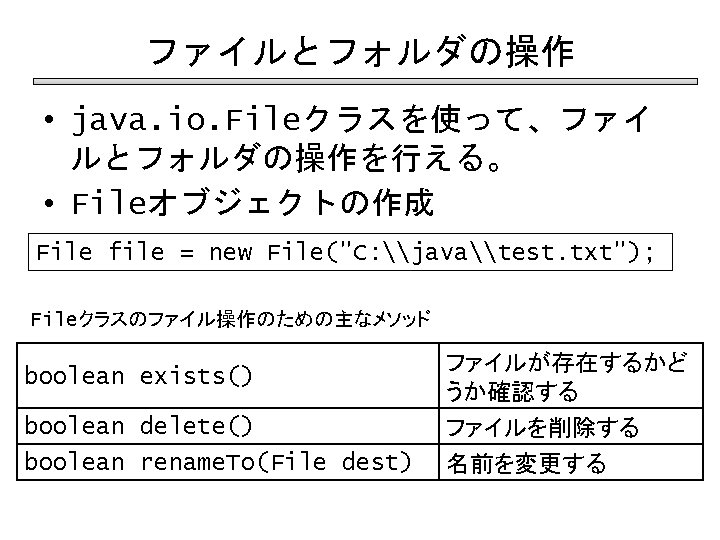
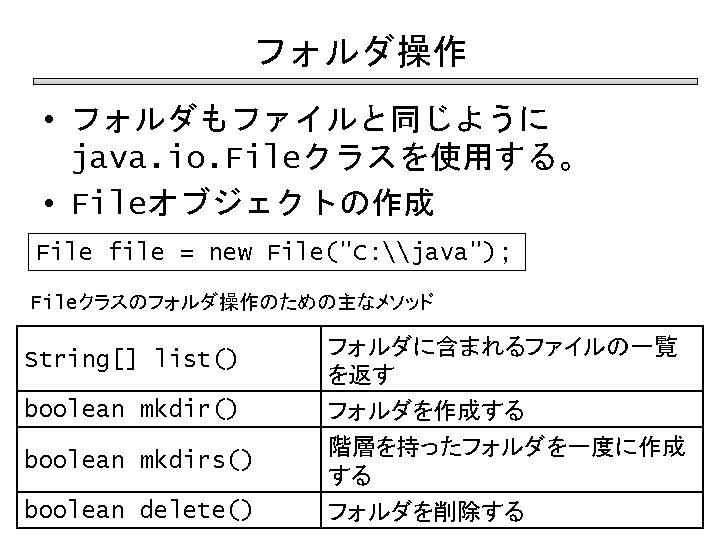
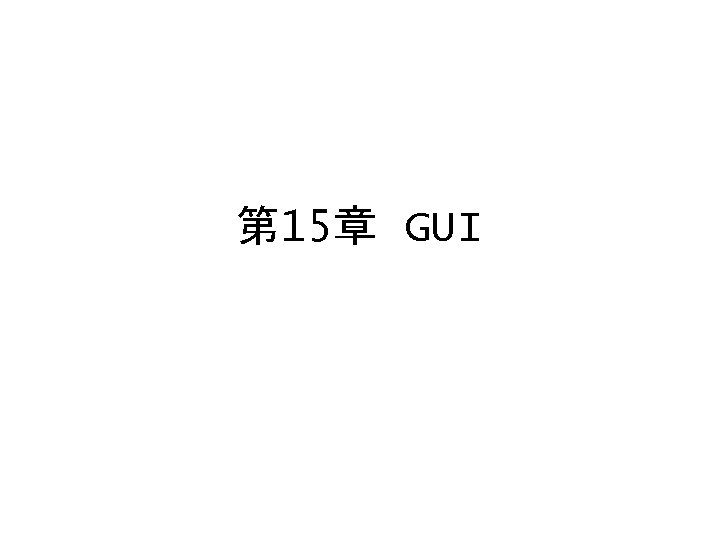
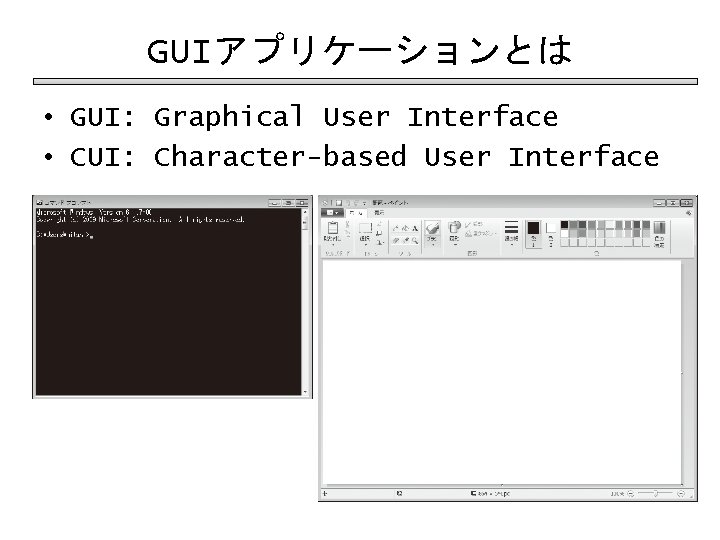
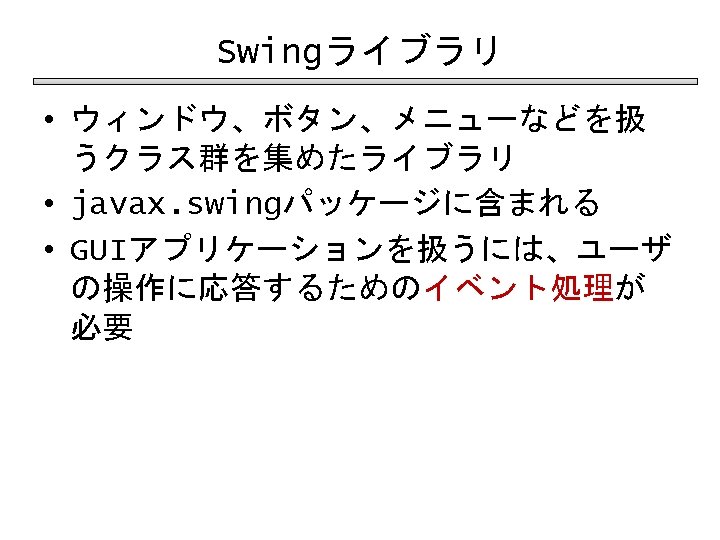
![フレームの作成 import javax. swing. *; class Simple. Frame. Example { public static void main(String[] フレームの作成 import javax. swing. *; class Simple. Frame. Example { public static void main(String[]](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-116.jpg)
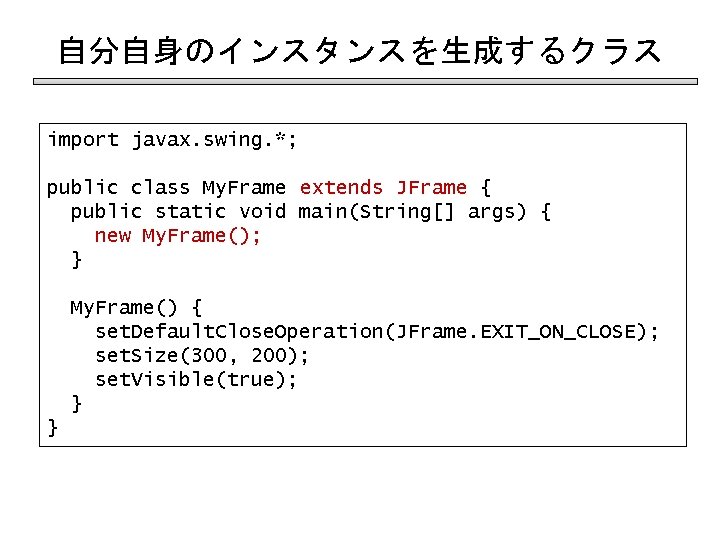
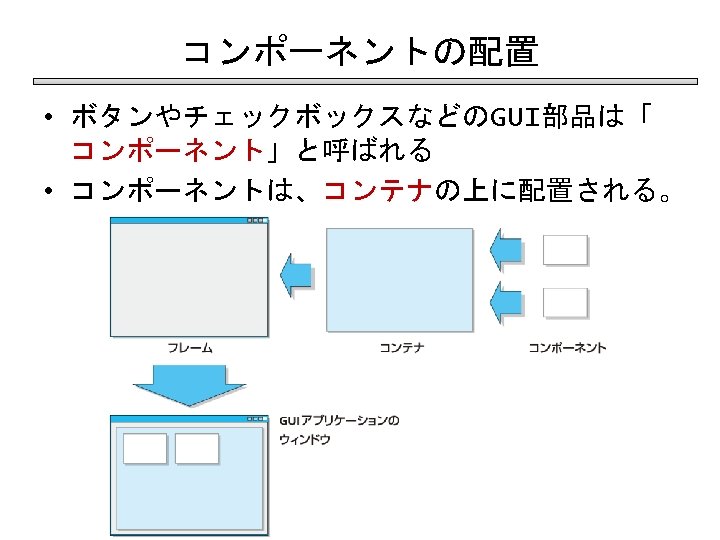
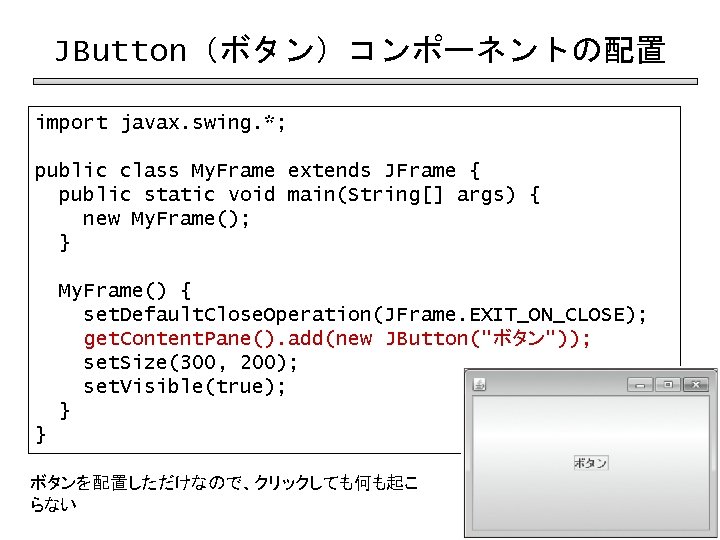
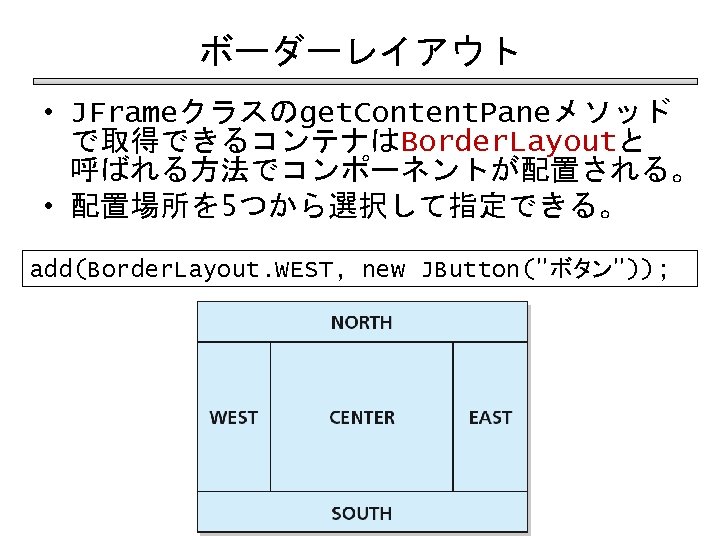
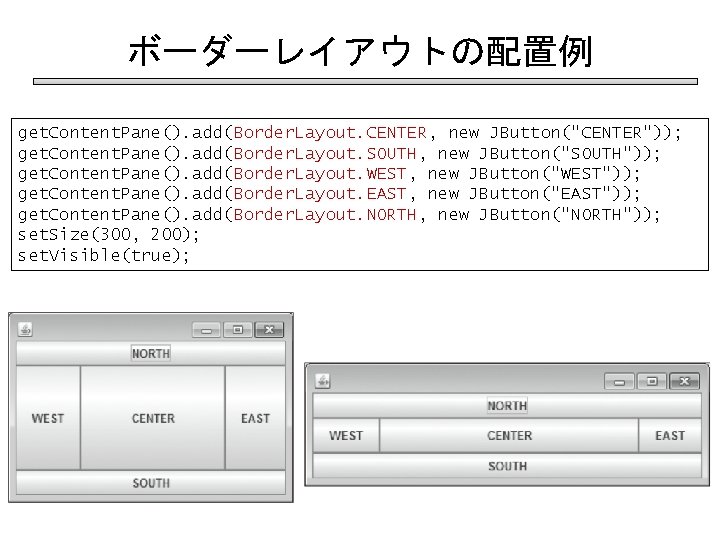
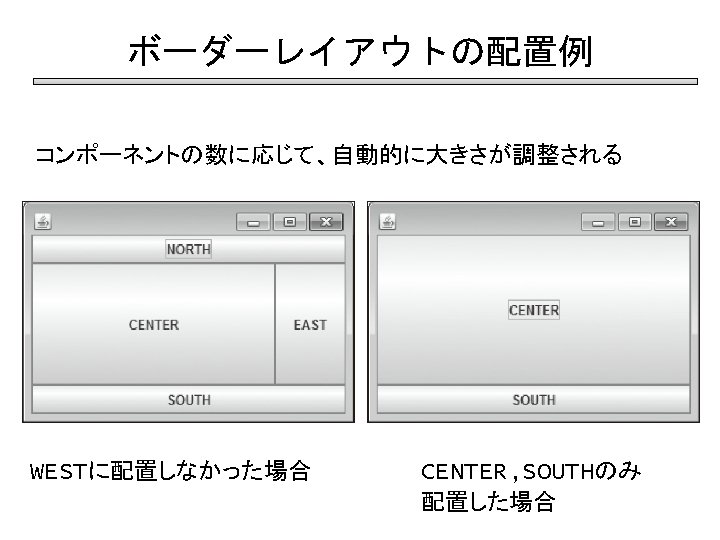
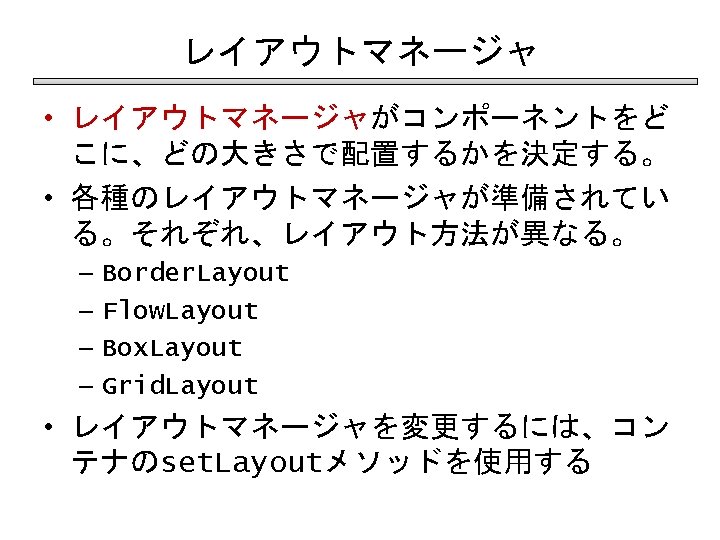
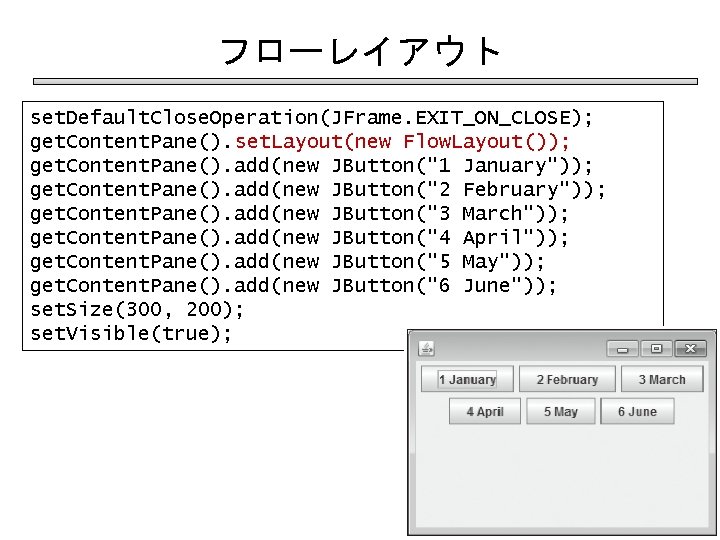
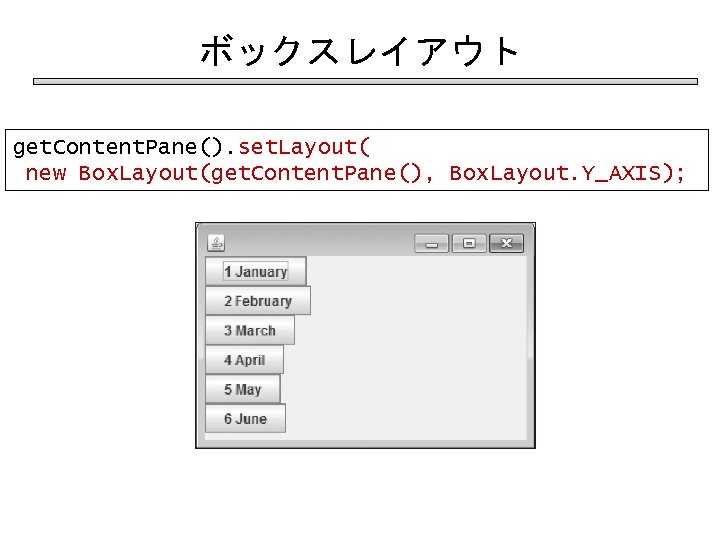
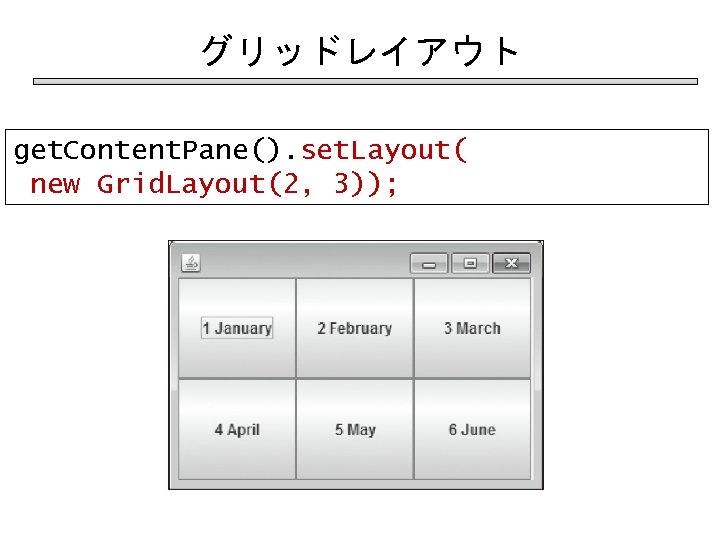
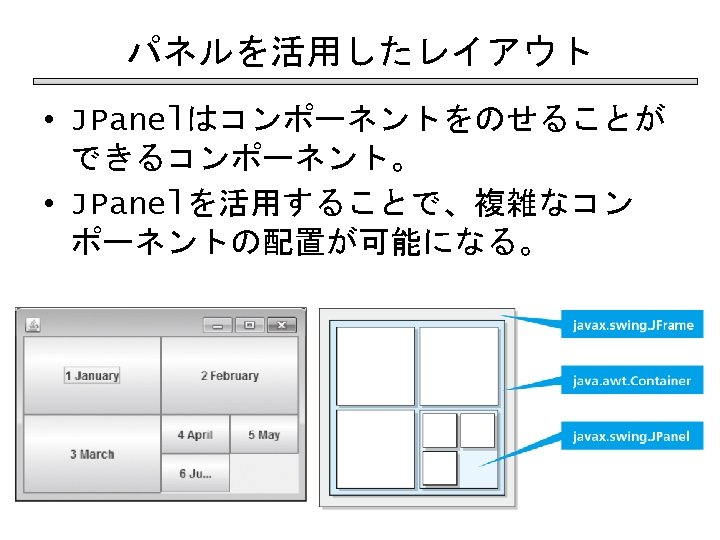
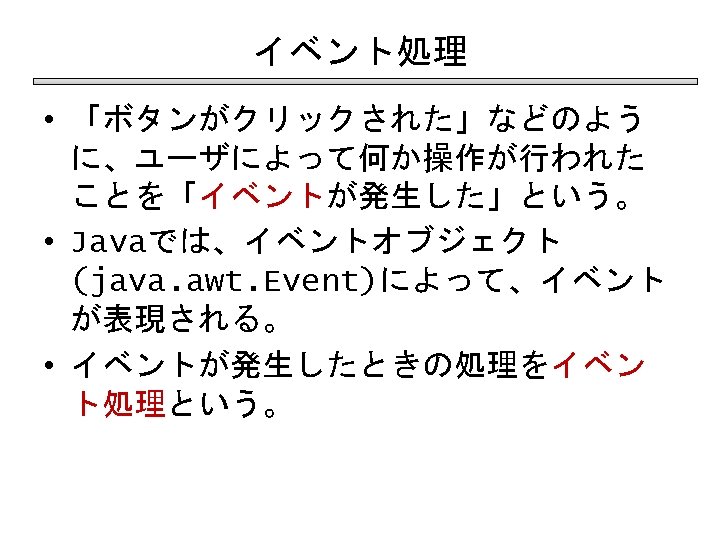
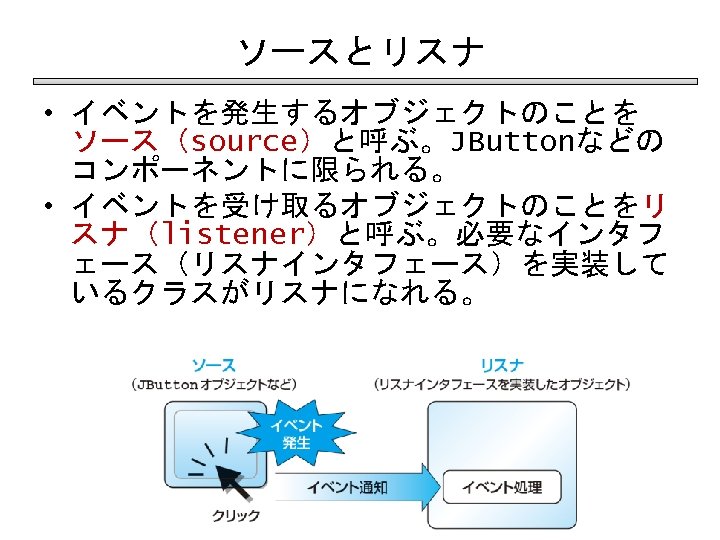
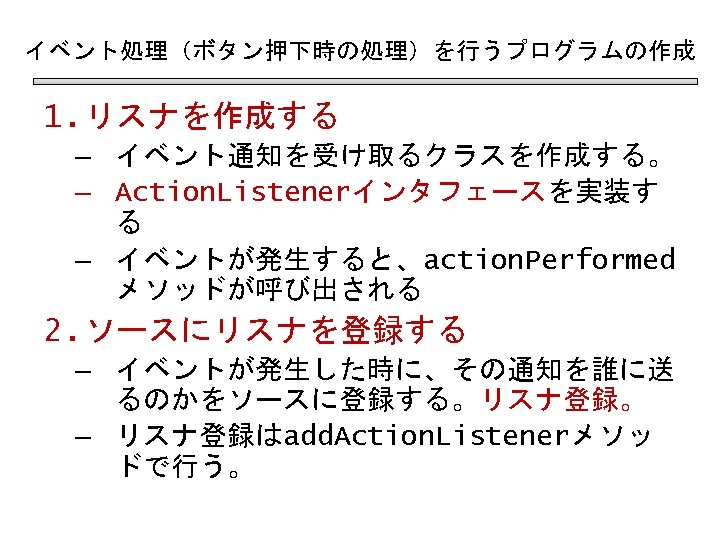
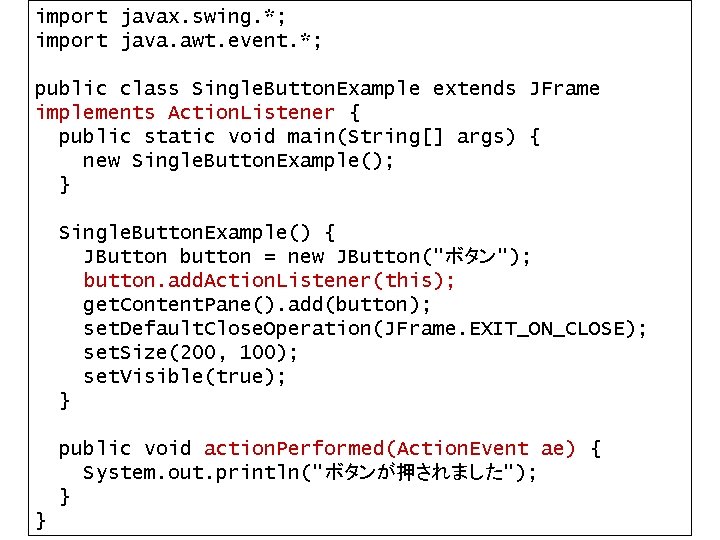
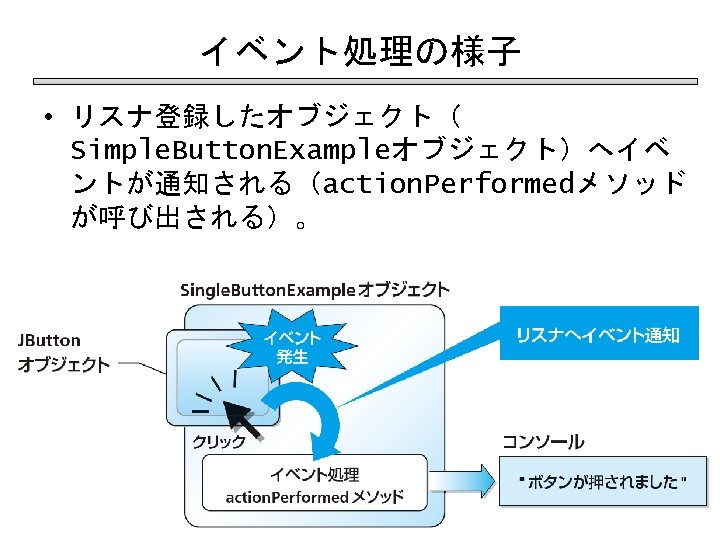
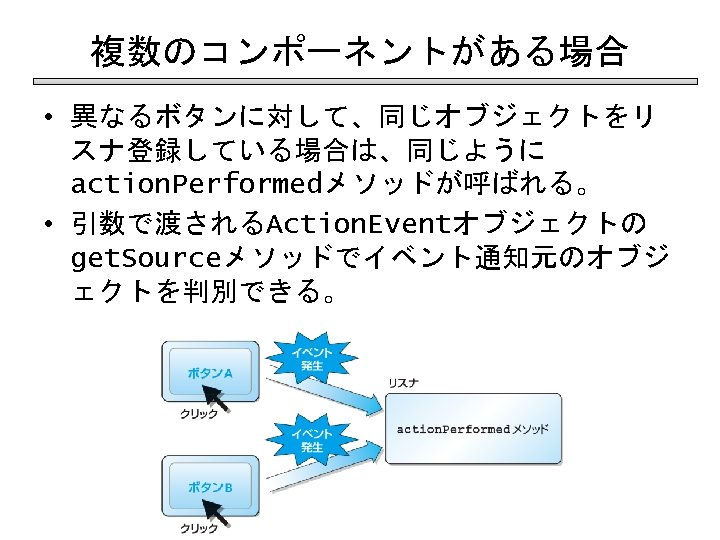
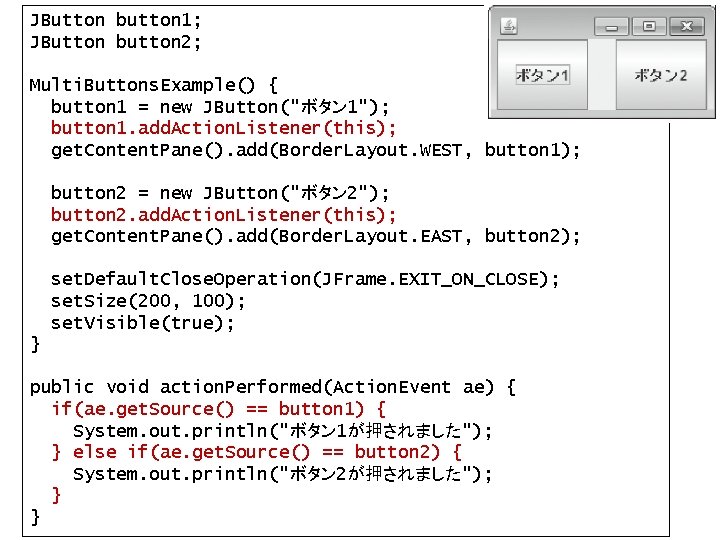
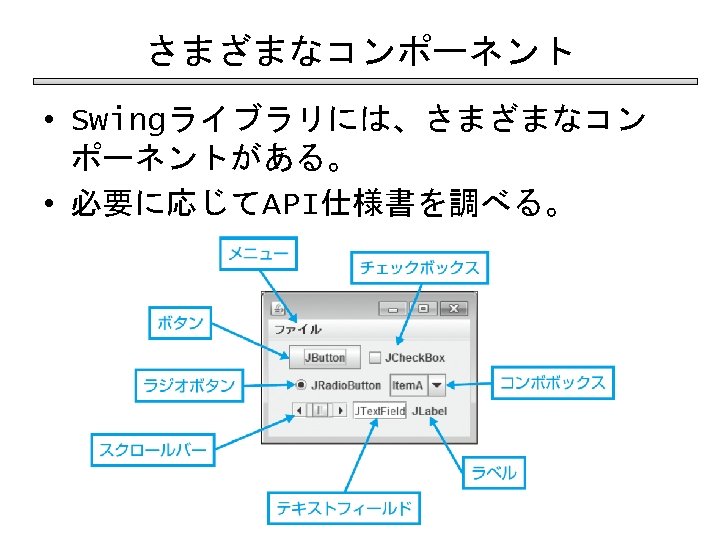
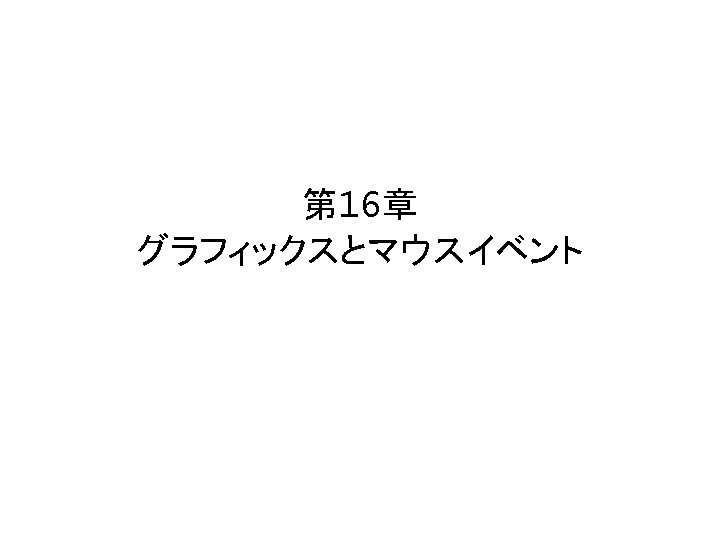
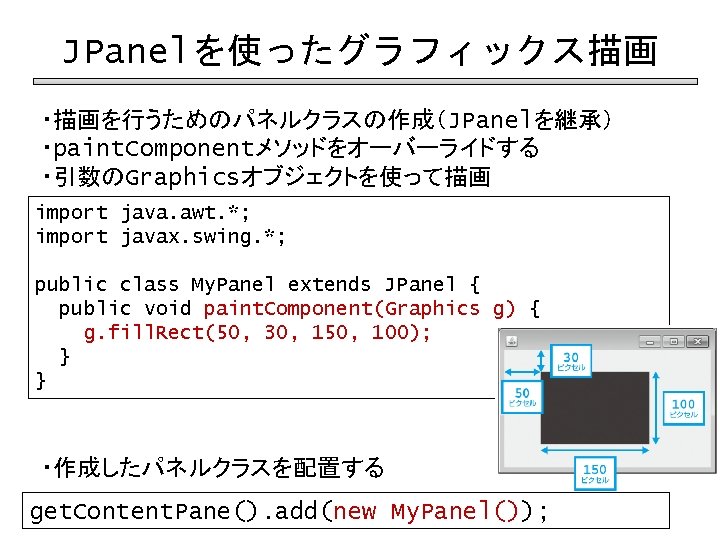
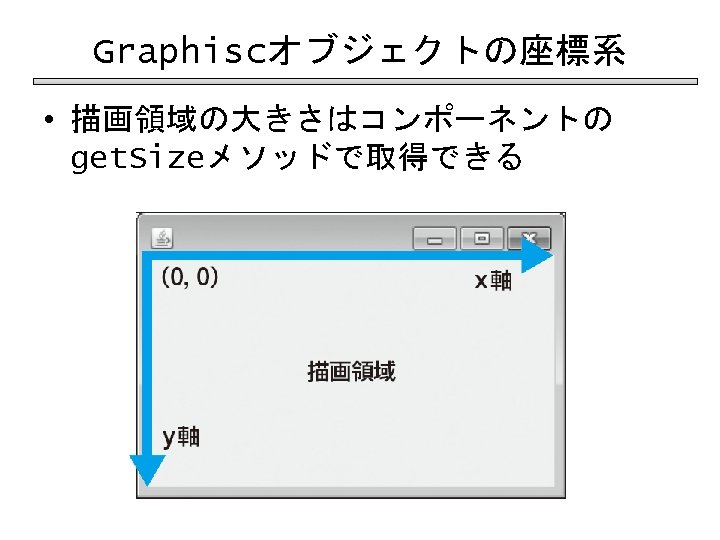
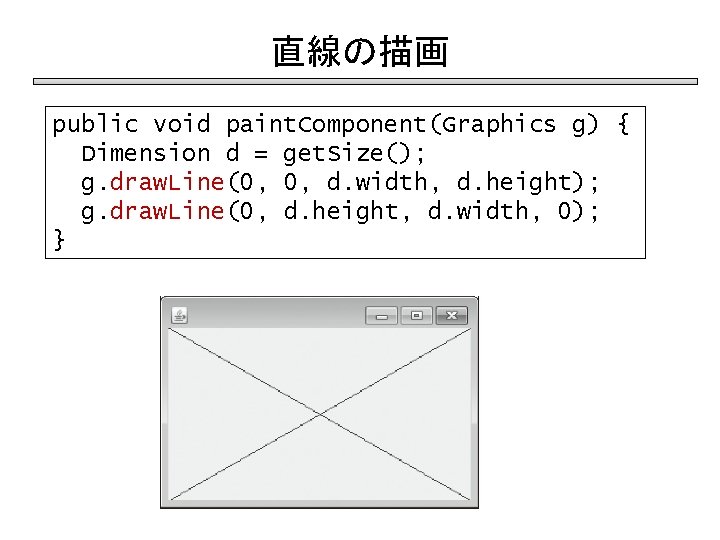
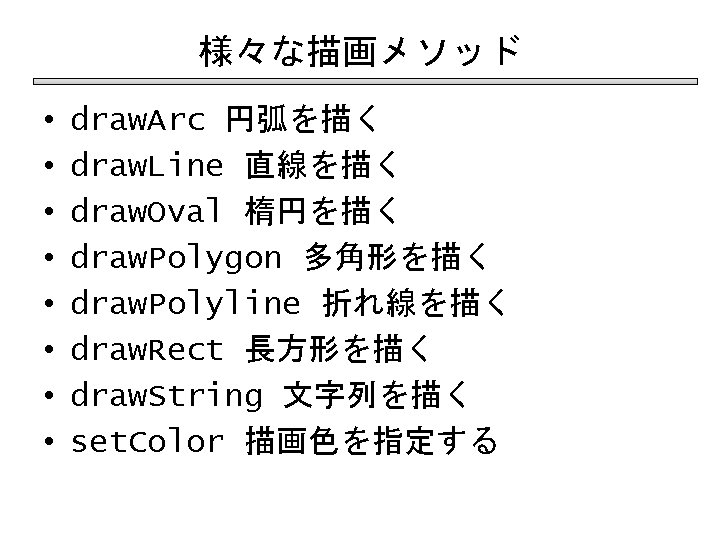
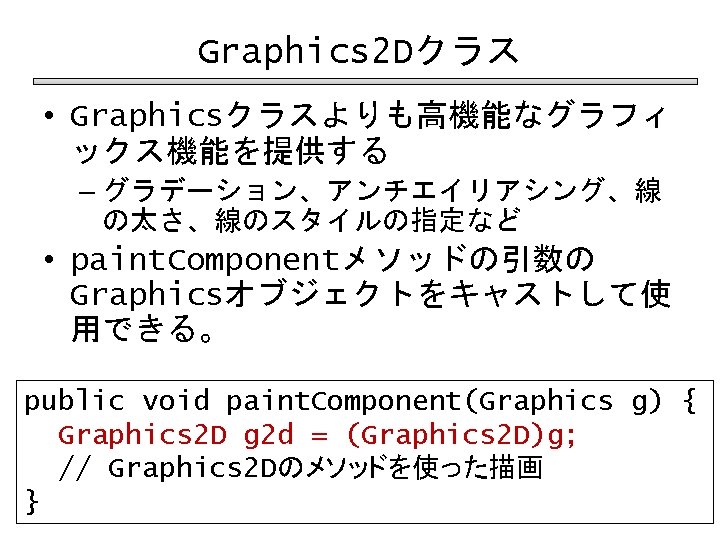
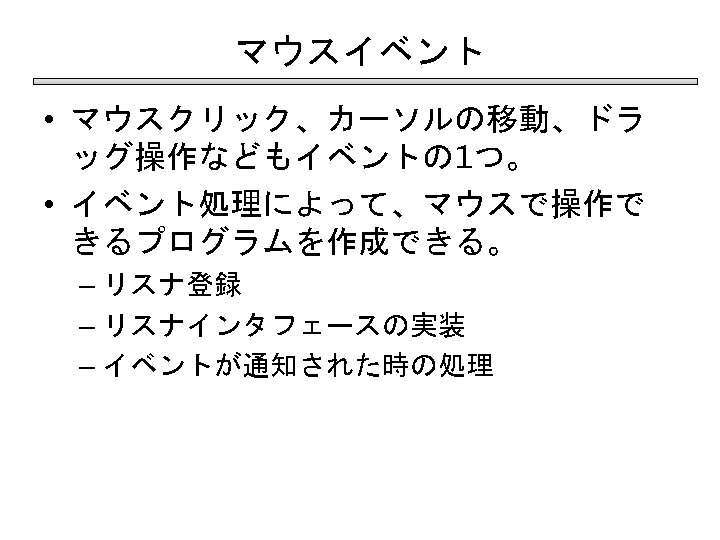
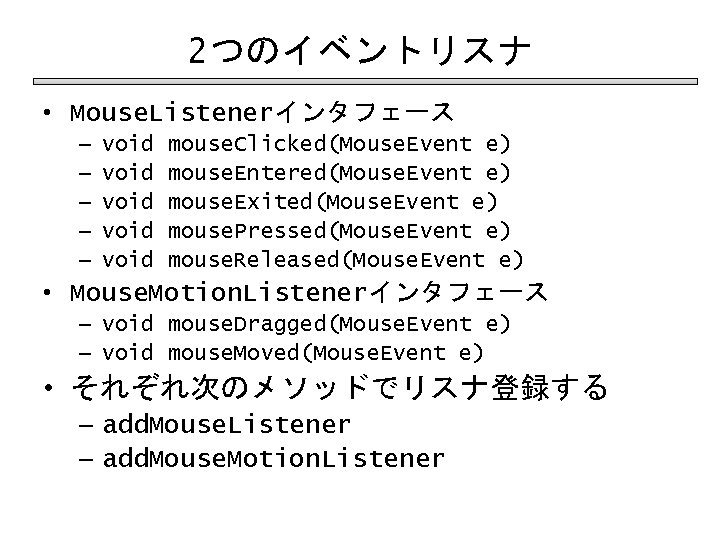
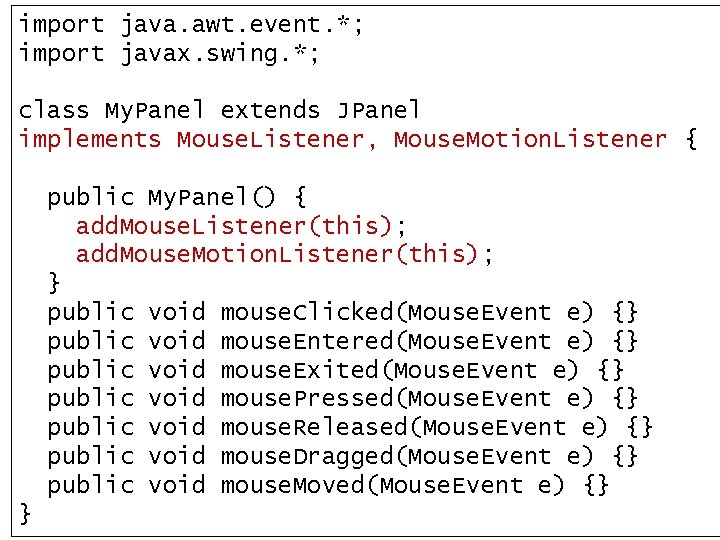
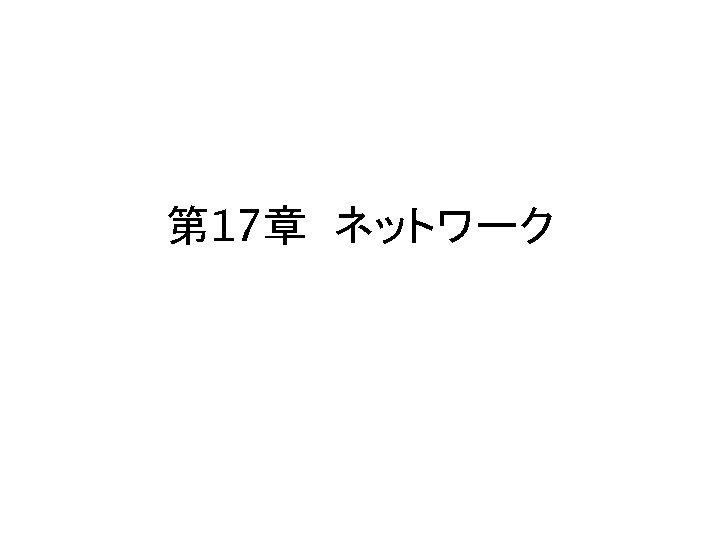
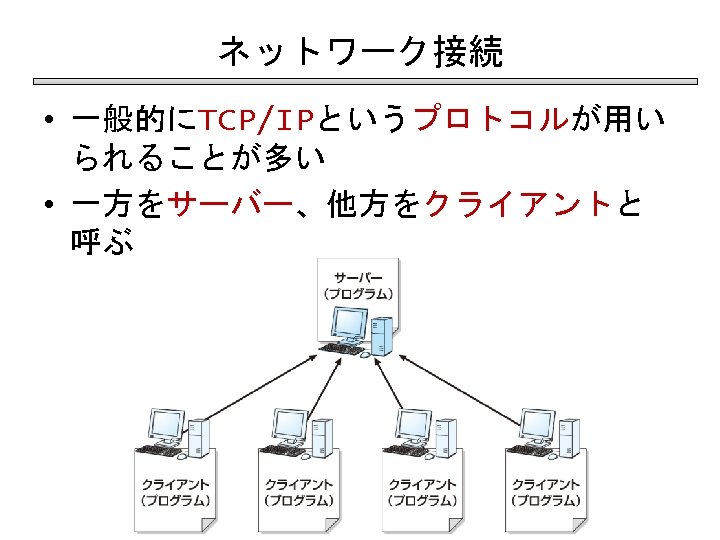
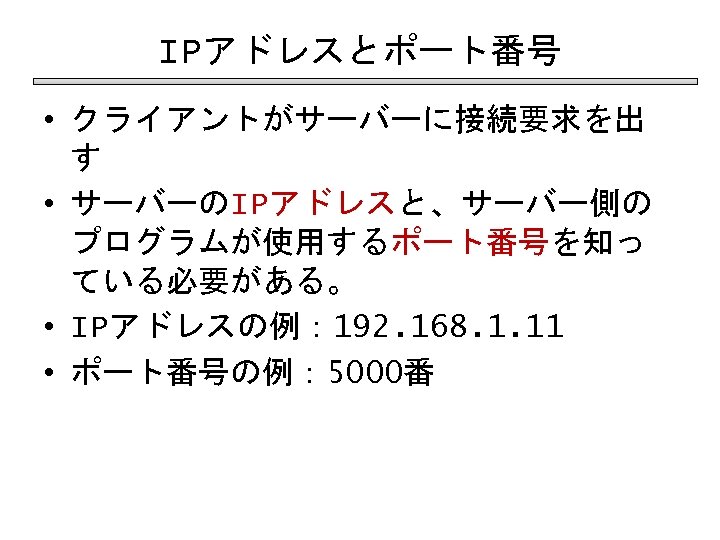
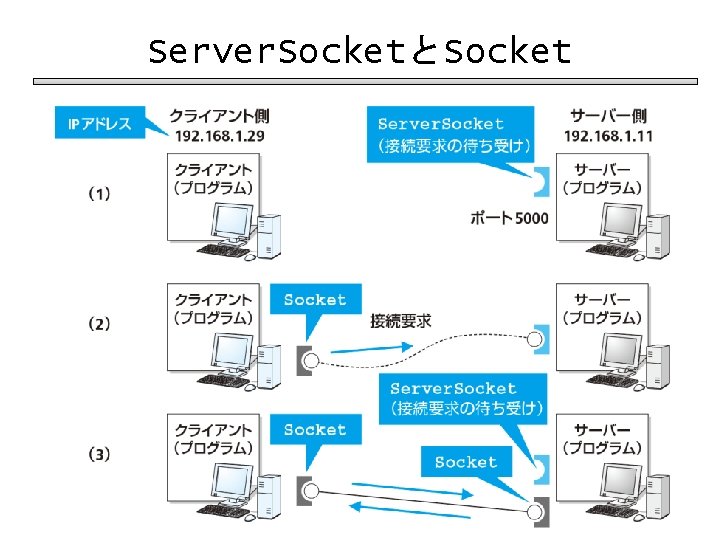
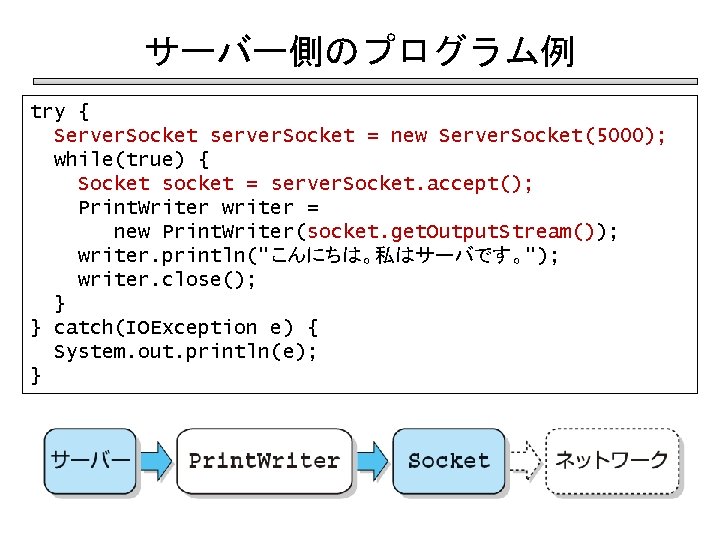
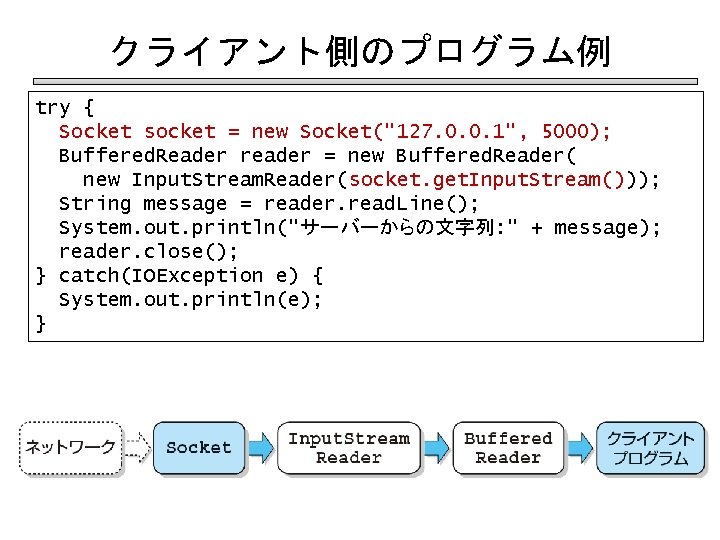
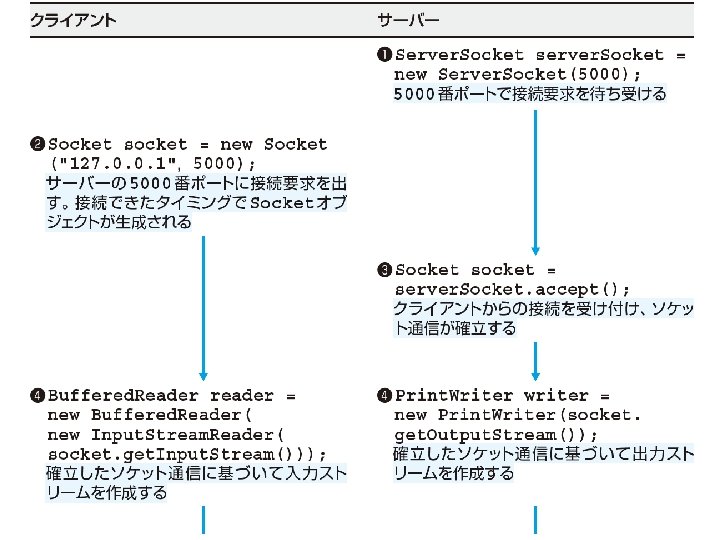
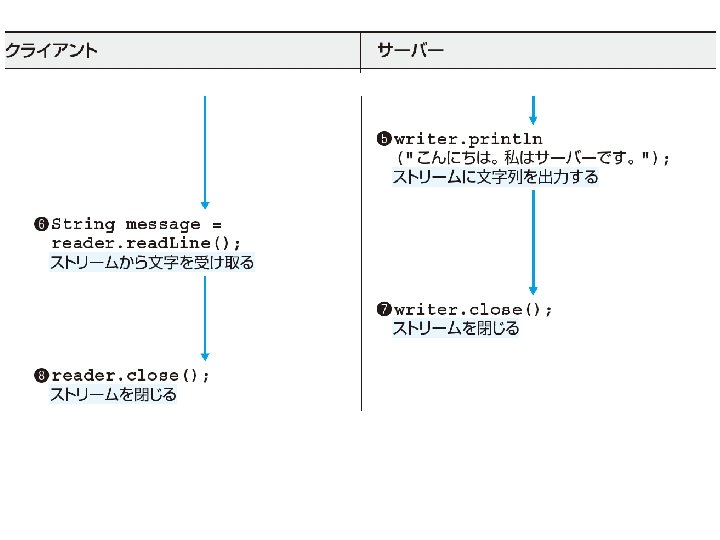
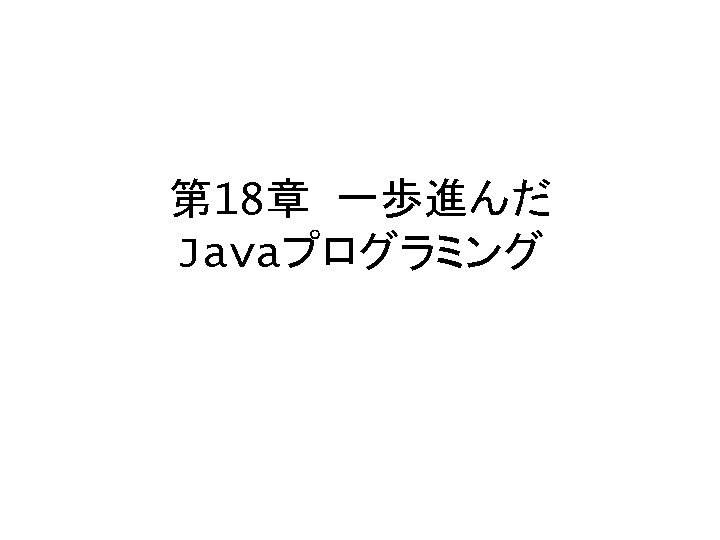
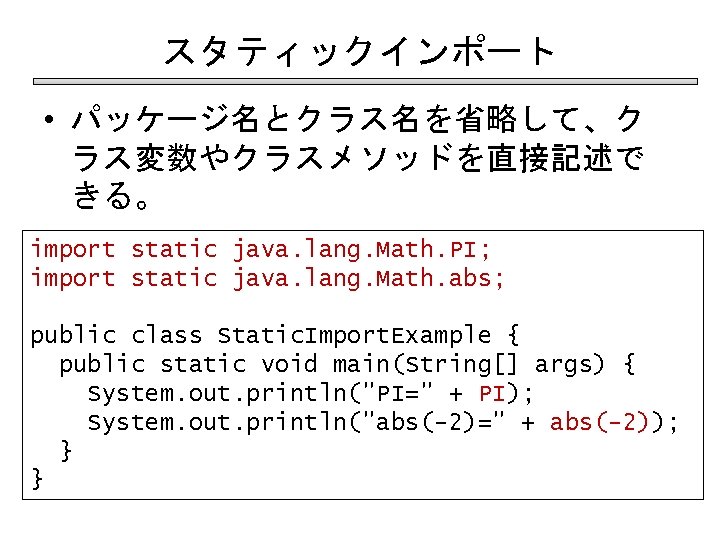
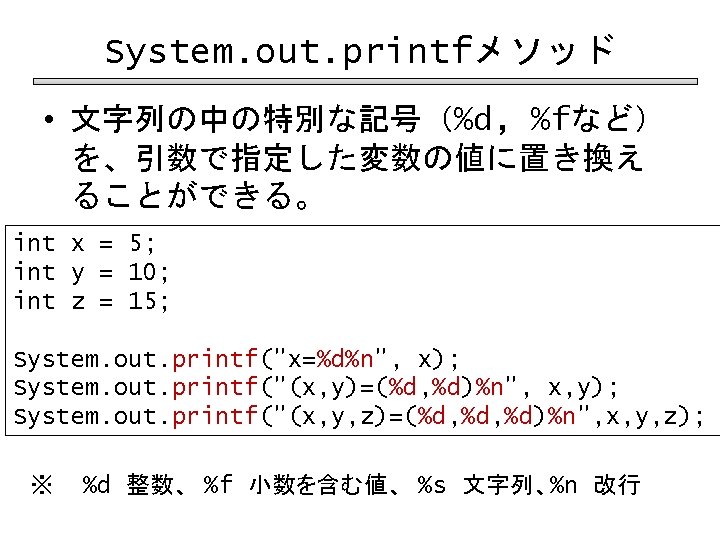
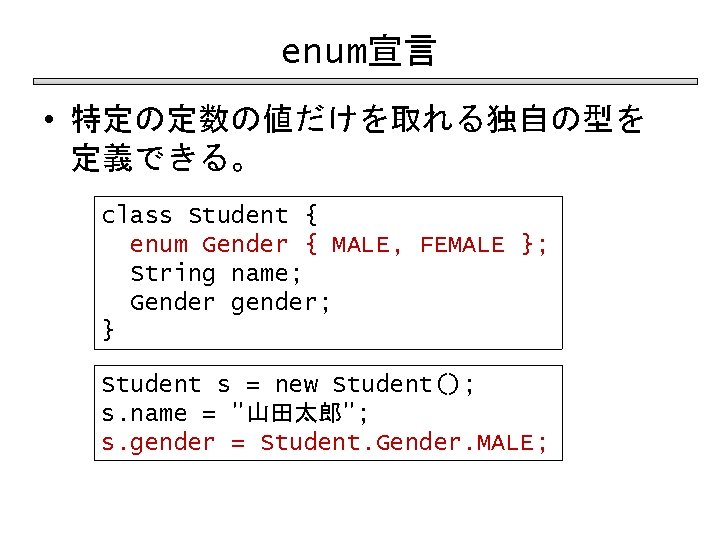
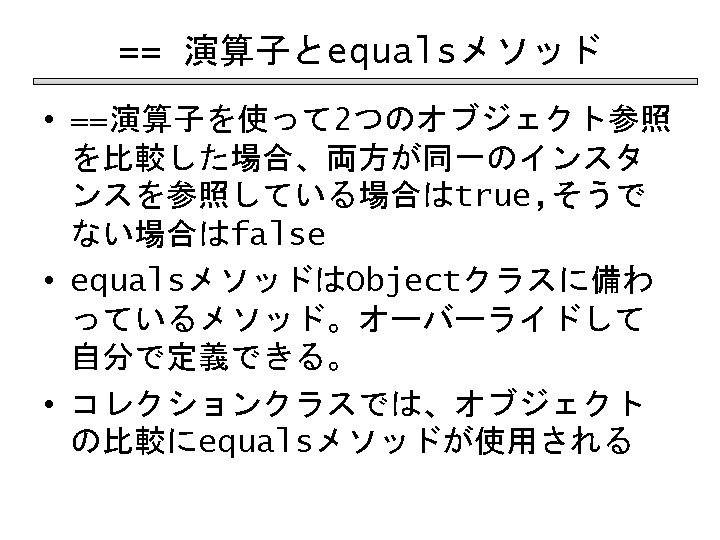
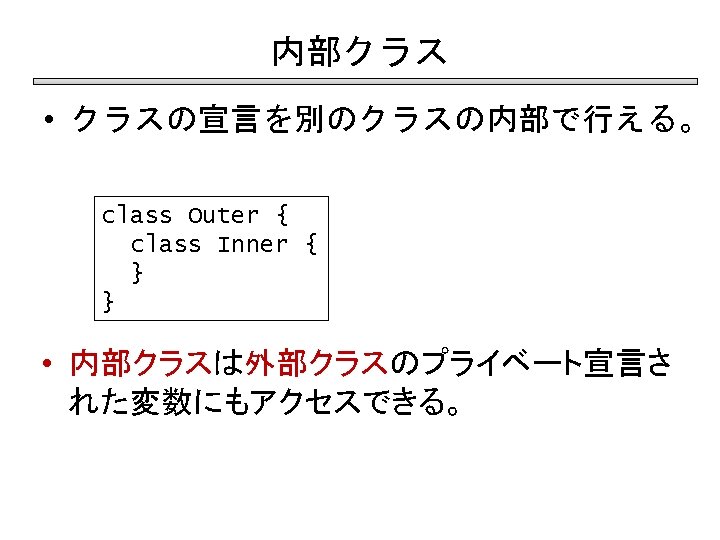
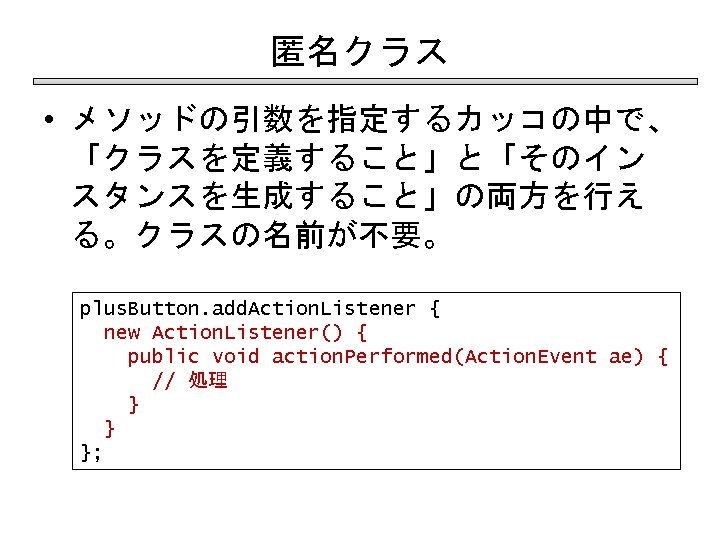
- Slides: 159
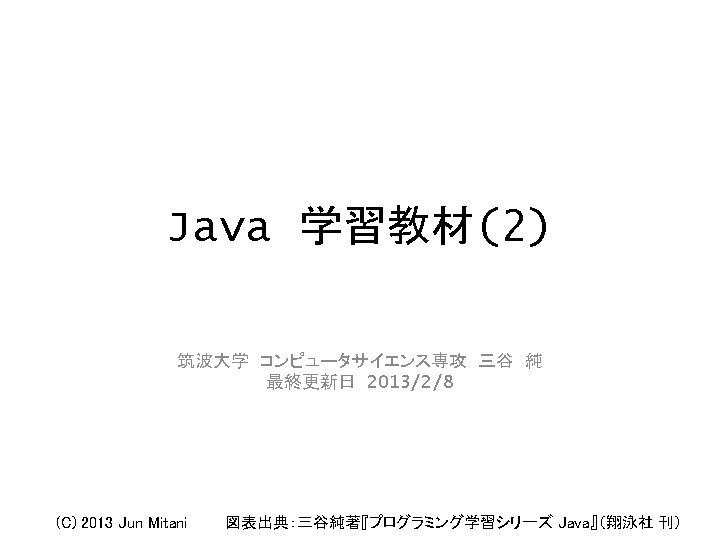
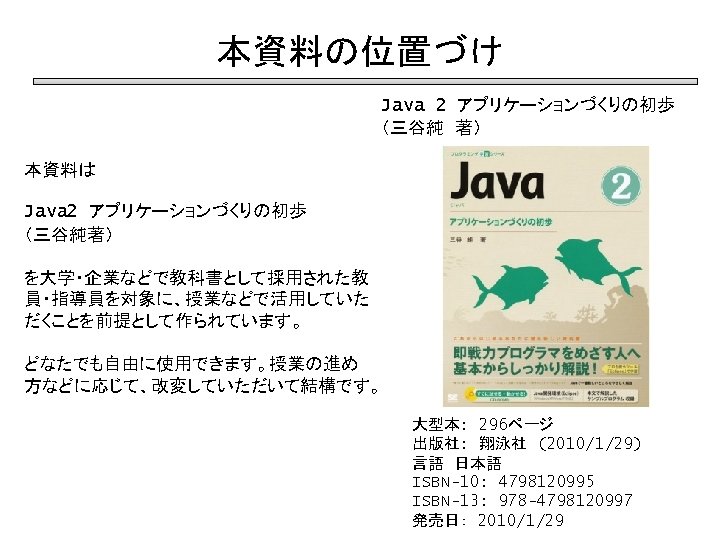
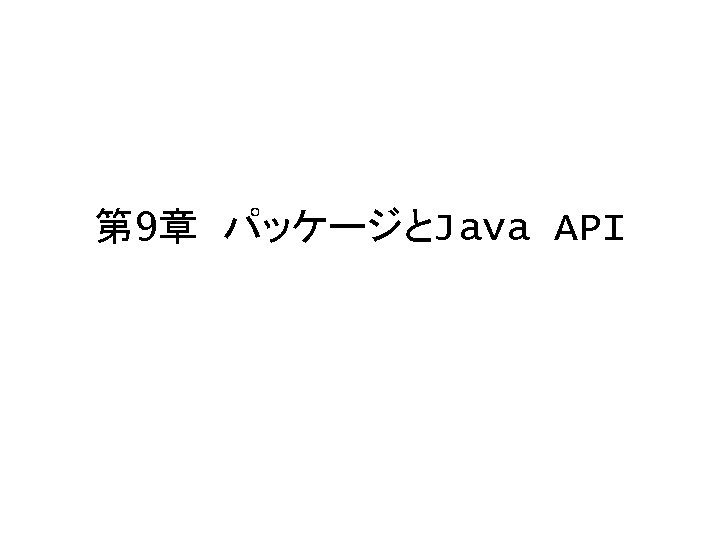
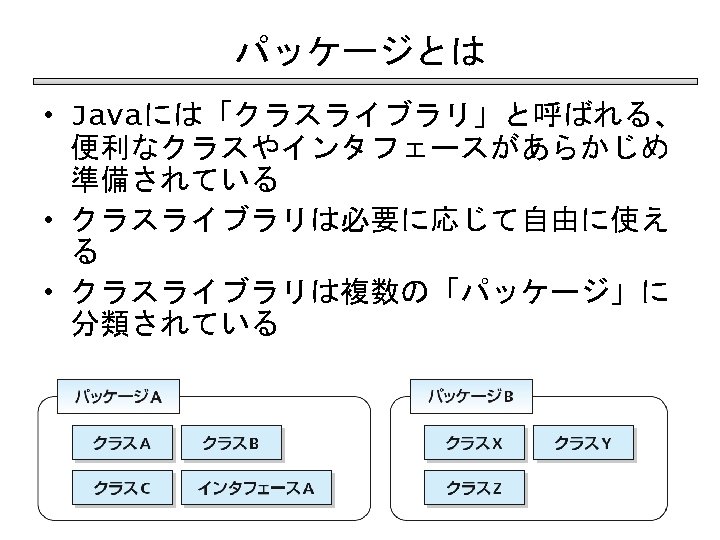
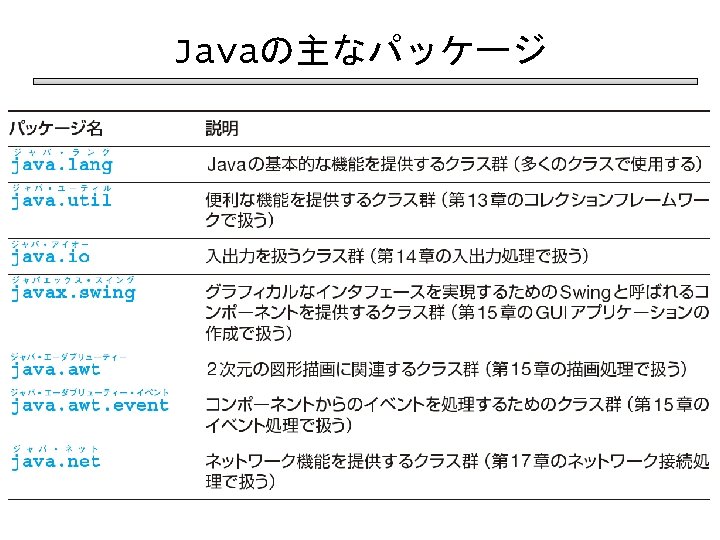
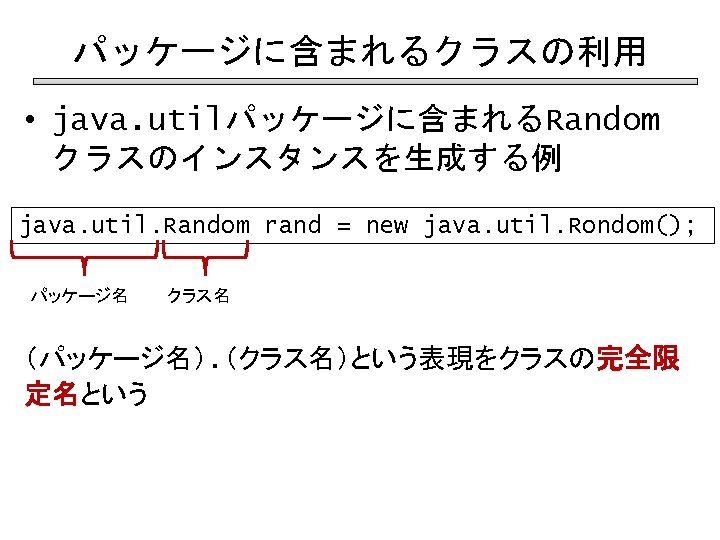
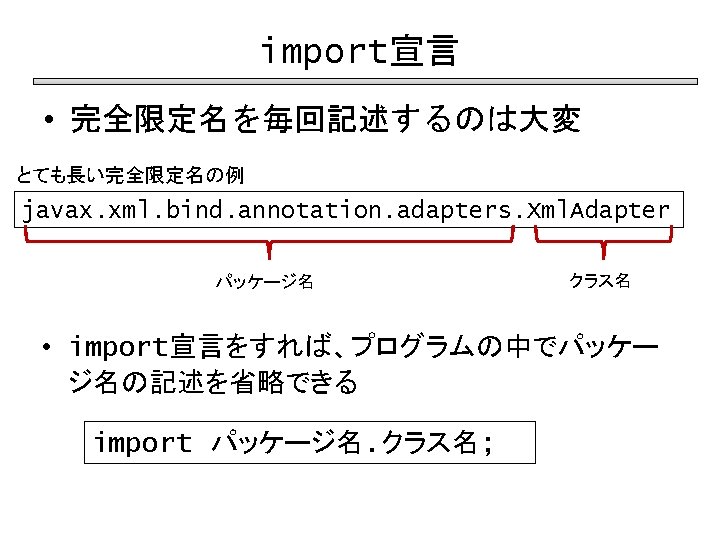
![import宣言の使用例 import java util Random class Import Example public static void mainString args import宣言の使用例 import java. util. Random; class Import. Example { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-8.jpg)
import宣言の使用例 import java. util. Random; class Import. Example { public static void main(String[] args) { Random rand = new Random(); // 0~ 1の間のランダムな値を出力する System. out. println(rand. next. Double()); } } java. util. Randomのimport宣言をしている
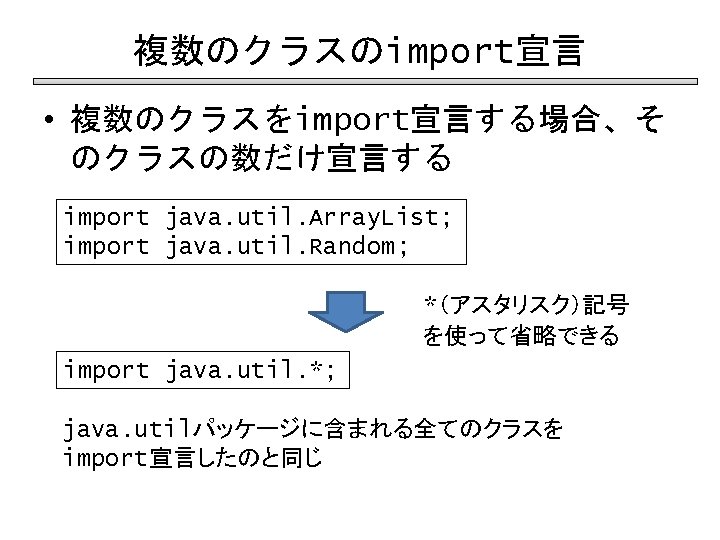
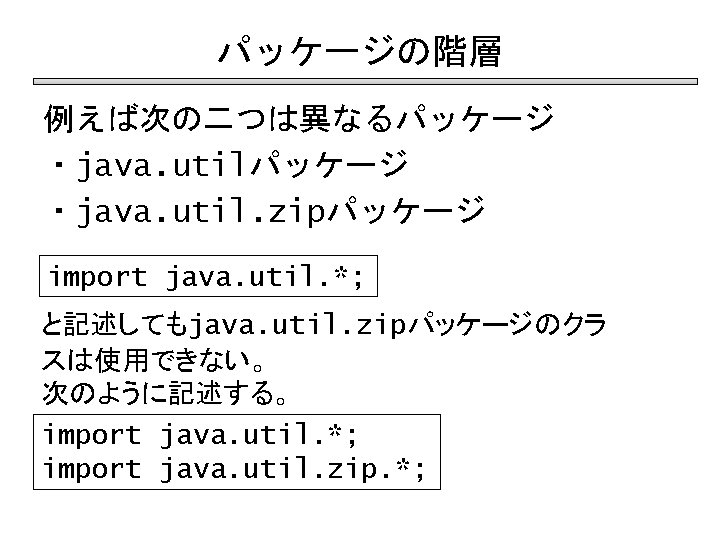
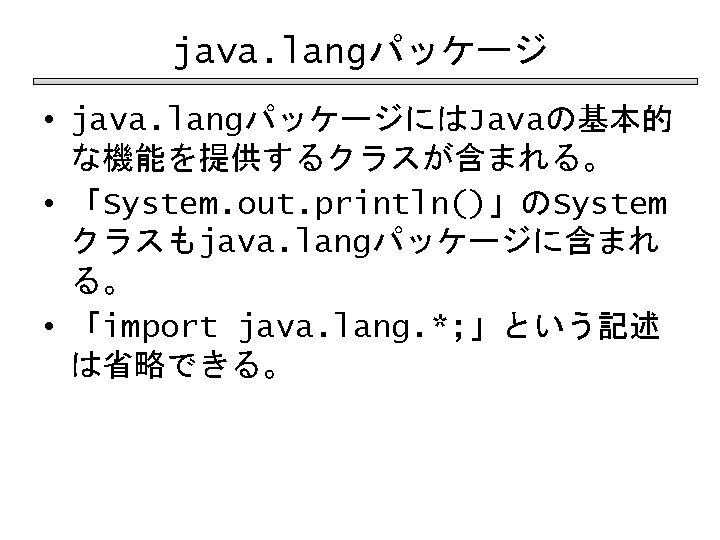
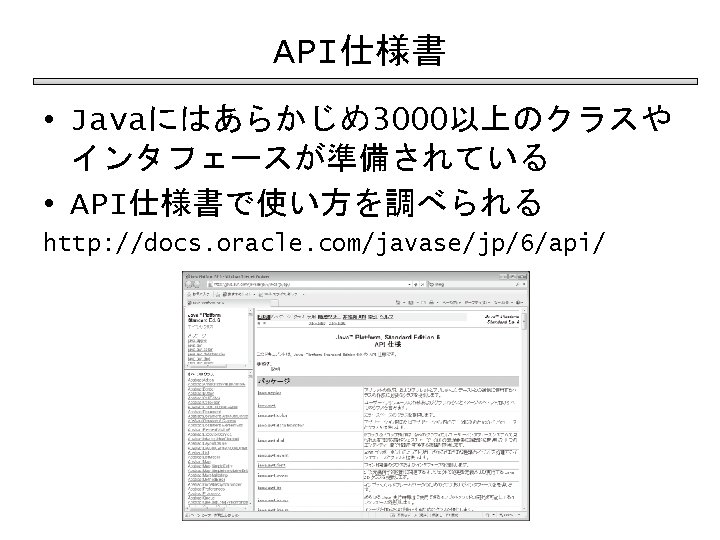
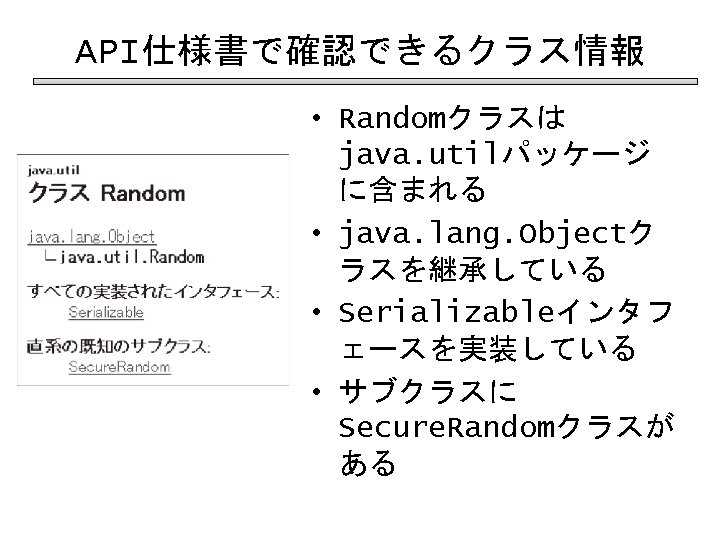
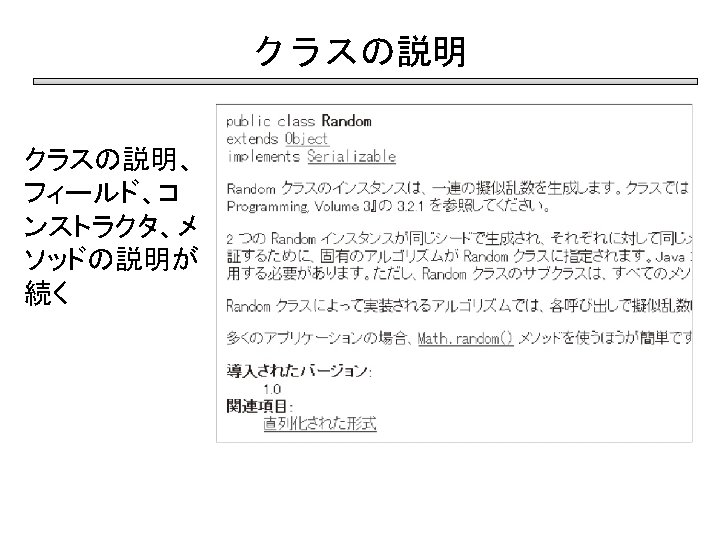
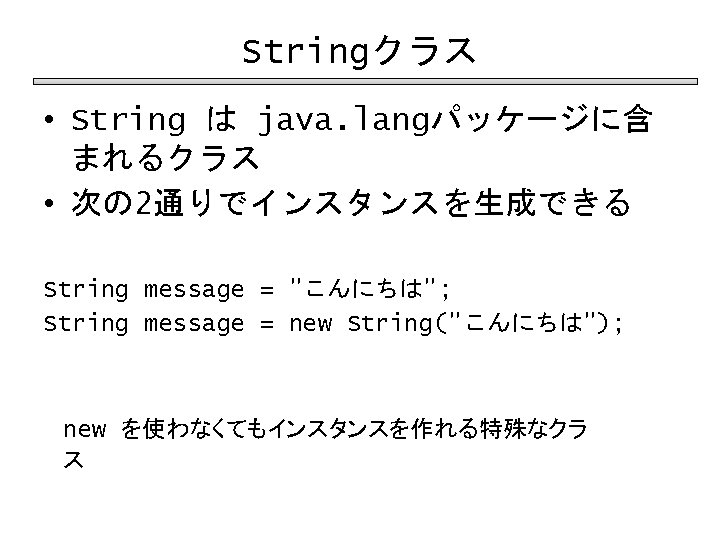
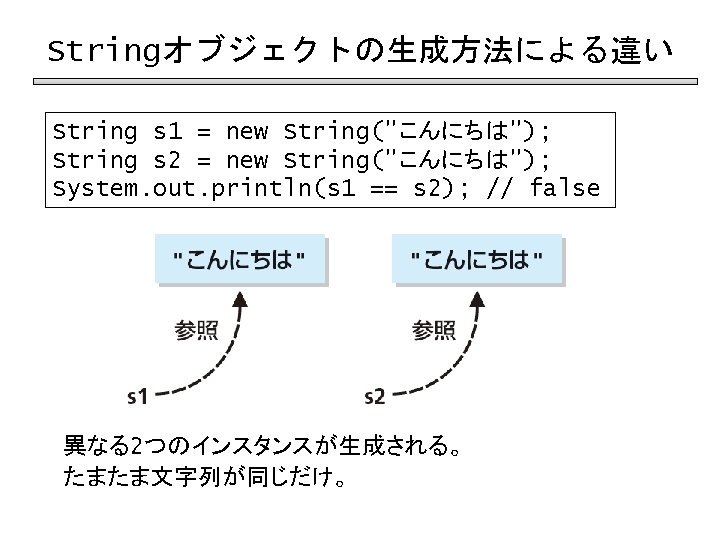
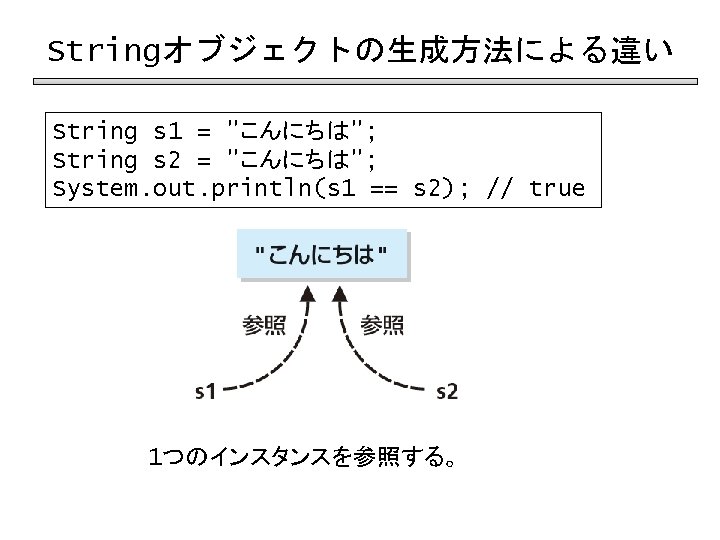
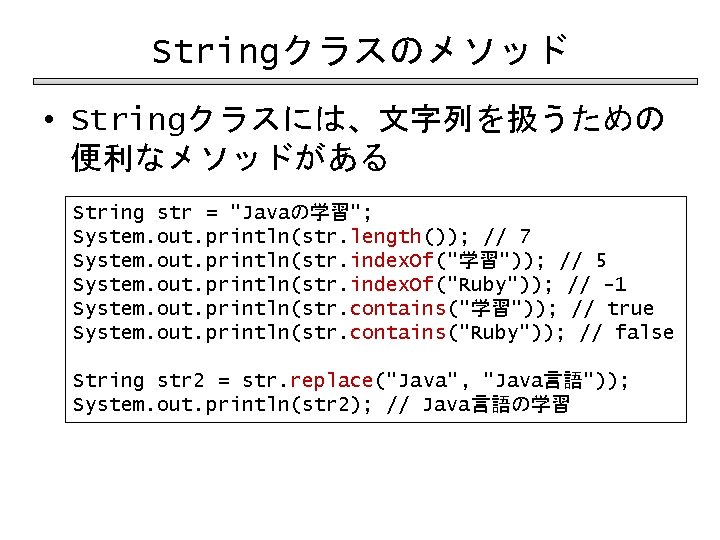
Stringクラスのメソッド • Stringクラスには、文字列を扱うための 便利なメソッドがある String str = "Javaの学習"; System. out. println(str. length()); // 7 System. out. println(str. index. Of("学習")); // 5 System. out. println(str. index. Of("Ruby")); // -1 System. out. println(str. contains("学習")); // true System. out. println(str. contains("Ruby")); // false String str 2 = str. replace("Java", "Java言語")); System. out. println(str 2); // Java言語の学習
![Stringクラスのメソッド 文字列を区切り記号で分割する例 String str 20121231 String items str split forint i Stringクラスのメソッド 文字列を区切り記号で分割する例 String str = "2012/12/31"; String[] items = str. split("/"); for(int i =](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-19.jpg)
Stringクラスのメソッド 文字列を区切り記号で分割する例 String str = "2012/12/31"; String[] items = str. split("/"); for(int i = 0; i < items. length; i++) { System. out. println(items[i]); } 実行結果 2012 12 31
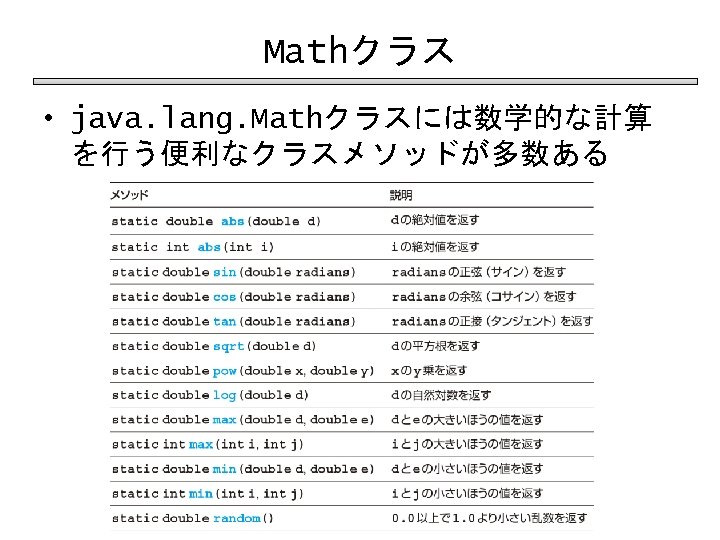
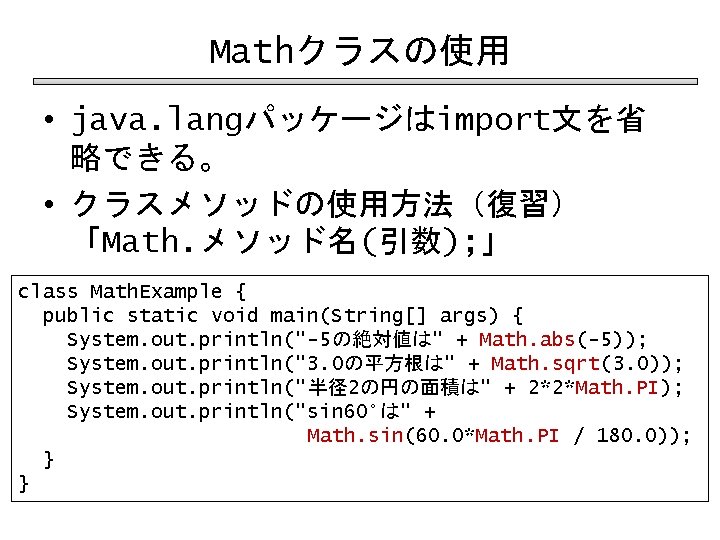
Mathクラスの使用 • java. langパッケージはimport文を省 略できる。 • クラスメソッドの使用方法(復習) 「Math. メソッド名(引数); 」 class Math. Example { public static void main(String[] args) { System. out. println("-5の絶対値は" + Math. abs(-5)); System. out. println("3. 0の平方根は" + Math. sqrt(3. 0)); System. out. println("半径2の円の面積は" + 2*2*Math. PI); System. out. println("sin 60°は" + Math. sin(60. 0*Math. PI / 180. 0)); } }
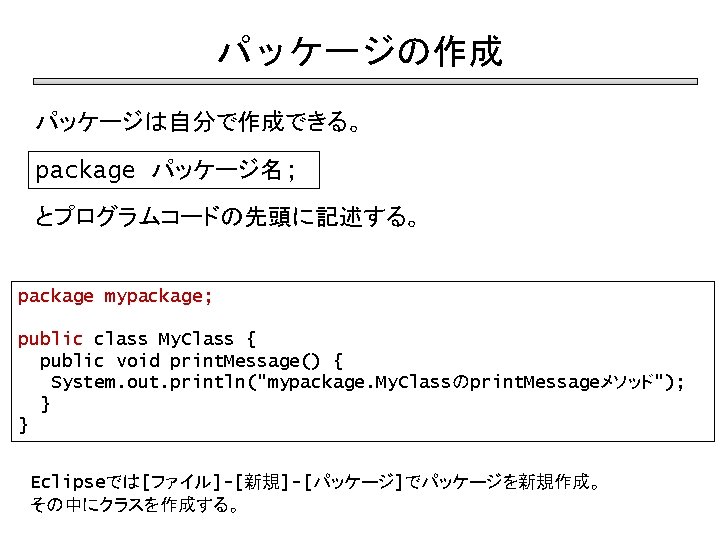
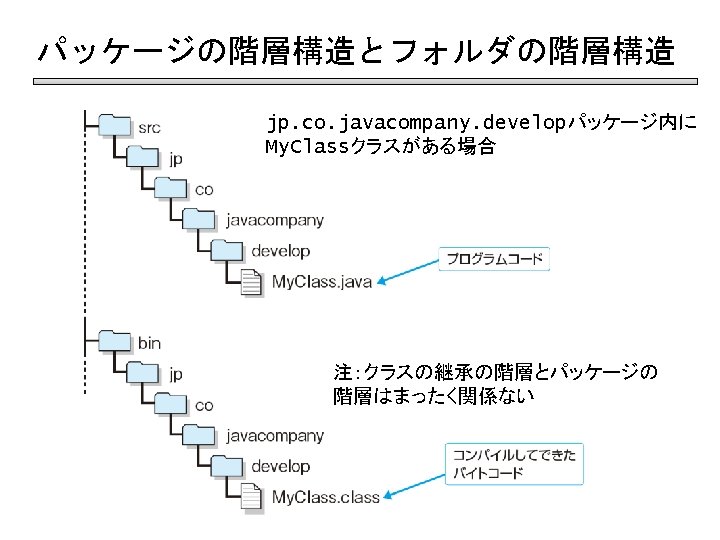
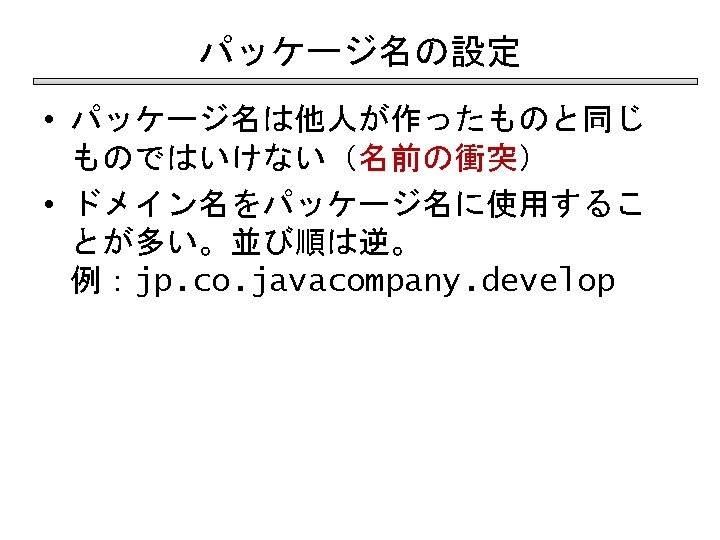
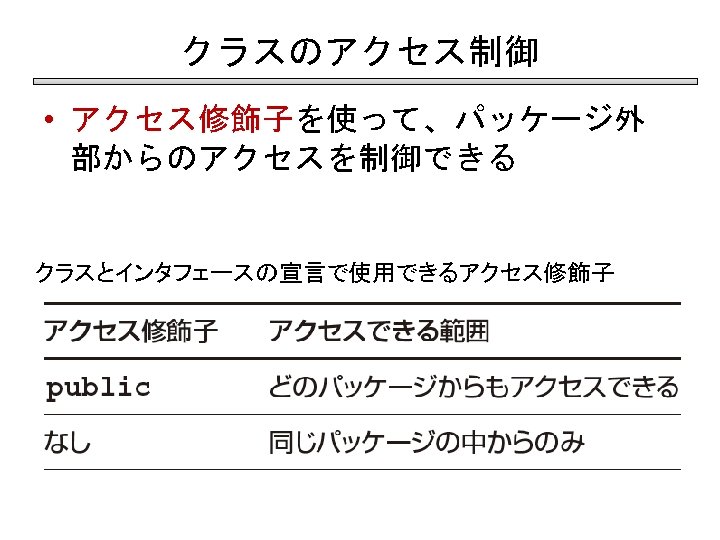
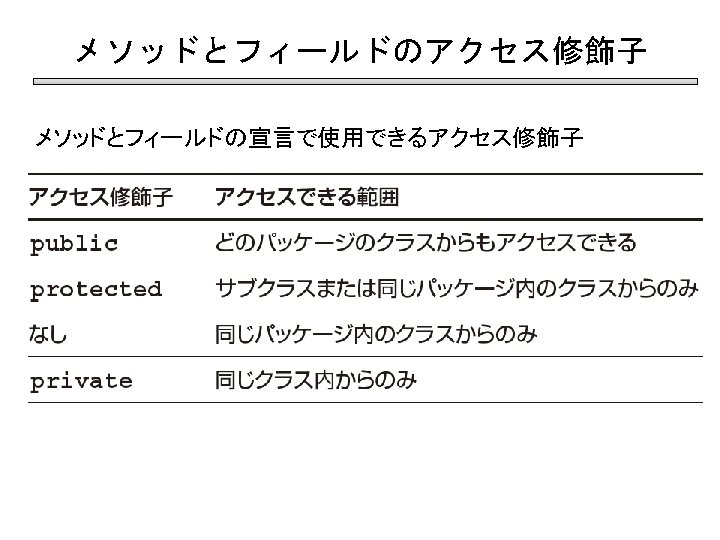
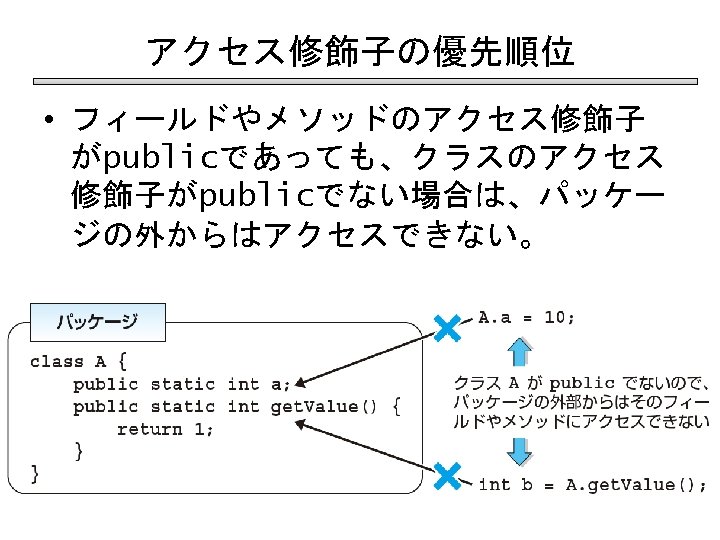
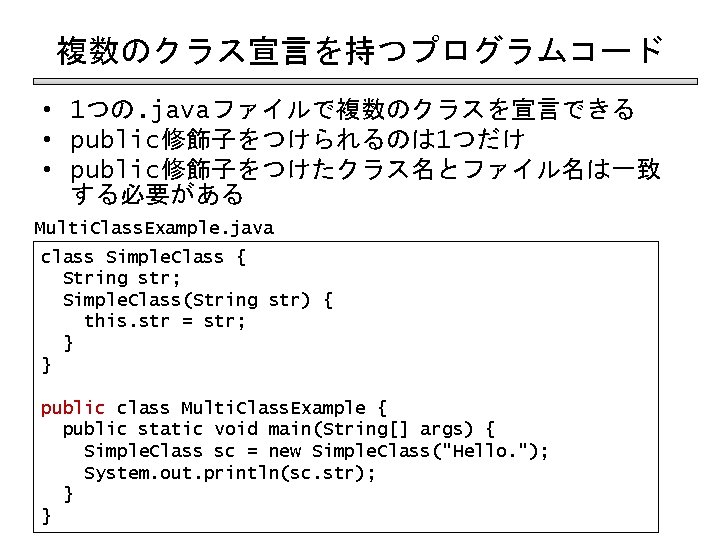
複数のクラス宣言を持つプログラムコード • 1つの. javaファイルで複数のクラスを宣言できる • public修飾子をつけられるのは 1つだけ • public修飾子をつけたクラス名とファイル名は一致 する必要がある Multi. Class. Example. java class Simple. Class { String str; Simple. Class(String str) { this. str = str; } } public class Multi. Class. Example { public static void main(String[] args) { Simple. Class sc = new Simple. Class("Hello. "); System. out. println(sc. str); } }
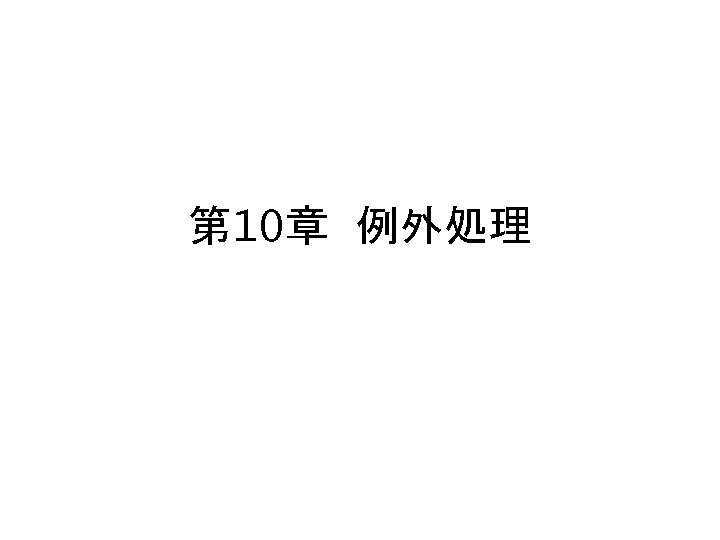
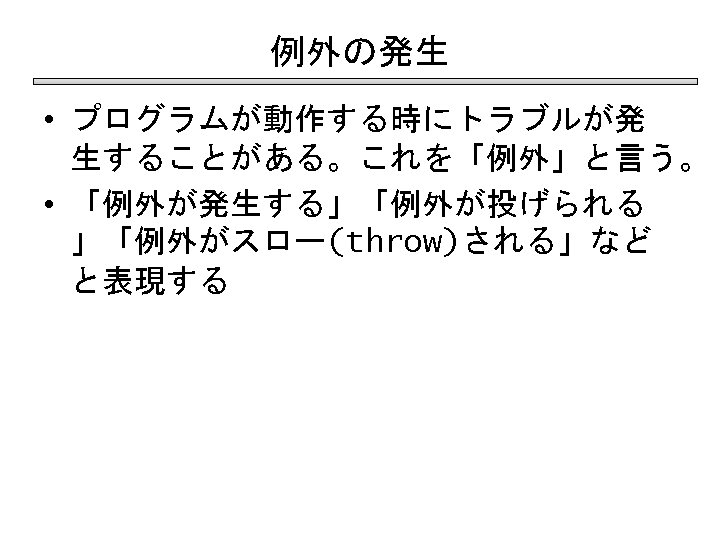
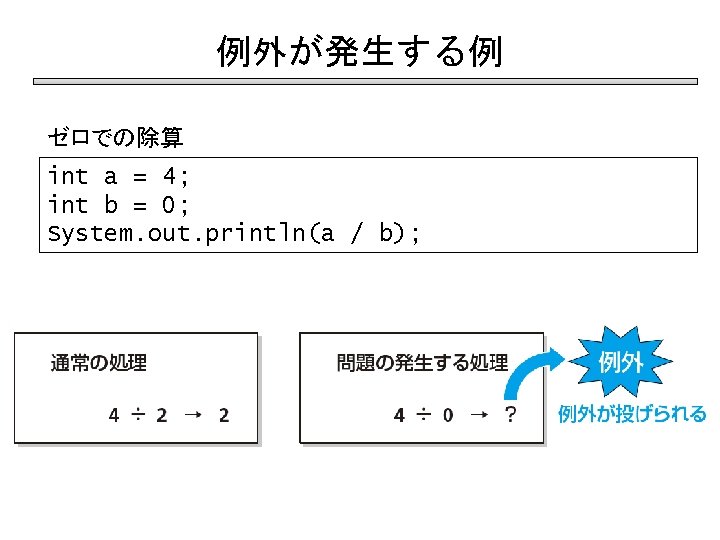
![例外が発生する例 範囲を超えたインデックスの参照 int scores new int3 scores0 50 scores1 55 scores2 例外が発生する例 範囲を超えたインデックスの参照 int[] scores = new int[3]; scores[0] = 50; scores[1] = 55; scores[2]](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-32.jpg)
例外が発生する例 範囲を超えたインデックスの参照 int[] scores = new int[3]; scores[0] = 50; scores[1] = 55; scores[2] = 70; scores[3] = 65;
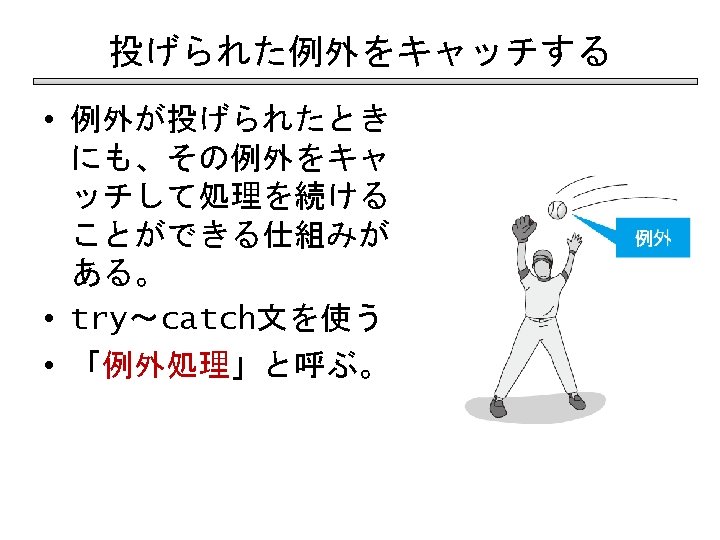
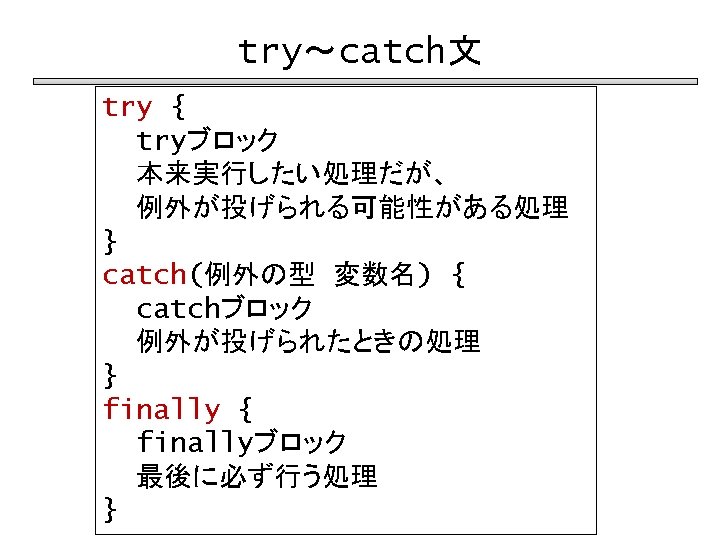
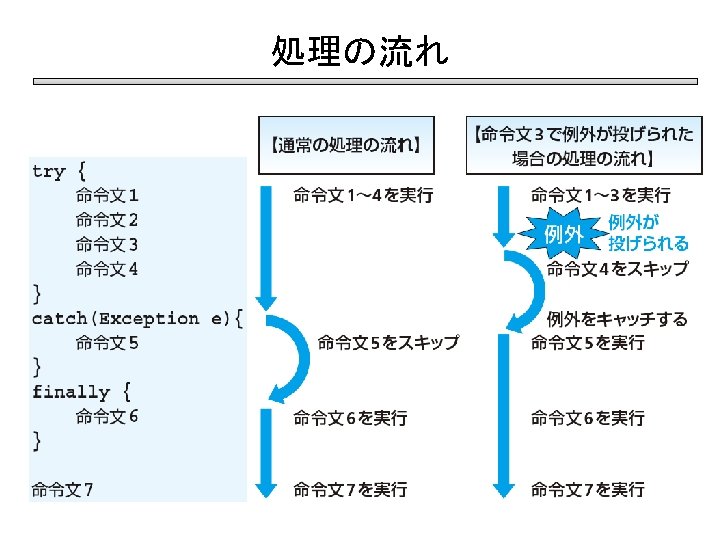
![例外処理の例 public class Exception Example 3 public static void mainString args int 例外処理の例 public class Exception. Example 3 { public static void main(String[] args) { int](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-36.jpg)
例外処理の例 public class Exception. Example 3 { public static void main(String[] args) { int a = 4; int b = 0; try { int c = a / b; System. out. println("cの値は" + c); } catch (Arithmetic. Exception e) { System. out. println("例外をキャッチしました"); System. out. println(e); } System. out. println("プログラムを終了します"); } }
![finallyの処理 public static void mainString args int a 4 int b finallyの処理 public static void main(String[] args) { int a = 4; int b =](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-37.jpg)
finallyの処理 public static void main(String[] args) { int a = 4; int b = 0; try { int c = a / b; System. out. println("cの値は" + c); } catch (Arithmetic. Exception e) { System. out. println("例外をキャッチしました"); System. out. println(e); return; } finally { System. out. println("finallyブロックの処理です"); } System. out. println("プログラムを終了します"); }
![catchブロックの検索 class Simple Class void do Something int array new int3 catchブロックの検索 class Simple. Class { void do. Something() { int array[] = new int[3];](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-38.jpg)
catchブロックの検索 class Simple. Class { void do. Something() { int array[] = new int[3]; array[10] = 99; // 例外が発生する System. out. println("do. Somethingメソッドを終了します"); } } public class Exception. Example 5 { public static void main(String args[]) { Simple. Class obj = new Simple. Class(); try { obj. do. Something(); // 例外の発生するメソッドの呼び出し } catch (Array. Index. Out. Of. Bounds. Exception e) { System. out. println("例外をキャッチしました"); e. print. Stack. Trace(); } } }
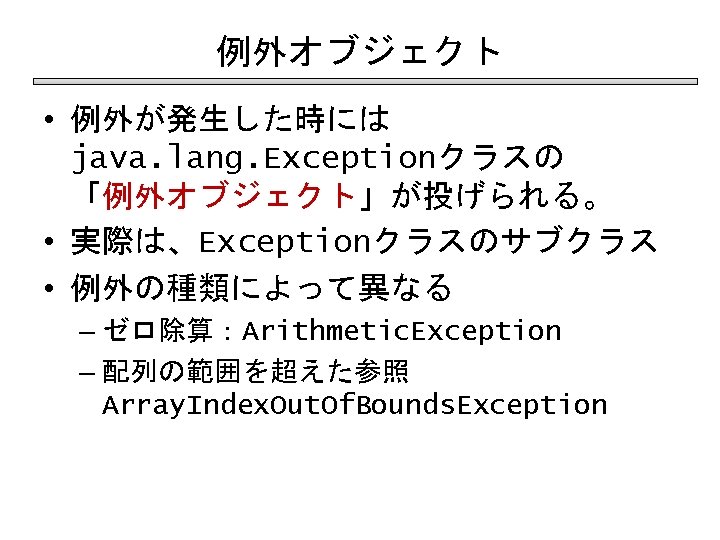
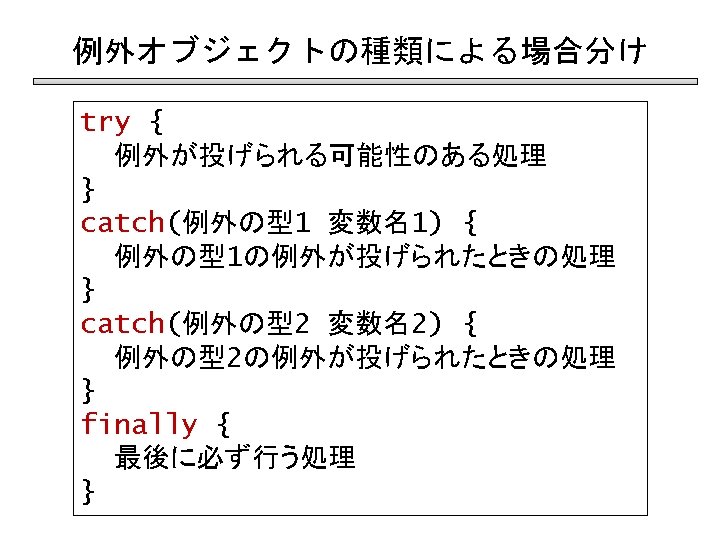
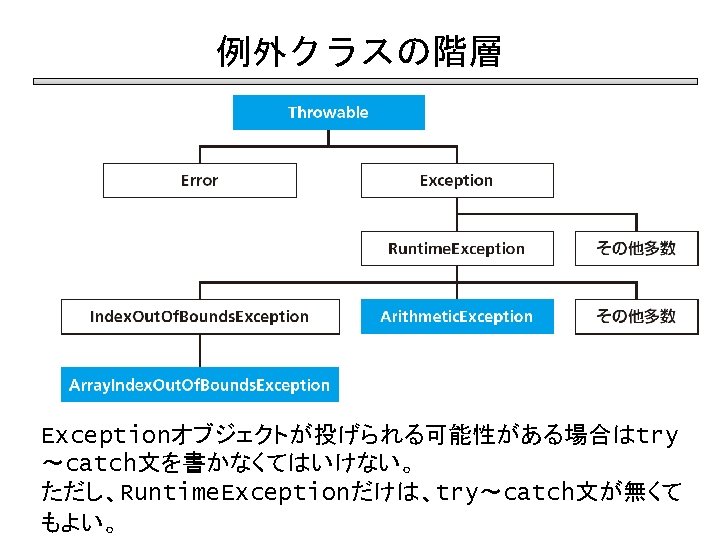
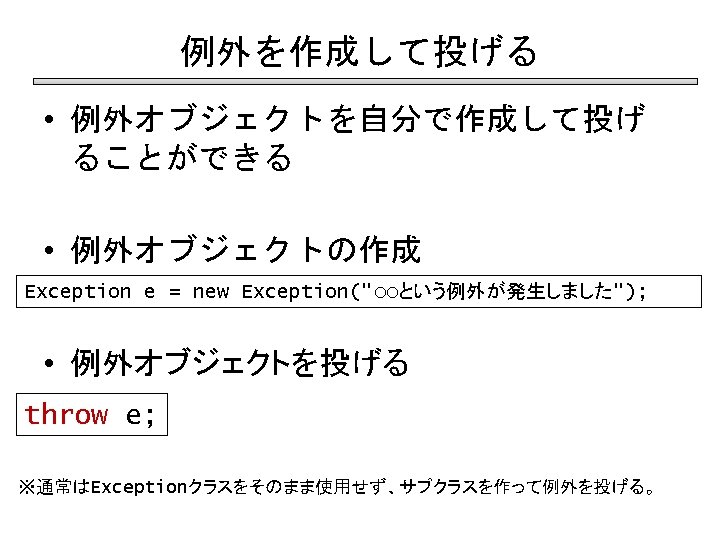
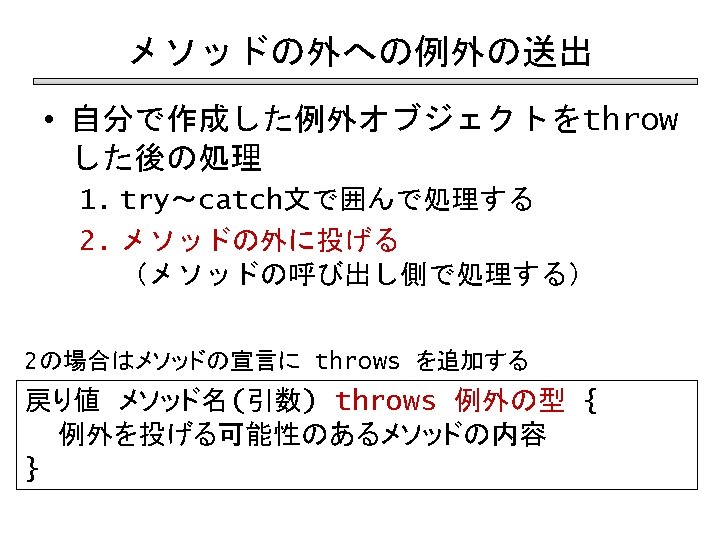
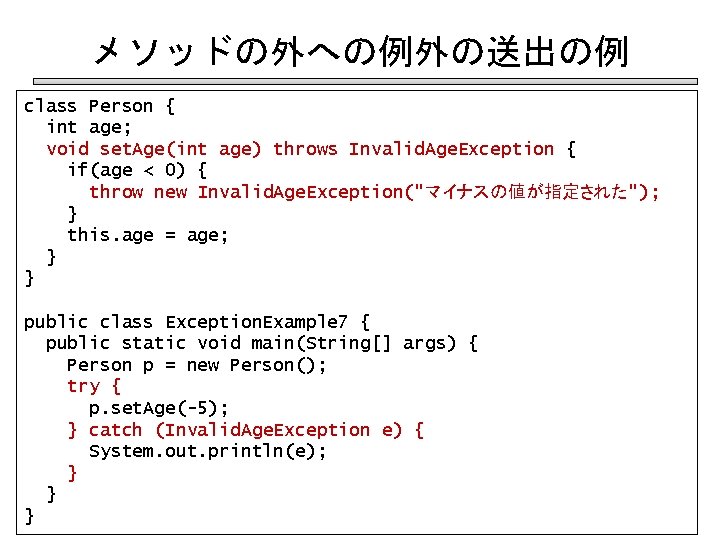
メソッドの外への例外の送出の例 class Person { int age; void set. Age(int age) throws Invalid. Age. Exception { if(age < 0) { throw new Invalid. Age. Exception("マイナスの値が指定された"); } this. age = age; } } public class Exception. Example 7 { public static void main(String[] args) { Person p = new Person(); try { p. set. Age(-5); } catch (Invalid. Age. Exception e) { System. out. println(e); } } }
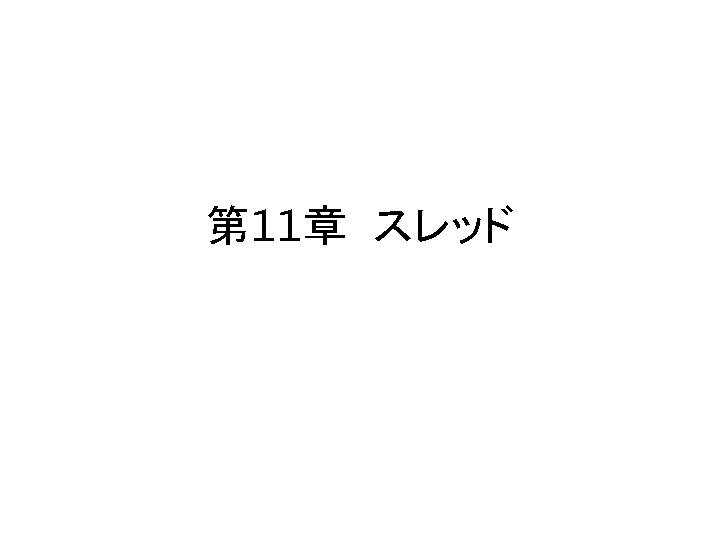
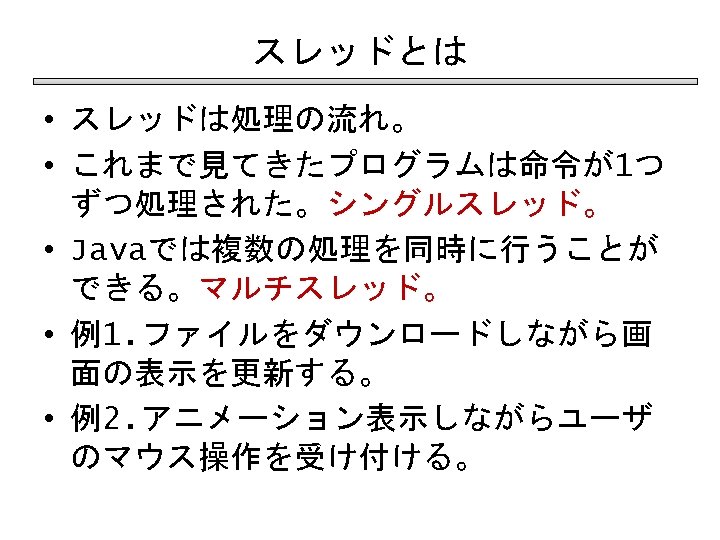
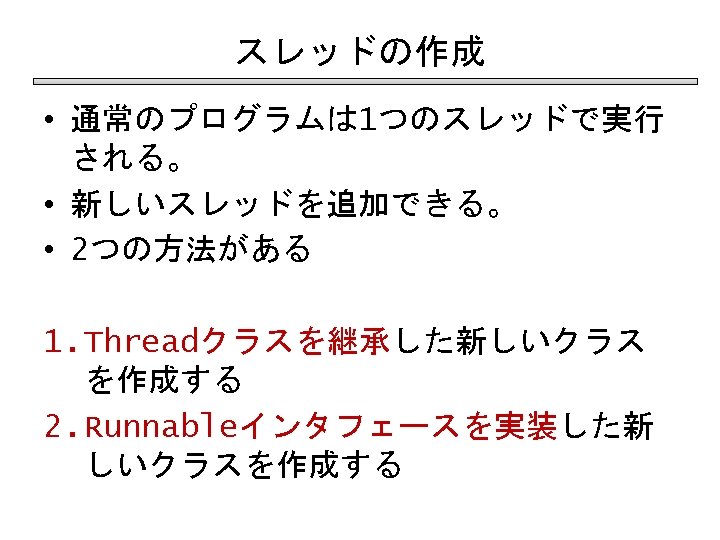
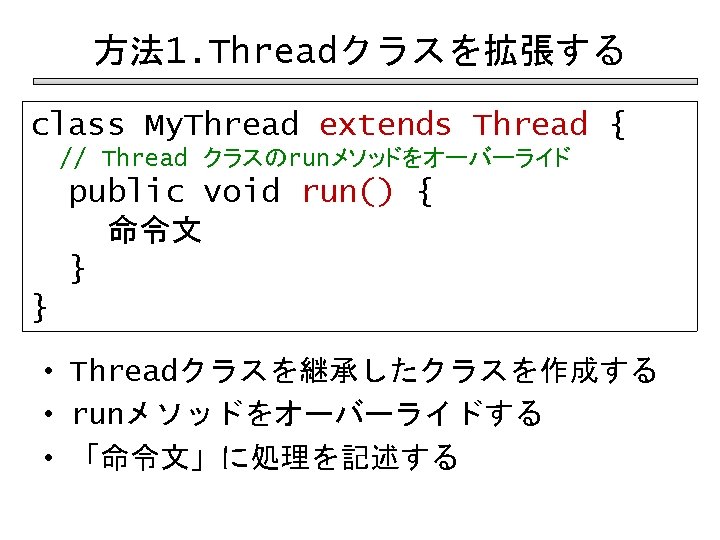
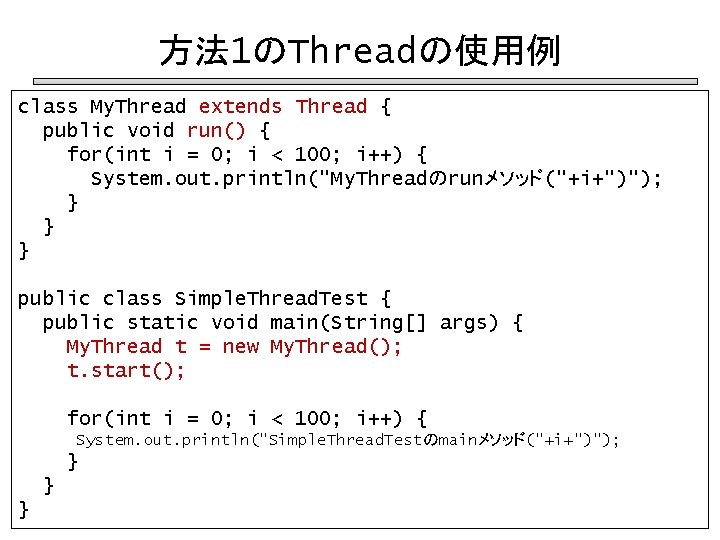
方法 1のThreadの使用例 class My. Thread extends Thread { public void run() { for(int i = 0; i < 100; i++) { System. out. println("My. Threadのrunメソッド("+i+")"); } } } public class Simple. Thread. Test { public static void main(String[] args) { My. Thread t = new My. Thread(); t. start(); for(int i = 0; i < 100; i++) { System. out. println("Simple. Thread. Testのmainメソッド("+i+")"); } } }
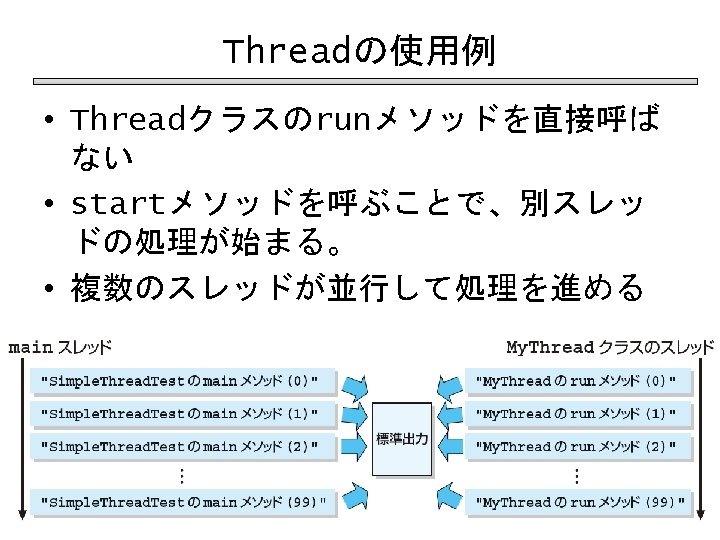
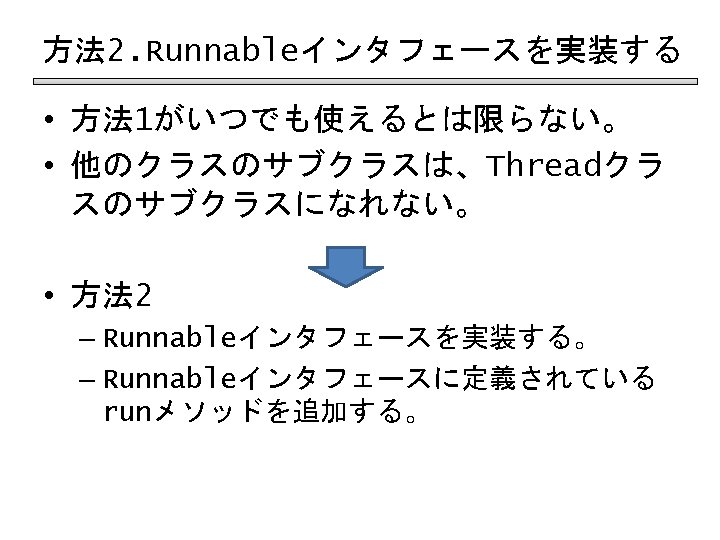
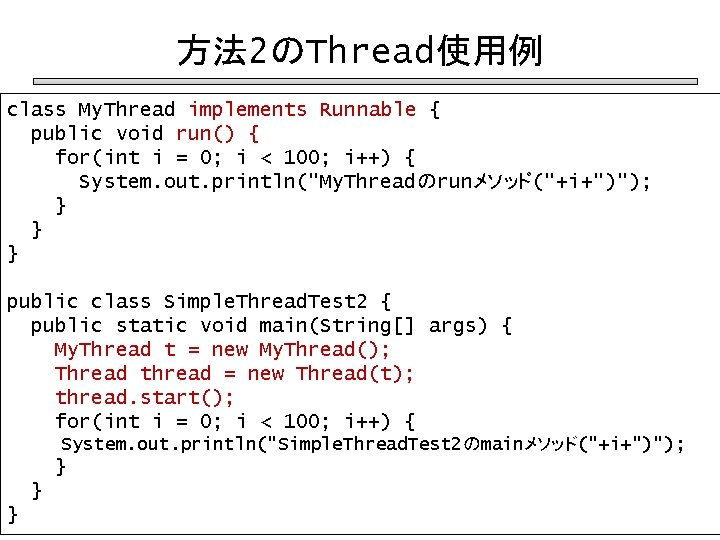
方法 2のThread使用例 class My. Thread implements Runnable { public void run() { for(int i = 0; i < 100; i++) { System. out. println("My. Threadのrunメソッド("+i+")"); } } } public class Simple. Thread. Test 2 { public static void main(String[] args) { My. Thread t = new My. Thread(); Thread thread = new Thread(t); thread. start(); for(int i = 0; i < 100; i++) { System. out. println("Simple. Thread. Test 2のmainメソッド("+i+")"); } } }
![スレッドを一定時間停止させる Thread sleep停止時間ミリ秒 public class Sleep Example public static void mainString args スレッドを一定時間停止させる Thread. sleep(停止時間(ミリ秒)); public class Sleep. Example { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-53.jpg)
スレッドを一定時間停止させる Thread. sleep(停止時間(ミリ秒)); public class Sleep. Example { public static void main(String[] args) { for(int i = 0; i < 10; i++) { try { Thread. sleep(1000); } catch (Interrupted. Exception e) { System. out. println(e); } System. out. print("*"); } } }
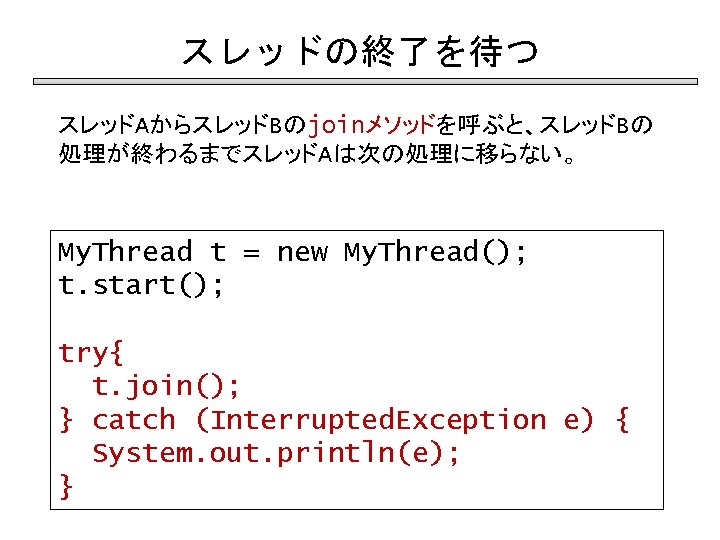
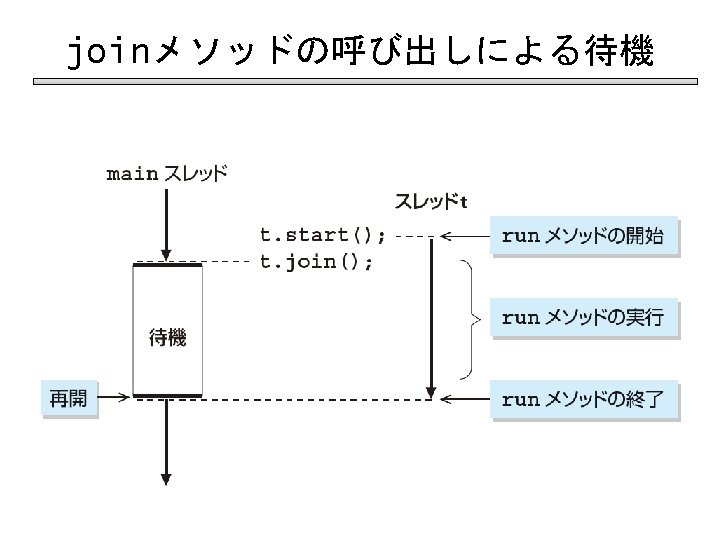
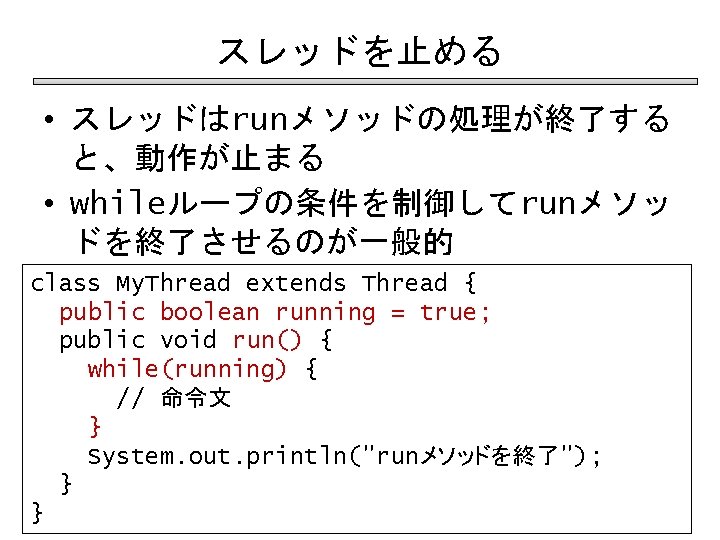
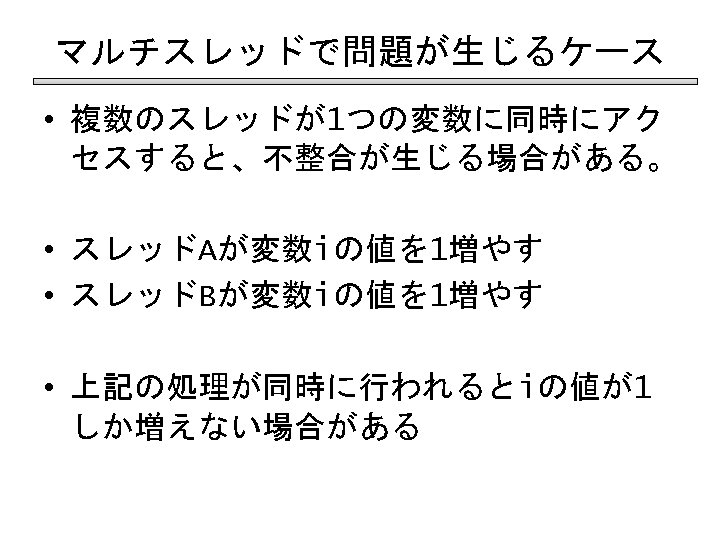
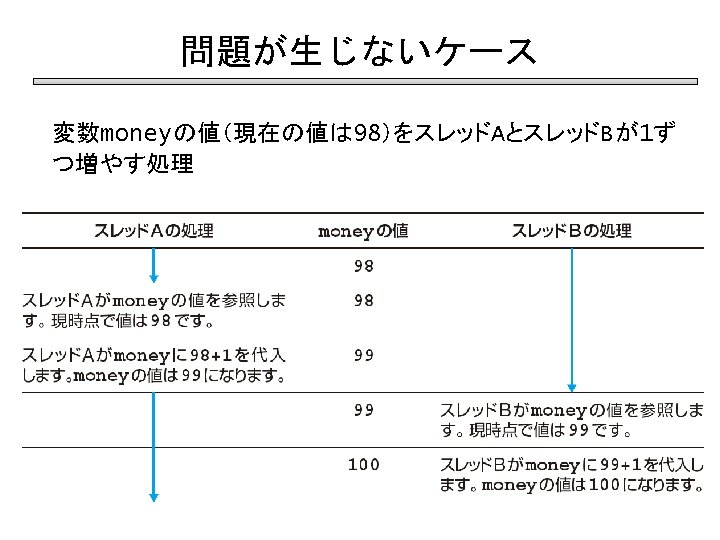
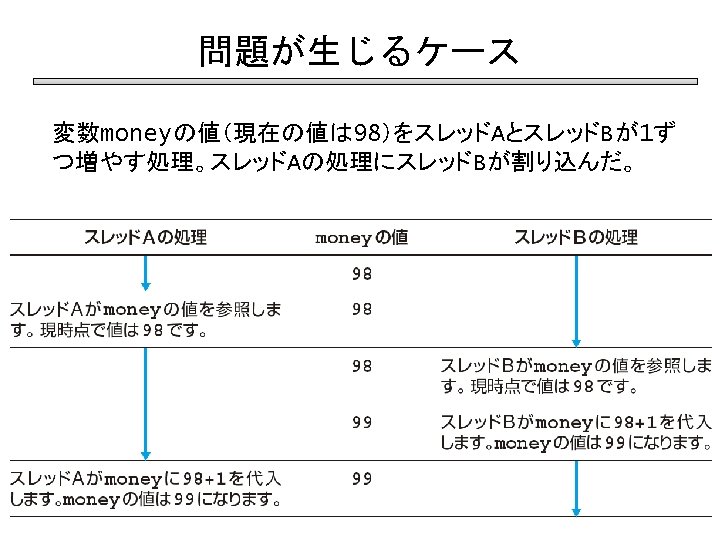
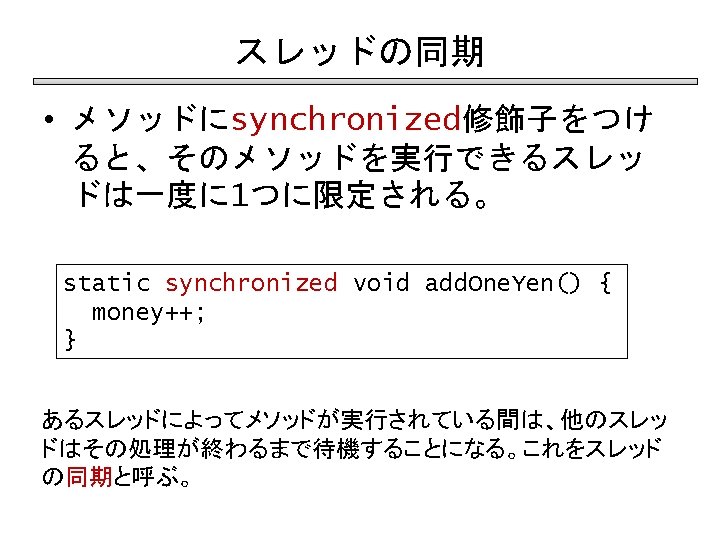
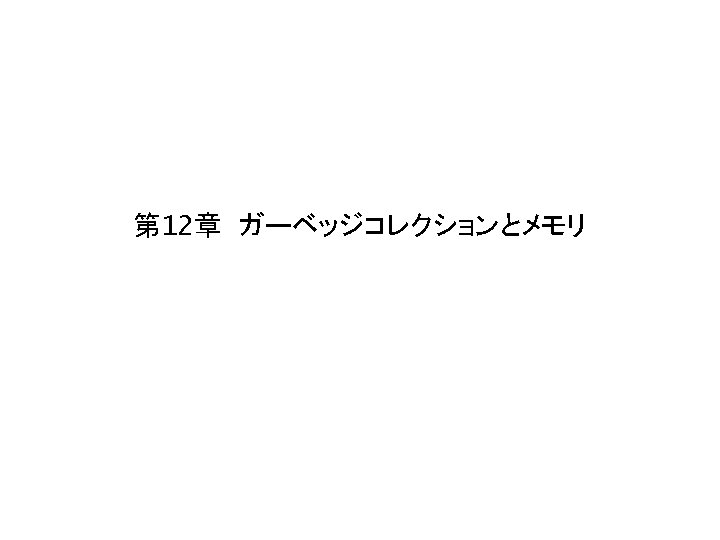
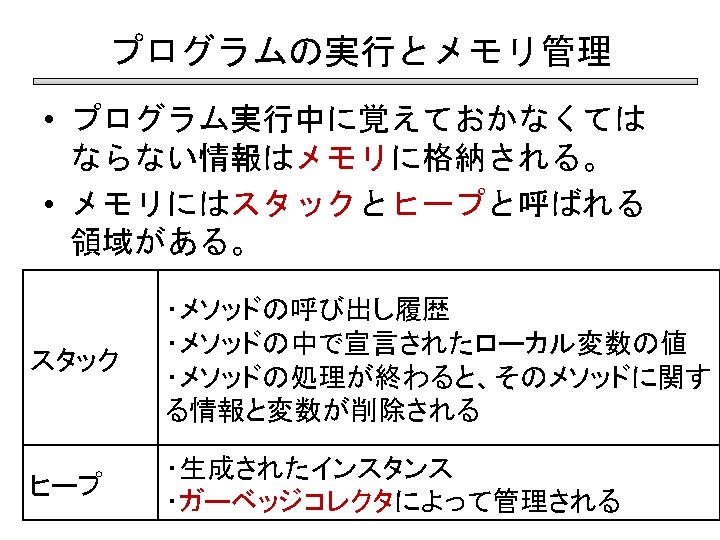
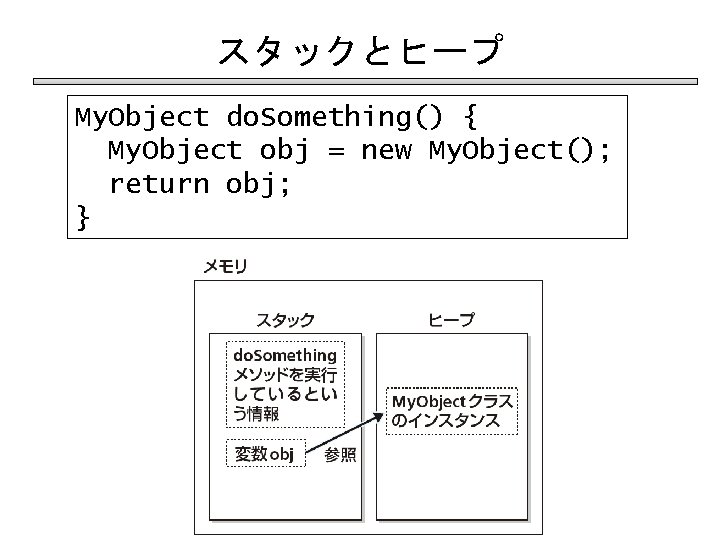
スタックとヒープ My. Object do. Something() { My. Object obj = new My. Object(); return obj; }
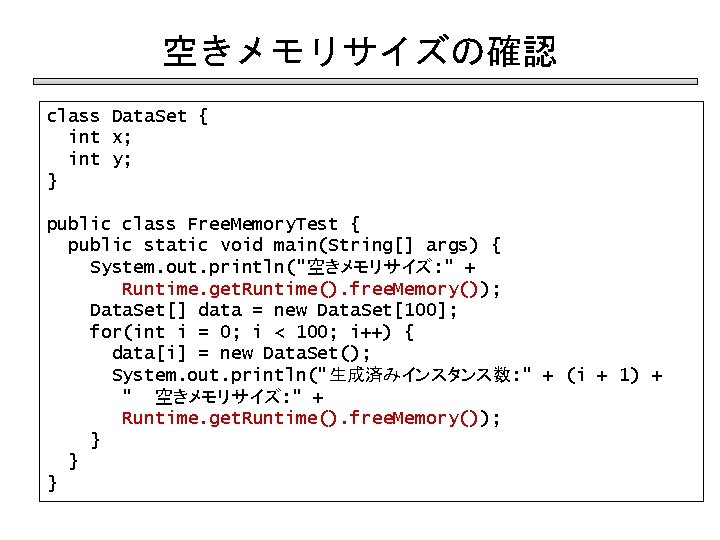
空きメモリサイズの確認 class Data. Set { int x; int y; } public class Free. Memory. Test { public static void main(String[] args) { System. out. println("空きメモリサイズ: " + Runtime. get. Runtime(). free. Memory()); Data. Set[] data = new Data. Set[100]; for(int i = 0; i < 100; i++) { data[i] = new Data. Set(); System. out. println("生成済みインスタンス数: " + (i + 1) + " 空きメモリサイズ: " + Runtime. get. Runtime(). free. Memory()); } } }
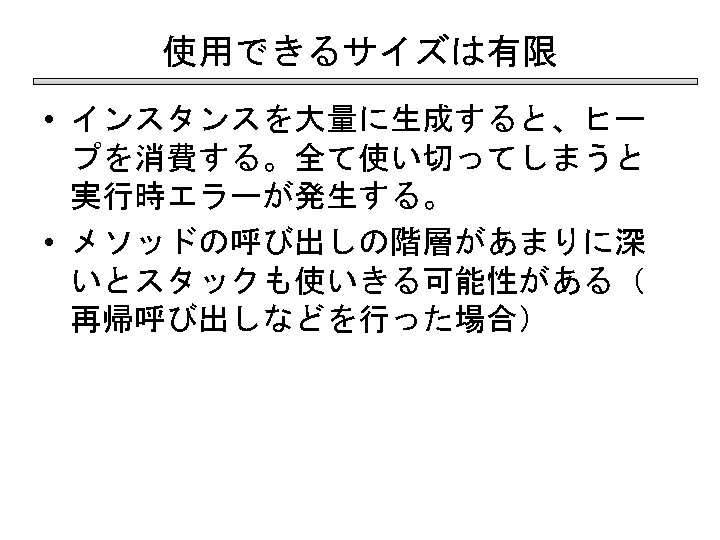
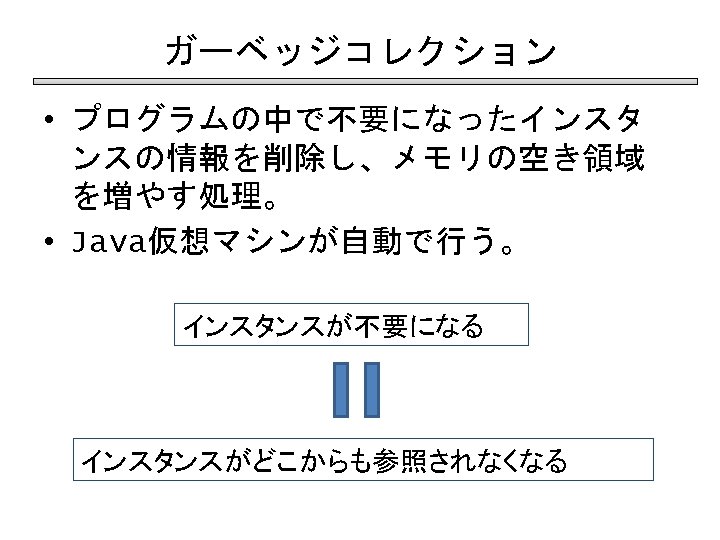
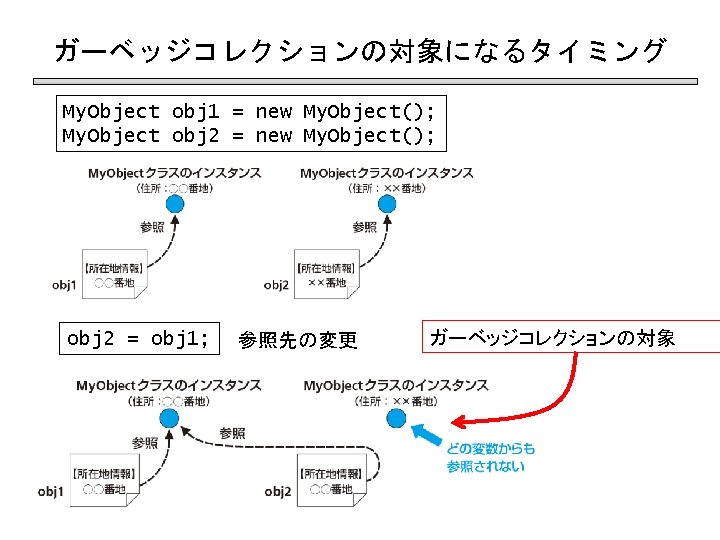
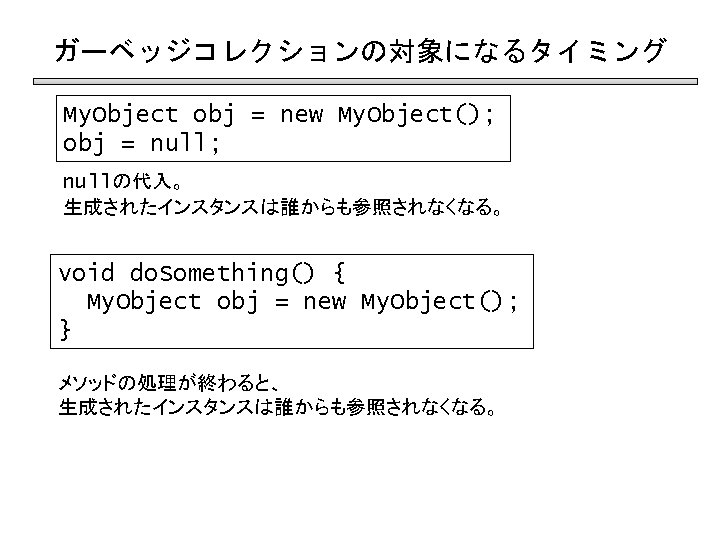
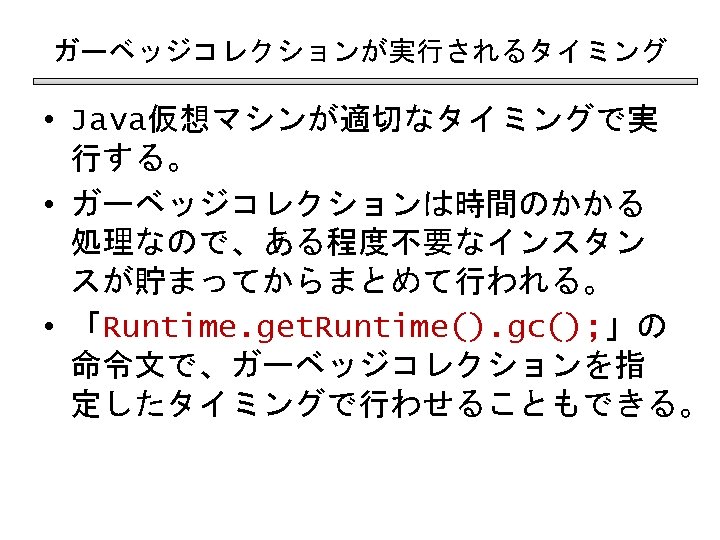
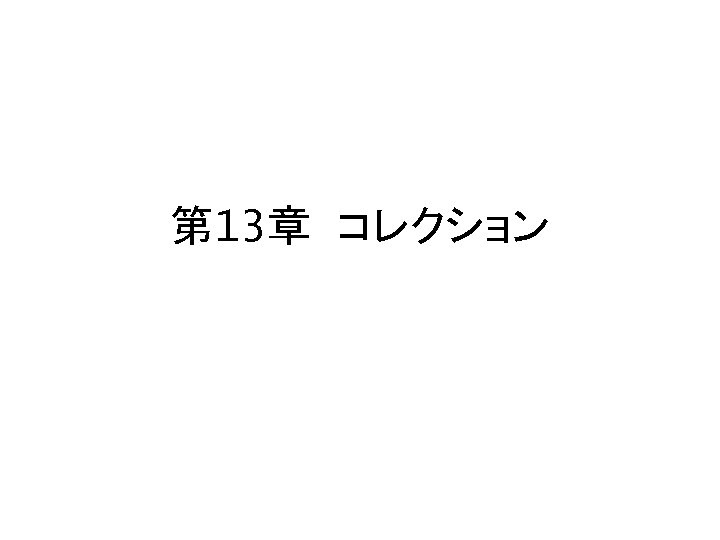
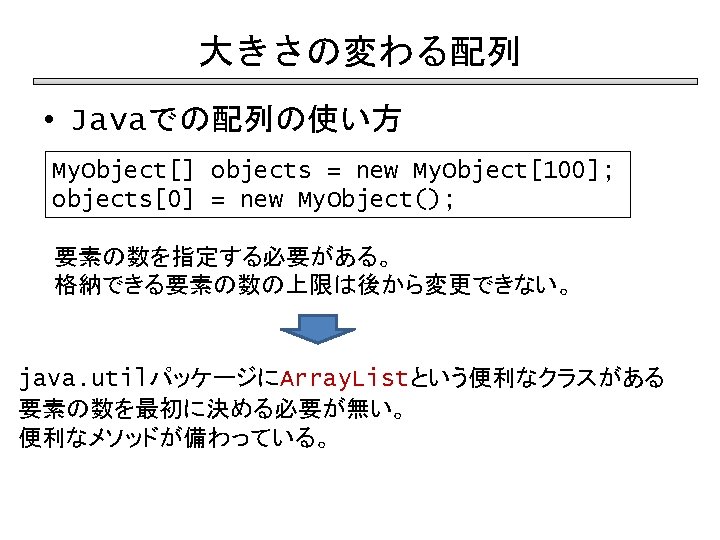
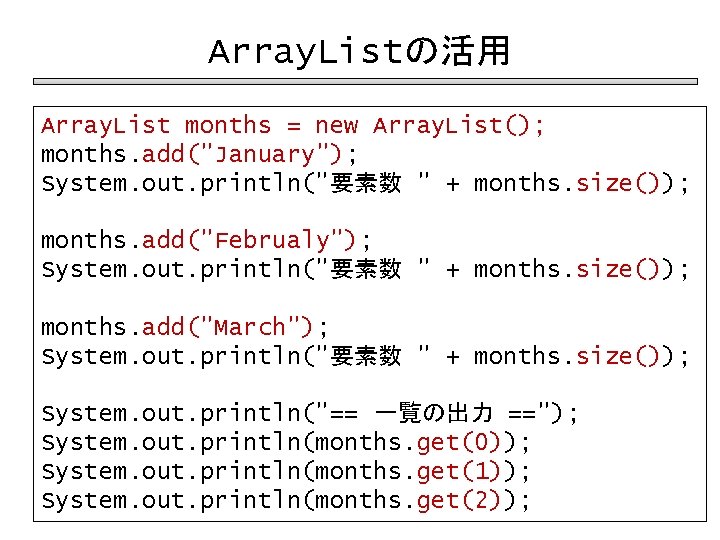
Array. Listの活用 Array. List months = new Array. List(); months. add("January"); System. out. println("要素数 " + months. size()); months. add("Februaly"); System. out. println("要素数 " + months. size()); months. add("March"); System. out. println("要素数 " + months. size()); System. out. println("== 一覧の出力 =="); System. out. println(months. get(0)); System. out. println(months. get(1)); System. out. println(months. get(2));
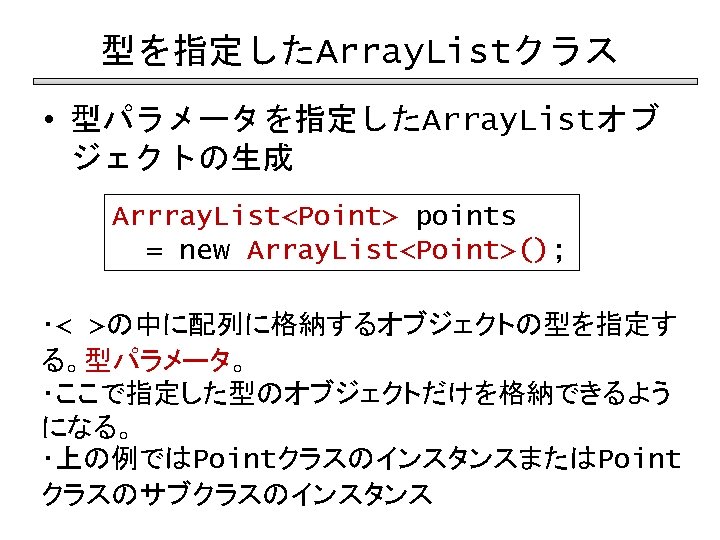
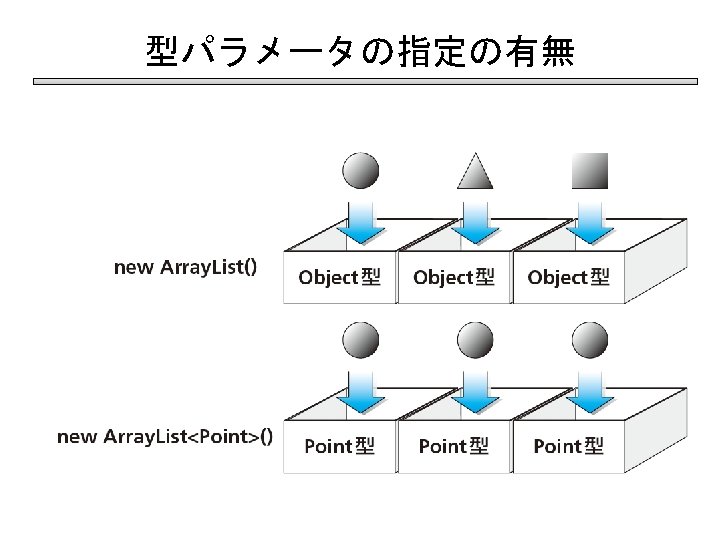
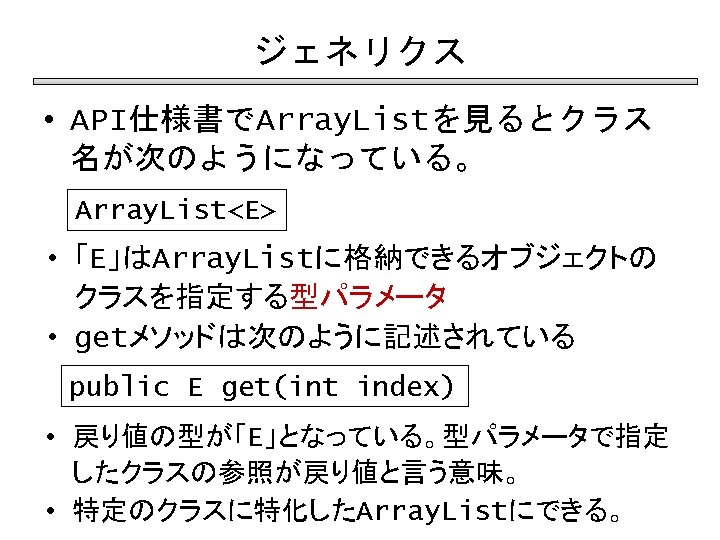
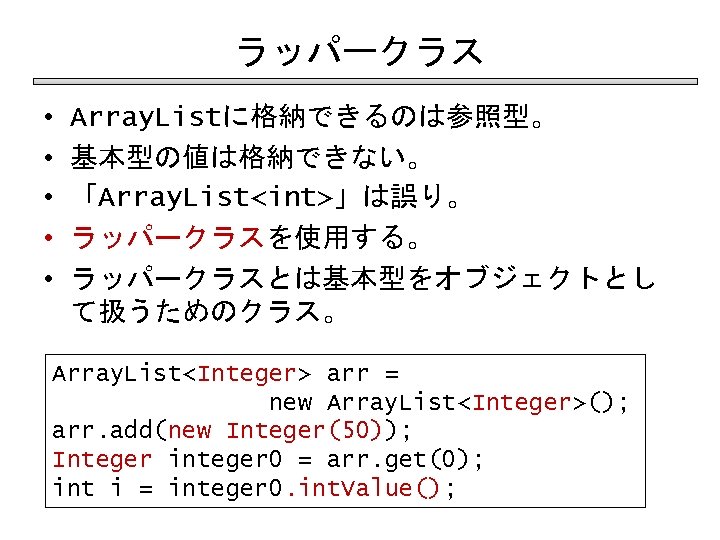
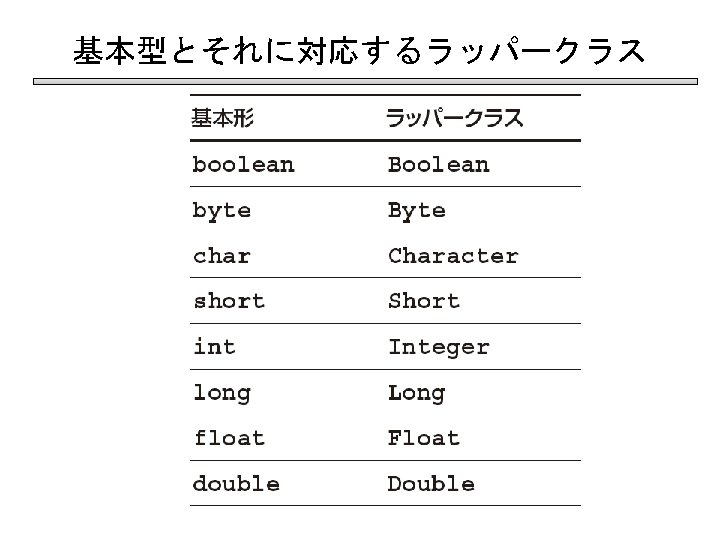
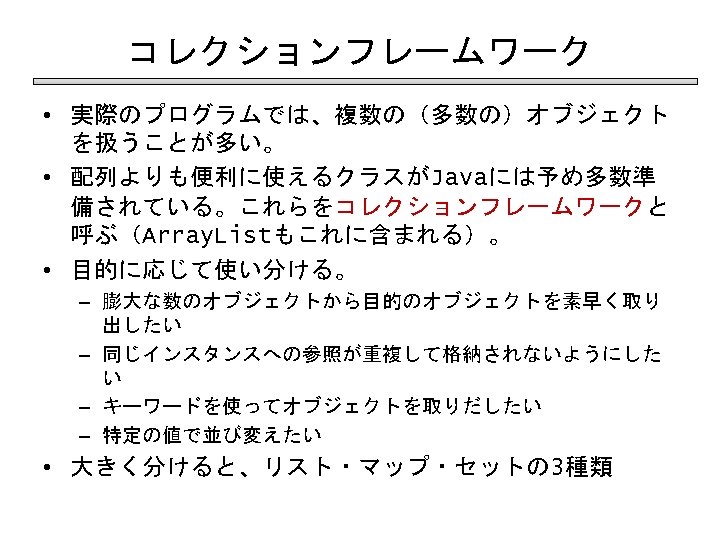
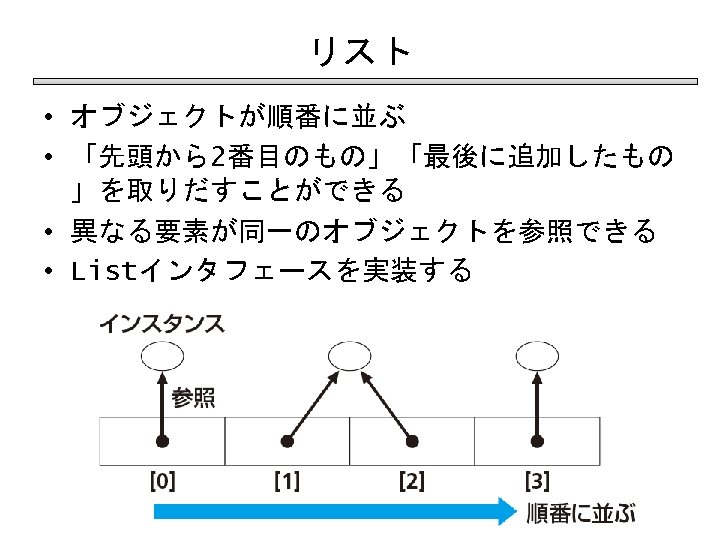
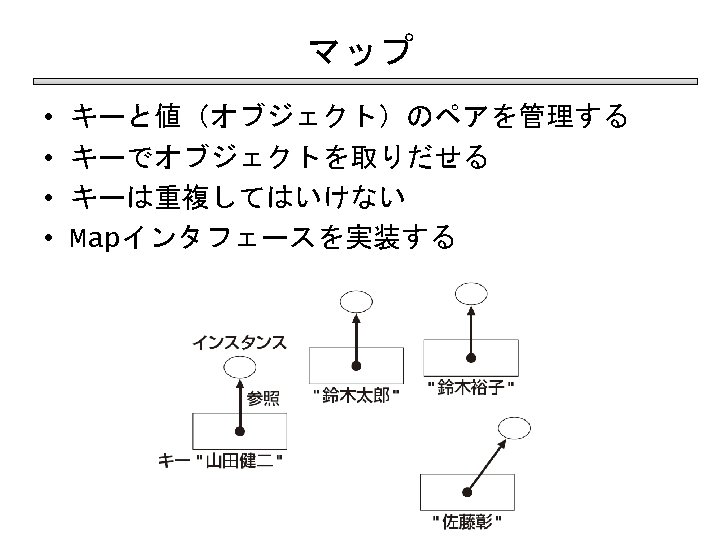
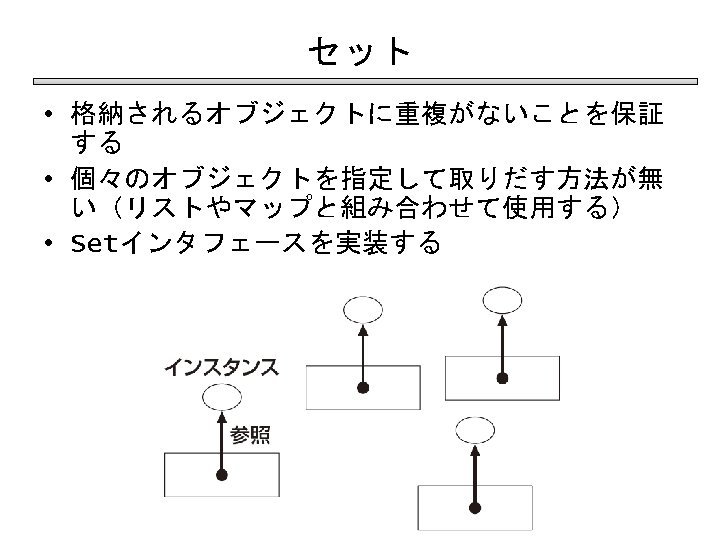
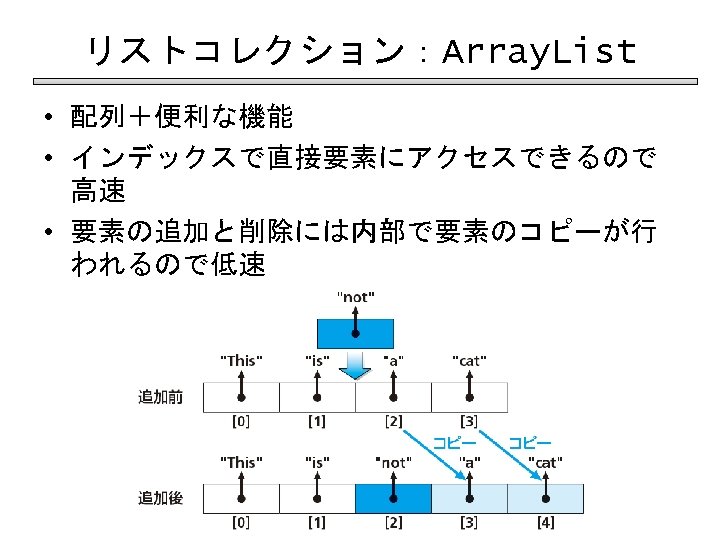
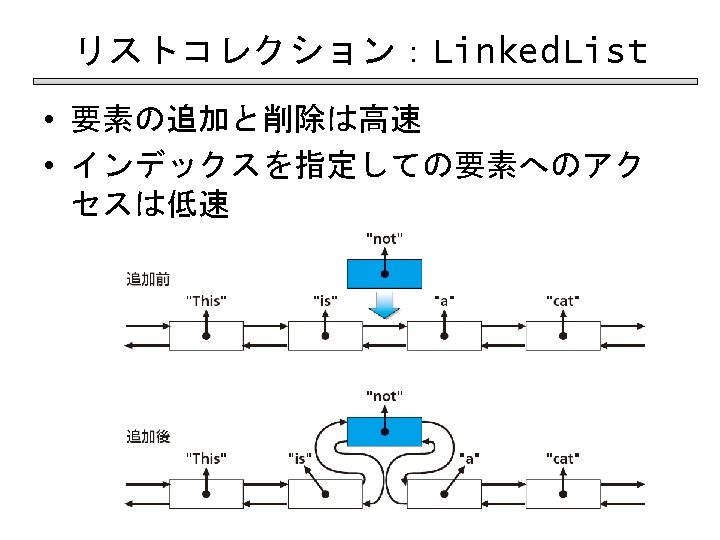
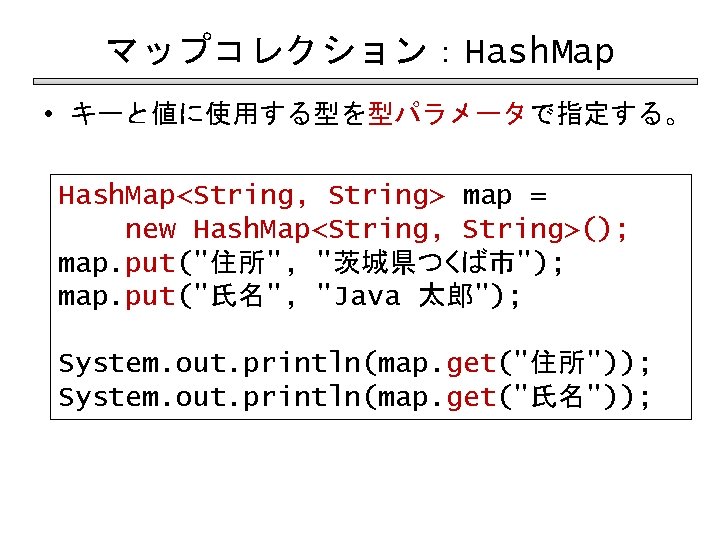
マップコレクション:Hash. Map • キーと値に使用する型を型パラメータで指定する。 Hash. Map<String, String> map = new Hash. Map<String, String>(); map. put("住所", "茨城県つくば市"); map. put("氏名", "Java 太郎"); System. out. println(map. get("住所")); System. out. println(map. get("氏名"));
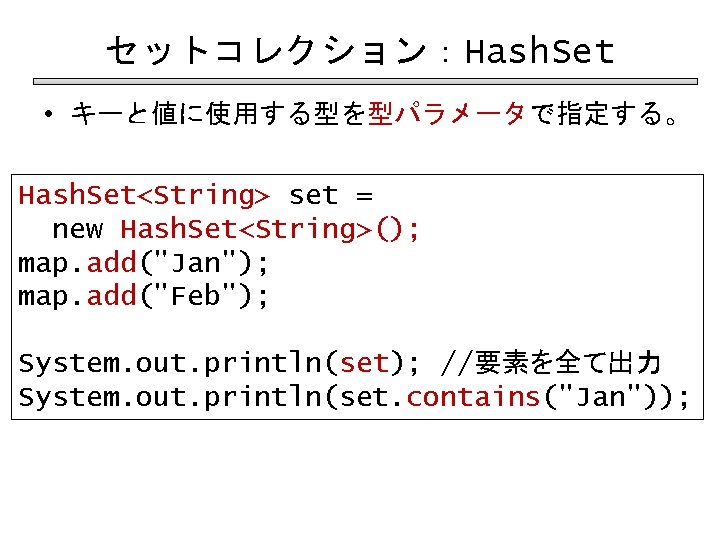
セットコレクション:Hash. Set • キーと値に使用する型を型パラメータで指定する。 Hash. Set<String> set = new Hash. Set<String>(); map. add("Jan"); map. add("Feb"); System. out. println(set); //要素を全て出力 System. out. println(set. contains("Jan"));
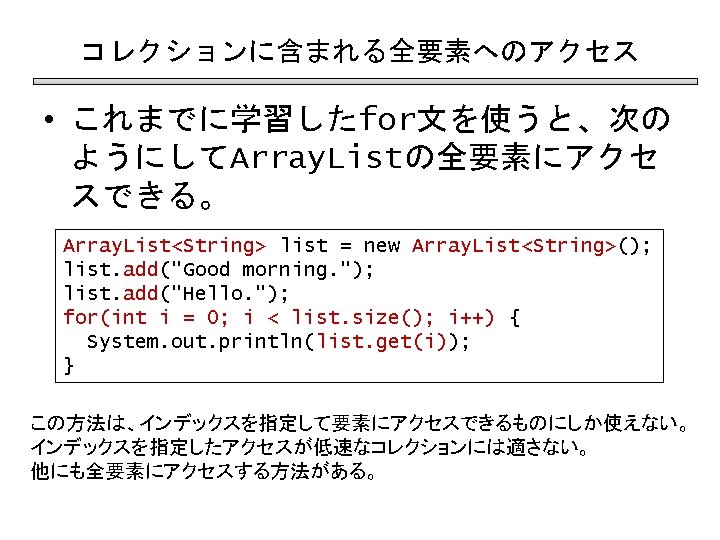
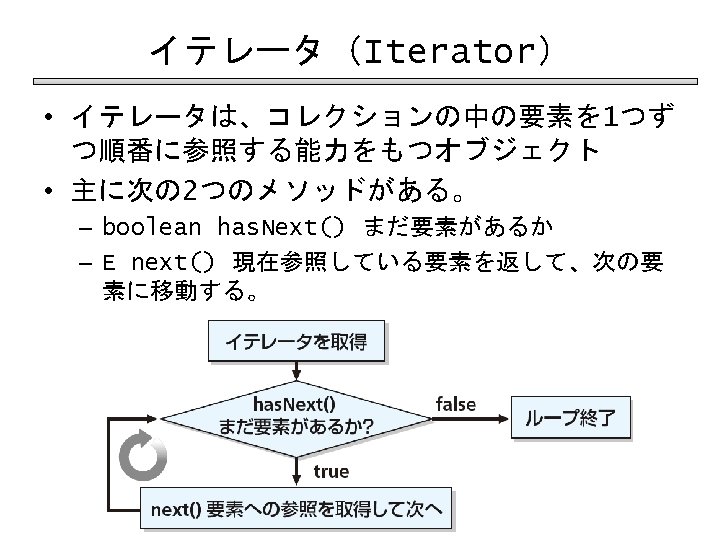
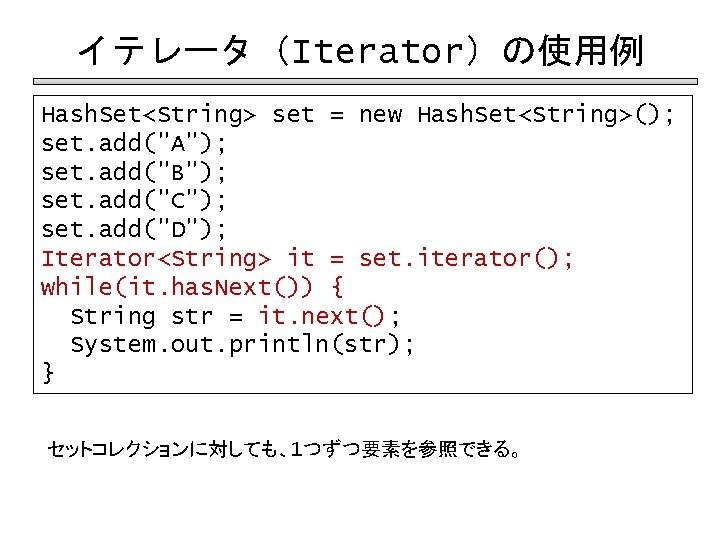
イテレータ(Iterator)の使用例 Hash. Set<String> set = new Hash. Set<String>(); set. add("A"); set. add("B"); set. add("C"); set. add("D"); Iterator<String> it = set. iterator(); while(it. has. Next()) { String str = it. next(); System. out. println(str); } セットコレクションに対しても、1つずつ要素を参照できる。
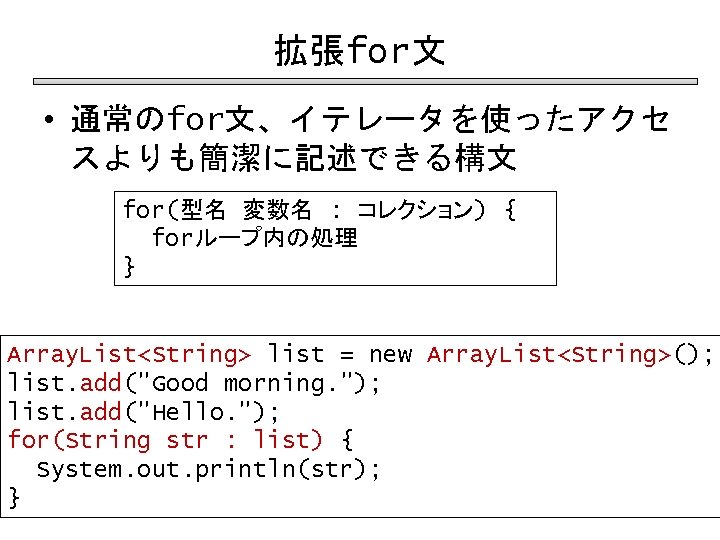
拡張for文 • 通常のfor文、イテレータを使ったアクセ スよりも簡潔に記述できる構文 for(型名 変数名 : コレクション) { forループ内の処理 } Array. List<String> list = new Array. List<String>(); list. add("Good morning. "); list. add("Hello. "); for(String str : list) { System. out. println(str); }
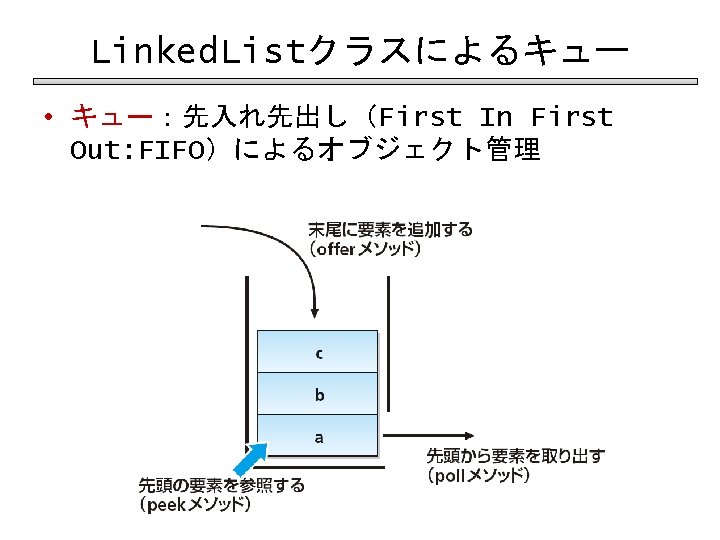
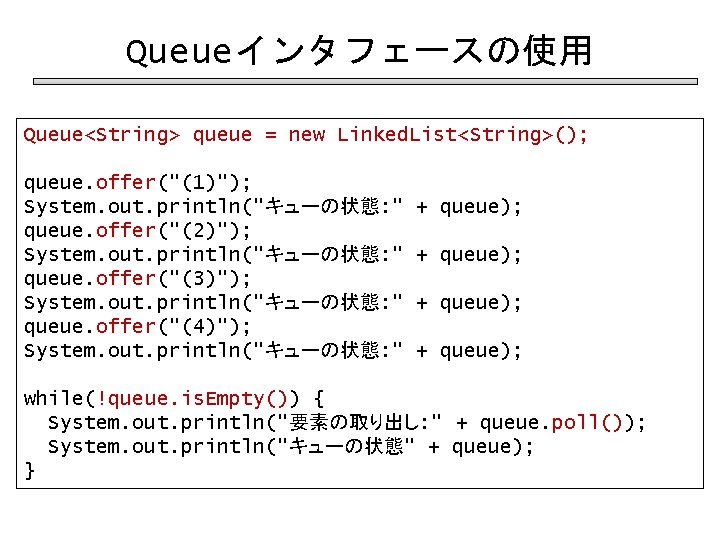
Queueインタフェースの使用 Queue<String> queue = new Linked. List<String>(); queue. offer("(1)"); System. out. println("キューの状態: " queue. offer("(2)"); System. out. println("キューの状態: " queue. offer("(3)"); System. out. println("キューの状態: " queue. offer("(4)"); System. out. println("キューの状態: " + queue); while(!queue. is. Empty()) { System. out. println("要素の取り出し: " + queue. poll()); System. out. println("キューの状態" + queue); }
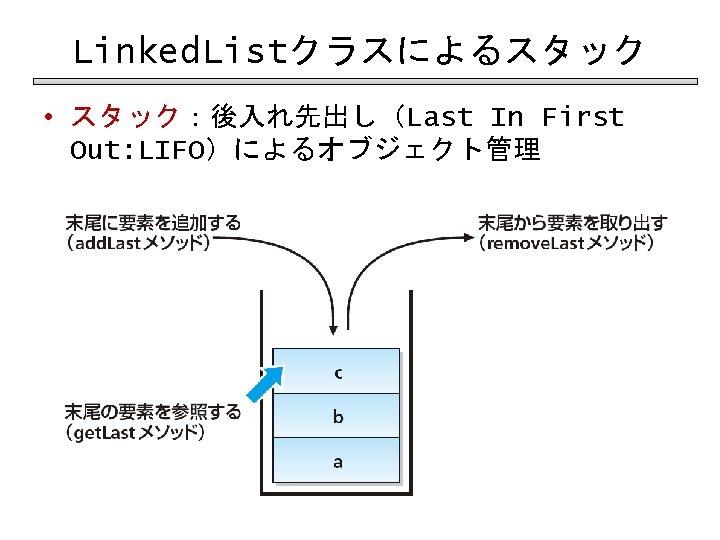
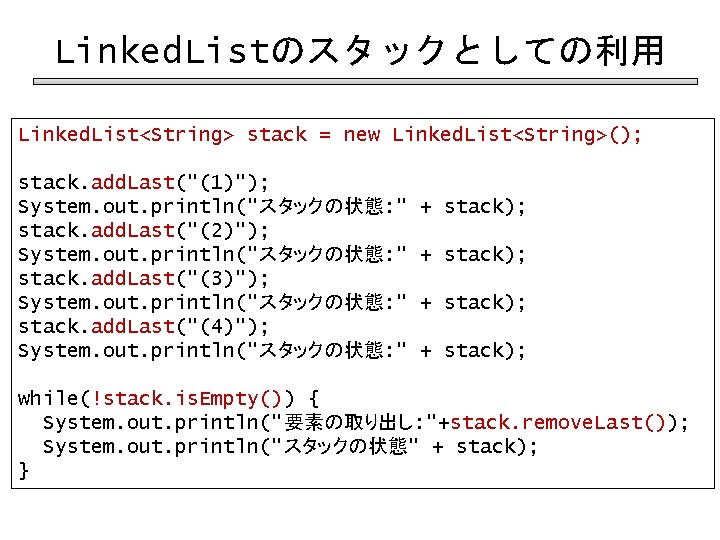
Linked. Listのスタックとしての利用 Linked. List<String> stack = new Linked. List<String>(); stack. add. Last("(1)"); System. out. println("スタックの状態: " stack. add. Last("(2)"); System. out. println("スタックの状態: " stack. add. Last("(3)"); System. out. println("スタックの状態: " stack. add. Last("(4)"); System. out. println("スタックの状態: " + stack); while(!stack. is. Empty()) { System. out. println("要素の取り出し: "+stack. remove. Last()); System. out. println("スタックの状態" + stack); }
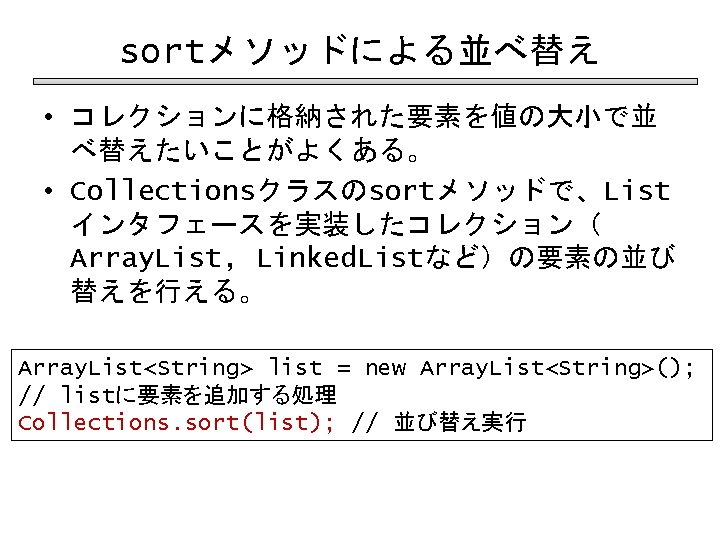
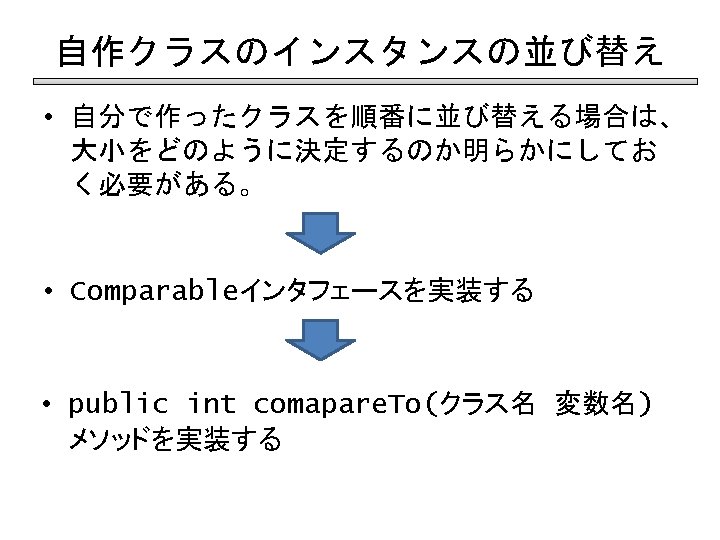
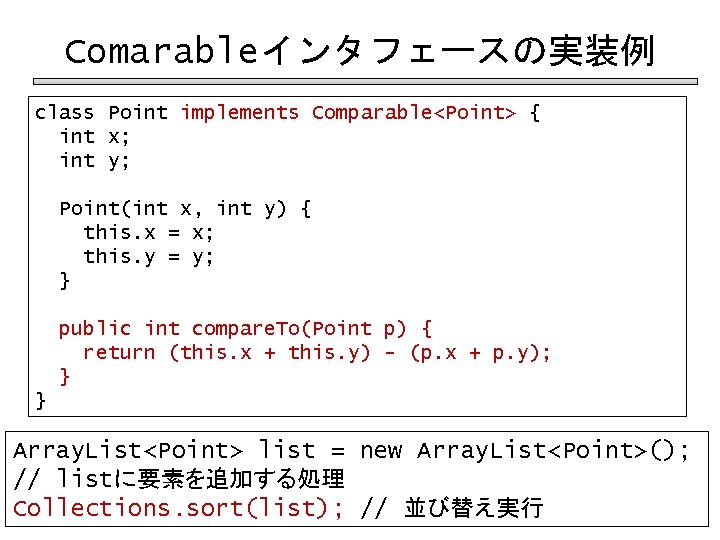
Comarableインタフェースの実装例 class Point implements Comparable<Point> { int x; int y; Point(int x, int y) { this. x = x; this. y = y; } public int compare. To(Point p) { return (this. x + this. y) - (p. x + p. y); } } Array. List<Point> list = new Array. List<Point>(); // listに要素を追加する処理 Collections. sort(list); // 並び替え実行
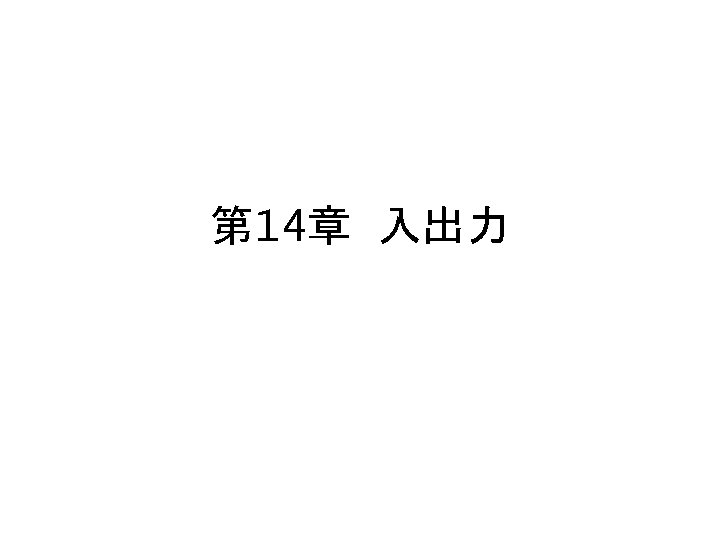
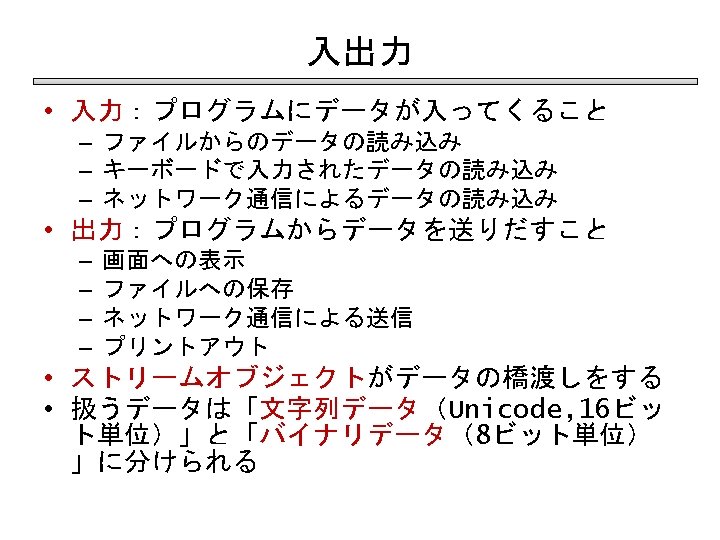
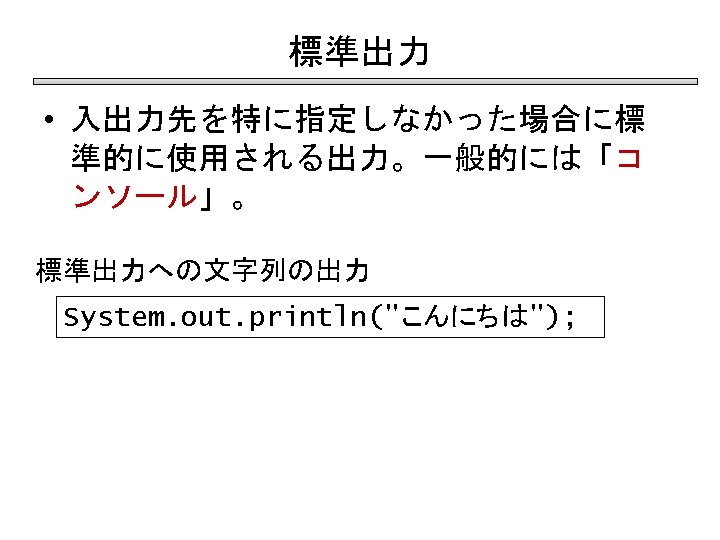
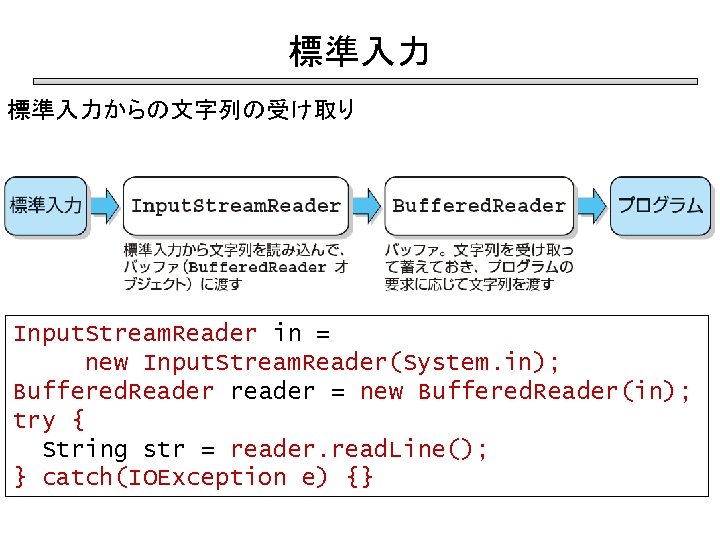
標準入力からの文字列の受け取り Input. Stream. Reader in = new Input. Stream. Reader(System. in); Buffered. Reader reader = new Buffered. Reader(in); try { String str = reader. read. Line(); } catch(IOException e) {}
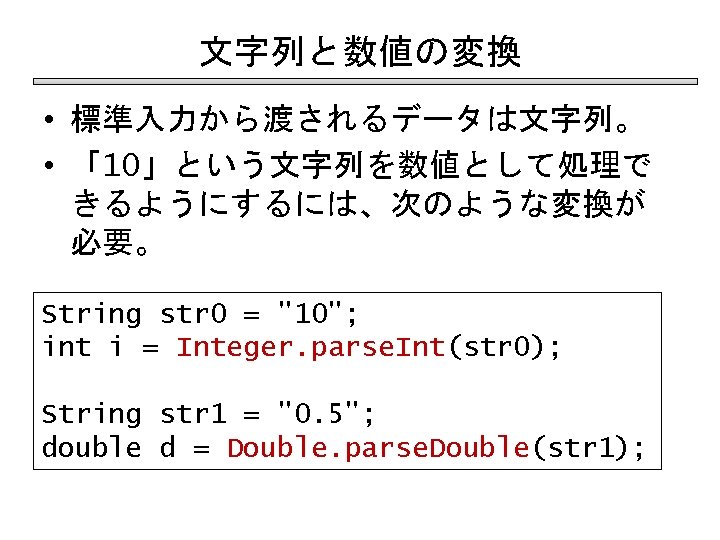
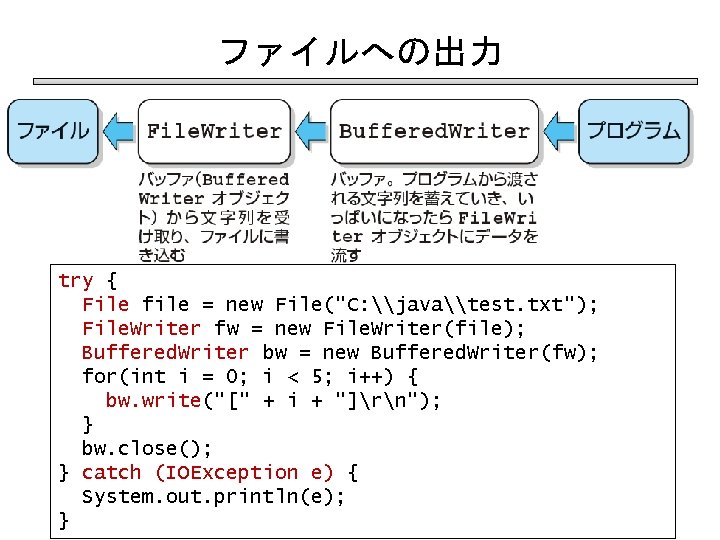
ファイルへの出力 try { File file = new File("C: \java\test. txt"); File. Writer fw = new File. Writer(file); Buffered. Writer bw = new Buffered. Writer(fw); for(int i = 0; i < 5; i++) { bw. write("[" + i + "]rn"); } bw. close(); } catch (IOException e) { System. out. println(e); }
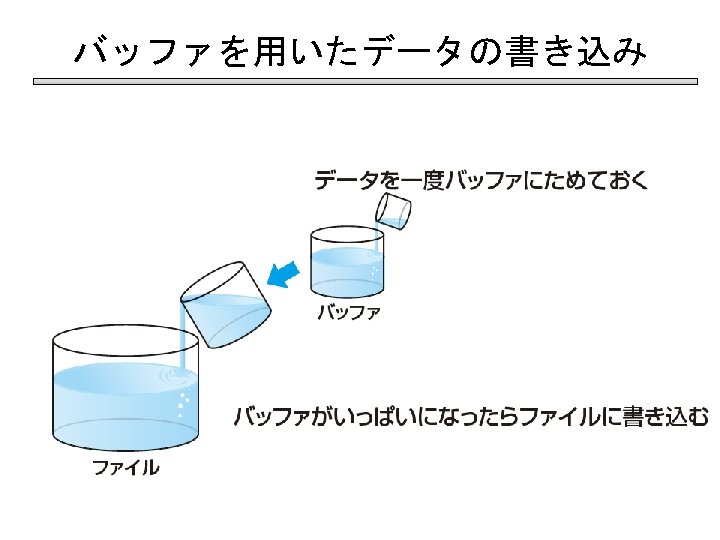
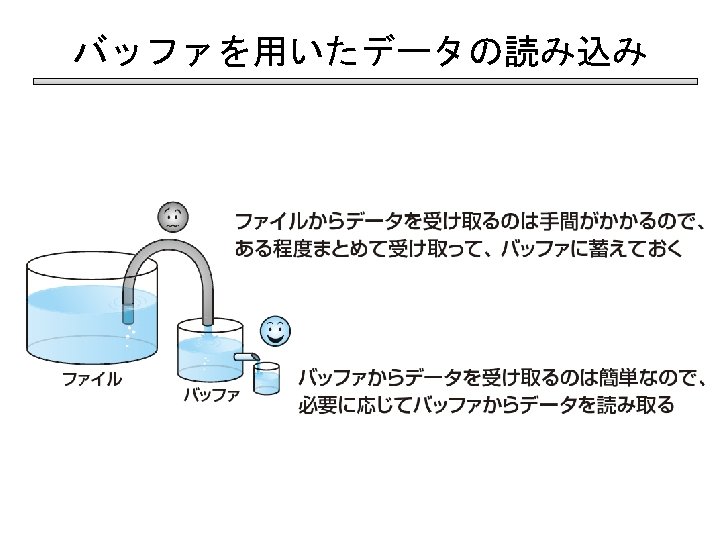
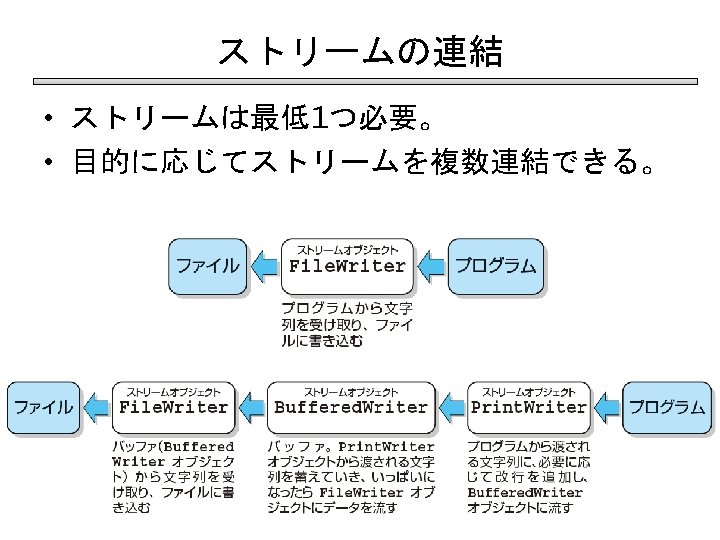
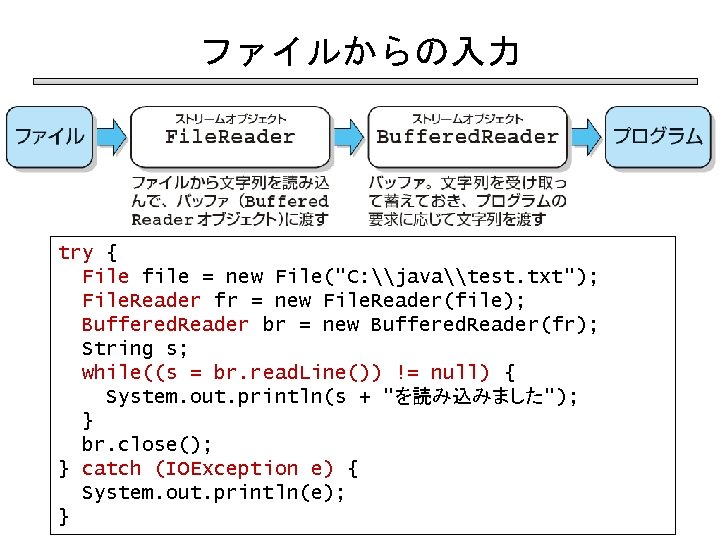
ファイルからの入力 try { File file = new File("C: \java\test. txt"); File. Reader fr = new File. Reader(file); Buffered. Reader br = new Buffered. Reader(fr); String s; while((s = br. read. Line()) != null) { System. out. println(s + "を読み込みました"); } br. close(); } catch (IOException e) { System. out. println(e); }
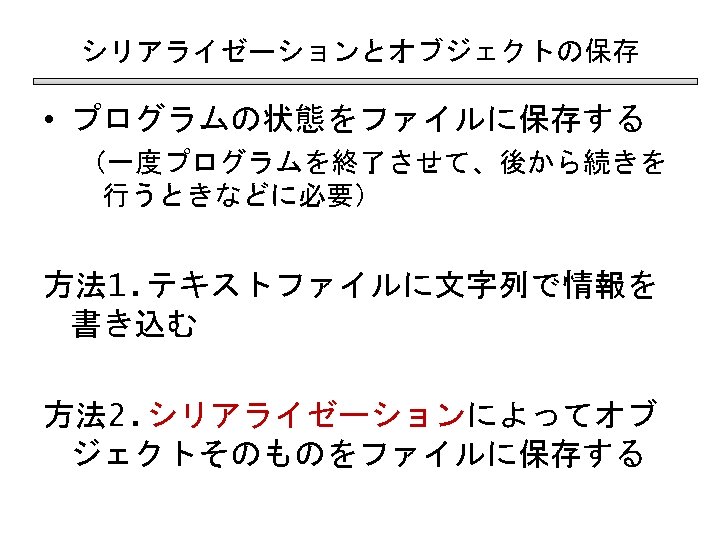
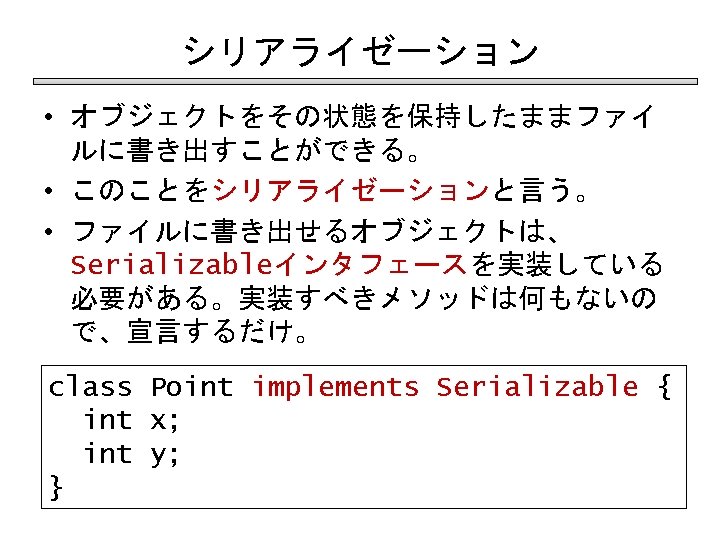
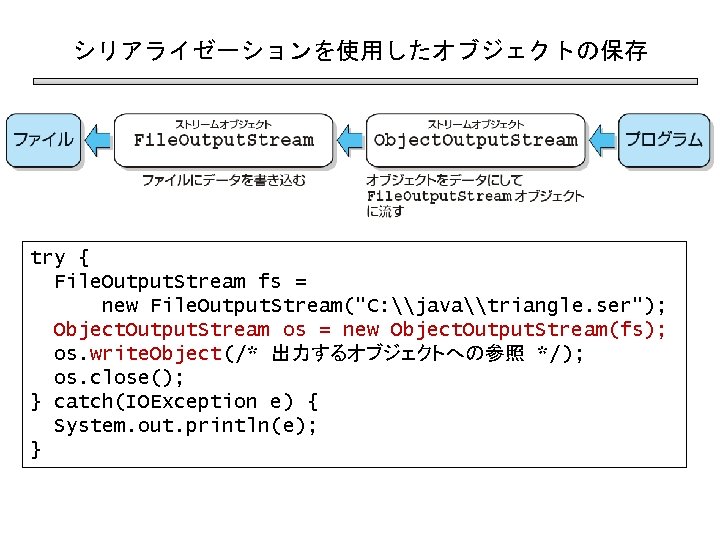
シリアライゼーションを使用したオブジェクトの保存 try { File. Output. Stream fs = new File. Output. Stream("C: \java\triangle. ser"); Object. Output. Stream os = new Object. Output. Stream(fs); os. write. Object(/* 出力するオブジェクトへの参照 */); os. close(); } catch(IOException e) { System. out. println(e); }
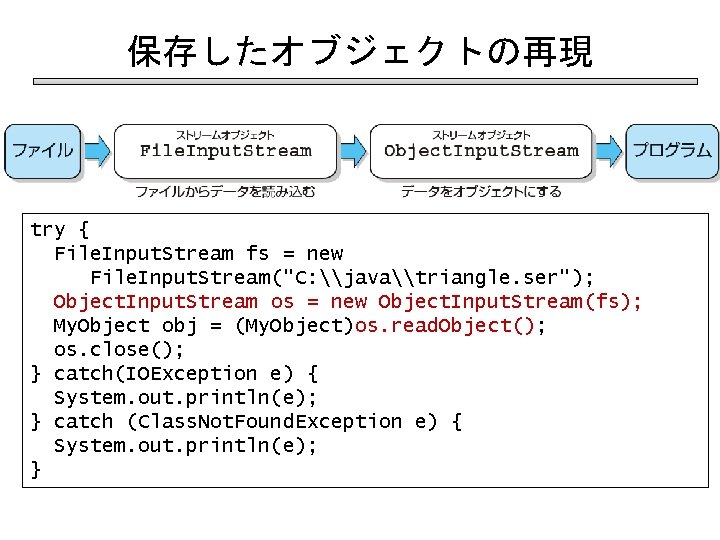
保存したオブジェクトの再現 try { File. Input. Stream fs = new File. Input. Stream("C: \java\triangle. ser"); Object. Input. Stream os = new Object. Input. Stream(fs); My. Object obj = (My. Object)os. read. Object(); os. close(); } catch(IOException e) { System. out. println(e); } catch (Class. Not. Found. Exception e) { System. out. println(e); }
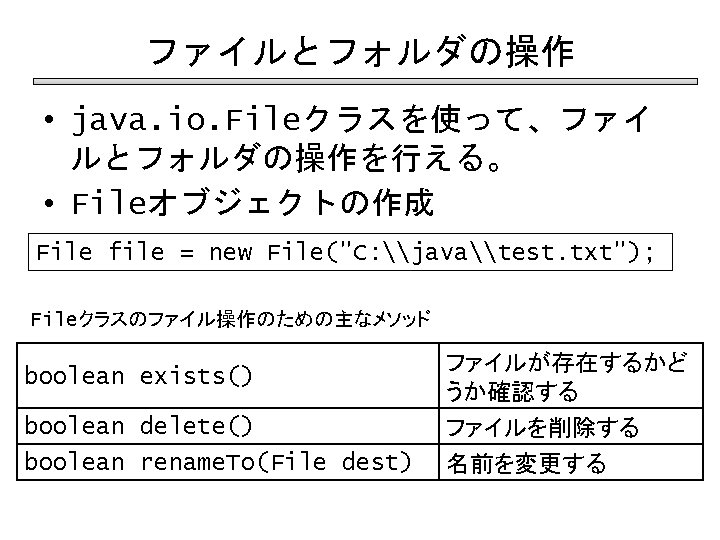
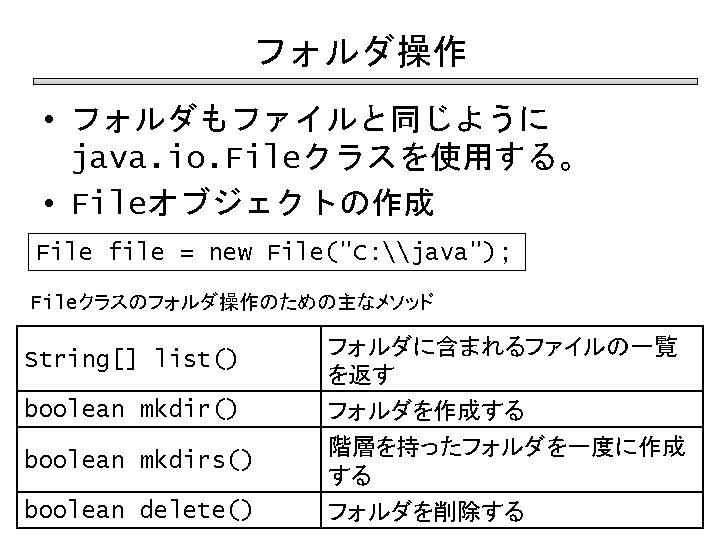
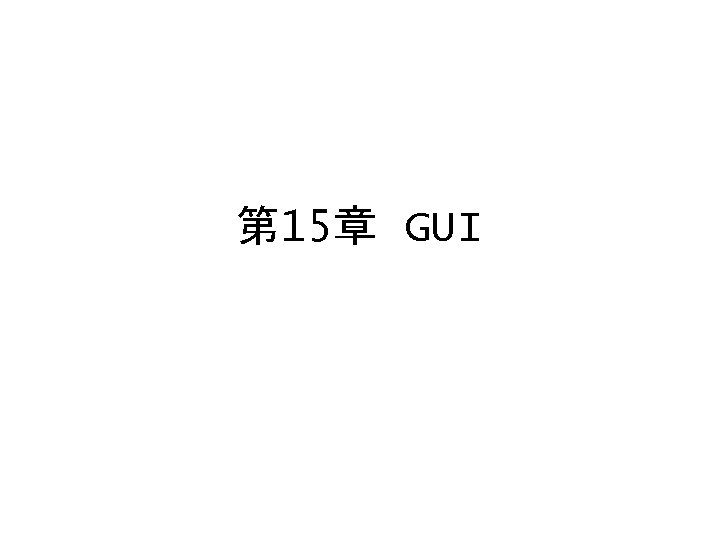
第 15章 GUI
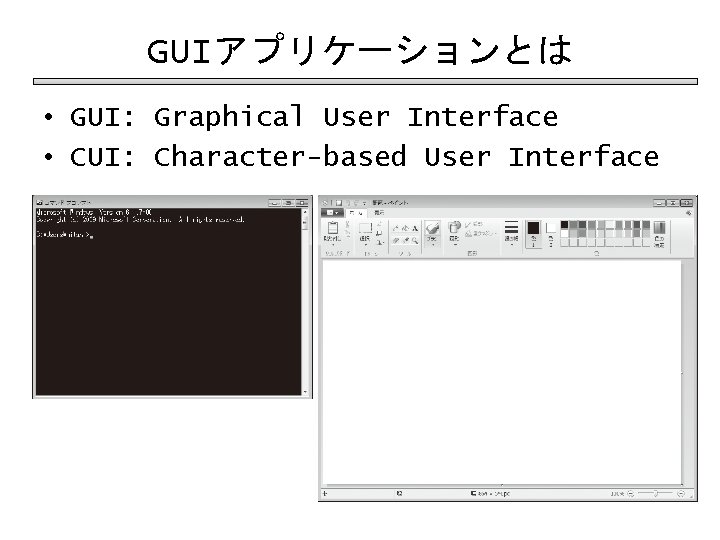
GUIアプリケーションとは • GUI: Graphical User Interface • CUI: Character-based User Interface
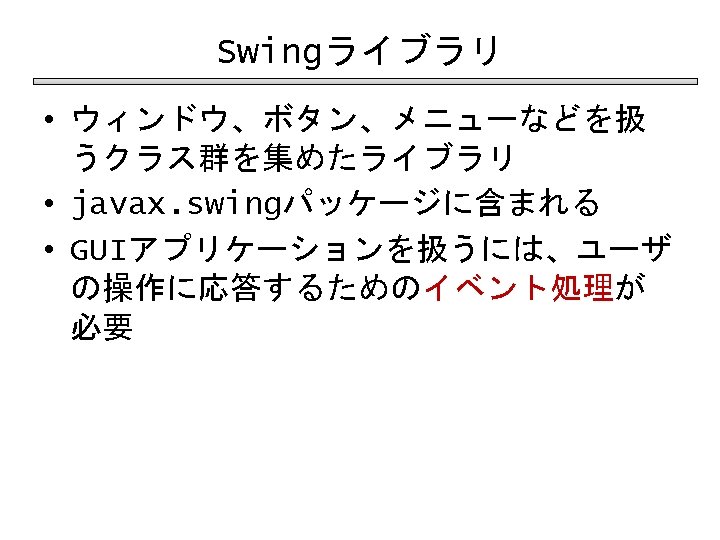
![フレームの作成 import javax swing class Simple Frame Example public static void mainString フレームの作成 import javax. swing. *; class Simple. Frame. Example { public static void main(String[]](https://slidetodoc.com/presentation_image_h/28f443ab3a5efa9f8a41f33031ca7c02/image-116.jpg)
フレームの作成 import javax. swing. *; class Simple. Frame. Example { public static void main(String[] args) { JFrame frame = new JFrame(); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); frame. set. Size(300, 200); frame. set. Visible(true); } }
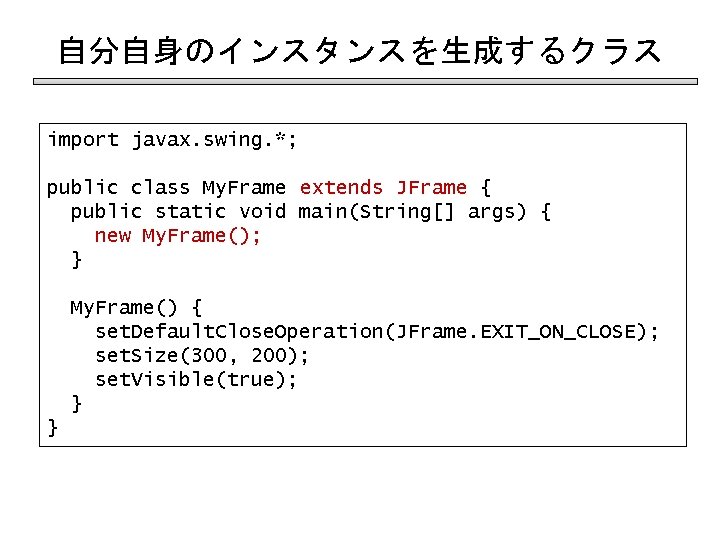
自分自身のインスタンスを生成するクラス import javax. swing. *; public class My. Frame extends JFrame { public static void main(String[] args) { new My. Frame(); } My. Frame() { set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); set. Size(300, 200); set. Visible(true); } }
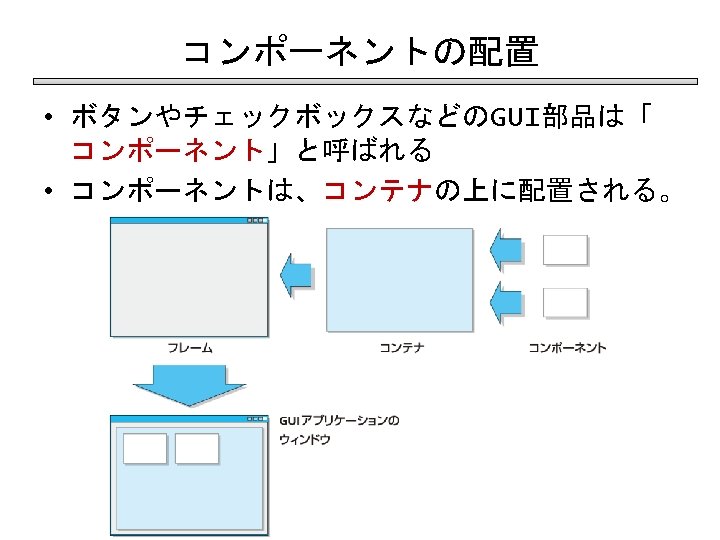
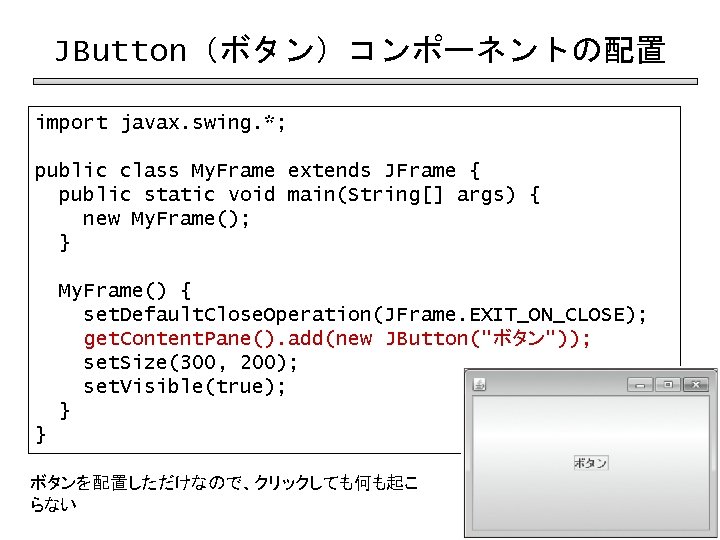
JButton(ボタン)コンポーネントの配置 import javax. swing. *; public class My. Frame extends JFrame { public static void main(String[] args) { new My. Frame(); } My. Frame() { set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); get. Content. Pane(). add(new JButton("ボタン")); set. Size(300, 200); set. Visible(true); } } ボタンを配置しただけなので、クリックしても何も起こ らない
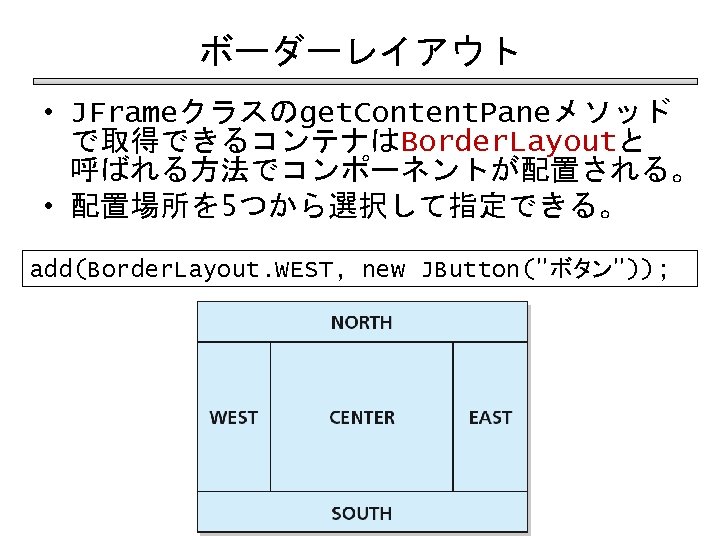
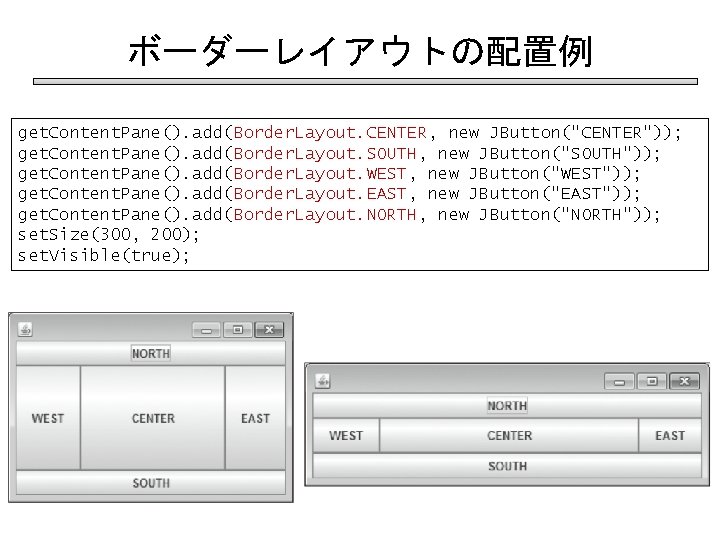
ボーダーレイアウトの配置例 get. Content. Pane(). add(Border. Layout. CENTER, new JButton("CENTER")); get. Content. Pane(). add(Border. Layout. SOUTH, new JButton("SOUTH")); get. Content. Pane(). add(Border. Layout. WEST, new JButton("WEST")); get. Content. Pane(). add(Border. Layout. EAST, new JButton("EAST")); get. Content. Pane(). add(Border. Layout. NORTH, new JButton("NORTH")); set. Size(300, 200); set. Visible(true);
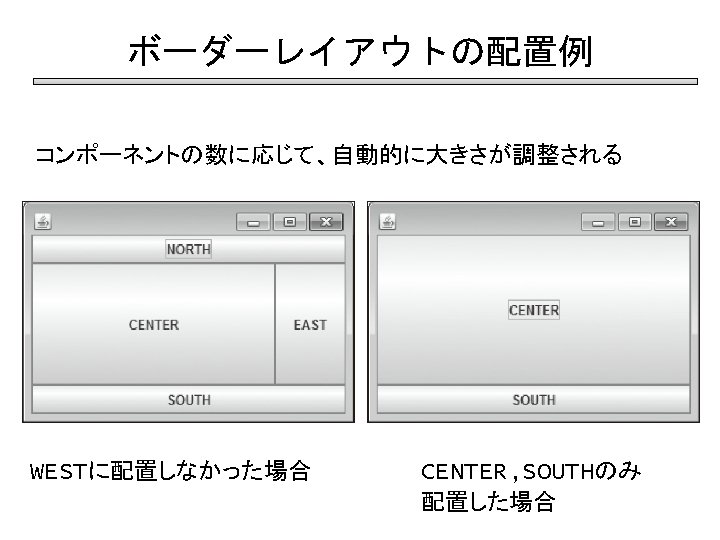
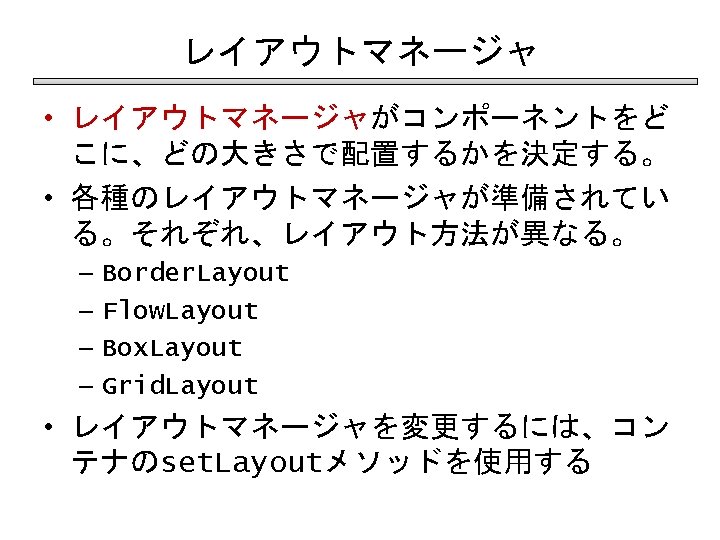
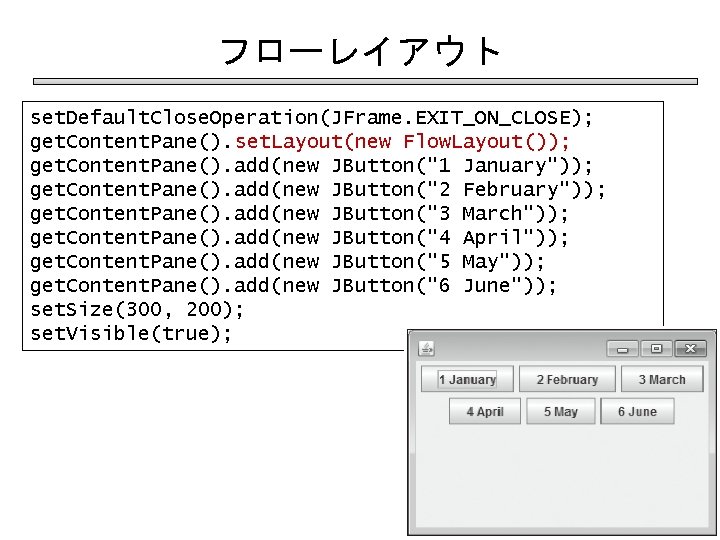
フローレイアウト set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); get. Content. Pane(). set. Layout(new Flow. Layout()); get. Content. Pane(). add(new JButton("1 January")); get. Content. Pane(). add(new JButton("2 February")); get. Content. Pane(). add(new JButton("3 March")); get. Content. Pane(). add(new JButton("4 April")); get. Content. Pane(). add(new JButton("5 May")); get. Content. Pane(). add(new JButton("6 June")); set. Size(300, 200); set. Visible(true);
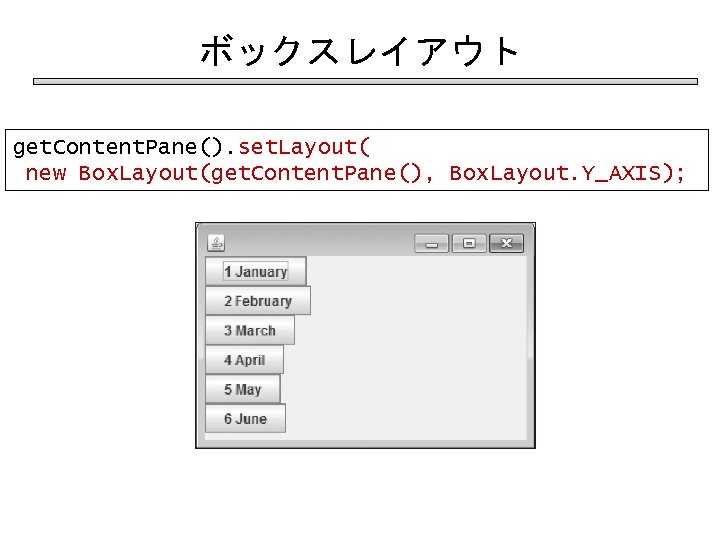
ボックスレイアウト get. Content. Pane(). set. Layout( new Box. Layout(get. Content. Pane(), Box. Layout. Y_AXIS);
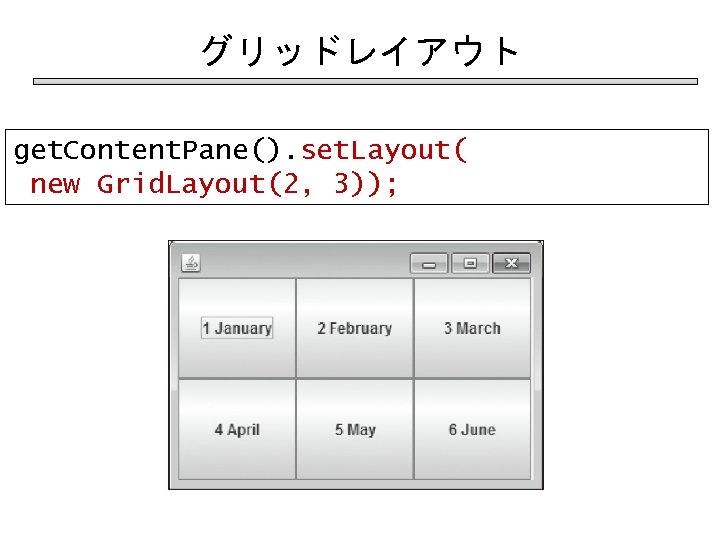
グリッドレイアウト get. Content. Pane(). set. Layout( new Grid. Layout(2, 3));
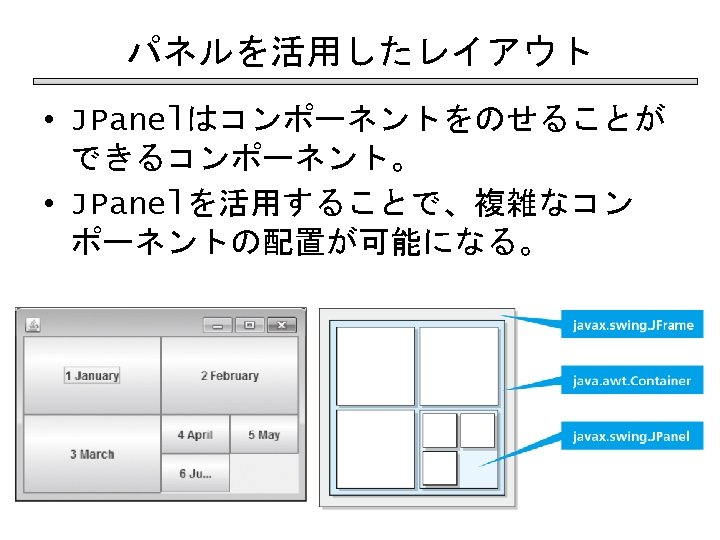
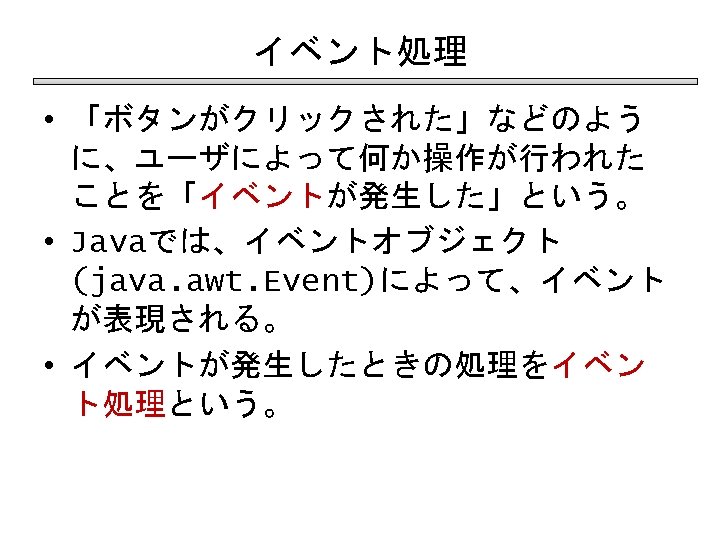
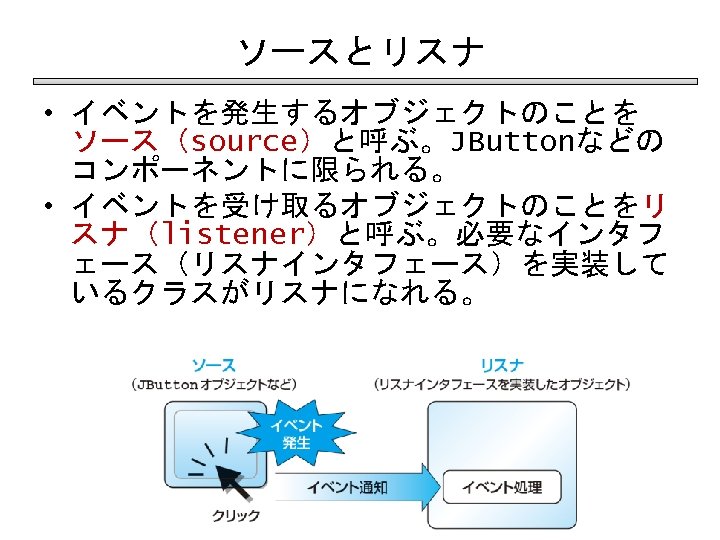
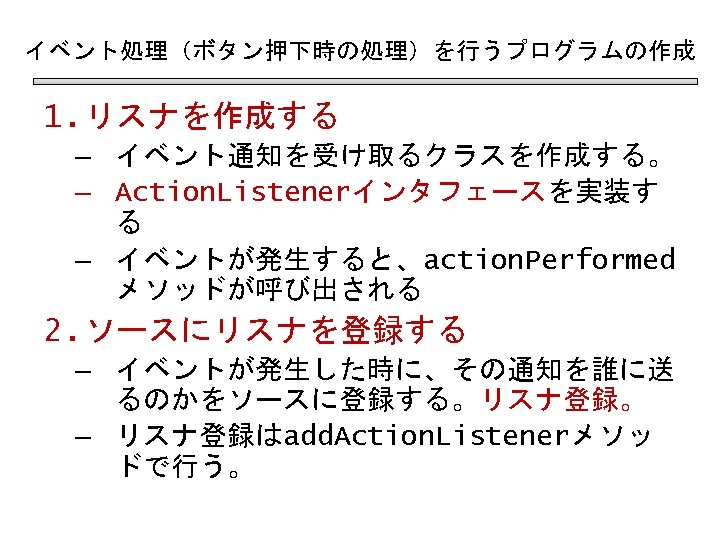
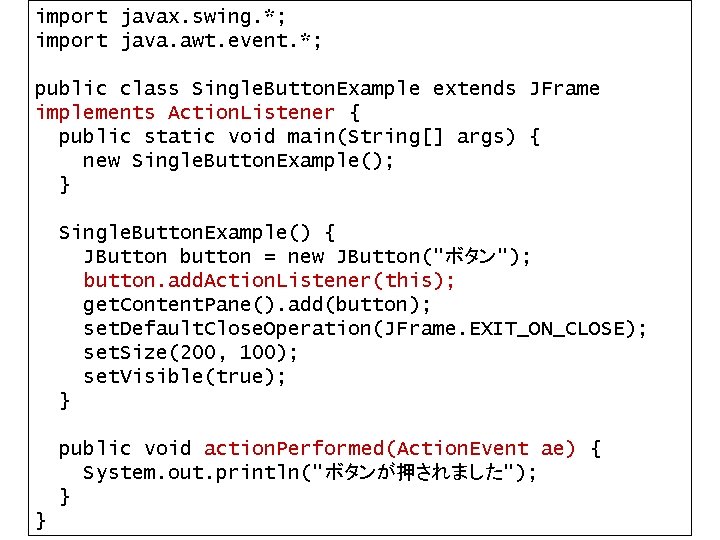
import javax. swing. *; import java. awt. event. *; public class Single. Button. Example extends JFrame implements Action. Listener { public static void main(String[] args) { new Single. Button. Example(); } Single. Button. Example() { JButton button = new JButton("ボタン"); button. add. Action. Listener(this); get. Content. Pane(). add(button); set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); set. Size(200, 100); set. Visible(true); } public void action. Performed(Action. Event ae) { System. out. println("ボタンが押されました"); } }
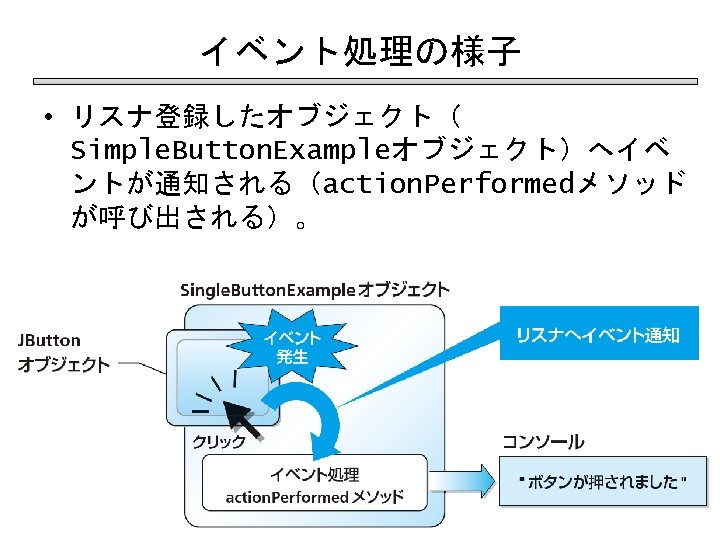
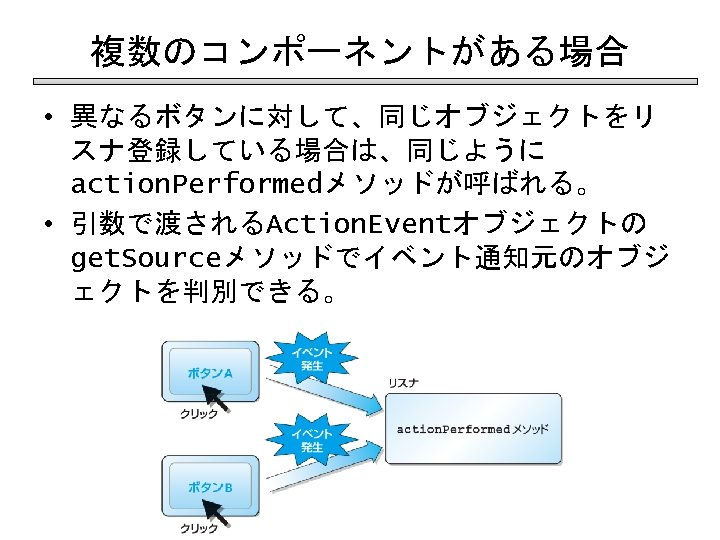
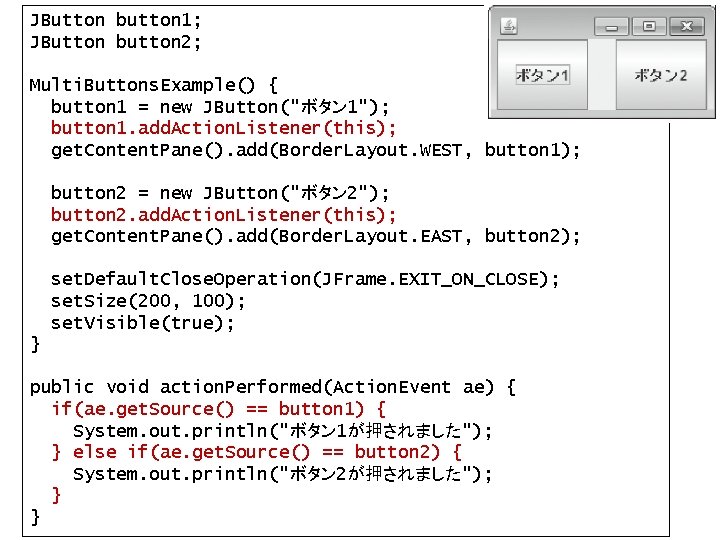
JButton button 1; JButton button 2; Multi. Buttons. Example() { button 1 = new JButton("ボタン 1"); button 1. add. Action. Listener(this); get. Content. Pane(). add(Border. Layout. WEST, button 1); button 2 = new JButton("ボタン 2"); button 2. add. Action. Listener(this); get. Content. Pane(). add(Border. Layout. EAST, button 2); set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); set. Size(200, 100); set. Visible(true); } public void action. Performed(Action. Event ae) { if(ae. get. Source() == button 1) { System. out. println("ボタン 1が押されました"); } else if(ae. get. Source() == button 2) { System. out. println("ボタン 2が押されました"); } }
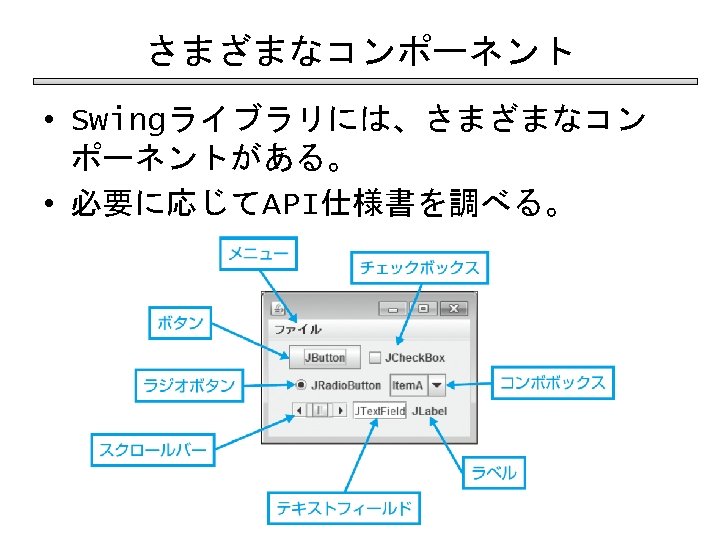
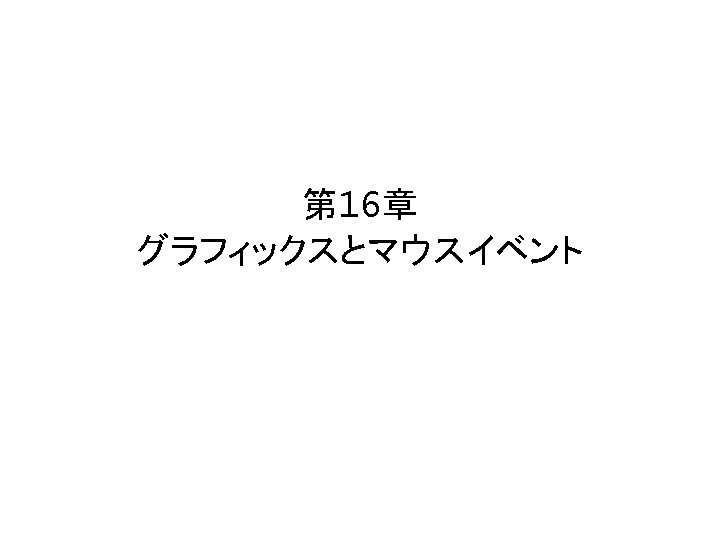
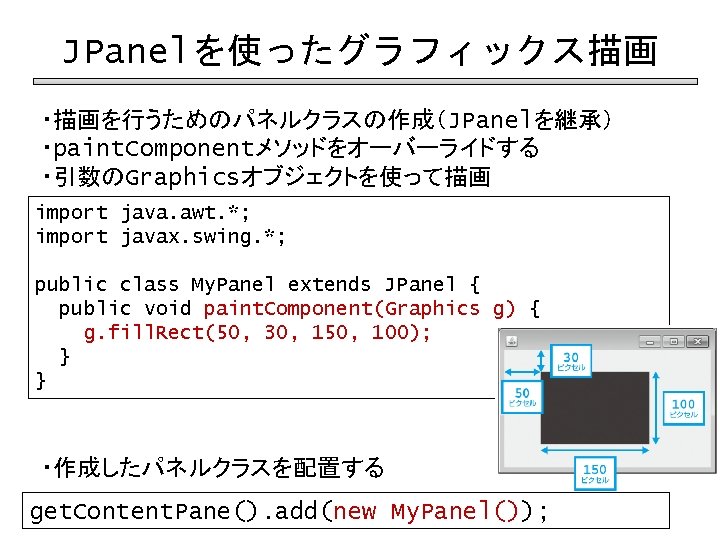
JPanelを使ったグラフィックス描画 ・描画を行うためのパネルクラスの作成(JPanelを継承) ・paint. Componentメソッドをオーバーライドする ・引数のGraphicsオブジェクトを使って描画 import java. awt. *; import javax. swing. *; public class My. Panel extends JPanel { public void paint. Component(Graphics g) { g. fill. Rect(50, 30, 150, 100); } } ・作成したパネルクラスを配置する get. Content. Pane(). add(new My. Panel());
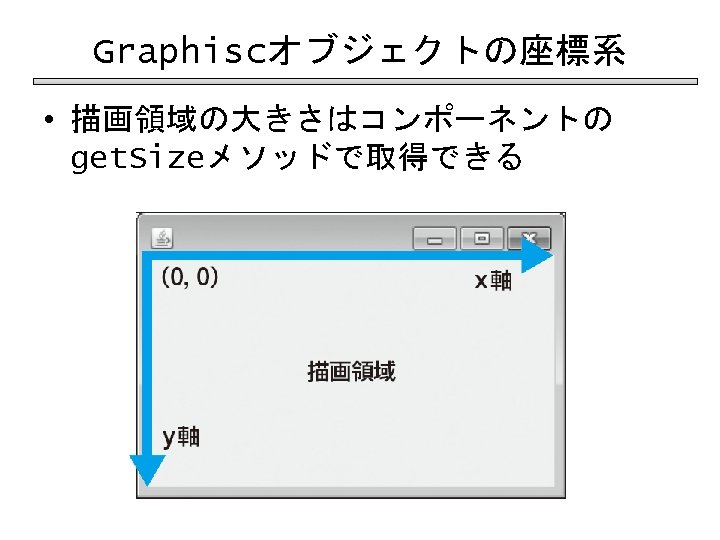
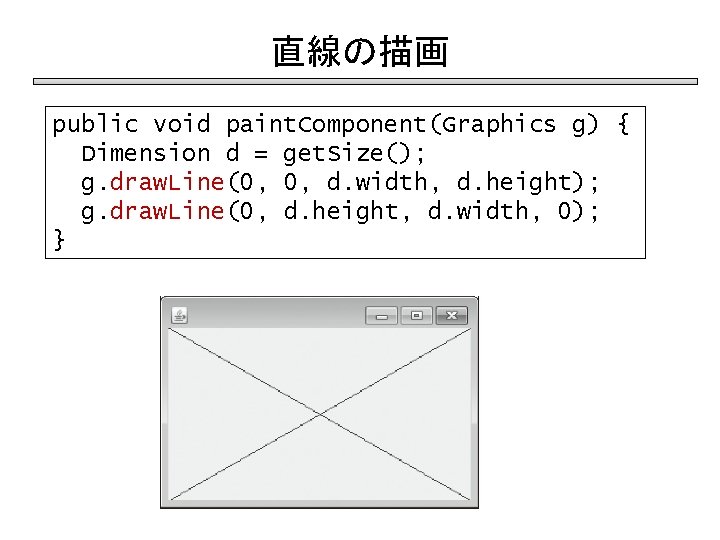
直線の描画 public void paint. Component(Graphics g) { Dimension d = get. Size(); g. draw. Line(0, 0, d. width, d. height); g. draw. Line(0, d. height, d. width, 0); }
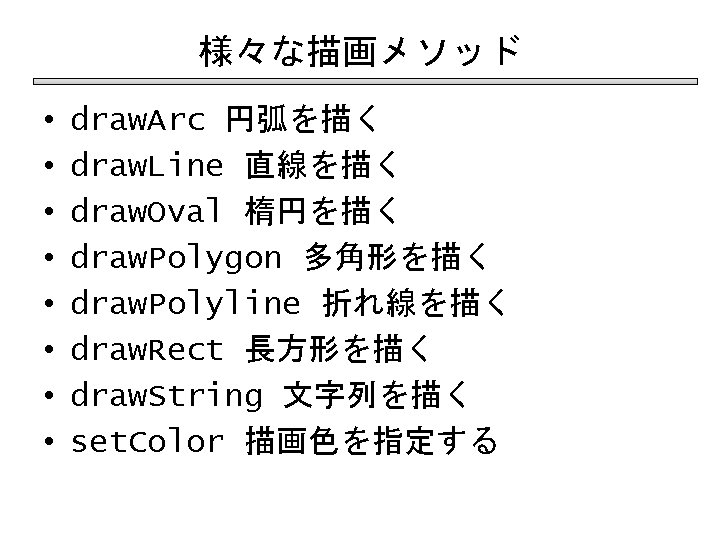
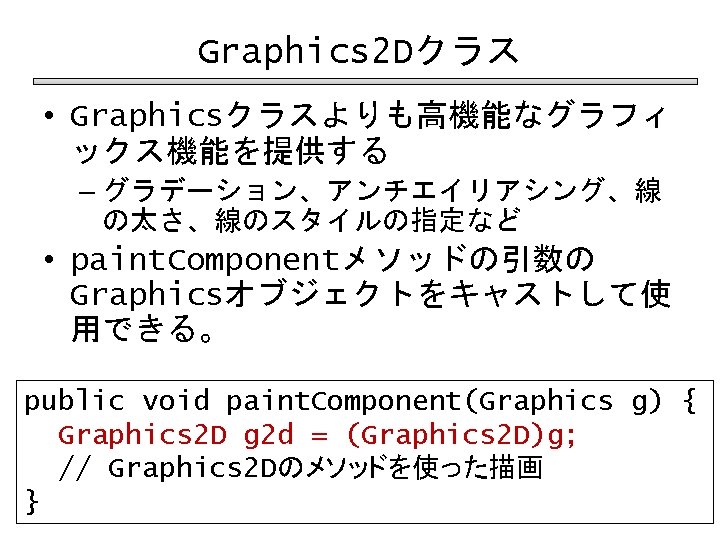
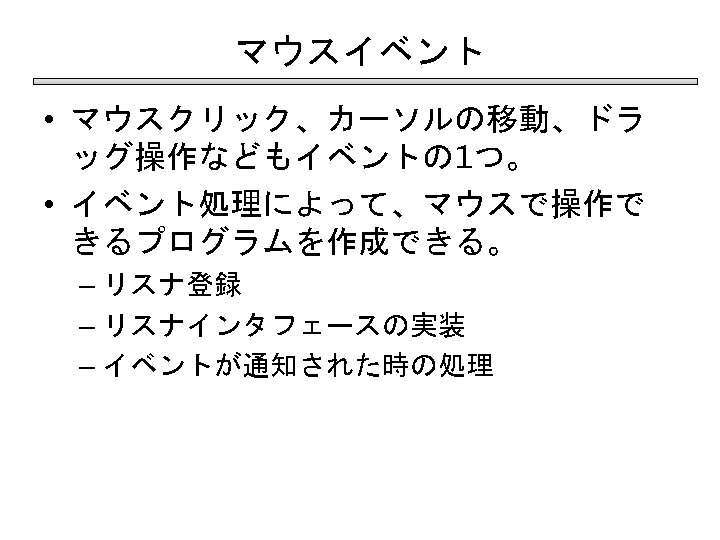
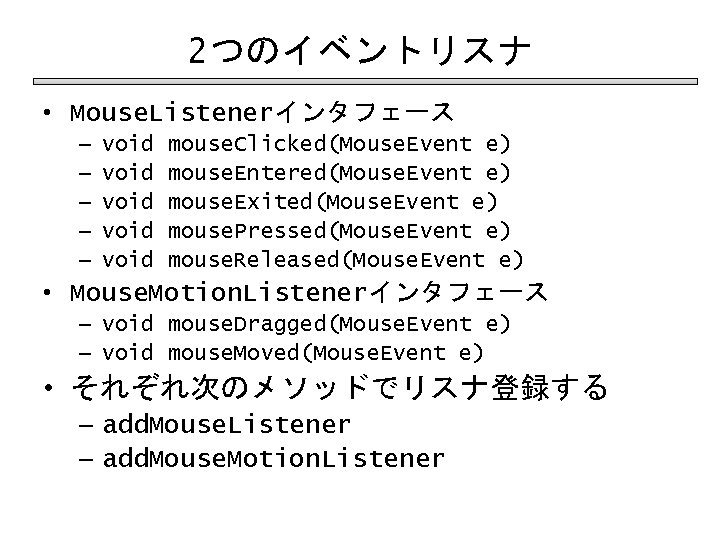
2つのイベントリスナ • Mouse. Listenerインタフェース – – – void void mouse. Clicked(Mouse. Event e) mouse. Entered(Mouse. Event e) mouse. Exited(Mouse. Event e) mouse. Pressed(Mouse. Event e) mouse. Released(Mouse. Event e) • Mouse. Motion. Listenerインタフェース – void mouse. Dragged(Mouse. Event e) – void mouse. Moved(Mouse. Event e) • それぞれ次のメソッドでリスナ登録する – add. Mouse. Listener – add. Mouse. Motion. Listener
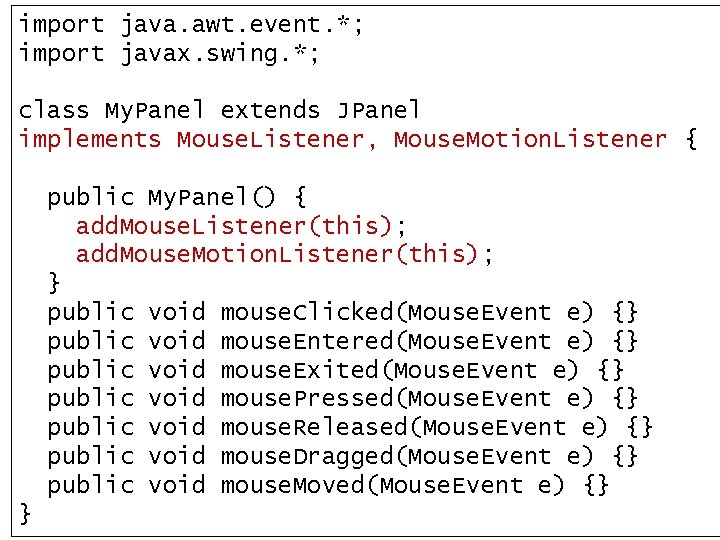
import java. awt. event. *; import javax. swing. *; class My. Panel extends JPanel implements Mouse. Listener, Mouse. Motion. Listener { public My. Panel() { add. Mouse. Listener(this); add. Mouse. Motion. Listener(this); } public void mouse. Clicked(Mouse. Event e) {} public void mouse. Entered(Mouse. Event e) {} public void mouse. Exited(Mouse. Event e) {} public void mouse. Pressed(Mouse. Event e) {} public void mouse. Released(Mouse. Event e) {} public void mouse. Dragged(Mouse. Event e) {} public void mouse. Moved(Mouse. Event e) {} }
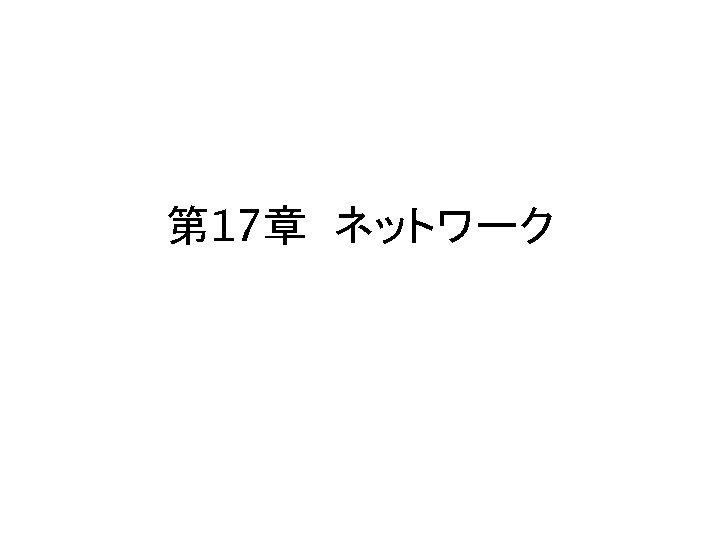
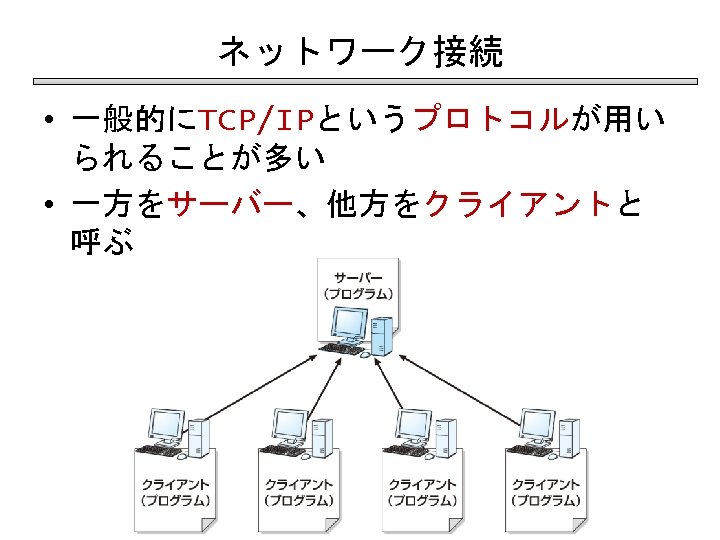
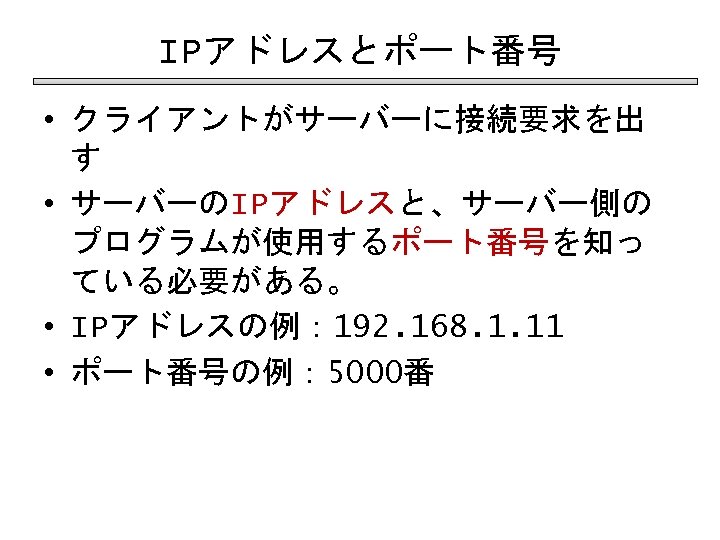
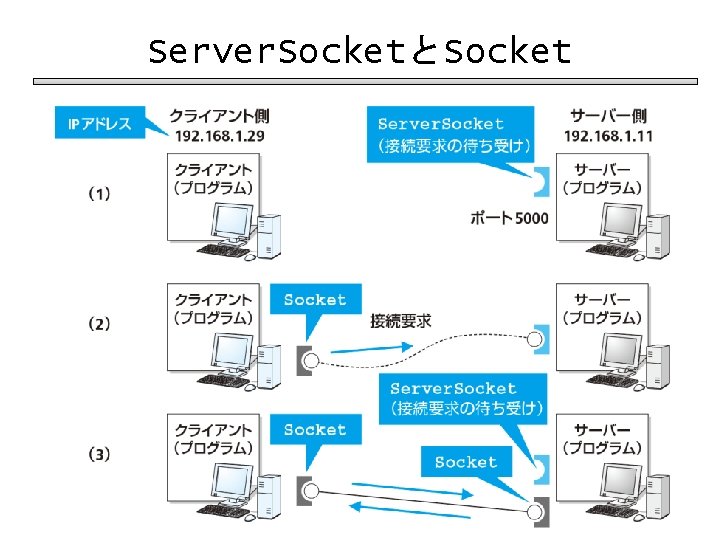
Server. SocketとSocket
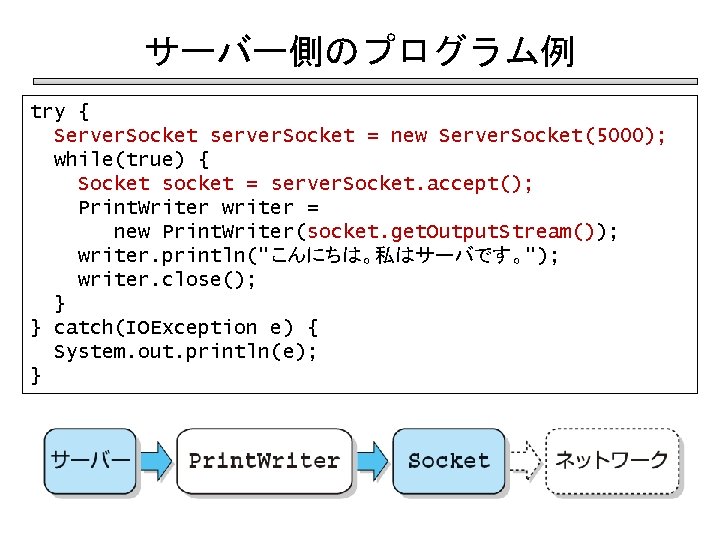
サーバー側のプログラム例 try { Server. Socket server. Socket = new Server. Socket(5000); while(true) { Socket socket = server. Socket. accept(); Print. Writer writer = new Print. Writer(socket. get. Output. Stream()); writer. println("こんにちは。私はサーバです。"); writer. close(); } } catch(IOException e) { System. out. println(e); }
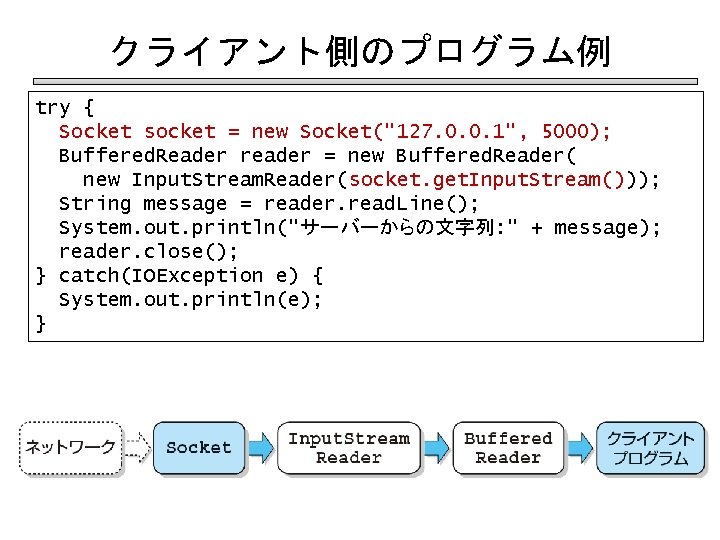
クライアント側のプログラム例 try { Socket socket = new Socket("127. 0. 0. 1", 5000); Buffered. Reader reader = new Buffered. Reader( new Input. Stream. Reader(socket. get. Input. Stream())); String message = reader. read. Line(); System. out. println("サーバーからの文字列: " + message); reader. close(); } catch(IOException e) { System. out. println(e); }
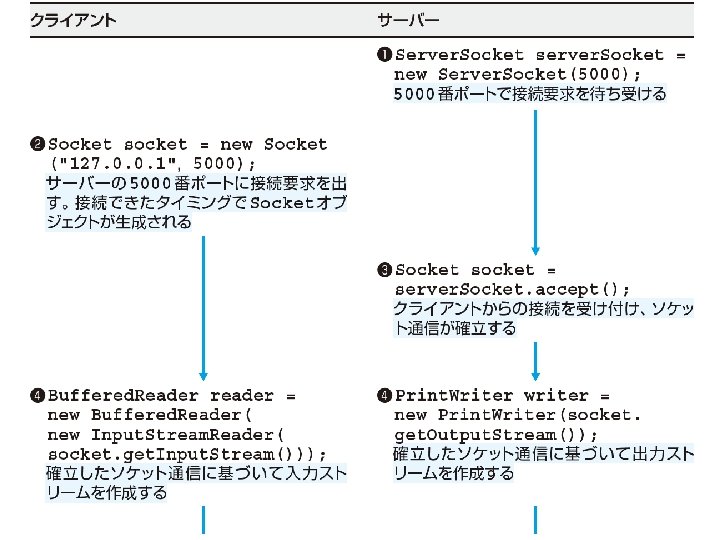
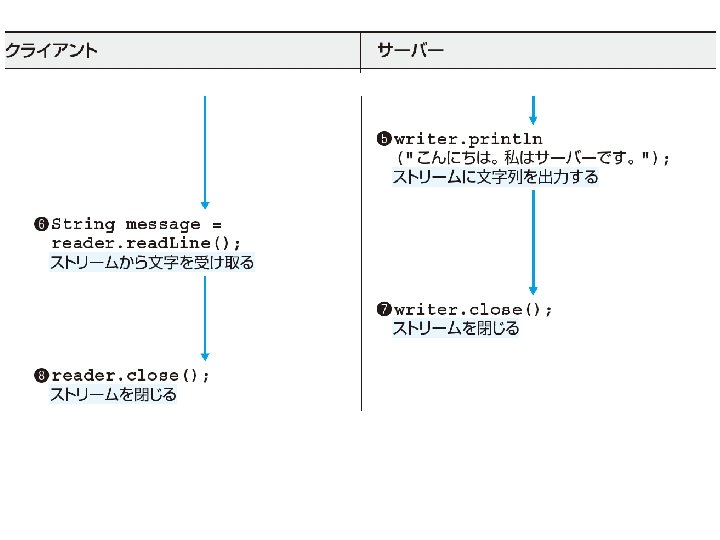
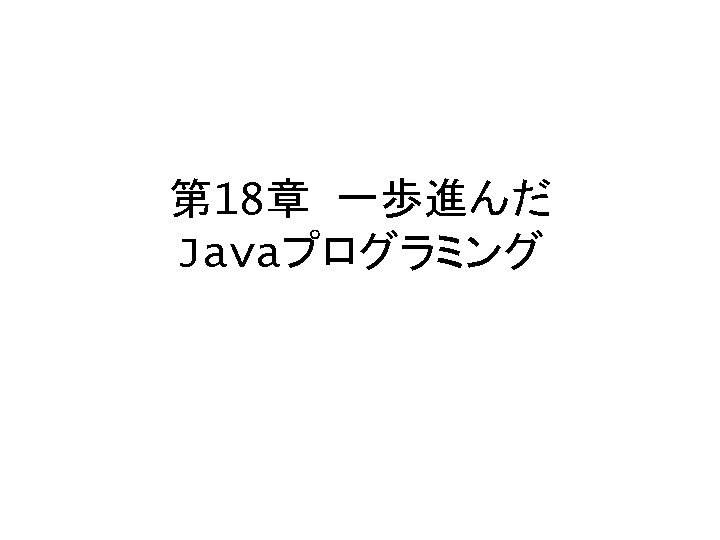
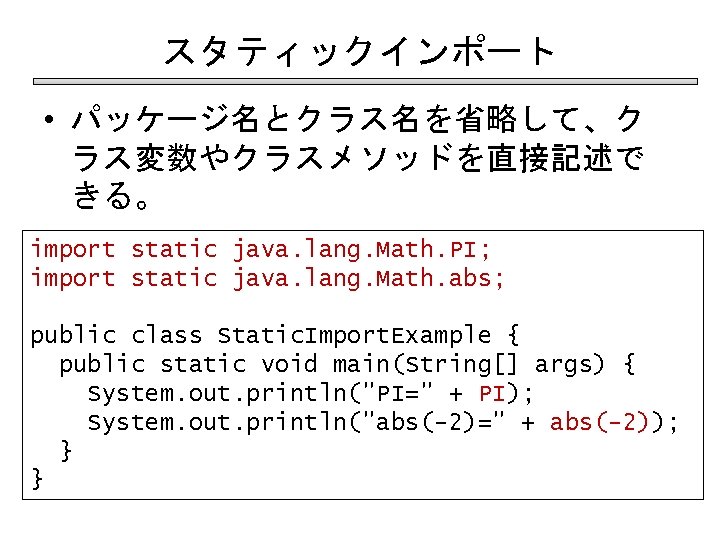
スタティックインポート • パッケージ名とクラス名を省略して、ク ラス変数やクラスメソッドを直接記述で きる。 import static java. lang. Math. PI; import static java. lang. Math. abs; public class Static. Import. Example { public static void main(String[] args) { System. out. println("PI=" + PI); System. out. println("abs(-2)=" + abs(-2)); } }
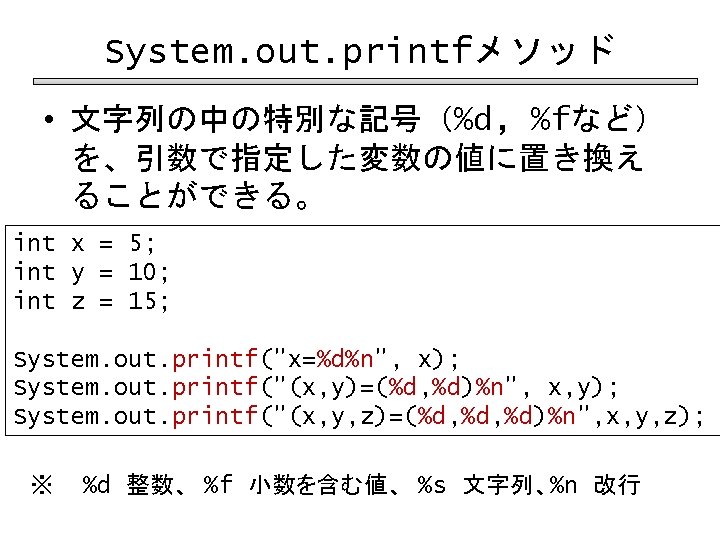
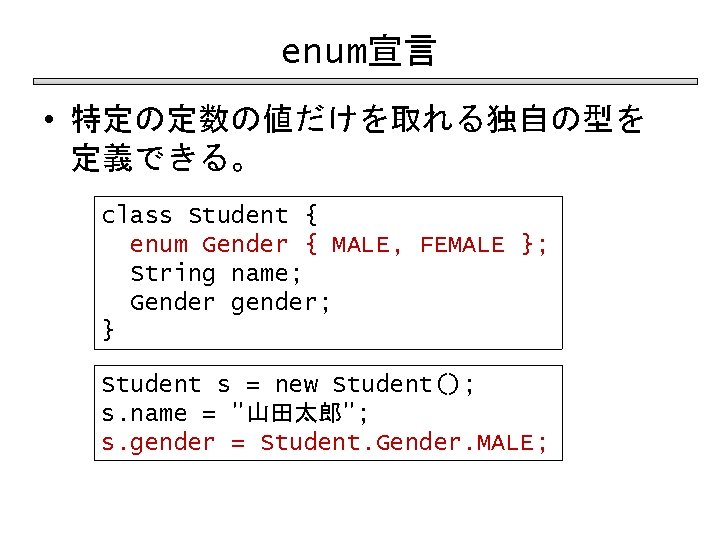
enum宣言 • 特定の定数の値だけを取れる独自の型を 定義できる。 class Student { enum Gender { MALE, FEMALE }; String name; Gender gender; } Student s = new Student(); s. name = "山田太郎"; s. gender = Student. Gender. MALE;
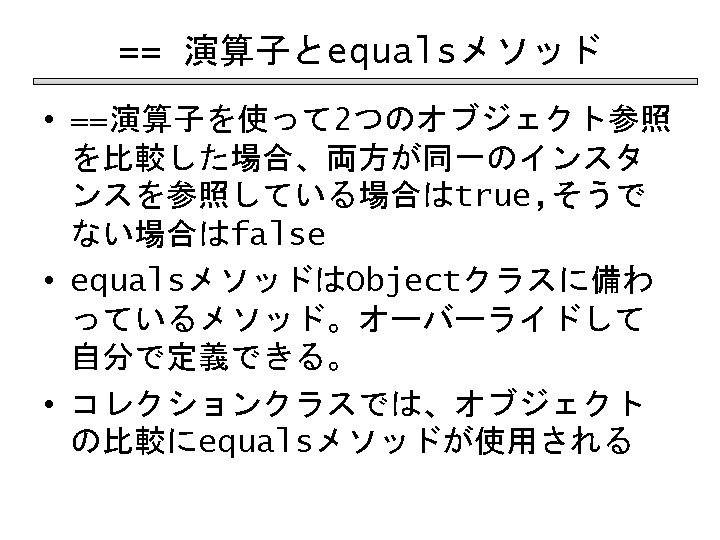
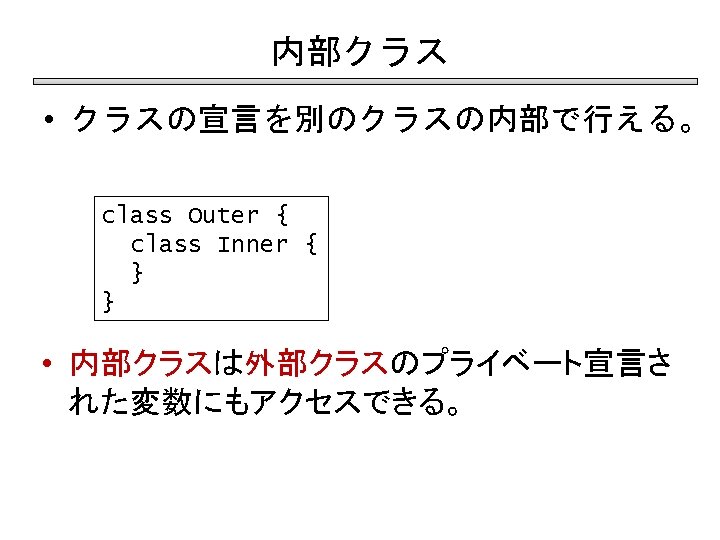
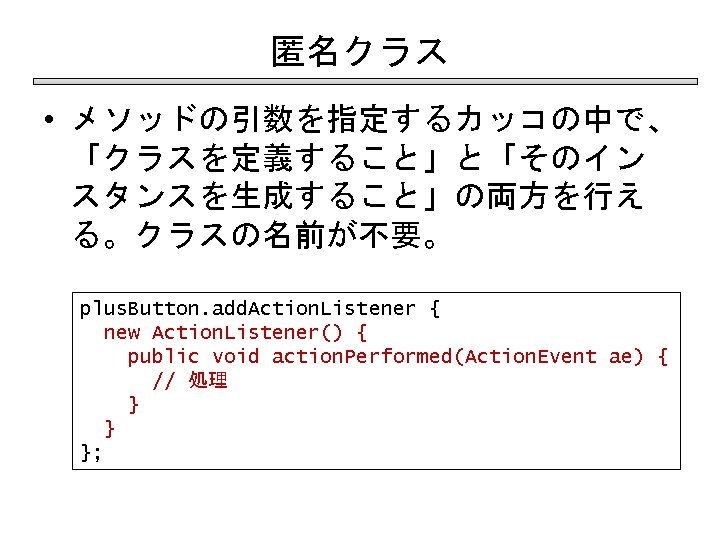
Java 讀檔
Import java.util.random;
Import java.util.* class vector
Import java.util.random;
Java import java.util.*
Import java.util.*
Java scanner import
Java import java.util.*
Import java.util.
Import java.util.* public class test
Java applet swing
Import java.awt.event
Boost tokenizer example
Import java.util.stringtokenizer;
Import java.util."; public
Import java.io.*;
Public class java
Strings in java
Using the random class requires an import statement.
Import java.io.*
Java thread import
What is lang in java
Java.lang.util
Random assignment vs random sampling
Random assignment vs random selection
From random import *
Import numpy as np import matplotlib.pyplot as plt
Random number generatore
Type random things
Diagram example
Public class demo
Uml 0..*
Java file class
Import javax.swing.*
Applet class in java
Tanslate
Import java.applet.applet
Import java.applet.applet
Java applet adalah
Import awt
Javax.servlet
Import java awt event
Difference between abstract class and interface
Import java color
Java static import
Import calendar java
Import java.
Jradio
Import java.applet.*
Problem solving
Java
Java scanner import
Java applet
Import java.awt
String tokenizer
Import java.awt.*
Java.io.file
Java
Import java.net.*;
Import java.awt.*;
Import javax
Sentirse util
Calcular o comprimento util de um rebite
Na figura abaixo embora puxe a carroça
2da timoteo 3 16
La ciencia es util
Serte util
Ejemplo de depreciación
Perbedaan antara java swing dan awt adalah
Language
Java bean vs enterprise java bean
Simple random sampling with replacement example
Random survival forest python
Random variable in probability distribution
Discrete vs continuous variable
Continuous random variable example
Continuous random variable example
Discrete random variables
Discrete random variables example
Random assignment example psychology
Variance of discrete random variable
Perceiving order in random events example
Gap test for random numbers
Random experiment
Systematic sampling example
6 random numbers
Cluster sampling
What are statistics
Hierarchy of thread class in java
Math class function in java
Www.classpointapp
Iterator inner class java
Abstract factory singleton
Dynamic code loading
Legacy class in java
Adapter classes in java
Java.lang.class
Primitif xxx
Keyboard class java
What is class
Oop java
Exception class hierarchy in java
Math package java
Abstract class java
Public class test public
Java dynamic class loading
Java class
Java abstract class properties
Array class java
Jframe uml
Class object in java
What is abstract class
Anatomy of a java class
Difference between abstract classes and interfaces
There is class today
Package mypackage class first class body
Abstract class vs concrete class
Lower class boundary of the modal class
Class i vs class ii mhc
Abstract concrete class relationship
Example of class interval
Stimulus
Stimuli vs stimulus
Therapeutic class and pharmacologic class
Class maths student student1 class student string name
How to find class boundaries
In greenfoot, you can cast an actor class to a world class?
Class 0 esd
Uml static
Class 2 class 3
Extends testcase
Package mypackage class first class body
Class third class
Component class has composite class as collaborator
Socket java example
Socket java example
Multithreading program in java
Lexical issues in java with example
Java cup
Ipc programming
Rsa encryption java
Event driven programming in java
Repetition control structure java
Java swing mvc example
Which are non executable statements in a program
Bank account java program using inheritance
Java applet example
Java fragment example
Abstract data type in java example
자바 스윙
Jdbc stand for
Java swing form example
Gui programmierung java
Java swing mvc example
Mac sha1
Java livelock example
Mongodb hadoop connector java example
Create a java rmi calculator application.
Class bike int speed =90
Java priority queue example