UNIT4 Concepts of Exception handling Exception hierarchy Types
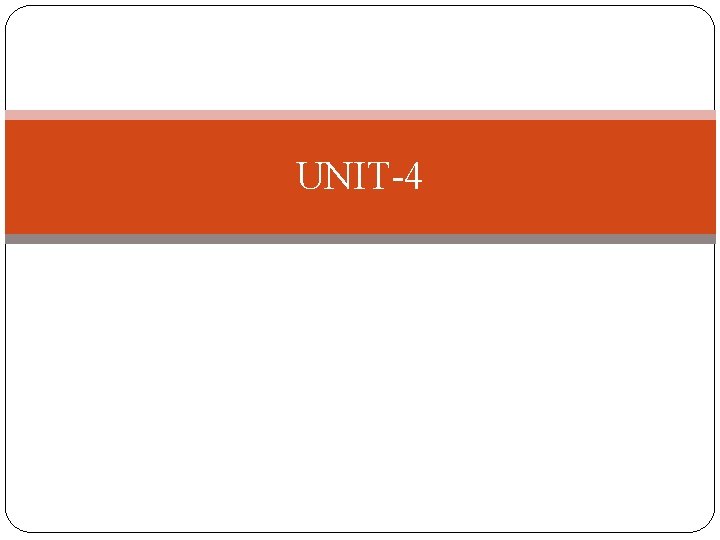
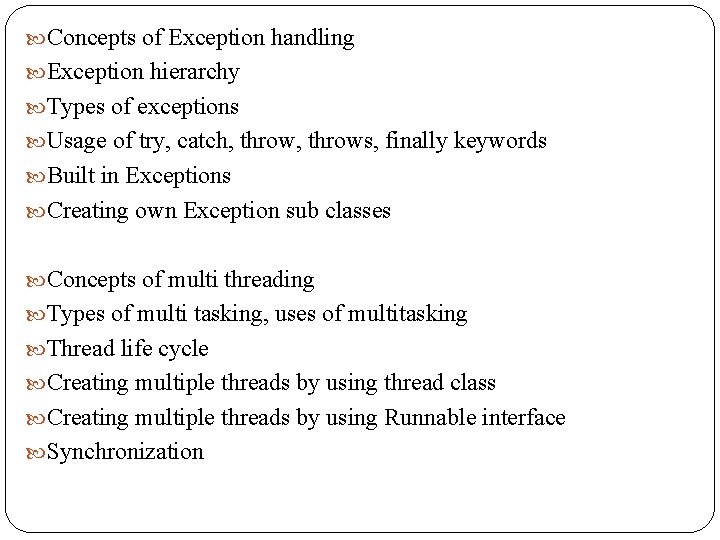
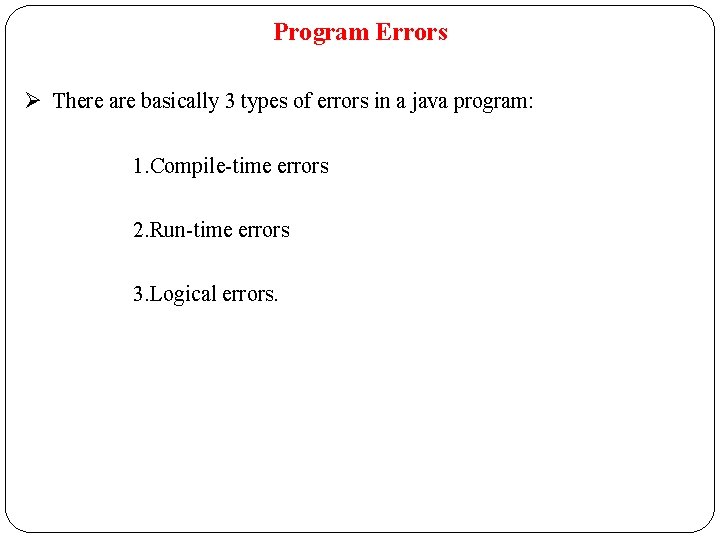
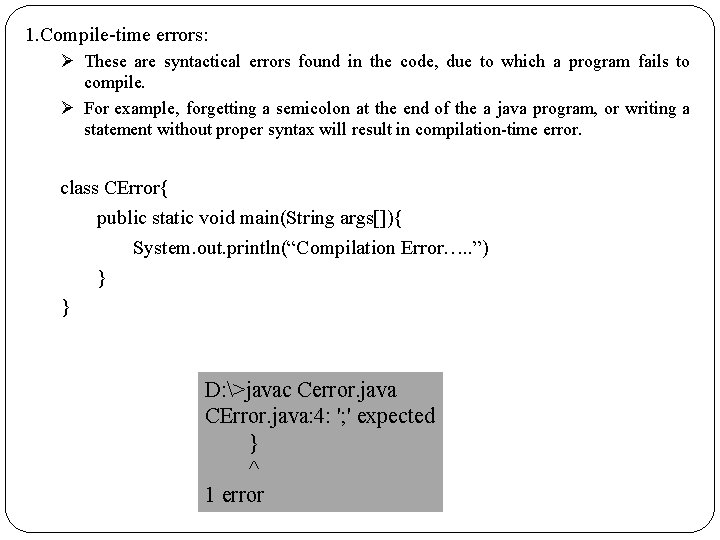
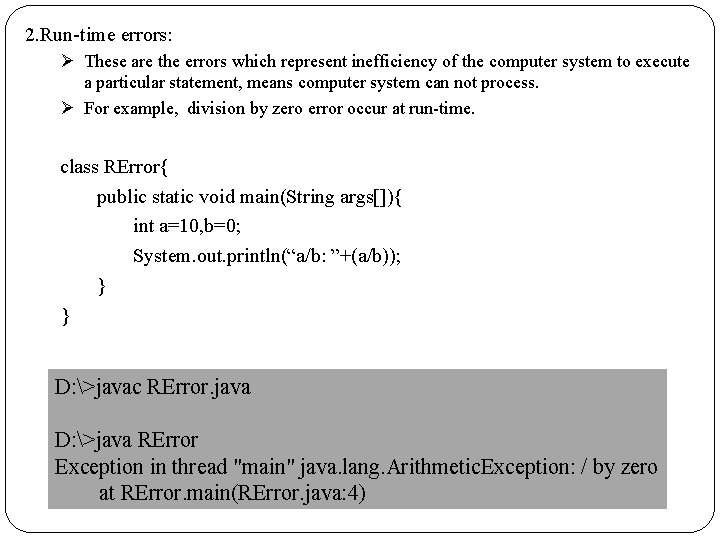
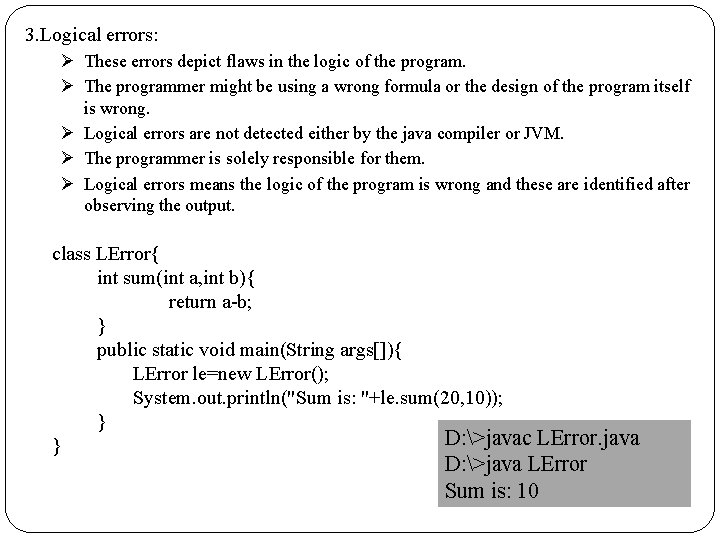
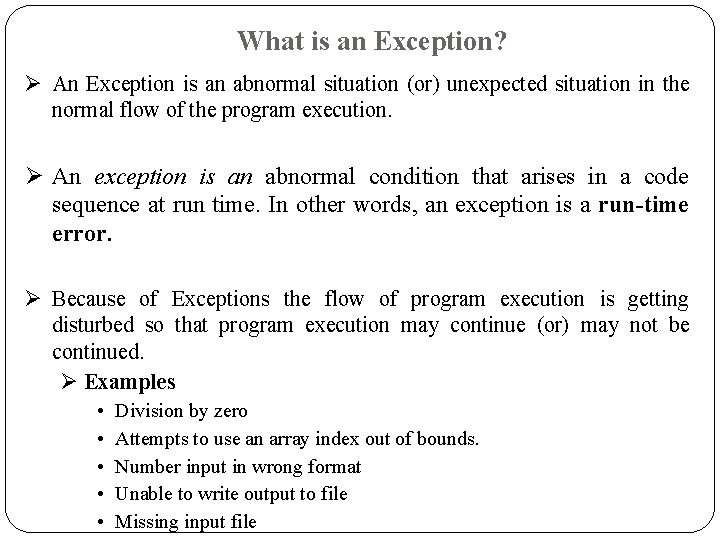
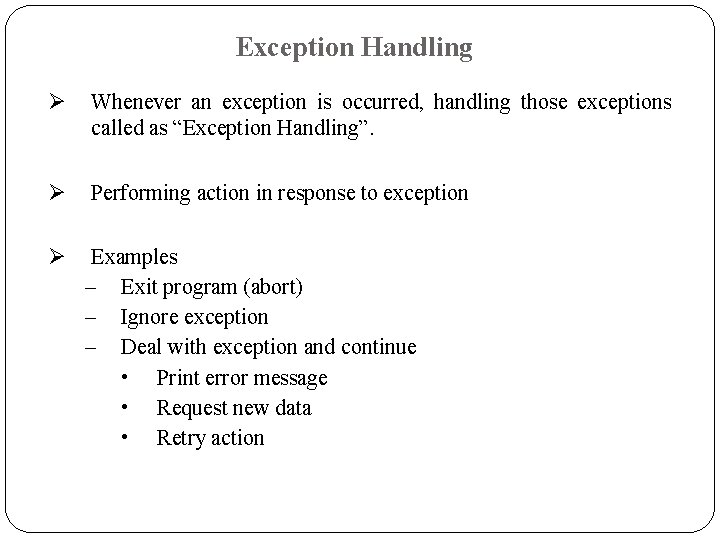
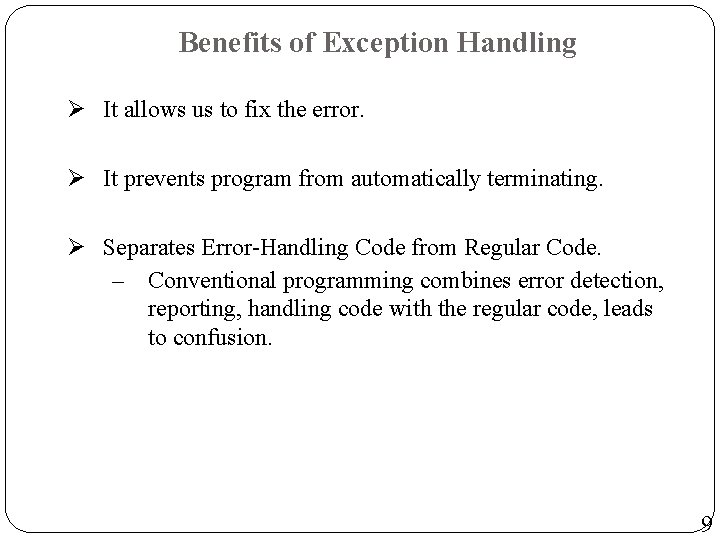
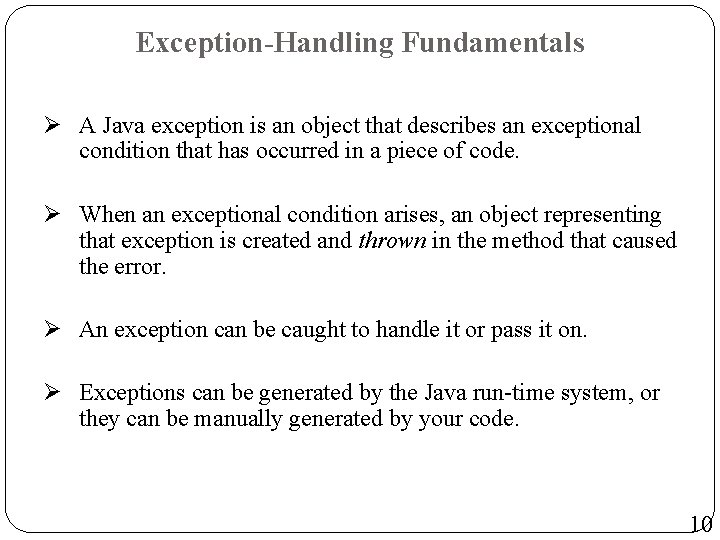
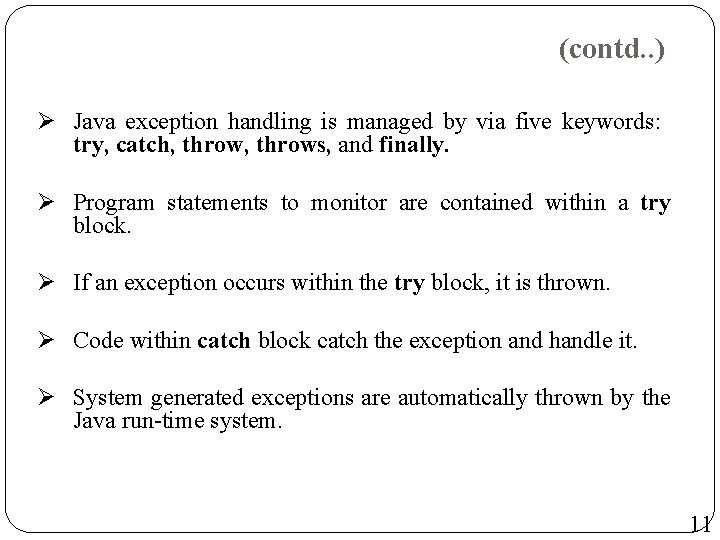
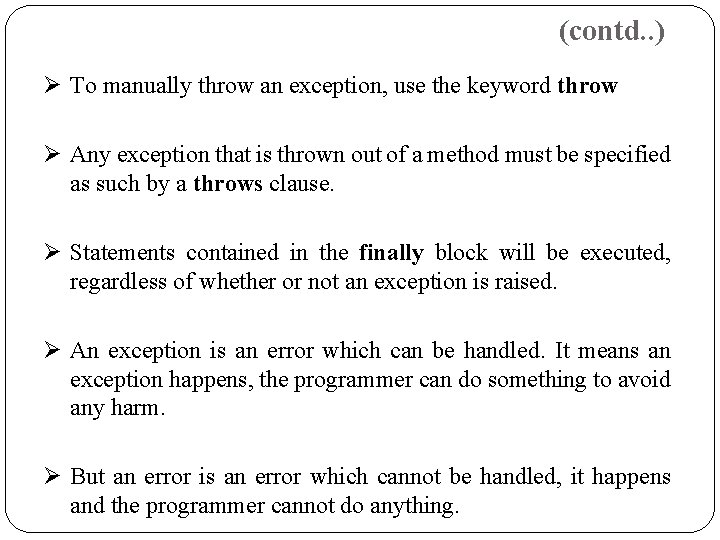
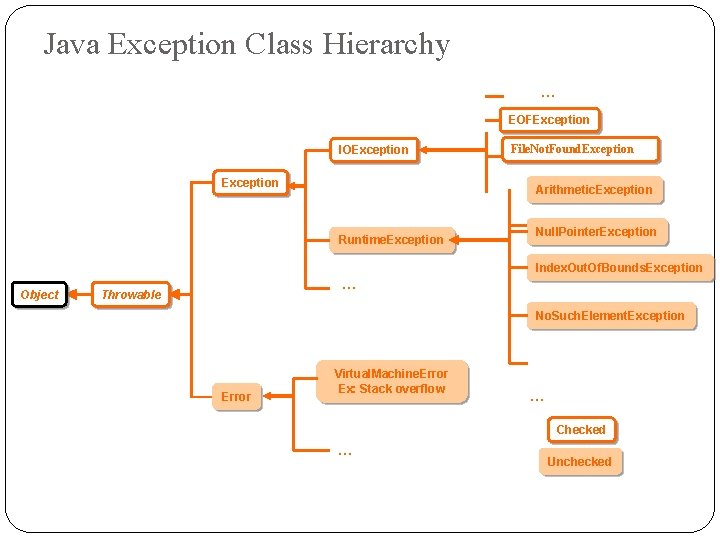
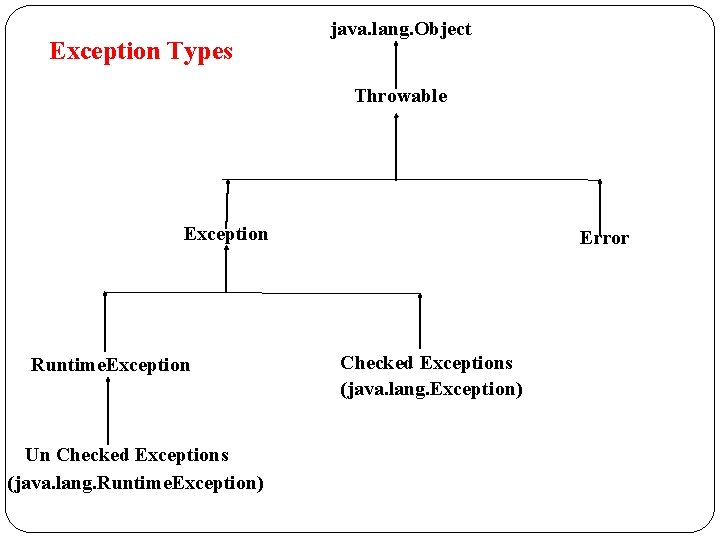
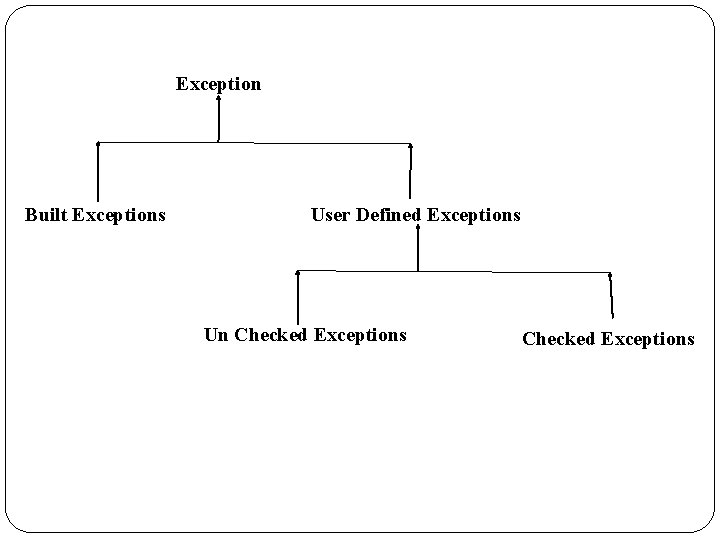
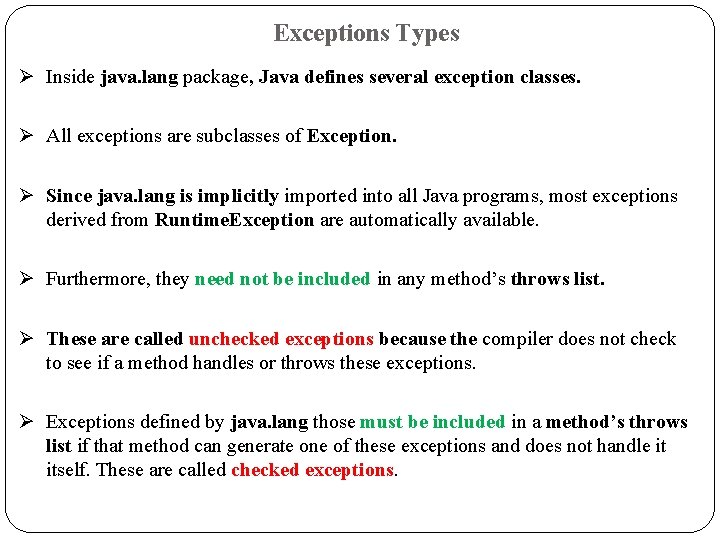
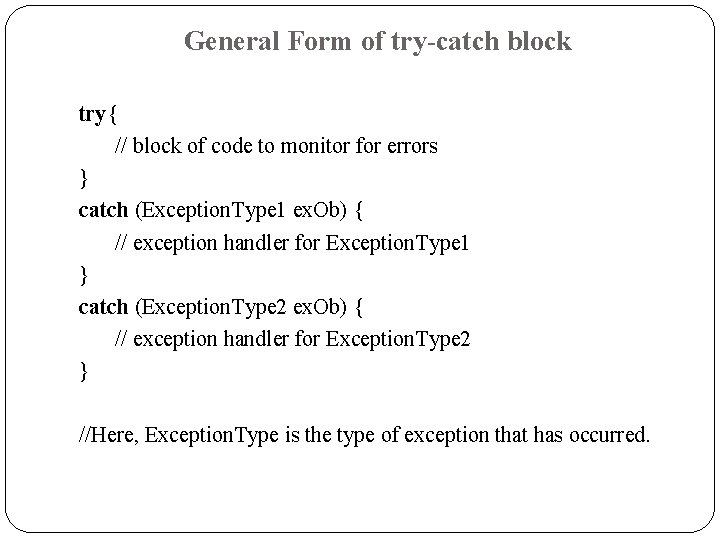
![Uncaught Exceptions class Exc 0 { public static void main(String args[]) { int d Uncaught Exceptions class Exc 0 { public static void main(String args[]) { int d](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-18.jpg)
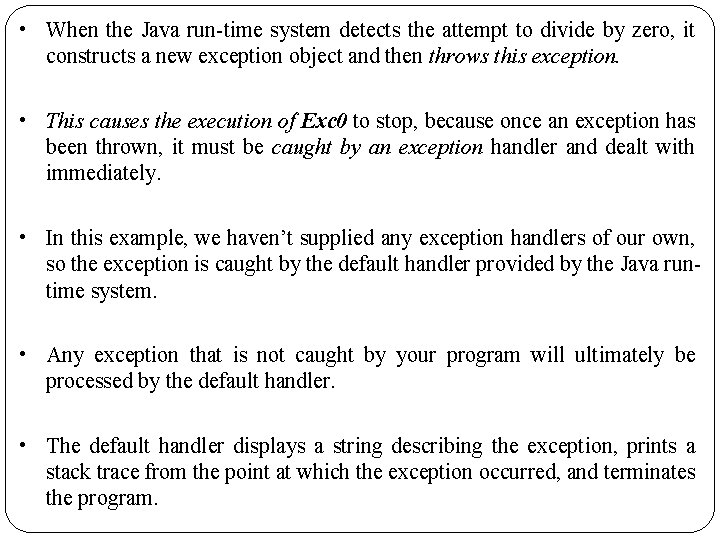
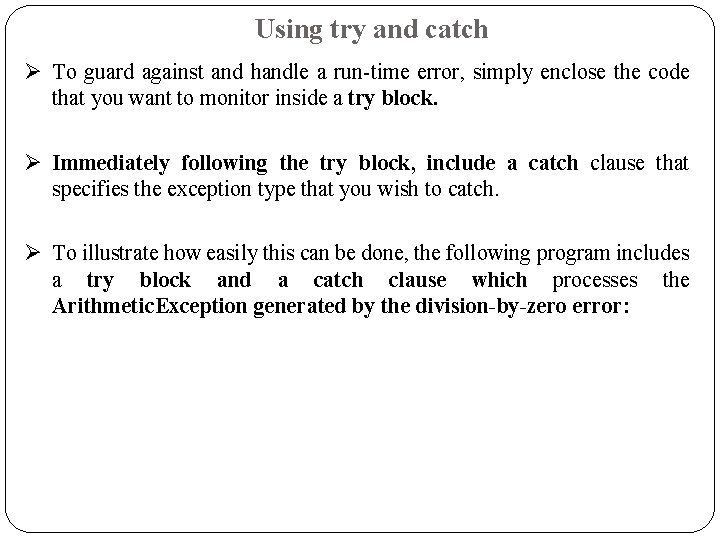
![class Exc 2 { public static void main(String args[]) { int d, a; try class Exc 2 { public static void main(String args[]) { int d, a; try](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-21.jpg)
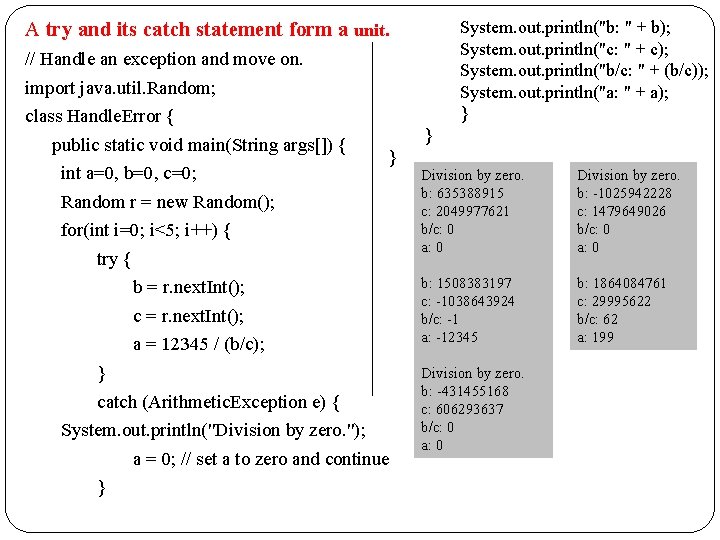
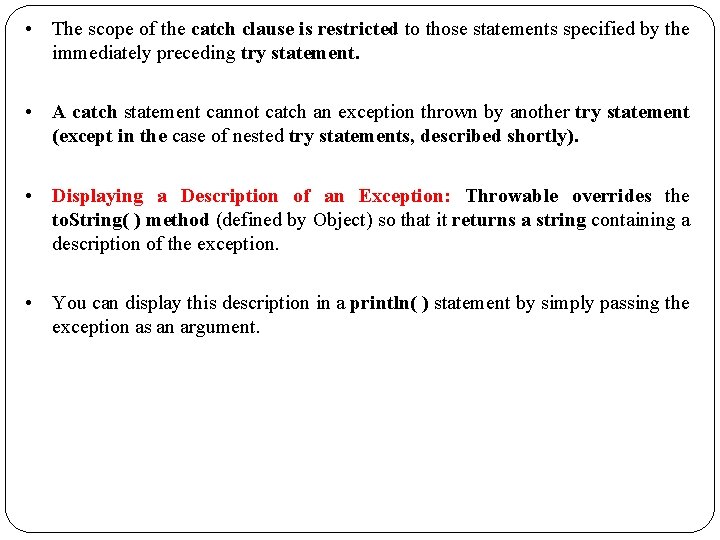
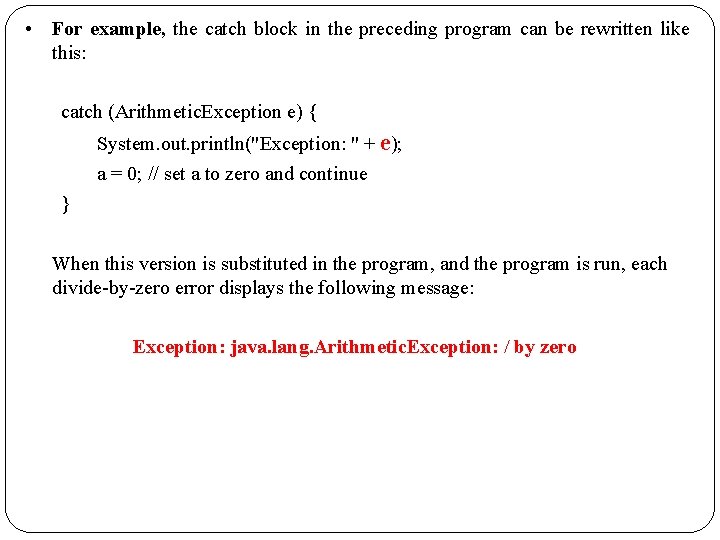
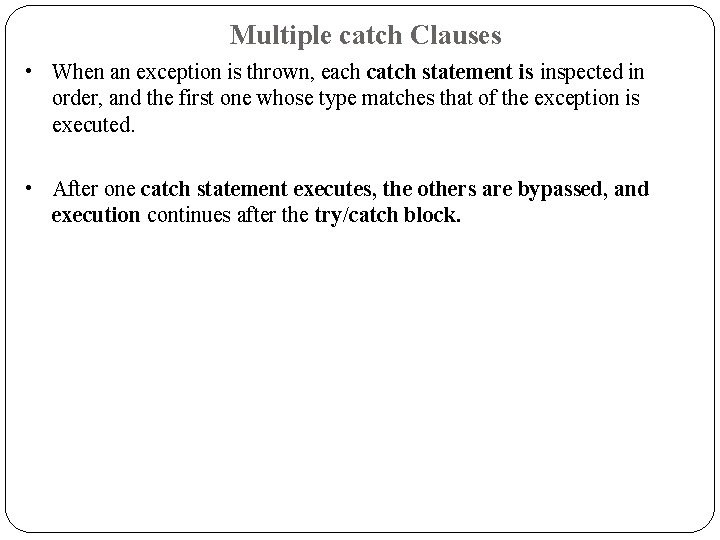
![class Multi. Catch { // Demonstrate multiple catch statements. public static void main(String args[]) class Multi. Catch { // Demonstrate multiple catch statements. public static void main(String args[])](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-26.jpg)
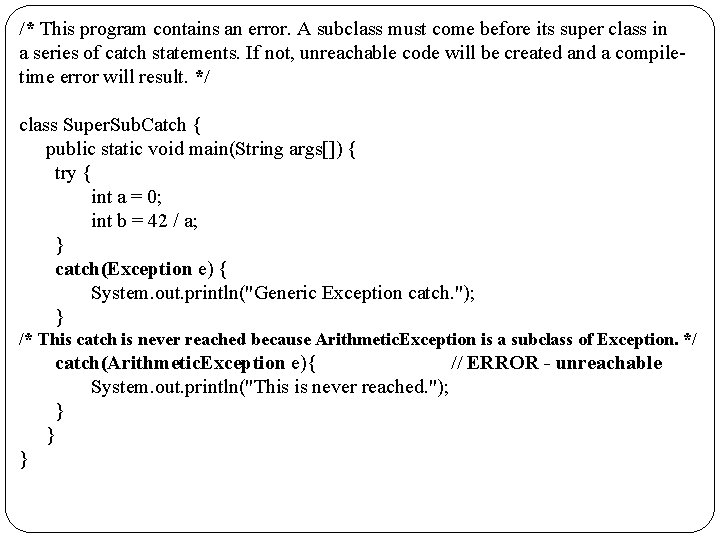
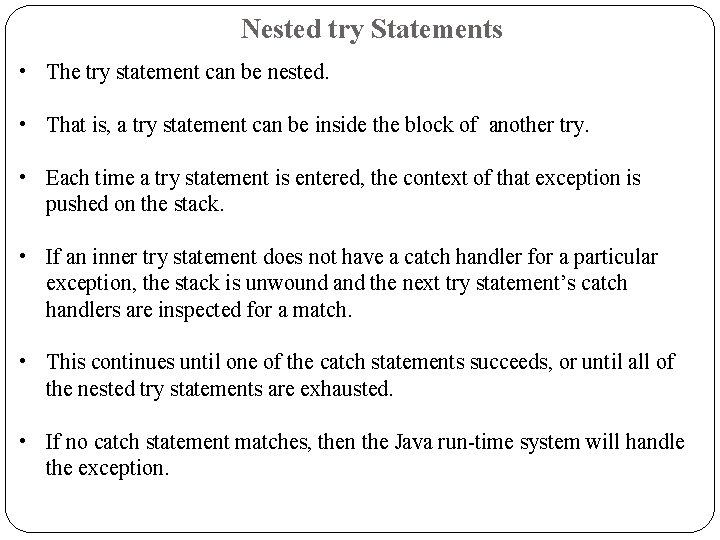
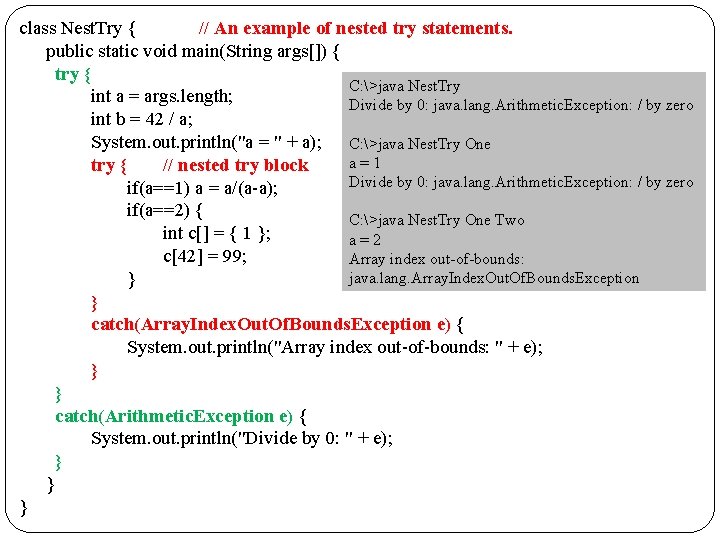
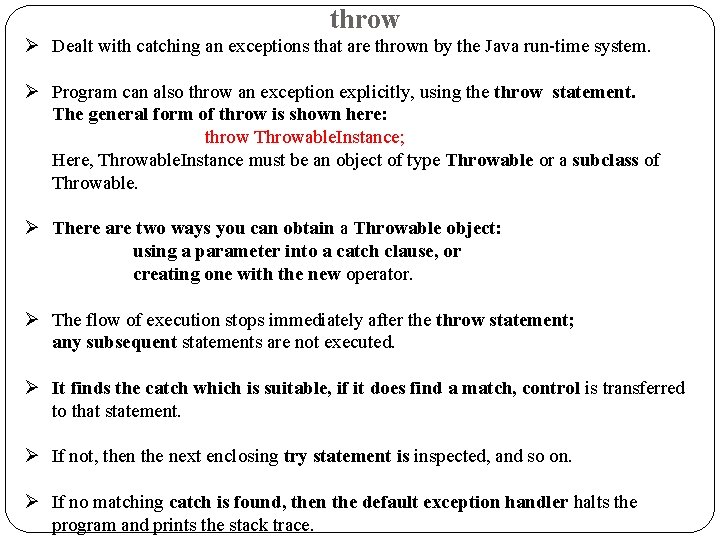
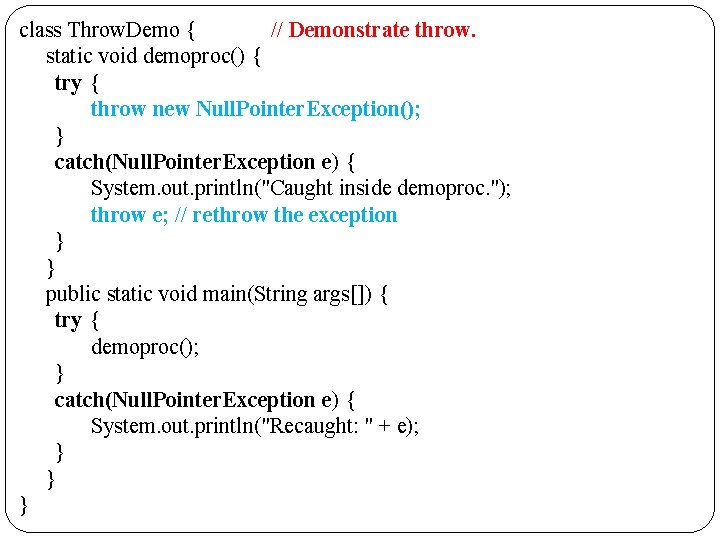
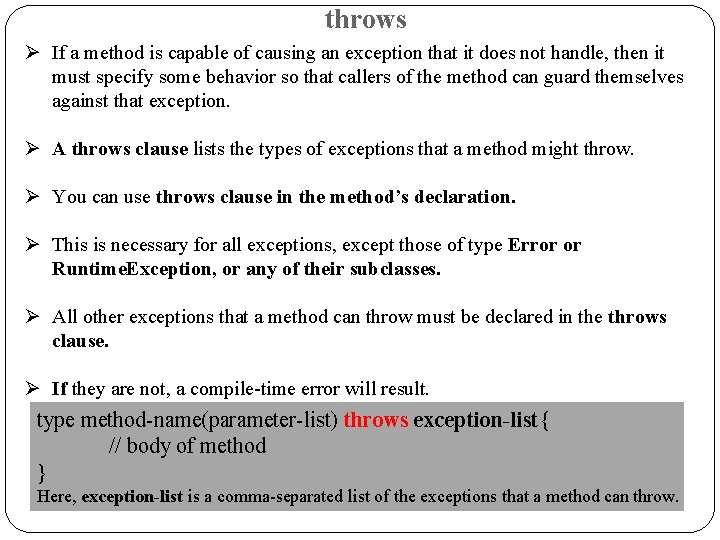
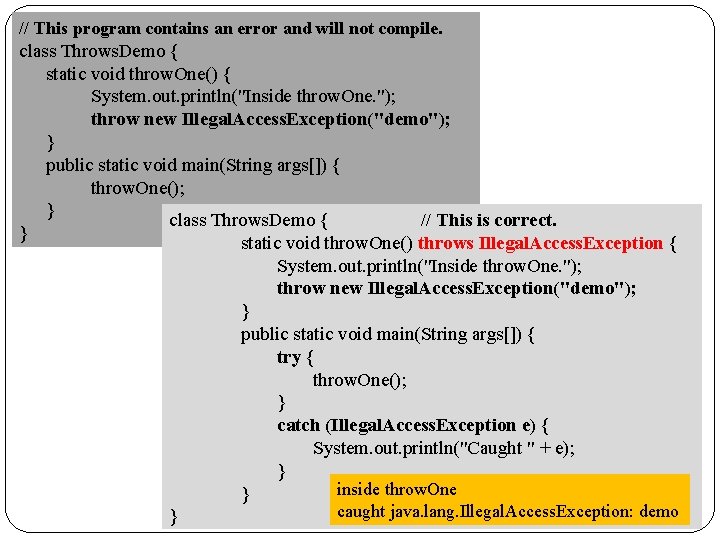
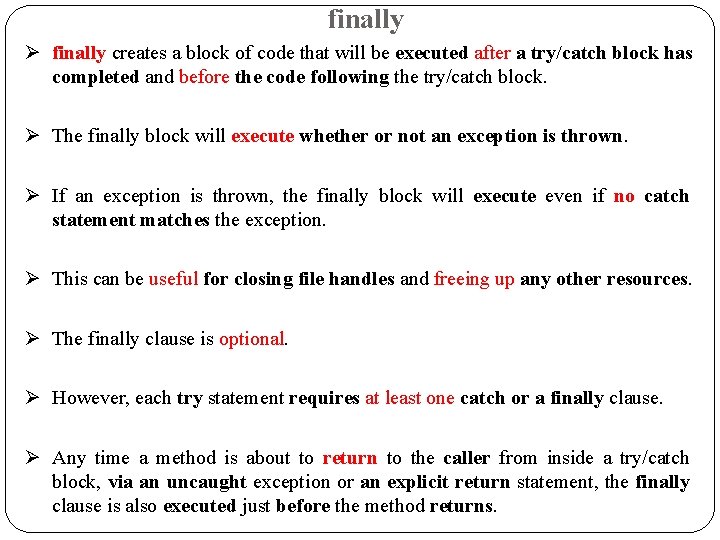
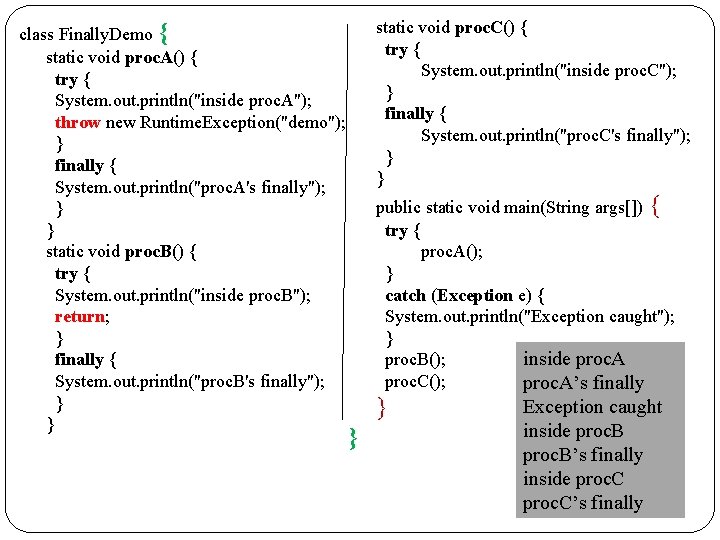
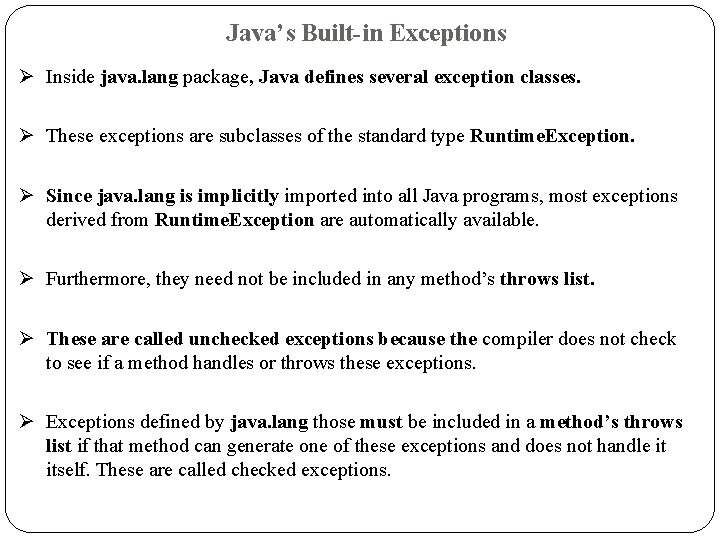
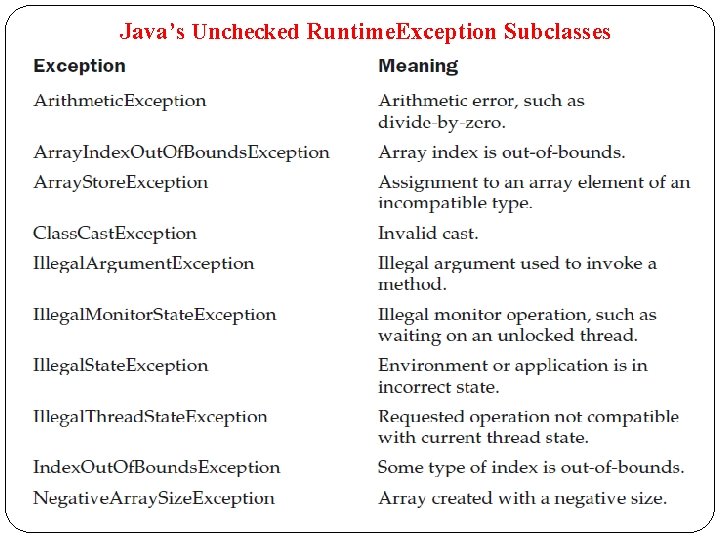
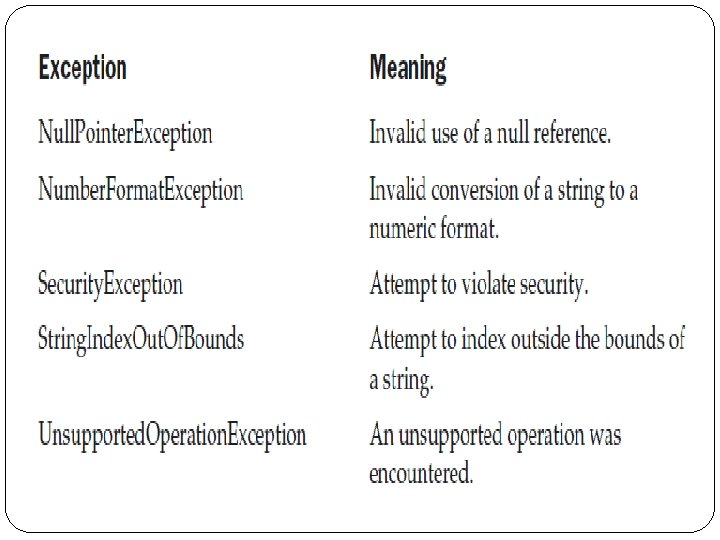
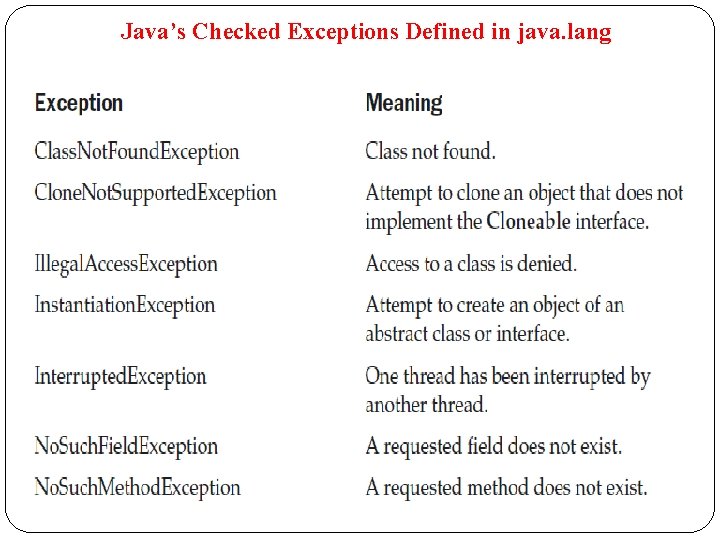
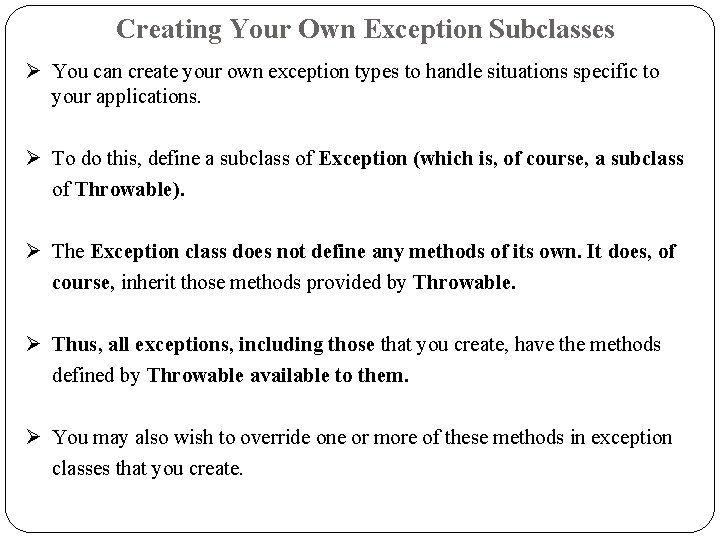
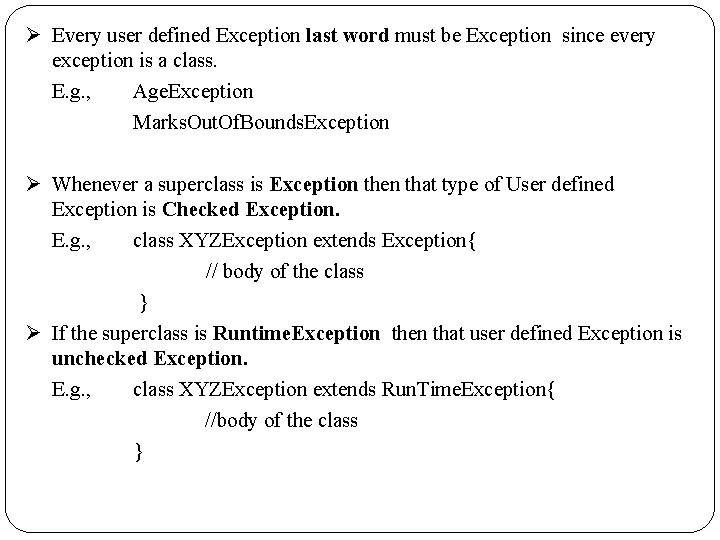
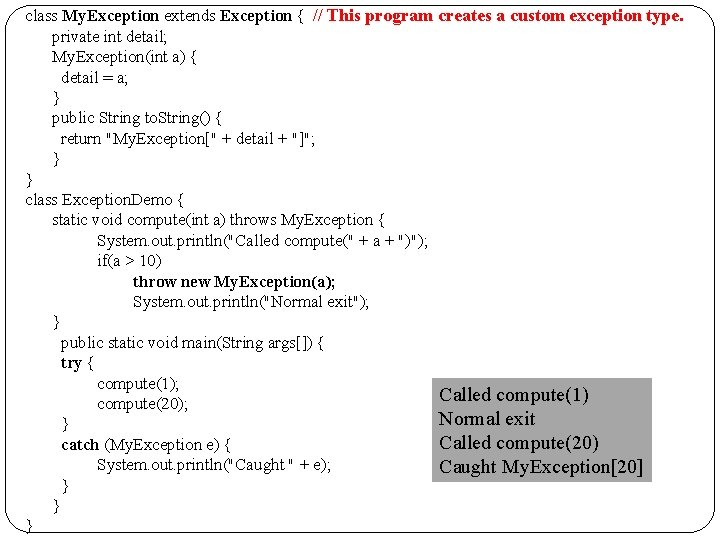
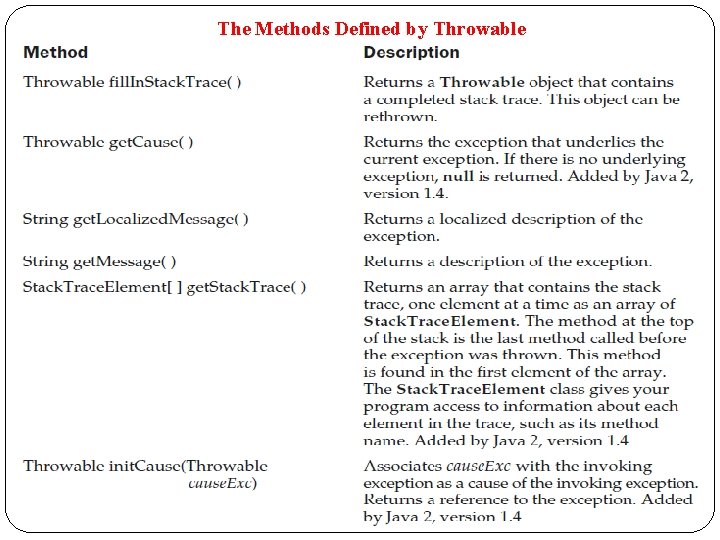
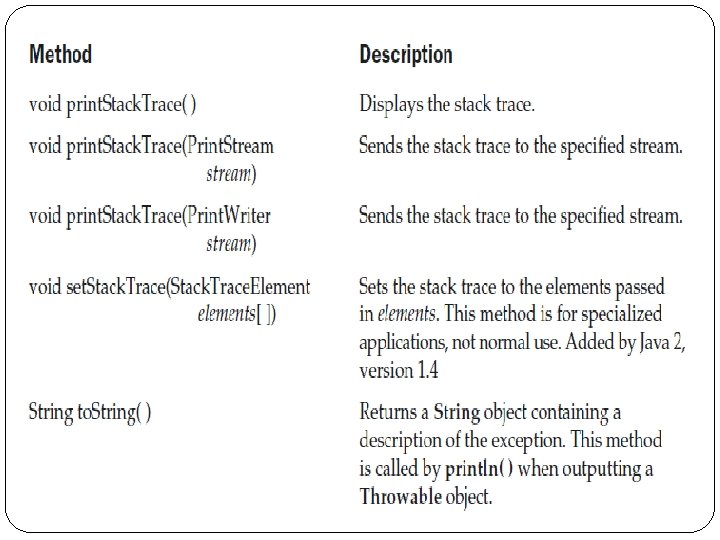
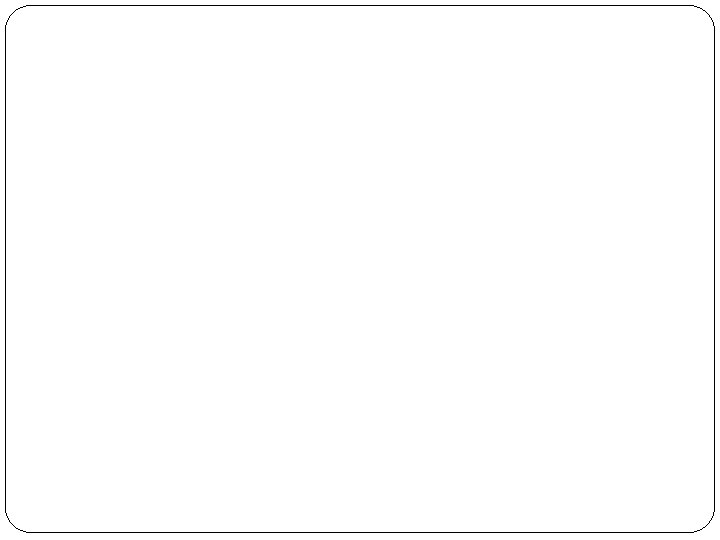
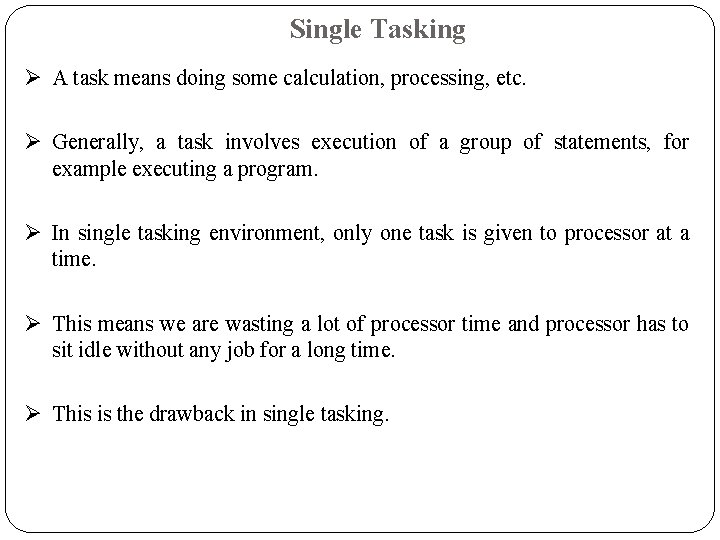
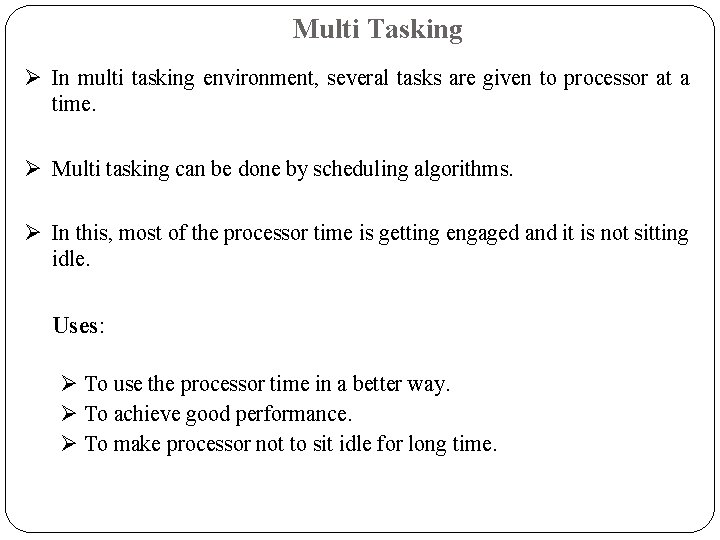
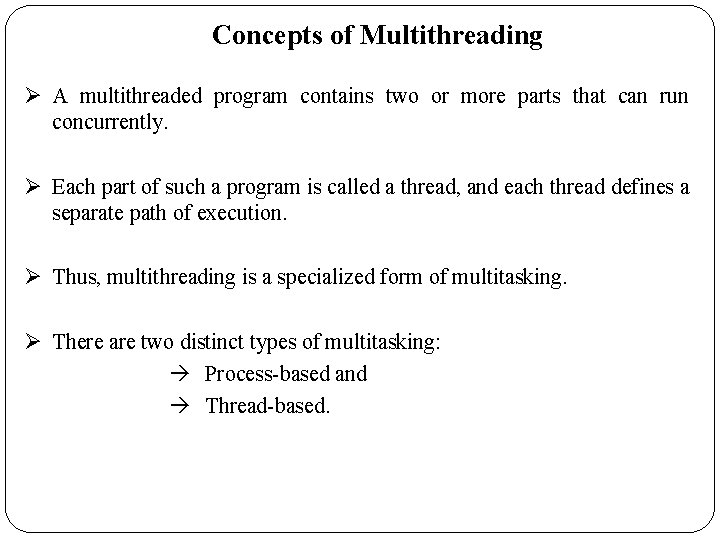
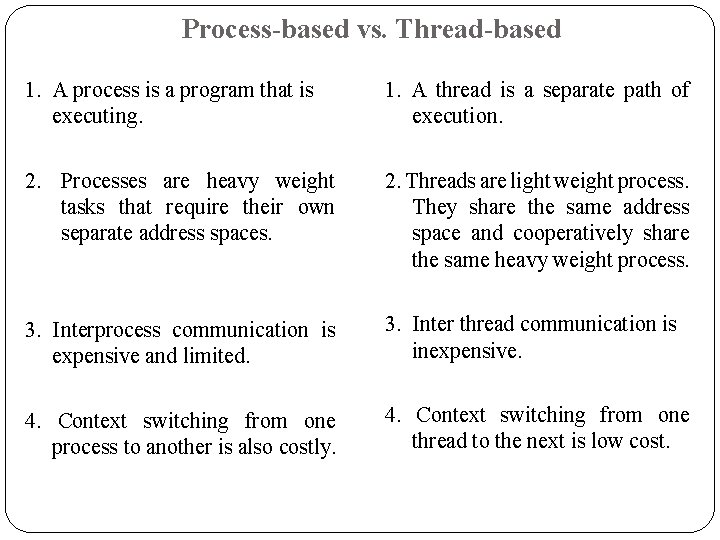
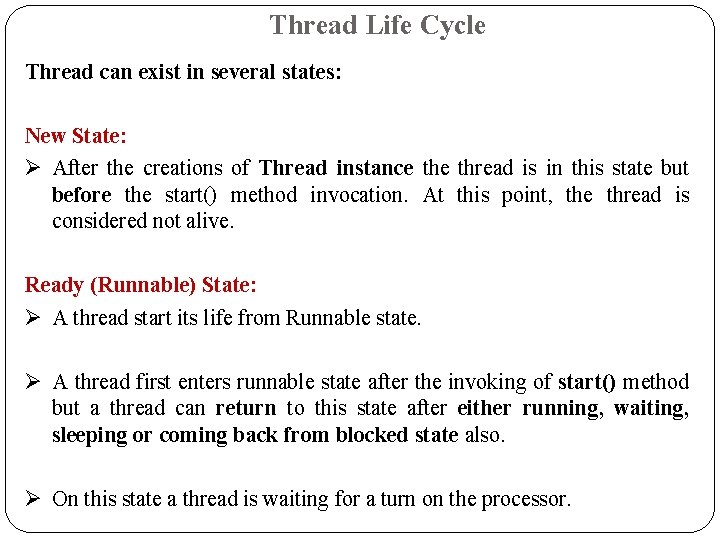
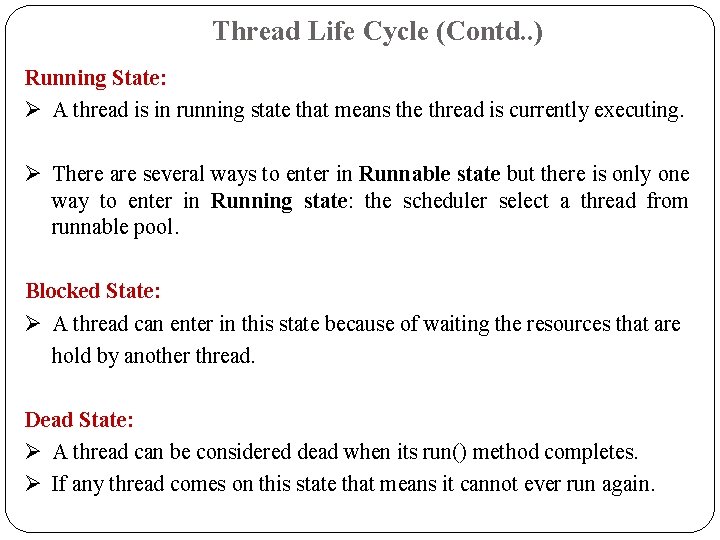
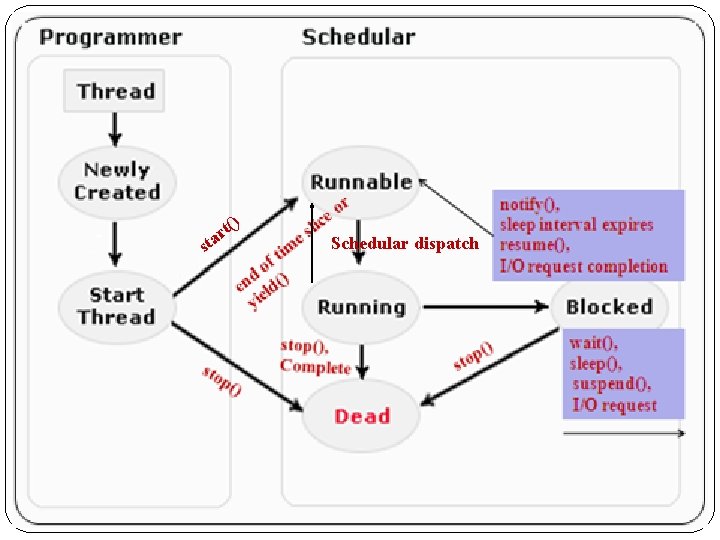
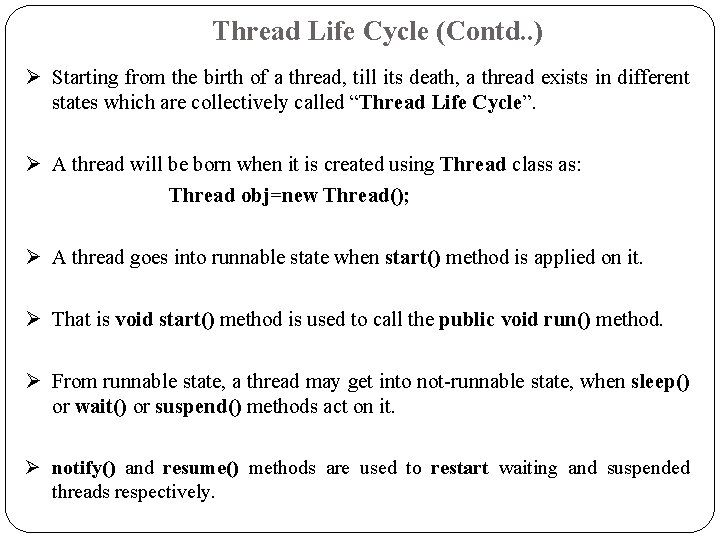
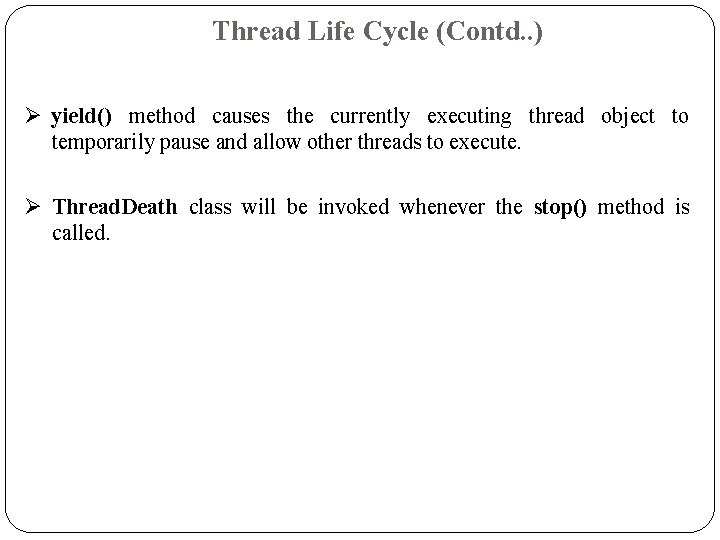
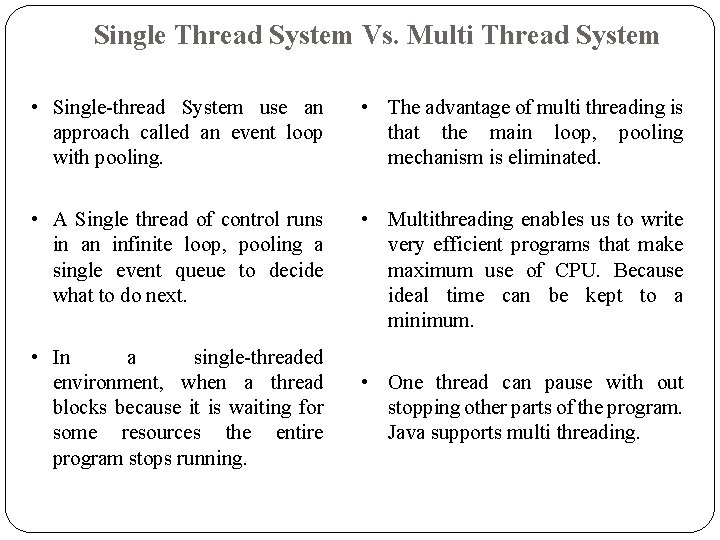
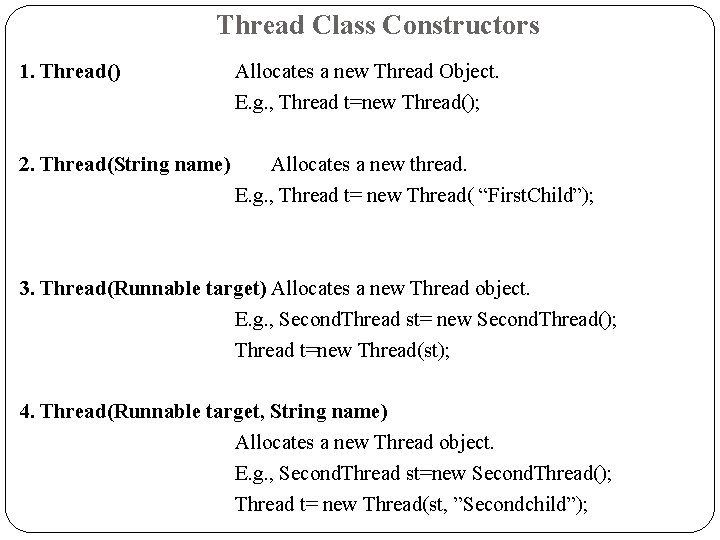
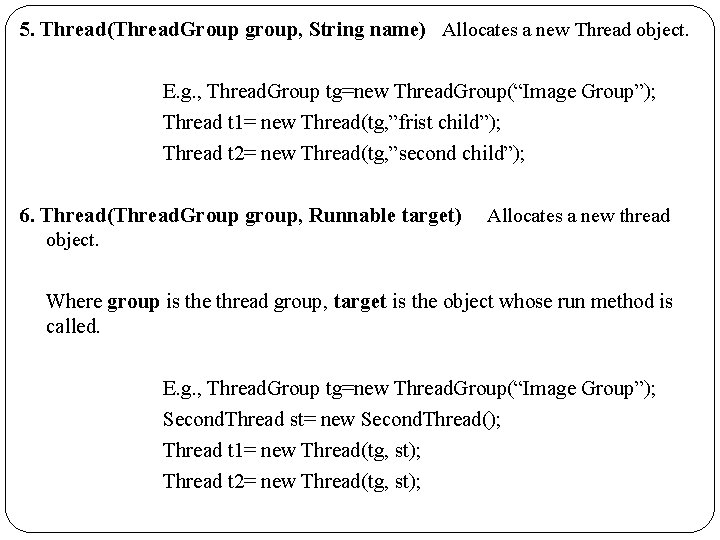
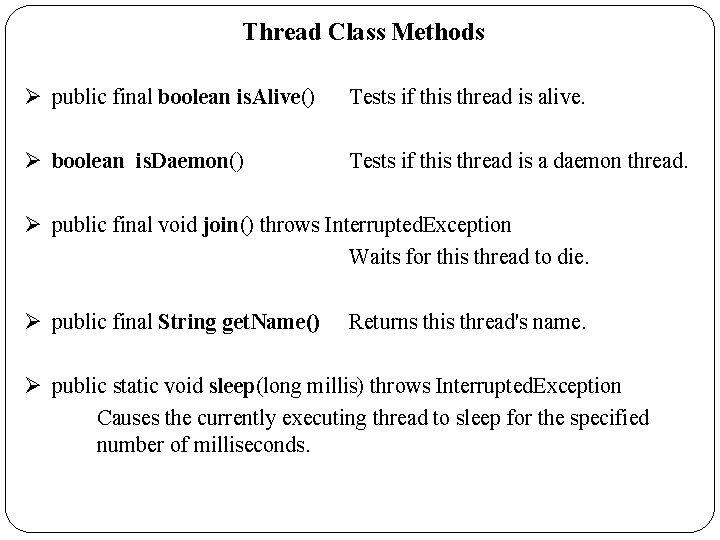
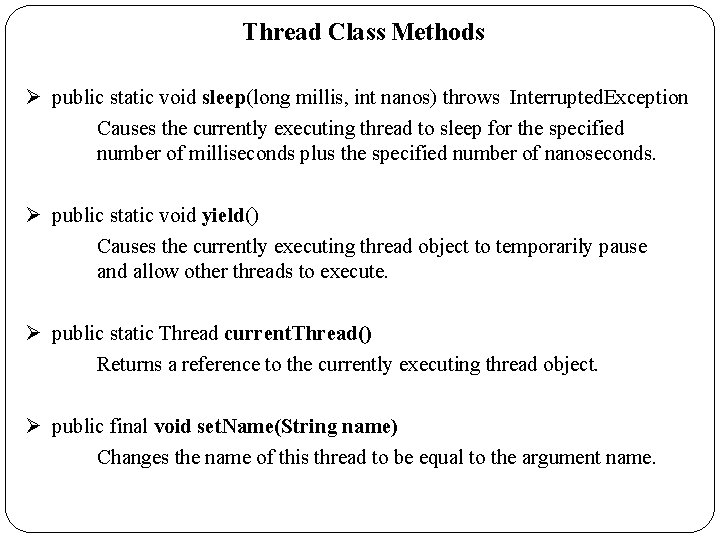
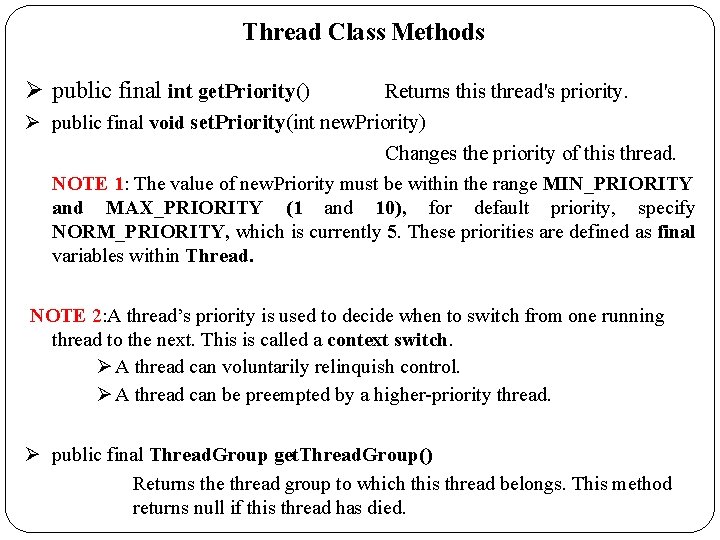
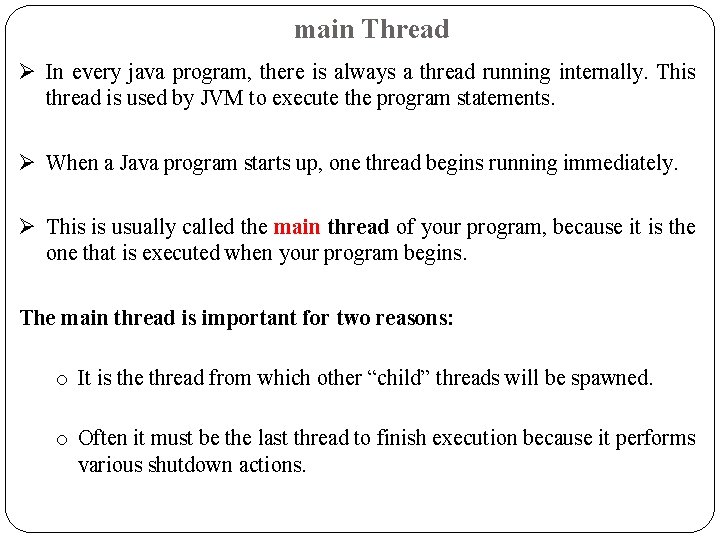
![class Current. Thread. Demo{ public static void main(String sree[]){ System. out. println(“Let us find class Current. Thread. Demo{ public static void main(String sree[]){ System. out. println(“Let us find](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-62.jpg)
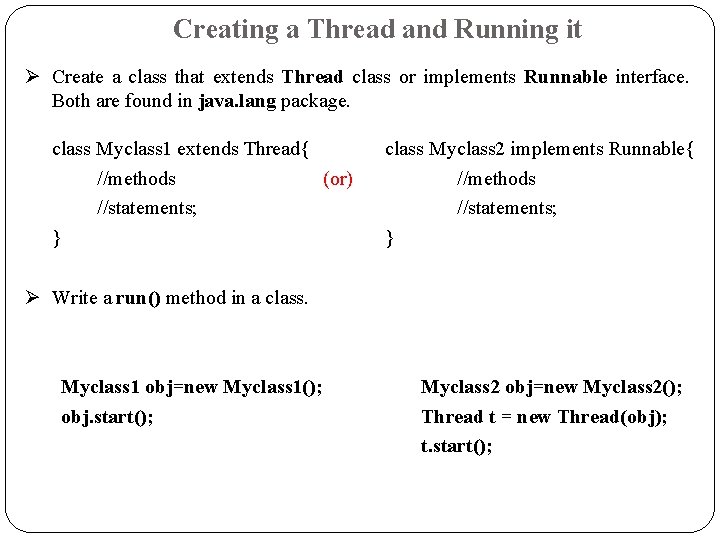
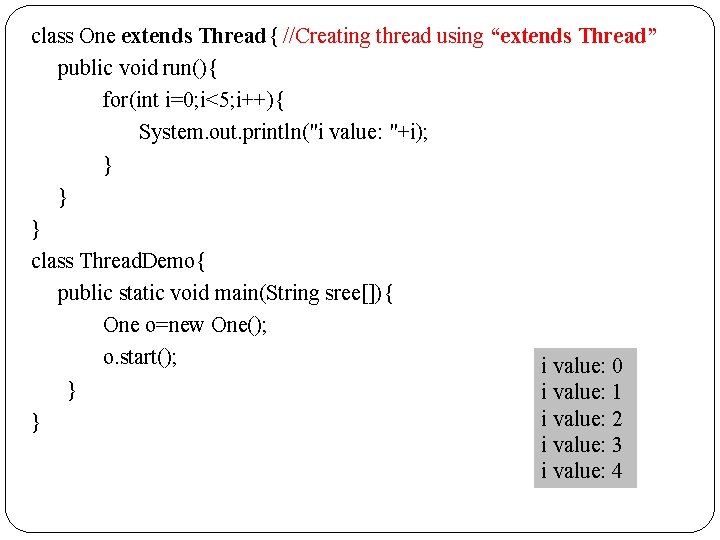
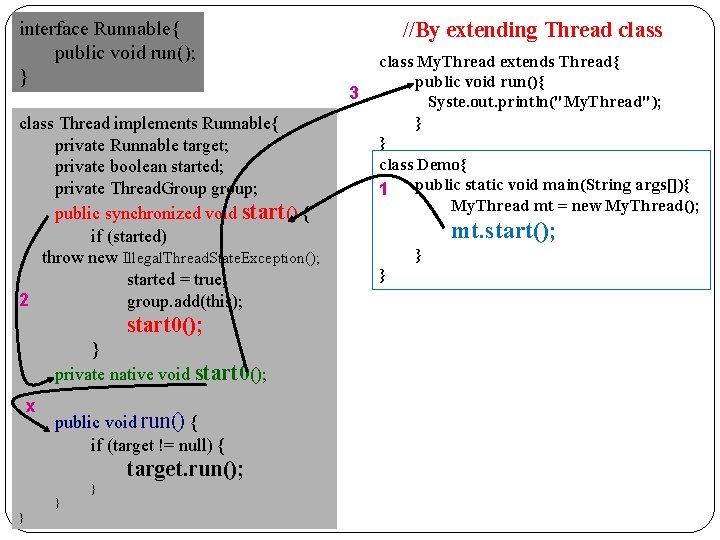
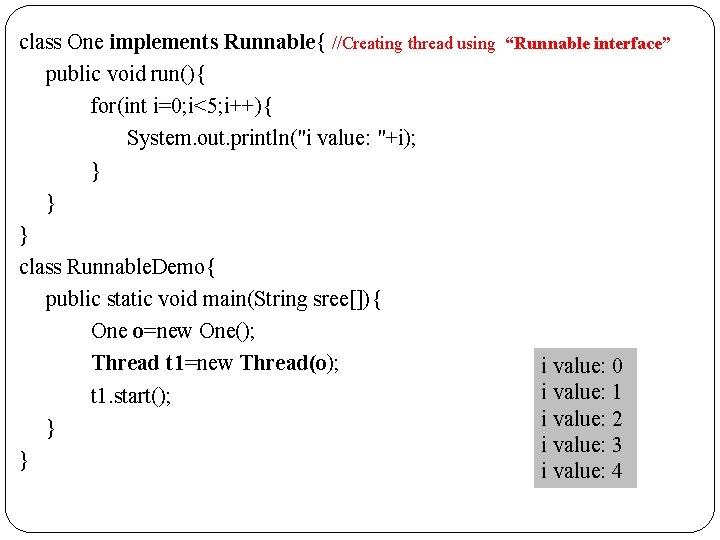
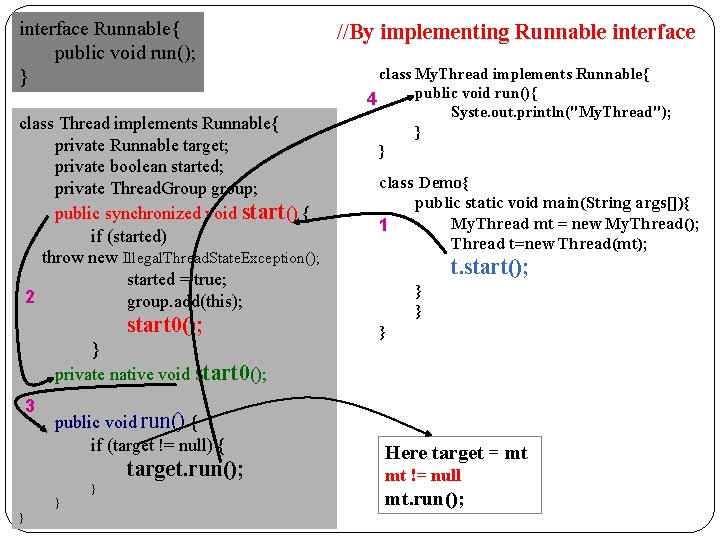
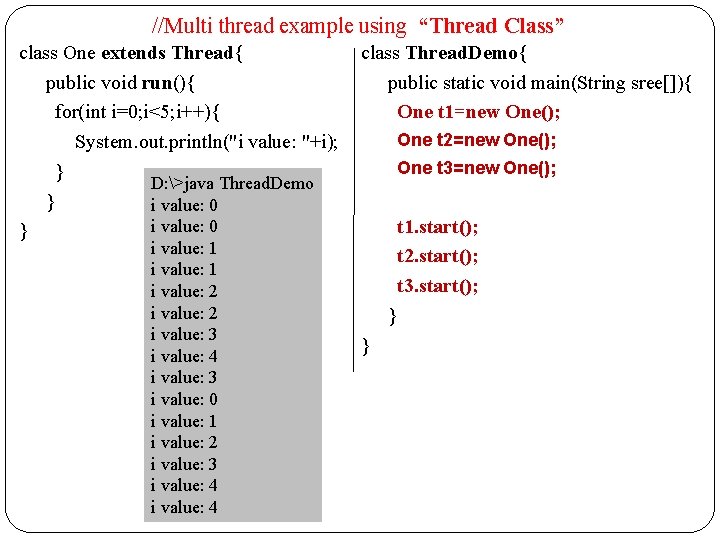
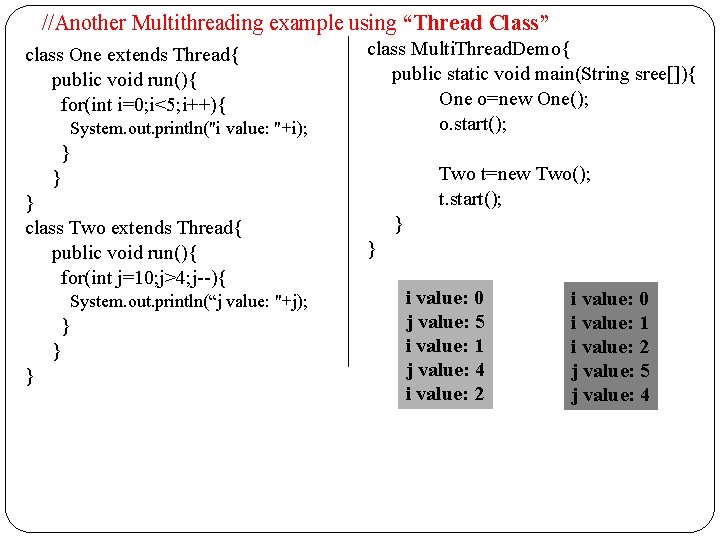
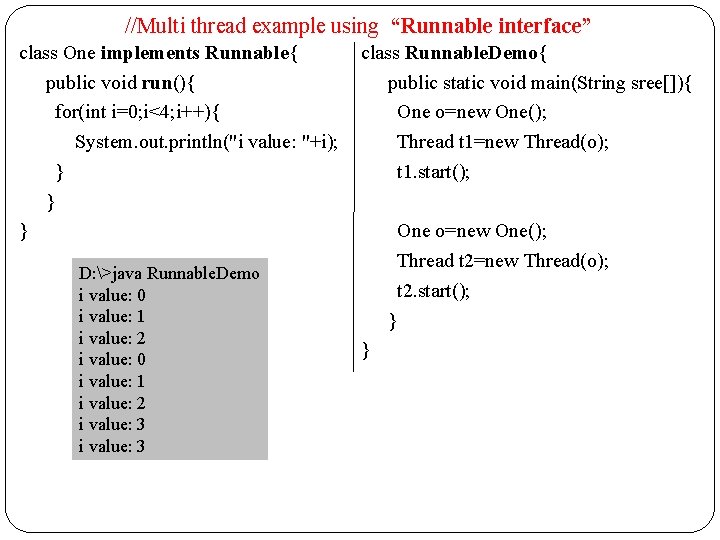
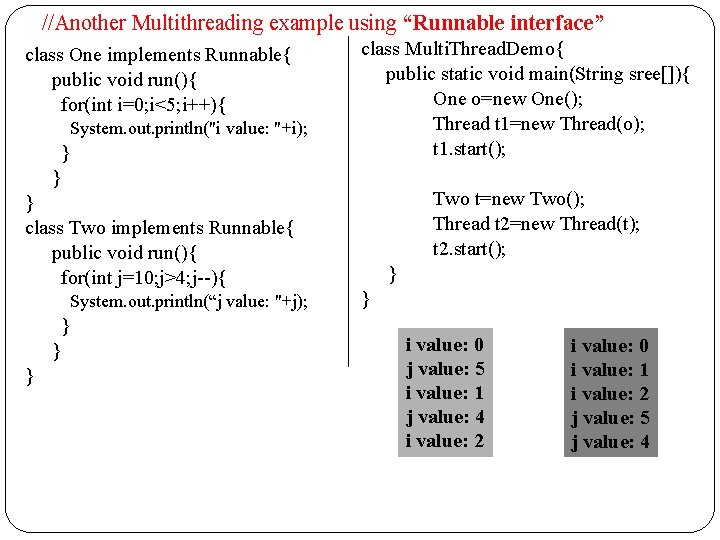
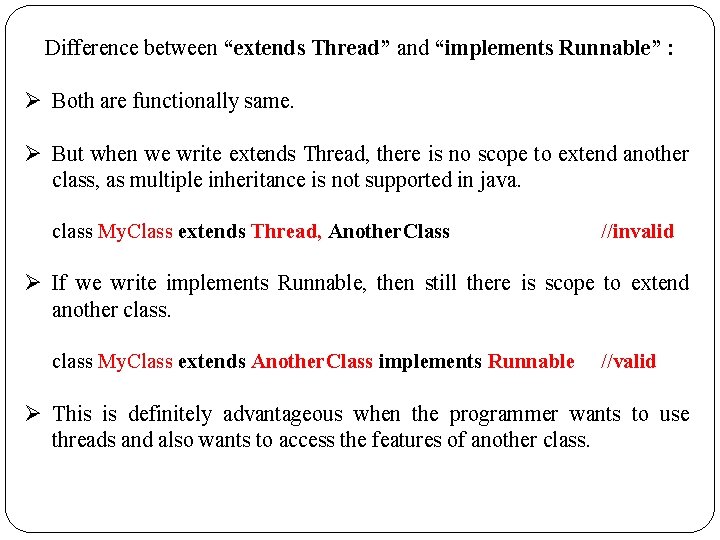
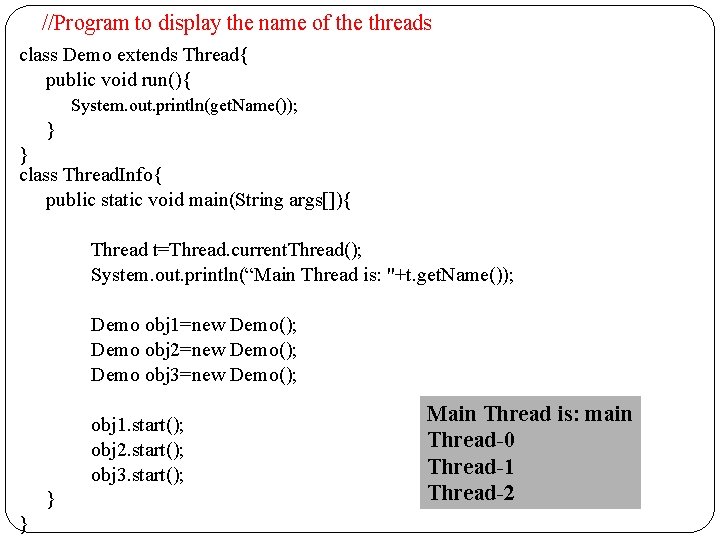
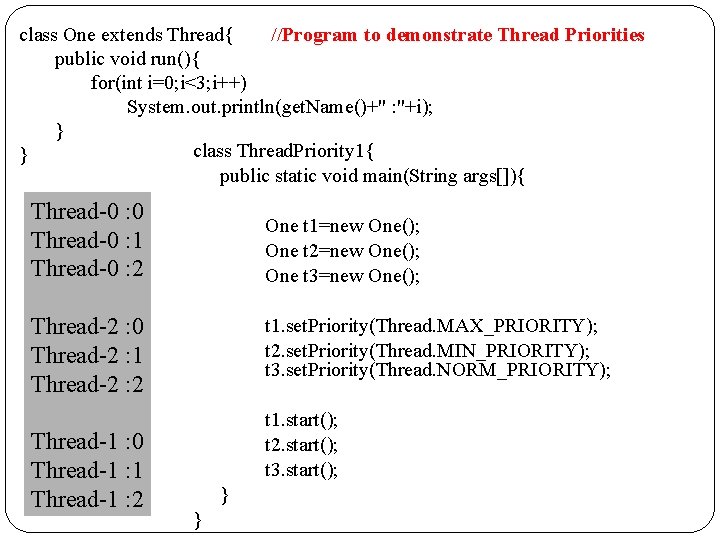
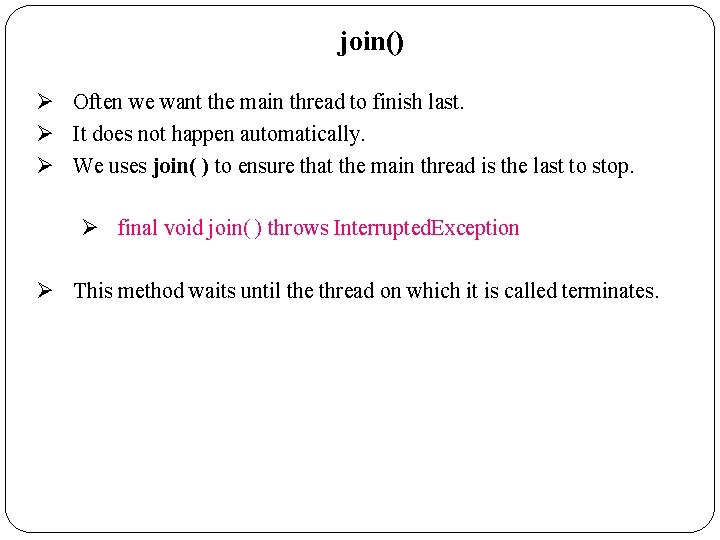
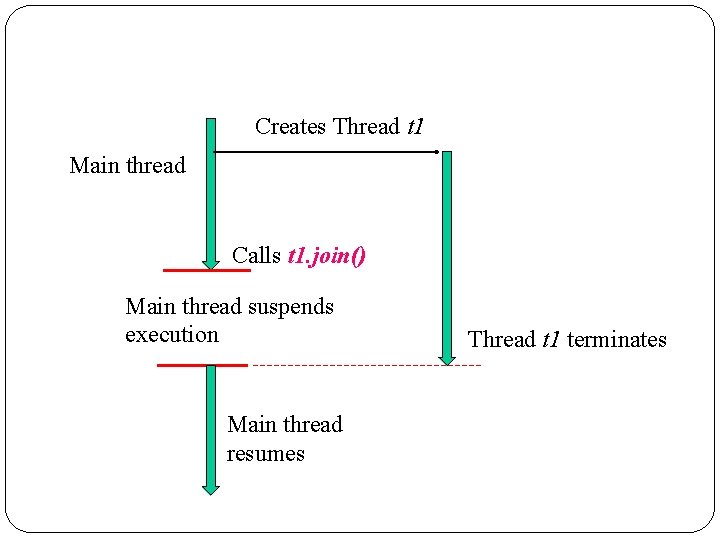
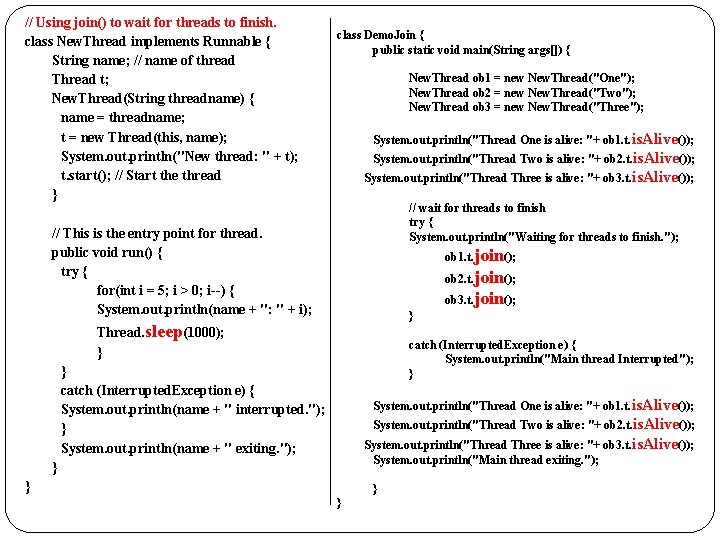
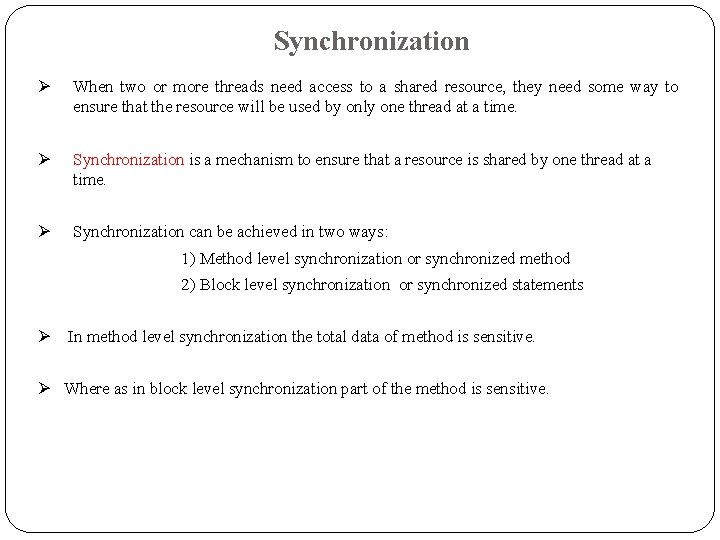
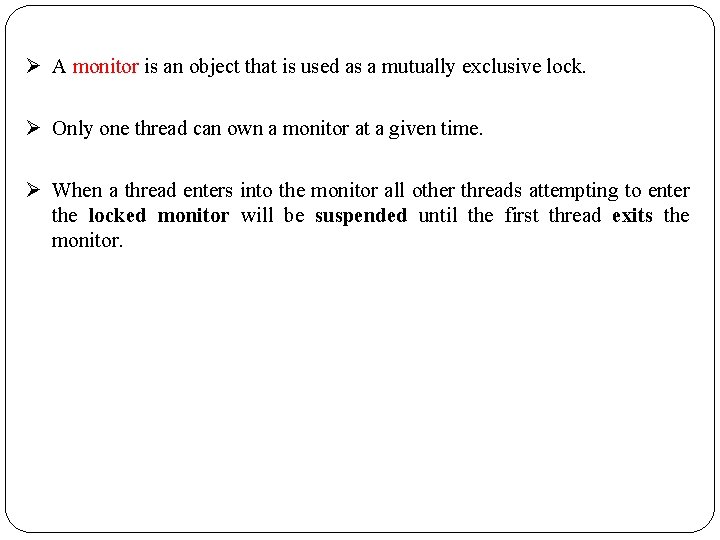
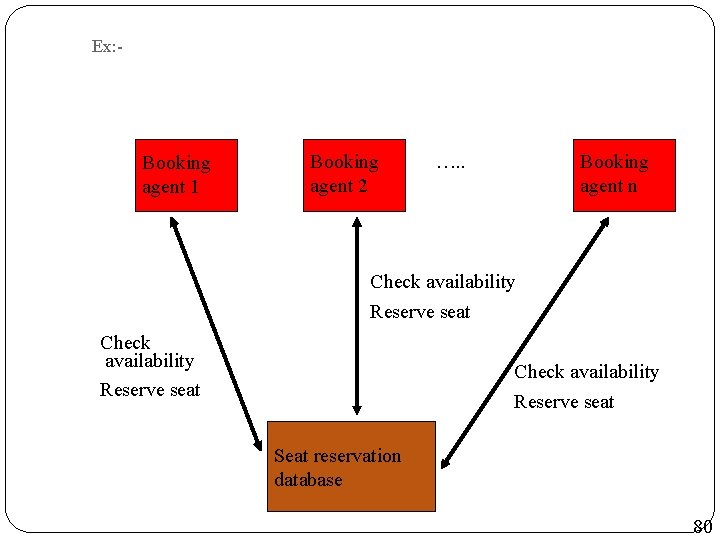
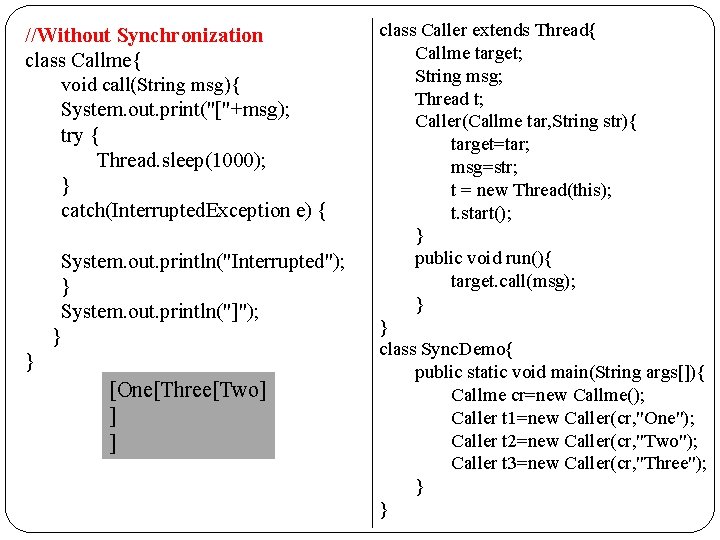
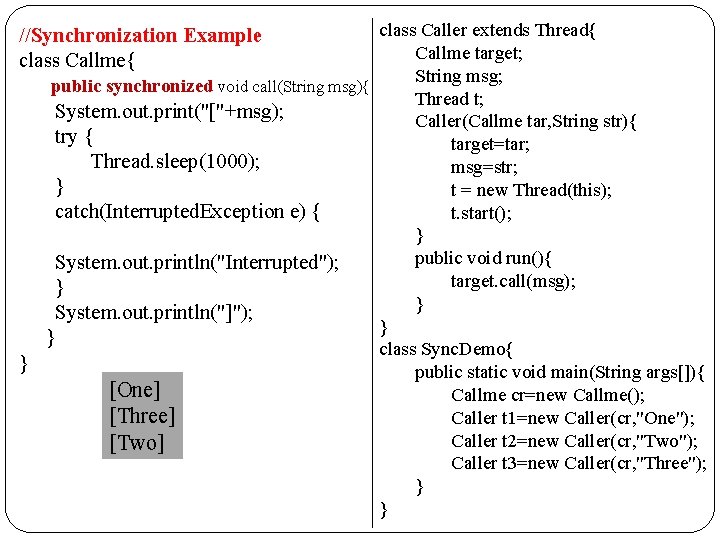
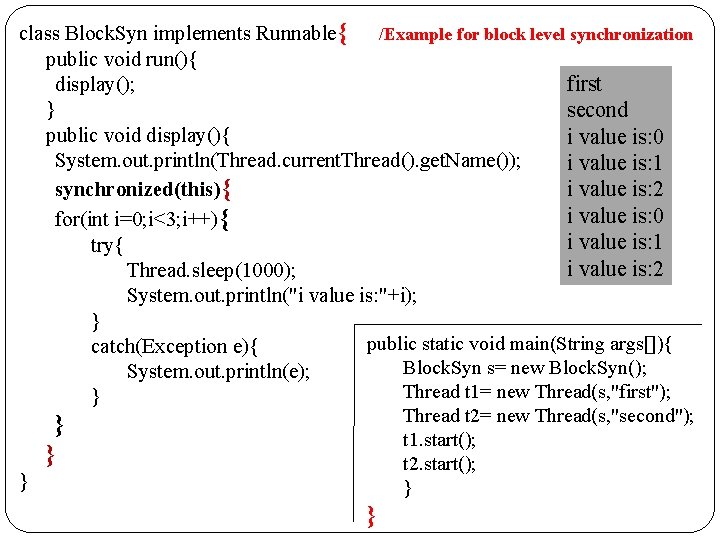
- Slides: 83
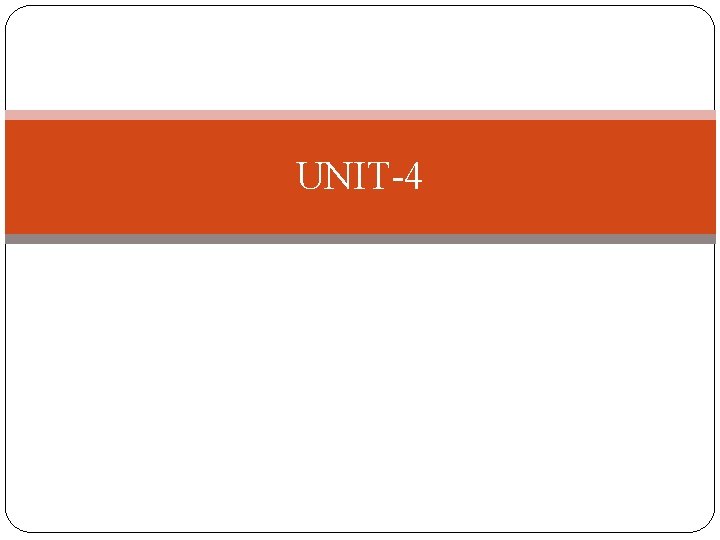
UNIT-4
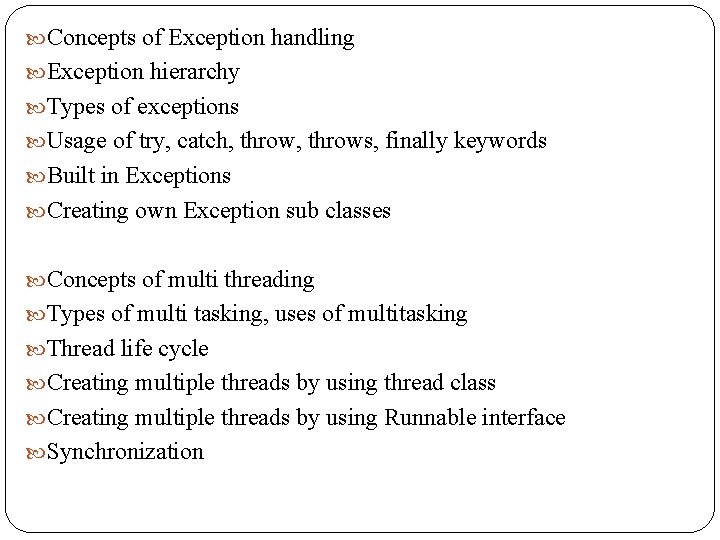
Concepts of Exception handling Exception hierarchy Types of exceptions Usage of try, catch, throws, finally keywords Built in Exceptions Creating own Exception sub classes Concepts of multi threading Types of multi tasking, uses of multitasking Thread life cycle Creating multiple threads by using thread class Creating multiple threads by using Runnable interface Synchronization
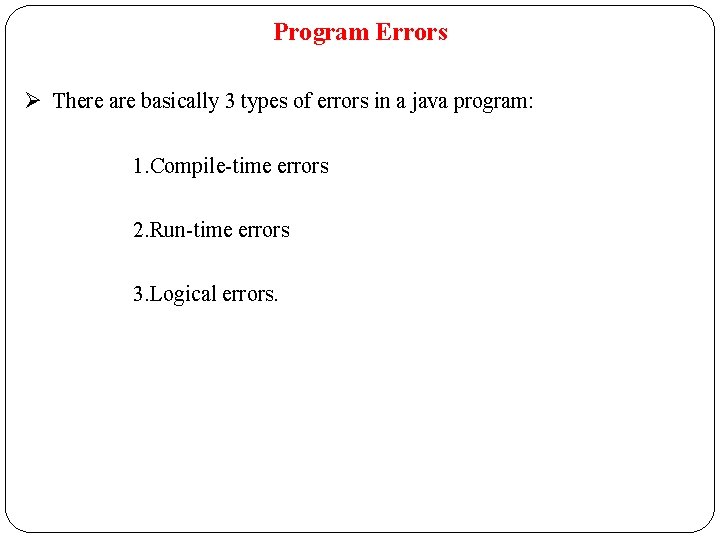
Program Errors Ø There are basically 3 types of errors in a java program: 1. Compile-time errors 2. Run-time errors 3. Logical errors.
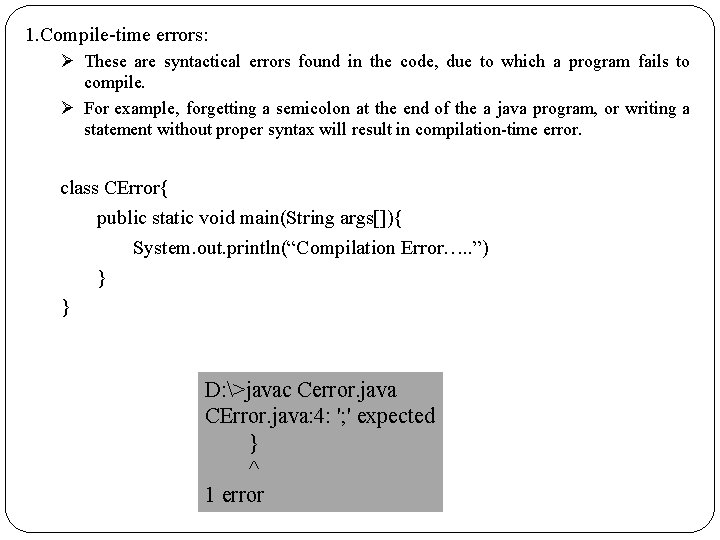
1. Compile-time errors: Ø These are syntactical errors found in the code, due to which a program fails to compile. Ø For example, forgetting a semicolon at the end of the a java program, or writing a statement without proper syntax will result in compilation-time error. class CError{ public static void main(String args[]){ System. out. println(“Compilation Error…. . ”) } } D: >javac Cerror. java CError. java: 4: '; ' expected } ^ 1 error
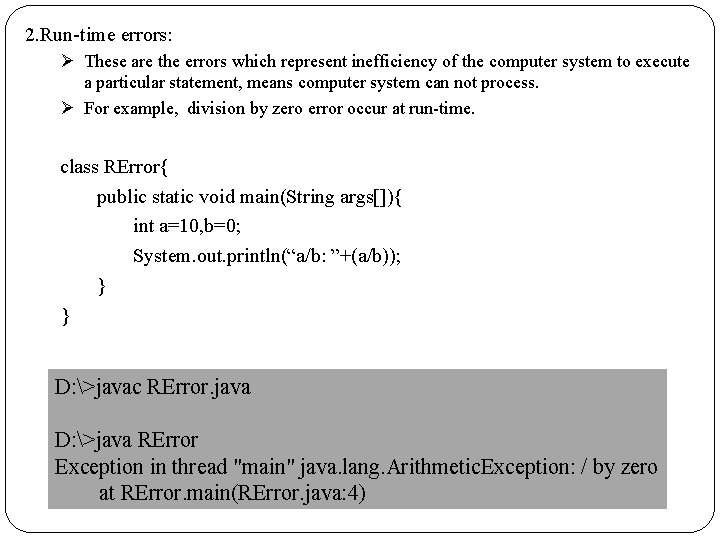
2. Run-time errors: Ø These are the errors which represent inefficiency of the computer system to execute a particular statement, means computer system can not process. Ø For example, division by zero error occur at run-time. class RError{ public static void main(String args[]){ int a=10, b=0; System. out. println(“a/b: ”+(a/b)); } } D: >javac RError. java D: >java RError Exception in thread "main" java. lang. Arithmetic. Exception: / by zero at RError. main(RError. java: 4)
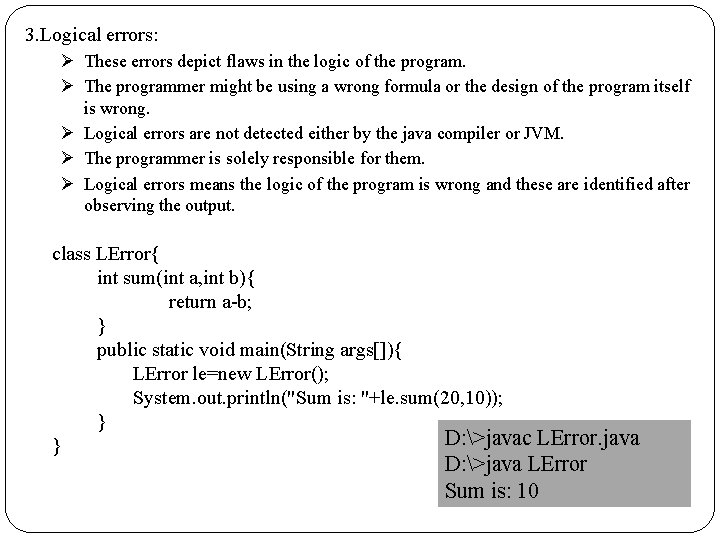
3. Logical errors: Ø These errors depict flaws in the logic of the program. Ø The programmer might be using a wrong formula or the design of the program itself is wrong. Ø Logical errors are not detected either by the java compiler or JVM. Ø The programmer is solely responsible for them. Ø Logical errors means the logic of the program is wrong and these are identified after observing the output. class LError{ int sum(int a, int b){ return a-b; } public static void main(String args[]){ LError le=new LError(); System. out. println("Sum is: "+le. sum(20, 10)); } D: >javac LError. java } D: >java LError Sum is: 10
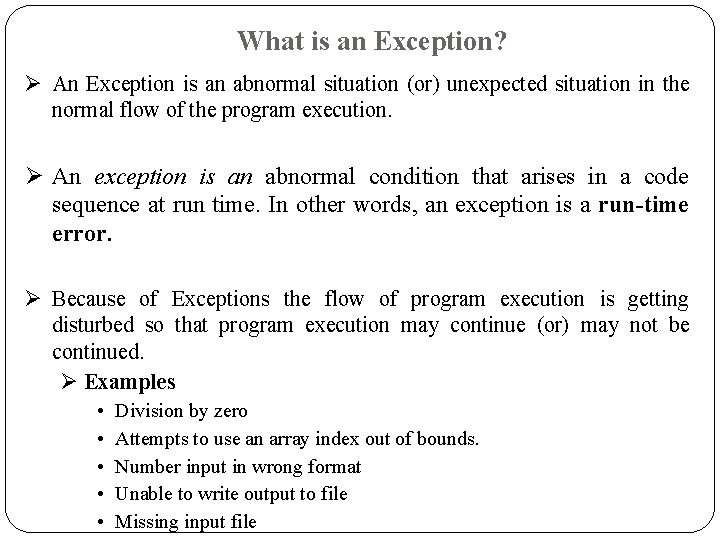
What is an Exception? Ø An Exception is an abnormal situation (or) unexpected situation in the normal flow of the program execution. Ø An exception is an abnormal condition that arises in a code sequence at run time. In other words, an exception is a run-time error. Ø Because of Exceptions the flow of program execution is getting disturbed so that program execution may continue (or) may not be continued. Ø Examples • • • Division by zero Attempts to use an array index out of bounds. Number input in wrong format Unable to write output to file Missing input file
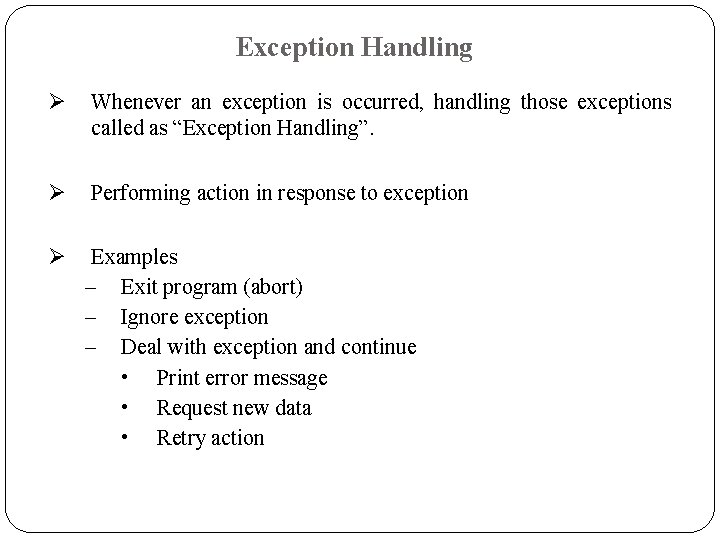
Exception Handling Ø Whenever an exception is occurred, handling those exceptions called as “Exception Handling”. Ø Performing action in response to exception Ø Examples – Exit program (abort) – Ignore exception – Deal with exception and continue • Print error message • Request new data • Retry action
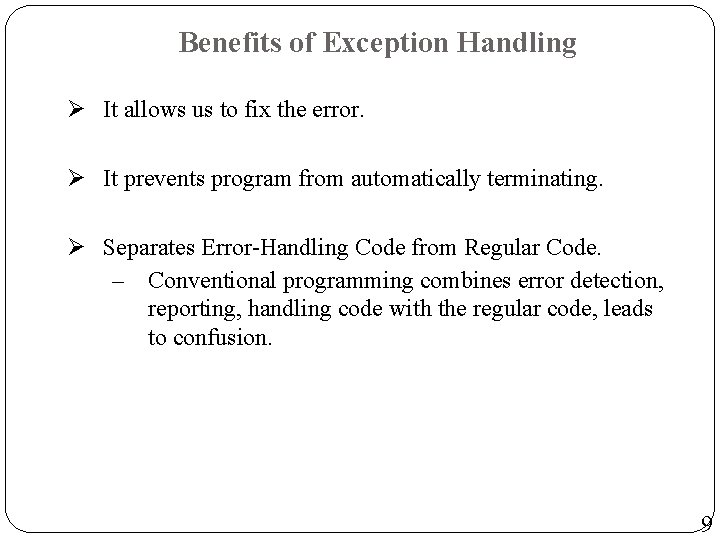
Benefits of Exception Handling Ø It allows us to fix the error. Ø It prevents program from automatically terminating. Ø Separates Error-Handling Code from Regular Code. – Conventional programming combines error detection, reporting, handling code with the regular code, leads to confusion. 9
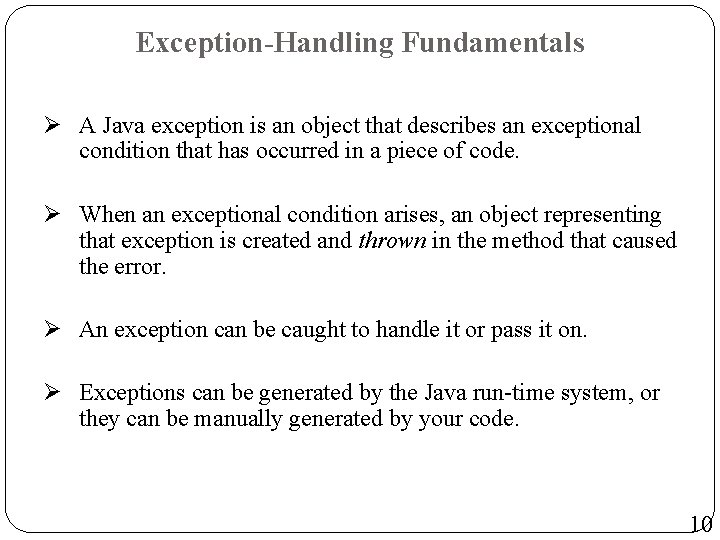
Exception-Handling Fundamentals Ø A Java exception is an object that describes an exceptional condition that has occurred in a piece of code. Ø When an exceptional condition arises, an object representing that exception is created and thrown in the method that caused the error. Ø An exception can be caught to handle it or pass it on. Ø Exceptions can be generated by the Java run-time system, or they can be manually generated by your code. 10
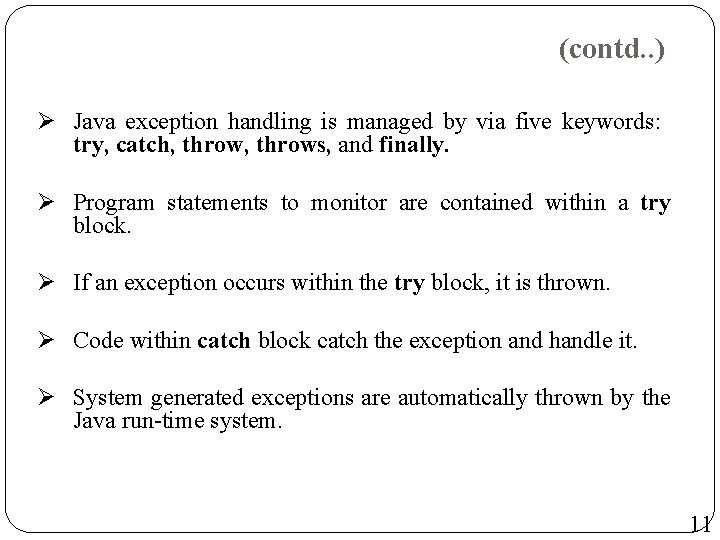
(contd. . ) Ø Java exception handling is managed by via five keywords: try, catch, throws, and finally. Ø Program statements to monitor are contained within a try block. Ø If an exception occurs within the try block, it is thrown. Ø Code within catch block catch the exception and handle it. Ø System generated exceptions are automatically thrown by the Java run-time system. 11
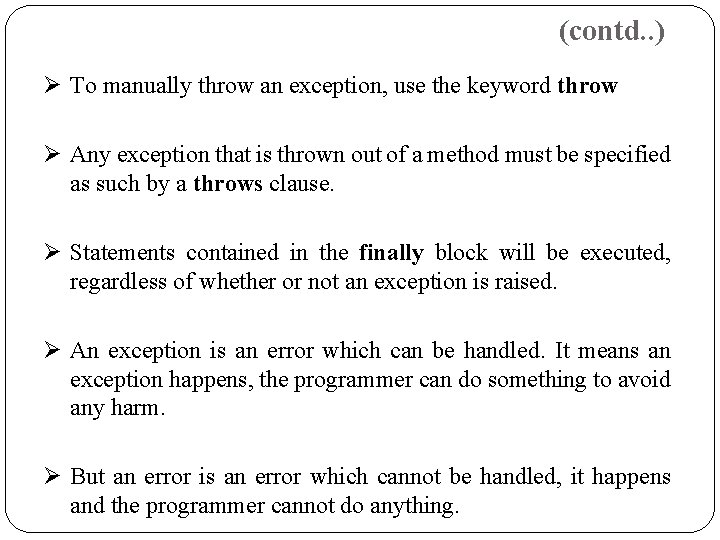
(contd. . ) Ø To manually throw an exception, use the keyword throw Ø Any exception that is thrown out of a method must be specified as such by a throws clause. Ø Statements contained in the finally block will be executed, regardless of whether or not an exception is raised. Ø An exception is an error which can be handled. It means an exception happens, the programmer can do something to avoid any harm. Ø But an error is an error which cannot be handled, it happens and the programmer cannot do anything.
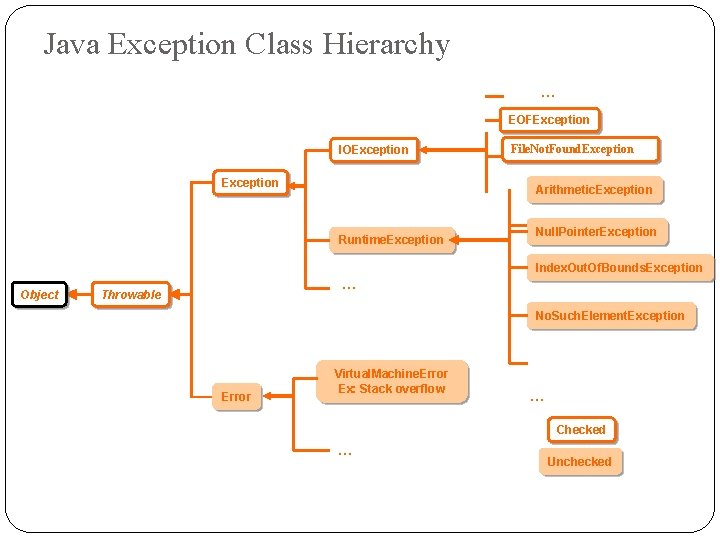
Java Exception Class Hierarchy … EOFException IOException File. Not. Found. Exception Arithmetic. Exception Runtime. Exception Null. Pointer. Exception Index. Out. Of. Bounds. Exception Object … Throwable No. Such. Element. Exception Error Virtual. Machine. Error Ex: Stack overflow … Checked … Unchecked
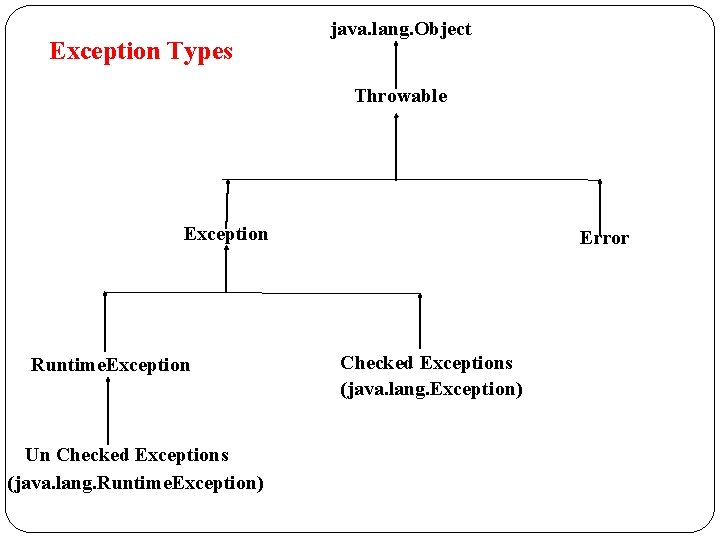
Exception Types java. lang. Object Throwable Exception Error Built –in Exception User-defined Exception Runtime. Exception Un Checked Exceptions (java. lang. Runtime. Exception) Checked Exceptions Checked (java. lang. Exception) Exceptions Unchecked Exceptions
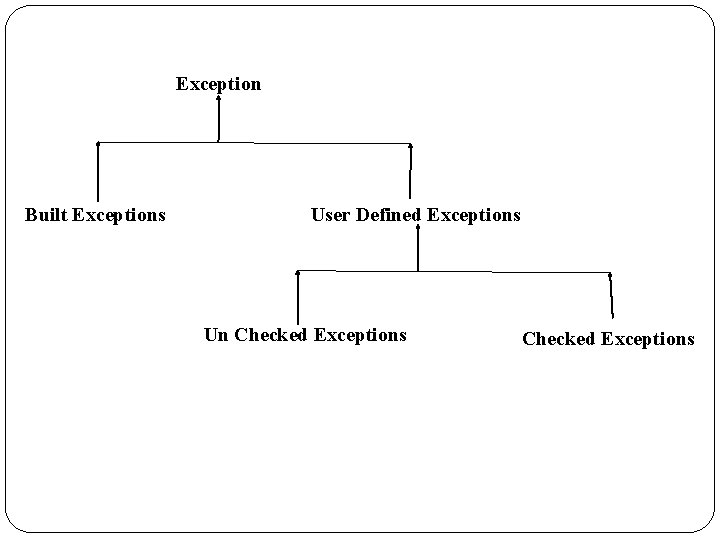
Exception Built Exceptions User Defined Exceptions Built –in Exception Un Checked Exceptions User-defined Exception Checked Exceptions Unchecked Exceptions
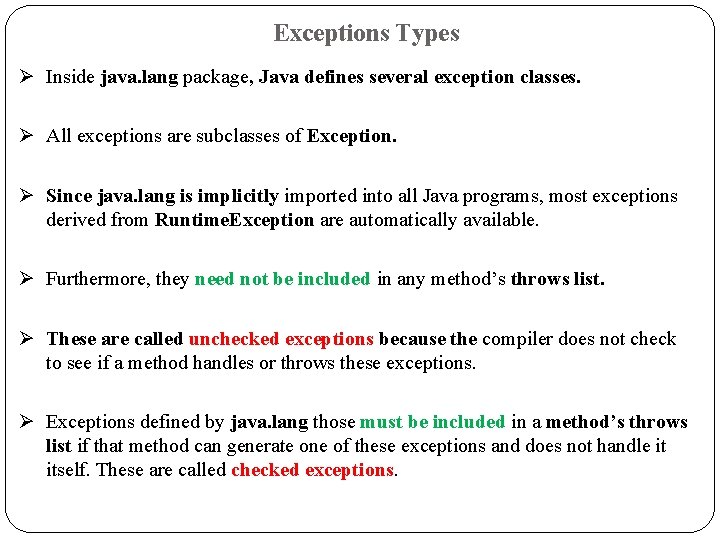
Exceptions Types Ø Inside java. lang package, Java defines several exception classes. Ø All exceptions are subclasses of Exception. Ø Since java. lang is implicitly imported into all Java programs, most exceptions derived from Runtime. Exception are automatically available. Ø Furthermore, they need not be included in any method’s throws list. Ø These are called unchecked exceptions because the compiler does not check to see if a method handles or throws these exceptions. Ø Exceptions defined by java. lang those must be included in a method’s throws list if that method can generate one of these exceptions and does not handle it itself. These are called checked exceptions.
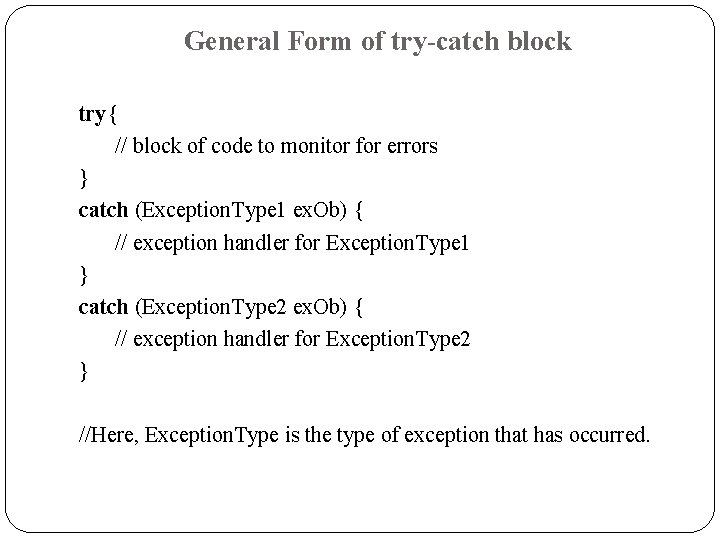
General Form of try-catch block try{ // block of code to monitor for errors } catch (Exception. Type 1 ex. Ob) { // exception handler for Exception. Type 1 } catch (Exception. Type 2 ex. Ob) { // exception handler for Exception. Type 2 } //Here, Exception. Type is the type of exception that has occurred.
![Uncaught Exceptions class Exc 0 public static void mainString args int d Uncaught Exceptions class Exc 0 { public static void main(String args[]) { int d](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-18.jpg)
Uncaught Exceptions class Exc 0 { public static void main(String args[]) { int d = 0; java. lang. Arithmetic. Exception: / by zero int a = 42 / d; at Exc 0. main(Exc 0. java: 4) } } class Exc 1 { static void subroutine() { int d = 0; int a = 10 / d; java. lang. Arithmetic. Exception: / by zero } at Exc 1. subroutine(Exc 1. java: 4) public static void main(String args[]) { at Exc 1. main(Exc 1. java: 7) Exc 1. subroutine(); } }
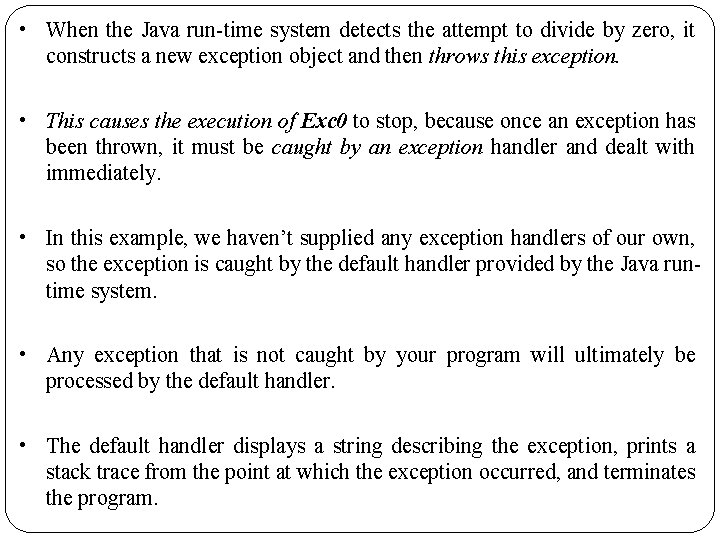
• When the Java run-time system detects the attempt to divide by zero, it constructs a new exception object and then throws this exception. • This causes the execution of Exc 0 to stop, because once an exception has been thrown, it must be caught by an exception handler and dealt with immediately. • In this example, we haven’t supplied any exception handlers of our own, so the exception is caught by the default handler provided by the Java runtime system. • Any exception that is not caught by your program will ultimately be processed by the default handler. • The default handler displays a string describing the exception, prints a stack trace from the point at which the exception occurred, and terminates the program.
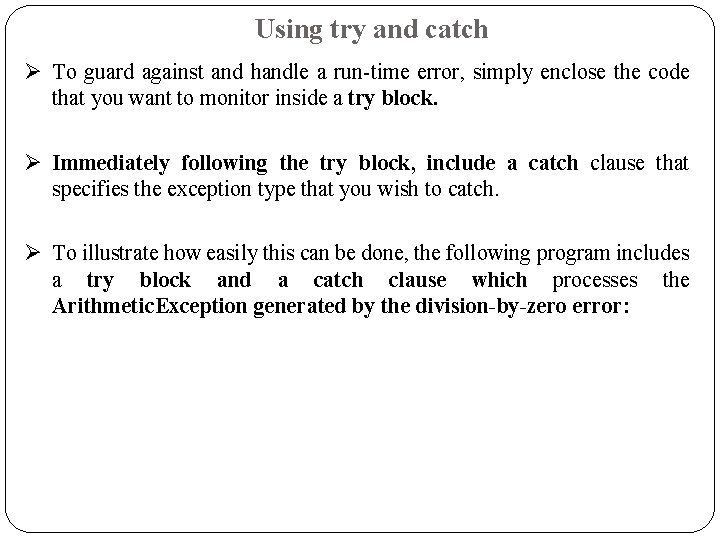
Using try and catch Ø To guard against and handle a run-time error, simply enclose the code that you want to monitor inside a try block. Ø Immediately following the try block, include a catch clause that specifies the exception type that you wish to catch. Ø To illustrate how easily this can be done, the following program includes a try block and a catch clause which processes the Arithmetic. Exception generated by the division-by-zero error:
![class Exc 2 public static void mainString args int d a try class Exc 2 { public static void main(String args[]) { int d, a; try](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-21.jpg)
class Exc 2 { public static void main(String args[]) { int d, a; try { // monitor a block of code. d = 0; a = 42 / d; System. out. println("This will not be printed. "); } catch (Arithmetic. Exception e) { // catch divide-by-zero error System. out. println("Division by zero. "); } System. out. println("After catch statement. "); } } Output: Division by zero. After catch statement.
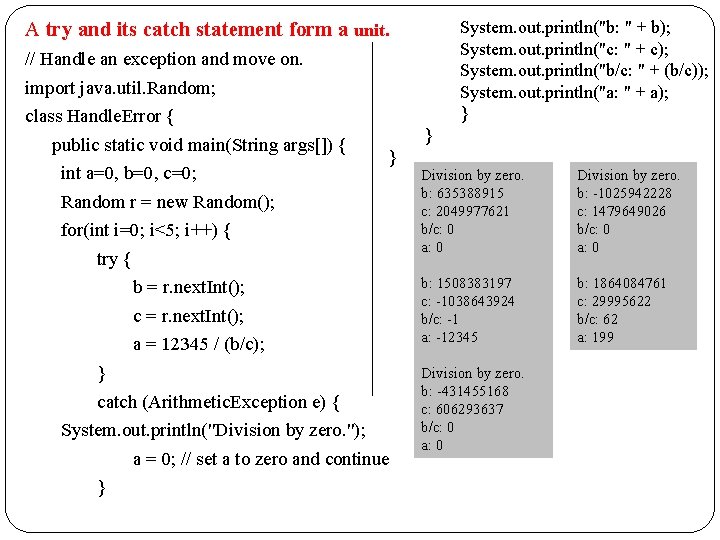
System. out. println("b: " + b); System. out. println("c: " + c); System. out. println("b/c: " + (b/c)); System. out. println("a: " + a); } A try and its catch statement form a unit. // Handle an exception and move on. import java. util. Random; class Handle. Error { public static void main(String args[]) { int a=0, b=0, c=0; } } Random r = new Random(); for(int i=0; i<5; i++) { try { b = r. next. Int(); c = r. next. Int(); a = 12345 / (b/c); } catch (Arithmetic. Exception e) { System. out. println("Division by zero. "); a = 0; // set a to zero and continue } Division by zero. b: 635388915 c: 2049977621 b/c: 0 a: 0 Division by zero. b: -1025942228 c: 1479649026 b/c: 0 a: 0 b: 1508383197 c: -1038643924 b/c: -1 a: -12345 b: 1864084761 c: 29995622 b/c: 62 a: 199 Division by zero. b: -431455168 c: 606293637 b/c: 0 a: 0
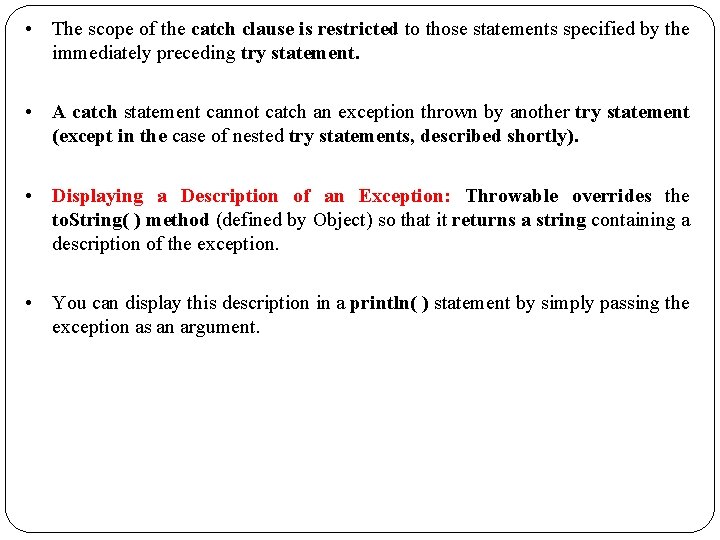
• The scope of the catch clause is restricted to those statements specified by the immediately preceding try statement. • A catch statement cannot catch an exception thrown by another try statement (except in the case of nested try statements, described shortly). • Displaying a Description of an Exception: Throwable overrides the to. String( ) method (defined by Object) so that it returns a string containing a description of the exception. • You can display this description in a println( ) statement by simply passing the exception as an argument.
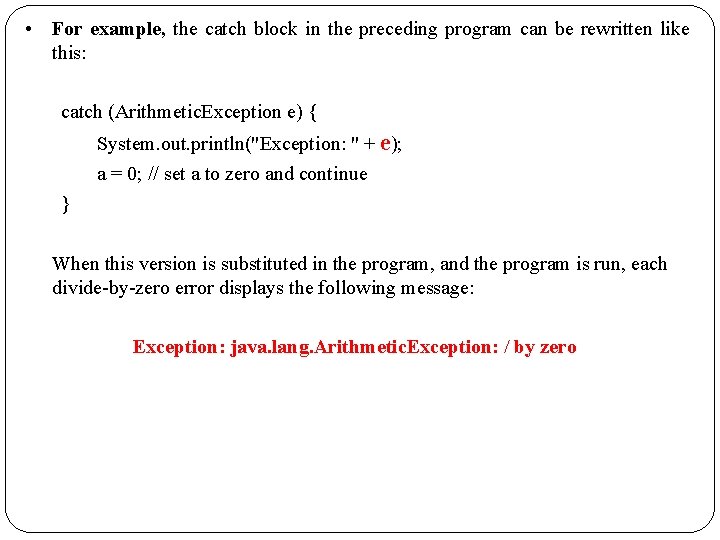
• For example, the catch block in the preceding program can be rewritten like this: catch (Arithmetic. Exception e) { System. out. println("Exception: " + e); a = 0; // set a to zero and continue } When this version is substituted in the program, and the program is run, each divide-by-zero error displays the following message: Exception: java. lang. Arithmetic. Exception: / by zero
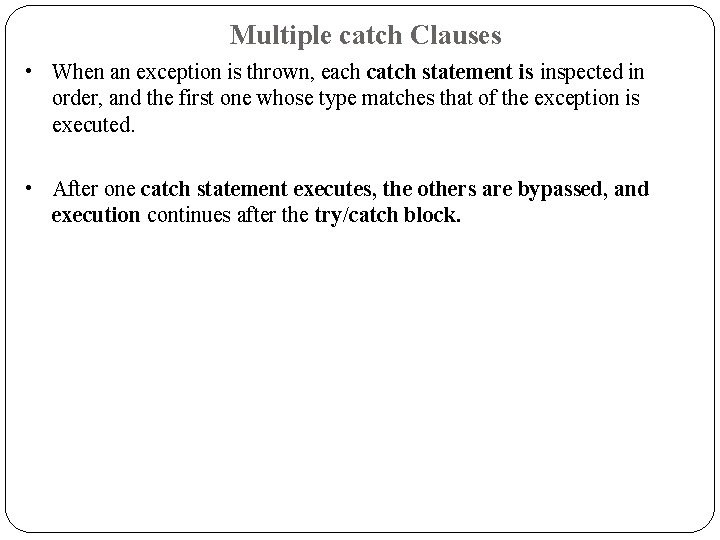
Multiple catch Clauses • When an exception is thrown, each catch statement is inspected in order, and the first one whose type matches that of the exception is executed. • After one catch statement executes, the others are bypassed, and execution continues after the try/catch block.
![class Multi Catch Demonstrate multiple catch statements public static void mainString args class Multi. Catch { // Demonstrate multiple catch statements. public static void main(String args[])](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-26.jpg)
class Multi. Catch { // Demonstrate multiple catch statements. public static void main(String args[]) { try { int a = args. length; System. out. println("a = " + a); int b = 42 / a; int c[] = { 1 }; c[42] = 99; } catch(Arithmetic. Exception e) { System. out. println("Divide by 0: " + e); } catch(Array. Index. Out. Of. Bounds. Exception e) { System. out. println("Array index oob: " + e); } System. out. println("After try/catch blocks. "); } C: >java Multi. Catch } a = 0 Divide by 0: java. lang. Arithmetic. Exception: / by zero After try/catch blocks. C: >java Multi. Catch Test. Arg a = 1 Array index oob: java. lang. Array. Index. Out. Of. Bounds. Exception After try/catch blocks.
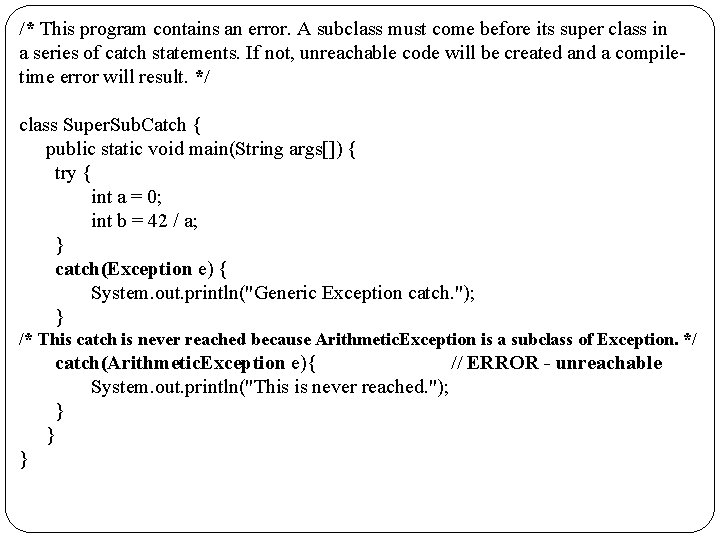
/* This program contains an error. A subclass must come before its super class in a series of catch statements. If not, unreachable code will be created and a compiletime error will result. */ class Super. Sub. Catch { public static void main(String args[]) { try { int a = 0; int b = 42 / a; } catch(Exception e) { System. out. println("Generic Exception catch. "); } /* This catch is never reached because Arithmetic. Exception is a subclass of Exception. */ catch(Arithmetic. Exception e){ // ERROR - unreachable System. out. println("This is never reached. "); } } }
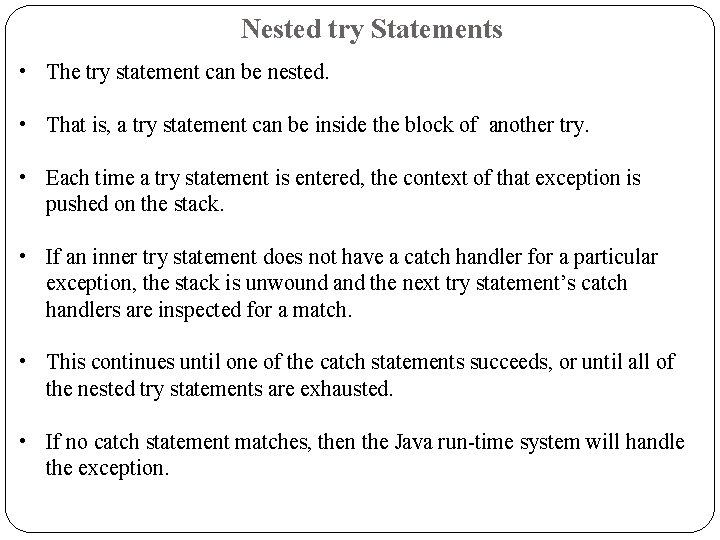
Nested try Statements • The try statement can be nested. • That is, a try statement can be inside the block of another try. • Each time a try statement is entered, the context of that exception is pushed on the stack. • If an inner try statement does not have a catch handler for a particular exception, the stack is unwound and the next try statement’s catch handlers are inspected for a match. • This continues until one of the catch statements succeeds, or until all of the nested try statements are exhausted. • If no catch statement matches, then the Java run-time system will handle the exception.
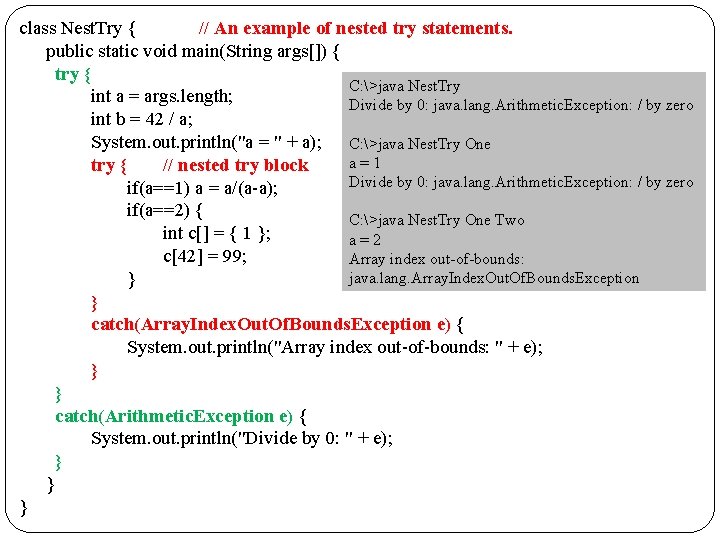
class Nest. Try { // An example of nested try statements. public static void main(String args[]) { try { C: >java Nest. Try int a = args. length; Divide by 0: java. lang. Arithmetic. Exception: / by zero int b = 42 / a; System. out. println("a = " + a); C: >java Nest. Try One a = 1 try { // nested try block Divide by 0: java. lang. Arithmetic. Exception: / by zero if(a==1) a = a/(a-a); if(a==2) { C: >java Nest. Try One Two int c[] = { 1 }; a = 2 c[42] = 99; Array index out-of-bounds: java. lang. Array. Index. Out. Of. Bounds. Exception } } catch(Array. Index. Out. Of. Bounds. Exception e) { System. out. println("Array index out-of-bounds: " + e); } } catch(Arithmetic. Exception e) { System. out. println("Divide by 0: " + e); } } }
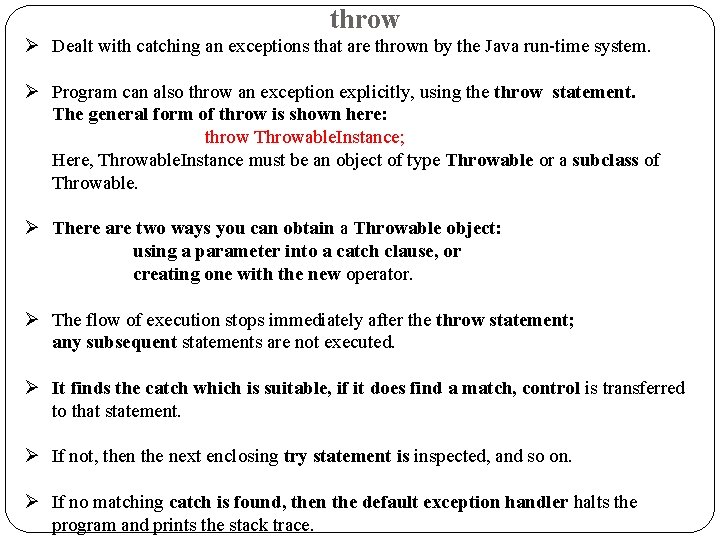
throw Ø Dealt with catching an exceptions that are thrown by the Java run-time system. Ø Program can also throw an exception explicitly, using the throw statement. The general form of throw is shown here: throw Throwable. Instance; Here, Throwable. Instance must be an object of type Throwable or a subclass of Throwable. Ø There are two ways you can obtain a Throwable object: using a parameter into a catch clause, or creating one with the new operator. Ø The flow of execution stops immediately after the throw statement; any subsequent statements are not executed. Ø It finds the catch which is suitable, if it does find a match, control is transferred to that statement. Ø If not, then the next enclosing try statement is inspected, and so on. Ø If no matching catch is found, then the default exception handler halts the program and prints the stack trace.
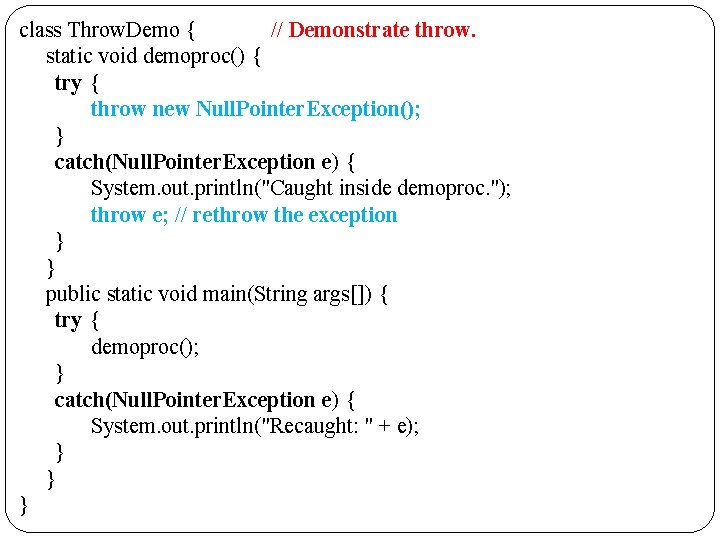
class Throw. Demo { // Demonstrate throw. static void demoproc() { try { throw new Null. Pointer. Exception(); } catch(Null. Pointer. Exception e) { System. out. println("Caught inside demoproc. "); throw e; // rethrow the exception } } public static void main(String args[]) { try { demoproc(); } catch(Null. Pointer. Exception e) { System. out. println("Recaught: " + e); } } }
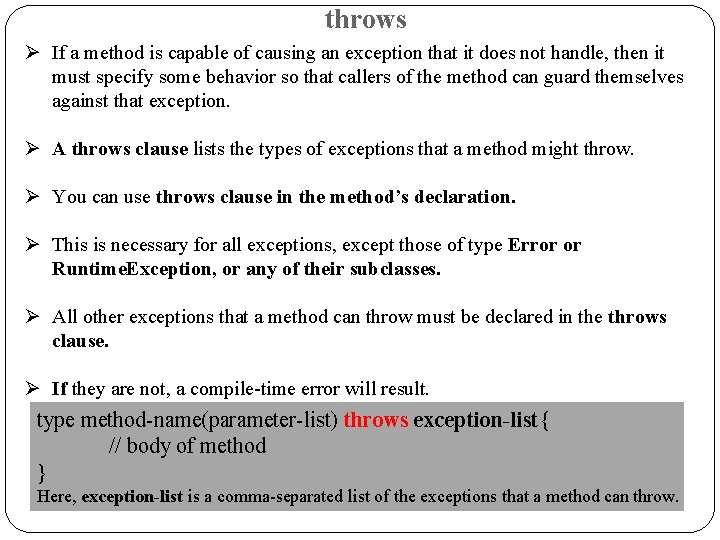
throws Ø If a method is capable of causing an exception that it does not handle, then it must specify some behavior so that callers of the method can guard themselves against that exception. Ø A throws clause lists the types of exceptions that a method might throw. Ø You can use throws clause in the method’s declaration. Ø This is necessary for all exceptions, except those of type Error or Runtime. Exception, or any of their subclasses. Ø All other exceptions that a method can throw must be declared in the throws clause. Ø If they are not, a compile-time error will result. type method-name(parameter-list) throws exception-list{ // body of method } Here, exception-list is a comma-separated list of the exceptions that a method can throw.
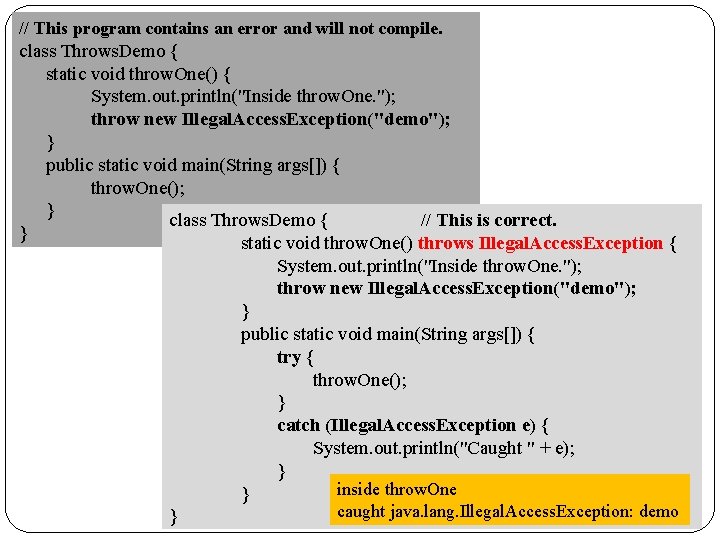
// This program contains an error and will not compile. class Throws. Demo { static void throw. One() { System. out. println("Inside throw. One. "); throw new Illegal. Access. Exception("demo"); } public static void main(String args[]) { throw. One(); } class Throws. Demo { // This is correct. } static void throw. One() throws Illegal. Access. Exception { System. out. println("Inside throw. One. "); throw new Illegal. Access. Exception("demo"); } public static void main(String args[]) { try { throw. One(); } catch (Illegal. Access. Exception e) { System. out. println("Caught " + e); } inside throw. One } caught java. lang. Illegal. Access. Exception: demo }
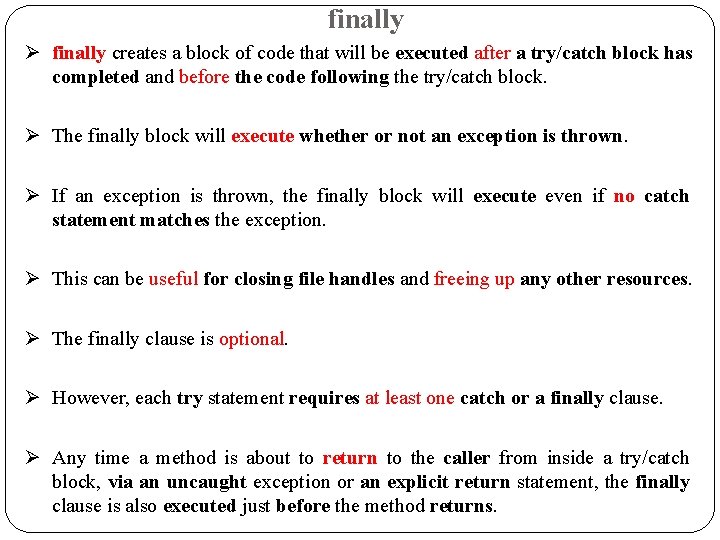
finally Ø finally creates a block of code that will be executed after a try/catch block has completed and before the code following the try/catch block. Ø The finally block will execute whether or not an exception is thrown. Ø If an exception is thrown, the finally block will execute even if no catch statement matches the exception. Ø This can be useful for closing file handles and freeing up any other resources. Ø The finally clause is optional. Ø However, each try statement requires at least one catch or a finally clause. Ø Any time a method is about to return to the caller from inside a try/catch block, via an uncaught exception or an explicit return statement, the finally clause is also executed just before the method returns.
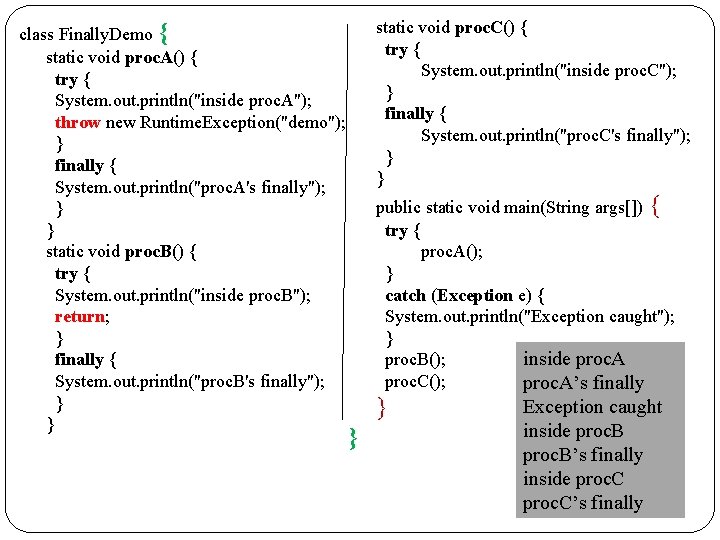
class Finally. Demo { static void proc. A() { try { System. out. println("inside proc. A"); throw new Runtime. Exception("demo"); } finally { System. out. println("proc. A's finally"); } } static void proc. B() { try { System. out. println("inside proc. B"); return; } finally { System. out. println("proc. B's finally"); } } static void proc. C() { try { System. out. println("inside proc. C"); } finally { System. out. println("proc. C's finally"); } } public static void main(String args[]) { try { proc. A(); } catch (Exception e) { System. out. println("Exception caught"); } inside proc. A proc. B(); proc. C(); proc. A’s finally } } Exception caught inside proc. B’s finally inside proc. C’s finally
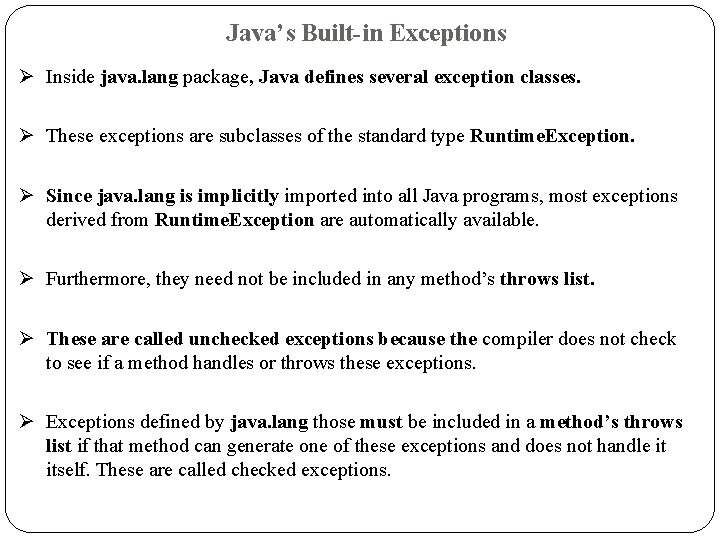
Java’s Built-in Exceptions Ø Inside java. lang package, Java defines several exception classes. Ø These exceptions are subclasses of the standard type Runtime. Exception. Ø Since java. lang is implicitly imported into all Java programs, most exceptions derived from Runtime. Exception are automatically available. Ø Furthermore, they need not be included in any method’s throws list. Ø These are called unchecked exceptions because the compiler does not check to see if a method handles or throws these exceptions. Ø Exceptions defined by java. lang those must be included in a method’s throws list if that method can generate one of these exceptions and does not handle it itself. These are called checked exceptions.
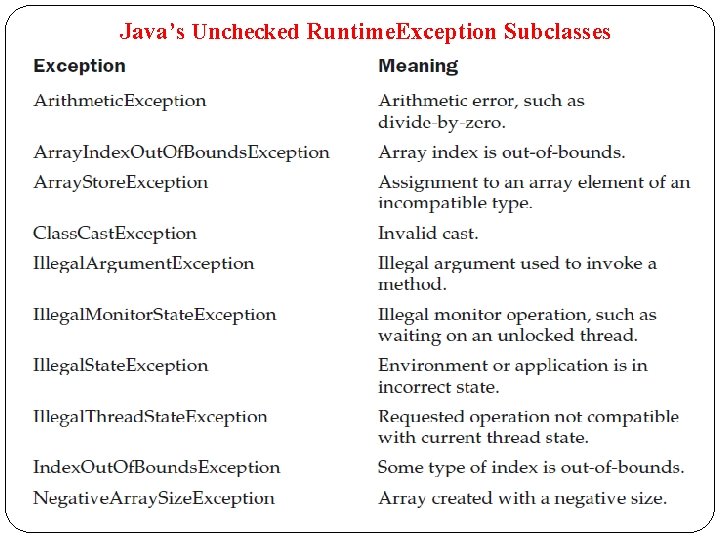
Java’s Unchecked Runtime. Exception Subclasses
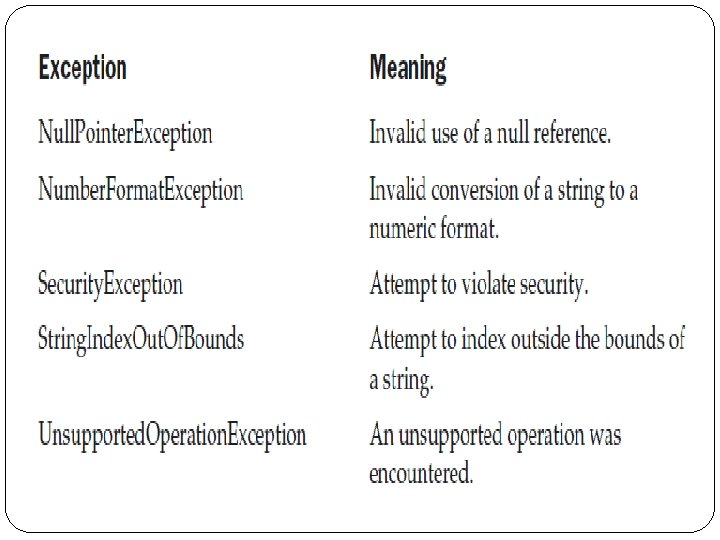
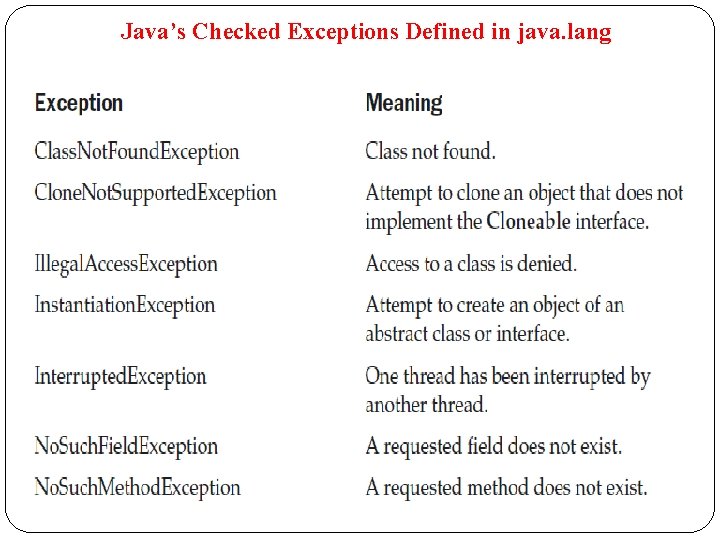
Java’s Checked Exceptions Defined in java. lang
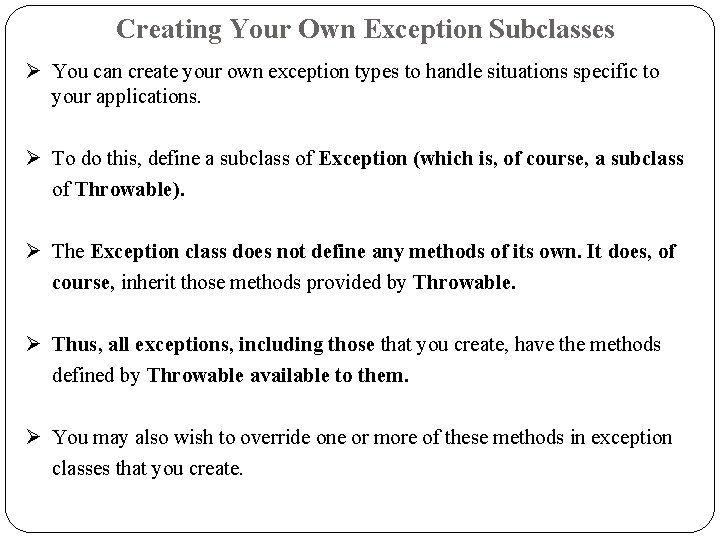
Creating Your Own Exception Subclasses Ø You can create your own exception types to handle situations specific to your applications. Ø To do this, define a subclass of Exception (which is, of course, a subclass of Throwable). Ø The Exception class does not define any methods of its own. It does, of course, inherit those methods provided by Throwable. Ø Thus, all exceptions, including those that you create, have the methods defined by Throwable available to them. Ø You may also wish to override one or more of these methods in exception classes that you create.
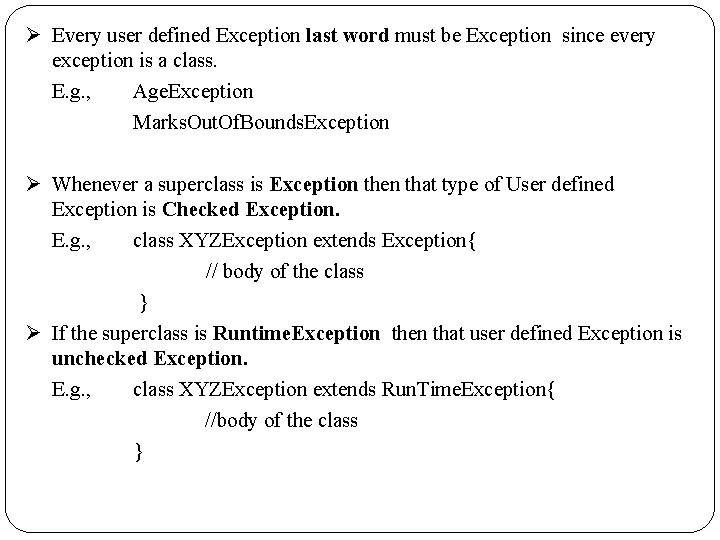
Ø Every user defined Exception last word must be Exception since every exception is a class. E. g. , Age. Exception Marks. Out. Of. Bounds. Exception Ø Whenever a superclass is Exception then that type of User defined Exception is Checked Exception. E. g. , class XYZException extends Exception{ // body of the class } Ø If the superclass is Runtime. Exception then that user defined Exception is unchecked Exception. E. g. , class XYZException extends Run. Time. Exception{ //body of the class }
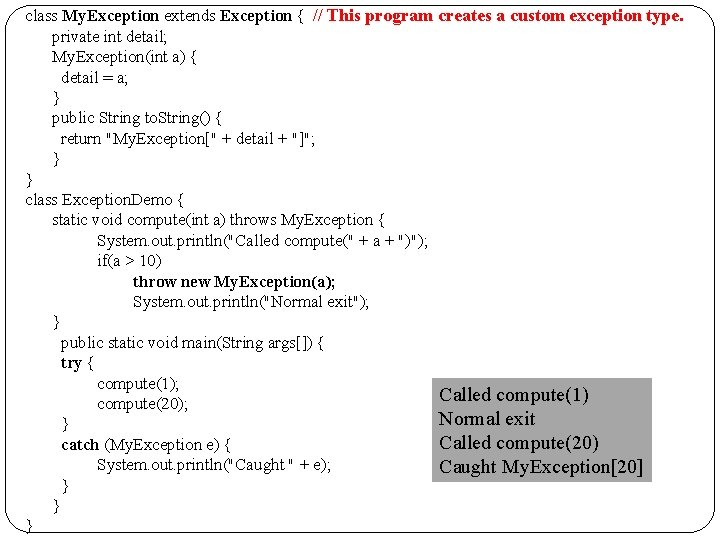
class My. Exception extends Exception { // This program creates a custom exception type. private int detail; My. Exception(int a) { detail = a; } public String to. String() { return "My. Exception[" + detail + "]"; } } class Exception. Demo { static void compute(int a) throws My. Exception { System. out. println("Called compute(" + a + ")"); if(a > 10) throw new My. Exception(a); System. out. println("Normal exit"); } public static void main(String args[]) { try { compute(1); Called compute(1) compute(20); Normal exit } Called compute(20) catch (My. Exception e) { System. out. println("Caught " + e); Caught My. Exception[20] } } }
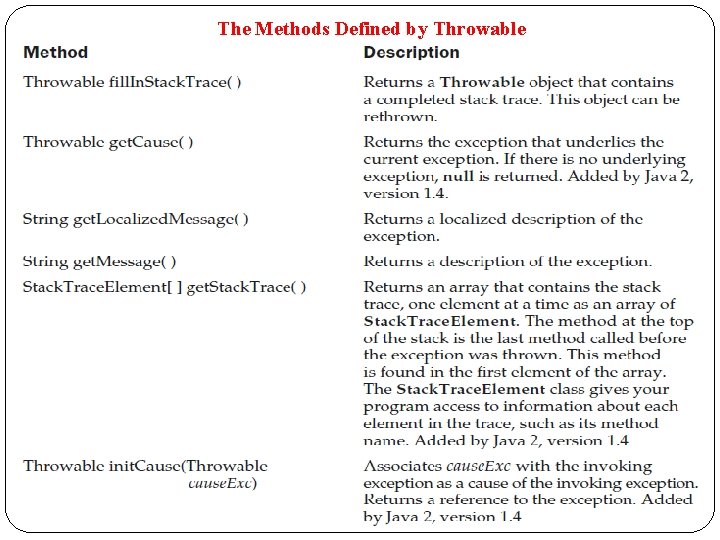
The Methods Defined by Throwable
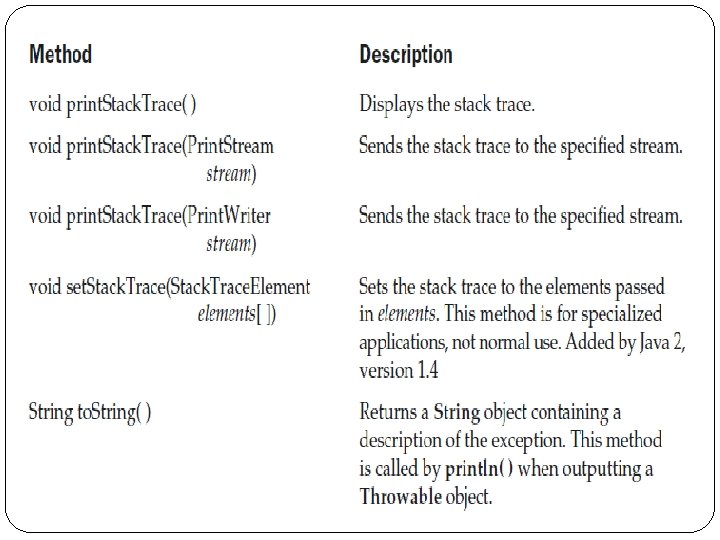
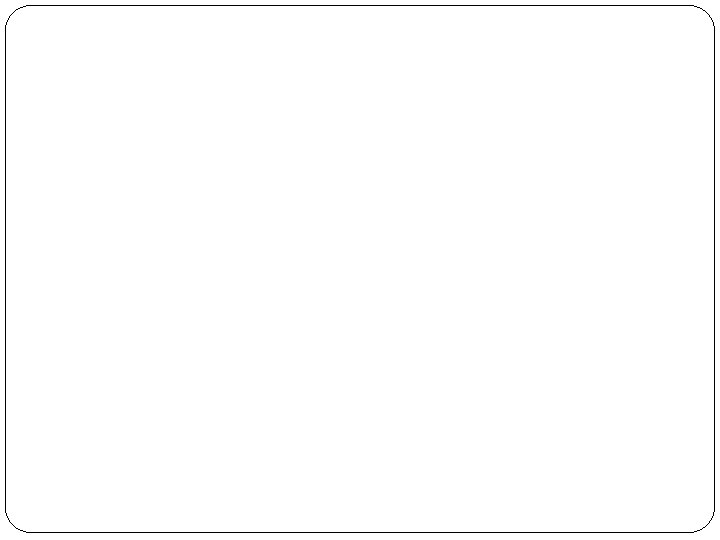
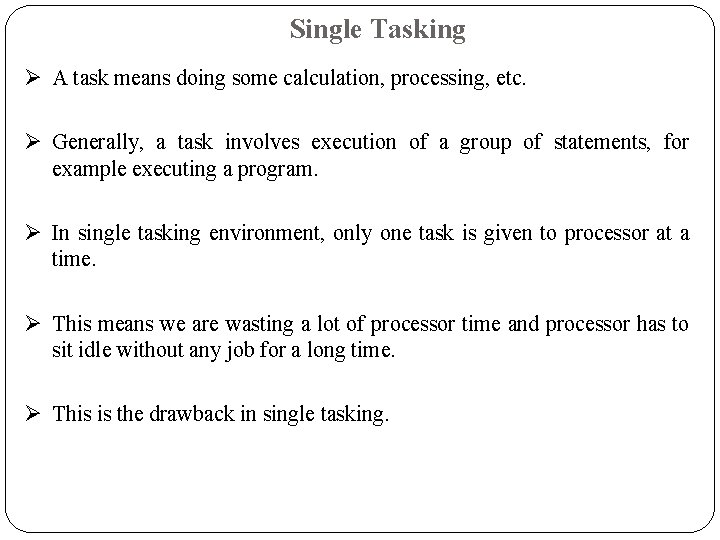
Single Tasking Ø A task means doing some calculation, processing, etc. Ø Generally, a task involves execution of a group of statements, for example executing a program. Ø In single tasking environment, only one task is given to processor at a time. Ø This means we are wasting a lot of processor time and processor has to sit idle without any job for a long time. Ø This is the drawback in single tasking.
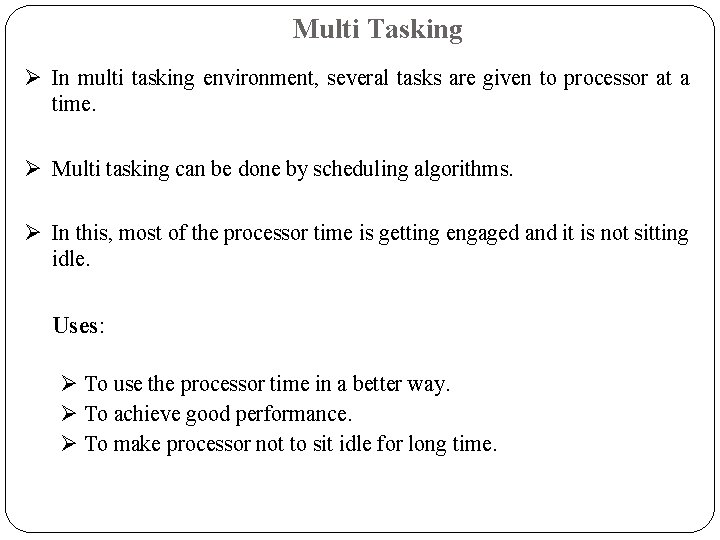
Multi Tasking Ø In multi tasking environment, several tasks are given to processor at a time. Ø Multi tasking can be done by scheduling algorithms. Ø In this, most of the processor time is getting engaged and it is not sitting idle. Uses: Ø To use the processor time in a better way. Ø To achieve good performance. Ø To make processor not to sit idle for long time.
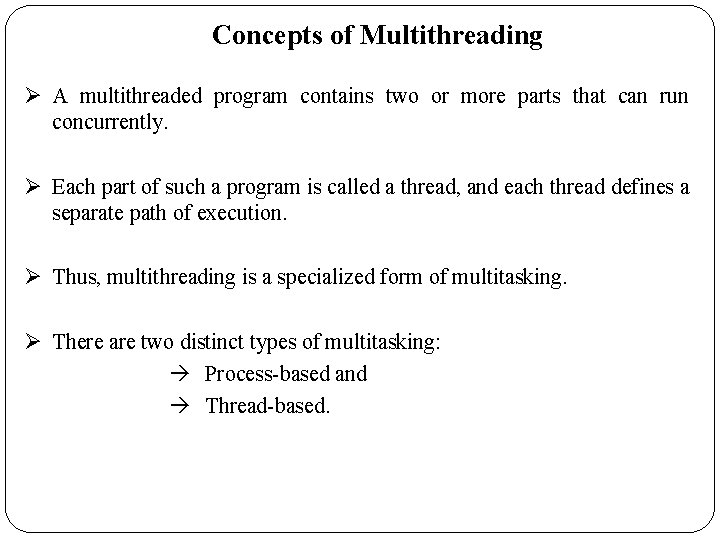
Concepts of Multithreading Ø A multithreaded program contains two or more parts that can run concurrently. Ø Each part of such a program is called a thread, and each thread defines a separate path of execution. Ø Thus, multithreading is a specialized form of multitasking. Ø There are two distinct types of multitasking: Process-based and Thread-based.
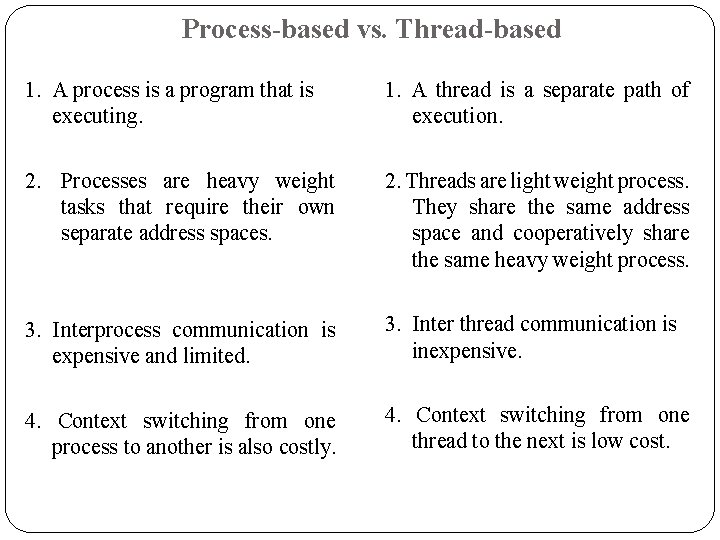
Process-based vs. Thread-based 1. A process is a program that is executing. 1. A thread is a separate path of execution. 2. Processes are heavy weight tasks that require their own separate address spaces. 2. Threads are light weight process. They share the same address space and cooperatively share the same heavy weight process. 3. Interprocess communication is expensive and limited. 3. Inter thread communication is inexpensive. 4. Context switching from one process to another is also costly. 4. Context switching from one thread to the next is low cost.
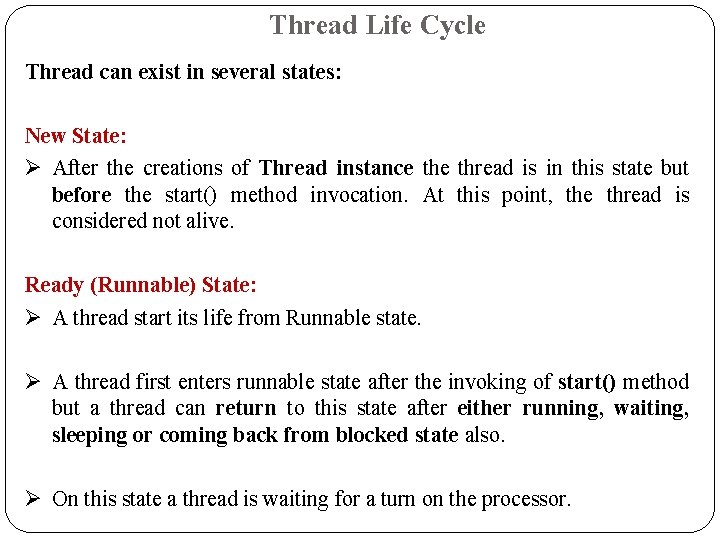
Thread Life Cycle Thread can exist in several states: New State: Ø After the creations of Thread instance thread is in this state but before the start() method invocation. At this point, the thread is considered not alive. Ready (Runnable) State: Ø A thread start its life from Runnable state. Ø A thread first enters runnable state after the invoking of start() method but a thread can return to this state after either running, waiting, sleeping or coming back from blocked state also. Ø On this state a thread is waiting for a turn on the processor.
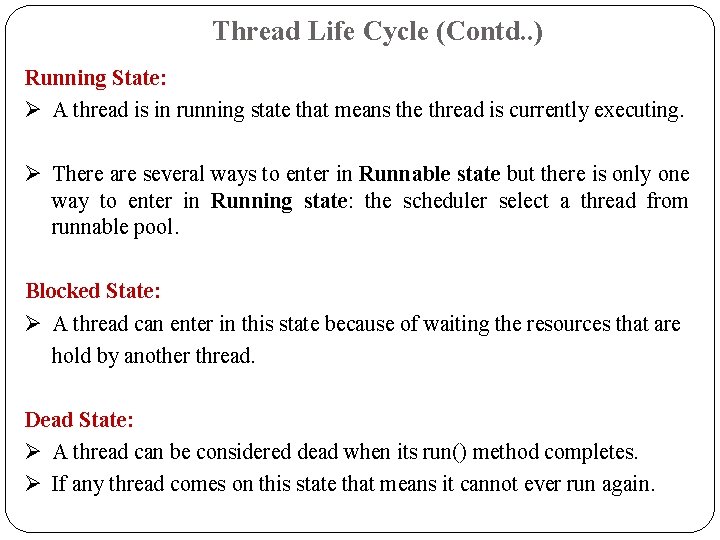
Thread Life Cycle (Contd. . ) Running State: Ø A thread is in running state that means the thread is currently executing. Ø There are several ways to enter in Runnable state but there is only one way to enter in Running state: the scheduler select a thread from runnable pool. Blocked State: Ø A thread can enter in this state because of waiting the resources that are hold by another thread. Dead State: Ø A thread can be considered dead when its run() method completes. Ø If any thread comes on this state that means it cannot ever run again.
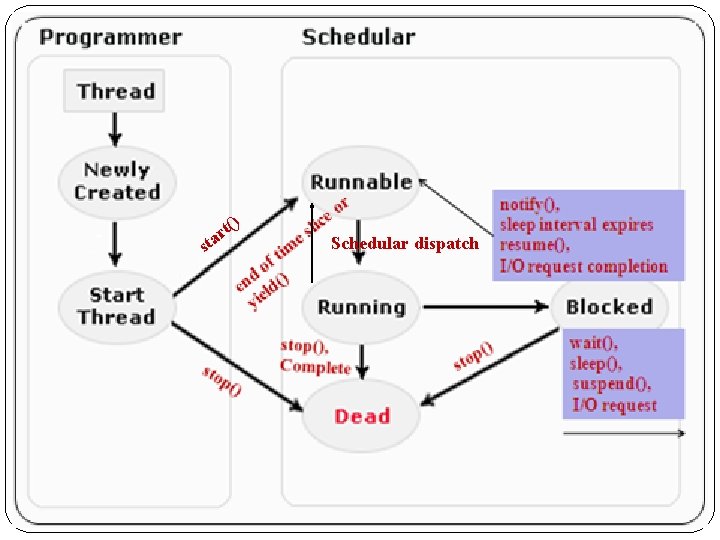
st t() r a Schedular dispatch
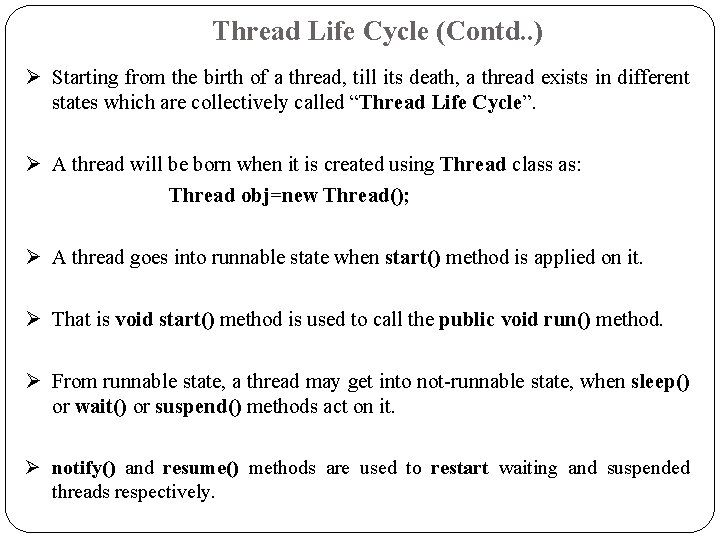
Thread Life Cycle (Contd. . ) Ø Starting from the birth of a thread, till its death, a thread exists in different states which are collectively called “Thread Life Cycle”. Ø A thread will be born when it is created using Thread class as: Thread obj=new Thread(); Ø A thread goes into runnable state when start() method is applied on it. Ø That is void start() method is used to call the public void run() method. Ø From runnable state, a thread may get into not-runnable state, when sleep() or wait() or suspend() methods act on it. Ø notify() and resume() methods are used to restart waiting and suspended threads respectively.
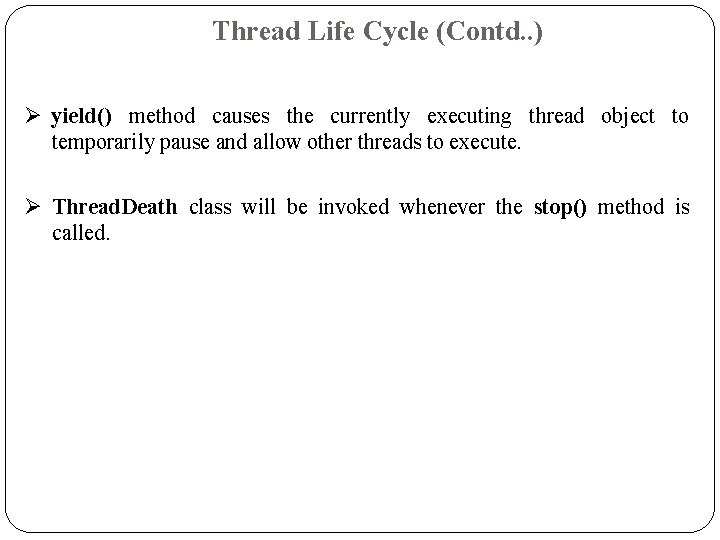
Thread Life Cycle (Contd. . ) Ø yield() method causes the currently executing thread object to temporarily pause and allow other threads to execute. Ø Thread. Death class will be invoked whenever the stop() method is called.
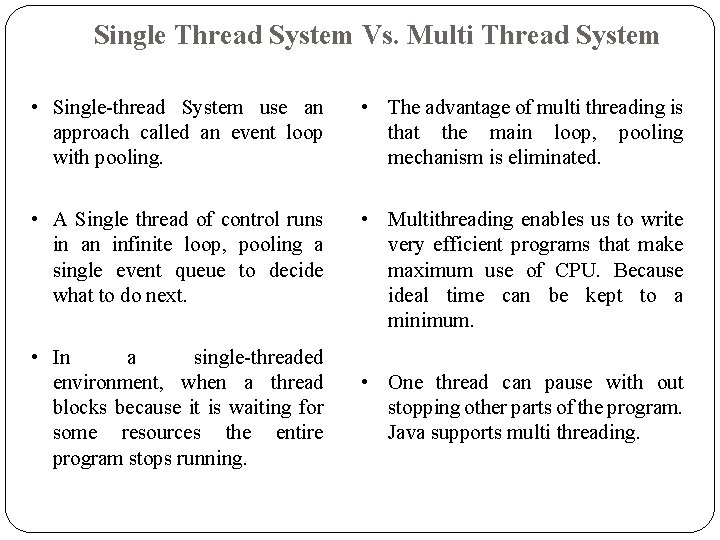
Single Thread System Vs. Multi Thread System • Single-thread System use an approach called an event loop with pooling. • The advantage of multi threading is that the main loop, pooling mechanism is eliminated. • A Single thread of control runs in an infinite loop, pooling a single event queue to decide what to do next. • Multithreading enables us to write very efficient programs that make maximum use of CPU. Because ideal time can be kept to a minimum. • In a single-threaded environment, when a thread blocks because it is waiting for some resources the entire program stops running. • One thread can pause with out stopping other parts of the program. Java supports multi threading.
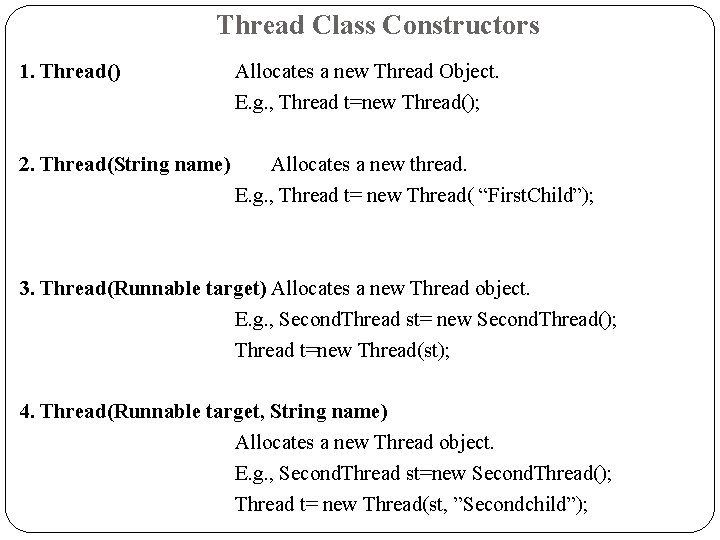
Thread Class Constructors 1. Thread() Allocates a new Thread Object. E. g. , Thread t=new Thread(); 2. Thread(String name) Allocates a new thread. E. g. , Thread t= new Thread( “First. Child”); 3. Thread(Runnable target) Allocates a new Thread object. E. g. , Second. Thread st= new Second. Thread(); Thread t=new Thread(st); 4. Thread(Runnable target, String name) Allocates a new Thread object. E. g. , Second. Thread st=new Second. Thread(); Thread t= new Thread(st, ”Secondchild”);
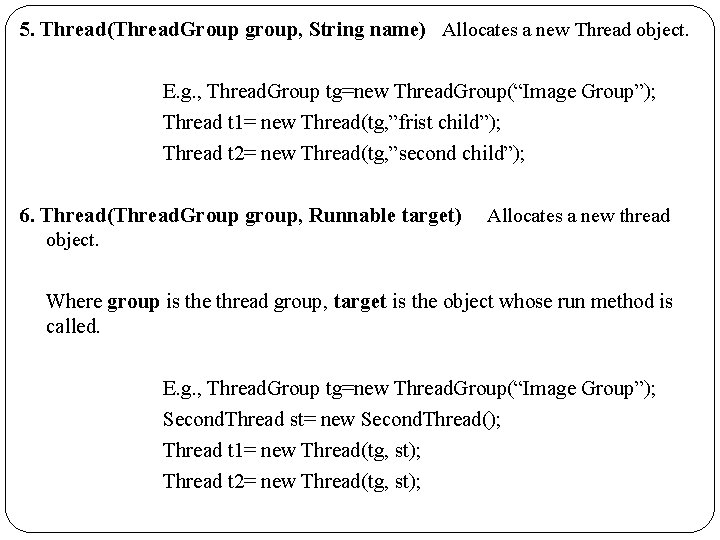
5. Thread(Thread. Group group, String name) Allocates a new Thread object. E. g. , Thread. Group tg=new Thread. Group(“Image Group”); Thread t 1= new Thread(tg, ”frist child”); Thread t 2= new Thread(tg, ”second child”); 6. Thread(Thread. Group group, Runnable target) Allocates a new thread object. Where group is the thread group, target is the object whose run method is called. E. g. , Thread. Group tg=new Thread. Group(“Image Group”); Second. Thread st= new Second. Thread(); Thread t 1= new Thread(tg, st); Thread t 2= new Thread(tg, st);
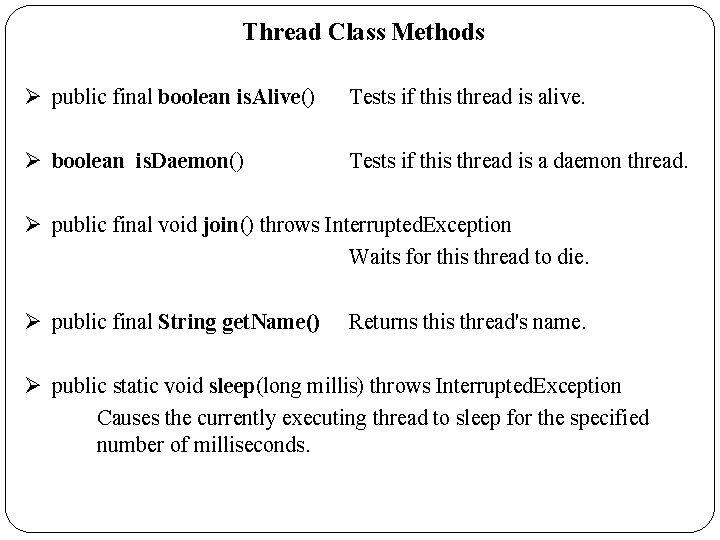
Thread Class Methods Ø public final boolean is. Alive() Tests if this thread is alive. Ø boolean is. Daemon() Tests if this thread is a daemon thread. Ø public final void join() throws Interrupted. Exception Waits for this thread to die. Ø public final String get. Name() Returns this thread's name. Ø public static void sleep(long millis) throws Interrupted. Exception Causes the currently executing thread to sleep for the specified number of milliseconds.
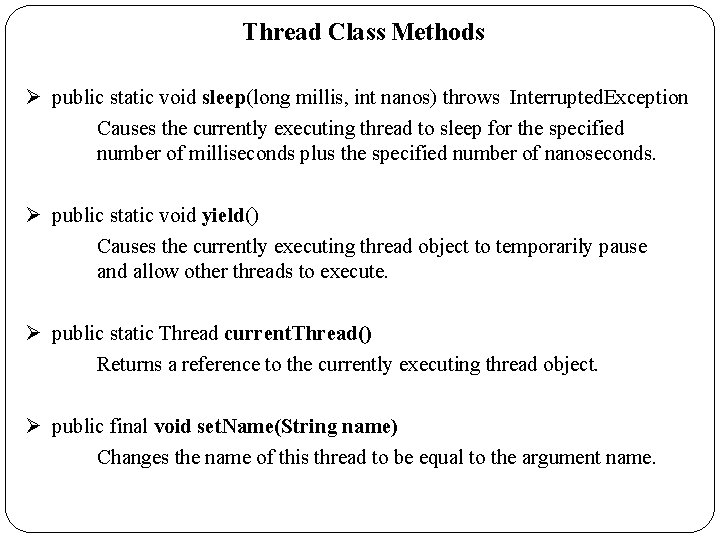
Thread Class Methods Ø public static void sleep(long millis, int nanos) throws Interrupted. Exception Causes the currently executing thread to sleep for the specified number of milliseconds plus the specified number of nanoseconds. Ø public static void yield() Causes the currently executing thread object to temporarily pause and allow other threads to execute. Ø public static Thread current. Thread() Returns a reference to the currently executing thread object. Ø public final void set. Name(String name) Changes the name of this thread to be equal to the argument name.
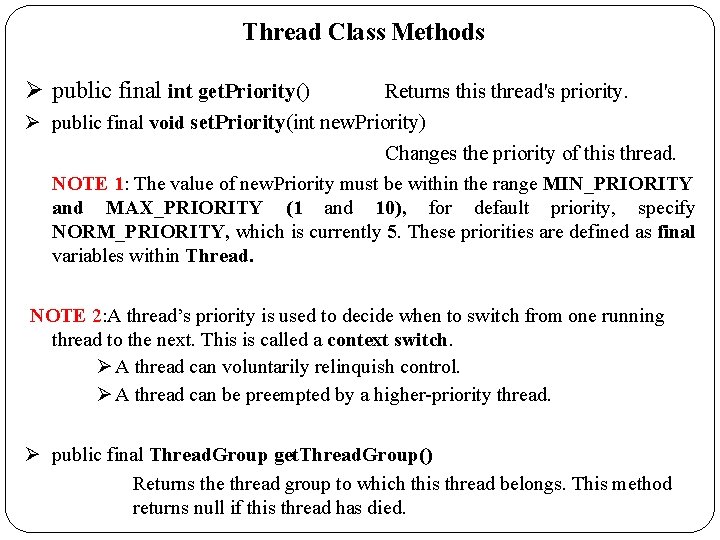
Thread Class Methods Ø public final int get. Priority() Returns this thread's priority. Ø public final void set. Priority(int new. Priority) Changes the priority of this thread. NOTE 1: The value of new. Priority must be within the range MIN_PRIORITY and MAX_PRIORITY (1 and 10), for default priority, specify NORM_PRIORITY, which is currently 5. These priorities are defined as final variables within Thread. NOTE 2: A thread’s priority is used to decide when to switch from one running thread to the next. This is called a context switch. Ø A thread can voluntarily relinquish control. Ø A thread can be preempted by a higher-priority thread. Ø public final Thread. Group get. Thread. Group() Returns the thread group to which this thread belongs. This method returns null if this thread has died.
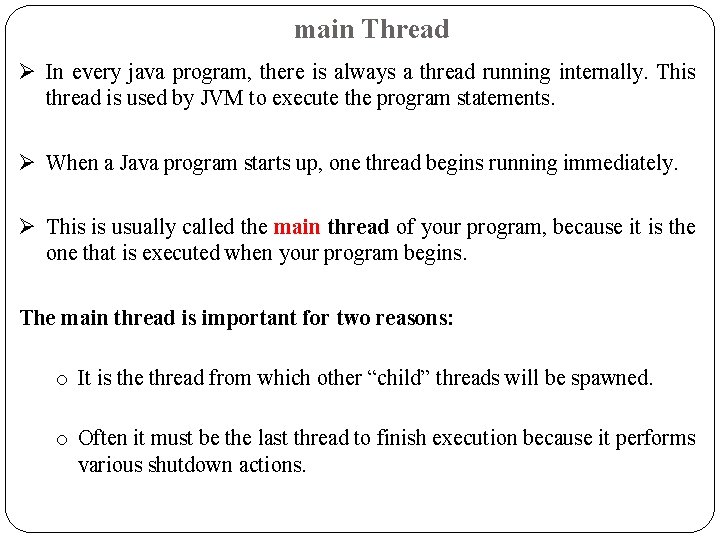
main Thread Ø In every java program, there is always a thread running internally. This thread is used by JVM to execute the program statements. Ø When a Java program starts up, one thread begins running immediately. Ø This is usually called the main thread of your program, because it is the one that is executed when your program begins. The main thread is important for two reasons: o It is the thread from which other “child” threads will be spawned. o Often it must be the last thread to finish execution because it performs various shutdown actions.
![class Current Thread Demo public static void mainString sree System out printlnLet us find class Current. Thread. Demo{ public static void main(String sree[]){ System. out. println(“Let us find](https://slidetodoc.com/presentation_image/f6df1212212c5df8992e104465d0ea3d/image-62.jpg)
class Current. Thread. Demo{ public static void main(String sree[]){ System. out. println(“Let us find the current thread…. . ”); Thread t = Thread. current. Thread(); System. out. println(“Current Thread= ”+t); System. out. println(“It’s name= ”+t. get. Name()); } } Let us find the current thread…. . Current Thread= Thread[main, 5, main] It’s name= main Which thread always runs in a java program by default? main thread
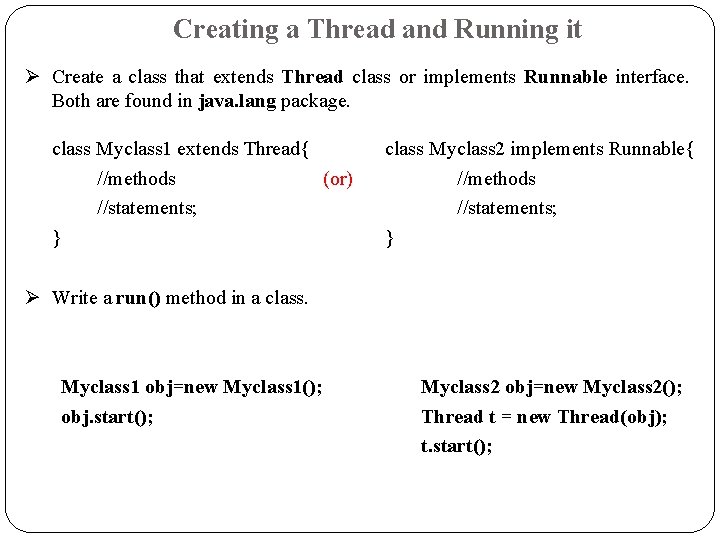
Creating a Thread and Running it Ø Create a class that extends Thread class or implements Runnable interface. Both are found in java. lang package. class Myclass 1 extends Thread{ //methods (or) //statements; } class Myclass 2 implements Runnable{ //methods //statements; } Ø Write a run() method in a class. Myclass 1 obj=new Myclass 1(); obj. start(); Myclass 2 obj=new Myclass 2(); Thread t = new Thread(obj); t. start();
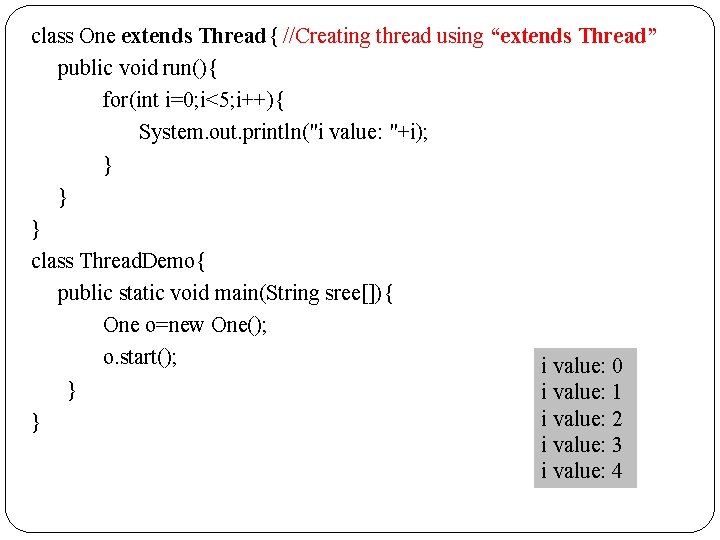
class One extends Thread{ //Creating thread using “extends Thread” public void run(){ for(int i=0; i<5; i++){ System. out. println("i value: "+i); } } } class Thread. Demo{ public static void main(String sree[]){ One o=new One(); o. start(); i value: 0 } i value: 1 i value: 2 } i value: 3 i value: 4
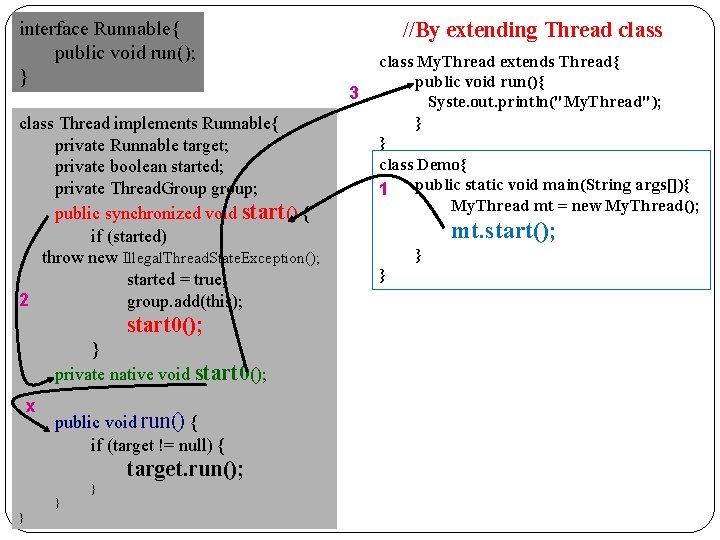
interface Runnable{ public void run(); } class Thread implements Runnable{ private Runnable target; private boolean started; private Thread. Group group; public synchronized void start() { if (started) throw new Illegal. Thread. State. Exception(); started = true; 2 group. add(this); start 0(); x } private native void start 0(); public void run() { if (target != null) { target. run(); } } } //By extending Thread class 3 class My. Thread extends Thread{ public void run(){ Syste. out. println("My. Thread"); } } class Demo{ 1 public static void main(String args[]){ My. Thread mt = new My. Thread(); mt. start(); } }
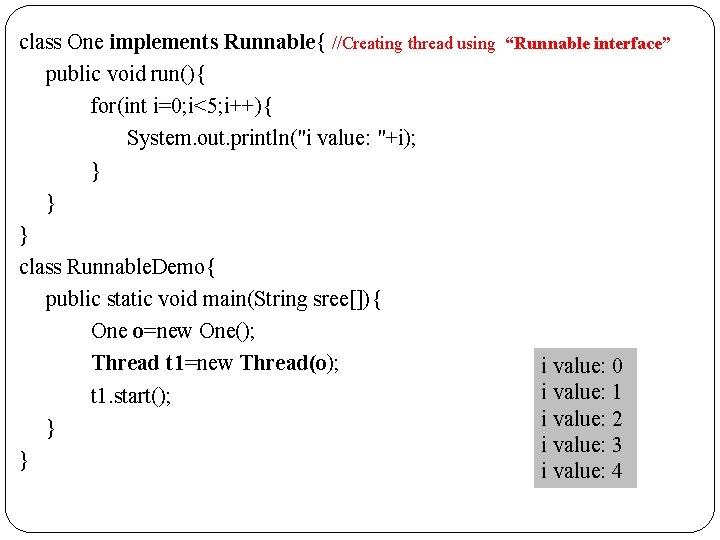
class One implements Runnable{ //Creating thread using “Runnable interface” public void run(){ for(int i=0; i<5; i++){ System. out. println("i value: "+i); } } } class Runnable. Demo{ public static void main(String sree[]){ One o=new One(); Thread t 1=new Thread(o); i value: 0 i value: 1 t 1. start(); i value: 2 } i value: 3 } i value: 4
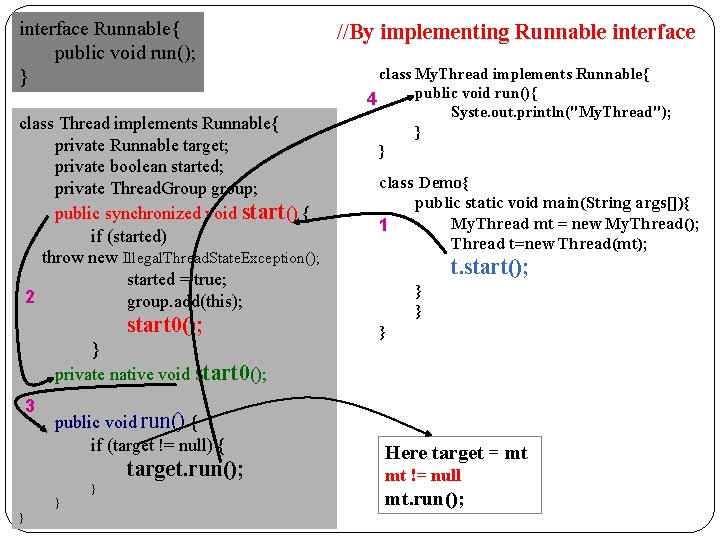
interface Runnable{ public void run(); } class Thread implements Runnable{ private Runnable target; private boolean started; private Thread. Group group; public synchronized void start() { if (started) throw new Illegal. Thread. State. Exception(); started = true; 2 group. add(this); start 0(); 3 } private native void start 0(); public void run() { if (target != null) { target. run(); } } } //By implementing Runnable interface class My. Thread implements Runnable{ public void run(){ 4 Syste. out. println("My. Thread"); } } class Demo{ public static void main(String args[]){ My. Thread mt = new My. Thread(); 1 Thread t=new Thread(mt); t. start(); } } } Here target = mt mt != null mt. run();
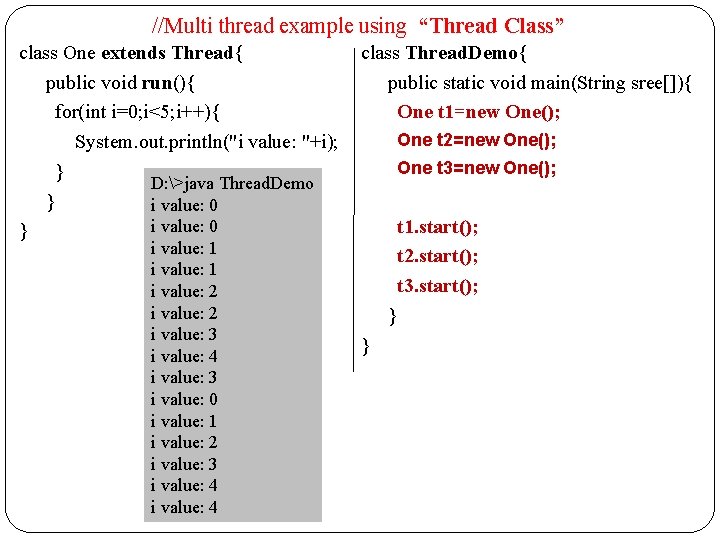
//Multi thread example using “Thread Class” class One extends Thread{ public void run(){ for(int i=0; i<5; i++){ System. out. println("i value: "+i); } D: >java Thread. Demo } i value: 0 } i value: 1 i value: 2 i value: 3 i value: 4 i value: 3 i value: 0 i value: 1 i value: 2 i value: 3 i value: 4 class Thread. Demo{ public static void main(String sree[]){ One t 1=new One(); One t 2=new One(); One t 3=new One(); t 1. start(); t 2. start(); t 3. start(); } }
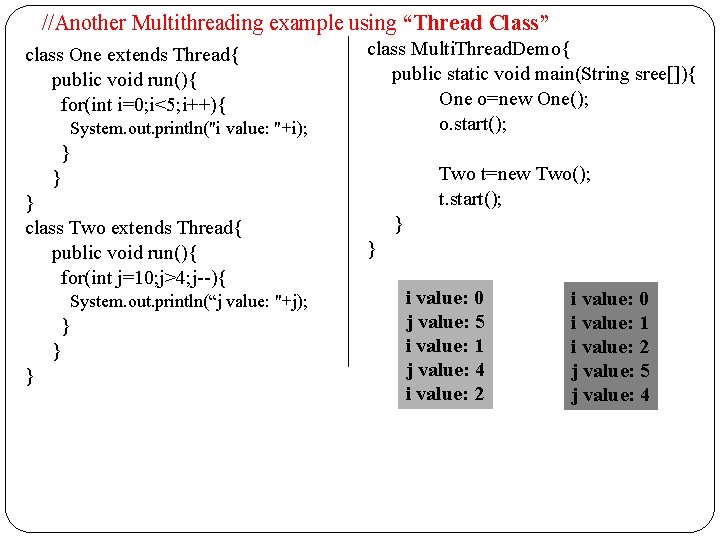
//Another Multithreading example using “Thread Class” class One extends Thread{ public void run(){ for(int i=0; i<5; i++){ System. out. println("i value: "+i); class Multi. Thread. Demo{ public static void main(String sree[]){ One o=new One(); o. start(); } } } class Two extends Thread{ public void run(){ for(int j=10; j>4; j--){ System. out. println(“j value: "+j); } } } Two t=new Two(); t. start(); } } i value: 0 j value: 5 i value: 1 j value: 4 i value: 2 i value: 0 i value: 1 i value: 2 j value: 5 j value: 4
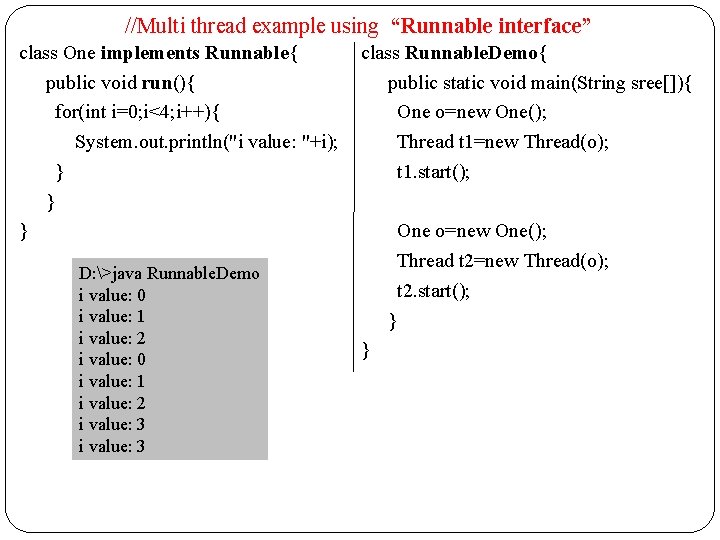
//Multi thread example using “Runnable interface” class One implements Runnable{ public void run(){ for(int i=0; i<4; i++){ System. out. println("i value: "+i); } } } D: >java Runnable. Demo i value: 0 i value: 1 i value: 2 i value: 3 class Runnable. Demo{ public static void main(String sree[]){ One o=new One(); Thread t 1=new Thread(o); t 1. start(); One o=new One(); Thread t 2=new Thread(o); t 2. start(); } }
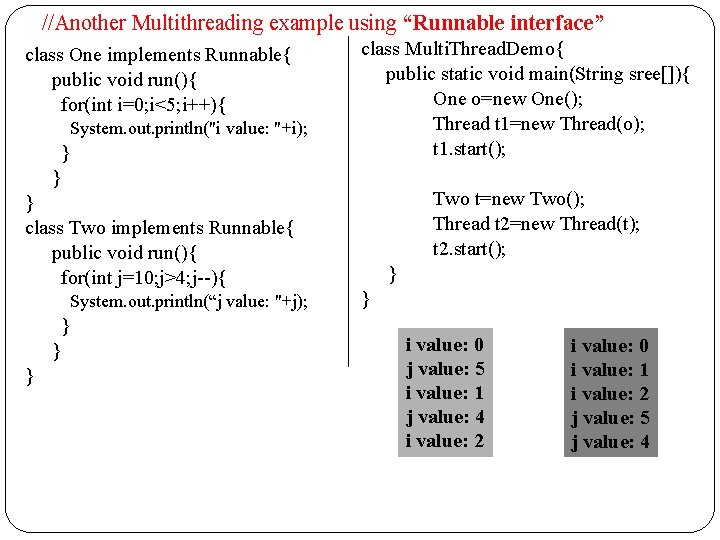
//Another Multithreading example using “Runnable interface” class One implements Runnable{ public void run(){ for(int i=0; i<5; i++){ System. out. println("i value: "+i); } } } class Two implements Runnable{ public void run(){ for(int j=10; j>4; j--){ System. out. println(“j value: "+j); } } } class Multi. Thread. Demo{ public static void main(String sree[]){ One o=new One(); Thread t 1=new Thread(o); t 1. start(); Two t=new Two(); Thread t 2=new Thread(t); t 2. start(); } } i value: 0 j value: 5 i value: 1 j value: 4 i value: 2 i value: 0 i value: 1 i value: 2 j value: 5 j value: 4
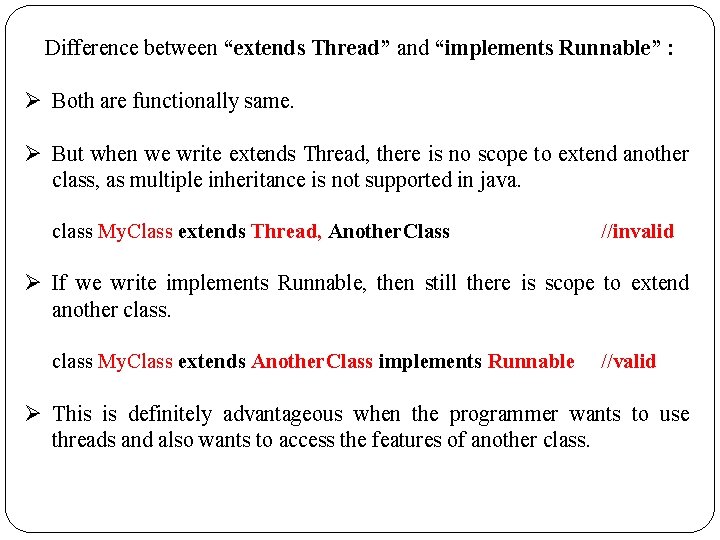
Difference between “extends Thread” and “implements Runnable” : Ø Both are functionally same. Ø But when we write extends Thread, there is no scope to extend another class, as multiple inheritance is not supported in java. class My. Class extends Thread, Another. Class //invalid Ø If we write implements Runnable, then still there is scope to extend another class My. Class extends Another. Class implements Runnable //valid Ø This is definitely advantageous when the programmer wants to use threads and also wants to access the features of another class.
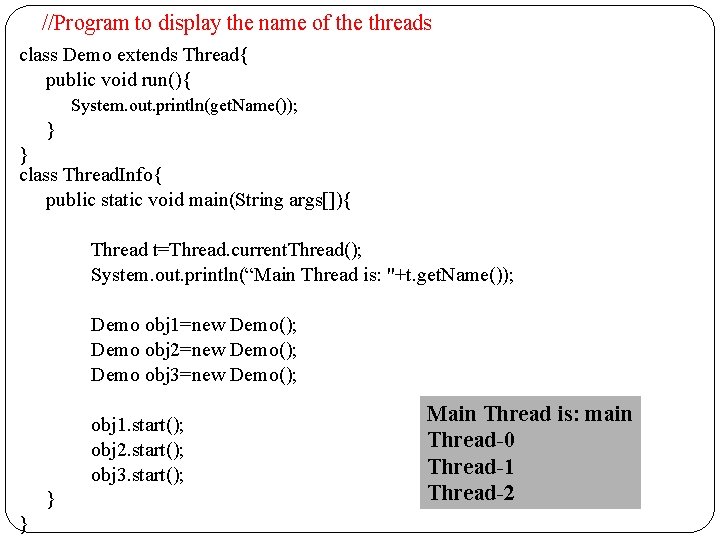
//Program to display the name of the threads class Demo extends Thread{ public void run(){ System. out. println(get. Name()); } } class Thread. Info{ public static void main(String args[]){ Thread t=Thread. current. Thread(); System. out. println(“Main Thread is: "+t. get. Name()); Demo obj 1=new Demo(); Demo obj 2=new Demo(); Demo obj 3=new Demo(); obj 1. start(); obj 2. start(); obj 3. start(); } } Main Thread is: main Thread-0 Thread-1 Thread-2
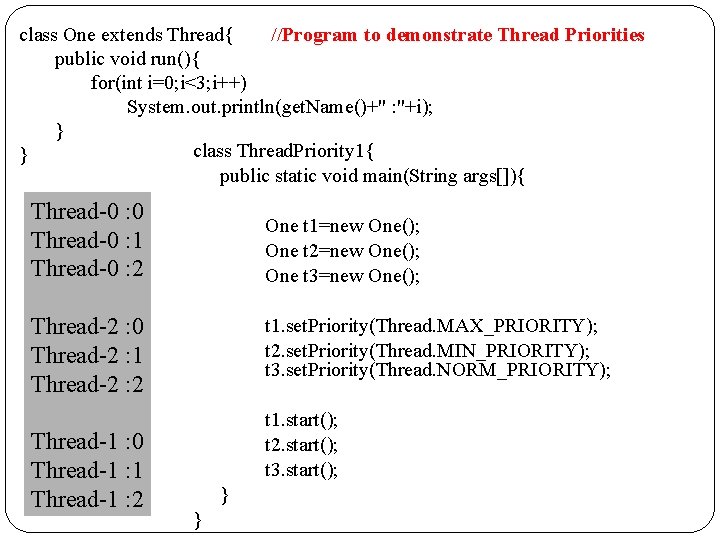
class One extends Thread{ //Program to demonstrate Thread Priorities public void run(){ for(int i=0; i<3; i++) System. out. println(get. Name()+" : "+i); } class Thread. Priority 1{ } public static void main(String args[]){ Thread-0 : 0 Thread-0 : 1 Thread-0 : 2 One t 1=new One(); One t 2=new One(); One t 3=new One(); Thread-2 : 0 Thread-2 : 1 Thread-2 : 2 Thread-1 : 0 Thread-1 : 1 Thread-1 : 2 t 1. set. Priority(Thread. MAX_PRIORITY); t 2. set. Priority(Thread. MIN_PRIORITY); t 3. set. Priority(Thread. NORM_PRIORITY); t 1. start(); t 2. start(); t 3. start(); } }
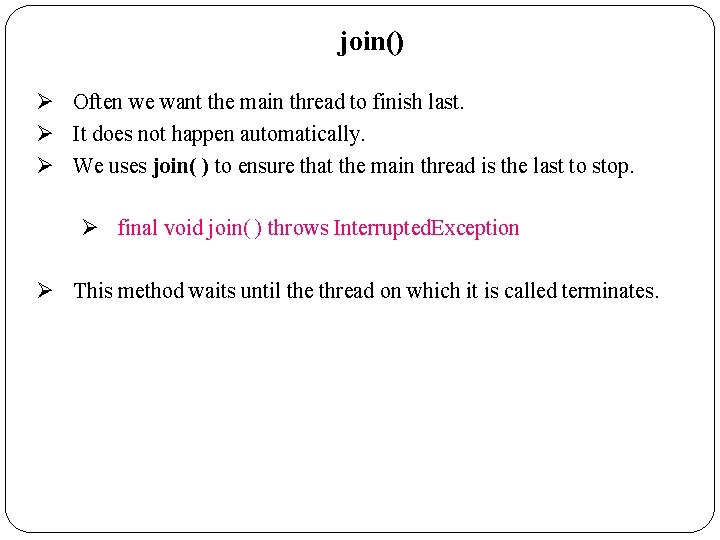
join() Ø Often we want the main thread to finish last. Ø It does not happen automatically. Ø We uses join( ) to ensure that the main thread is the last to stop. Ø final void join( ) throws Interrupted. Exception Ø This method waits until the thread on which it is called terminates.
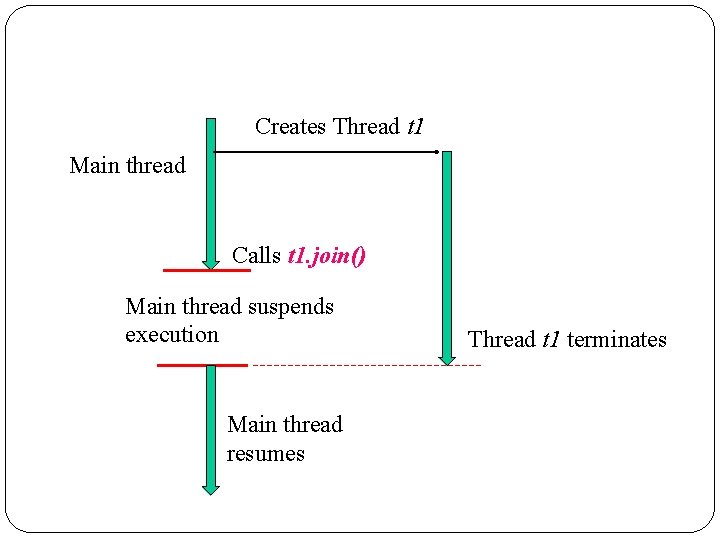
Creates Thread t 1 Main thread Calls t 1. join() Main thread suspends execution Main thread resumes Thread t 1 terminates
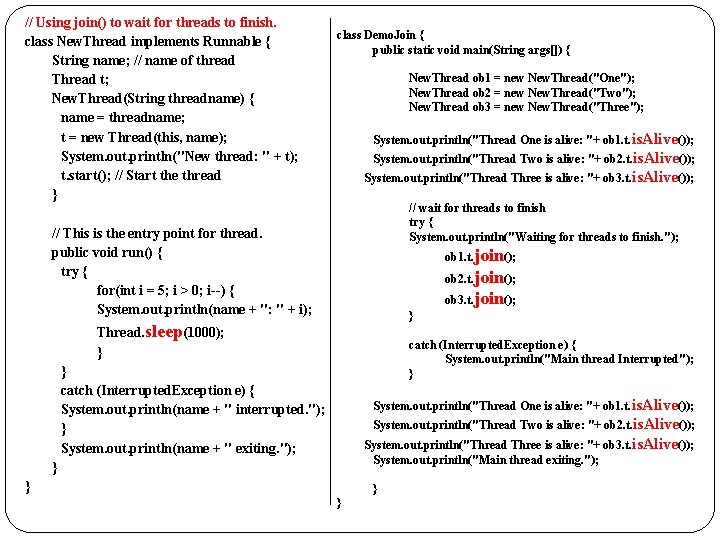
// Using join() to wait for threads to finish. class New. Thread implements Runnable { String name; // name of thread Thread t; New. Thread(String threadname) { name = threadname; t = new Thread(this, name); System. out. println("New thread: " + t); t. start(); // Start the thread } class Demo. Join { public static void main(String args[]) { New. Thread ob 1 = new New. Thread("One"); New. Thread ob 2 = new New. Thread("Two"); New. Thread ob 3 = new New. Thread("Three"); System. out. println("Thread One is alive: "+ ob 1. t. is. Alive()); System. out. println("Thread Two is alive: "+ ob 2. t. is. Alive()); System. out. println("Thread Three is alive: "+ ob 3. t. is. Alive()); // wait for threads to finish try { System. out. println("Waiting for threads to finish. "); // This is the entry point for thread. public void run() { try { for(int i = 5; i > 0; i--) { System. out. println(name + ": " + i); ob 1. t. join(); ob 2. t. join(); ob 3. t. join(); } Thread. sleep(1000); } catch (Interrupted. Exception e) { System. out. println("Main thread Interrupted"); } } catch (Interrupted. Exception e) { System. out. println("Thread One is alive: "+ ob 1. t. is. Alive()); System. out. println(name + " interrupted. "); System. out. println("Thread Two is alive: "+ ob 2. t. is. Alive()); } System. out. println("Thread Three is alive: "+ ob 3. t. is. Alive()); System. out. println(name + " exiting. "); System. out. println("Main thread exiting. "); } }
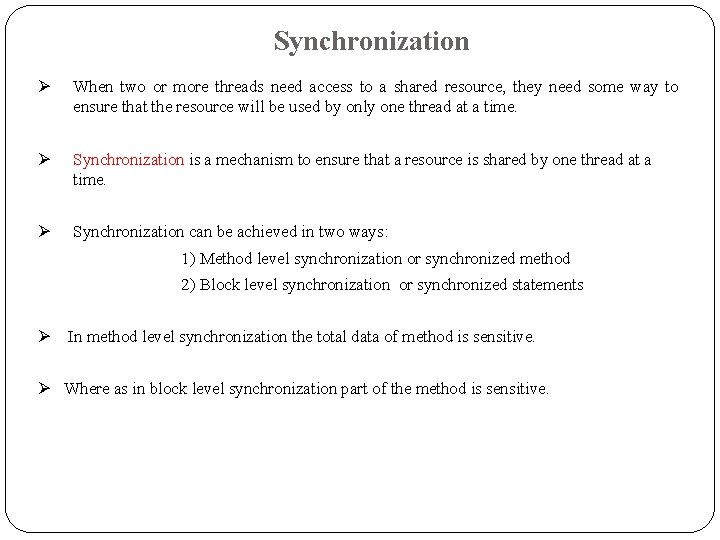
Synchronization Ø When two or more threads need access to a shared resource, they need some way to ensure that the resource will be used by only one thread at a time. Ø Synchronization is a mechanism to ensure that a resource is shared by one thread at a time. Ø Synchronization can be achieved in two ways: 1) Method level synchronization or synchronized method 2) Block level synchronization or synchronized statements Ø In method level synchronization the total data of method is sensitive. Ø Where as in block level synchronization part of the method is sensitive.
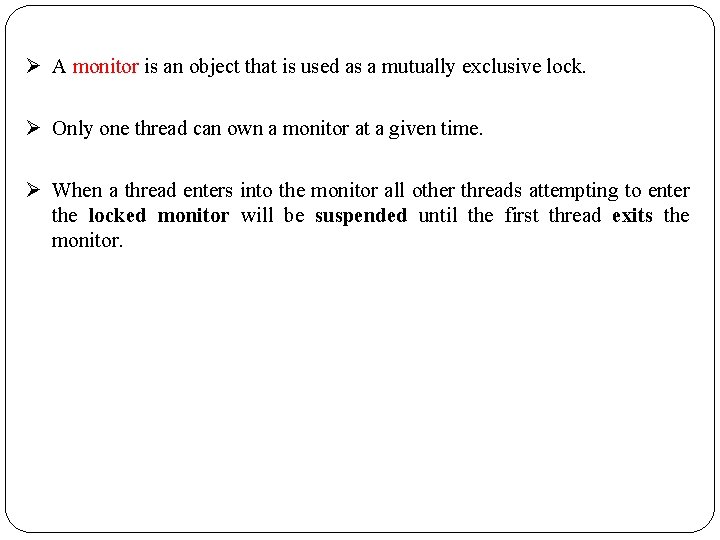
Ø A monitor is an object that is used as a mutually exclusive lock. Ø Only one thread can own a monitor at a given time. Ø When a thread enters into the monitor all other threads attempting to enter the locked monitor will be suspended until the first thread exits the monitor.
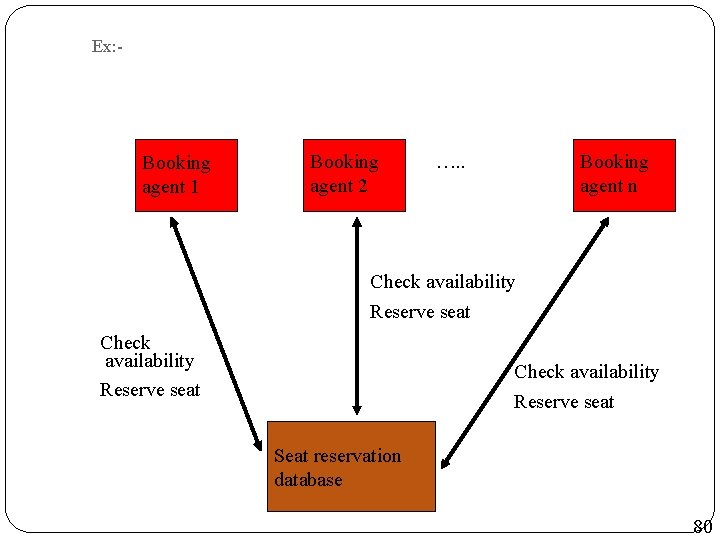
Ex: - Booking agent 1 Booking agent 2 …. . Booking agent n Check availability Reserve seat Seat reservation database 80
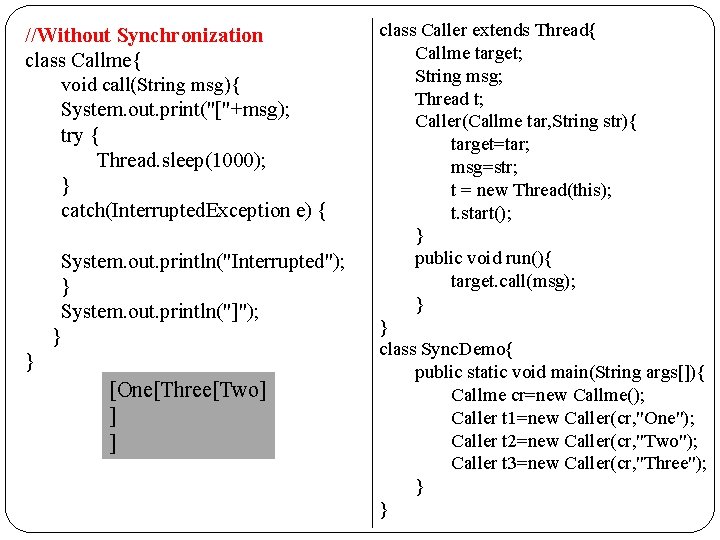
//Without Synchronization class Callme{ void call(String msg){ System. out. print("["+msg); try { Thread. sleep(1000); } catch(Interrupted. Exception e) { System. out. println("Interrupted"); } System. out. println("]"); } } [One[Three[Two] ] ] class Caller extends Thread{ Callme target; String msg; Thread t; Caller(Callme tar, String str){ target=tar; msg=str; t = new Thread(this); t. start(); } public void run(){ target. call(msg); } } class Sync. Demo{ public static void main(String args[]){ Callme cr=new Callme(); Caller t 1=new Caller(cr, "One"); Caller t 2=new Caller(cr, "Two"); Caller t 3=new Caller(cr, "Three"); } }
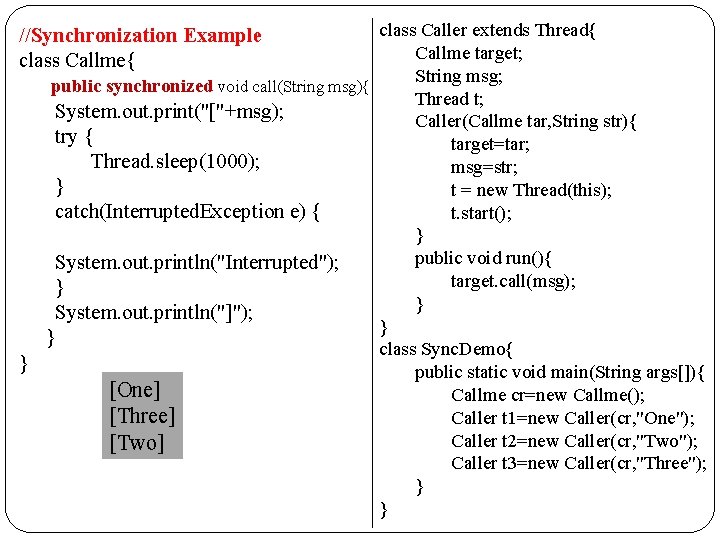
class Caller extends Thread{ //Synchronization Example Callme target; class Callme{ String msg; public synchronized void call(String msg){ Thread t; System. out. print("["+msg); Caller(Callme tar, String str){ try { target=tar; Thread. sleep(1000); msg=str; } t = new Thread(this); catch(Interrupted. Exception e) { t. start(); System. out. println("Interrupted"); } System. out. println("]"); } } [One] [Three] [Two] } public void run(){ target. call(msg); } } class Sync. Demo{ public static void main(String args[]){ Callme cr=new Callme(); Caller t 1=new Caller(cr, "One"); Caller t 2=new Caller(cr, "Two"); Caller t 3=new Caller(cr, "Three"); } }
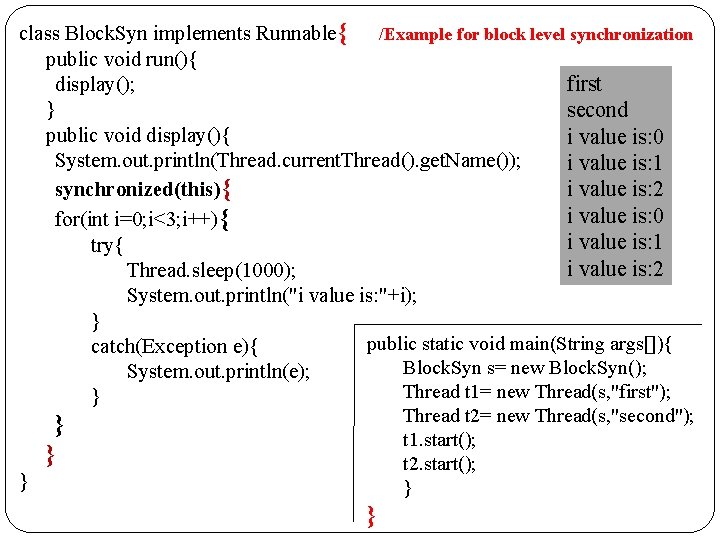
class Block. Syn implements Runnable{ /Example for block level synchronization public void run(){ first display(); } second public void display(){ i value is: 0 System. out. println(Thread. current. Thread(). get. Name()); i value is: 1 synchronized(this){ i value is: 2 i value is: 0 for(int i=0; i<3; i++){ i value is: 1 try{ i value is: 2 Thread. sleep(1000); System. out. println("i value is: "+i); } public static void main(String args[]){ catch(Exception e){ Block. Syn s= new Block. Syn(); System. out. println(e); Thread t 1= new Thread(s, "first"); } Thread t 2= new Thread(s, "second"); } t 1. start(); } t 2. start(); } } }