Session 05 Java Strings and Files Exercise Complete
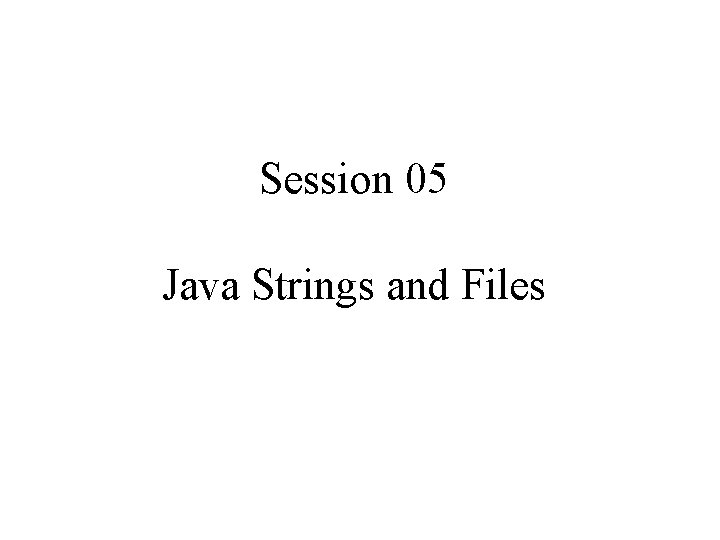
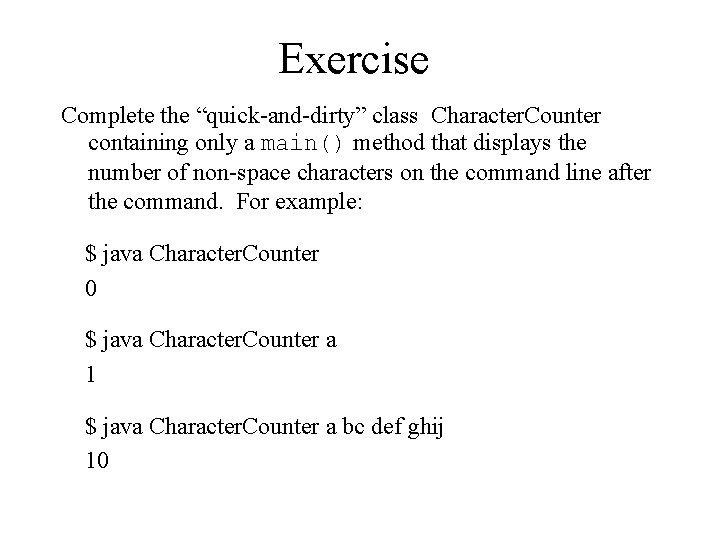
![Character. Count template public class Character. Counter { public static void main( String[] args Character. Count template public class Character. Counter { public static void main( String[] args](https://slidetodoc.com/presentation_image_h/8021b8f217285bf410b91dabe1145755/image-3.jpg)
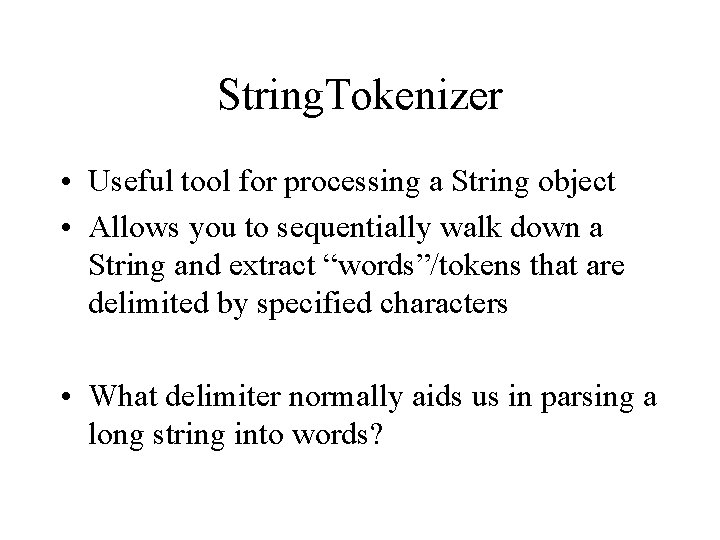
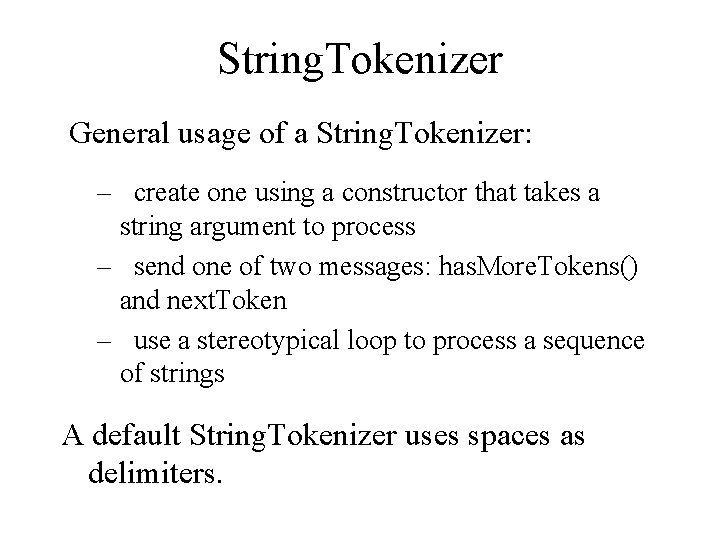
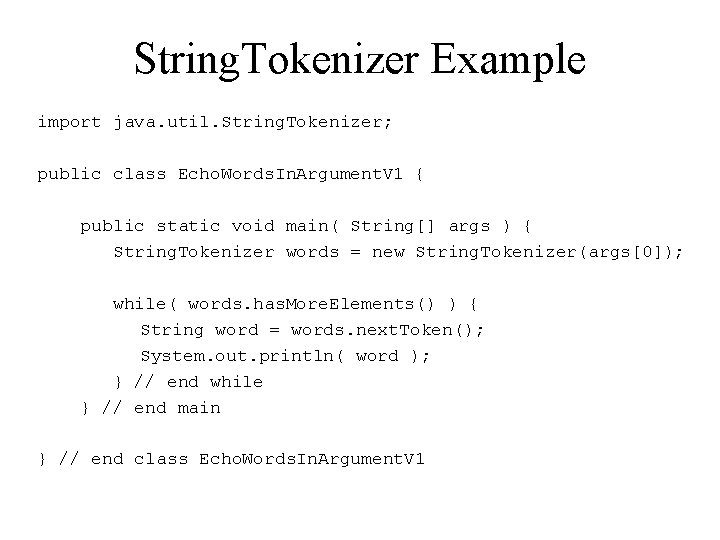
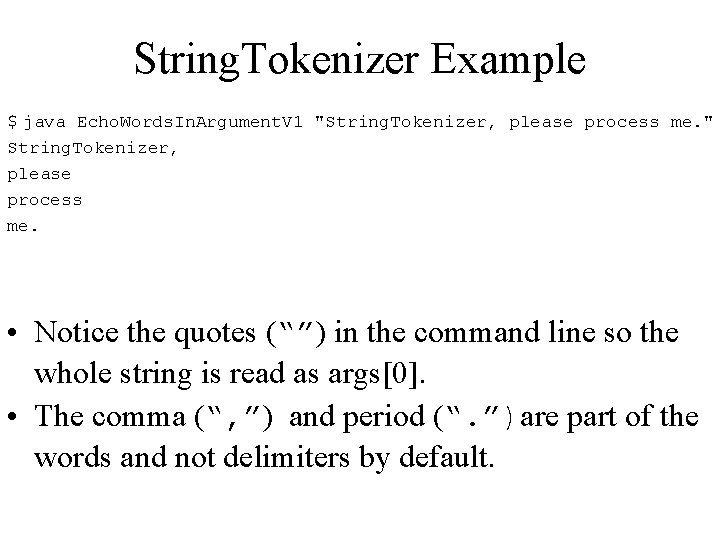
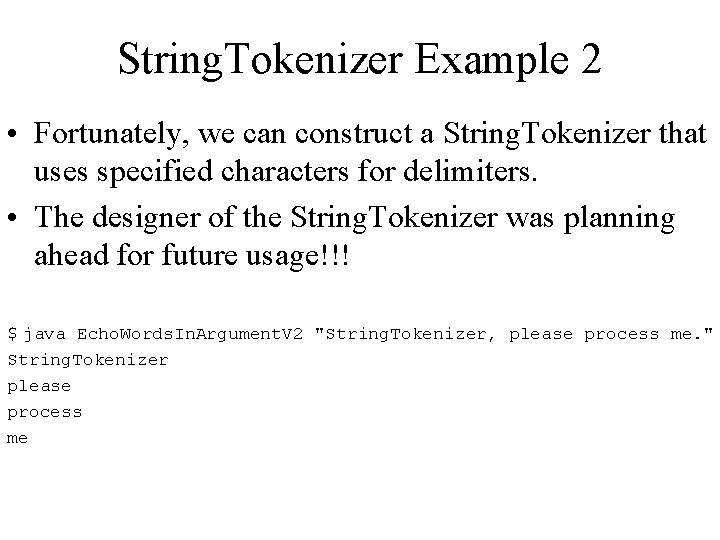
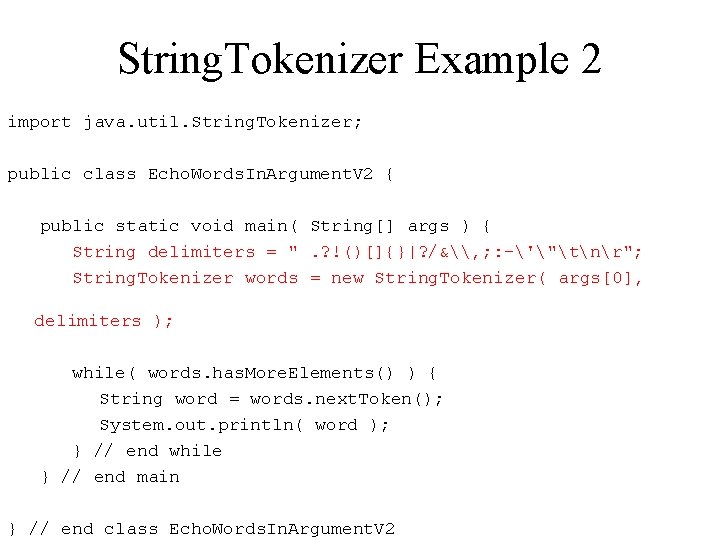
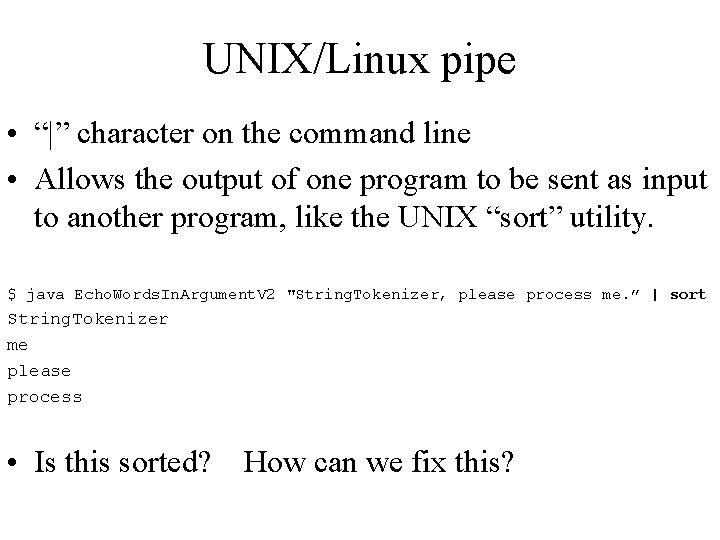
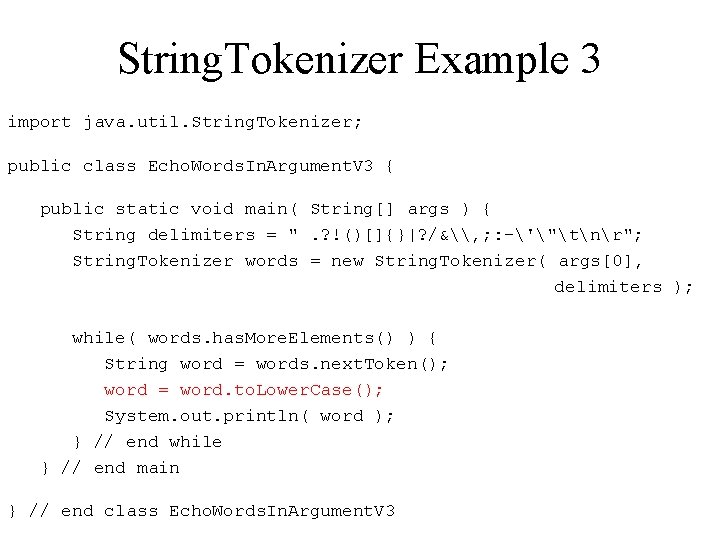
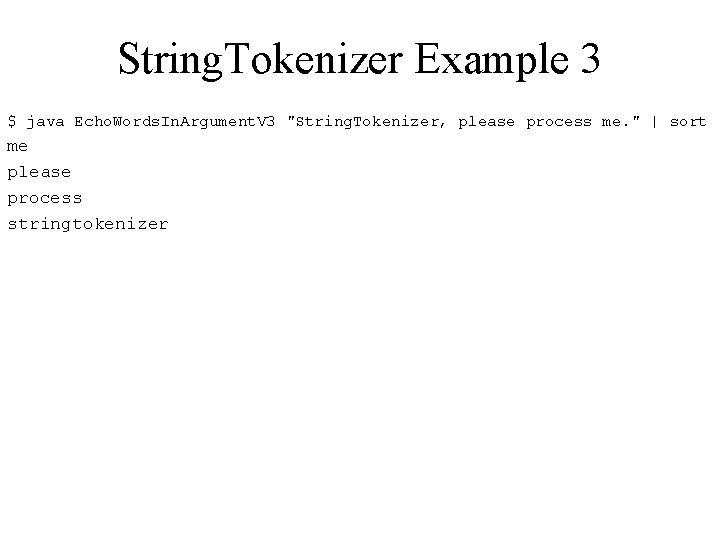
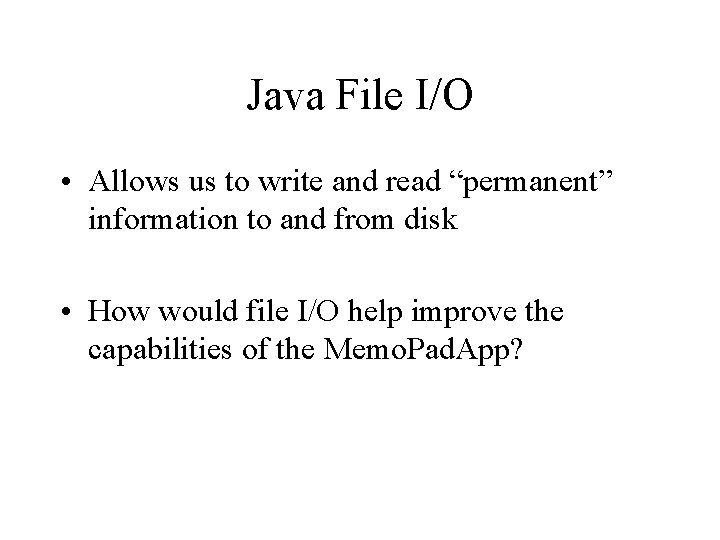
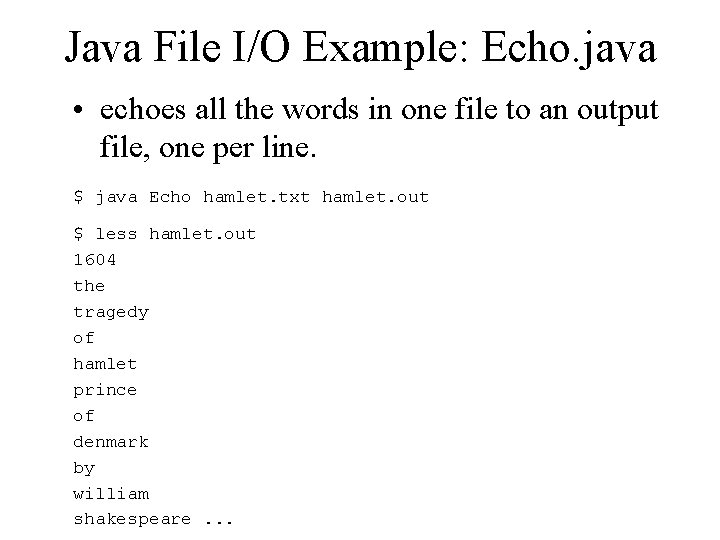
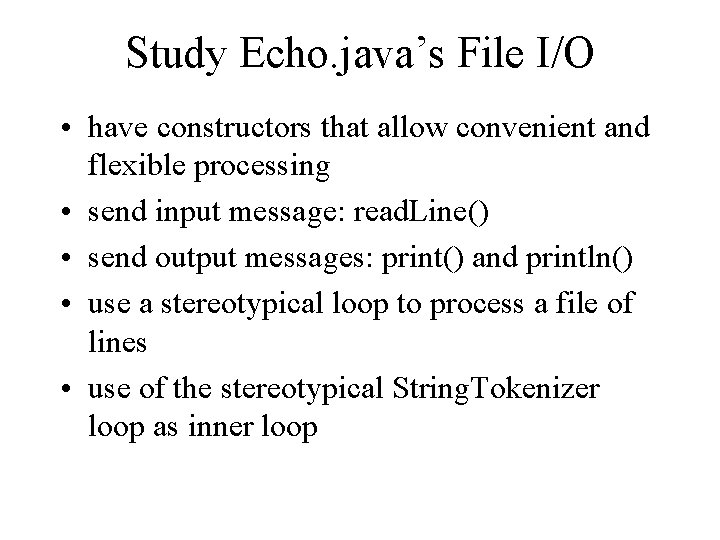
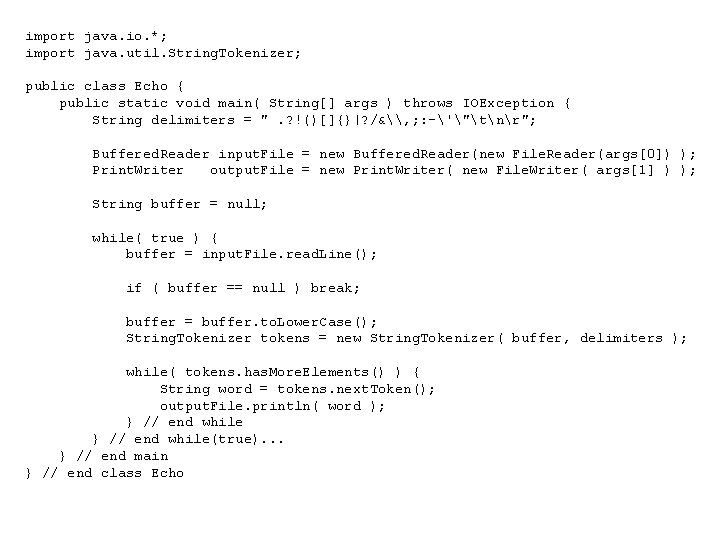
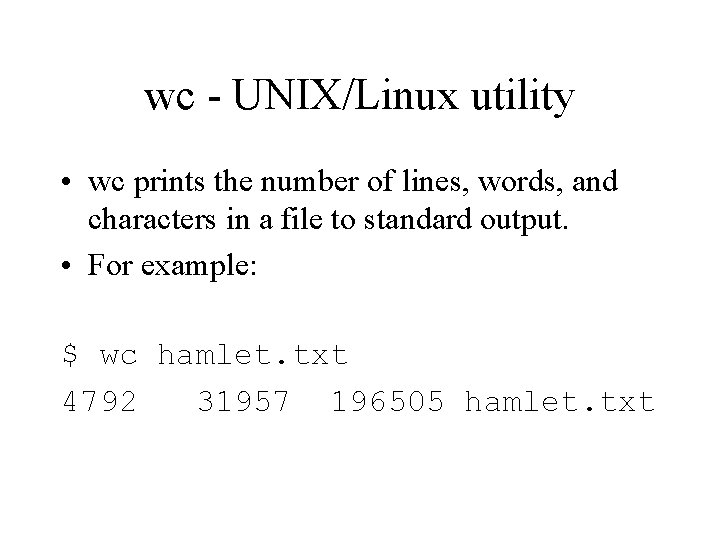
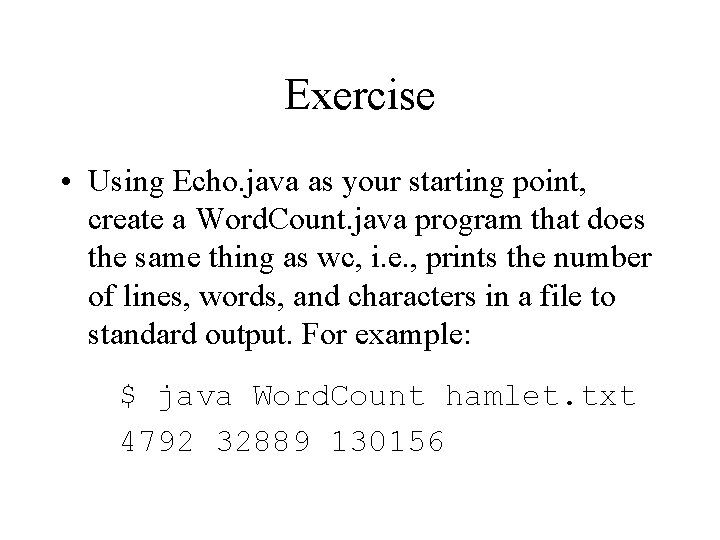
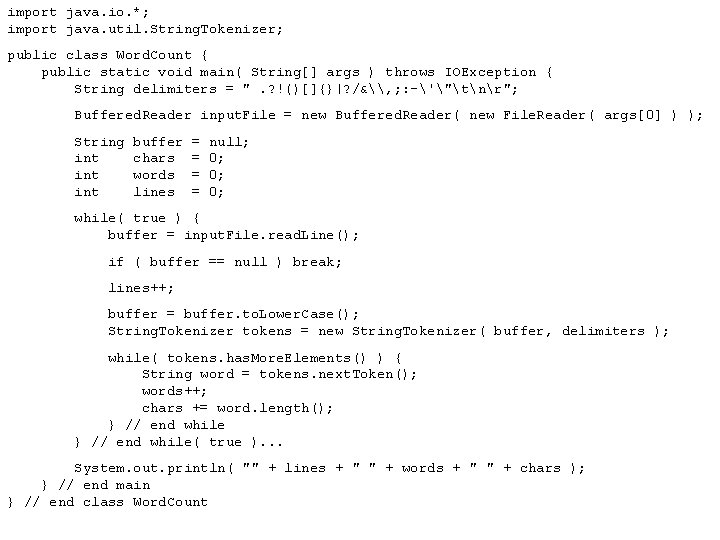
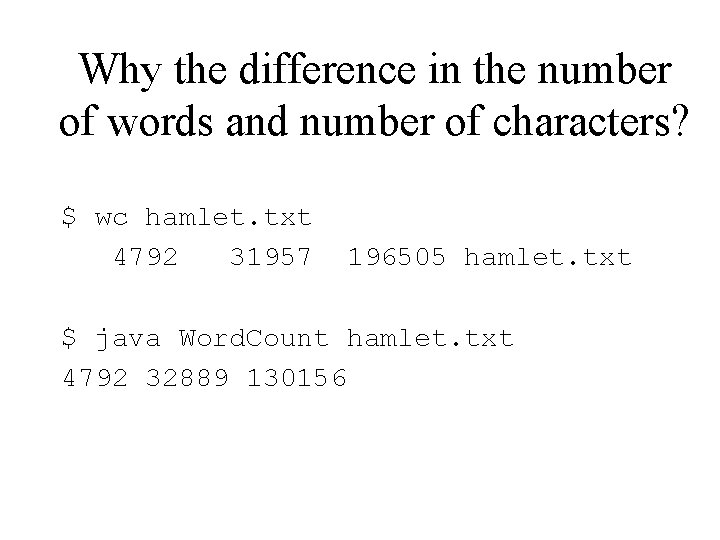
- Slides: 20
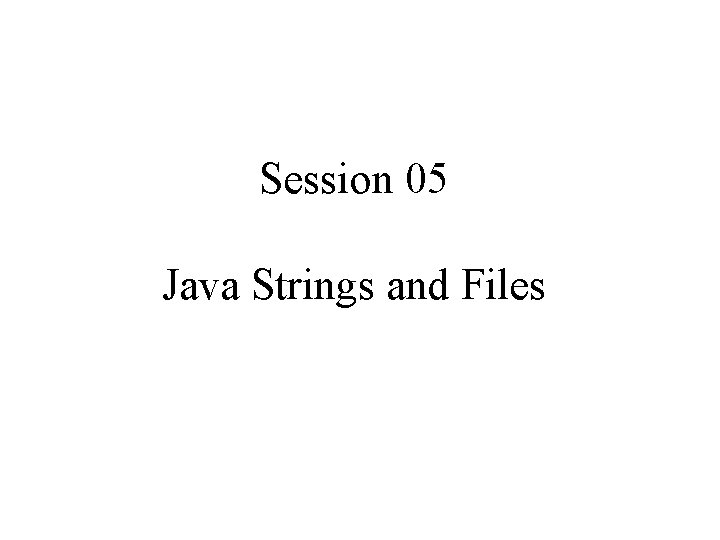
Session 05 Java Strings and Files
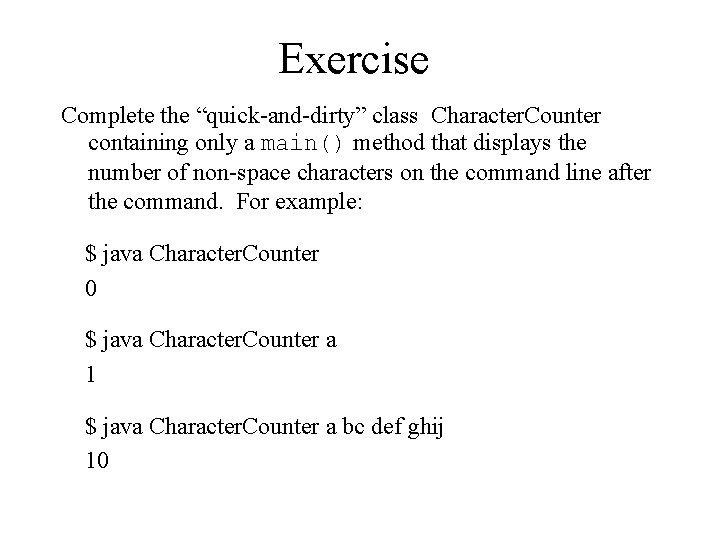
Exercise Complete the “quick-and-dirty” class Character. Counter containing only a main() method that displays the number of non-space characters on the command line after the command. For example: $ java Character. Counter 0 $ java Character. Counter a 1 $ java Character. Counter a bc def ghij 10
![Character Count template public class Character Counter public static void main String args Character. Count template public class Character. Counter { public static void main( String[] args](https://slidetodoc.com/presentation_image_h/8021b8f217285bf410b91dabe1145755/image-3.jpg)
Character. Count template public class Character. Counter { public static void main( String[] args ) { int character. Count = 0 ; } // end main } // end class Character. Counter
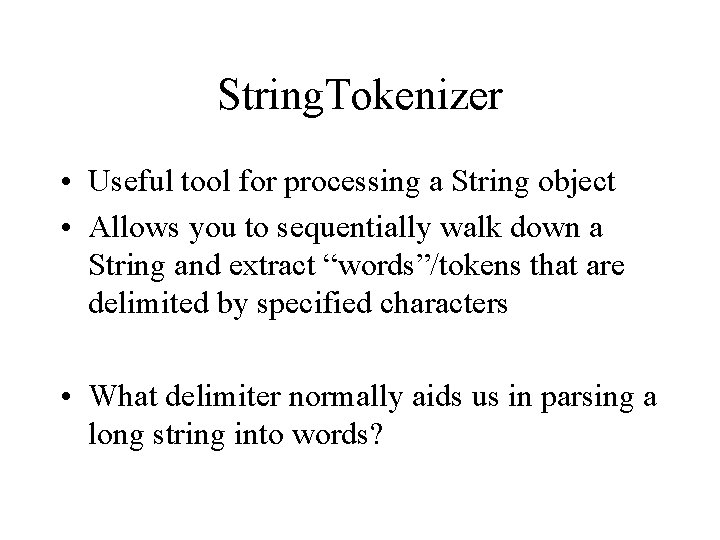
String. Tokenizer • Useful tool for processing a String object • Allows you to sequentially walk down a String and extract “words”/tokens that are delimited by specified characters • What delimiter normally aids us in parsing a long string into words?
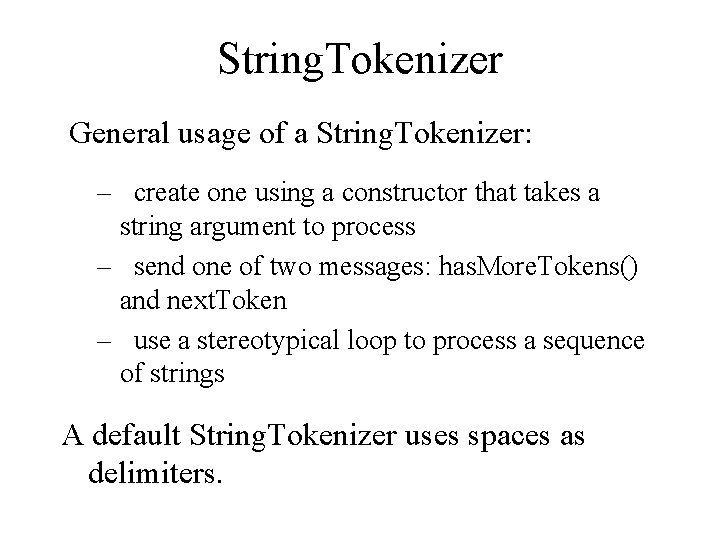
String. Tokenizer General usage of a String. Tokenizer: – create one using a constructor that takes a string argument to process – send one of two messages: has. More. Tokens() and next. Token – use a stereotypical loop to process a sequence of strings A default String. Tokenizer uses spaces as delimiters.
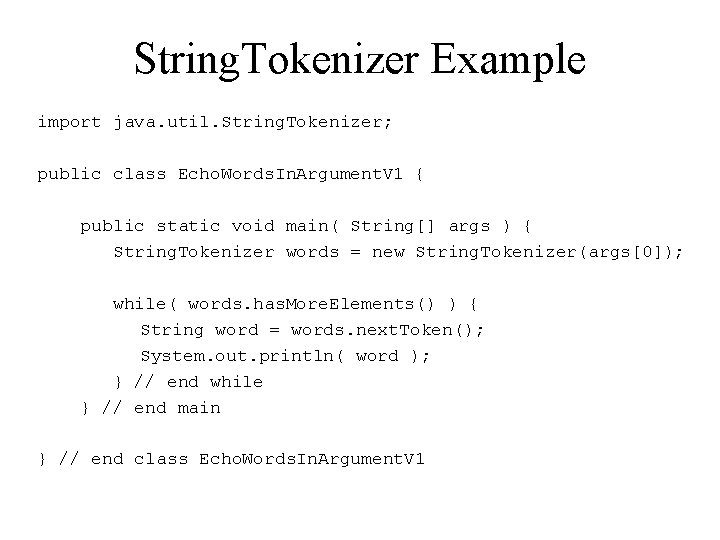
String. Tokenizer Example import java. util. String. Tokenizer; public class Echo. Words. In. Argument. V 1 { public static void main( String[] args ) { String. Tokenizer words = new String. Tokenizer(args[0]); while( words. has. More. Elements() ) { String word = words. next. Token(); System. out. println( word ); } // end while } // end main } // end class Echo. Words. In. Argument. V 1
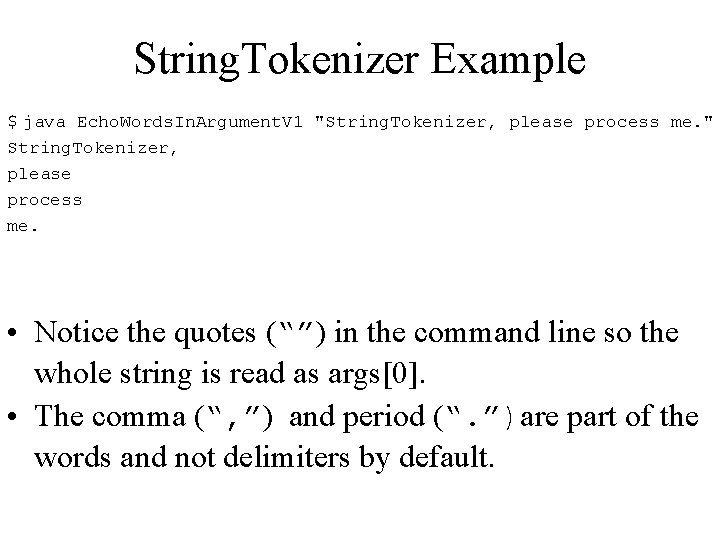
String. Tokenizer Example $ java Echo. Words. In. Argument. V 1 "String. Tokenizer, please process me. " String. Tokenizer, please process me. • Notice the quotes (“”) in the command line so the whole string is read as args[0]. • The comma (“, ”) and period (“. ”)are part of the words and not delimiters by default.
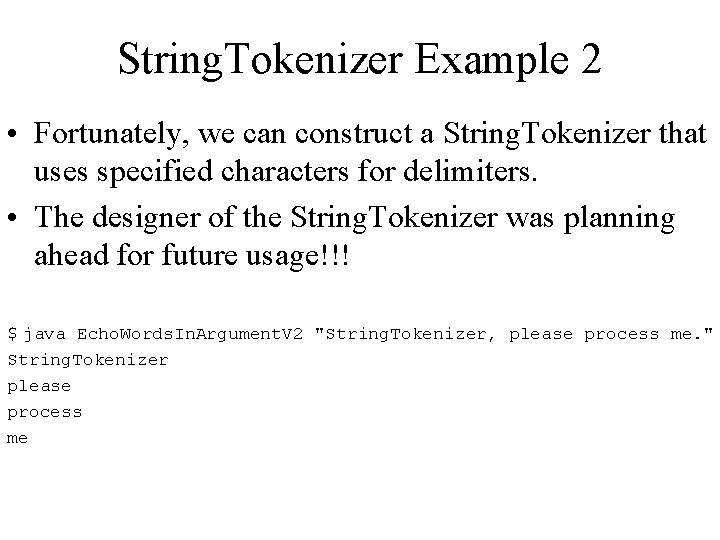
String. Tokenizer Example 2 • Fortunately, we can construct a String. Tokenizer that uses specified characters for delimiters. • The designer of the String. Tokenizer was planning ahead for future usage!!! $ java Echo. Words. In. Argument. V 2 "String. Tokenizer, please process me. " String. Tokenizer please process me
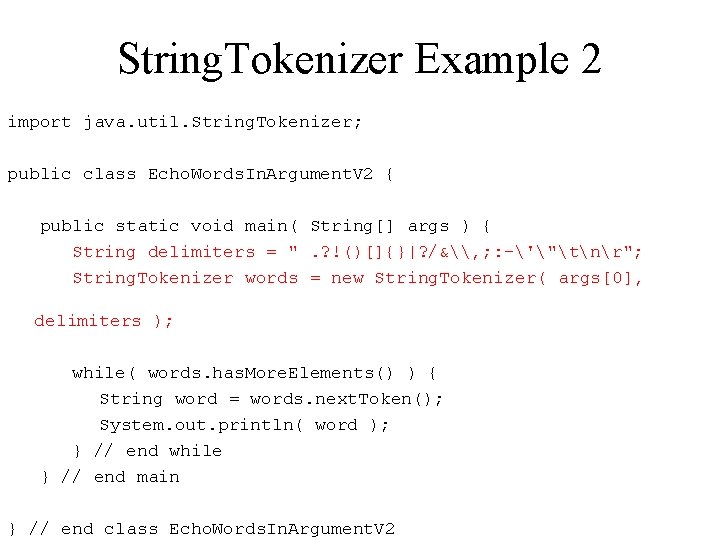
String. Tokenizer Example 2 import java. util. String. Tokenizer; public class Echo. Words. In. Argument. V 2 { public static void main( String[] args ) { String delimiters = ". ? !()[]{}|? /&\, ; : -'"tnr"; String. Tokenizer words = new String. Tokenizer( args[0], delimiters ); while( words. has. More. Elements() ) { String word = words. next. Token(); System. out. println( word ); } // end while } // end main } // end class Echo. Words. In. Argument. V 2
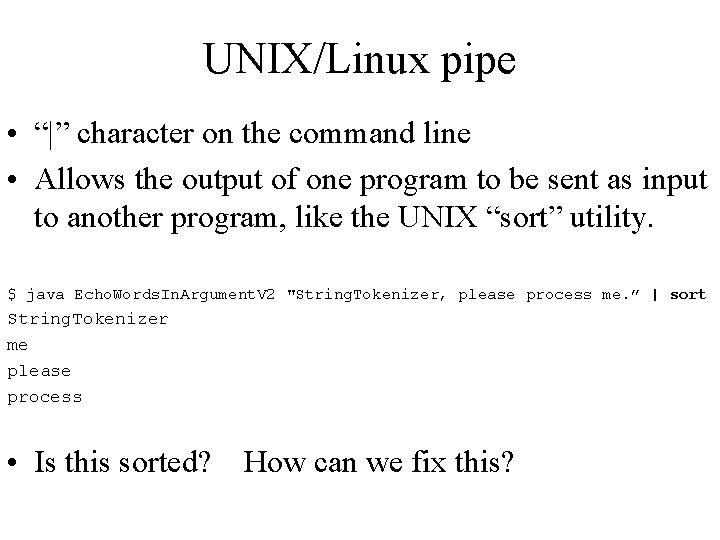
UNIX/Linux pipe • “|” character on the command line • Allows the output of one program to be sent as input to another program, like the UNIX “sort” utility. $ java Echo. Words. In. Argument. V 2 "String. Tokenizer, please process me. ” | sort String. Tokenizer me please process • Is this sorted? How can we fix this?
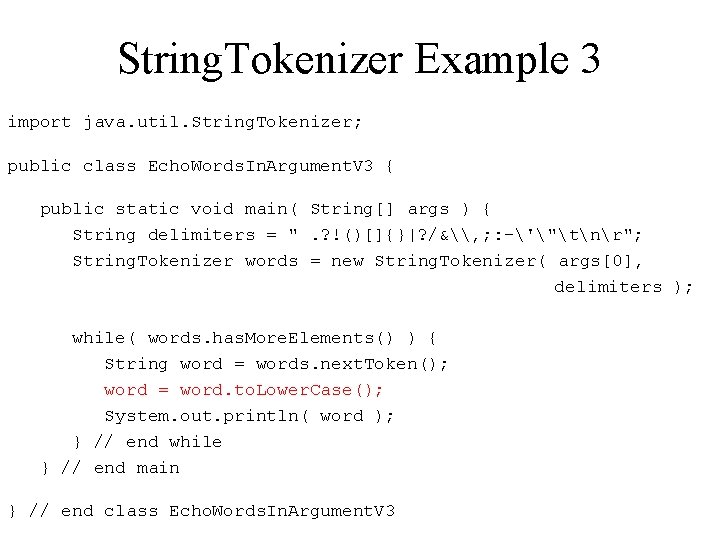
String. Tokenizer Example 3 import java. util. String. Tokenizer; public class Echo. Words. In. Argument. V 3 { public static void main( String[] args ) { String delimiters = ". ? !()[]{}|? /&\, ; : -'"tnr"; String. Tokenizer words = new String. Tokenizer( args[0], delimiters ); while( words. has. More. Elements() ) { String word = words. next. Token(); word = word. to. Lower. Case(); System. out. println( word ); } // end while } // end main } // end class Echo. Words. In. Argument. V 3
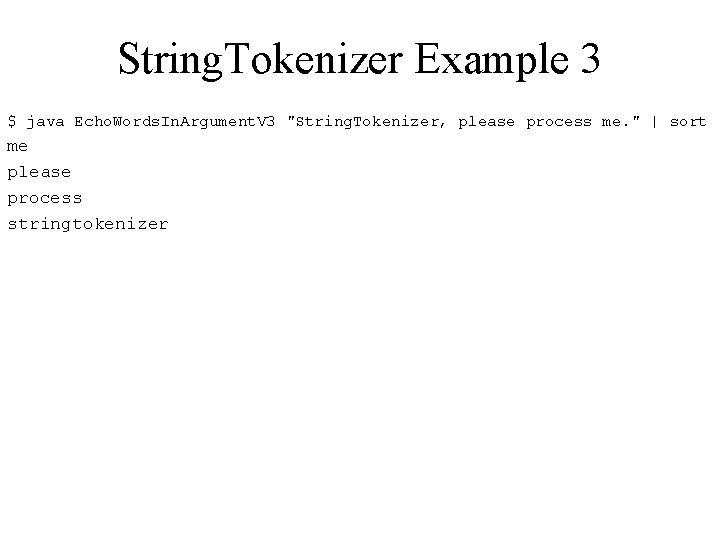
String. Tokenizer Example 3 $ java Echo. Words. In. Argument. V 3 "String. Tokenizer, please process me. " | sort me please process stringtokenizer
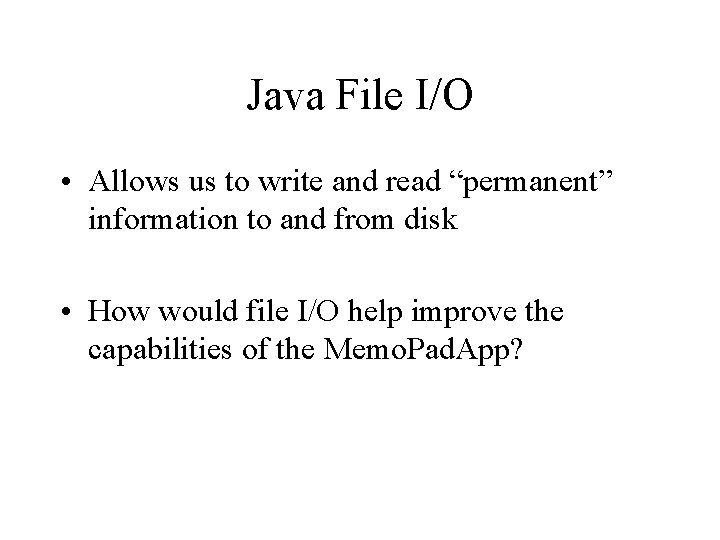
Java File I/O • Allows us to write and read “permanent” information to and from disk • How would file I/O help improve the capabilities of the Memo. Pad. App?
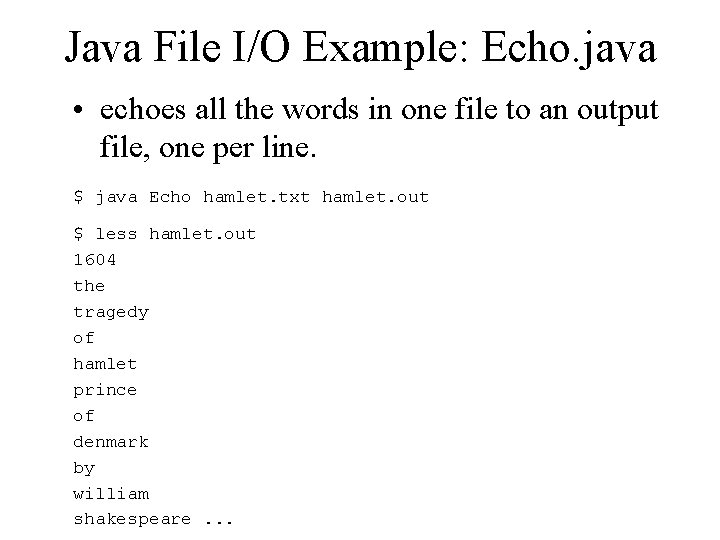
Java File I/O Example: Echo. java • echoes all the words in one file to an output file, one per line. $ java Echo hamlet. txt hamlet. out $ less hamlet. out 1604 the tragedy of hamlet prince of denmark by william shakespeare. . .
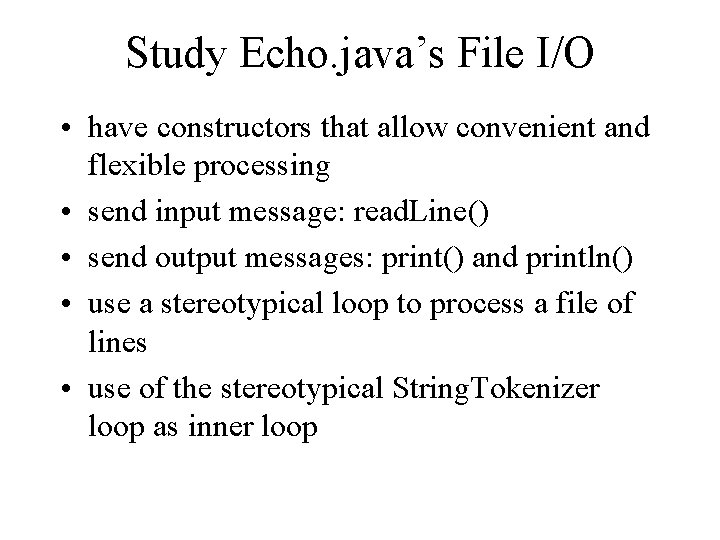
Study Echo. java’s File I/O • have constructors that allow convenient and flexible processing • send input message: read. Line() • send output messages: print() and println() • use a stereotypical loop to process a file of lines • use of the stereotypical String. Tokenizer loop as inner loop
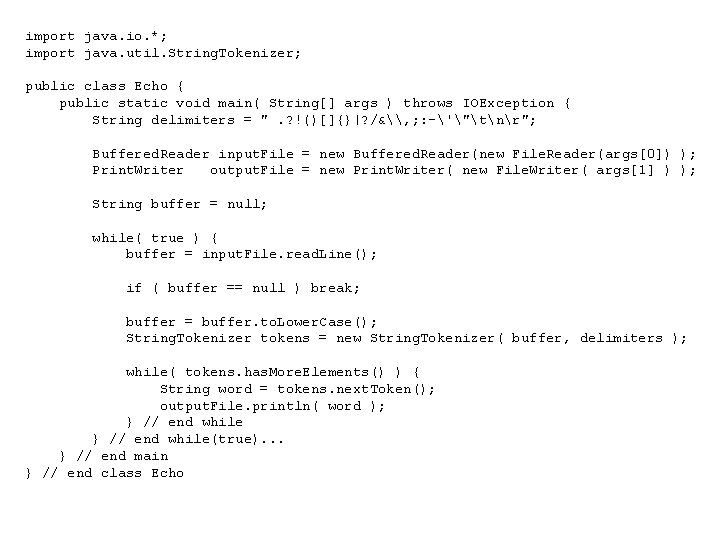
import java. io. *; import java. util. String. Tokenizer; public class Echo { public static void main( String[] args ) throws IOException { String delimiters = ". ? !()[]{}|? /&\, ; : -'"tnr"; Buffered. Reader input. File = new Buffered. Reader(new File. Reader(args[0]) ); Print. Writer output. File = new Print. Writer( new File. Writer( args[1] ) ); String buffer = null; while( true ) { buffer = input. File. read. Line(); if ( buffer == null ) break; buffer = buffer. to. Lower. Case(); String. Tokenizer tokens = new String. Tokenizer( buffer, delimiters ); while( tokens. has. More. Elements() ) { String word = tokens. next. Token(); output. File. println( word ); } // end while(true). . . } // end main } // end class Echo
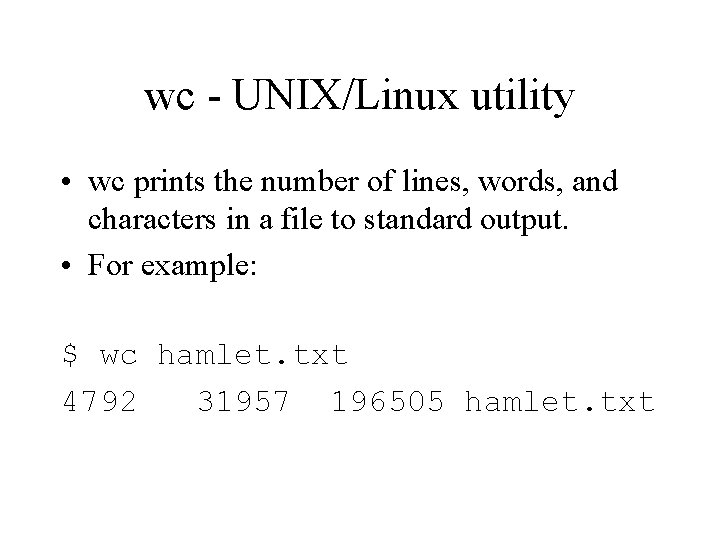
wc - UNIX/Linux utility • wc prints the number of lines, words, and characters in a file to standard output. • For example: $ wc hamlet. txt 4792 31957 196505 hamlet. txt
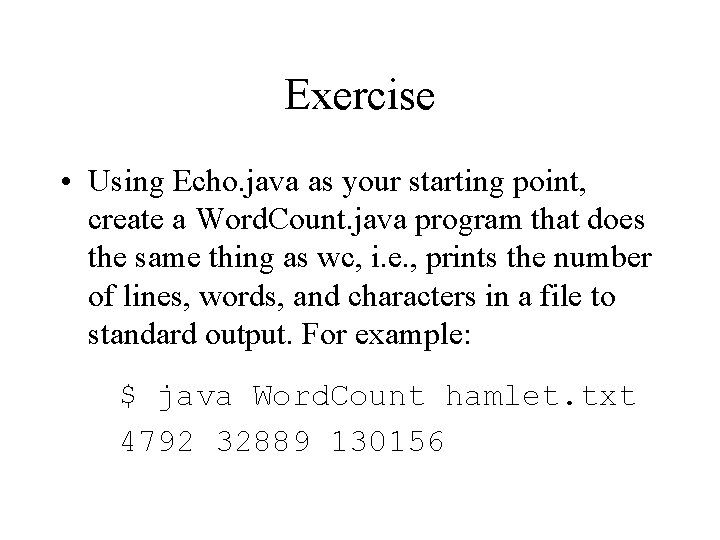
Exercise • Using Echo. java as your starting point, create a Word. Count. java program that does the same thing as wc, i. e. , prints the number of lines, words, and characters in a file to standard output. For example: $ java Word. Count hamlet. txt 4792 32889 130156
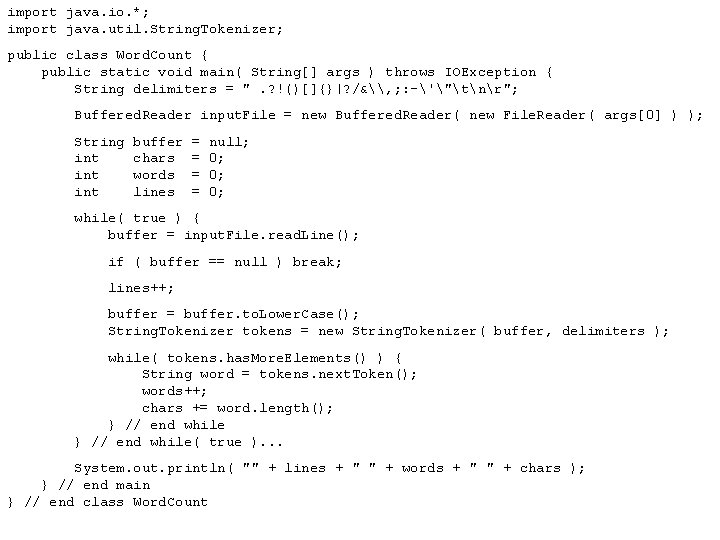
import java. io. *; import java. util. String. Tokenizer; public class Word. Count { public static void main( String[] args ) throws IOException { String delimiters = ". ? !()[]{}|? /&\, ; : -'"tnr"; Buffered. Reader input. File = new Buffered. Reader( new File. Reader( args[0] ) ); String int int buffer chars words lines = = null; 0; 0; 0; while( true ) { buffer = input. File. read. Line(); if ( buffer == null ) break; lines++; buffer = buffer. to. Lower. Case(); String. Tokenizer tokens = new String. Tokenizer( buffer, delimiters ); while( tokens. has. More. Elements() ) { String word = tokens. next. Token(); words++; chars += word. length(); } // end while( true ). . . System. out. println( "" + lines + " " + words + " " + chars ); } // end main } // end class Word. Count
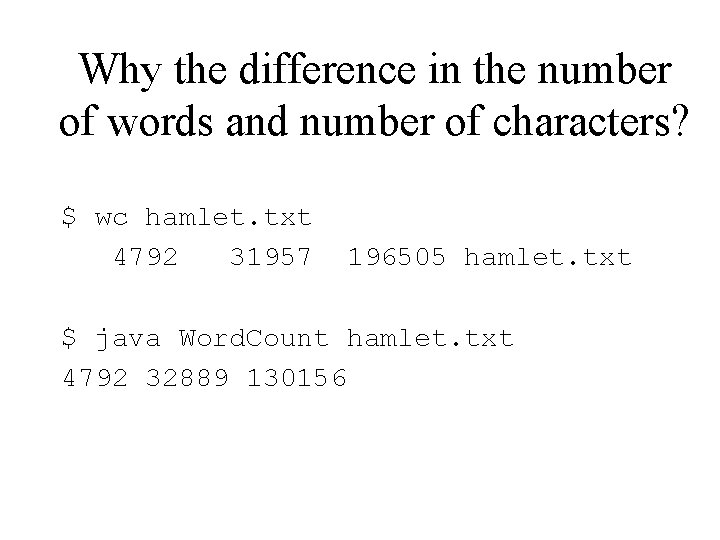
Why the difference in the number of words and number of characters? $ wc hamlet. txt 4792 31957 196505 hamlet. txt $ java Word. Count hamlet. txt 4792 32889 130156