class Student 1 public Student class Student pravite
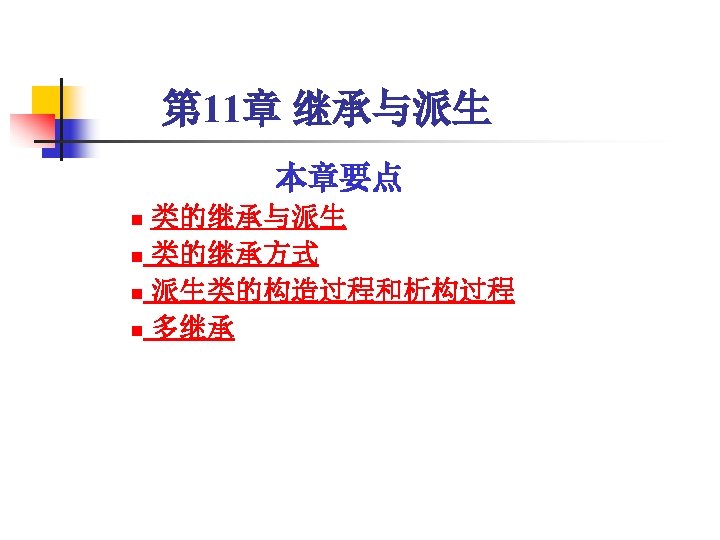
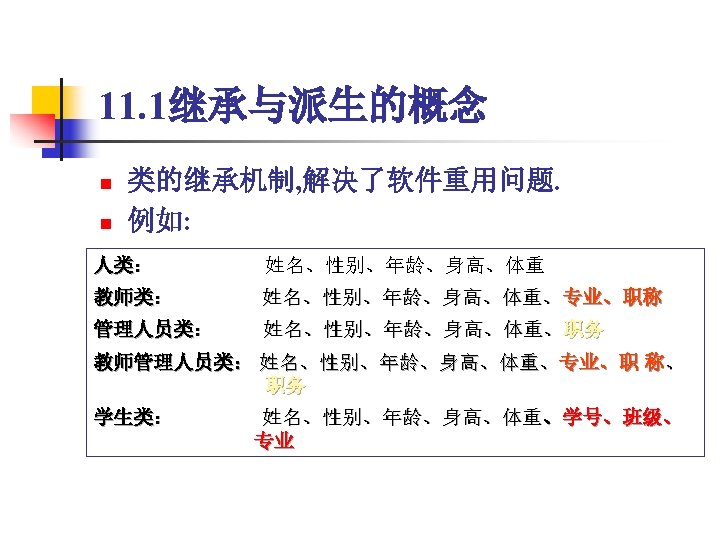
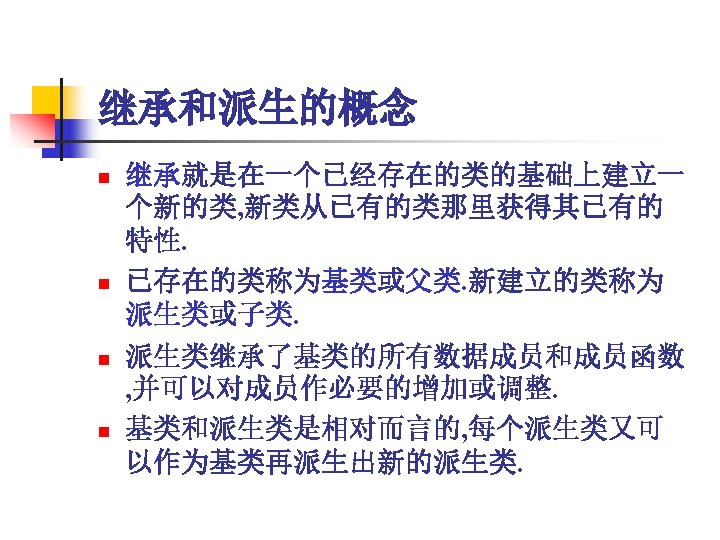
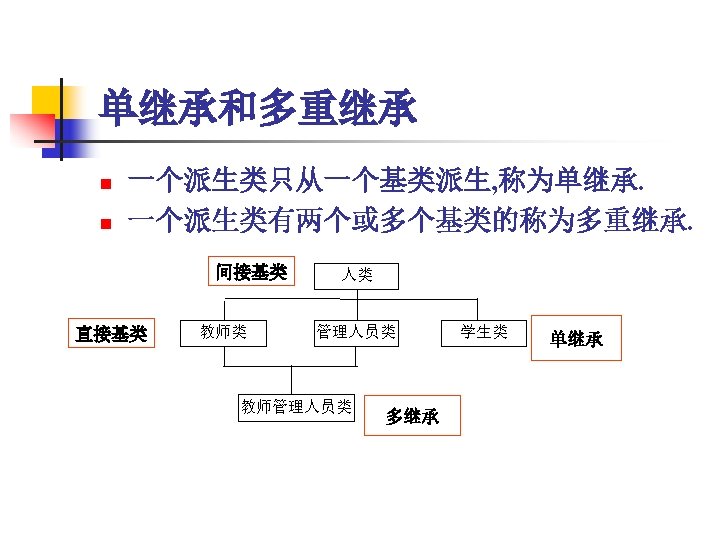
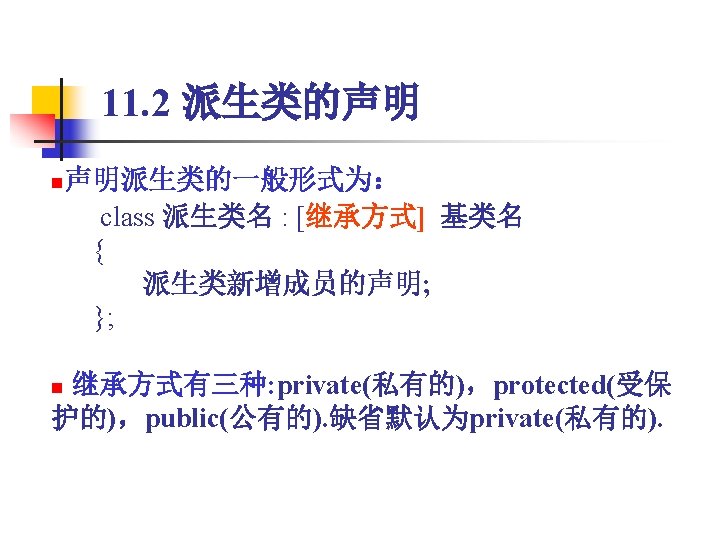
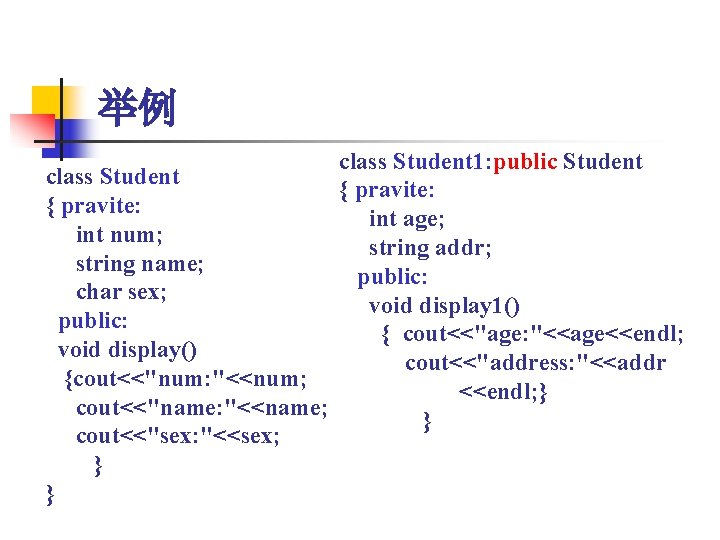
举例 class Student 1: public Student class Student { pravite: int age; int num; string addr; string name; public: char sex; void display 1() public: { cout<<"age: "<<age<<endl; void display() cout<<"address: "<<addr {cout<<"num: "<<num; <<endl; } cout<<"name: "<<name; } cout<<"sex: "<<sex; } }
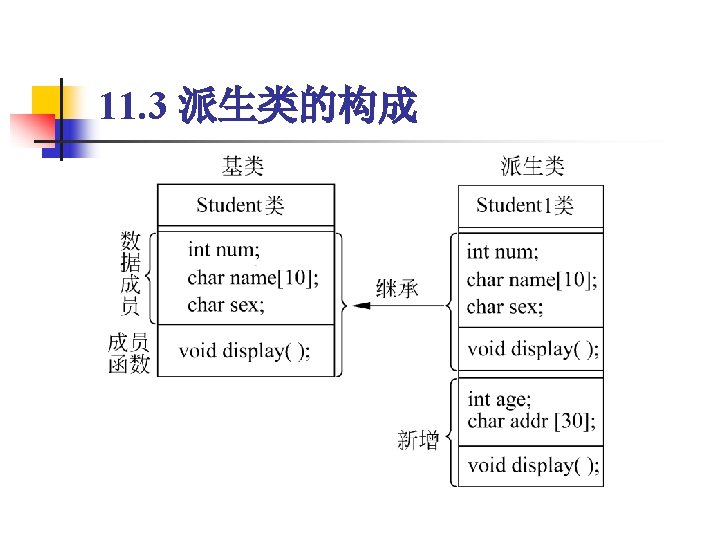
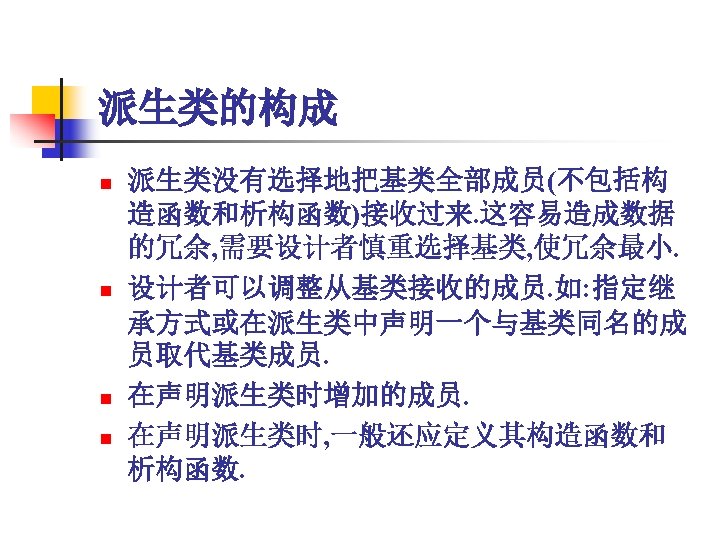
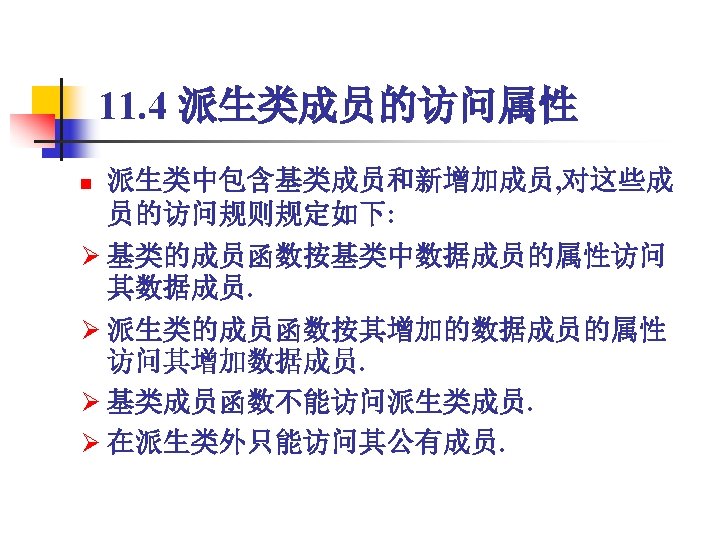
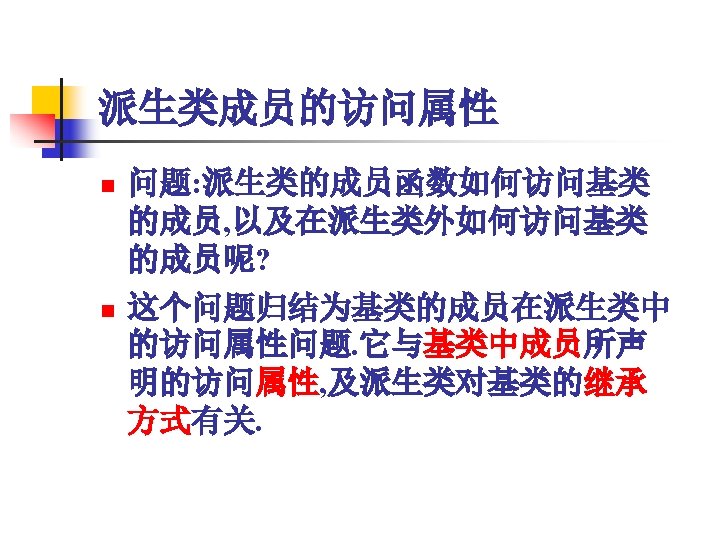
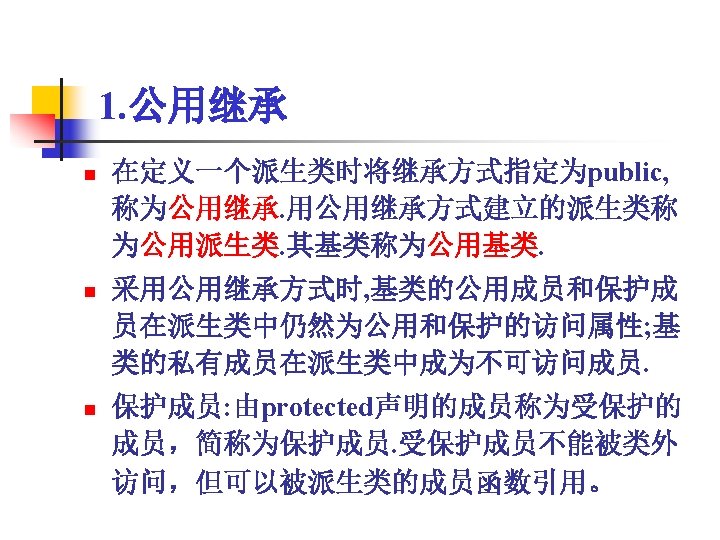
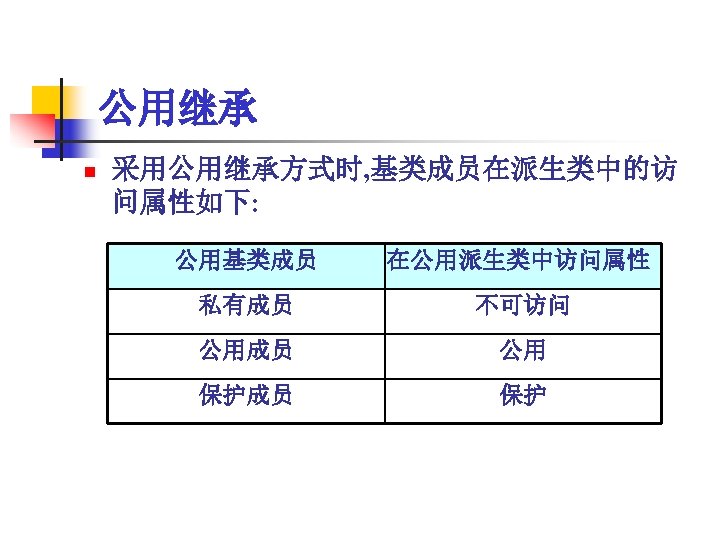
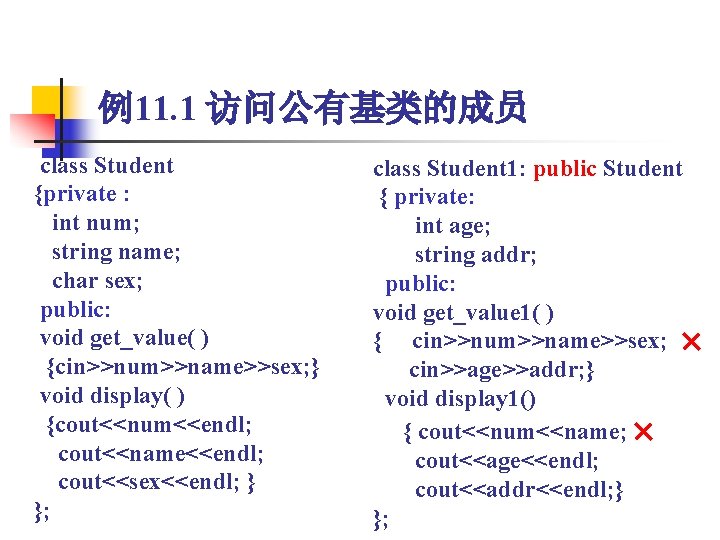
例11. 1 访问公有基类的成员 class Student {private : int num; string name; char sex; public: void get_value( ) {cin>>num>>name>>sex; } void display( ) {cout<<num<<endl; cout<<name<<endl; cout<<sex<<endl; } }; class Student 1: public Student { private: int age; string addr; public: void get_value 1( ) { cin>>num>>name>>sex; × cin>>age>>addr; } void display 1() { cout<<num<<name; × cout<<age<<endl; cout<<addr<<endl; } };
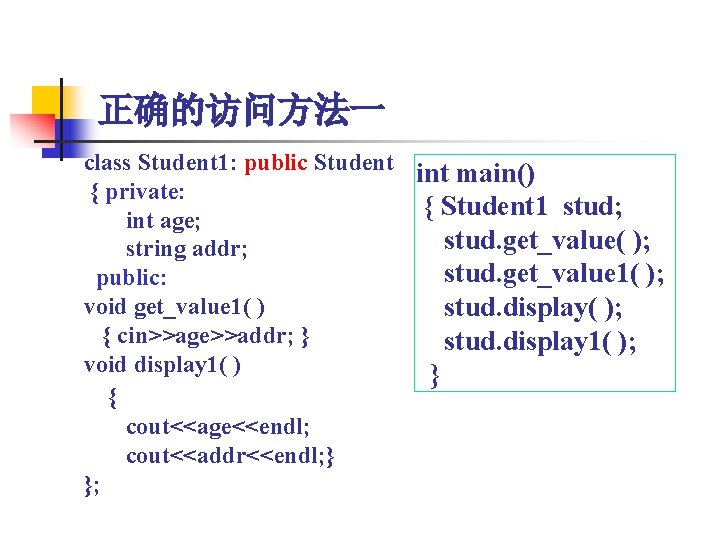
正确的访问方法一 class Student 1: public Student int main() { private: { Student 1 stud; int age; stud. get_value( ); string addr; stud. get_value 1( ); public: void get_value 1( ) stud. display( ); { cin>>age>>addr; } stud. display 1( ); void display 1( ) } { cout<<age<<endl; cout<<addr<<endl; } };
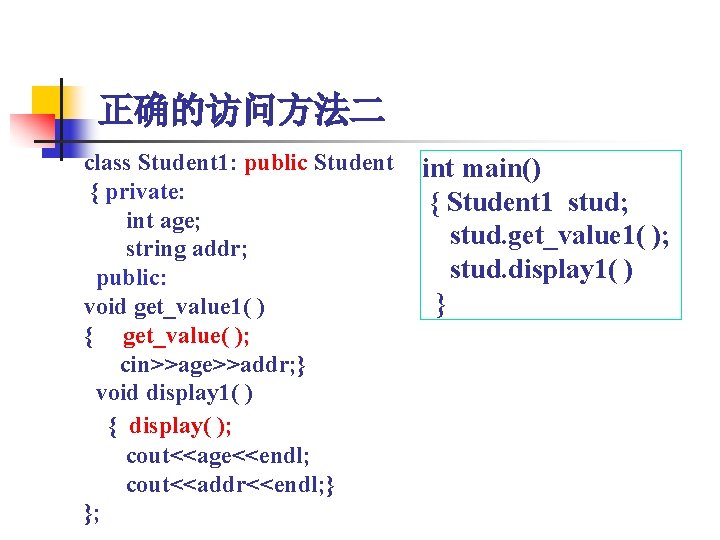
正确的访问方法二 class Student 1: public Student { private: int age; string addr; public: void get_value 1( ) { get_value( ); cin>>age>>addr; } void display 1( ) { display( ); cout<<age<<endl; cout<<addr<<endl; } }; int main() { Student 1 stud; stud. get_value 1( ); stud. display 1( ) }
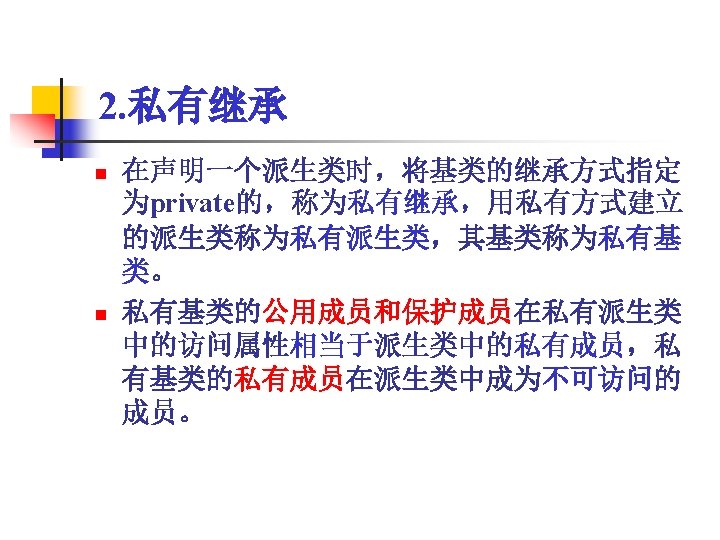
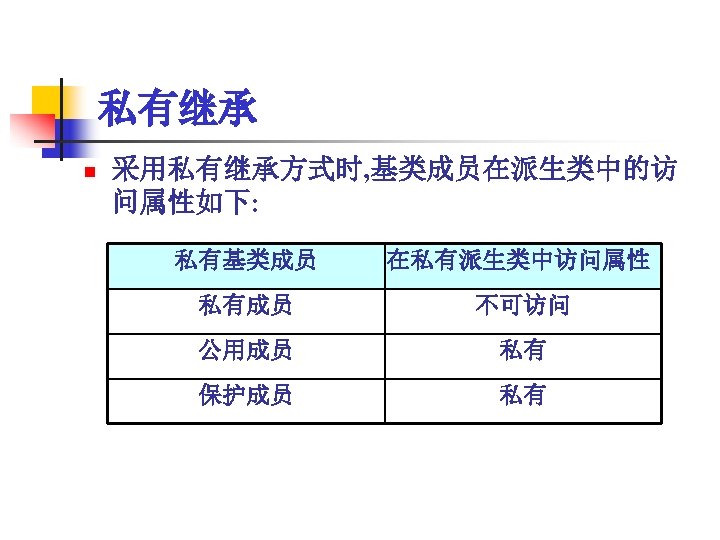
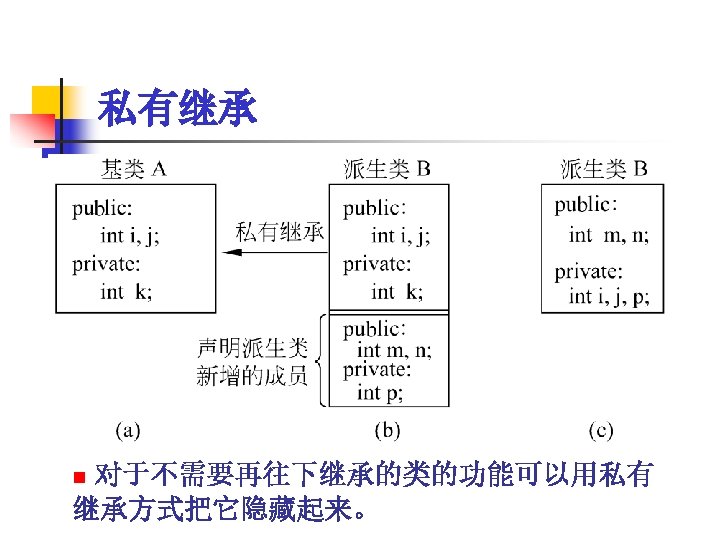
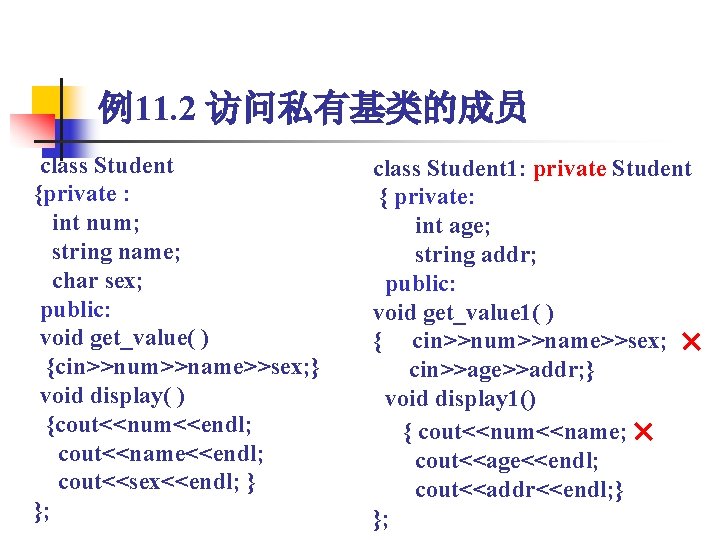
例11. 2 访问私有基类的成员 class Student {private : int num; string name; char sex; public: void get_value( ) {cin>>num>>name>>sex; } void display( ) {cout<<num<<endl; cout<<name<<endl; cout<<sex<<endl; } }; class Student 1: private Student { private: int age; string addr; public: void get_value 1( ) { cin>>num>>name>>sex; × cin>>age>>addr; } void display 1() { cout<<num<<name; × cout<<age<<endl; cout<<addr<<endl; } };
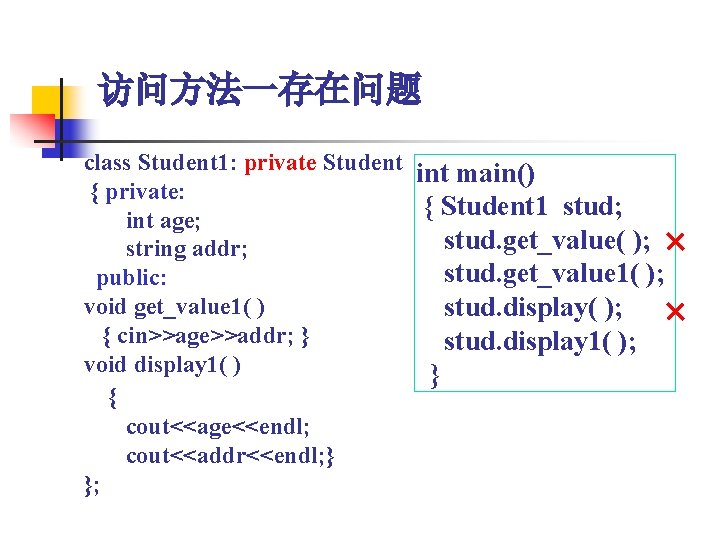
访问方法一存在问题 class Student 1: private Student int main() { private: { Student 1 stud; int age; stud. get_value( ); × string addr; stud. get_value 1( ); public: void get_value 1( ) stud. display( ); × { cin>>age>>addr; } stud. display 1( ); void display 1( ) } { cout<<age<<endl; cout<<addr<<endl; } };
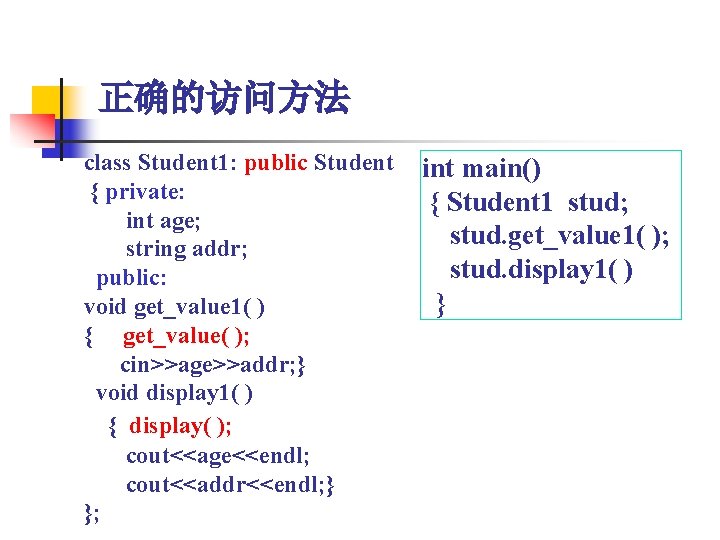
正确的访问方法 class Student 1: public Student { private: int age; string addr; public: void get_value 1( ) { get_value( ); cin>>age>>addr; } void display 1( ) { display( ); cout<<age<<endl; cout<<addr<<endl; } }; int main() { Student 1 stud; stud. get_value 1( ); stud. display 1( ) }
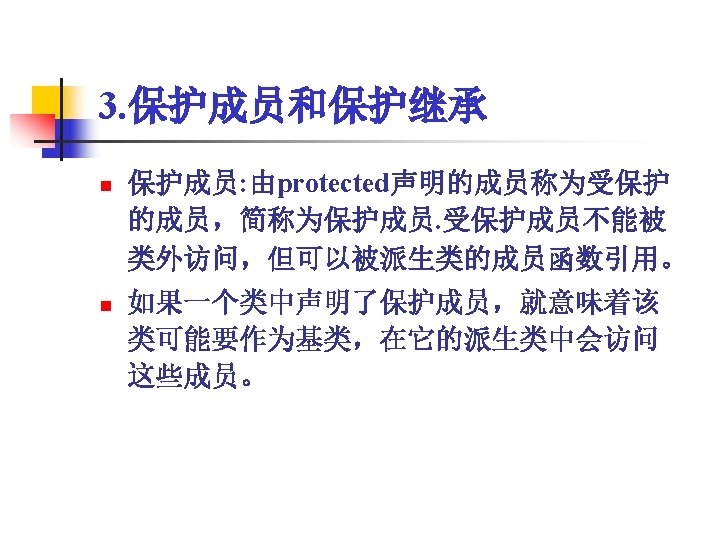
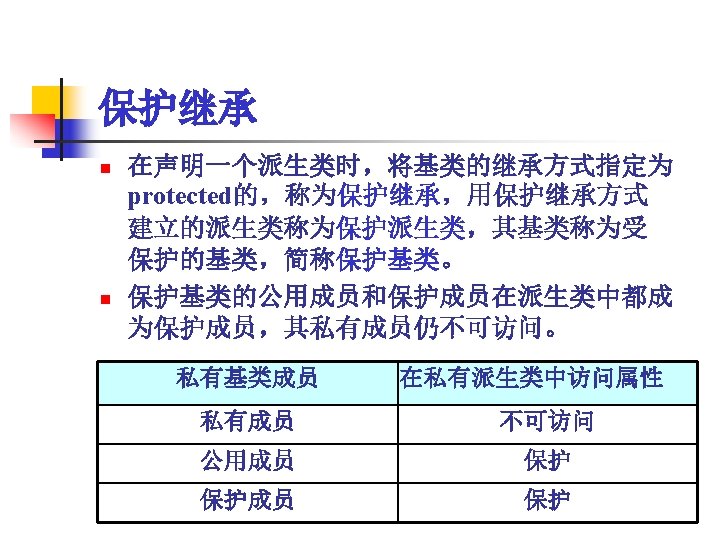
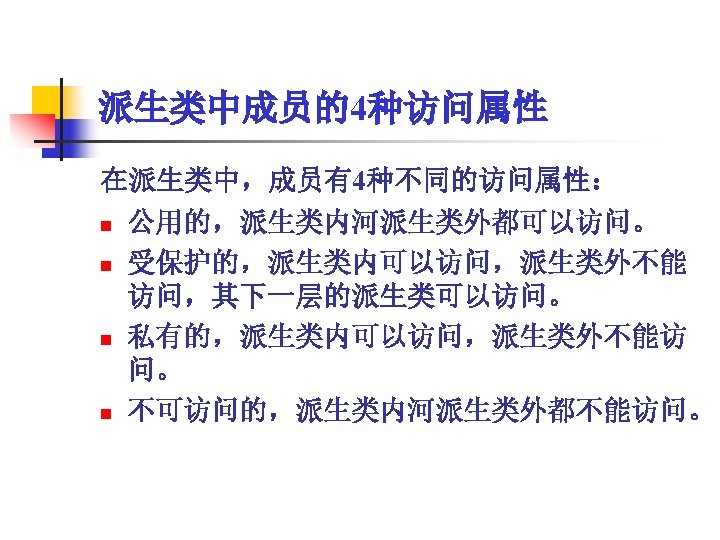
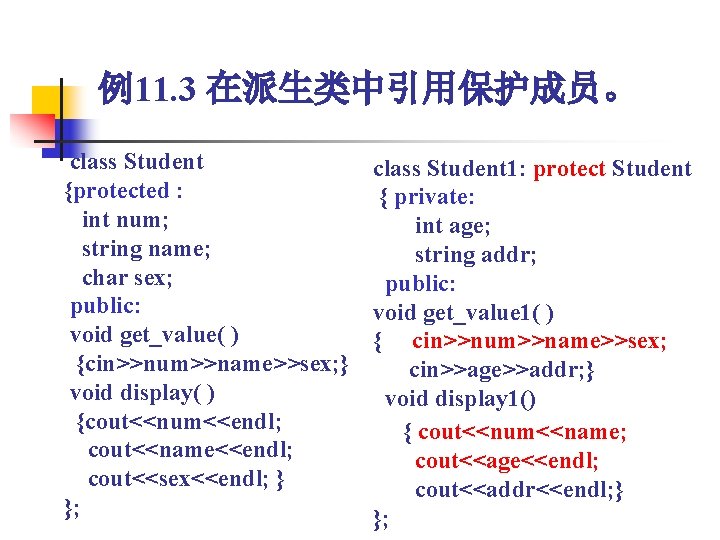
例11. 3 在派生类中引用保护成员。 class Student {protected : int num; string name; char sex; public: void get_value( ) {cin>>num>>name>>sex; } void display( ) {cout<<num<<endl; cout<<name<<endl; cout<<sex<<endl; } }; class Student 1: protect Student { private: int age; string addr; public: void get_value 1( ) { cin>>num>>name>>sex; cin>>age>>addr; } void display 1() { cout<<num<<name; cout<<age<<endl; cout<<addr<<endl; } };
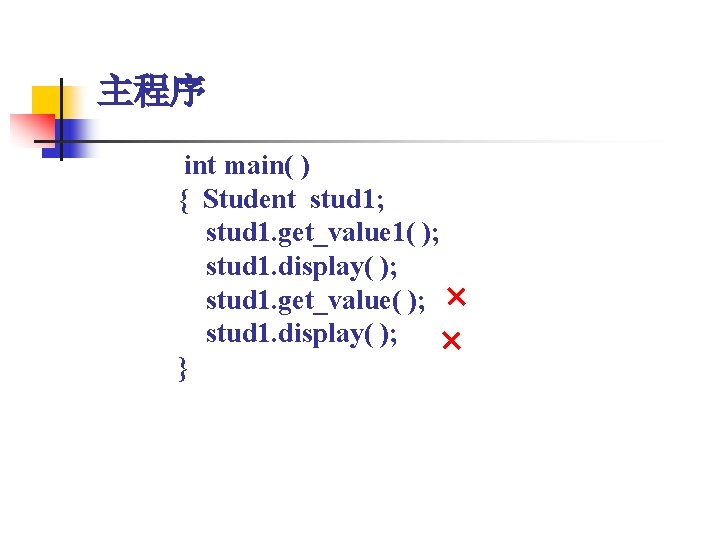
主程序 int main( ) { Student stud 1; stud 1. get_value 1( ); stud 1. display( ); stud 1. get_value( ); × stud 1. display( ); × }
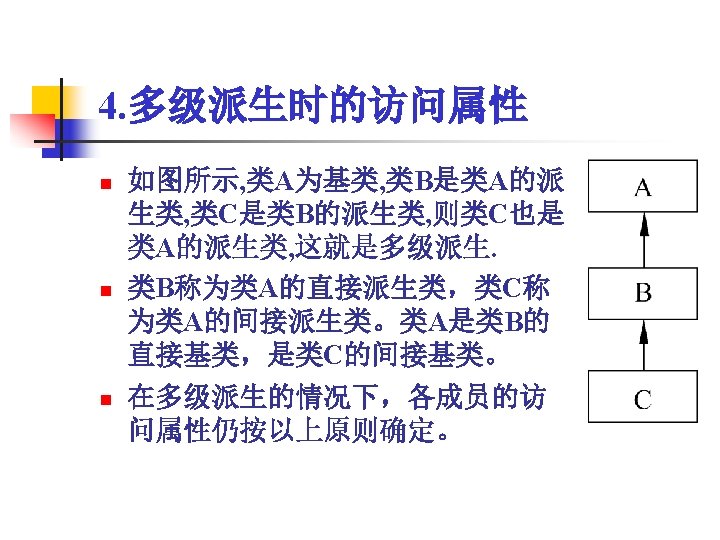
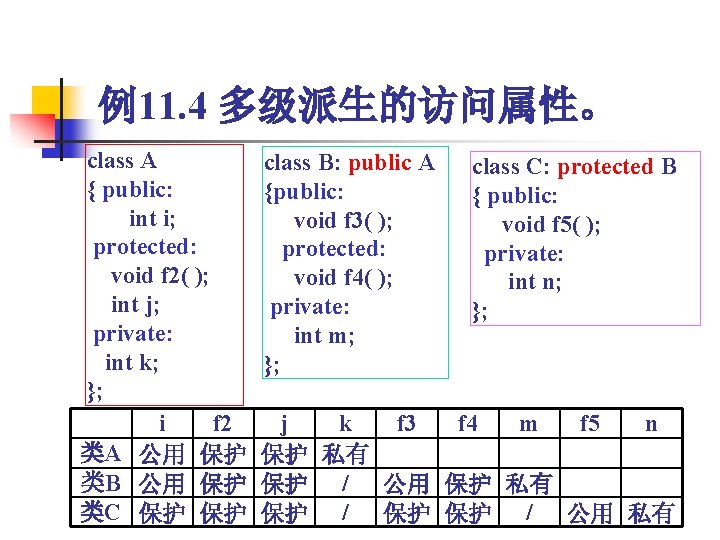
例11. 4 多级派生的访问属性。 class A { public: int i; protected: void f 2( ); int j; private: int k; }; i f 2 类A 公用 保护 类B 公用 保护 类C 保护 保护 class B: public A {public: void f 3( ); protected: void f 4( ); private: int m; }; class C: protected B { public: void f 5( ); private: int n; }; j k f 3 f 4 m f 5 n 保护 私有 保护 / 公用 保护 私有 保护 / 保护 保护 / 公用 私有
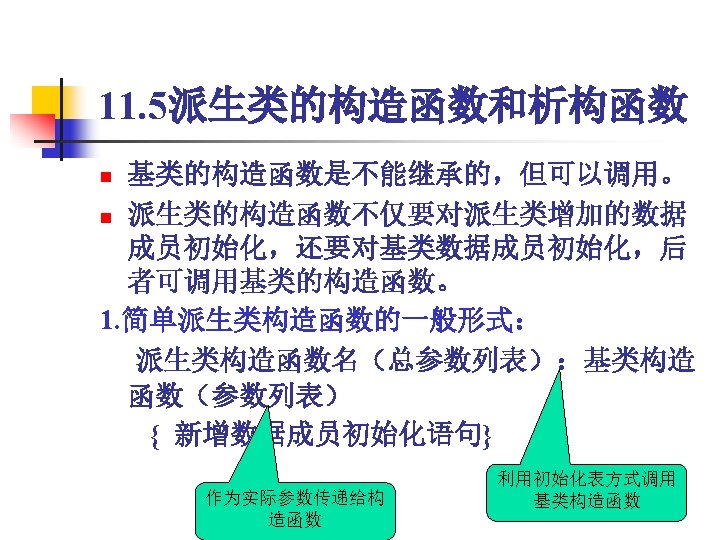
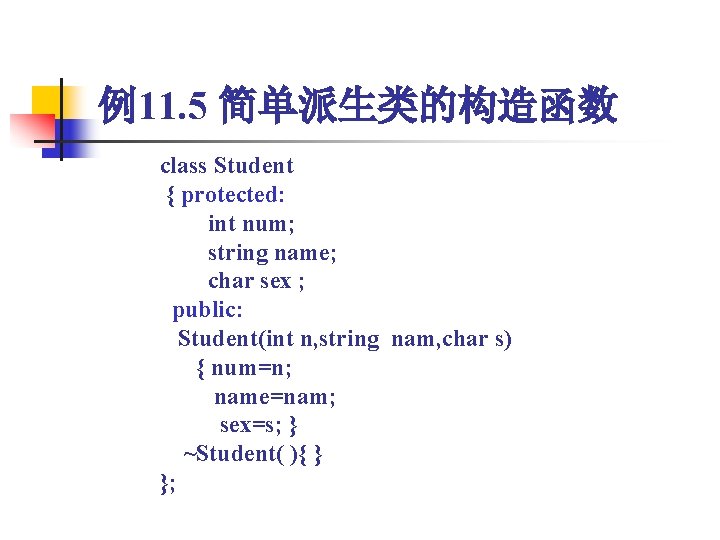
例11. 5 简单派生类的构造函数 class Student { protected: int num; string name; char sex ; public: Student(int n, string nam, char s) { num=n; name=nam; sex=s; } ~Student( ){ } };
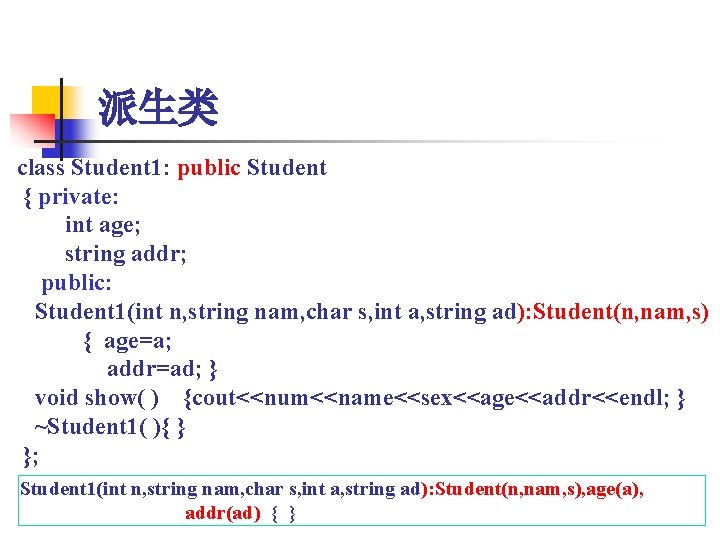
派生类 class Student 1: public Student { private: int age; string addr; public: Student 1(int n, string nam, char s, int a, string ad): Student(n, nam, s) { age=a; addr=ad; } void show( ) {cout<<num<<name<<sex<<age<<addr<<endl; } ~Student 1( ){ } }; Student 1(int n, string nam, char s, int a, string ad): Student(n, nam, s), age(a), addr(ad) { }
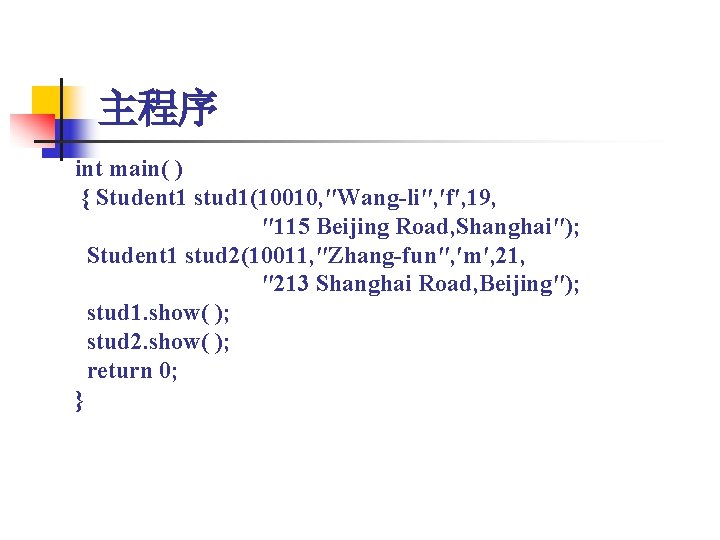
主程序 int main( ) { Student 1 stud 1(10010, ″Wang-li″, ′f′, 19, ″ 115 Beijing Road, Shanghai″); Student 1 stud 2(10011, ″Zhang-fun″, ′m′, 21, ″ 213 Shanghai Road, Beijing″); stud 1. show( ); stud 2. show( ); return 0; }
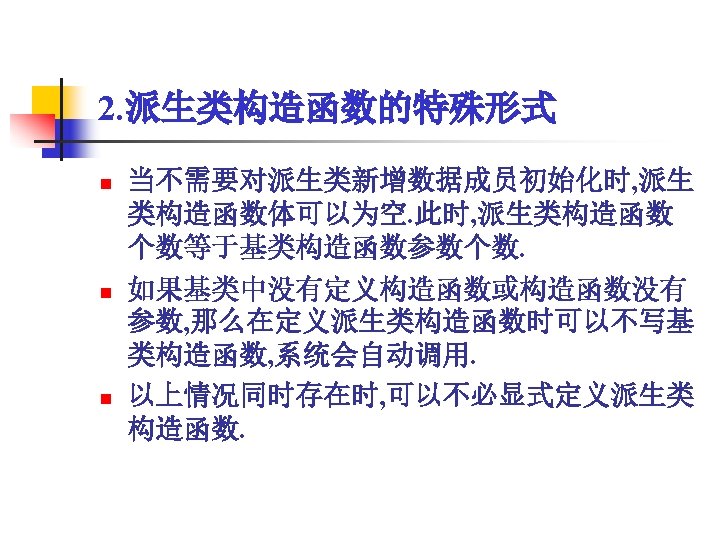
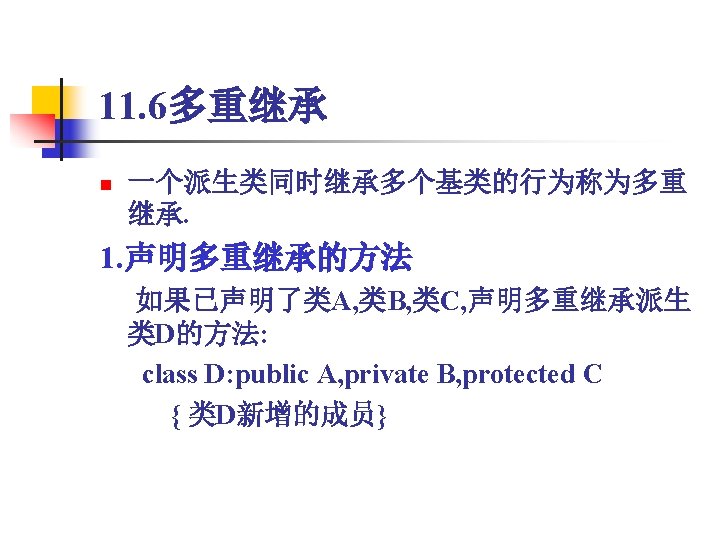
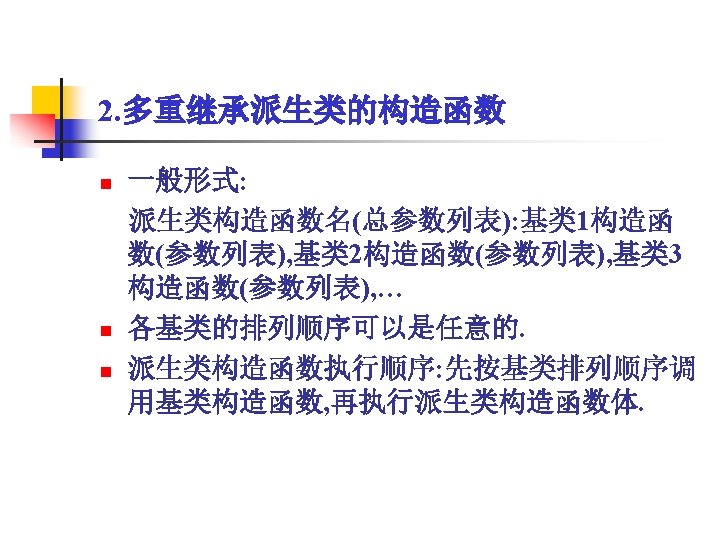
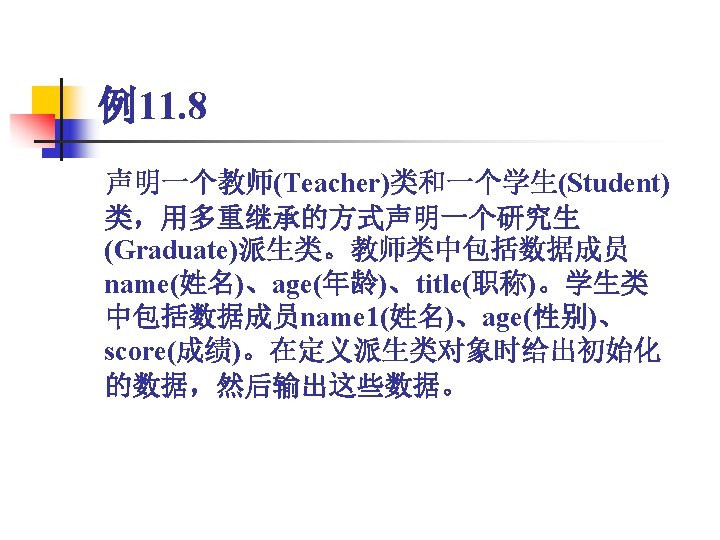
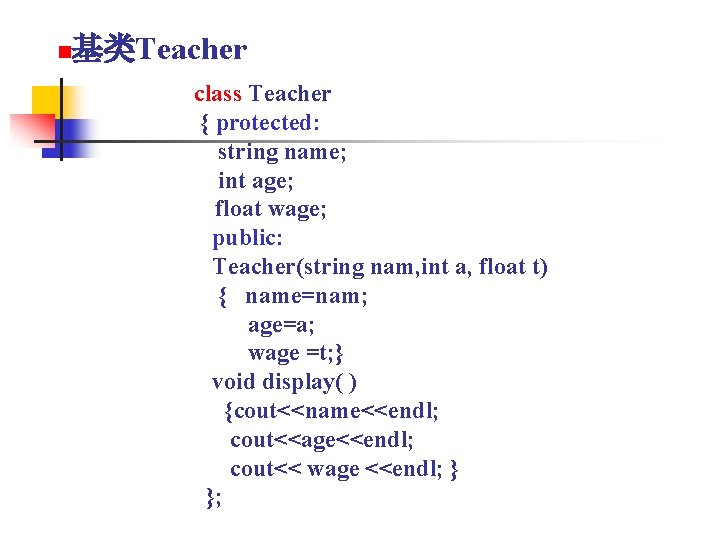
n 基类Teacher class Teacher { protected: string name; int age; float wage; public: Teacher(string nam, int a, float t) { name=nam; age=a; wage =t; } void display( ) {cout<<name<<endl; cout<<age<<endl; cout<< wage <<endl; } };
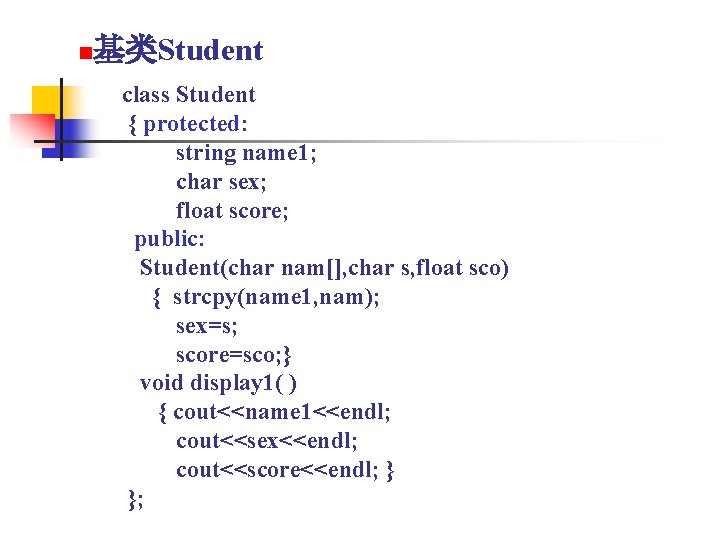
n 基类Student class Student { protected: string name 1; char sex; float score; public: Student(char nam[], char s, float sco) { strcpy(name 1, nam); sex=s; score=sco; } void display 1( ) { cout<<name 1<<endl; cout<<sex<<endl; cout<<score<<endl; } };
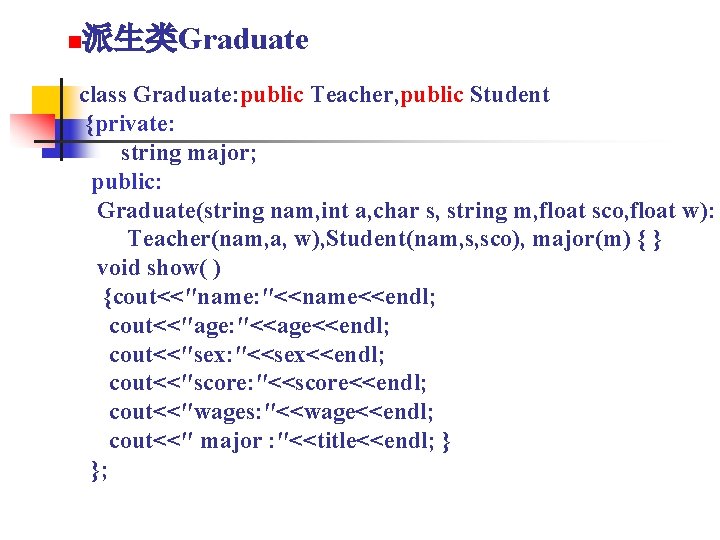
n 派生类Graduate class Graduate: public Teacher, public Student {private: string major; public: Graduate(string nam, int a, char s, string m, float sco, float w): Teacher(nam, a, w), Student(nam, s, sco), major(m) { } void show( ) {cout<<″name: ″<<name<<endl; cout<<″age: ″<<age<<endl; cout<<″sex: ″<<sex<<endl; cout<<″score: ″<<score<<endl; cout<<″wages: ″<<wage<<endl; cout<<″ major : ″<<title<<endl; } };
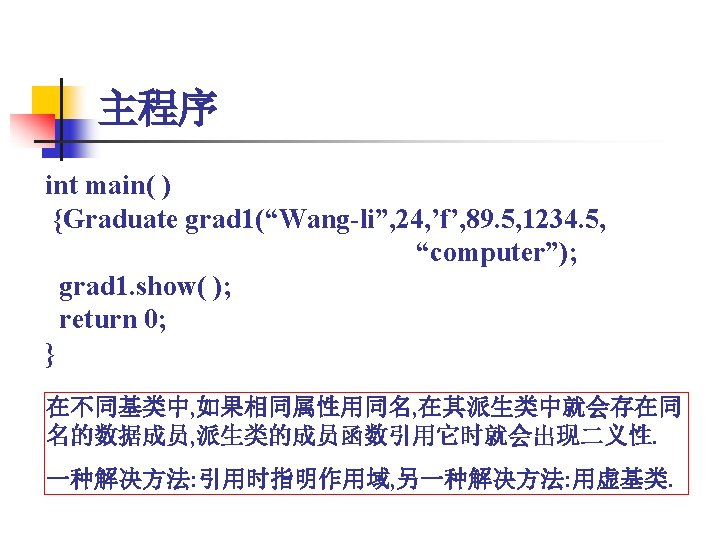
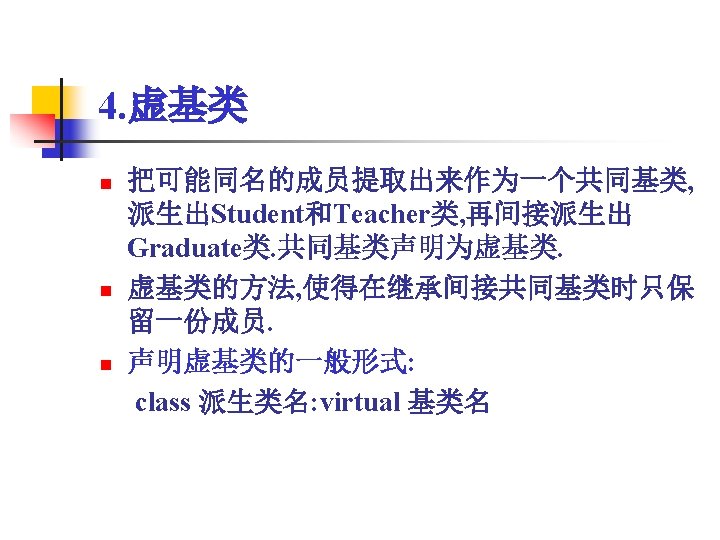
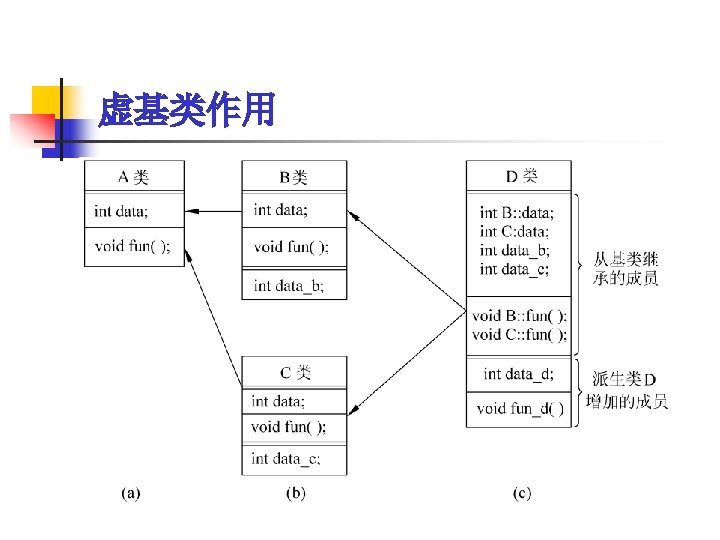
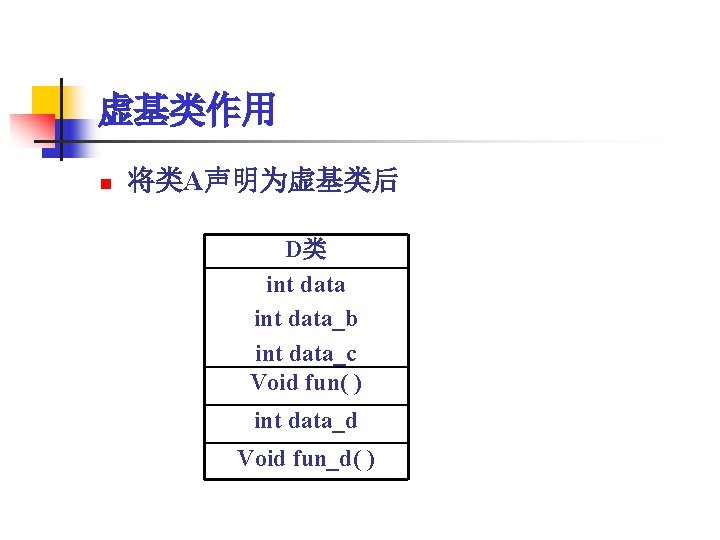
虚基类作用 n 将类A声明为虚基类后 D类 int data_b int data_c Void fun( ) int data_d Void fun_d( )
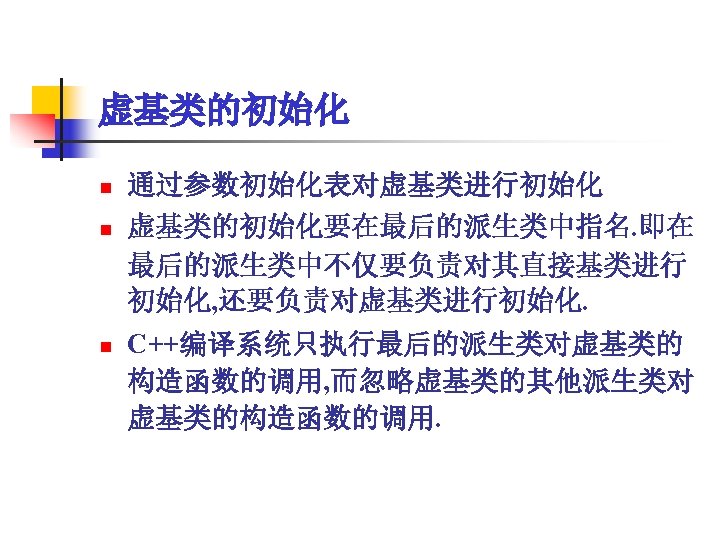
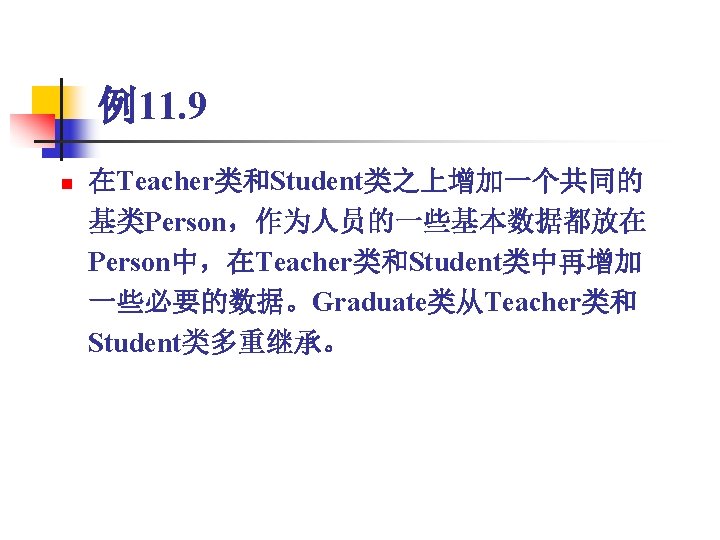
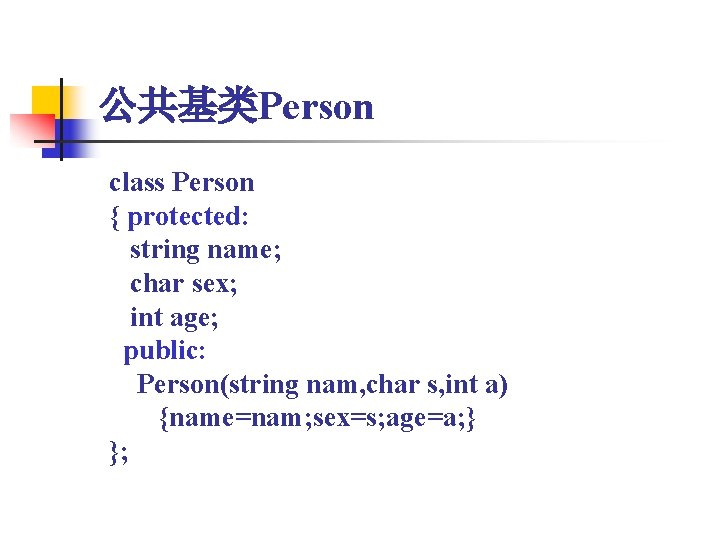
公共基类Person class Person { protected: string name; char sex; int age; public: Person(string nam, char s, int a) {name=nam; sex=s; age=a; } };
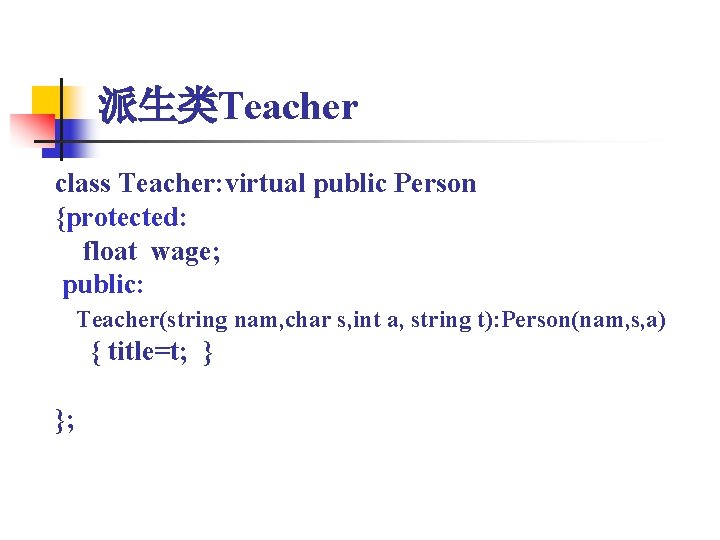
派生类Teacher class Teacher: virtual public Person {protected: float wage; public: Teacher(string nam, char s, int a, string t): Person(nam, s, a) { title=t; } };
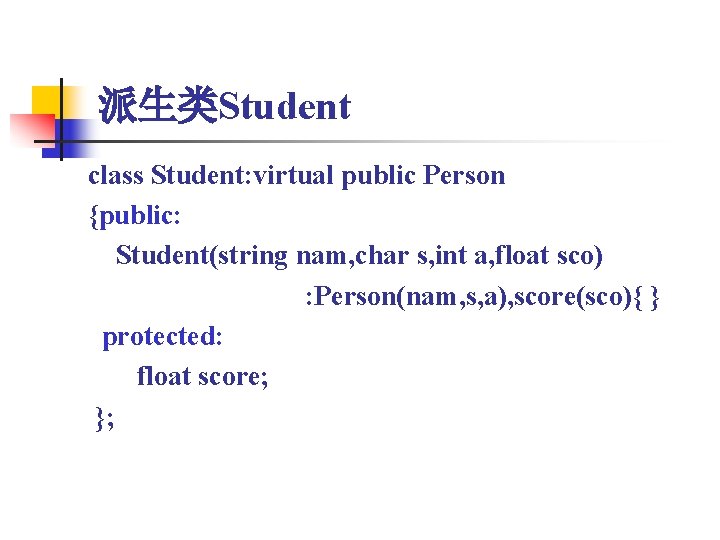
派生类Student class Student: virtual public Person {public: Student(string nam, char s, int a, float sco) : Person(nam, s, a), score(sco){ } protected: float score; };
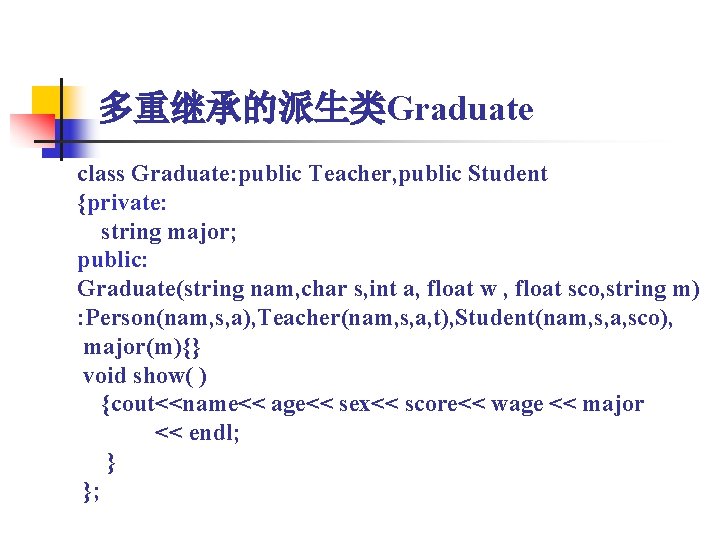
多重继承的派生类Graduate class Graduate: public Teacher, public Student {private: string major; public: Graduate(string nam, char s, int a, float w , float sco, string m) : Person(nam, s, a), Teacher(nam, s, a, t), Student(nam, s, a, sco), major(m){} void show( ) {cout<<name<< age<< sex<< score<< wage << major << endl; } };
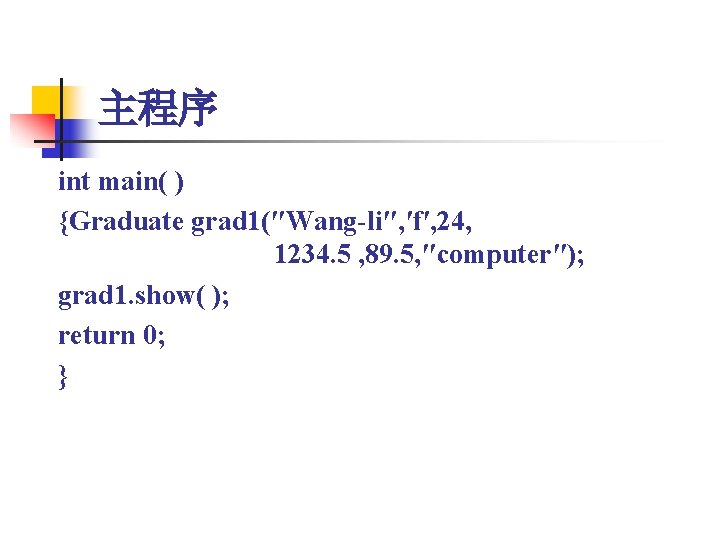
主程序 int main( ) {Graduate grad 1(″Wang-li″, ′f′, 24, 1234. 5 , 89. 5, ″computer″); grad 1. show( ); return 0; }
- Slides: 50