String Class in Java java lang Class String
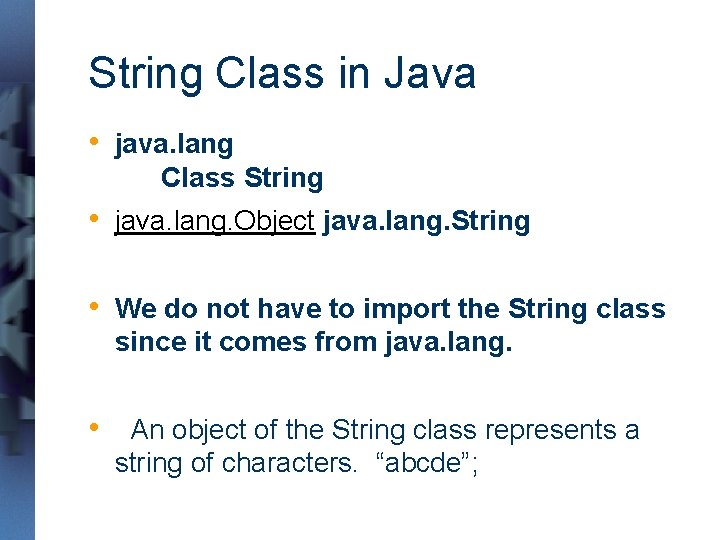
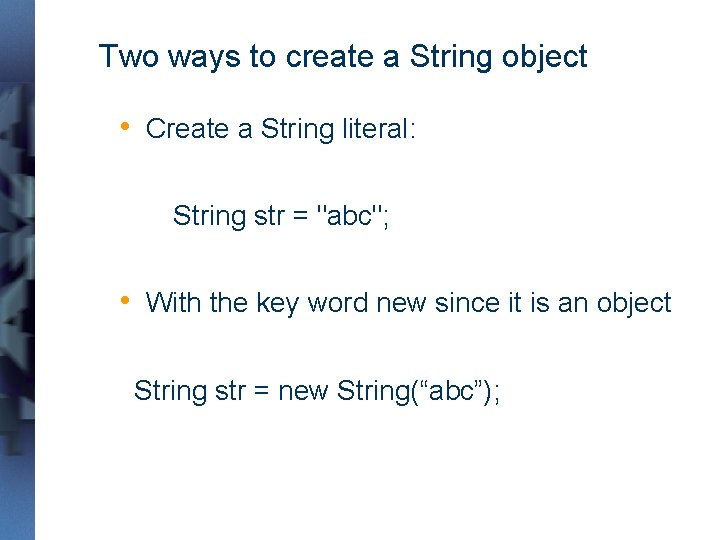
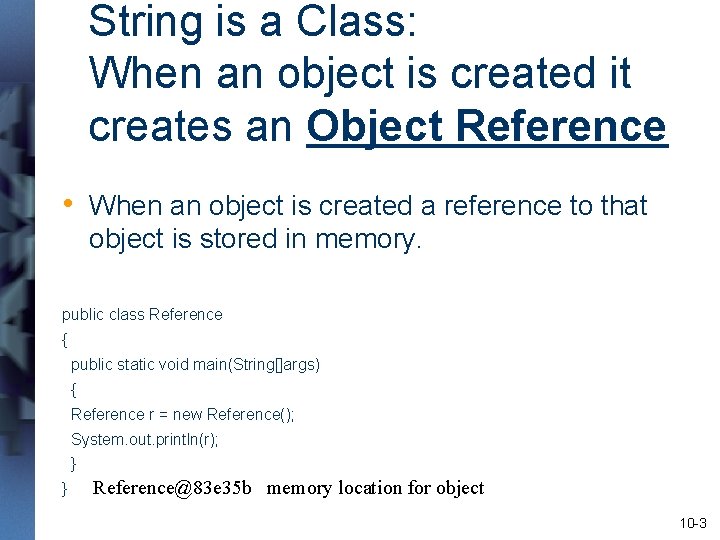
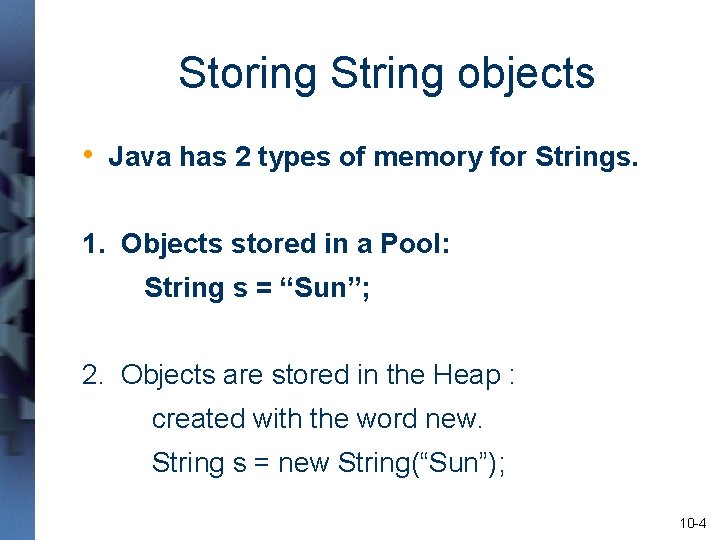
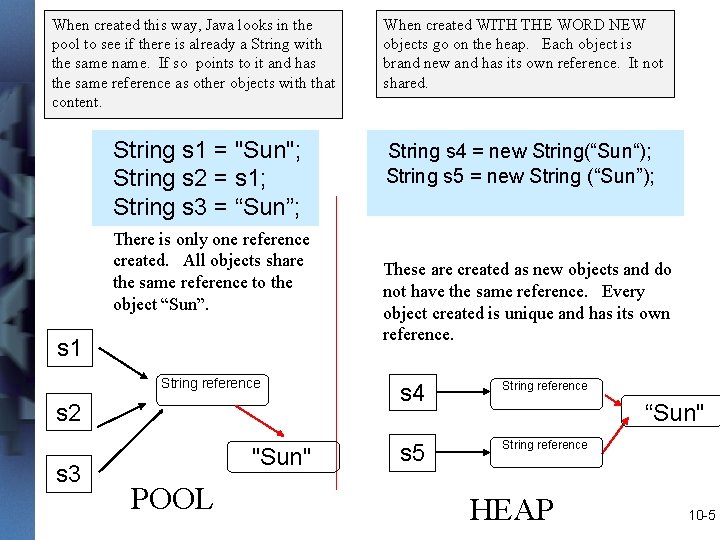
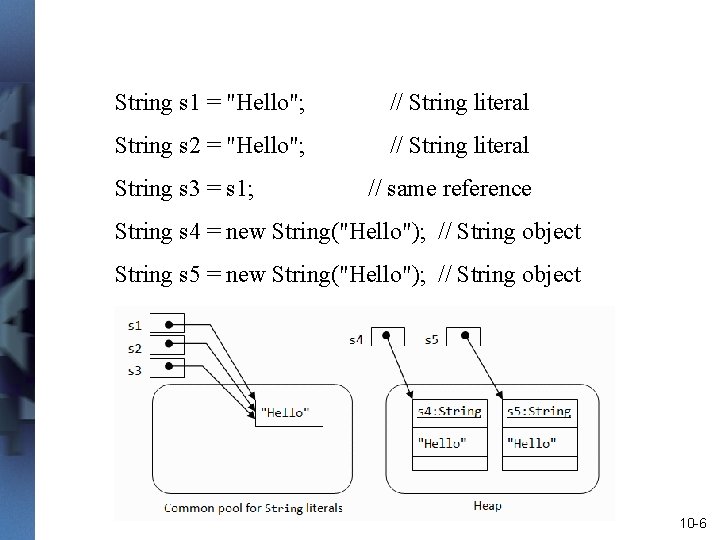
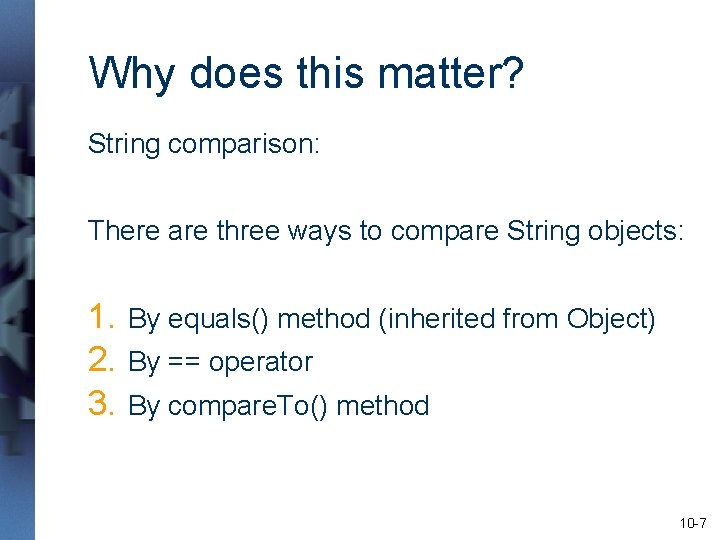
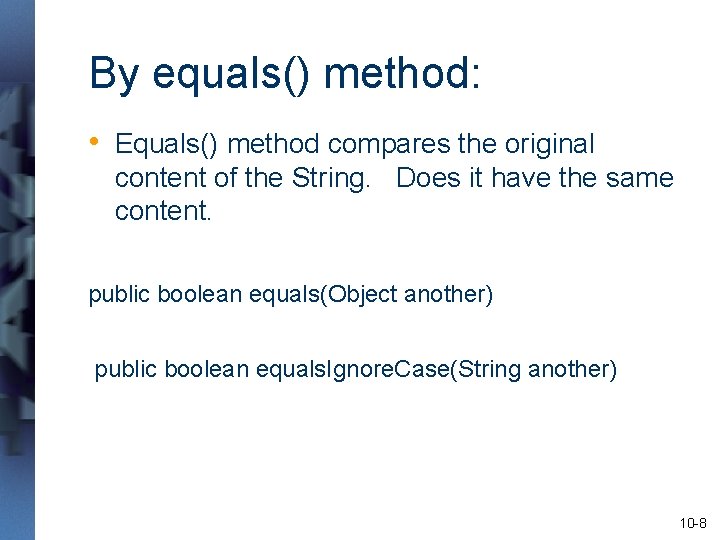
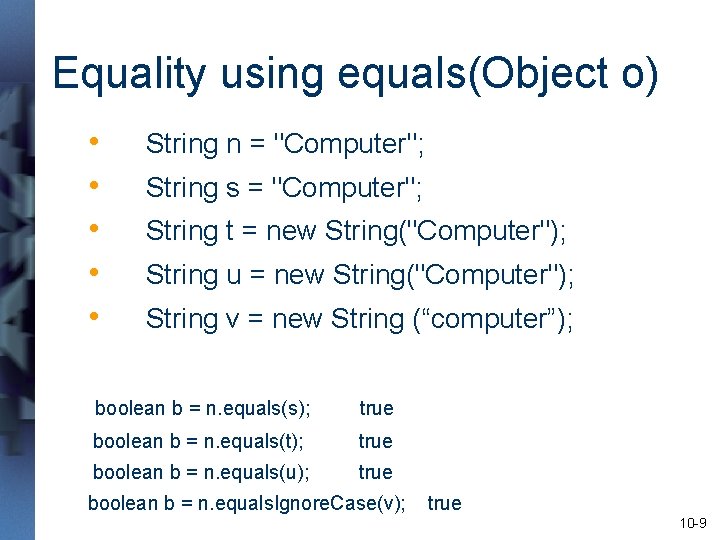
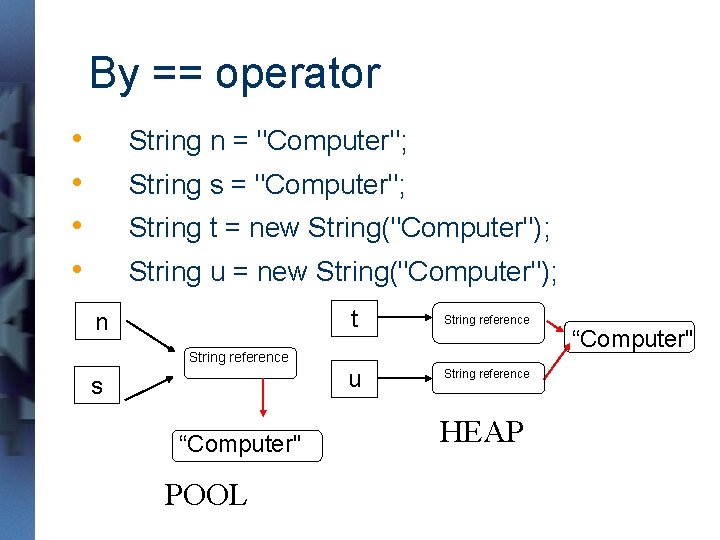
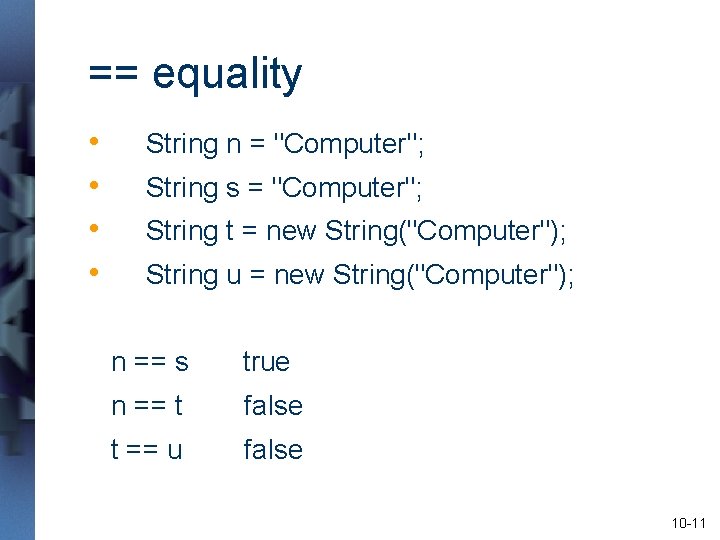
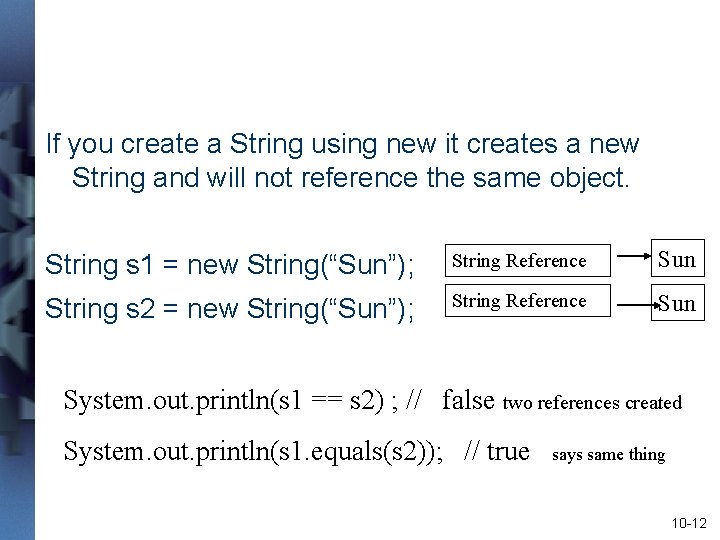
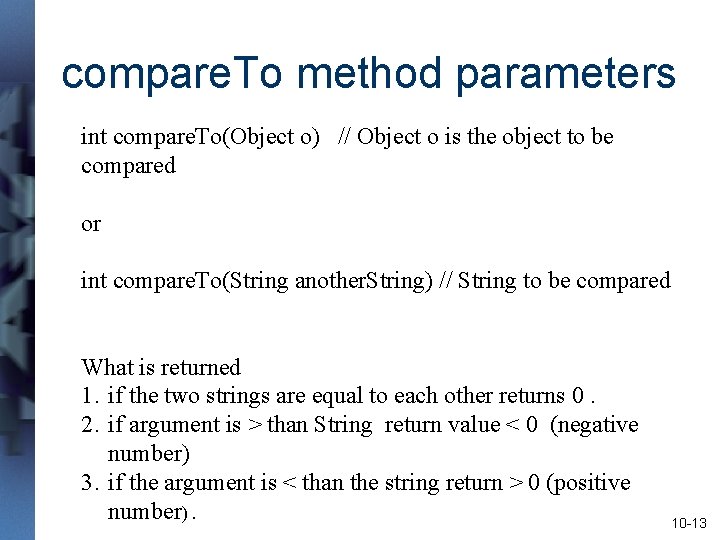
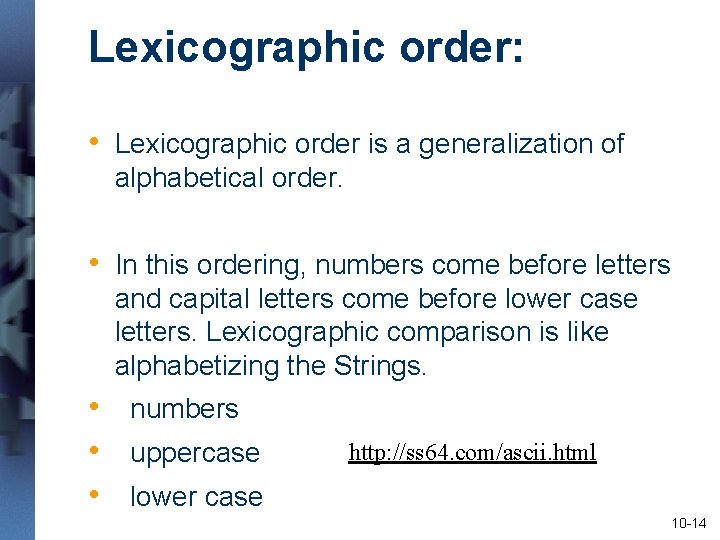
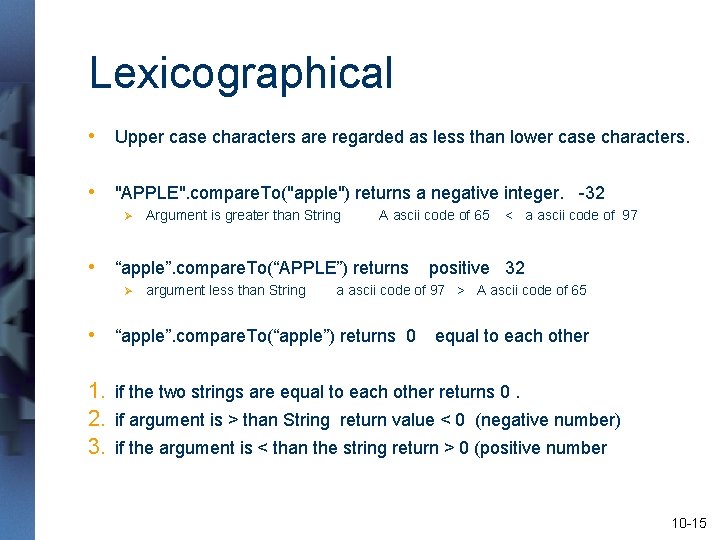
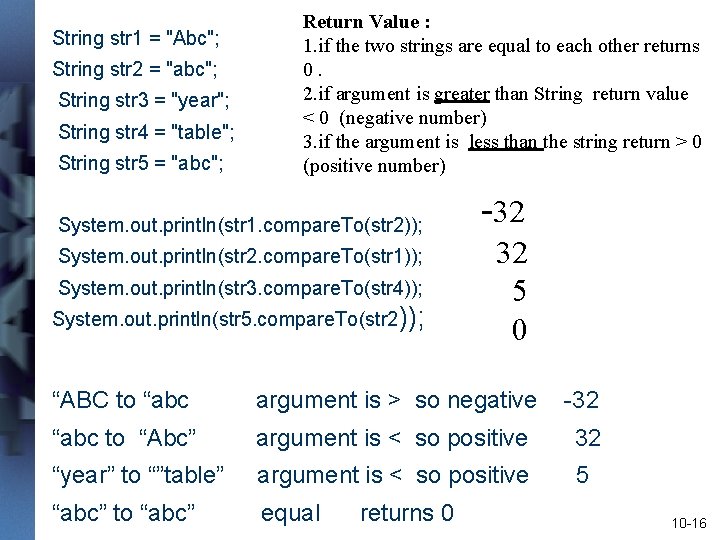
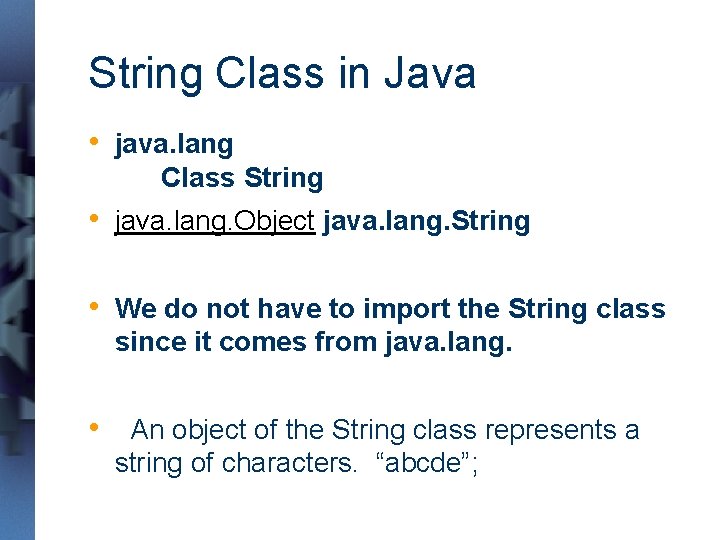
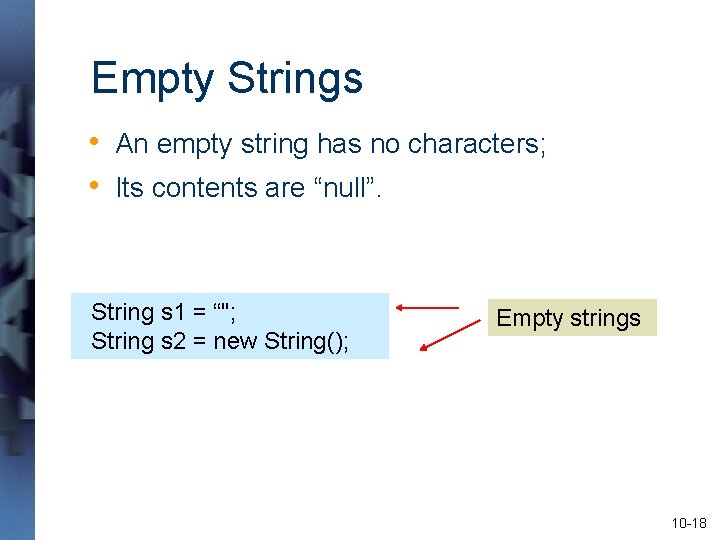
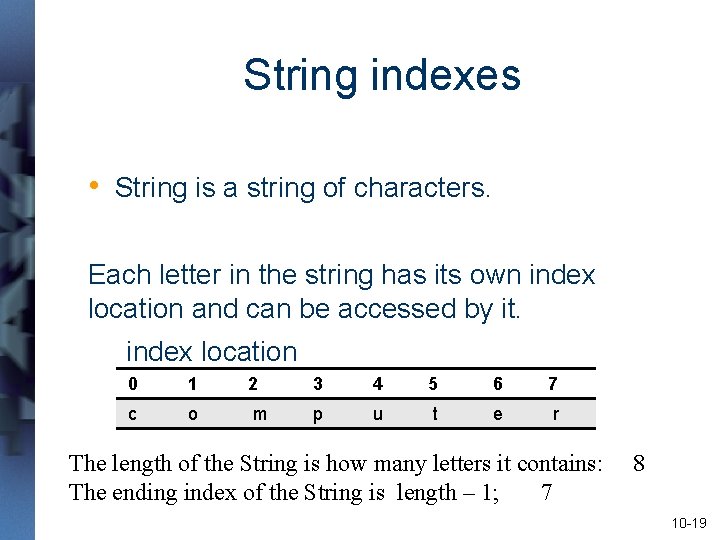
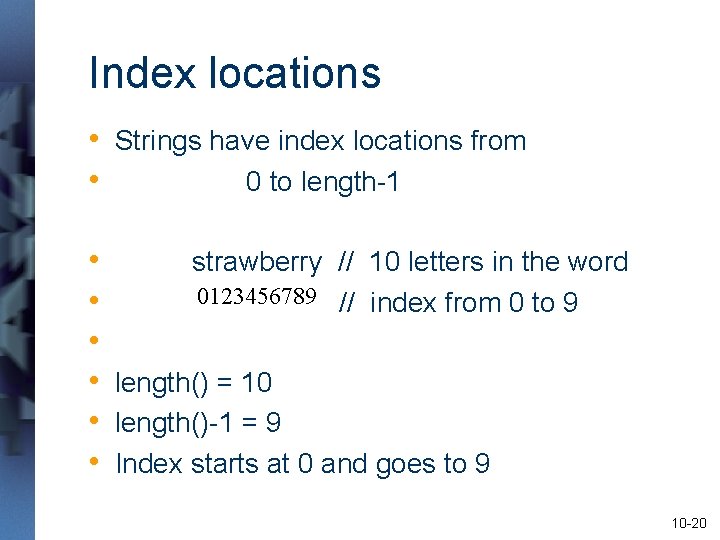
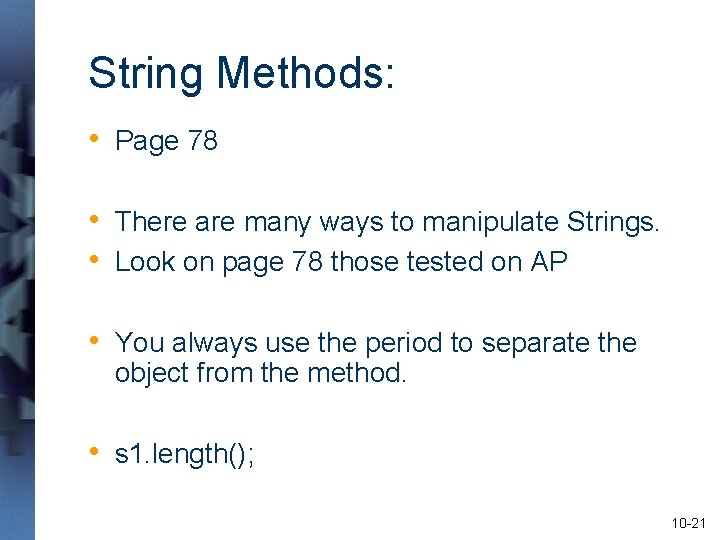
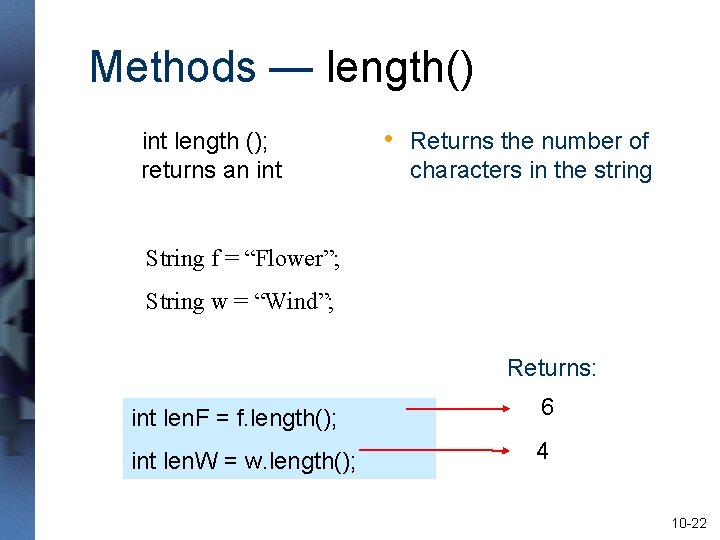
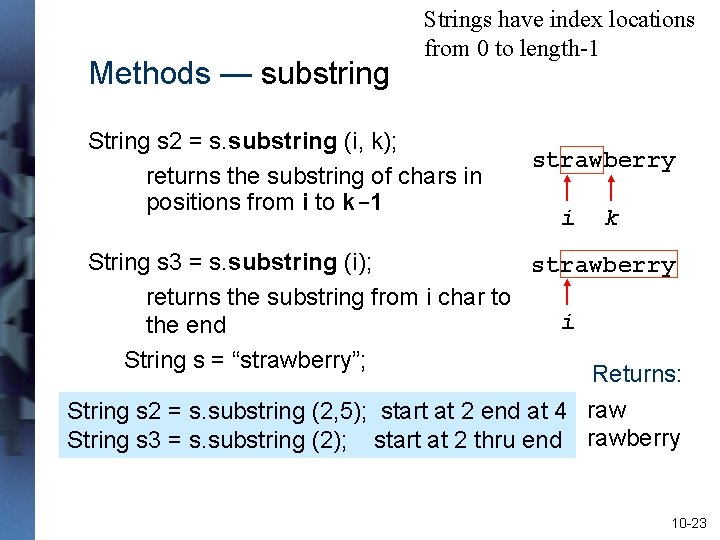
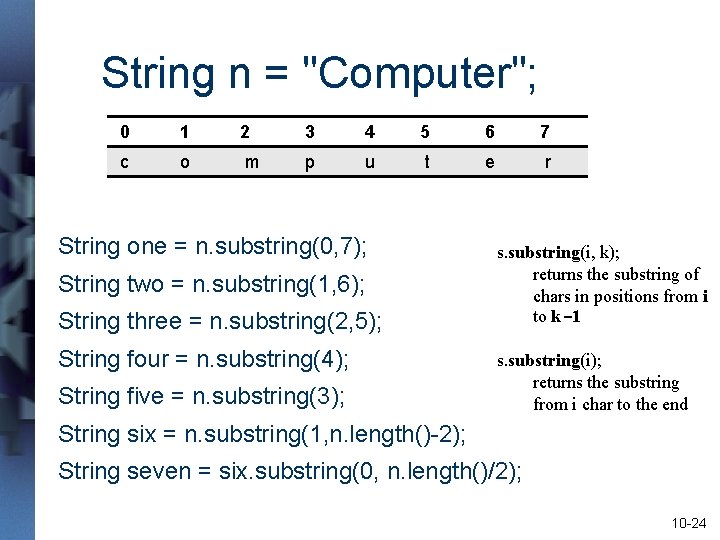
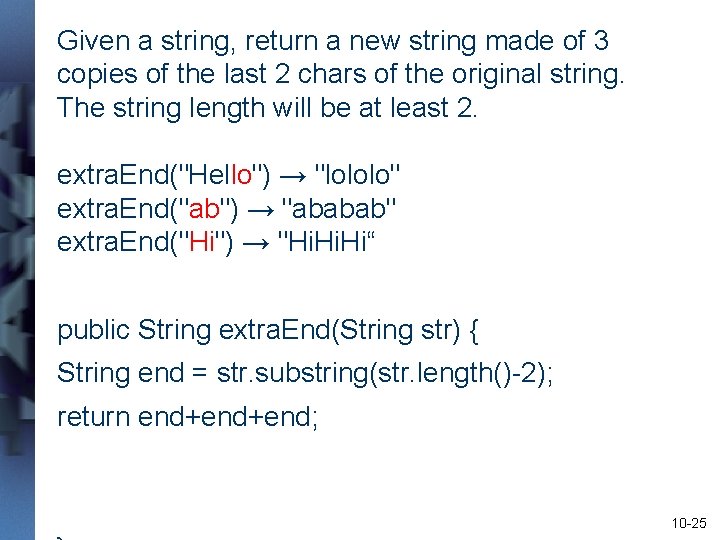
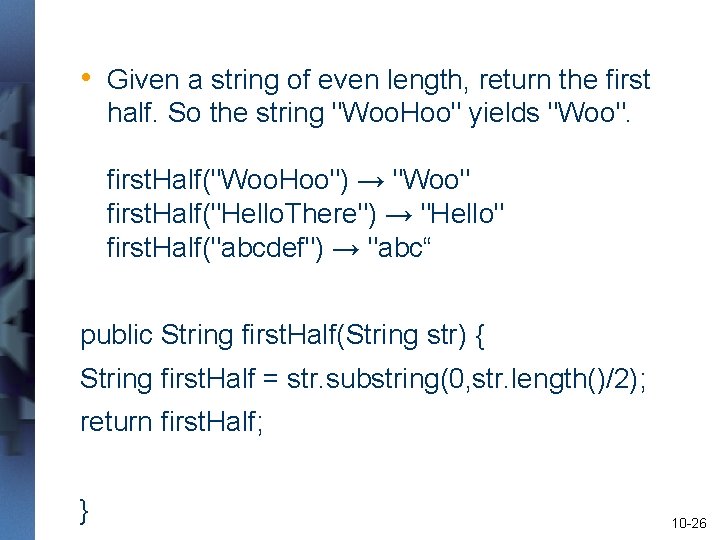
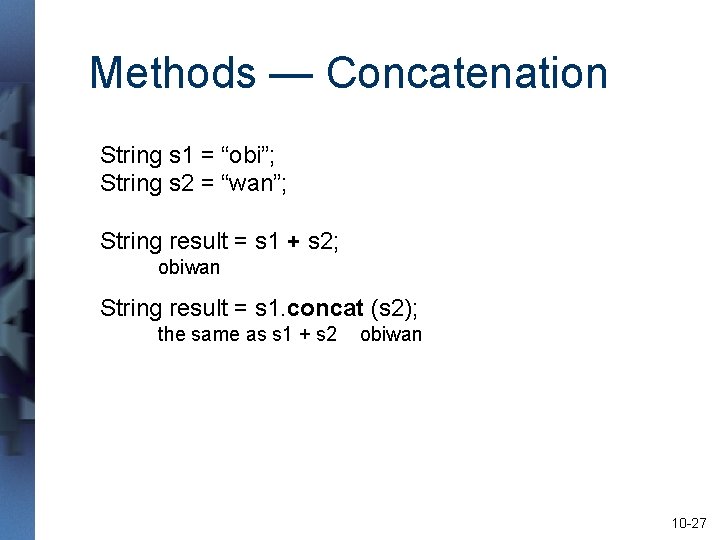
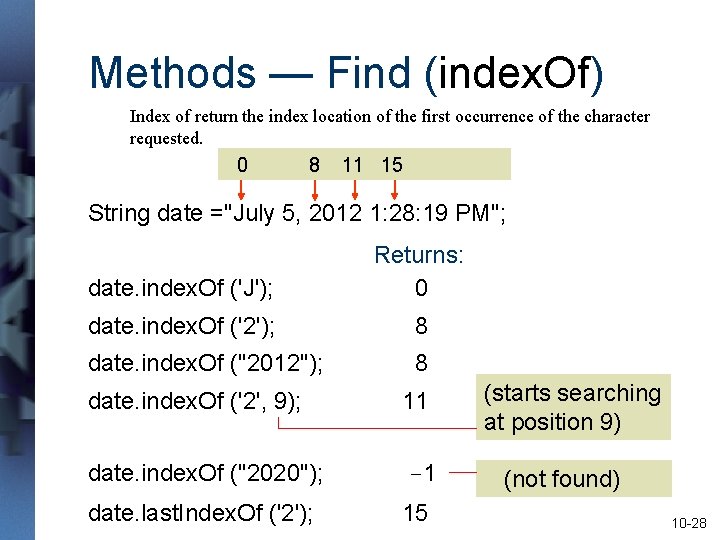
- Slides: 28
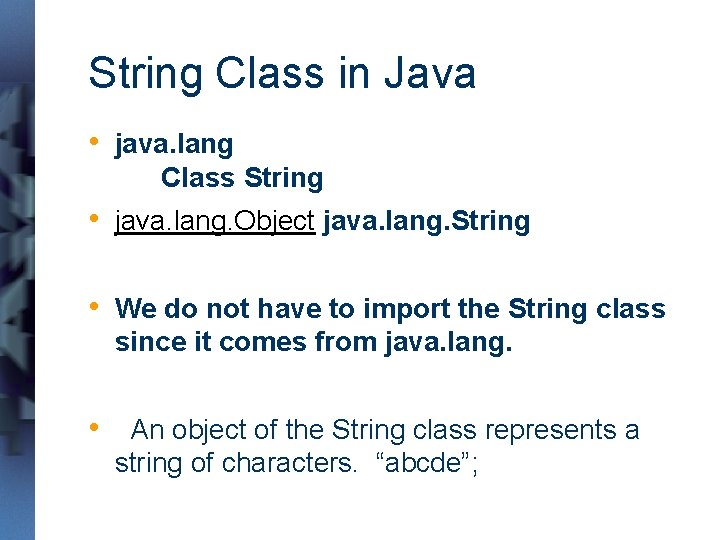
String Class in Java • java. lang Class String • java. lang. Object java. lang. String • We do not have to import the String class since it comes from java. lang. • An object of the String class represents a string of characters. “abcde”;
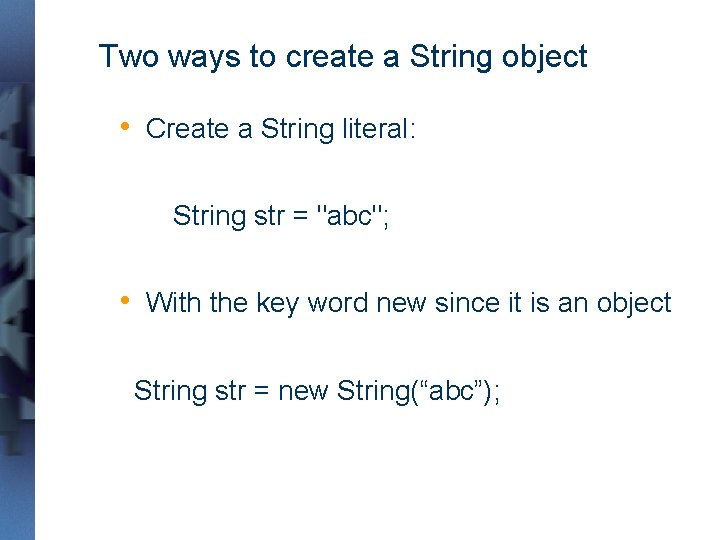
Two ways to create a String object • Create a String literal: String str = "abc"; • With the key word new since it is an object String str = new String(“abc”);
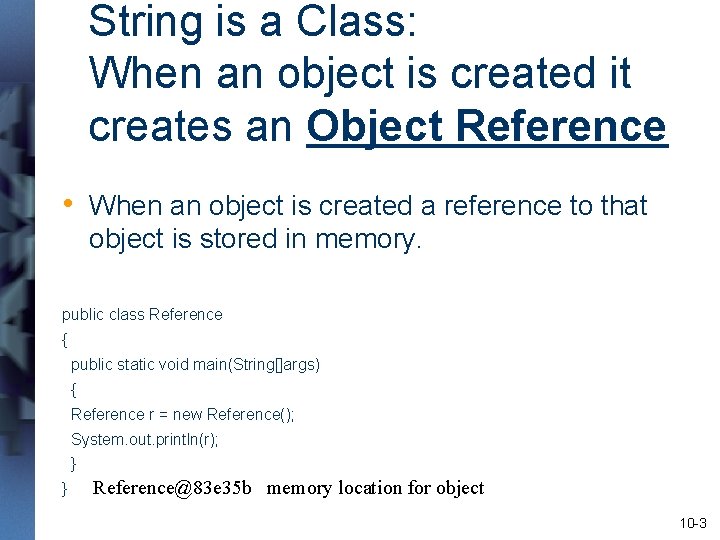
String is a Class: When an object is created it creates an Object Reference • When an object is created a reference to that object is stored in memory. public class Reference { public static void main(String[]args) { Reference r = new Reference(); System. out. println(r); } } Reference@83 e 35 b memory location for object 10 -3
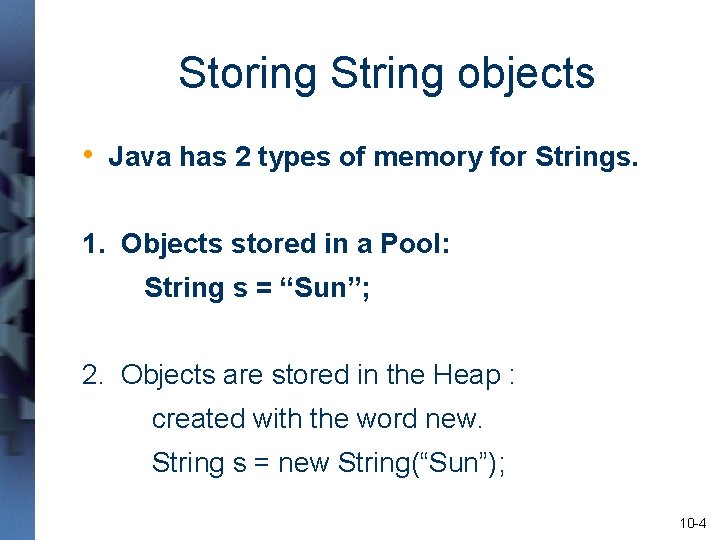
Storing String objects • Java has 2 types of memory for Strings. 1. Objects stored in a Pool: String s = “Sun”; 2. Objects are stored in the Heap : created with the word new. String s = new String(“Sun”); 10 -4
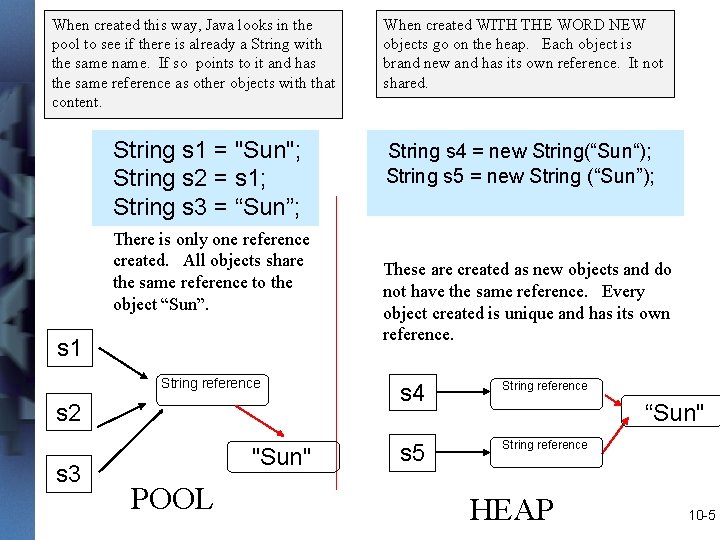
When created this way, Java looks in the pool to see if there is already a String with the same name. If so points to it and has the same reference as other objects with that content. String s 1 = "Sun"; String s 2 = s 1; String s 3 = “Sun”; There is only one reference created. All objects share the same reference to the object “Sun”. s 1 String reference s 2 s 3 "Sun" POOL When created WITH THE WORD NEW objects go on the heap. Each object is brand new and has its own reference. It not shared. String s 4 = new String(“Sun“); String s 5 = new String (“Sun”); These are created as new objects and do not have the same reference. Every object created is unique and has its own reference. s 4 String reference s 5 String reference “Sun" HEAP 10 -5
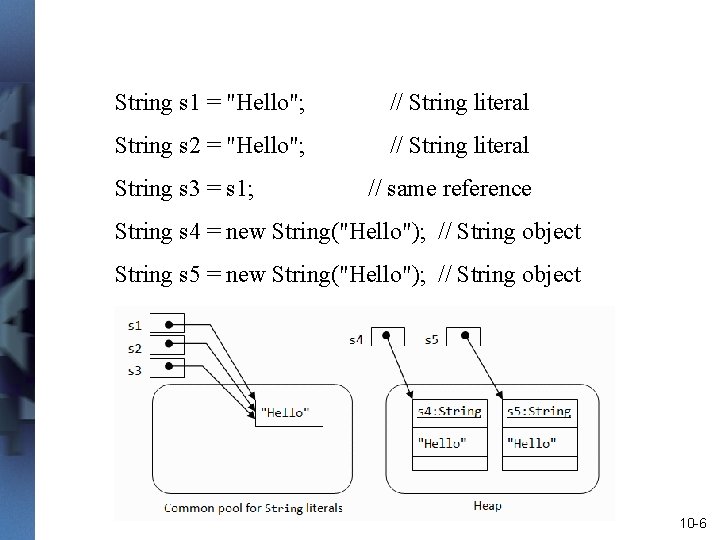
String s 1 = "Hello"; // String literal String s 2 = "Hello"; // String literal String s 3 = s 1; // same reference String s 4 = new String("Hello"); // String object String s 5 = new String("Hello"); // String object 10 -6
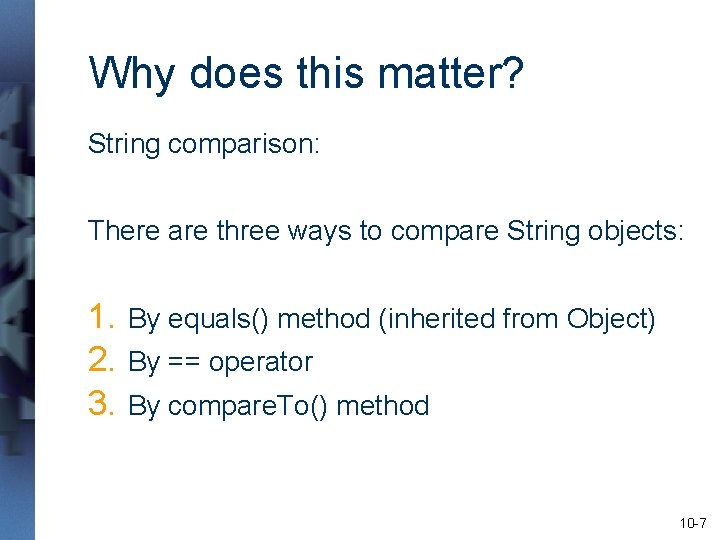
Why does this matter? String comparison: There are three ways to compare String objects: 1. By equals() method (inherited from Object) 2. By == operator 3. By compare. To() method 10 -7
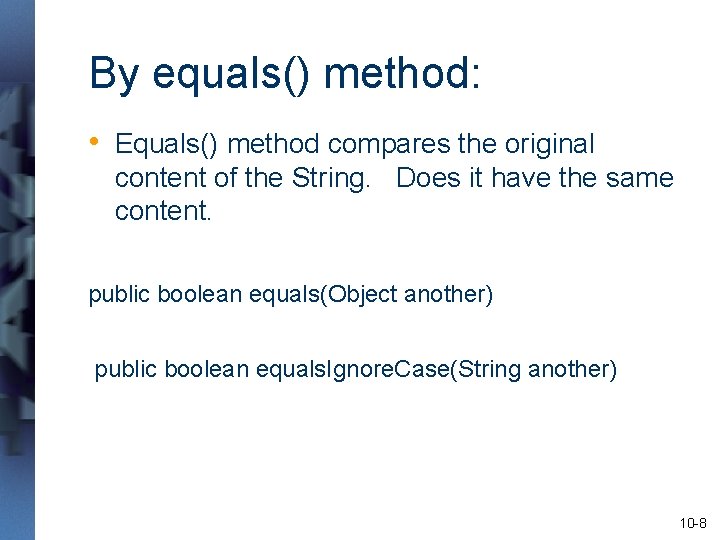
By equals() method: • Equals() method compares the original content of the String. Does it have the same content. public boolean equals(Object another) public boolean equals. Ignore. Case(String another) 10 -8
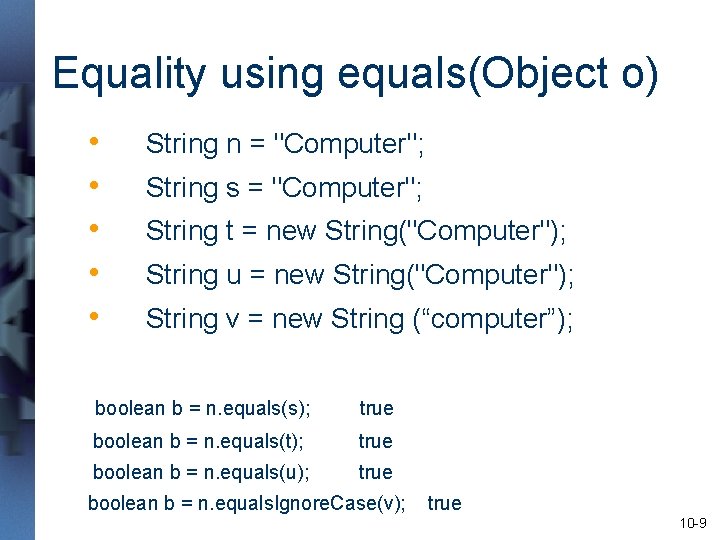
Equality using equals(Object o) • • • String n = "Computer"; String s = "Computer"; String t = new String("Computer"); String u = new String("Computer"); String v = new String (“computer”); boolean b = n. equals(s); true boolean b = n. equals(t); true boolean b = n. equals(u); true boolean b = n. equals. Ignore. Case(v); true 10 -9
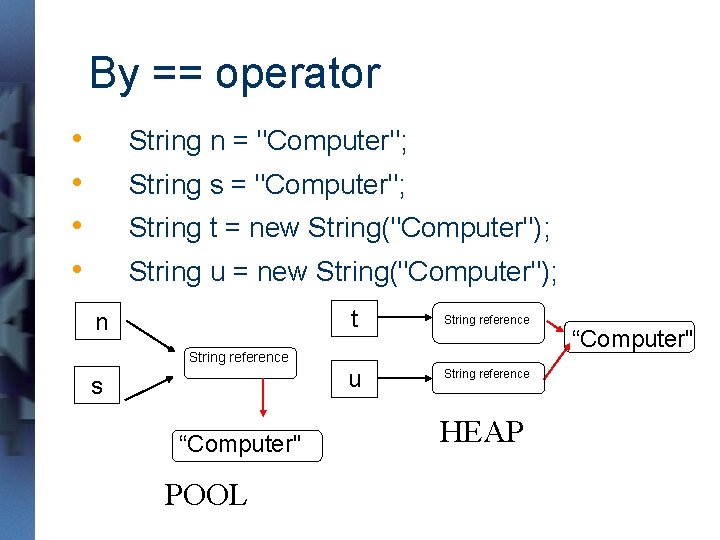
By == operator • • String n = "Computer"; String s = "Computer"; String t = new String("Computer"); String u = new String("Computer"); n t String reference u String reference s “Computer" POOL HEAP “Computer"
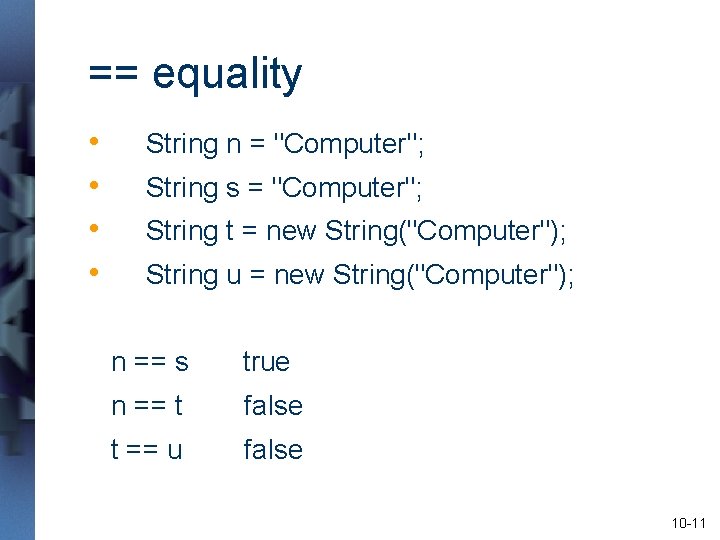
== equality • • String n = "Computer"; String s = "Computer"; String t = new String("Computer"); String u = new String("Computer"); n == s true n == t false t == u false 10 -11
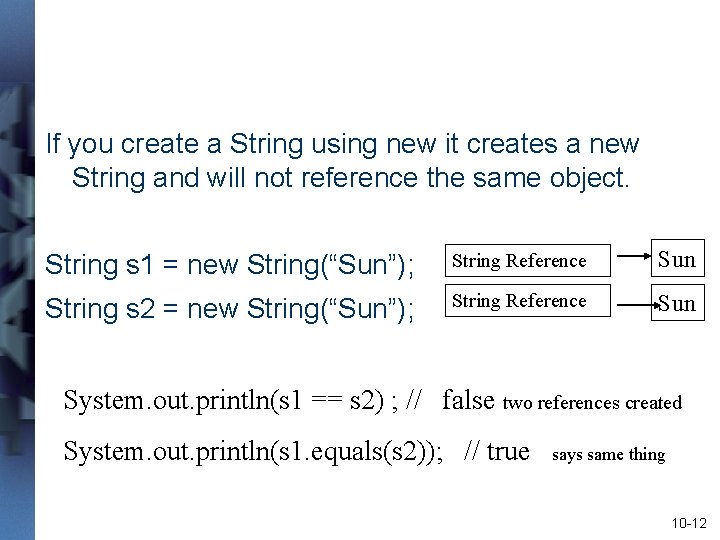
If you create a String using new it creates a new String and will not reference the same object. String s 1 = new String(“Sun”); String Reference Sun String s 2 = new String(“Sun”); String Reference Sun System. out. println(s 1 == s 2) ; // false two references created System. out. println(s 1. equals(s 2)); // true says same thing 10 -12
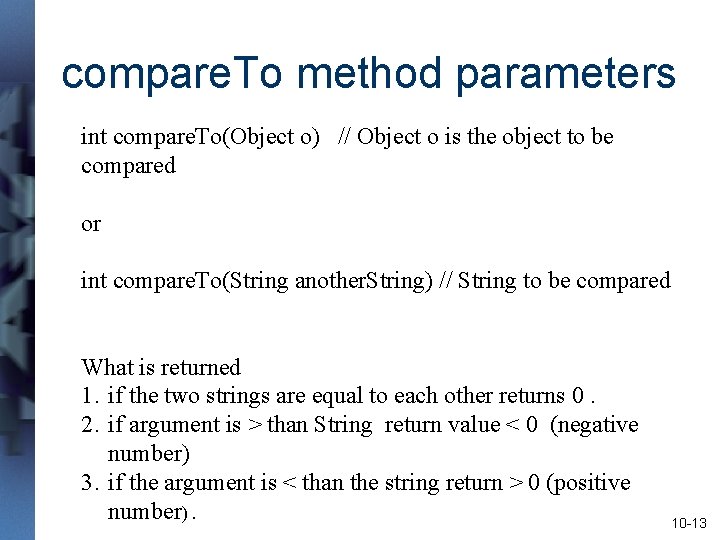
compare. To method parameters int compare. To(Object o) // Object o is the object to be compared or int compare. To(String another. String) // String to be compared What is returned 1. if the two strings are equal to each other returns 0. 2. if argument is > than String return value < 0 (negative number) 3. if the argument is < than the string return > 0 (positive number). 10 -13
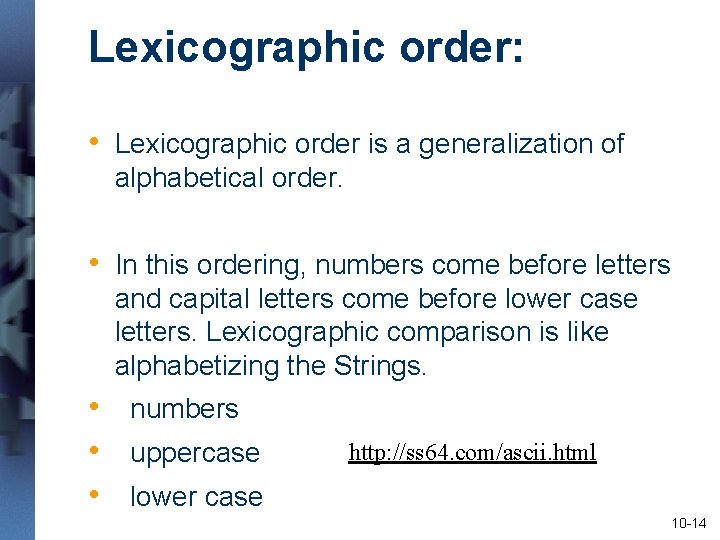
Lexicographic order: • Lexicographic order is a generalization of alphabetical order. • In this ordering, numbers come before letters and capital letters come before lower case letters. Lexicographic comparison is like alphabetizing the Strings. • numbers • uppercase • lower case http: //ss 64. com/ascii. html 10 -14
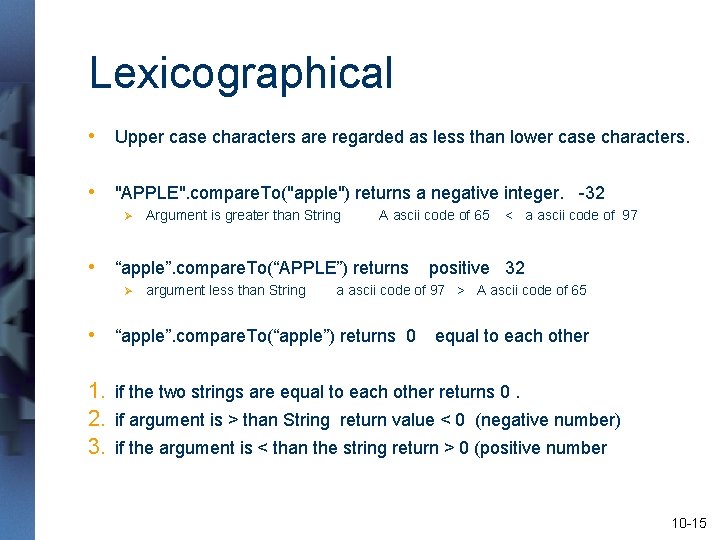
Lexicographical • Upper case characters are regarded as less than lower case characters. • "APPLE". compare. To("apple") returns a negative integer. -32 Ø Argument is greater than String A ascii code of 65 < a ascii code of 97 • “apple”. compare. To(“APPLE”) returns positive 32 Ø argument less than String a ascii code of 97 > A ascii code of 65 • “apple”. compare. To(“apple”) returns 0 equal to each other 1. if the two strings are equal to each other returns 0. 2. if argument is > than String return value < 0 (negative number) 3. if the argument is < than the string return > 0 (positive number 10 -15
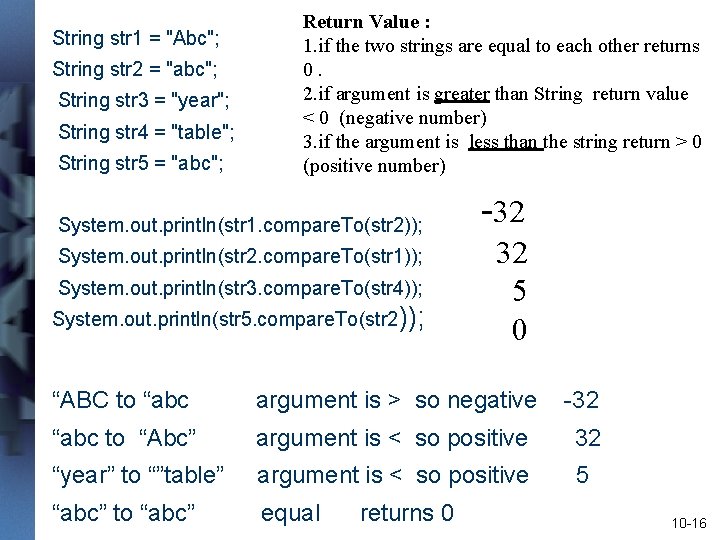
String str 1 = "Abc"; String str 2 = "abc"; String str 3 = "year"; String str 4 = "table"; String str 5 = "abc"; Return Value : 1. if the two strings are equal to each other returns 0. 2. if argument is greater than String return value < 0 (negative number) 3. if the argument is less than the string return > 0 (positive number) System. out. println(str 1. compare. To(str 2)); System. out. println(str 2. compare. To(str 1)); System. out. println(str 3. compare. To(str 4)); System. out. println(str 5. compare. To(str 2)); -32 32 5 0 “ABC to “abc argument is > so negative -32 “abc to “Abc” argument is < so positive 32 “year” to “”table” argument is < so positive 5 “abc” to “abc” equal returns 0 10 -16
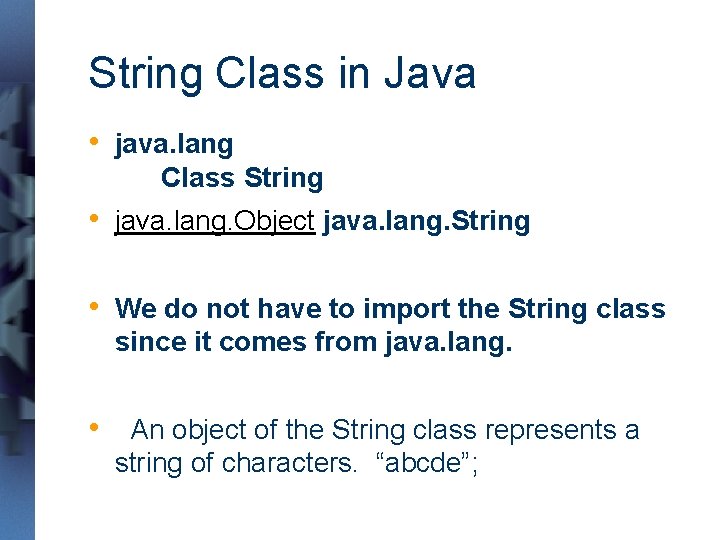
String Class in Java • java. lang Class String • java. lang. Object java. lang. String • We do not have to import the String class since it comes from java. lang. • An object of the String class represents a string of characters. “abcde”;
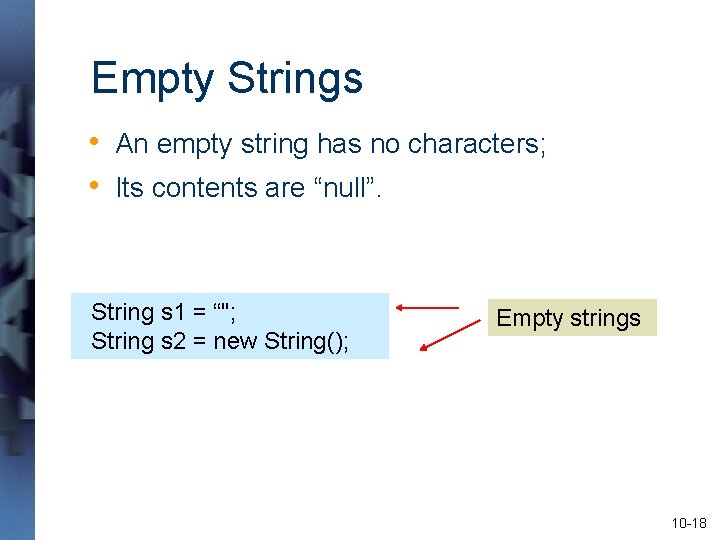
Empty Strings • An empty string has no characters; • Its contents are “null”. String s 1 = “"; String s 2 = new String(); Empty strings 10 -18
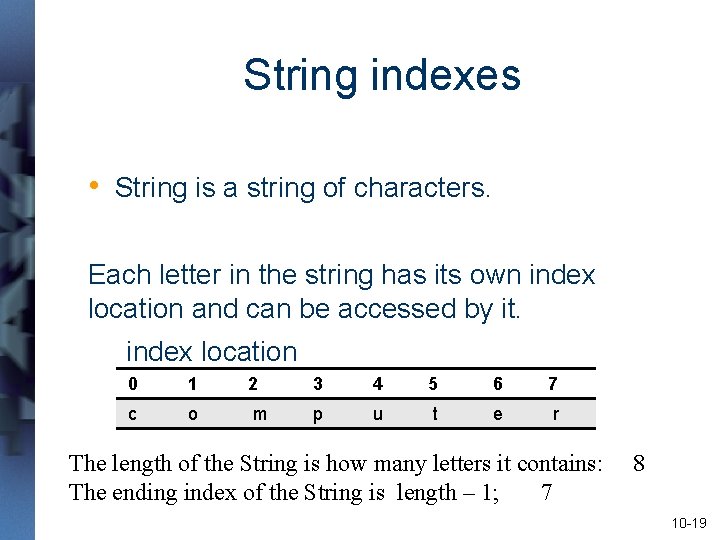
String indexes • String is a string of characters. Each letter in the string has its own index location and can be accessed by it. index location 0 1 2 3 4 5 6 7 c o m p u t e r The length of the String is how many letters it contains: The ending index of the String is length – 1; 7 8 10 -19
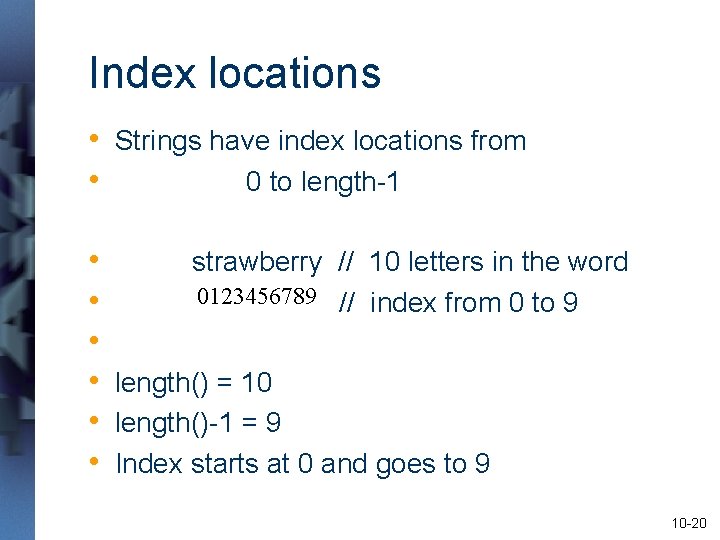
Index locations • Strings have index locations from • 0 to length-1 • strawberry // 10 letters in the word 0123456789 // index from 0 to 9 • • • length() = 10 • length()-1 = 9 • Index starts at 0 and goes to 9 10 -20
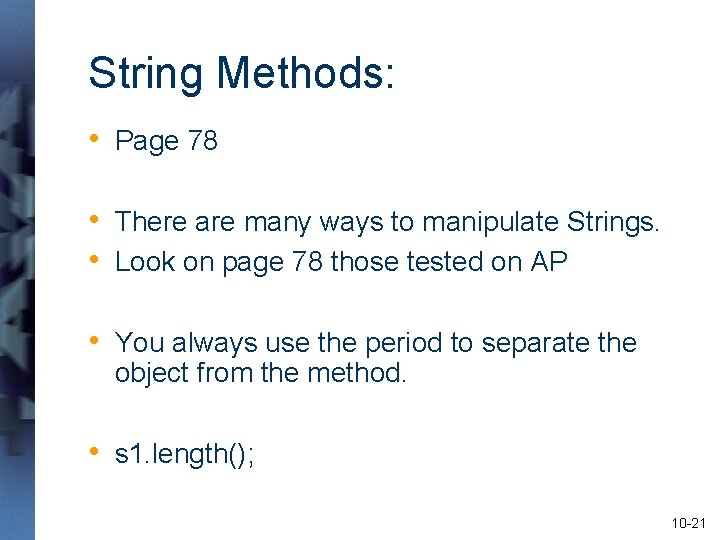
String Methods: • Page 78 • There are many ways to manipulate Strings. • Look on page 78 those tested on AP • You always use the period to separate the object from the method. • s 1. length(); 10 -21
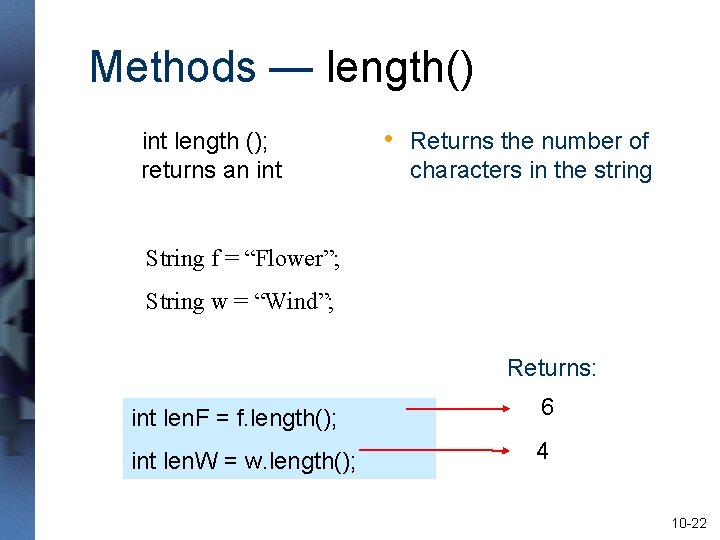
Methods — length() int length (); returns an int • Returns the number of characters in the string String f = “Flower”; String w = “Wind”; Returns: int len. F = f. length(); 6 int len. W = w. length(); 4 10 -22
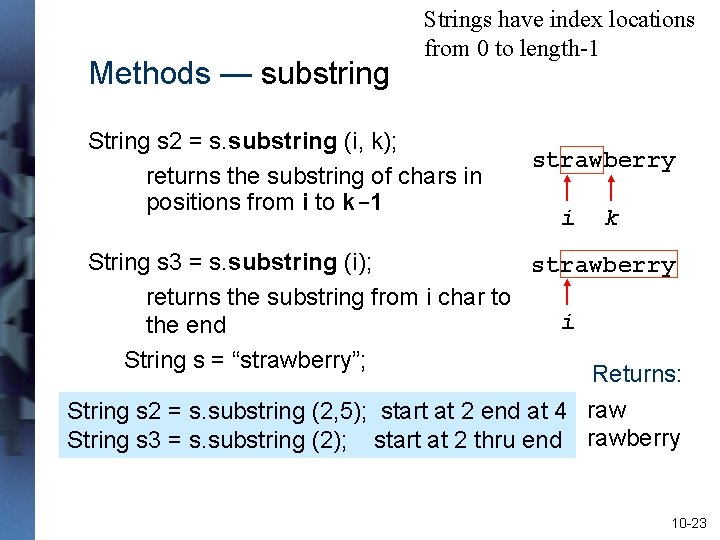
Methods — substring Strings have index locations from 0 to length-1 String s 2 = s. substring (i, k); returns the substring of chars in positions from i to k-1 strawberry i k String s 3 = s. substring (i); strawberry returns the substring from i char to i the end String s = “strawberry”; Returns: String s 2 = s. substring (2, 5); start at 2 end at 4 raw String s 3 = s. substring (2); start at 2 thru end rawberry 10 -23
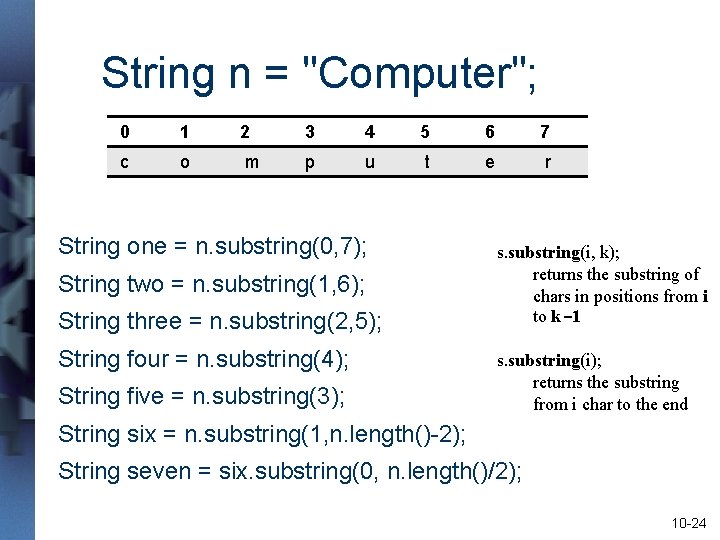
String n = "Computer"; 0 1 2 3 4 5 6 7 c o m p u t e r String one = n. substring(0, 7); String two = n. substring(1, 6); String three = n. substring(2, 5); String four = n. substring(4); String five = n. substring(3); s. substring(i, k); returns the substring of chars in positions from i to k-1 s. substring(i); returns the substring from i char to the end String six = n. substring(1, n. length()-2); String seven = six. substring(0, n. length()/2); 10 -24
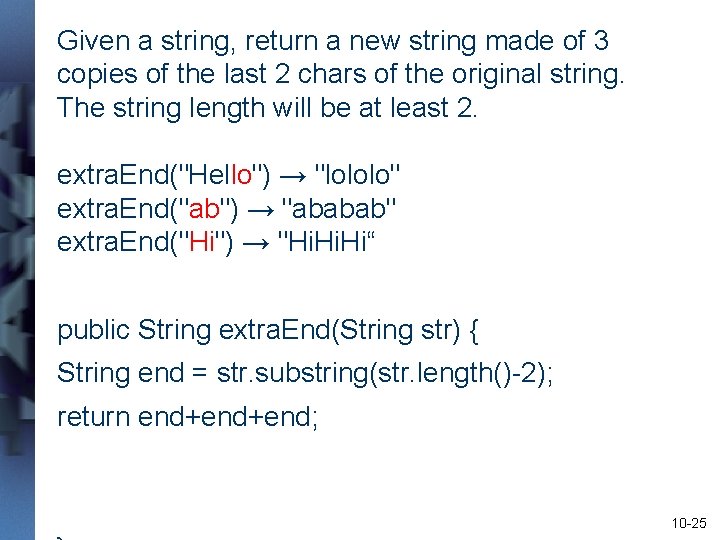
Given a string, return a new string made of 3 copies of the last 2 chars of the original string. The string length will be at least 2. extra. End("Hello") → "lololo" extra. End("ab") → "ababab" extra. End("Hi") → "Hi. Hi“ public String extra. End(String str) { String end = str. substring(str. length()-2); return end+end; 10 -25
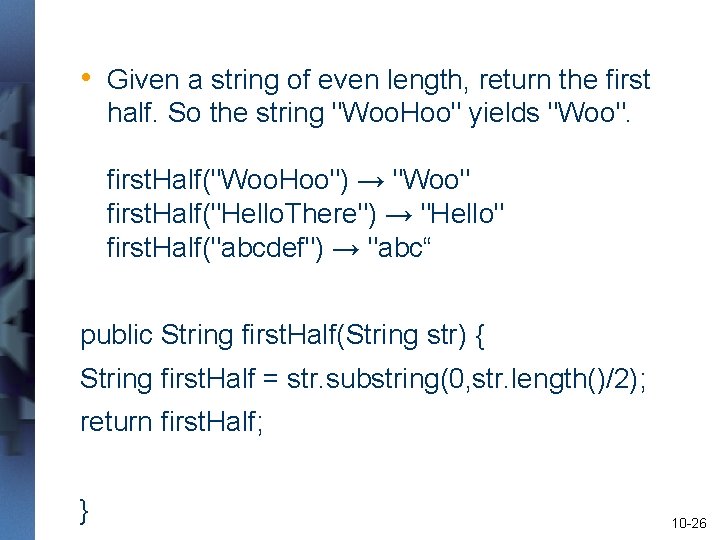
• Given a string of even length, return the first half. So the string "Woo. Hoo" yields "Woo". first. Half("Woo. Hoo") → "Woo" first. Half("Hello. There") → "Hello" first. Half("abcdef") → "abc“ public String first. Half(String str) { String first. Half = str. substring(0, str. length()/2); return first. Half; } 10 -26
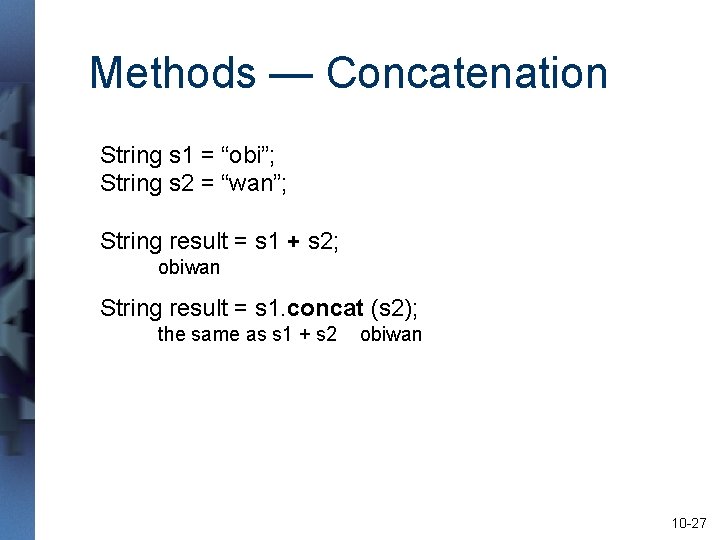
Methods — Concatenation String s 1 = “obi”; String s 2 = “wan”; String result = s 1 + s 2; obiwan String result = s 1. concat (s 2); the same as s 1 + s 2 obiwan 10 -27
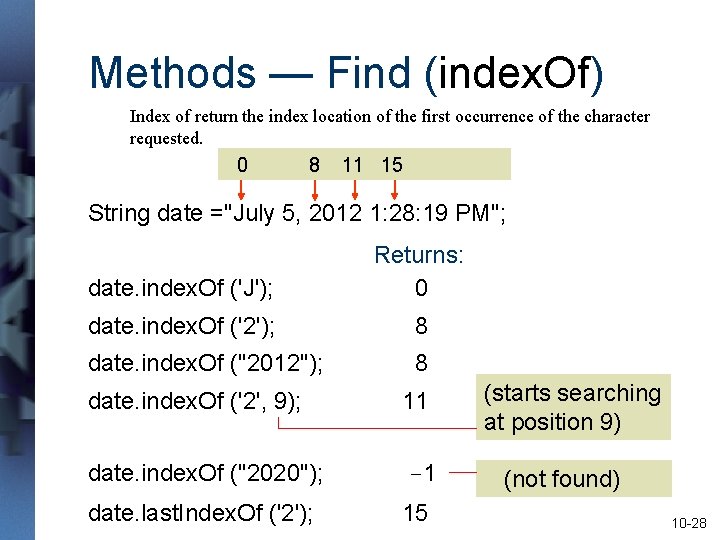
Methods — Find (index. Of) Index of return the index location of the first occurrence of the character requested. 0 8 11 15 String date ="July 5, 2012 1: 28: 19 PM"; date. index. Of ('J'); Returns: 0 date. index. Of ('2'); 8 date. index. Of ("2012"); 8 date. index. Of ('2', 9); 11 date. index. Of ("2020"); -1 date. last. Index. Of ('2'); 15 (starts searching at position 9) (not found) 10 -28
Http protocol description
Lexicographic order java
Java lang math class
Java string new string
Private.com
Java.lang.util
Import java.util.*
Java.lang package
Spliterator
Class maths student student1 class student string name
Const char *s=""
Java string manipulation
Java string operation
Private string java
String data type
Java string methods
String conversion
Java string methods
Unit 2
Strings in java
Klasa string java
Private string[]
Java string conditional statement
C++ string initialization
Find a string in java
Public class student
String constructor in java
Private string
Public class person private string name