The string data type String String in general
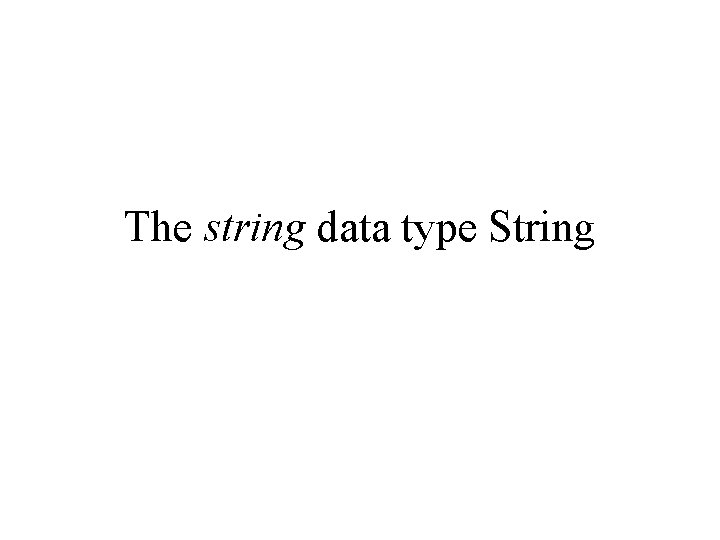
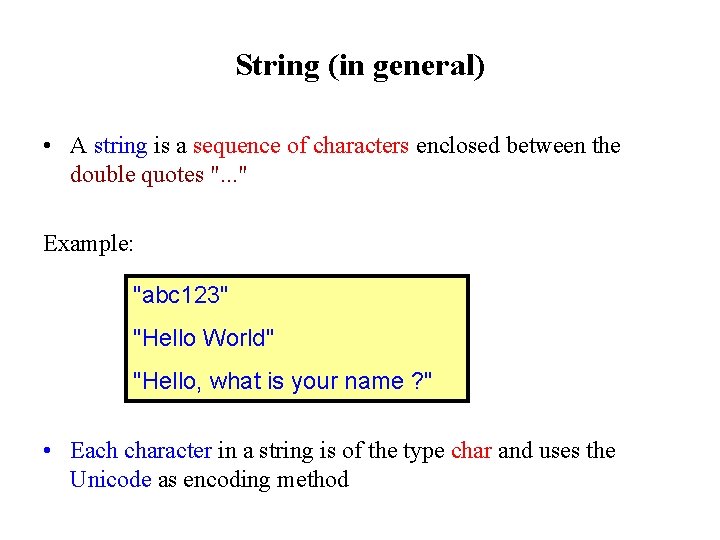
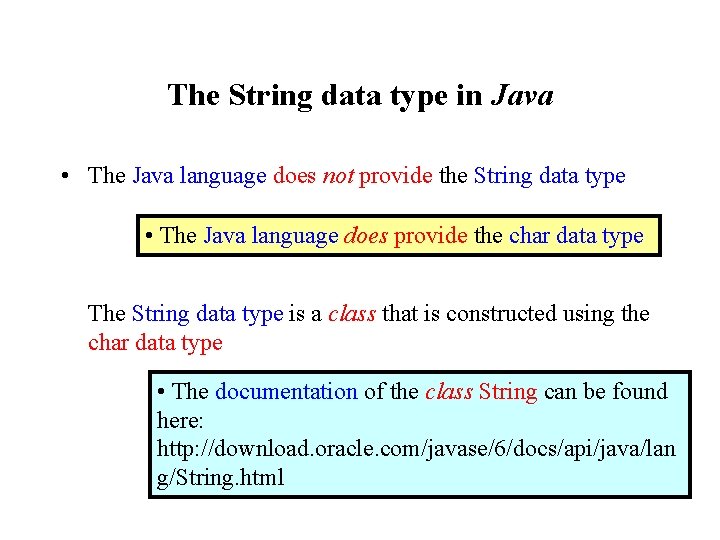
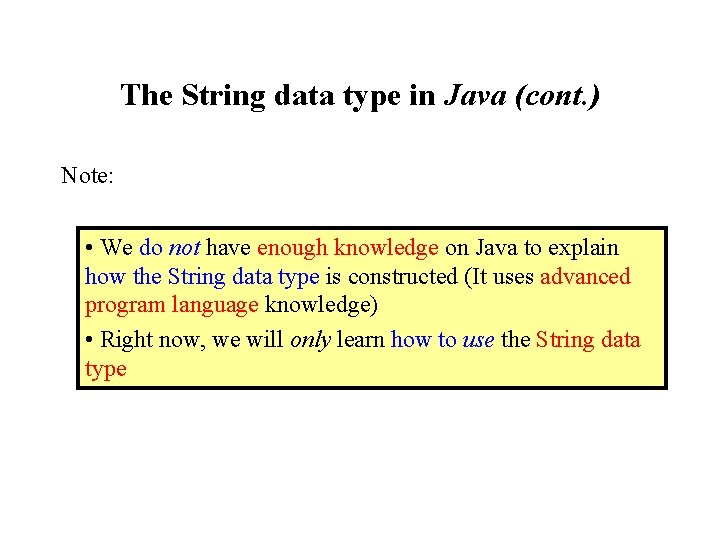
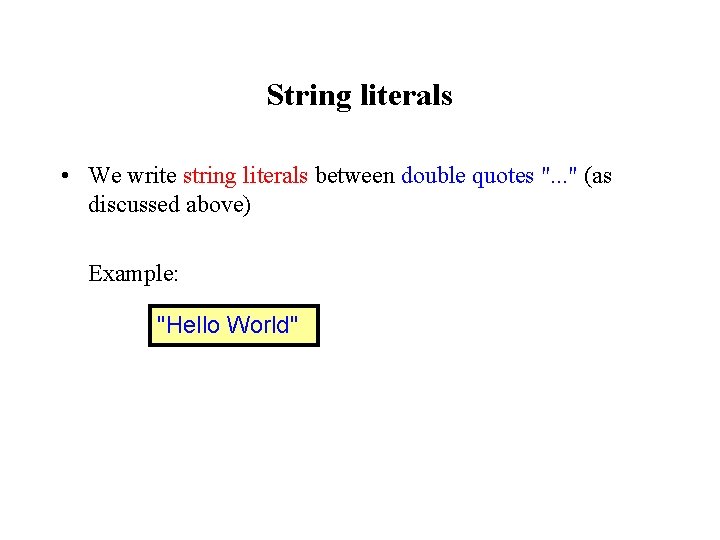
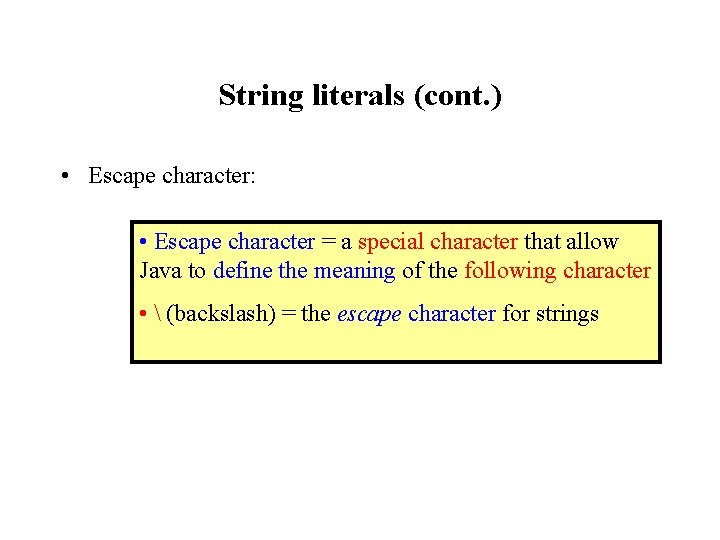
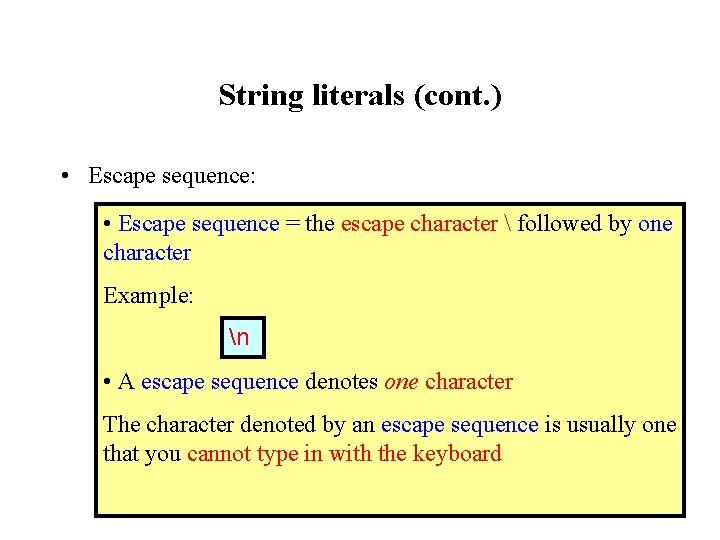
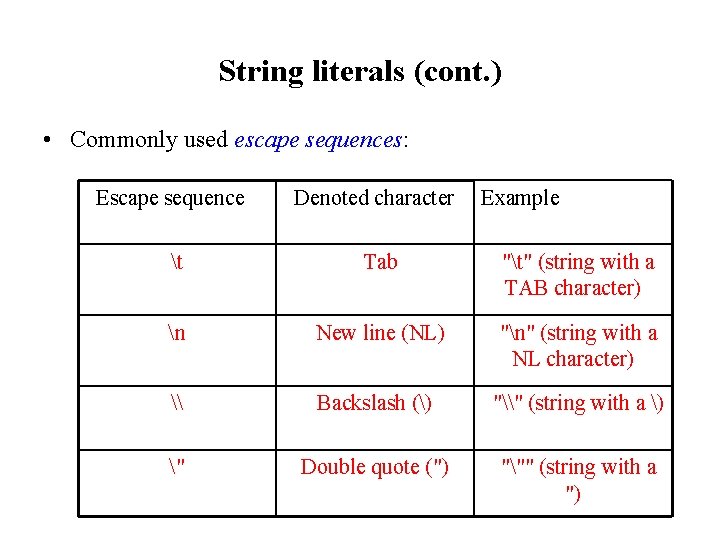
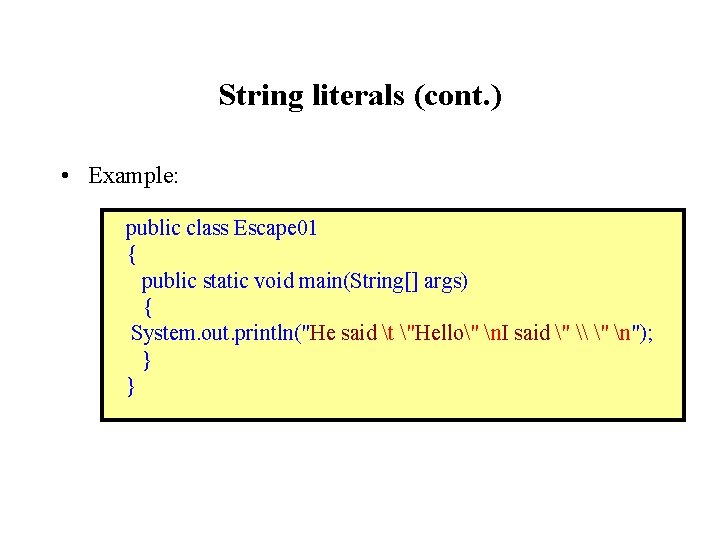
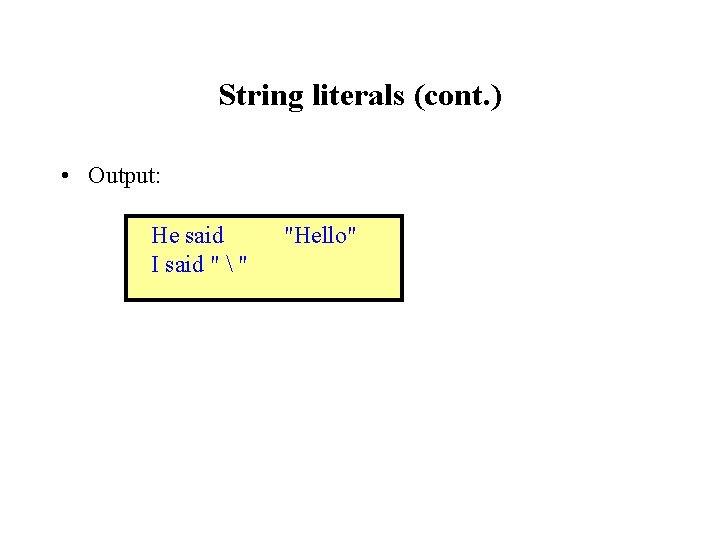
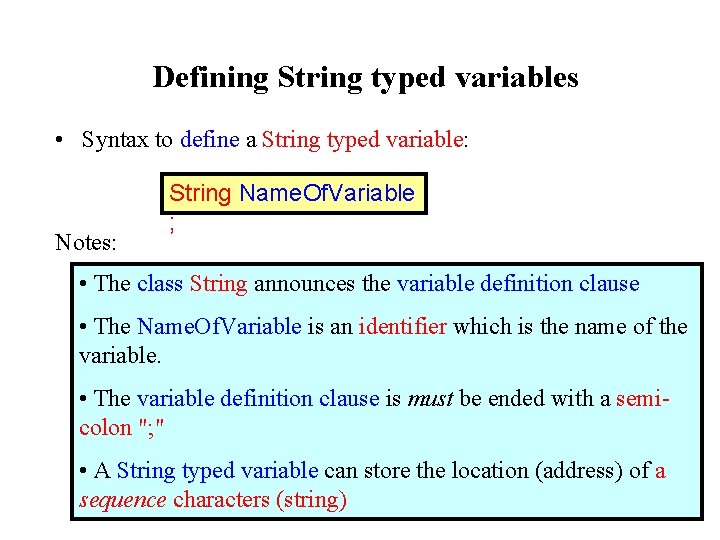
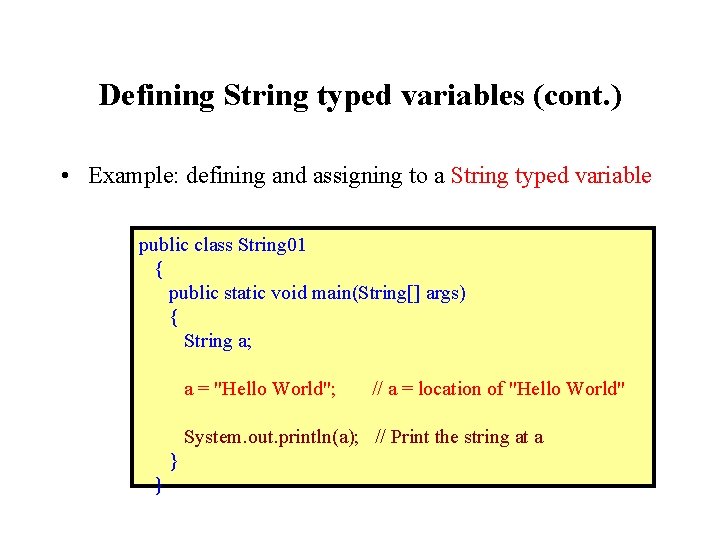
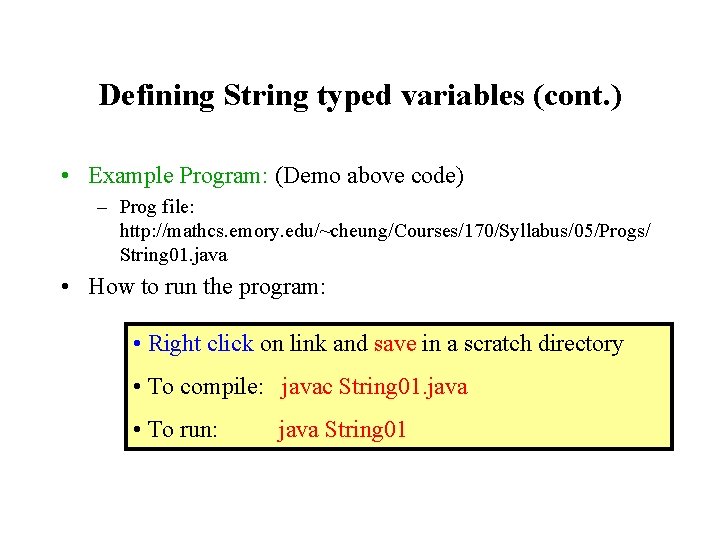
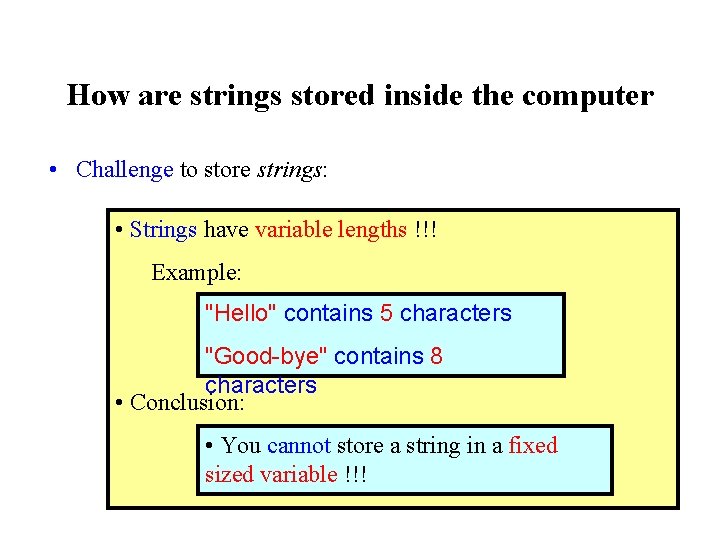
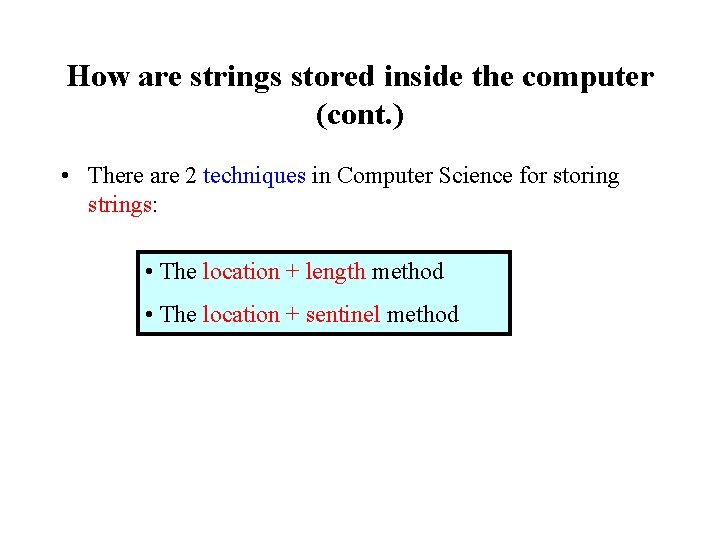
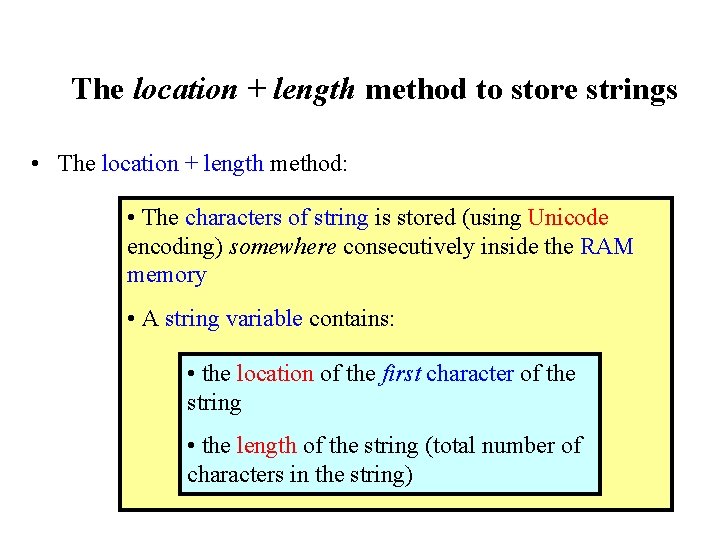
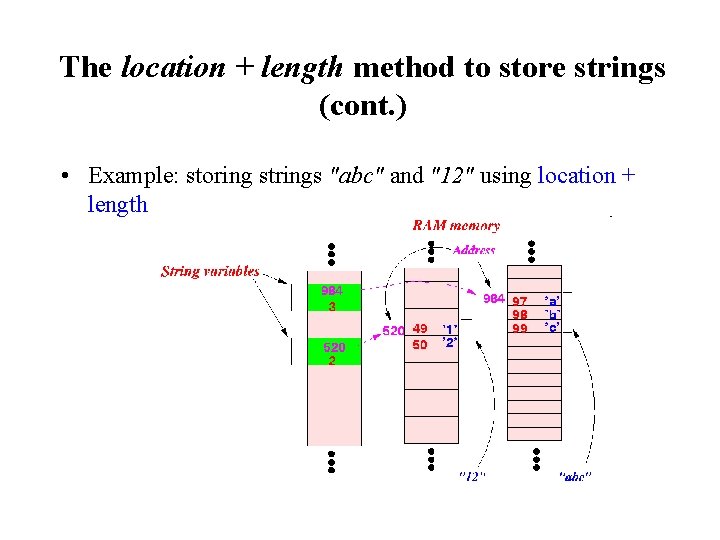
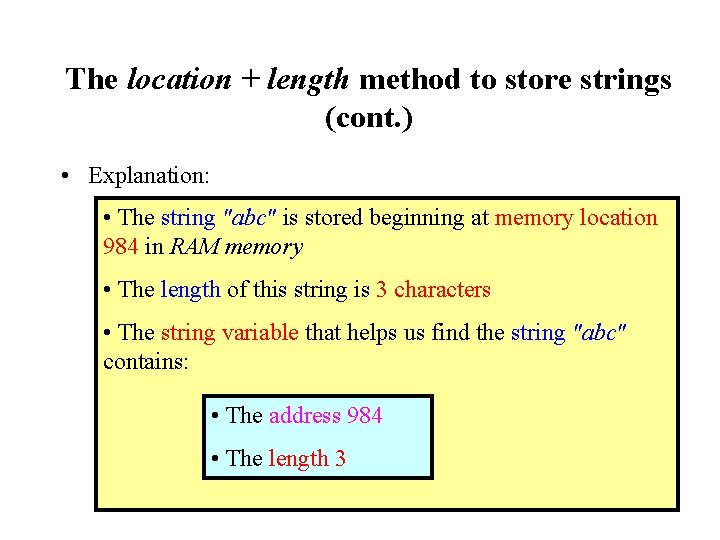
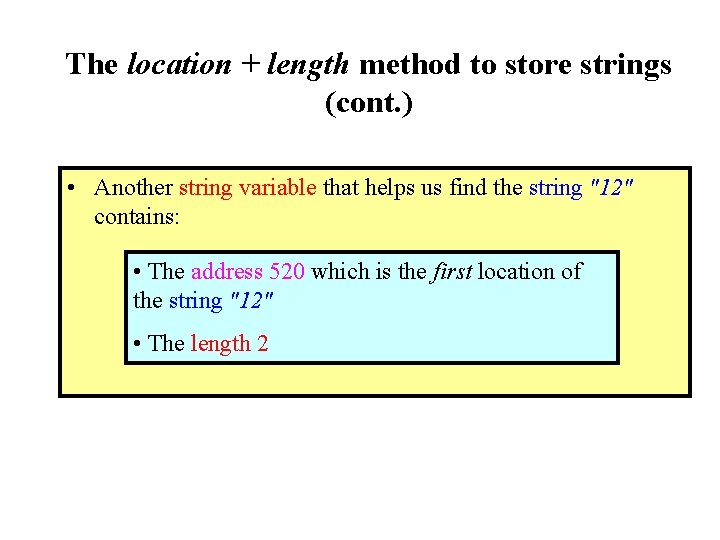
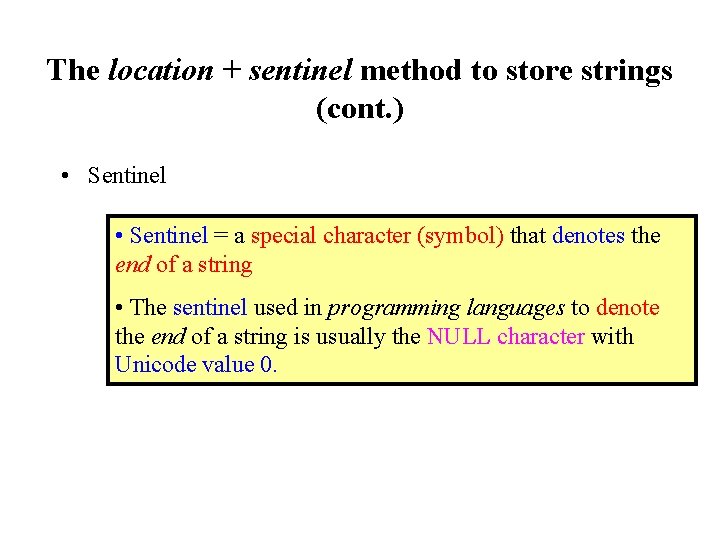
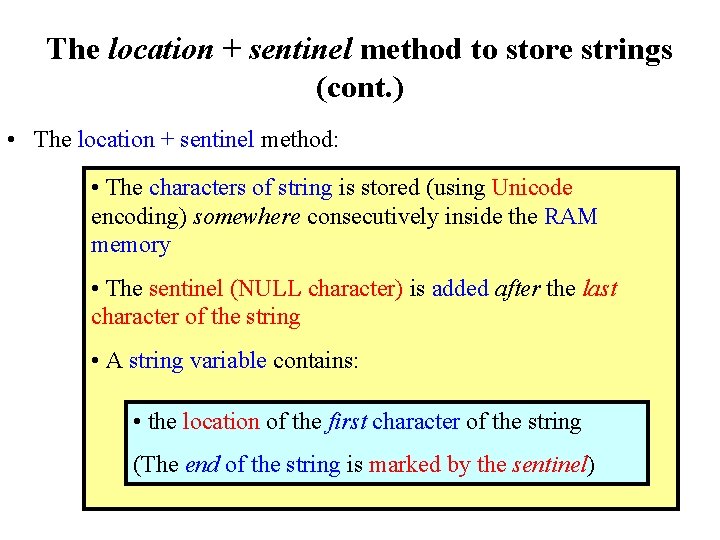
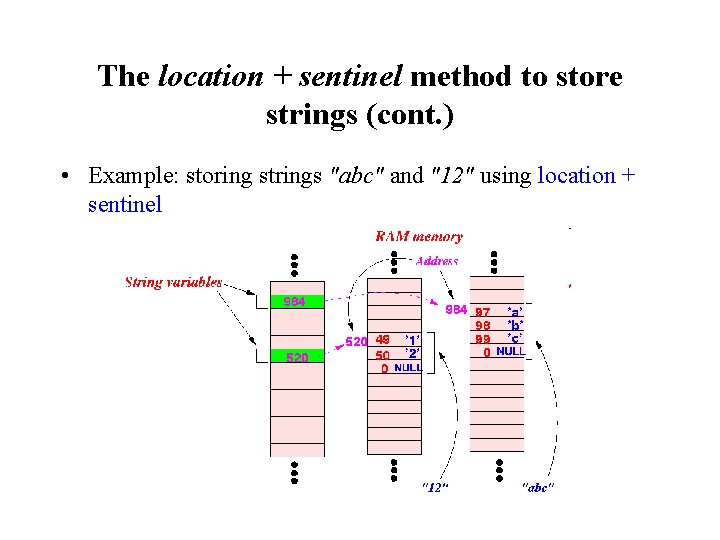
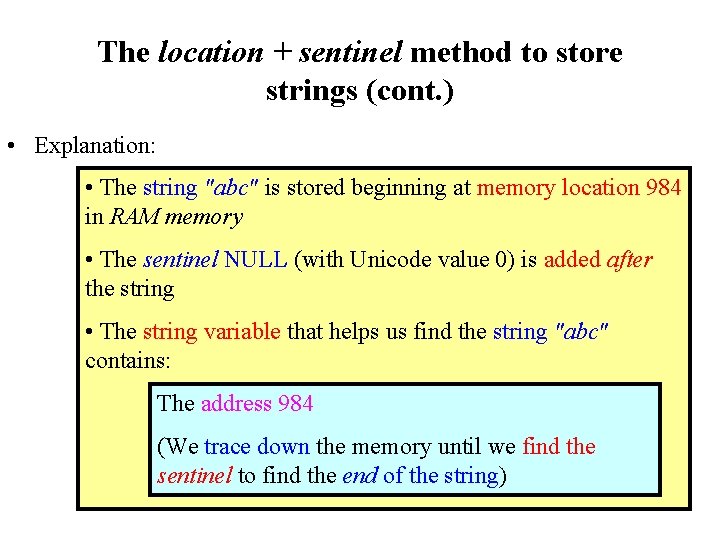
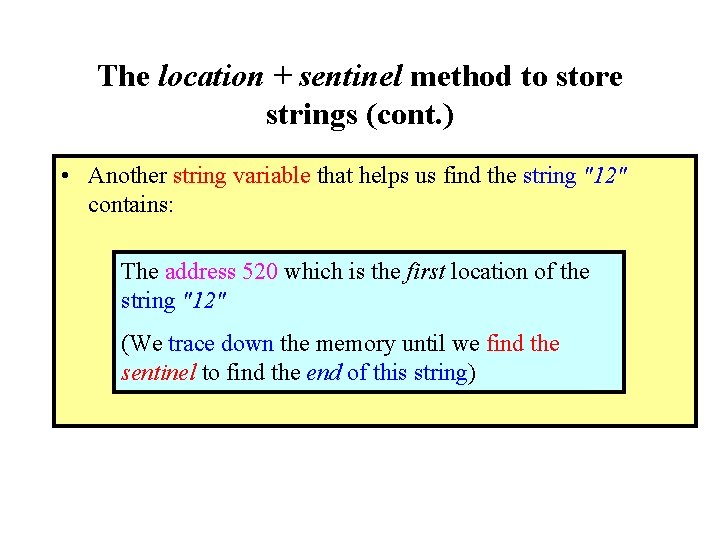
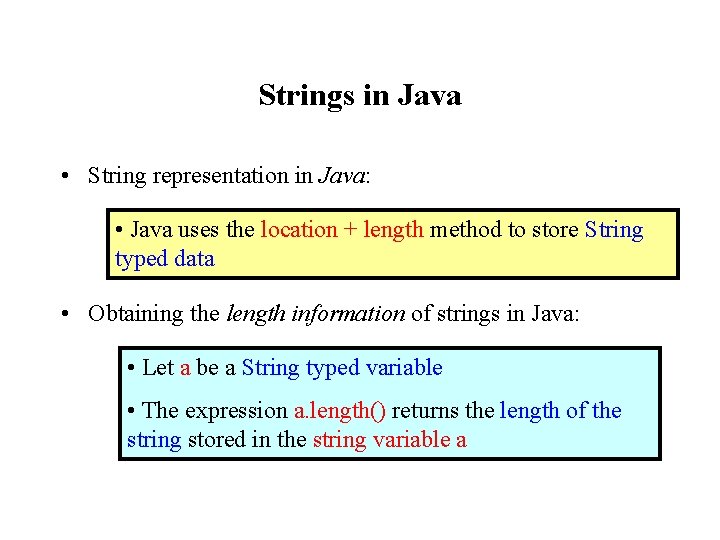
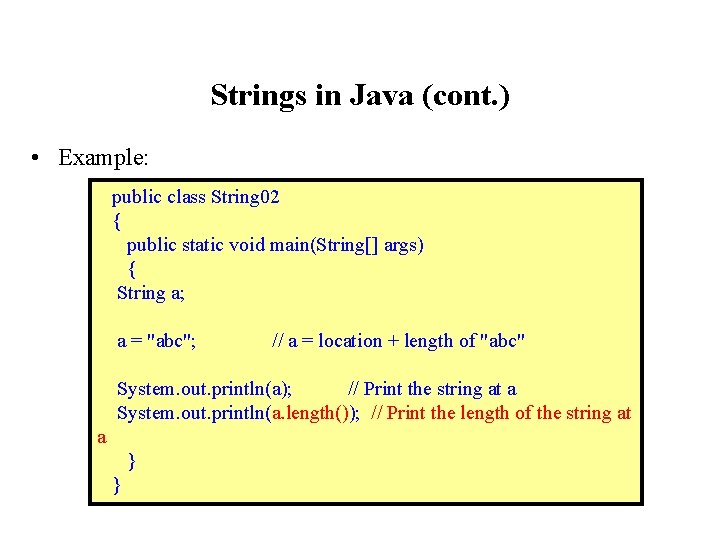
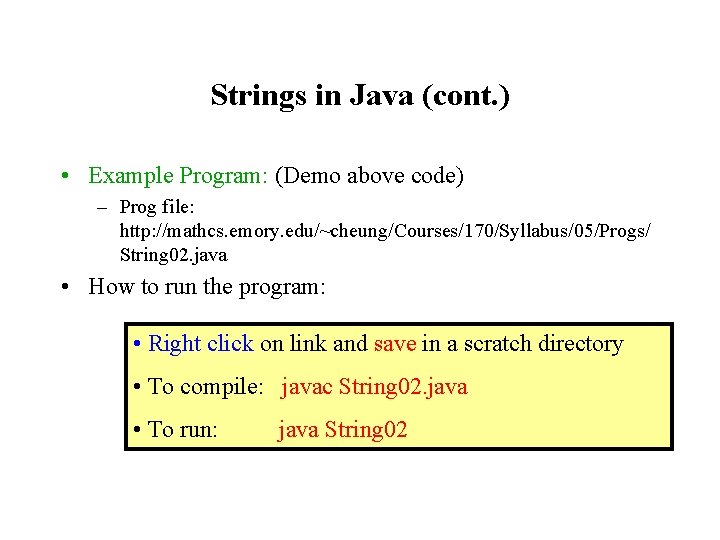
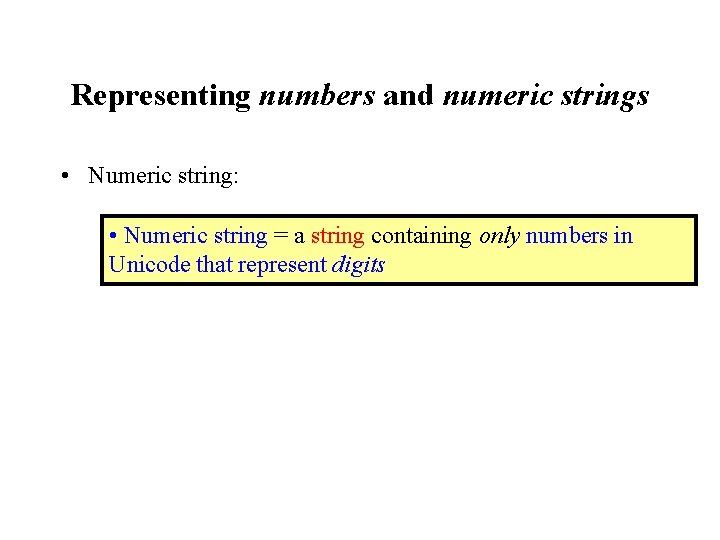
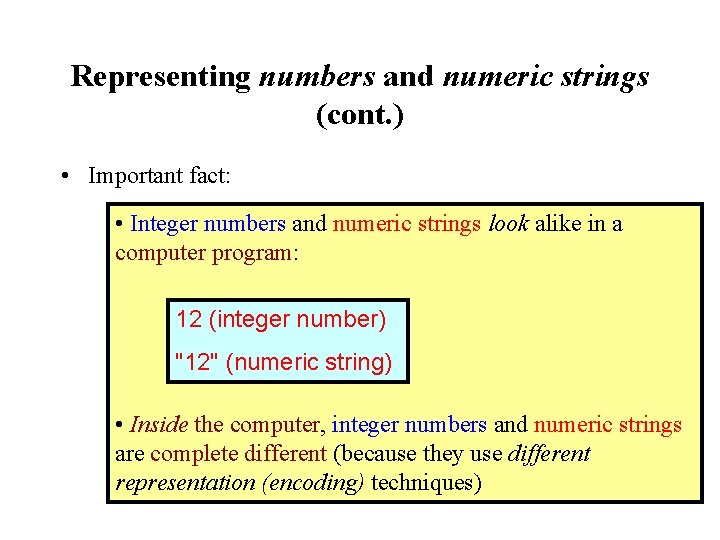
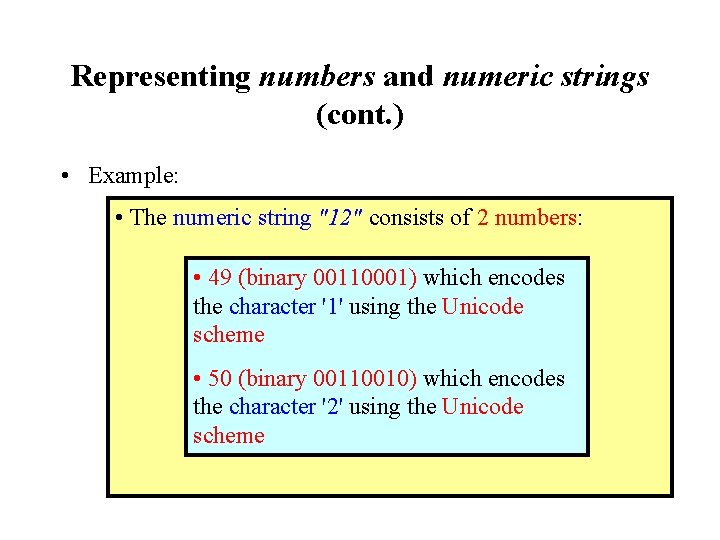
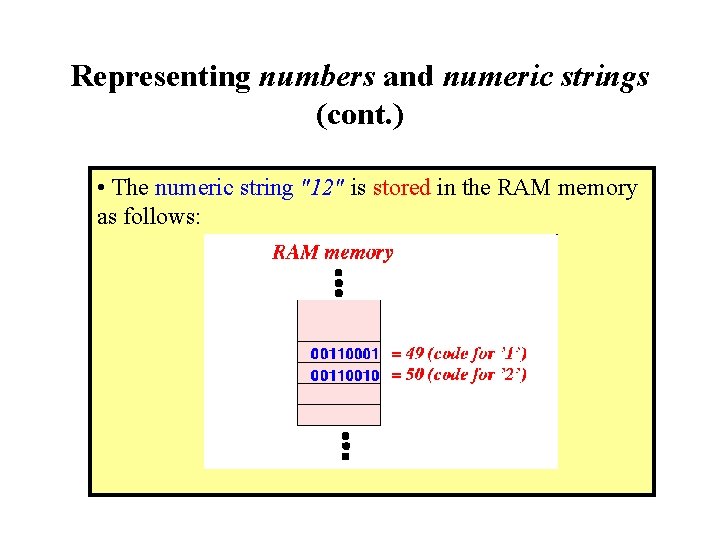
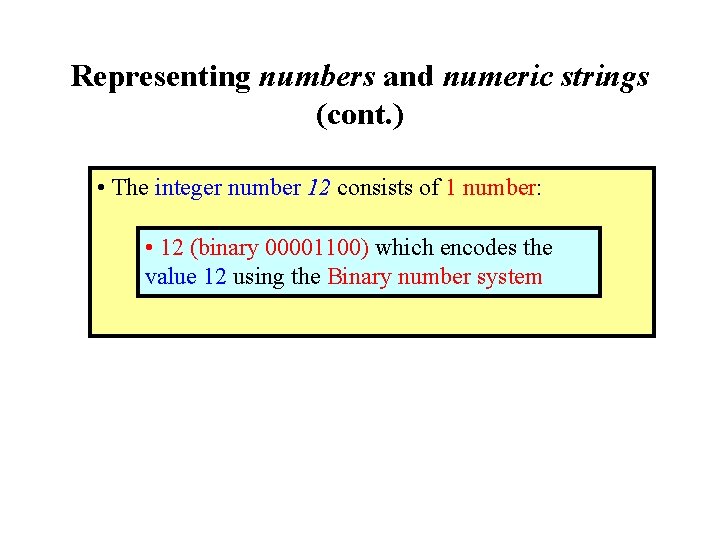
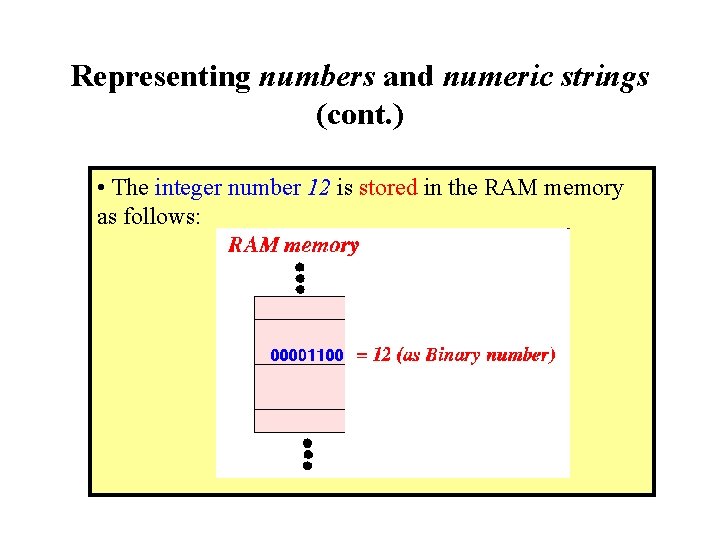
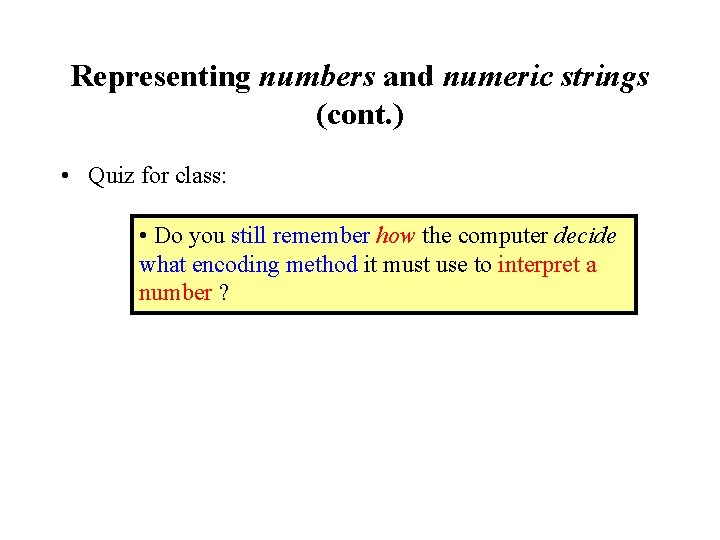
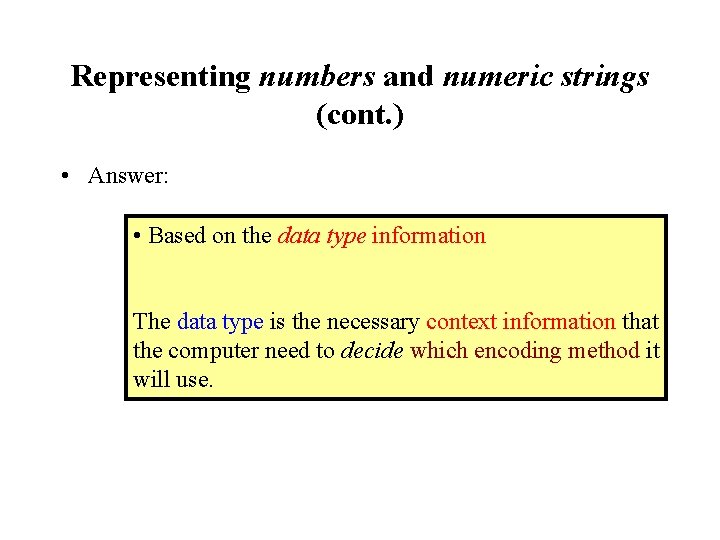
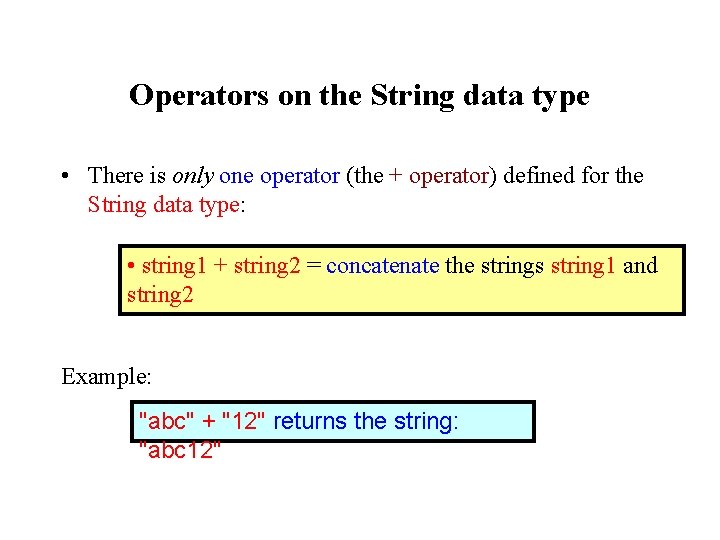
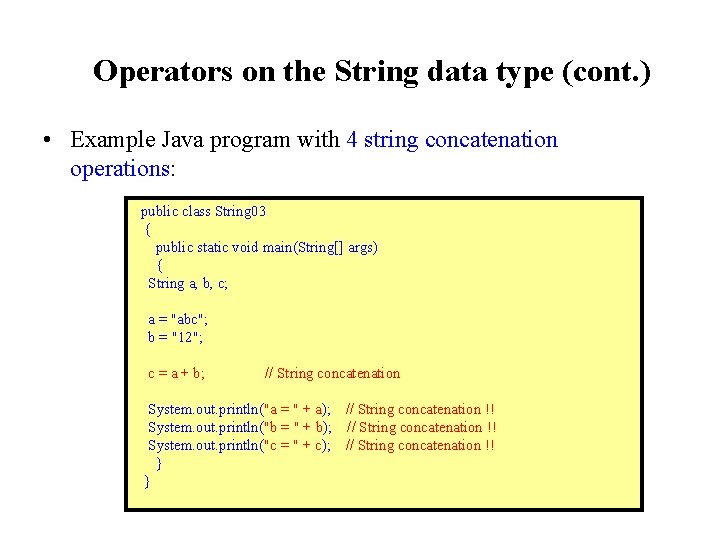
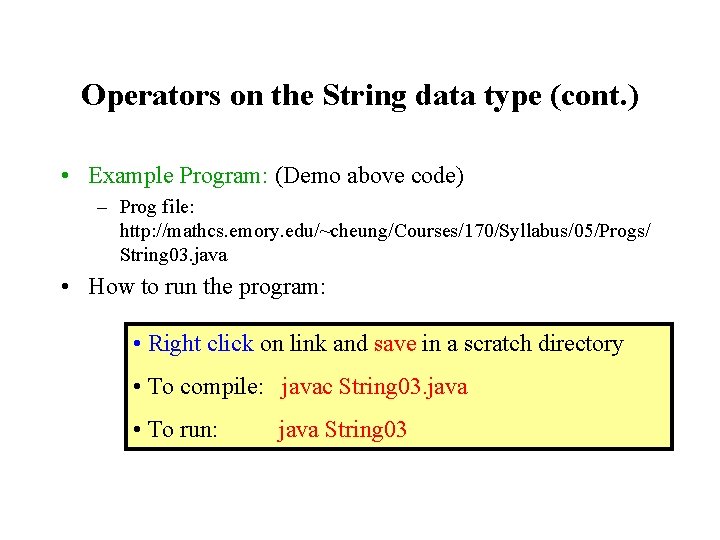
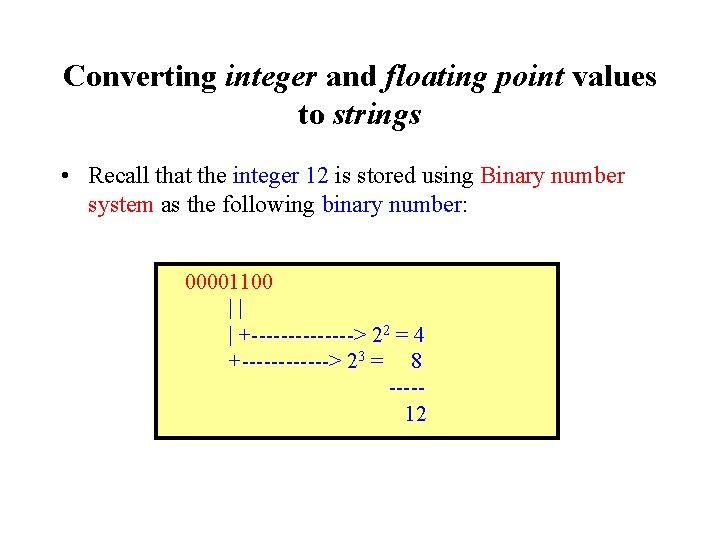
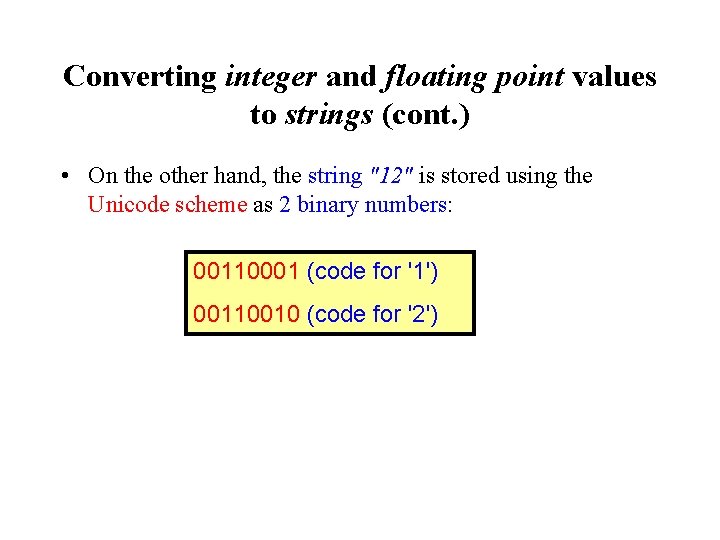
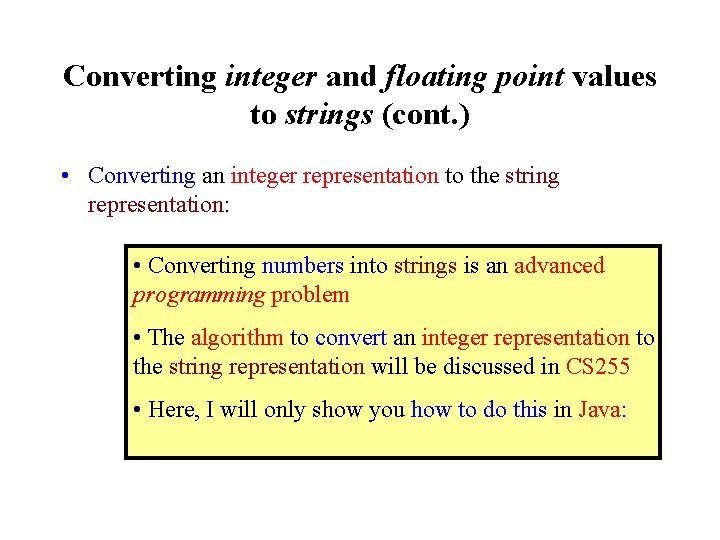
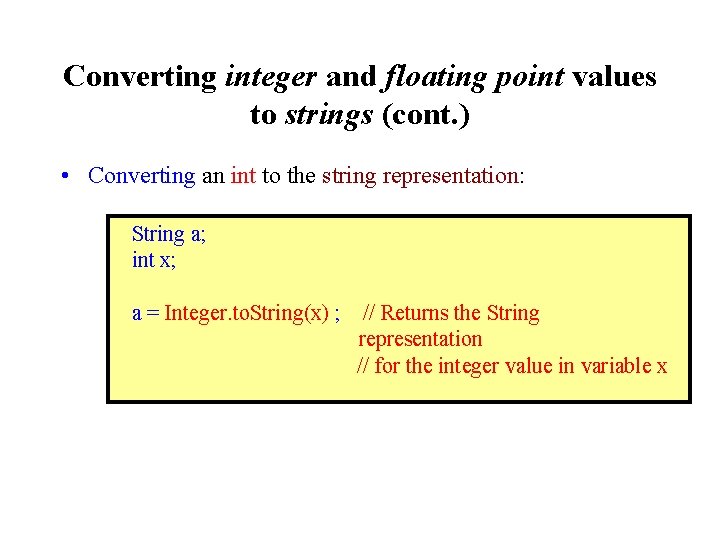
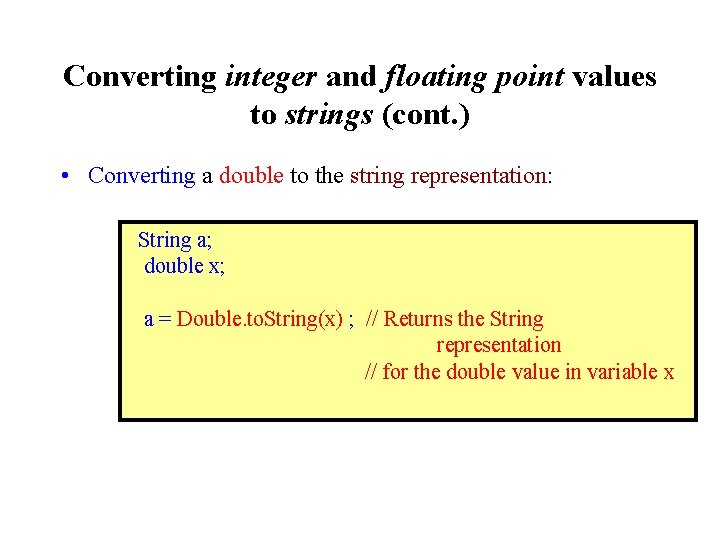
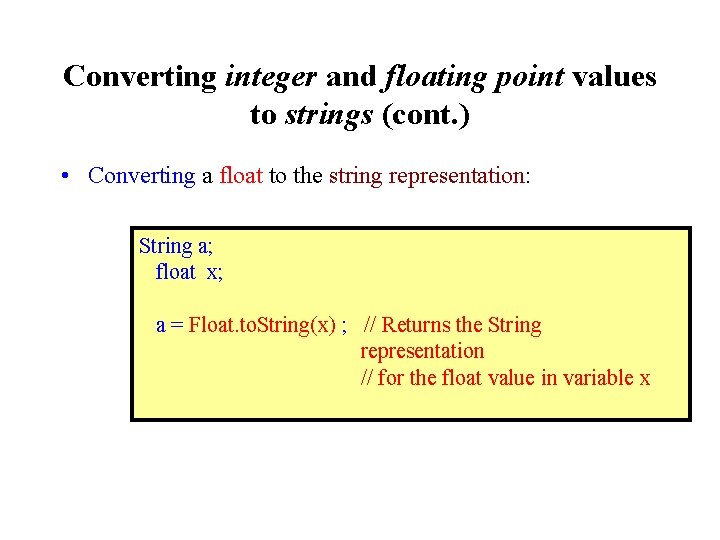
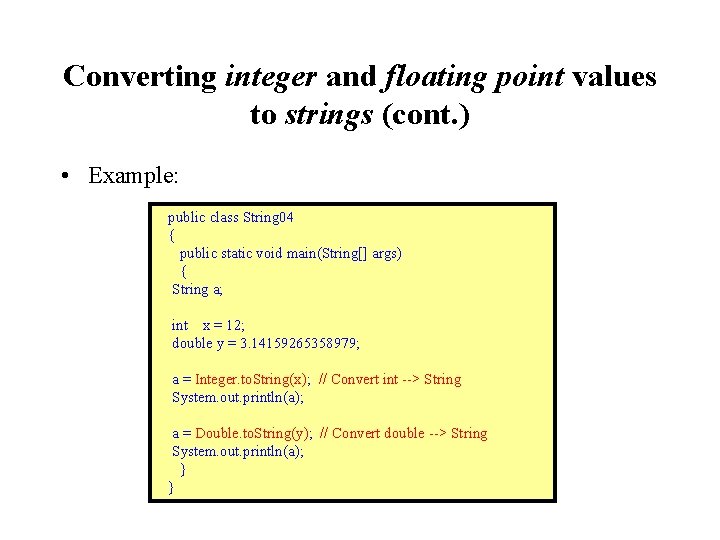
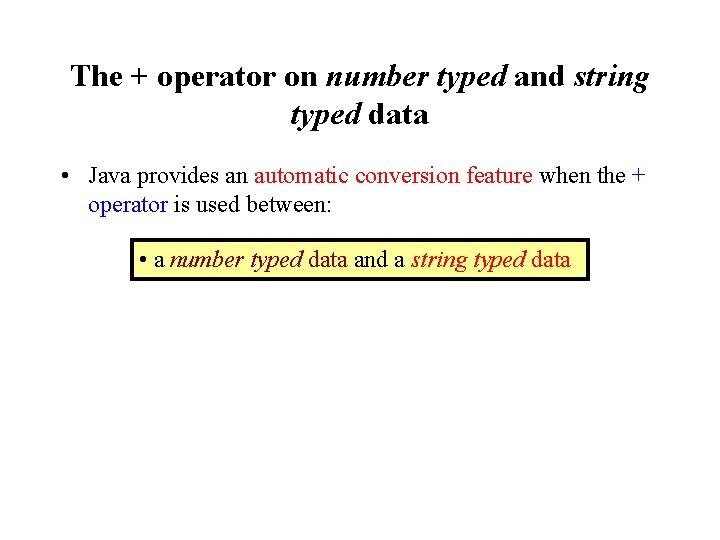
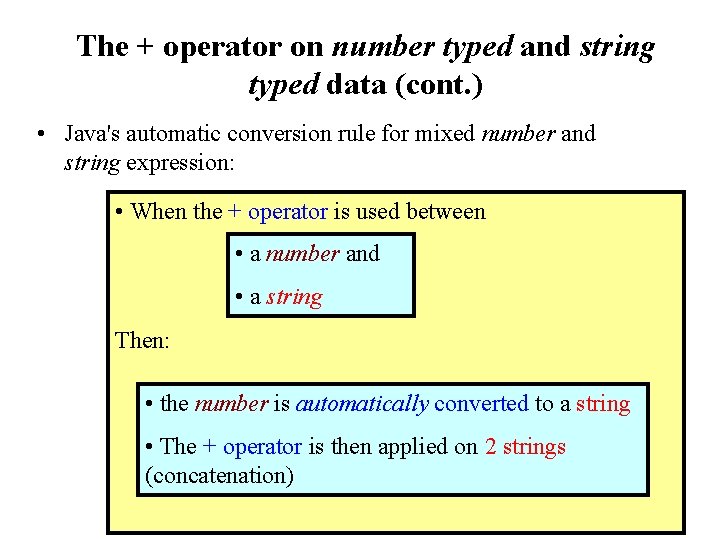
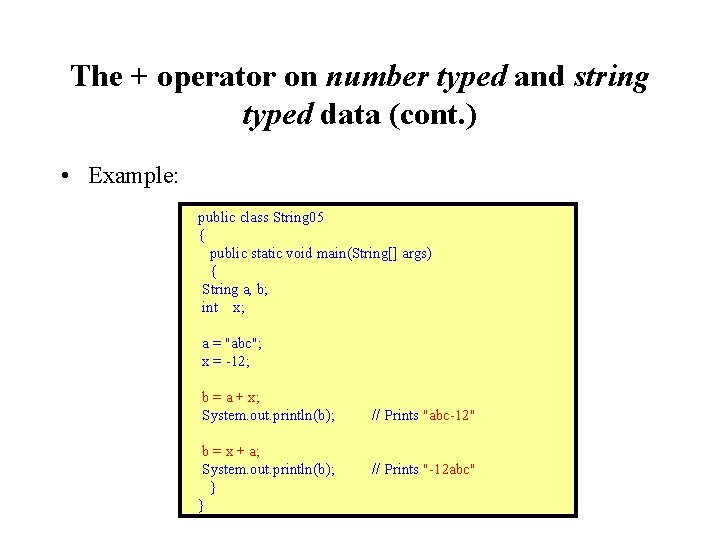
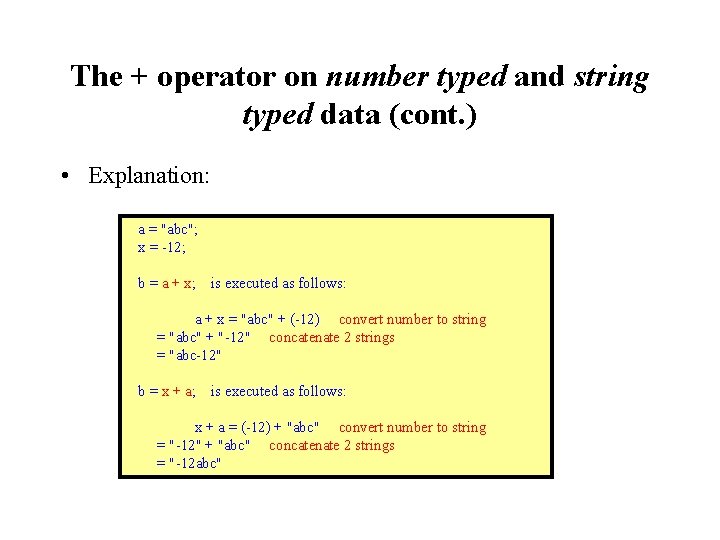
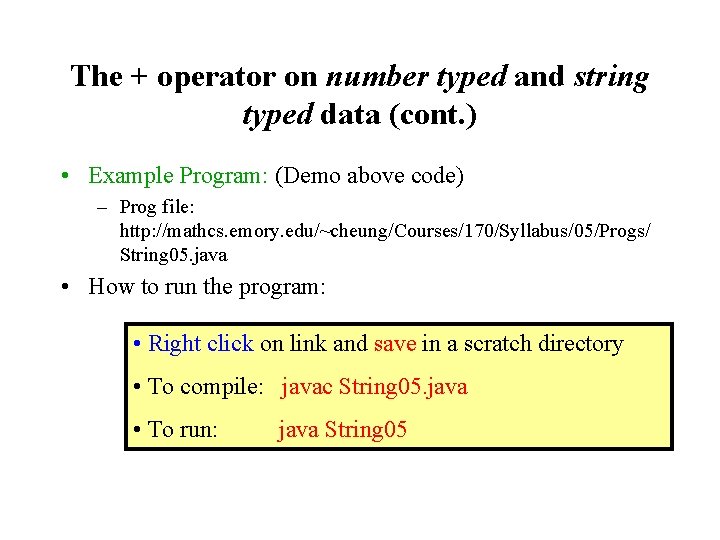
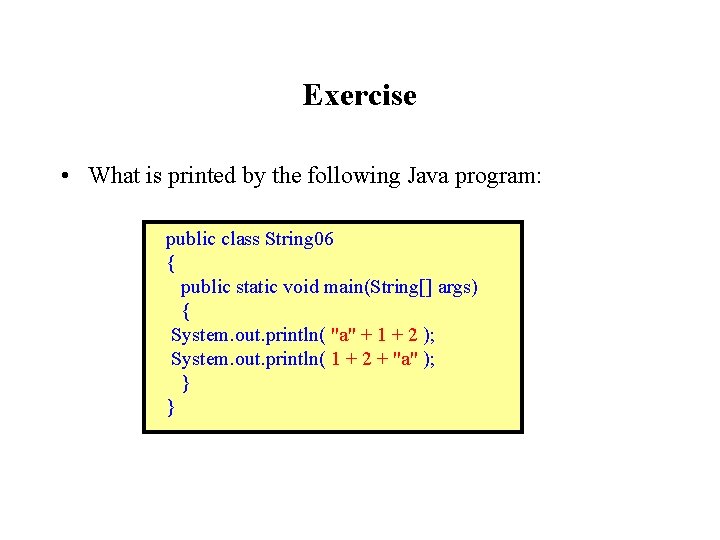
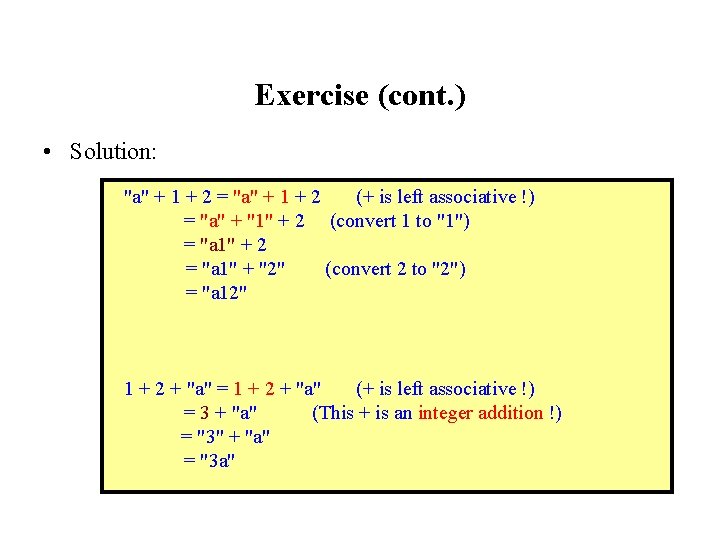
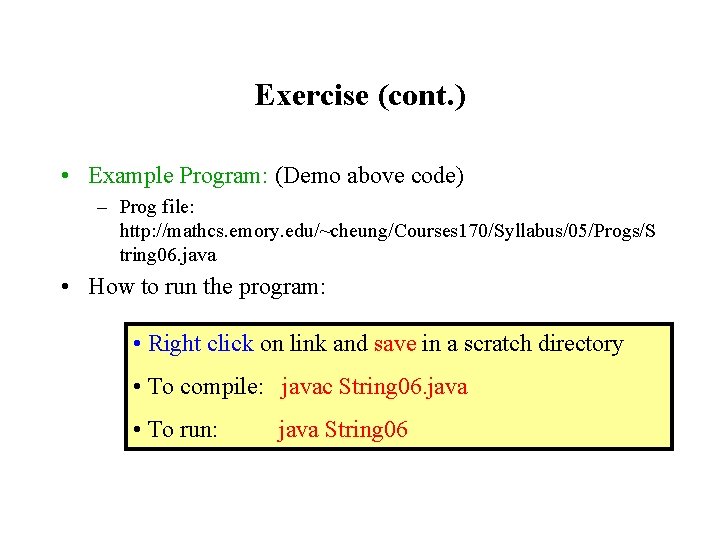
- Slides: 53
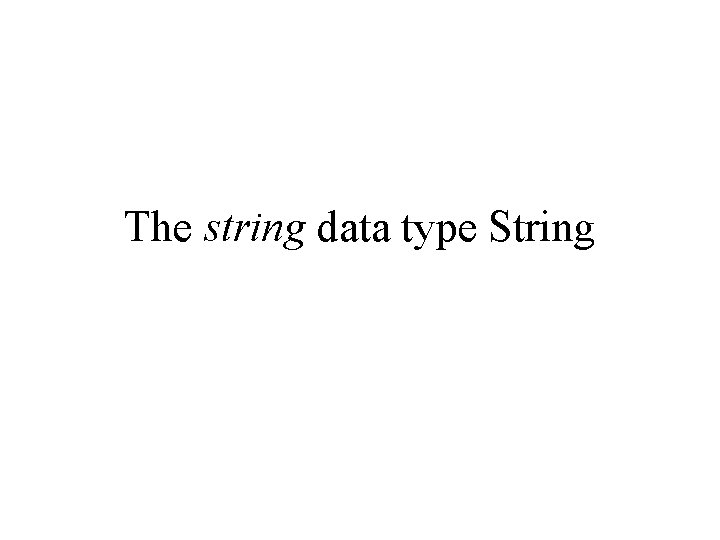
The string data type String
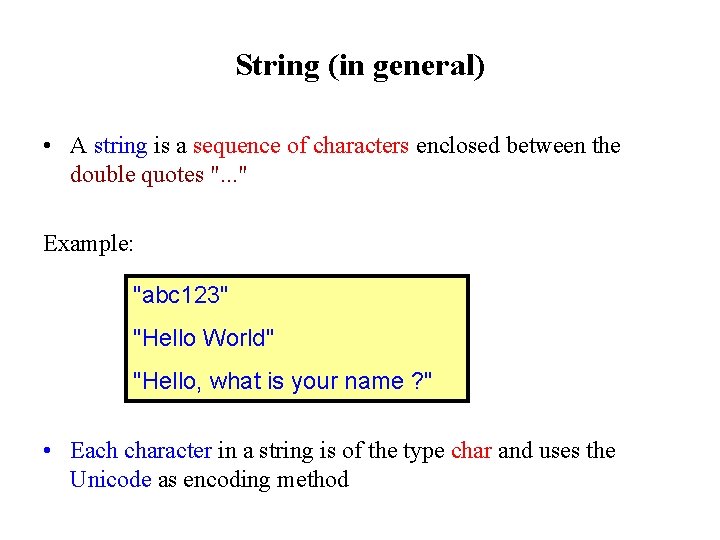
String (in general) • A string is a sequence of characters enclosed between the double quotes ". . . " Example: "abc 123" "Hello World" "Hello, what is your name ? " • Each character in a string is of the type char and uses the Unicode as encoding method
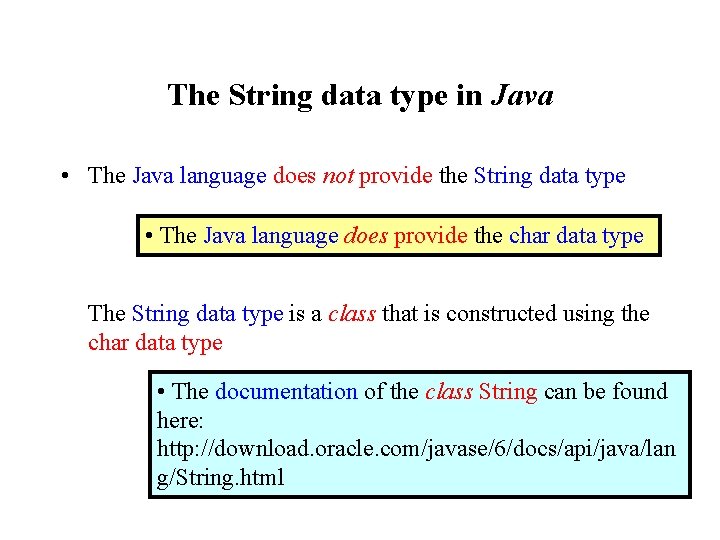
The String data type in Java • The Java language does not provide the String data type • The Java language does provide the char data type The String data type is a class that is constructed using the char data type • The documentation of the class String can be found here: http: //download. oracle. com/javase/6/docs/api/java/lan g/String. html
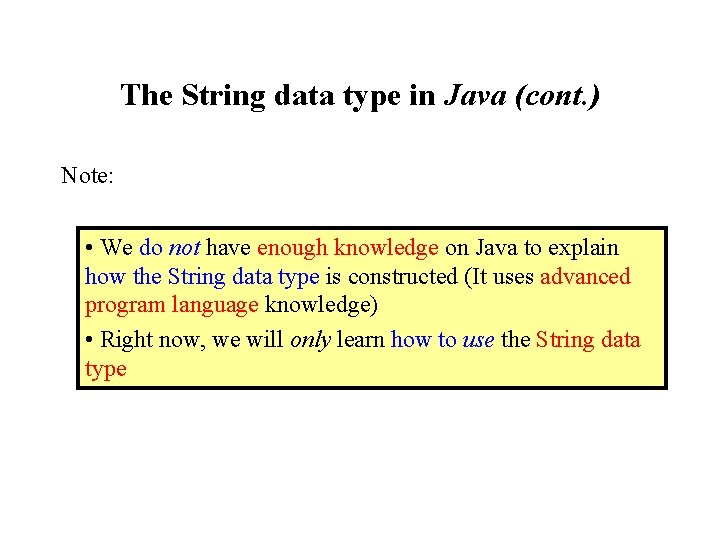
The String data type in Java (cont. ) Note: • We do not have enough knowledge on Java to explain how the String data type is constructed (It uses advanced program language knowledge) • Right now, we will only learn how to use the String data type
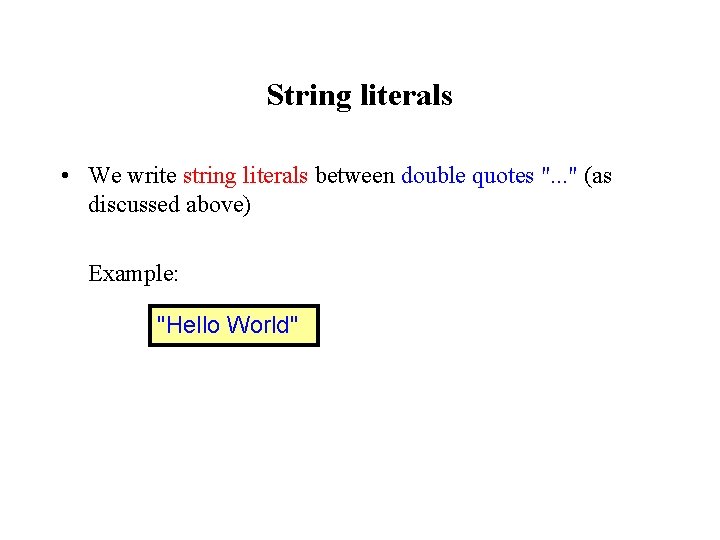
String literals • We write string literals between double quotes ". . . " (as discussed above) Example: "Hello World"
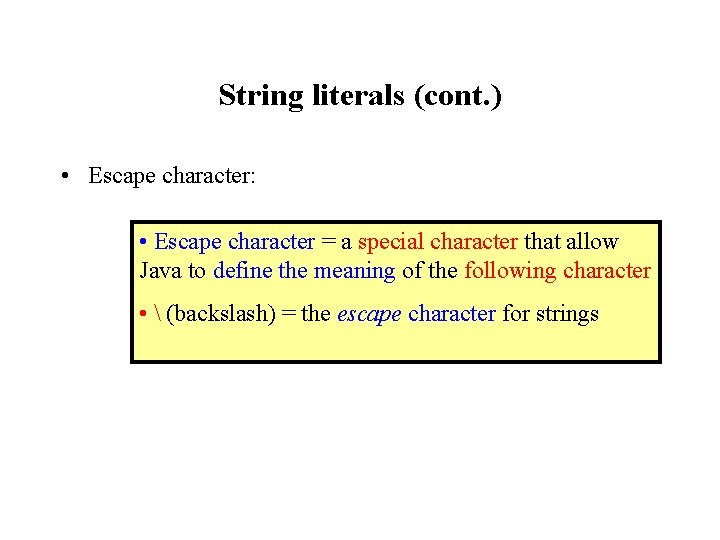
String literals (cont. ) • Escape character: • Escape character = a special character that allow Java to define the meaning of the following character • (backslash) = the escape character for strings
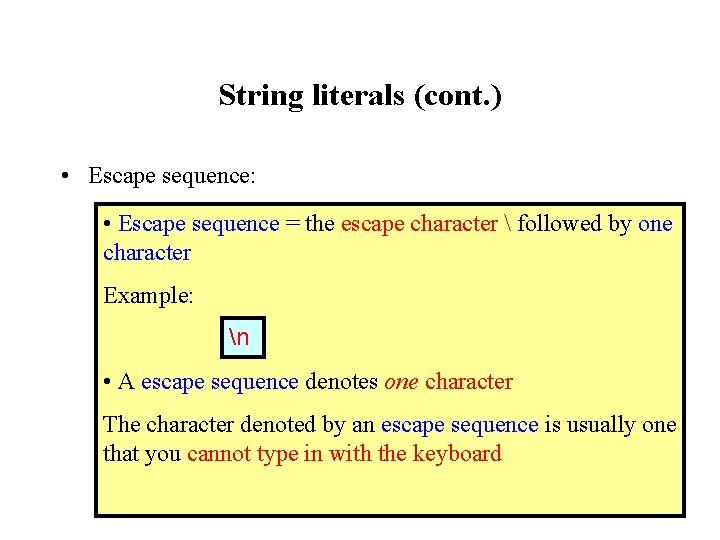
String literals (cont. ) • Escape sequence: • Escape sequence = the escape character followed by one character Example: n • A escape sequence denotes one character The character denoted by an escape sequence is usually one that you cannot type in with the keyboard
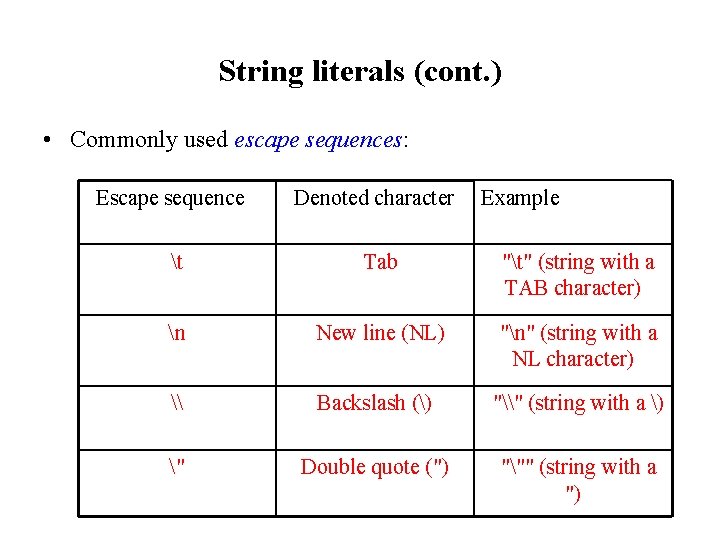
String literals (cont. ) • Commonly used escape sequences: Escape sequence Denoted character Example t Tab "t" (string with a TAB character) n New line (NL) "n" (string with a NL character) \ Backslash () "\" (string with a ) " Double quote (") """ (string with a ")
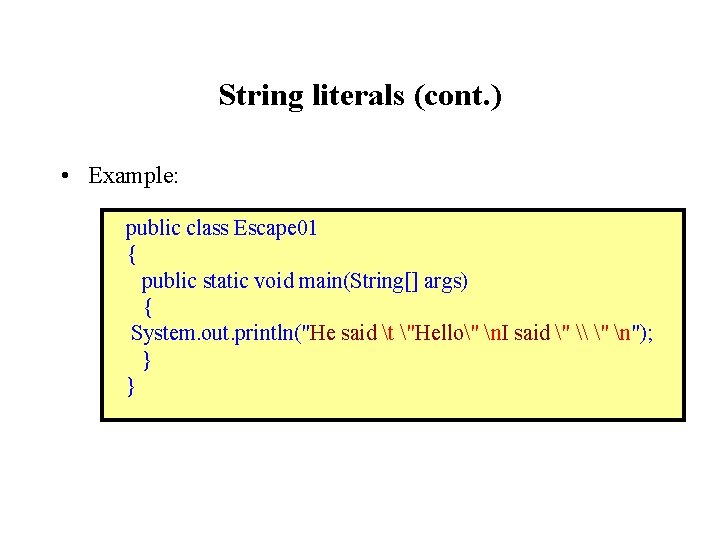
String literals (cont. ) • Example: public class Escape 01 { public static void main(String[] args) { System. out. println("He said t "Hello" n. I said " \ " n"); } }
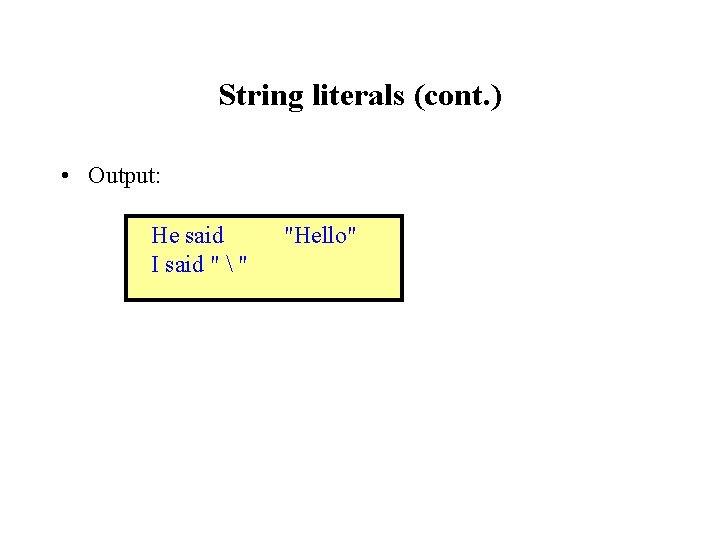
String literals (cont. ) • Output: He said "Hello" I said " "
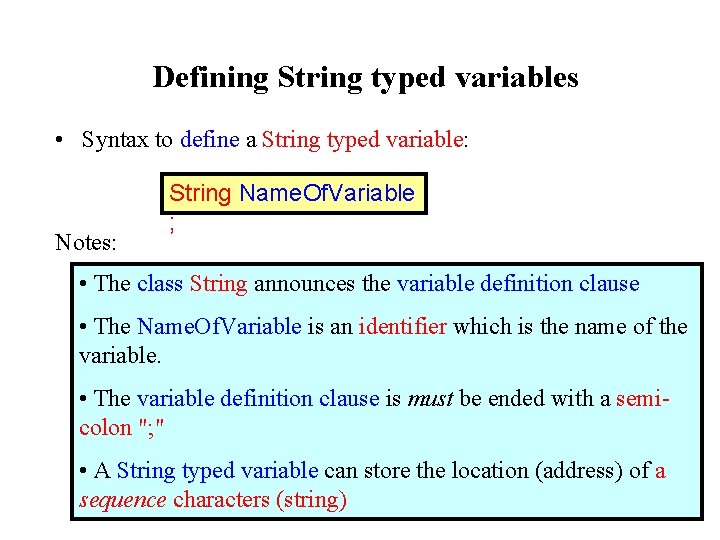
Defining String typed variables • Syntax to define a String typed variable: Notes: String Name. Of. Variable ; • The class String announces the variable definition clause • The Name. Of. Variable is an identifier which is the name of the variable. • The variable definition clause is must be ended with a semicolon "; " • A String typed variable can store the location (address) of a sequence characters (string)
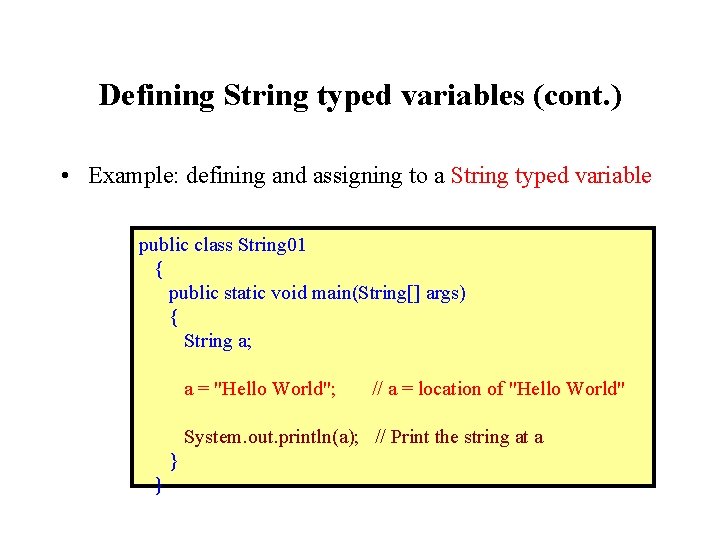
Defining String typed variables (cont. ) • Example: defining and assigning to a String typed variable public class String 01 { public static void main(String[] args) { String a; a = "Hello World"; // a = location of "Hello World" System. out. println(a); // Print the string at a } }
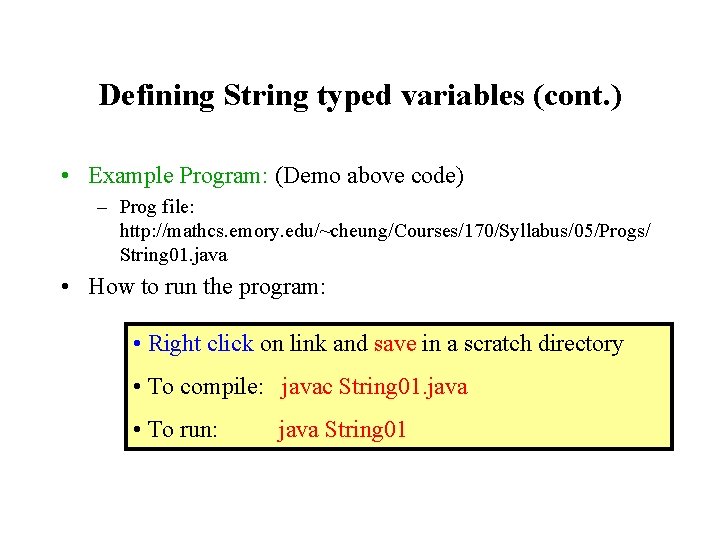
Defining String typed variables (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ String 01. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac String 01. java • To run: java String 01
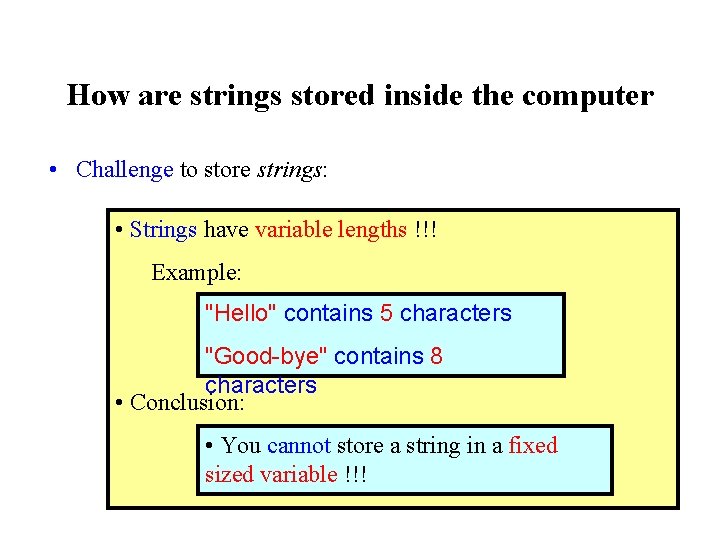
How are strings stored inside the computer • Challenge to store strings: • Strings have variable lengths !!! Example: "Hello" contains 5 characters "Good-bye" contains 8 characters • Conclusion: • You cannot store a string in a fixed sized variable !!!
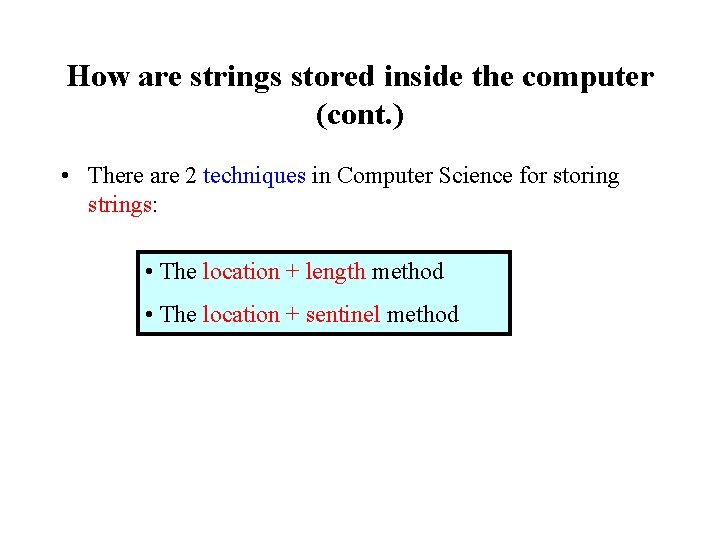
How are strings stored inside the computer (cont. ) • There are 2 techniques in Computer Science for storing strings: • The location + length method • The location + sentinel method
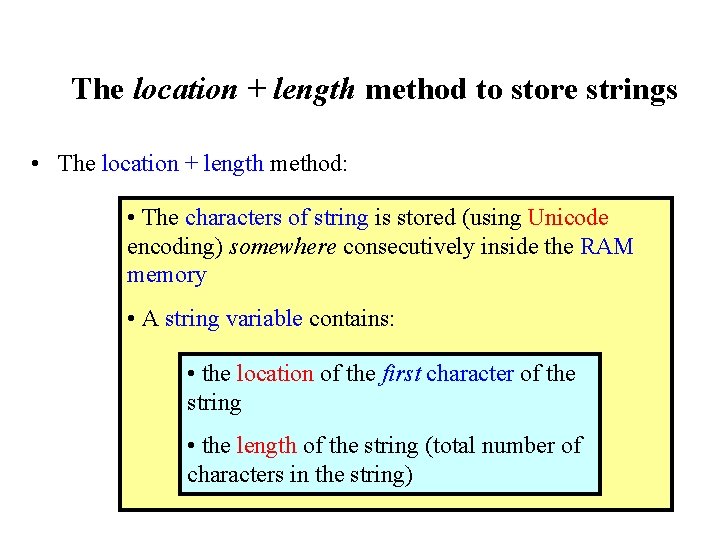
The location + length method to store strings • The location + length method: • The characters of string is stored (using Unicode encoding) somewhere consecutively inside the RAM memory • A string variable contains: • the location of the first character of the string • the length of the string (total number of characters in the string)
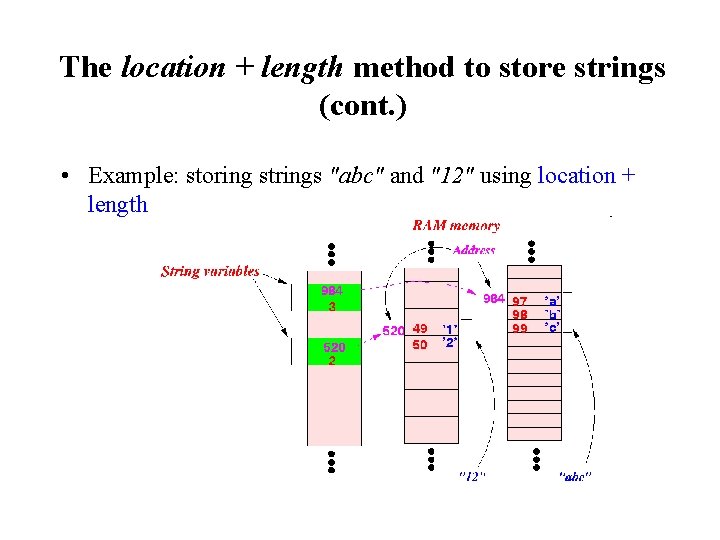
The location + length method to store strings (cont. ) • Example: storing strings "abc" and "12" using location + length
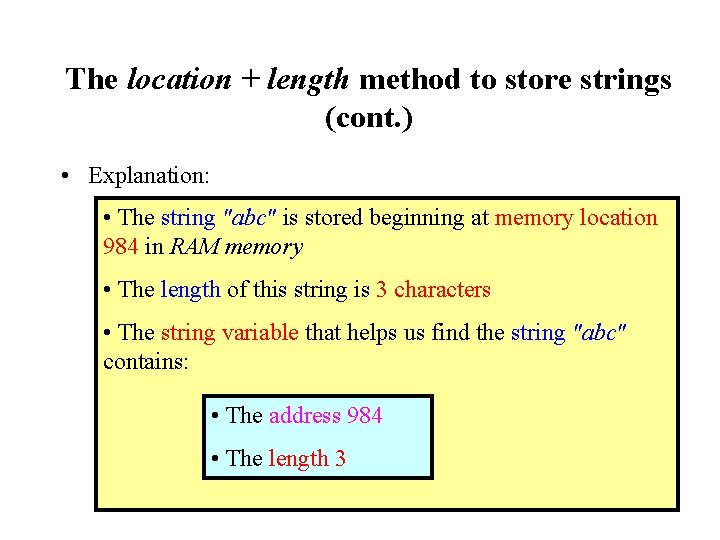
The location + length method to store strings (cont. ) • Explanation: • The string "abc" is stored beginning at memory location 984 in RAM memory • The length of this string is 3 characters • The string variable that helps us find the string "abc" contains: • The address 984 • The length 3
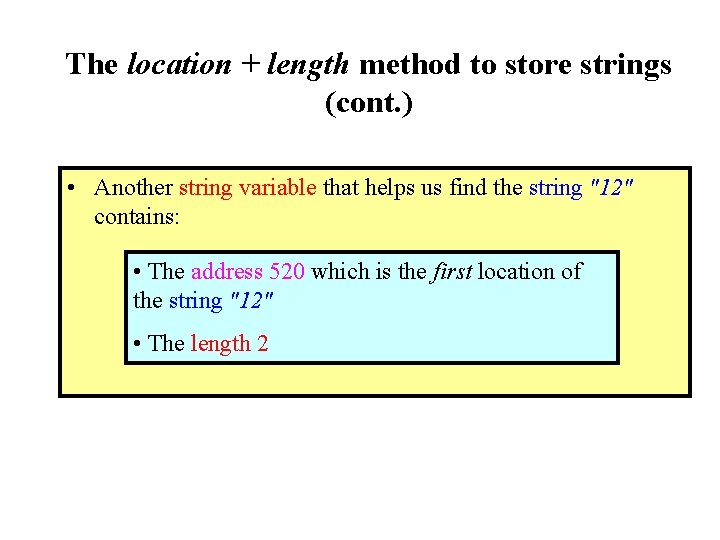
The location + length method to store strings (cont. ) • Another string variable that helps us find the string "12" contains: • The address 520 which is the first location of the string "12" • The length 2
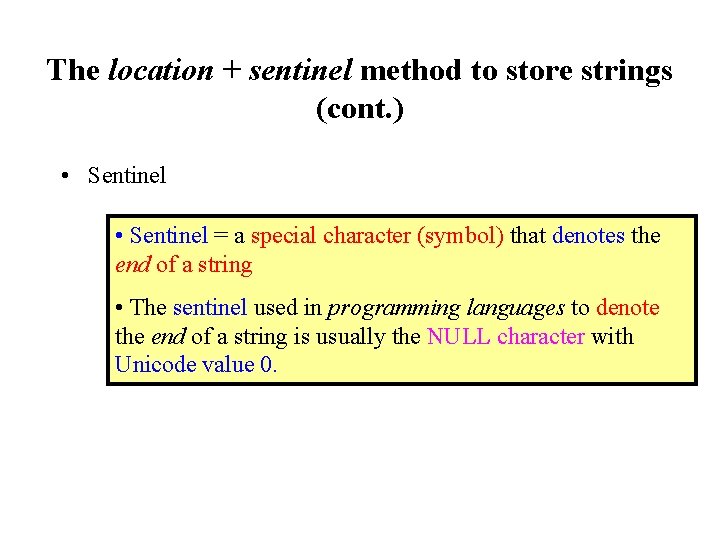
The location + sentinel method to store strings (cont. ) • Sentinel = a special character (symbol) that denotes the end of a string • The sentinel used in programming languages to denote the end of a string is usually the NULL character with Unicode value 0.
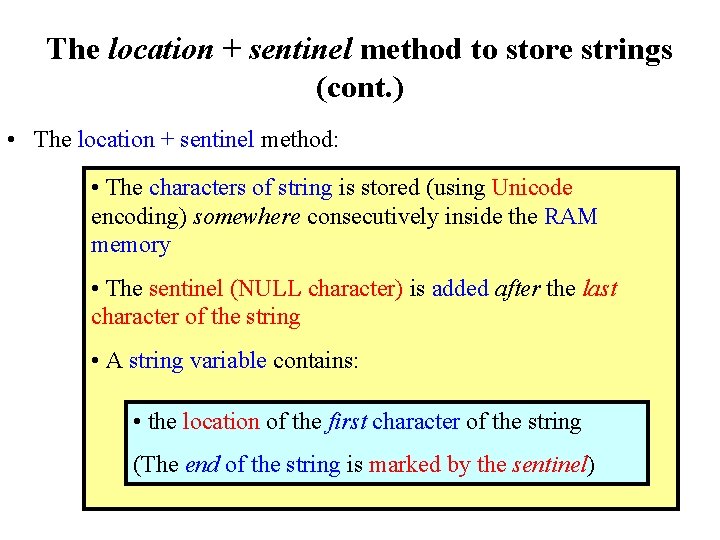
The location + sentinel method to store strings (cont. ) • The location + sentinel method: • The characters of string is stored (using Unicode encoding) somewhere consecutively inside the RAM memory • The sentinel (NULL character) is added after the last character of the string • A string variable contains: • the location of the first character of the string (The end of the string is marked by the sentinel)
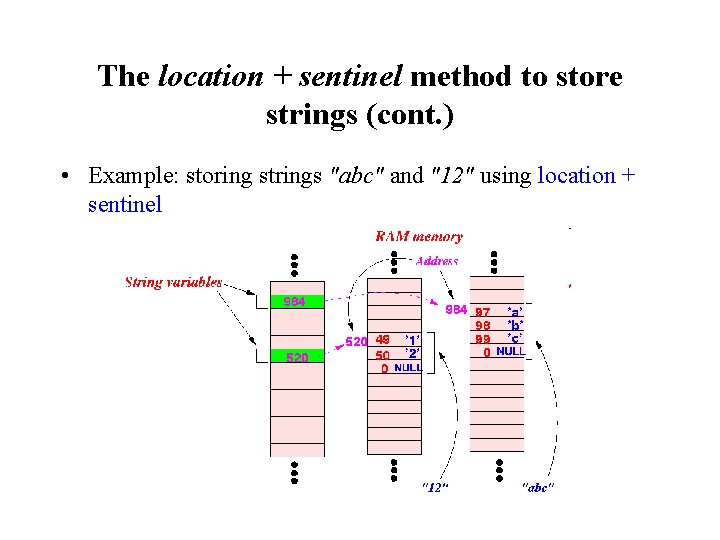
The location + sentinel method to store strings (cont. ) • Example: storing strings "abc" and "12" using location + sentinel
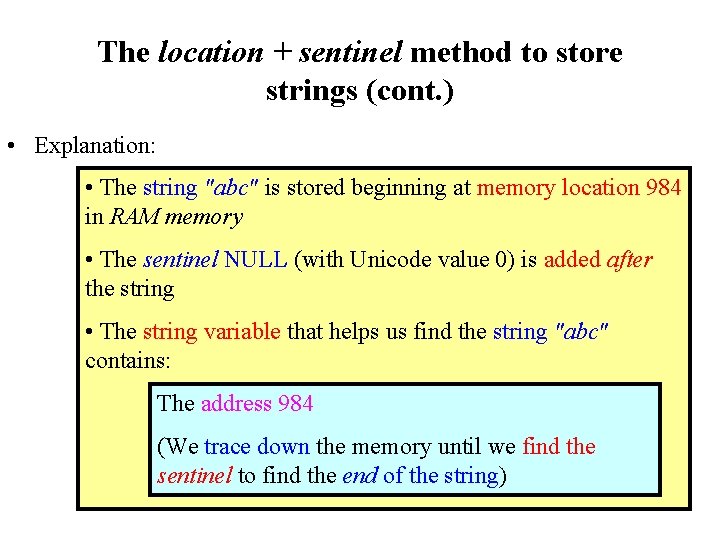
The location + sentinel method to store strings (cont. ) • Explanation: • The string "abc" is stored beginning at memory location 984 in RAM memory • The sentinel NULL (with Unicode value 0) is added after the string • The string variable that helps us find the string "abc" contains: The address 984 (We trace down the memory until we find the sentinel to find the end of the string)
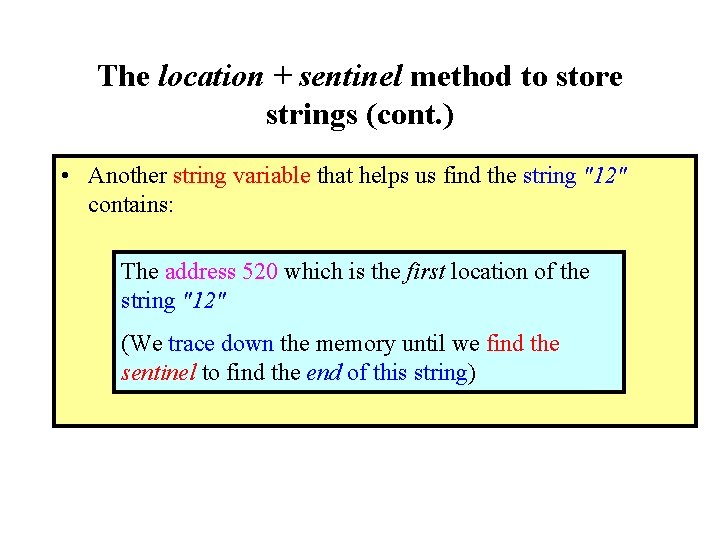
The location + sentinel method to store strings (cont. ) • Another string variable that helps us find the string "12" contains: The address 520 which is the first location of the string "12" (We trace down the memory until we find the sentinel to find the end of this string)
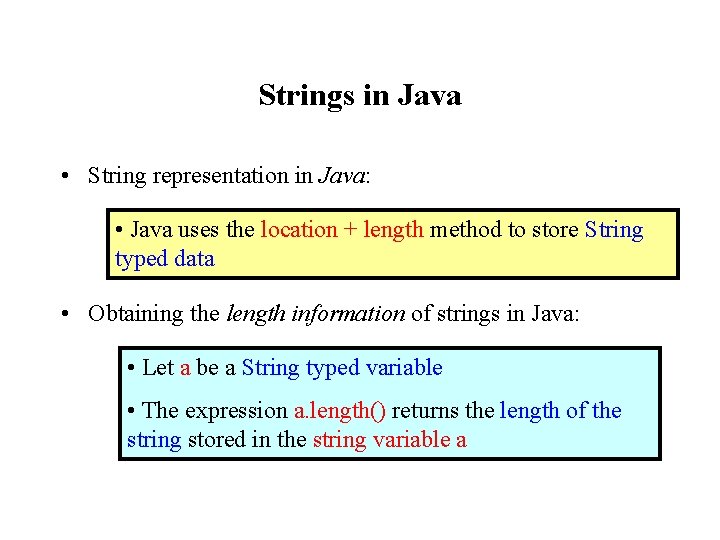
Strings in Java • String representation in Java: • Java uses the location + length method to store String typed data • Obtaining the length information of strings in Java: • Let a be a String typed variable • The expression a. length() returns the length of the string stored in the string variable a
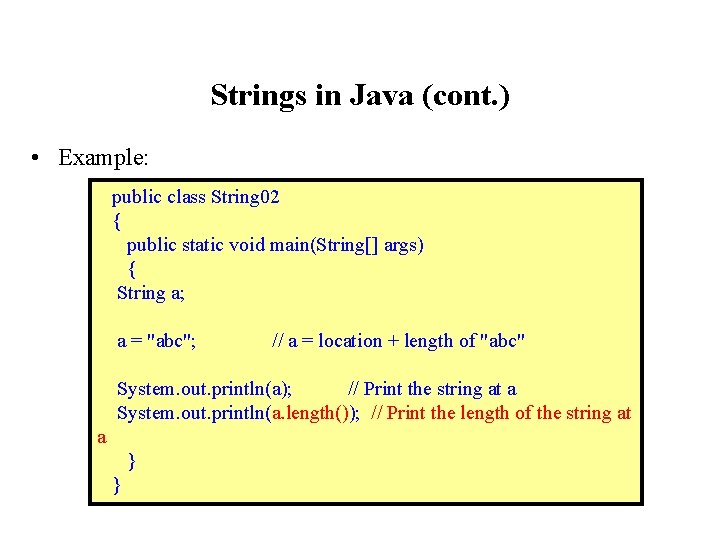
Strings in Java (cont. ) • Example: public class String 02 { public static void main(String[] args) { String a; a = "abc"; // a = location + length of "abc" System. out. println(a); // Print the string at a System. out. println(a. length()); // Print the length of the string at a } }
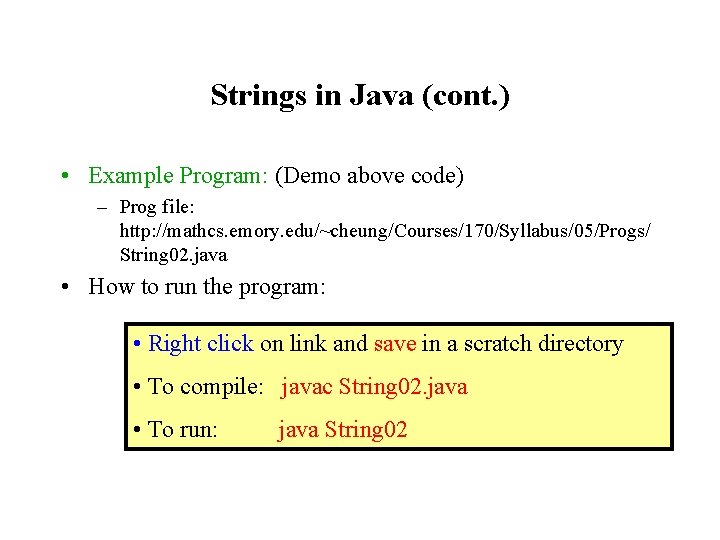
Strings in Java (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ String 02. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac String 02. java • To run: java String 02
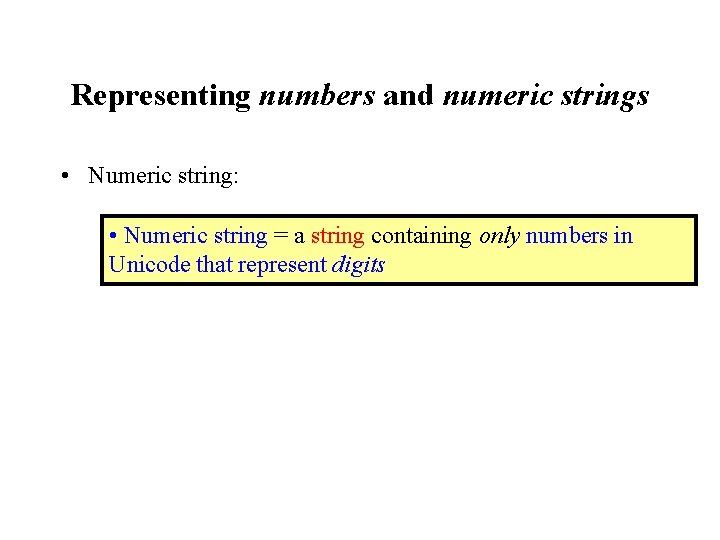
Representing numbers and numeric strings • Numeric string: • Numeric string = a string containing only numbers in Unicode that represent digits
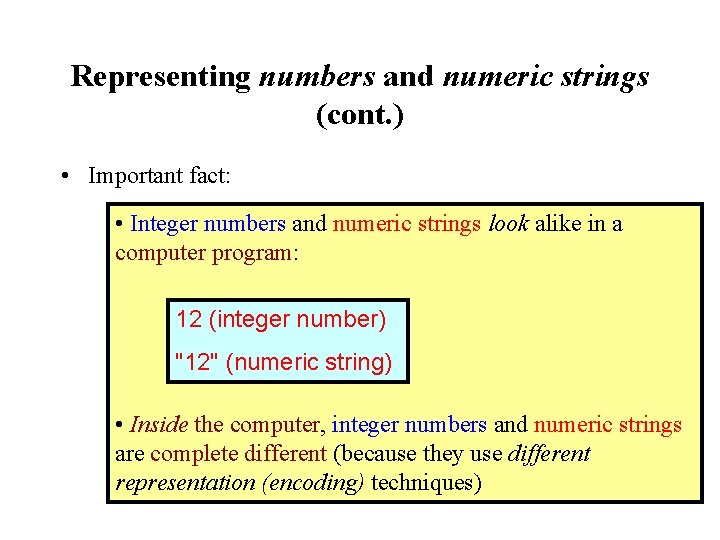
Representing numbers and numeric strings (cont. ) • Important fact: • Integer numbers and numeric strings look alike in a computer program: 12 (integer number) "12" (numeric string) • Inside the computer, integer numbers and numeric strings are complete different (because they use different representation (encoding) techniques)
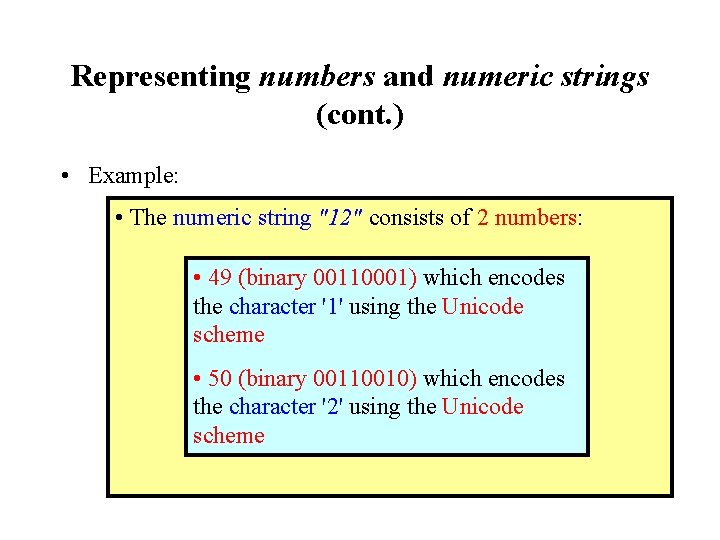
Representing numbers and numeric strings (cont. ) • Example: • The numeric string "12" consists of 2 numbers: • 49 (binary 00110001) which encodes the character '1' using the Unicode scheme • 50 (binary 00110010) which encodes the character '2' using the Unicode scheme
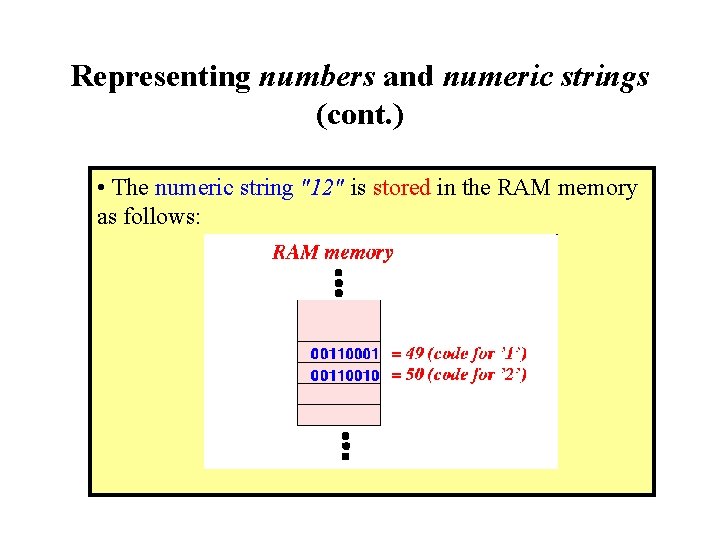
Representing numbers and numeric strings (cont. ) • The numeric string "12" is stored in the RAM memory as follows:
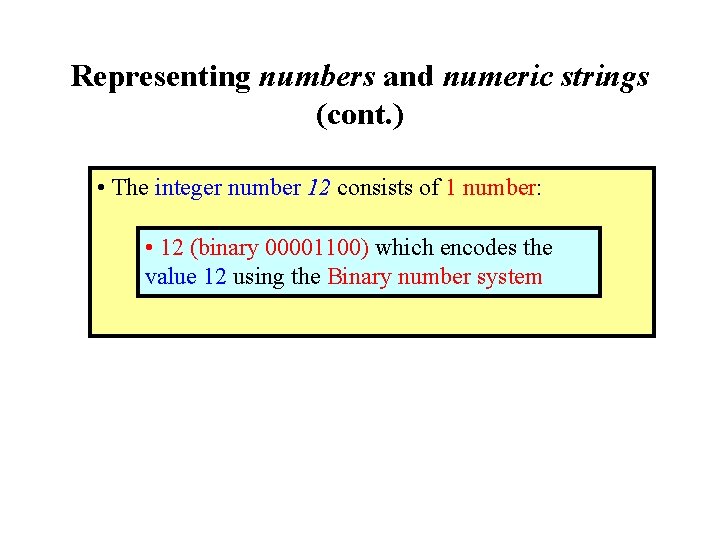
Representing numbers and numeric strings (cont. ) • The integer number 12 consists of 1 number: • 12 (binary 00001100) which encodes the value 12 using the Binary number system
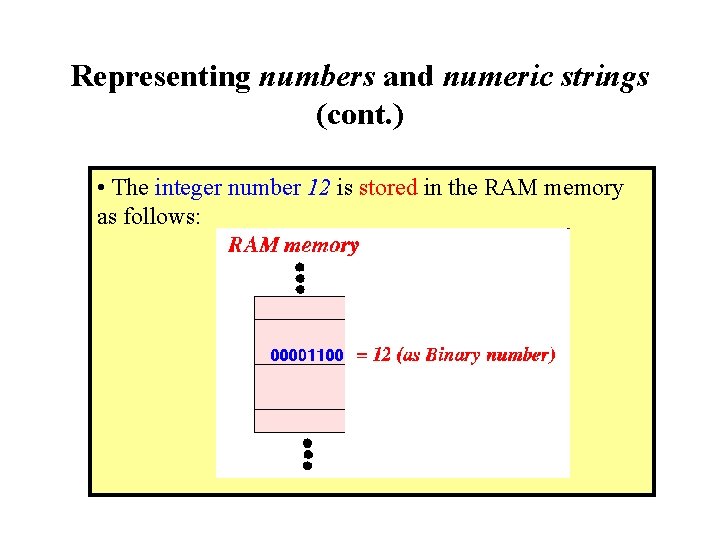
Representing numbers and numeric strings (cont. ) • The integer number 12 is stored in the RAM memory as follows:
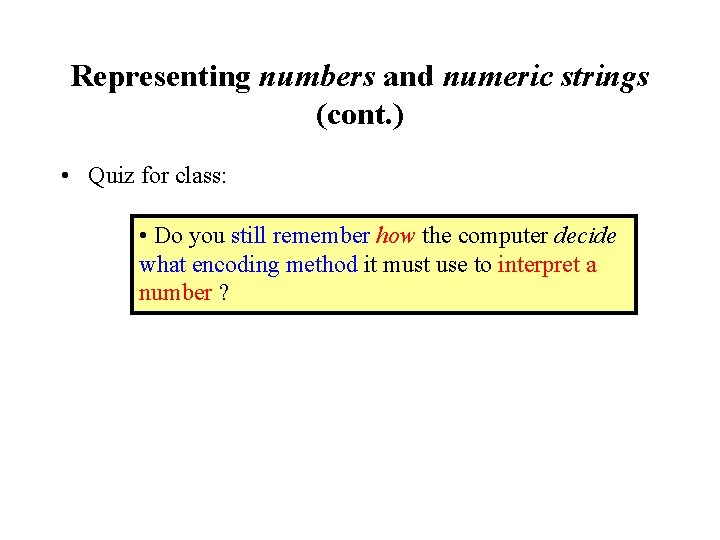
Representing numbers and numeric strings (cont. ) • Quiz for class: • Do you still remember how the computer decide what encoding method it must use to interpret a number ?
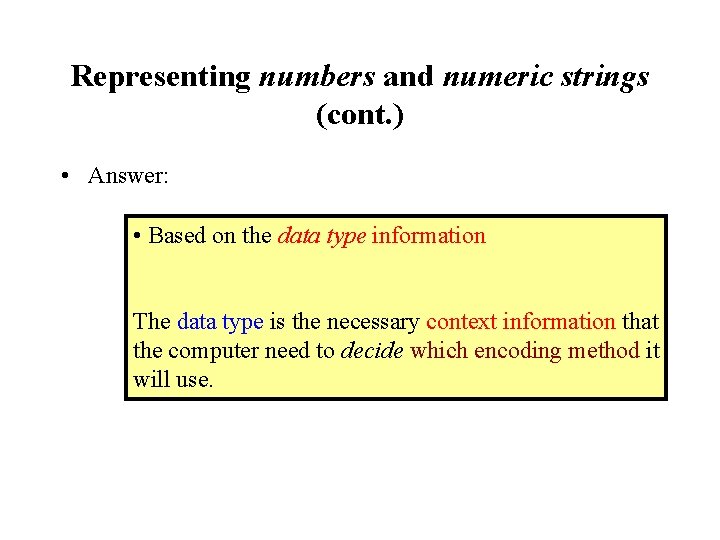
Representing numbers and numeric strings (cont. ) • Answer: • Based on the data type information The data type is the necessary context information that the computer need to decide which encoding method it will use.
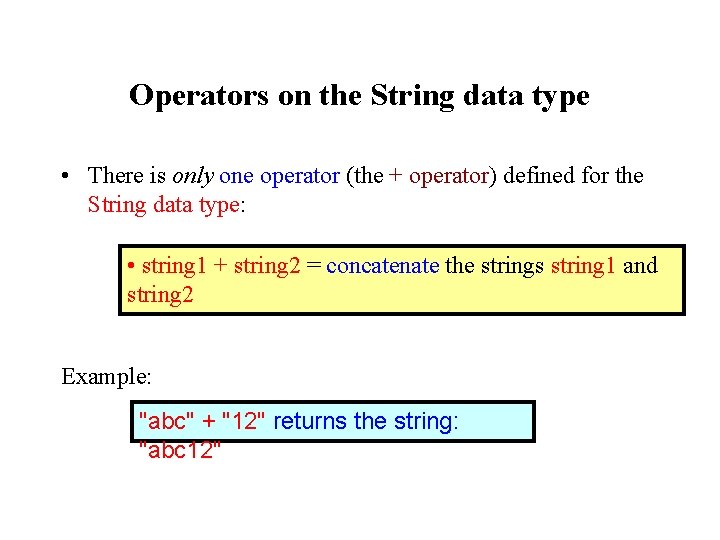
Operators on the String data type • There is only one operator (the + operator) defined for the String data type: • string 1 + string 2 = concatenate the strings string 1 and string 2 Example: "abc" + "12" returns the string: "abc 12"
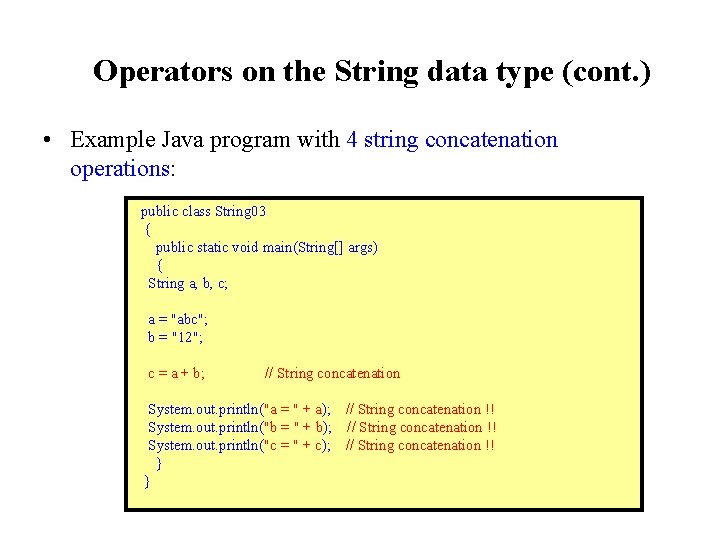
Operators on the String data type (cont. ) • Example Java program with 4 string concatenation operations: public class String 03 { public static void main(String[] args) { String a, b, c; a = "abc"; b = "12"; c = a + b; // String concatenation System. out. println("a = " + a); // String concatenation !! System. out. println("b = " + b); // String concatenation !! System. out. println("c = " + c); // String concatenation !! } }
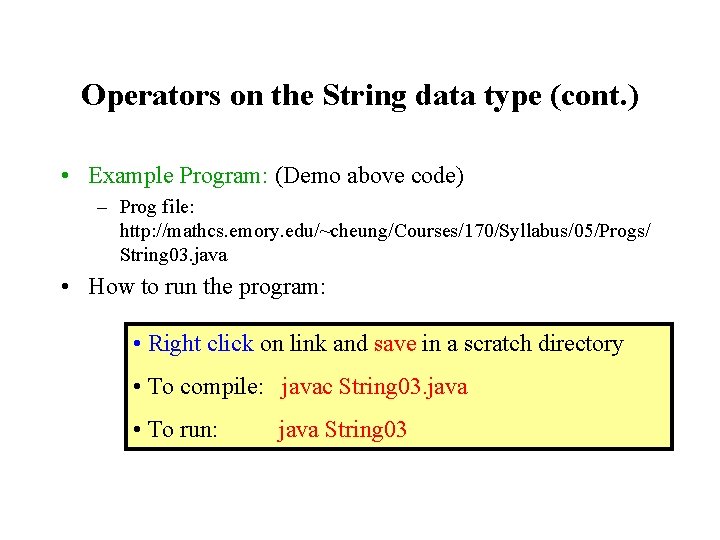
Operators on the String data type (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ String 03. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac String 03. java • To run: java String 03
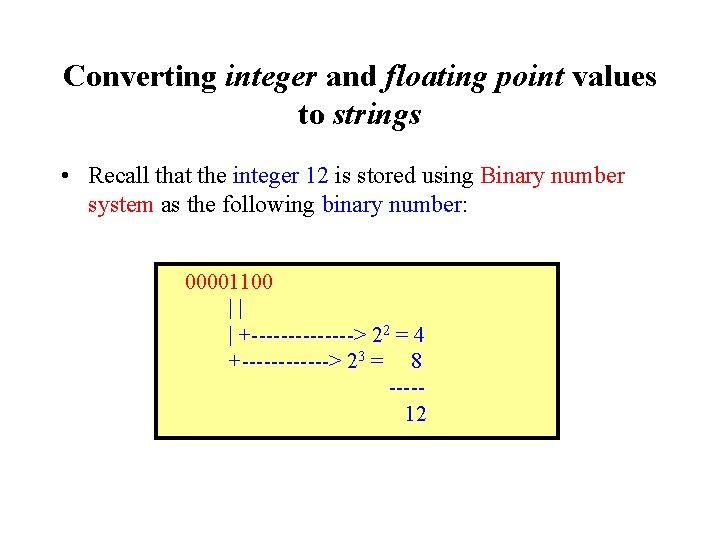
Converting integer and floating point values to strings • Recall that the integer 12 is stored using Binary number system as the following binary number: 00001100 | | | +-------> 22 = 4 +------> 23 = 8 ----- 12
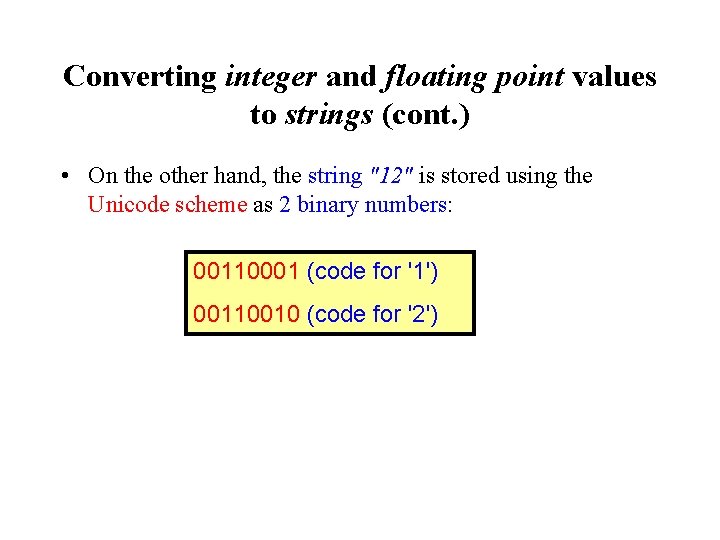
Converting integer and floating point values to strings (cont. ) • On the other hand, the string "12" is stored using the Unicode scheme as 2 binary numbers: 00110001 (code for '1') 00110010 (code for '2')
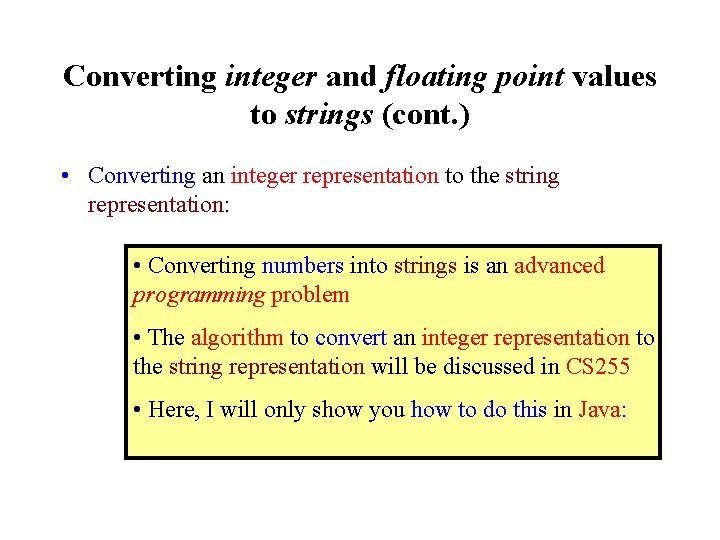
Converting integer and floating point values to strings (cont. ) • Converting an integer representation to the string representation: • Converting numbers into strings is an advanced programming problem • The algorithm to convert an integer representation to the string representation will be discussed in CS 255 • Here, I will only show you how to do this in Java:
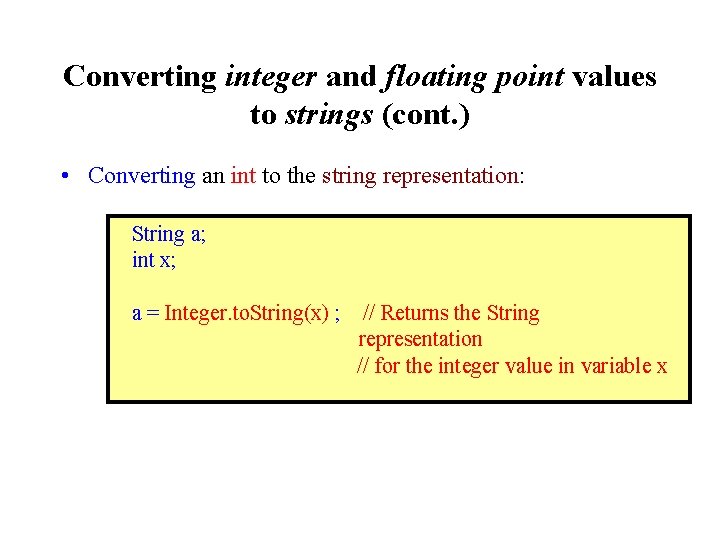
Converting integer and floating point values to strings (cont. ) • Converting an int to the string representation: String a; int x; a = Integer. to. String(x) ; // Returns the String representation // for the integer value in variable x
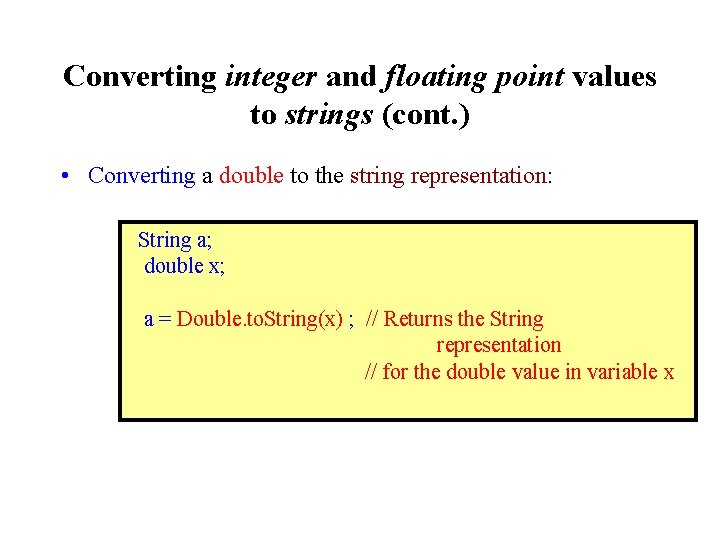
Converting integer and floating point values to strings (cont. ) • Converting a double to the string representation: String a; double x; a = Double. to. String(x) ; // Returns the String representation // for the double value in variable x
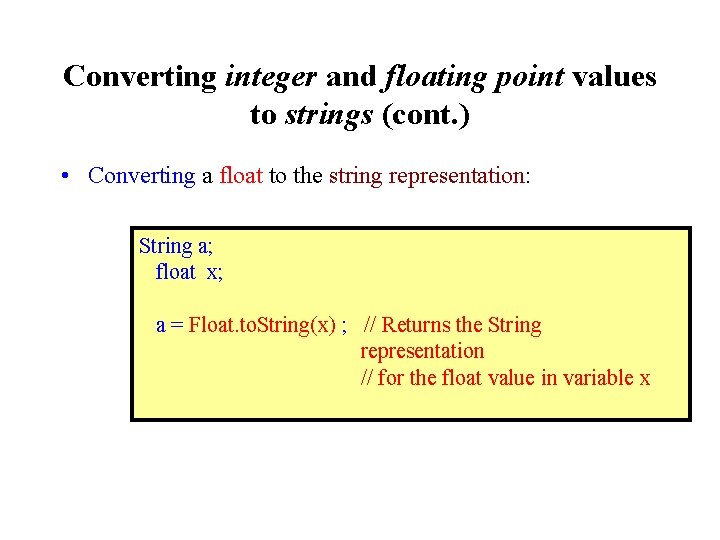
Converting integer and floating point values to strings (cont. ) • Converting a float to the string representation: String a; float x; a = Float. to. String(x) ; // Returns the String representation // for the float value in variable x
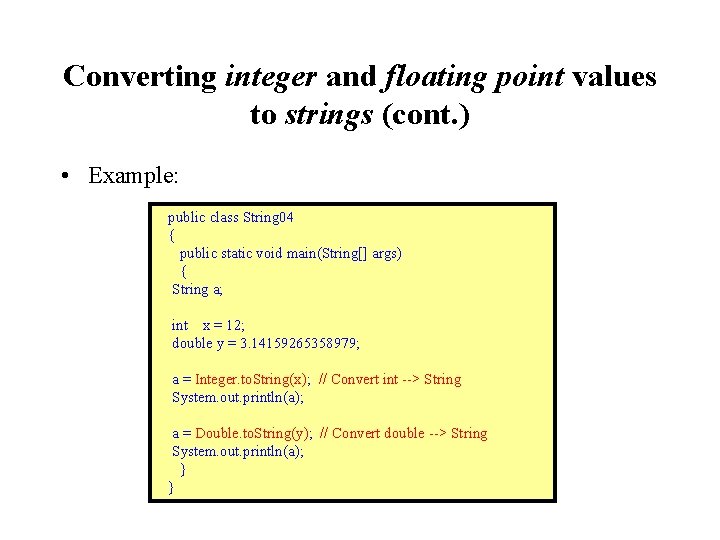
Converting integer and floating point values to strings (cont. ) • Example: public class String 04 { public static void main(String[] args) { String a; int x = 12; double y = 3. 14159265358979; a = Integer. to. String(x); // Convert int --> String System. out. println(a); a = Double. to. String(y); // Convert double --> String System. out. println(a); } }
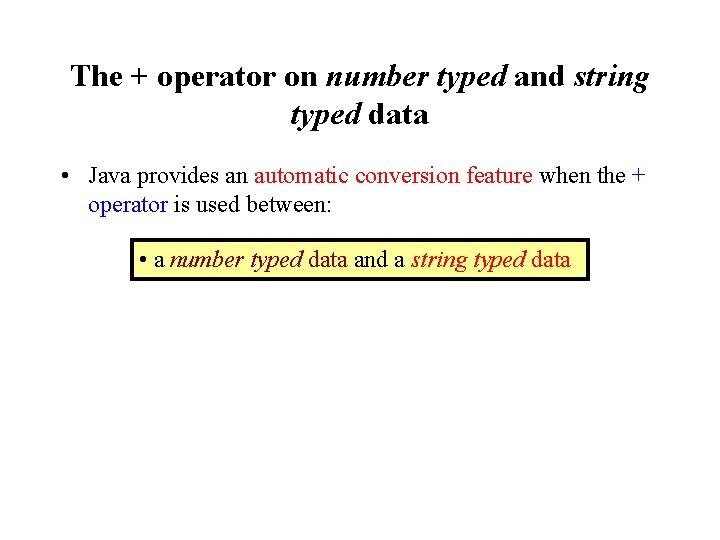
The + operator on number typed and string typed data • Java provides an automatic conversion feature when the + operator is used between: • a number typed data and a string typed data
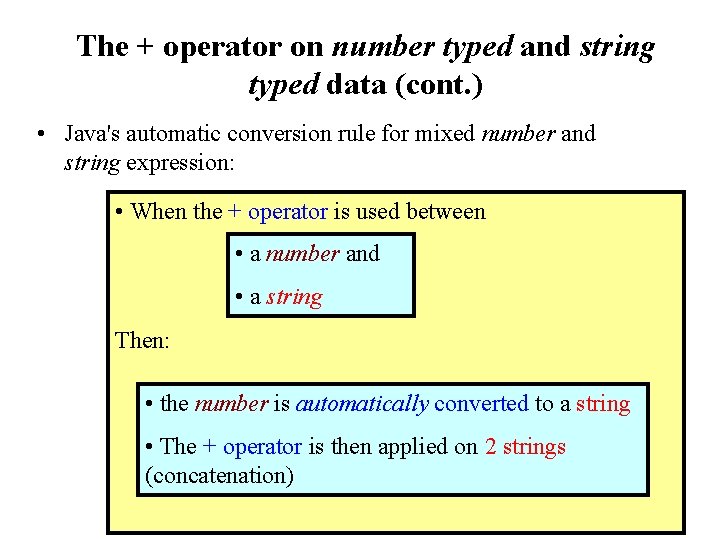
The + operator on number typed and string typed data (cont. ) • Java's automatic conversion rule for mixed number and string expression: • When the + operator is used between • a number and • a string Then: • the number is automatically converted to a string • The + operator is then applied on 2 strings (concatenation)
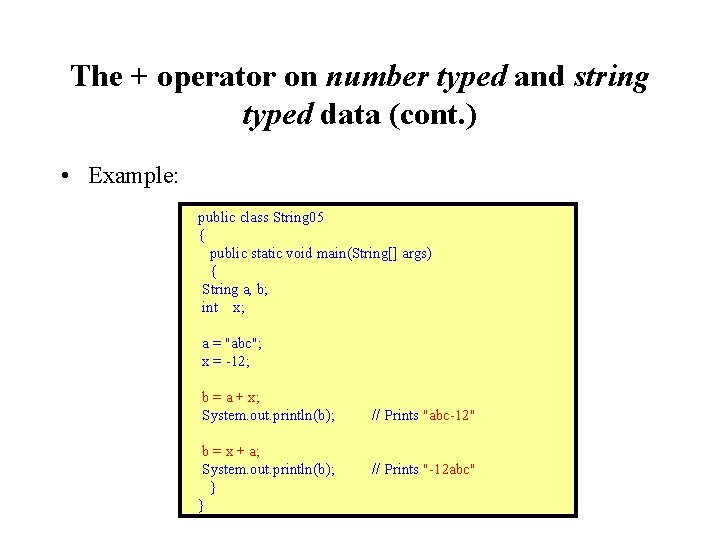
The + operator on number typed and string typed data (cont. ) • Example: public class String 05 { public static void main(String[] args) { String a, b; int x; a = "abc"; x = -12; b = a + x; System. out. println(b); // Prints "abc-12" b = x + a; System. out. println(b); // Prints "-12 abc" } }
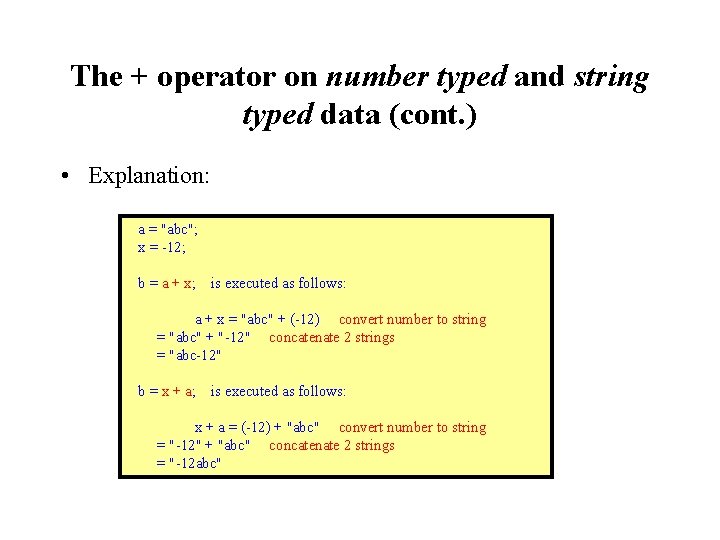
The + operator on number typed and string typed data (cont. ) • Explanation: a = "abc"; x = -12; b = a + x; is executed as follows: a + x = "abc" + (-12) convert number to string = "abc" + "-12" concatenate 2 strings = "abc-12" b = x + a; is executed as follows: x + a = (-12) + "abc" convert number to string = "-12" + "abc" concatenate 2 strings = "-12 abc"
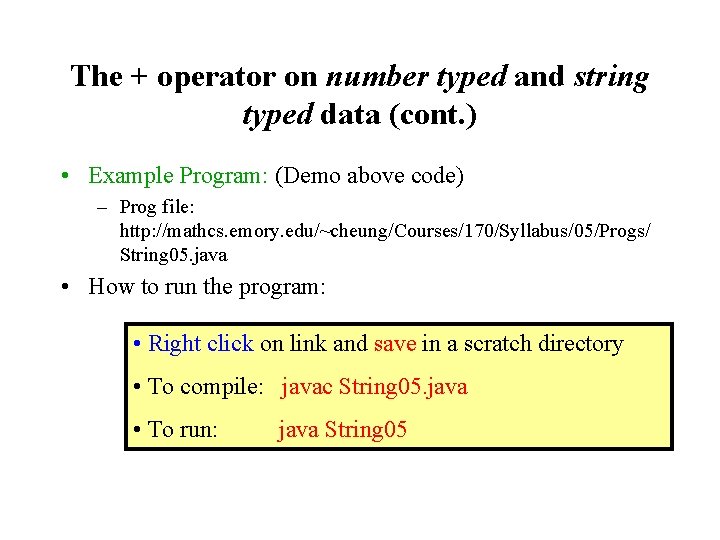
The + operator on number typed and string typed data (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ String 05. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac String 05. java • To run: java String 05
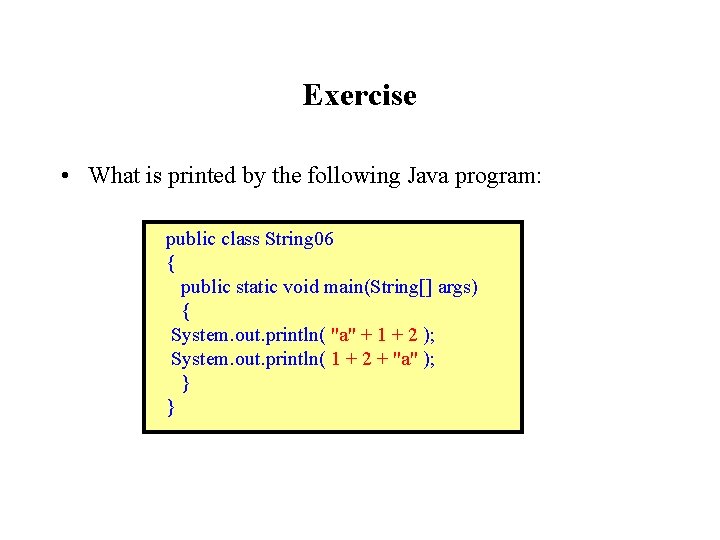
Exercise • What is printed by the following Java program: public class String 06 { public static void main(String[] args) { System. out. println( "a" + 1 + 2 ); System. out. println( 1 + 2 + "a" ); } }
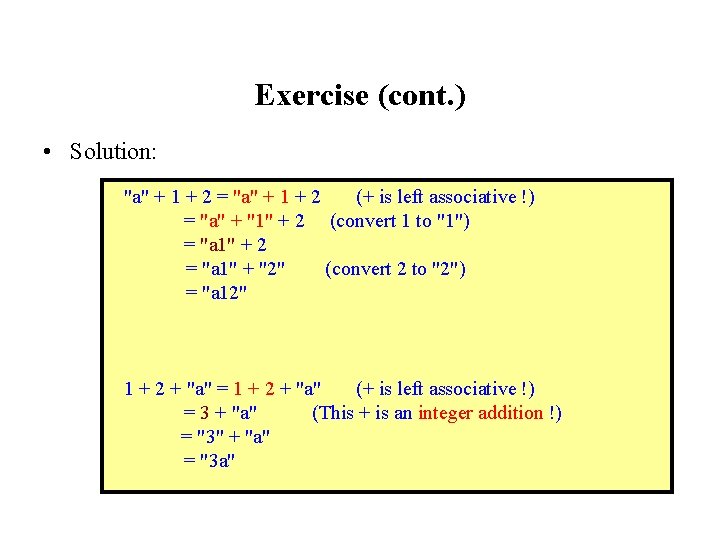
Exercise (cont. ) • Solution: "a" + 1 + 2 = "a" + 1 + 2 (+ is left associative !) = "a" + "1" + 2 (convert 1 to "1") = "a 1" + 2 = "a 1" + "2" (convert 2 to "2") = "a 12" 1 + 2 + "a" = 1 + 2 + "a" (+ is left associative !) = 3 + "a" (This + is an integer addition !) = "3" + "a" = "3 a"
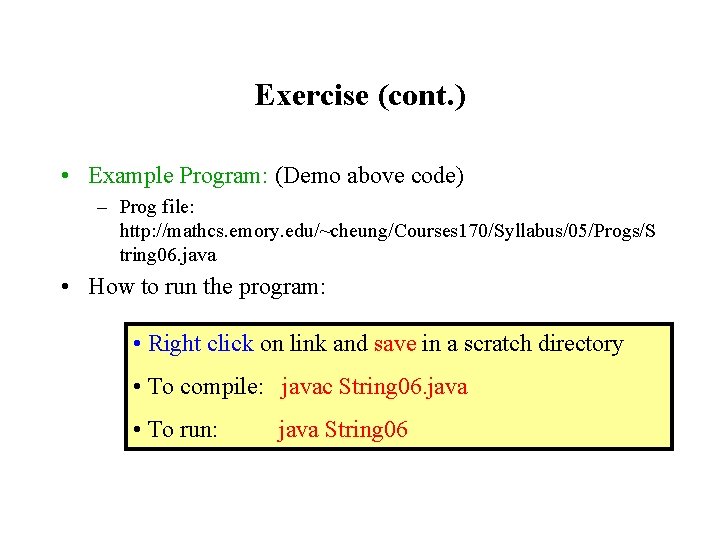
Exercise (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses 170/Syllabus/05/Progs/S tring 06. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac String 06. java • To run: java String 06