Purchase java Number Format import java util Scanner
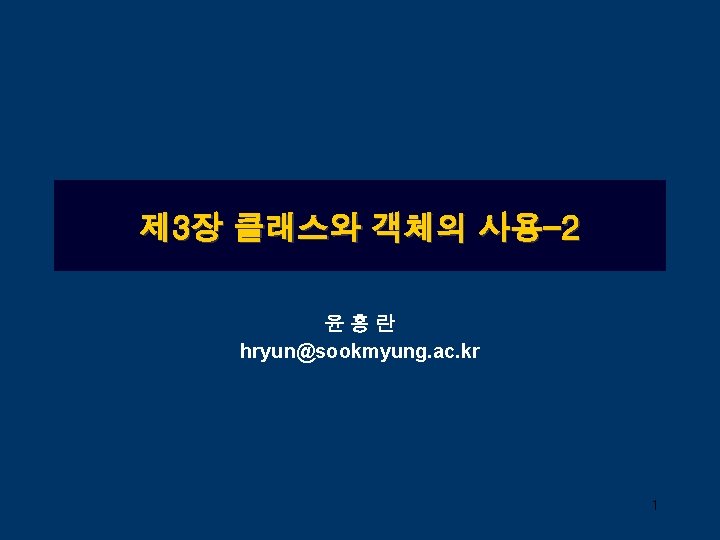
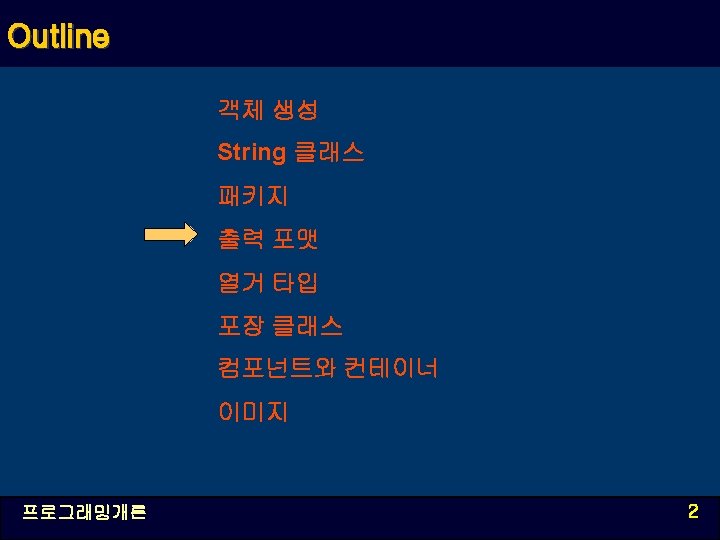
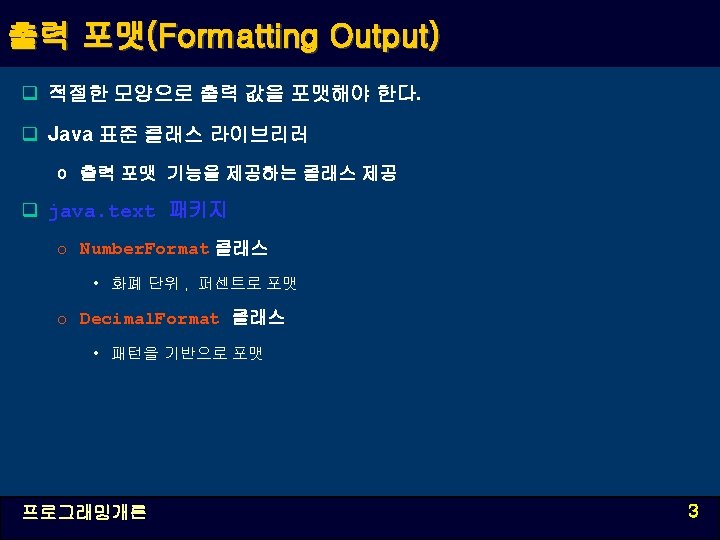
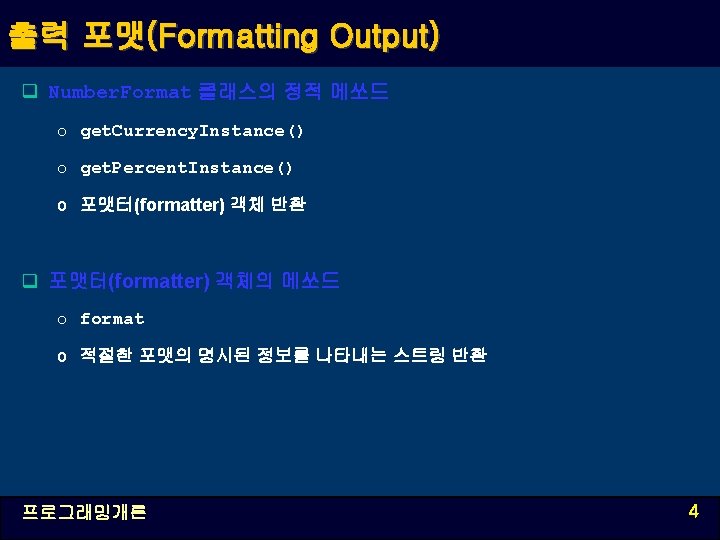
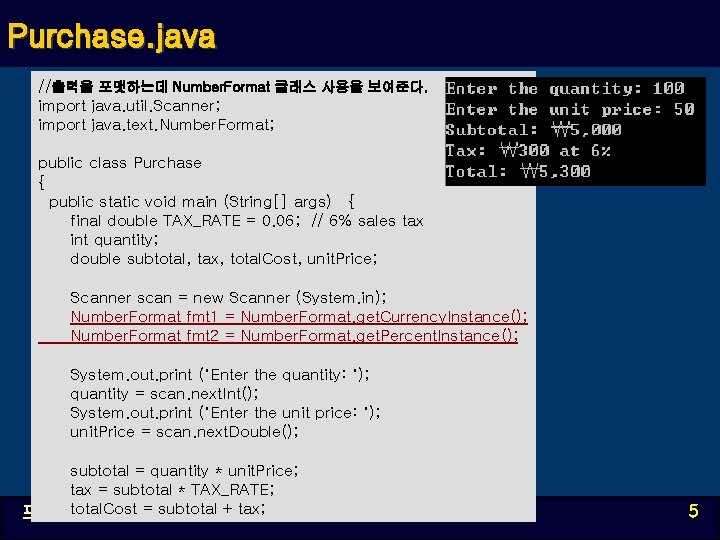
Purchase. java //출력을 포맷하는데 Number. Format 클래스 사용을 보여준다. import java. util. Scanner; import java. text. Number. Format; public class Purchase { public static void main (String[] args) { final double TAX_RATE = 0. 06; // 6% sales tax int quantity; double subtotal, tax, total. Cost, unit. Price; Scanner scan = new Scanner (System. in); Number. Format fmt 1 = Number. Format. get. Currency. Instance(); Number. Format fmt 2 = Number. Format. get. Percent. Instance(); System. out. print ("Enter the quantity: "); quantity = scan. next. Int(); System. out. print ("Enter the unit price: "); unit. Price = scan. next. Double(); subtotal = quantity * unit. Price; tax = subtotal * TAX_RATE; total. Cost = subtotal + tax; 프로그래밍개론 5
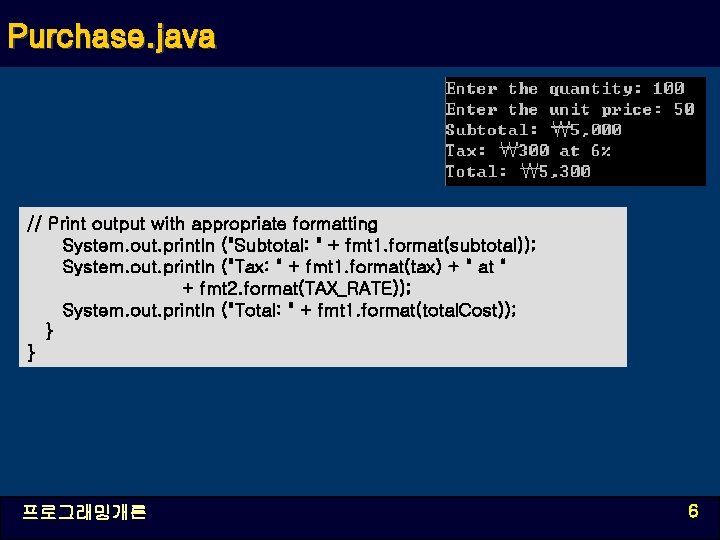
Purchase. java // Print output with appropriate formatting System. out. println ("Subtotal: " + fmt 1. format(subtotal)); System. out. println ("Tax: " + fmt 1. format(tax) + " at " + fmt 2. format(TAX_RATE)); System. out. println ("Total: " + fmt 1. format(total. Cost)); } } 프로그래밍개론 6
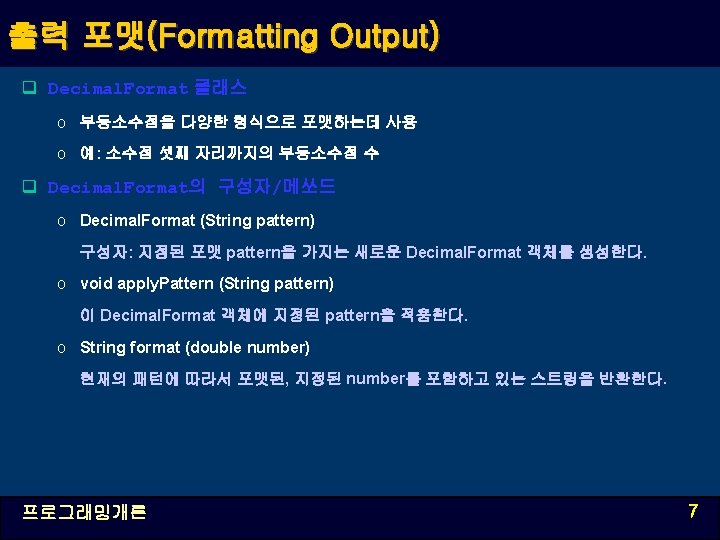
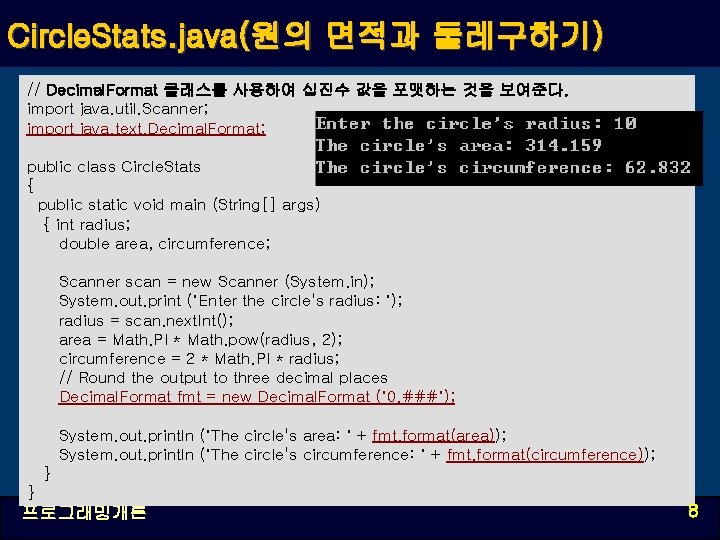
Circle. Stats. java(원의 면적과 둘레구하기) // Decimal. Format 클래스를 사용하여 십진수 값을 포맷하는 것을 보여준다. import java. util. Scanner; import java. text. Decimal. Format; public class Circle. Stats { public static void main (String[] args) { int radius; double area, circumference; Scanner scan = new Scanner (System. in); System. out. print ("Enter the circle's radius: "); radius = scan. next. Int(); area = Math. PI * Math. pow(radius, 2); circumference = 2 * Math. PI * radius; // Round the output to three decimal places Decimal. Format fmt = new Decimal. Format ("0. ###"); System. out. println ("The circle's area: " + fmt. format(area)); System. out. println ("The circle's circumference: " + fmt. format(circumference)); } } 프로그래밍개론 8
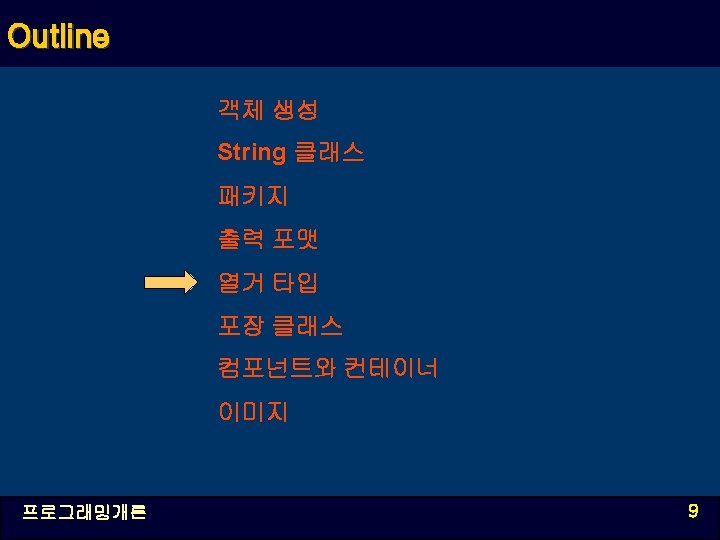
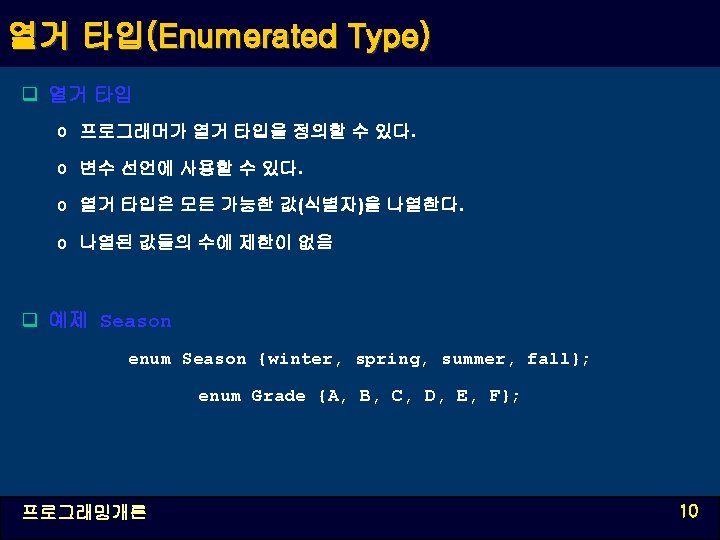
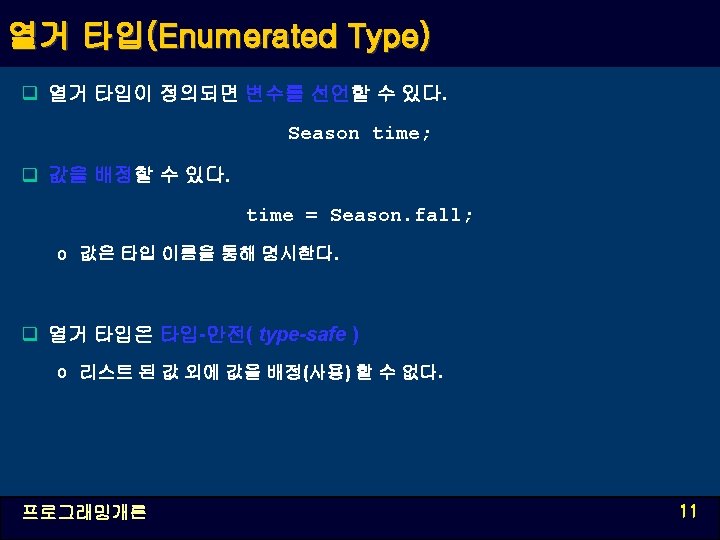
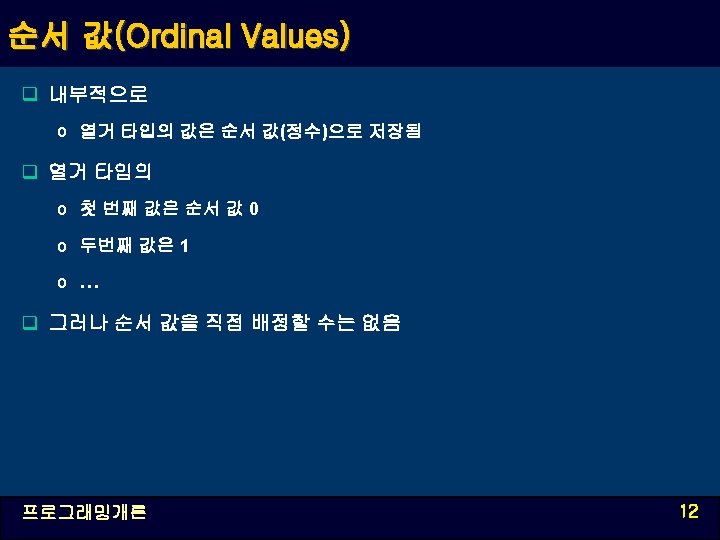
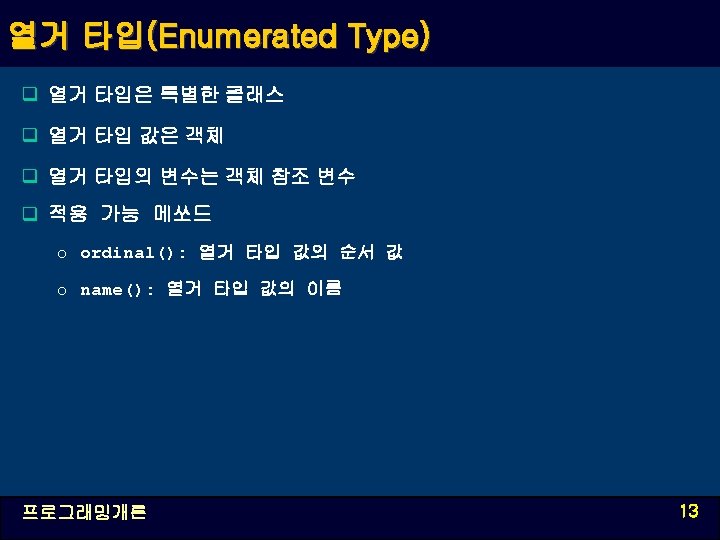
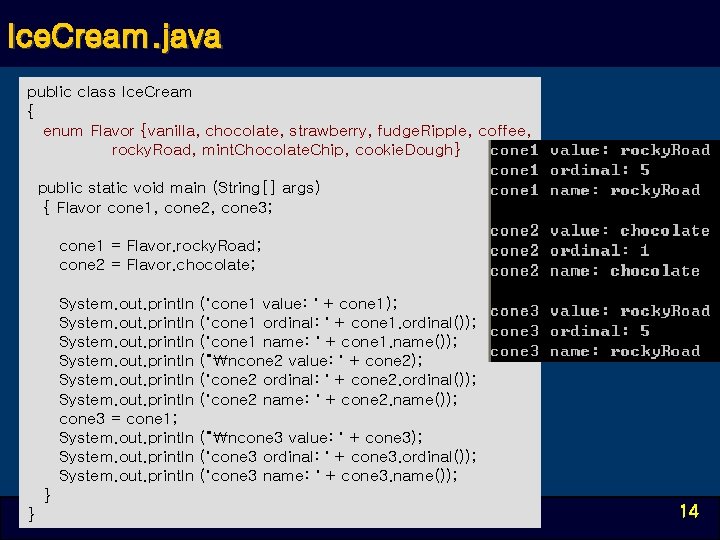
Ice. Cream. java public class Ice. Cream { enum Flavor {vanilla, chocolate, strawberry, fudge. Ripple, coffee, rocky. Road, mint. Chocolate. Chip, cookie. Dough} public static void main (String[] args) { Flavor cone 1, cone 2, cone 3; cone 1 = Flavor. rocky. Road; cone 2 = Flavor. chocolate; System. out. println cone 3 = cone 1; System. out. println } 프로그래밍개론 } ("cone 1 value: " + cone 1); ("cone 1 ordinal: " + cone 1. ordinal()); ("cone 1 name: " + cone 1. name()); (“ncone 2 value: " + cone 2); ("cone 2 ordinal: " + cone 2. ordinal()); ("cone 2 name: " + cone 2. name()); (“ncone 3 value: " + cone 3); ("cone 3 ordinal: " + cone 3. ordinal()); ("cone 3 name: " + cone 3. name()); 14
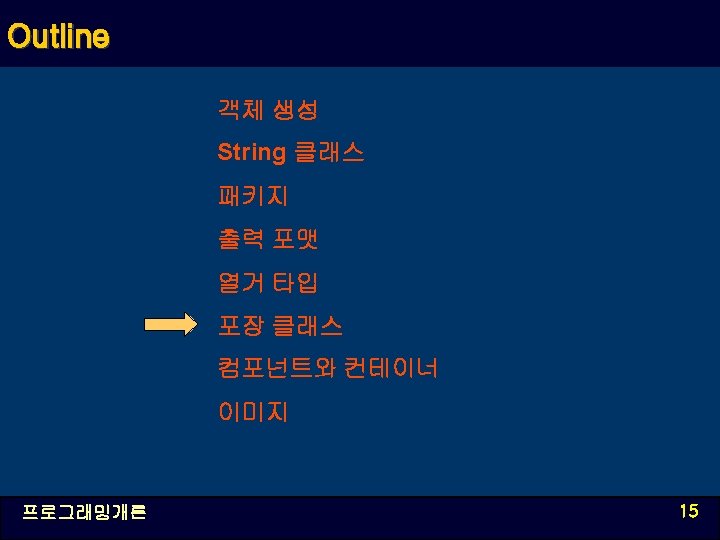
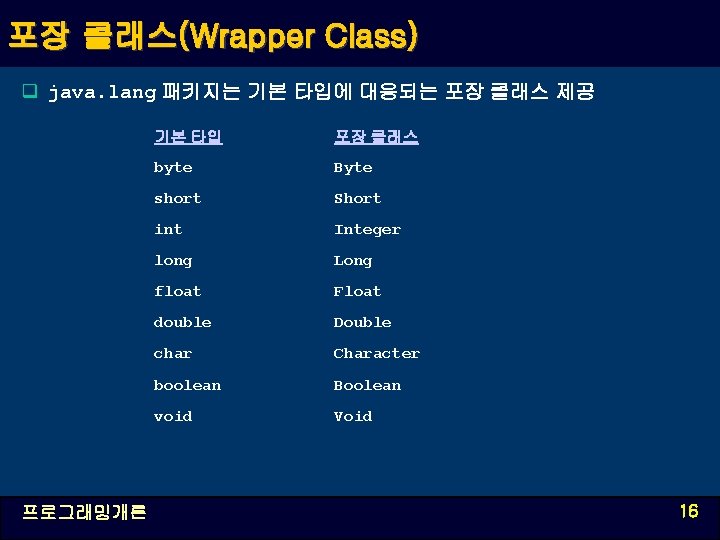
포장 클래스(Wrapper Class) q java. lang 패키지는 기본 타입에 대응되는 포장 클래스 제공 프로그래밍개론 기본 타입 포장 클래스 byte Byte short Short int Integer long Long float Float double Double char Character boolean Boolean void Void 16
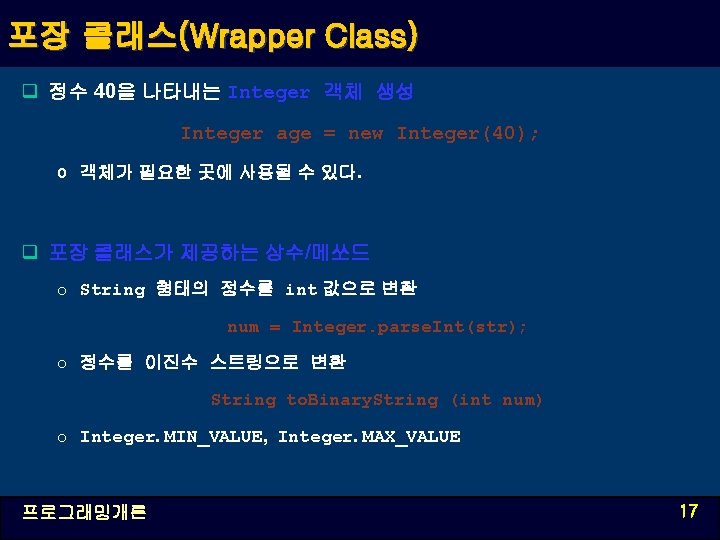
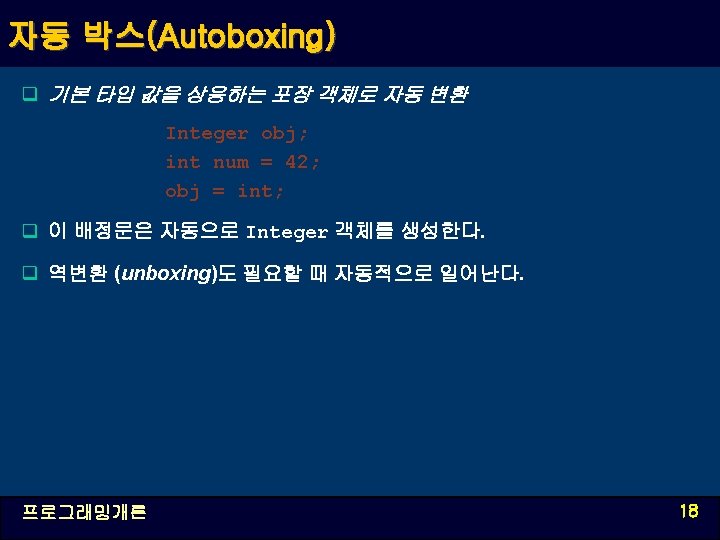
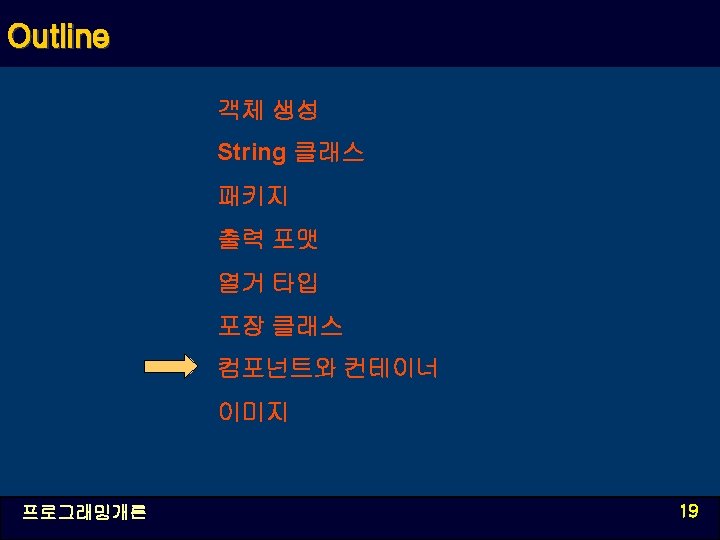
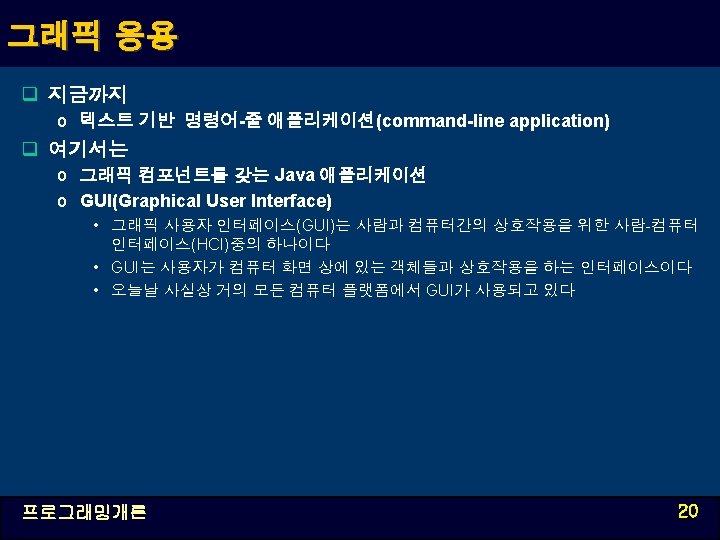
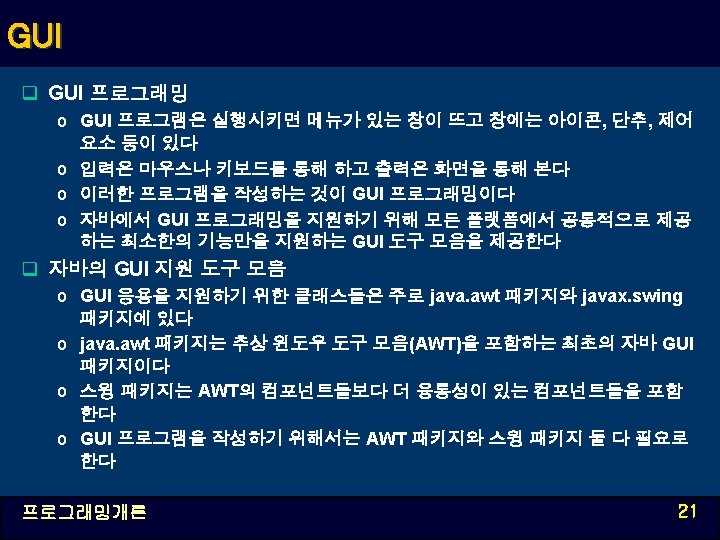
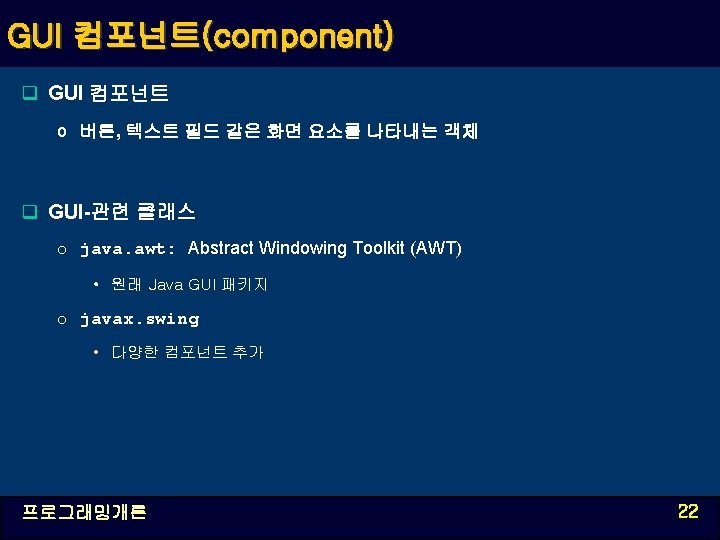
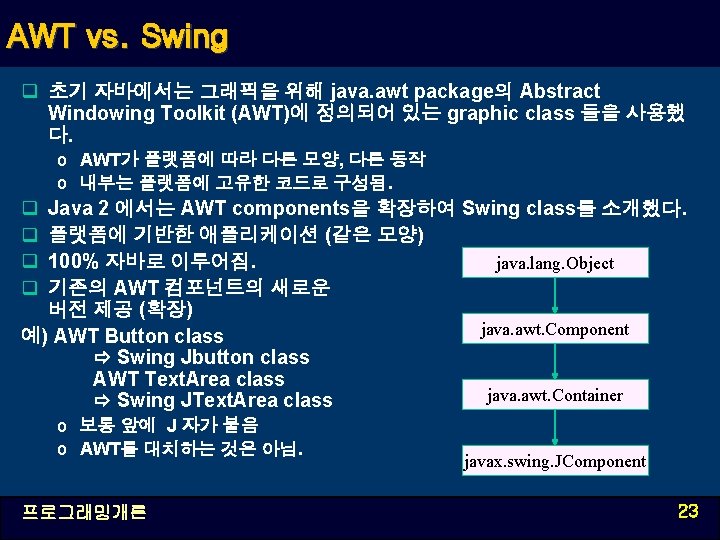
AWT vs. Swing q 초기 자바에서는 그래픽을 위해 java. awt package의 Abstract Windowing Toolkit (AWT)에 정의되어 있는 graphic class 들을 사용했 다. o AWT가 플랫폼에 따라 다른 모양, 다른 동작 o 내부는 플랫폼에 고유한 코드로 구성됨. q q Java 2 에서는 AWT components을 확장하여 Swing class를 소개했다. 플랫폼에 기반한 애플리케이션 (같은 모양) 100% 자바로 이루어짐. java. lang. Object 기존의 AWT 컴포넌트의 새로운 버전 제공 (확장) java. awt. Component 예) AWT Button class Swing Jbutton class AWT Text. Area class java. awt. Container Swing JText. Area class o 보통 앞에 J 자가 붙음 o AWT를 대치하는 것은 아님. 프로그래밍개론 javax. swing. JComponent 23
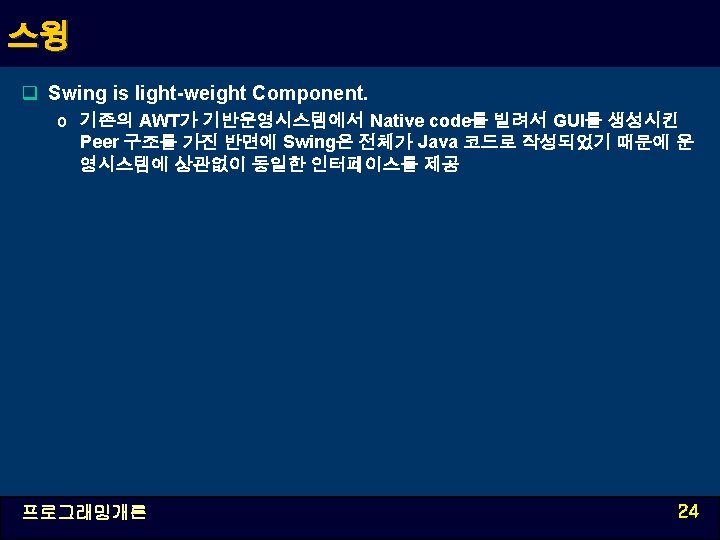
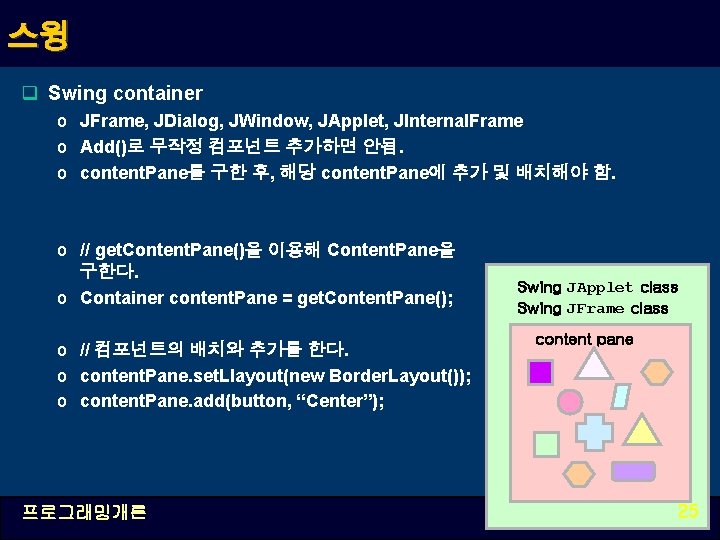
스윙 q Swing container o JFrame, JDialog, JWindow, JApplet, JInternal. Frame o Add()로 무작정 컴포넌트 추가하면 안됨. o content. Pane를 구한 후, 해당 content. Pane에 추가 및 배치해야 함. o // get. Content. Pane()을 이용해 Content. Pane을 구한다. o Container content. Pane = get. Content. Pane(); o // 컴포넌트의 배치와 추가를 한다. o content. Pane. set. Llayout(new Border. Layout()); o content. Pane. add(button, “Center”); 프로그래밍개론 Swing JApplet class Swing JFrame class content pane 25
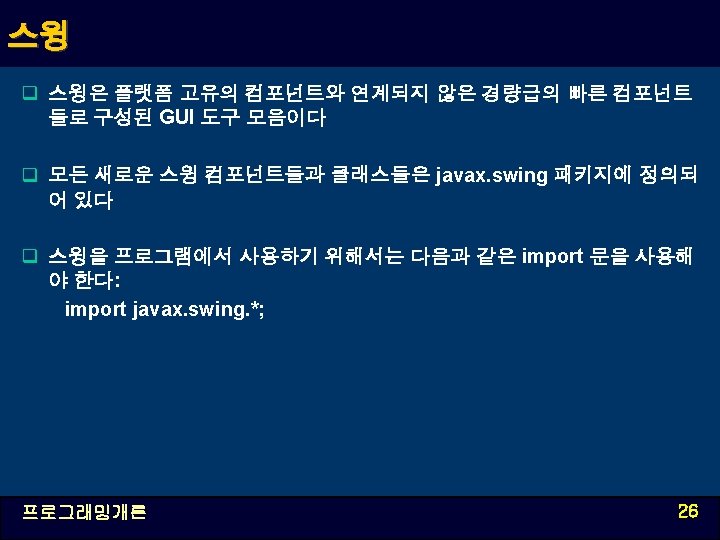
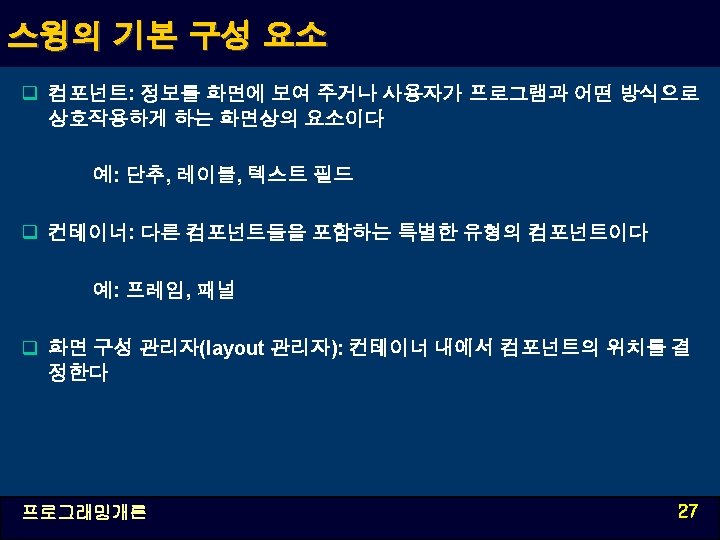
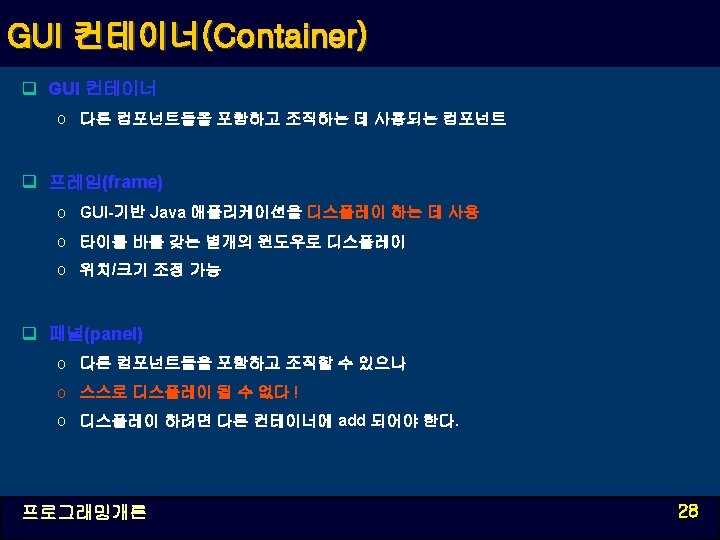
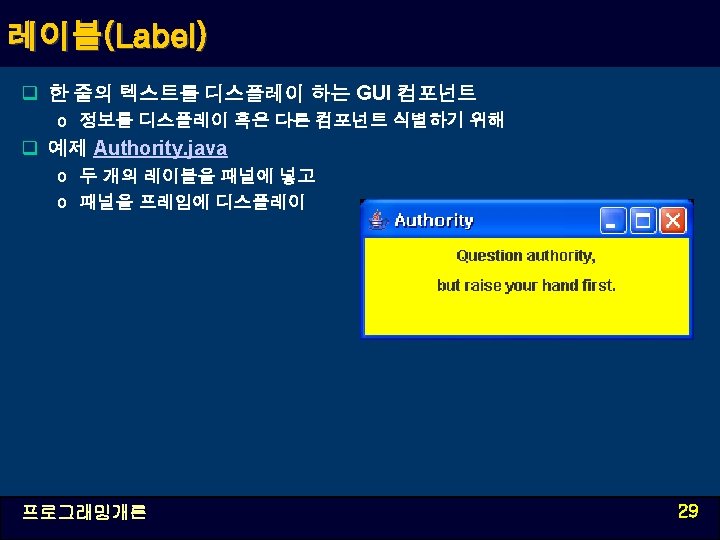
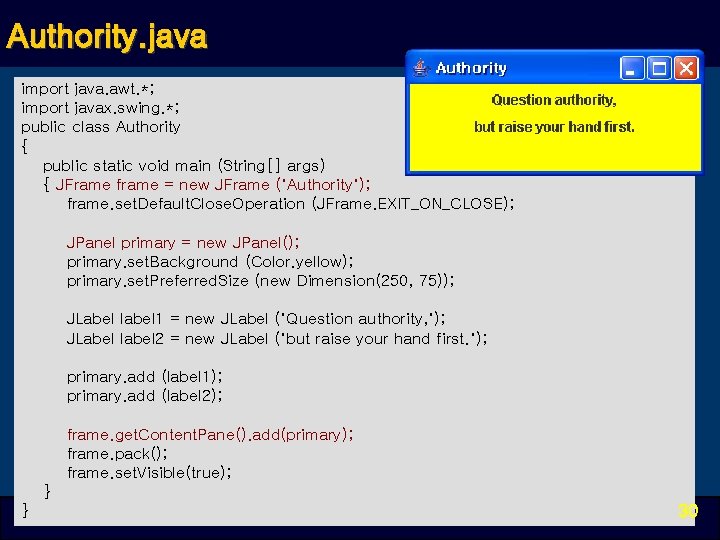
Authority. java import java. awt. *; import javax. swing. *; public class Authority { public static void main (String[] args) { JFrame frame = new JFrame ("Authority"); frame. set. Default. Close. Operation (JFrame. EXIT_ON_CLOSE); JPanel primary = new JPanel(); primary. set. Background (Color. yellow); primary. set. Preferred. Size (new Dimension(250, 75)); JLabel label 1 = new JLabel ("Question authority, "); JLabel label 2 = new JLabel ("but raise your hand first. "); primary. add (label 1); primary. add (label 2); frame. get. Content. Pane(). add(primary); frame. pack(); frame. set. Visible(true); } } 프로그래밍개론 30
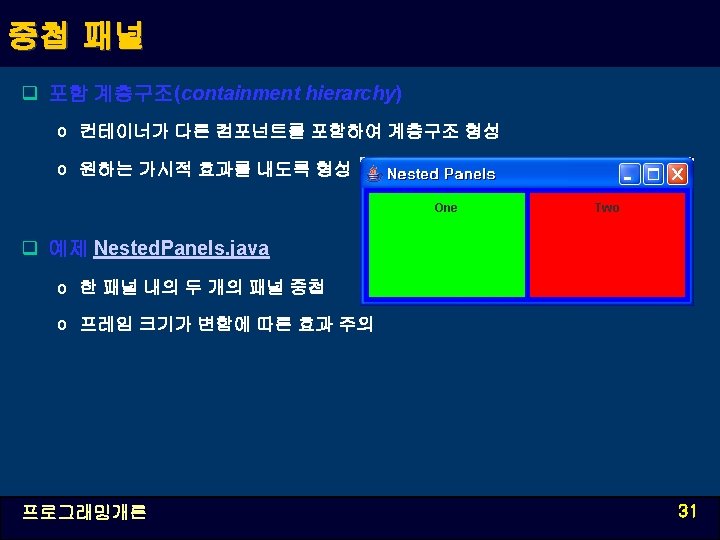
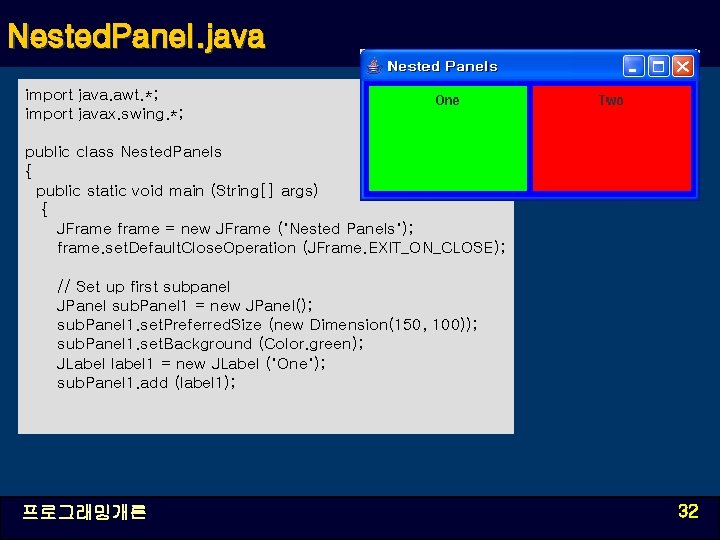
Nested. Panel. java import java. awt. *; import javax. swing. *; public class Nested. Panels { public static void main (String[] args) { JFrame frame = new JFrame ("Nested Panels"); frame. set. Default. Close. Operation (JFrame. EXIT_ON_CLOSE); // Set up first subpanel JPanel sub. Panel 1 = new JPanel(); sub. Panel 1. set. Preferred. Size (new Dimension(150, 100)); sub. Panel 1. set. Background (Color. green); JLabel label 1 = new JLabel ("One"); sub. Panel 1. add (label 1); 프로그래밍개론 32
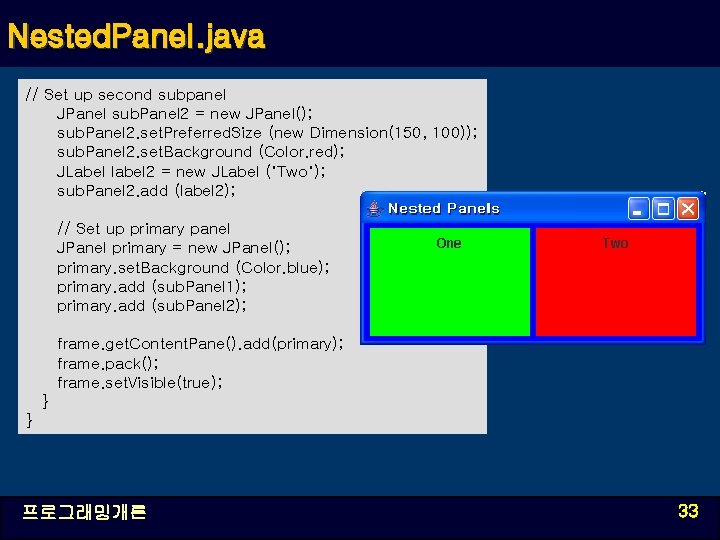
Nested. Panel. java // Set up second subpanel JPanel sub. Panel 2 = new JPanel(); sub. Panel 2. set. Preferred. Size (new Dimension(150, 100)); sub. Panel 2. set. Background (Color. red); JLabel label 2 = new JLabel ("Two"); sub. Panel 2. add (label 2); // Set up primary panel JPanel primary = new JPanel(); primary. set. Background (Color. blue); primary. add (sub. Panel 1); primary. add (sub. Panel 2); frame. get. Content. Pane(). add(primary); frame. pack(); frame. set. Visible(true); } } 프로그래밍개론 33
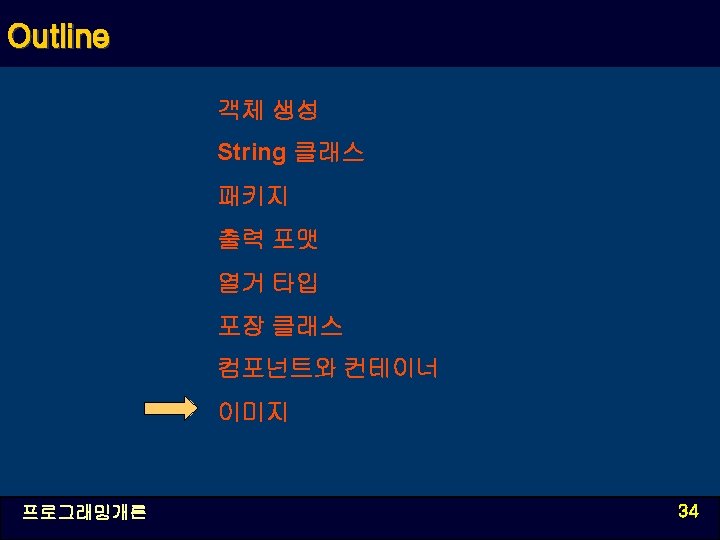
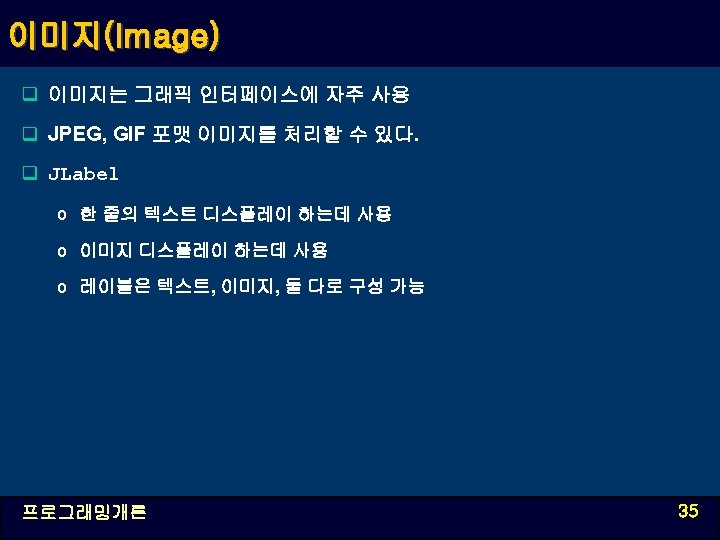
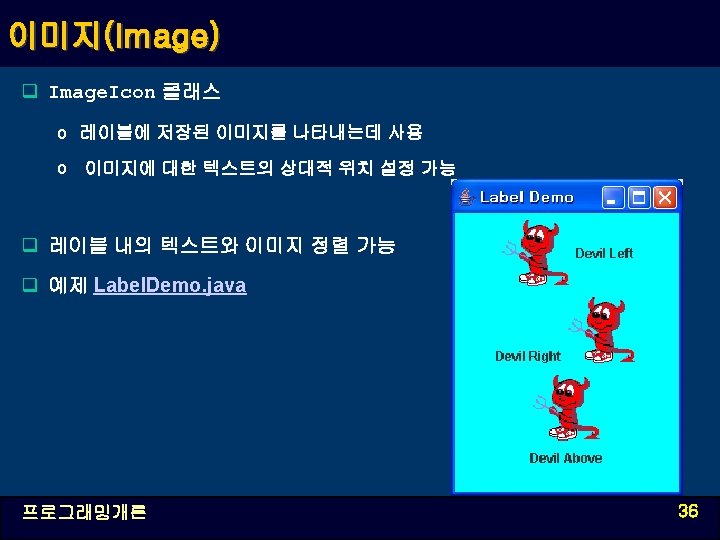
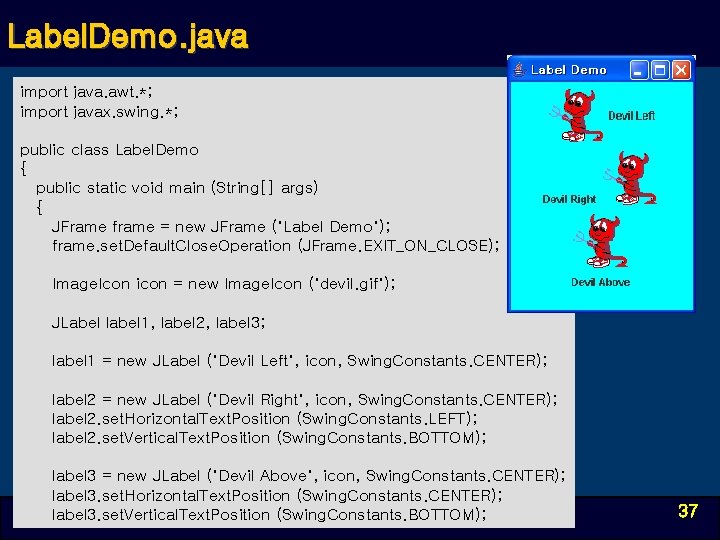
Label. Demo. java import java. awt. *; import javax. swing. *; public class Label. Demo { public static void main (String[] args) { JFrame frame = new JFrame ("Label Demo"); frame. set. Default. Close. Operation (JFrame. EXIT_ON_CLOSE); Image. Icon icon = new Image. Icon ("devil. gif"); JLabel label 1, label 2, label 3; label 1 = new JLabel ("Devil Left", icon, Swing. Constants. CENTER); label 2 = new JLabel ("Devil Right", icon, Swing. Constants. CENTER); label 2. set. Horizontal. Text. Position (Swing. Constants. LEFT); label 2. set. Vertical. Text. Position (Swing. Constants. BOTTOM); label 3 = new JLabel ("Devil Above", icon, Swing. Constants. CENTER); label 3. set. Horizontal. Text. Position (Swing. Constants. CENTER); 프로그래밍개론 label 3. set. Vertical. Text. Position (Swing. Constants. BOTTOM); 37
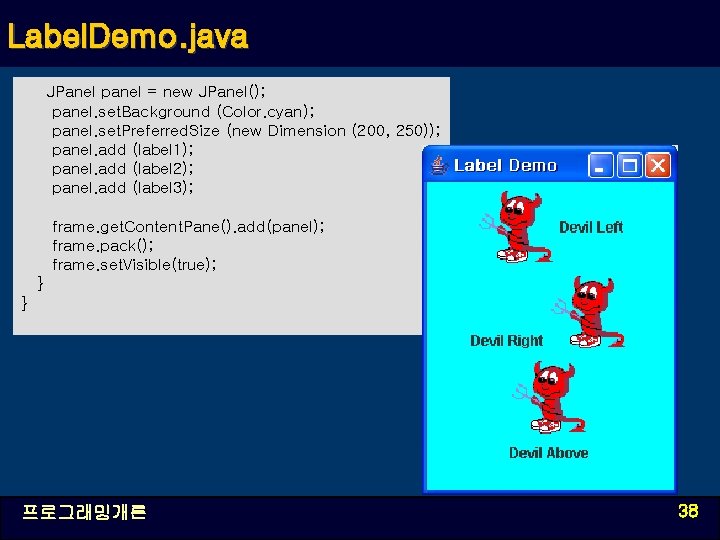
Label. Demo. java JPanel panel = new JPanel(); panel. set. Background (Color. cyan); panel. set. Preferred. Size (new Dimension (200, 250)); panel. add (label 1); panel. add (label 2); panel. add (label 3); frame. get. Content. Pane(). add(panel); frame. pack(); frame. set. Visible(true); } } 프로그래밍개론 38
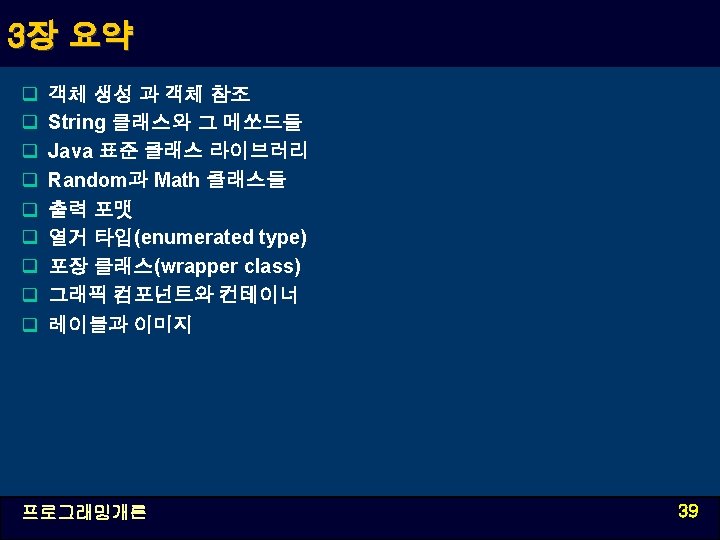
- Slides: 39