Java Collections and Generics 1 Collections in java
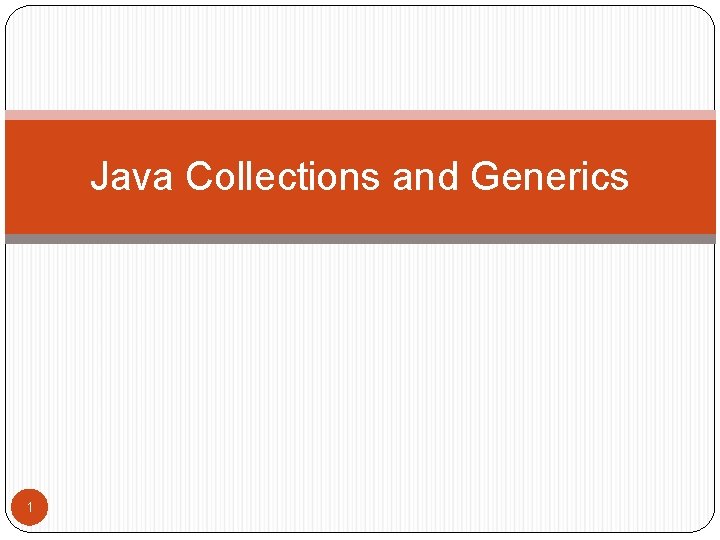
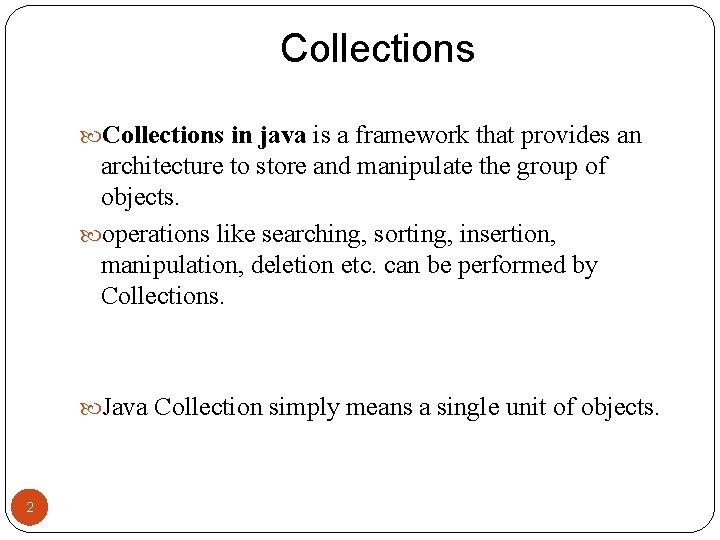
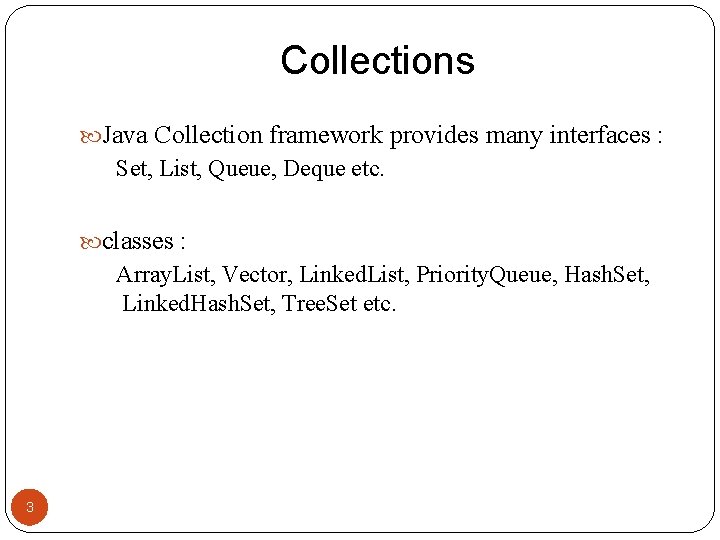
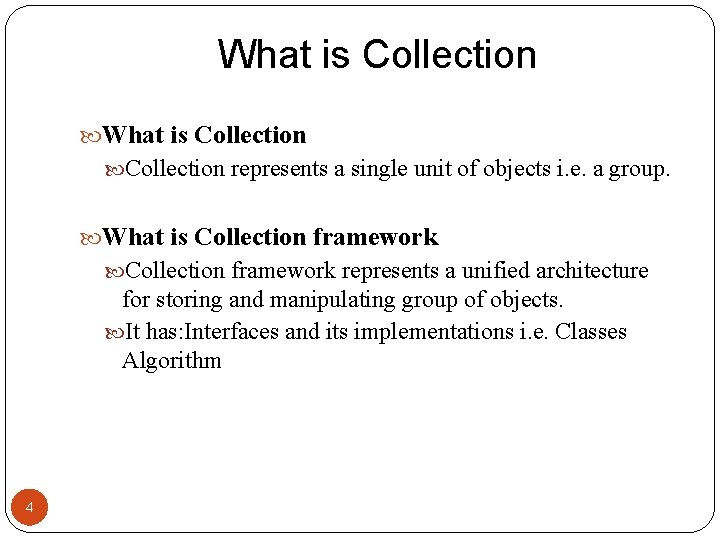
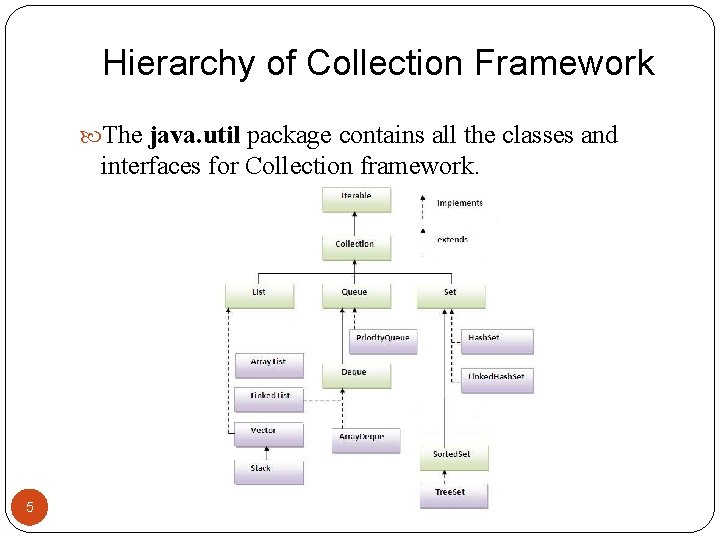
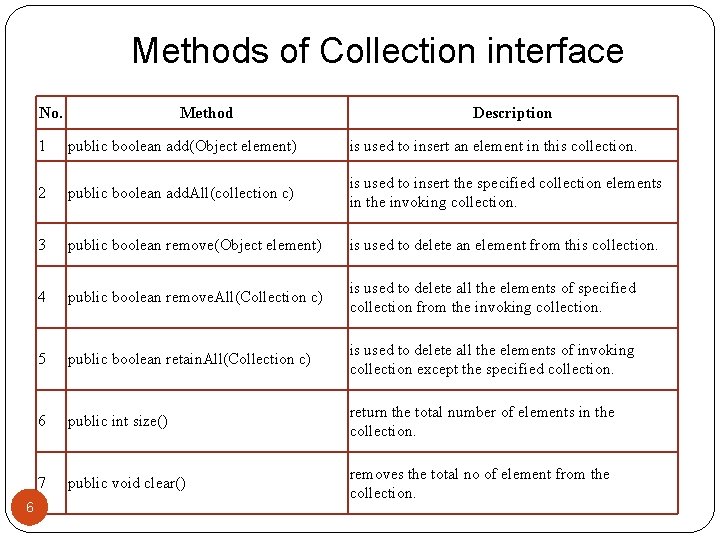
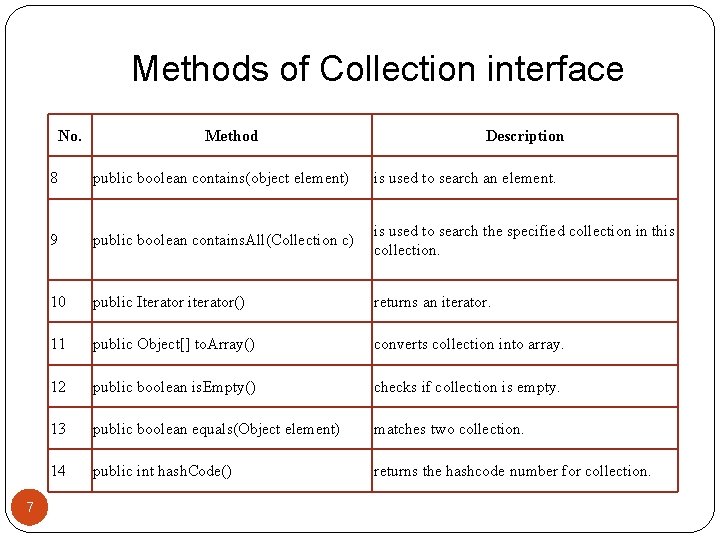
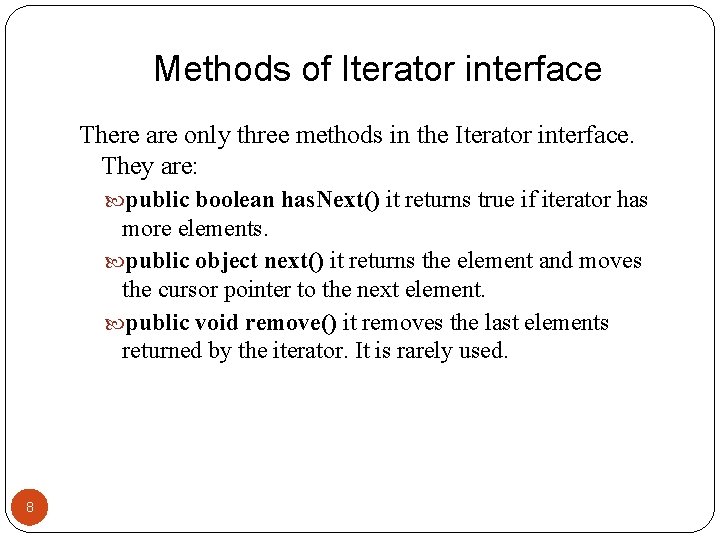
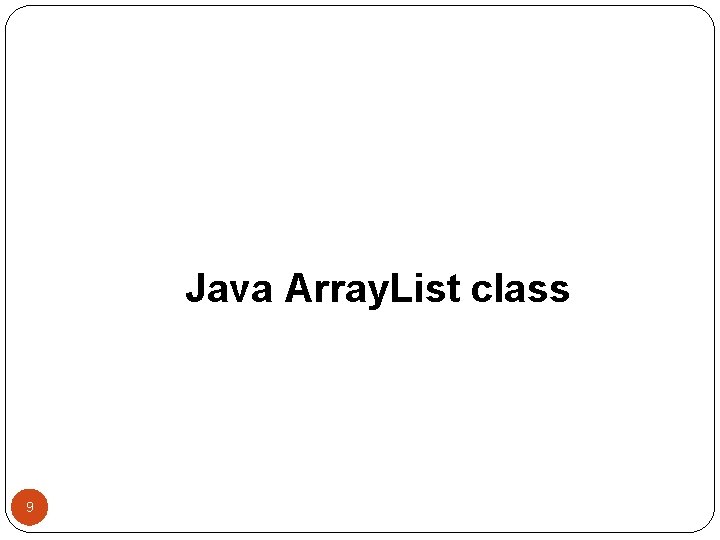
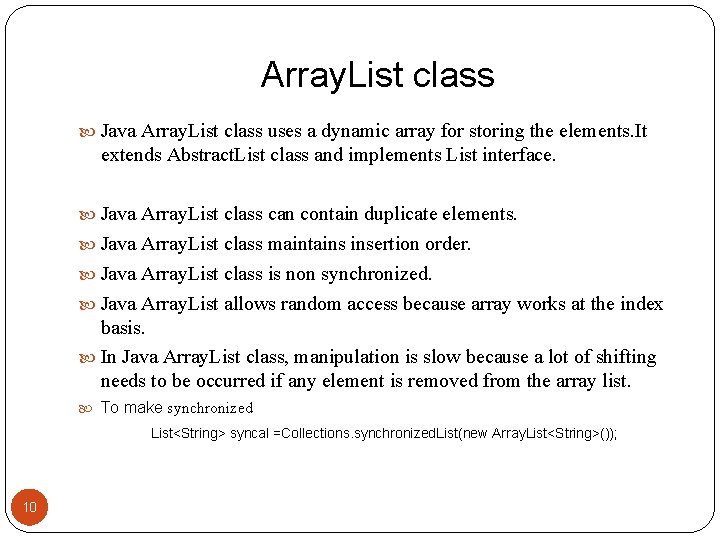
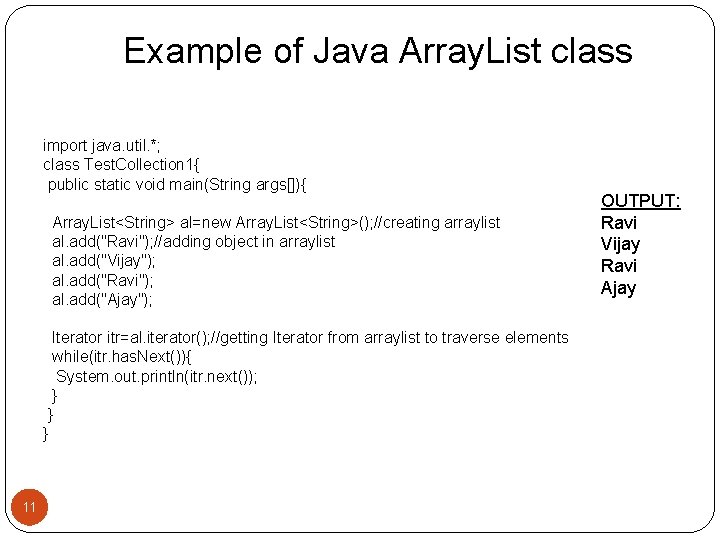
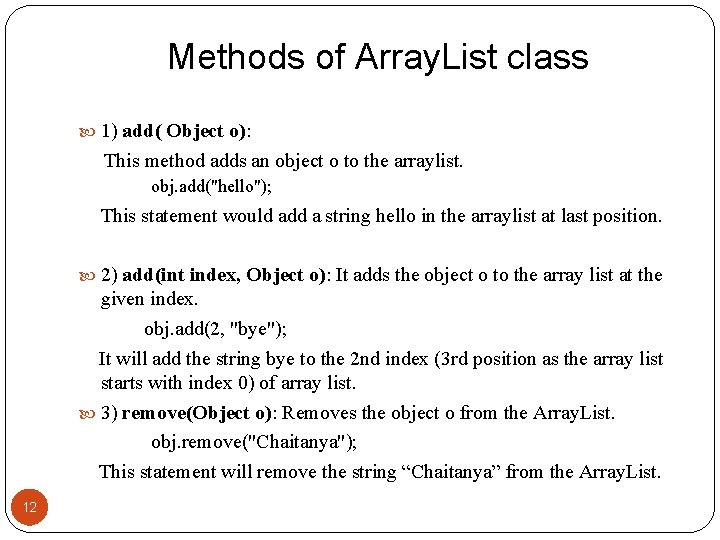
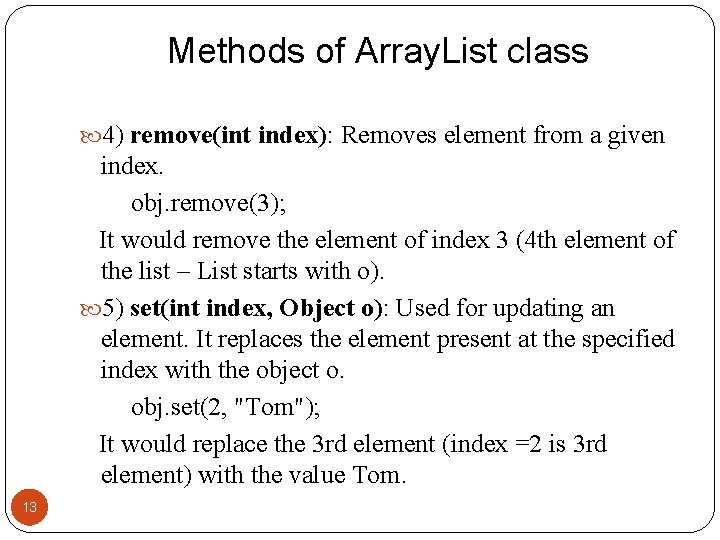
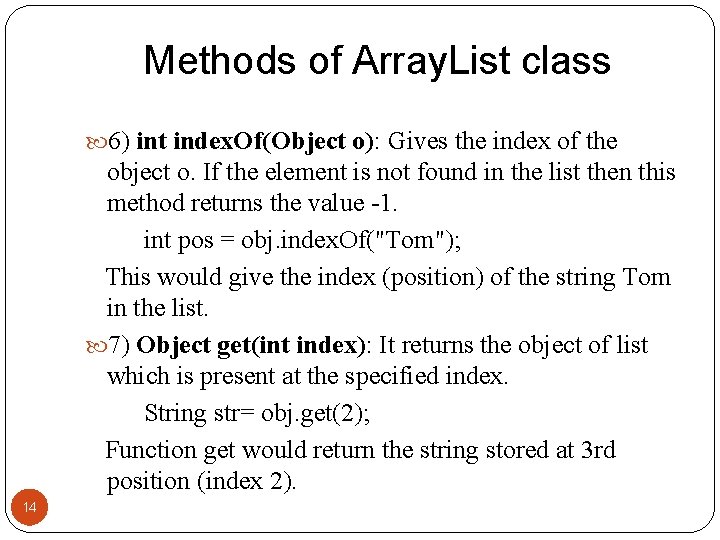
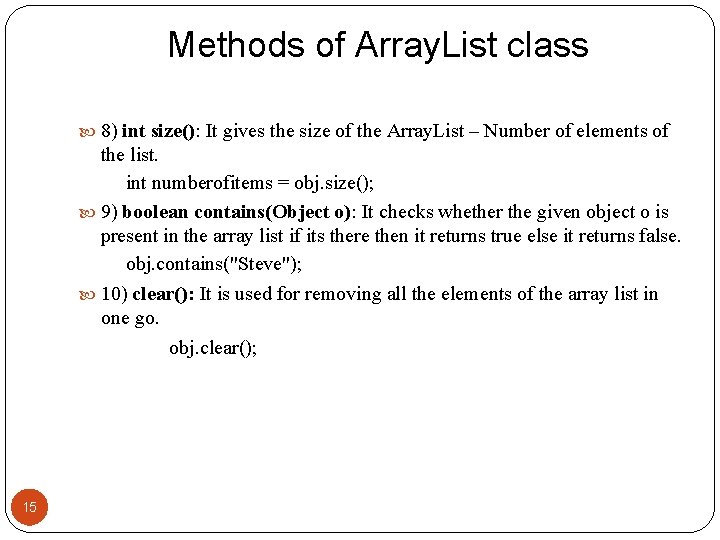
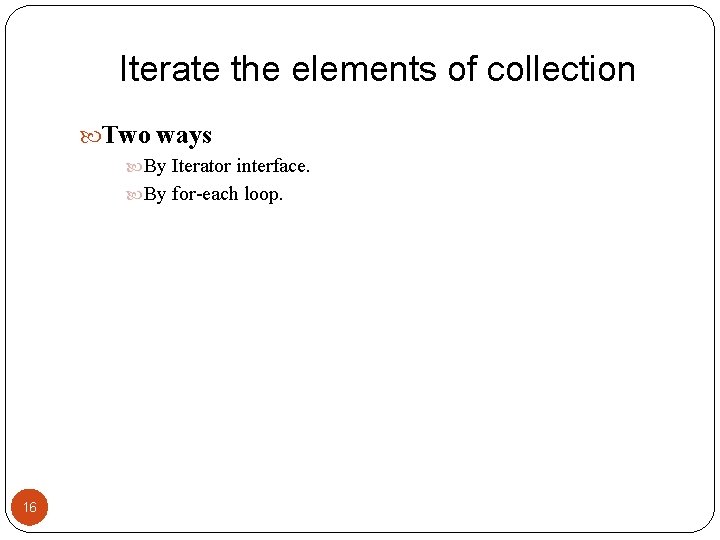
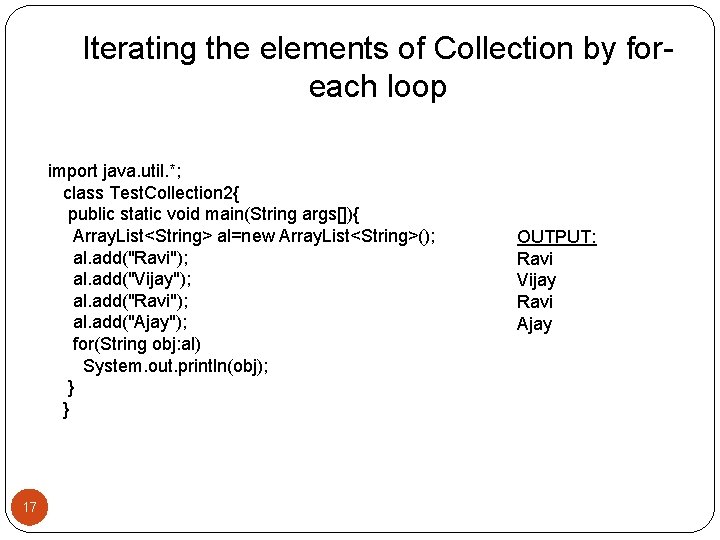
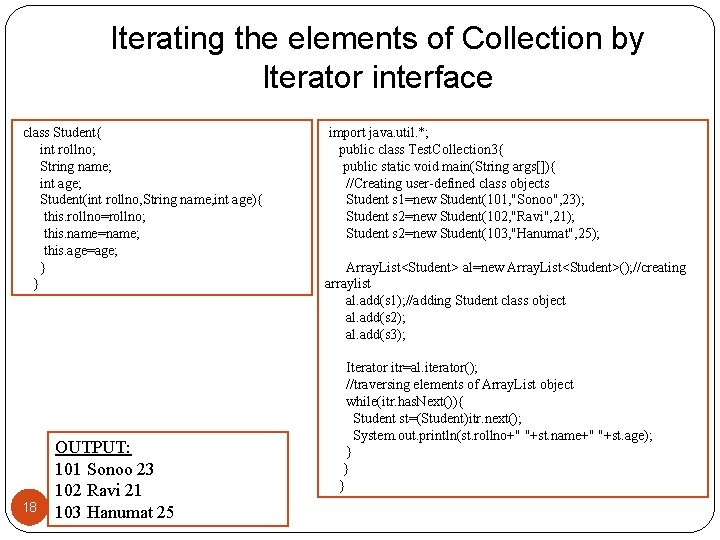
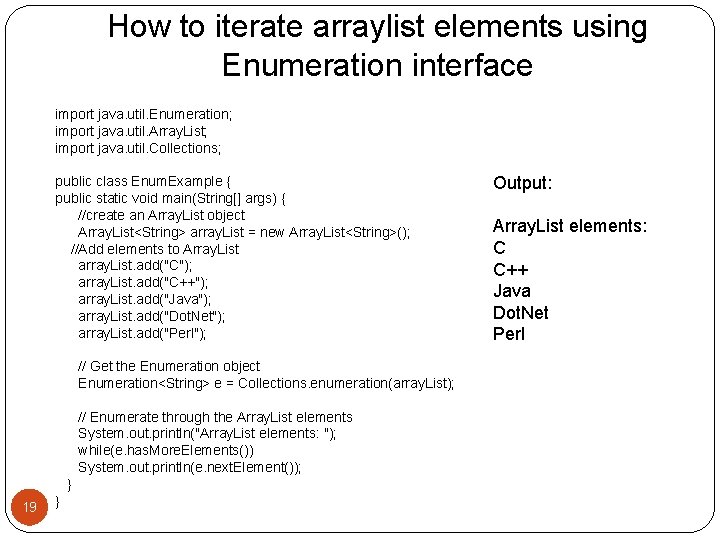
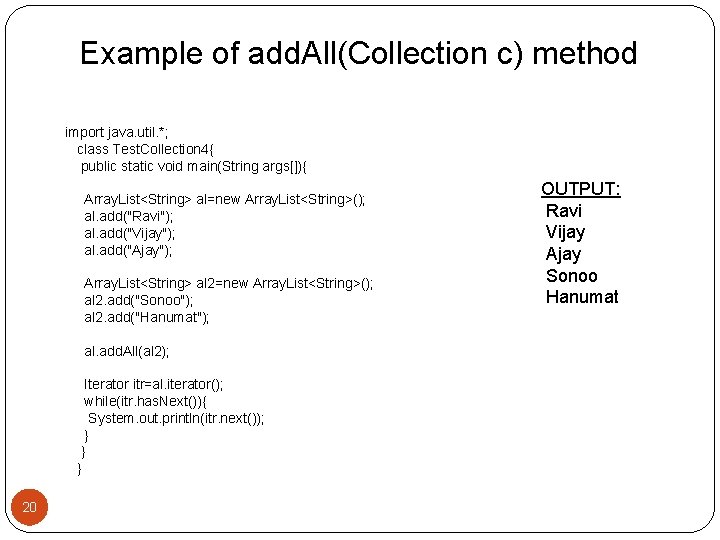
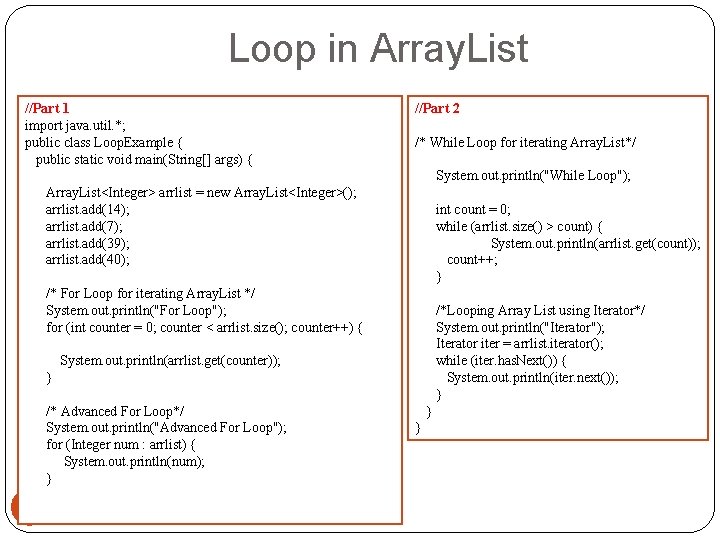
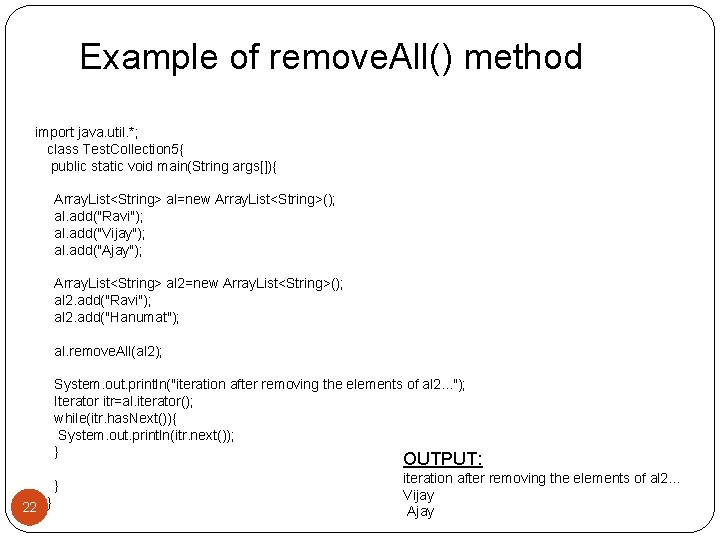
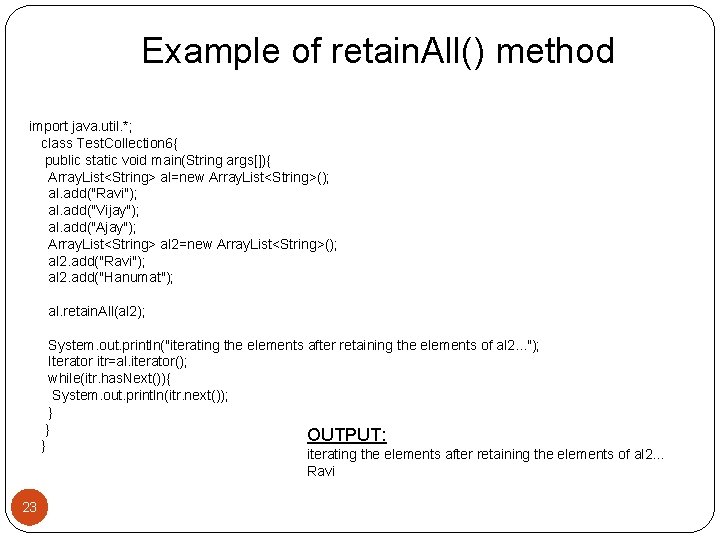
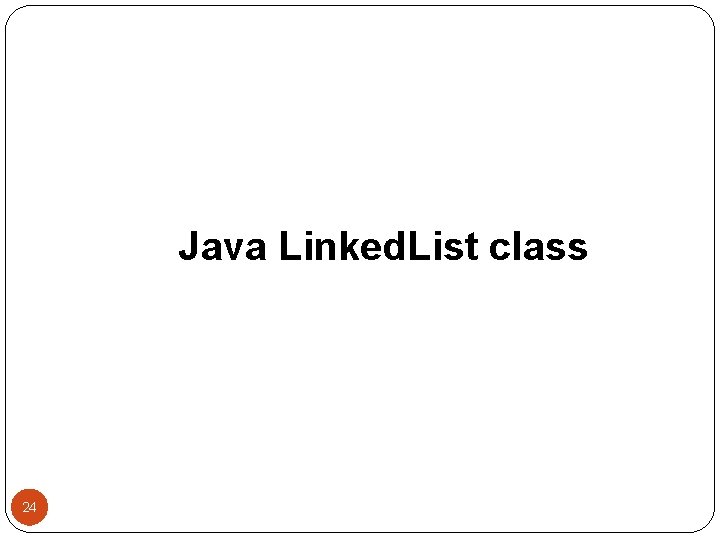
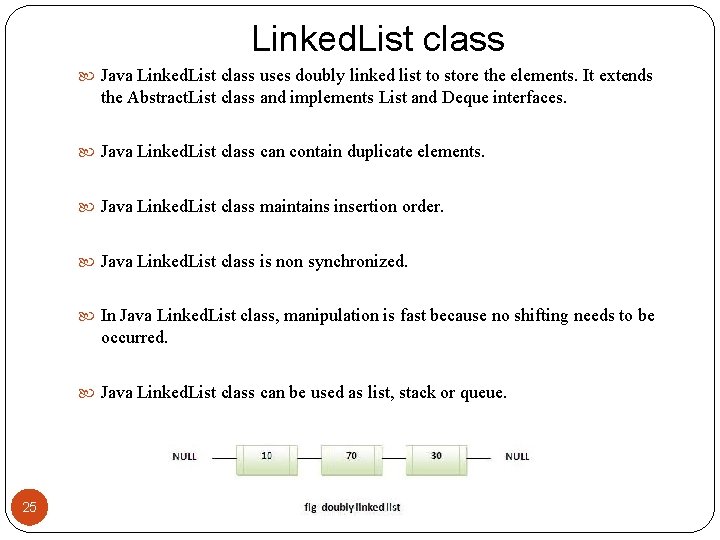
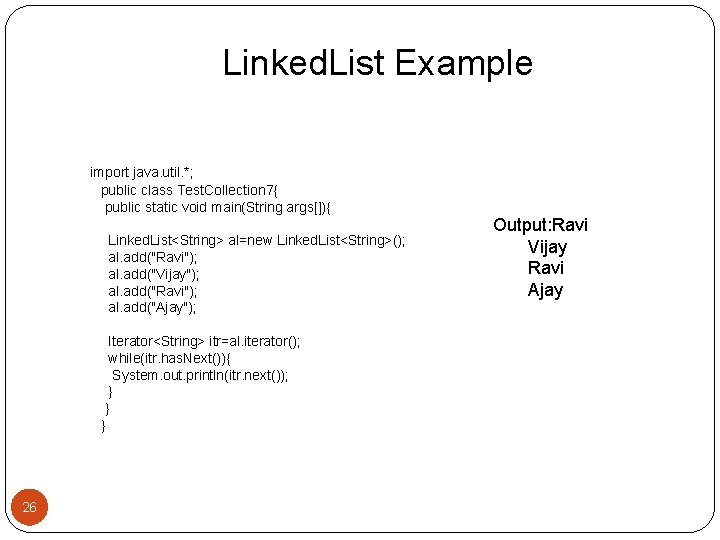
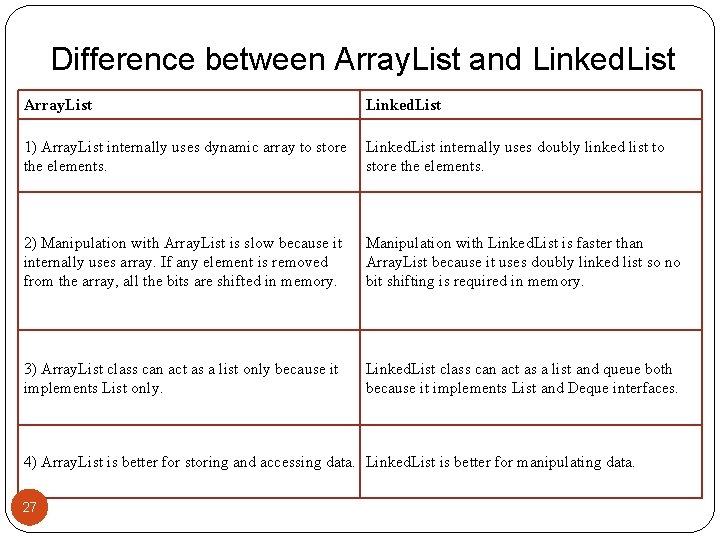
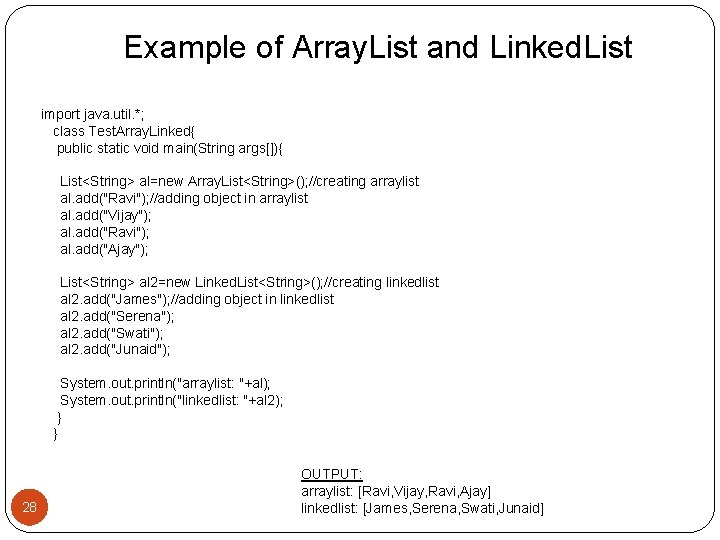
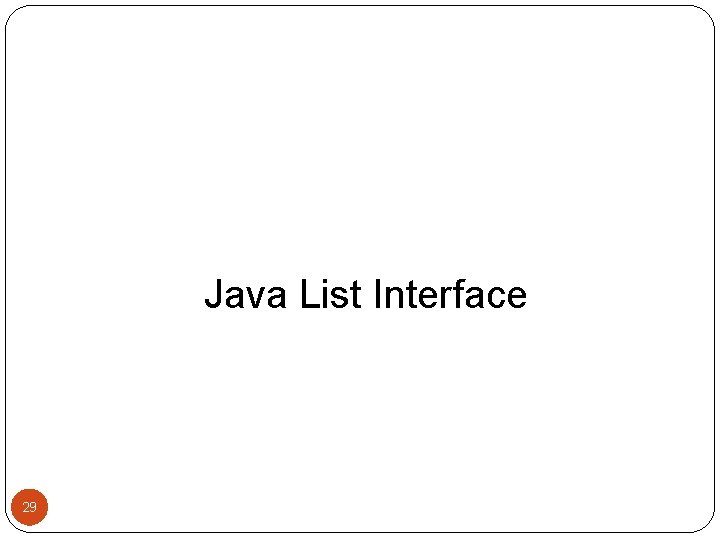
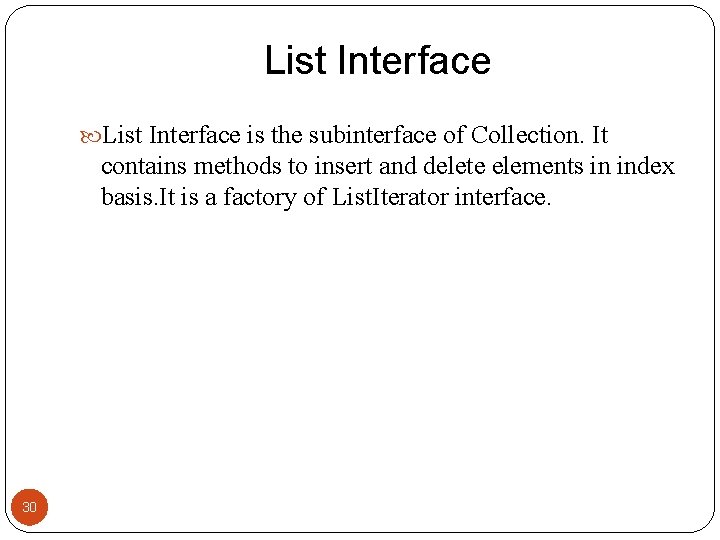
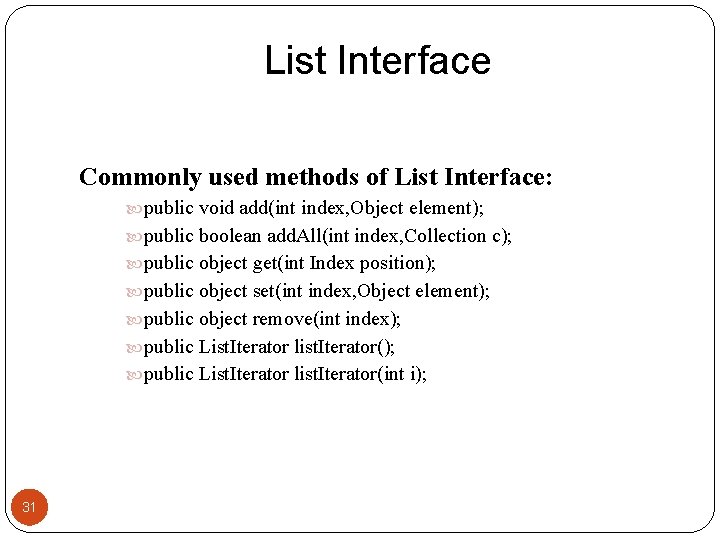
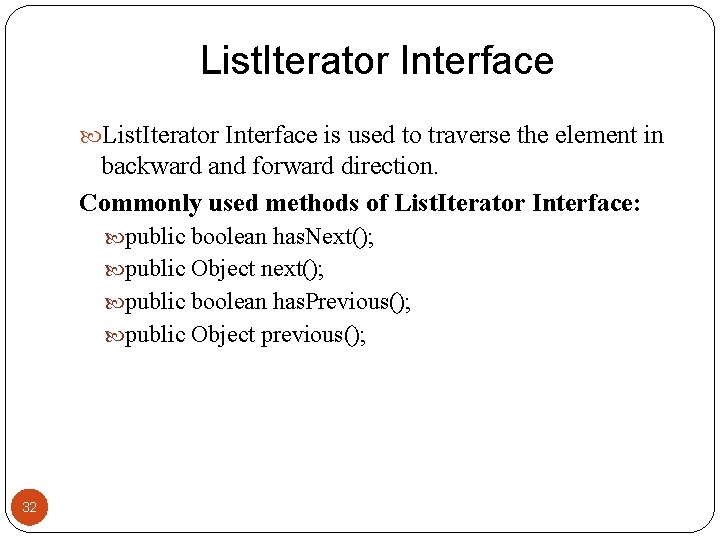
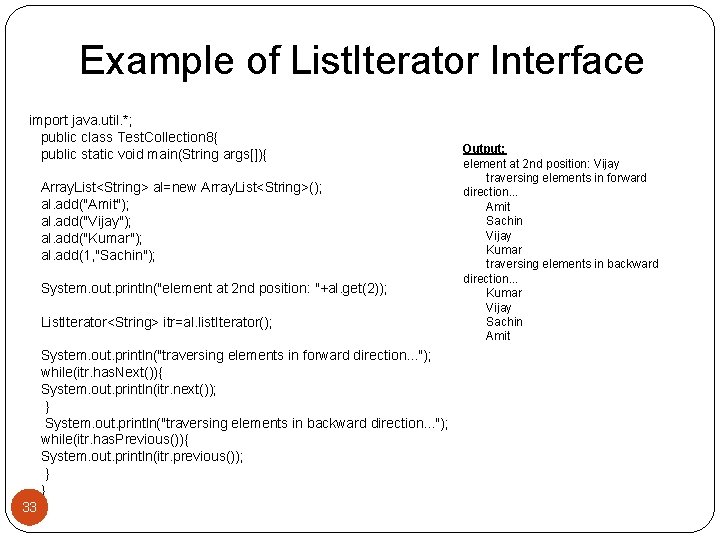
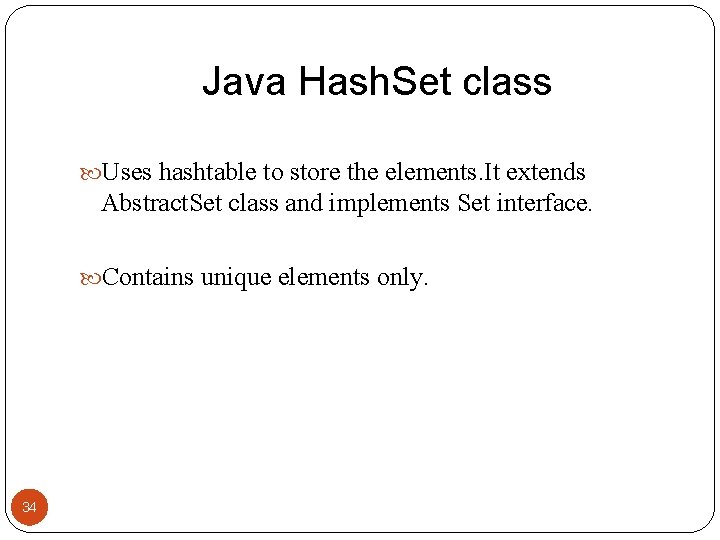
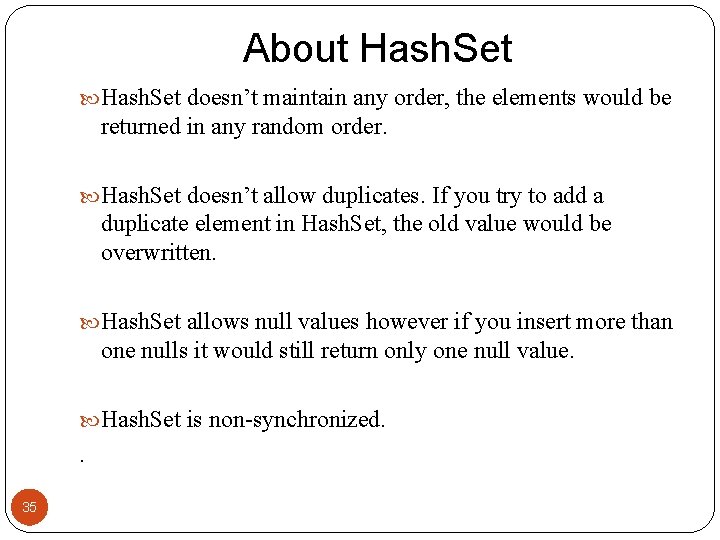
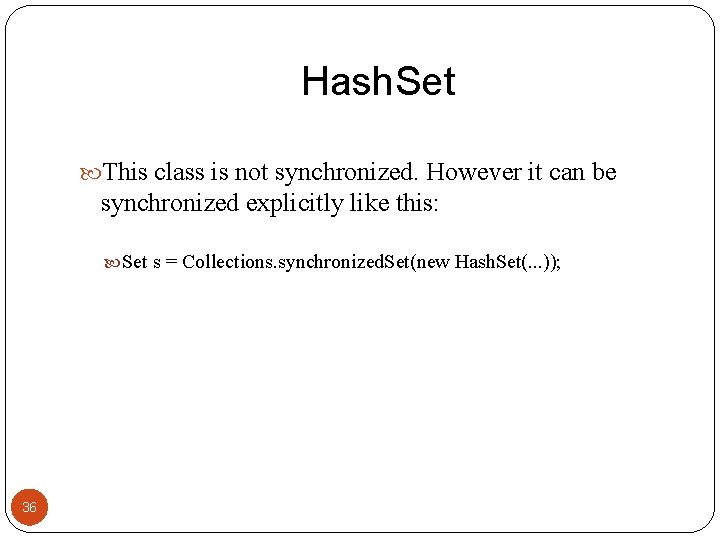
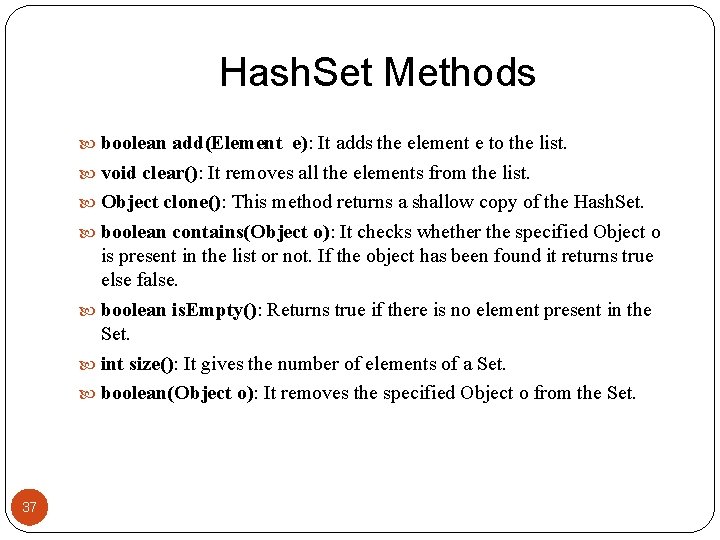
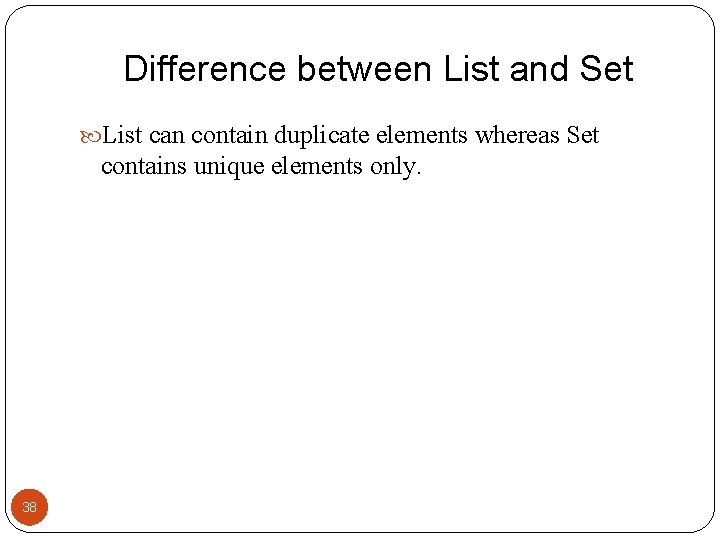
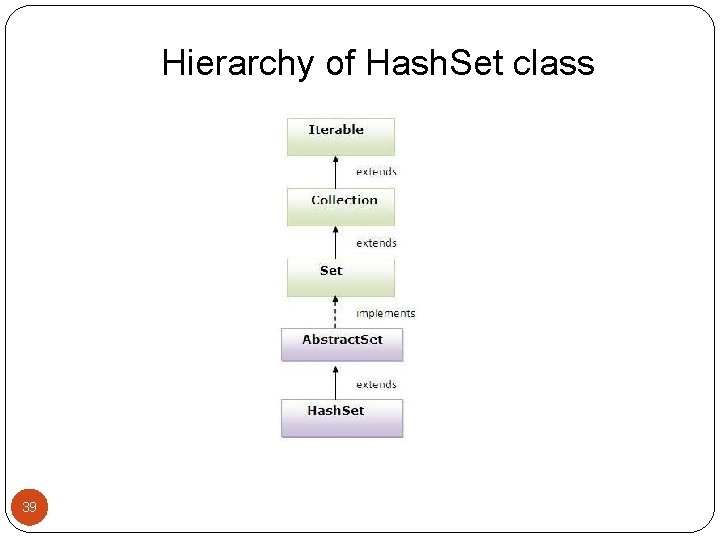
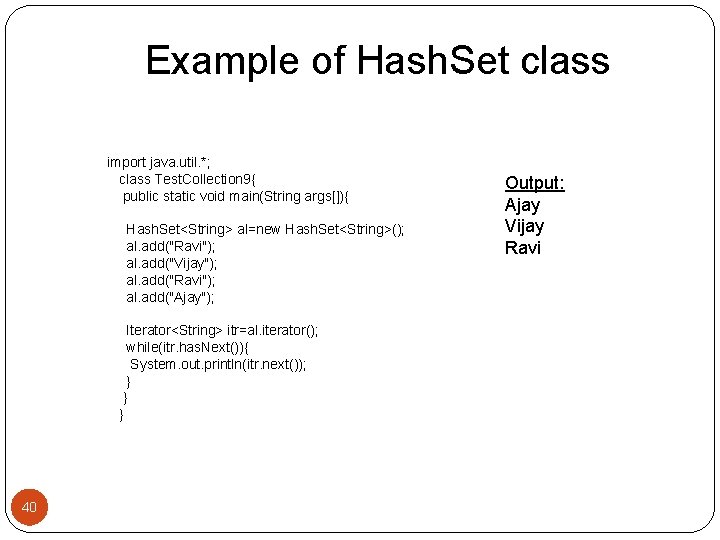
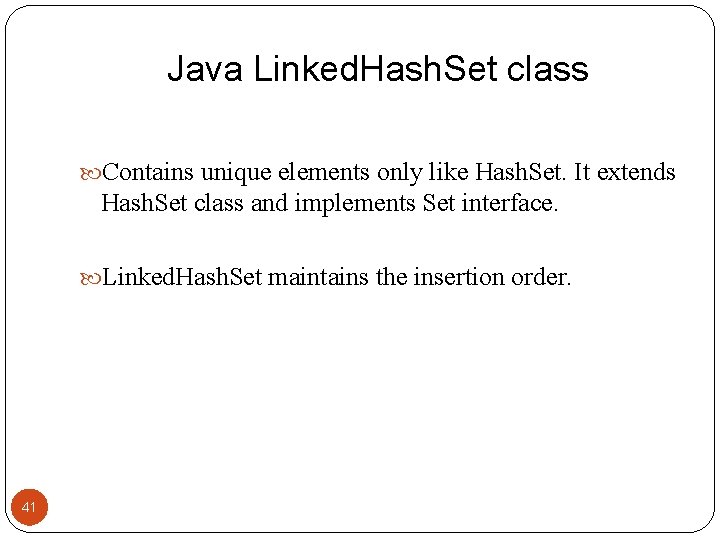
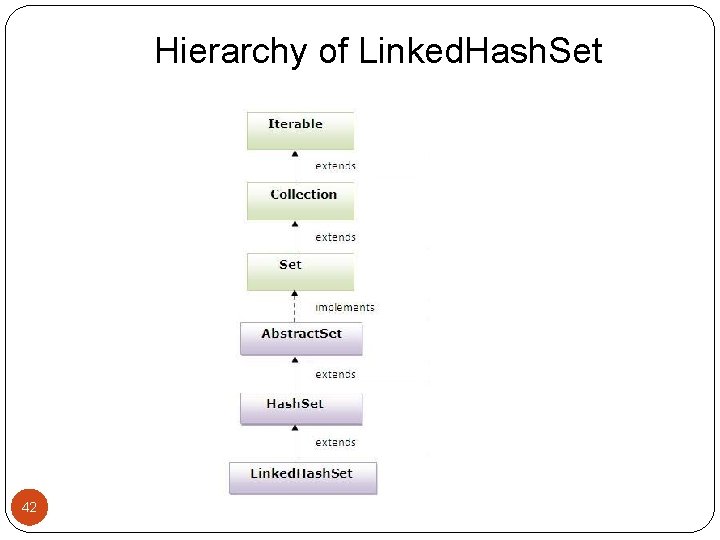
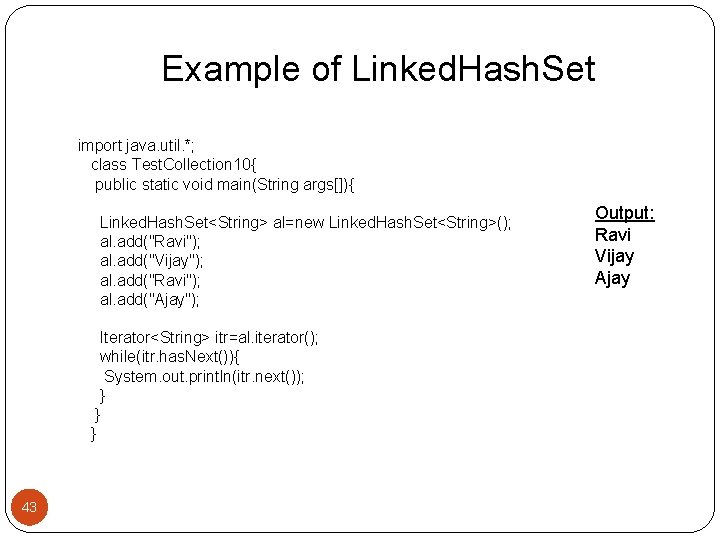
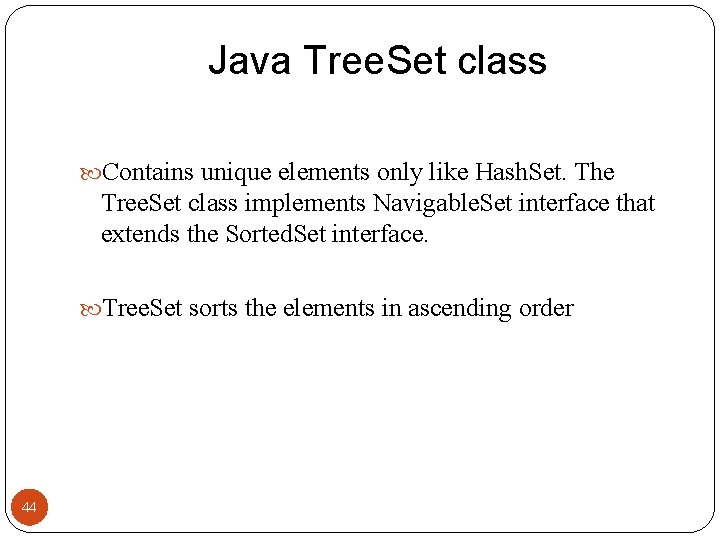
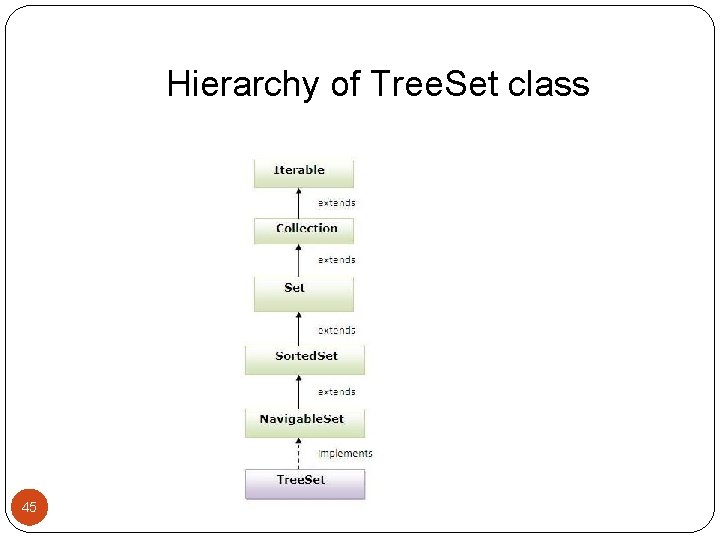
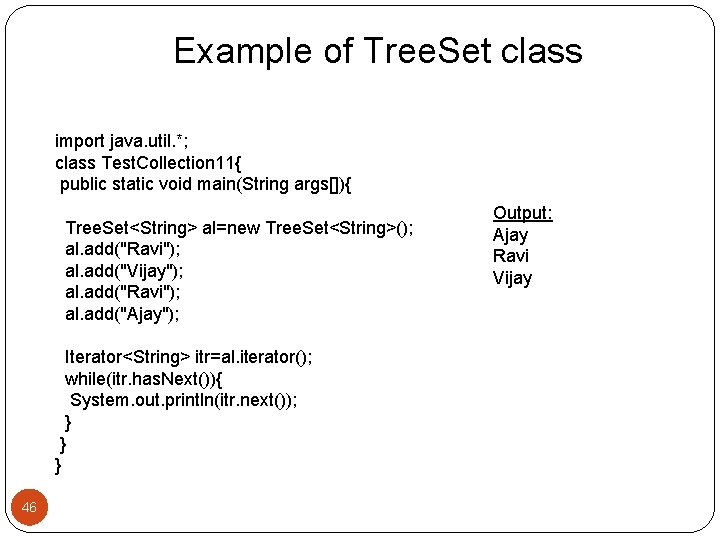
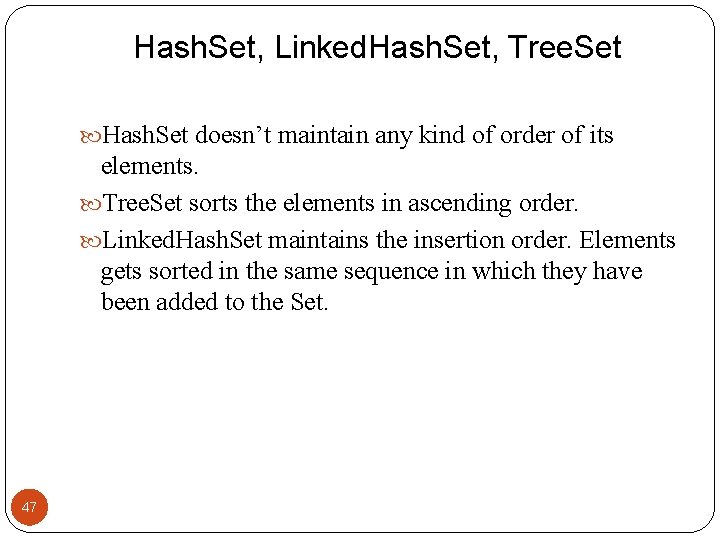
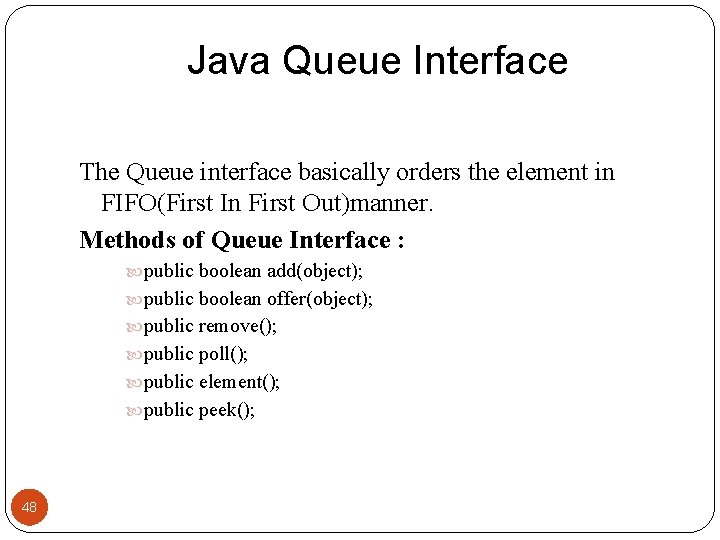
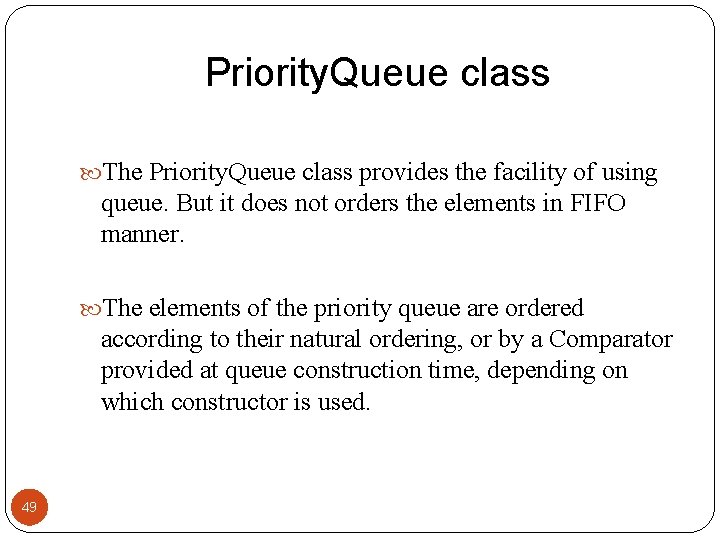
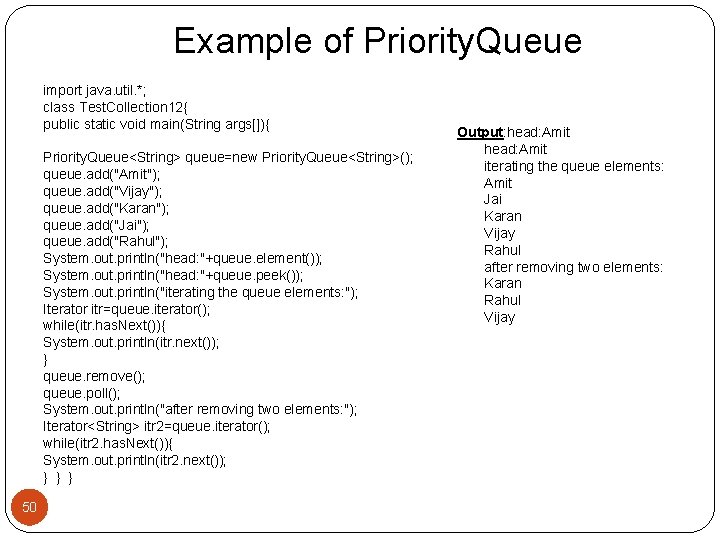
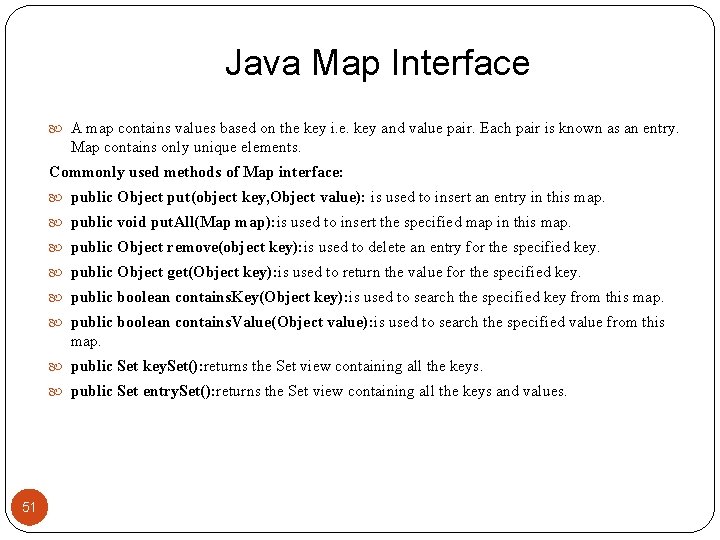
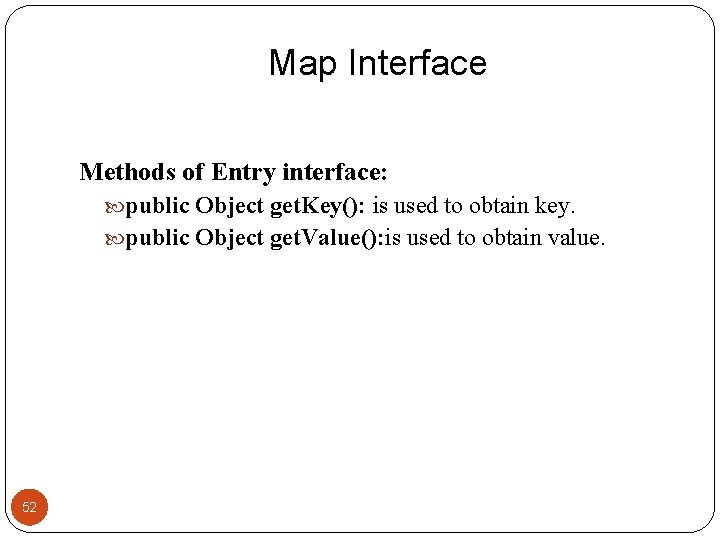
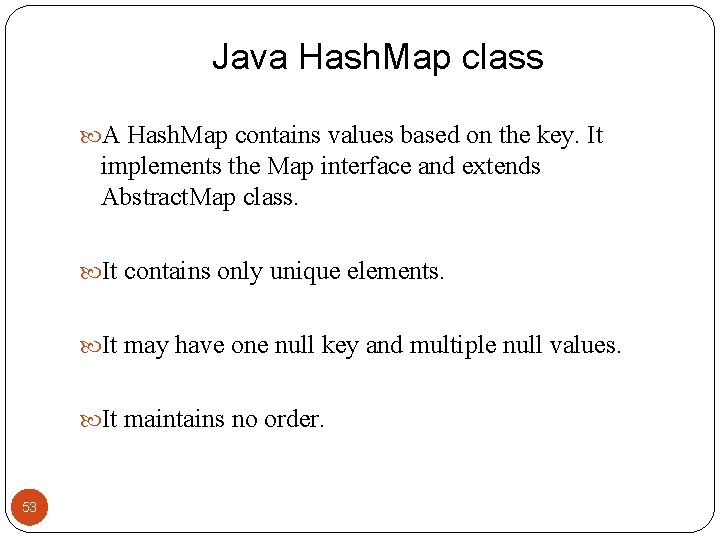
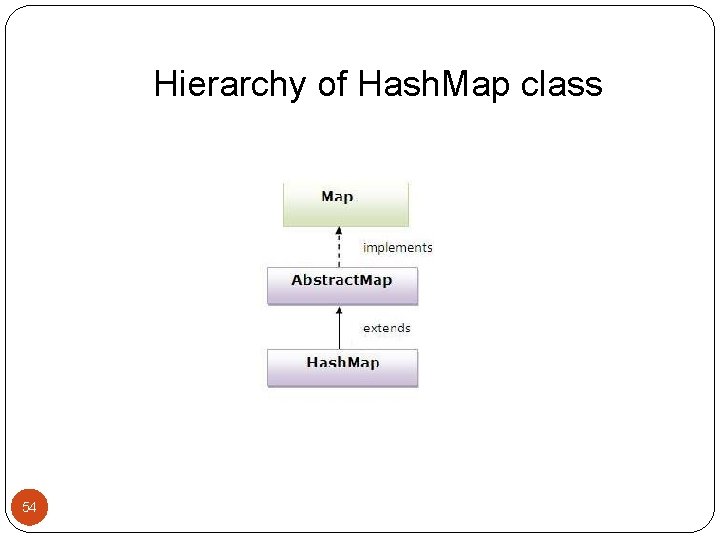
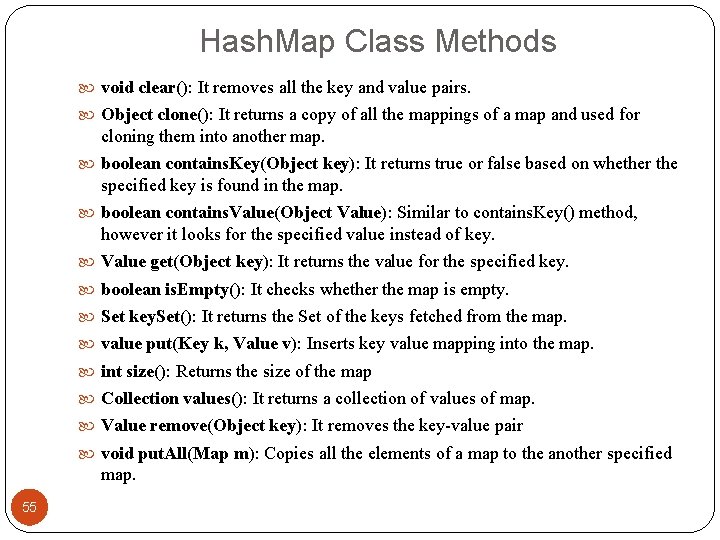
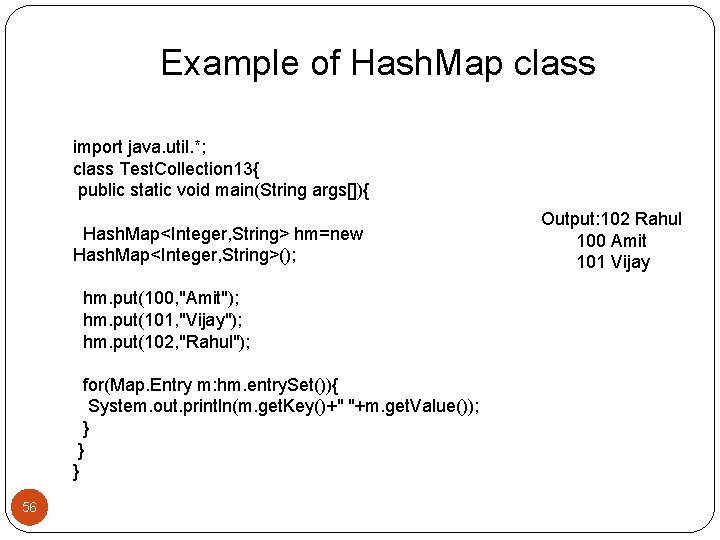
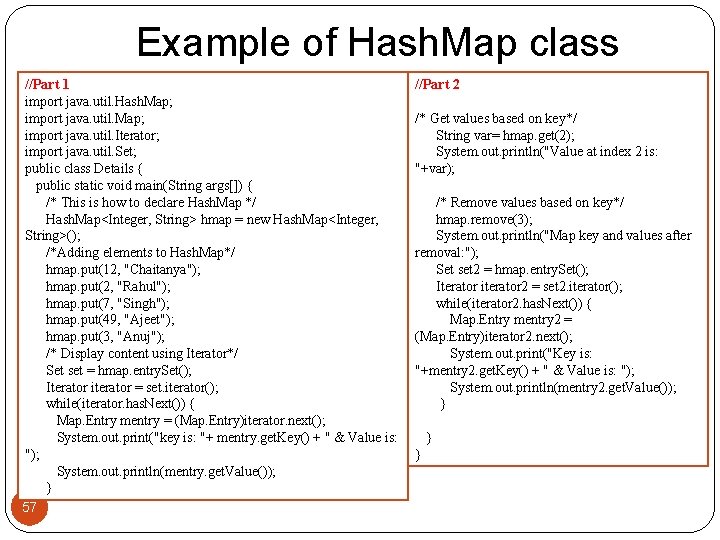
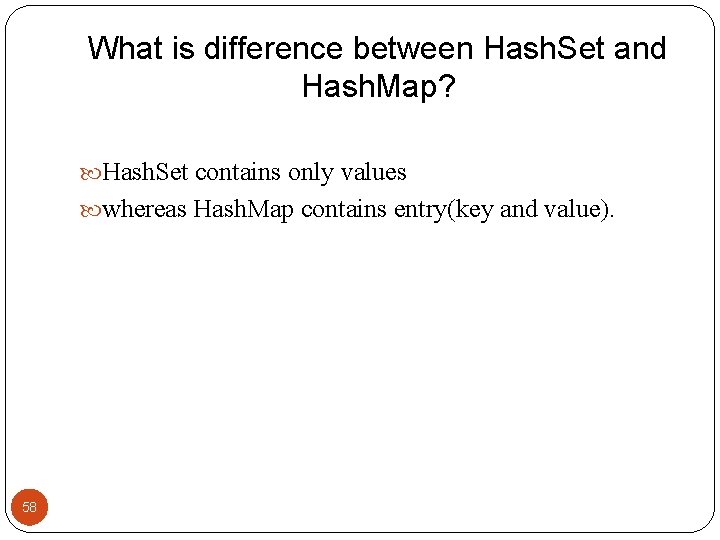
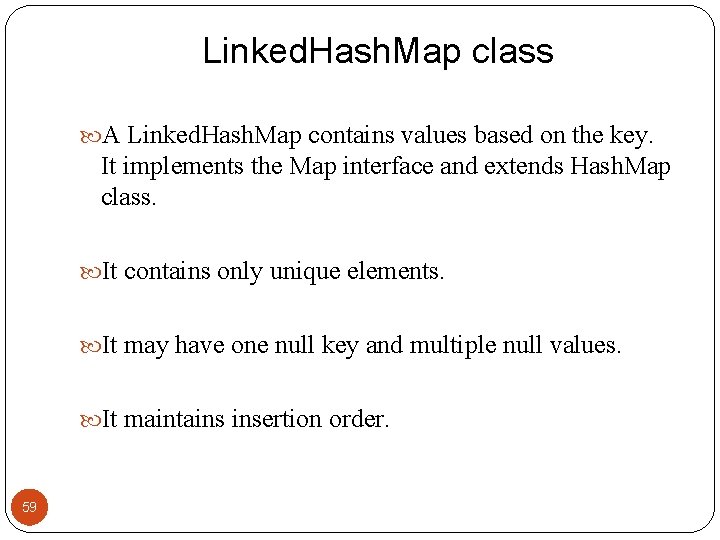
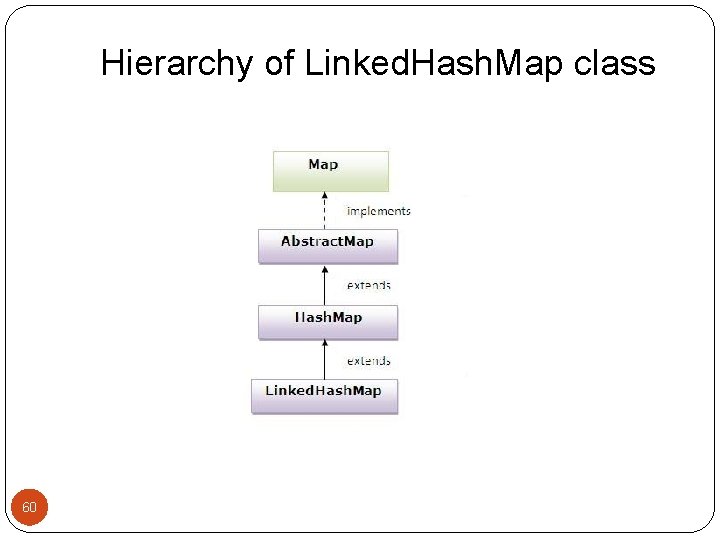
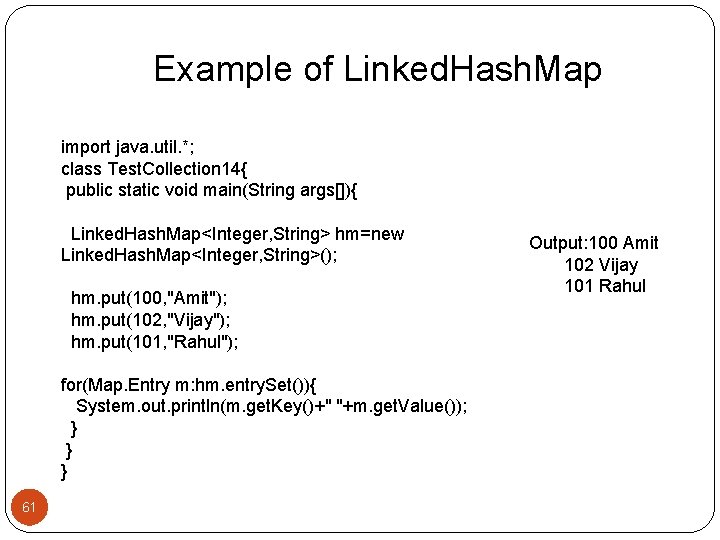
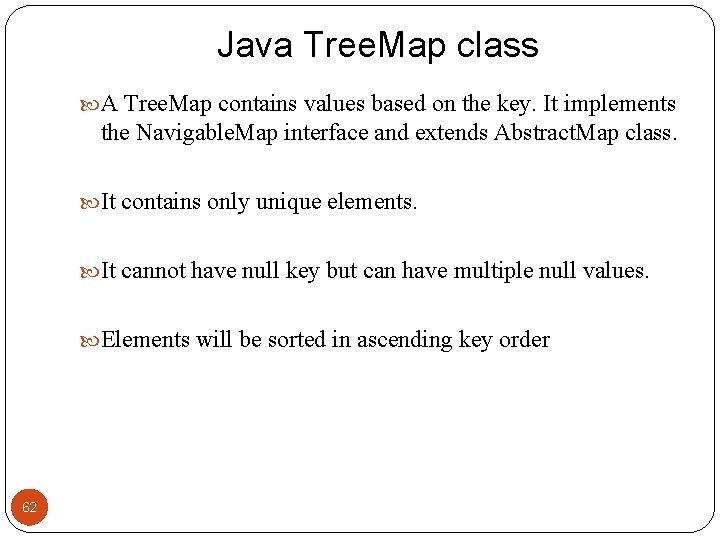
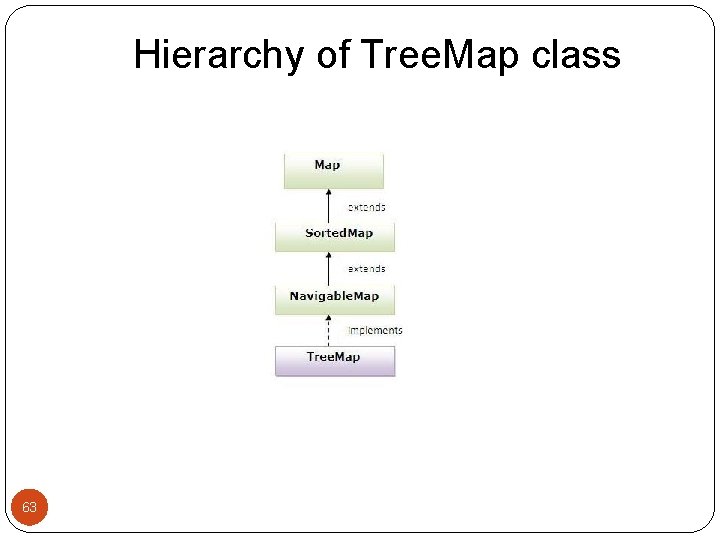
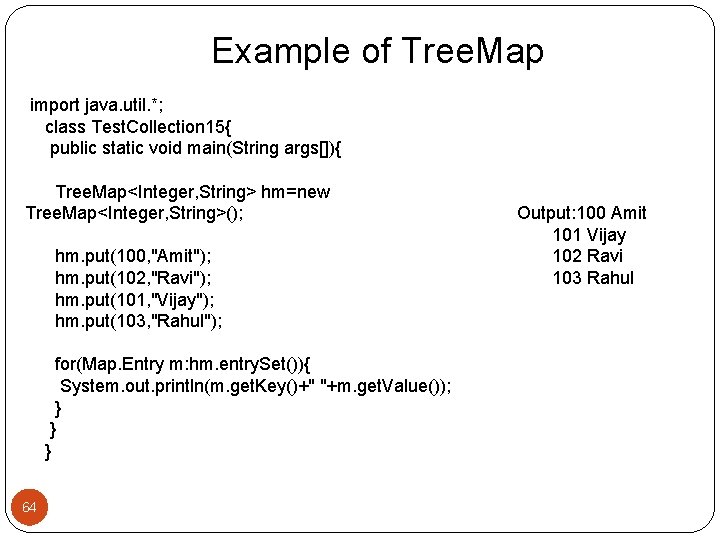
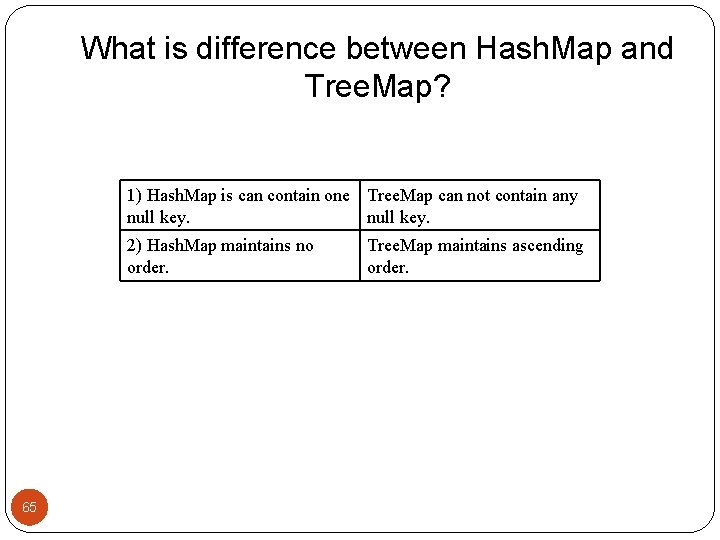
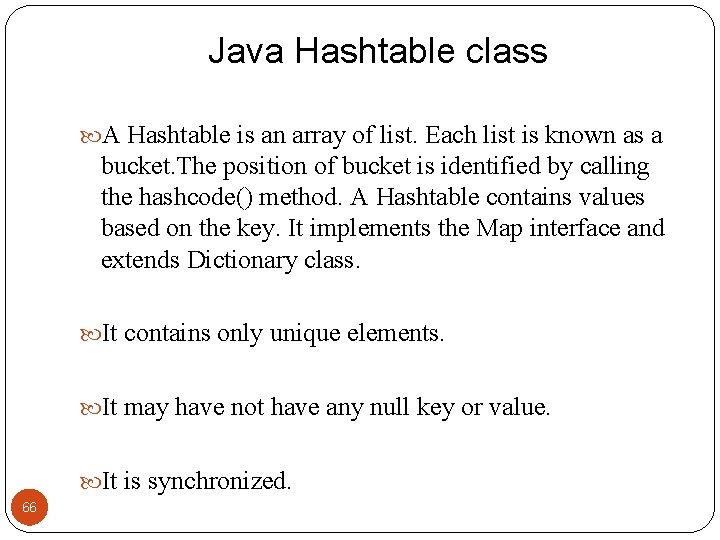
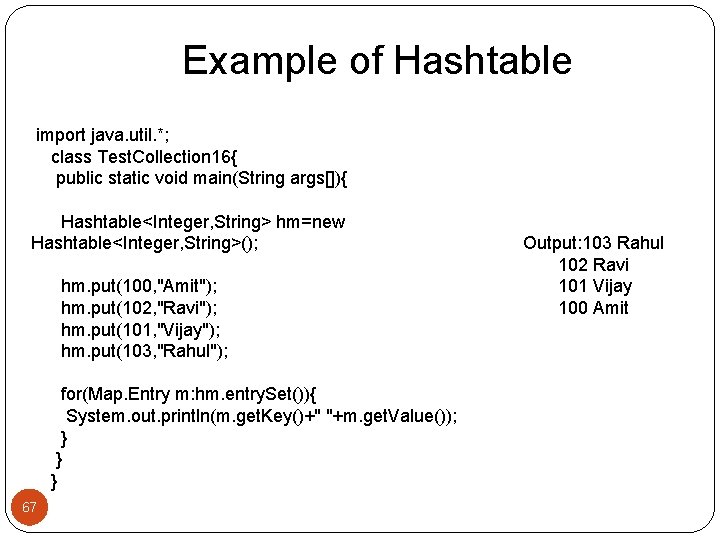
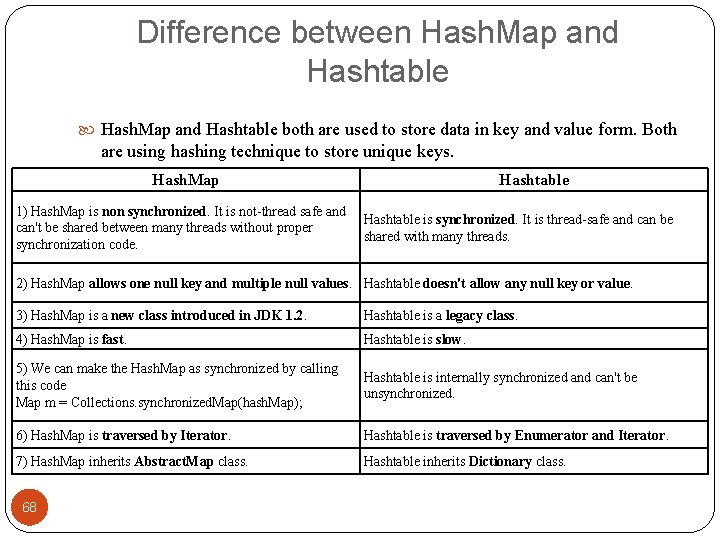
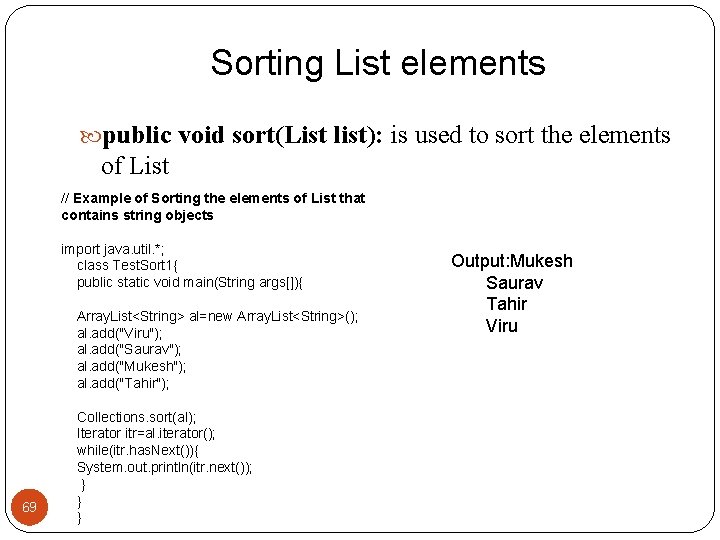
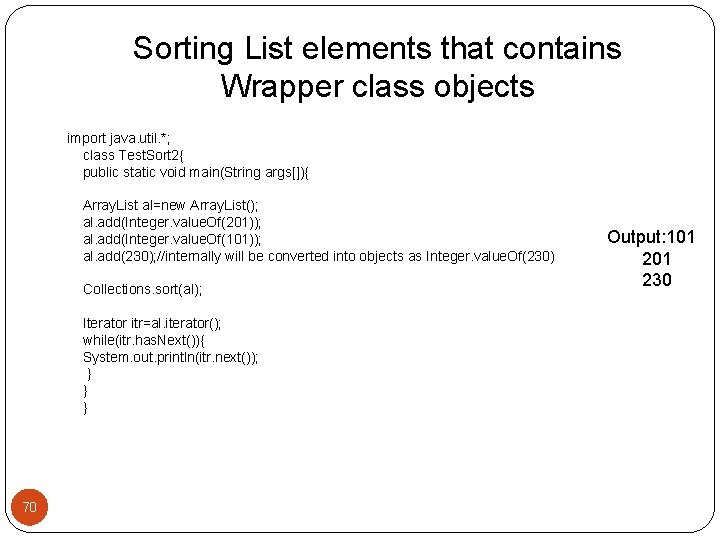
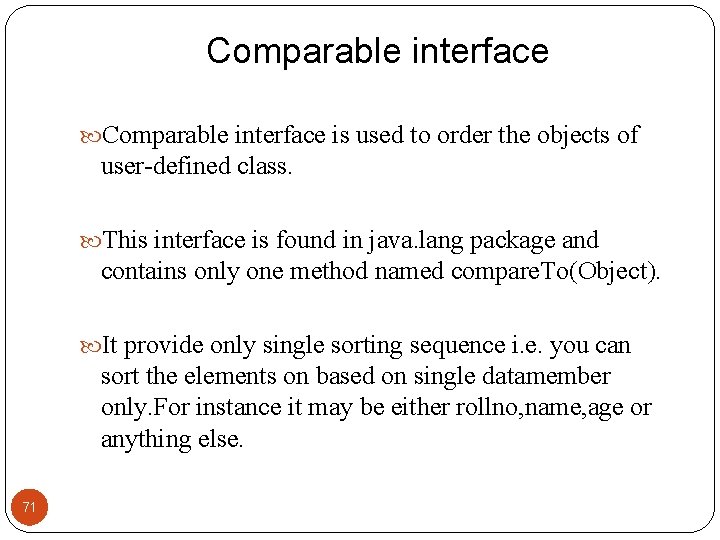
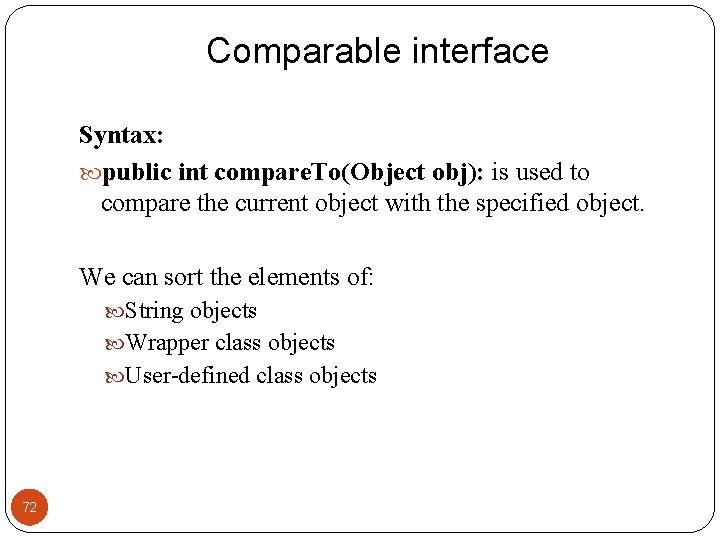
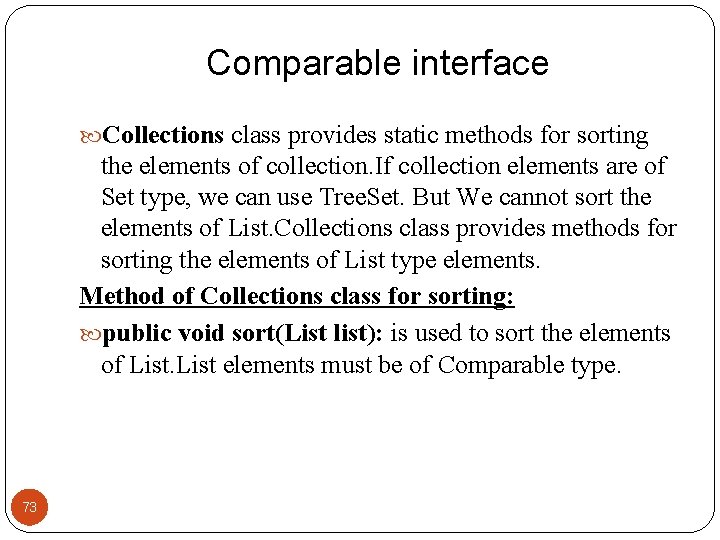
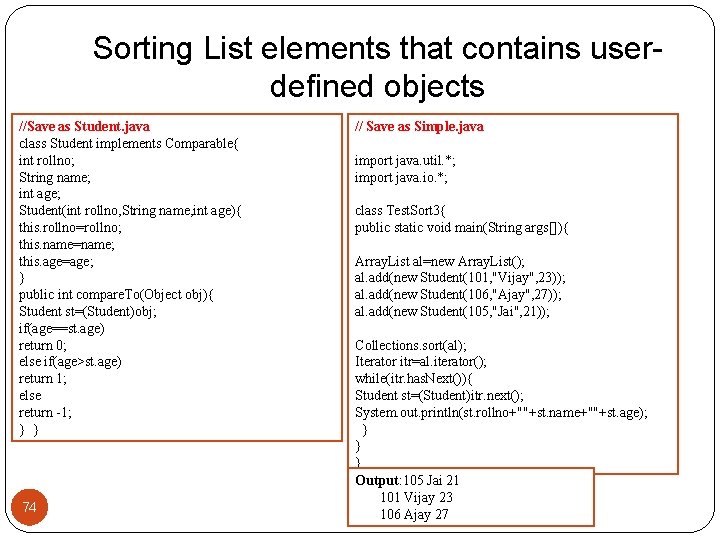
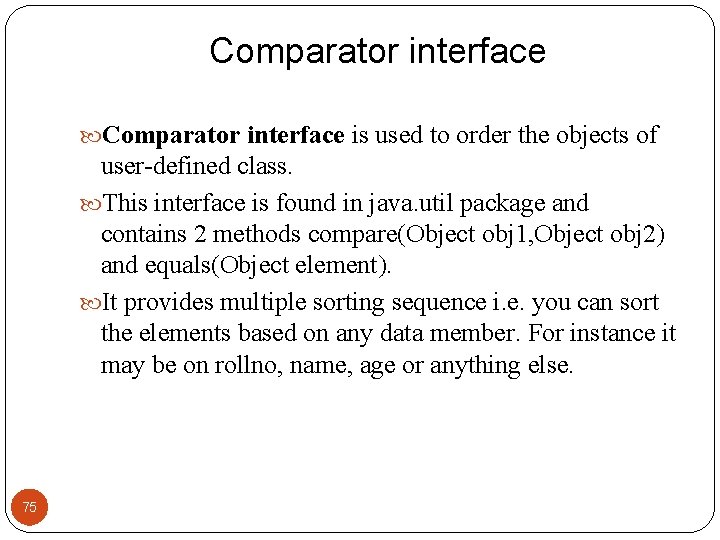
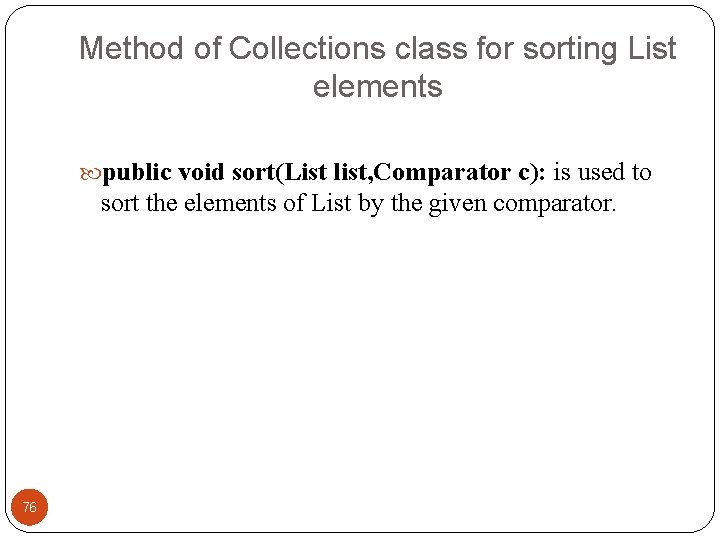
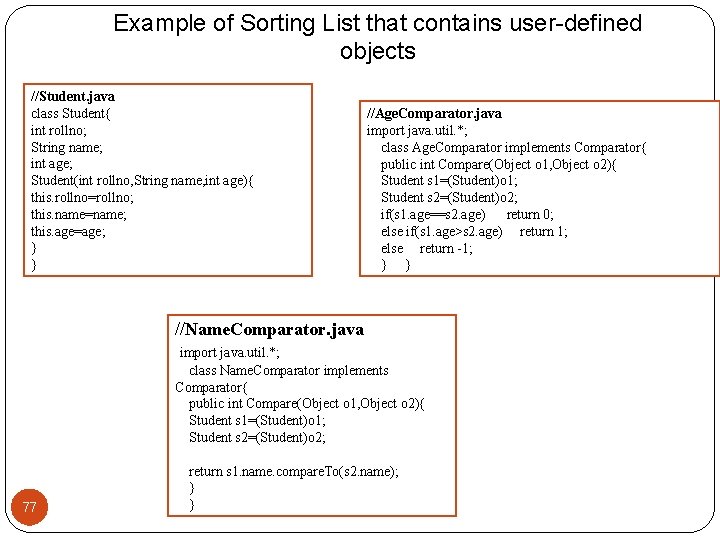
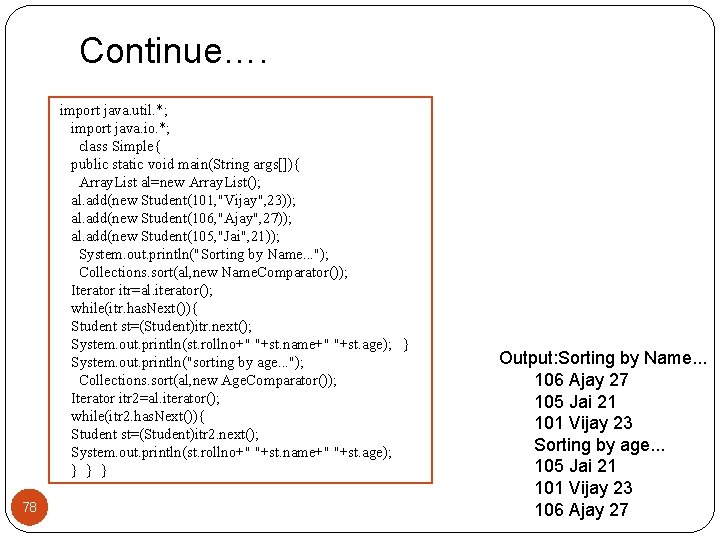
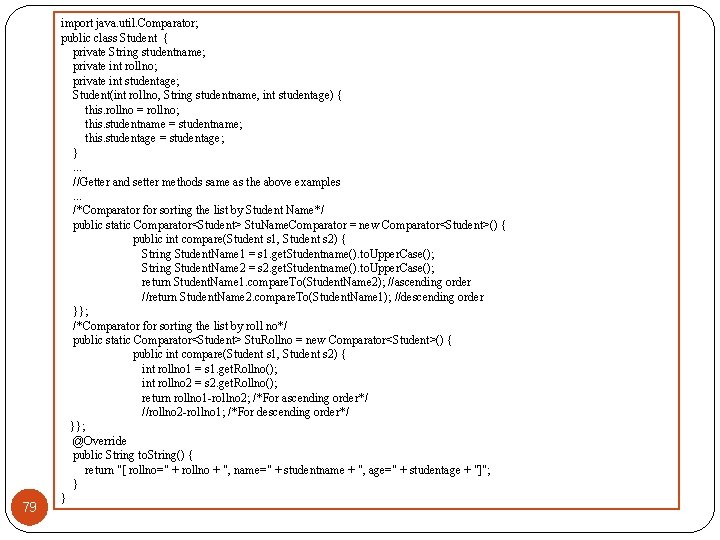
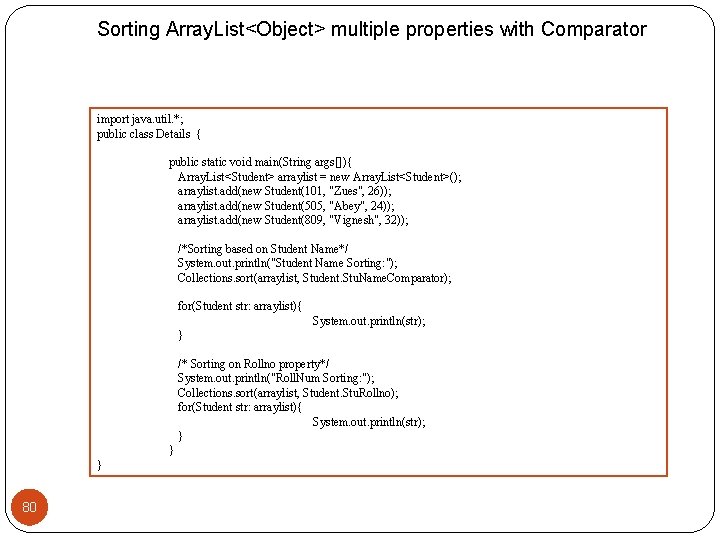
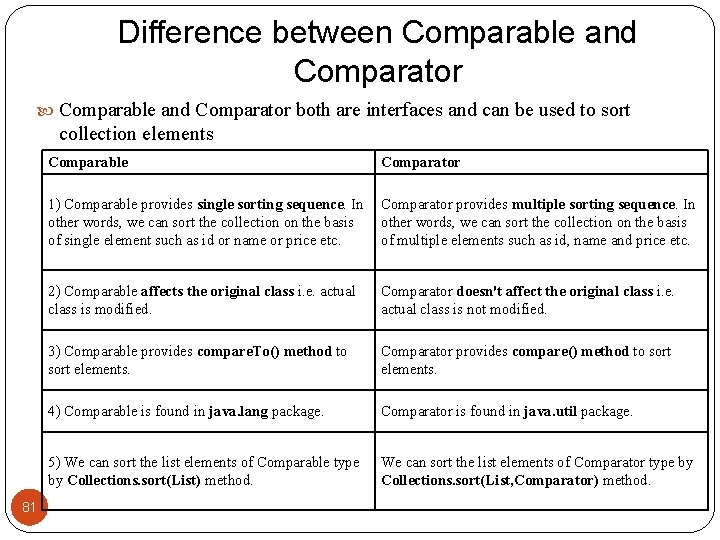
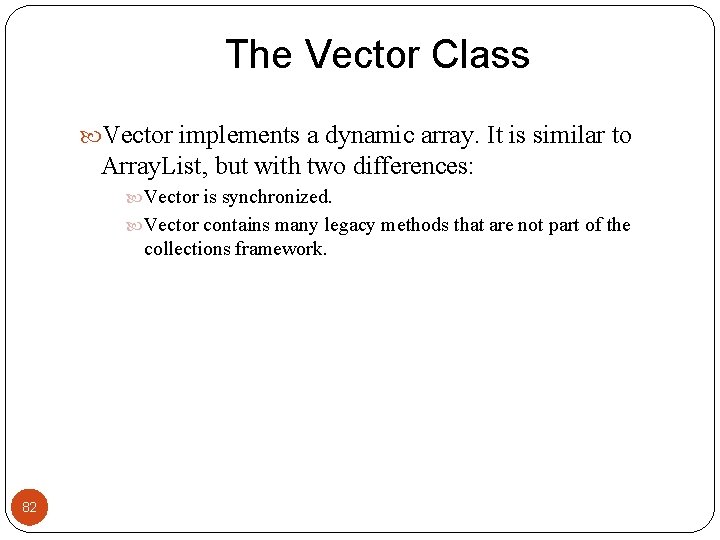
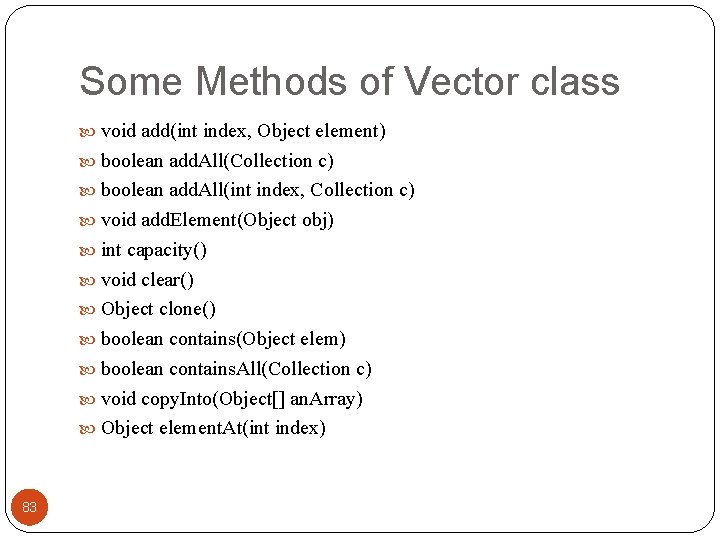
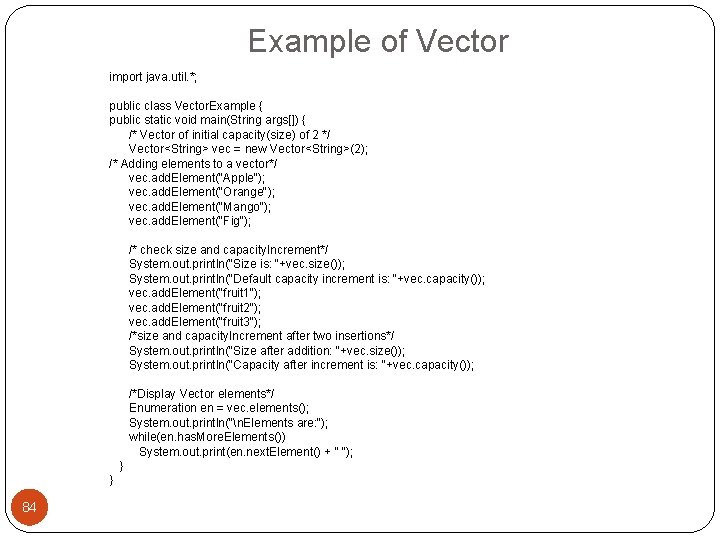
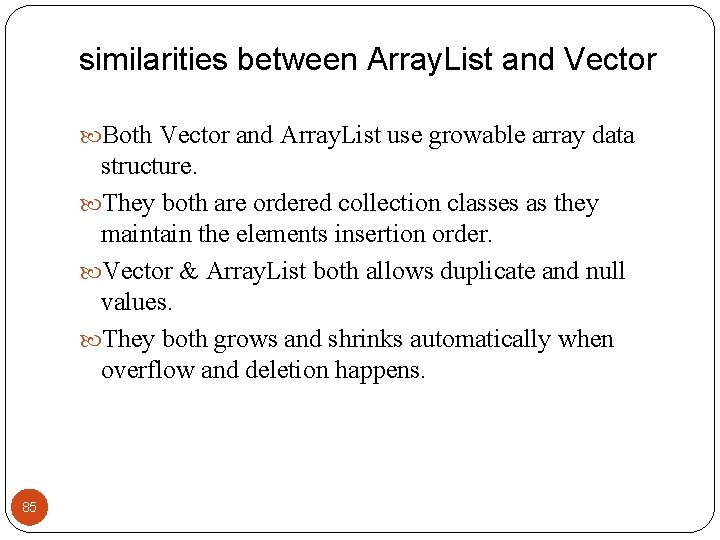
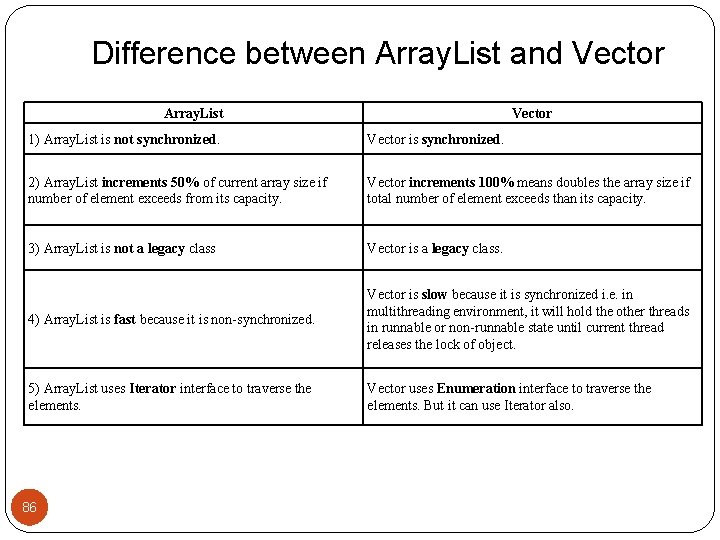
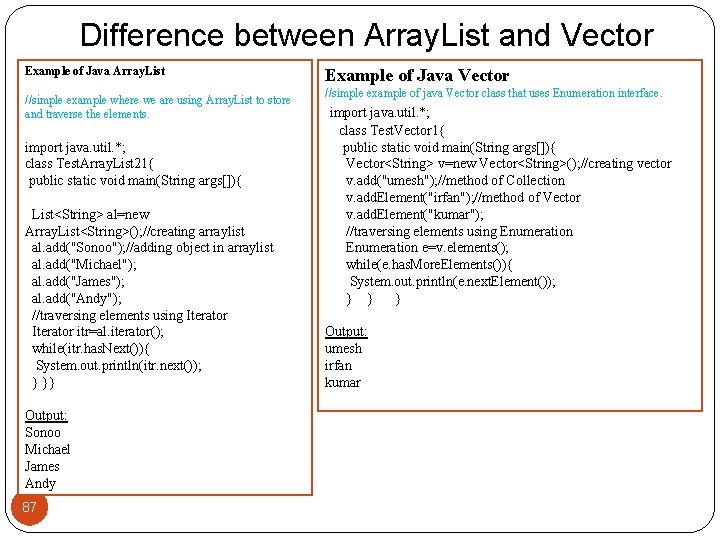
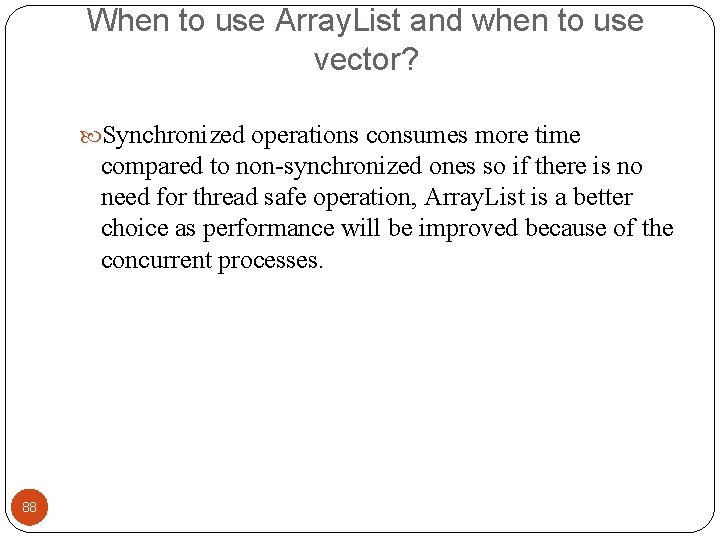
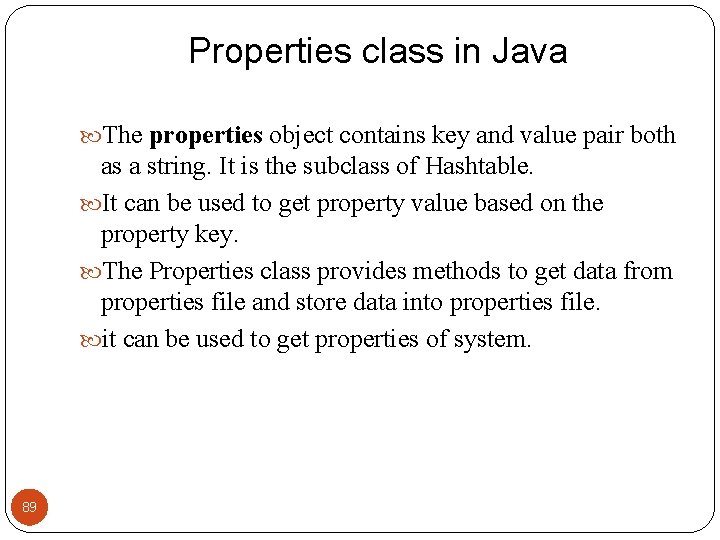
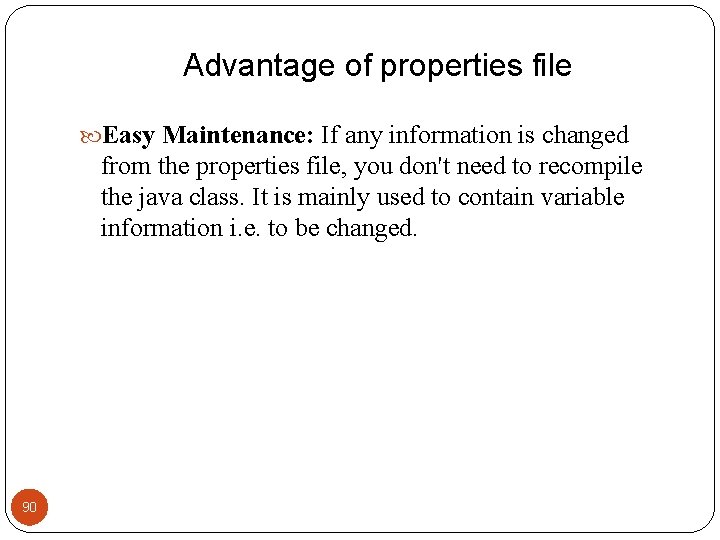
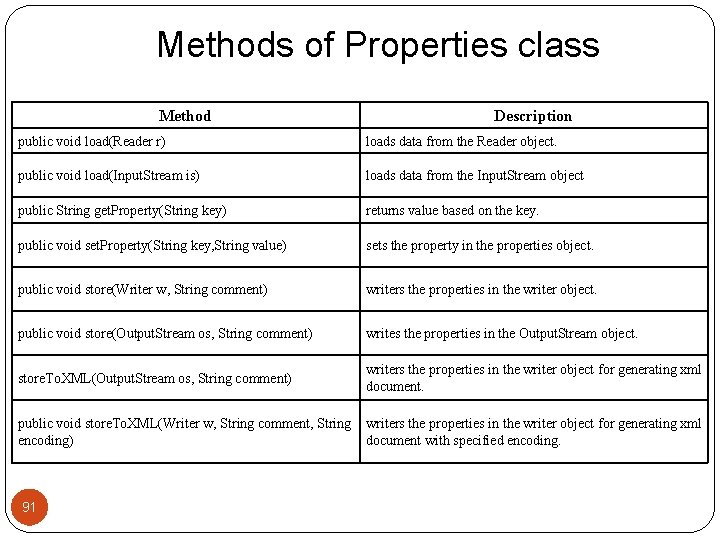
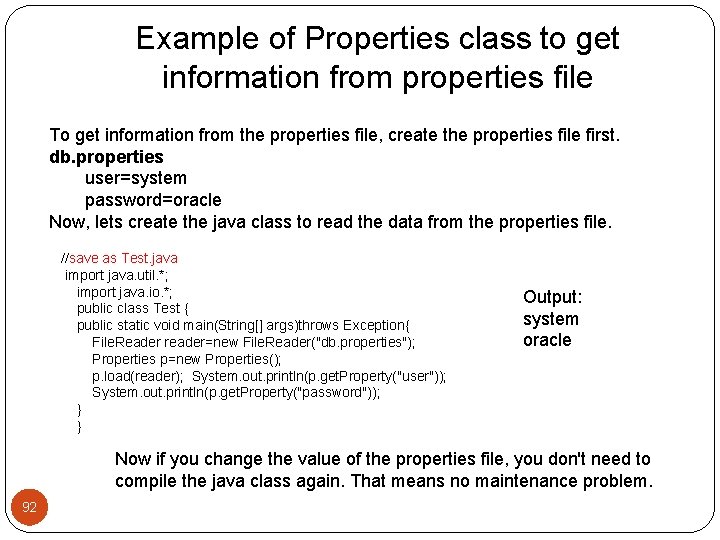
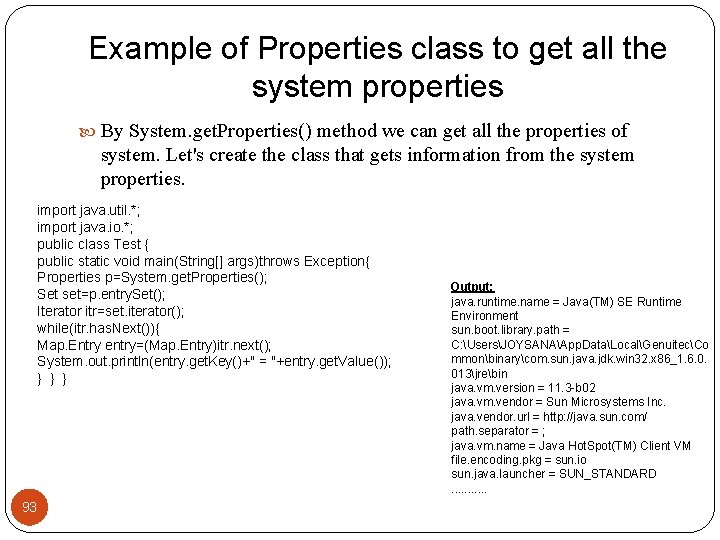
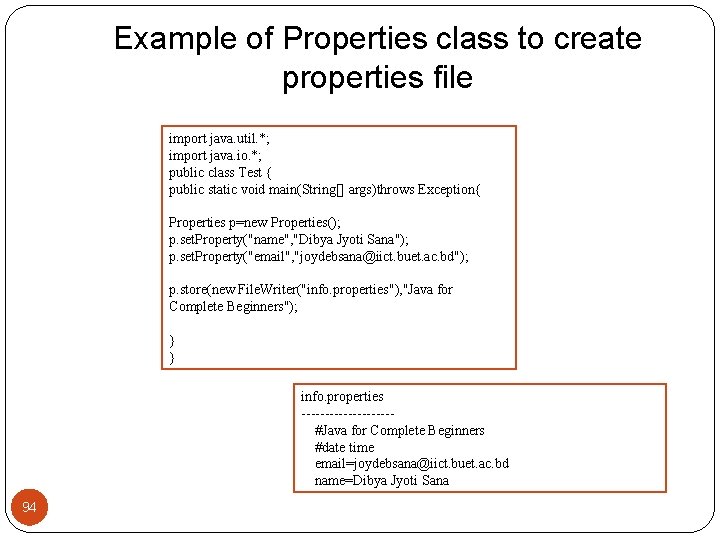
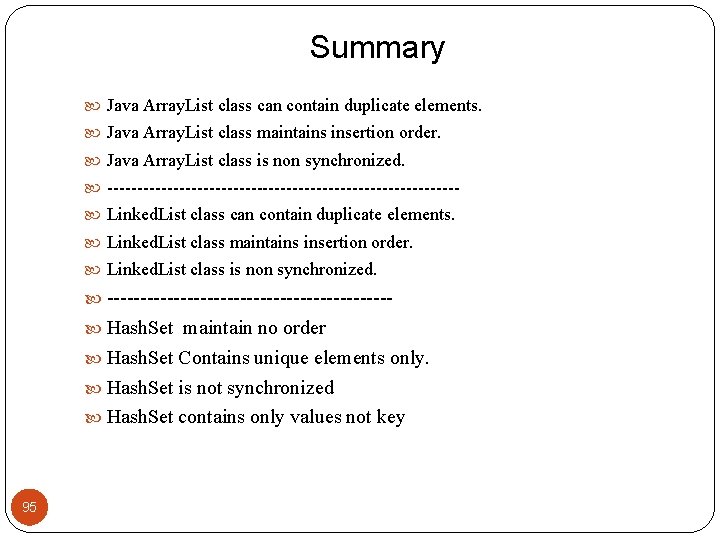
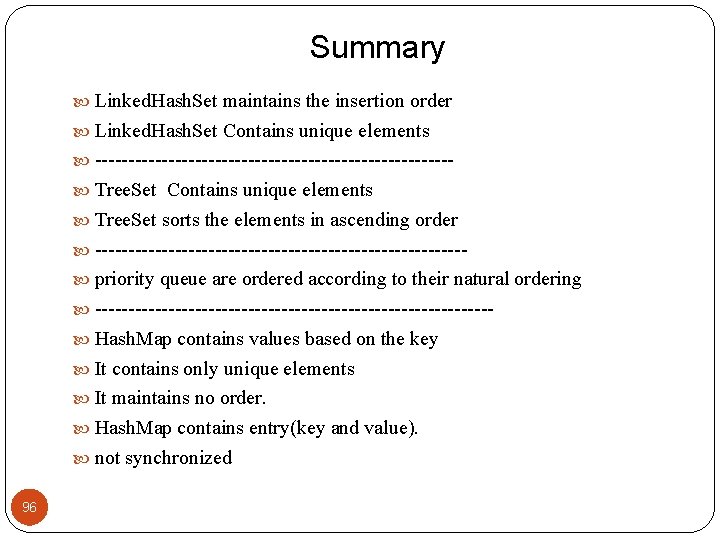
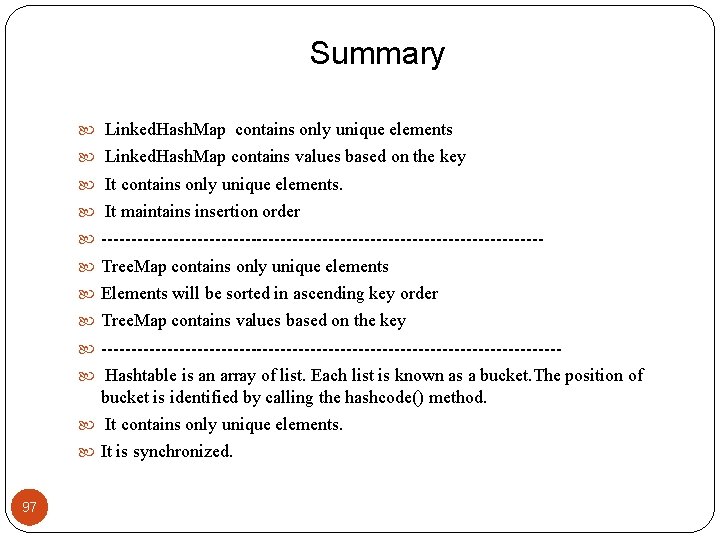
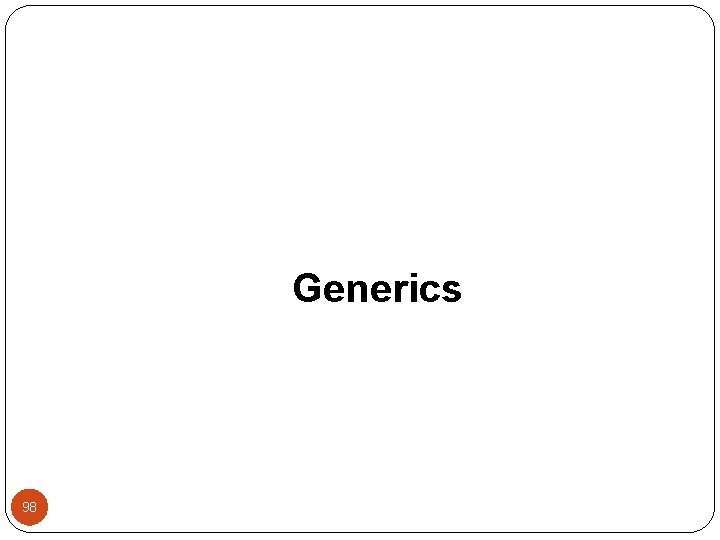
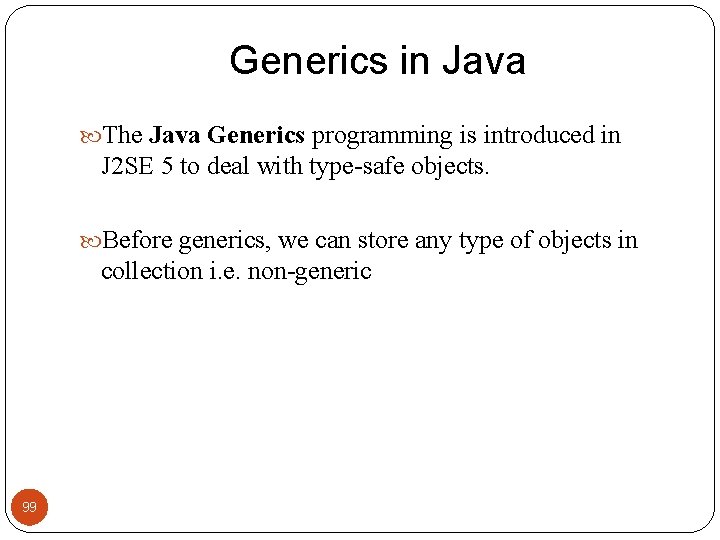
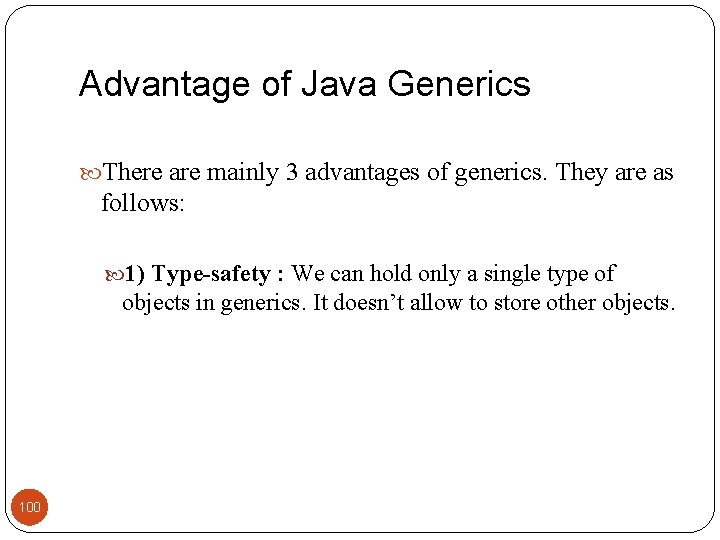
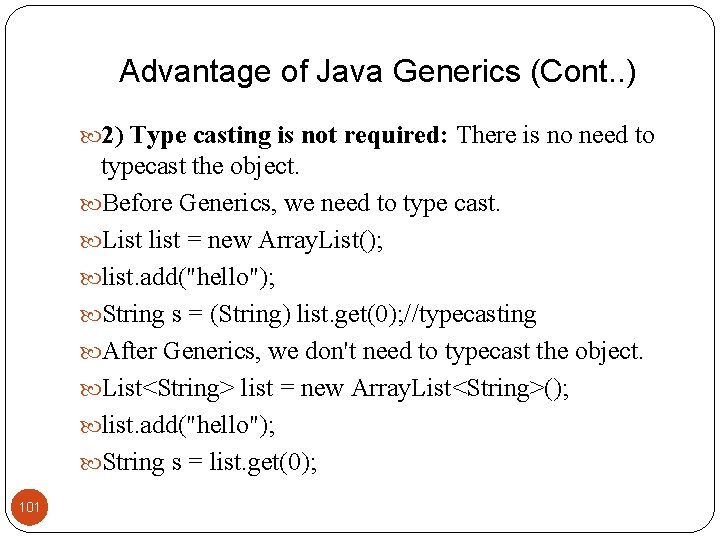
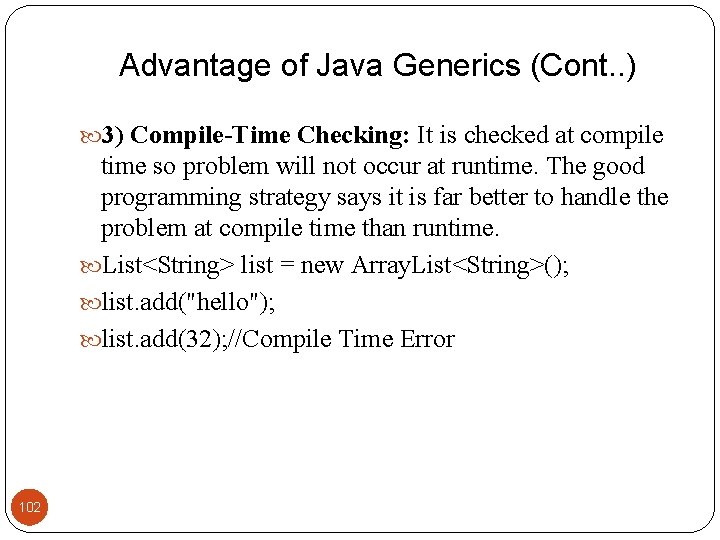
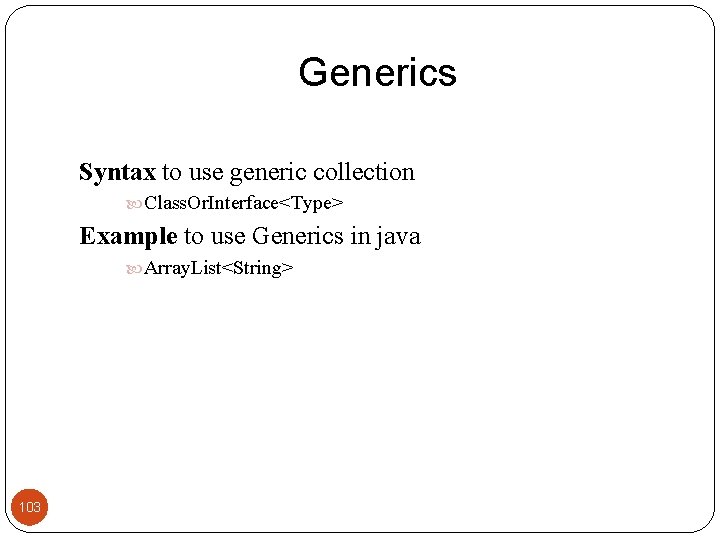
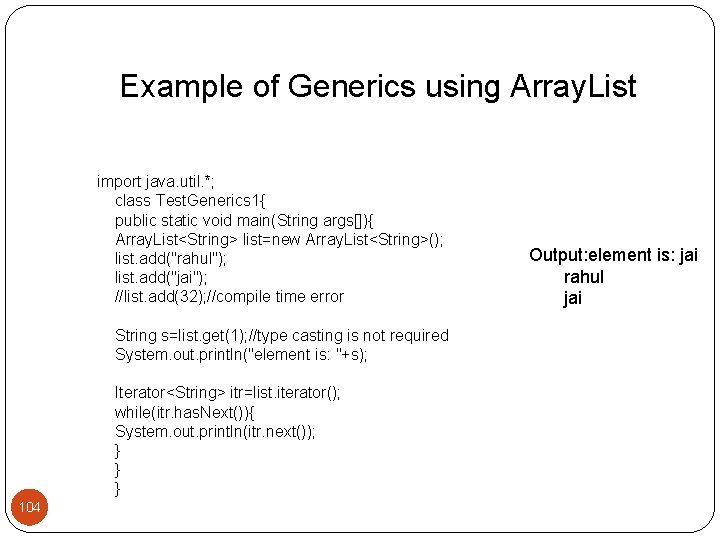
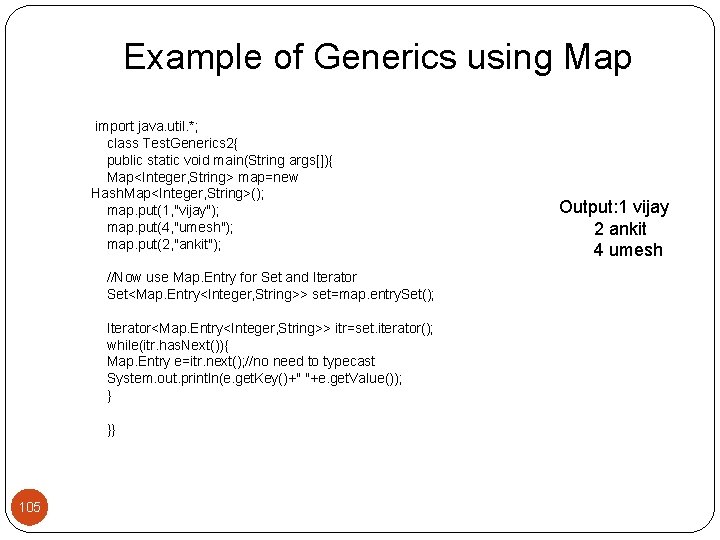
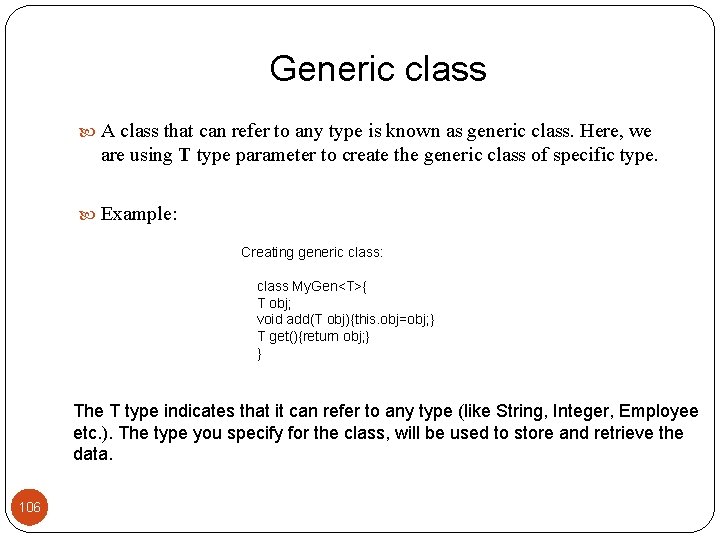
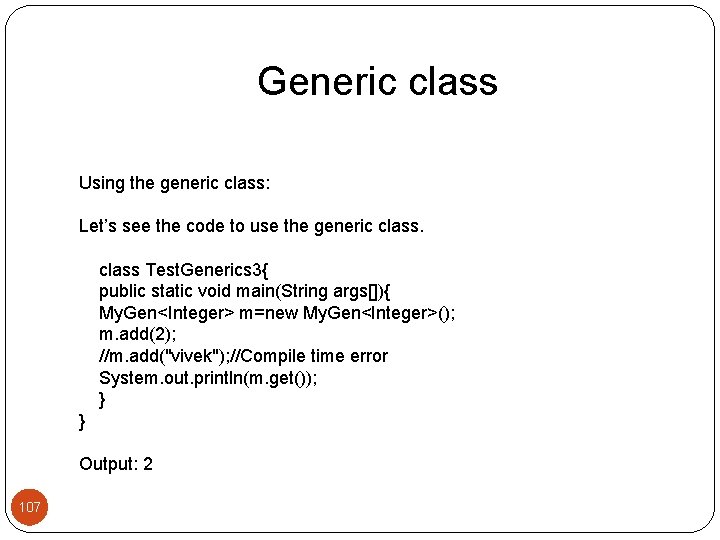
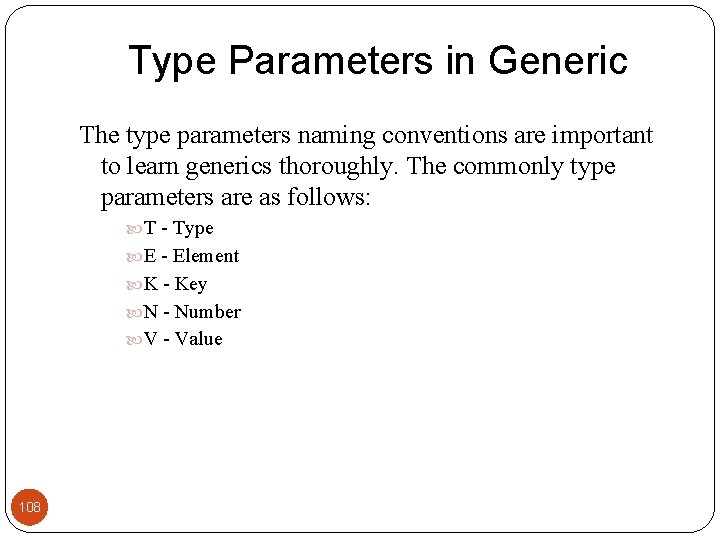
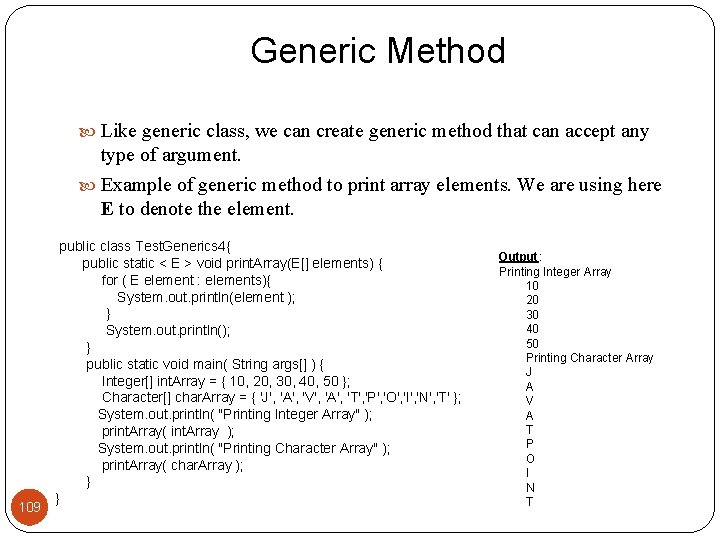
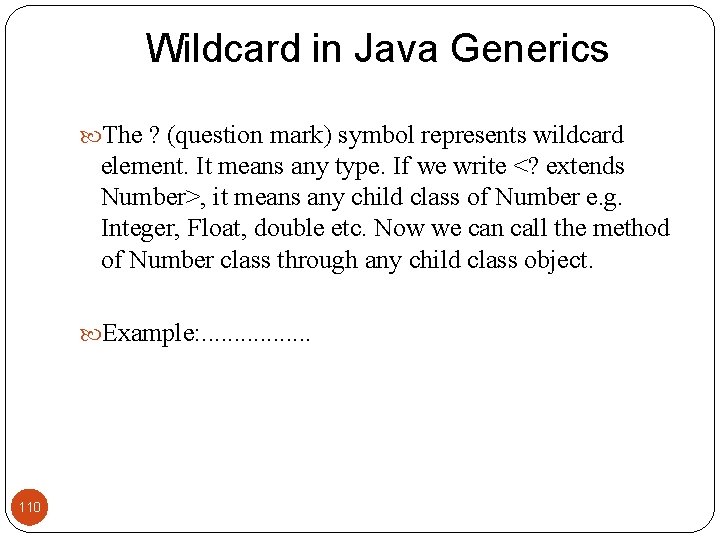
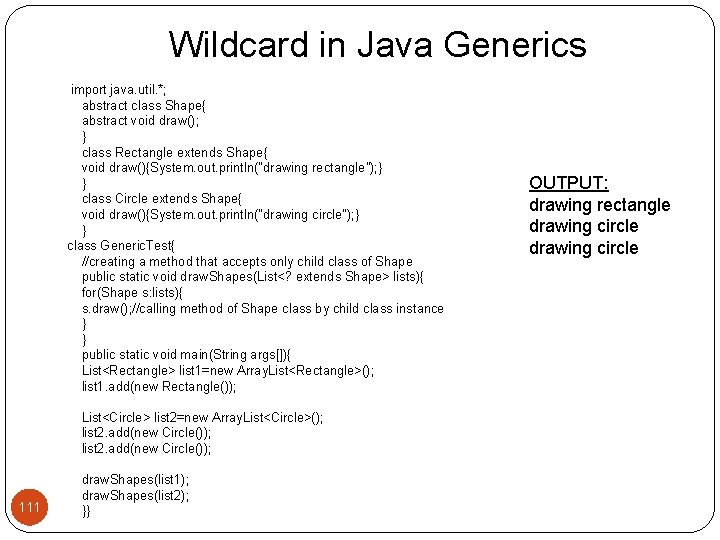
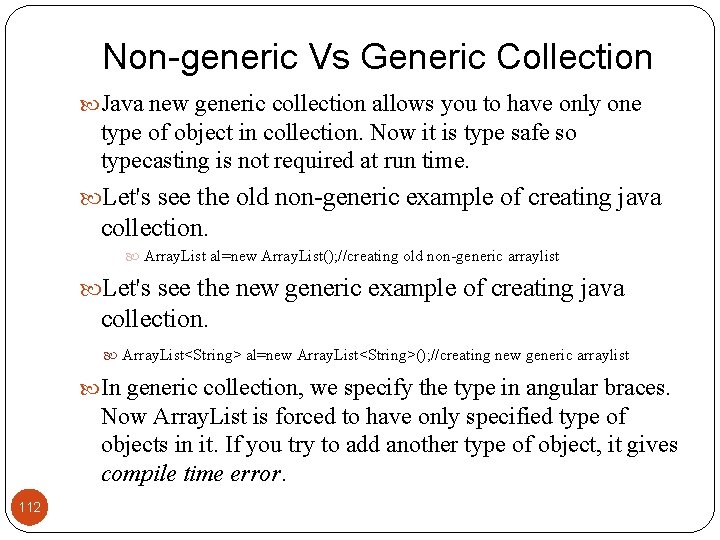
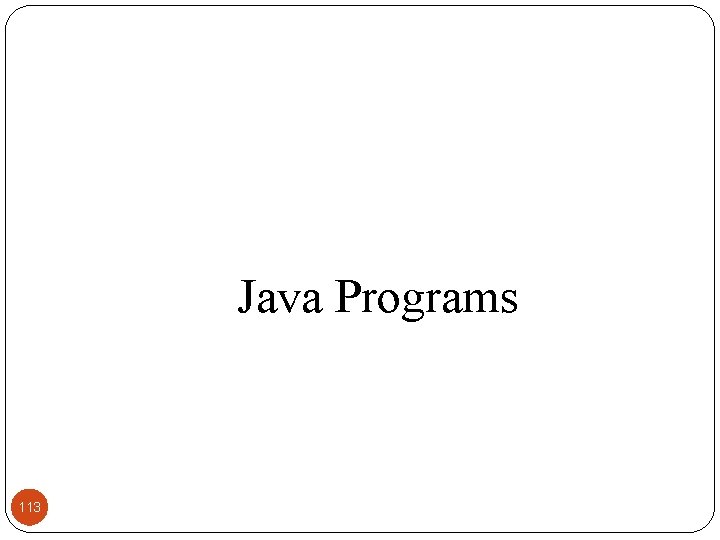
- Slides: 113
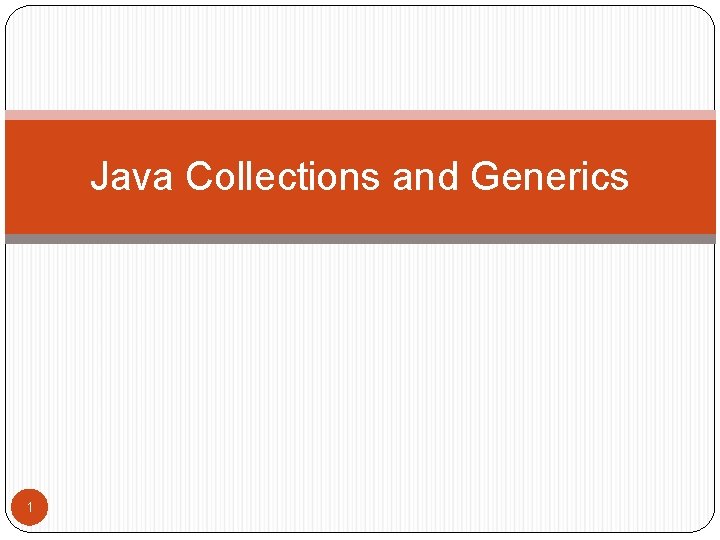
Java Collections and Generics 1
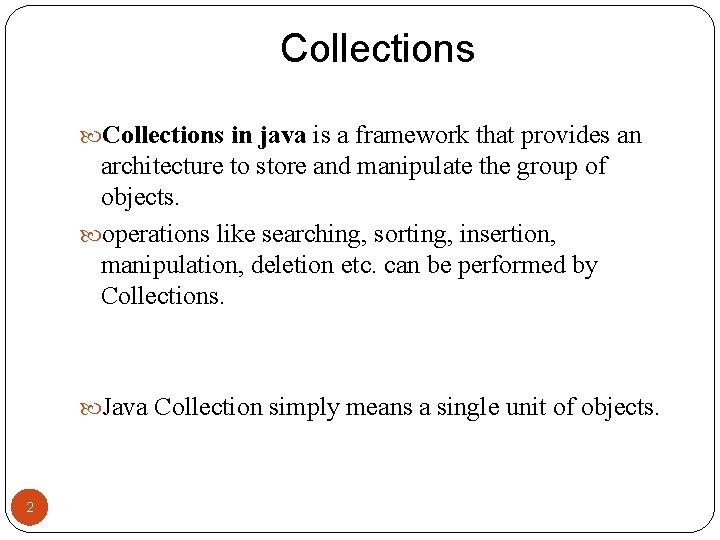
Collections in java is a framework that provides an architecture to store and manipulate the group of objects. operations like searching, sorting, insertion, manipulation, deletion etc. can be performed by Collections. Java Collection simply means a single unit of objects. 2
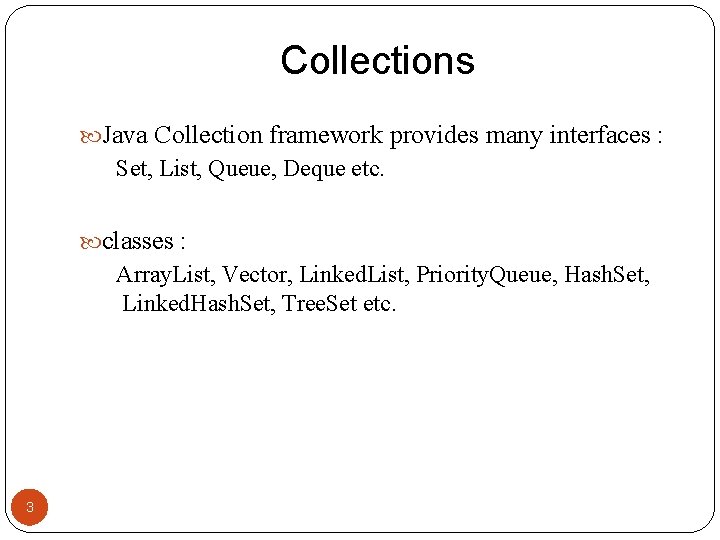
Collections Java Collection framework provides many interfaces : Set, List, Queue, Deque etc. classes : Array. List, Vector, Linked. List, Priority. Queue, Hash. Set, Linked. Hash. Set, Tree. Set etc. 3
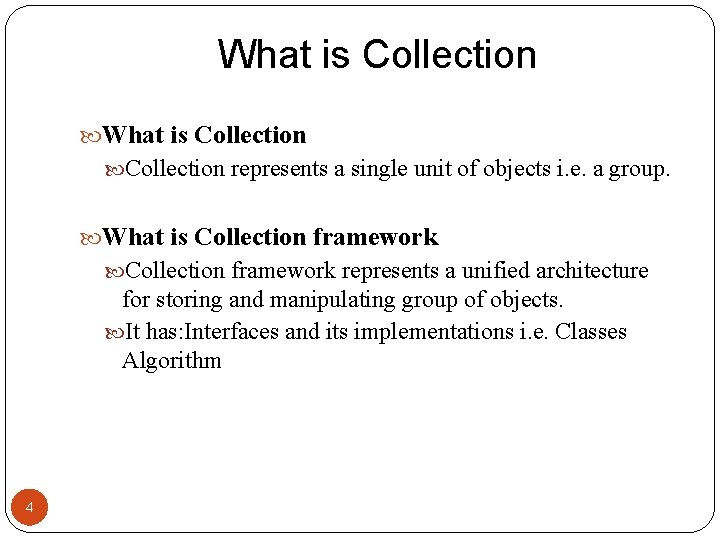
What is Collection represents a single unit of objects i. e. a group. What is Collection framework represents a unified architecture for storing and manipulating group of objects. It has: Interfaces and its implementations i. e. Classes Algorithm 4
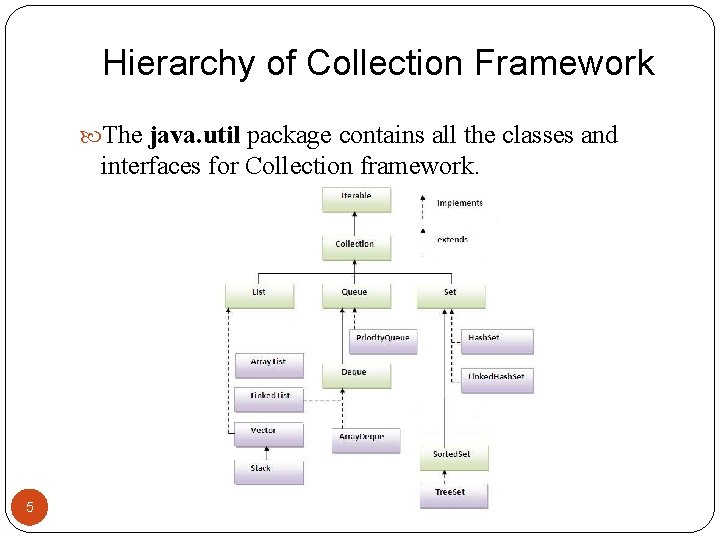
Hierarchy of Collection Framework The java. util package contains all the classes and interfaces for Collection framework. 5
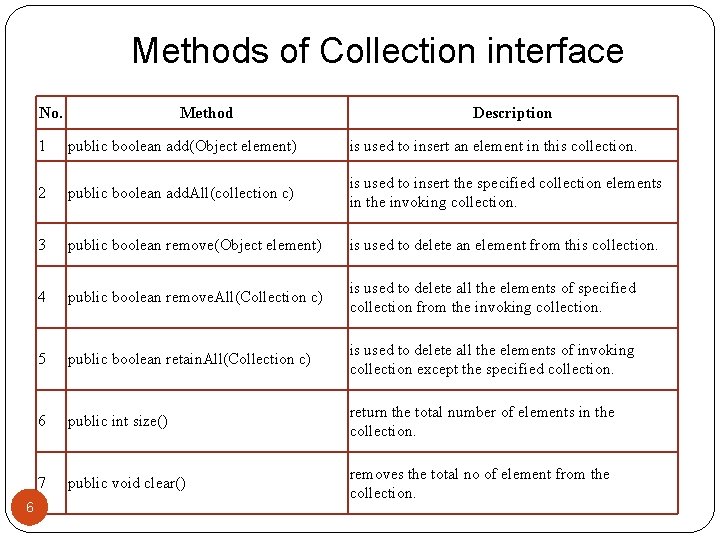
Methods of Collection interface No. 6 Method Description 1 public boolean add(Object element) is used to insert an element in this collection. 2 public boolean add. All(collection c) is used to insert the specified collection elements in the invoking collection. 3 public boolean remove(Object element) is used to delete an element from this collection. 4 public boolean remove. All(Collection c) is used to delete all the elements of specified collection from the invoking collection. 5 public boolean retain. All(Collection c) is used to delete all the elements of invoking collection except the specified collection. 6 public int size() return the total number of elements in the collection. 7 public void clear() removes the total no of element from the collection.
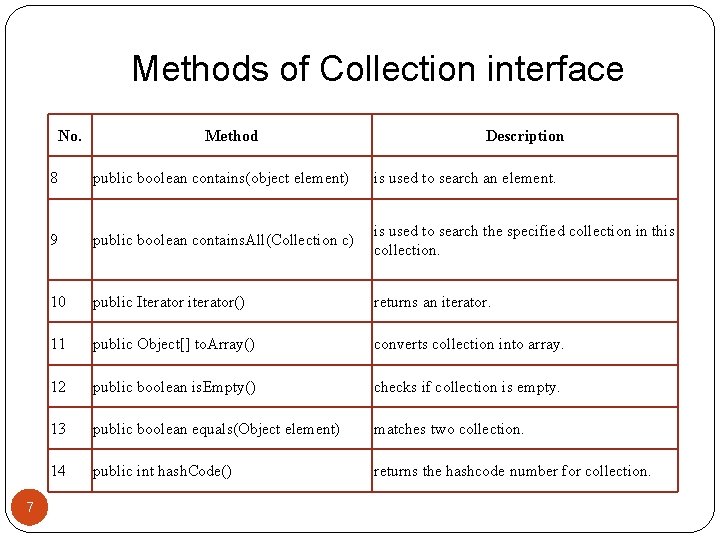
Methods of Collection interface No. 7 Method Description 8 public boolean contains(object element) is used to search an element. 9 public boolean contains. All(Collection c) is used to search the specified collection in this collection. 10 public Iterator iterator() returns an iterator. 11 public Object[] to. Array() converts collection into array. 12 public boolean is. Empty() checks if collection is empty. 13 public boolean equals(Object element) matches two collection. 14 public int hash. Code() returns the hashcode number for collection.
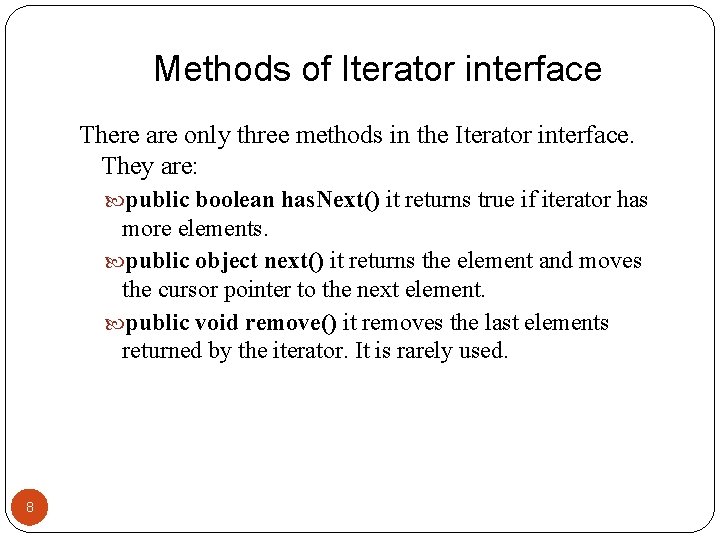
Methods of Iterator interface There are only three methods in the Iterator interface. They are: public boolean has. Next() it returns true if iterator has more elements. public object next() it returns the element and moves the cursor pointer to the next element. public void remove() it removes the last elements returned by the iterator. It is rarely used. 8
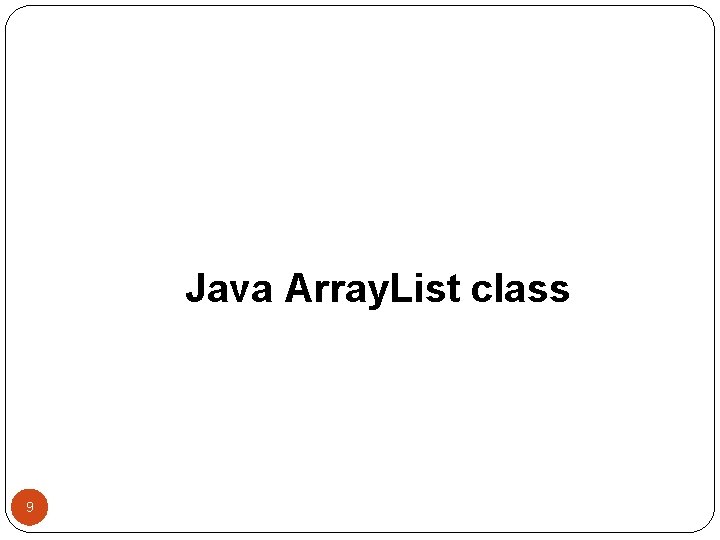
Java Array. List class 9
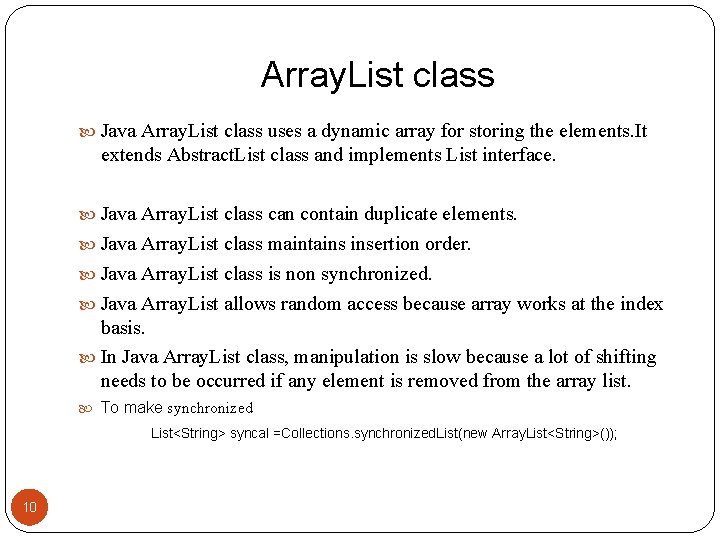
Array. List class Java Array. List class uses a dynamic array for storing the elements. It extends Abstract. List class and implements List interface. Java Array. List class can contain duplicate elements. Java Array. List class maintains insertion order. Java Array. List class is non synchronized. Java Array. List allows random access because array works at the index basis. In Java Array. List class, manipulation is slow because a lot of shifting needs to be occurred if any element is removed from the array list. To make synchronized 10 List<String> syncal =Collections. synchronized. List(new Array. List<String>());
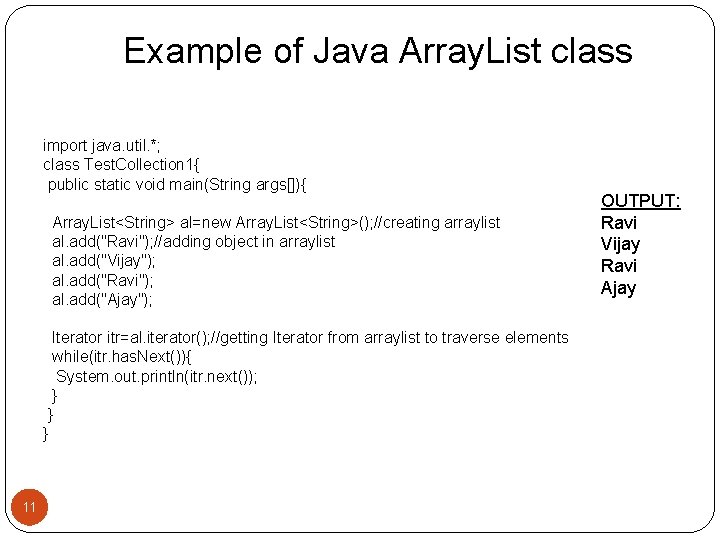
Example of Java Array. List class import java. util. *; class Test. Collection 1{ public static void main(String args[]){ Array. List<String> al=new Array. List<String>(); //creating arraylist al. add("Ravi"); //adding object in arraylist al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); Iterator itr=al. iterator(); //getting Iterator from arraylist to traverse elements while(itr. has. Next()){ System. out. println(itr. next()); } } } 11 OUTPUT: Ravi Vijay Ravi Ajay
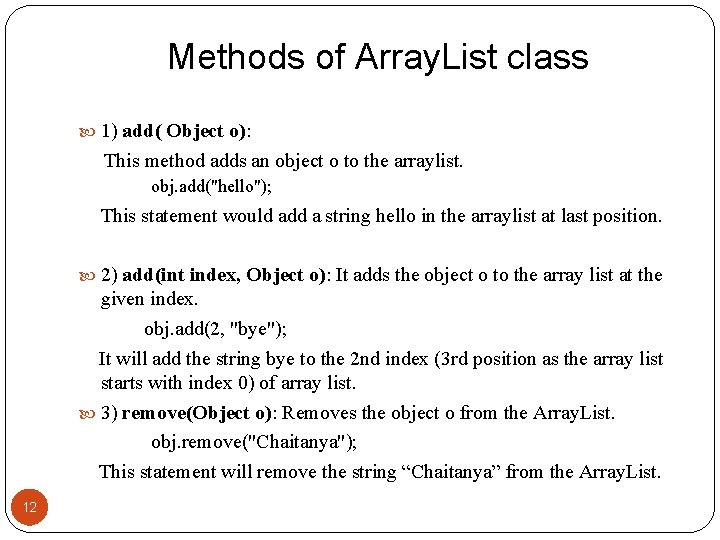
Methods of Array. List class 1) add( Object o): This method adds an object o to the arraylist. obj. add("hello"); This statement would add a string hello in the arraylist at last position. 2) add(int index, Object o): It adds the object o to the array list at the given index. obj. add(2, "bye"); It will add the string bye to the 2 nd index (3 rd position as the array list starts with index 0) of array list. 3) remove(Object o): Removes the object o from the Array. List. obj. remove("Chaitanya"); This statement will remove the string “Chaitanya” from the Array. List. 12
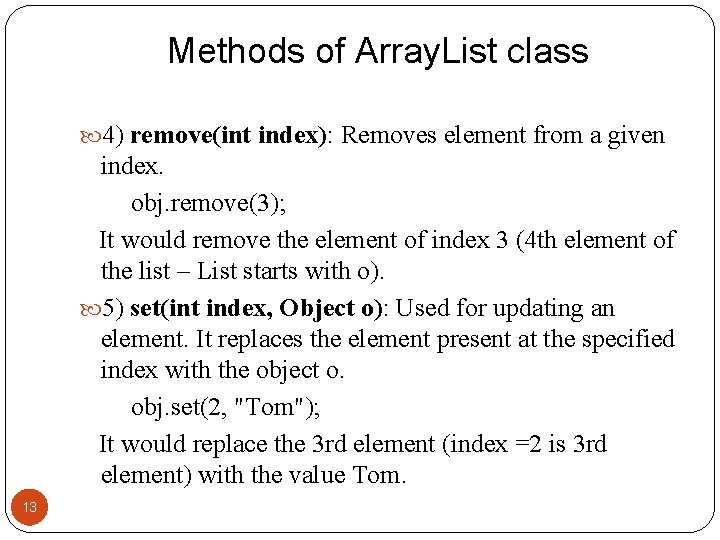
Methods of Array. List class 4) remove(int index): Removes element from a given index. obj. remove(3); It would remove the element of index 3 (4 th element of the list – List starts with o). 5) set(int index, Object o): Used for updating an element. It replaces the element present at the specified index with the object o. obj. set(2, "Tom"); It would replace the 3 rd element (index =2 is 3 rd element) with the value Tom. 13
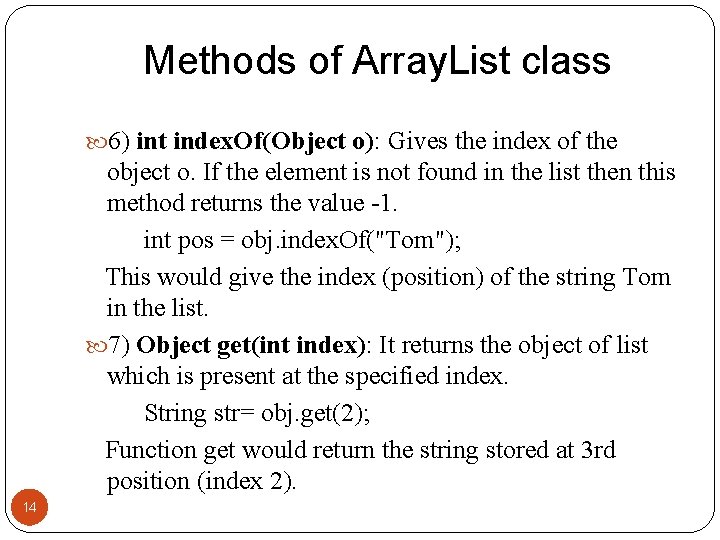
Methods of Array. List class 6) int index. Of(Object o): Gives the index of the object o. If the element is not found in the list then this method returns the value -1. int pos = obj. index. Of("Tom"); This would give the index (position) of the string Tom in the list. 7) Object get(int index): It returns the object of list which is present at the specified index. String str= obj. get(2); Function get would return the string stored at 3 rd position (index 2). 14
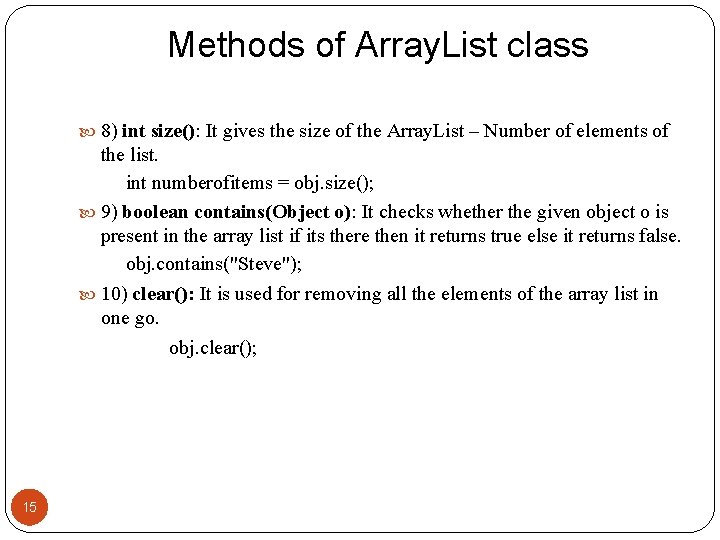
Methods of Array. List class 8) int size(): It gives the size of the Array. List – Number of elements of the list. int numberofitems = obj. size(); 9) boolean contains(Object o): It checks whether the given object o is present in the array list if its there then it returns true else it returns false. obj. contains("Steve"); 10) clear(): It is used for removing all the elements of the array list in one go. obj. clear(); 15
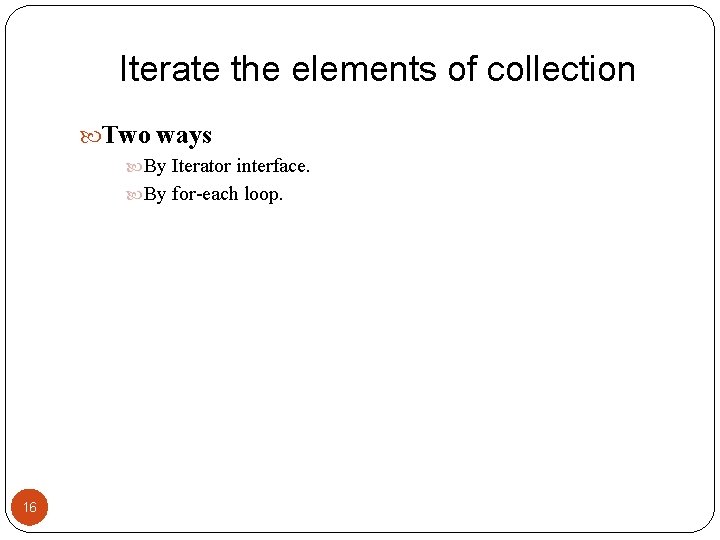
Iterate the elements of collection Two ways By Iterator interface. By for-each loop. 16
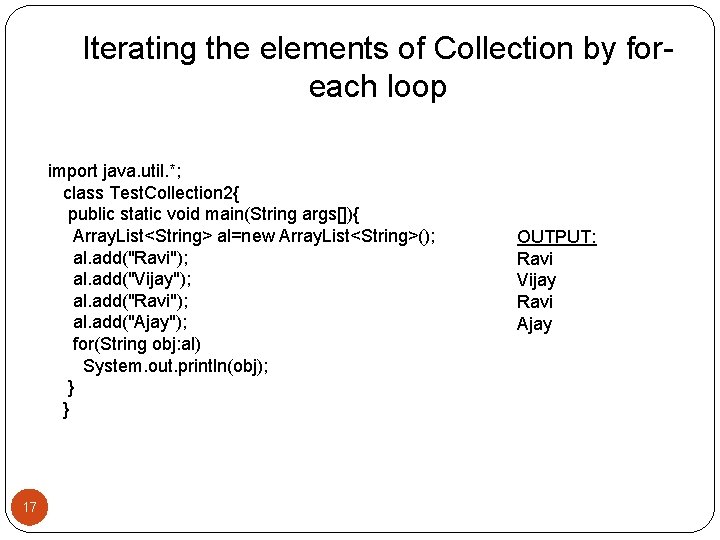
Iterating the elements of Collection by foreach loop import java. util. *; class Test. Collection 2{ public static void main(String args[]){ Array. List<String> al=new Array. List<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); for(String obj: al) System. out. println(obj); } } 17 OUTPUT: Ravi Vijay Ravi Ajay
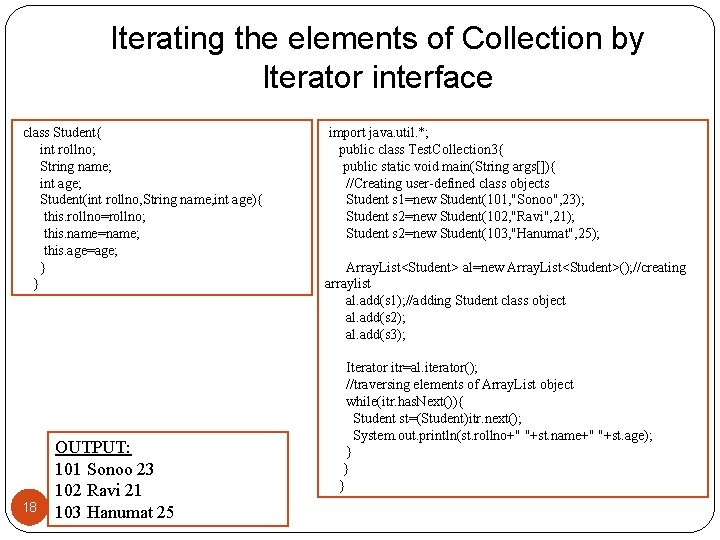
Iterating the elements of Collection by Iterator interface class Student{ int rollno; String name; int age; Student(int rollno, String name, int age){ this. rollno=rollno; this. name=name; this. age=age; } } 18 OUTPUT: 101 Sonoo 23 102 Ravi 21 103 Hanumat 25 import java. util. *; public class Test. Collection 3{ public static void main(String args[]){ //Creating user-defined class objects Student s 1=new Student(101, "Sonoo", 23); Student s 2=new Student(102, "Ravi", 21); Student s 2=new Student(103, "Hanumat", 25); Array. List<Student> al=new Array. List<Student>(); //creating arraylist al. add(s 1); //adding Student class object al. add(s 2); al. add(s 3); Iterator itr=al. iterator(); //traversing elements of Array. List object while(itr. has. Next()){ Student st=(Student)itr. next(); System. out. println(st. rollno+" "+st. name+" "+st. age); } } }
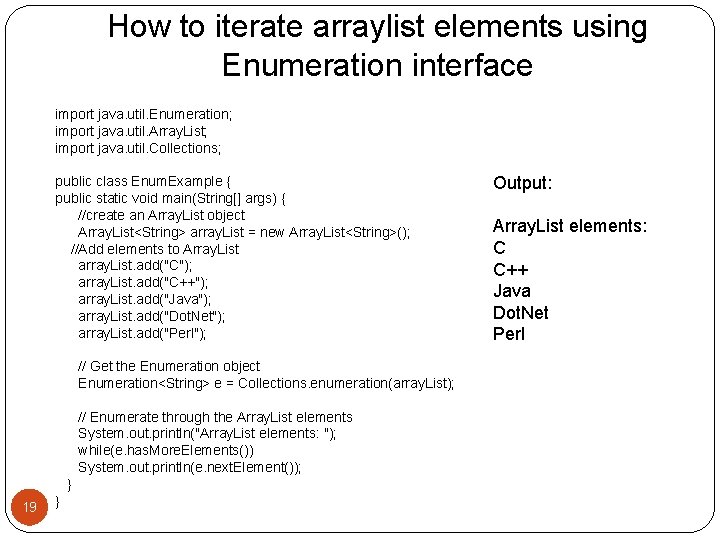
How to iterate arraylist elements using Enumeration interface 19 import java. util. Enumeration; import java. util. Array. List; import java. util. Collections; public class Enum. Example { public static void main(String[] args) { //create an Array. List object Array. List<String> array. List = new Array. List<String>(); //Add elements to Array. List array. List. add("C"); array. List. add("C++"); array. List. add("Java"); array. List. add("Dot. Net"); array. List. add("Perl"); // Get the Enumeration object Enumeration<String> e = Collections. enumeration(array. List); // Enumerate through the Array. List elements System. out. println("Array. List elements: "); while(e. has. More. Elements()) System. out. println(e. next. Element()); } } Output: Array. List elements: C C++ Java Dot. Net Perl
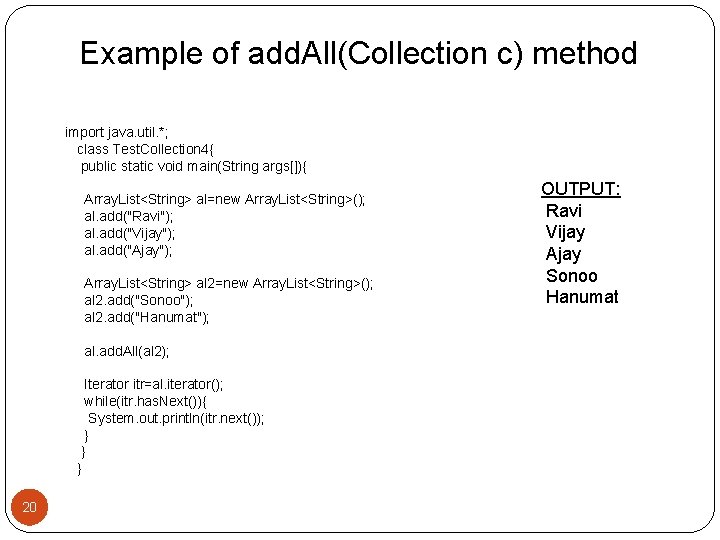
Example of add. All(Collection c) method import java. util. *; class Test. Collection 4{ public static void main(String args[]){ Array. List<String> al=new Array. List<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ajay"); Array. List<String> al 2=new Array. List<String>(); al 2. add("Sonoo"); al 2. add("Hanumat"); al. add. All(al 2); Iterator itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } 20 OUTPUT: Ravi Vijay Ajay Sonoo Hanumat
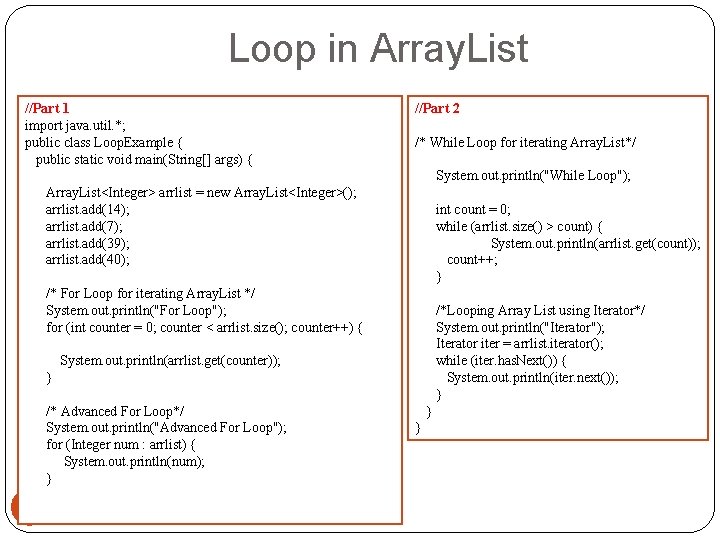
Loop in Array. List //Part 1 import java. util. *; public class Loop. Example { public static void main(String[] args) { //Part 2 /* While Loop for iterating Array. List*/ System. out. println("While Loop"); Array. List<Integer> arrlist = new Array. List<Integer>(); arrlist. add(14); arrlist. add(7); arrlist. add(39); arrlist. add(40); /* For Loop for iterating Array. List */ System. out. println("For Loop"); for (int counter = 0; counter < arrlist. size(); counter++) { System. out. println(arrlist. get(counter)); } /* Advanced For Loop*/ System. out. println("Advanced For Loop"); for (Integer num : arrlist) { System. out. println(num); } 21 int count = 0; while (arrlist. size() > count) { System. out. println(arrlist. get(count)); count++; } /*Looping Array List using Iterator*/ System. out. println("Iterator"); Iterator iter = arrlist. iterator(); while (iter. has. Next()) { System. out. println(iter. next()); } } }
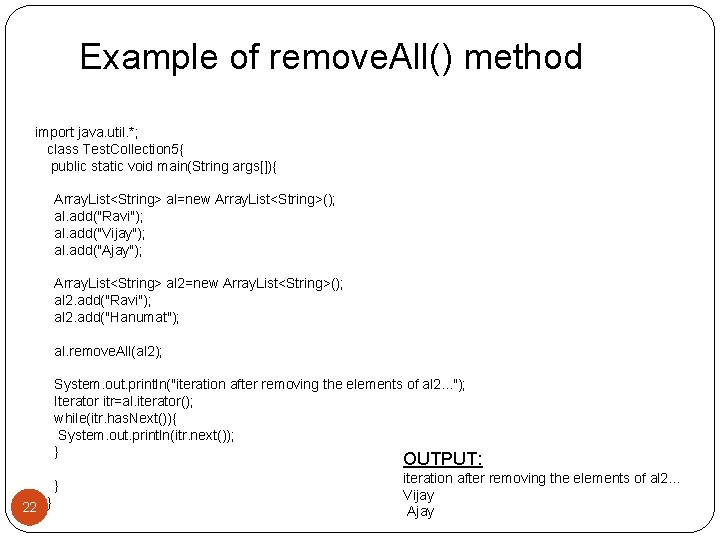
Example of remove. All() method import java. util. *; class Test. Collection 5{ public static void main(String args[]){ Array. List<String> al=new Array. List<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ajay"); Array. List<String> al 2=new Array. List<String>(); al 2. add("Ravi"); al 2. add("Hanumat"); al. remove. All(al 2); System. out. println("iteration after removing the elements of al 2. . . "); Iterator itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } OUTPUT: iteration after removing the elements of al 2. . . } Vijay } 22 Ajay
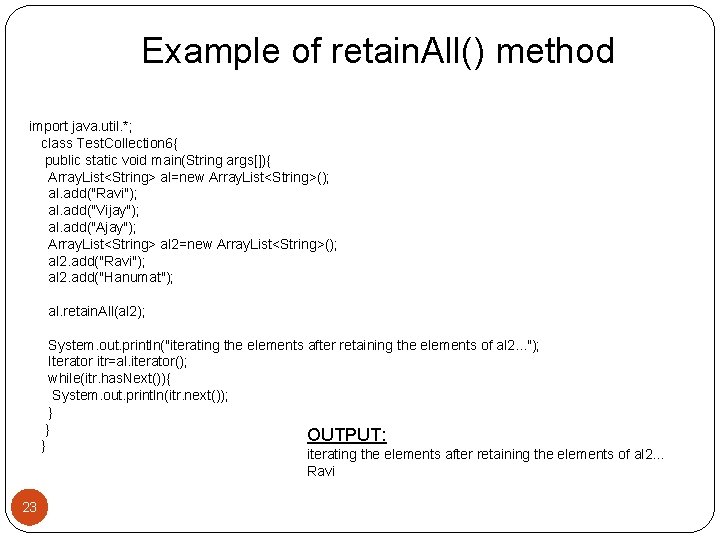
Example of retain. All() method import java. util. *; class Test. Collection 6{ public static void main(String args[]){ Array. List<String> al=new Array. List<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ajay"); Array. List<String> al 2=new Array. List<String>(); al 2. add("Ravi"); al 2. add("Hanumat"); al. retain. All(al 2); System. out. println("iterating the elements after retaining the elements of al 2. . . "); Iterator itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } OUTPUT: } iterating the elements after retaining the elements of al 2. . . Ravi 23
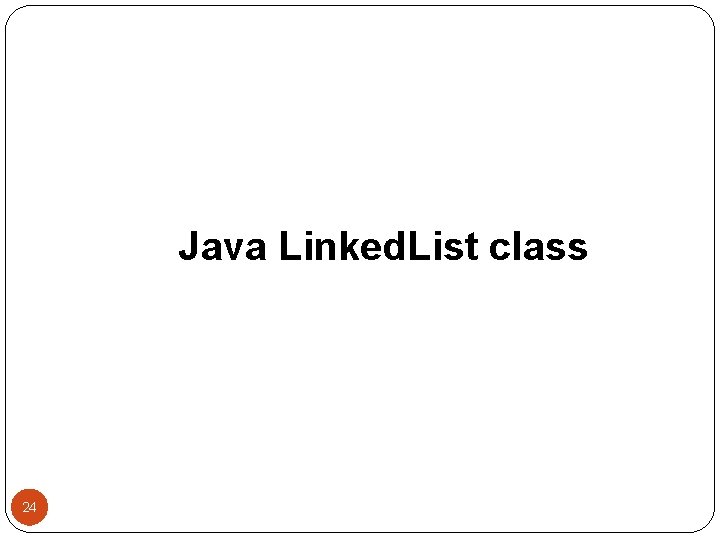
Java Linked. List class 24
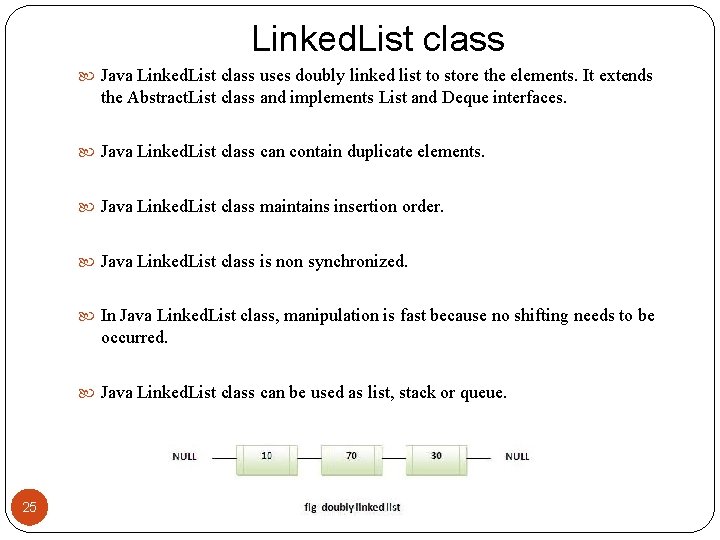
Linked. List class Java Linked. List class uses doubly linked list to store the elements. It extends the Abstract. List class and implements List and Deque interfaces. Java Linked. List class can contain duplicate elements. Java Linked. List class maintains insertion order. Java Linked. List class is non synchronized. In Java Linked. List class, manipulation is fast because no shifting needs to be occurred. Java Linked. List class can be used as list, stack or queue. 25
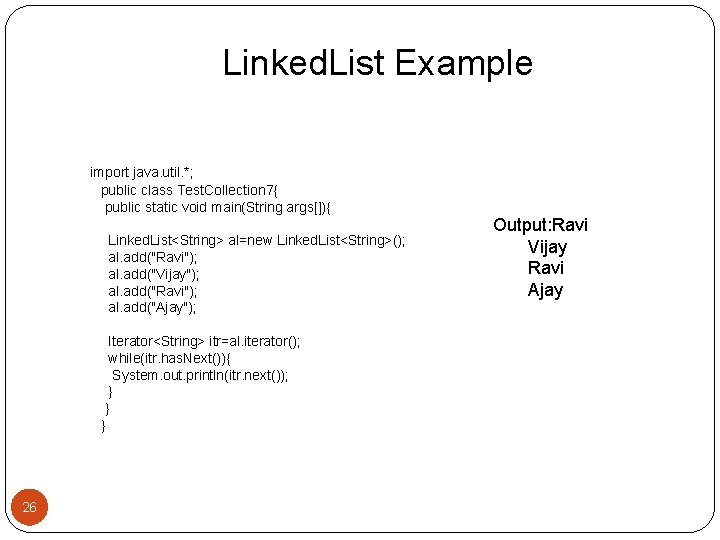
Linked. List Example import java. util. *; public class Test. Collection 7{ public static void main(String args[]){ Linked. List<String> al=new Linked. List<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); Iterator<String> itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } 26 Output: Ravi Vijay Ravi Ajay
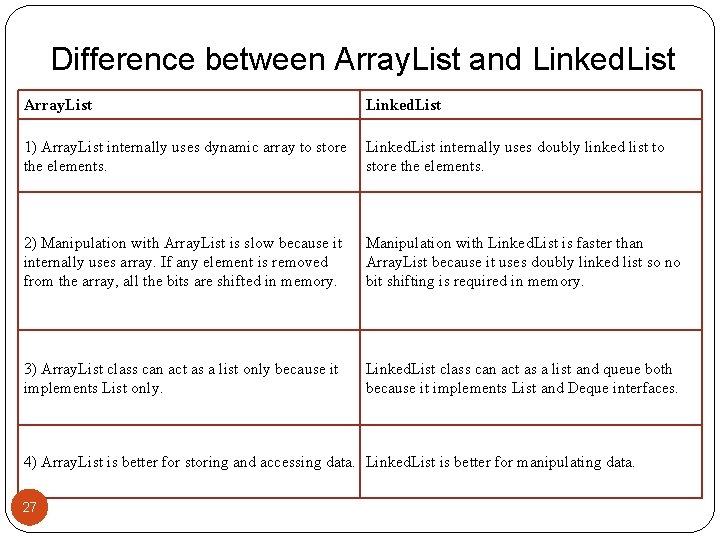
Difference between Array. List and Linked. List Array. List Linked. List 1) Array. List internally uses dynamic array to store Linked. List internally uses doubly linked list to the elements. store the elements. 2) Manipulation with Array. List is slow because it internally uses array. If any element is removed from the array, all the bits are shifted in memory. Manipulation with Linked. List is faster than Array. List because it uses doubly linked list so no bit shifting is required in memory. 3) Array. List class can act as a list only because it implements List only. Linked. List class can act as a list and queue both because it implements List and Deque interfaces. 4) Array. List is better for storing and accessing data. Linked. List is better for manipulating data. 27
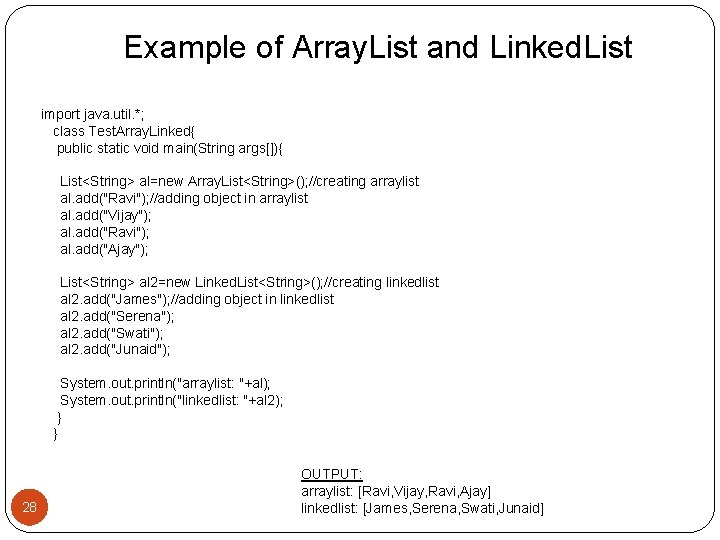
Example of Array. List and Linked. List import java. util. *; class Test. Array. Linked{ public static void main(String args[]){ List<String> al=new Array. List<String>(); //creating arraylist al. add("Ravi"); //adding object in arraylist al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); List<String> al 2=new Linked. List<String>(); //creating linkedlist al 2. add("James"); //adding object in linkedlist al 2. add("Serena"); al 2. add("Swati"); al 2. add("Junaid"); System. out. println("arraylist: "+al); System. out. println("linkedlist: "+al 2); } 28 OUTPUT: arraylist: [Ravi, Vijay, Ravi, Ajay] linkedlist: [James, Serena, Swati, Junaid]
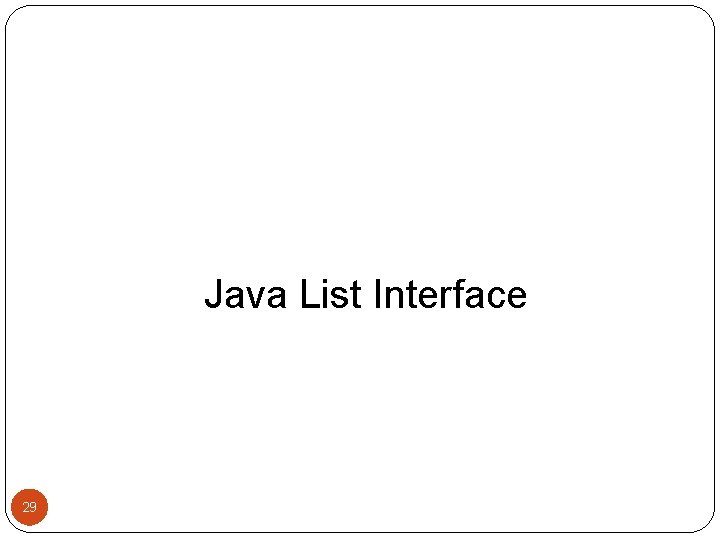
Java List Interface 29
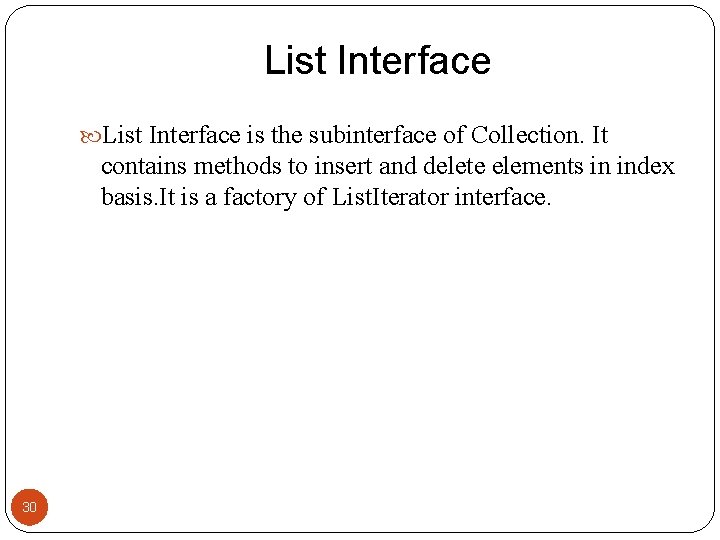
List Interface is the subinterface of Collection. It contains methods to insert and delete elements in index basis. It is a factory of List. Iterator interface. 30
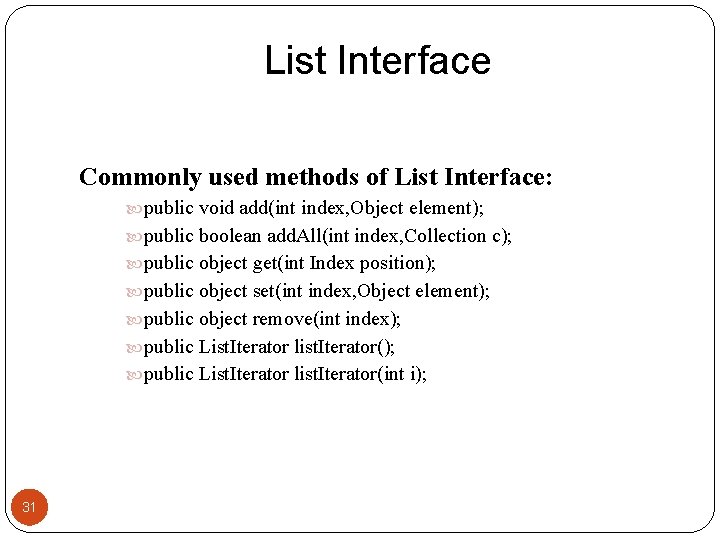
List Interface Commonly used methods of List Interface: public void add(int index, Object element); public boolean add. All(int index, Collection c); public object get(int Index position); public object set(int index, Object element); public object remove(int index); public List. Iterator list. Iterator(int i); 31
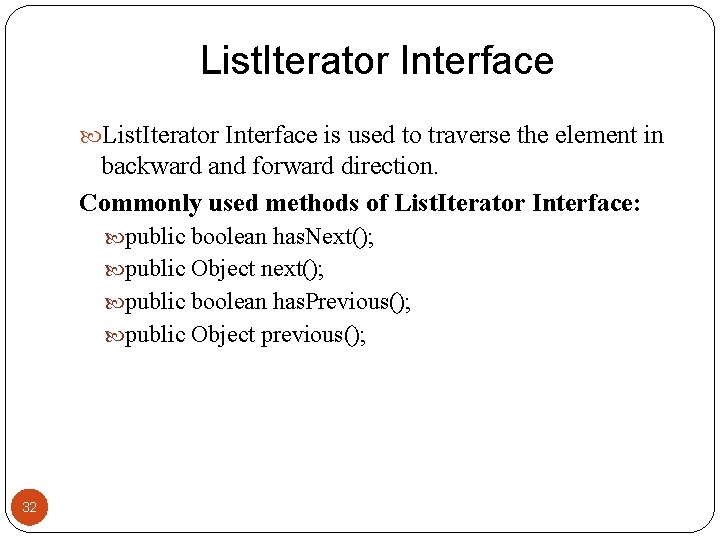
List. Iterator Interface is used to traverse the element in backward and forward direction. Commonly used methods of List. Iterator Interface: public boolean has. Next(); public Object next(); public boolean has. Previous(); public Object previous(); 32
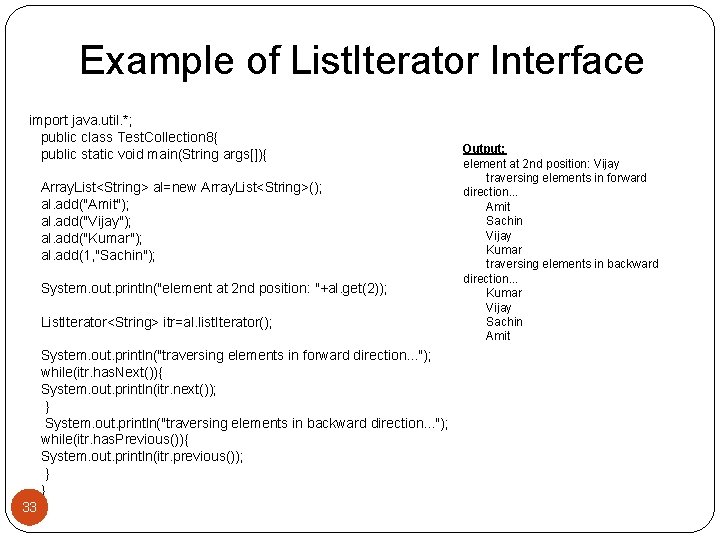
Example of List. Iterator Interface import java. util. *; public class Test. Collection 8{ public static void main(String args[]){ Array. List<String> al=new Array. List<String>(); al. add("Amit"); al. add("Vijay"); al. add("Kumar"); al. add(1, "Sachin"); System. out. println("element at 2 nd position: "+al. get(2)); List. Iterator<String> itr=al. list. Iterator(); System. out. println("traversing elements in forward direction. . . "); while(itr. has. Next()){ System. out. println(itr. next()); } System. out. println("traversing elements in backward direction. . . "); while(itr. has. Previous()){ System. out. println(itr. previous()); } } } 33 Output: element at 2 nd position: Vijay traversing elements in forward direction. . . Amit Sachin Vijay Kumar traversing elements in backward direction. . . Kumar Vijay Sachin Amit
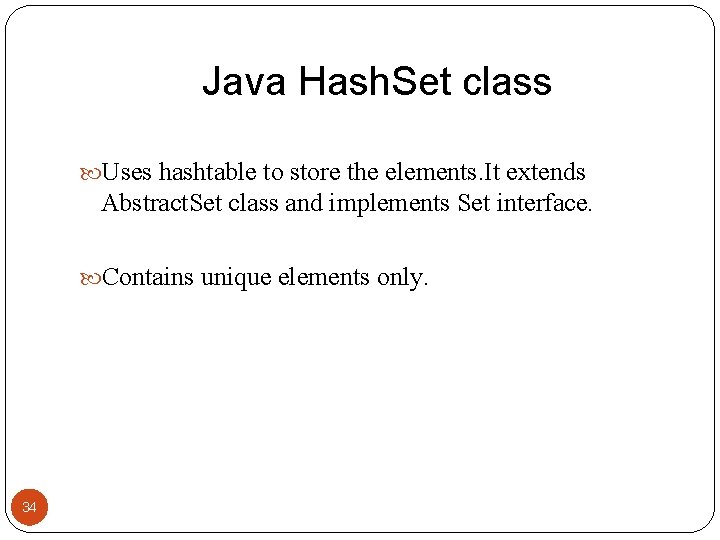
Java Hash. Set class Uses hashtable to store the elements. It extends Abstract. Set class and implements Set interface. Contains unique elements only. 34
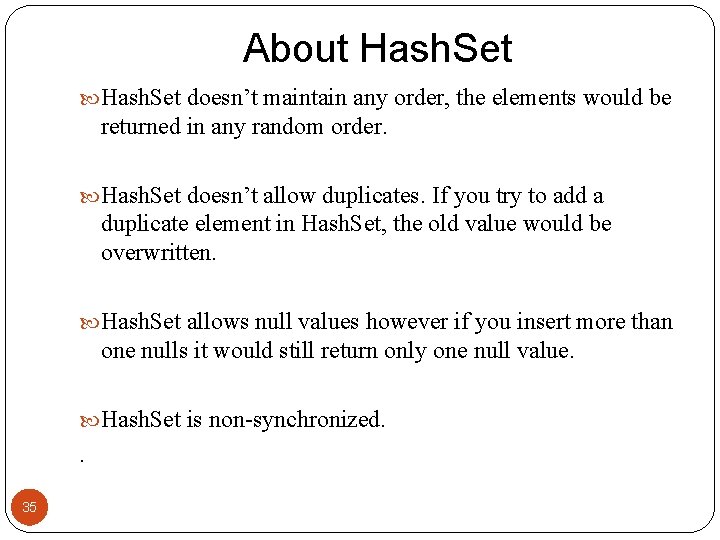
About Hash. Set doesn’t maintain any order, the elements would be returned in any random order. Hash. Set doesn’t allow duplicates. If you try to add a duplicate element in Hash. Set, the old value would be overwritten. Hash. Set allows null values however if you insert more than one nulls it would still return only one null value. Hash. Set is non-synchronized. . 35
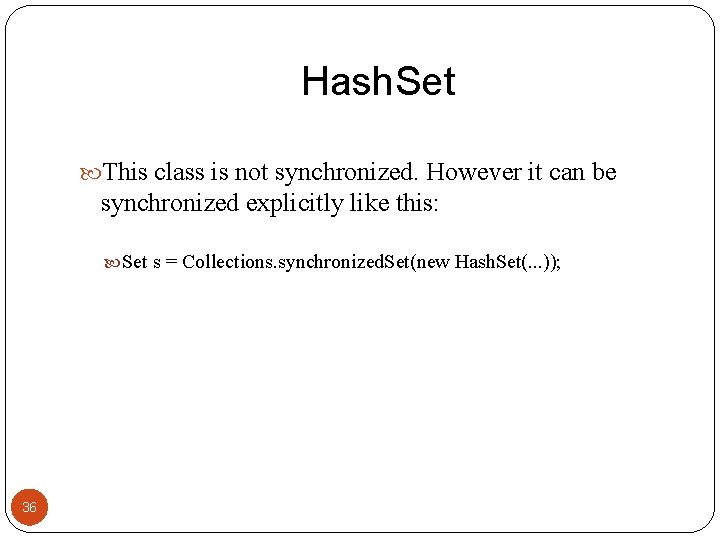
Hash. Set This class is not synchronized. However it can be synchronized explicitly like this: Set s = Collections. synchronized. Set(new Hash. Set(. . . )); 36
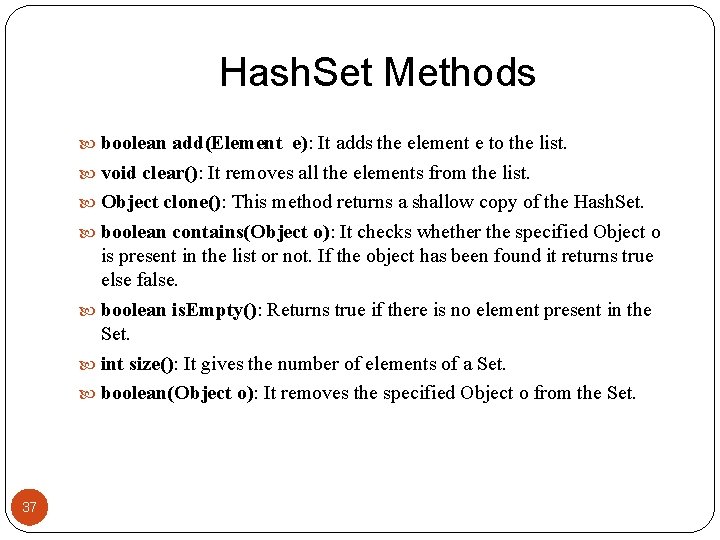
Hash. Set Methods boolean add(Element e): It adds the element e to the list. void clear(): It removes all the elements from the list. Object clone(): This method returns a shallow copy of the Hash. Set. boolean contains(Object o): It checks whether the specified Object o is present in the list or not. If the object has been found it returns true else false. boolean is. Empty(): Returns true if there is no element present in the Set. int size(): It gives the number of elements of a Set. boolean(Object o): It removes the specified Object o from the Set. 37
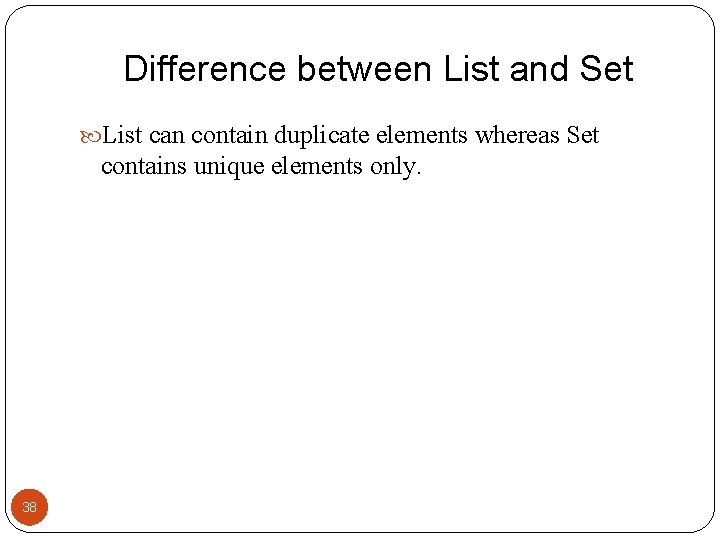
Difference between List and Set List can contain duplicate elements whereas Set contains unique elements only. 38
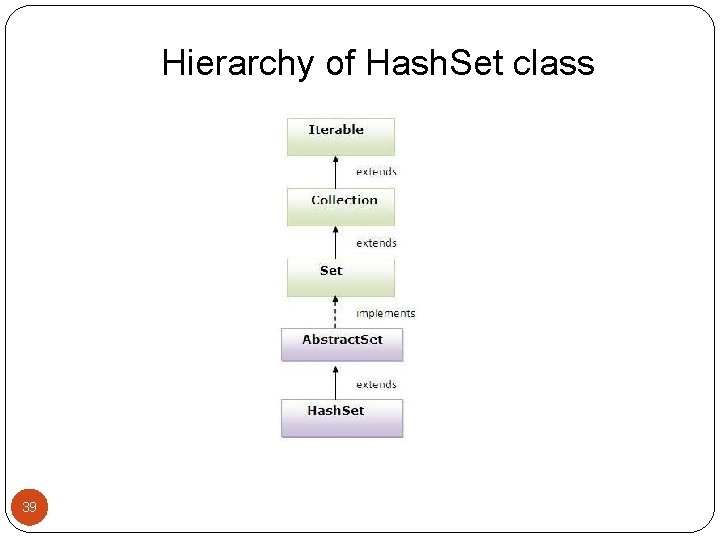
Hierarchy of Hash. Set class 39
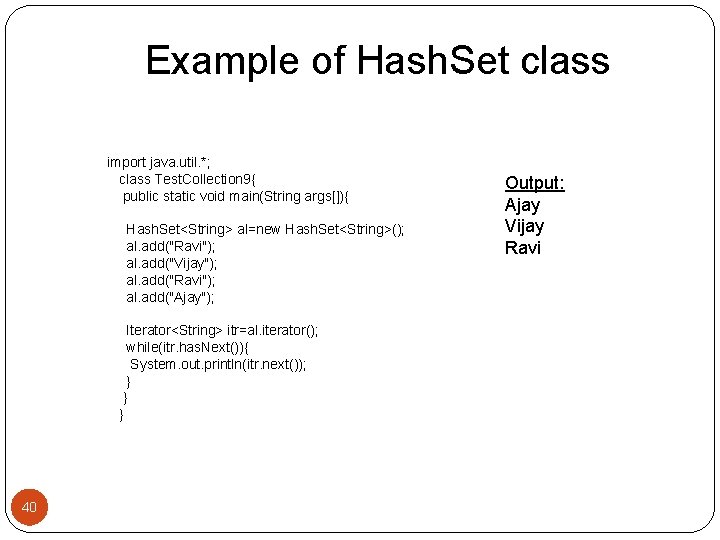
Example of Hash. Set class import java. util. *; class Test. Collection 9{ public static void main(String args[]){ Hash. Set<String> al=new Hash. Set<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); Iterator<String> itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } 40 Output: Ajay Vijay Ravi
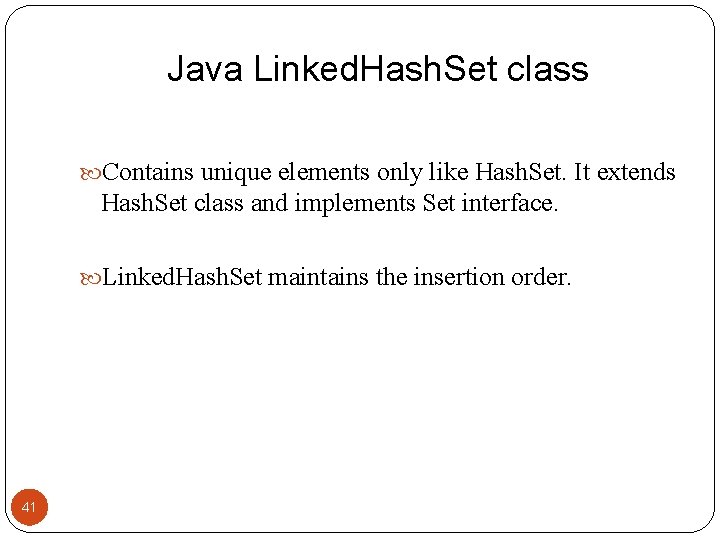
Java Linked. Hash. Set class Contains unique elements only like Hash. Set. It extends Hash. Set class and implements Set interface. Linked. Hash. Set maintains the insertion order. 41
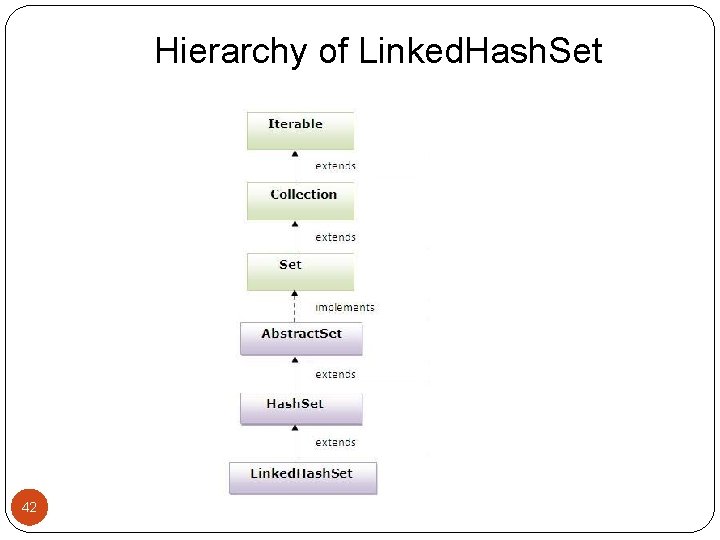
Hierarchy of Linked. Hash. Set 42
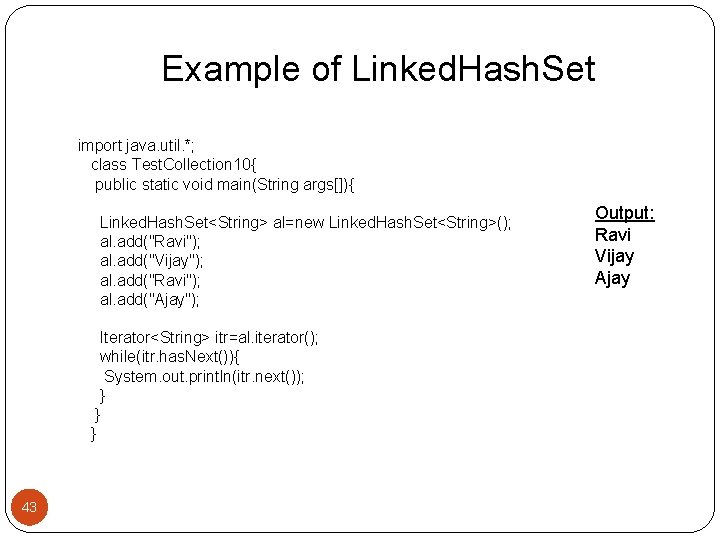
Example of Linked. Hash. Set import java. util. *; class Test. Collection 10{ public static void main(String args[]){ Linked. Hash. Set<String> al=new Linked. Hash. Set<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); Iterator<String> itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } 43 Output: Ravi Vijay Ajay
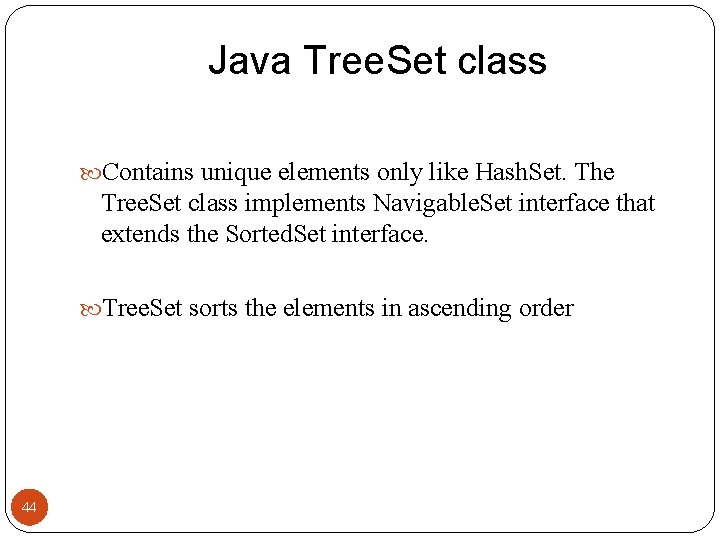
Java Tree. Set class Contains unique elements only like Hash. Set. The Tree. Set class implements Navigable. Set interface that extends the Sorted. Set interface. Tree. Set sorts the elements in ascending order 44
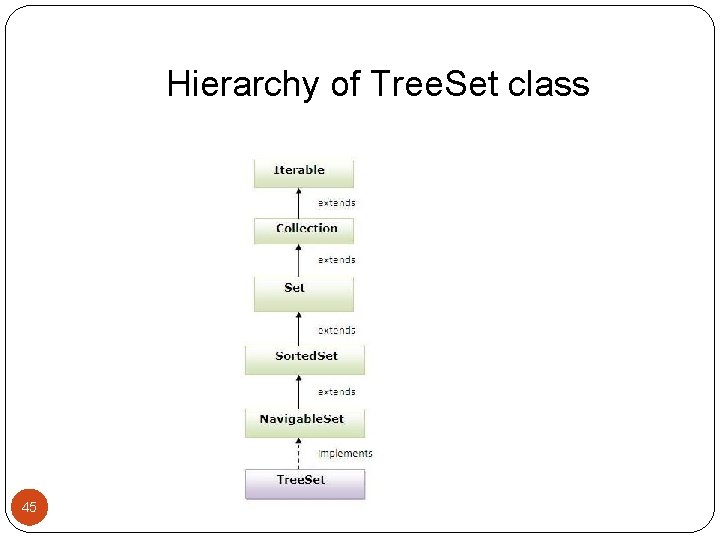
Hierarchy of Tree. Set class 45
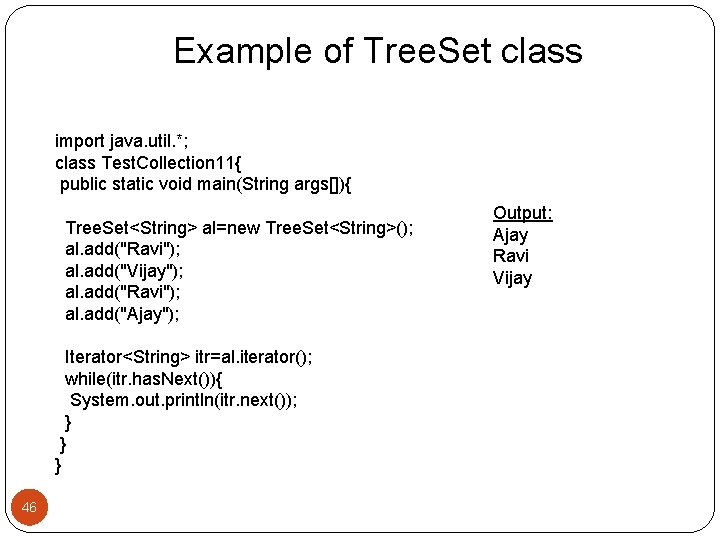
Example of Tree. Set class import java. util. *; class Test. Collection 11{ public static void main(String args[]){ Tree. Set<String> al=new Tree. Set<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); Iterator<String> itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } 46 Output: Ajay Ravi Vijay
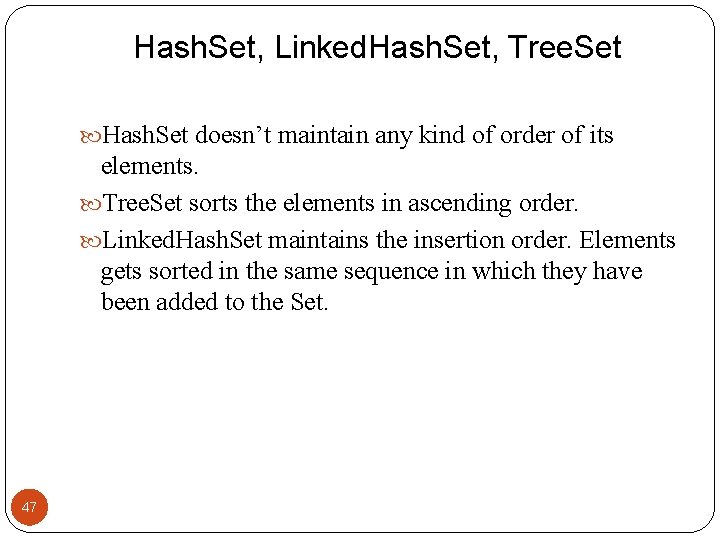
Hash. Set, Linked. Hash. Set, Tree. Set Hash. Set doesn’t maintain any kind of order of its elements. Tree. Set sorts the elements in ascending order. Linked. Hash. Set maintains the insertion order. Elements gets sorted in the same sequence in which they have been added to the Set. 47
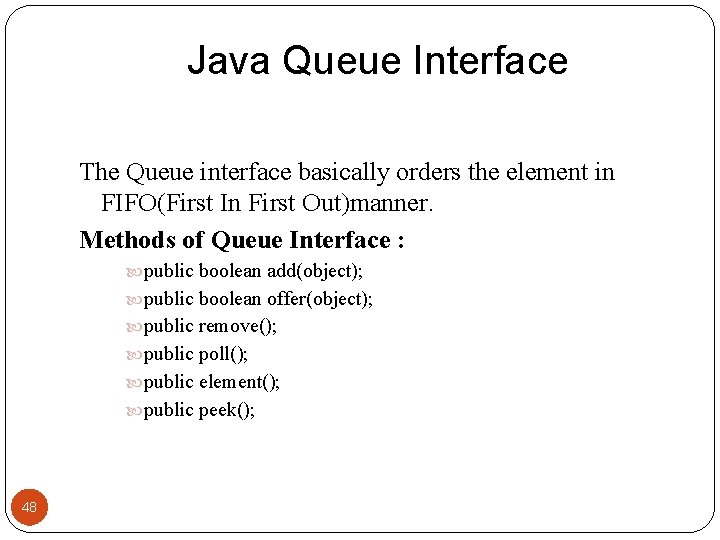
Java Queue Interface The Queue interface basically orders the element in FIFO(First In First Out)manner. Methods of Queue Interface : public boolean add(object); public boolean offer(object); public remove(); public poll(); public element(); public peek(); 48
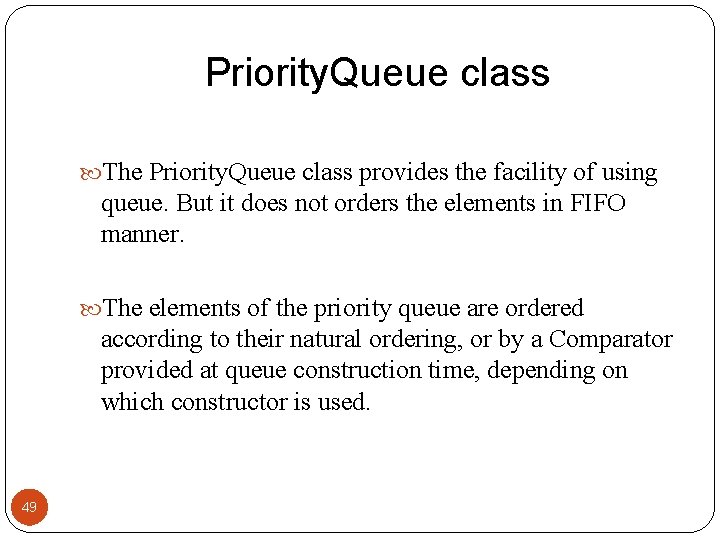
Priority. Queue class The Priority. Queue class provides the facility of using queue. But it does not orders the elements in FIFO manner. The elements of the priority queue are ordered according to their natural ordering, or by a Comparator provided at queue construction time, depending on which constructor is used. 49
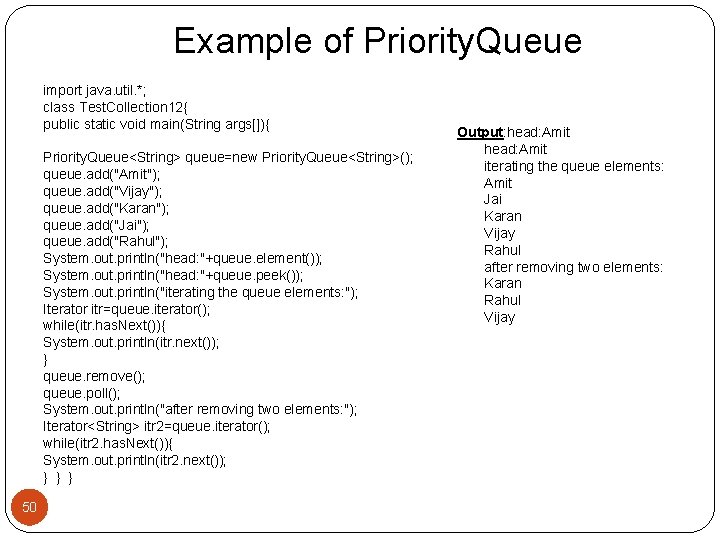
Example of Priority. Queue import java. util. *; class Test. Collection 12{ public static void main(String args[]){ Priority. Queue<String> queue=new Priority. Queue<String>(); queue. add("Amit"); queue. add("Vijay"); queue. add("Karan"); queue. add("Jai"); queue. add("Rahul"); System. out. println("head: "+queue. element()); System. out. println("head: "+queue. peek()); System. out. println("iterating the queue elements: "); Iterator itr=queue. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } queue. remove(); queue. poll(); System. out. println("after removing two elements: "); Iterator<String> itr 2=queue. iterator(); while(itr 2. has. Next()){ System. out. println(itr 2. next()); } } } 50 Output: head: Amit iterating the queue elements: Amit Jai Karan Vijay Rahul after removing two elements: Karan Rahul Vijay
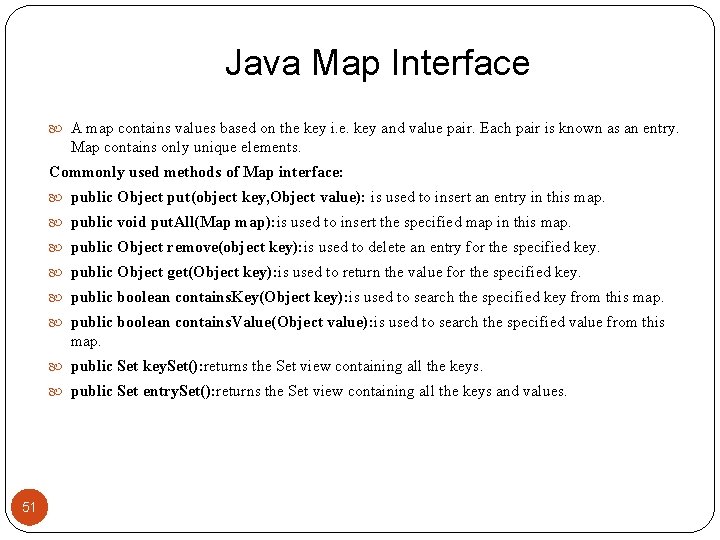
Java Map Interface A map contains values based on the key i. e. key and value pair. Each pair is known as an entry. Map contains only unique elements. Commonly used methods of Map interface: public Object put(object key, Object value): is used to insert an entry in this map. public void put. All(Map map): is used to insert the specified map in this map. public Object remove(object key): is used to delete an entry for the specified key. public Object get(Object key): is used to return the value for the specified key. public boolean contains. Key(Object key): is used to search the specified key from this map. public boolean contains. Value(Object value): is used to search the specified value from this map. public Set key. Set(): returns the Set view containing all the keys. public Set entry. Set(): returns the Set view containing all the keys and values. 51
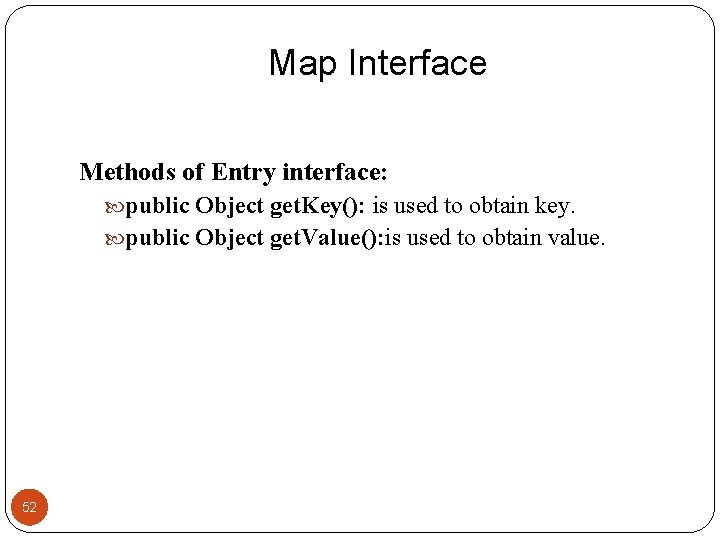
Map Interface Methods of Entry interface: public Object get. Key(): is used to obtain key. public Object get. Value(): is used to obtain value. 52
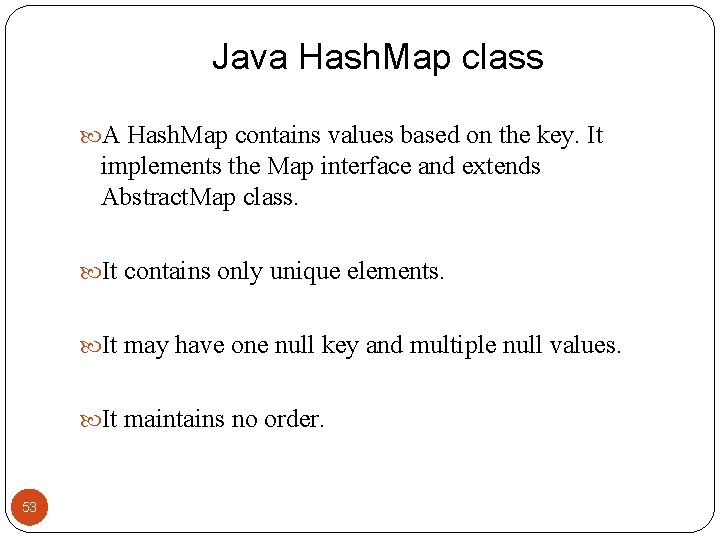
Java Hash. Map class A Hash. Map contains values based on the key. It implements the Map interface and extends Abstract. Map class. It contains only unique elements. It may have one null key and multiple null values. It maintains no order. 53
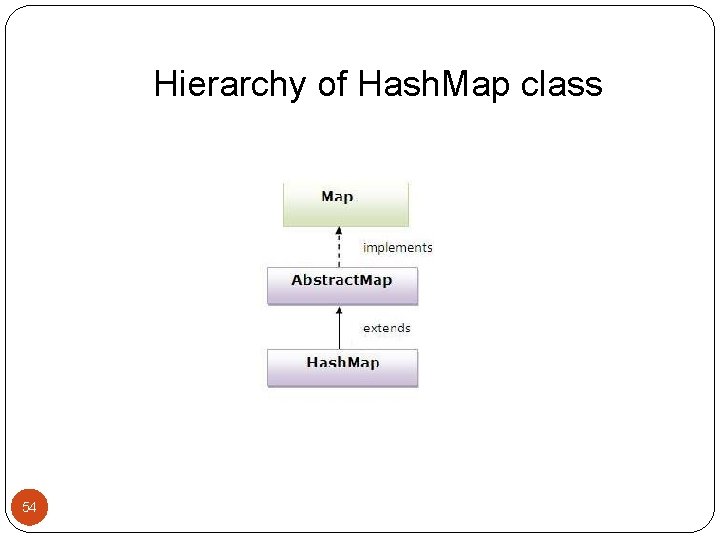
Hierarchy of Hash. Map class 54
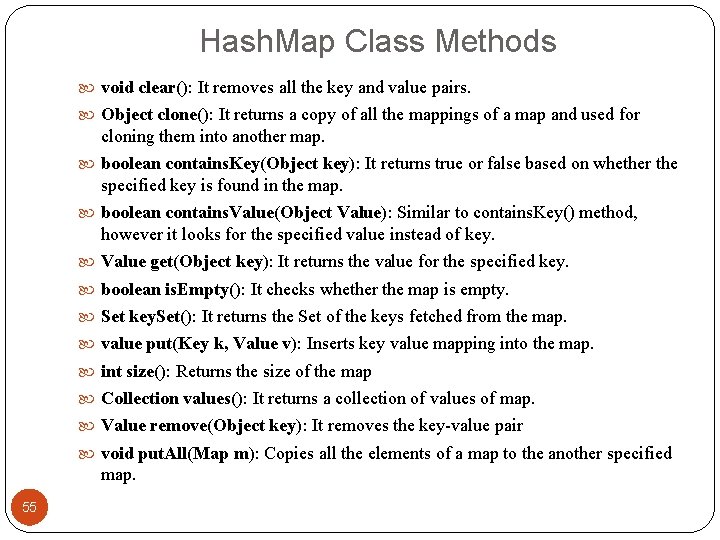
Hash. Map Class Methods void clear(): It removes all the key and value pairs. Object clone(): It returns a copy of all the mappings of a map and used for cloning them into another map. boolean contains. Key(Object key): It returns true or false based on whether the specified key is found in the map. boolean contains. Value(Object Value): Similar to contains. Key() method, however it looks for the specified value instead of key. Value get(Object key): It returns the value for the specified key. boolean is. Empty(): It checks whether the map is empty. Set key. Set(): It returns the Set of the keys fetched from the map. value put(Key k, Value v): Inserts key value mapping into the map. int size(): Returns the size of the map Collection values(): It returns a collection of values of map. Value remove(Object key): It removes the key-value pair void put. All(Map m): Copies all the elements of a map to the another specified map. 55
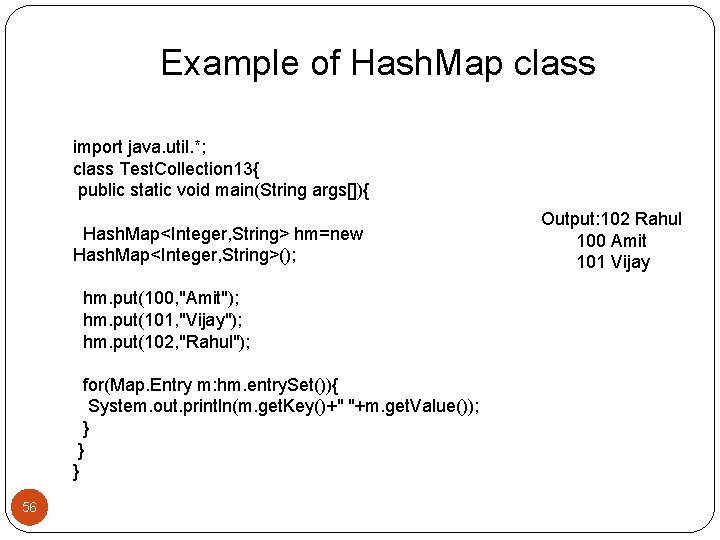
Example of Hash. Map class import java. util. *; class Test. Collection 13{ public static void main(String args[]){ Hash. Map<Integer, String> hm=new Hash. Map<Integer, String>(); hm. put(100, "Amit"); hm. put(101, "Vijay"); hm. put(102, "Rahul"); for(Map. Entry m: hm. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); } } } 56 Output: 102 Rahul 100 Amit 101 Vijay
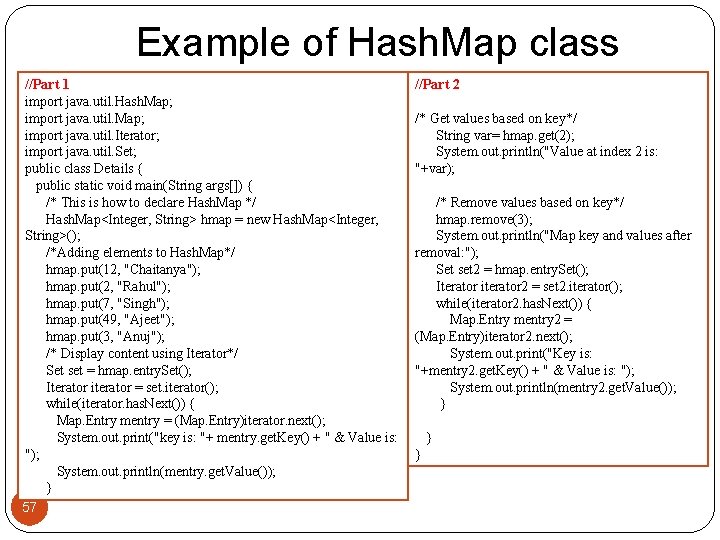
Example of Hash. Map class //Part 1 import java. util. Hash. Map; import java. util. Iterator; import java. util. Set; public class Details { public static void main(String args[]) { /* This is how to declare Hash. Map */ Hash. Map<Integer, String> hmap = new Hash. Map<Integer, String>(); /*Adding elements to Hash. Map*/ hmap. put(12, "Chaitanya"); hmap. put(2, "Rahul"); hmap. put(7, "Singh"); hmap. put(49, "Ajeet"); hmap. put(3, "Anuj"); /* Display content using Iterator*/ Set set = hmap. entry. Set(); Iterator iterator = set. iterator(); while(iterator. has. Next()) { Map. Entry mentry = (Map. Entry)iterator. next(); System. out. print("key is: "+ mentry. get. Key() + " & Value is: "); System. out. println(mentry. get. Value()); } 57 //Part 2 /* Get values based on key*/ String var= hmap. get(2); System. out. println("Value at index 2 is: "+var); /* Remove values based on key*/ hmap. remove(3); System. out. println("Map key and values after removal: "); Set set 2 = hmap. entry. Set(); Iterator iterator 2 = set 2. iterator(); while(iterator 2. has. Next()) { Map. Entry mentry 2 = (Map. Entry)iterator 2. next(); System. out. print("Key is: "+mentry 2. get. Key() + " & Value is: "); System. out. println(mentry 2. get. Value()); } } }
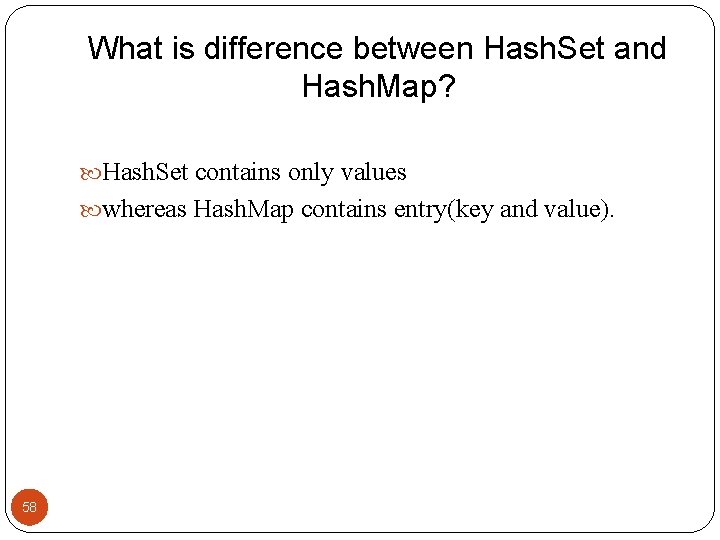
What is difference between Hash. Set and Hash. Map? Hash. Set contains only values whereas Hash. Map contains entry(key and value). 58
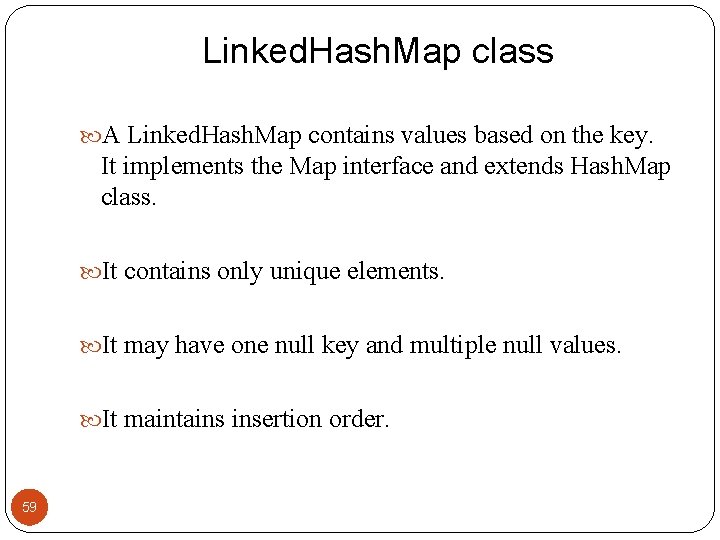
Linked. Hash. Map class A Linked. Hash. Map contains values based on the key. It implements the Map interface and extends Hash. Map class. It contains only unique elements. It may have one null key and multiple null values. It maintains insertion order. 59
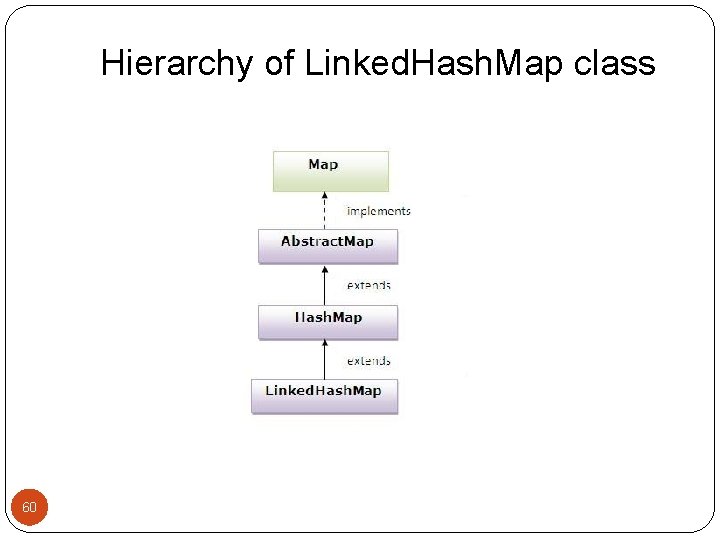
Hierarchy of Linked. Hash. Map class 60
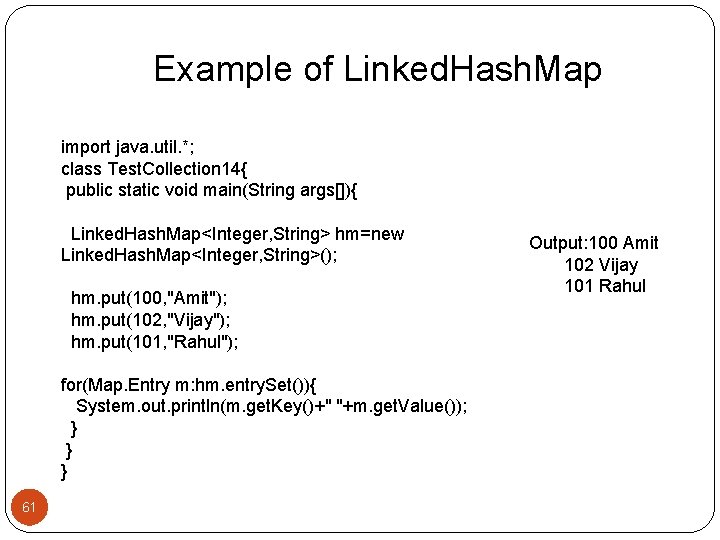
Example of Linked. Hash. Map import java. util. *; class Test. Collection 14{ public static void main(String args[]){ Linked. Hash. Map<Integer, String> hm=new Linked. Hash. Map<Integer, String>(); hm. put(100, "Amit"); hm. put(102, "Vijay"); hm. put(101, "Rahul"); for(Map. Entry m: hm. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); } } } 61 Output: 100 Amit 102 Vijay 101 Rahul
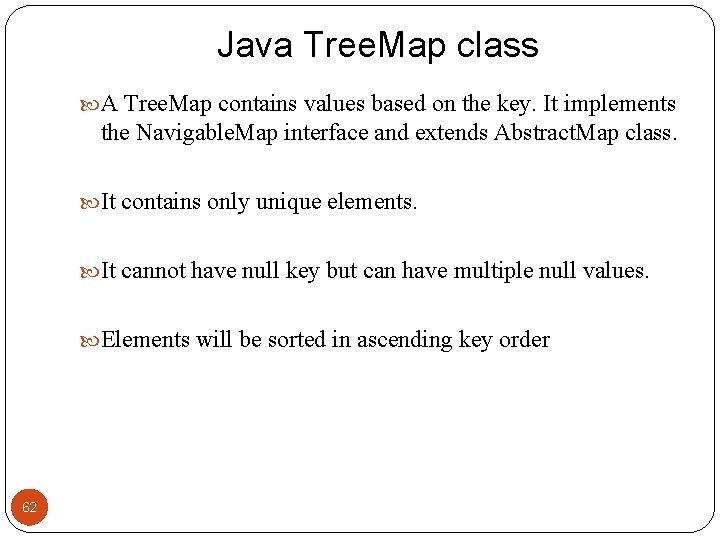
Java Tree. Map class A Tree. Map contains values based on the key. It implements the Navigable. Map interface and extends Abstract. Map class. It contains only unique elements. It cannot have null key but can have multiple null values. Elements will be sorted in ascending key order 62
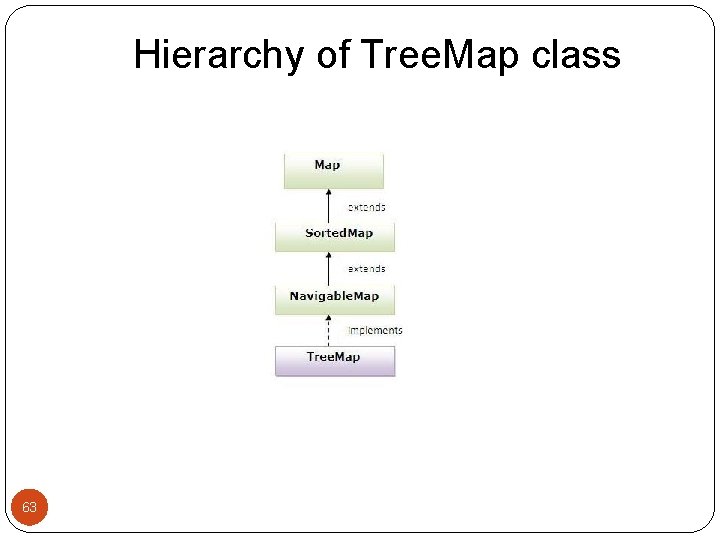
Hierarchy of Tree. Map class 63
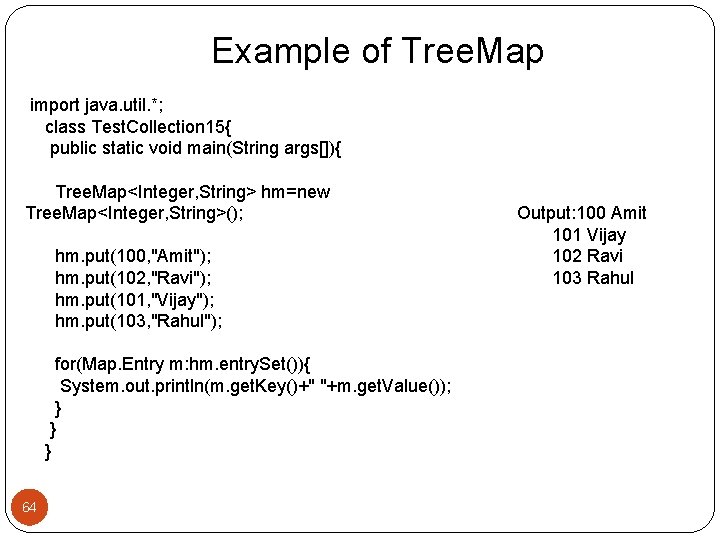
Example of Tree. Map import java. util. *; class Test. Collection 15{ public static void main(String args[]){ Tree. Map<Integer, String> hm=new Tree. Map<Integer, String>(); hm. put(100, "Amit"); hm. put(102, "Ravi"); hm. put(101, "Vijay"); hm. put(103, "Rahul"); for(Map. Entry m: hm. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); } } } 64 Output: 100 Amit 101 Vijay 102 Ravi 103 Rahul
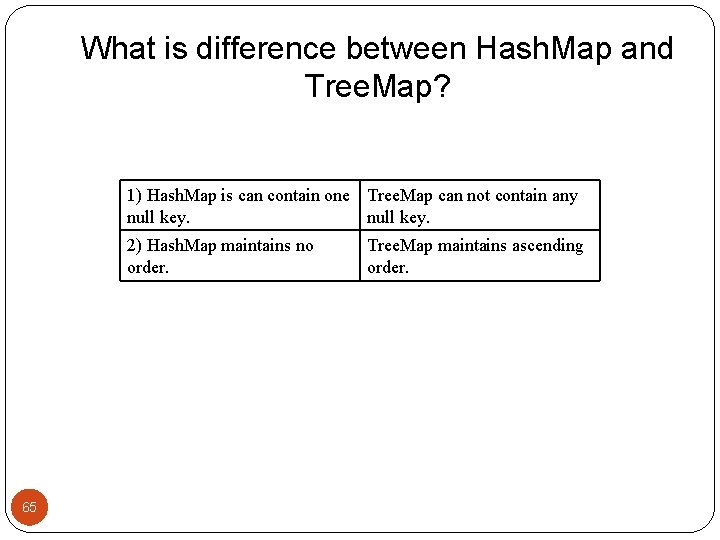
What is difference between Hash. Map and Tree. Map? 1) Hash. Map is can contain one Tree. Map can not contain any null key. 2) Hash. Map maintains no order. 65 Tree. Map maintains ascending order.
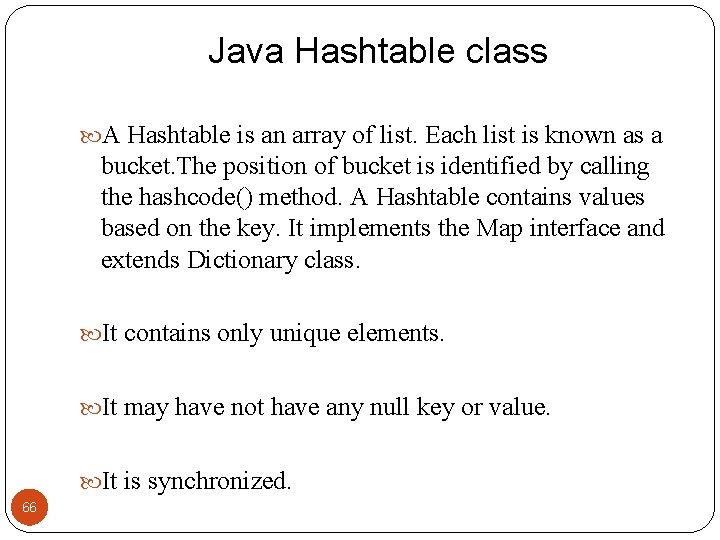
Java Hashtable class A Hashtable is an array of list. Each list is known as a bucket. The position of bucket is identified by calling the hashcode() method. A Hashtable contains values based on the key. It implements the Map interface and extends Dictionary class. It contains only unique elements. It may have not have any null key or value. It is synchronized. 66
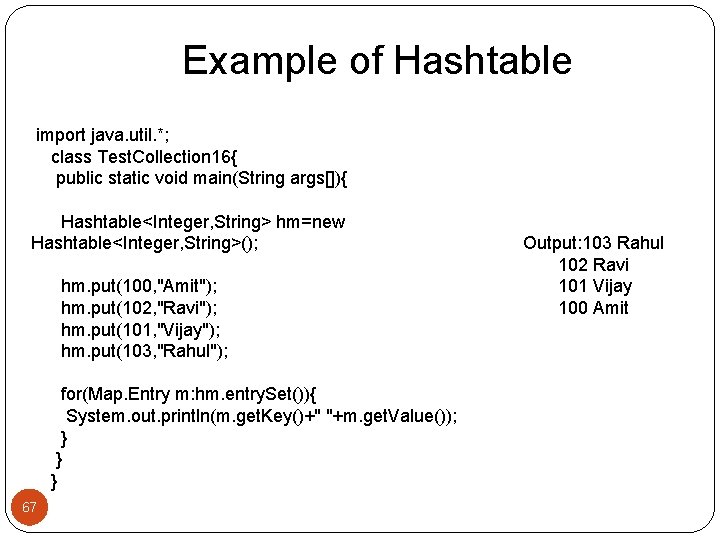
Example of Hashtable import java. util. *; class Test. Collection 16{ public static void main(String args[]){ Hashtable<Integer, String> hm=new Hashtable<Integer, String>(); hm. put(100, "Amit"); hm. put(102, "Ravi"); hm. put(101, "Vijay"); hm. put(103, "Rahul"); for(Map. Entry m: hm. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); } } } 67 Output: 103 Rahul 102 Ravi 101 Vijay 100 Amit
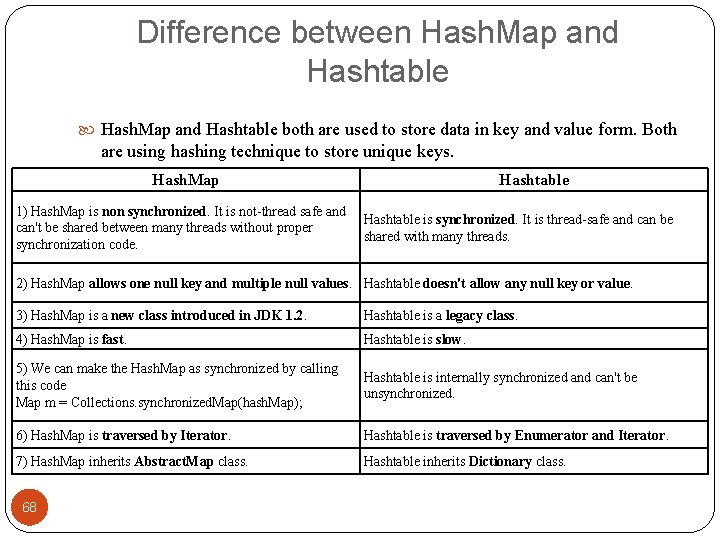
Difference between Hash. Map and Hashtable both are used to store data in key and value form. Both are using hashing technique to store unique keys. Hash. Map 1) Hash. Map is non synchronized. It is not-thread safe and can't be shared between many threads without proper synchronization code. Hashtable is synchronized. It is thread-safe and can be shared with many threads. 2) Hash. Map allows one null key and multiple null values. Hashtable doesn't allow any null key or value. 3) Hash. Map is a new class introduced in JDK 1. 2. Hashtable is a legacy class. 4) Hash. Map is fast. Hashtable is slow. 5) We can make the Hash. Map as synchronized by calling this code Map m = Collections. synchronized. Map(hash. Map); Hashtable is internally synchronized and can't be unsynchronized. 6) Hash. Map is traversed by Iterator. Hashtable is traversed by Enumerator and Iterator. 7) Hash. Map inherits Abstract. Map class. Hashtable inherits Dictionary class. 68
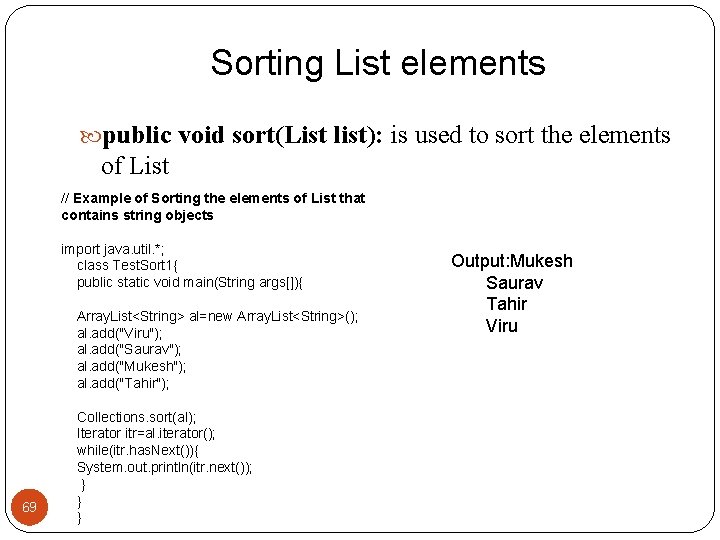
Sorting List elements public void sort(List list): is used to sort the elements of List // Example of Sorting the elements of List that contains string objects 69 import java. util. *; class Test. Sort 1{ public static void main(String args[]){ Array. List<String> al=new Array. List<String>(); al. add("Viru"); al. add("Saurav"); al. add("Mukesh"); al. add("Tahir"); Collections. sort(al); Iterator itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } Output: Mukesh Saurav Tahir Viru
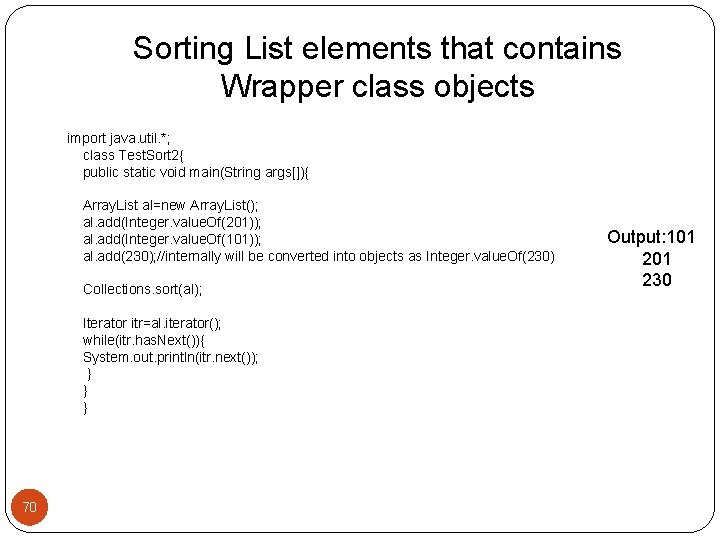
Sorting List elements that contains Wrapper class objects import java. util. *; class Test. Sort 2{ public static void main(String args[]){ Array. List al=new Array. List(); al. add(Integer. value. Of(201)); al. add(Integer. value. Of(101)); al. add(230); //internally will be converted into objects as Integer. value. Of(230) Collections. sort(al); Iterator itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } 70 Output: 101 230
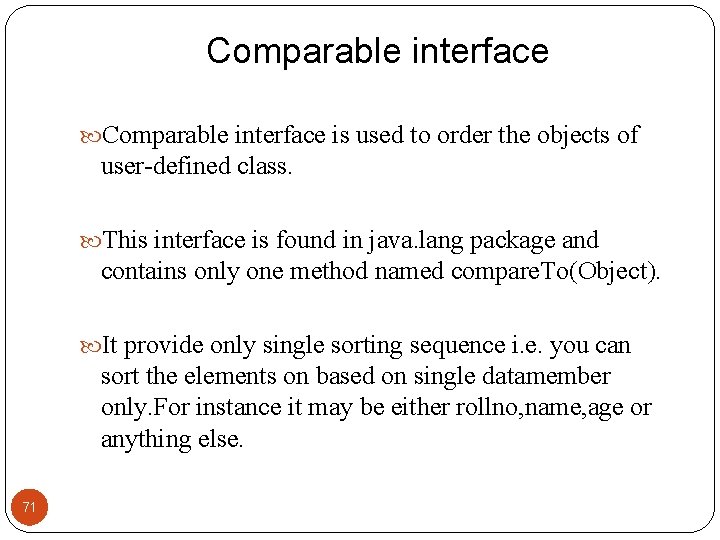
Comparable interface is used to order the objects of user-defined class. This interface is found in java. lang package and contains only one method named compare. To(Object). It provide only single sorting sequence i. e. you can sort the elements on based on single datamember only. For instance it may be either rollno, name, age or anything else. 71
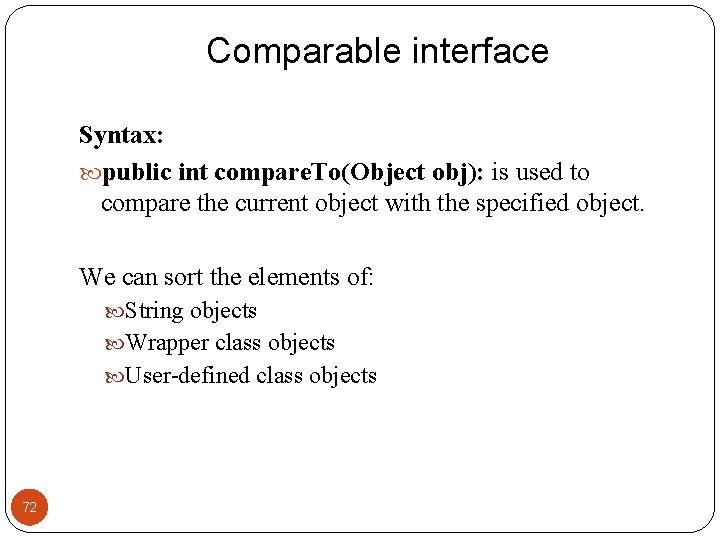
Comparable interface Syntax: public int compare. To(Object obj): is used to compare the current object with the specified object. We can sort the elements of: String objects Wrapper class objects User-defined class objects 72
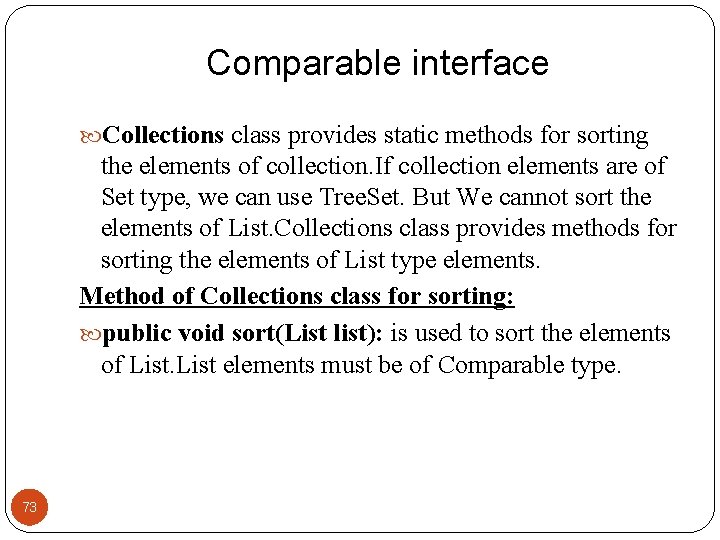
Comparable interface Collections class provides static methods for sorting the elements of collection. If collection elements are of Set type, we can use Tree. Set. But We cannot sort the elements of List. Collections class provides methods for sorting the elements of List type elements. Method of Collections class for sorting: public void sort(List list): is used to sort the elements of List elements must be of Comparable type. 73
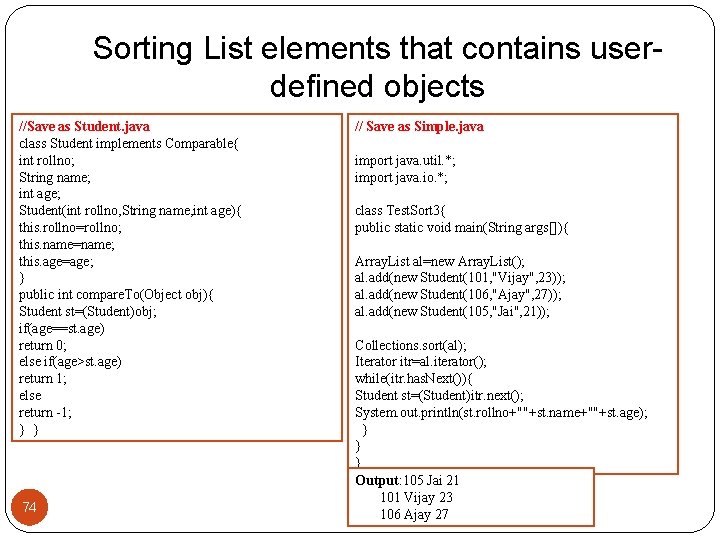
Sorting List elements that contains userdefined objects //Save as Student. java class Student implements Comparable{ int rollno; String name; int age; Student(int rollno, String name, int age){ this. rollno=rollno; this. name=name; this. age=age; } public int compare. To(Object obj){ Student st=(Student)obj; if(age==st. age) return 0; else if(age>st. age) return 1; else return -1; } } 74 // Save as Simple. java import java. util. *; import java. io. *; class Test. Sort 3{ public static void main(String args[]){ Array. List al=new Array. List(); al. add(new Student(101, "Vijay", 23)); al. add(new Student(106, "Ajay", 27)); al. add(new Student(105, "Jai", 21)); Collections. sort(al); Iterator itr=al. iterator(); while(itr. has. Next()){ Student st=(Student)itr. next(); System. out. println(st. rollno+""+st. name+""+st. age); } } } Output: 105 Jai 21 101 Vijay 23 106 Ajay 27
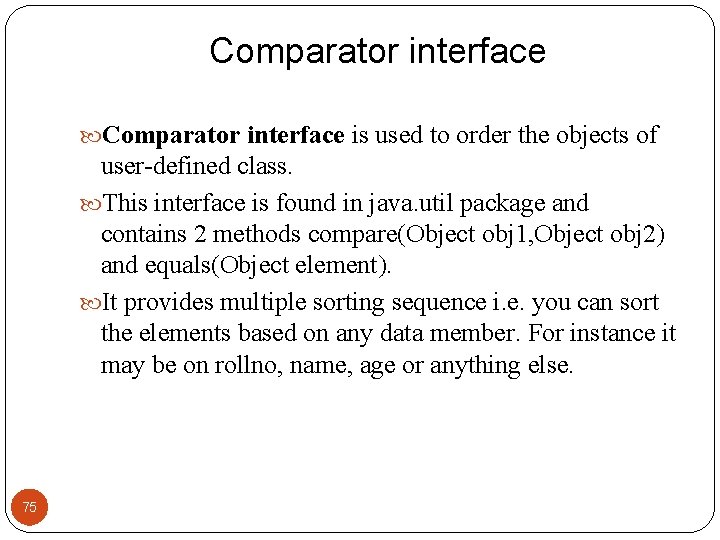
Comparator interface is used to order the objects of user-defined class. This interface is found in java. util package and contains 2 methods compare(Object obj 1, Object obj 2) and equals(Object element). It provides multiple sorting sequence i. e. you can sort the elements based on any data member. For instance it may be on rollno, name, age or anything else. 75
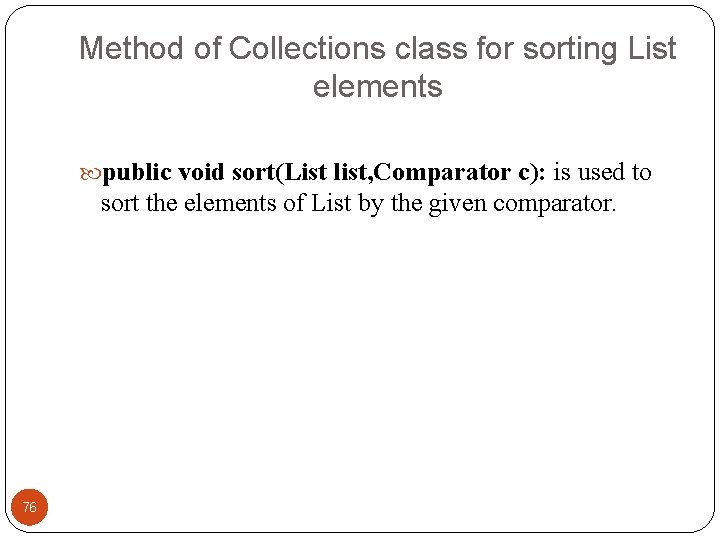
Method of Collections class for sorting List elements public void sort(List list, Comparator c): is used to sort the elements of List by the given comparator. 76
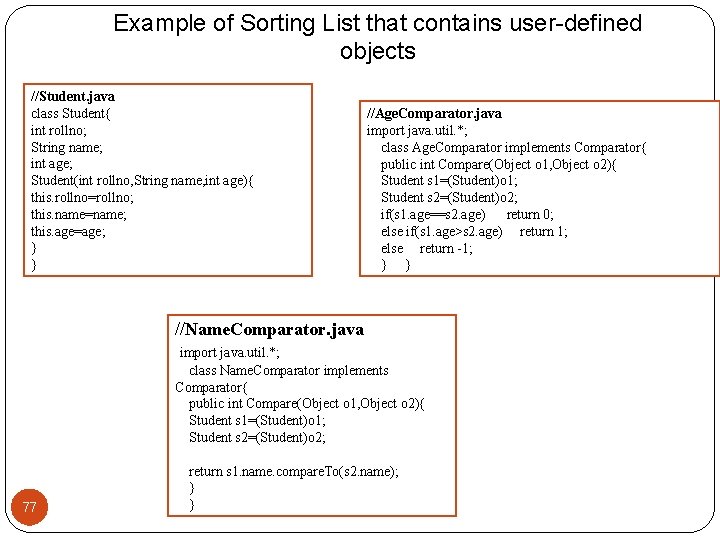
Example of Sorting List that contains user-defined objects //Student. java class Student{ int rollno; String name; int age; Student(int rollno, String name, int age){ this. rollno=rollno; this. name=name; this. age=age; } } //Age. Comparator. java import java. util. *; class Age. Comparator implements Comparator{ public int Compare(Object o 1, Object o 2){ Student s 1=(Student)o 1; Student s 2=(Student)o 2; if(s 1. age==s 2. age) return 0; else if(s 1. age>s 2. age) return 1; else return -1; } } //Name. Comparator. java import java. util. *; 77 class Name. Comparator implements Comparator{ public int Compare(Object o 1, Object o 2){ Student s 1=(Student)o 1; Student s 2=(Student)o 2; return s 1. name. compare. To(s 2. name); } }
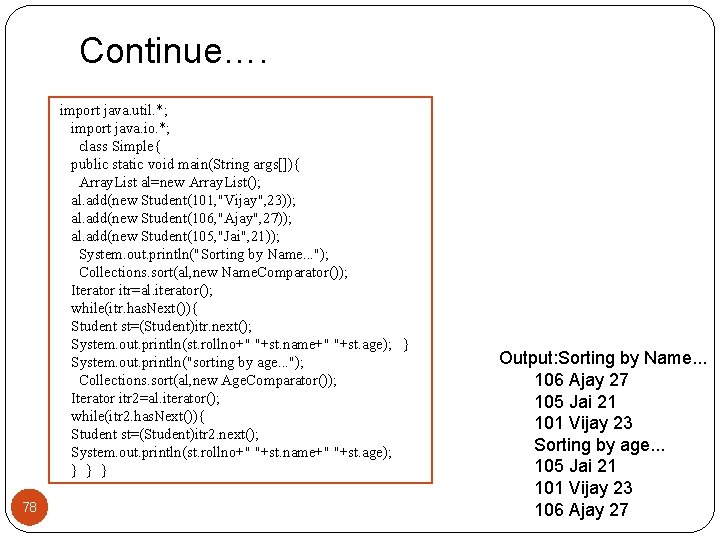
Continue…. import java. util. *; import java. io. *; class Simple{ public static void main(String args[]){ Array. List al=new Array. List(); al. add(new Student(101, "Vijay", 23)); al. add(new Student(106, "Ajay", 27)); al. add(new Student(105, "Jai", 21)); System. out. println("Sorting by Name. . . "); Collections. sort(al, new Name. Comparator()); Iterator itr=al. iterator(); while(itr. has. Next()){ Student st=(Student)itr. next(); System. out. println(st. rollno+" "+st. name+" "+st. age); } System. out. println("sorting by age. . . "); Collections. sort(al, new Age. Comparator()); Iterator itr 2=al. iterator(); while(itr 2. has. Next()){ Student st=(Student)itr 2. next(); System. out. println(st. rollno+" "+st. name+" "+st. age); } } } 78 Output: Sorting by Name. . . 106 Ajay 27 105 Jai 21 101 Vijay 23 Sorting by age. . . 105 Jai 21 101 Vijay 23 106 Ajay 27
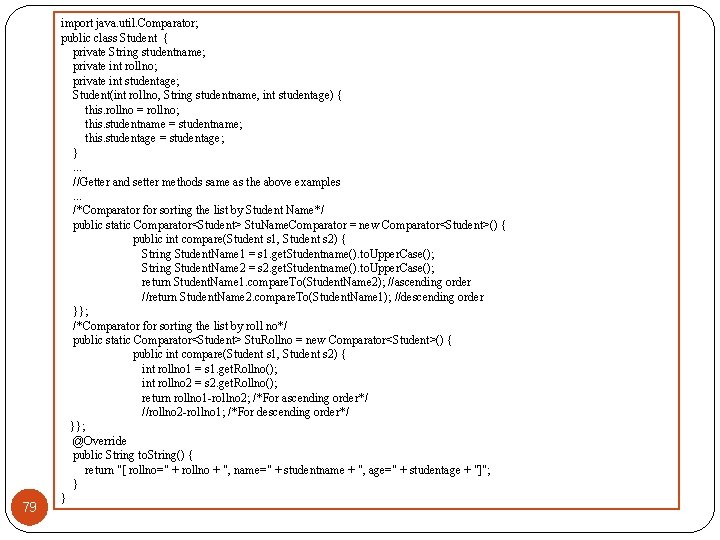
79 import java. util. Comparator; public class Student { private String studentname; private int rollno; private int studentage; Student(int rollno, String studentname, int studentage) { this. rollno = rollno; this. studentname = studentname; this. studentage = studentage; } . . . //Getter and setter methods same as the above examples . . . /*Comparator for sorting the list by Student Name*/ public static Comparator<Student> Stu. Name. Comparator = new Comparator<Student>() { public int compare(Student s 1, Student s 2) { String Student. Name 1 = s 1. get. Studentname(). to. Upper. Case(); String Student. Name 2 = s 2. get. Studentname(). to. Upper. Case(); return Student. Name 1. compare. To(Student. Name 2); //ascending order //return Student. Name 2. compare. To(Student. Name 1); //descending order }}; /*Comparator for sorting the list by roll no*/ public static Comparator<Student> Stu. Rollno = new Comparator<Student>() { public int compare(Student s 1, Student s 2) { int rollno 1 = s 1. get. Rollno(); int rollno 2 = s 2. get. Rollno(); return rollno 1 -rollno 2; /*For ascending order*/ //rollno 2 -rollno 1; /*For descending order*/ }}; @Override public String to. String() { return "[ rollno=" + rollno + ", name=" + studentname + ", age=" + studentage + "]"; } }
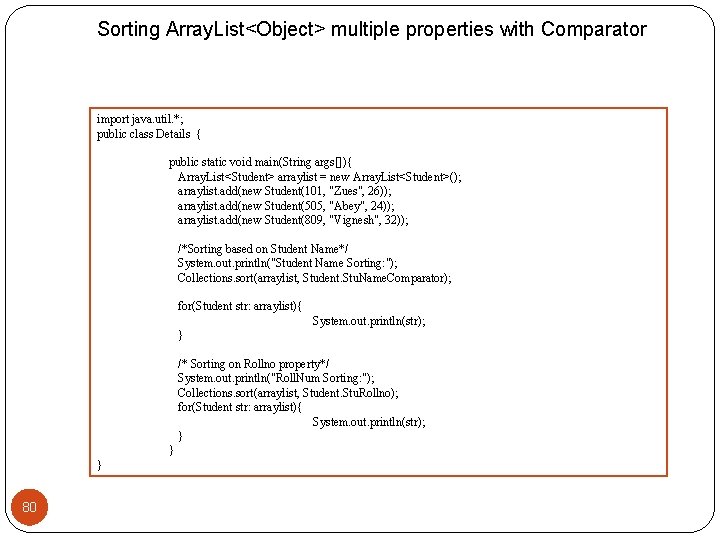
Sorting Array. List<Object> multiple properties with Comparator import java. util. *; public class Details { public static void main(String args[]){ Array. List<Student> arraylist = new Array. List<Student>(); arraylist. add(new Student(101, "Zues", 26)); arraylist. add(new Student(505, "Abey", 24)); arraylist. add(new Student(809, "Vignesh", 32)); /*Sorting based on Student Name*/ System. out. println("Student Name Sorting: "); Collections. sort(arraylist, Student. Stu. Name. Comparator); for(Student str: arraylist){ System. out. println(str); } /* Sorting on Rollno property*/ System. out. println("Roll. Num Sorting: "); Collections. sort(arraylist, Student. Stu. Rollno); for(Student str: arraylist){ System. out. println(str); } } } 80
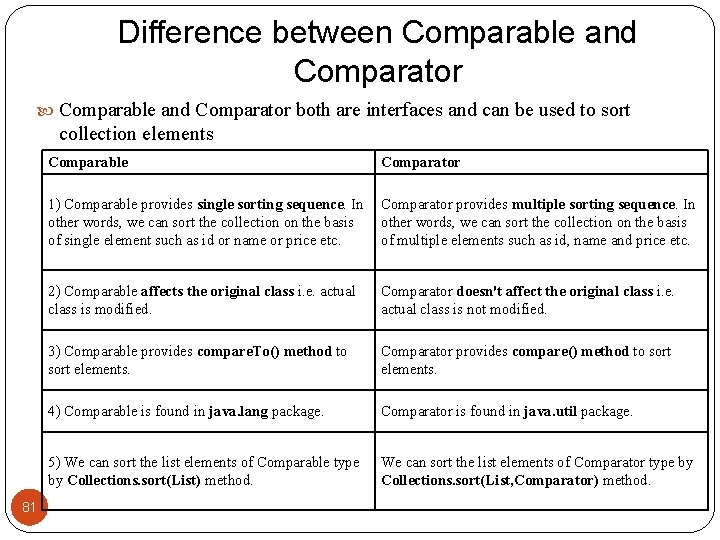
Difference between Comparable and Comparator both are interfaces and can be used to sort collection elements Comparable Comparator 1) Comparable provides single sorting sequence. In Comparator provides multiple sorting sequence. In other words, we can sort the collection on the basis of single element such as id or name or price etc. of multiple elements such as id, name and price etc. 81 2) Comparable affects the original class i. e. actual class is modified. Comparator doesn't affect the original class i. e. actual class is not modified. 3) Comparable provides compare. To() method to sort elements. Comparator provides compare() method to sort elements. 4) Comparable is found in java. lang package. Comparator is found in java. util package. 5) We can sort the list elements of Comparable type by Collections. sort(List) method. We can sort the list elements of Comparator type by Collections. sort(List, Comparator) method.
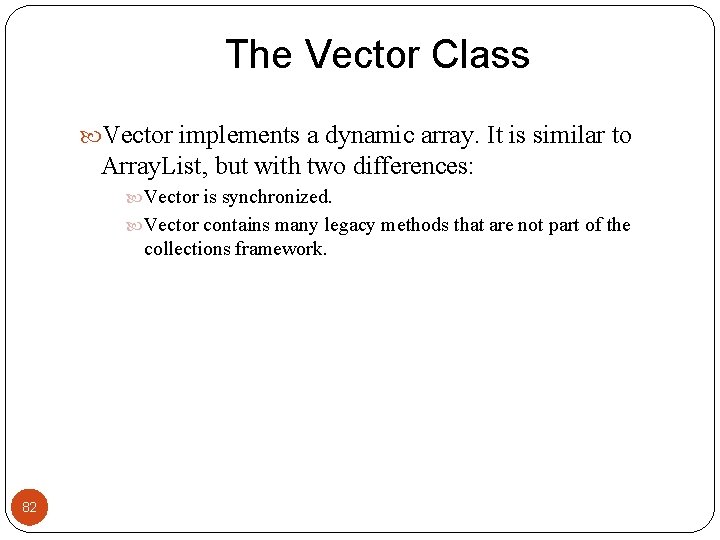
The Vector Class Vector implements a dynamic array. It is similar to Array. List, but with two differences: Vector is synchronized. Vector contains many legacy methods that are not part of the collections framework. 82
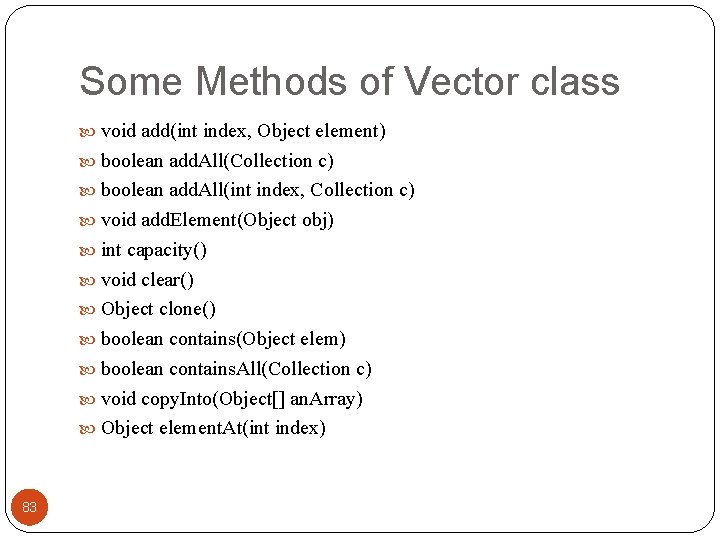
Some Methods of Vector class void add(int index, Object element) boolean add. All(Collection c) boolean add. All(int index, Collection c) void add. Element(Object obj) int capacity() void clear() Object clone() boolean contains(Object elem) boolean contains. All(Collection c) void copy. Into(Object[] an. Array) Object element. At(int index) 83
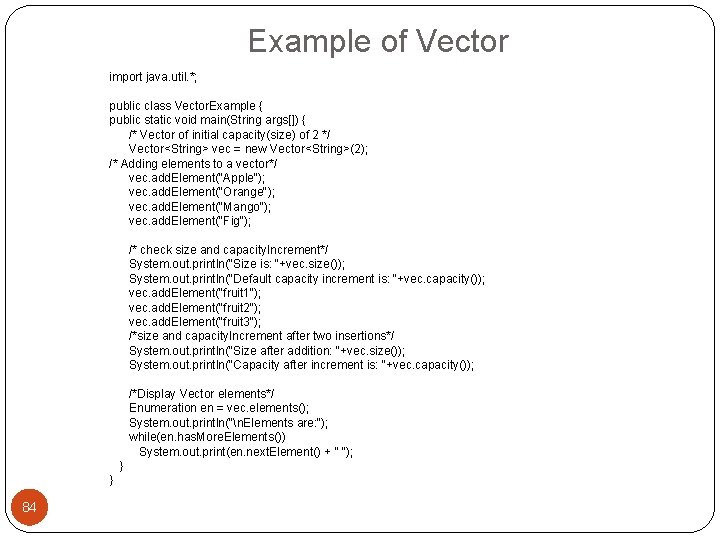
Example of Vector import java. util. *; public class Vector. Example { public static void main(String args[]) { /* Vector of initial capacity(size) of 2 */ Vector<String> vec = new Vector<String>(2); /* Adding elements to a vector*/ vec. add. Element("Apple"); vec. add. Element("Orange"); vec. add. Element("Mango"); vec. add. Element("Fig"); /* check size and capacity. Increment*/ System. out. println("Size is: "+vec. size()); System. out. println("Default capacity increment is: "+vec. capacity()); vec. add. Element("fruit 1"); vec. add. Element("fruit 2"); vec. add. Element("fruit 3"); /*size and capacity. Increment after two insertions*/ System. out. println("Size after addition: "+vec. size()); System. out. println("Capacity after increment is: "+vec. capacity()); /*Display Vector elements*/ Enumeration en = vec. elements(); System. out. println("n. Elements are: "); while(en. has. More. Elements()) System. out. print(en. next. Element() + " "); } } 84
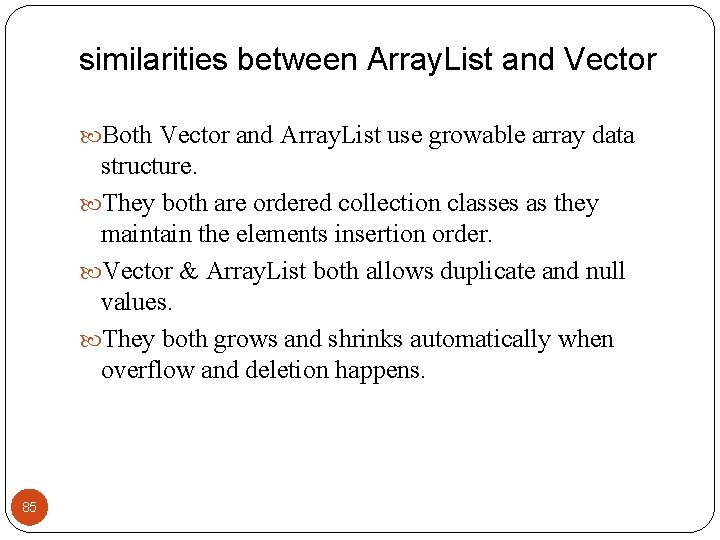
similarities between Array. List and Vector Both Vector and Array. List use growable array data structure. They both are ordered collection classes as they maintain the elements insertion order. Vector & Array. List both allows duplicate and null values. They both grows and shrinks automatically when overflow and deletion happens. 85
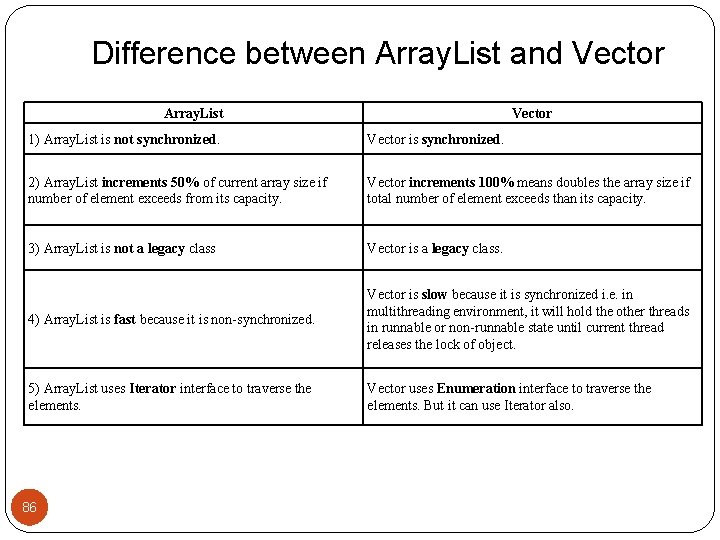
Difference between Array. List and Vector Array. List Vector 1) Array. List is not synchronized. Vector is synchronized. 2) Array. List increments 50% of current array size if number of element exceeds from its capacity. Vector increments 100% means doubles the array size if total number of element exceeds than its capacity. 3) Array. List is not a legacy class Vector is a legacy class. 4) Array. List is fast because it is non-synchronized. Vector is slow because it is synchronized i. e. in multithreading environment, it will hold the other threads in runnable or non-runnable state until current thread releases the lock of object. 5) Array. List uses Iterator interface to traverse the elements. Vector uses Enumeration interface to traverse the elements. But it can use Iterator also. 86
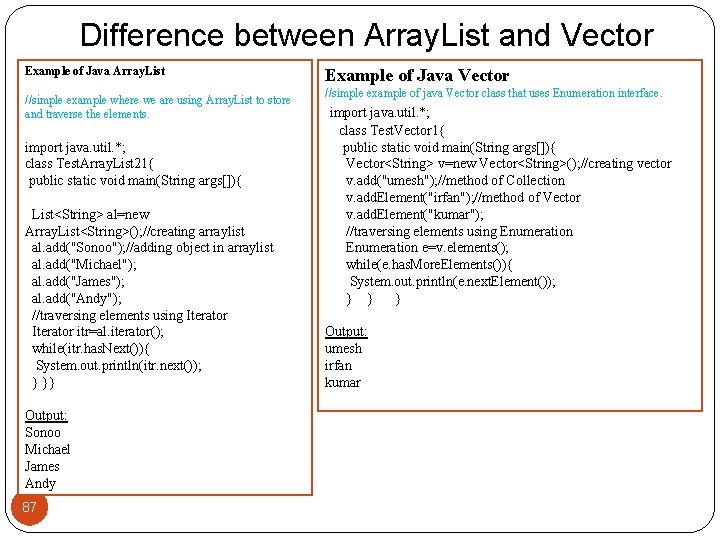
Difference between Array. List and Vector Example of Java Array. List //simple example where we are using Array. List to store and traverse the elements. import java. util. *; class Test. Array. List 21{ public static void main(String args[]){ List<String> al=new Array. List<String>(); //creating arraylist al. add("Sonoo"); //adding object in arraylist al. add("Michael"); al. add("James"); al. add("Andy"); //traversing elements using Iterator itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } }} Output: Sonoo Michael James Andy 87 Example of Java Vector //simple example of java Vector class that uses Enumeration interface. import java. util. *; class Test. Vector 1{ public static void main(String args[]){ Vector<String> v=new Vector<String>(); //creating vector v. add("umesh"); //method of Collection v. add. Element("irfan"); //method of Vector v. add. Element("kumar"); //traversing elements using Enumeration e=v. elements(); while(e. has. More. Elements()){ System. out. println(e. next. Element()); } } } Output: umesh irfan kumar
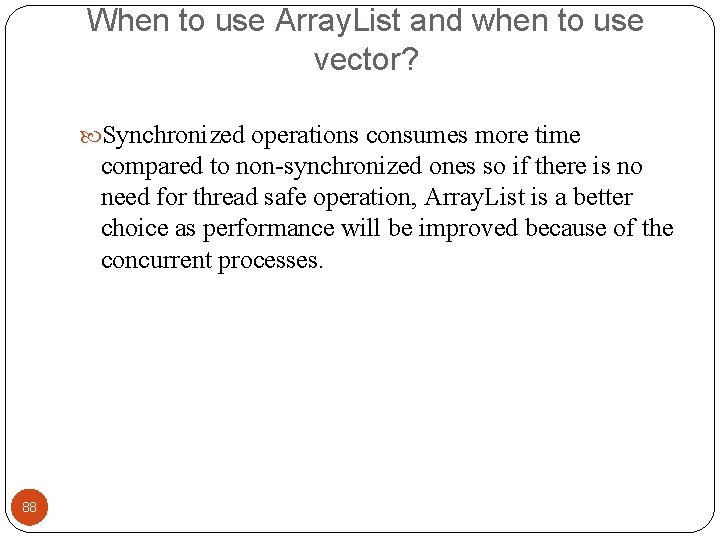
When to use Array. List and when to use vector? Synchronized operations consumes more time compared to non-synchronized ones so if there is no need for thread safe operation, Array. List is a better choice as performance will be improved because of the concurrent processes. 88
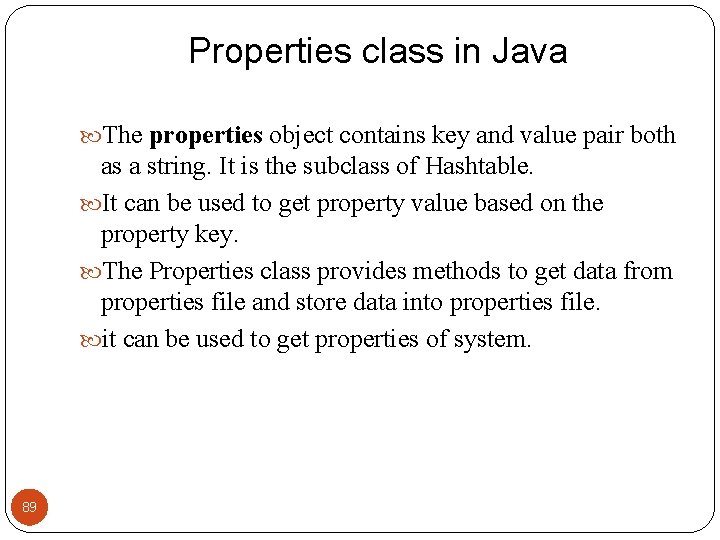
Properties class in Java The properties object contains key and value pair both as a string. It is the subclass of Hashtable. It can be used to get property value based on the property key. The Properties class provides methods to get data from properties file and store data into properties file. it can be used to get properties of system. 89
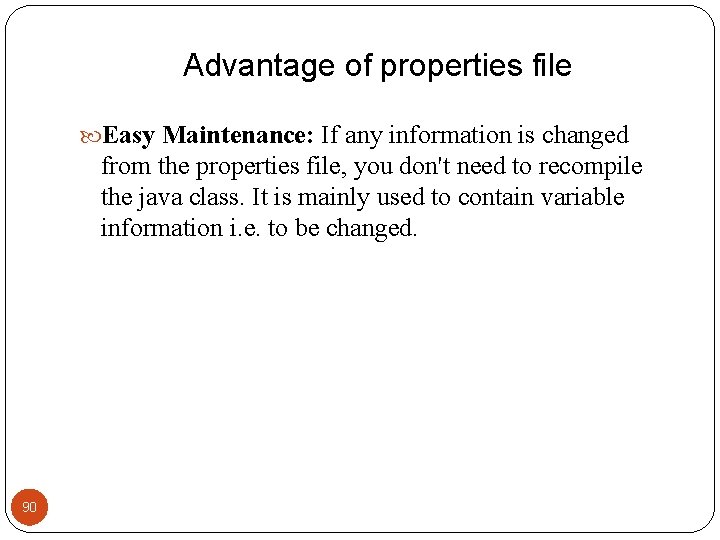
Advantage of properties file Easy Maintenance: If any information is changed from the properties file, you don't need to recompile the java class. It is mainly used to contain variable information i. e. to be changed. 90
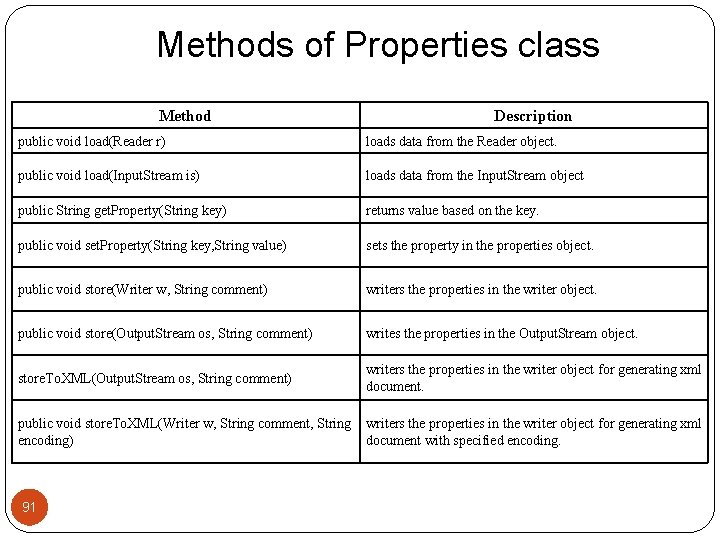
Methods of Properties class Method Description public void load(Reader r) loads data from the Reader object. public void load(Input. Stream is) loads data from the Input. Stream object public String get. Property(String key) returns value based on the key. public void set. Property(String key, String value) sets the property in the properties object. public void store(Writer w, String comment) writers the properties in the writer object. public void store(Output. Stream os, String comment) writes the properties in the Output. Stream object. store. To. XML(Output. Stream os, String comment) writers the properties in the writer object for generating xml document. public void store. To. XML(Writer w, String comment, String writers the properties in the writer object for generating xml encoding) document with specified encoding. 91
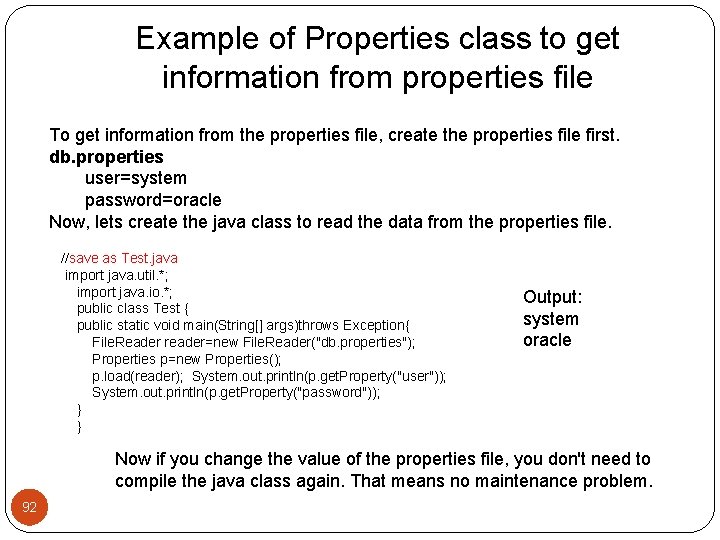
Example of Properties class to get information from properties file To get information from the properties file, create the properties file first. db. properties user=system password=oracle Now, lets create the java class to read the data from the properties file. //save as Test. java import java. util. *; import java. io. *; public class Test { public static void main(String[] args)throws Exception{ File. Reader reader=new File. Reader("db. properties"); Properties p=new Properties(); p. load(reader); System. out. println(p. get. Property("user")); System. out. println(p. get. Property("password")); } } Output: system oracle Now if you change the value of the properties file, you don't need to compile the java class again. That means no maintenance problem. 92
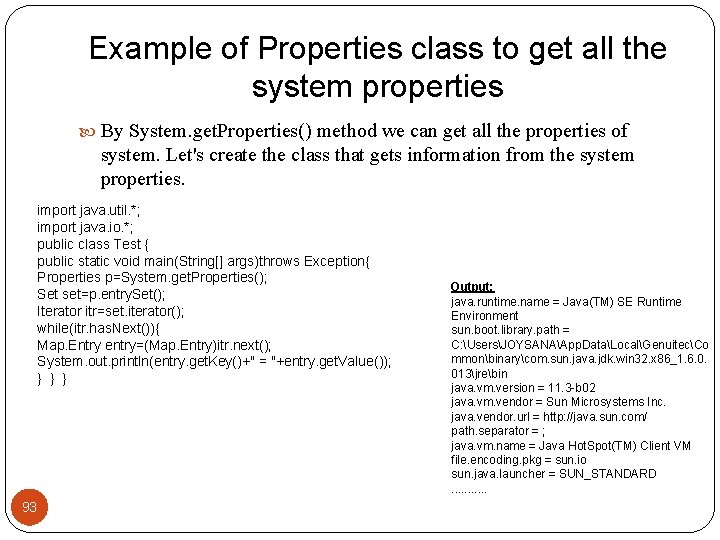
Example of Properties class to get all the system properties By System. get. Properties() method we can get all the properties of system. Let's create the class that gets information from the system properties. import java. util. *; import java. io. *; public class Test { public static void main(String[] args)throws Exception{ Properties p=System. get. Properties(); Set set=p. entry. Set(); Iterator itr=set. iterator(); while(itr. has. Next()){ Map. Entry entry=(Map. Entry)itr. next(); System. out. println(entry. get. Key()+" = "+entry. get. Value()); } } } 93 Output: java. runtime. name = Java(TM) SE Runtime Environment sun. boot. library. path = C: UsersJOYSANAApp. DataLocalGenuitecCo mmonbinarycom. sun. java. jdk. win 32. x 86_1. 6. 0. 013jrebin java. vm. version = 11. 3 -b 02 java. vm. vendor = Sun Microsystems Inc. java. vendor. url = http: //java. sun. com/ path. separator = ; java. vm. name = Java Hot. Spot(TM) Client VM file. encoding. pkg = sun. io sun. java. launcher = SUN_STANDARD. . .
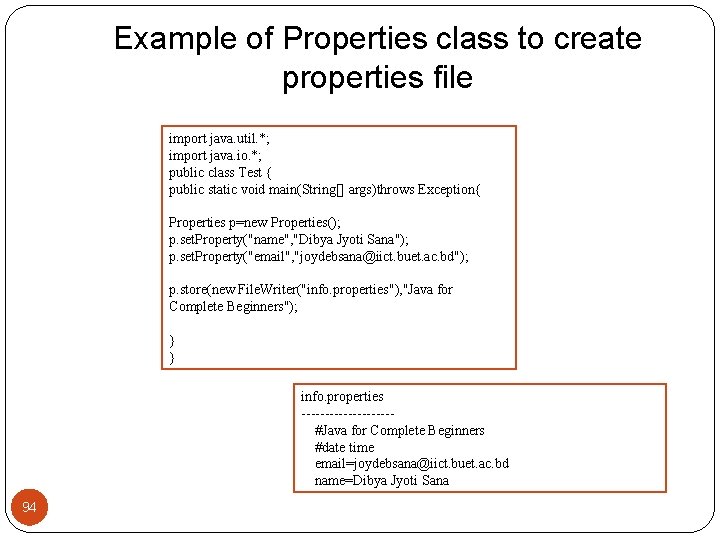
Example of Properties class to create properties file import java. util. *; import java. io. *; public class Test { public static void main(String[] args)throws Exception{ Properties p=new Properties(); p. set. Property("name", "Dibya Jyoti Sana"); p. set. Property("email", "joydebsana@iict. buet. ac. bd"); p. store(new File. Writer("info. properties"), "Java for Complete Beginners"); } } info. properties ---------- #Java for Complete Beginners #date time email=joydebsana@iict. buet. ac. bd name=Dibya Jyoti Sana 94
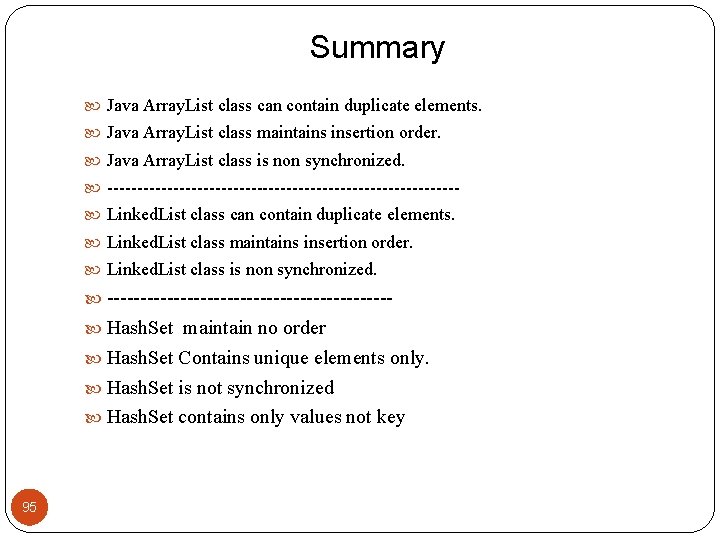
Summary Java Array. List class can contain duplicate elements. Java Array. List class maintains insertion order. Java Array. List class is non synchronized. ----------------------------- Linked. List class can contain duplicate elements. Linked. List class maintains insertion order. Linked. List class is non synchronized. --------------------- Hash. Set maintain no order Hash. Set Contains unique elements only. Hash. Set is not synchronized Hash. Set contains only values not key 95
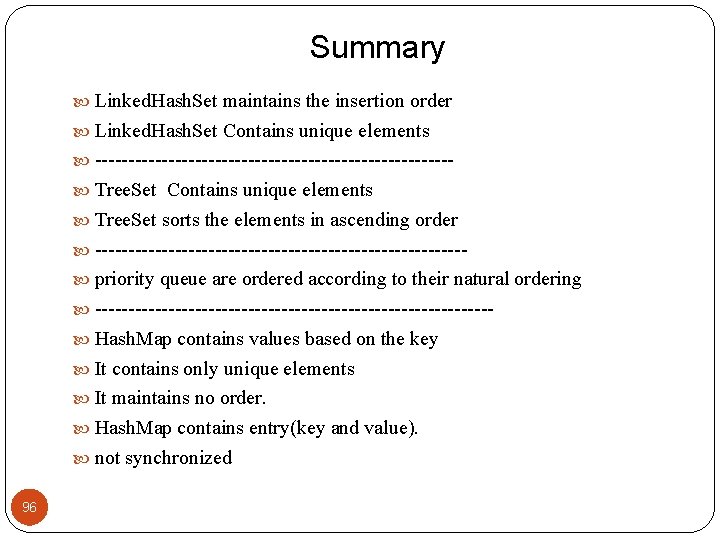
Summary Linked. Hash. Set maintains the insertion order Linked. Hash. Set Contains unique elements --------------------------- Tree. Set Contains unique elements Tree. Set sorts the elements in ascending order ---------------------------- priority queue are ordered according to their natural ordering ------------------------------ Hash. Map contains values based on the key It contains only unique elements It maintains no order. Hash. Map contains entry(key and value). not synchronized 96
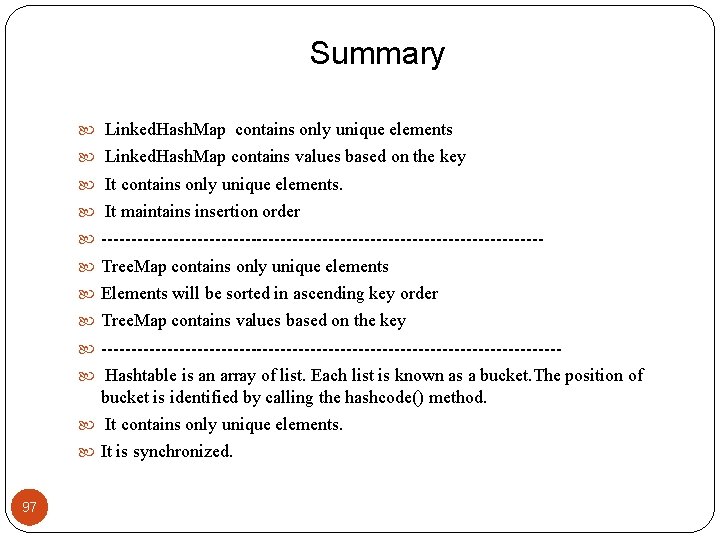
Summary Linked. Hash. Map contains only unique elements Linked. Hash. Map contains values based on the key It contains only unique elements. It maintains insertion order ------------------------------------- Tree. Map contains only unique elements Elements will be sorted in ascending key order Tree. Map contains values based on the key --------------------------------------- Hashtable is an array of list. Each list is known as a bucket. The position of bucket is identified by calling the hashcode() method. It contains only unique elements. It is synchronized. 97
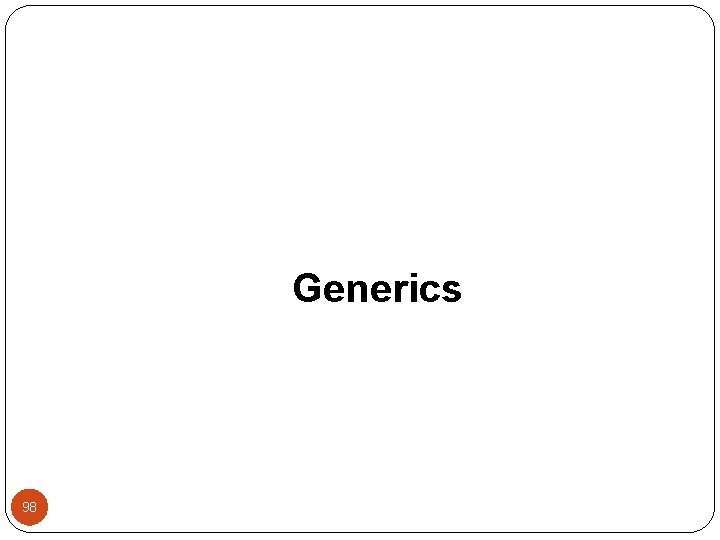
Generics 98
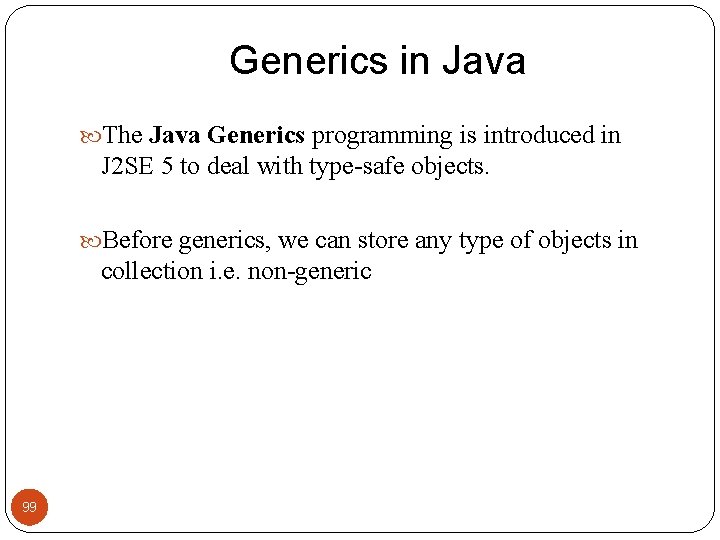
Generics in Java The Java Generics programming is introduced in J 2 SE 5 to deal with type-safe objects. Before generics, we can store any type of objects in collection i. e. non-generic 99
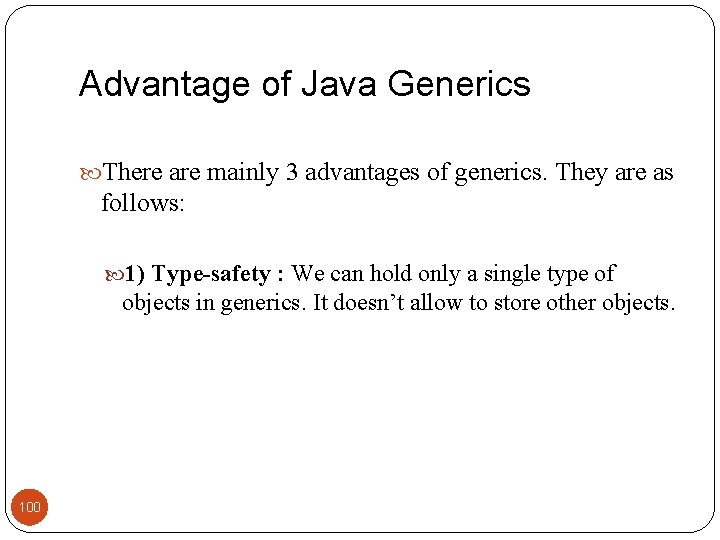
Advantage of Java Generics There are mainly 3 advantages of generics. They are as follows: 1) Type-safety : We can hold only a single type of objects in generics. It doesn’t allow to store other objects. 100
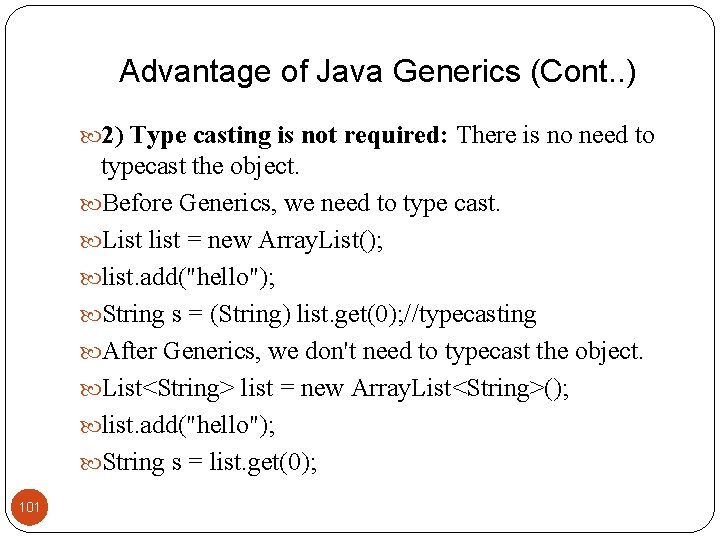
Advantage of Java Generics (Cont. . ) 2) Type casting is not required: There is no need to typecast the object. Before Generics, we need to type cast. List list = new Array. List(); list. add("hello"); String s = (String) list. get(0); //typecasting After Generics, we don't need to typecast the object. List<String> list = new Array. List<String>(); list. add("hello"); String s = list. get(0); 101
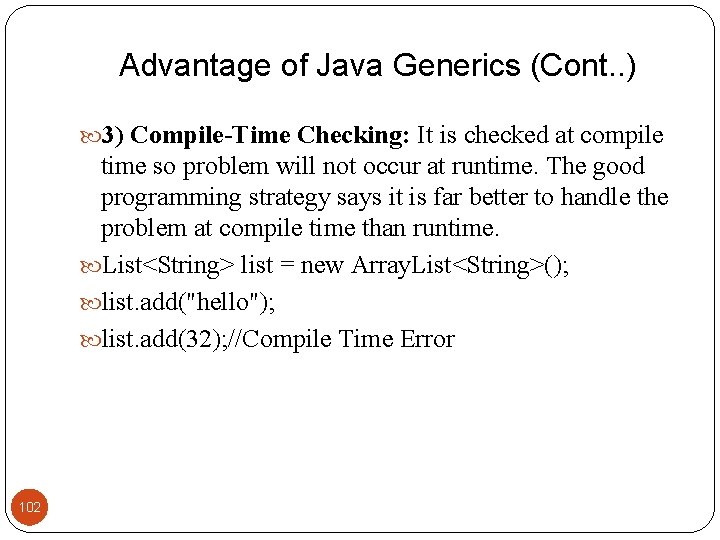
Advantage of Java Generics (Cont. . ) 3) Compile-Time Checking: It is checked at compile time so problem will not occur at runtime. The good programming strategy says it is far better to handle the problem at compile time than runtime. List<String> list = new Array. List<String>(); list. add("hello"); list. add(32); //Compile Time Error 102
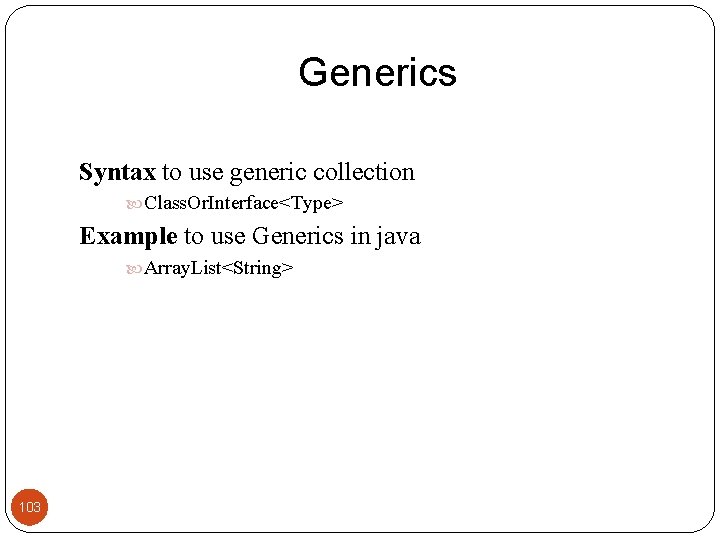
Generics Syntax to use generic collection Class. Or. Interface<Type> Example to use Generics in java Array. List<String> 103
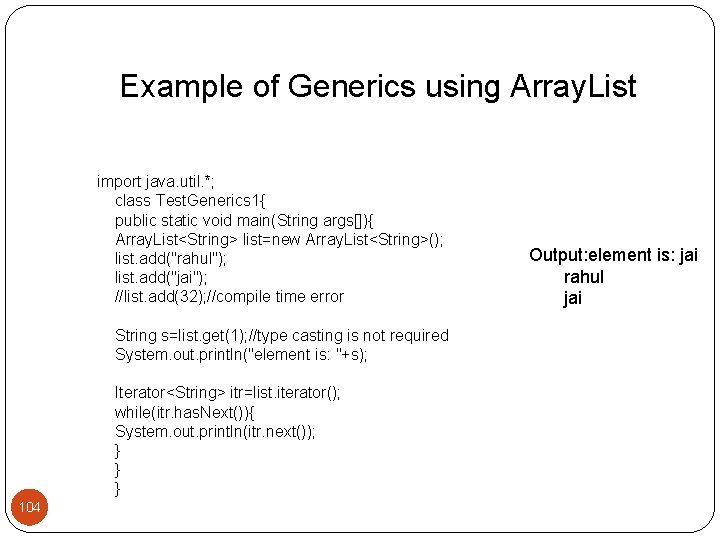
Example of Generics using Array. List import java. util. *; class Test. Generics 1{ public static void main(String args[]){ Array. List<String> list=new Array. List<String>(); list. add("rahul"); list. add("jai"); //list. add(32); //compile time error String s=list. get(1); //type casting is not required System. out. println("element is: "+s); Iterator<String> itr=list. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } 104 Output: element is: jai rahul jai
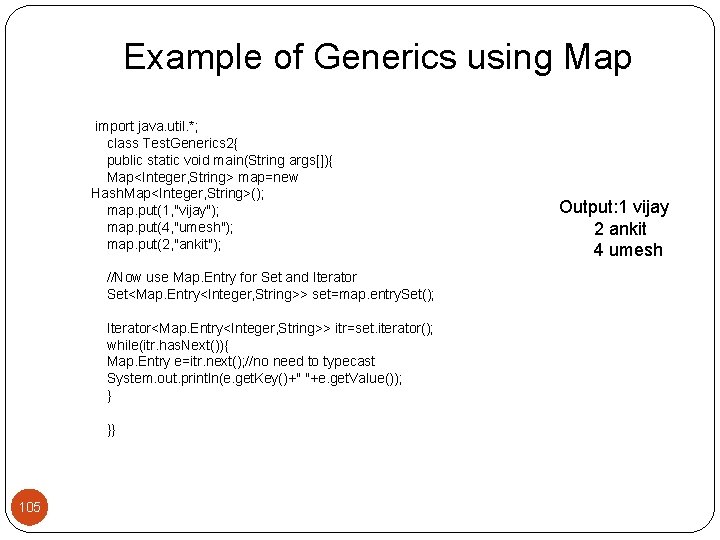
Example of Generics using Map import java. util. *; class Test. Generics 2{ public static void main(String args[]){ Map<Integer, String> map=new Hash. Map<Integer, String>(); map. put(1, "vijay"); map. put(4, "umesh"); map. put(2, "ankit"); //Now use Map. Entry for Set and Iterator Set<Map. Entry<Integer, String>> set=map. entry. Set(); Iterator<Map. Entry<Integer, String>> itr=set. iterator(); while(itr. has. Next()){ Map. Entry e=itr. next(); //no need to typecast System. out. println(e. get. Key()+" "+e. get. Value()); } }} 105 Output: 1 vijay 2 ankit 4 umesh
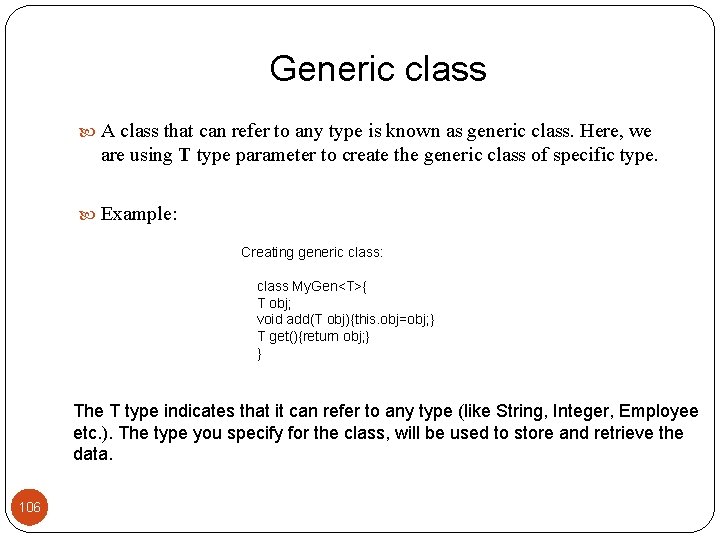
Generic class A class that can refer to any type is known as generic class. Here, we are using T type parameter to create the generic class of specific type. Example: Creating generic class: class My. Gen<T>{ T obj; void add(T obj){this. obj=obj; } T get(){return obj; } } The T type indicates that it can refer to any type (like String, Integer, Employee etc. ). The type you specify for the class, will be used to store and retrieve the data. 106
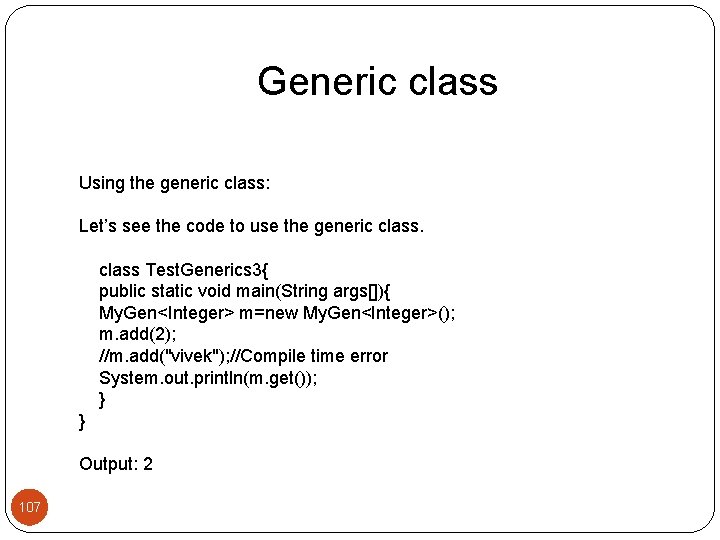
Generic class Using the generic class: Let’s see the code to use the generic class Test. Generics 3{ public static void main(String args[]){ My. Gen<Integer> m=new My. Gen<Integer>(); m. add(2); //m. add("vivek"); //Compile time error System. out. println(m. get()); } } Output: 2 107
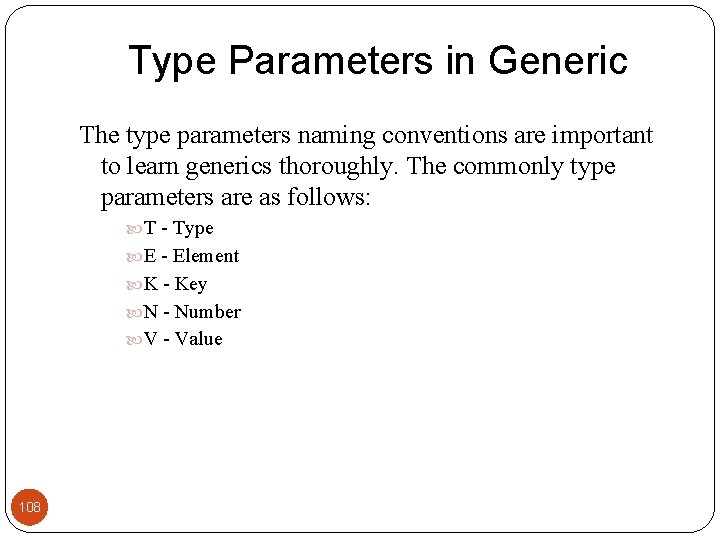
Type Parameters in Generic The type parameters naming conventions are important to learn generics thoroughly. The commonly type parameters are as follows: T - Type E - Element K - Key N - Number V - Value 108
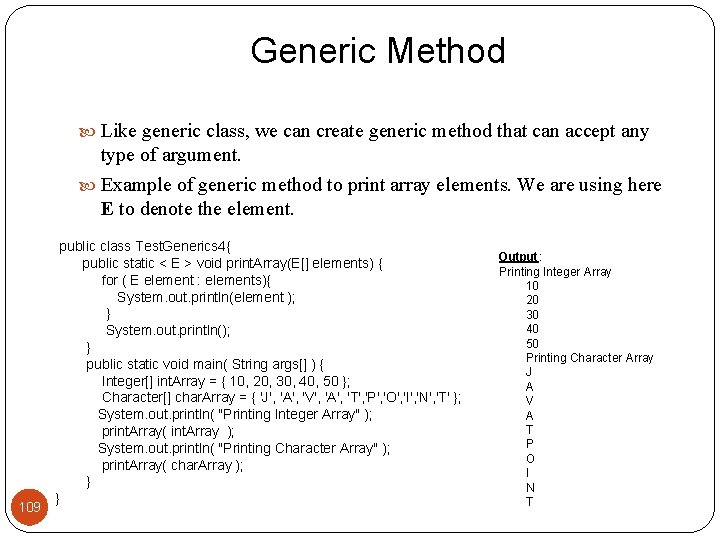
Generic Method Like generic class, we can create generic method that can accept any type of argument. Example of generic method to print array elements. We are using here E to denote the element. 109 public class Test. Generics 4{ public static < E > void print. Array(E[] elements) { for ( E element : elements){ System. out. println(element ); } System. out. println(); } public static void main( String args[] ) { Integer[] int. Array = { 10, 20, 30, 40, 50 }; Character[] char. Array = { 'J', 'A', 'V', 'A', 'T', 'P', 'O', 'I', 'N', 'T' }; System. out. println( "Printing Integer Array" ); print. Array( int. Array ); System. out. println( "Printing Character Array" ); print. Array( char. Array ); } } Output: Printing Integer Array 10 20 30 40 50 Printing Character Array J A V A T P O I N T
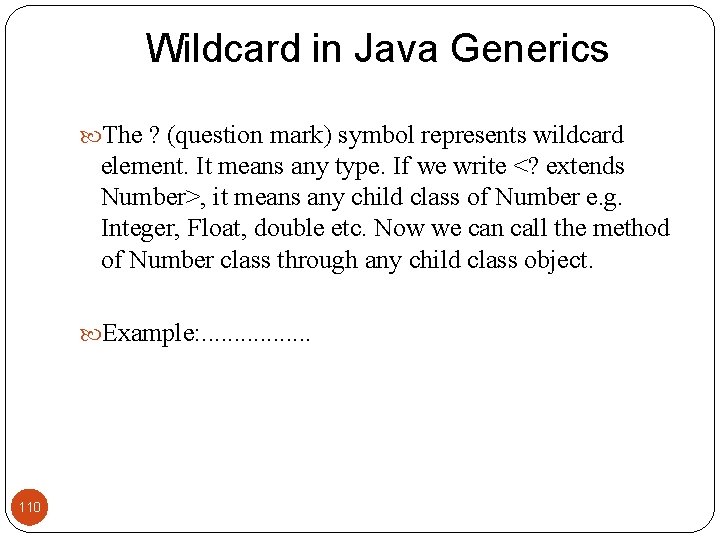
Wildcard in Java Generics The ? (question mark) symbol represents wildcard element. It means any type. If we write <? extends Number>, it means any child class of Number e. g. Integer, Float, double etc. Now we can call the method of Number class through any child class object. Example: . . . . 110
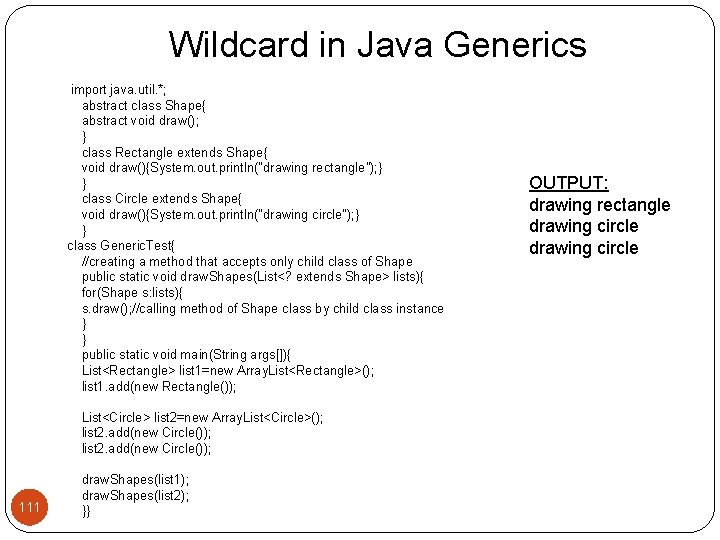
Wildcard in Java Generics 111 import java. util. *; abstract class Shape{ abstract void draw(); } class Rectangle extends Shape{ void draw(){System. out. println("drawing rectangle"); } } class Circle extends Shape{ void draw(){System. out. println("drawing circle"); } } class Generic. Test{ //creating a method that accepts only child class of Shape public static void draw. Shapes(List<? extends Shape> lists){ for(Shape s: lists){ s. draw(); //calling method of Shape class by child class instance } } public static void main(String args[]){ List<Rectangle> list 1=new Array. List<Rectangle>(); list 1. add(new Rectangle()); List<Circle> list 2=new Array. List<Circle>(); list 2. add(new Circle()); draw. Shapes(list 1); draw. Shapes(list 2); }} OUTPUT: drawing rectangle drawing circle
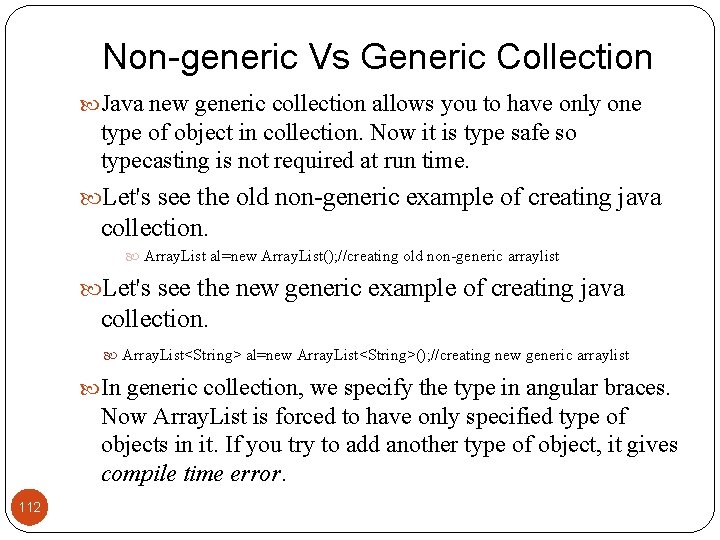
Non-generic Vs Generic Collection Java new generic collection allows you to have only one type of object in collection. Now it is type safe so typecasting is not required at run time. Let's see the old non-generic example of creating java collection. Array. List al=new Array. List(); //creating old non-generic arraylist Let's see the new generic example of creating java collection. In generic collection, we specify the type in angular braces. Now Array. List is forced to have only specified type of objects in it. If you try to add another type of object, it gives compile time error. Array. List<String> al=new Array. List<String>(); //creating new generic arraylist 112
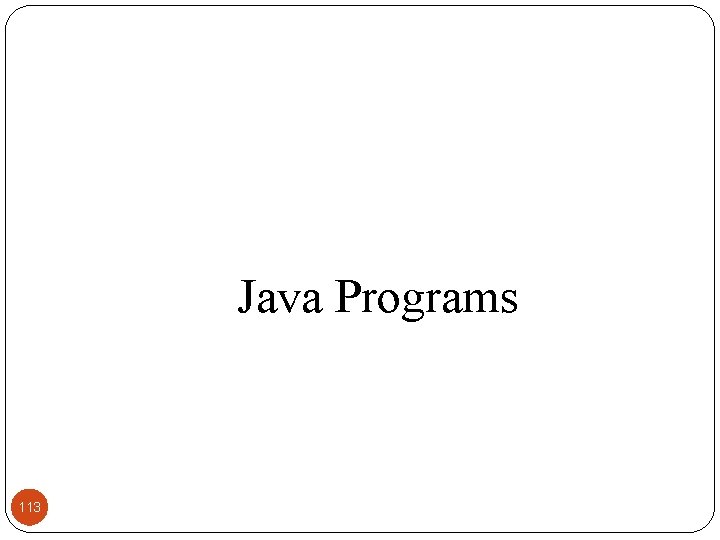
Java Programs 113
Using system.collections
Java generics array
Generics java erklärung
Advantages of generics in java
Java generics array
Hospitality generics
Kotlinx serialization generics
C generics
Trees in java collections
Java collections overview
Java collections framework diagram
Java collections hierarchy
Difference between array and string
Google collections java
Pseg credit and collections
Chapter 20 patient collections and financial management
The html
Collection of specialized cells and cell products
Aba therapy billing and insurance services
Williams and fudge payments
Brown bin tonbridge and malling
Collections trust spectrum
Hltpat001
Largest galaxy
Are all the methods in the collections class static?
631-828-3140
Collection strategy in sap
Financial data systems debt collector
Cara menampilkan preview file di windows 10
Adt collections
System.collections.generic namespace
Musnad collections of hadith
Jisc licence manager
Jennifer weintraub
Shared services alaska
Fms collections
Collections
Eton college collections
Sruthika collections
I dig bio
Collections
Reflectionit
Revenue cycle sales to cash collections
Arm industry collections
Iupui digital collections
Benchmarks in collections care
Processes neuron
Ucsd dda
Set interface in java
Customers typically pay according to each invoice with the
C# collections overview
Import java.util.*
Import java.util.*
Import java.applet.applet
Import java util scanner
Import java.io.file
Java gcd
Import java.util.random
Import java.io.*
Import java.util.
Java import java.io.*
Awt adalah
Import java.awt.event.*
Language
Javatpoint ejb
List array python difference
Overriding and overloading in java
Problem solving
Advantages and disadvantages of inheritance in java
Class person
Divide and conquer
Mutator and accessor methods in java
Mutator and accessor methods in java
Java stacks and queues
Common gui event types and listener interfaces in java
Application
Java naming directory interface
Data structures and abstractions with java
Java input and output statements
Searching and sorting in java
Eric s roberts
Sets and maps java
Java an introduction to problem solving and programming
Adt in ooad
Data abstraction and problem solving with java
Searching and sorting in java
Overloading methods and constructors in java
Big o java
Searching and sorting in java
Difference between java and visual basic
Static vs dynamic class loading in java
Mutator adalah
What is rule and convention in java
Java file input and output
Console input and output in java
Java pointers and references
E balagurusamy object oriented programming with c++
Java stacks and queues
Java pointers and references
Java introduction to problem solving and programming
Skyline problem divide and conquer java
Generalization and specialization in java
Mixed mode assignment allowed in c and java
Difference between abstract class and interface
Red orange yellow blue green purple
Java soap xml 파싱
Java xml
West java leaders reading challenge
List of java exceptions
Jux java
Vadim java
Mathematical functions in java
App inventor java bridge
Extends and implements difference