Pointers and References Java vs C 04 Dec20
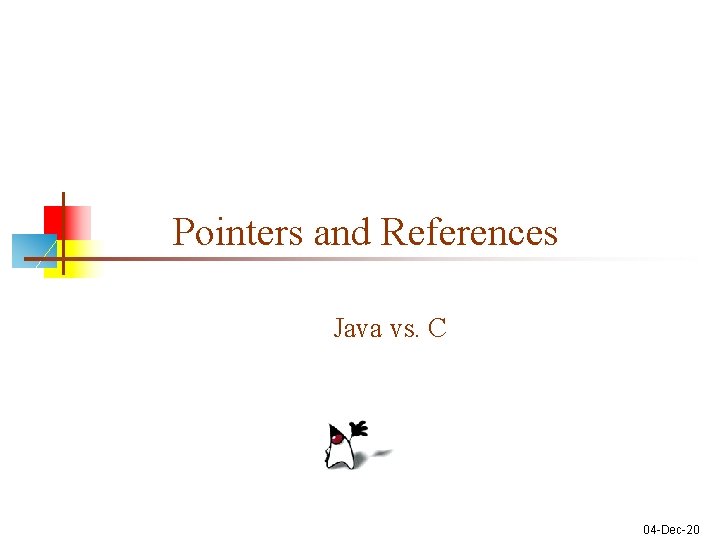
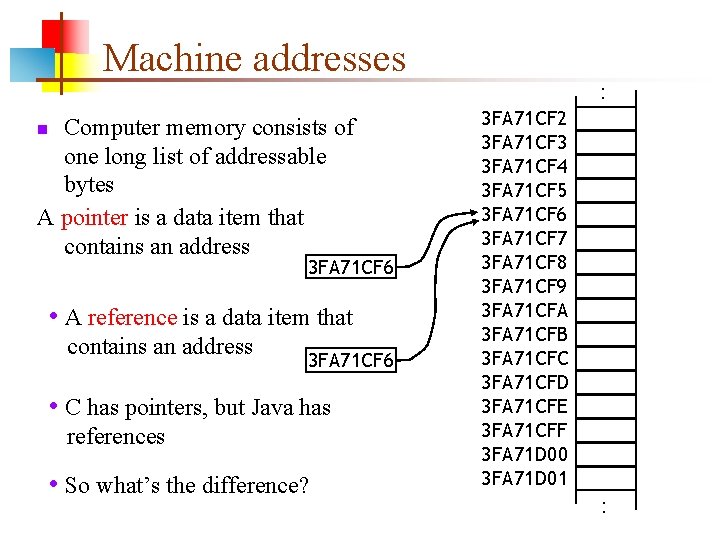
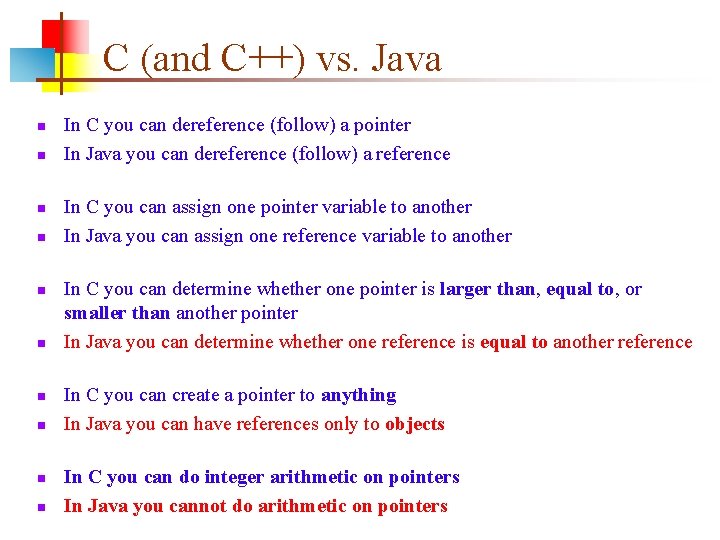
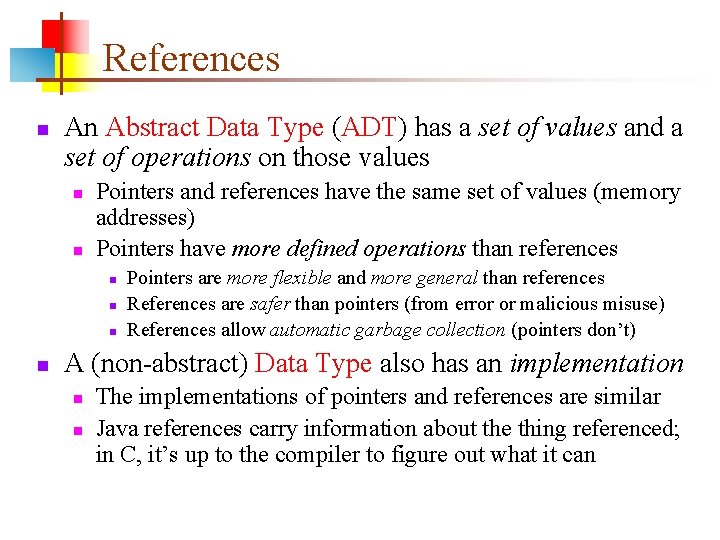
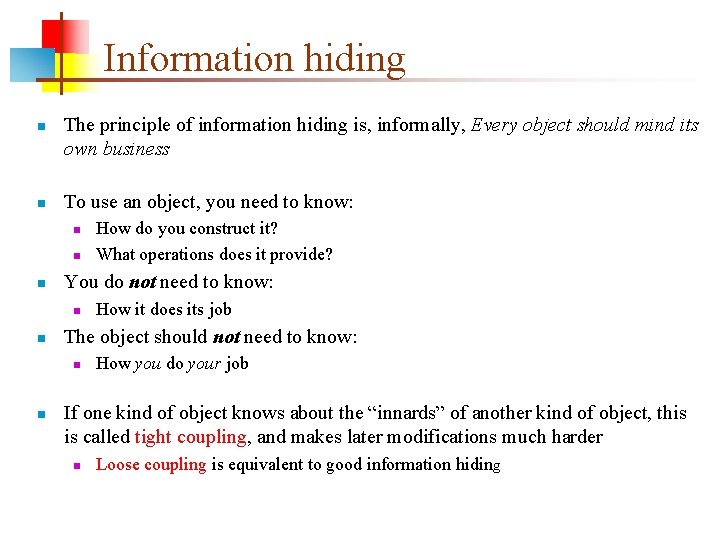
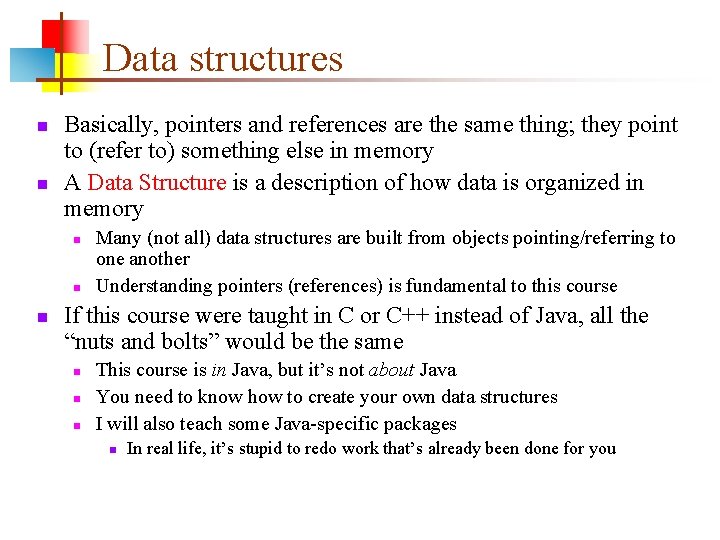
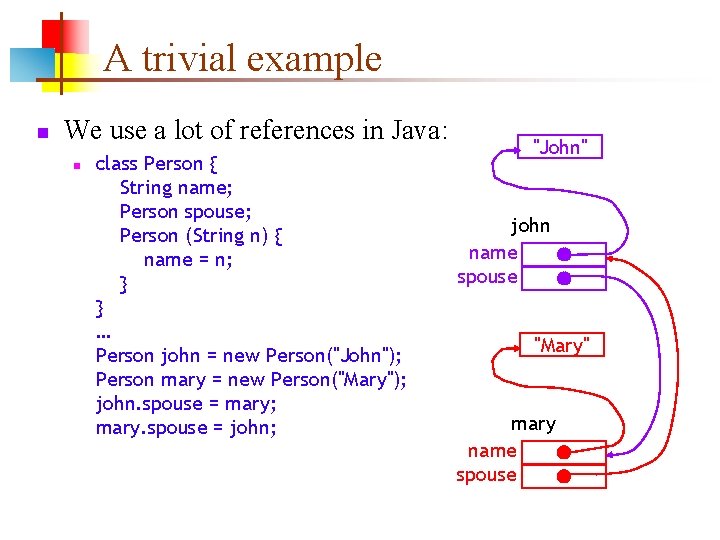
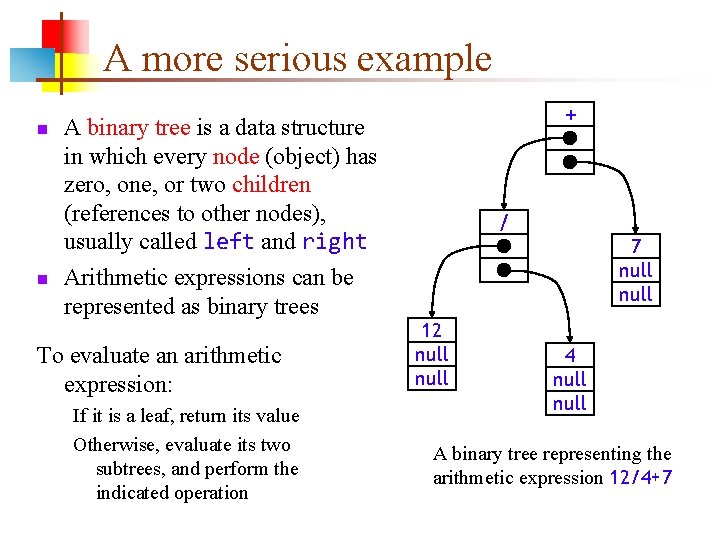
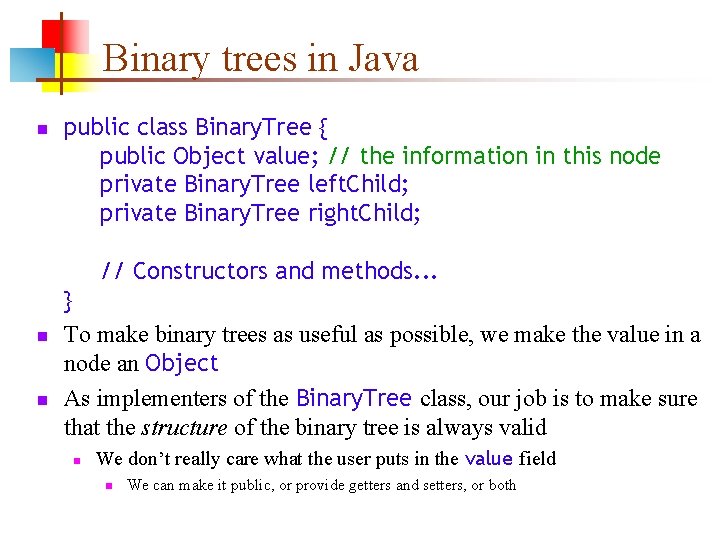
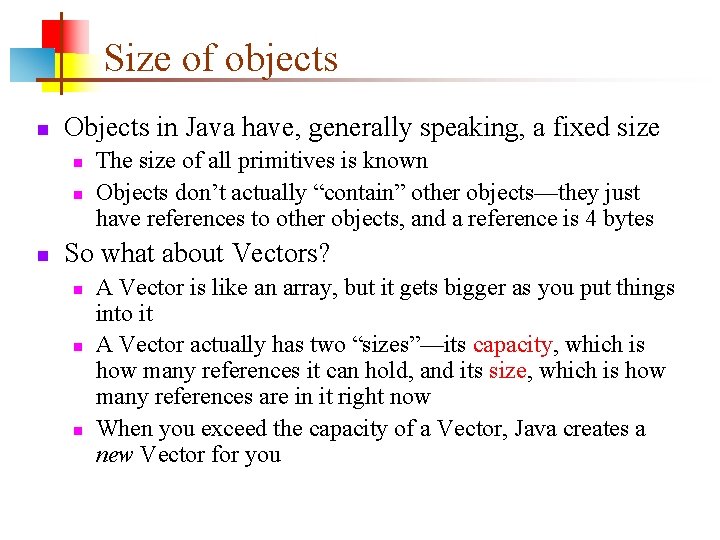
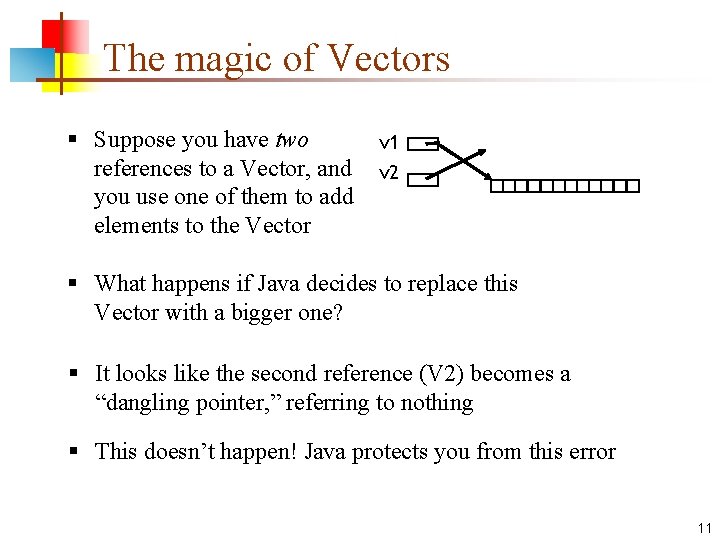
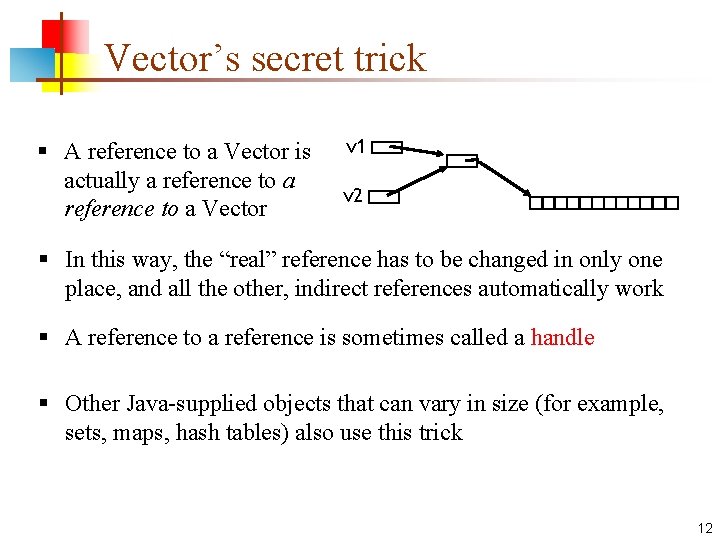
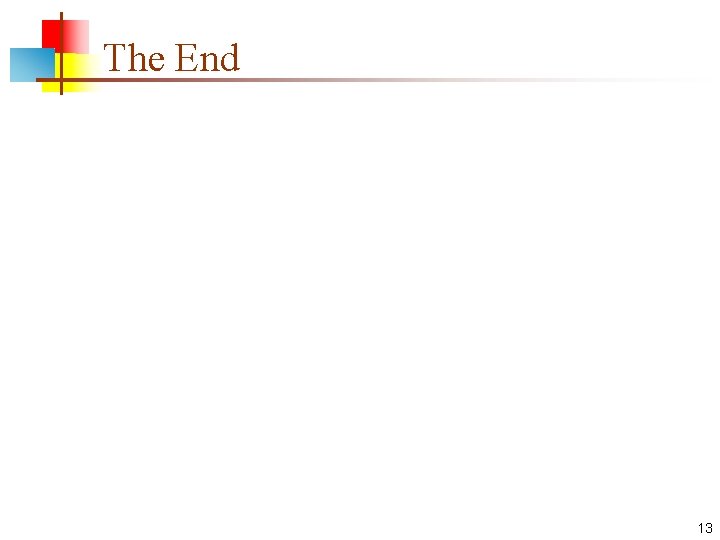
- Slides: 13
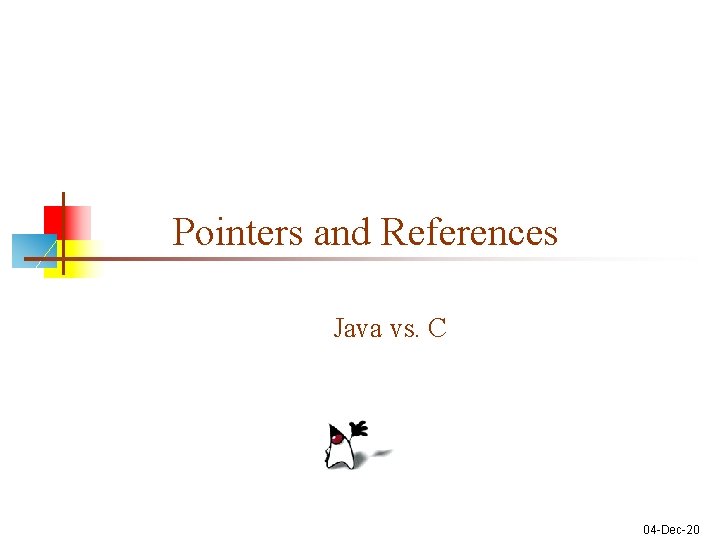
Pointers and References Java vs. C 04 -Dec-20
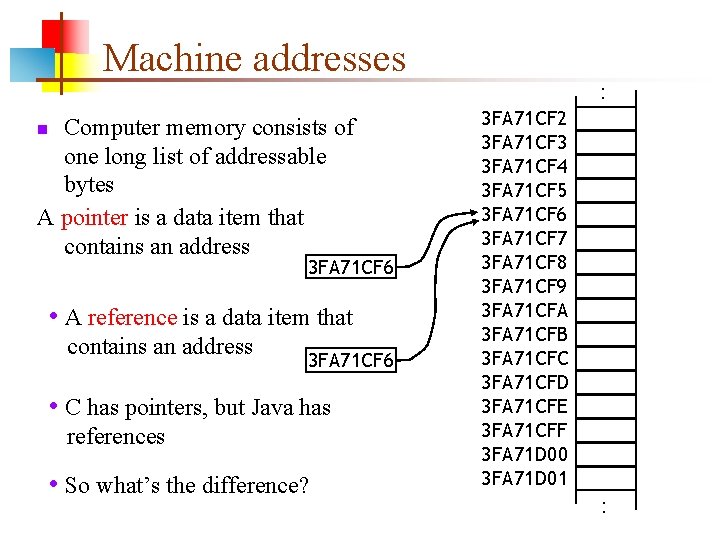
Machine addresses Computer memory consists of one long list of addressable bytes A pointer is a data item that contains an address 3 FA 71 CF 6 • A reference is a data item that contains an address 3 FA 71 CF 6 • C has pointers, but Java has references • So what’s the difference? : 3 FA 71 CF 2 3 FA 71 CF 3 3 FA 71 CF 4 3 FA 71 CF 5 3 FA 71 CF 6 3 FA 71 CF 7 3 FA 71 CF 8 3 FA 71 CF 9 3 FA 71 CFA 3 FA 71 CFB 3 FA 71 CFC 3 FA 71 CFD 3 FA 71 CFE 3 FA 71 CFF 3 FA 71 D 00 3 FA 71 D 01 :
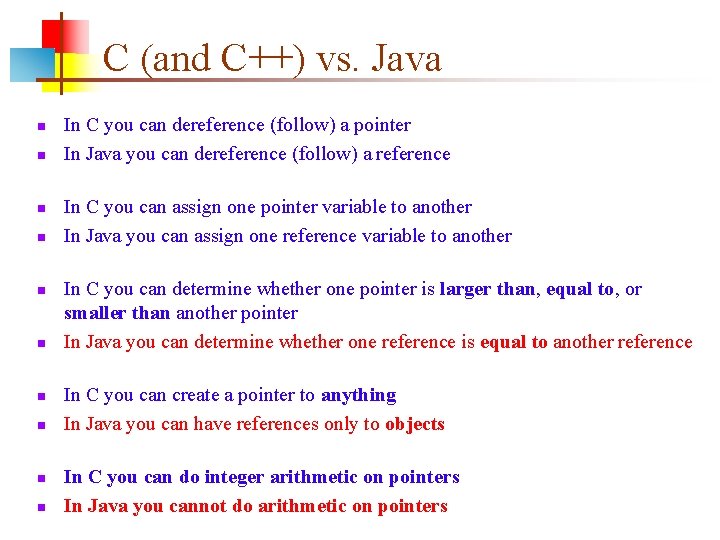
C (and C++) vs. Java In C you can dereference (follow) a pointer In Java you can dereference (follow) a reference In C you can assign one pointer variable to another In Java you can assign one reference variable to another In C you can determine whether one pointer is larger than, equal to, or smaller than another pointer In Java you can determine whether one reference is equal to another reference In C you can create a pointer to anything In Java you can have references only to objects In C you can do integer arithmetic on pointers In Java you cannot do arithmetic on pointers
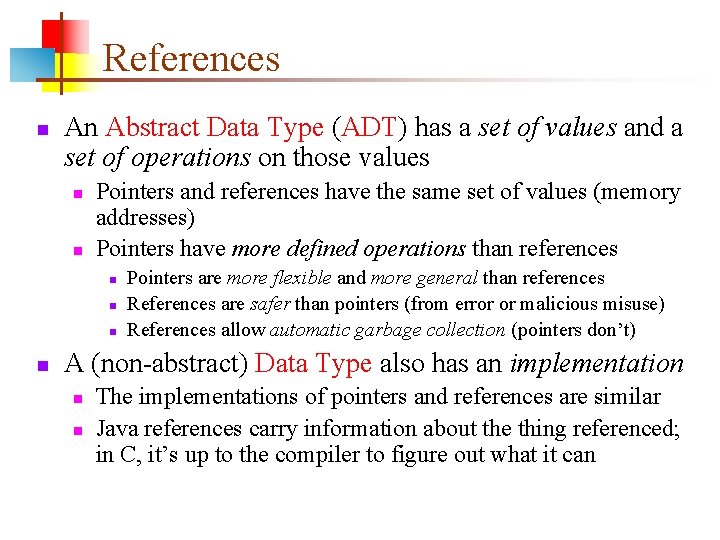
References An Abstract Data Type (ADT) has a set of values and a set of operations on those values Pointers and references have the same set of values (memory addresses) Pointers have more defined operations than references Pointers are more flexible and more general than references References are safer than pointers (from error or malicious misuse) References allow automatic garbage collection (pointers don’t) A (non-abstract) Data Type also has an implementation The implementations of pointers and references are similar Java references carry information about the thing referenced; in C, it’s up to the compiler to figure out what it can
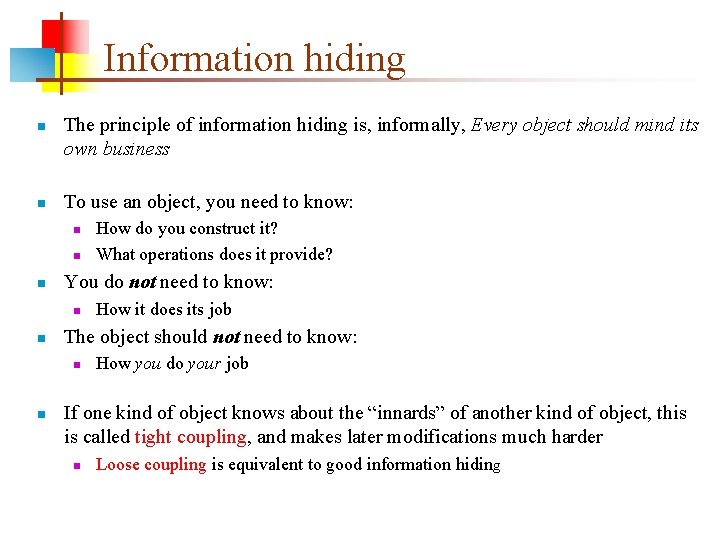
Information hiding The principle of information hiding is, informally, Every object should mind its own business To use an object, you need to know: You do not need to know: How it does its job The object should not need to know: How do you construct it? What operations does it provide? How you do your job If one kind of object knows about the “innards” of another kind of object, this is called tight coupling, and makes later modifications much harder Loose coupling is equivalent to good information hiding
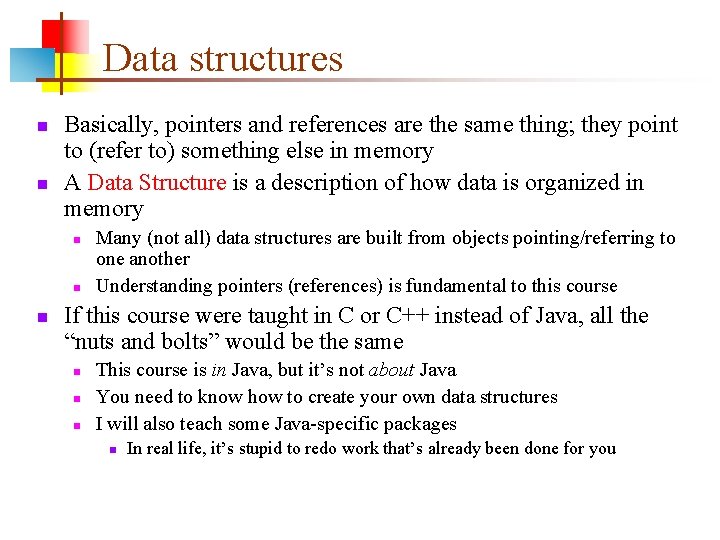
Data structures Basically, pointers and references are the same thing; they point to (refer to) something else in memory A Data Structure is a description of how data is organized in memory Many (not all) data structures are built from objects pointing/referring to one another Understanding pointers (references) is fundamental to this course If this course were taught in C or C++ instead of Java, all the “nuts and bolts” would be the same This course is in Java, but it’s not about Java You need to know how to create your own data structures I will also teach some Java-specific packages In real life, it’s stupid to redo work that’s already been done for you
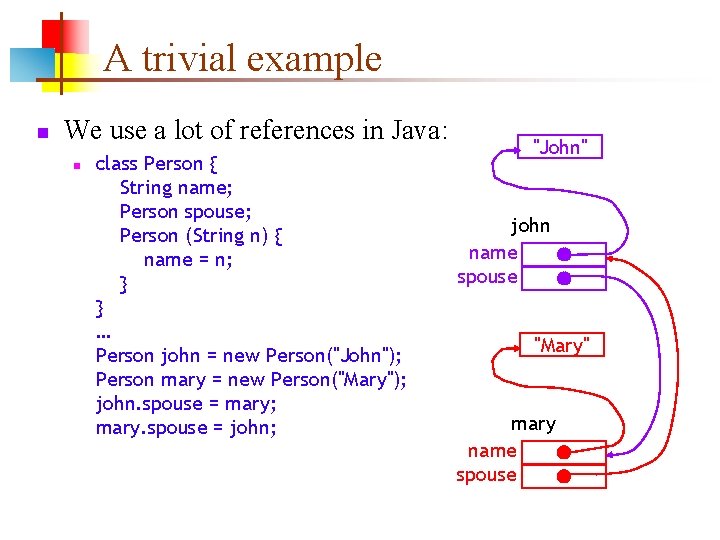
A trivial example We use a lot of references in Java: class Person { String name; Person spouse; Person (String n) { name = n; } } … Person john = new Person("John"); Person mary = new Person("Mary"); john. spouse = mary; mary. spouse = john; "John" john name spouse "Mary" mary name spouse
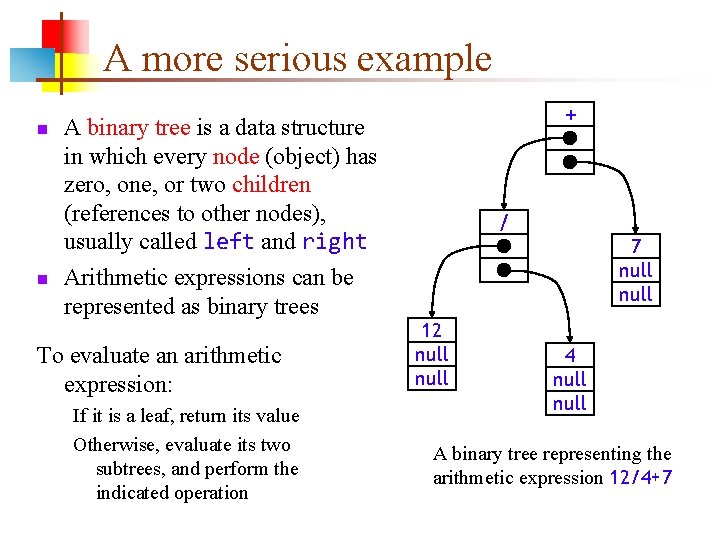
A more serious example A binary tree is a data structure in which every node (object) has zero, one, or two children (references to other nodes), usually called left and right Arithmetic expressions can be represented as binary trees To evaluate an arithmetic expression: If it is a leaf, return its value Otherwise, evaluate its two subtrees, and perform the indicated operation + / 12 null 7 null 4 null A binary tree representing the arithmetic expression 12/4+7
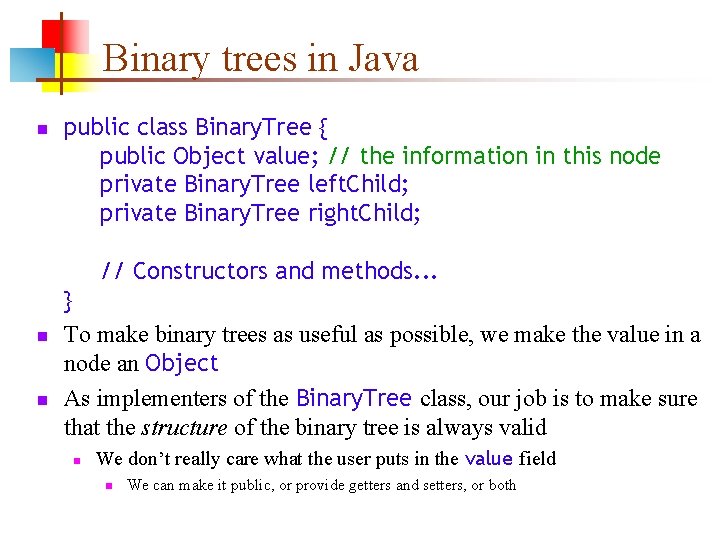
Binary trees in Java public class Binary. Tree { public Object value; // the information in this node private Binary. Tree left. Child; private Binary. Tree right. Child; // Constructors and methods. . . } To make binary trees as useful as possible, we make the value in a node an Object As implementers of the Binary. Tree class, our job is to make sure that the structure of the binary tree is always valid We don’t really care what the user puts in the value field We can make it public, or provide getters and setters, or both
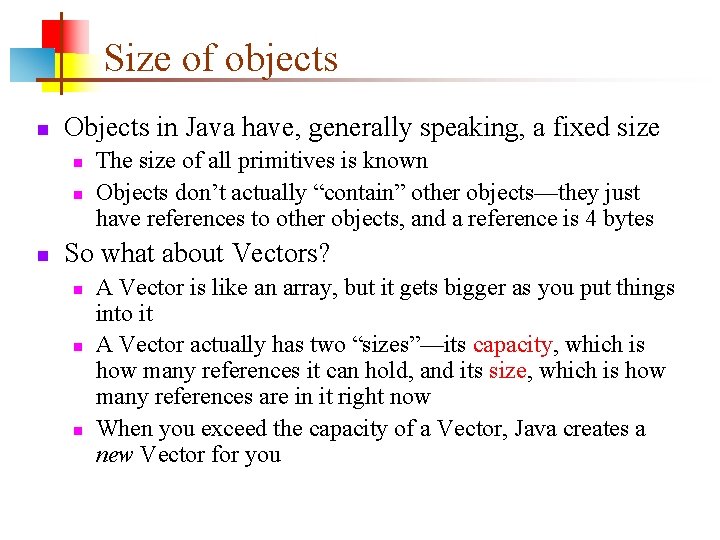
Size of objects Objects in Java have, generally speaking, a fixed size The size of all primitives is known Objects don’t actually “contain” other objects—they just have references to other objects, and a reference is 4 bytes So what about Vectors? A Vector is like an array, but it gets bigger as you put things into it A Vector actually has two “sizes”—its capacity, which is how many references it can hold, and its size, which is how many references are in it right now When you exceed the capacity of a Vector, Java creates a new Vector for you
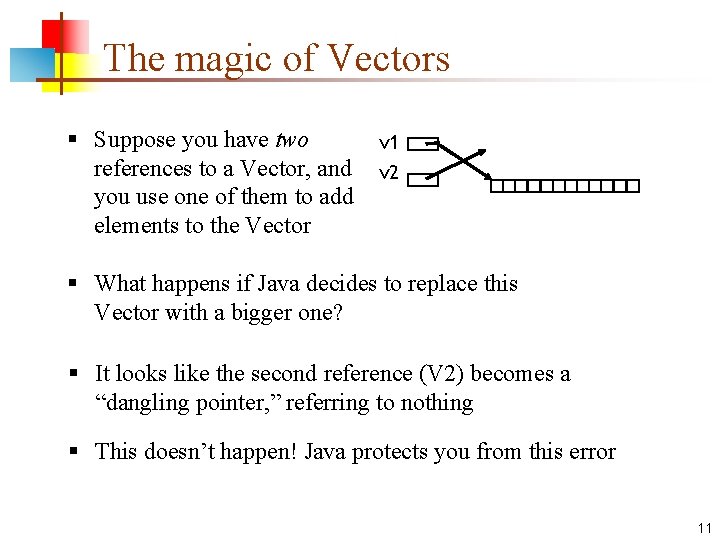
The magic of Vectors § Suppose you have two references to a Vector, and you use one of them to add elements to the Vector v 1 v 2 § What happens if Java decides to replace this Vector with a bigger one? § It looks like the second reference (V 2) becomes a “dangling pointer, ” referring to nothing § This doesn’t happen! Java protects you from this error 11
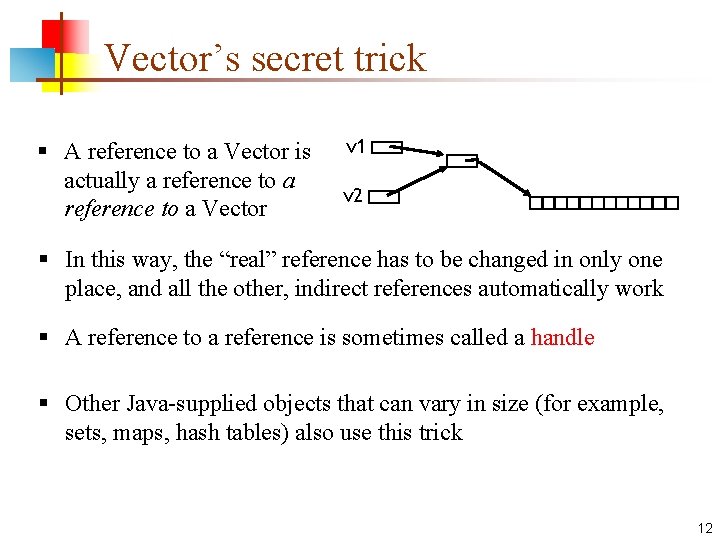
Vector’s secret trick § A reference to a Vector is actually a reference to a Vector v 1 v 2 § In this way, the “real” reference has to be changed in only one place, and all the other, indirect references automatically work § A reference to a reference is sometimes called a handle § Other Java-supplied objects that can vary in size (for example, sets, maps, hash tables) also use this trick 12
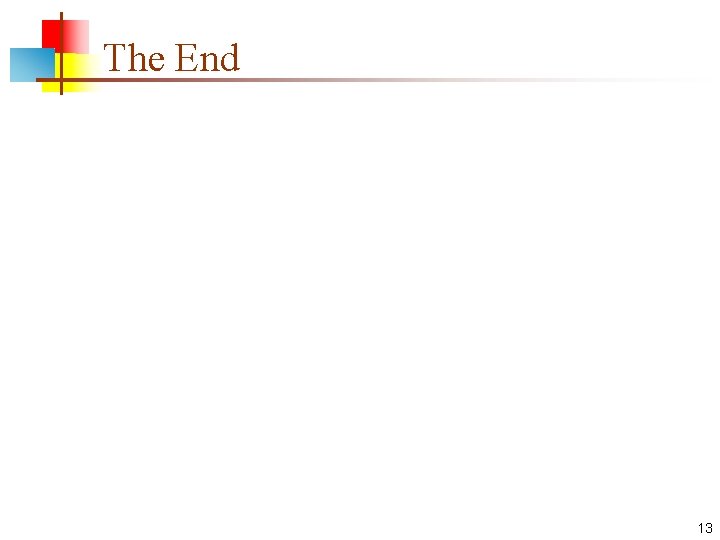
The End 13