Chapter 2 Java Fundamentals Input and Output statements
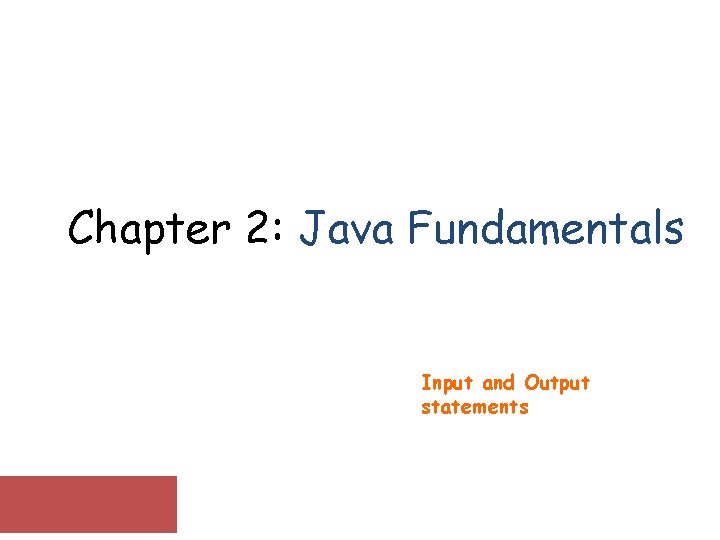
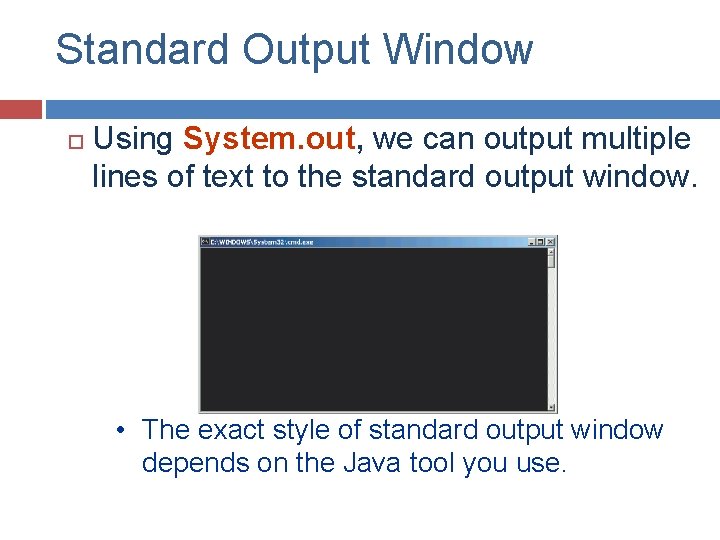
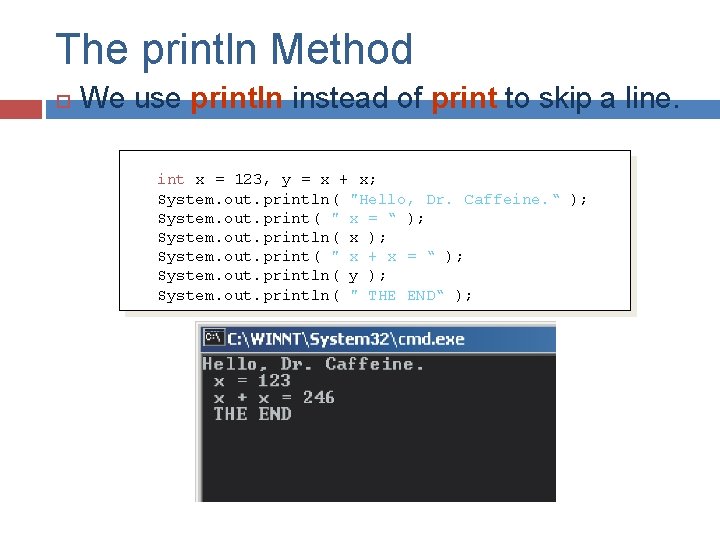
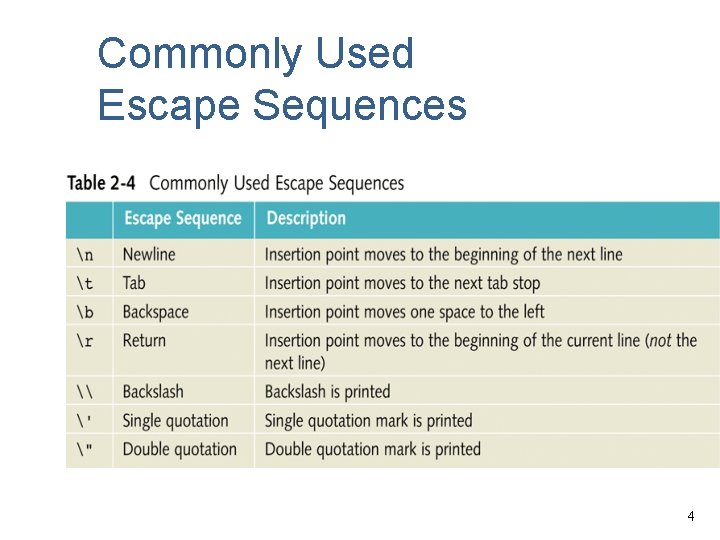
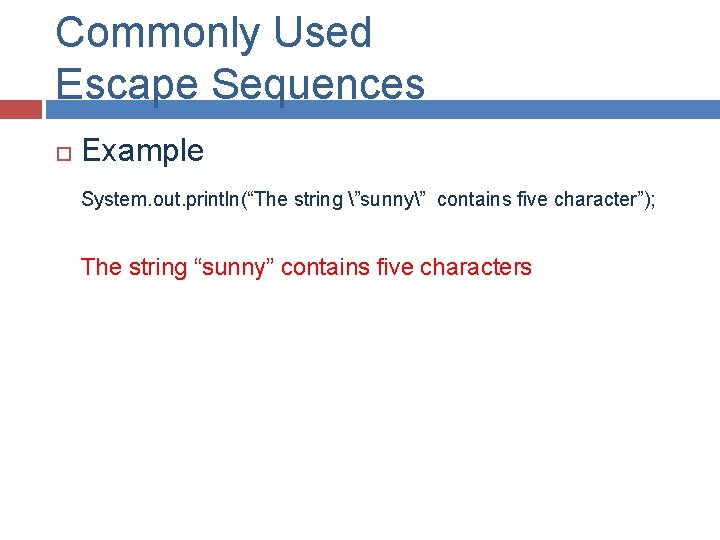
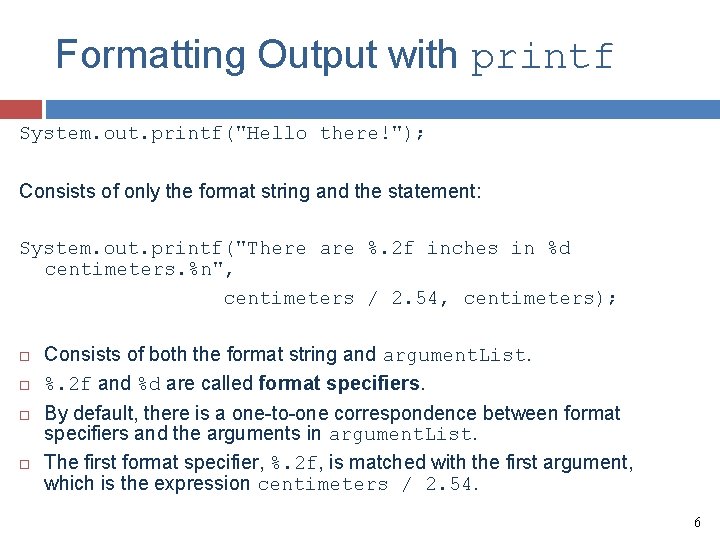
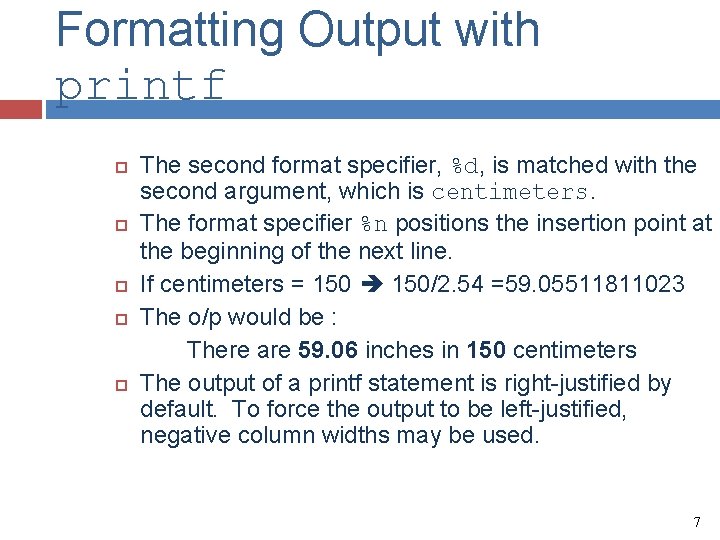
![Example 1 public class Example 3_6 { public static void main (String[] args) { Example 1 public class Example 3_6 { public static void main (String[] args) {](https://slidetodoc.com/presentation_image_h2/4a70918d6db06fd43898fd3de0754c30/image-8.jpg)
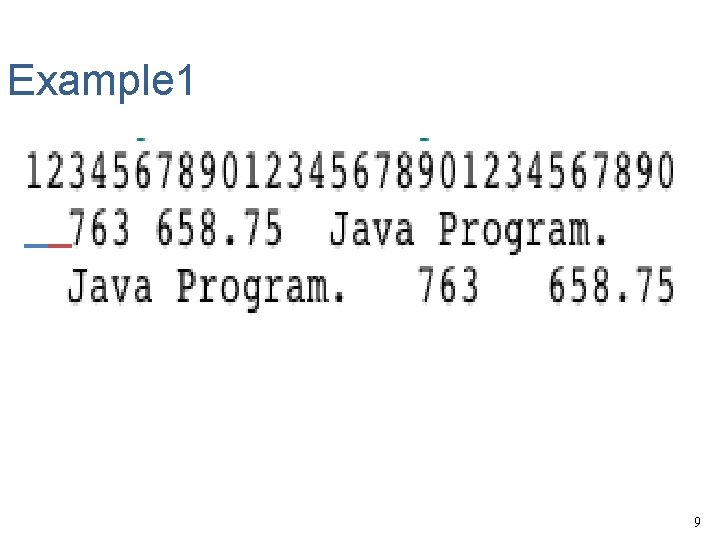
![Example 2 public class Example 3_7 { public static void main (String[] args) { Example 2 public class Example 3_7 { public static void main (String[] args) {](https://slidetodoc.com/presentation_image_h2/4a70918d6db06fd43898fd3de0754c30/image-10.jpg)
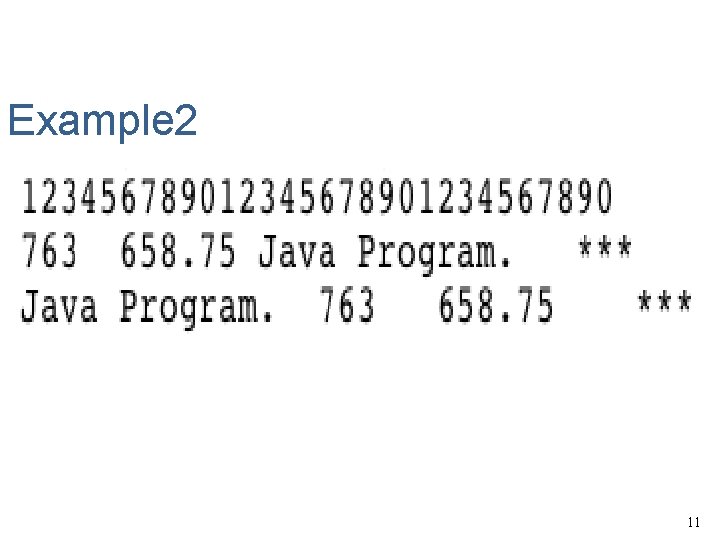
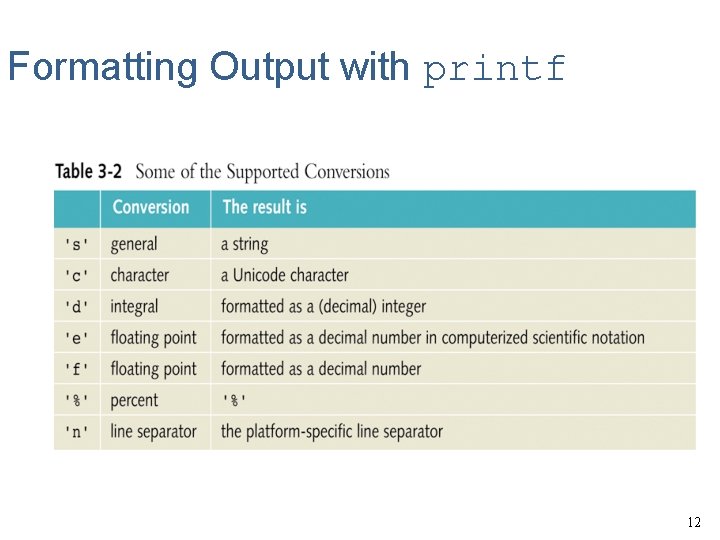
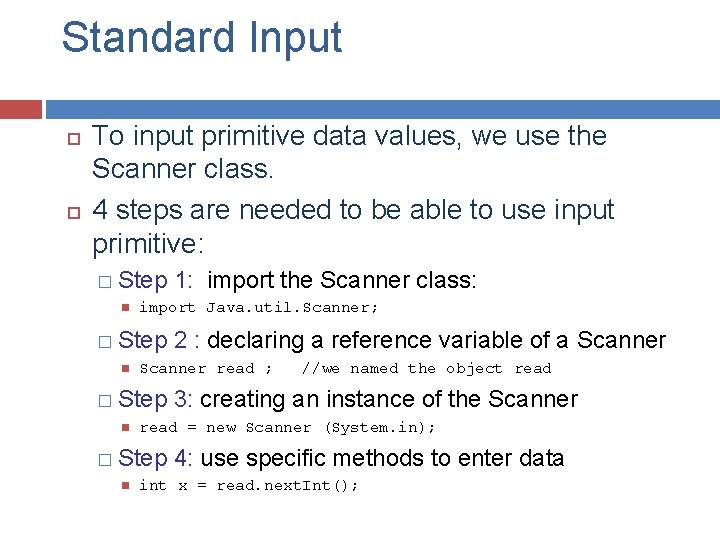
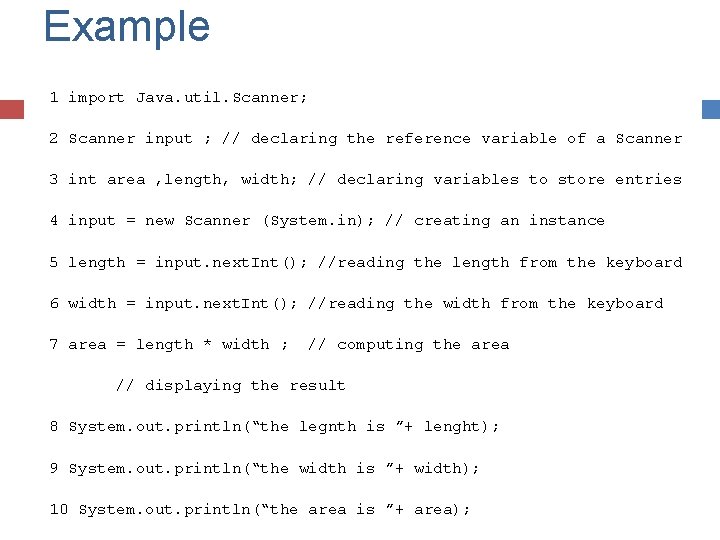
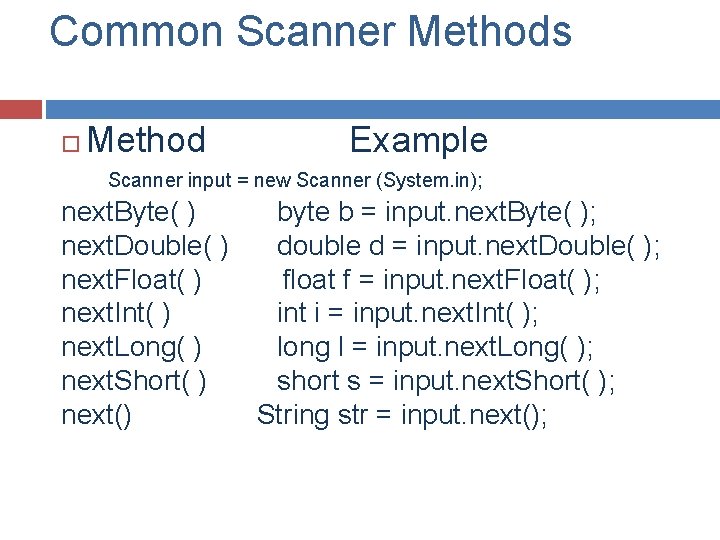
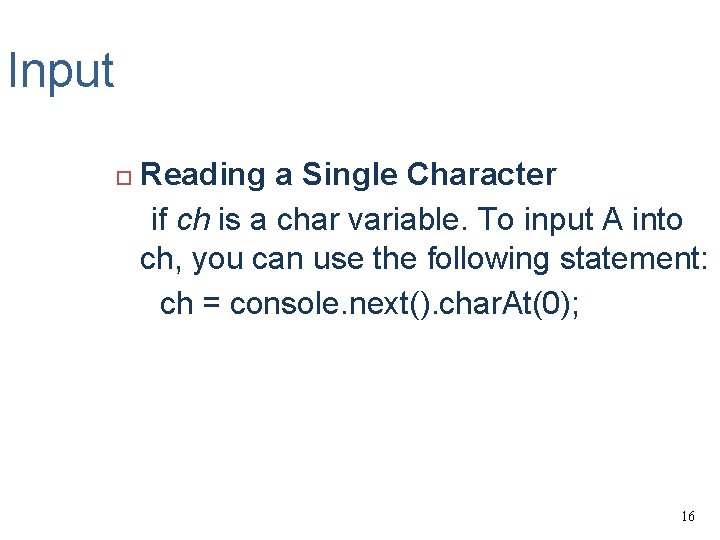
- Slides: 16
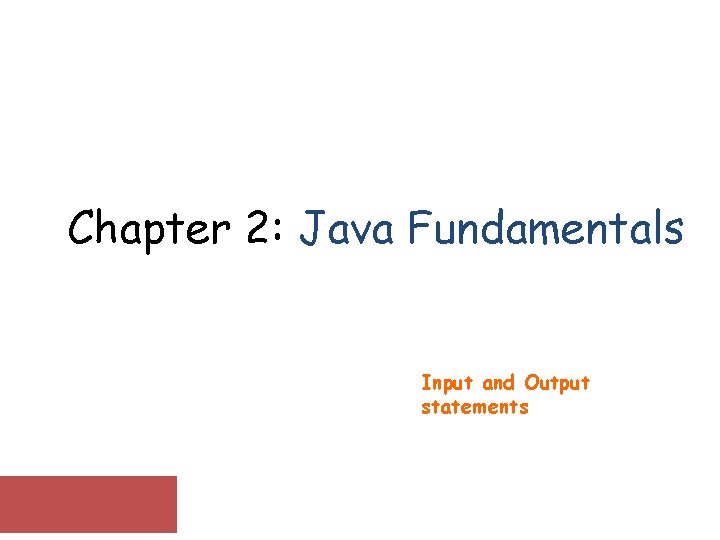
Chapter 2: Java Fundamentals Input and Output statements
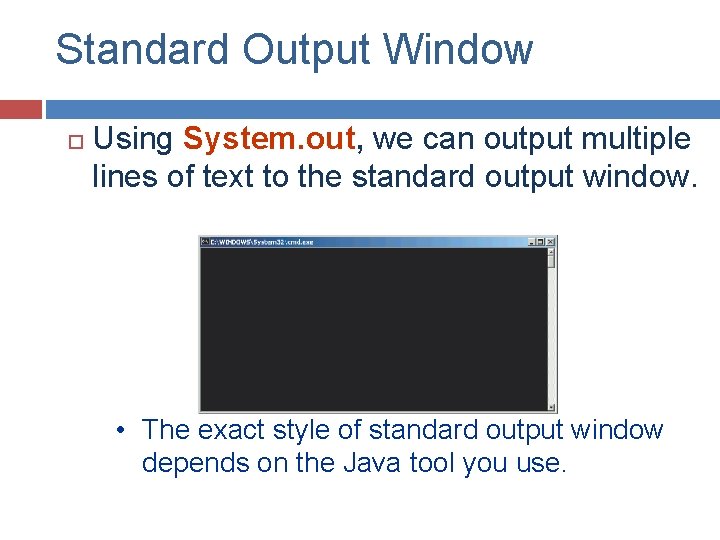
Standard Output Window Using System. out, we can output multiple lines of text to the standard output window. • The exact style of standard output window depends on the Java tool you use.
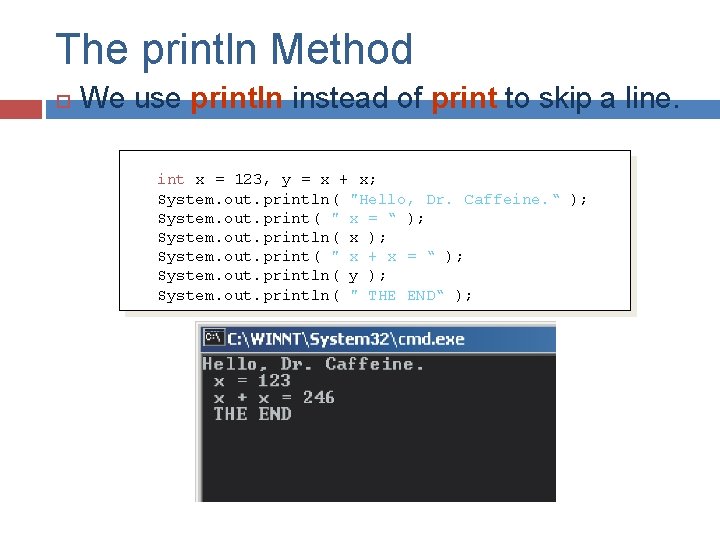
The println Method We use println instead of print to skip a line. int x = 123, y = x + x; System. out. println( "Hello, Dr. Caffeine. “ ); System. out. print( " x = “ ); System. out. println( x ); System. out. print( " x + x = “ ); System. out. println( y ); System. out. println( " THE END“ );
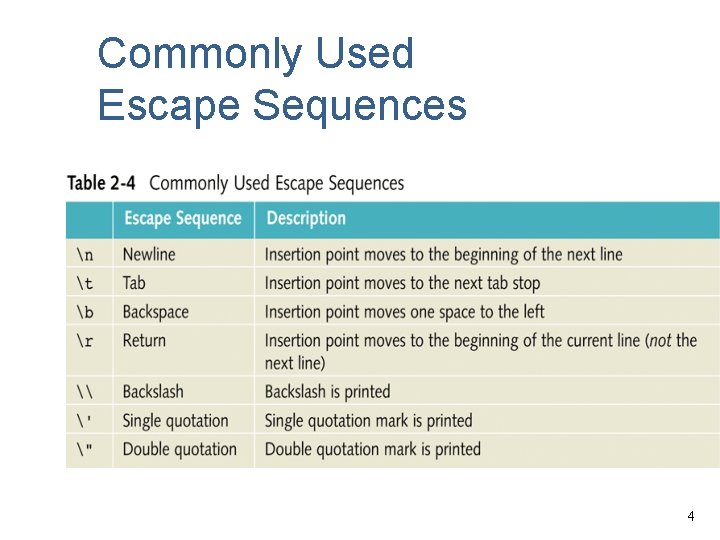
Commonly Used Escape Sequences 4
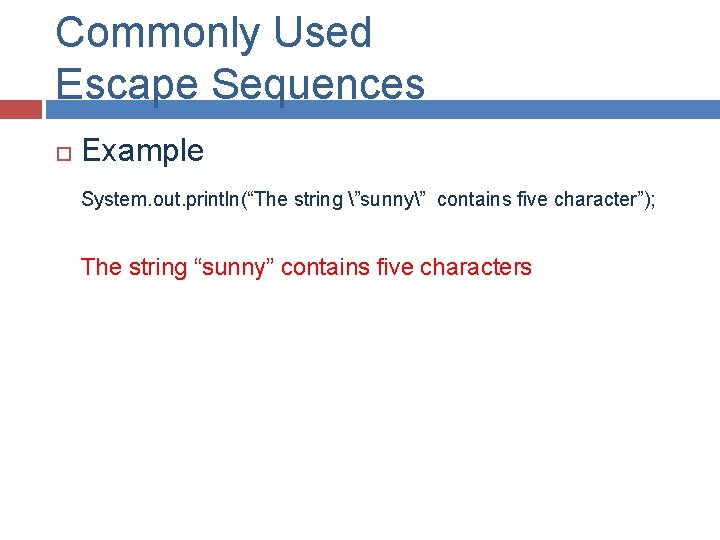
Commonly Used Escape Sequences Example System. out. println(“The string ”sunny” contains five character”); The string “sunny” contains five characters
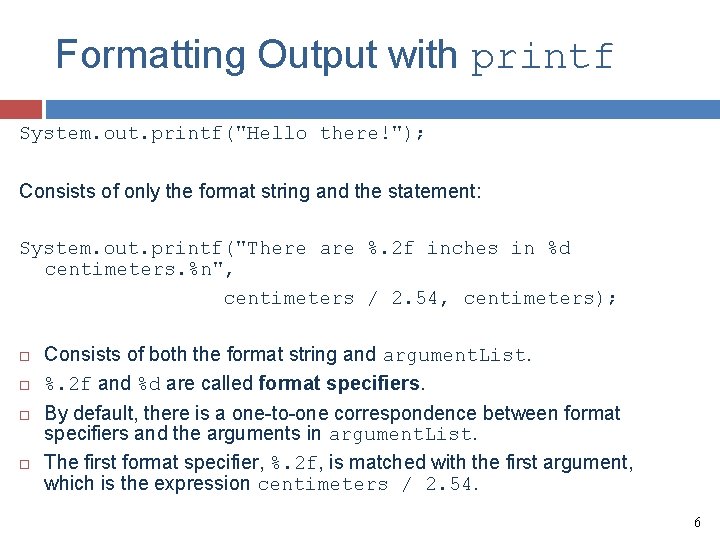
Formatting Output with printf System. out. printf("Hello there!"); Consists of only the format string and the statement: System. out. printf("There are %. 2 f inches in %d centimeters. %n", centimeters / 2. 54, centimeters); Consists of both the format string and argument. List. %. 2 f and %d are called format specifiers. By default, there is a one-to-one correspondence between format specifiers and the arguments in argument. List. The first format specifier, %. 2 f, is matched with the first argument, which is the expression centimeters / 2. 54. 6
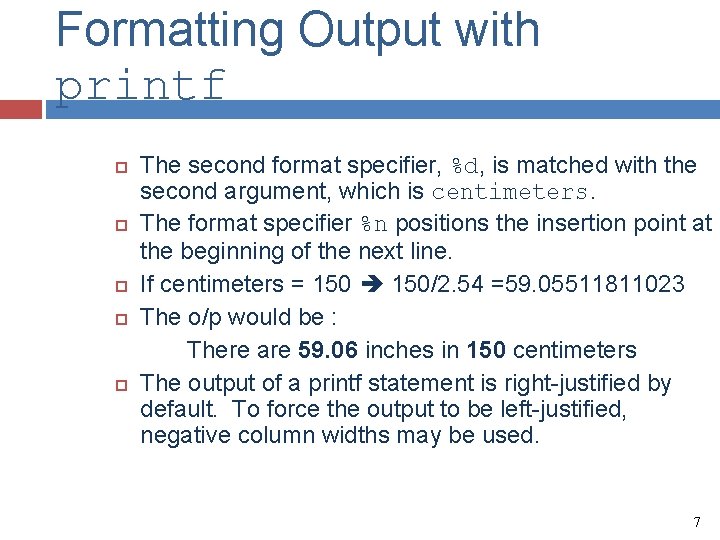
Formatting Output with printf The second format specifier, %d, is matched with the second argument, which is centimeters. The format specifier %n positions the insertion point at the beginning of the next line. If centimeters = 150/2. 54 =59. 05511811023 The o/p would be : There are 59. 06 inches in 150 centimeters The output of a printf statement is right-justified by default. To force the output to be left-justified, negative column widths may be used. 7
![Example 1 public class Example 36 public static void main String args Example 1 public class Example 3_6 { public static void main (String[] args) {](https://slidetodoc.com/presentation_image_h2/4a70918d6db06fd43898fd3de0754c30/image-8.jpg)
Example 1 public class Example 3_6 { public static void main (String[] args) { int num = 763; double x = 658. 75; String str = "Java Program. "; System. out. println("12345678901234567890"); System. out. printf ( "%5 d%7. 2 f%15 s%n", num, x, str); } } System. out. printf ("%15 s%6 d%9. 2 f %n", str, num, x); 8
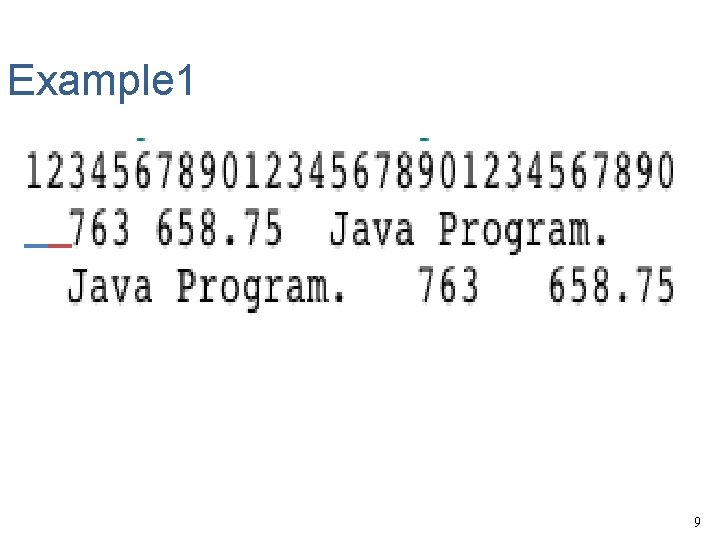
Example 1 9
![Example 2 public class Example 37 public static void main String args Example 2 public class Example 3_7 { public static void main (String[] args) {](https://slidetodoc.com/presentation_image_h2/4a70918d6db06fd43898fd3de0754c30/image-10.jpg)
Example 2 public class Example 3_7 { public static void main (String[] args) { int num = 763; double x = 658. 75; String str = "Java Program. "; System. out. println("12345678901234567890"); System. out. printf("%-5 d%-7. 2 f%-15 s ***%n", num, x, str); System. out. printf("%-15 s%-6 d%-9. 2 f ***%n", str, num, x); } } 10
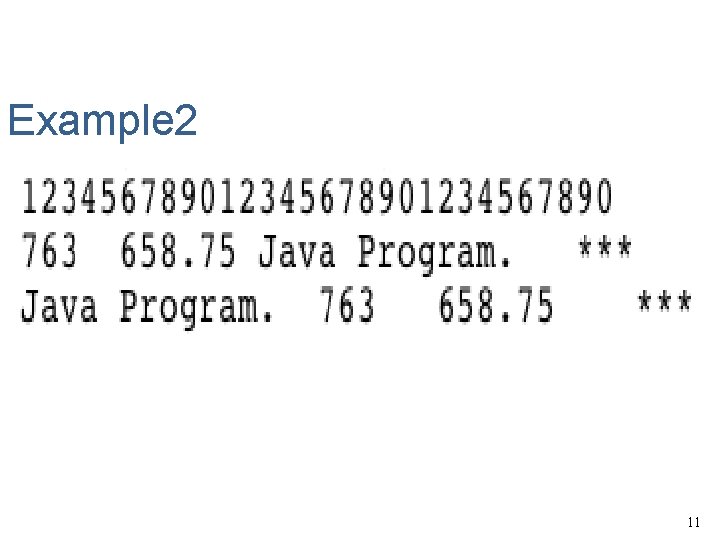
Example 2 11
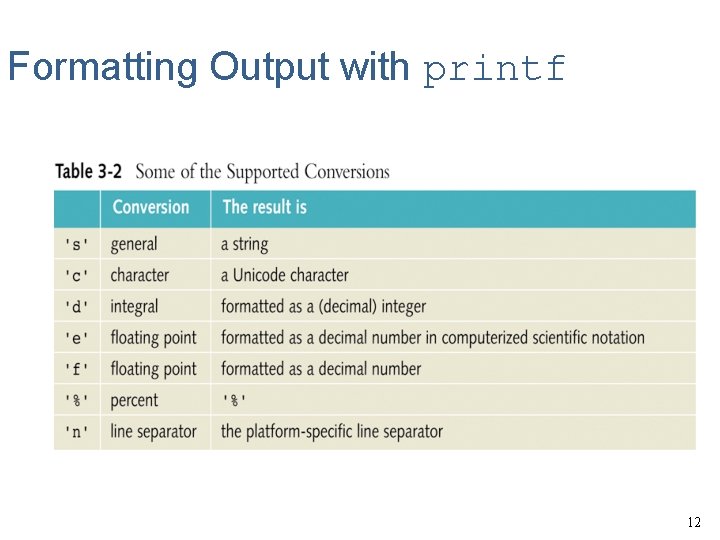
Formatting Output with printf 12
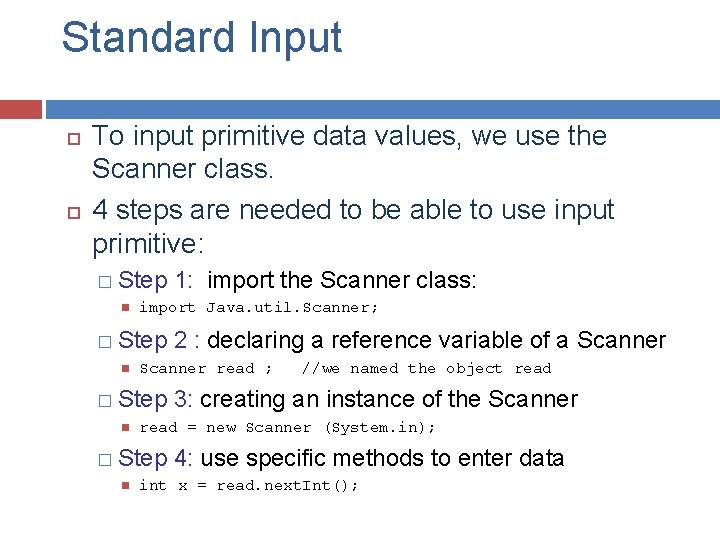
Standard Input To input primitive data values, we use the Scanner class. 4 steps are needed to be able to use input primitive: � Step import Java. util. Scanner; � Step //we named the object read 3: creating an instance of the Scanner read = new Scanner (System. in); � Step 2 : declaring a reference variable of a Scanner read ; � Step 1: import the Scanner class: 4: use specific methods to enter data int x = read. next. Int();
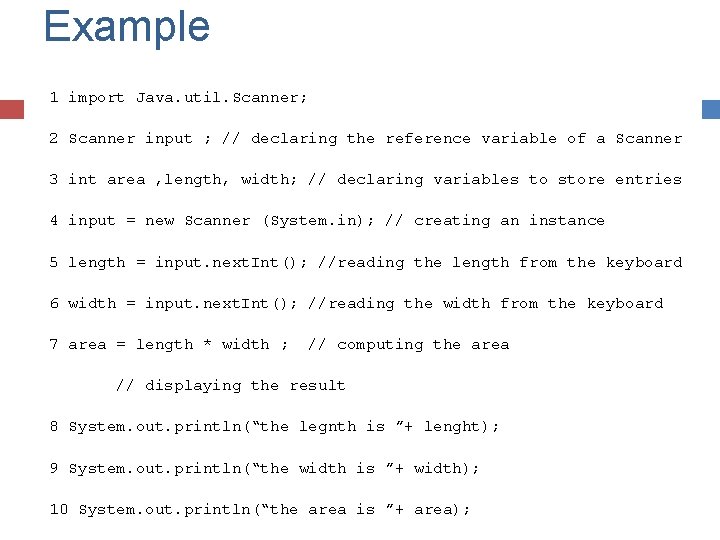
Example 1 import Java. util. Scanner; 2 Scanner input ; // declaring the reference variable of a Scanner 3 int area , length, width; // declaring variables to store entries 4 input = new Scanner (System. in); // creating an instance 5 length = input. next. Int(); //reading the length from the keyboard 6 width = input. next. Int(); //reading the width from the keyboard 7 area = length * width ; // computing the area // displaying the result 8 System. out. println(“the legnth is ”+ lenght); 9 System. out. println(“the width is ”+ width); 10 System. out. println(“the area is ”+ area);
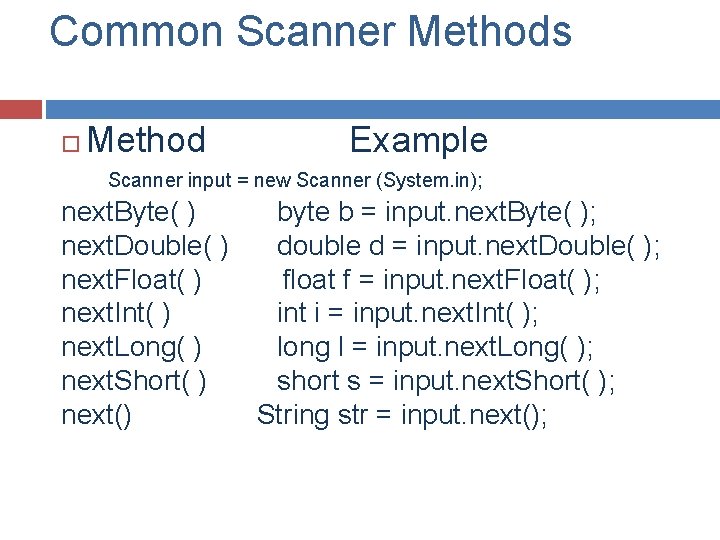
Common Scanner Methods Method Example Scanner input = new Scanner (System. in); next. Byte( ) byte b = input. next. Byte( ); next. Double( ) double d = input. next. Double( ); next. Float( ) float f = input. next. Float( ); next. Int( ) int i = input. next. Int( ); next. Long( ) long l = input. next. Long( ); next. Short( ) short s = input. next. Short( ); next() String str = input. next();
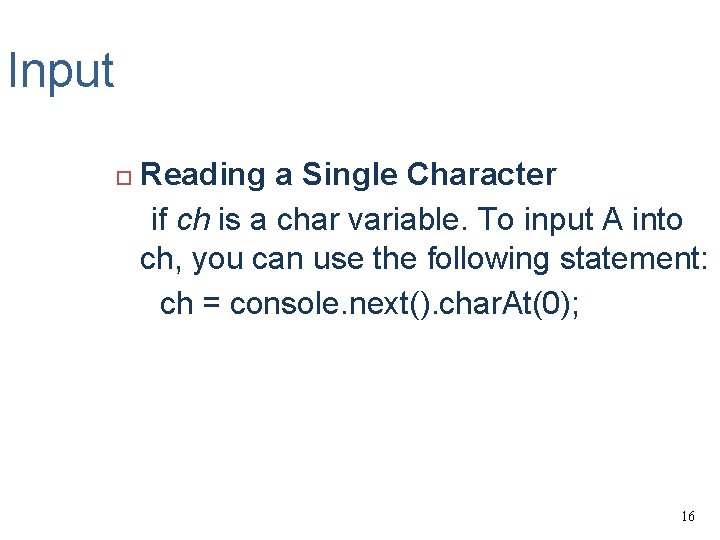
Input Reading a Single Character if ch is a char variable. To input A into ch, you can use the following statement: ch = console. next(). char. At(0); 16
Java input and output statements
Productivity = output/input
Chapter 15 input output java
Java file input and output
Console input and output in java
Java file input output
Chapter 6 input and output
Input and output devices chapter 2
Chapter 2 input processing and output
Chapter 2 lab java fundamentals
Input output design
Advantages of input devices
Vr input devices
Vat input
Input vs output vat
Input vat and output vat
Conclusion for input and output devices of computer