Collections Class This class consists exclusively of static
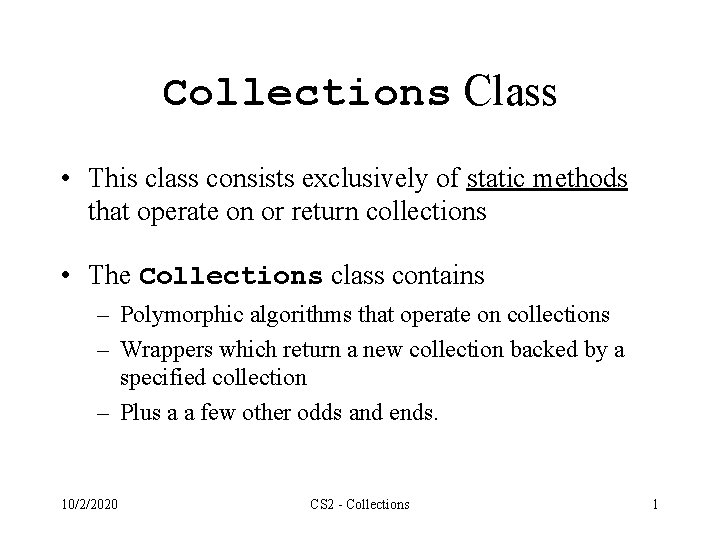
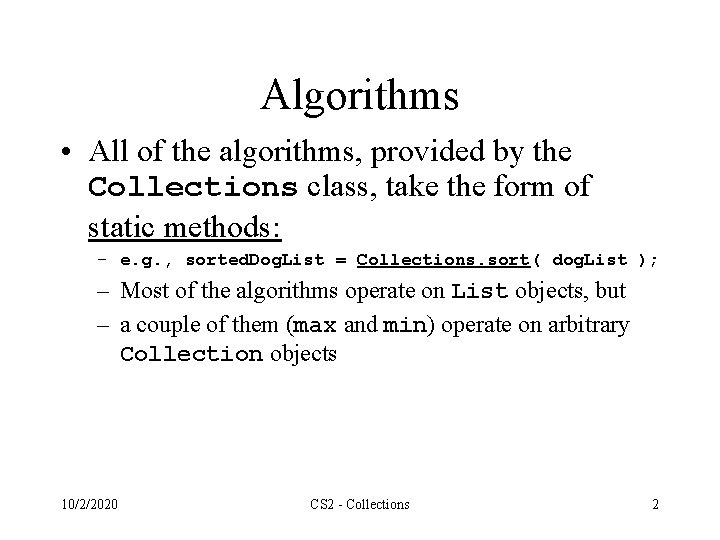
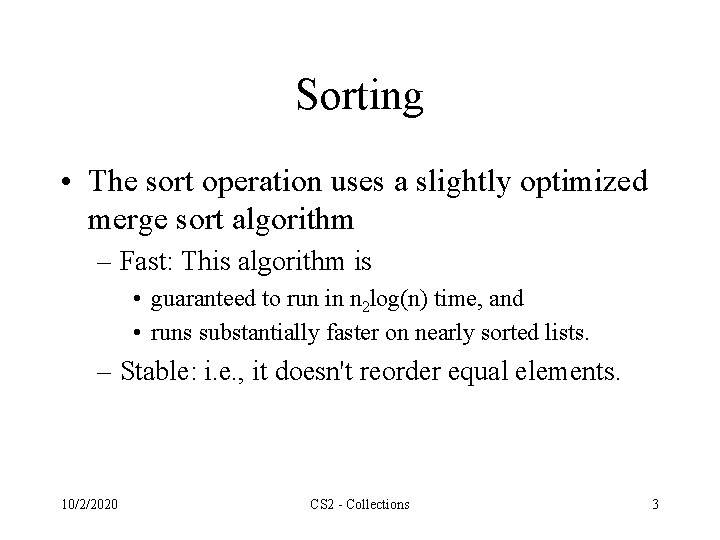
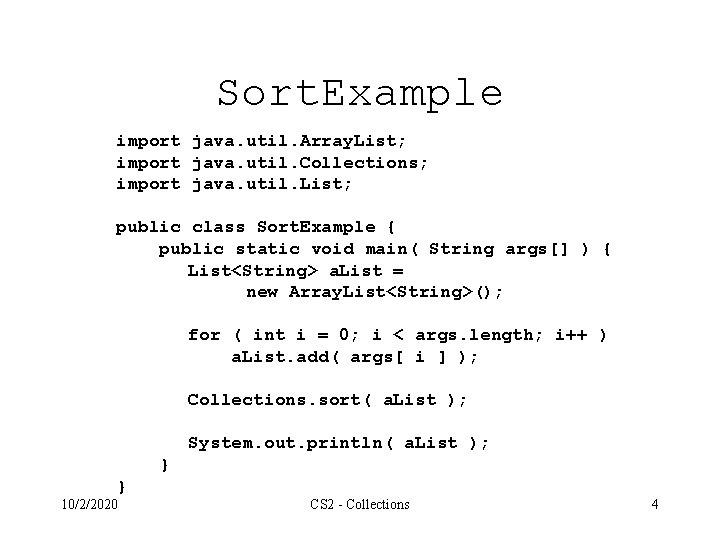
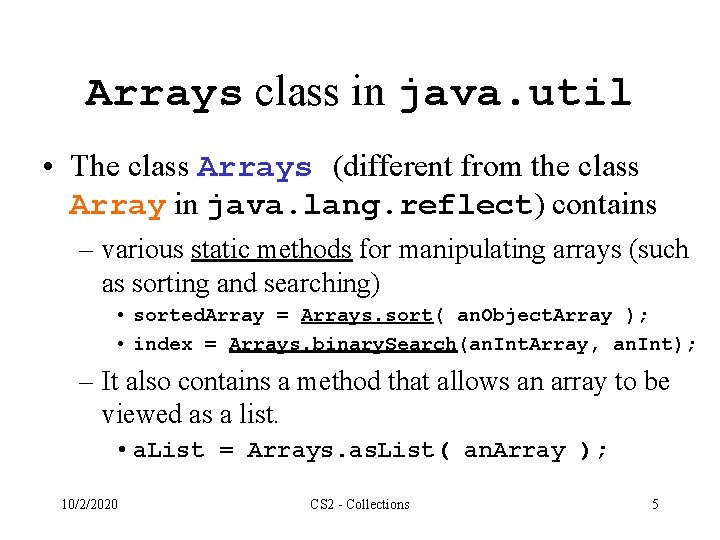
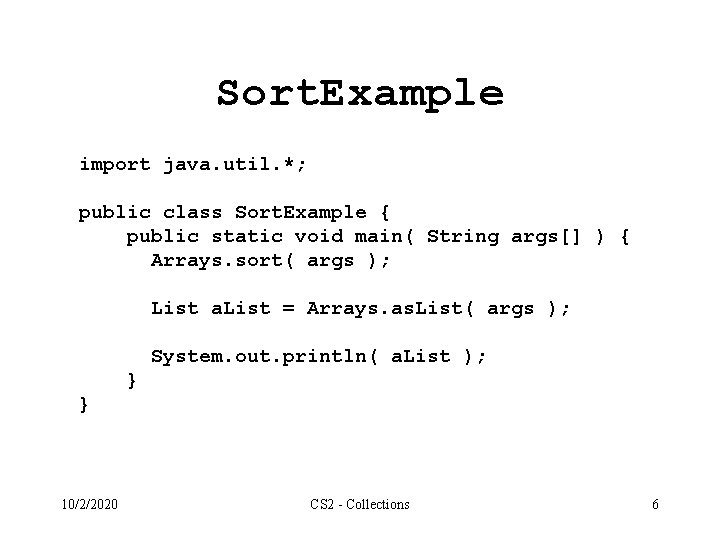
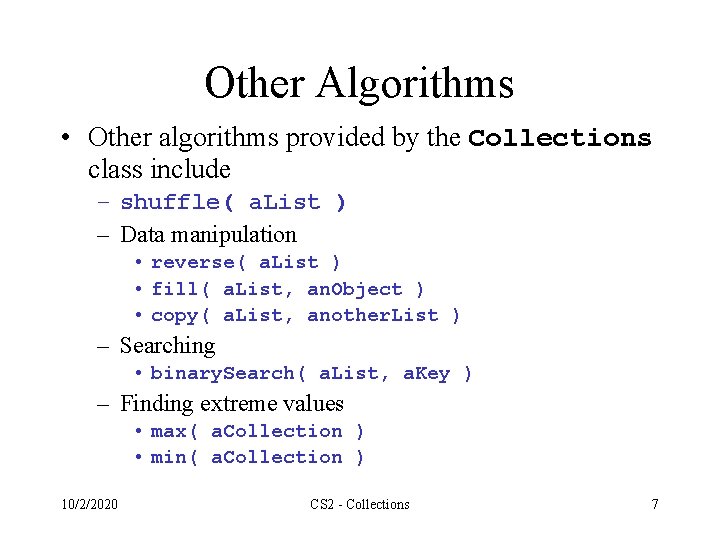
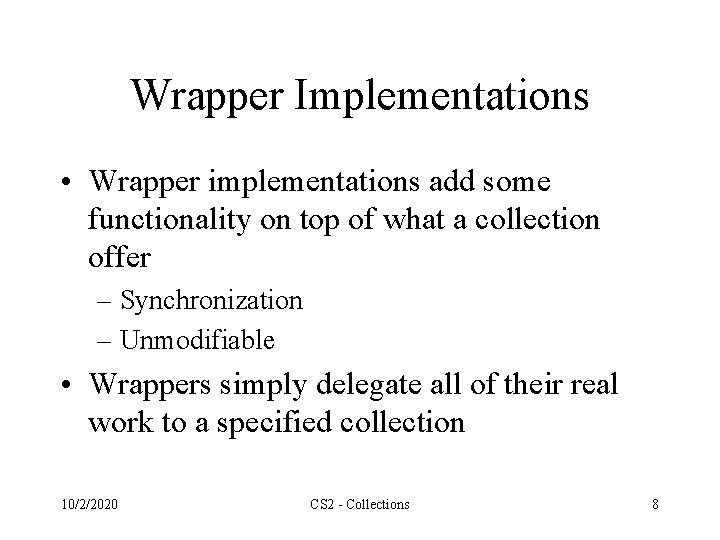
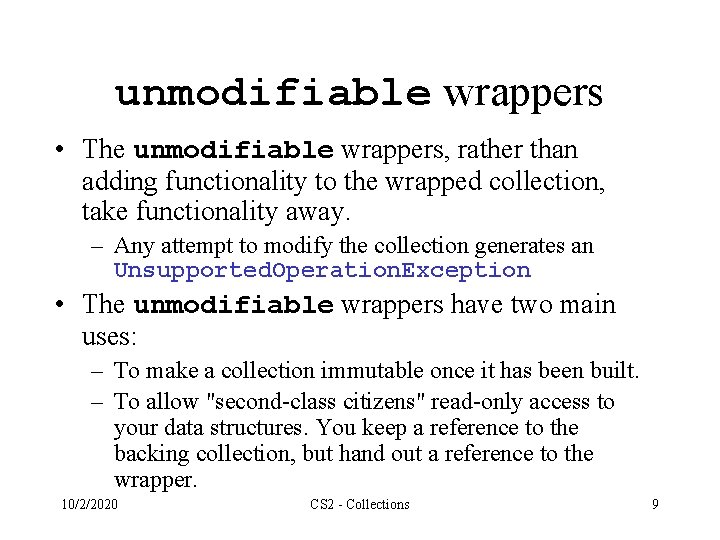
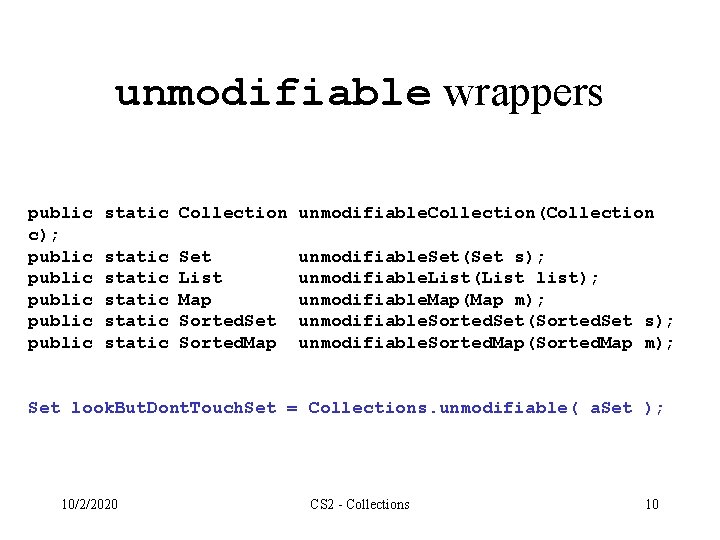
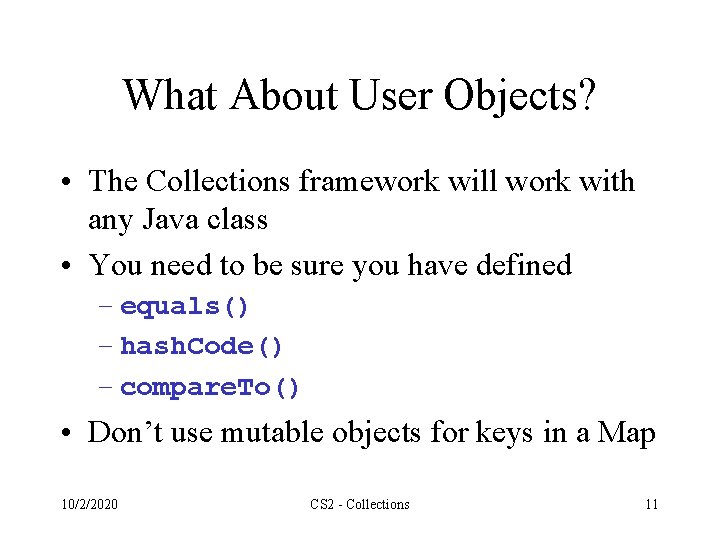
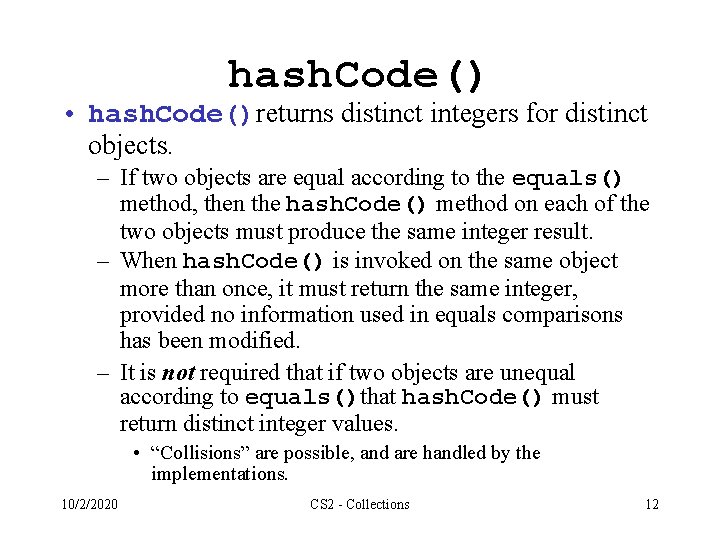
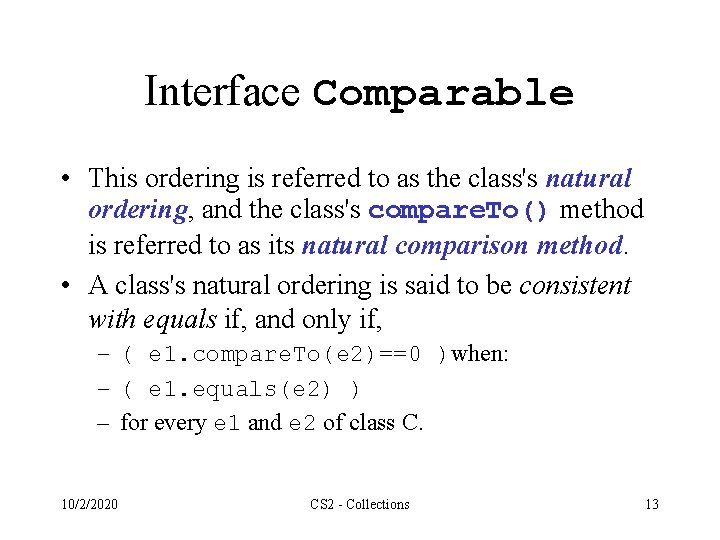
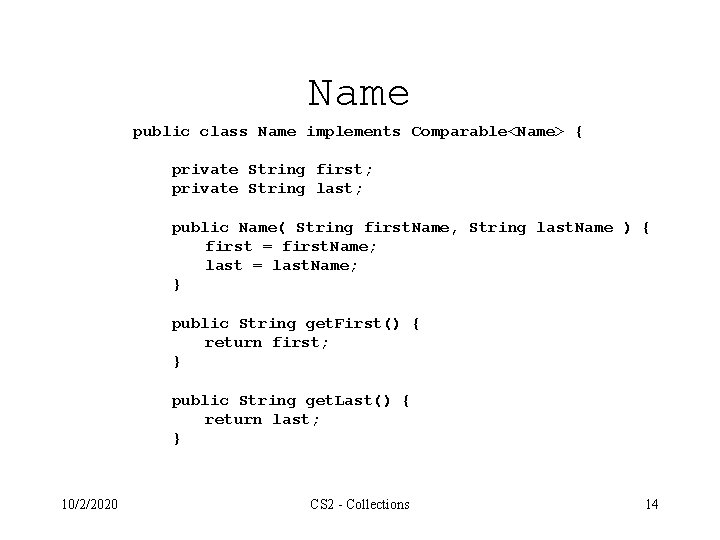
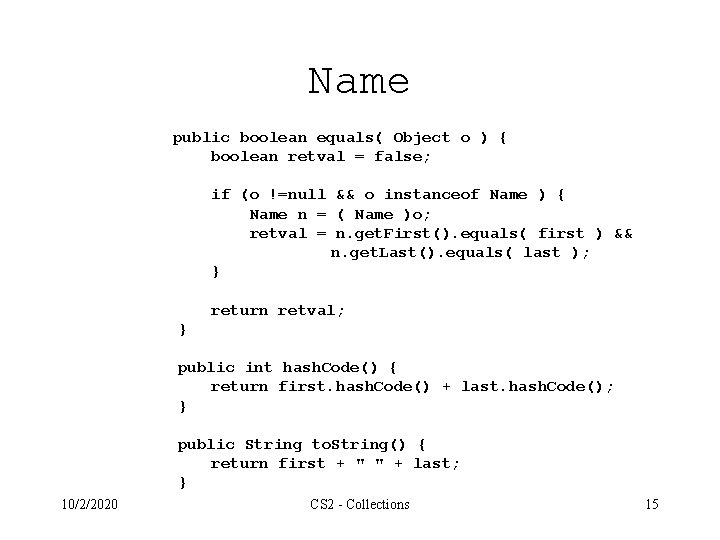
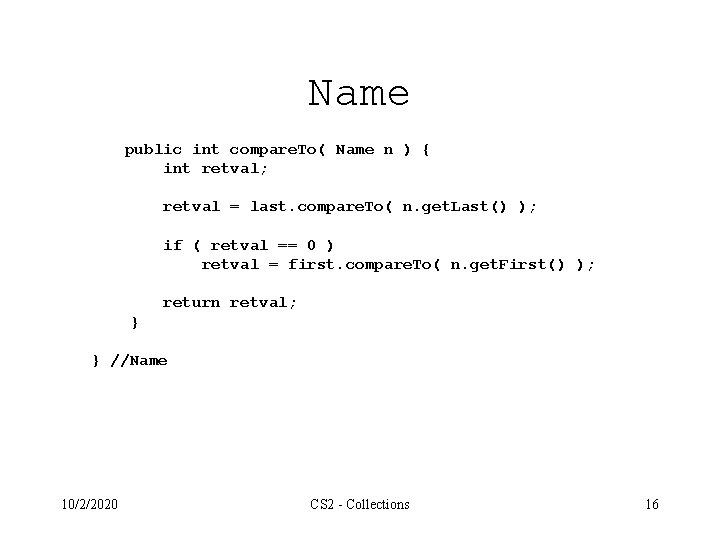
- Slides: 16
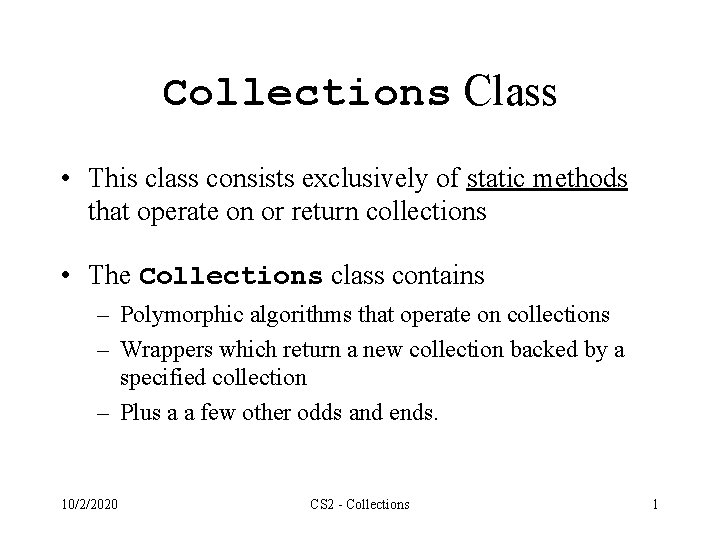
Collections Class • This class consists exclusively of static methods that operate on or return collections • The Collections class contains – Polymorphic algorithms that operate on collections – Wrappers which return a new collection backed by a specified collection – Plus a a few other odds and ends. 10/2/2020 CS 2 - Collections 1
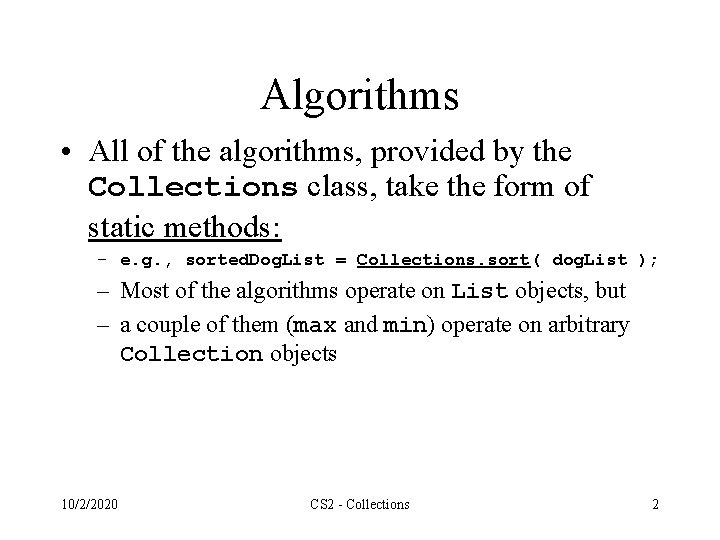
Algorithms • All of the algorithms, provided by the Collections class, take the form of static methods: – e. g. , sorted. Dog. List = Collections. sort( dog. List ); – Most of the algorithms operate on List objects, but – a couple of them (max and min) operate on arbitrary Collection objects 10/2/2020 CS 2 - Collections 2
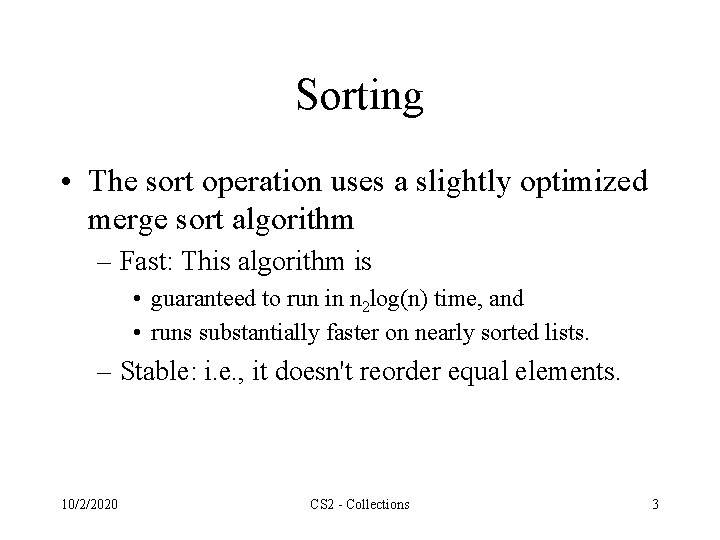
Sorting • The sort operation uses a slightly optimized merge sort algorithm – Fast: This algorithm is • guaranteed to run in n 2 log(n) time, and • runs substantially faster on nearly sorted lists. – Stable: i. e. , it doesn't reorder equal elements. 10/2/2020 CS 2 - Collections 3
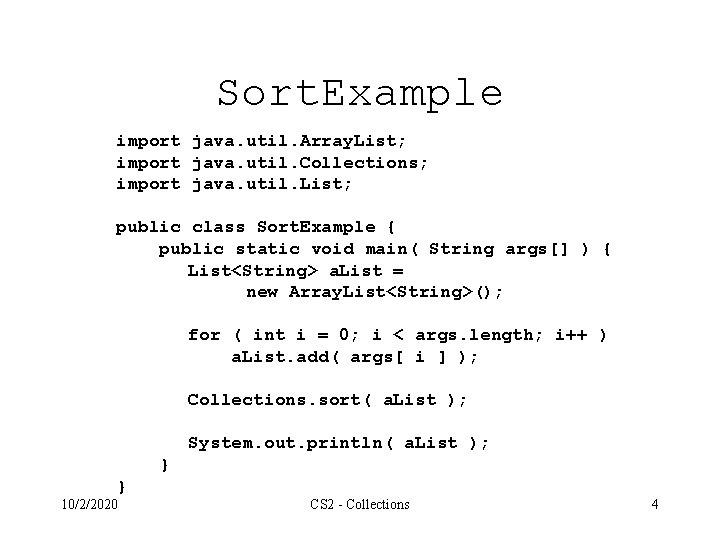
Sort. Example import java. util. Array. List; import java. util. Collections; import java. util. List; public class Sort. Example { public static void main( String args[] ) { List<String> a. List = new Array. List<String>(); for ( int i = 0; i < args. length; i++ ) a. List. add( args[ i ] ); Collections. sort( a. List ); System. out. println( a. List ); } } 10/2/2020 CS 2 - Collections 4
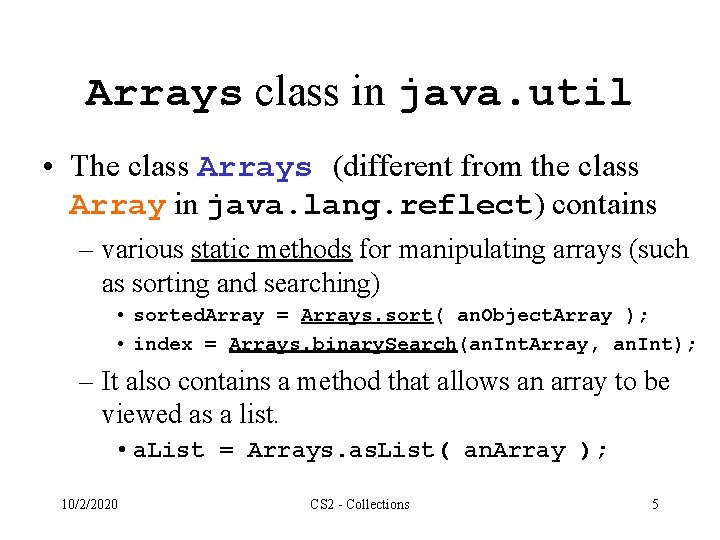
Arrays class in java. util • The class Arrays (different from the class Array in java. lang. reflect) contains – various static methods for manipulating arrays (such as sorting and searching) • sorted. Array = Arrays. sort( an. Object. Array ); • index = Arrays. binary. Search(an. Int. Array, an. Int); – It also contains a method that allows an array to be viewed as a list. • a. List = Arrays. as. List( an. Array ); 10/2/2020 CS 2 - Collections 5
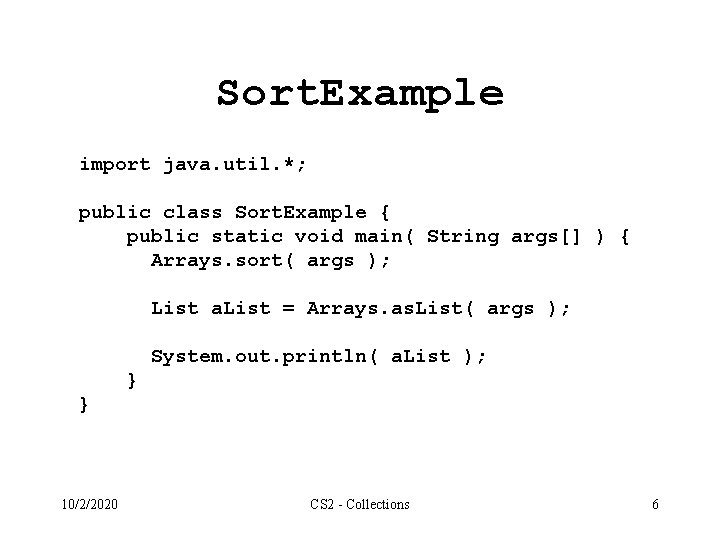
Sort. Example import java. util. *; public class Sort. Example { public static void main( String args[] ) { Arrays. sort( args ); List a. List = Arrays. as. List( args ); System. out. println( a. List ); } } 10/2/2020 CS 2 - Collections 6
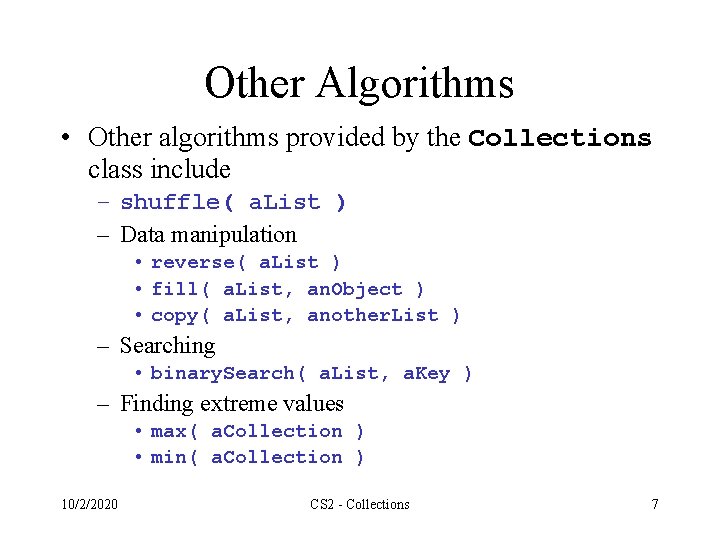
Other Algorithms • Other algorithms provided by the Collections class include – shuffle( a. List ) – Data manipulation • reverse( a. List ) • fill( a. List, an. Object ) • copy( a. List, another. List ) – Searching • binary. Search( a. List, a. Key ) – Finding extreme values • max( a. Collection ) • min( a. Collection ) 10/2/2020 CS 2 - Collections 7
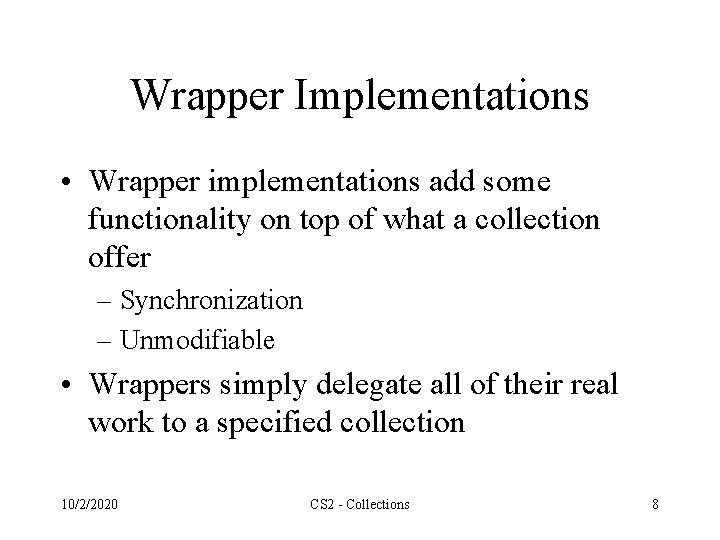
Wrapper Implementations • Wrapper implementations add some functionality on top of what a collection offer – Synchronization – Unmodifiable • Wrappers simply delegate all of their real work to a specified collection 10/2/2020 CS 2 - Collections 8
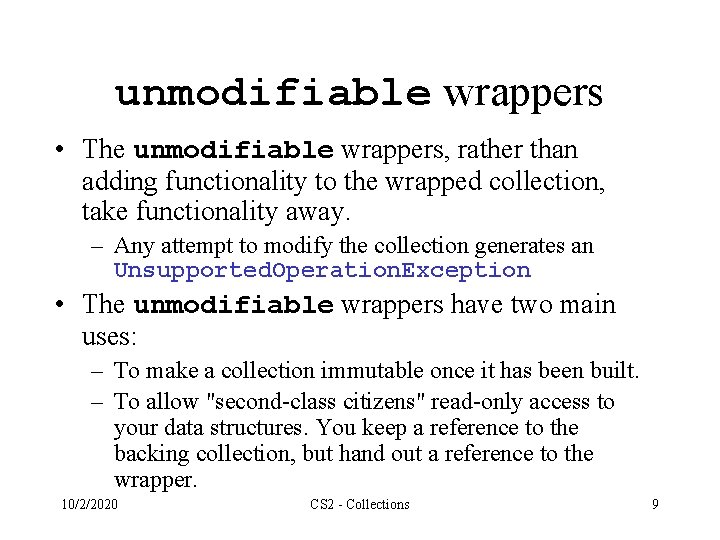
unmodifiable wrappers • The unmodifiable wrappers, rather than adding functionality to the wrapped collection, take functionality away. – Any attempt to modify the collection generates an Unsupported. Operation. Exception • The unmodifiable wrappers have two main uses: – To make a collection immutable once it has been built. – To allow "second-class citizens" read-only access to your data structures. You keep a reference to the backing collection, but hand out a reference to the wrapper. 10/2/2020 CS 2 - Collections 9
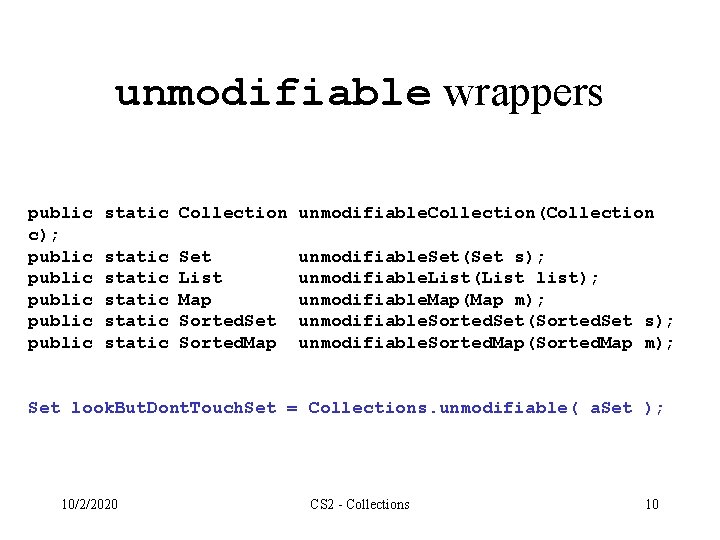
unmodifiable wrappers public c); public public static Collection unmodifiable. Collection(Collection static static Set List Map Sorted. Set Sorted. Map unmodifiable. Set(Set s); unmodifiable. List(List list); unmodifiable. Map(Map m); unmodifiable. Sorted. Set(Sorted. Set s); unmodifiable. Sorted. Map(Sorted. Map m); Set look. But. Dont. Touch. Set = Collections. unmodifiable( a. Set ); 10/2/2020 CS 2 - Collections 10
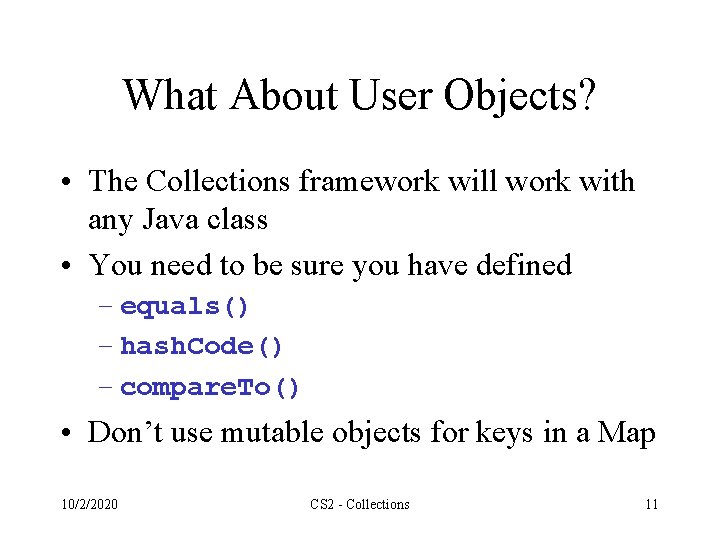
What About User Objects? • The Collections framework will work with any Java class • You need to be sure you have defined – equals() – hash. Code() – compare. To() • Don’t use mutable objects for keys in a Map 10/2/2020 CS 2 - Collections 11
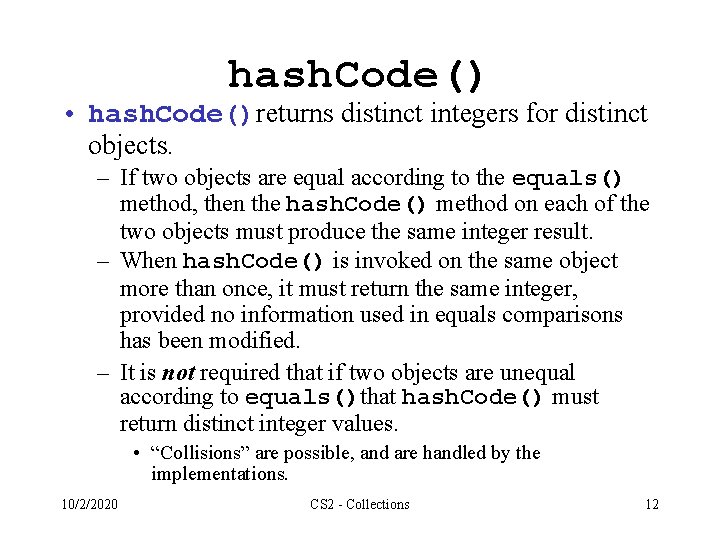
hash. Code() • hash. Code()returns distinct integers for distinct objects. – If two objects are equal according to the equals() method, then the hash. Code() method on each of the two objects must produce the same integer result. – When hash. Code() is invoked on the same object more than once, it must return the same integer, provided no information used in equals comparisons has been modified. – It is not required that if two objects are unequal according to equals()that hash. Code() must return distinct integer values. • “Collisions” are possible, and are handled by the implementations. 10/2/2020 CS 2 - Collections 12
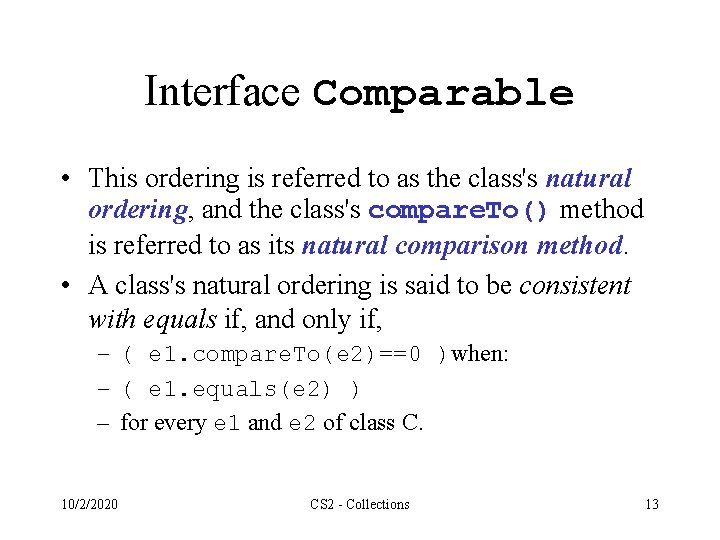
Interface Comparable • This ordering is referred to as the class's natural ordering, and the class's compare. To() method is referred to as its natural comparison method. • A class's natural ordering is said to be consistent with equals if, and only if, – ( e 1. compare. To(e 2)==0 )when: – ( e 1. equals(e 2) ) – for every e 1 and e 2 of class C. 10/2/2020 CS 2 - Collections 13
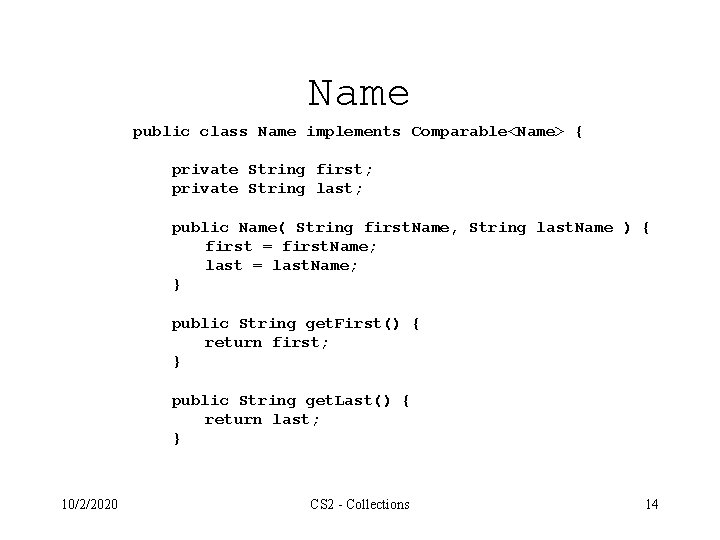
Name public class Name implements Comparable<Name> { private String first; private String last; public Name( String first. Name, String last. Name ) { first = first. Name; last = last. Name; } public String get. First() { return first; } public String get. Last() { return last; } 10/2/2020 CS 2 - Collections 14
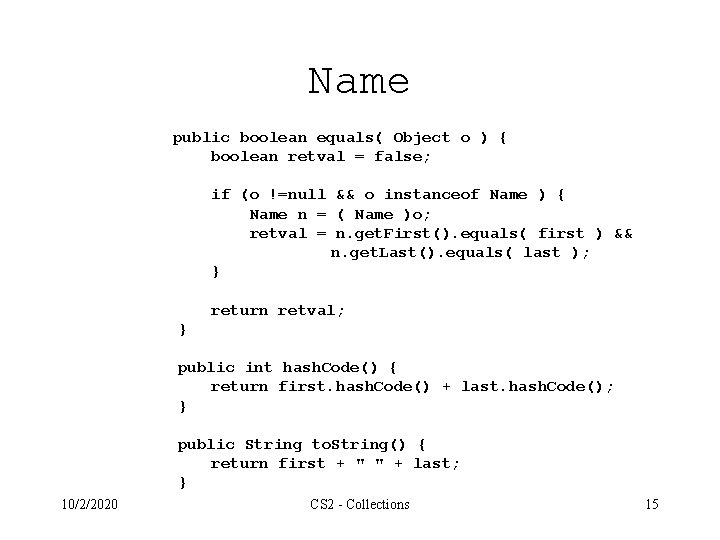
Name public boolean equals( Object o ) { boolean retval = false; if (o !=null && o instanceof Name ) { Name n = ( Name )o; retval = n. get. First(). equals( first ) && n. get. Last(). equals( last ); } return retval; } public int hash. Code() { return first. hash. Code() + last. hash. Code(); } public String to. String() { return first + " " + last; } 10/2/2020 CS 2 - Collections 15
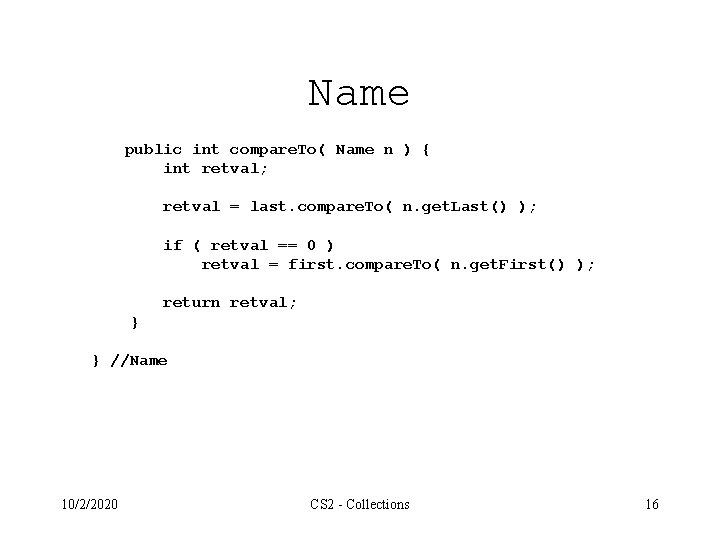
Name public int compare. To( Name n ) { int retval; retval = last. compare. To( n. get. Last() ); if ( retval == 0 ) retval = first. compare. To( n. get. First() ); return retval; } } //Name 10/2/2020 CS 2 - Collections 16