Servlet Filters import java io import javax servlet
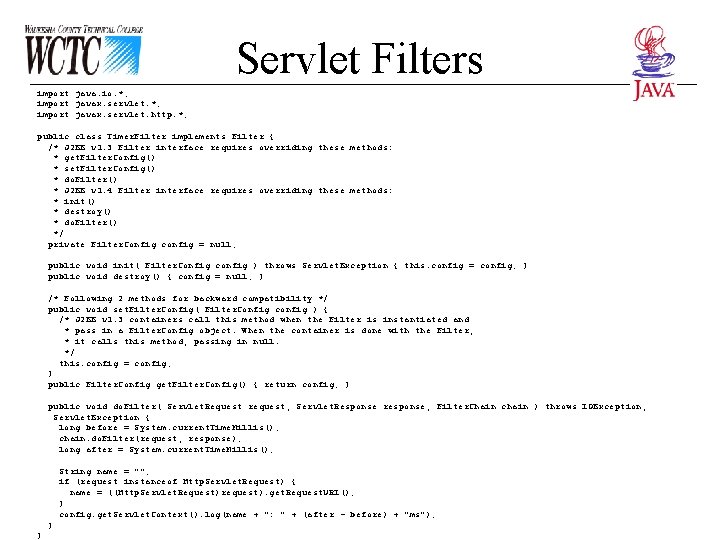
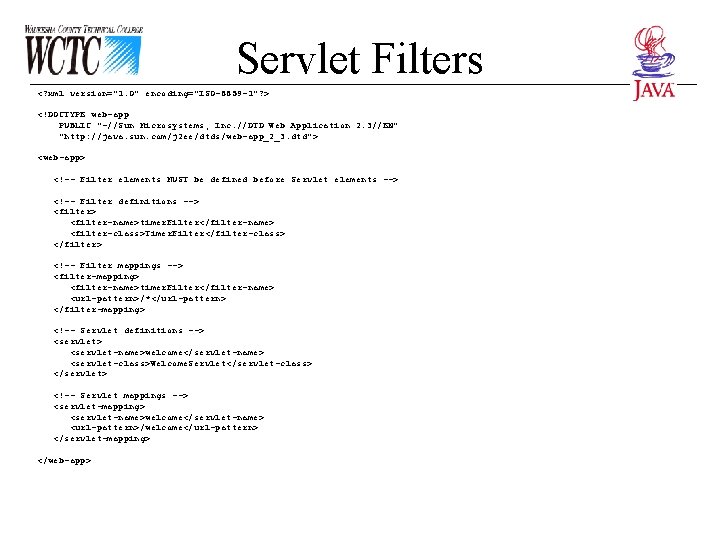
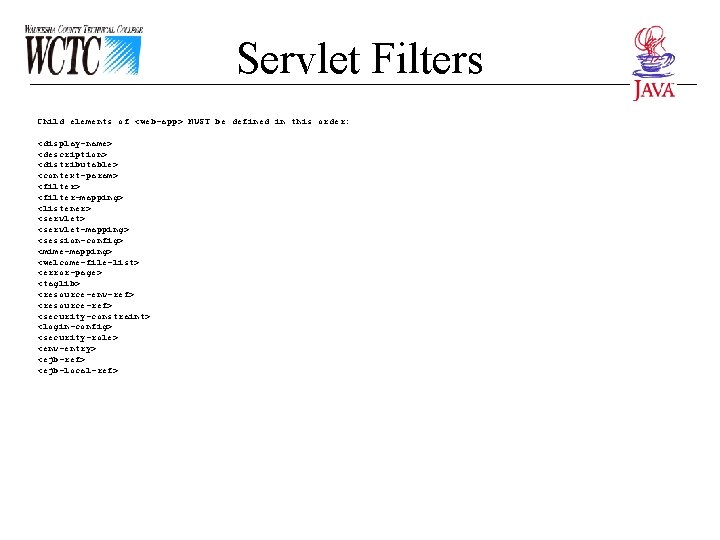
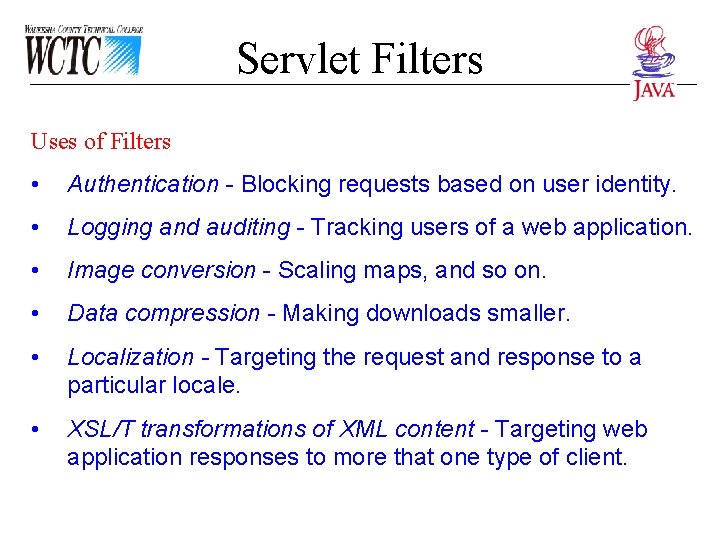
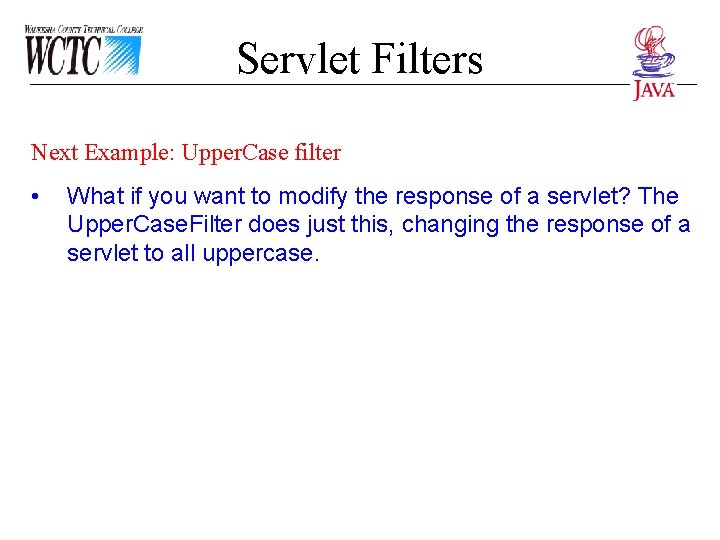
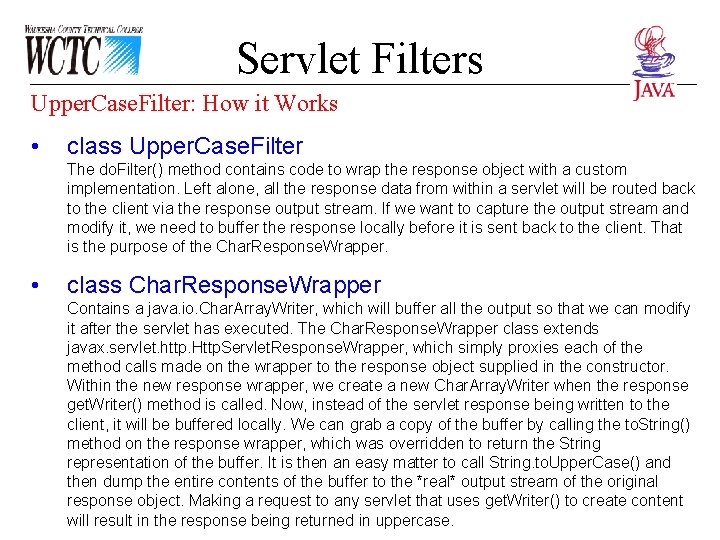
- Slides: 6
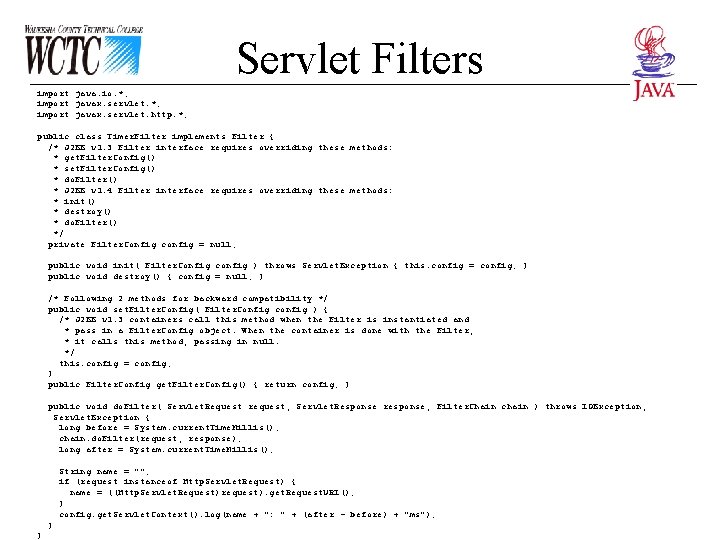
Servlet Filters import java. io. *; import javax. servlet. http. *; public class Timer. Filter implements Filter { /* J 2 EE v 1. 3 Filter interface requires overriding these methods: * get. Filter. Config() * set. Filter. Config() * do. Filter() * J 2 EE v 1. 4 Filter interface requires overriding these methods: * init() * destroy() * do. Filter() */ private Filter. Config config = null; public void init( Filter. Config config ) throws Servlet. Exception { this. config = config; } public void destroy() { config = null; } /* Following 2 methods for backward compatibility */ public void set. Filter. Config( Filter. Config config ) { /* J 2 EE v 1. 3 containers call this method when the Filter is instantiated and * pass in a Filter. Config object. When the container is done with the Filter, * it calls this method, passing in null. */ this. config = config; } public Filter. Config get. Filter. Config() { return config; } public void do. Filter( Servlet. Request request, Servlet. Response response, Filter. Chain chain ) throws IOException, Servlet. Exception { long before = System. current. Time. Millis(); chain. do. Filter(request, response); long after = System. current. Time. Millis(); String name = ""; if (request instanceof Http. Servlet. Request) { name = ((Http. Servlet. Request)request). get. Request. URI(); } config. get. Servlet. Context(). log(name + ": " + (after - before) + "ms"); } }
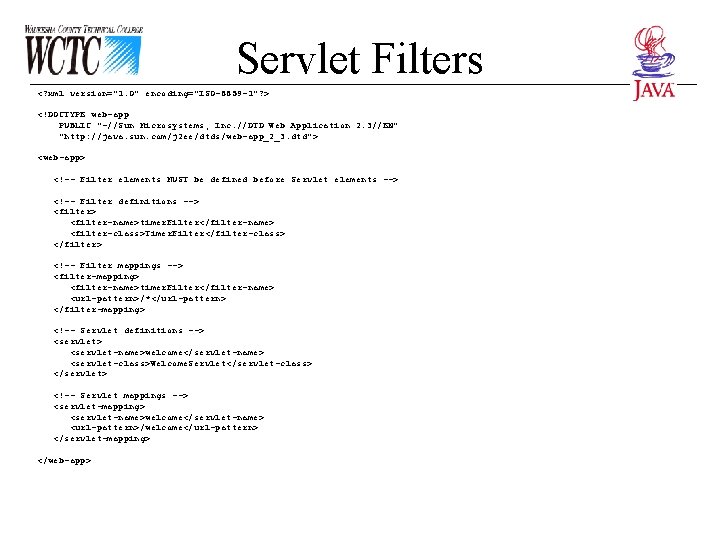
Servlet Filters <? xml version="1. 0" encoding="ISO-8859 -1"? > <!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc. //DTD Web Application 2. 3//EN" "http: //java. sun. com/j 2 ee/dtds/web-app_2_3. dtd"> <web-app> <!-- Filter elements MUST be defined before Servlet elements --> <!-- Filter definitions --> <filter-name>timer. Filter</filter-name> <filter-class>Timer. Filter</filter-class> </filter> <!-- Filter mappings --> <filter-mapping> <filter-name>timer. Filter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!-- Servlet definitions --> <servlet-name>welcome</servlet-name> <servlet-class>Welcome. Servlet</servlet-class> </servlet> <!-- Servlet mappings --> <servlet-mapping> <servlet-name>welcome</servlet-name> <url-pattern>/welcome</url-pattern> </servlet-mapping> </web-app>
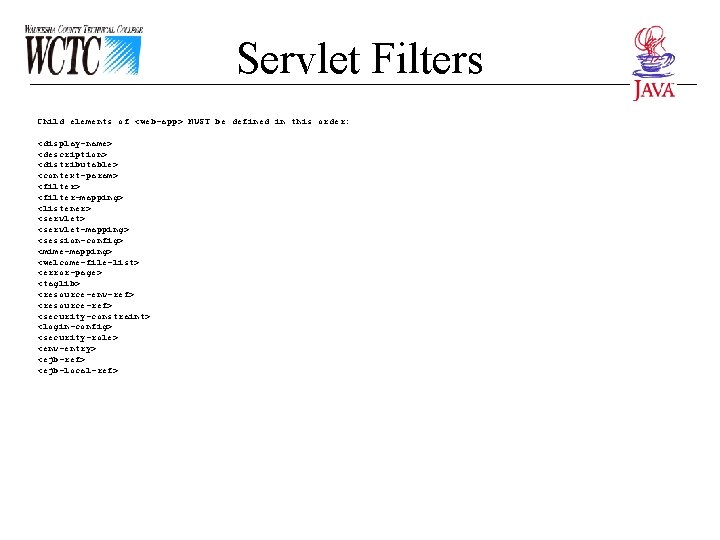
Servlet Filters Child elements of <web-app> MUST be defined in this order: <display-name> <description> <distributable> <context-param> <filter-mapping> <listener> <servlet-mapping> <session-config> <mime-mapping> <welcome-file-list> <error-page> <taglib> <resource-env-ref> <resource-ref> <security-constraint> <login-config> <security-role> <env-entry> <ejb-ref> <ejb-local-ref>
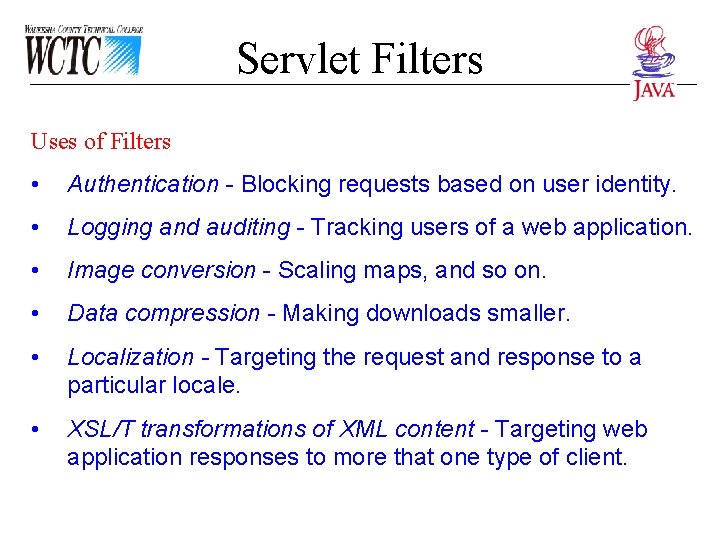
Servlet Filters Uses of Filters • Authentication - Blocking requests based on user identity. • Logging and auditing - Tracking users of a web application. • Image conversion - Scaling maps, and so on. • Data compression - Making downloads smaller. • Localization - Targeting the request and response to a particular locale. • XSL/T transformations of XML content - Targeting web application responses to more that one type of client.
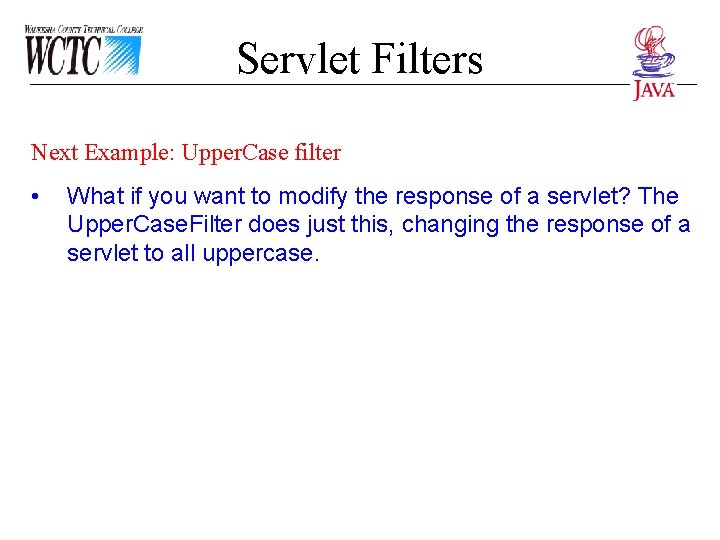
Servlet Filters Next Example: Upper. Case filter • What if you want to modify the response of a servlet? The Upper. Case. Filter does just this, changing the response of a servlet to all uppercase.
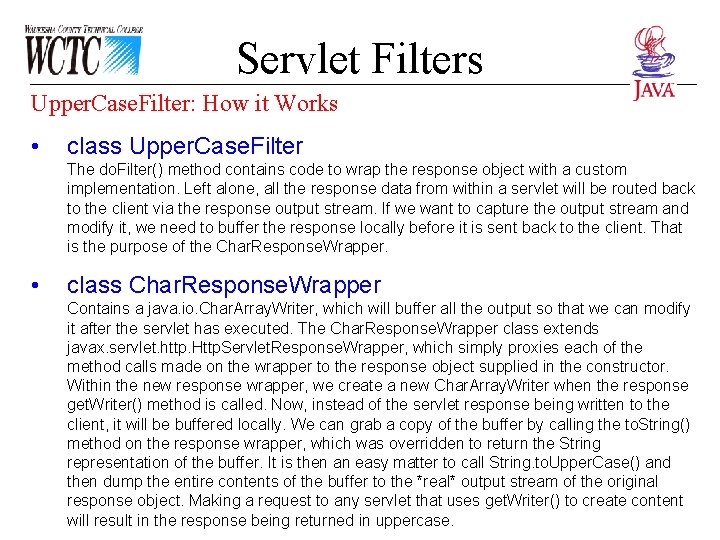
Servlet Filters Upper. Case. Filter: How it Works • class Upper. Case. Filter The do. Filter() method contains code to wrap the response object with a custom implementation. Left alone, all the response data from within a servlet will be routed back to the client via the response output stream. If we want to capture the output stream and modify it, we need to buffer the response locally before it is sent back to the client. That is the purpose of the Char. Response. Wrapper. • class Char. Response. Wrapper Contains a java. io. Char. Array. Writer, which will buffer all the output so that we can modify it after the servlet has executed. The Char. Response. Wrapper class extends javax. servlet. http. Http. Servlet. Response. Wrapper, which simply proxies each of the method calls made on the wrapper to the response object supplied in the constructor. Within the new response wrapper, we create a new Char. Array. Writer when the response get. Writer() method is called. Now, instead of the servlet response being written to the client, it will be buffered locally. We can grab a copy of the buffer by calling the to. String() method on the response wrapper, which was overridden to return the String representation of the buffer. It is then an easy matter to call String. to. Upper. Case() and then dump the entire contents of the buffer to the *real* output stream of the original response object. Making a request to any servlet that uses get. Writer() to create content will result in the response being returned in uppercase.