the Math class Java provides certain math functions
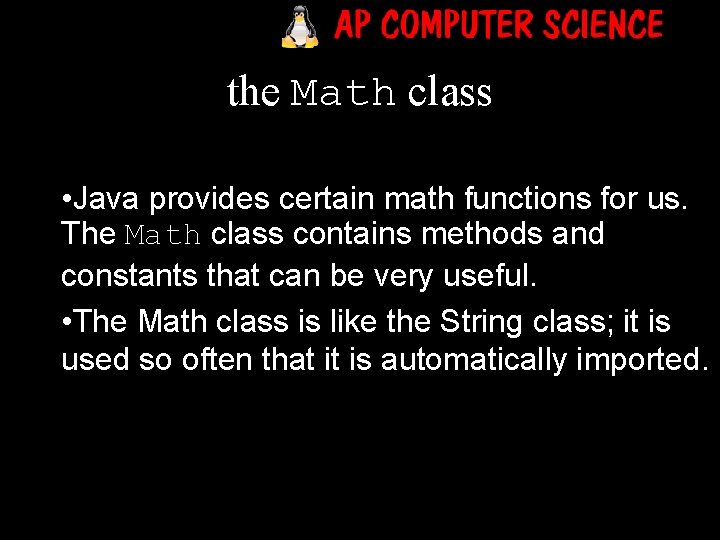
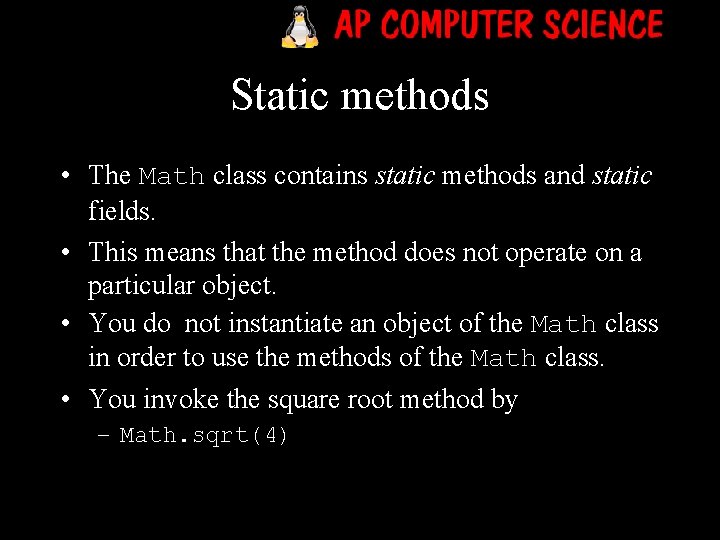
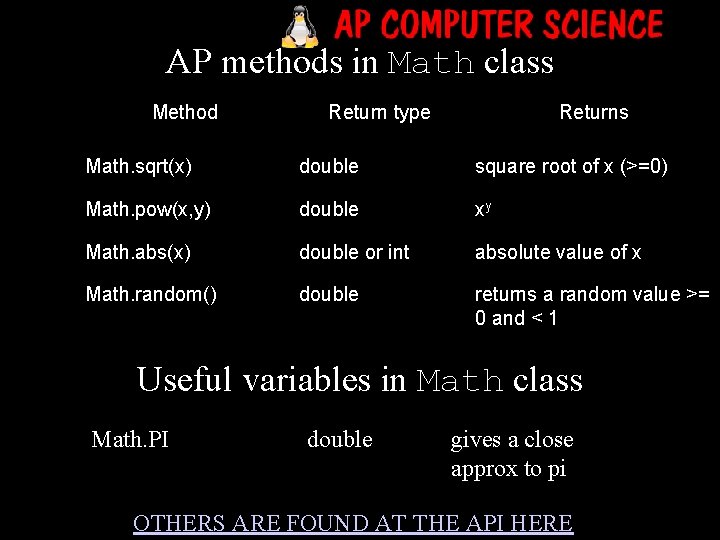
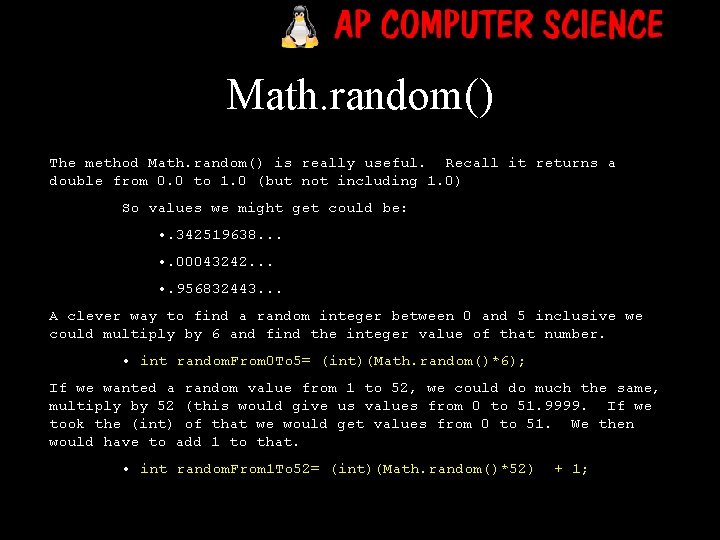
![Example public static void main(String[] args) { System. out. println(Math. sqrt(16)); System. out. println(Math. Example public static void main(String[] args) { System. out. println(Math. sqrt(16)); System. out. println(Math.](https://slidetodoc.com/presentation_image_h/290514456445e8e86c2bdf93ea0a644d/image-5.jpg)
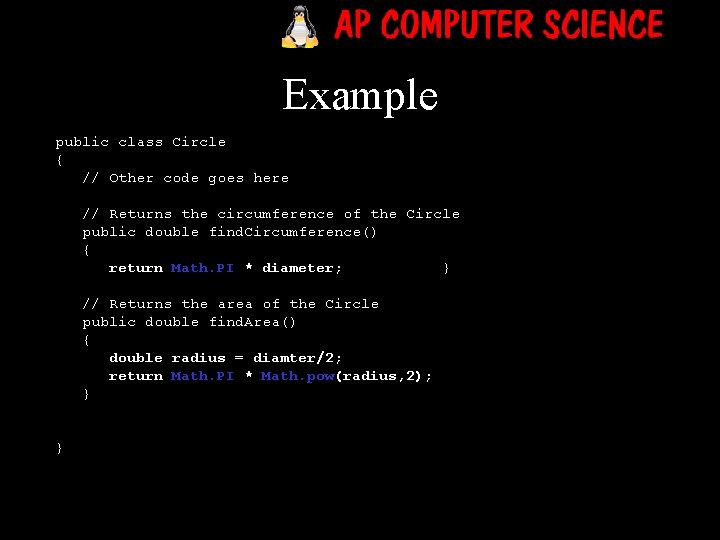
- Slides: 6
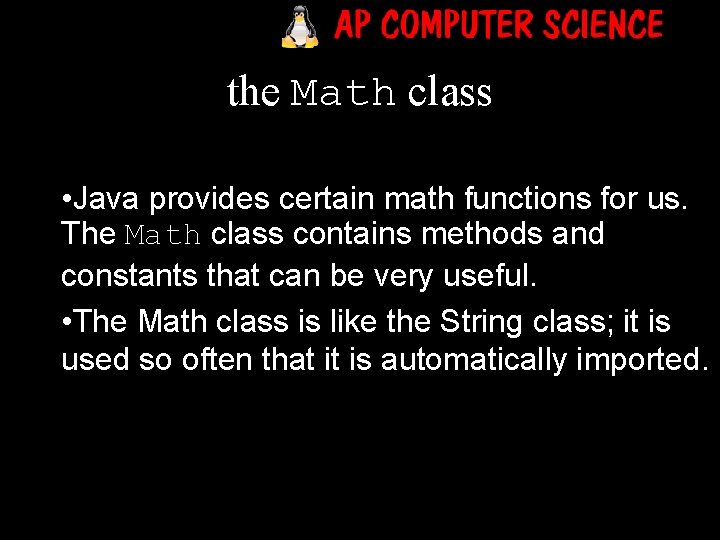
the Math class • Java provides certain math functions for us. The Math class contains methods and constants that can be very useful. • The Math class is like the String class; it is used so often that it is automatically imported.
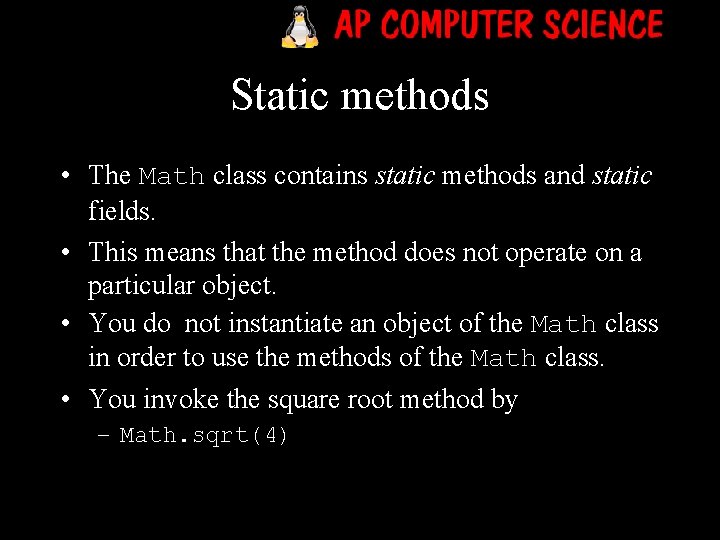
Static methods • The Math class contains static methods and static fields. • This means that the method does not operate on a particular object. • You do not instantiate an object of the Math class in order to use the methods of the Math class. • You invoke the square root method by – Math. sqrt(4)
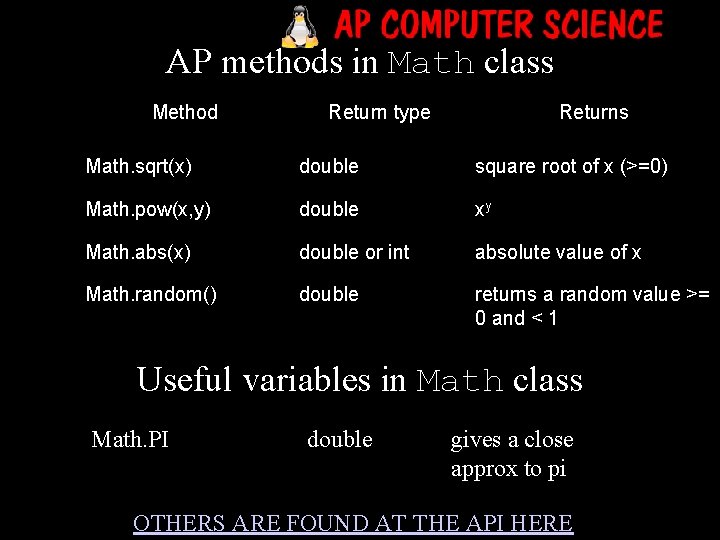
AP methods in Math class Method Return type Returns Math. sqrt(x) double square root of x (>=0) Math. pow(x, y) double xy Math. abs(x) double or int absolute value of x Math. random() double returns a random value >= 0 and < 1 Useful variables in Math class Math. PI double gives a close approx to pi OTHERS ARE FOUND AT THE API HERE
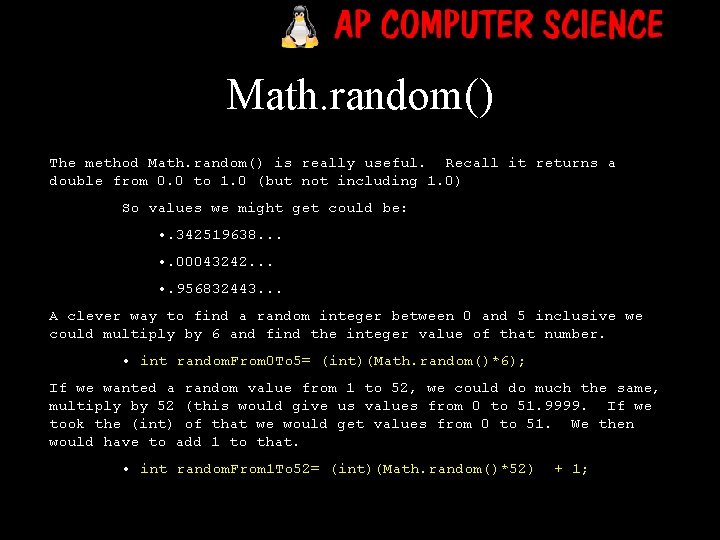
Math. random() The method Math. random() is really useful. Recall it returns a double from 0. 0 to 1. 0 (but not including 1. 0) So values we might get could be: • . 342519638. . . • . 00043242. . . • . 956832443. . . A clever way to find a random integer between 0 and 5 inclusive we could multiply by 6 and find the integer value of that number. • int random. From 0 To 5= (int)(Math. random()*6); If we wanted a random value from 1 to 52, we could do much the same, multiply by 52 (this would give us values from 0 to 51. 9999. If we took the (int) of that we would get values from 0 to 51. We then would have to add 1 to that. • int random. From 1 To 52= (int)(Math. random()*52) + 1;
![Example public static void mainString args System out printlnMath sqrt16 System out printlnMath Example public static void main(String[] args) { System. out. println(Math. sqrt(16)); System. out. println(Math.](https://slidetodoc.com/presentation_image_h/290514456445e8e86c2bdf93ea0a644d/image-5.jpg)
Example public static void main(String[] args) { System. out. println(Math. sqrt(16)); System. out. println(Math. random()); System. out. println((int)(Math. random()*3+1)); System. out. println(Math. abs(-5)); System. out. println(Math. pow(2, 5)); } 4. 0 0. 7872152671821442 1 5 32. 0
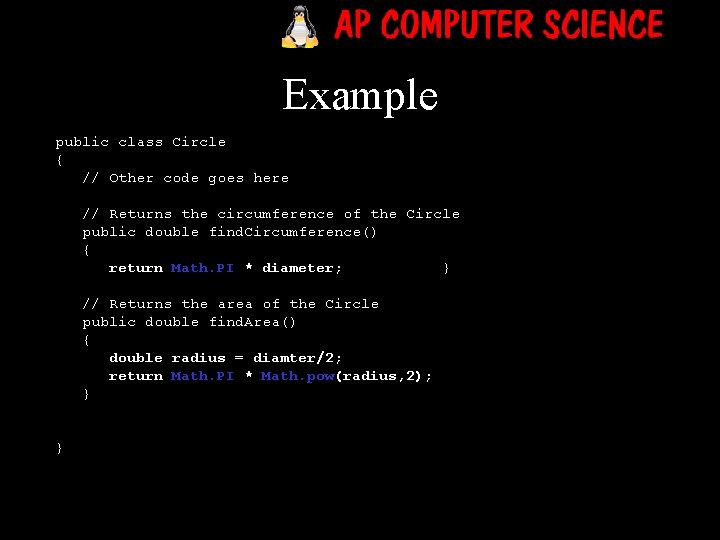
Example public class Circle { // Other code goes here // Returns the circumference of the Circle public double find. Circumference() { return Math. PI * diameter; } // Returns the area of the Circle public double find. Area() { double radius = diamter/2; return Math. PI * Math. pow(radius, 2); } }