Introduction to Java import java util Scanner Import
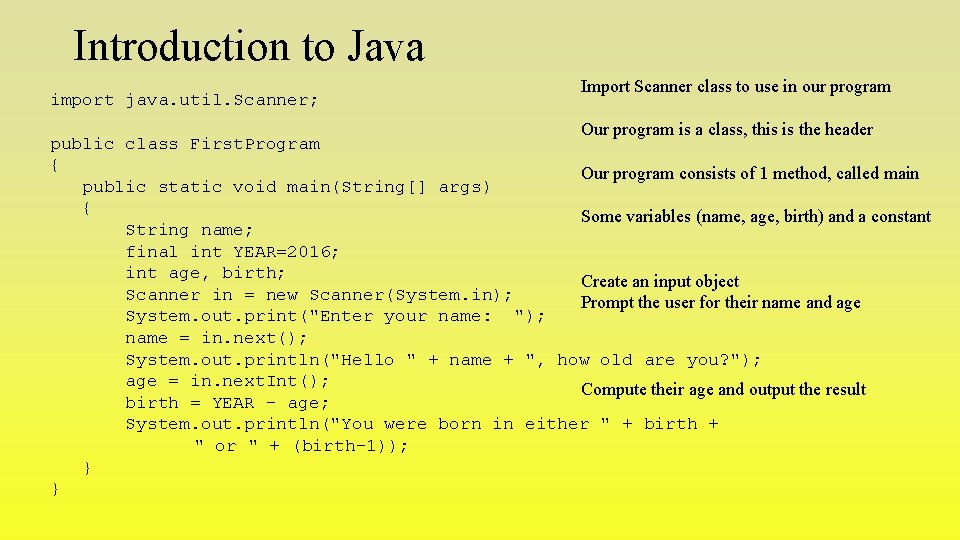
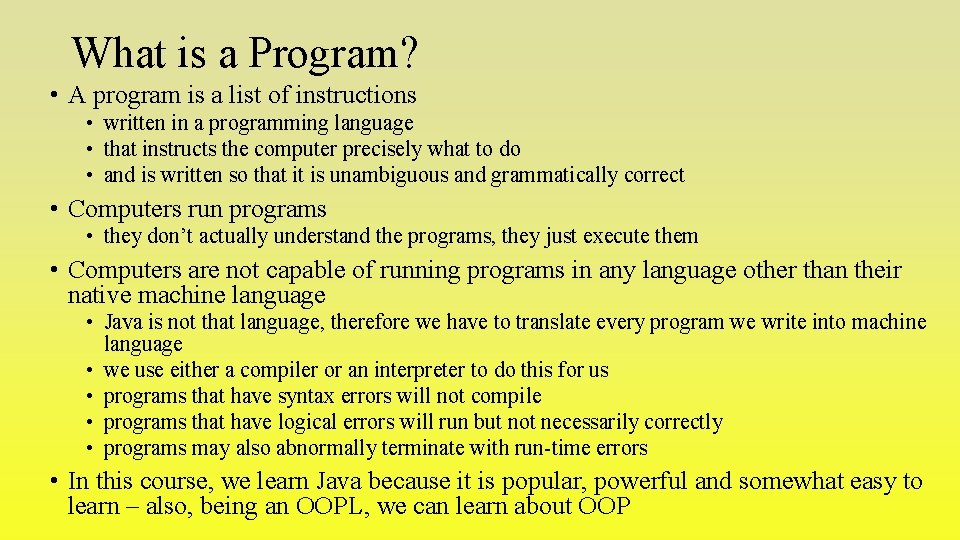
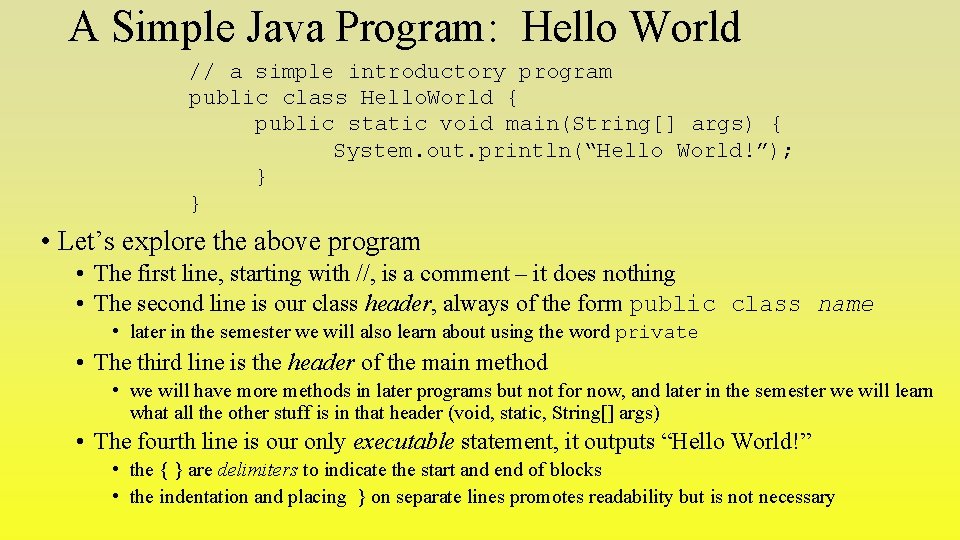
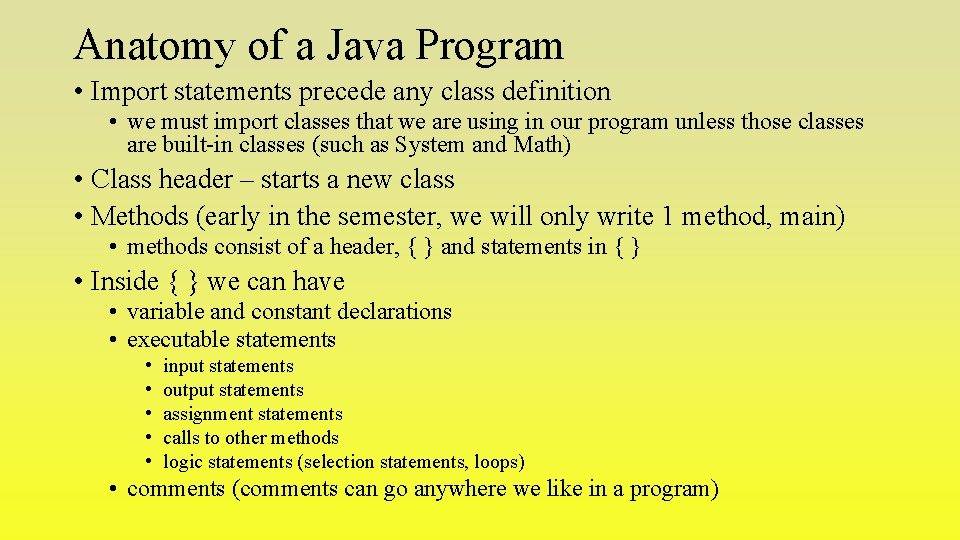
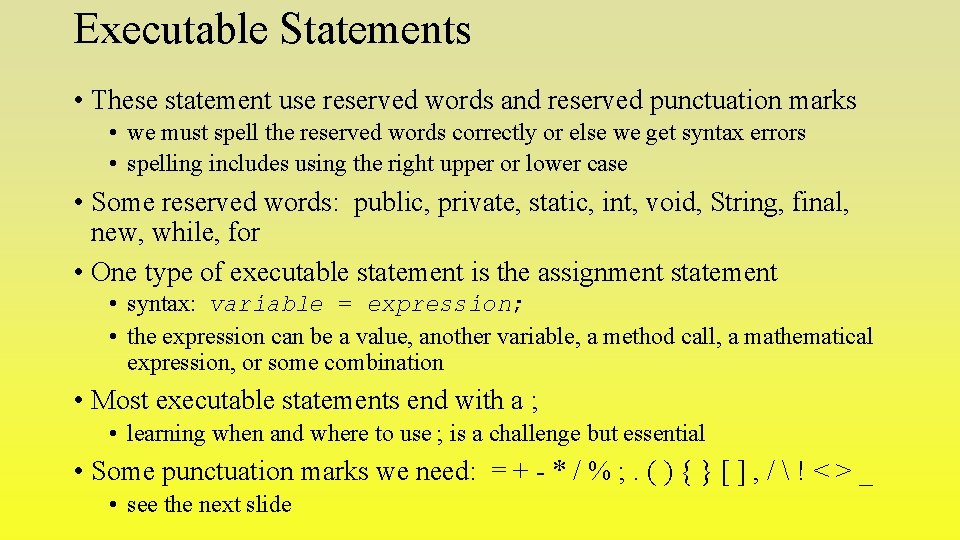
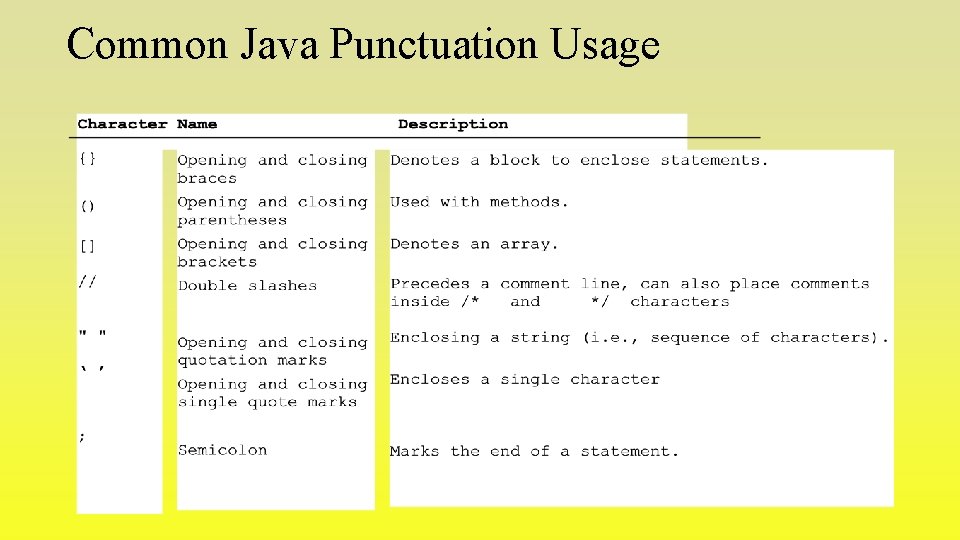
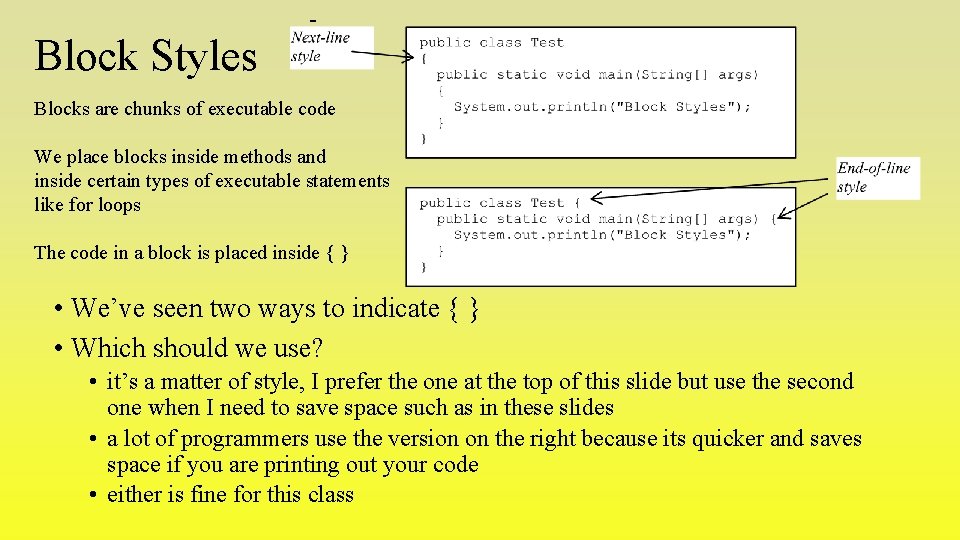
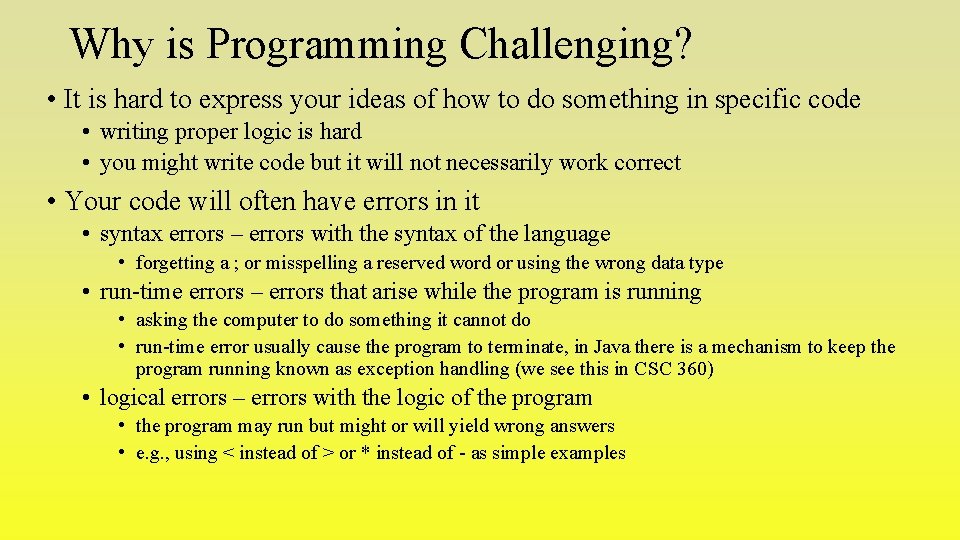
![Locate the Syntax Errors public class An. Error { public static void main(String[] args) Locate the Syntax Errors public class An. Error { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/a747d10b7376634f13e7dbfef36259cc/image-9.jpg)
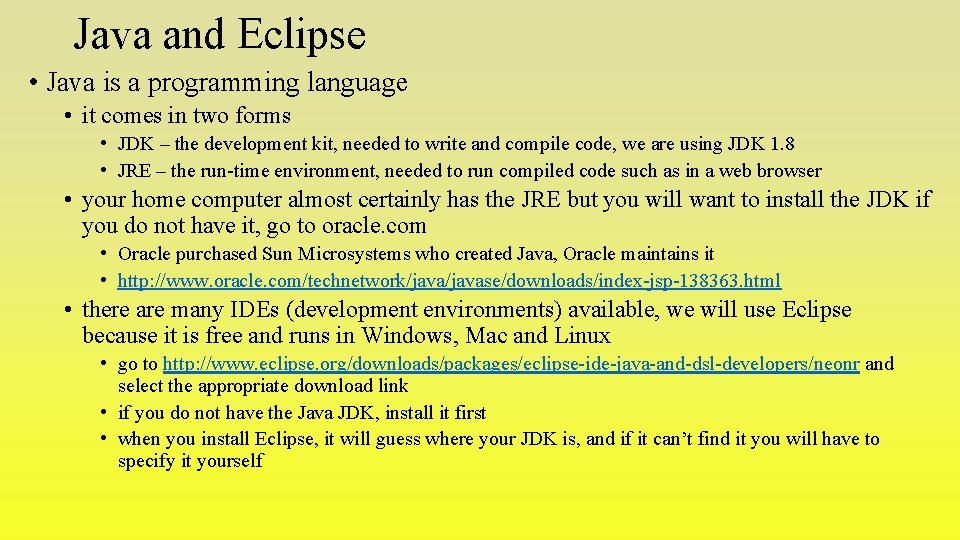
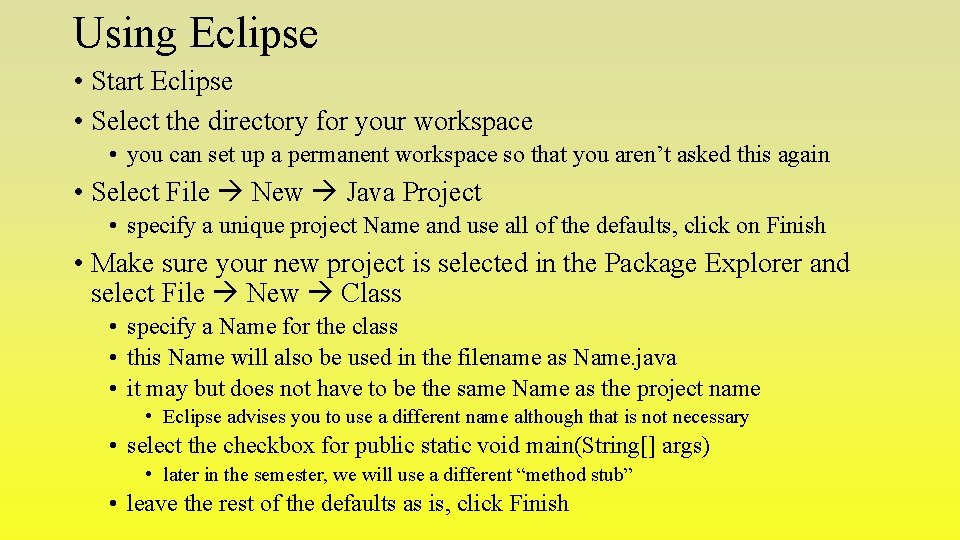
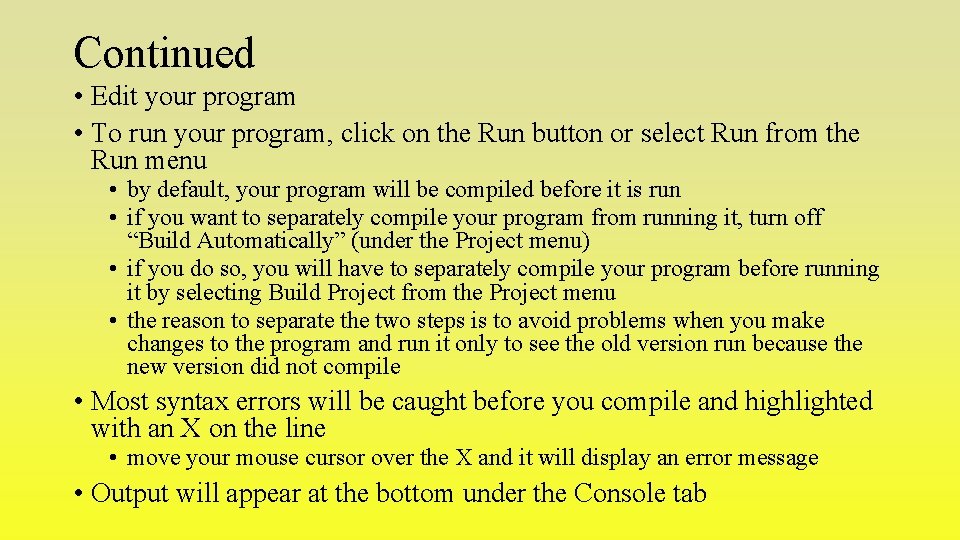
- Slides: 12
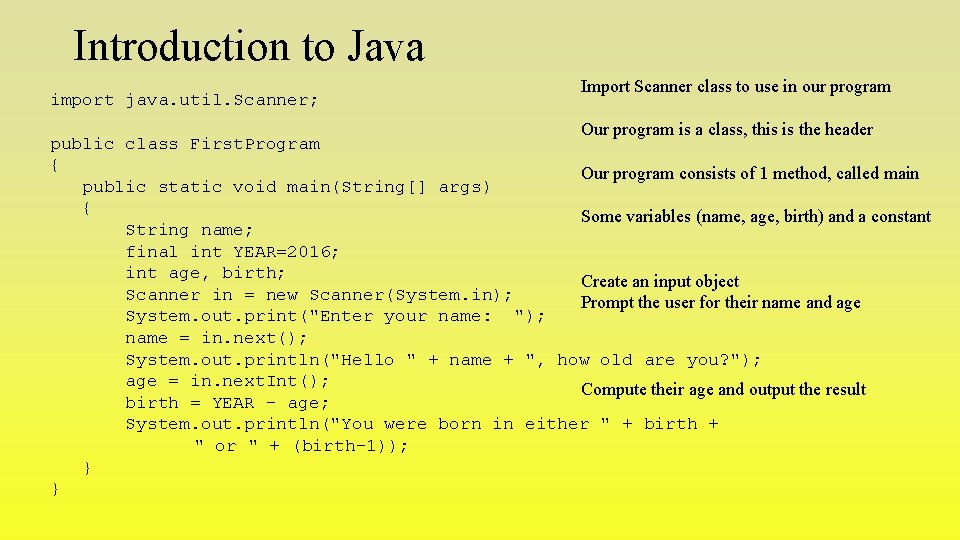
Introduction to Java import java. util. Scanner; Import Scanner class to use in our program Our program is a class, this is the header public class First. Program { Our program consists of 1 method, called main public static void main(String[] args) { Some variables (name, age, birth) and a constant String name; final int YEAR=2016; int age, birth; Create an input object Scanner in = new Scanner(System. in); Prompt the user for their name and age System. out. print("Enter your name: "); name = in. next(); System. out. println("Hello " + name + ", how old are you? "); age = in. next. Int(); Compute their age and output the result birth = YEAR - age; System. out. println("You were born in either " + birth + " or " + (birth-1)); } }
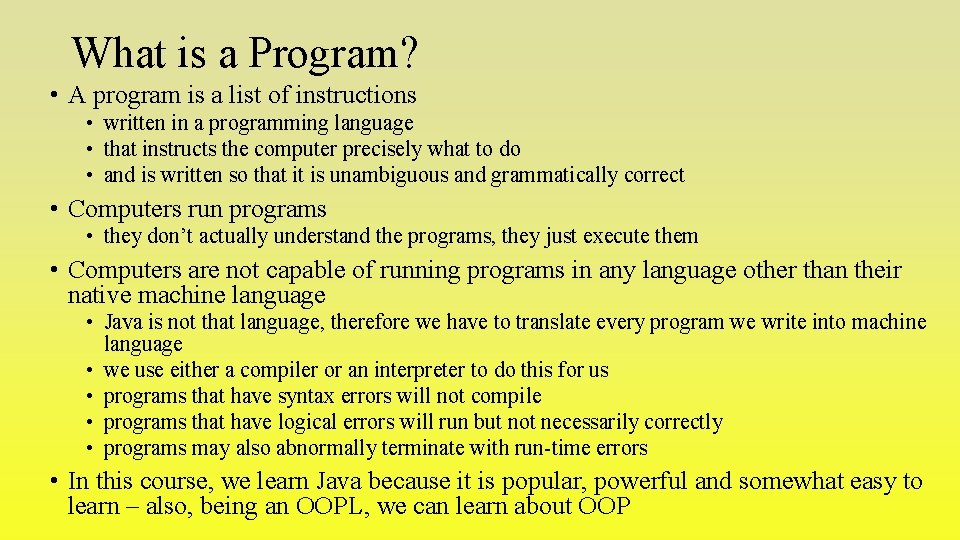
What is a Program? • A program is a list of instructions • written in a programming language • that instructs the computer precisely what to do • and is written so that it is unambiguous and grammatically correct • Computers run programs • they don’t actually understand the programs, they just execute them • Computers are not capable of running programs in any language other than their native machine language • Java is not that language, therefore we have to translate every program we write into machine language • we use either a compiler or an interpreter to do this for us • programs that have syntax errors will not compile • programs that have logical errors will run but not necessarily correctly • programs may also abnormally terminate with run-time errors • In this course, we learn Java because it is popular, powerful and somewhat easy to learn – also, being an OOPL, we can learn about OOP
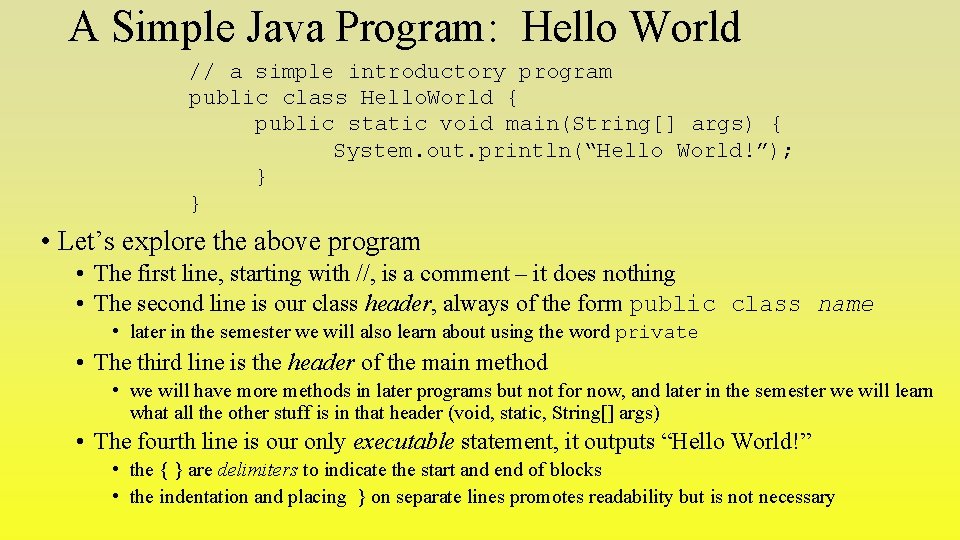
A Simple Java Program: Hello World // a simple introductory program public class Hello. World { public static void main(String[] args) { System. out. println(“Hello World!”); } } • Let’s explore the above program • The first line, starting with //, is a comment – it does nothing • The second line is our class header, always of the form public class name • later in the semester we will also learn about using the word private • The third line is the header of the main method • we will have more methods in later programs but not for now, and later in the semester we will learn what all the other stuff is in that header (void, static, String[] args) • The fourth line is our only executable statement, it outputs “Hello World!” • the { } are delimiters to indicate the start and end of blocks • the indentation and placing } on separate lines promotes readability but is not necessary
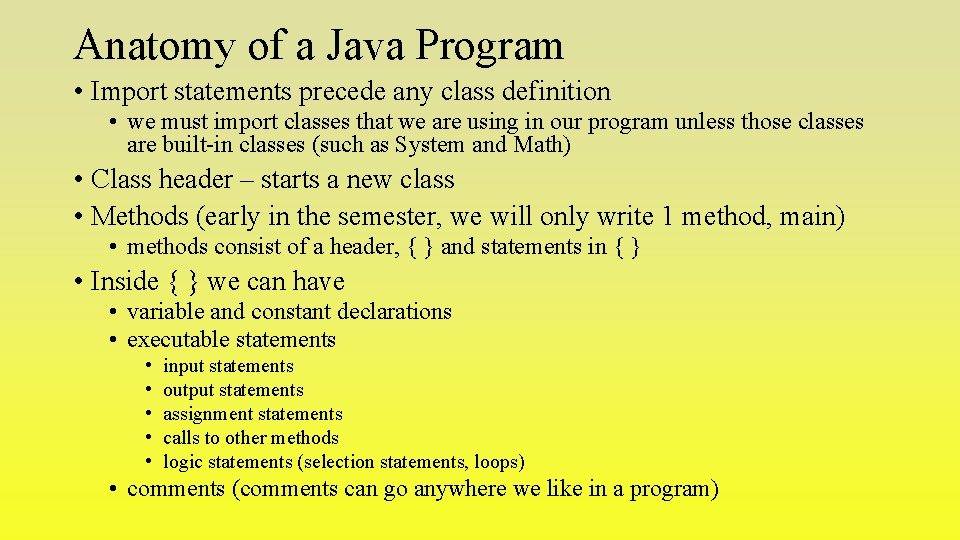
Anatomy of a Java Program • Import statements precede any class definition • we must import classes that we are using in our program unless those classes are built-in classes (such as System and Math) • Class header – starts a new class • Methods (early in the semester, we will only write 1 method, main) • methods consist of a header, { } and statements in { } • Inside { } we can have • variable and constant declarations • executable statements • • • input statements output statements assignment statements calls to other methods logic statements (selection statements, loops) • comments (comments can go anywhere we like in a program)
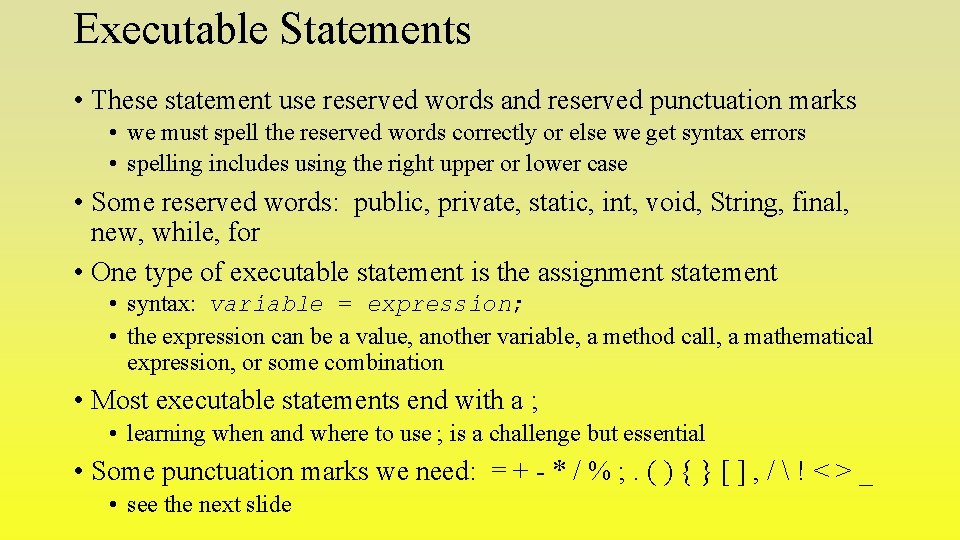
Executable Statements • These statement use reserved words and reserved punctuation marks • we must spell the reserved words correctly or else we get syntax errors • spelling includes using the right upper or lower case • Some reserved words: public, private, static, int, void, String, final, new, while, for • One type of executable statement is the assignment statement • syntax: variable = expression; • the expression can be a value, another variable, a method call, a mathematical expression, or some combination • Most executable statements end with a ; • learning when and where to use ; is a challenge but essential • Some punctuation marks we need: = + - * / % ; . ( ) { } [ ] , / ! < > _ • see the next slide
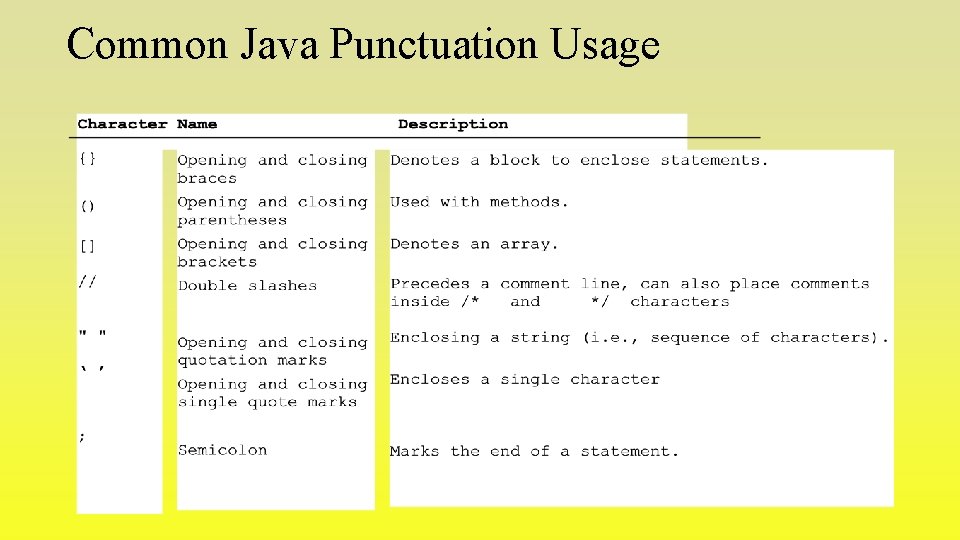
Common Java Punctuation Usage
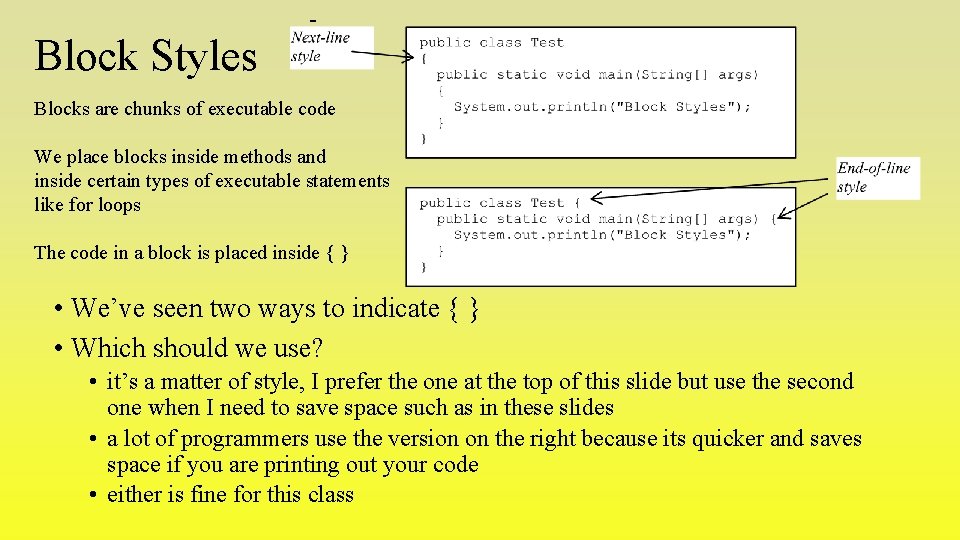
Block Styles Blocks are chunks of executable code We place blocks inside methods and inside certain types of executable statements like for loops The code in a block is placed inside { } • We’ve seen two ways to indicate { } • Which should we use? • it’s a matter of style, I prefer the one at the top of this slide but use the second one when I need to save space such as in these slides • a lot of programmers use the version on the right because its quicker and saves space if you are printing out your code • either is fine for this class
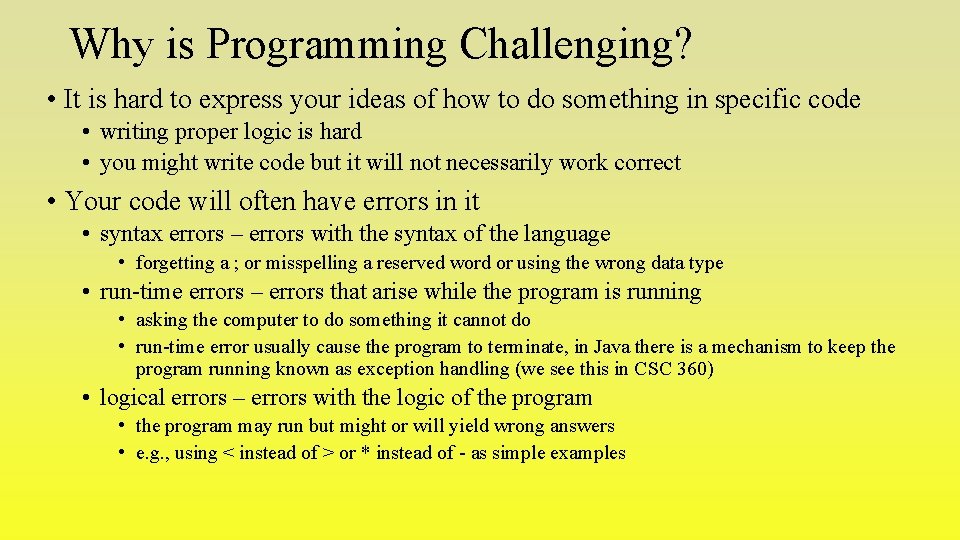
Why is Programming Challenging? • It is hard to express your ideas of how to do something in specific code • writing proper logic is hard • you might write code but it will not necessarily work correct • Your code will often have errors in it • syntax errors – errors with the syntax of the language • forgetting a ; or misspelling a reserved word or using the wrong data type • run-time errors – errors that arise while the program is running • asking the computer to do something it cannot do • run-time error usually cause the program to terminate, in Java there is a mechanism to keep the program running known as exception handling (we see this in CSC 360) • logical errors – errors with the logic of the program • the program may run but might or will yield wrong answers • e. g. , using < instead of > or * instead of - as simple examples
![Locate the Syntax Errors public class An Error public static void mainString args Locate the Syntax Errors public class An. Error { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/a747d10b7376634f13e7dbfef36259cc/image-9.jpg)
Locate the Syntax Errors public class An. Error { public static void main(String[] args) { System. out. println(“Hello world); } } public class An. Error 2 { public static void main(String[] foo) System. out. println(“Hello world”); } } private class An. Error 3 { public static void main(String[] args) { System. out. println(“Hello world”) } }
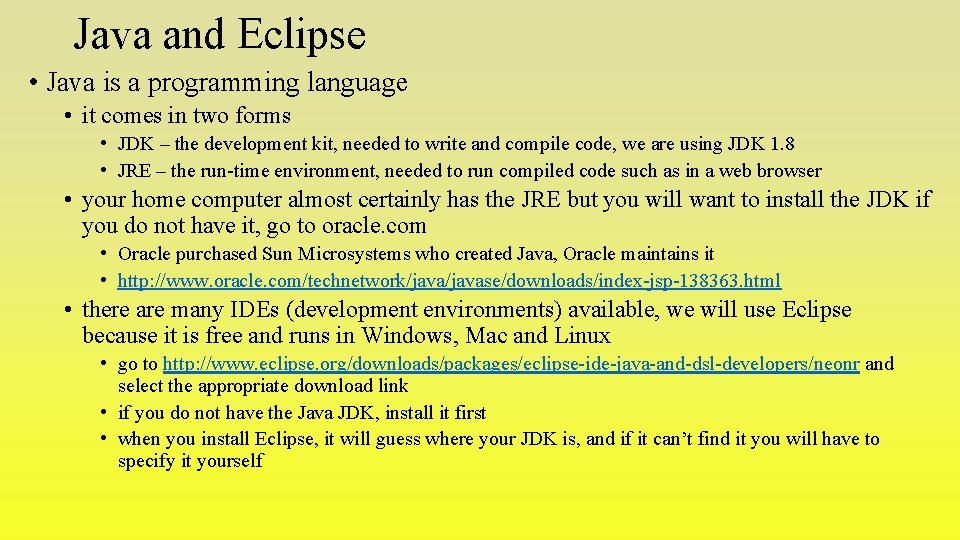
Java and Eclipse • Java is a programming language • it comes in two forms • JDK – the development kit, needed to write and compile code, we are using JDK 1. 8 • JRE – the run-time environment, needed to run compiled code such as in a web browser • your home computer almost certainly has the JRE but you will want to install the JDK if you do not have it, go to oracle. com • Oracle purchased Sun Microsystems who created Java, Oracle maintains it • http: //www. oracle. com/technetwork/javase/downloads/index-jsp-138363. html • there are many IDEs (development environments) available, we will use Eclipse because it is free and runs in Windows, Mac and Linux • go to http: //www. eclipse. org/downloads/packages/eclipse-ide-java-and-dsl-developers/neonr and select the appropriate download link • if you do not have the Java JDK, install it first • when you install Eclipse, it will guess where your JDK is, and if it can’t find it you will have to specify it yourself
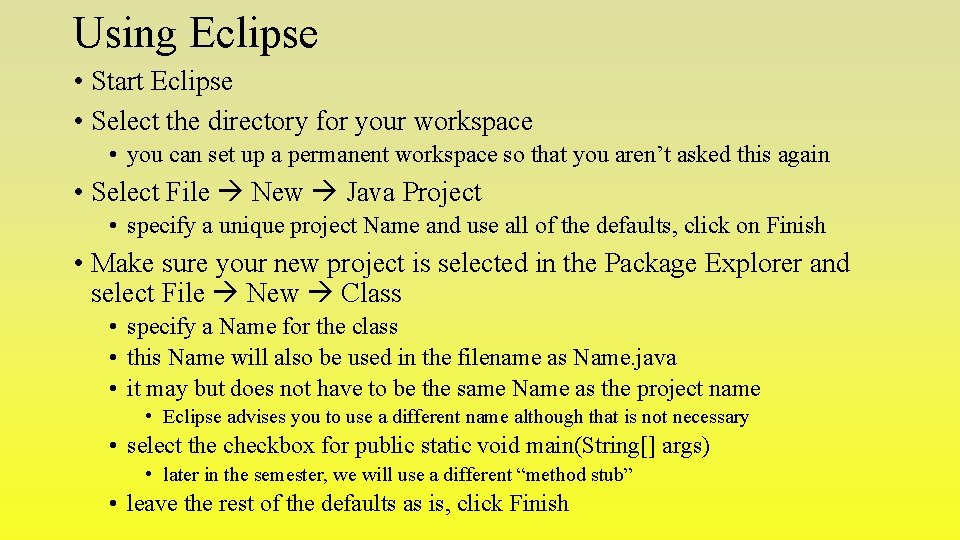
Using Eclipse • Start Eclipse • Select the directory for your workspace • you can set up a permanent workspace so that you aren’t asked this again • Select File New Java Project • specify a unique project Name and use all of the defaults, click on Finish • Make sure your new project is selected in the Package Explorer and select File New Class • specify a Name for the class • this Name will also be used in the filename as Name. java • it may but does not have to be the same Name as the project name • Eclipse advises you to use a different name although that is not necessary • select the checkbox for public static void main(String[] args) • later in the semester, we will use a different “method stub” • leave the rest of the defaults as is, click Finish
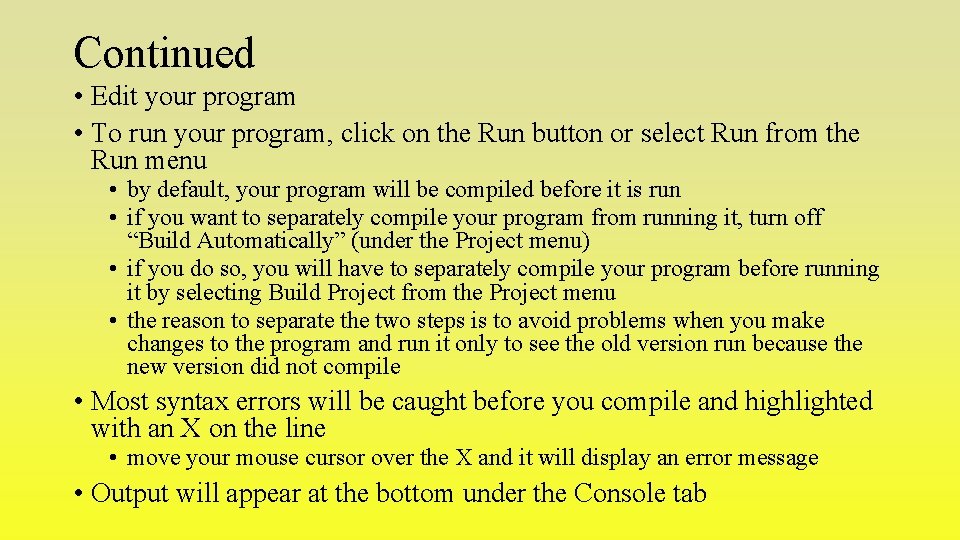
Continued • Edit your program • To run your program, click on the Run button or select Run from the Run menu • by default, your program will be compiled before it is run • if you want to separately compile your program from running it, turn off “Build Automatically” (under the Project menu) • if you do so, you will have to separately compile your program before running it by selecting Build Project from the Project menu • the reason to separate the two steps is to avoid problems when you make changes to the program and run it only to see the old version run because the new version did not compile • Most syntax errors will be caught before you compile and highlighted with an X on the line • move your mouse cursor over the X and it will display an error message • Output will appear at the bottom under the Console tab