Java and OOP Part 3 Extending classes OOP
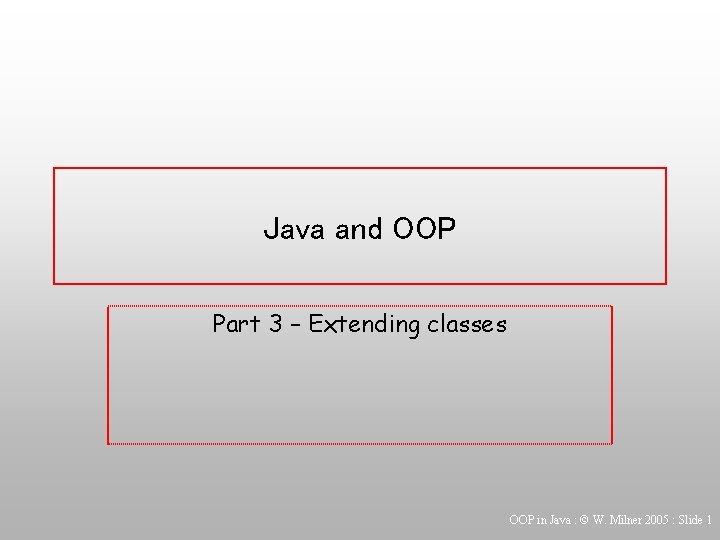
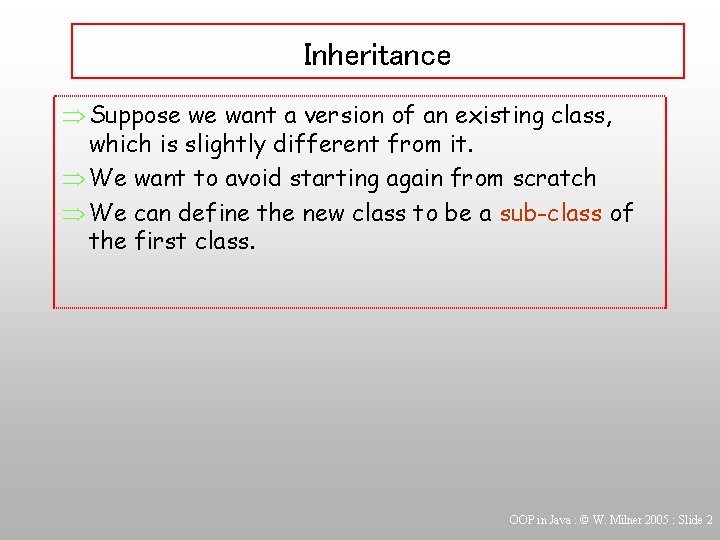
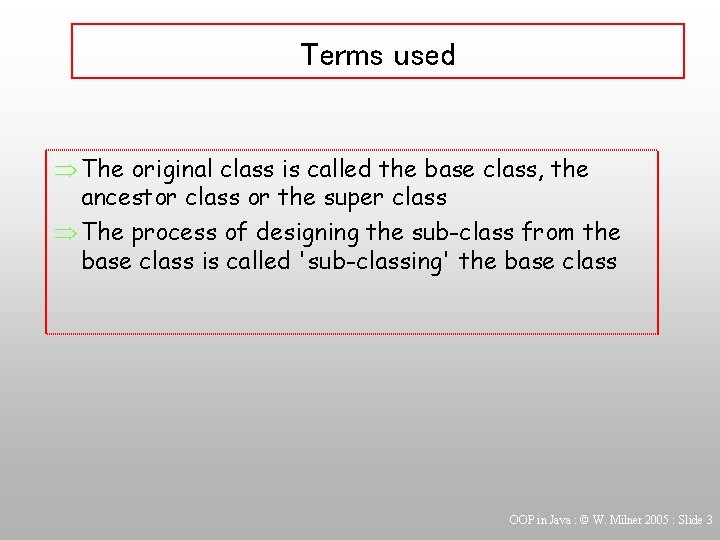
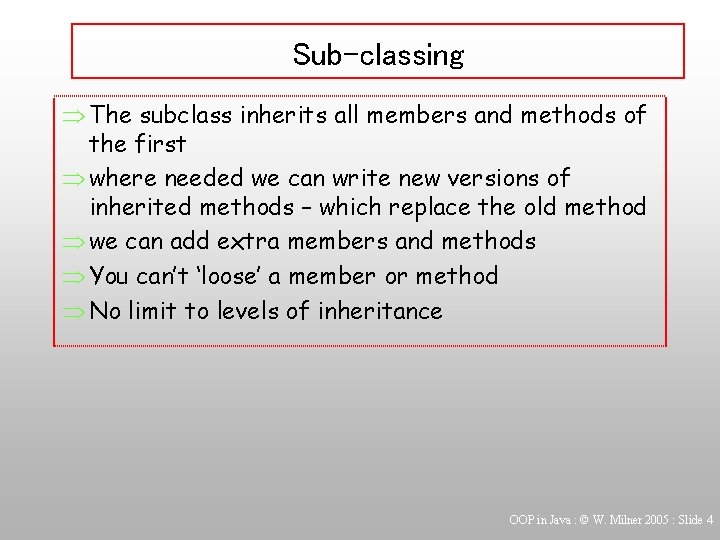
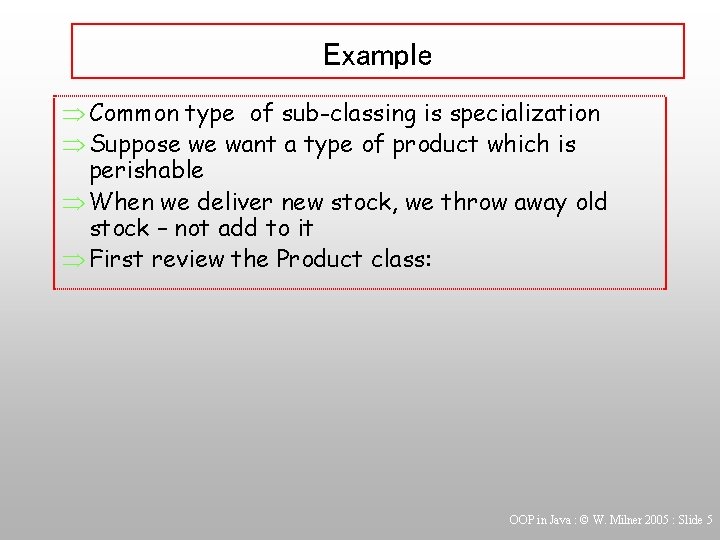
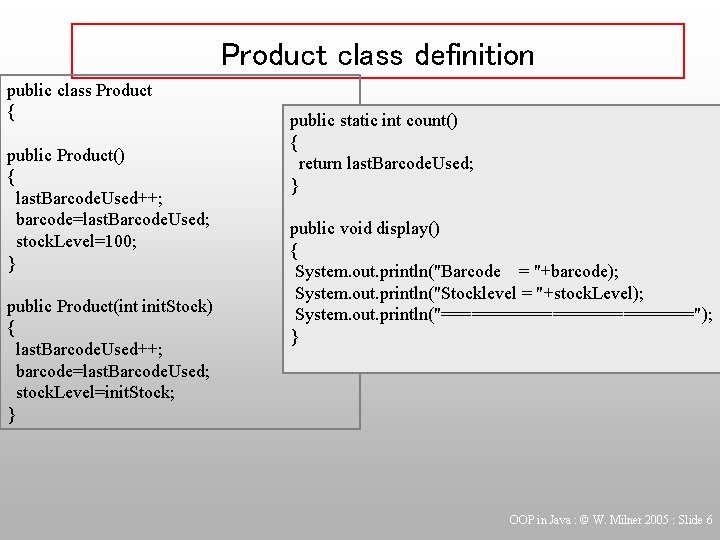
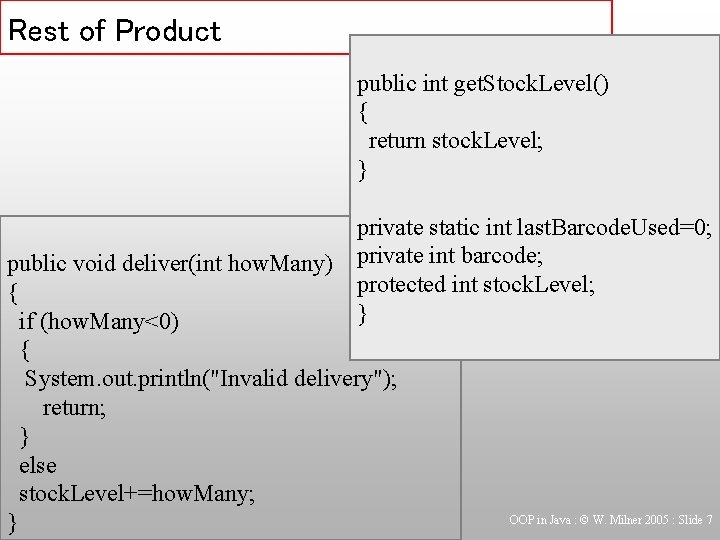
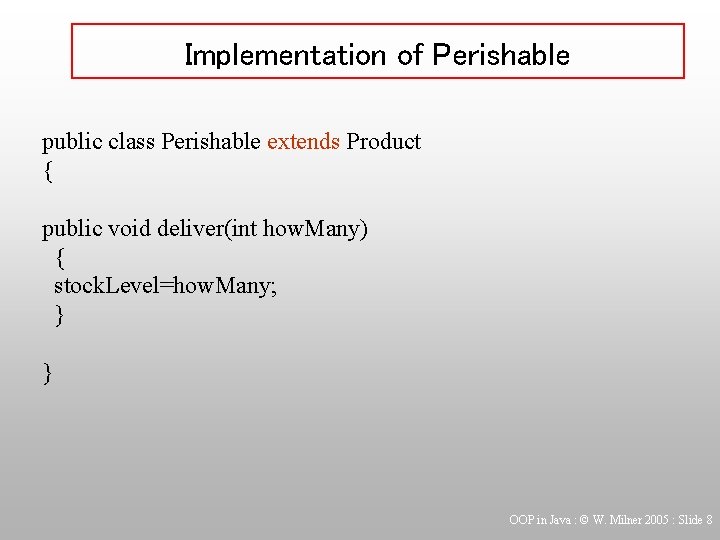
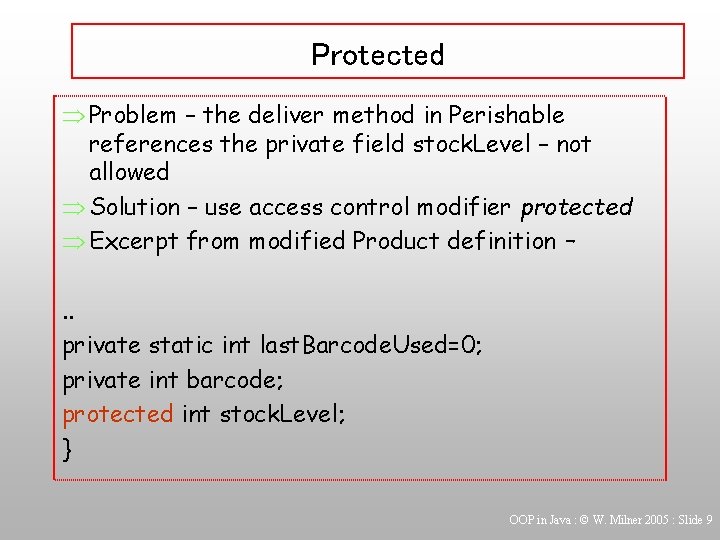
![public class First { public static void main(String[] args) { Product prod = new public class First { public static void main(String[] args) { Product prod = new](https://slidetodoc.com/presentation_image/7de7a26068aff99123eb3989a40381dc/image-10.jpg)
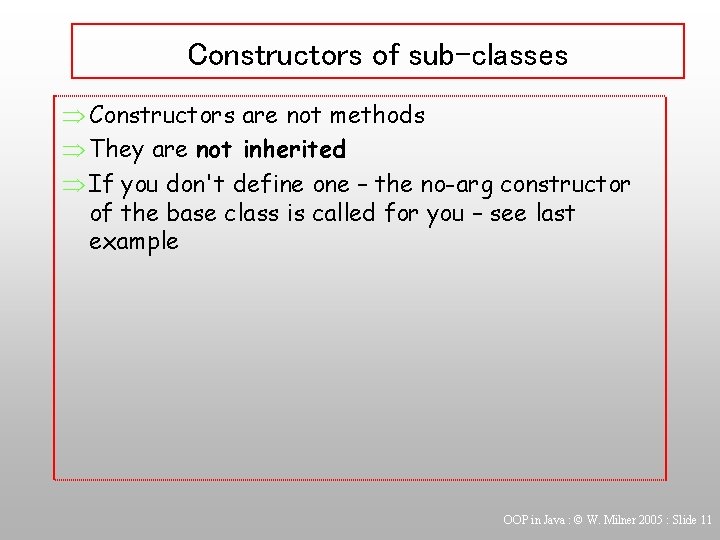
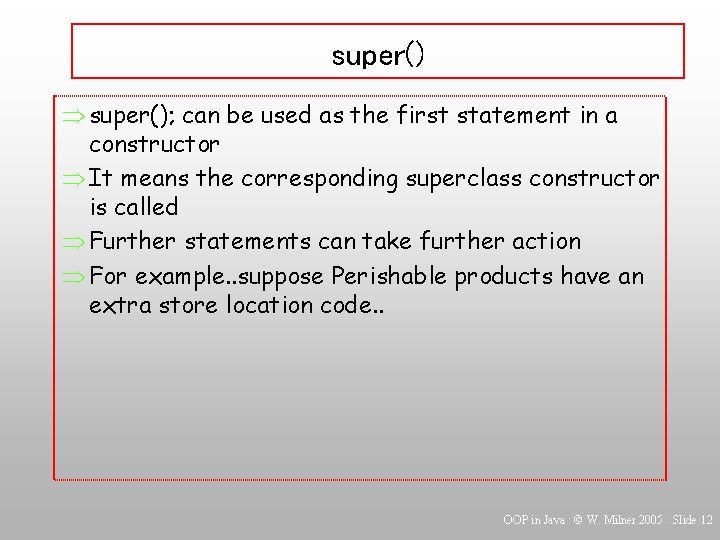
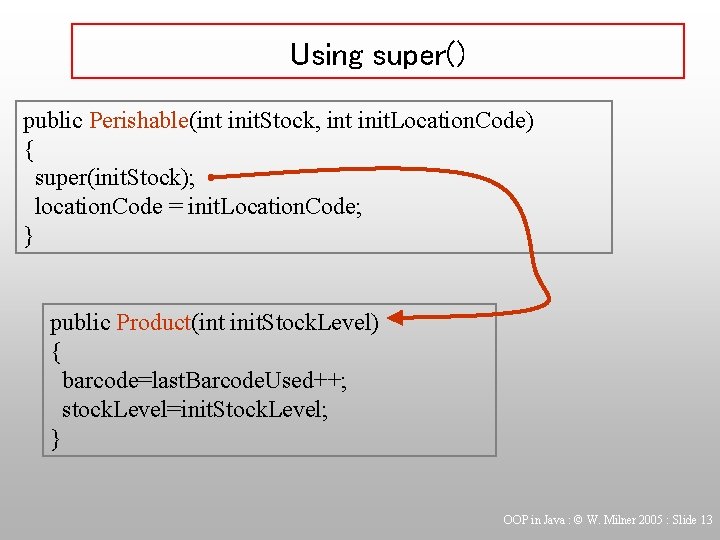
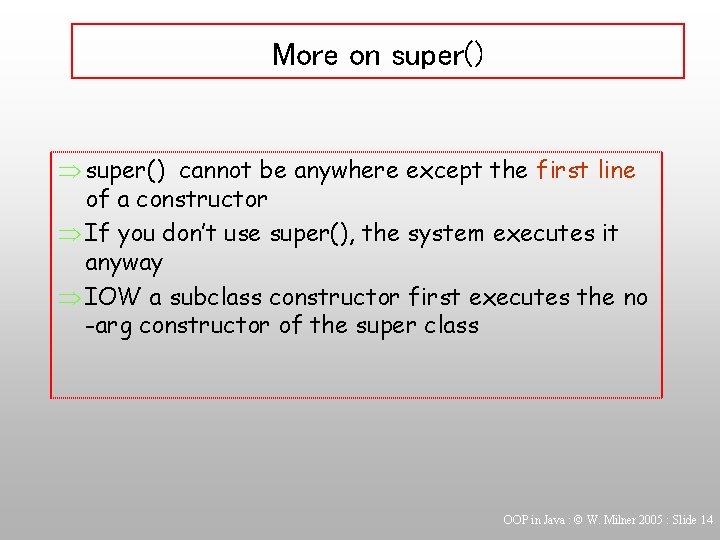
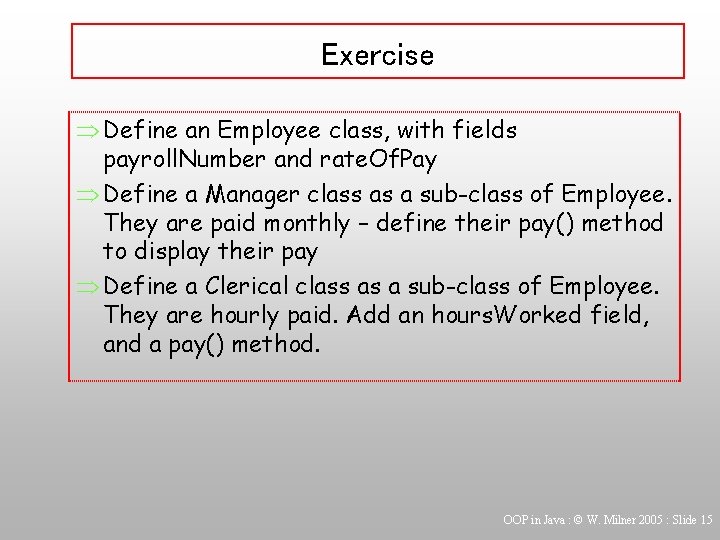
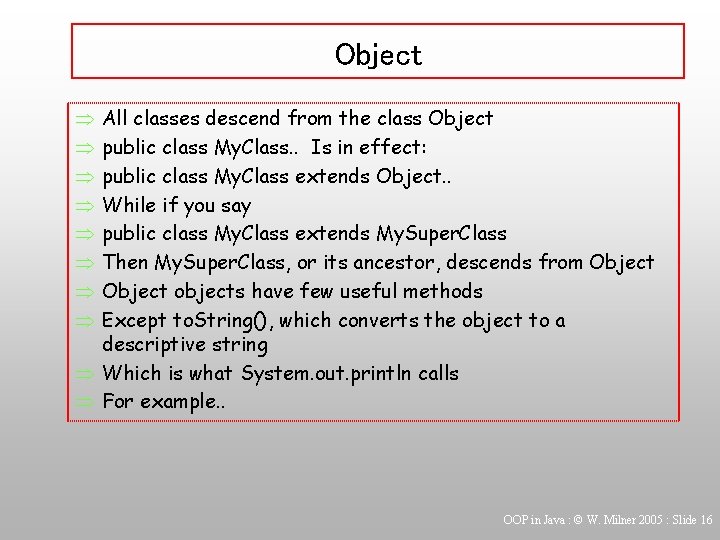
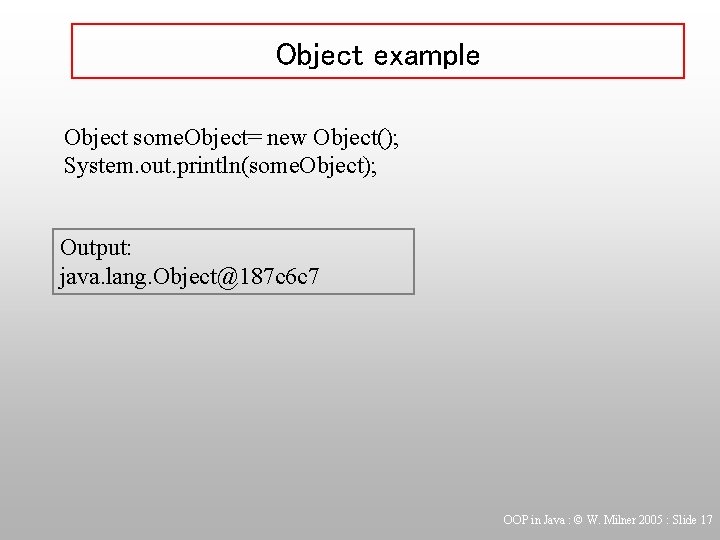
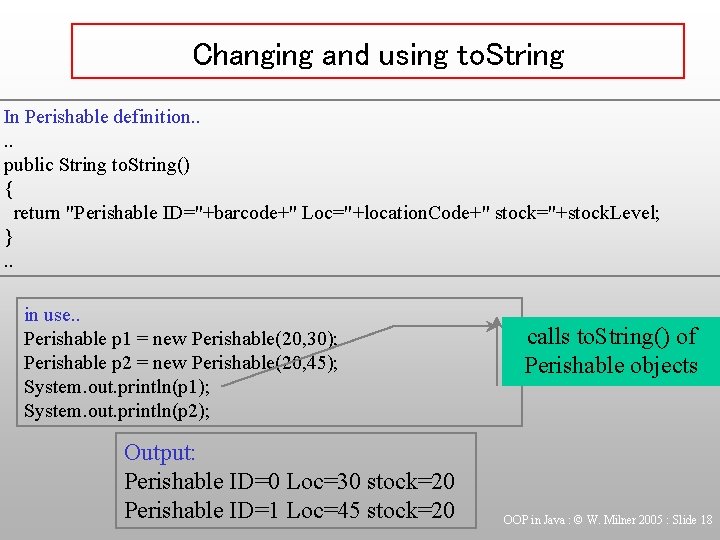
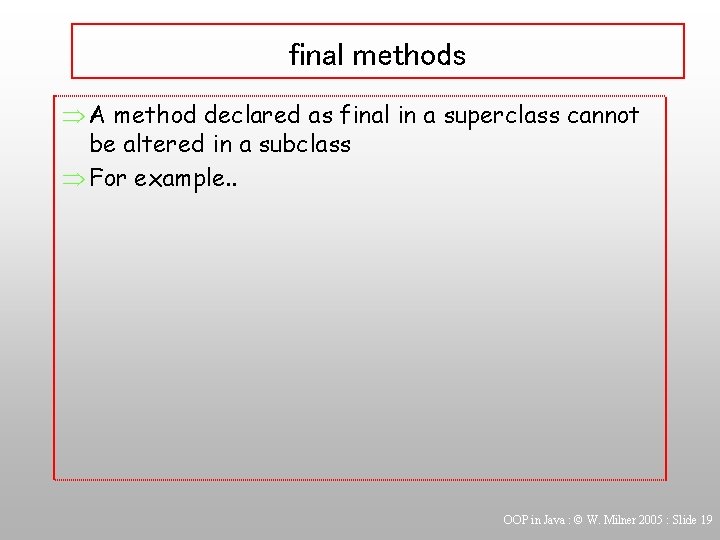
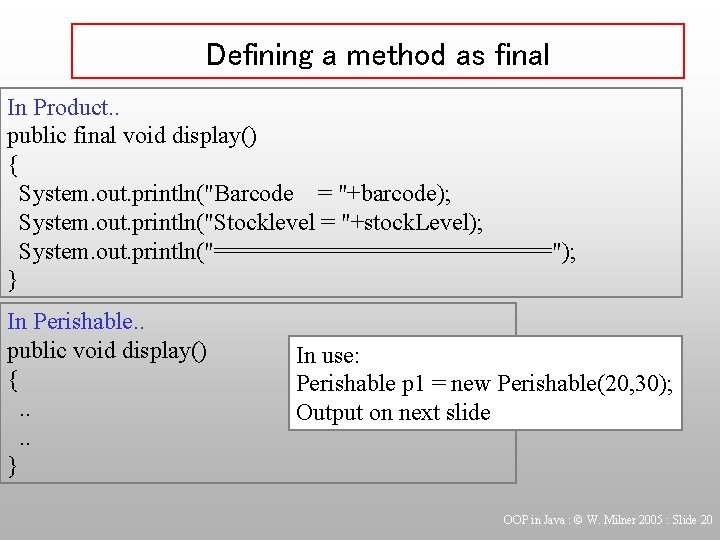
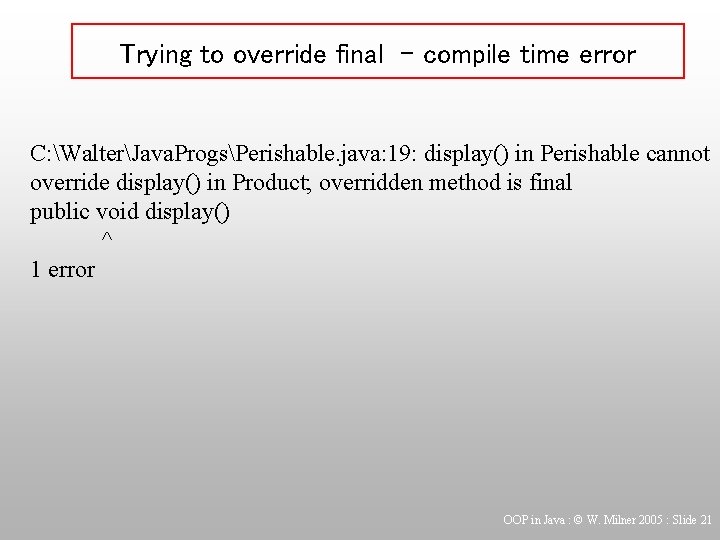
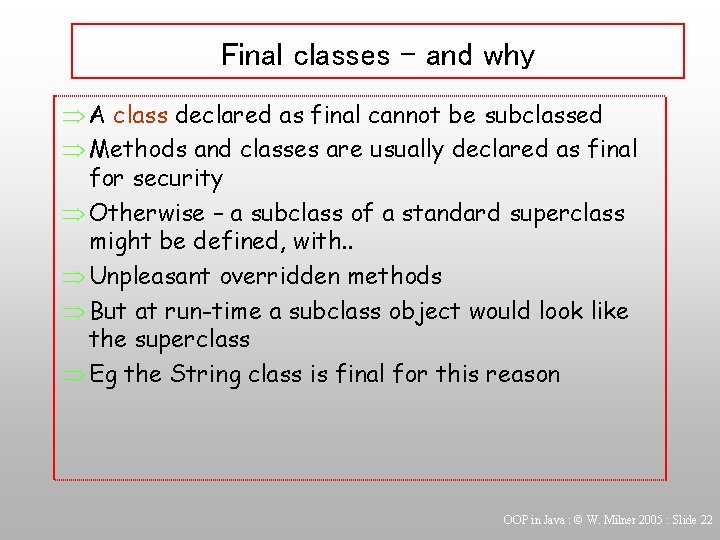
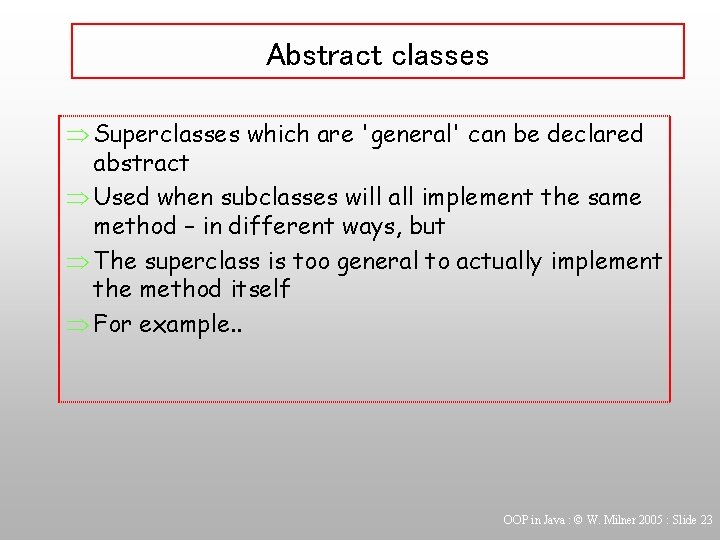
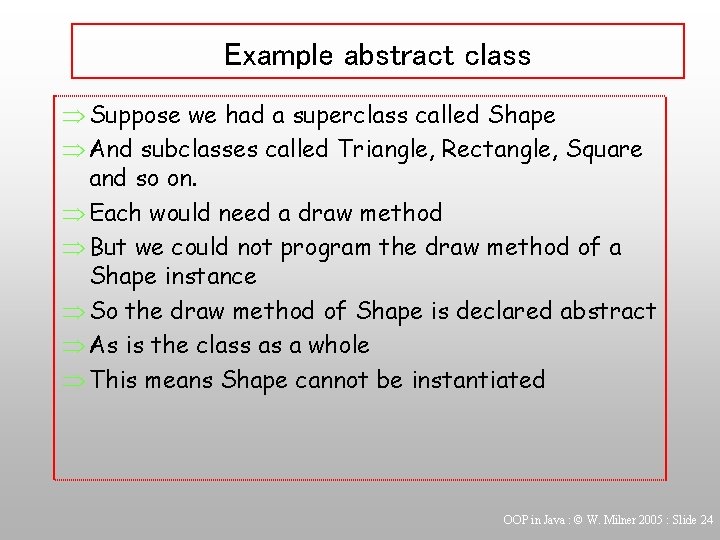
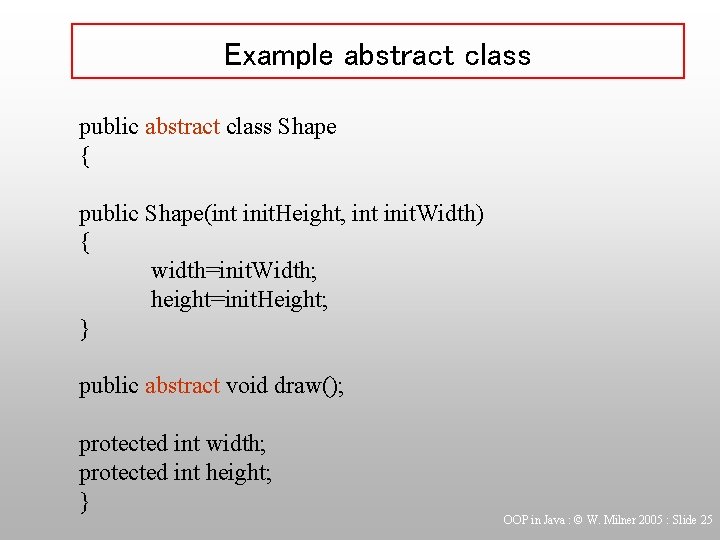
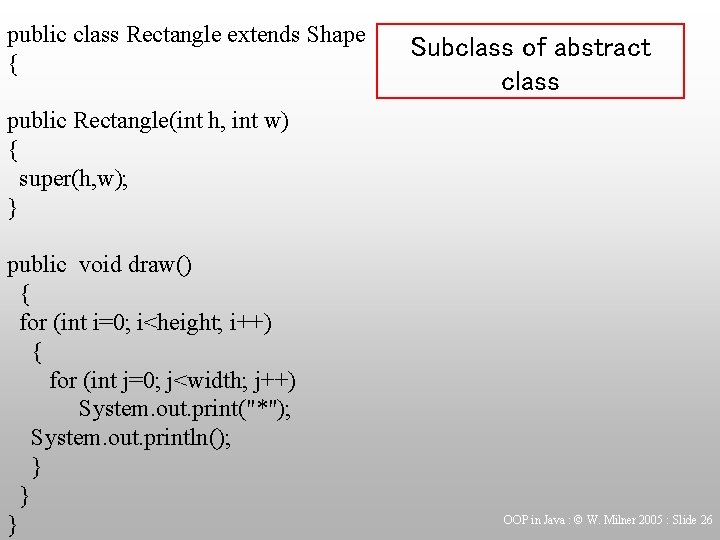
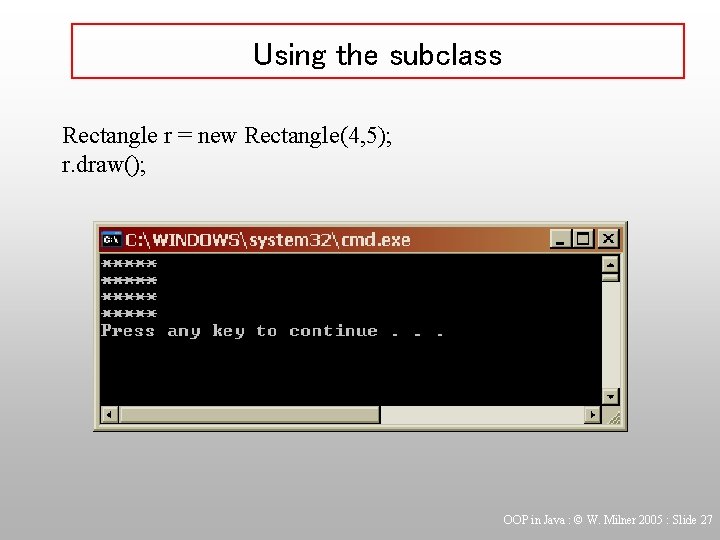
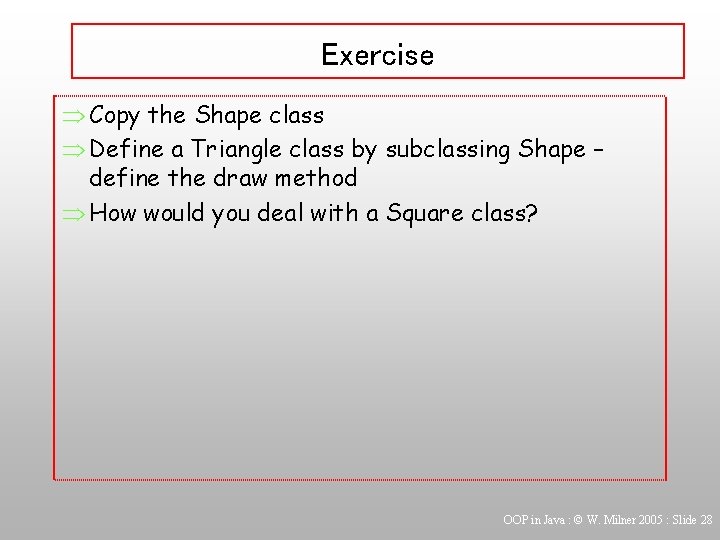
- Slides: 28
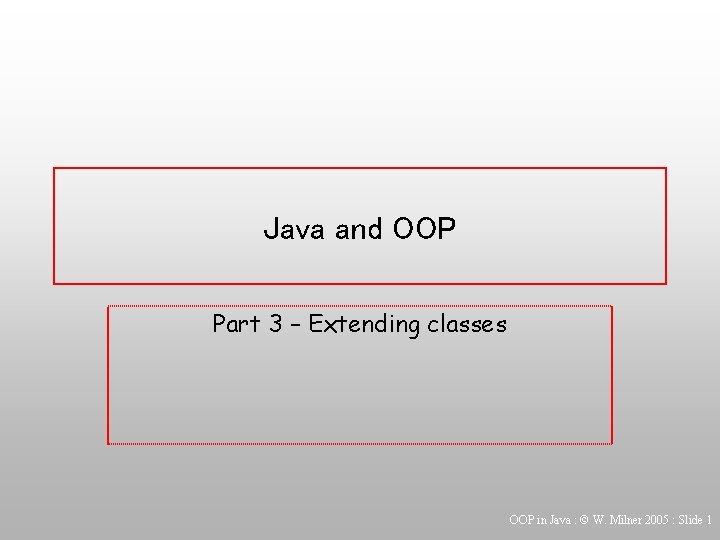
Java and OOP Part 3 – Extending classes OOP in Java : © W. Milner 2005 : Slide 1
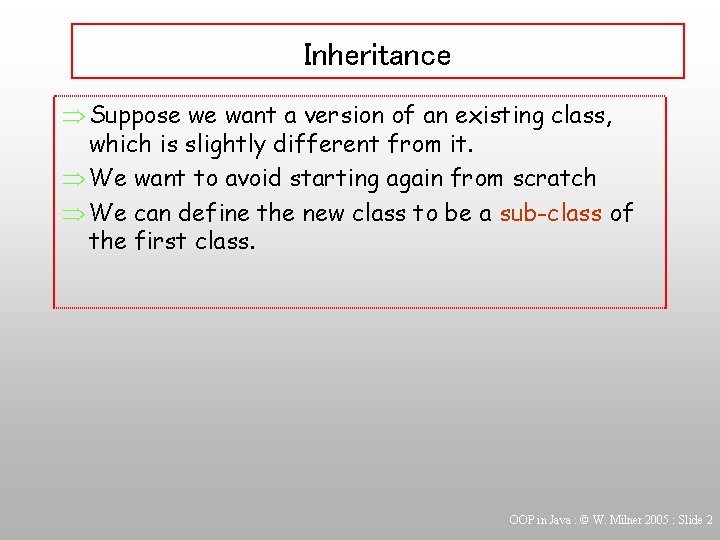
Inheritance Þ Suppose we want a version of an existing class, which is slightly different from it. Þ We want to avoid starting again from scratch Þ We can define the new class to be a sub-class of the first class. OOP in Java : © W. Milner 2005 : Slide 2
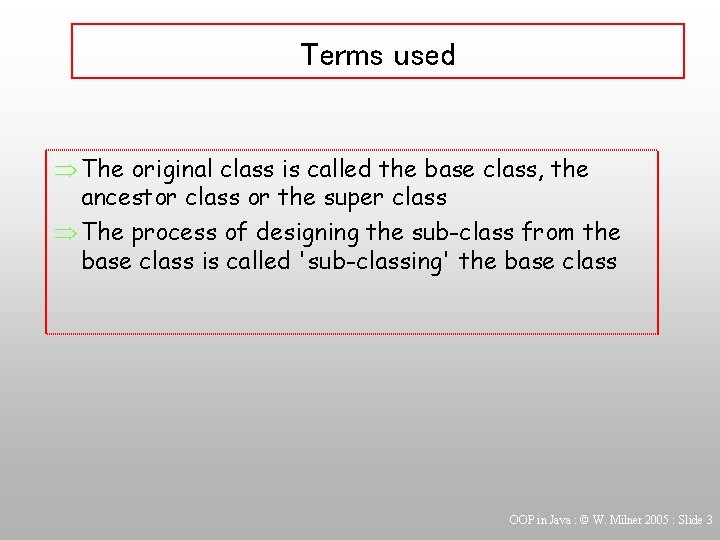
Terms used Þ The original class is called the base class, the ancestor class or the super class Þ The process of designing the sub-class from the base class is called 'sub-classing' the base class OOP in Java : © W. Milner 2005 : Slide 3
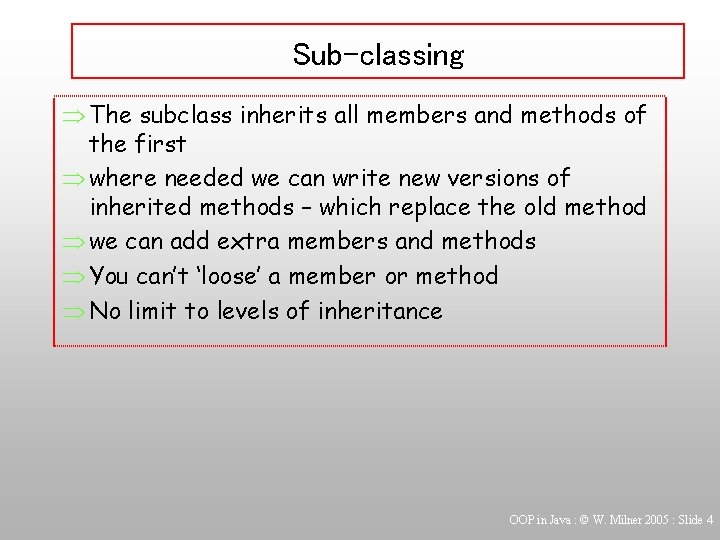
Sub-classing Þ The subclass inherits all members and methods of the first Þ where needed we can write new versions of inherited methods – which replace the old method Þ we can add extra members and methods Þ You can’t ‘loose’ a member or method Þ No limit to levels of inheritance OOP in Java : © W. Milner 2005 : Slide 4
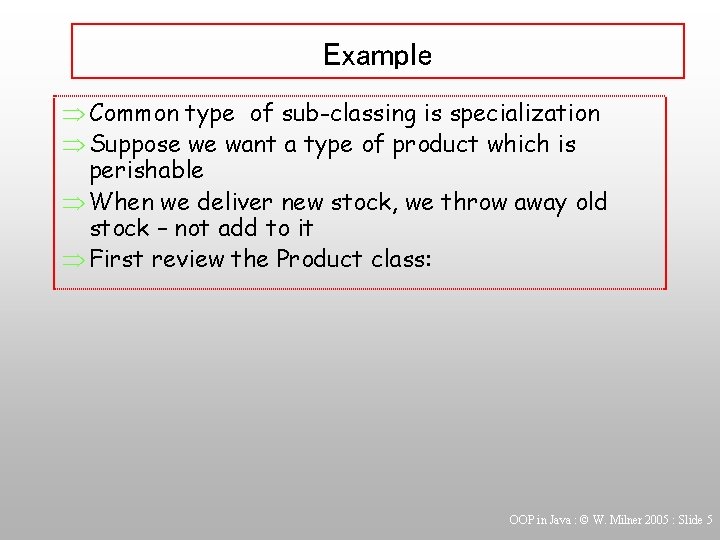
Example Þ Common type of sub-classing is specialization Þ Suppose we want a type of product which is perishable Þ When we deliver new stock, we throw away old stock – not add to it Þ First review the Product class: OOP in Java : © W. Milner 2005 : Slide 5
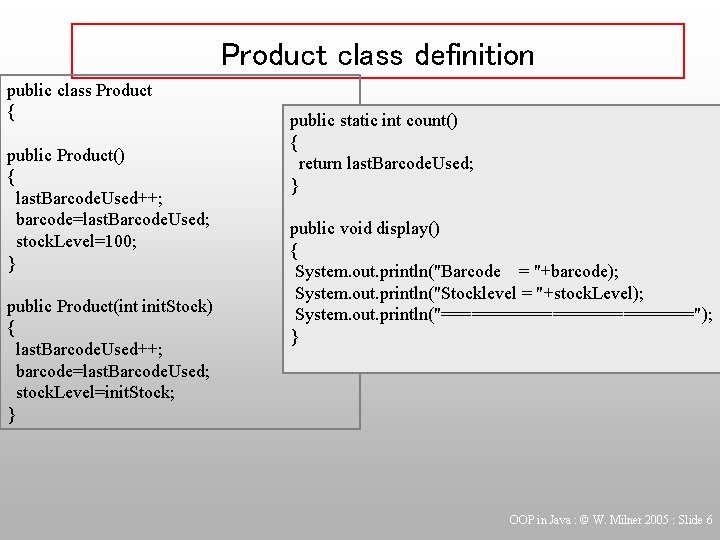
Product class definition public class Product { public Product() { last. Barcode. Used++; barcode=last. Barcode. Used; stock. Level=100; } public Product(int init. Stock) { last. Barcode. Used++; barcode=last. Barcode. Used; stock. Level=init. Stock; } public static int count() { return last. Barcode. Used; } public void display() { System. out. println("Barcode = "+barcode); System. out. println("Stocklevel = "+stock. Level); System. out. println("============="); } OOP in Java : © W. Milner 2005 : Slide 6
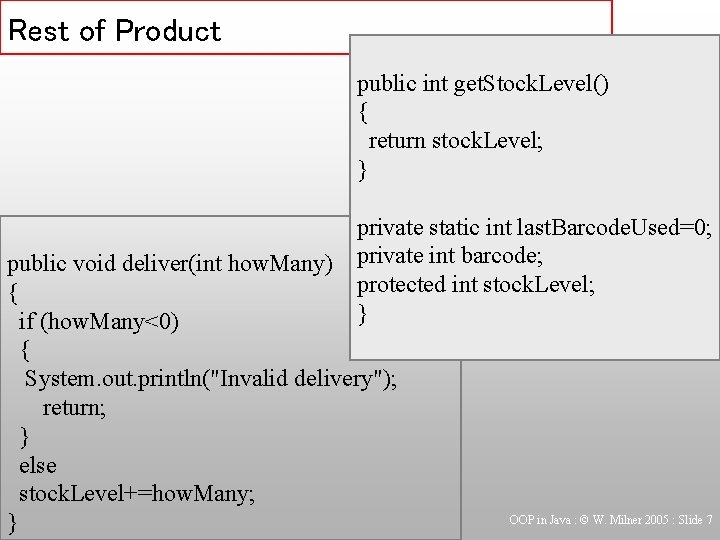
Rest of Product public int get. Stock. Level() { return stock. Level; } private static int last. Barcode. Used=0; public void deliver(int how. Many) private int barcode; protected int stock. Level; { } if (how. Many<0) { System. out. println("Invalid delivery"); return; } else stock. Level+=how. Many; } OOP in Java : © W. Milner 2005 : Slide 7
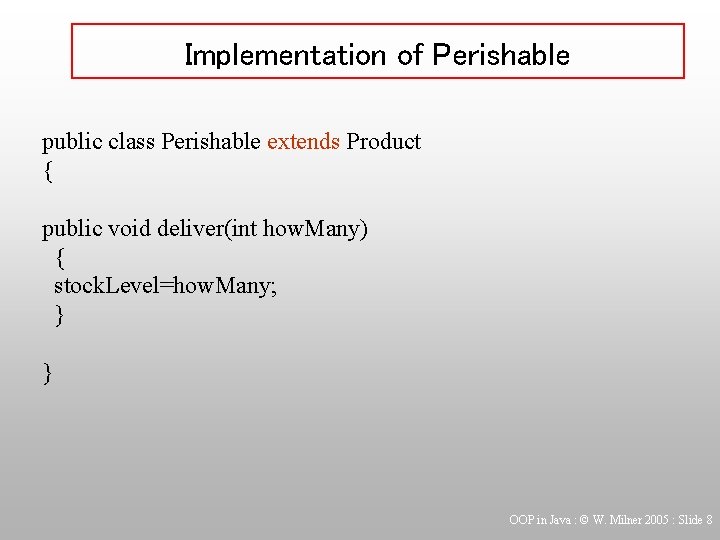
Implementation of Perishable public class Perishable extends Product { public void deliver(int how. Many) { stock. Level=how. Many; } } OOP in Java : © W. Milner 2005 : Slide 8
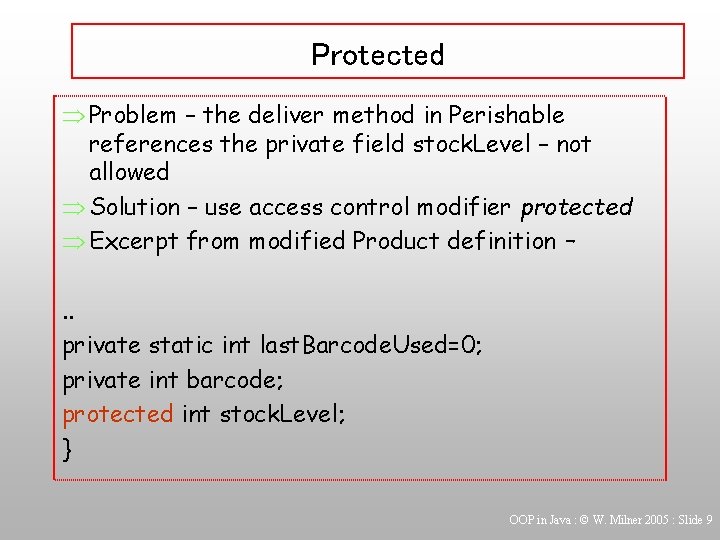
Protected Þ Problem – the deliver method in Perishable references the private field stock. Level – not allowed Þ Solution – use access control modifier protected Þ Excerpt from modified Product definition –. . private static int last. Barcode. Used=0; private int barcode; protected int stock. Level; } OOP in Java : © W. Milner 2005 : Slide 9
![public class First public static void mainString args Product prod new public class First { public static void main(String[] args) { Product prod = new](https://slidetodoc.com/presentation_image/7de7a26068aff99123eb3989a40381dc/image-10.jpg)
public class First { public static void main(String[] args) { Product prod = new Product(); prod. deliver(100); Perishable perish 1 = new Perishable(); Perishable perish 2 = new Perishable(); perish 1. deliver(50); perish 2. deliver(60); prod. display(); perish 1. display(); perish 2. display(); } } Using the subclass All 3 use default constructor =stocklevel 100 Barcode = 1 Stocklevel = 200 ========== Barcode = 2 Stocklevel = 50 ========== Barcode = 3 Stocklevel = 60 ========== OOP in Java : © W. Milner 2005 : Slide 10
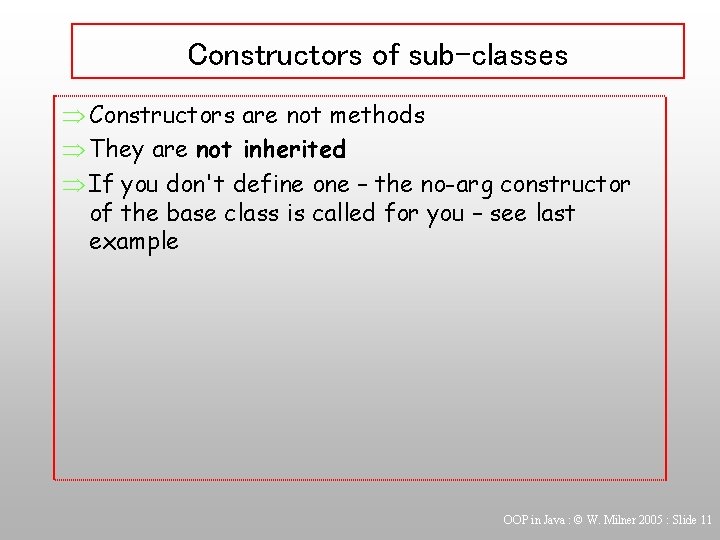
Constructors of sub-classes Þ Constructors are not methods Þ They are not inherited Þ If you don't define one – the no-arg constructor of the base class is called for you – see last example OOP in Java : © W. Milner 2005 : Slide 11
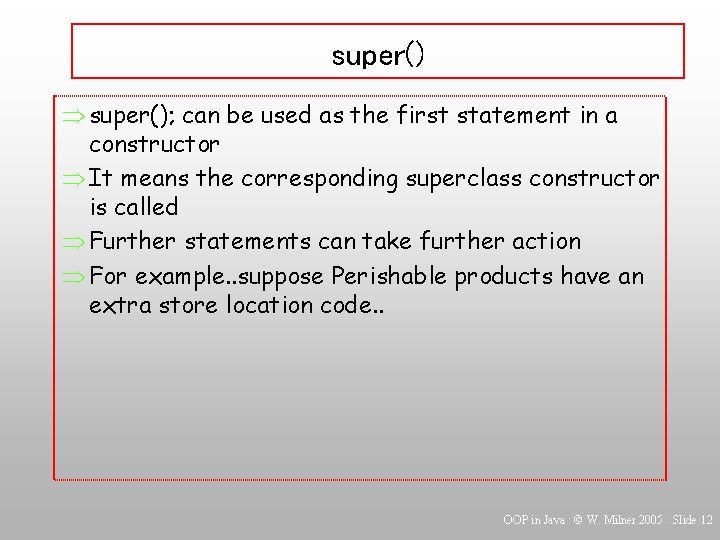
super() Þ super(); can be used as the first statement in a constructor Þ It means the corresponding superclass constructor is called Þ Further statements can take further action Þ For example. . suppose Perishable products have an extra store location code. . OOP in Java : © W. Milner 2005 : Slide 12
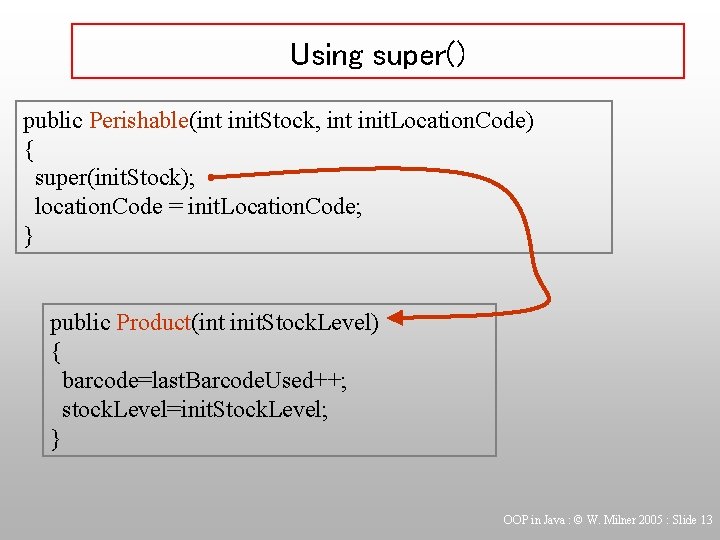
Using super() public Perishable(int init. Stock, int init. Location. Code) { super(init. Stock); location. Code = init. Location. Code; } public Product(int init. Stock. Level) { barcode=last. Barcode. Used++; stock. Level=init. Stock. Level; } OOP in Java : © W. Milner 2005 : Slide 13
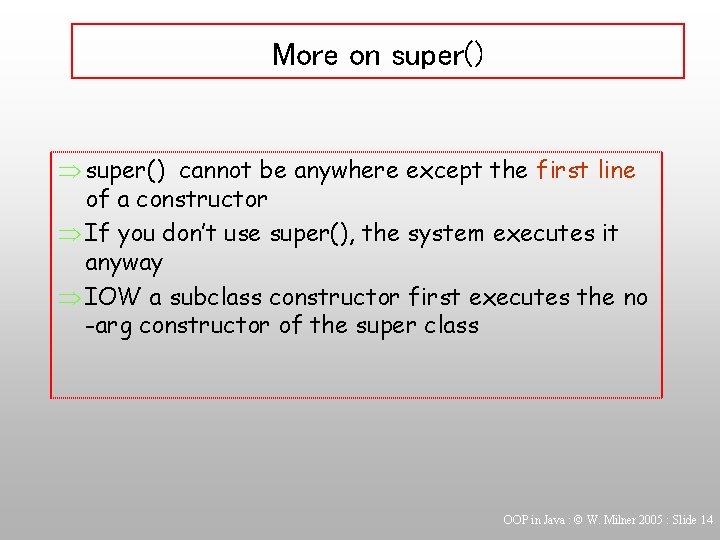
More on super() Þ super() cannot be anywhere except the first line of a constructor Þ If you don’t use super(), the system executes it anyway Þ IOW a subclass constructor first executes the no -arg constructor of the super class OOP in Java : © W. Milner 2005 : Slide 14
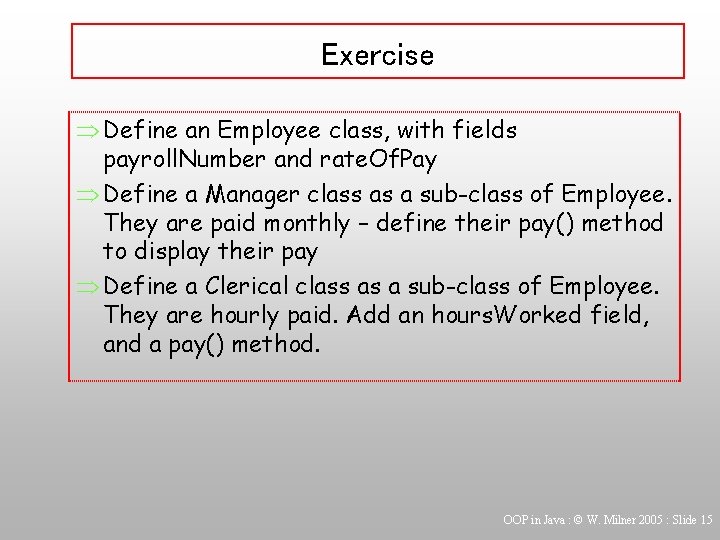
Exercise Þ Define an Employee class, with fields payroll. Number and rate. Of. Pay Þ Define a Manager class as a sub-class of Employee. They are paid monthly – define their pay() method to display their pay Þ Define a Clerical class as a sub-class of Employee. They are hourly paid. Add an hours. Worked field, and a pay() method. OOP in Java : © W. Milner 2005 : Slide 15
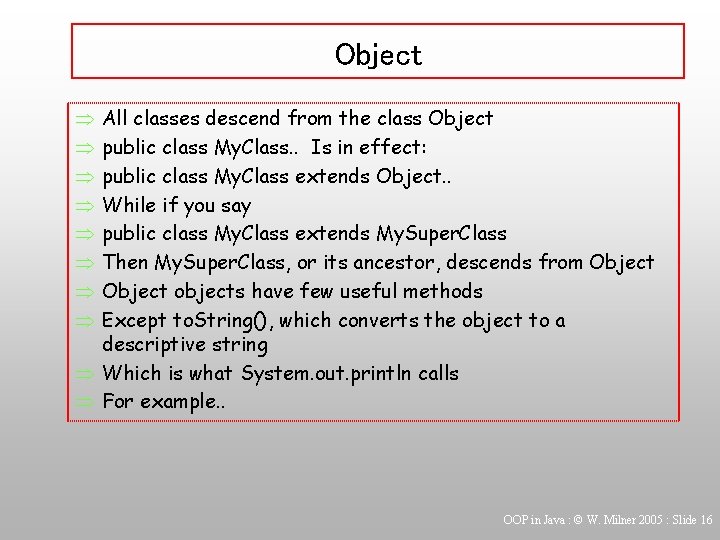
Object All classes descend from the class Object public class My. Class. . Is in effect: public class My. Class extends Object. . While if you say public class My. Class extends My. Super. Class Then My. Super. Class, or its ancestor, descends from Object objects have few useful methods Except to. String(), which converts the object to a descriptive string Þ Which is what System. out. println calls Þ For example. . Þ Þ Þ Þ OOP in Java : © W. Milner 2005 : Slide 16
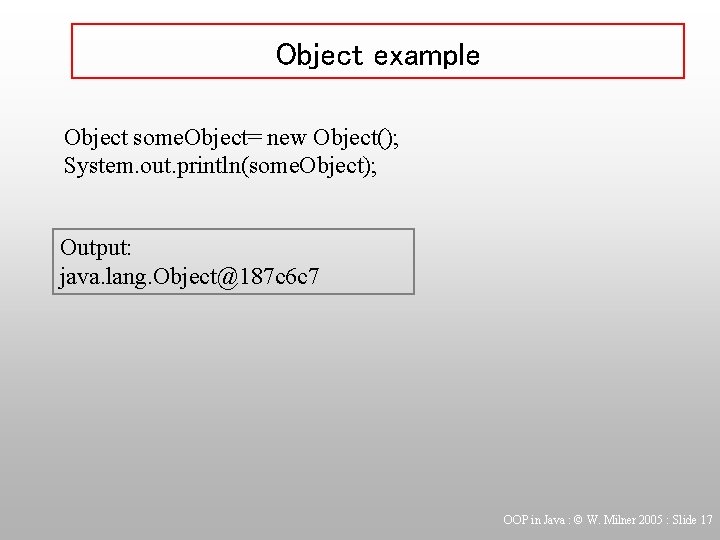
Object example Object some. Object= new Object(); System. out. println(some. Object); Output: java. lang. Object@187 c 6 c 7 OOP in Java : © W. Milner 2005 : Slide 17
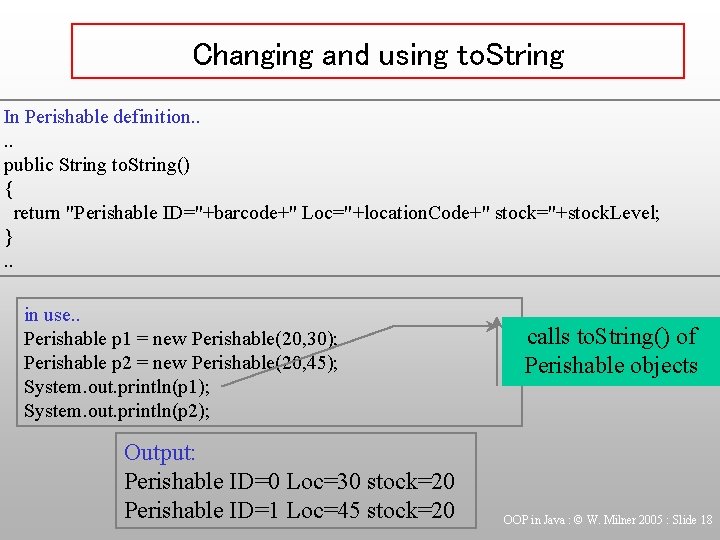
Changing and using to. String In Perishable definition. . public String to. String() { return "Perishable ID="+barcode+" Loc="+location. Code+" stock="+stock. Level; }. . in use. . Perishable p 1 = new Perishable(20, 30); Perishable p 2 = new Perishable(20, 45); System. out. println(p 1); System. out. println(p 2); Output: Perishable ID=0 Loc=30 stock=20 Perishable ID=1 Loc=45 stock=20 calls to. String() of Perishable objects OOP in Java : © W. Milner 2005 : Slide 18
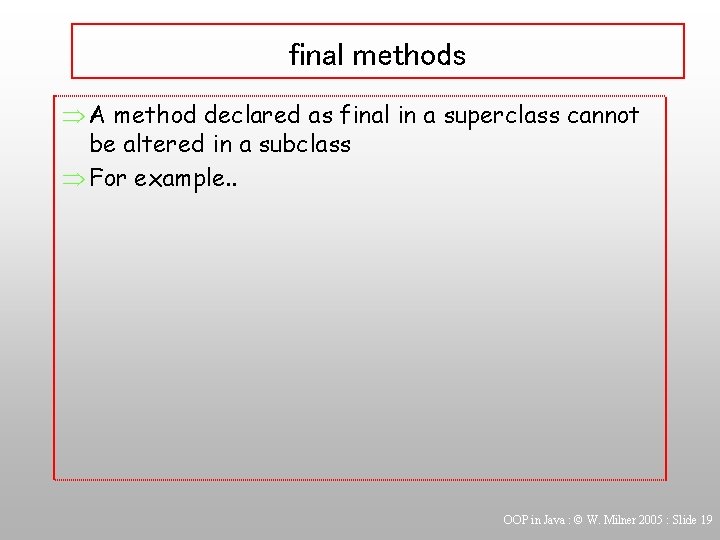
final methods Þ A method declared as final in a superclass cannot be altered in a subclass Þ For example. . OOP in Java : © W. Milner 2005 : Slide 19
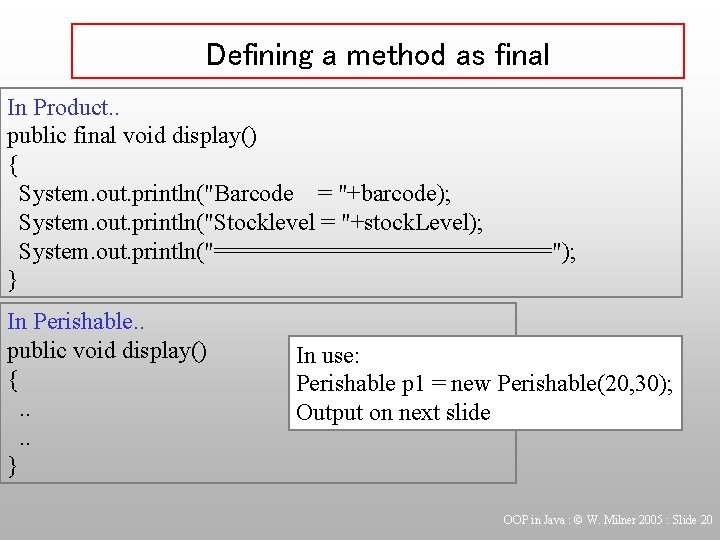
Defining a method as final In Product. . public final void display() { System. out. println("Barcode = "+barcode); System. out. println("Stocklevel = "+stock. Level); System. out. println("============="); } In Perishable. . public void display() {. . } In use: Perishable p 1 = new Perishable(20, 30); Output on next slide OOP in Java : © W. Milner 2005 : Slide 20
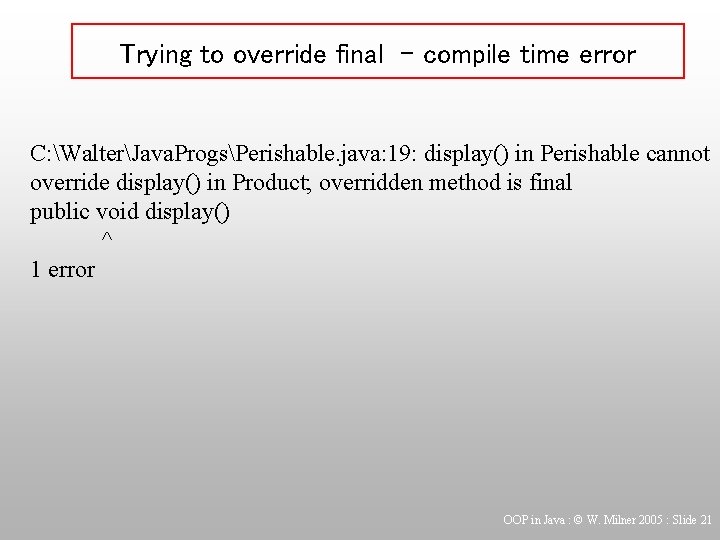
Trying to override final - compile time error C: WalterJava. ProgsPerishable. java: 19: display() in Perishable cannot override display() in Product; overridden method is final public void display() ^ 1 error OOP in Java : © W. Milner 2005 : Slide 21
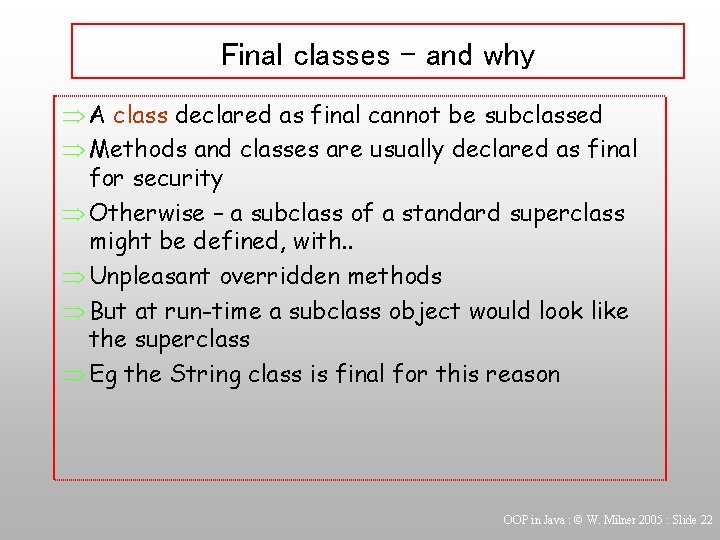
Final classes – and why Þ A class declared as final cannot be subclassed Þ Methods and classes are usually declared as final for security Þ Otherwise – a subclass of a standard superclass might be defined, with. . Þ Unpleasant overridden methods Þ But at run-time a subclass object would look like the superclass Þ Eg the String class is final for this reason OOP in Java : © W. Milner 2005 : Slide 22
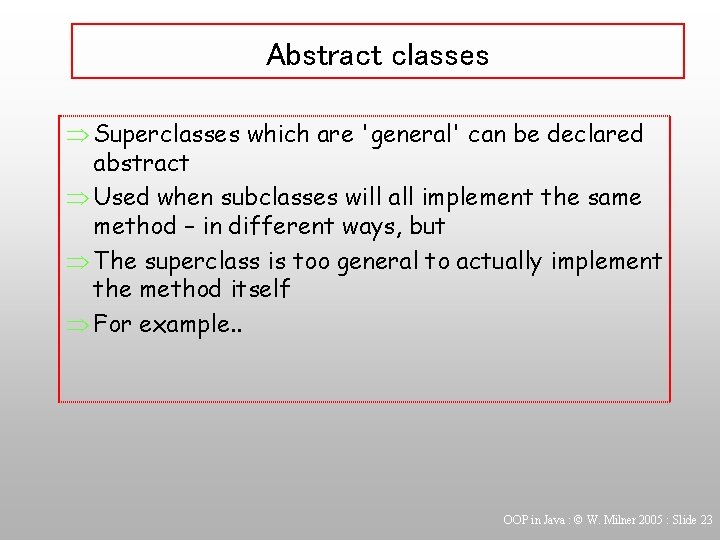
Abstract classes Þ Superclasses which are 'general' can be declared abstract Þ Used when subclasses will all implement the same method – in different ways, but Þ The superclass is too general to actually implement the method itself Þ For example. . OOP in Java : © W. Milner 2005 : Slide 23
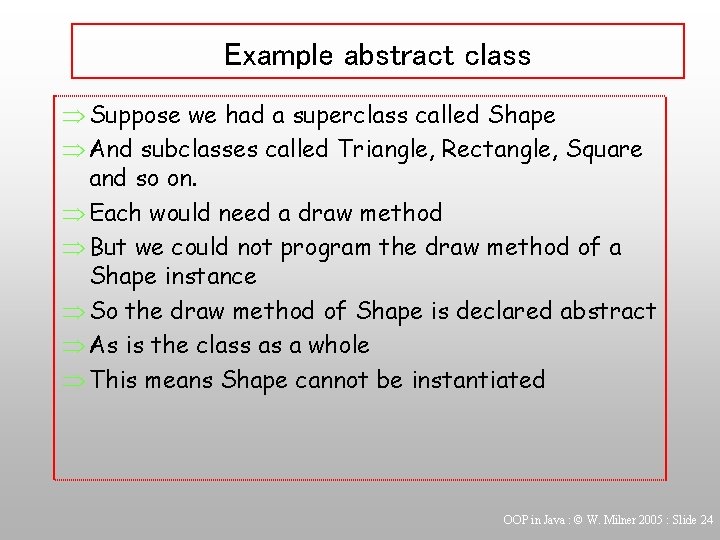
Example abstract class Þ Suppose we had a superclass called Shape Þ And subclasses called Triangle, Rectangle, Square and so on. Þ Each would need a draw method Þ But we could not program the draw method of a Shape instance Þ So the draw method of Shape is declared abstract Þ As is the class as a whole Þ This means Shape cannot be instantiated OOP in Java : © W. Milner 2005 : Slide 24
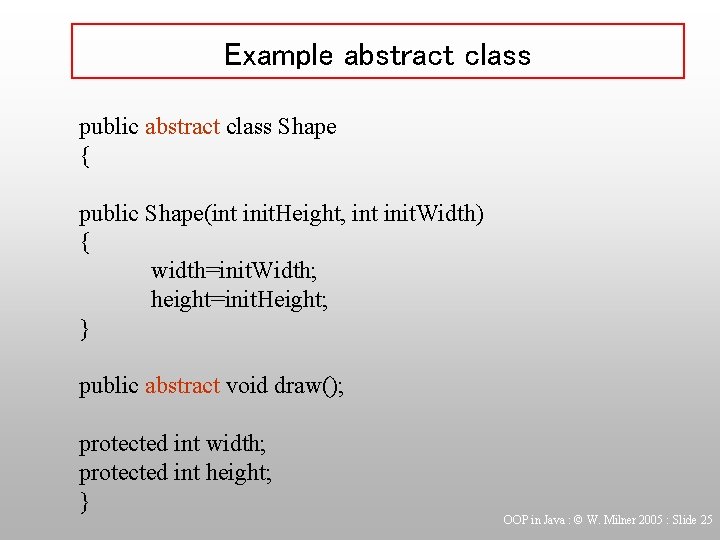
Example abstract class public abstract class Shape { public Shape(int init. Height, int init. Width) { width=init. Width; height=init. Height; } public abstract void draw(); protected int width; protected int height; } OOP in Java : © W. Milner 2005 : Slide 25
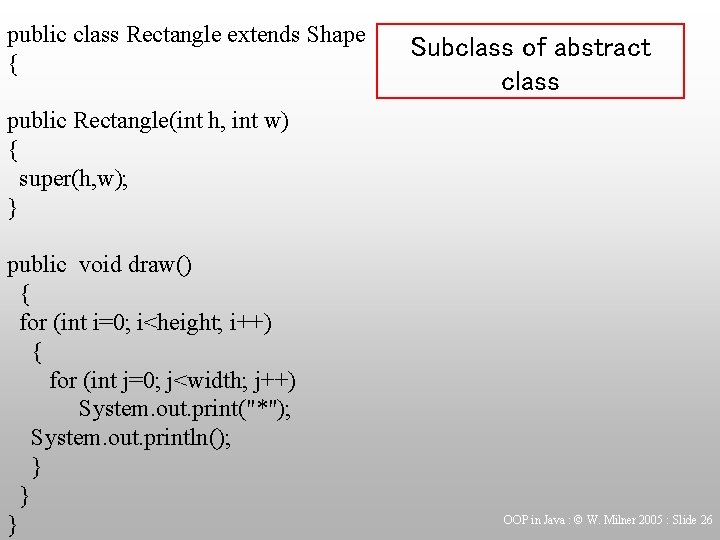
public class Rectangle extends Shape { Subclass of abstract class public Rectangle(int h, int w) { super(h, w); } public void draw() { for (int i=0; i<height; i++) { for (int j=0; j<width; j++) System. out. print("*"); System. out. println(); } } } OOP in Java : © W. Milner 2005 : Slide 26
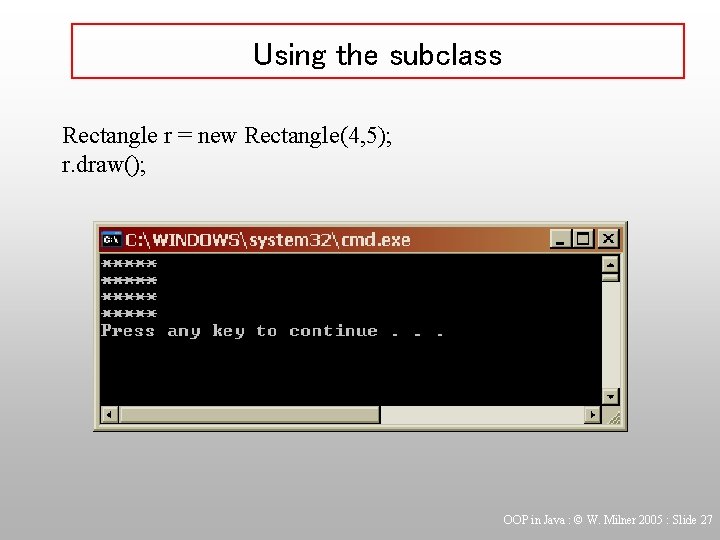
Using the subclass Rectangle r = new Rectangle(4, 5); r. draw(); OOP in Java : © W. Milner 2005 : Slide 27
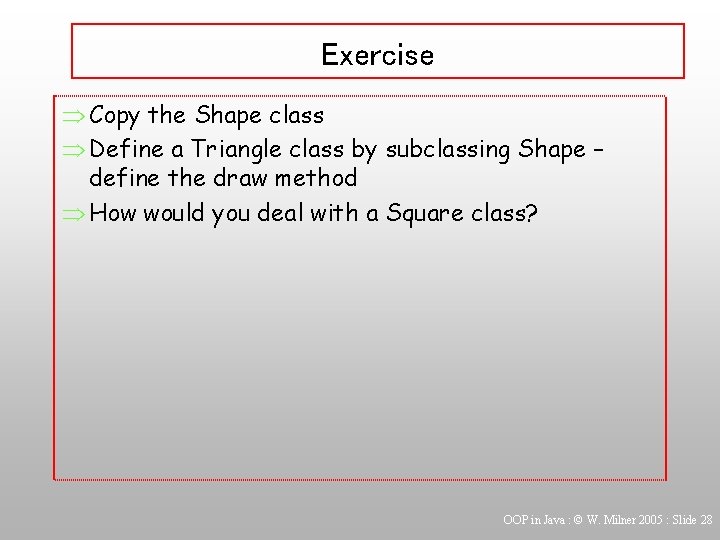
Exercise Þ Copy the Shape class Þ Define a Triangle class by subclassing Shape – define the draw method Þ How would you deal with a Square class? OOP in Java : © W. Milner 2005 : Slide 28