Inherited Classes in Java CSCI 392 Classes Part
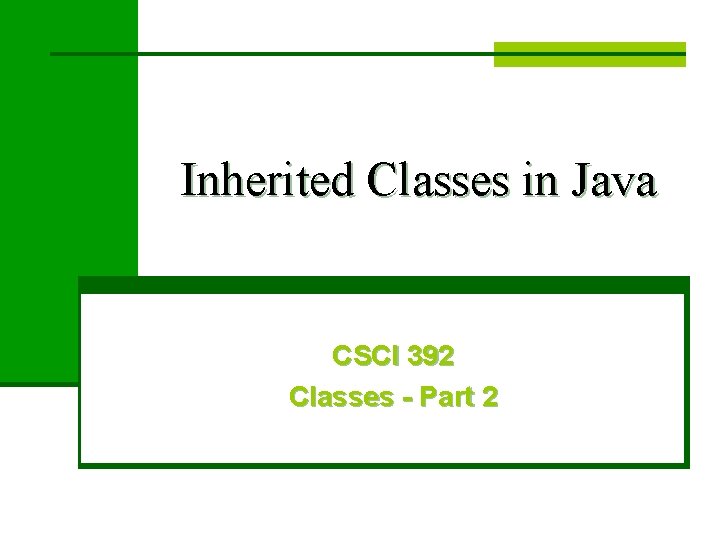
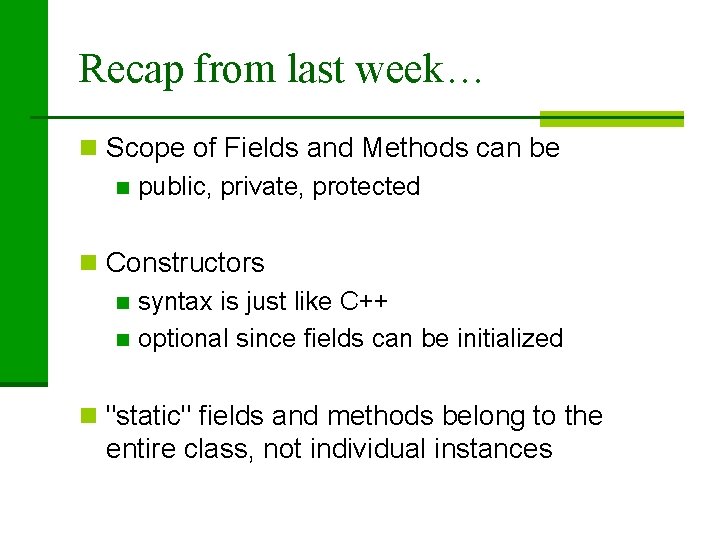
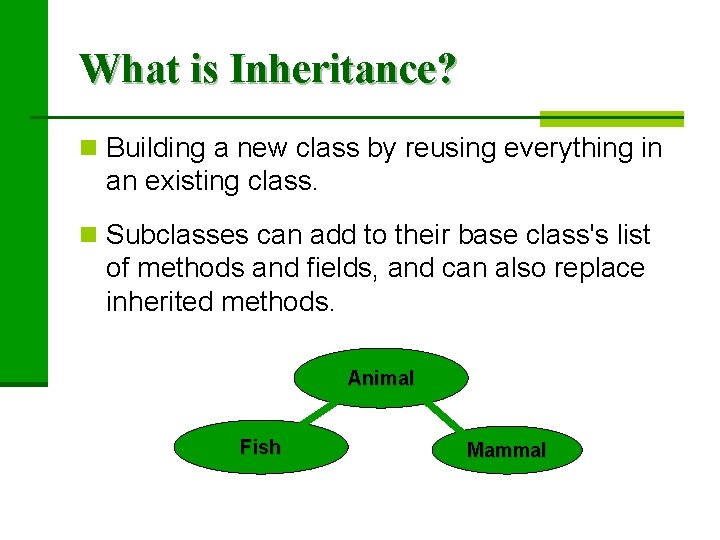
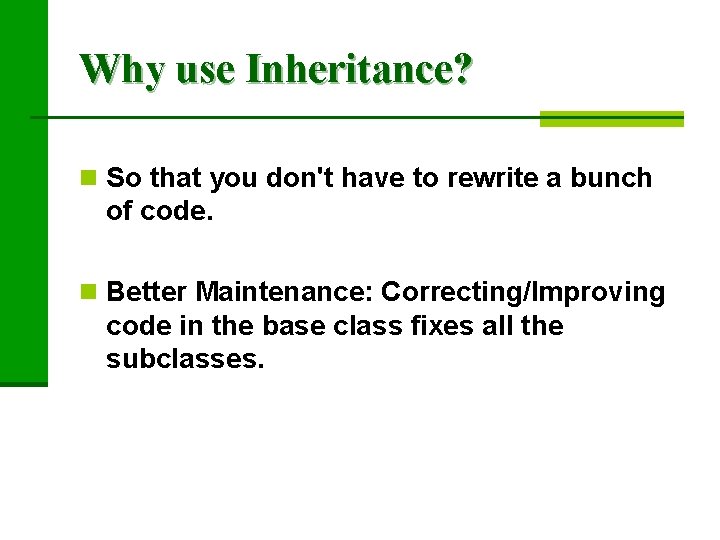
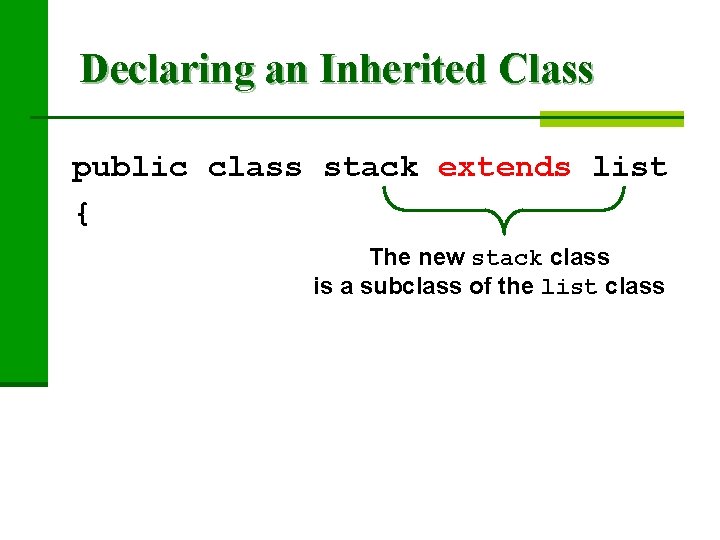
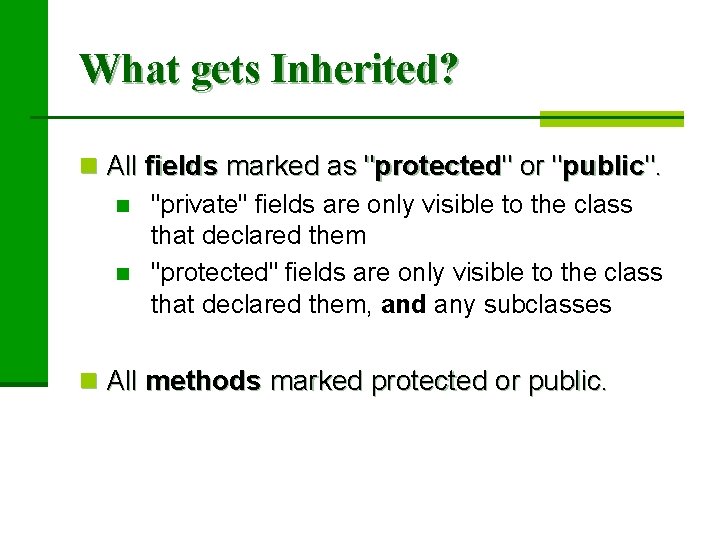
![public class list { protected int[] values; private int size; . . . public public class list { protected int[] values; private int size; . . . public](https://slidetodoc.com/presentation_image_h2/e4fd97893a806f7f3405d92f06a36767/image-7.jpg)
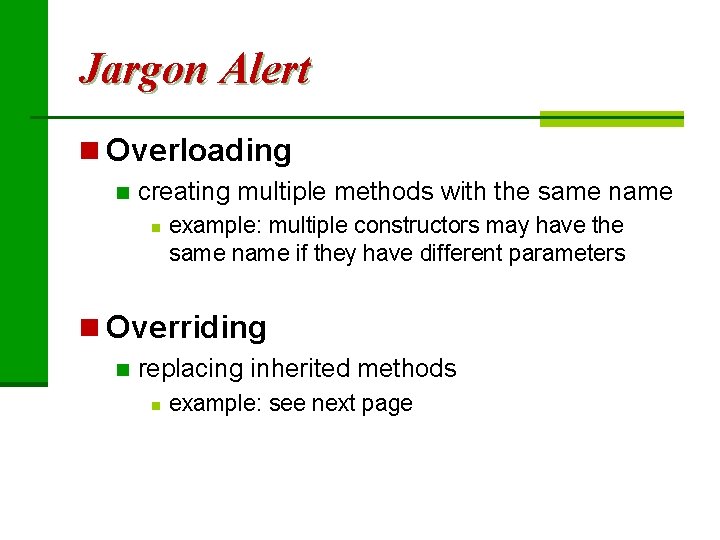
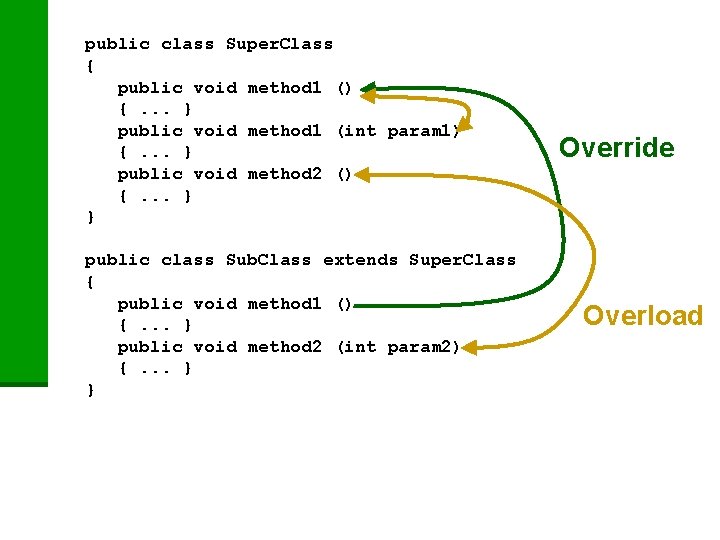
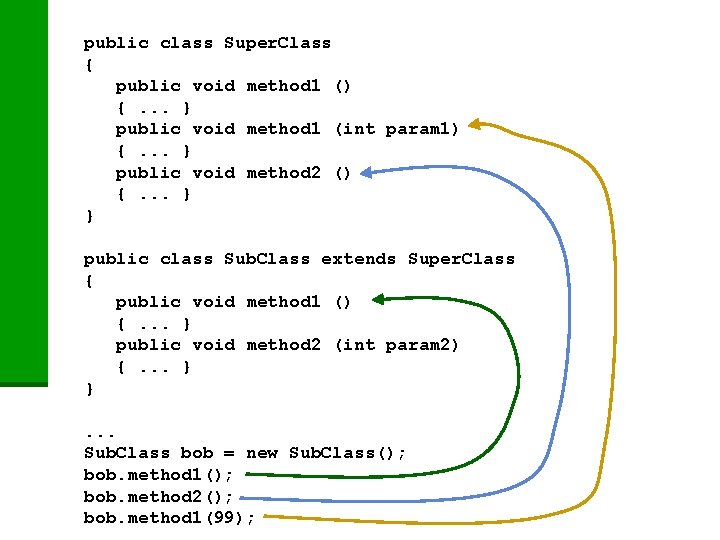
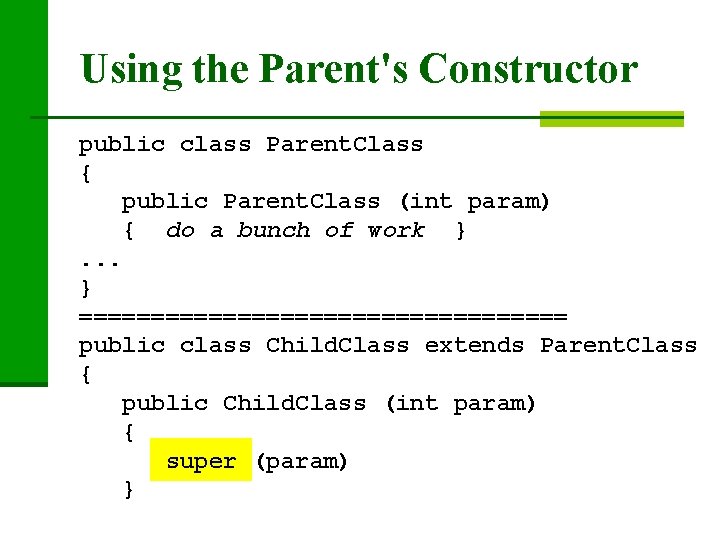
- Slides: 11
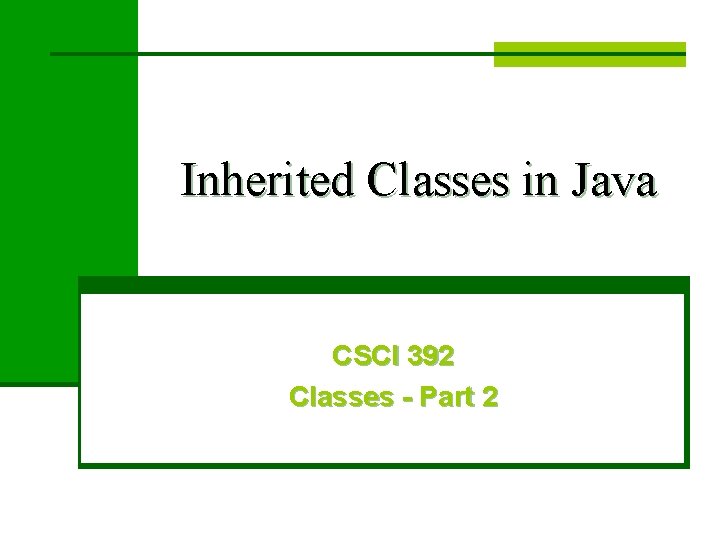
Inherited Classes in Java CSCI 392 Classes - Part 2
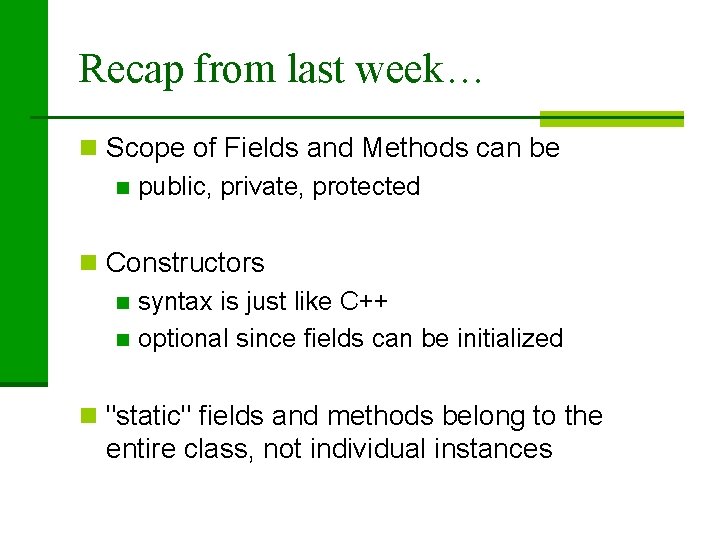
Recap from last week… n Scope of Fields and Methods can be n public, private, protected n Constructors n syntax is just like C++ n optional since fields can be initialized n "static" fields and methods belong to the entire class, not individual instances
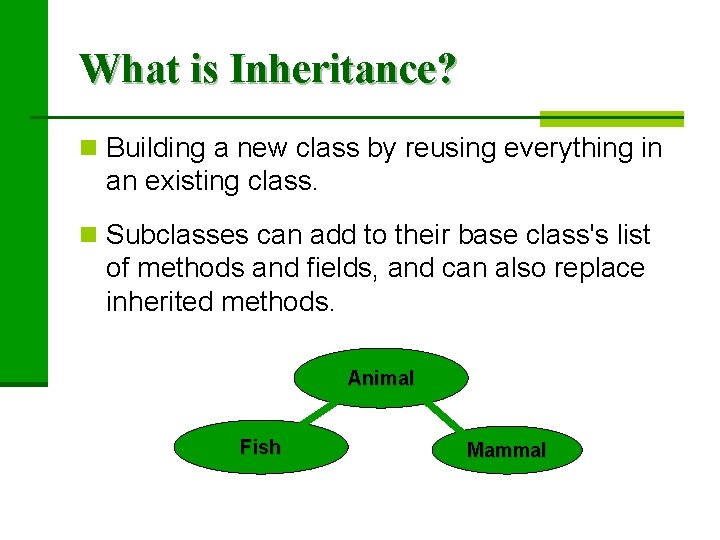
What is Inheritance? n Building a new class by reusing everything in an existing class. n Subclasses can add to their base class's list of methods and fields, and can also replace inherited methods. Animal Fish Mammal
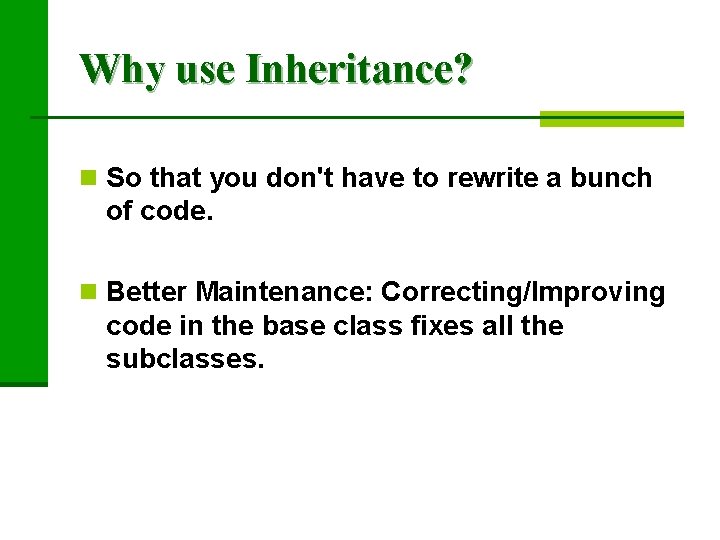
Why use Inheritance? n So that you don't have to rewrite a bunch of code. n Better Maintenance: Correcting/Improving code in the base class fixes all the subclasses.
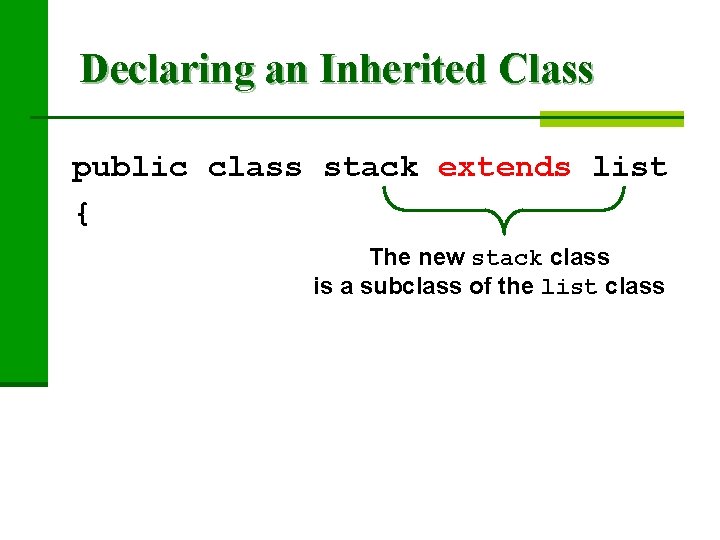
Declaring an Inherited Class public class stack extends list { The new stack class is a subclass of the list class
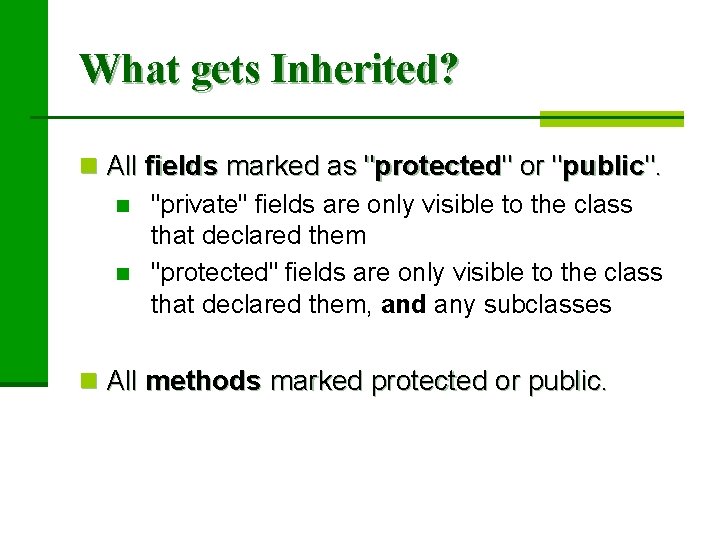
What gets Inherited? n All fields marked as "protected" or "public". n "private" fields are only visible to the class that declared them n "protected" fields are only visible to the class that declared them, and any subclasses n All methods marked protected or public.
![public class list protected int values private int size public public class list { protected int[] values; private int size; . . . public](https://slidetodoc.com/presentation_image_h2/e4fd97893a806f7f3405d92f06a36767/image-7.jpg)
public class list { protected int[] values; private int size; . . . public class stack extends list {. . . public void some_method () { values[i] = myinteger; // legal size++; // illegal
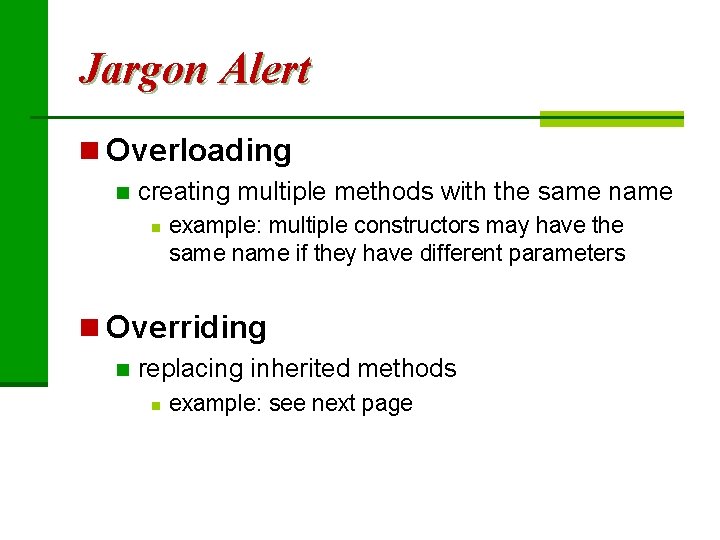
Jargon Alert n Overloading n creating multiple methods with the same n example: multiple constructors may have the same name if they have different parameters n Overriding n replacing inherited methods n example: see next page
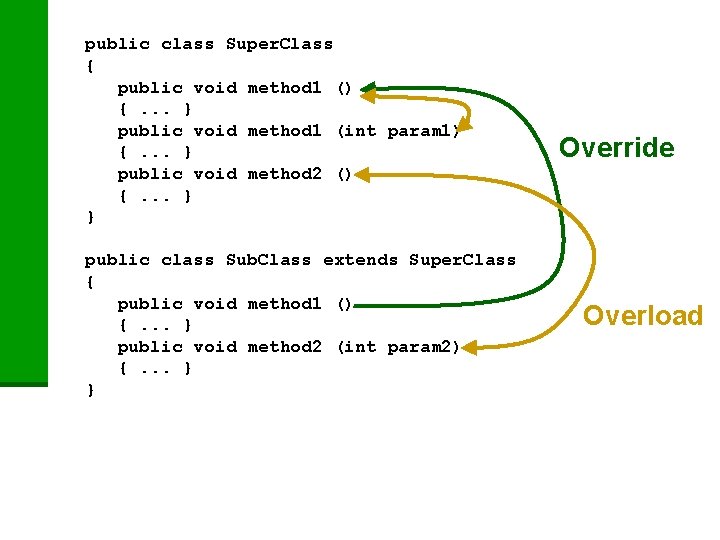
public class Super. Class { public void method 1 () {. . . } public void method 1 (int param 1) {. . . } public void method 2 () {. . . } } public class Sub. Class extends Super. Class { public void method 1 () {. . . } public void method 2 (int param 2) {. . . } } Override Overload
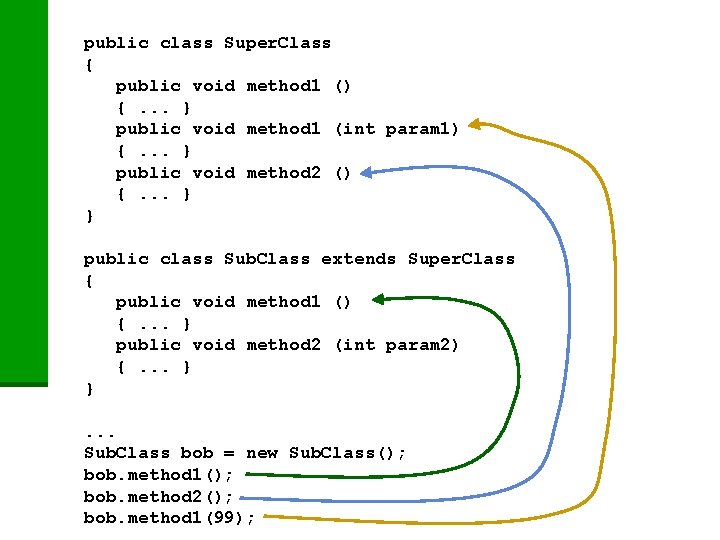
public class Super. Class { public void method 1 () {. . . } public void method 1 (int param 1) {. . . } public void method 2 () {. . . } } public class Sub. Class extends Super. Class { public void method 1 () {. . . } public void method 2 (int param 2) {. . . } }. . . Sub. Class bob = new Sub. Class(); bob. method 1(); bob. method 2(); bob. method 1(99);
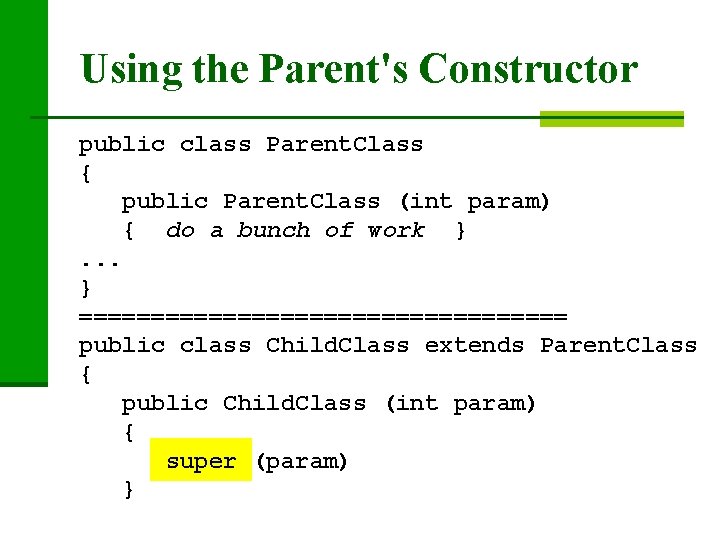
Using the Parent's Constructor public class Parent. Class { public Parent. Class (int param) { do a bunch of work }. . . } ================= public class Child. Class extends Parent. Class { public Child. Class (int param) { super (param) }
Włącz czynnik pod pierwiastek
Kj 392 not angka
Classes e subclasses de palavras exercicios
Pre ap classes vs regular classes
Thalassemia trait
Similarities and differences between parents and offspring
Acquired traits for animals
Elephant inherited traits
Inherited traits and learned behaviors
Examples of instincts
Acquired traits in animals
Homocigoto dominante