Java OOP Some standard packages OOP in Java
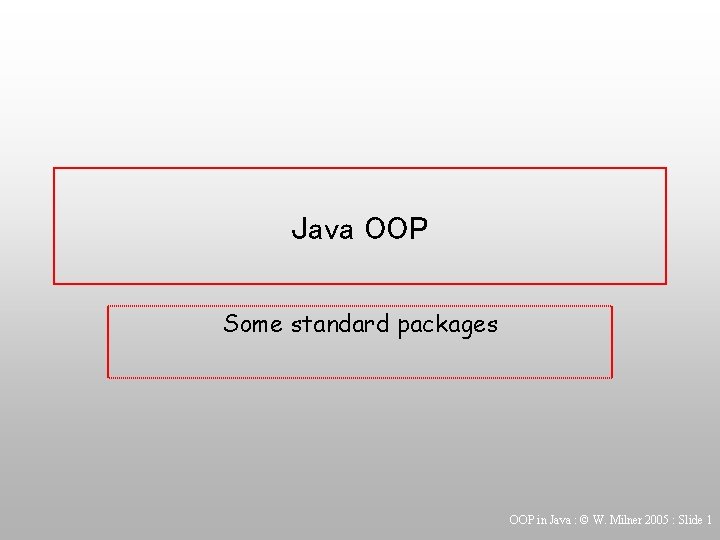
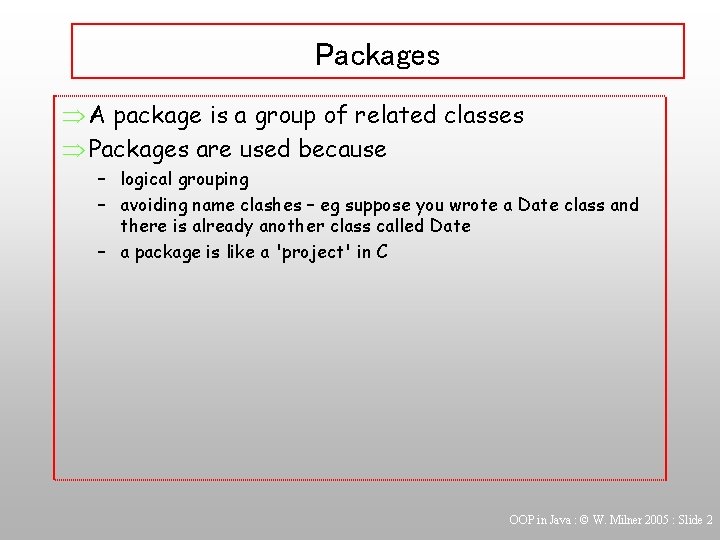
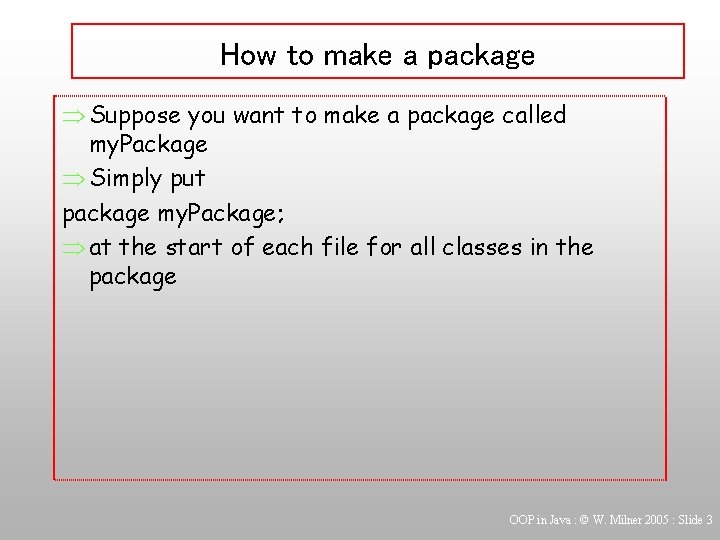
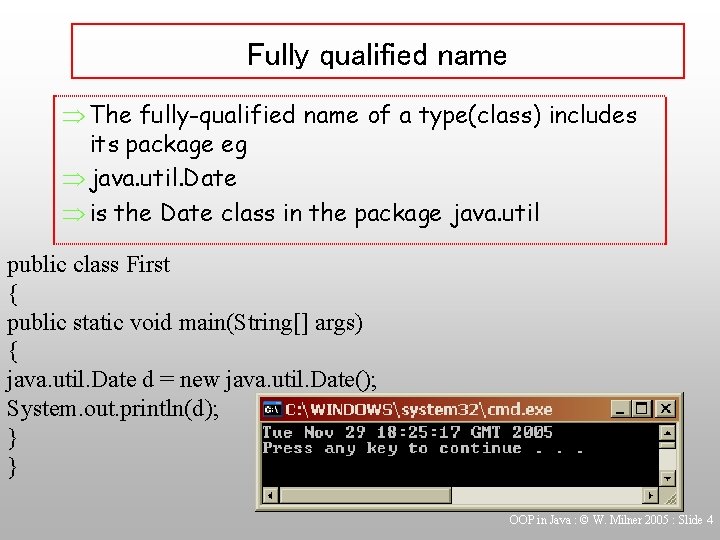
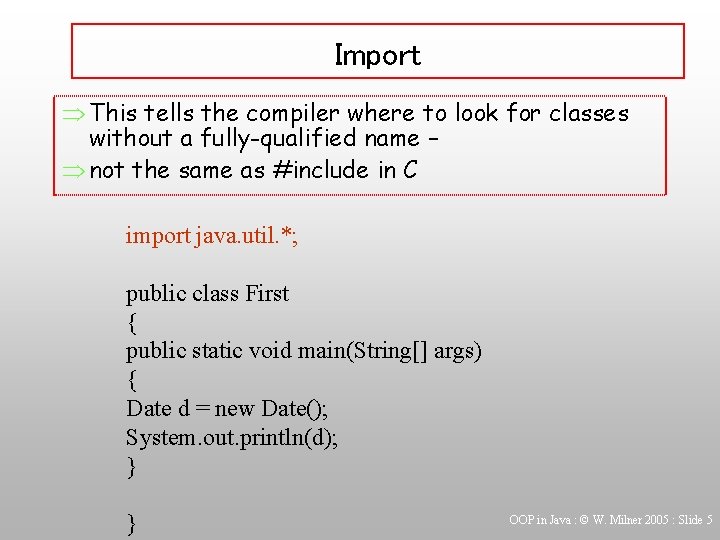
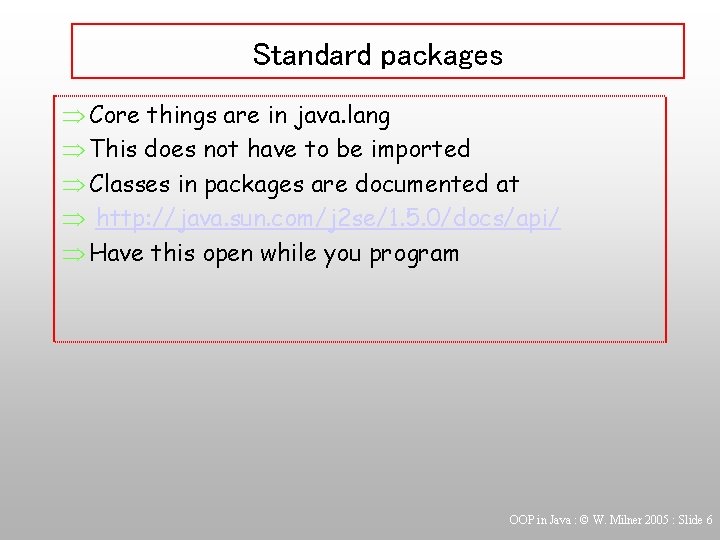
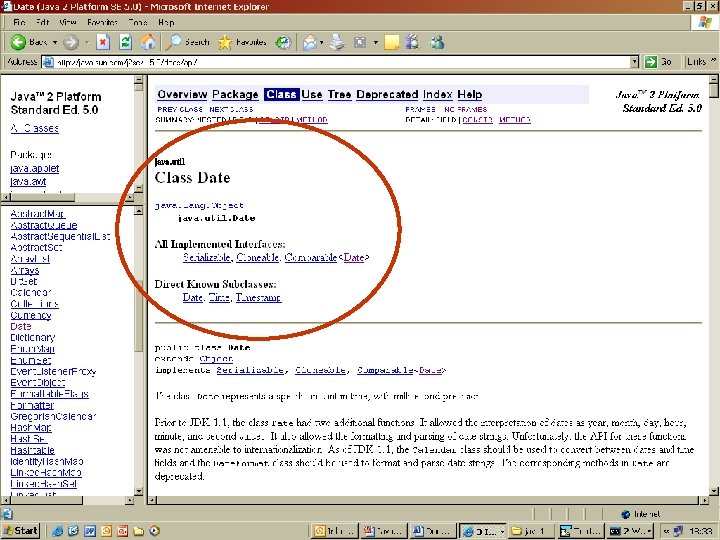
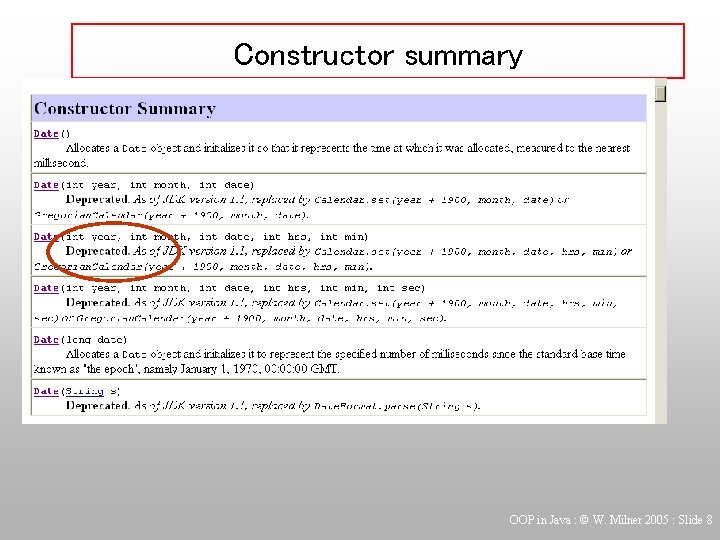
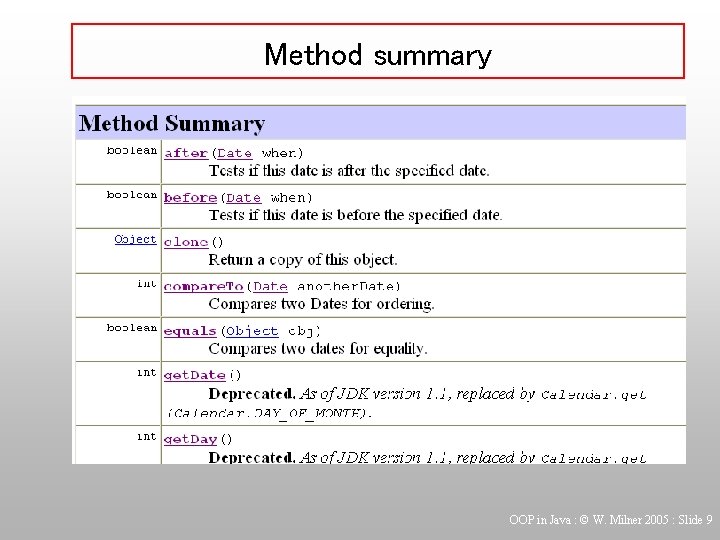
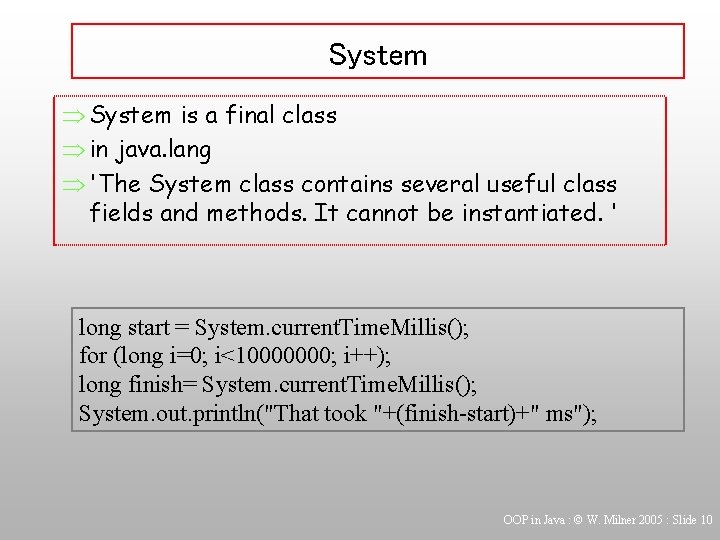
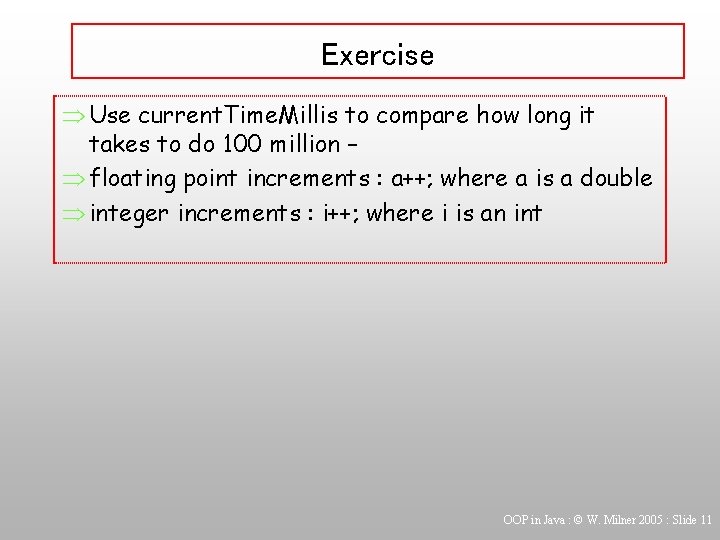
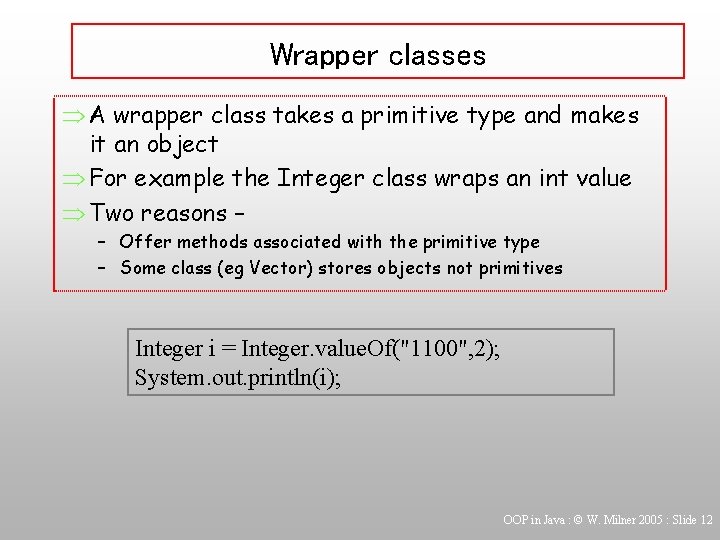
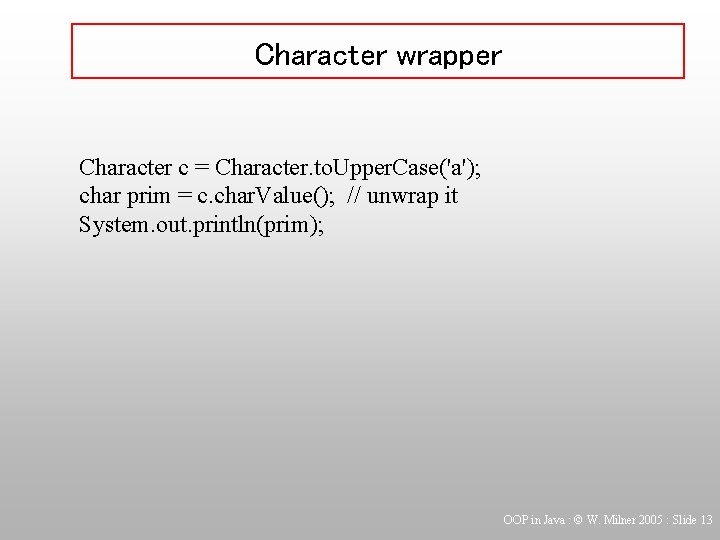
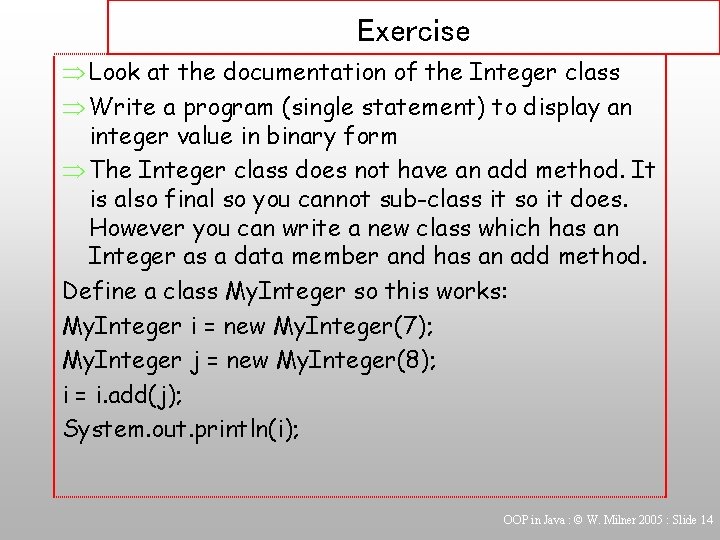
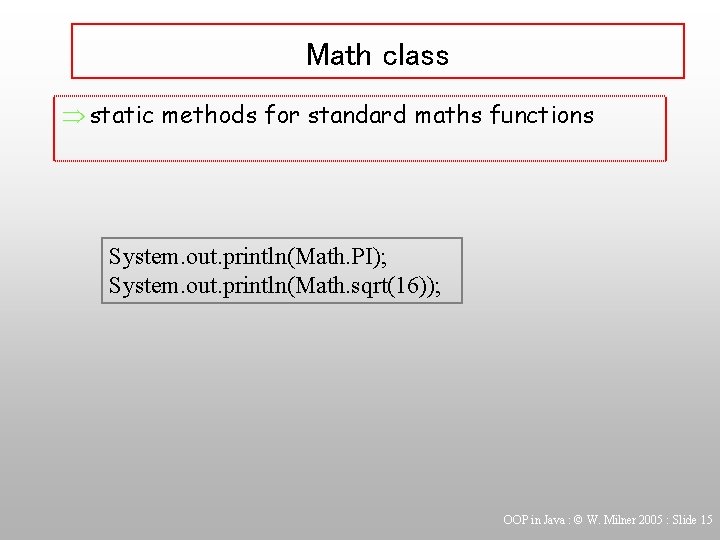
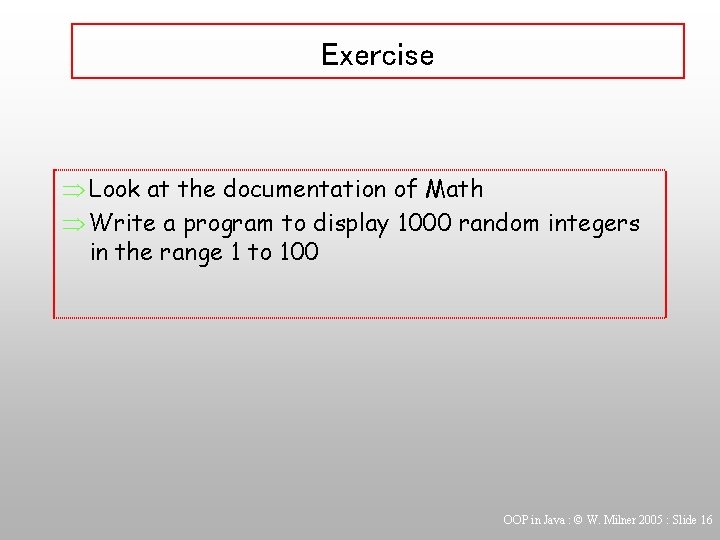
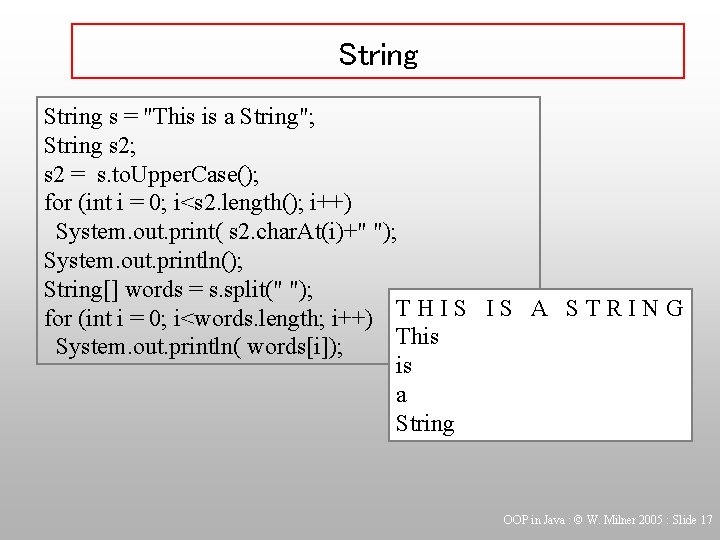
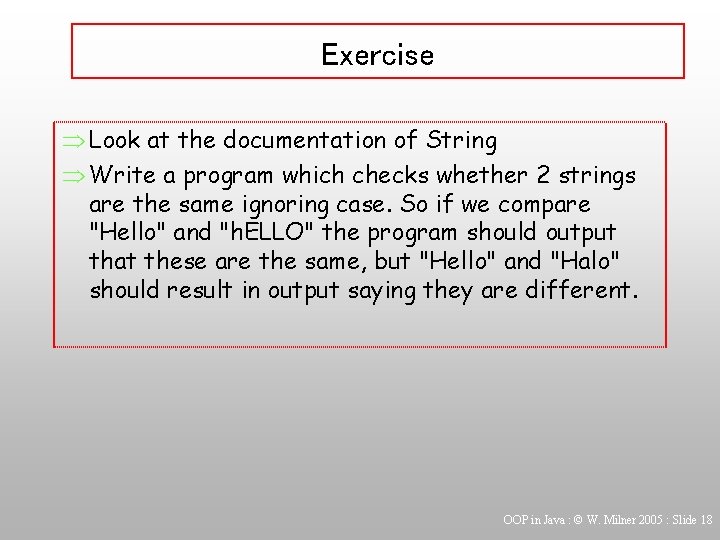
- Slides: 18
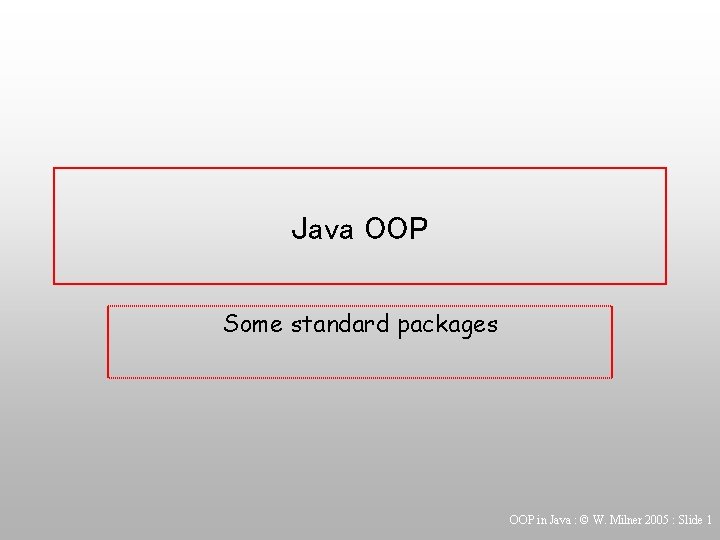
Java OOP Some standard packages OOP in Java : © W. Milner 2005 : Slide 1
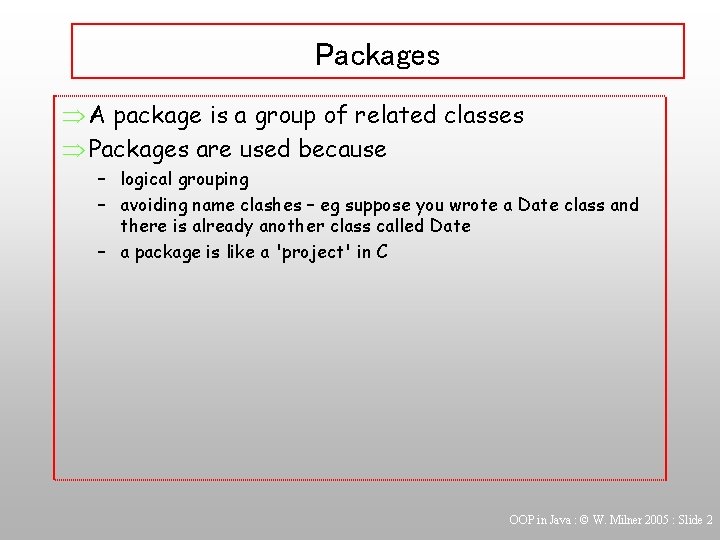
Packages Þ A package is a group of related classes Þ Packages are used because – logical grouping – avoiding name clashes – eg suppose you wrote a Date class and there is already another class called Date – a package is like a 'project' in C OOP in Java : © W. Milner 2005 : Slide 2
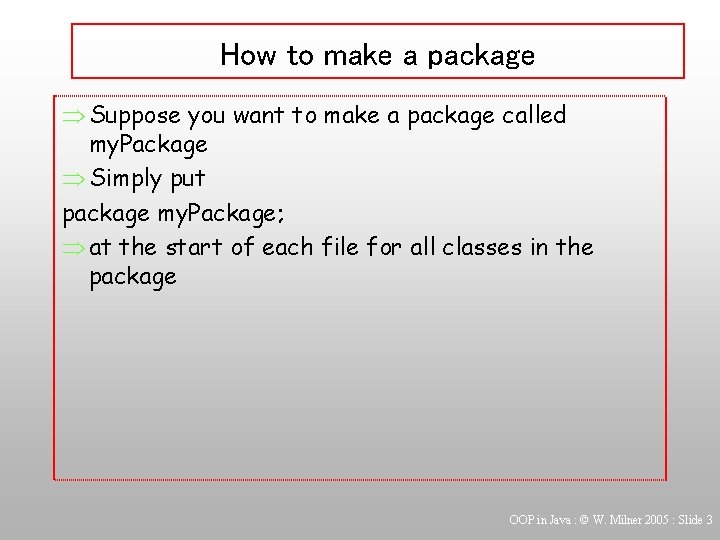
How to make a package Þ Suppose you want to make a package called my. Package Þ Simply put package my. Package; Þ at the start of each file for all classes in the package OOP in Java : © W. Milner 2005 : Slide 3
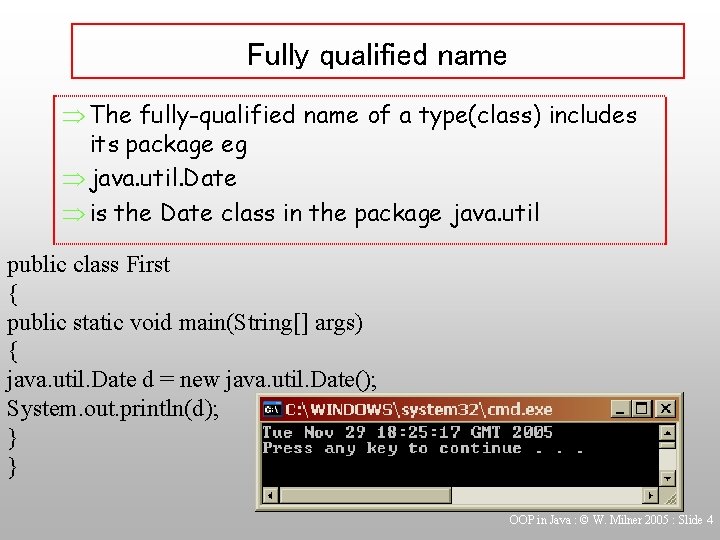
Fully qualified name Þ The fully-qualified name of a type(class) includes its package eg Þ java. util. Date Þ is the Date class in the package java. util public class First { public static void main(String[] args) { java. util. Date d = new java. util. Date(); System. out. println(d); } } OOP in Java : © W. Milner 2005 : Slide 4
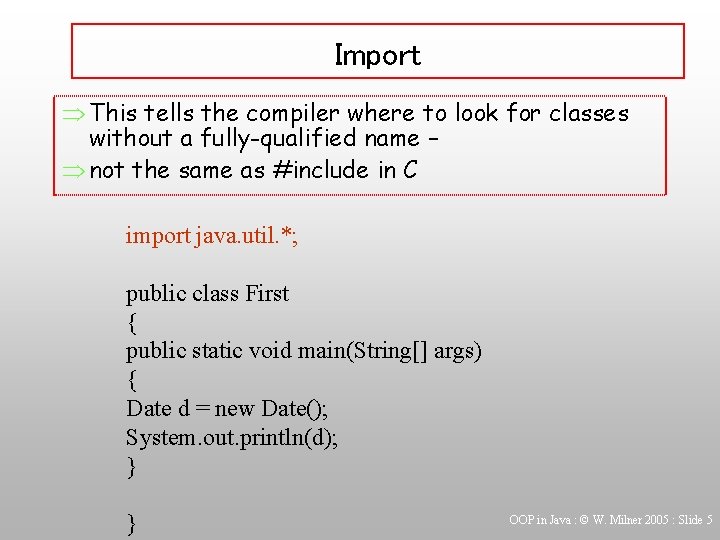
Import Þ This tells the compiler where to look for classes without a fully-qualified name – Þ not the same as #include in C import java. util. *; public class First { public static void main(String[] args) { Date d = new Date(); System. out. println(d); } } OOP in Java : © W. Milner 2005 : Slide 5
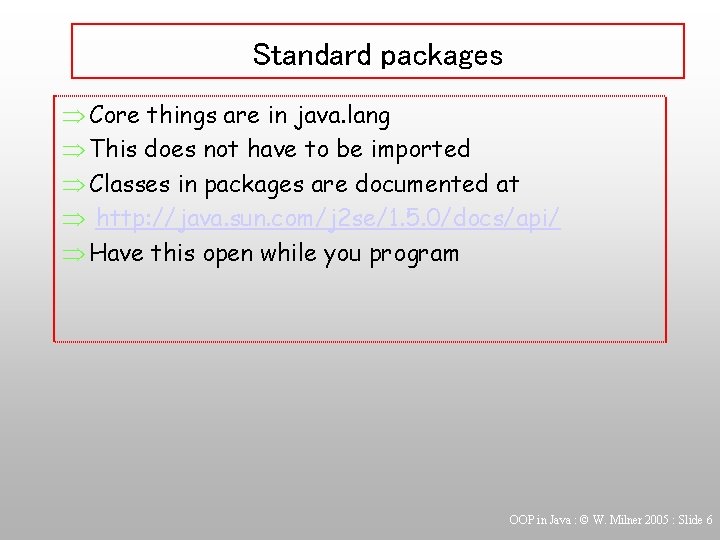
Standard packages Þ Core things are in java. lang Þ This does not have to be imported Þ Classes in packages are documented at Þ http: //java. sun. com/j 2 se/1. 5. 0/docs/api/ Þ Have this open while you program OOP in Java : © W. Milner 2005 : Slide 6
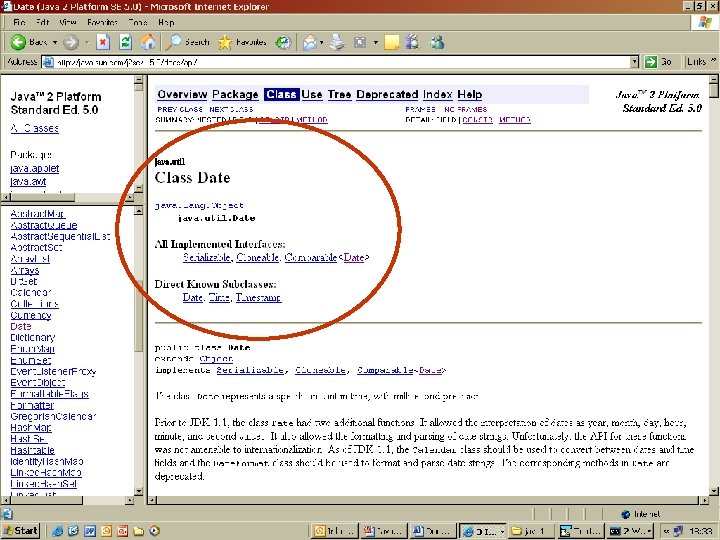
OOP in Java : © W. Milner 2005 : Slide 7
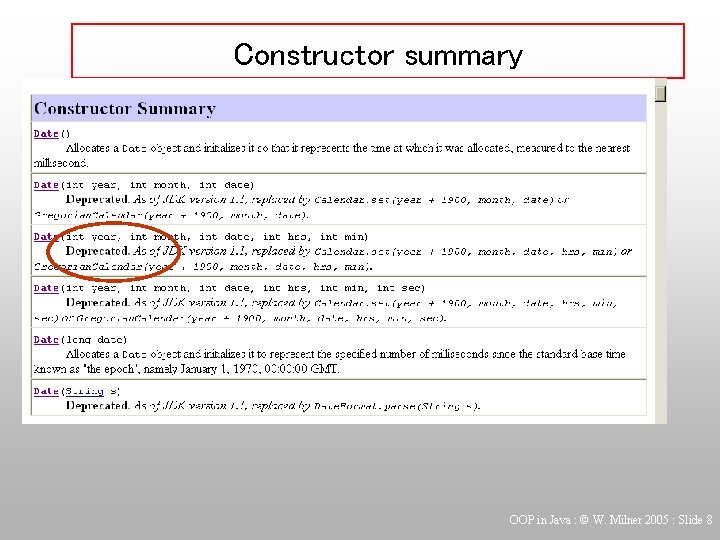
Constructor summary OOP in Java : © W. Milner 2005 : Slide 8
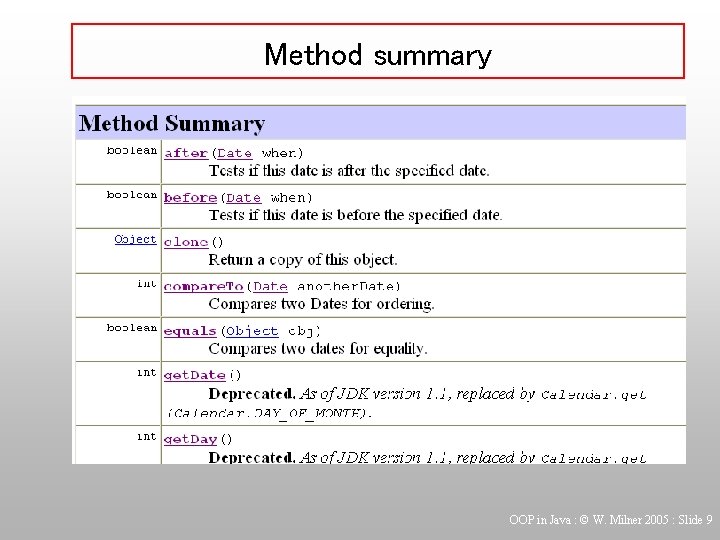
Method summary OOP in Java : © W. Milner 2005 : Slide 9
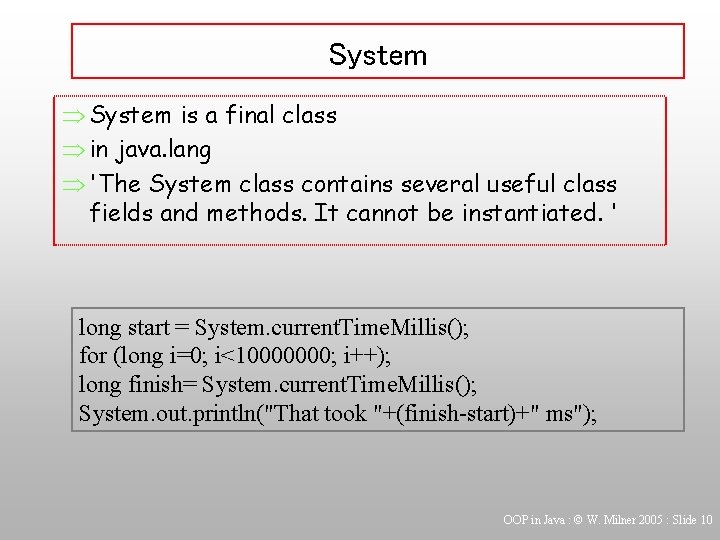
System Þ System is a final class Þ in java. lang Þ 'The System class contains several useful class fields and methods. It cannot be instantiated. ' long start = System. current. Time. Millis(); for (long i=0; i<10000000; i++); long finish= System. current. Time. Millis(); System. out. println("That took "+(finish-start)+" ms"); OOP in Java : © W. Milner 2005 : Slide 10
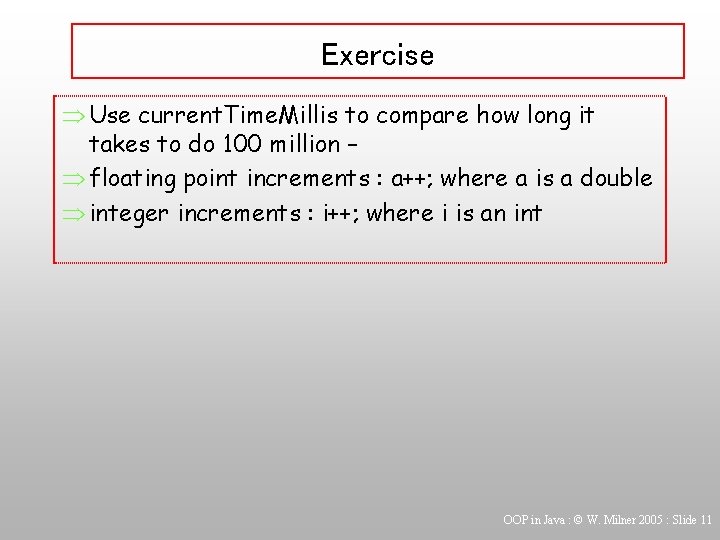
Exercise Þ Use current. Time. Millis to compare how long it takes to do 100 million – Þ floating point increments : a++; where a is a double Þ integer increments : i++; where i is an int OOP in Java : © W. Milner 2005 : Slide 11
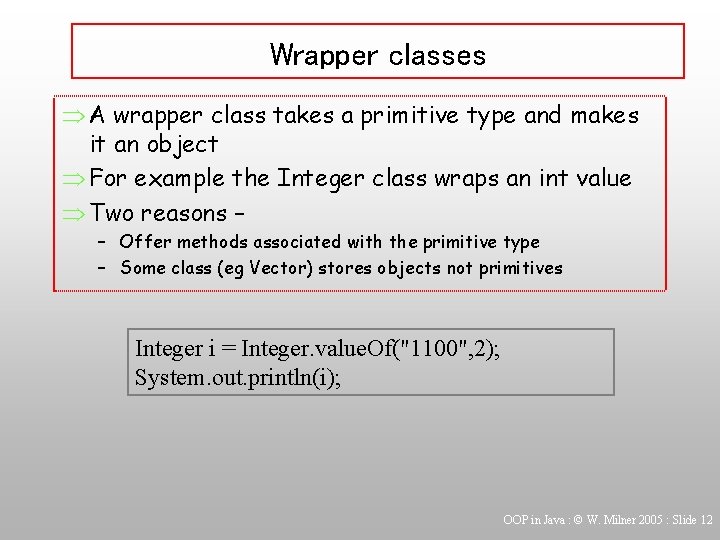
Wrapper classes Þ A wrapper class takes a primitive type and makes it an object Þ For example the Integer class wraps an int value Þ Two reasons – – Offer methods associated with the primitive type – Some class (eg Vector) stores objects not primitives Integer i = Integer. value. Of("1100", 2); System. out. println(i); OOP in Java : © W. Milner 2005 : Slide 12
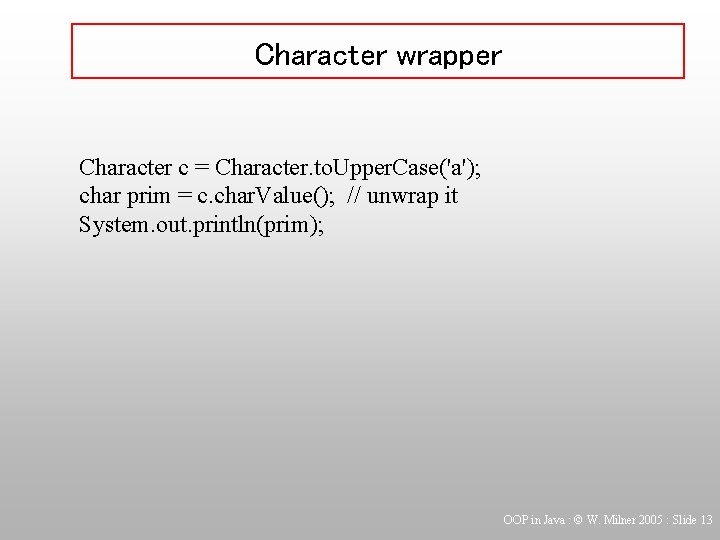
Character wrapper Character c = Character. to. Upper. Case('a'); char prim = c. char. Value(); // unwrap it System. out. println(prim); OOP in Java : © W. Milner 2005 : Slide 13
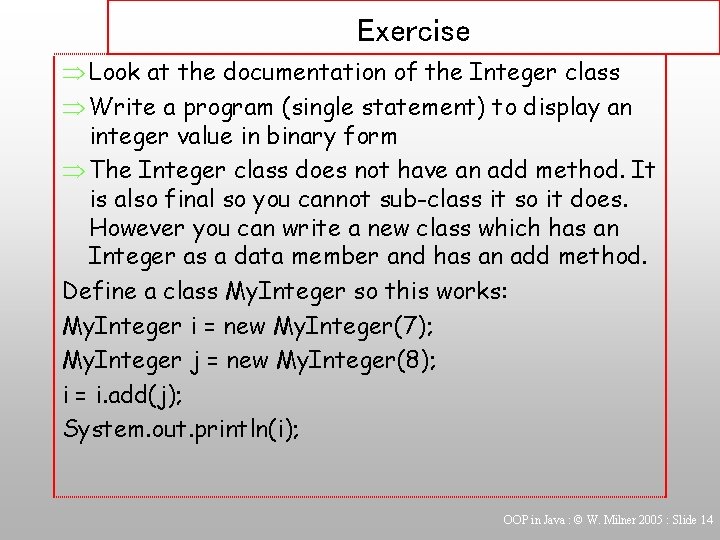
Exercise Þ Look at the documentation of the Integer class Þ Write a program (single statement) to display an integer value in binary form Þ The Integer class does not have an add method. It is also final so you cannot sub-class it so it does. However you can write a new class which has an Integer as a data member and has an add method. Define a class My. Integer so this works: My. Integer i = new My. Integer(7); My. Integer j = new My. Integer(8); i = i. add(j); System. out. println(i); OOP in Java : © W. Milner 2005 : Slide 14
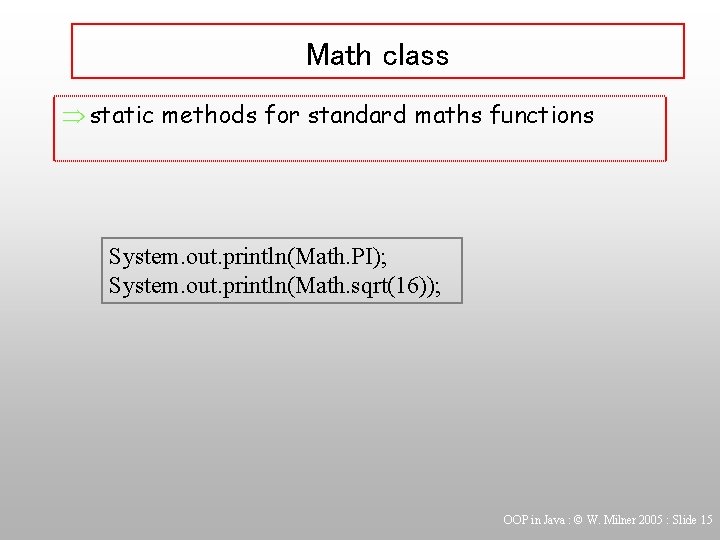
Math class Þ static methods for standard maths functions System. out. println(Math. PI); System. out. println(Math. sqrt(16)); OOP in Java : © W. Milner 2005 : Slide 15
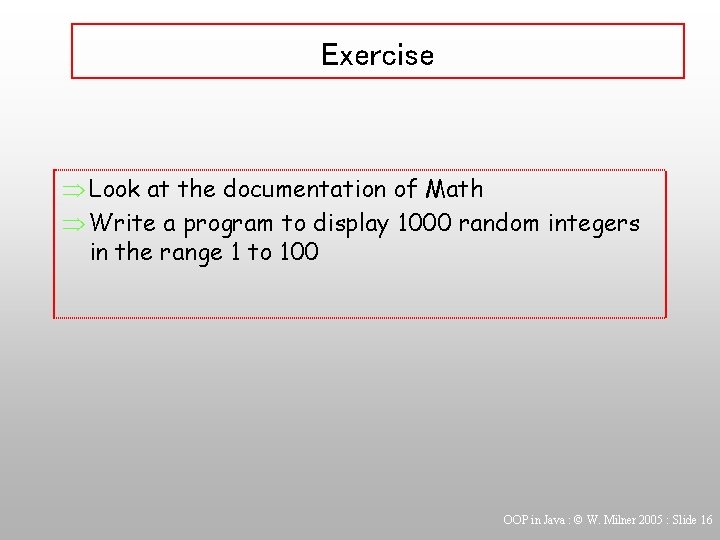
Exercise Þ Look at the documentation of Math Þ Write a program to display 1000 random integers in the range 1 to 100 OOP in Java : © W. Milner 2005 : Slide 16
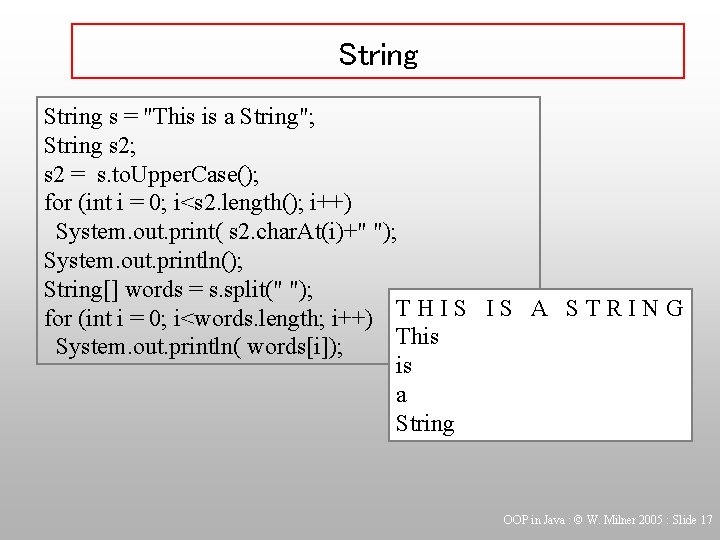
String s = "This is a String"; String s 2; s 2 = s. to. Upper. Case(); for (int i = 0; i<s 2. length(); i++) System. out. print( s 2. char. At(i)+" "); System. out. println(); String[] words = s. split(" "); for (int i = 0; i<words. length; i++) T H I S A S T R I N G This System. out. println( words[i]); is a String OOP in Java : © W. Milner 2005 : Slide 17
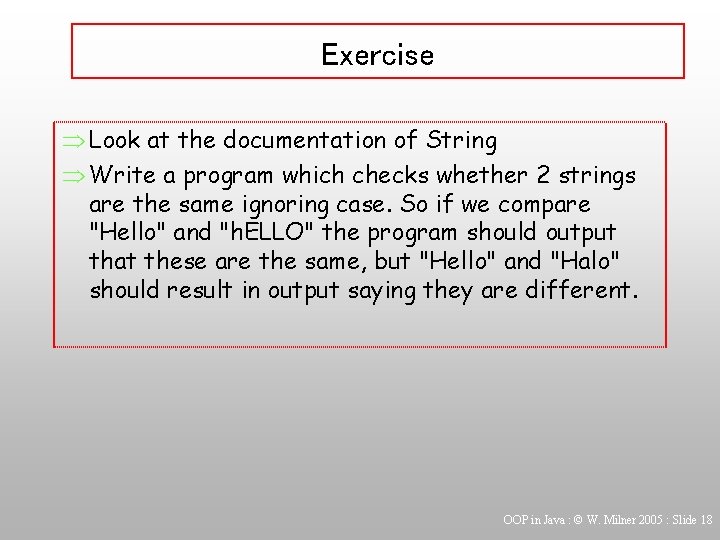
Exercise Þ Look at the documentation of String Þ Write a program which checks whether 2 strings are the same ignoring case. So if we compare "Hello" and "h. ELLO" the program should output that these are the same, but "Hello" and "Halo" should result in output saying they are different. OOP in Java : © W. Milner 2005 : Slide 18