Chapter 5 Control Structures II Java Programming From
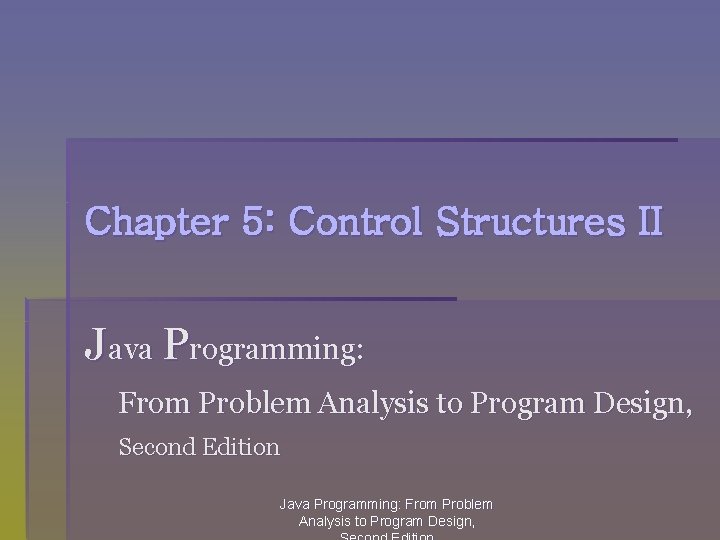
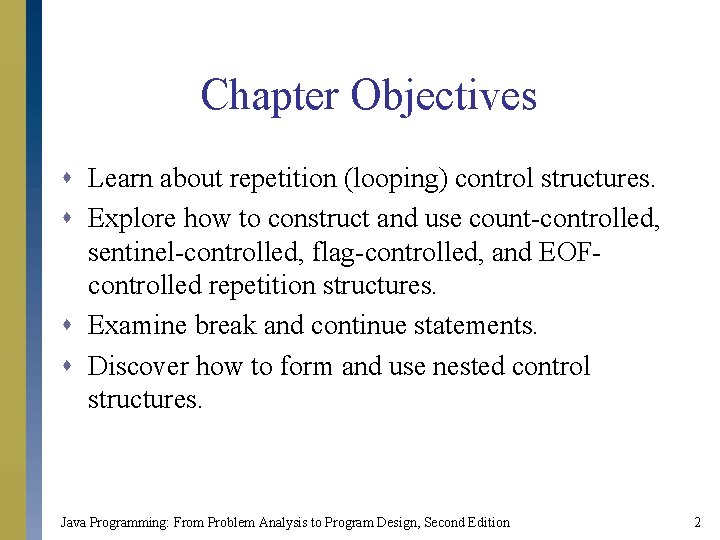
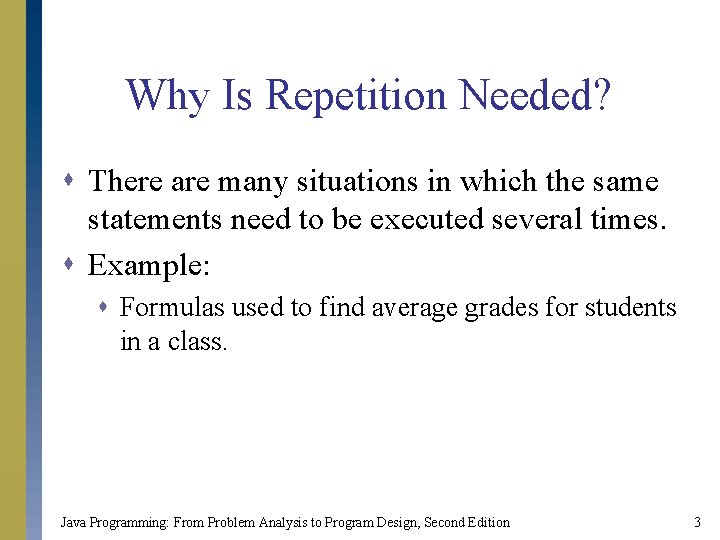
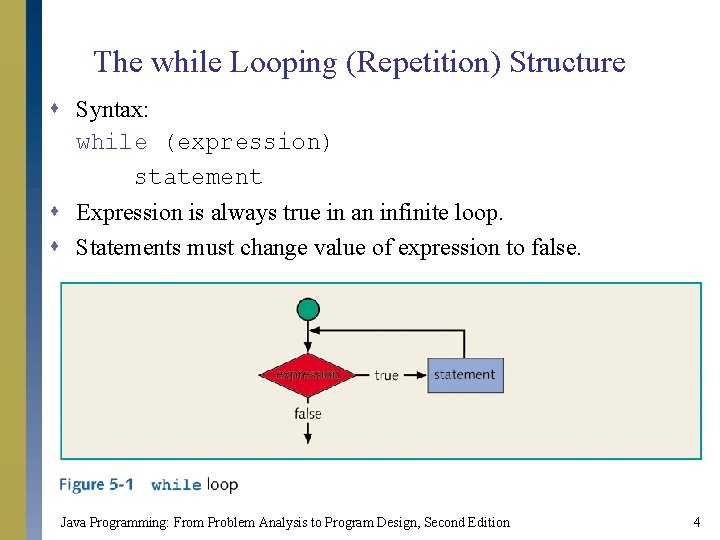
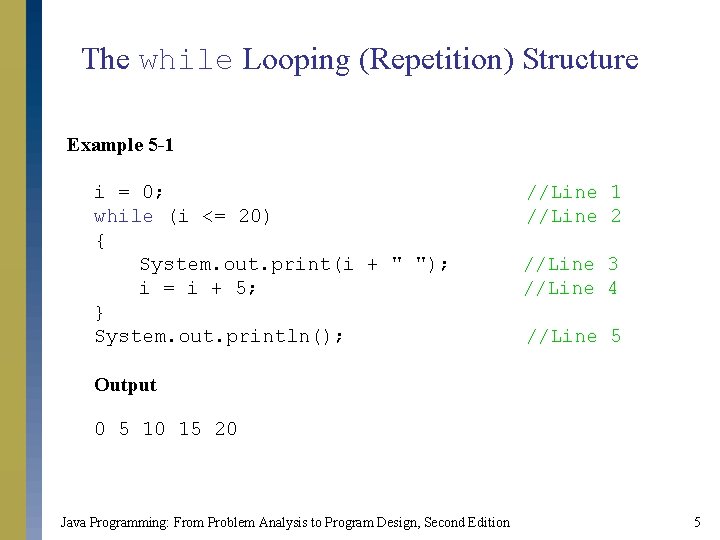
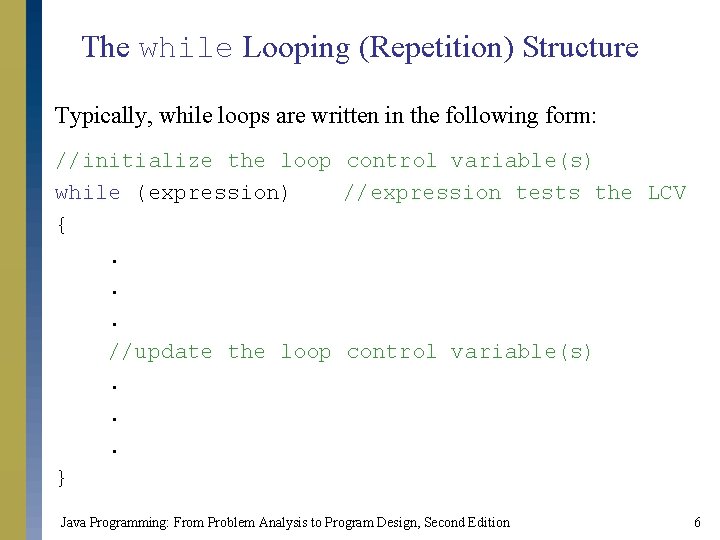
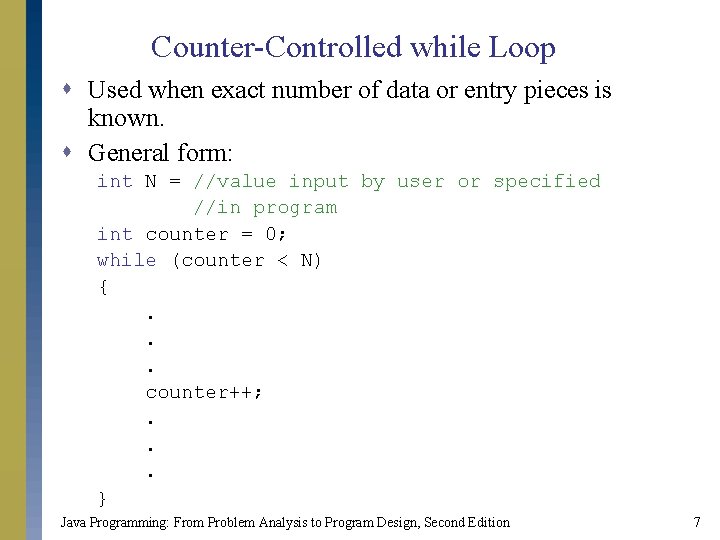
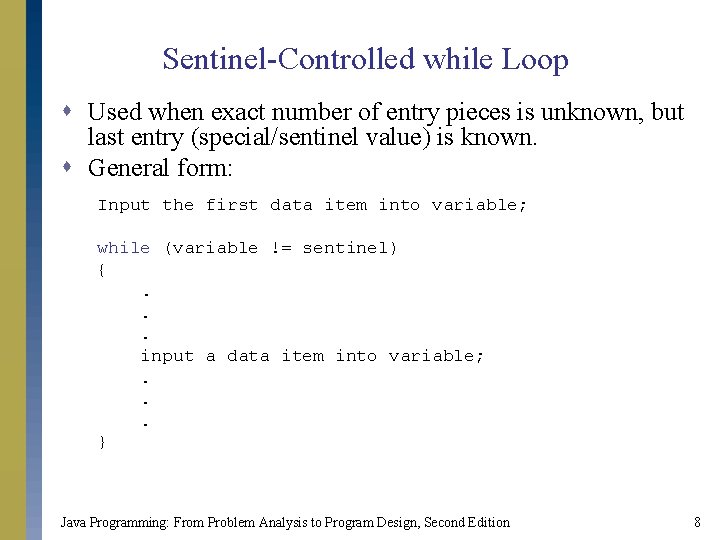
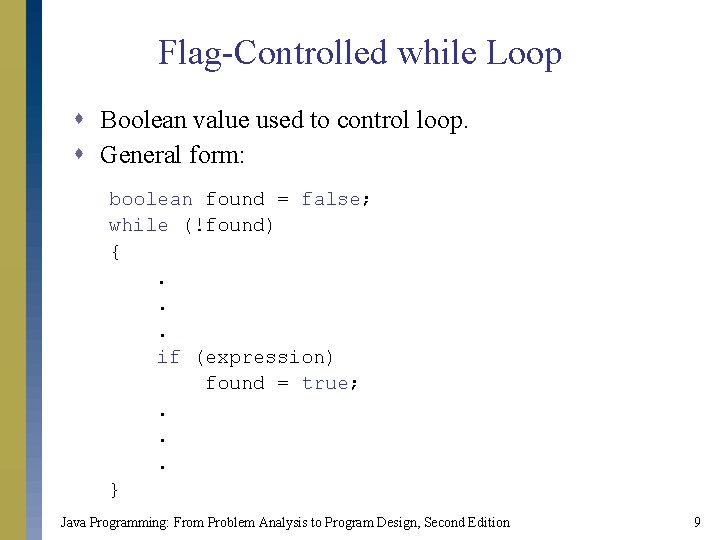
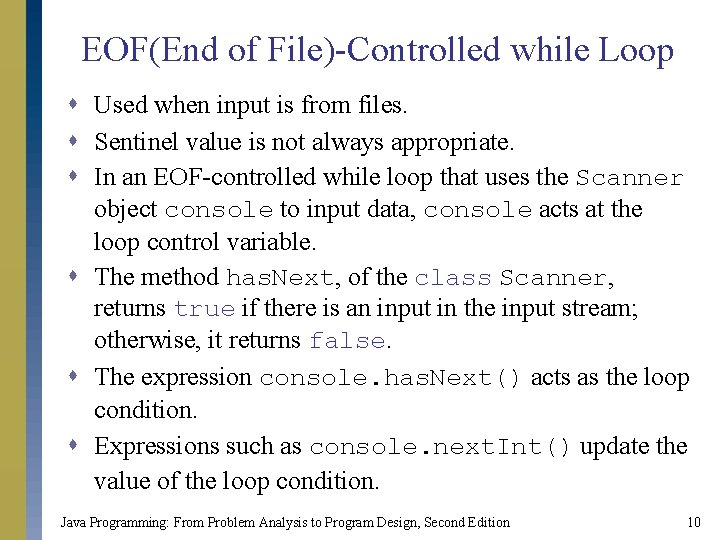
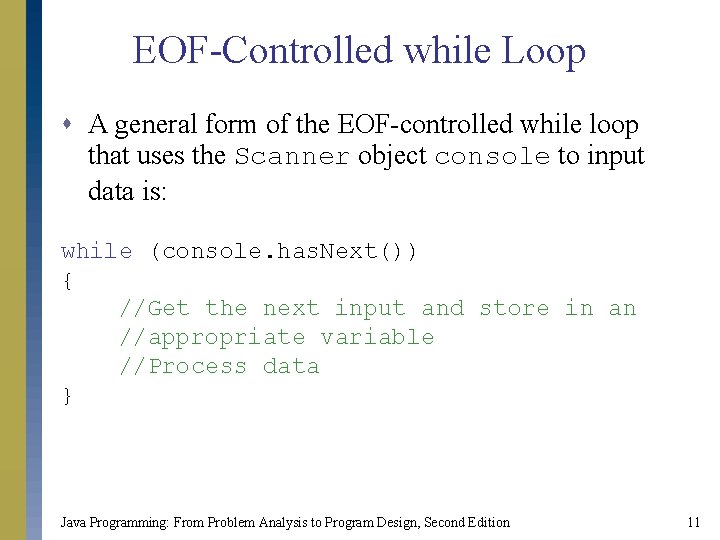
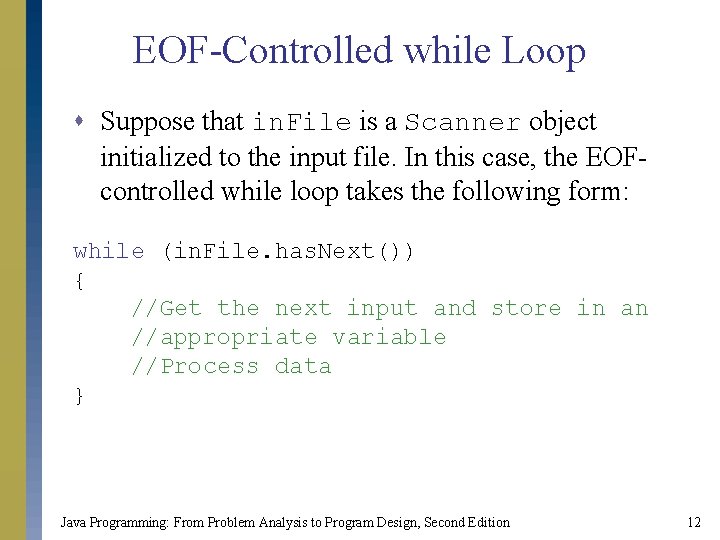
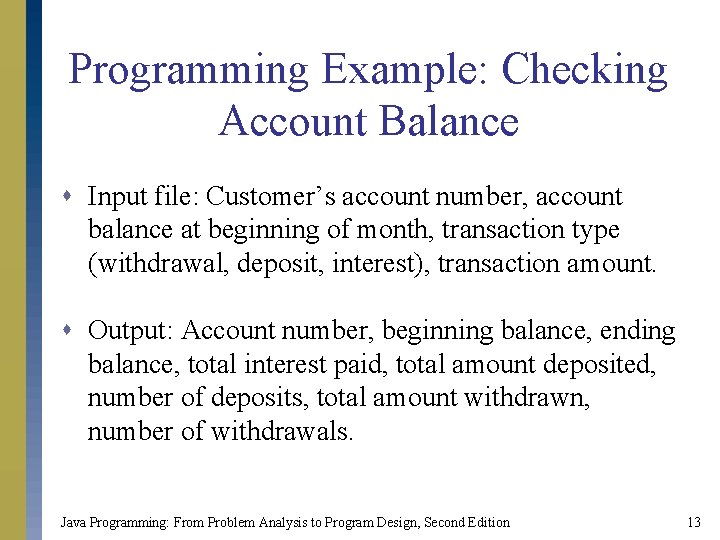
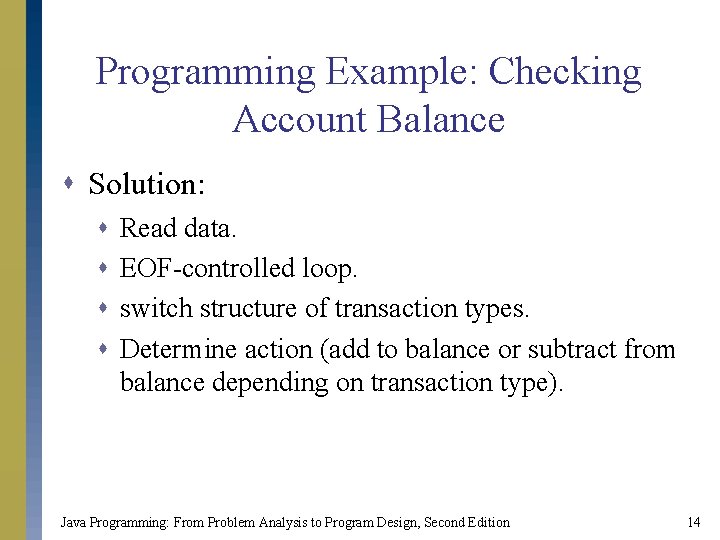
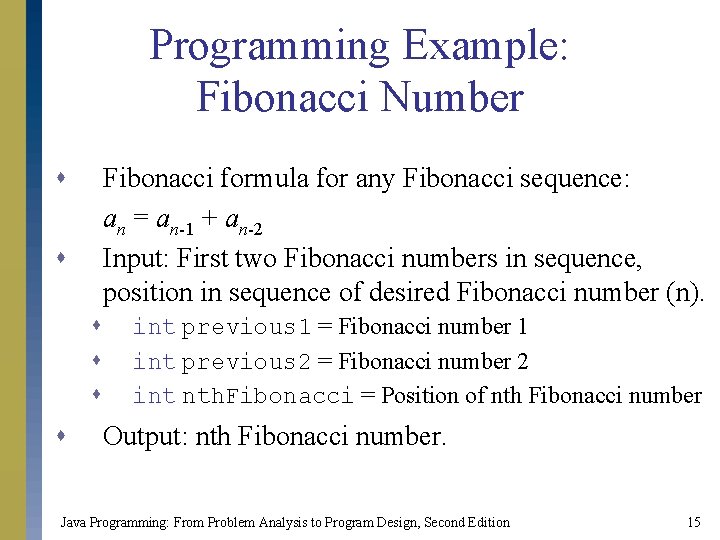
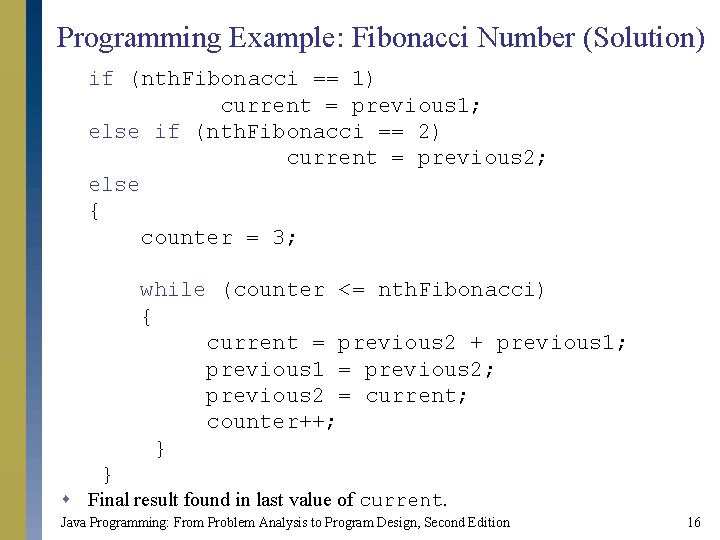
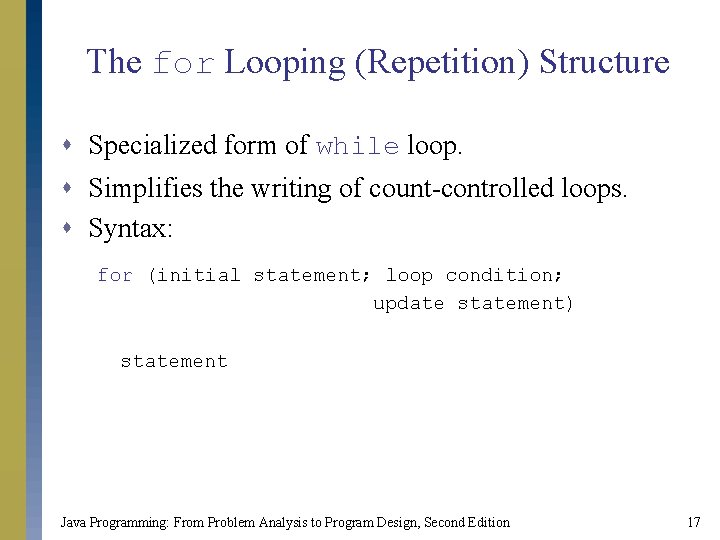
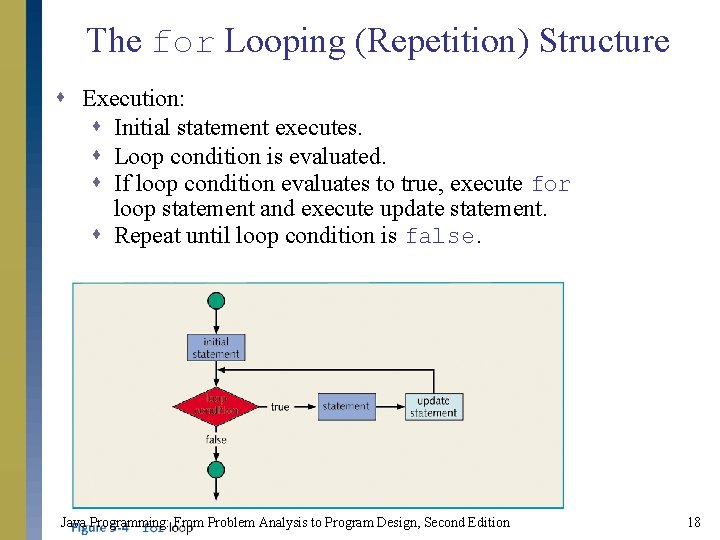
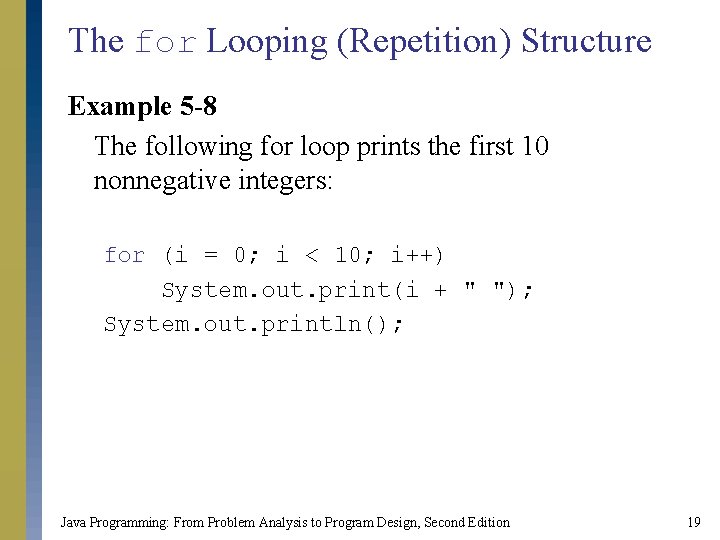
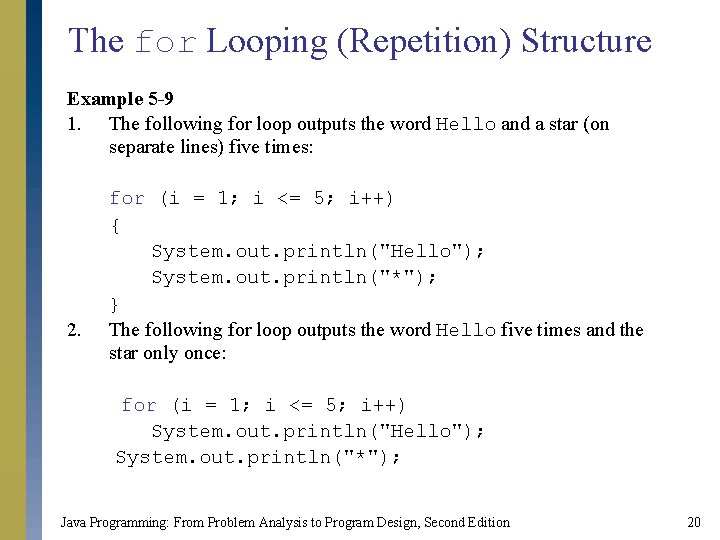
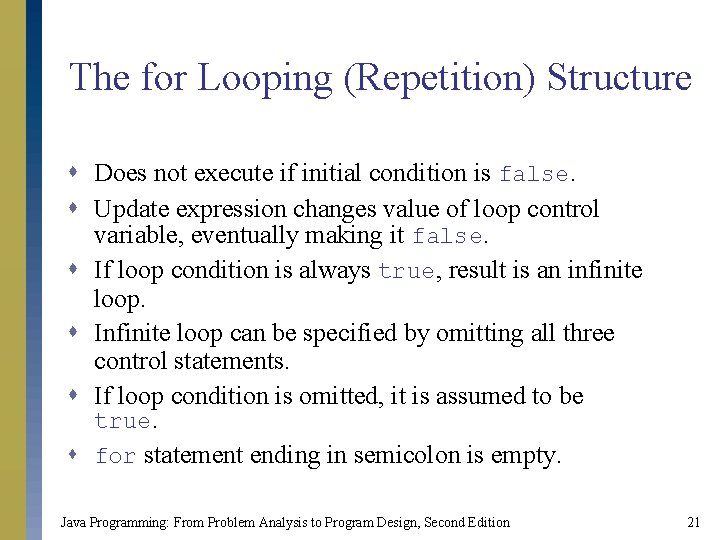
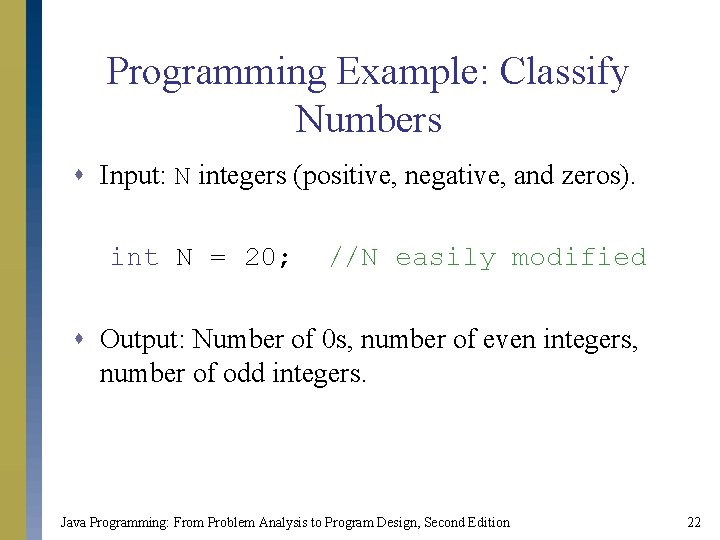
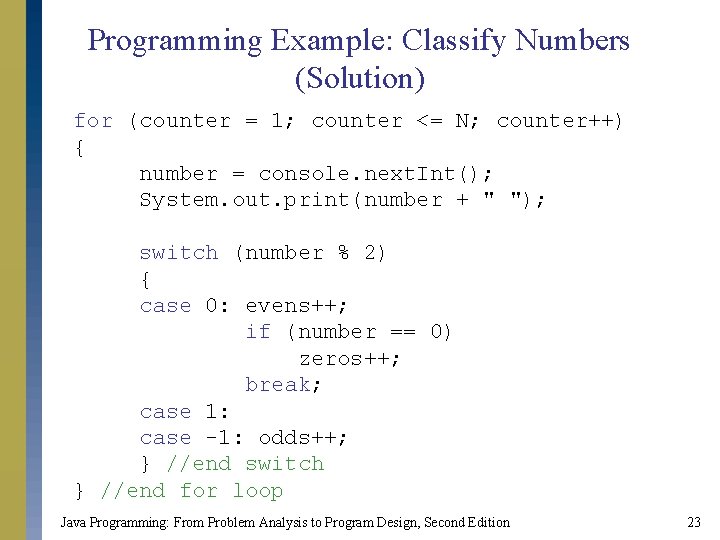
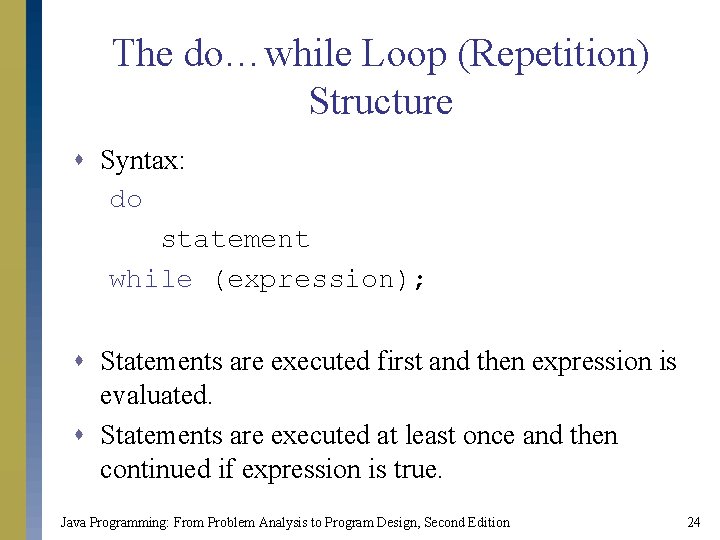
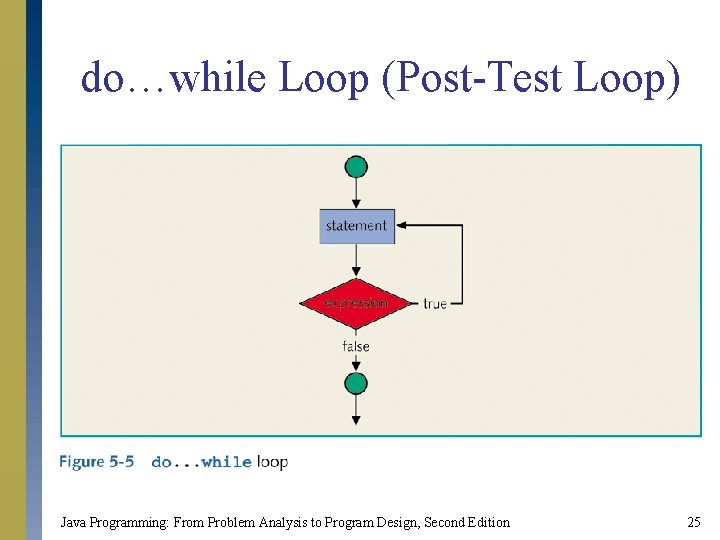
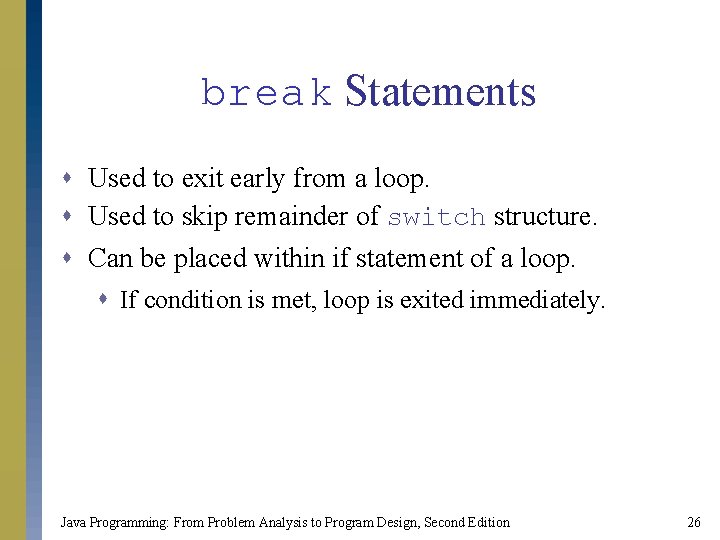
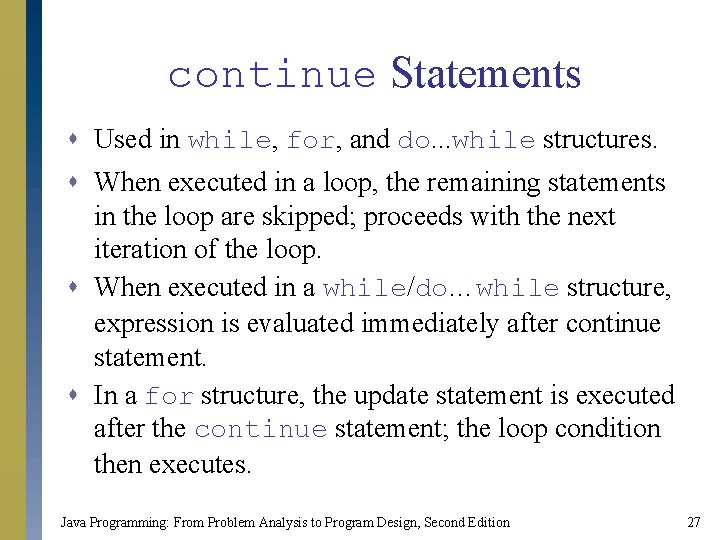
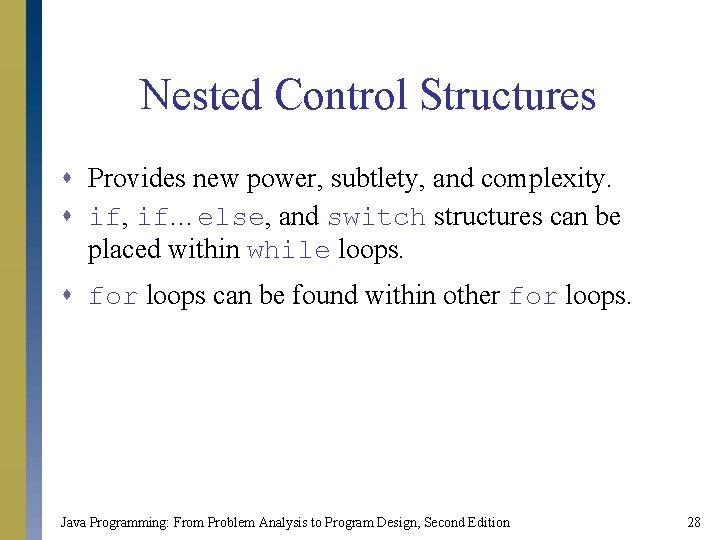
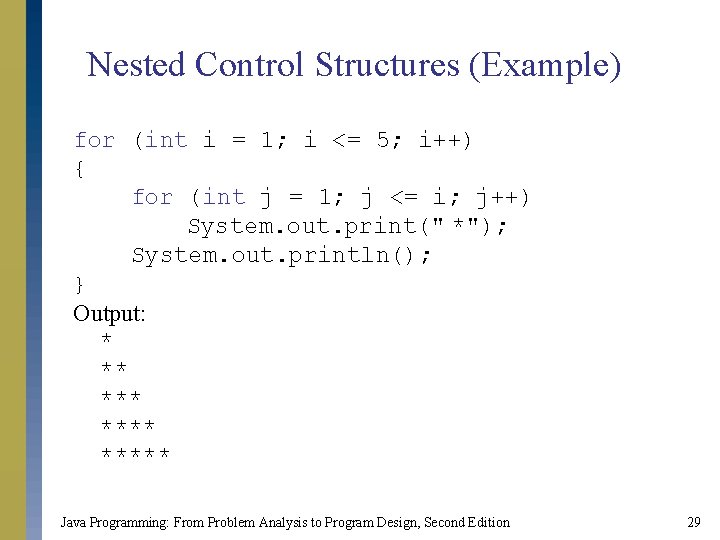
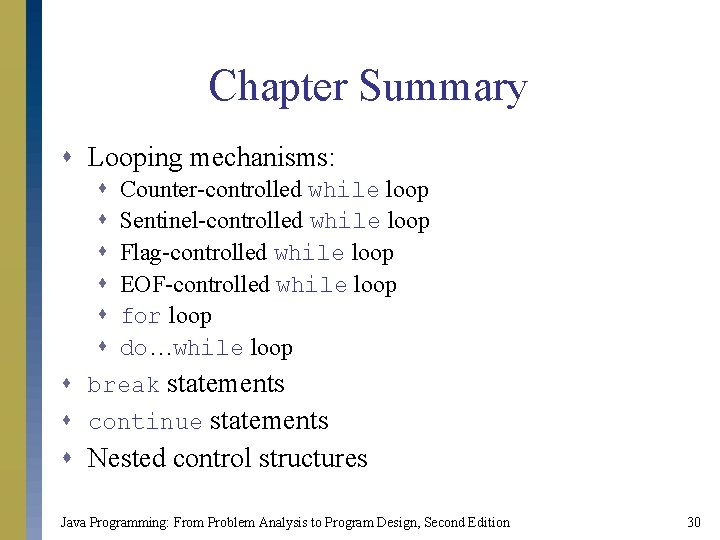
- Slides: 30
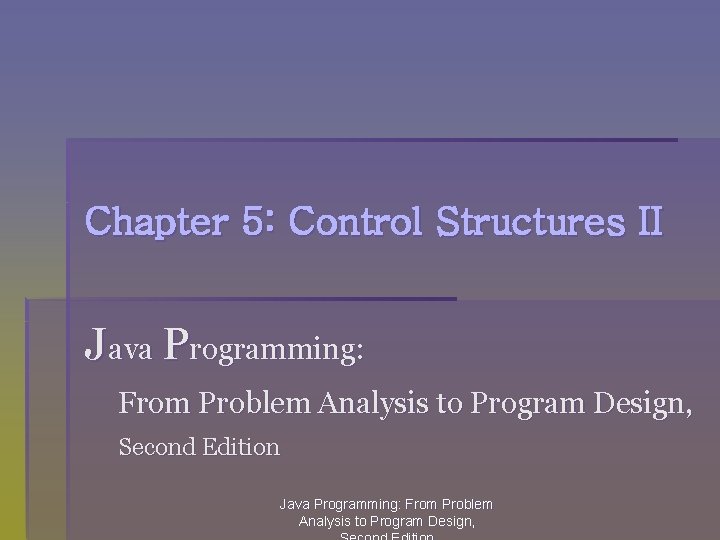
Chapter 5: Control Structures II Java Programming: From Problem Analysis to Program Design, Second Edition Java Programming: From Problem Analysis to Program Design,
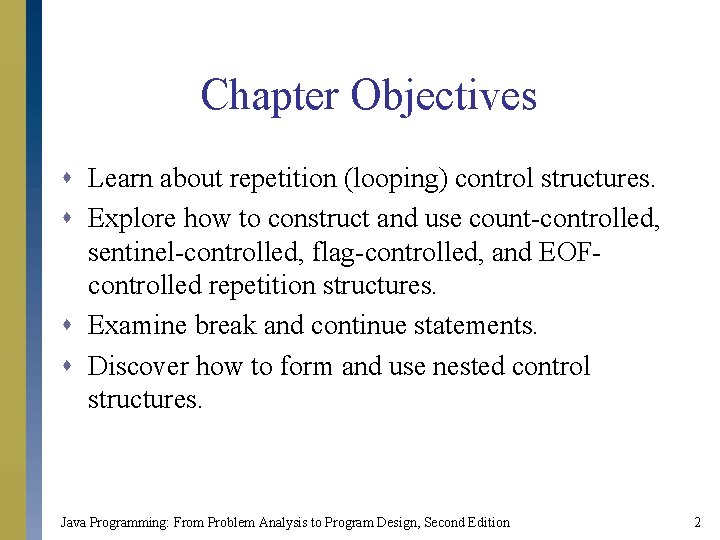
Chapter Objectives s Learn about repetition (looping) control structures. s Explore how to construct and use count-controlled, sentinel-controlled, flag-controlled, and EOFcontrolled repetition structures. s Examine break and continue statements. s Discover how to form and use nested control structures. Java Programming: From Problem Analysis to Program Design, Second Edition 2
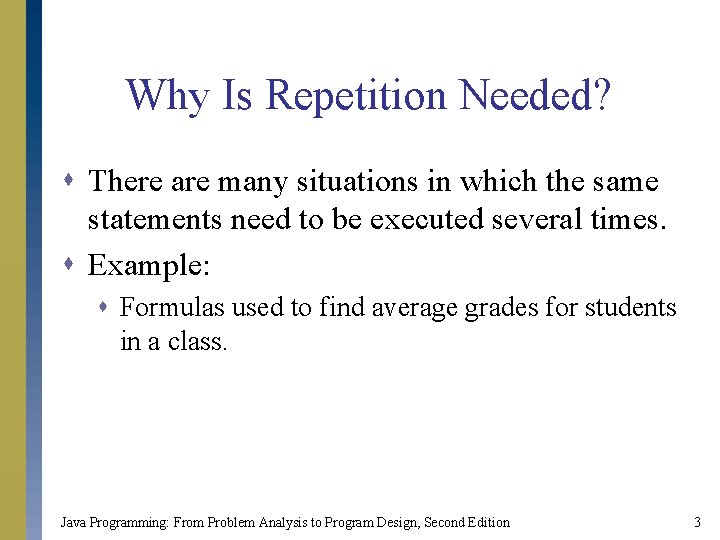
Why Is Repetition Needed? s There are many situations in which the same statements need to be executed several times. s Example: s Formulas used to find average grades for students in a class. Java Programming: From Problem Analysis to Program Design, Second Edition 3
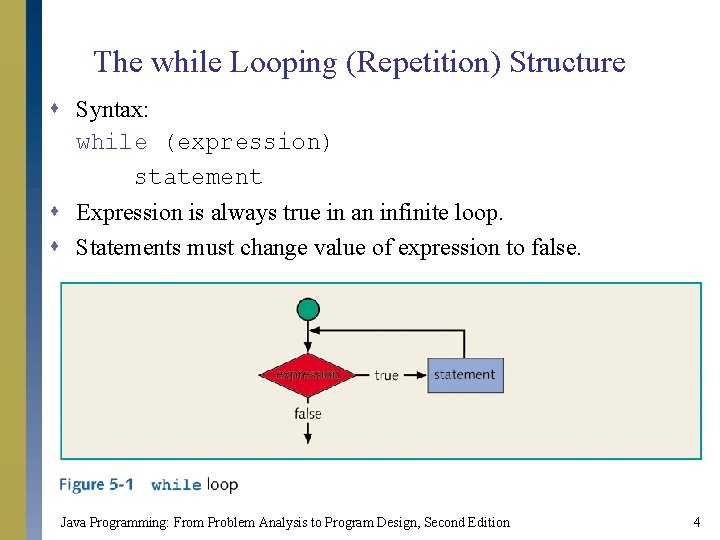
The while Looping (Repetition) Structure s Syntax: while (expression) statement s Expression is always true in an infinite loop. s Statements must change value of expression to false. Java Programming: From Problem Analysis to Program Design, Second Edition 4
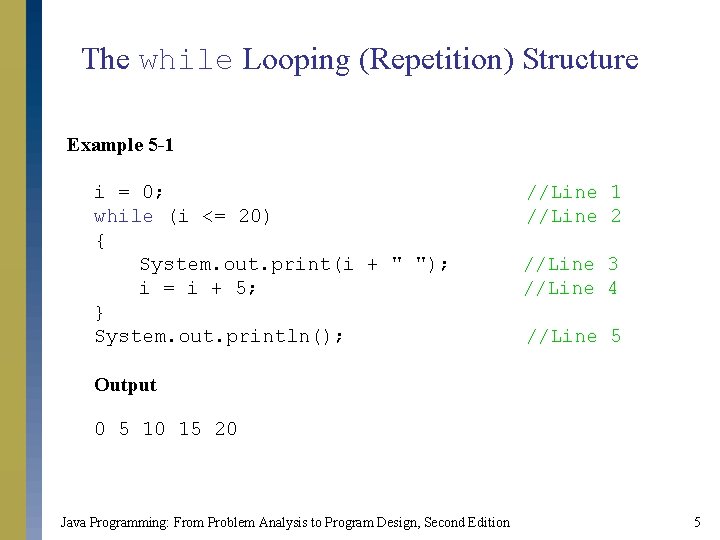
The while Looping (Repetition) Structure Example 5 -1 i = 0; while (i <= 20) { System. out. print(i + " "); i = i + 5; } System. out. println(); //Line 1 //Line 2 //Line 3 //Line 4 //Line 5 Output 0 5 10 15 20 Java Programming: From Problem Analysis to Program Design, Second Edition 5
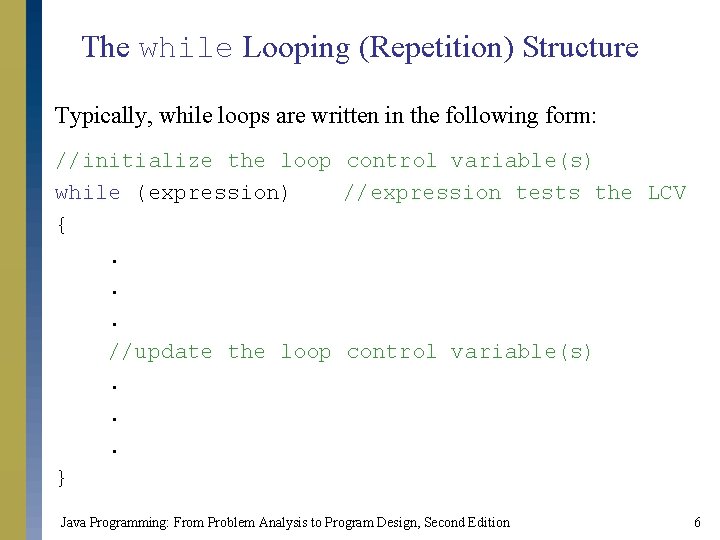
The while Looping (Repetition) Structure Typically, while loops are written in the following form: //initialize the loop control variable(s) while (expression) //expression tests the LCV {. . . //update the loop control variable(s). . . } Java Programming: From Problem Analysis to Program Design, Second Edition 6
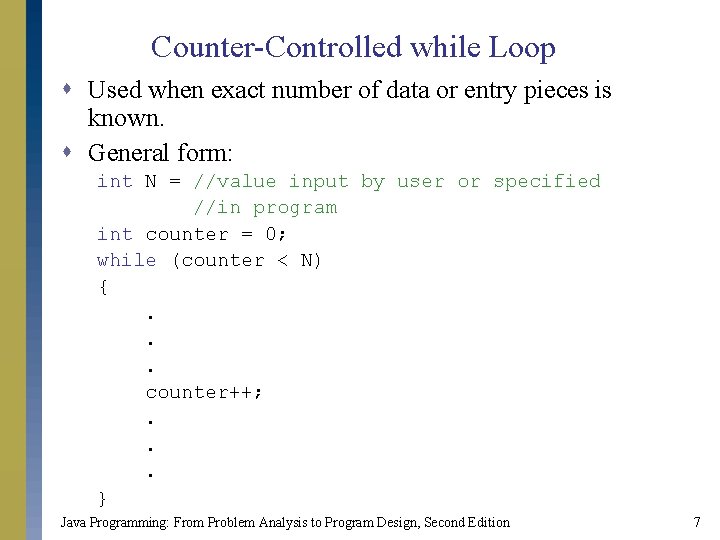
Counter-Controlled while Loop s Used when exact number of data or entry pieces is known. s General form: int N = //value input by user or specified //in program int counter = 0; while (counter < N) {. . . counter++; . . . } Java Programming: From Problem Analysis to Program Design, Second Edition 7
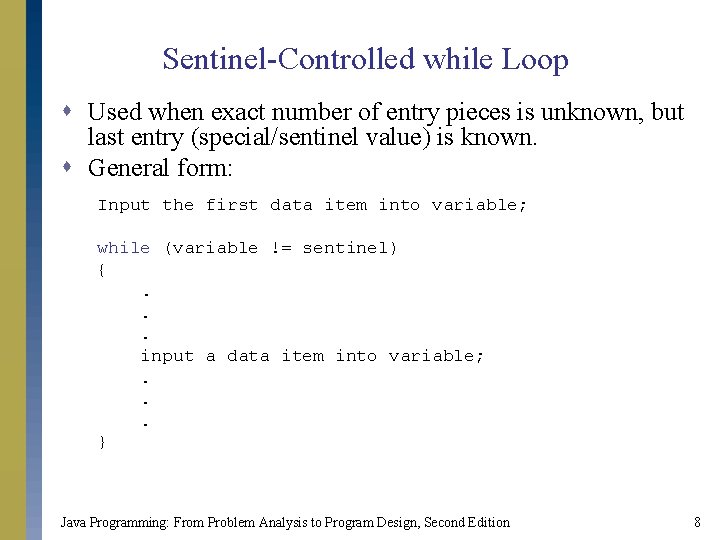
Sentinel-Controlled while Loop s Used when exact number of entry pieces is unknown, but last entry (special/sentinel value) is known. s General form: Input the first data item into variable; while (variable != sentinel) {. . . input a data item into variable; . . . } Java Programming: From Problem Analysis to Program Design, Second Edition 8
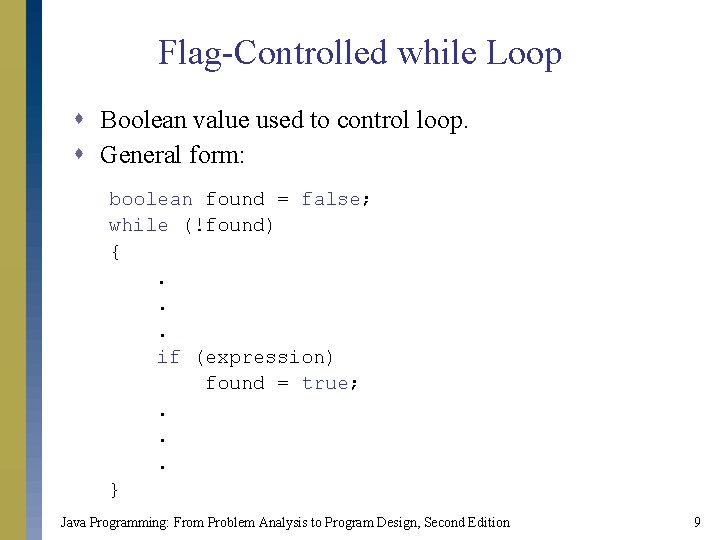
Flag-Controlled while Loop s Boolean value used to control loop. s General form: boolean found = false; while (!found) {. . . if (expression) found = true; . . . } Java Programming: From Problem Analysis to Program Design, Second Edition 9
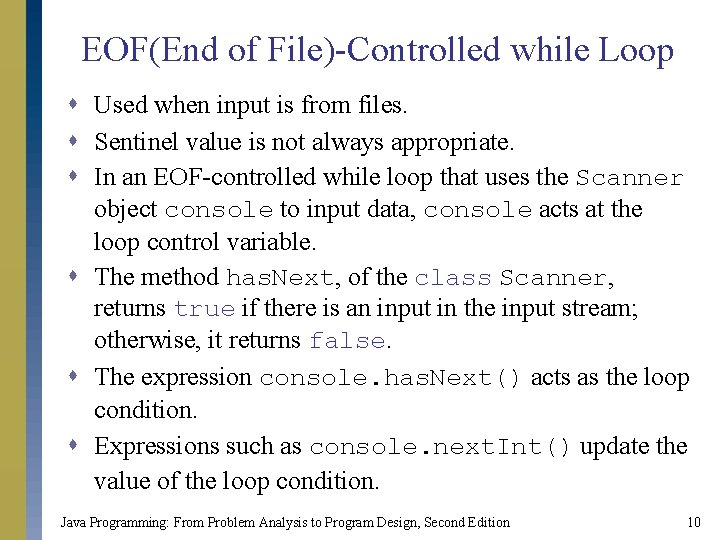
EOF(End of File)-Controlled while Loop s Used when input is from files. s Sentinel value is not always appropriate. s In an EOF-controlled while loop that uses the Scanner object console to input data, console acts at the loop control variable. s The method has. Next, of the class Scanner, returns true if there is an input in the input stream; otherwise, it returns false. s The expression console. has. Next() acts as the loop condition. s Expressions such as console. next. Int() update the value of the loop condition. Java Programming: From Problem Analysis to Program Design, Second Edition 10
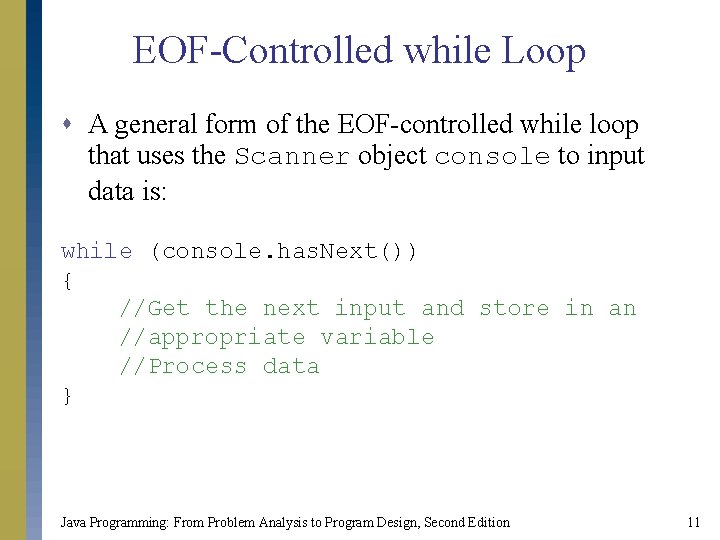
EOF-Controlled while Loop s A general form of the EOF-controlled while loop that uses the Scanner object console to input data is: while (console. has. Next()) { //Get the next input and store in an //appropriate variable //Process data } Java Programming: From Problem Analysis to Program Design, Second Edition 11
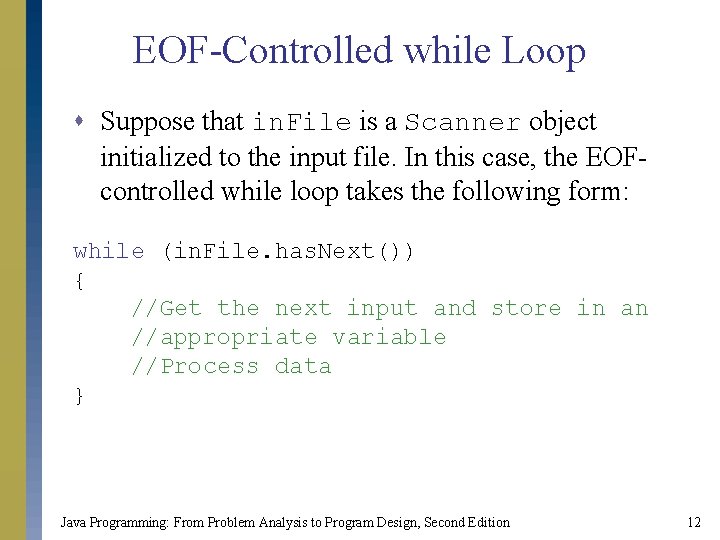
EOF-Controlled while Loop s Suppose that in. File is a Scanner object initialized to the input file. In this case, the EOFcontrolled while loop takes the following form: while (in. File. has. Next()) { //Get the next input and store in an //appropriate variable //Process data } Java Programming: From Problem Analysis to Program Design, Second Edition 12
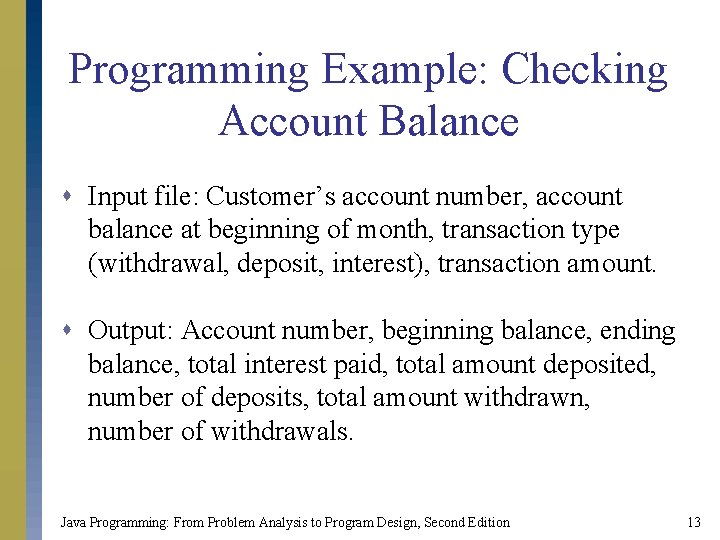
Programming Example: Checking Account Balance s Input file: Customer’s account number, account balance at beginning of month, transaction type (withdrawal, deposit, interest), transaction amount. s Output: Account number, beginning balance, ending balance, total interest paid, total amount deposited, number of deposits, total amount withdrawn, number of withdrawals. Java Programming: From Problem Analysis to Program Design, Second Edition 13
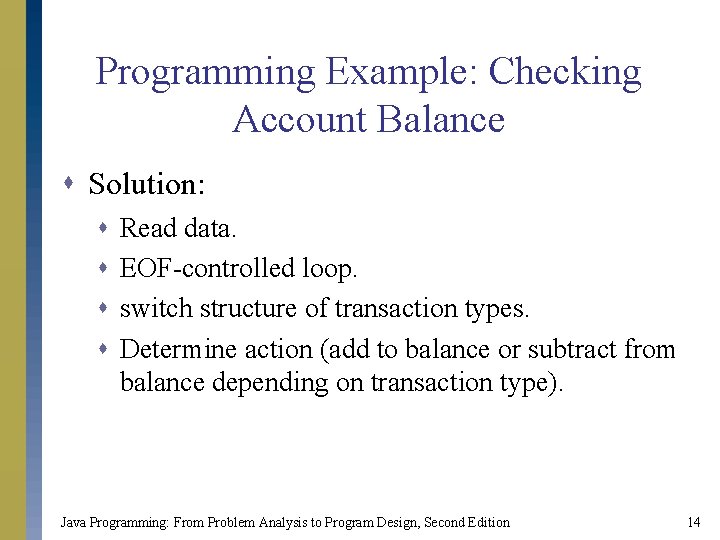
Programming Example: Checking Account Balance s Solution: s s Read data. EOF-controlled loop. switch structure of transaction types. Determine action (add to balance or subtract from balance depending on transaction type). Java Programming: From Problem Analysis to Program Design, Second Edition 14
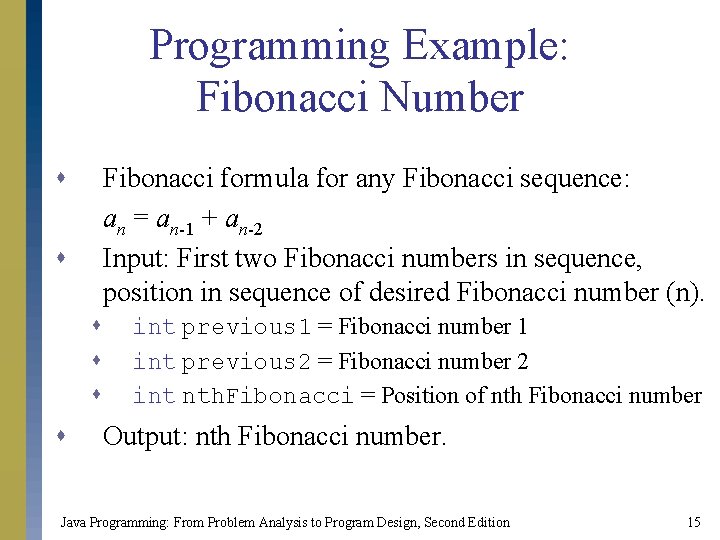
Programming Example: Fibonacci Number s Fibonacci formula for any Fibonacci sequence: an = an-1 + an-2 Input: First two Fibonacci numbers in sequence, position in sequence of desired Fibonacci number (n). s s s int previous 1 = Fibonacci number 1 int previous 2 = Fibonacci number 2 int nth. Fibonacci = Position of nth Fibonacci number Output: nth Fibonacci number. Java Programming: From Problem Analysis to Program Design, Second Edition 15
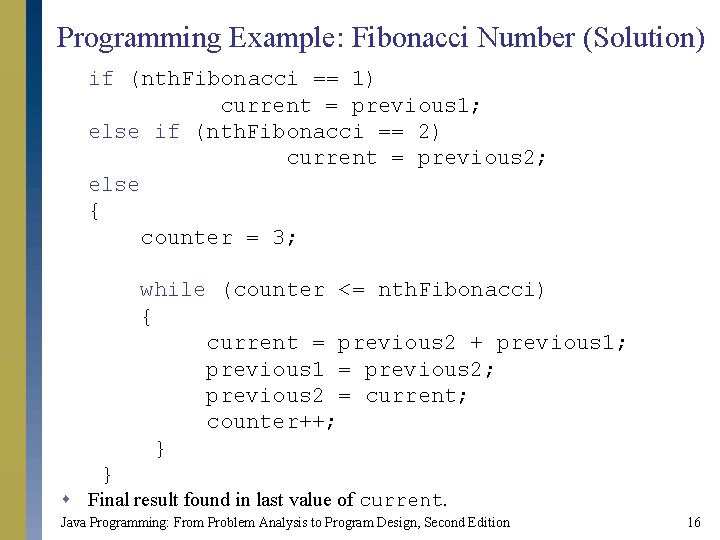
Programming Example: Fibonacci Number (Solution) if (nth. Fibonacci == 1) current = previous 1; else if (nth. Fibonacci == 2) current = previous 2; else { counter = 3; while (counter <= nth. Fibonacci) { current = previous 2 + previous 1; previous 1 = previous 2; previous 2 = current; counter++; } } s Final result found in last value of current. Java Programming: From Problem Analysis to Program Design, Second Edition 16
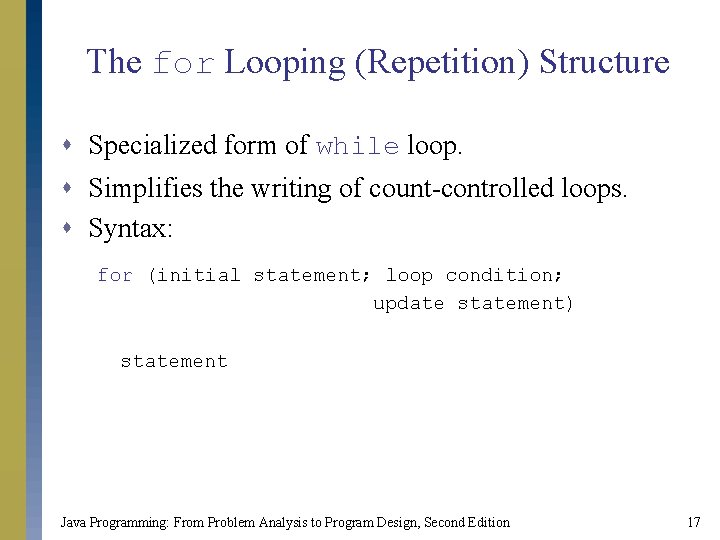
The for Looping (Repetition) Structure s Specialized form of while loop. s Simplifies the writing of count-controlled loops. s Syntax: for (initial statement; loop condition; update statement) statement Java Programming: From Problem Analysis to Program Design, Second Edition 17
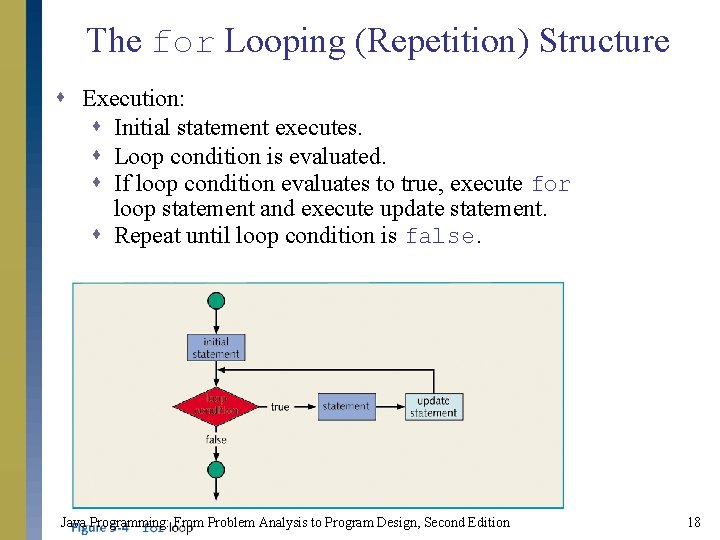
The for Looping (Repetition) Structure s Execution: s Initial statement executes. s Loop condition is evaluated. s If loop condition evaluates to true, execute for loop statement and execute update statement. s Repeat until loop condition is false. Java Programming: From Problem Analysis to Program Design, Second Edition 18
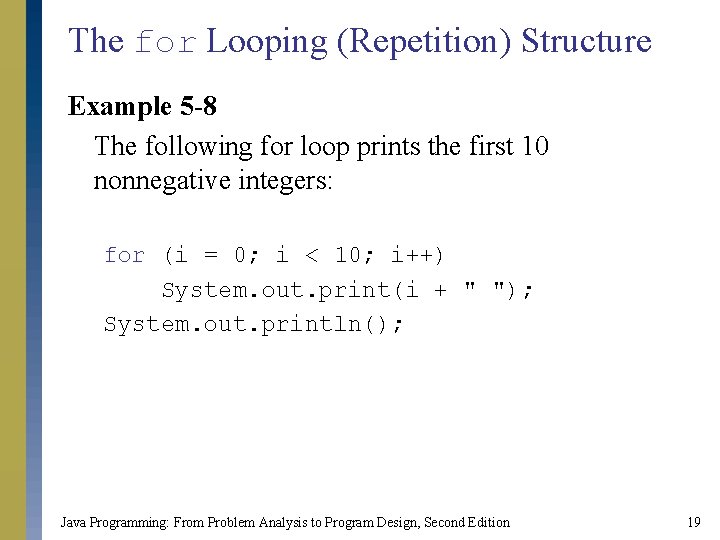
The for Looping (Repetition) Structure Example 5 -8 The following for loop prints the first 10 nonnegative integers: for (i = 0; i < 10; i++) System. out. print(i + " "); System. out. println(); Java Programming: From Problem Analysis to Program Design, Second Edition 19
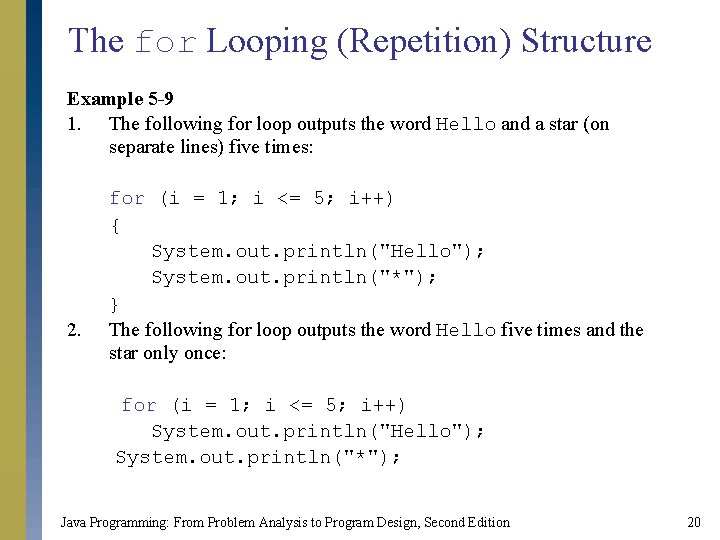
The for Looping (Repetition) Structure Example 5 -9 1. The following for loop outputs the word Hello and a star (on separate lines) five times: 2. for (i = 1; i <= 5; i++) { System. out. println("Hello"); System. out. println("*"); } The following for loop outputs the word Hello five times and the star only once: for (i = 1; i <= 5; i++) System. out. println("Hello"); System. out. println("*"); Java Programming: From Problem Analysis to Program Design, Second Edition 20
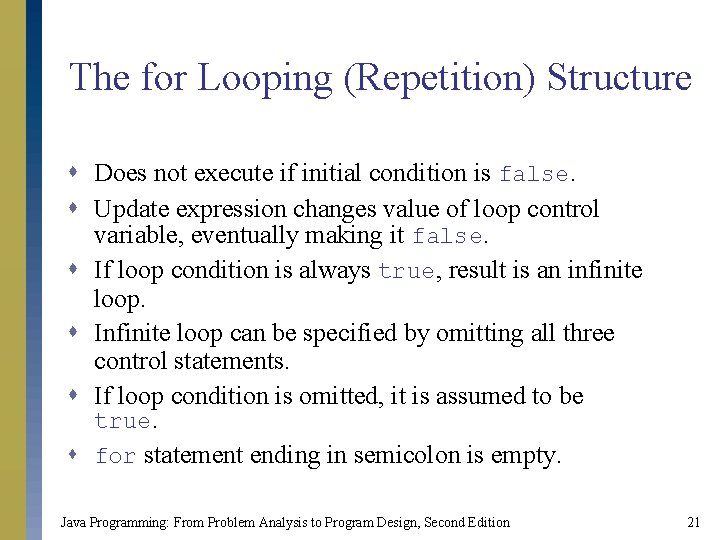
The for Looping (Repetition) Structure s Does not execute if initial condition is false. s Update expression changes value of loop control variable, eventually making it false. s If loop condition is always true, result is an infinite loop. s Infinite loop can be specified by omitting all three control statements. s If loop condition is omitted, it is assumed to be true. s for statement ending in semicolon is empty. Java Programming: From Problem Analysis to Program Design, Second Edition 21
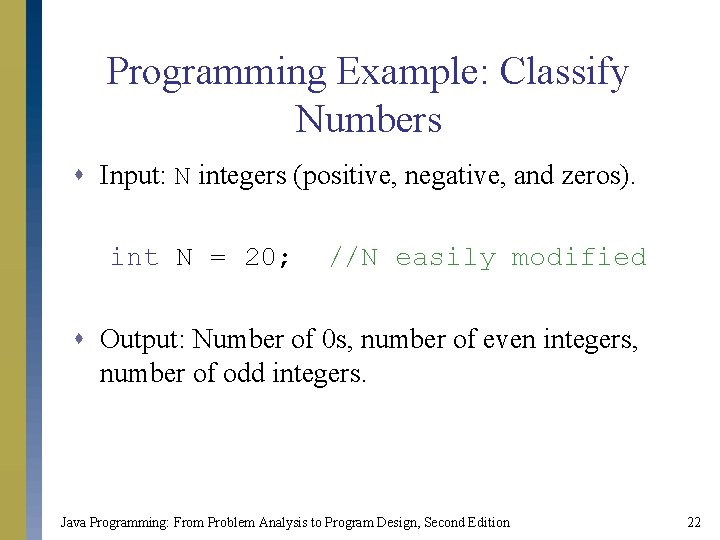
Programming Example: Classify Numbers s Input: N integers (positive, negative, and zeros). int N = 20; //N easily modified s Output: Number of 0 s, number of even integers, number of odd integers. Java Programming: From Problem Analysis to Program Design, Second Edition 22
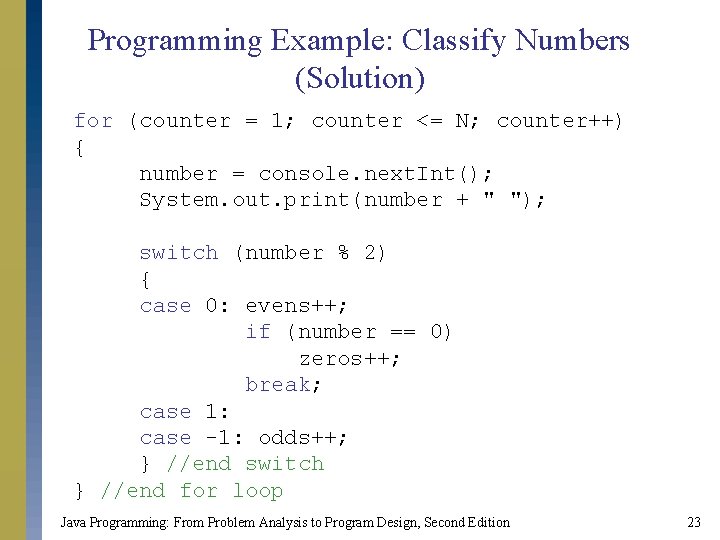
Programming Example: Classify Numbers (Solution) for (counter = 1; counter <= N; counter++) { number = console. next. Int(); System. out. print(number + " "); switch (number % 2) { case 0: evens++; if (number == 0) zeros++; break; case 1: case -1: odds++; } //end switch } //end for loop Java Programming: From Problem Analysis to Program Design, Second Edition 23
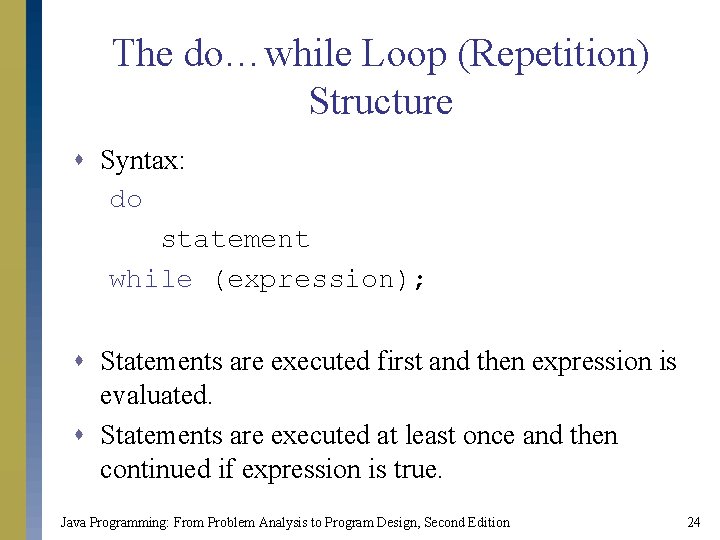
The do…while Loop (Repetition) Structure s Syntax: do statement while (expression); s Statements are executed first and then expression is evaluated. s Statements are executed at least once and then continued if expression is true. Java Programming: From Problem Analysis to Program Design, Second Edition 24
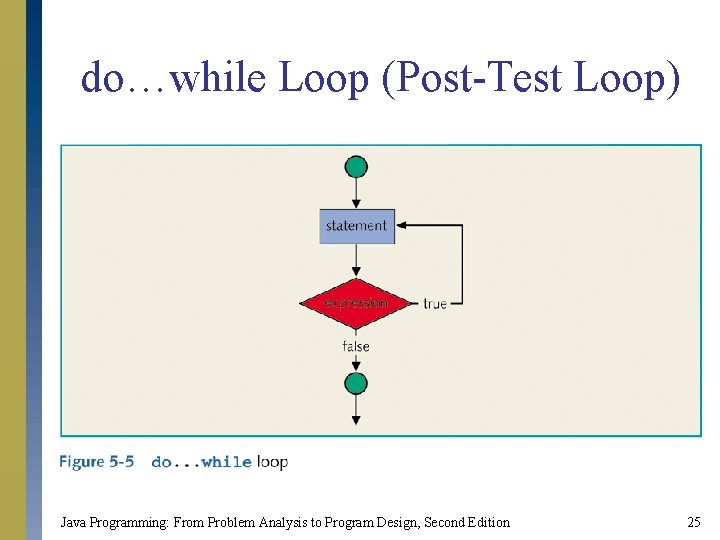
do…while Loop (Post-Test Loop) Java Programming: From Problem Analysis to Program Design, Second Edition 25
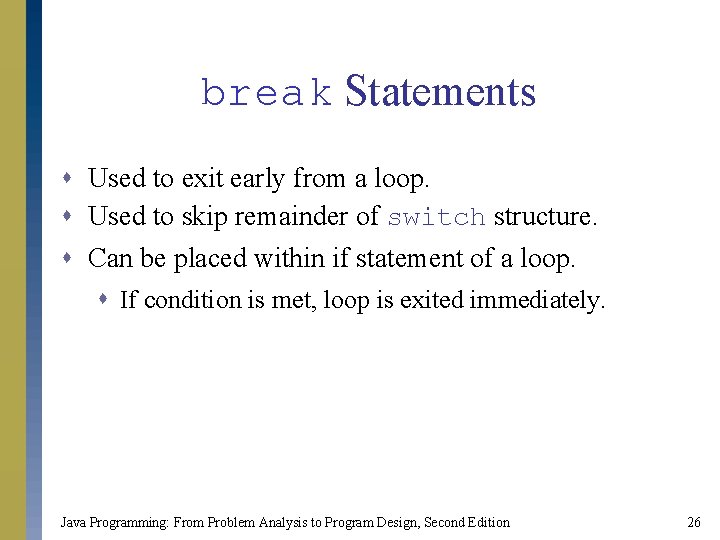
break Statements s Used to exit early from a loop. s Used to skip remainder of switch structure. s Can be placed within if statement of a loop. s If condition is met, loop is exited immediately. Java Programming: From Problem Analysis to Program Design, Second Edition 26
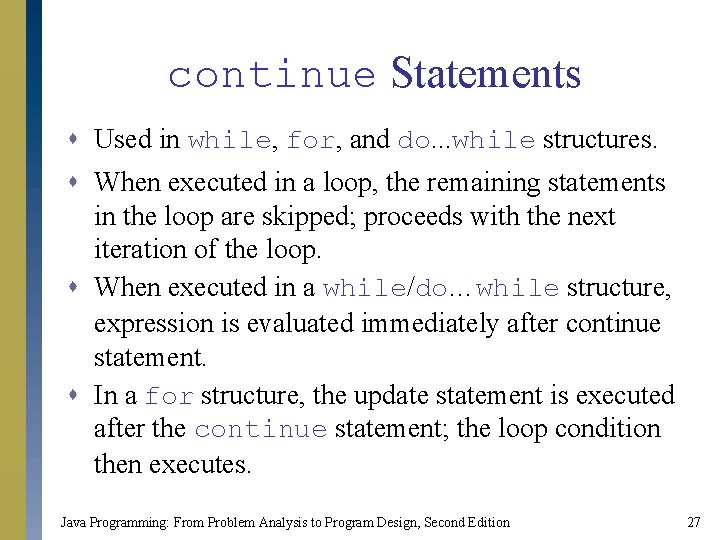
continue Statements s Used in while, for, and do. . . while structures. s When executed in a loop, the remaining statements in the loop are skipped; proceeds with the next iteration of the loop. s When executed in a while/do…while structure, expression is evaluated immediately after continue statement. s In a for structure, the update statement is executed after the continue statement; the loop condition then executes. Java Programming: From Problem Analysis to Program Design, Second Edition 27
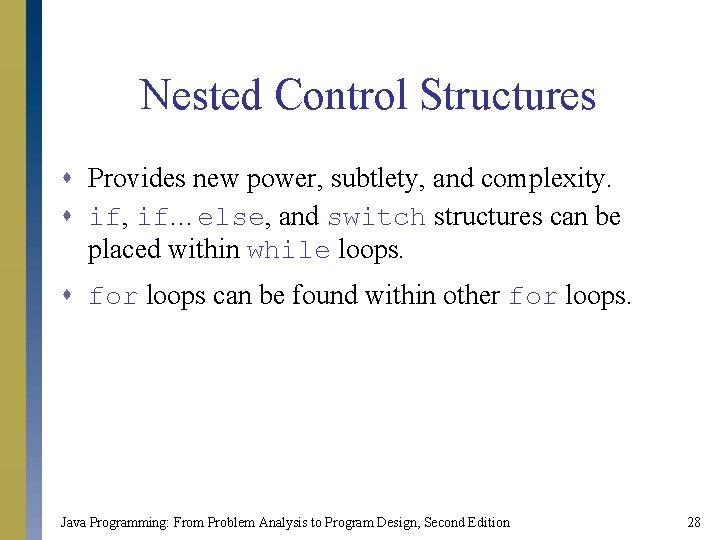
Nested Control Structures s Provides new power, subtlety, and complexity. s if, if…else, and switch structures can be placed within while loops. s for loops can be found within other for loops. Java Programming: From Problem Analysis to Program Design, Second Edition 28
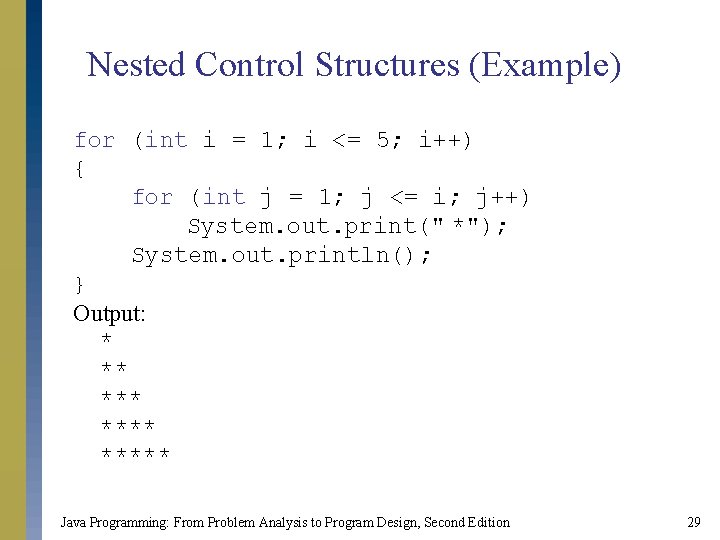
Nested Control Structures (Example) for (int i = 1; i <= 5; i++) { for (int j = 1; j <= i; j++) System. out. print(" *"); System. out. println(); } Output: * ** ***** Java Programming: From Problem Analysis to Program Design, Second Edition 29
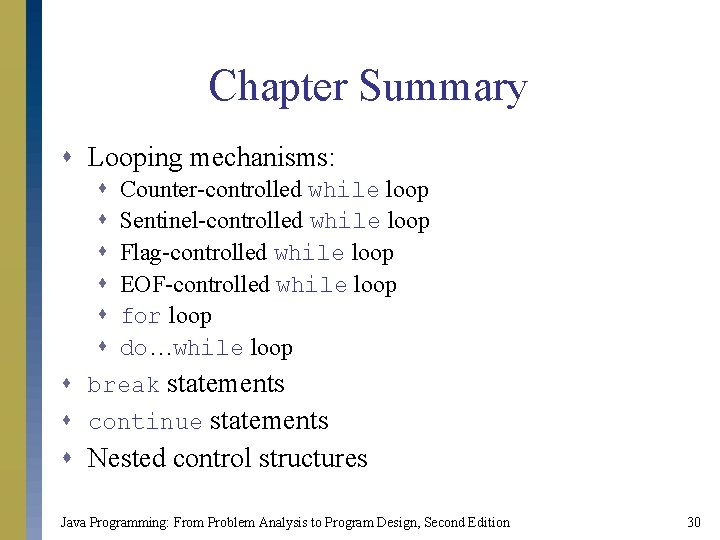
Chapter Summary s Looping mechanisms: s s s Counter-controlled while loop Sentinel-controlled while loop Flag-controlled while loop EOF-controlled while loop for loop do…while loop s break statements s continue statements s Nested control structures Java Programming: From Problem Analysis to Program Design, Second Edition 30