Java Applets A First Program Applet Example The
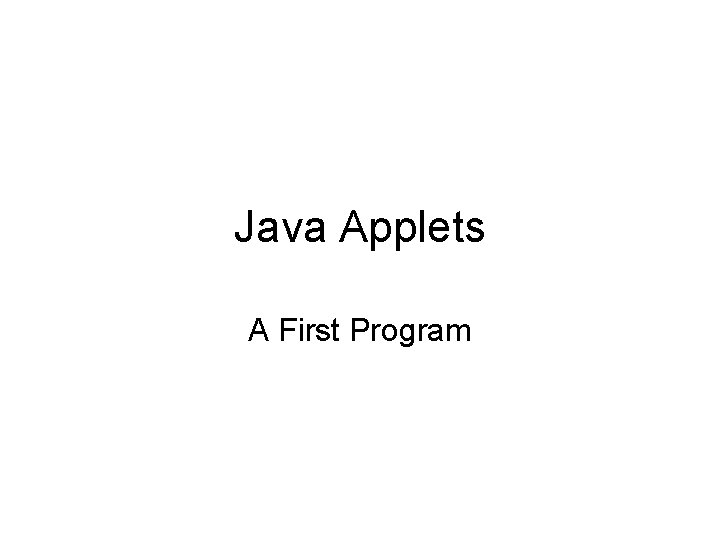
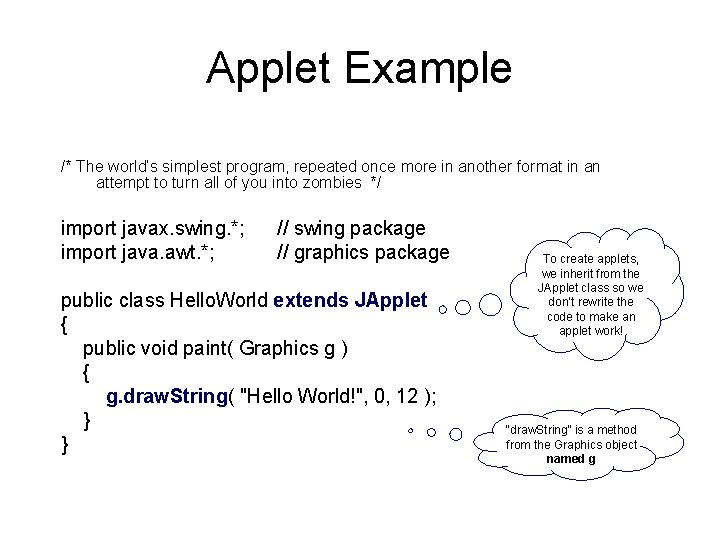
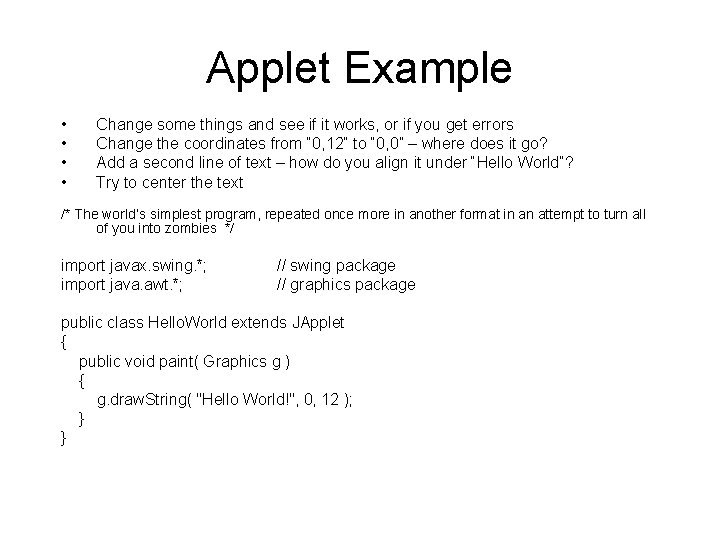
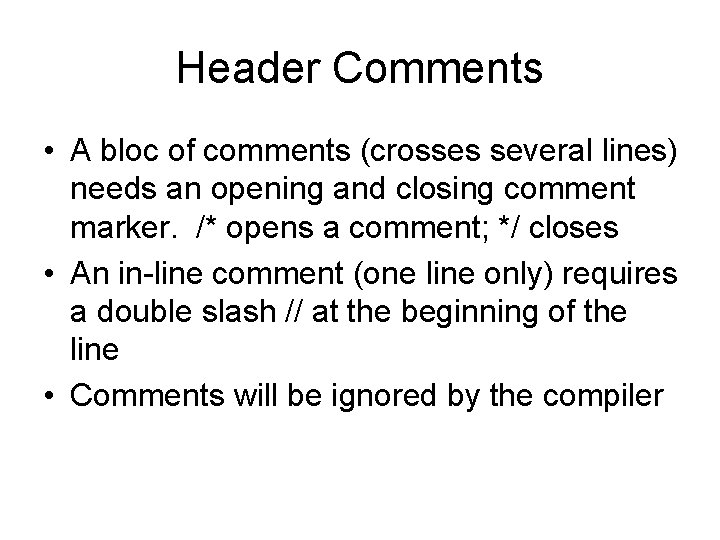
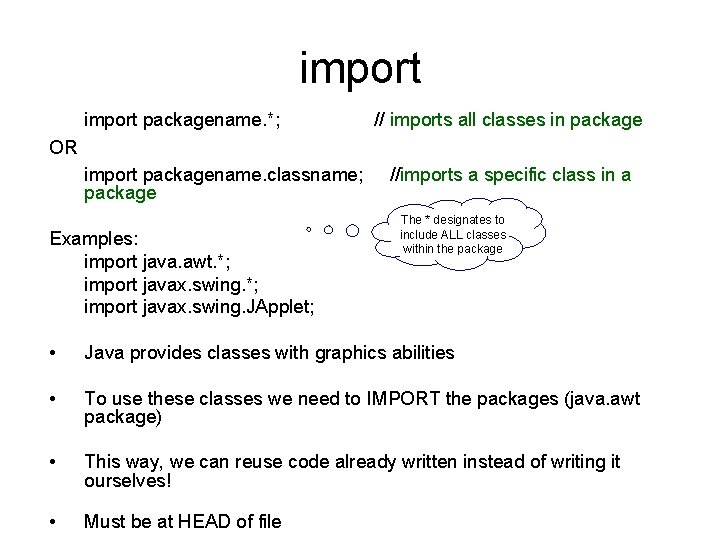
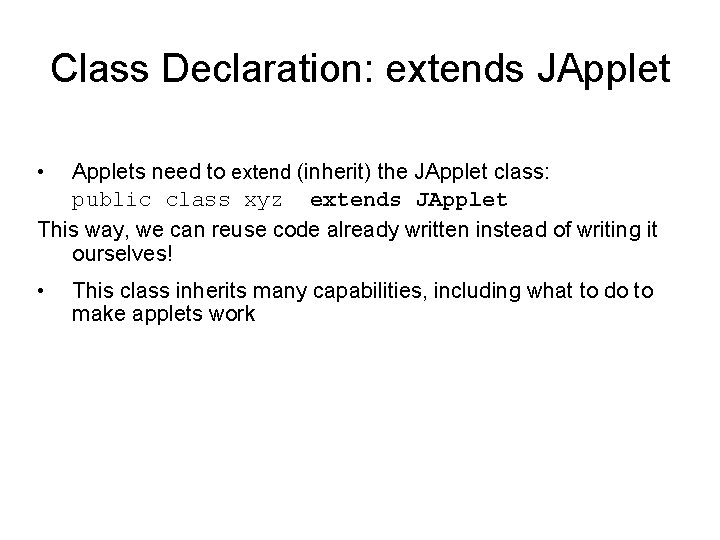
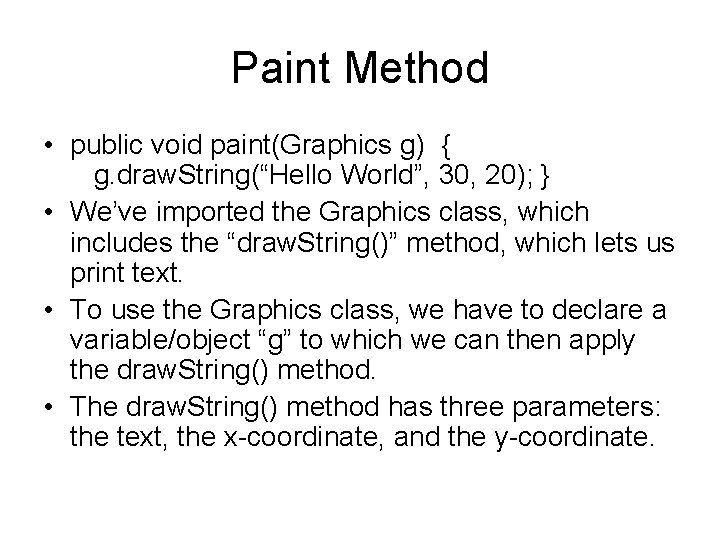
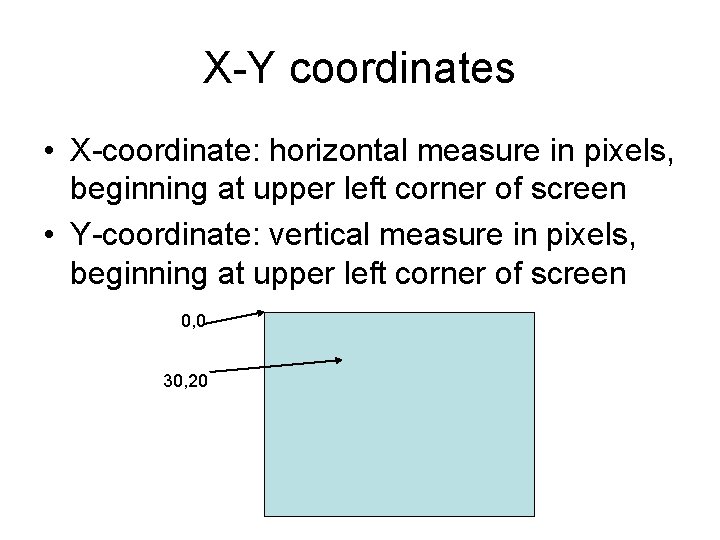
- Slides: 8
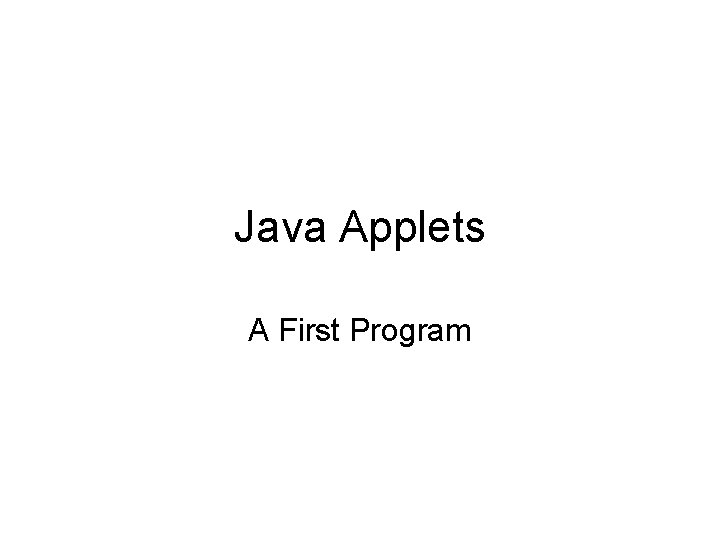
Java Applets A First Program
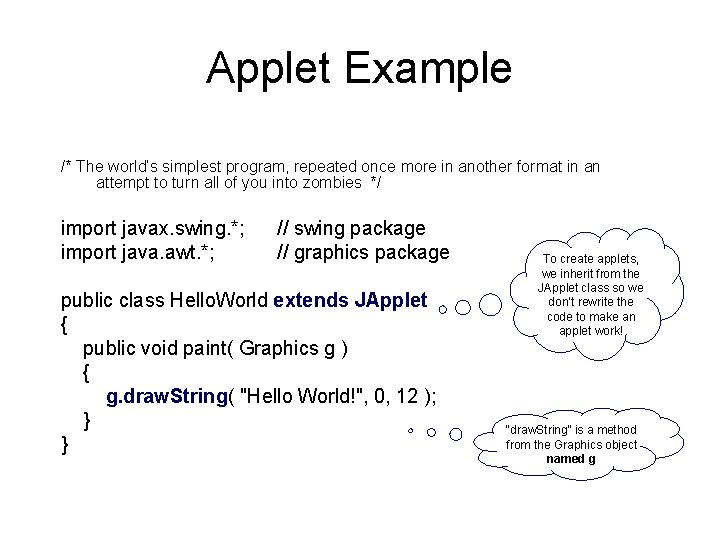
Applet Example /* The world’s simplest program, repeated once more in another format in an attempt to turn all of you into zombies */ import javax. swing. *; import java. awt. *; // swing package // graphics package public class Hello. World extends JApplet { public void paint( Graphics g ) { g. draw. String( "Hello World!", 0, 12 ); } } To create applets, we inherit from the JApplet class so we don’t rewrite the code to make an applet work! “draw. String” is a method from the Graphics object named g
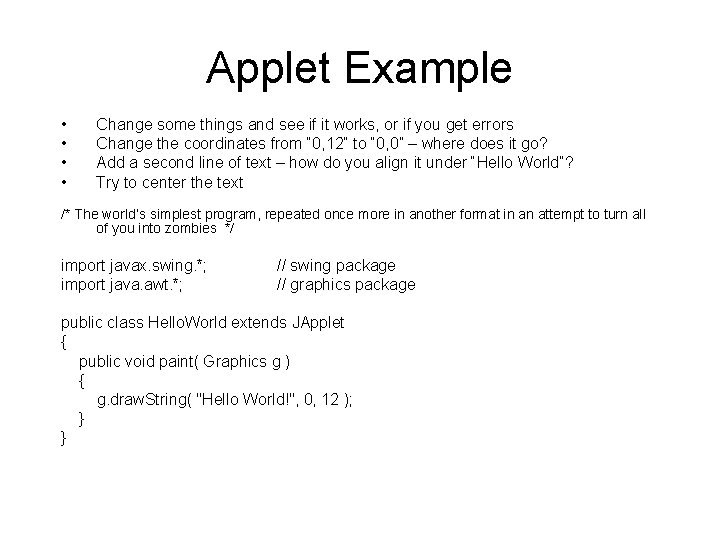
Applet Example • • Change some things and see if it works, or if you get errors Change the coordinates from “ 0, 12” to “ 0, 0” – where does it go? Add a second line of text – how do you align it under “Hello World”? Try to center the text /* The world’s simplest program, repeated once more in another format in an attempt to turn all of you into zombies */ import javax. swing. *; import java. awt. *; // swing package // graphics package public class Hello. World extends JApplet { public void paint( Graphics g ) { g. draw. String( "Hello World!", 0, 12 ); } }
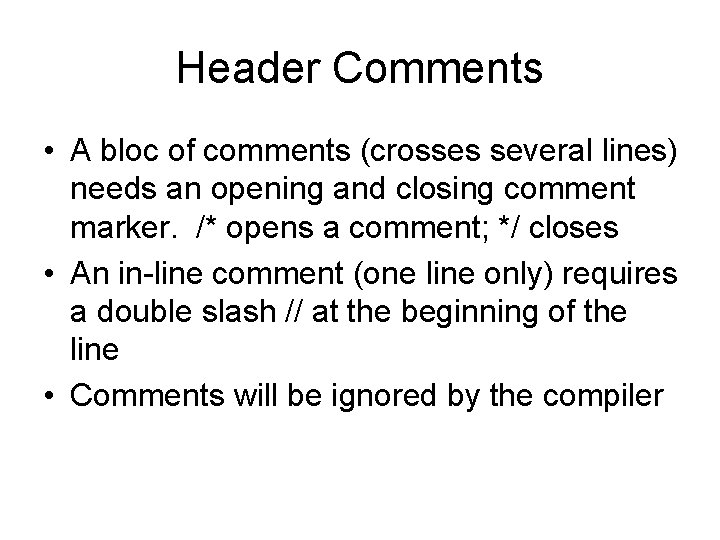
Header Comments • A bloc of comments (crosses several lines) needs an opening and closing comment marker. /* opens a comment; */ closes • An in-line comment (one line only) requires a double slash // at the beginning of the line • Comments will be ignored by the compiler
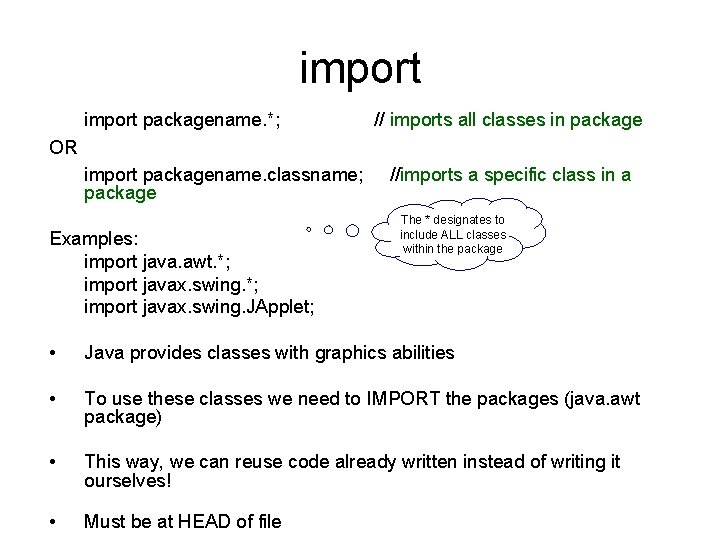
import packagename. *; // imports all classes in package OR import packagename. classname; package Examples: import java. awt. *; import javax. swing. JApplet; //imports a specific class in a The * designates to include ALL classes within the package • Java provides classes with graphics abilities • To use these classes we need to IMPORT the packages (java. awt package) • This way, we can reuse code already written instead of writing it ourselves! • Must be at HEAD of file
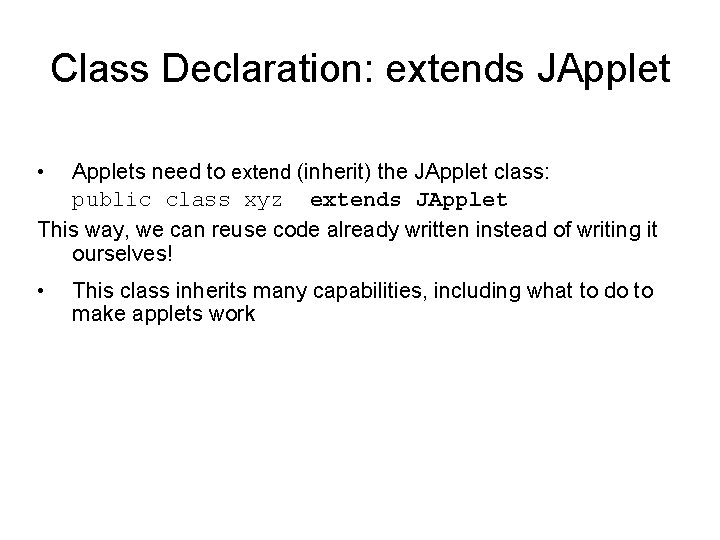
Class Declaration: extends JApplet • Applets need to extend (inherit) the JApplet class: public class xyz extends JApplet This way, we can reuse code already written instead of writing it ourselves! • This class inherits many capabilities, including what to do to make applets work
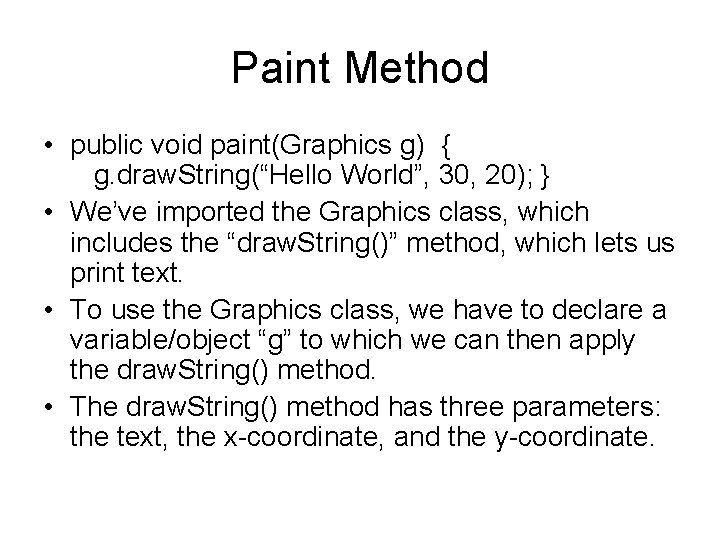
Paint Method • public void paint(Graphics g) { g. draw. String(“Hello World”, 30, 20); } • We’ve imported the Graphics class, which includes the “draw. String()” method, which lets us print text. • To use the Graphics class, we have to declare a variable/object “g” to which we can then apply the draw. String() method. • The draw. String() method has three parameters: the text, the x-coordinate, and the y-coordinate.
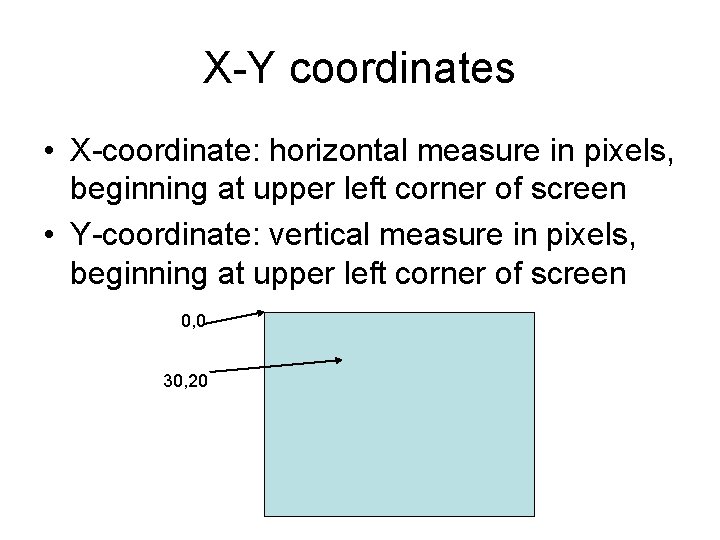
X-Y coordinates • X-coordinate: horizontal measure in pixels, beginning at upper left corner of screen • Y-coordinate: vertical measure in pixels, beginning at upper left corner of screen 0, 0 30, 20