The Art of Programming Languages Flowcharts 1 First
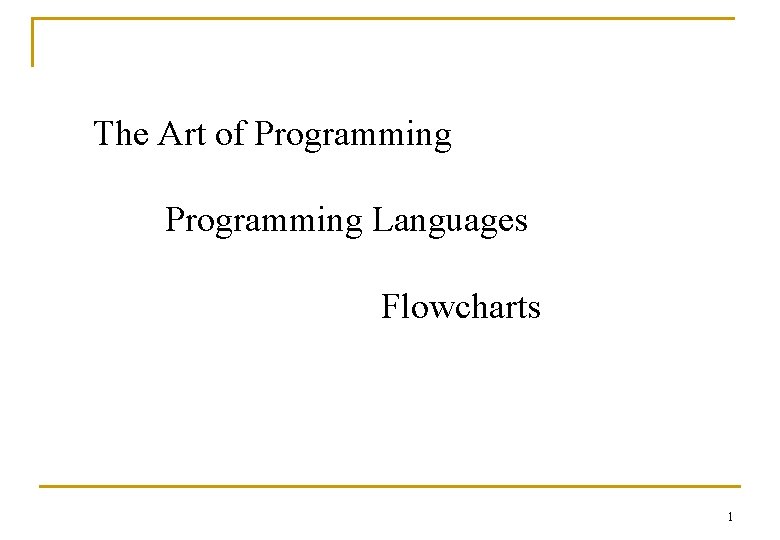
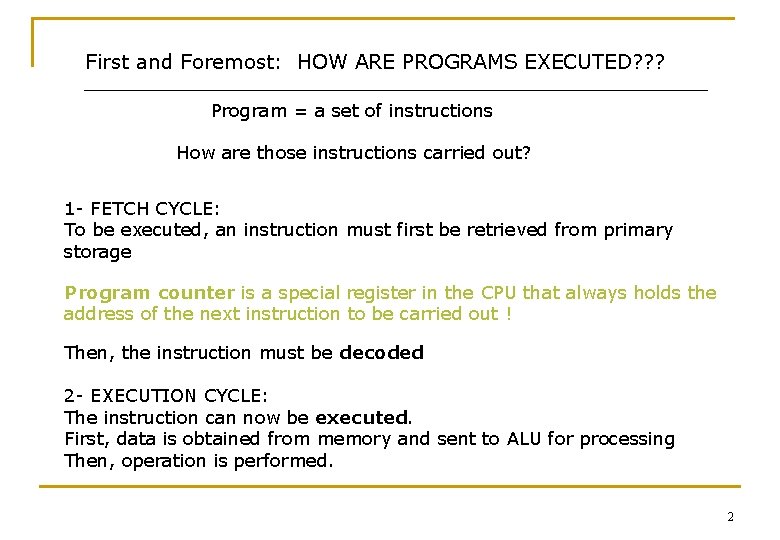
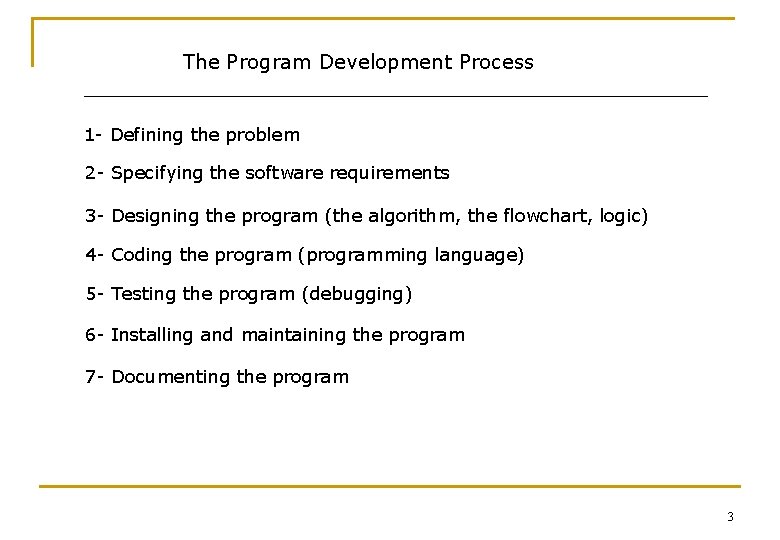
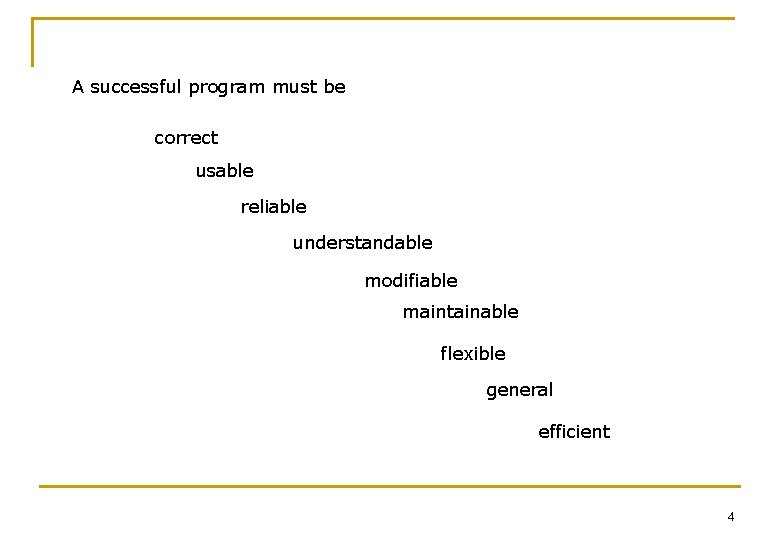
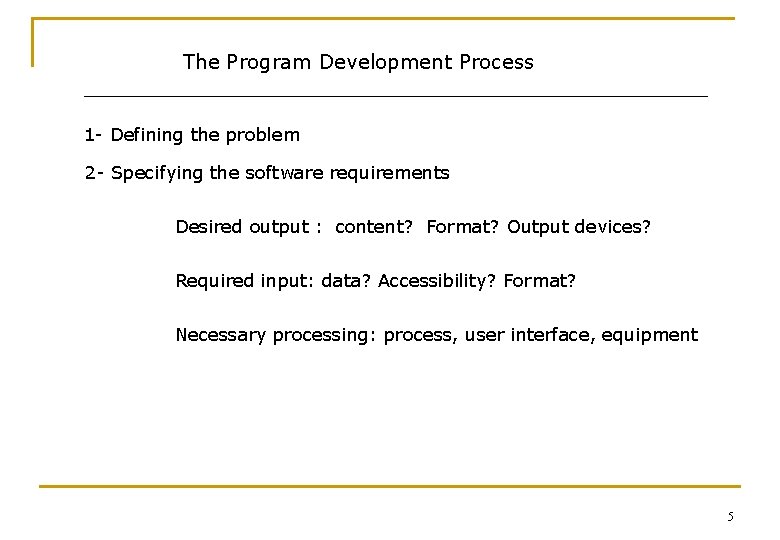
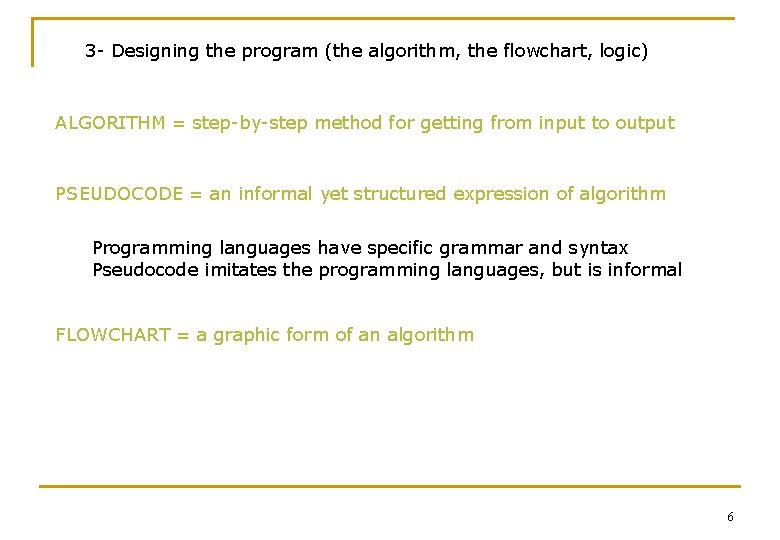
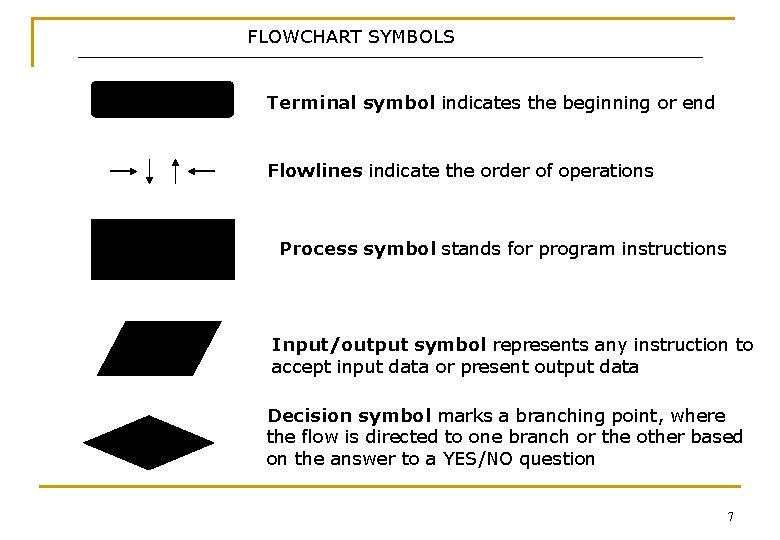
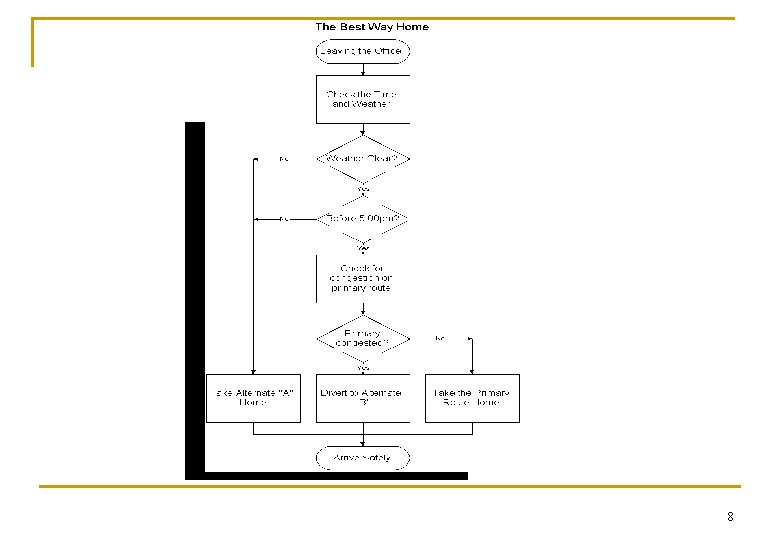
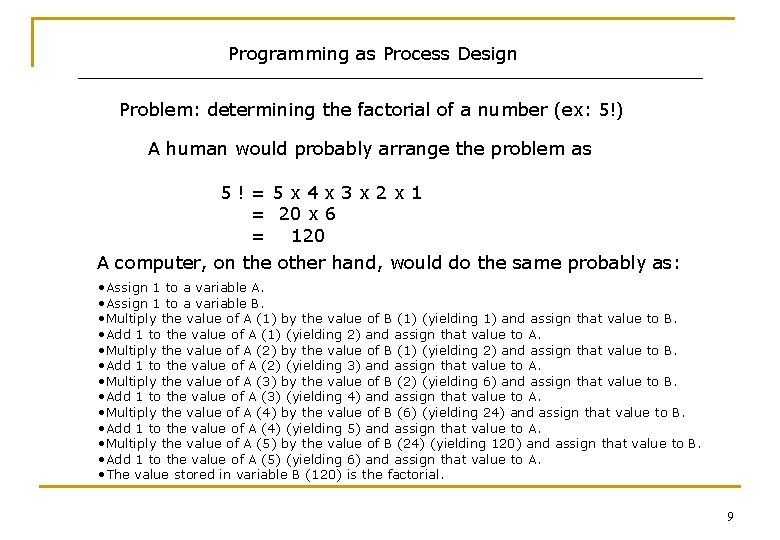
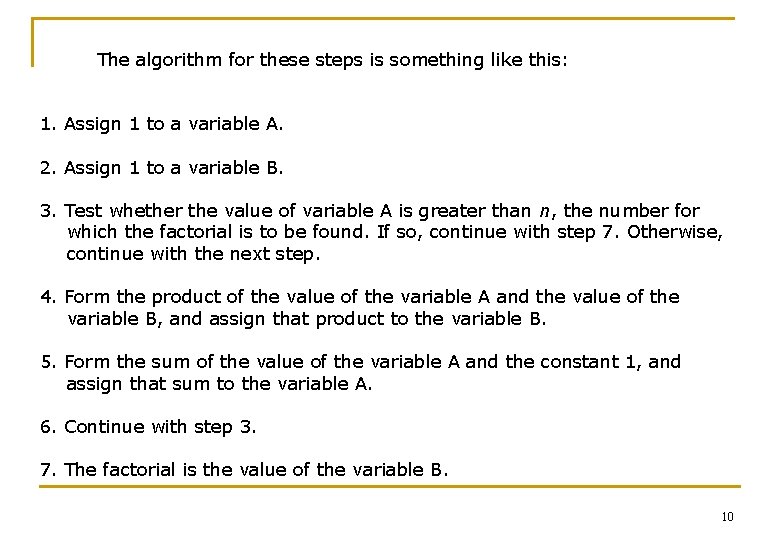
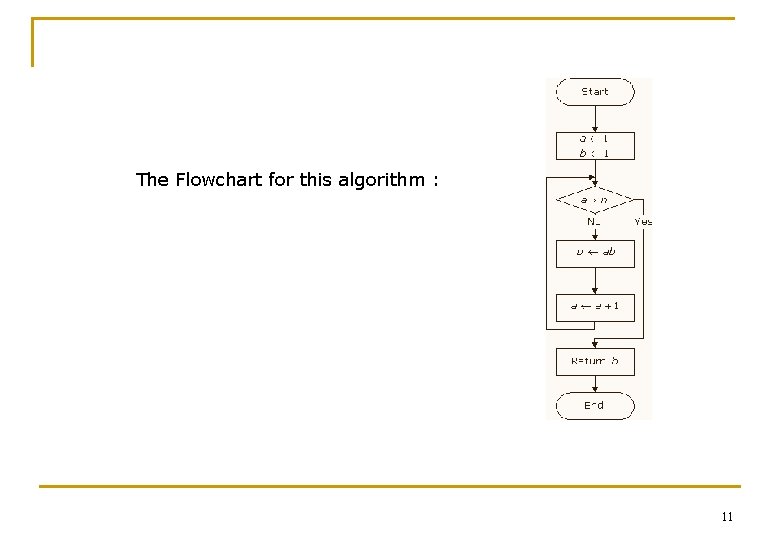
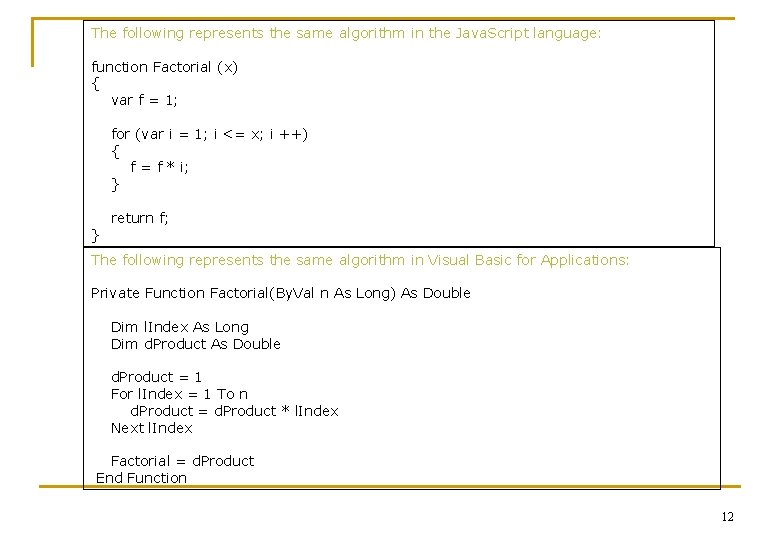
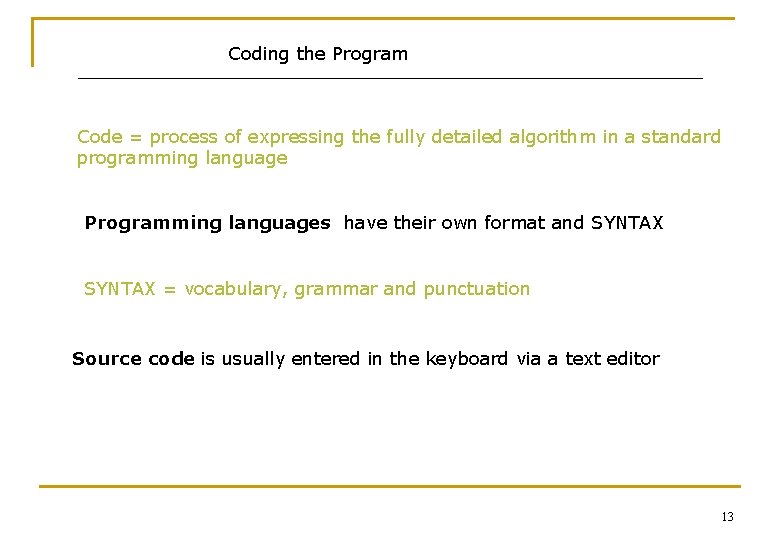
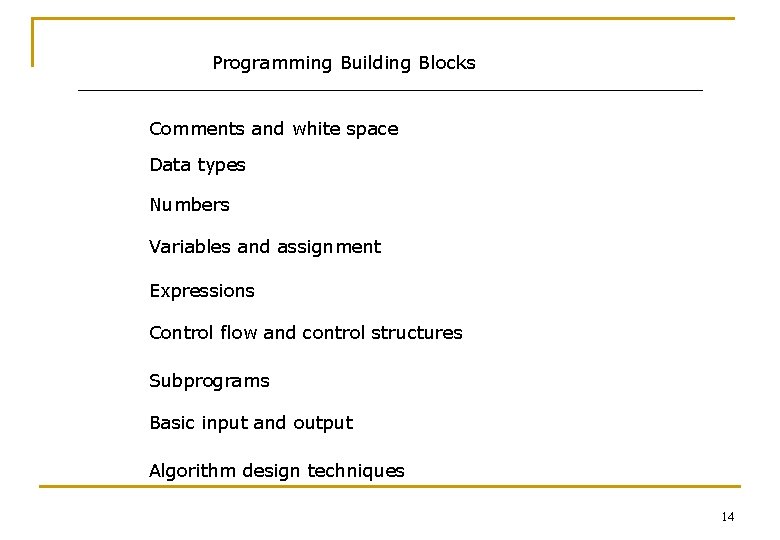
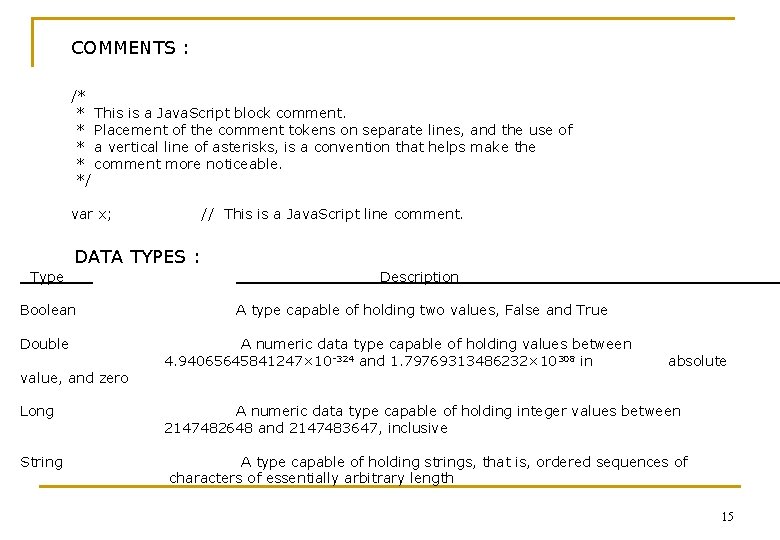
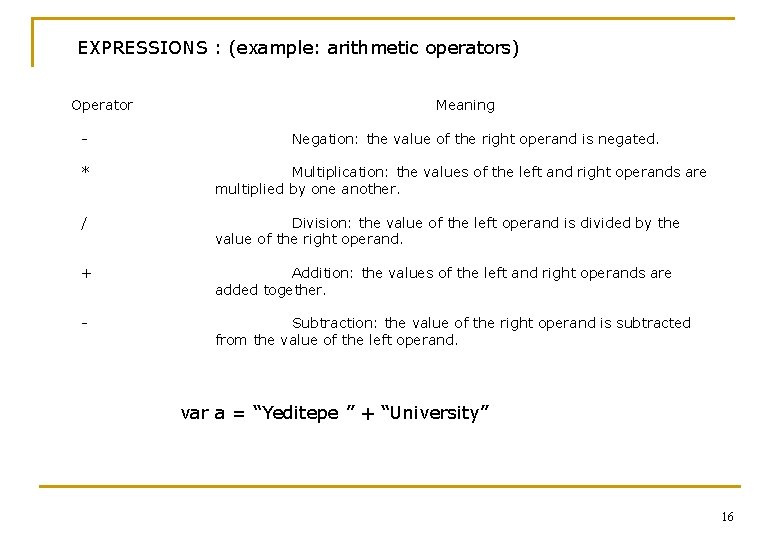
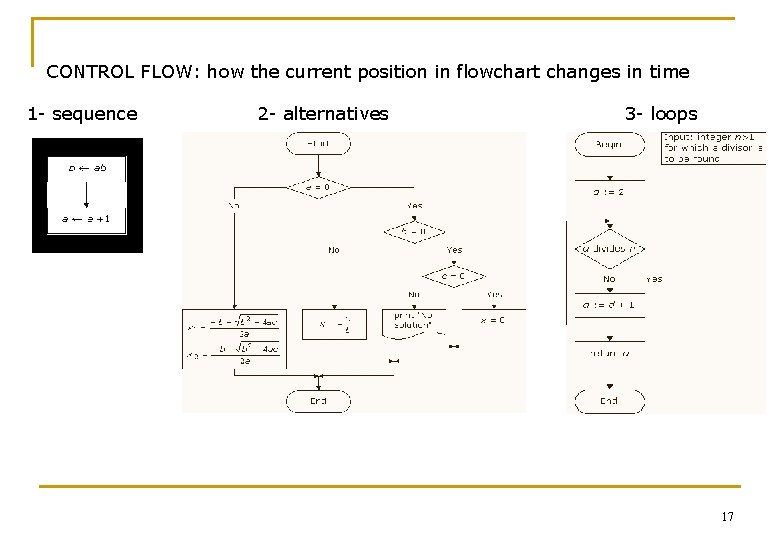
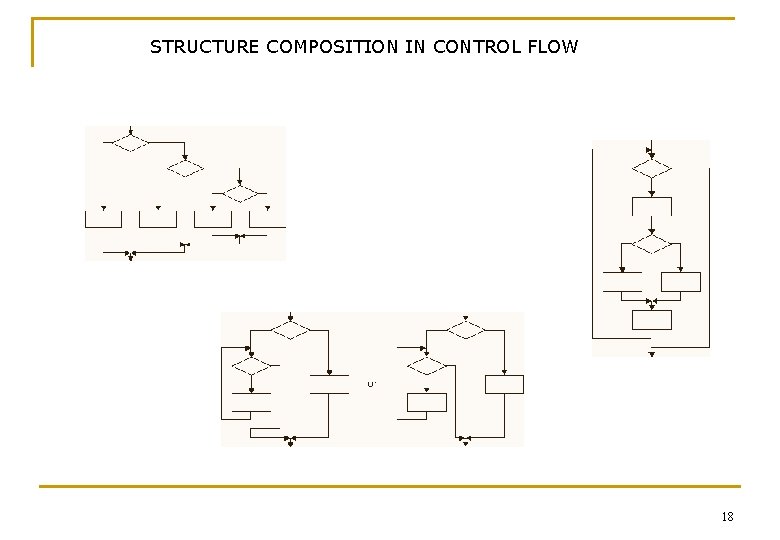
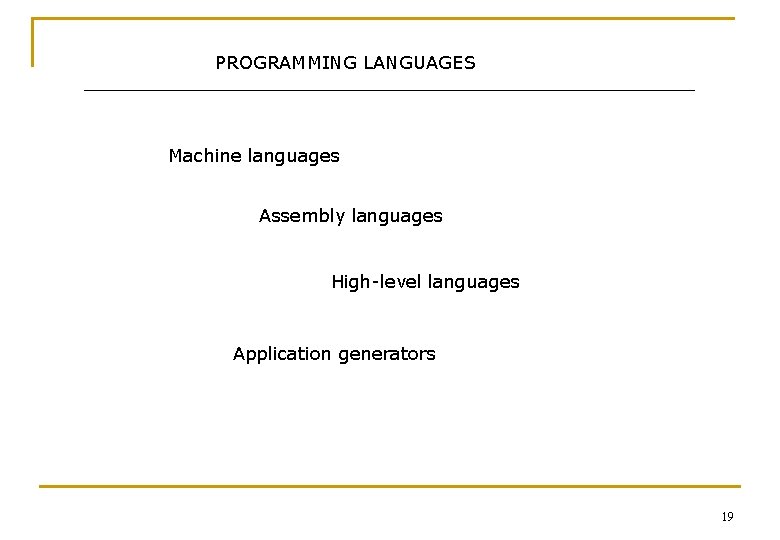
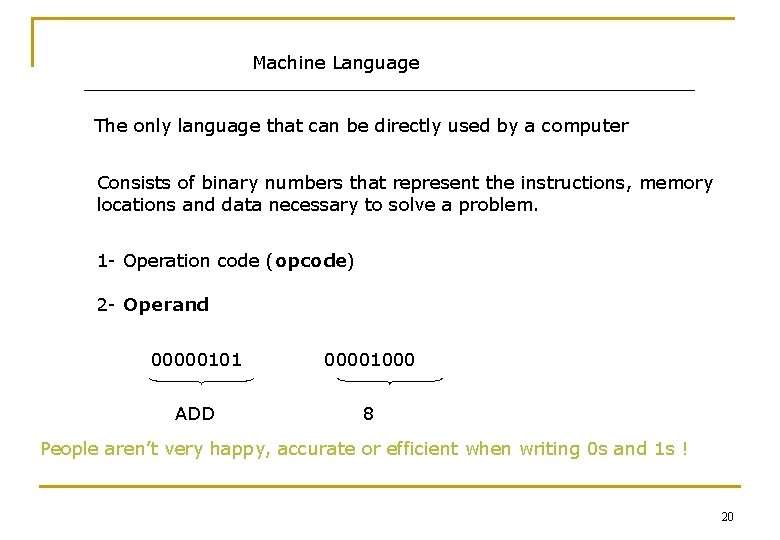
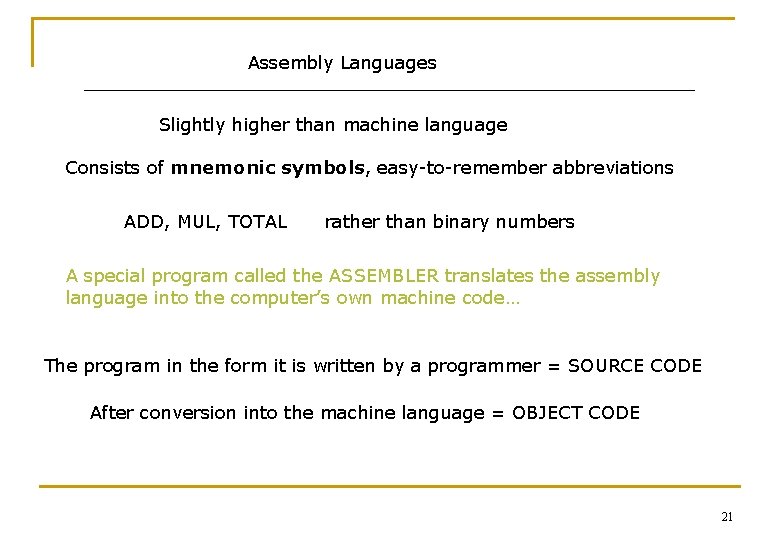
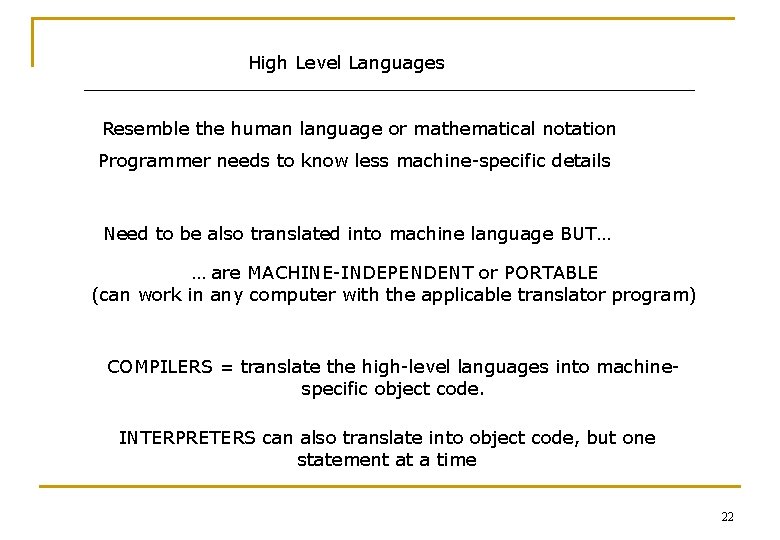
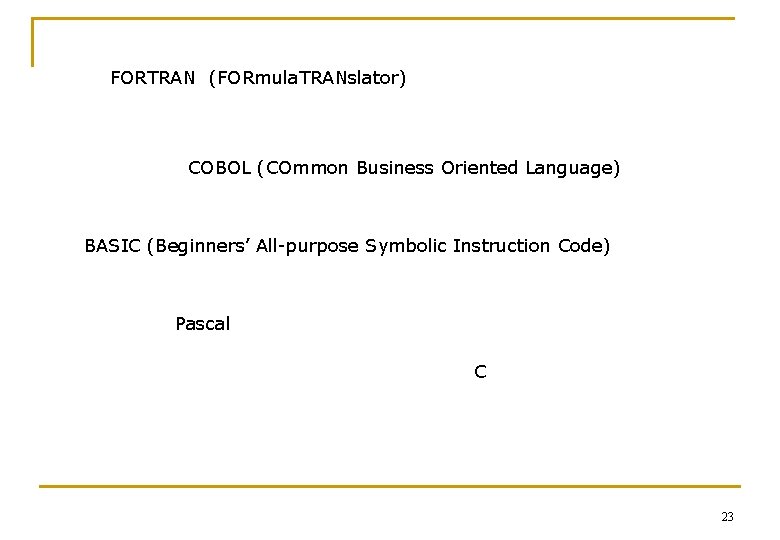
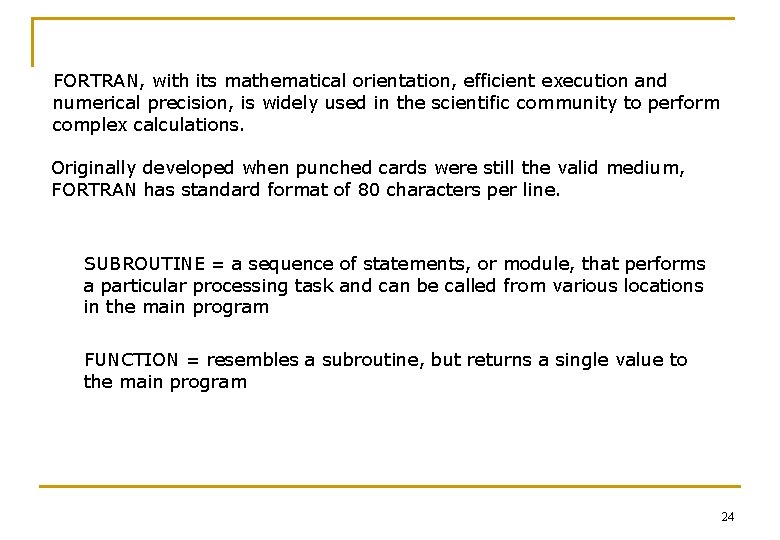
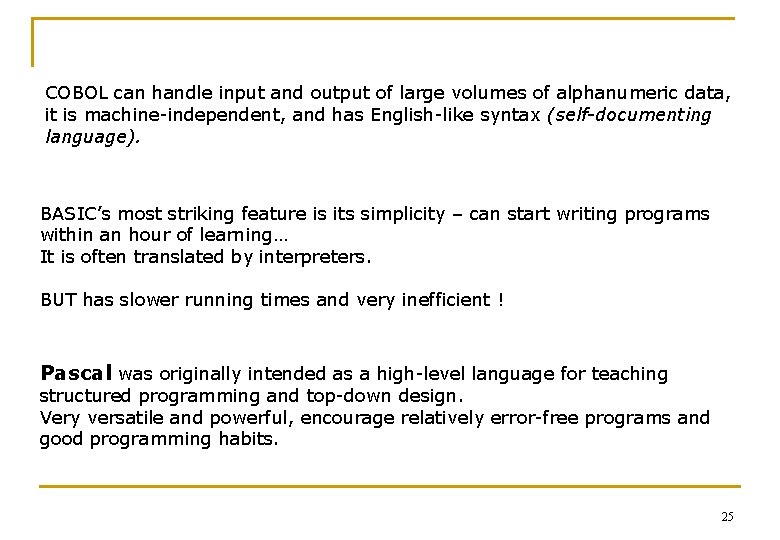
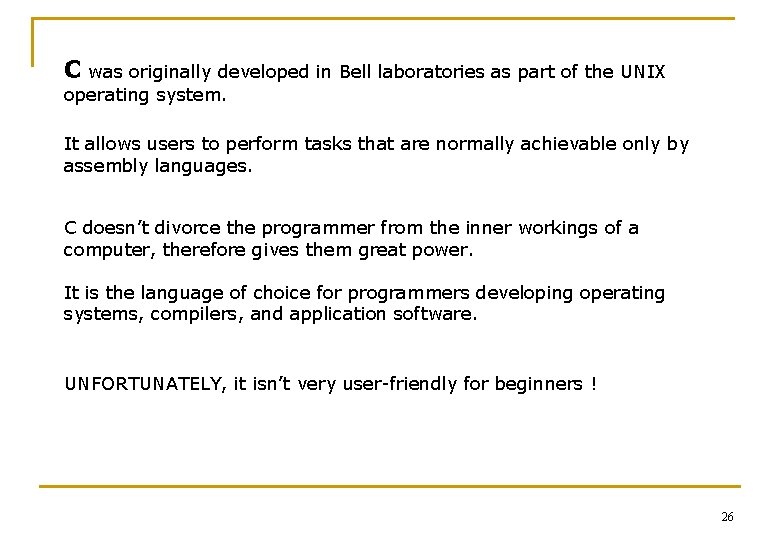
- Slides: 26
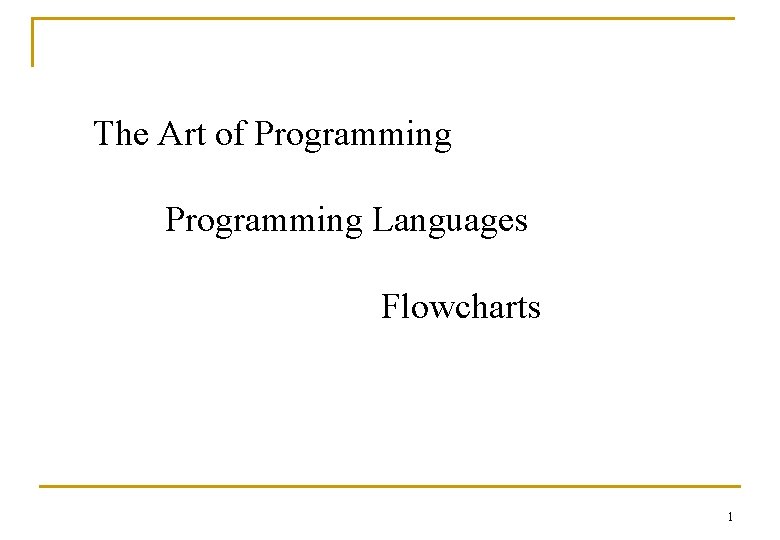
The Art of Programming Languages Flowcharts 1
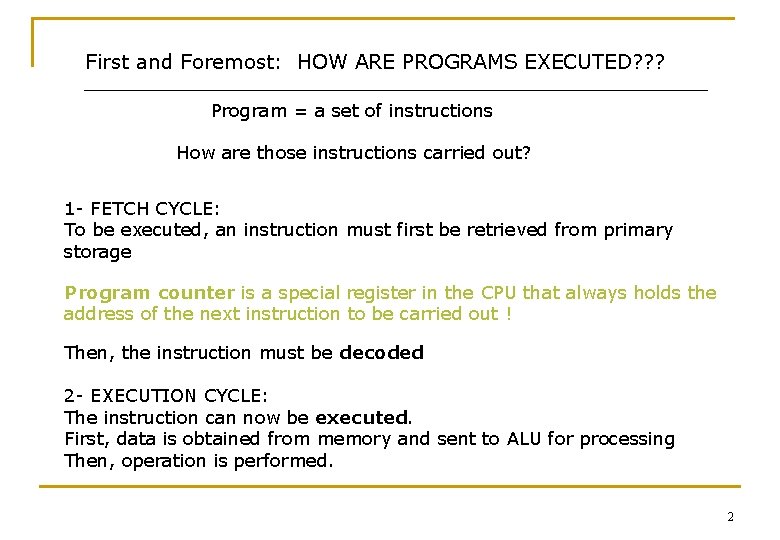
First and Foremost: HOW ARE PROGRAMS EXECUTED? ? ? Program = a set of instructions How are those instructions carried out? 1 - FETCH CYCLE: To be executed, an instruction must first be retrieved from primary storage Program counter is a special register in the CPU that always holds the address of the next instruction to be carried out ! Then, the instruction must be decoded 2 - EXECUTION CYCLE: The instruction can now be executed. First, data is obtained from memory and sent to ALU for processing Then, operation is performed. 2
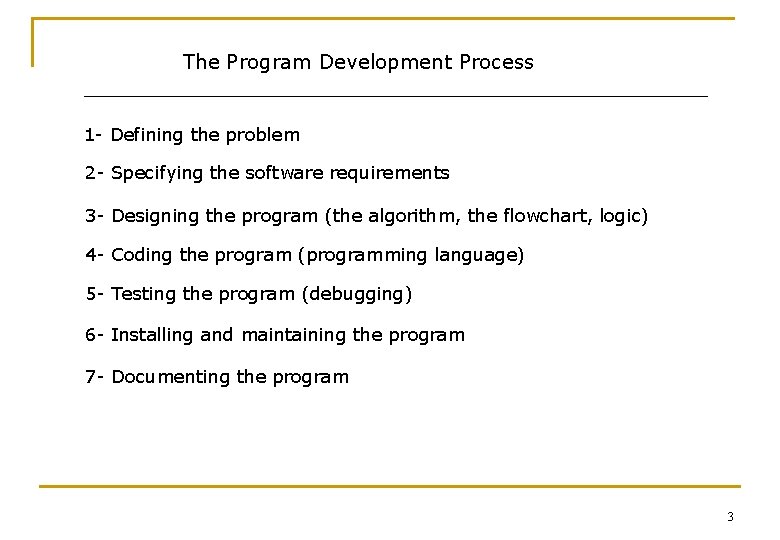
The Program Development Process 1 - Defining the problem 2 - Specifying the software requirements 3 - Designing the program (the algorithm, the flowchart, logic) 4 - Coding the program (programming language) 5 - Testing the program (debugging) 6 - Installing and maintaining the program 7 - Documenting the program 3
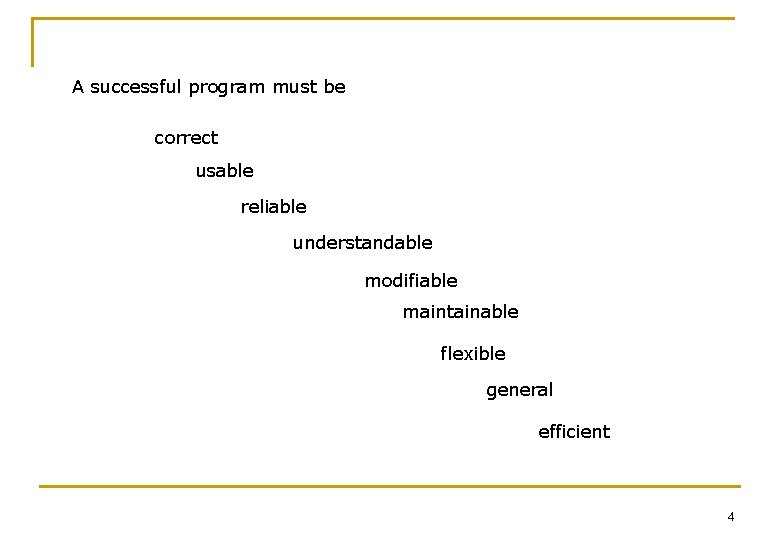
A successful program must be correct usable reliable understandable modifiable maintainable flexible general efficient 4
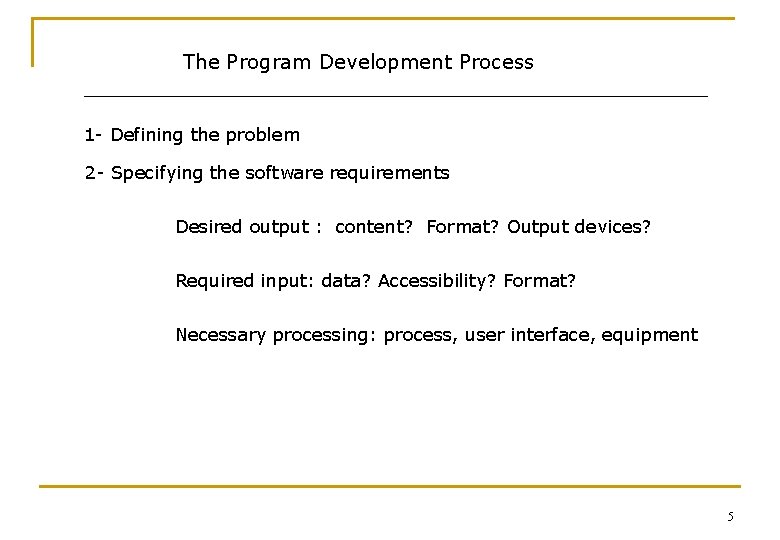
The Program Development Process 1 - Defining the problem 2 - Specifying the software requirements Desired output : content? Format? Output devices? Required input: data? Accessibility? Format? Necessary processing: process, user interface, equipment 5
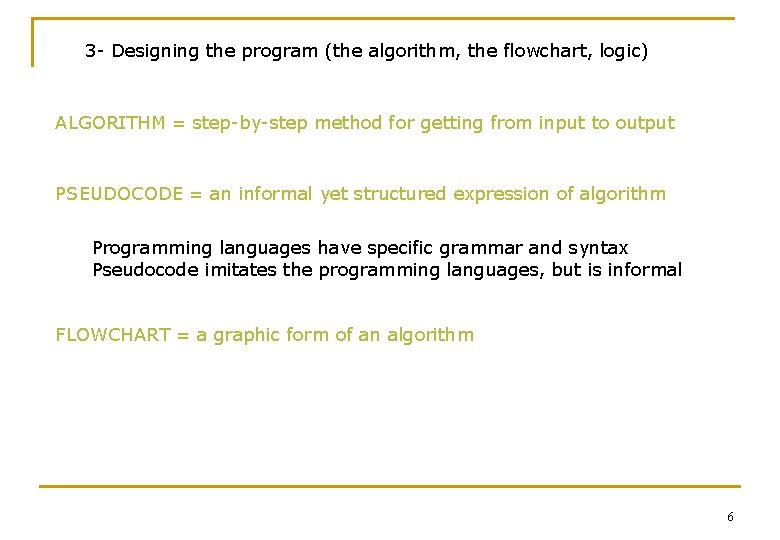
3 - Designing the program (the algorithm, the flowchart, logic) ALGORITHM = step-by-step method for getting from input to output PSEUDOCODE = an informal yet structured expression of algorithm Programming languages have specific grammar and syntax Pseudocode imitates the programming languages, but is informal FLOWCHART = a graphic form of an algorithm 6
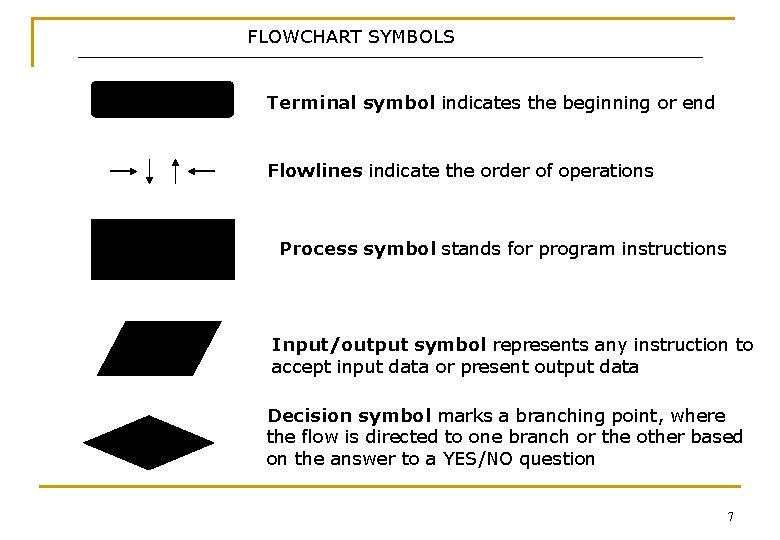
FLOWCHART SYMBOLS Terminal symbol indicates the beginning or end Flowlines indicate the order of operations Process symbol stands for program instructions Input/output symbol represents any instruction to accept input data or present output data Decision symbol marks a branching point, where the flow is directed to one branch or the other based on the answer to a YES/NO question 7
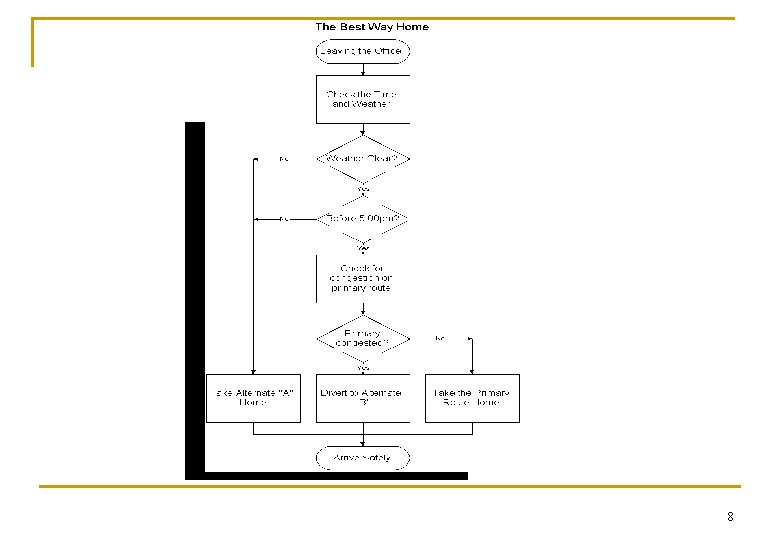
8
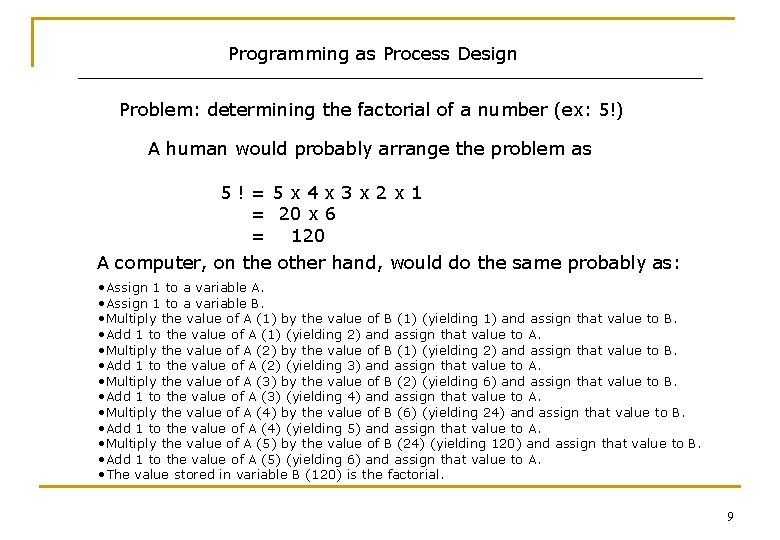
Programming as Process Design Problem: determining the factorial of a number (ex: 5!) A human would probably arrange the problem as 5!=5 x 4 x 3 x 2 x 1 = 20 x 6 = 120 A computer, on the other hand, would do the same probably as: • Assign 1 to a variable A. • Assign 1 to a variable B. • Multiply the value of A (1) by the value of B (1) (yielding 1) and assign that value to B. • Add 1 to the value of A (1) (yielding 2) and assign that value to A. • Multiply the value of A (2) by the value of B (1) (yielding 2) and assign that value to B. • Add 1 to the value of A (2) (yielding 3) and assign that value to A. • Multiply the value of A (3) by the value of B (2) (yielding 6) and assign that value to B. • Add 1 to the value of A (3) (yielding 4) and assign that value to A. • Multiply the value of A (4) by the value of B (6) (yielding 24) and assign that value to B. • Add 1 to the value of A (4) (yielding 5) and assign that value to A. • Multiply the value of A (5) by the value of B (24) (yielding 120) and assign that value to B. • Add 1 to the value of A (5) (yielding 6) and assign that value to A. • The value stored in variable B (120) is the factorial. 9
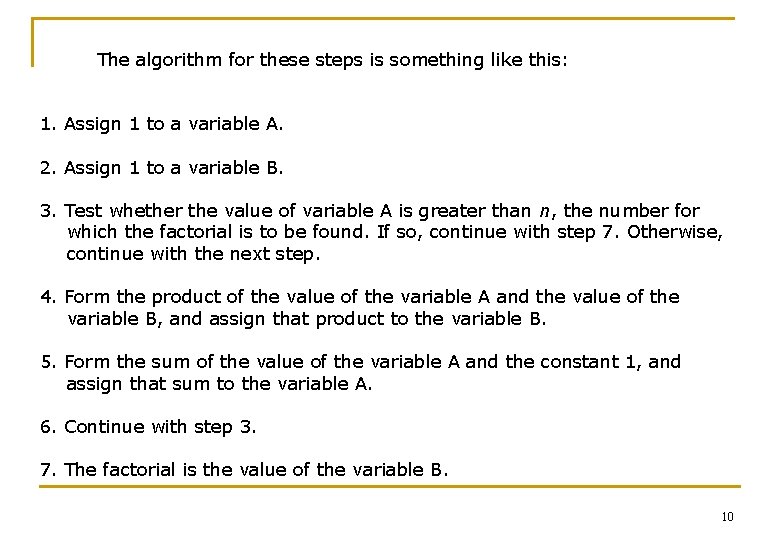
The algorithm for these steps is something like this: 1. Assign 1 to a variable A. 2. Assign 1 to a variable B. 3. Test whether the value of variable A is greater than n, the number for which the factorial is to be found. If so, continue with step 7. Otherwise, continue with the next step. 4. Form the product of the value of the variable A and the value of the variable B, and assign that product to the variable B. 5. Form the sum of the value of the variable A and the constant 1, and assign that sum to the variable A. 6. Continue with step 3. 7. The factorial is the value of the variable B. 10
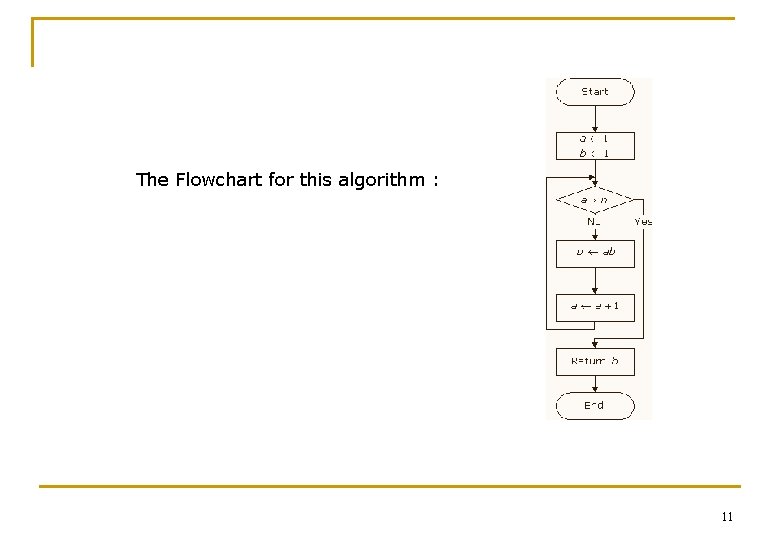
The Flowchart for this algorithm : 11
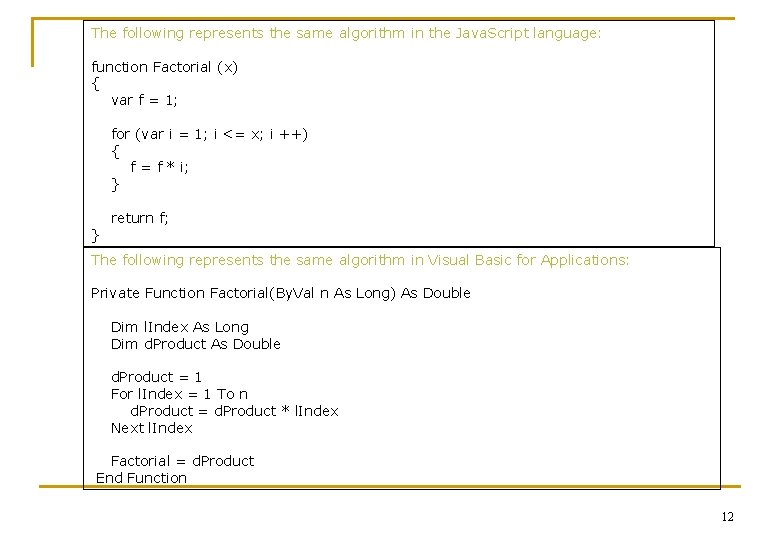
The following represents the same algorithm in the Java. Script language: function Factorial (x) { var f = 1; for (var i = 1; i <= x; i ++) { f = f * i; } } return f; The following represents the same algorithm in Visual Basic for Applications: Private Function Factorial(By. Val n As Long) As Double Dim l. Index As Long Dim d. Product As Double d. Product = 1 For l. Index = 1 To n d. Product = d. Product * l. Index Next l. Index Factorial = d. Product End Function 12
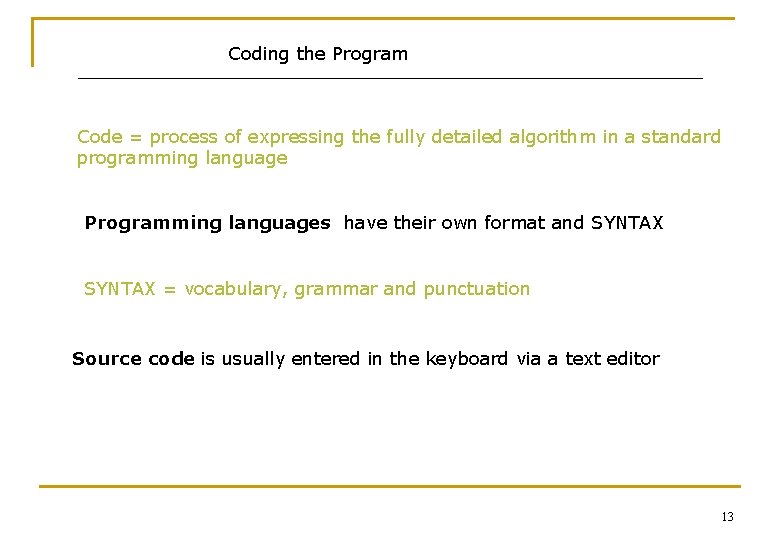
Coding the Program Code = process of expressing the fully detailed algorithm in a standard programming language Programming languages have their own format and SYNTAX = vocabulary, grammar and punctuation Source code is usually entered in the keyboard via a text editor 13
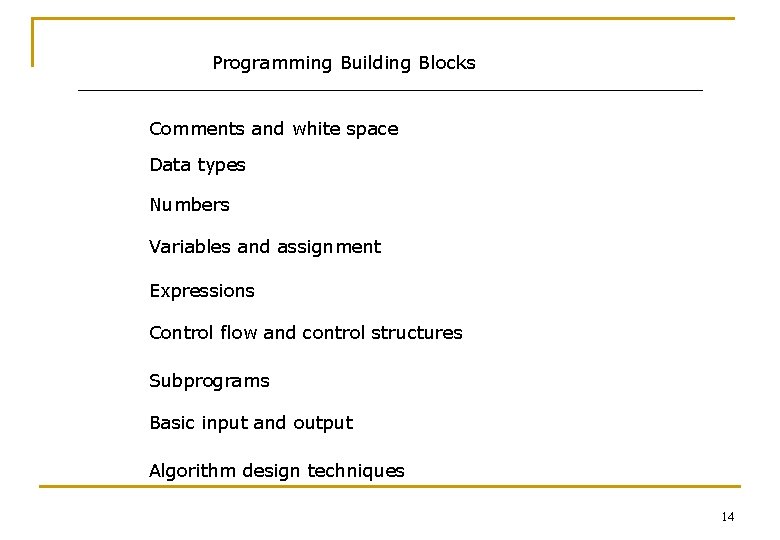
Programming Building Blocks Comments and white space Data types Numbers Variables and assignment Expressions Control flow and control structures Subprograms Basic input and output Algorithm design techniques 14
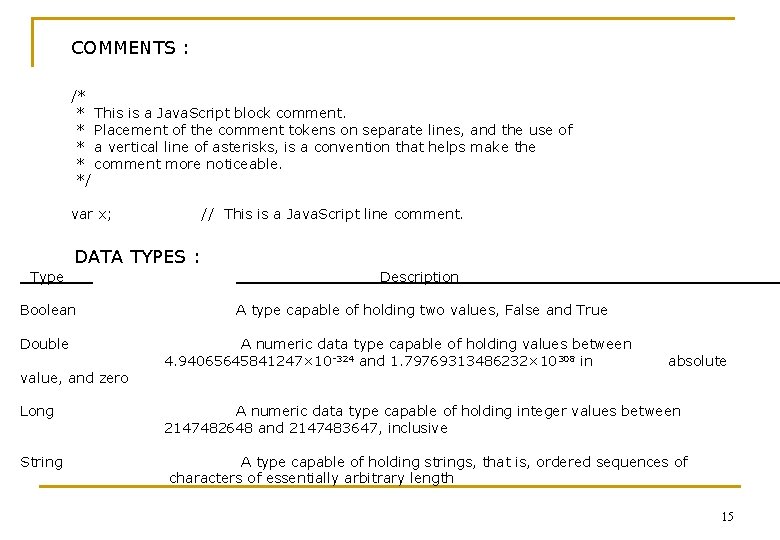
COMMENTS : /* * This is a Java. Script block comment. * Placement of the comment tokens on separate lines, and the use of * a vertical line of asterisks, is a convention that helps make the * comment more noticeable. */ var x; // This is a Java. Script line comment. DATA TYPES : Type Boolean Double value, and zero Long String Description_________ A type capable of holding two values, False and True A numeric data type capable of holding values between 4. 94065645841247× 10 -324 and 1. 79769313486232× 10308 in absolute A numeric data type capable of holding integer values between 2147482648 and 2147483647, inclusive A type capable of holding strings, that is, ordered sequences of characters of essentially arbitrary length 15
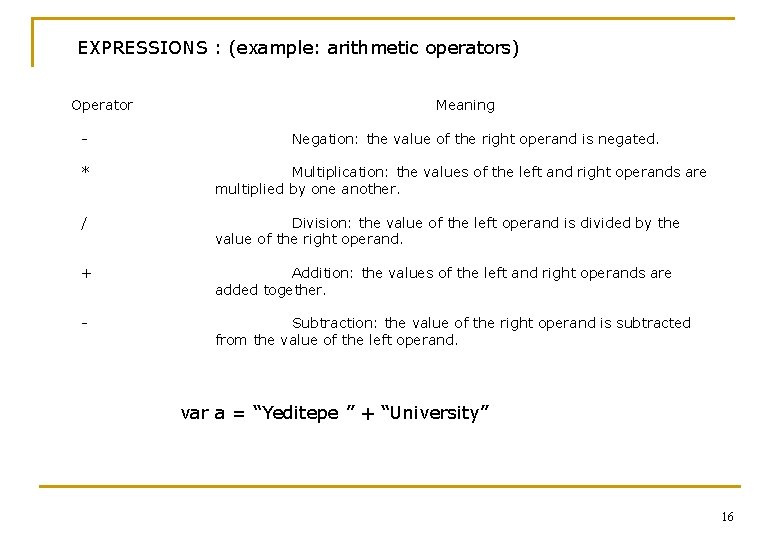
EXPRESSIONS : (example: arithmetic operators) Operator - Meaning Negation: the value of the right operand is negated. * Multiplication: the values of the left and right operands are multiplied by one another. / Division: the value of the left operand is divided by the value of the right operand. + Addition: the values of the left and right operands are added together. - Subtraction: the value of the right operand is subtracted from the value of the left operand. var a = “Yeditepe ” + “University” 16
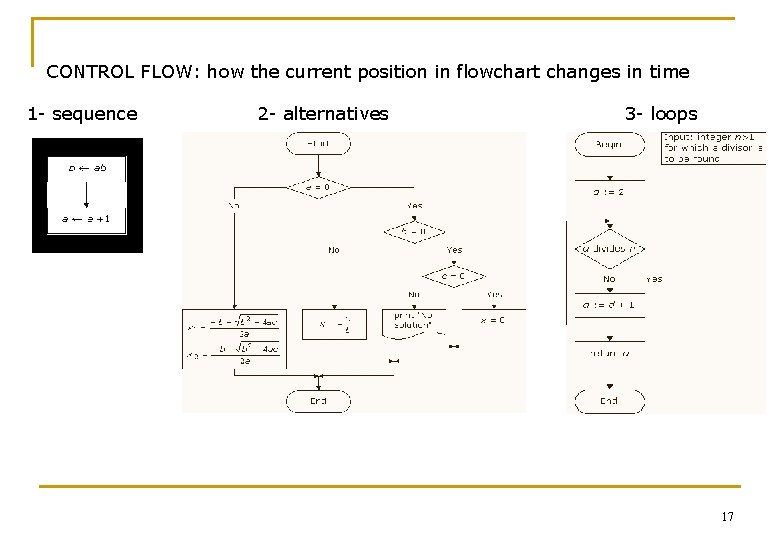
CONTROL FLOW: how the current position in flowchart changes in time 1 - sequence 2 - alternatives 3 - loops 17
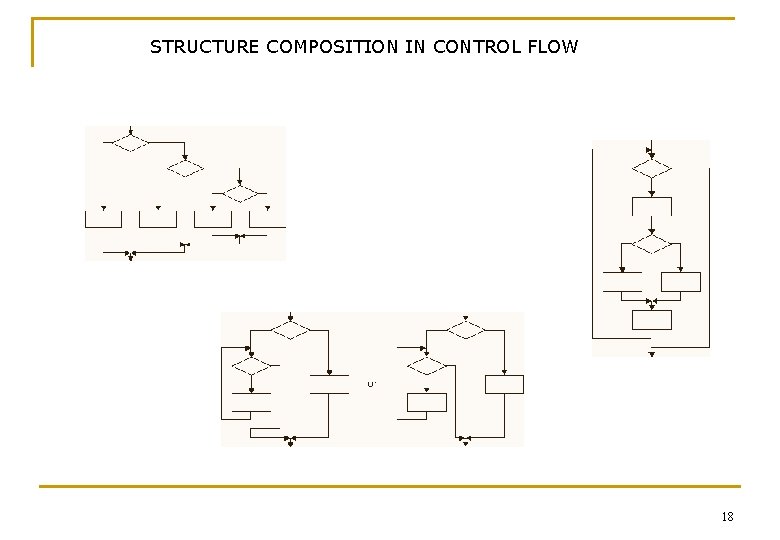
STRUCTURE COMPOSITION IN CONTROL FLOW 18
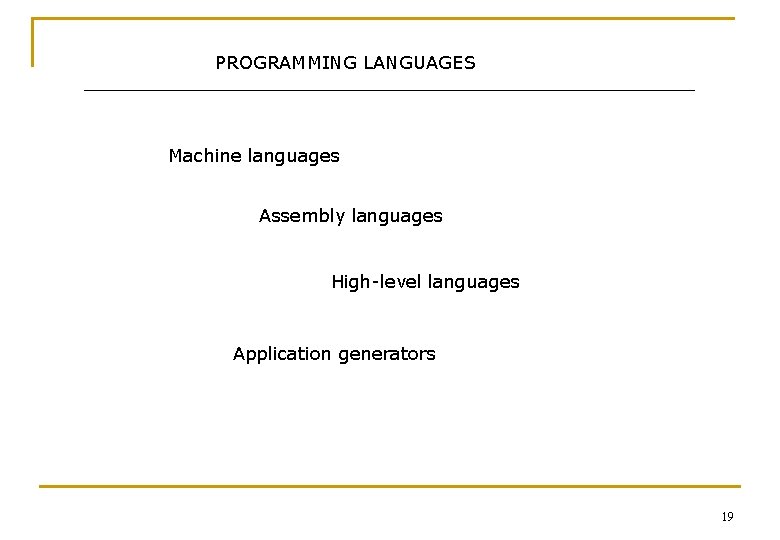
PROGRAMMING LANGUAGES Machine languages Assembly languages High-level languages Application generators 19
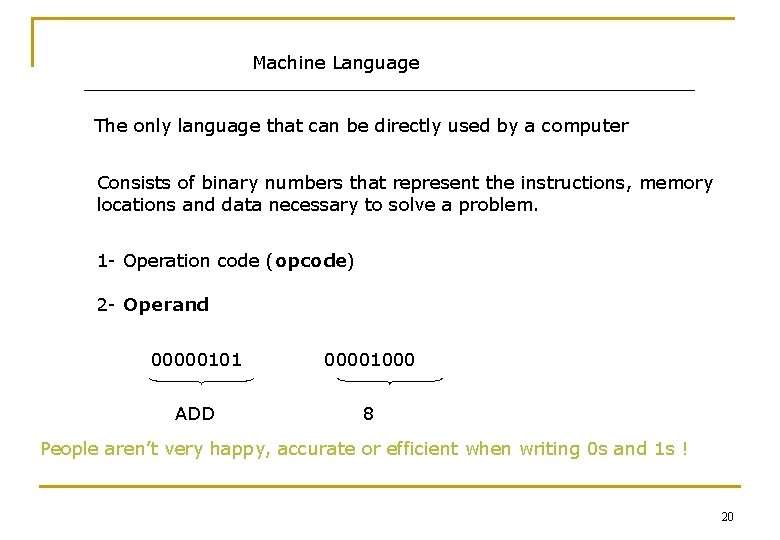
Machine Language The only language that can be directly used by a computer Consists of binary numbers that represent the instructions, memory locations and data necessary to solve a problem. 1 - Operation code (opcode) 2 - Operand 00000101 00001000 ADD 8 People aren’t very happy, accurate or efficient when writing 0 s and 1 s ! 20
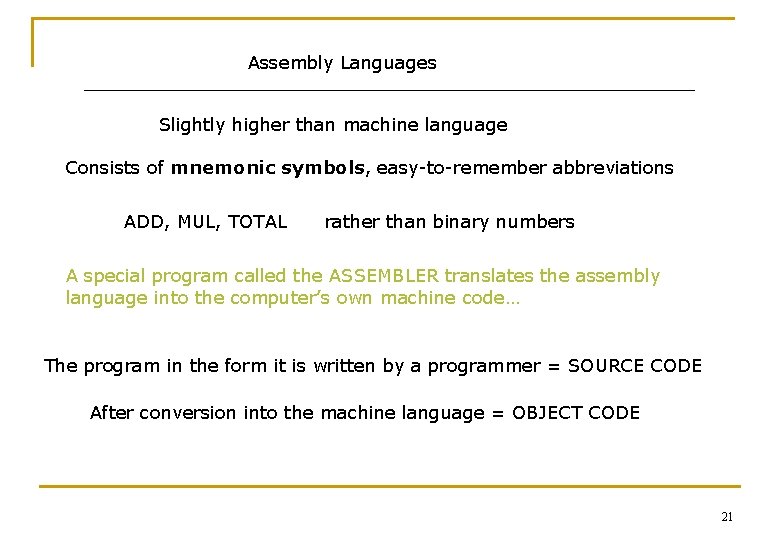
Assembly Languages Slightly higher than machine language Consists of mnemonic symbols, easy-to-remember abbreviations ADD, MUL, TOTAL rather than binary numbers A special program called the ASSEMBLER translates the assembly language into the computer’s own machine code… The program in the form it is written by a programmer = SOURCE CODE After conversion into the machine language = OBJECT CODE 21
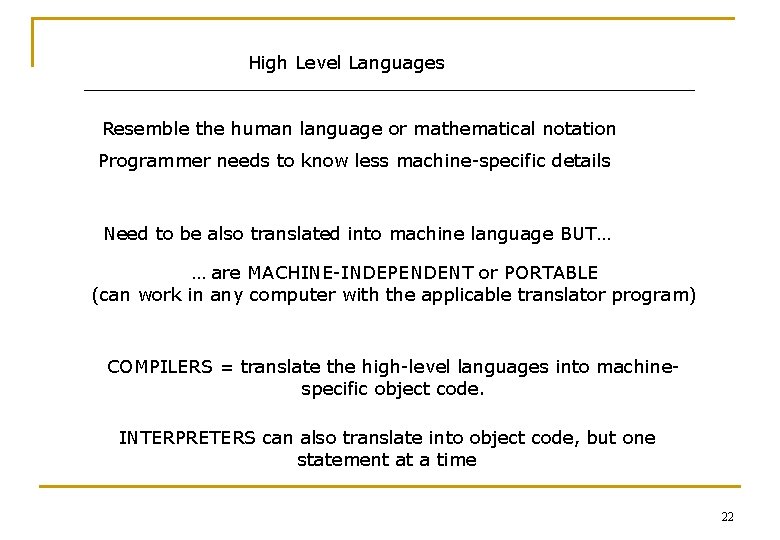
High Level Languages Resemble the human language or mathematical notation Programmer needs to know less machine-specific details Need to be also translated into machine language BUT… … are MACHINE-INDEPENDENT or PORTABLE (can work in any computer with the applicable translator program) COMPILERS = translate the high-level languages into machinespecific object code. INTERPRETERS can also translate into object code, but one statement at a time 22
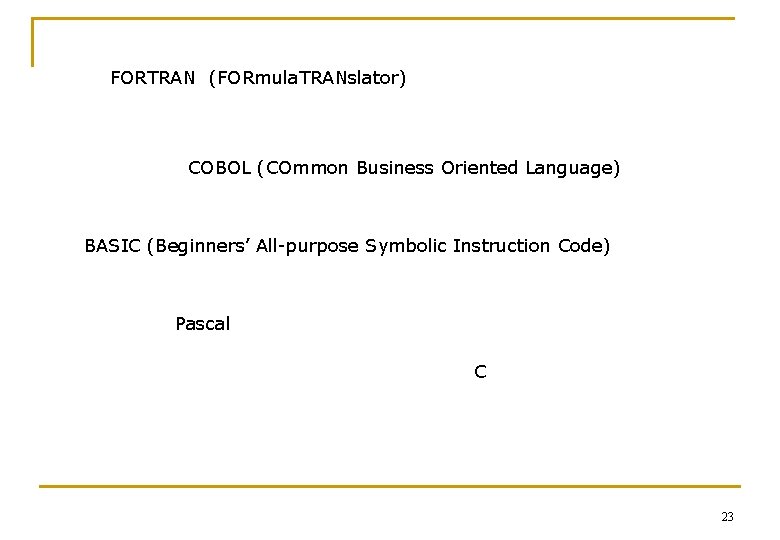
FORTRAN (FORmula. TRANslator) COBOL (COmmon Business Oriented Language) BASIC (Beginners’ All-purpose Symbolic Instruction Code) Pascal C 23
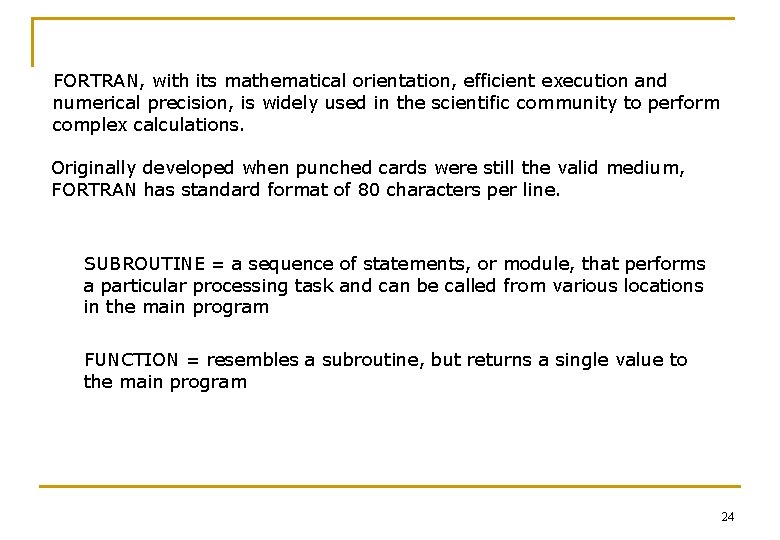
FORTRAN, with its mathematical orientation, efficient execution and numerical precision, is widely used in the scientific community to perform complex calculations. Originally developed when punched cards were still the valid medium, FORTRAN has standard format of 80 characters per line. SUBROUTINE = a sequence of statements, or module, that performs a particular processing task and can be called from various locations in the main program FUNCTION = resembles a subroutine, but returns a single value to the main program 24
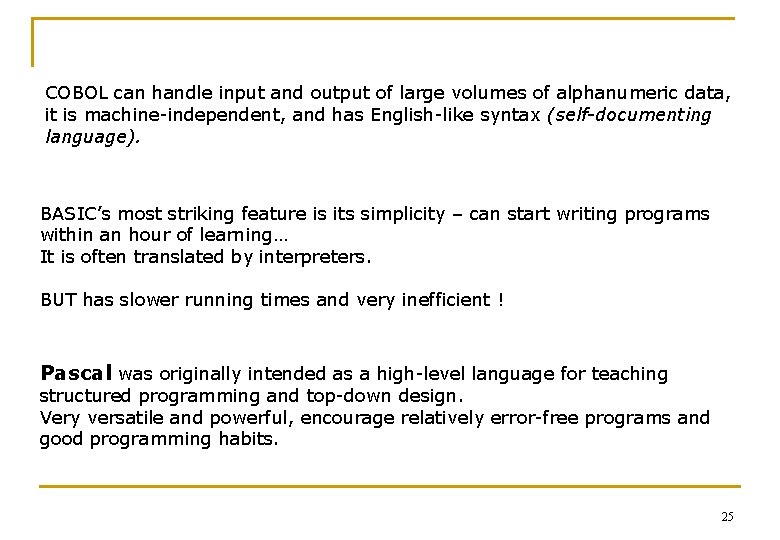
COBOL can handle input and output of large volumes of alphanumeric data, it is machine-independent, and has English-like syntax (self-documenting language). BASIC’s most striking feature is its simplicity – can start writing programs within an hour of learning… It is often translated by interpreters. BUT has slower running times and very inefficient ! Pascal was originally intended as a high-level language for teaching structured programming and top-down design. Very versatile and powerful, encourage relatively error-free programs and good programming habits. 25
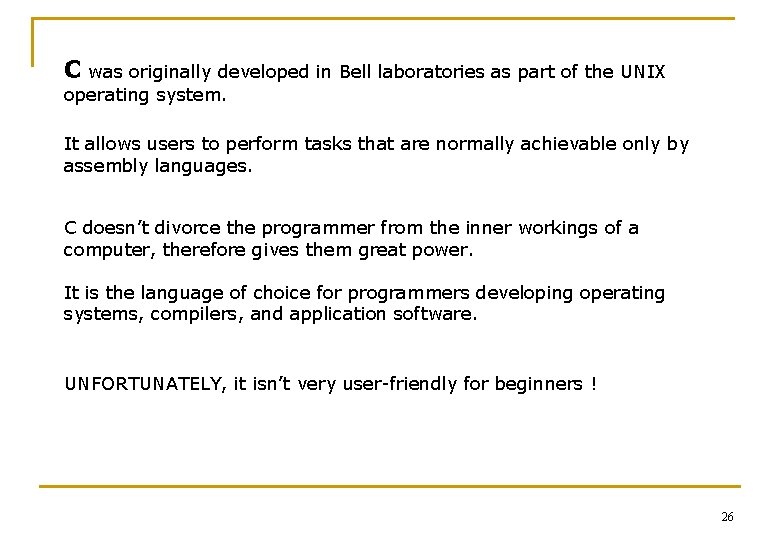
C was originally developed in Bell laboratories as part of the UNIX operating system. It allows users to perform tasks that are normally achievable only by assembly languages. C doesn’t divorce the programmer from the inner workings of a computer, therefore gives them great power. It is the language of choice for programmers developing operating systems, compilers, and application software. UNFORTUNATELY, it isn’t very user-friendly for beginners ! 26
3-3 assignment: introduction to pseudocode and flowcharts
Algorithms and flowcharts
Flowcharts and pseudocode
Pseudocode for baking bread
Xkcd flowcharts
Hierarchy of pseudocode
Real-time systems and programming languages
Cs 421 programming languages and compilers
Multithreading program in java
Programming languages levels
Introduction to programming languages
Plc
Joey paquet
Comparative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Cse 340 principles of programming languages
Integral data type is
Xenia programming languages
Advantages of application software
Mainstream programming languages
Cse 340 principles of programming languages
Programing languages
Programming languages
Programming languages
Programming languages