Elementary Programming Liang Introduction to Java Programming Seventh
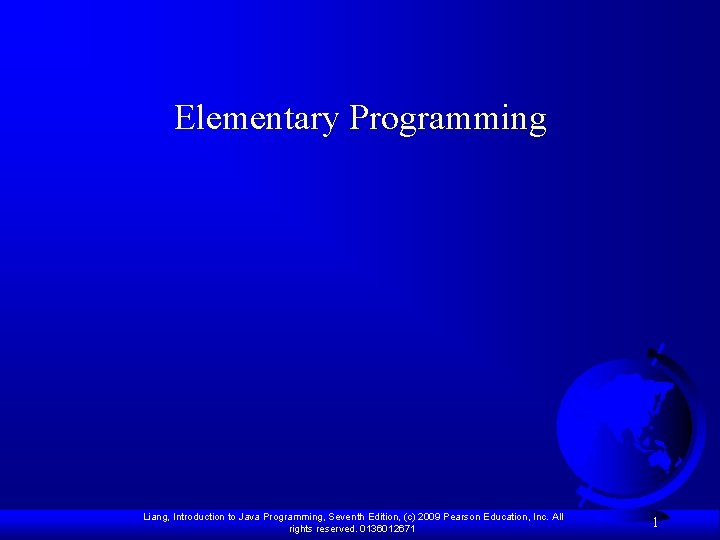
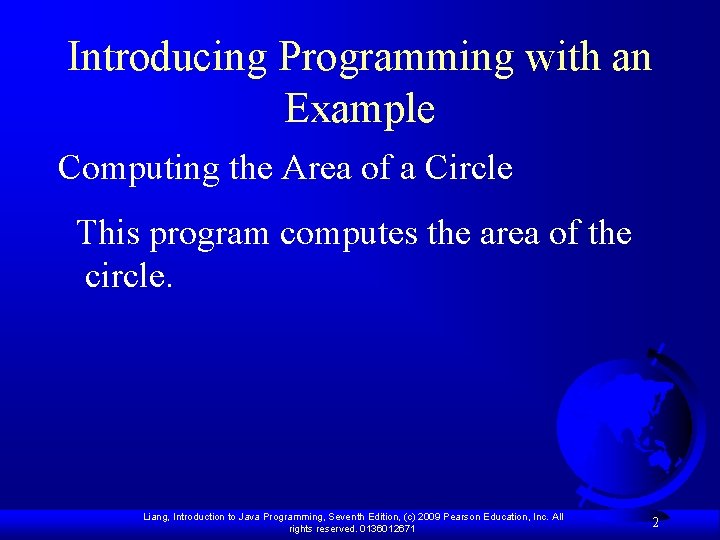
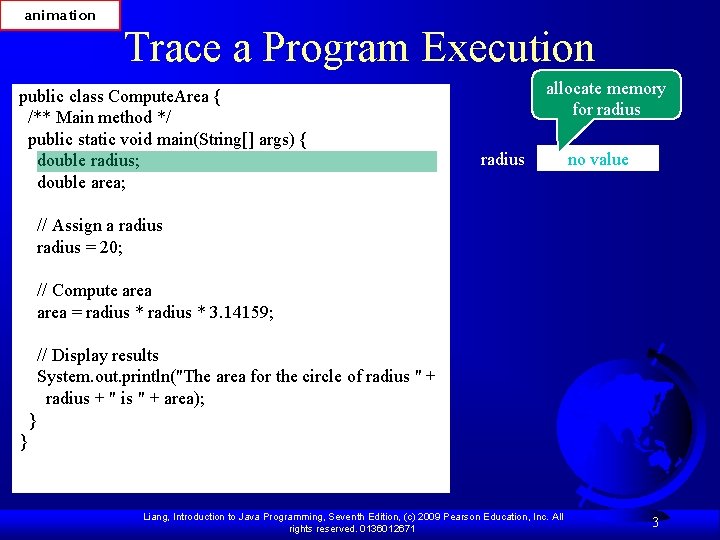
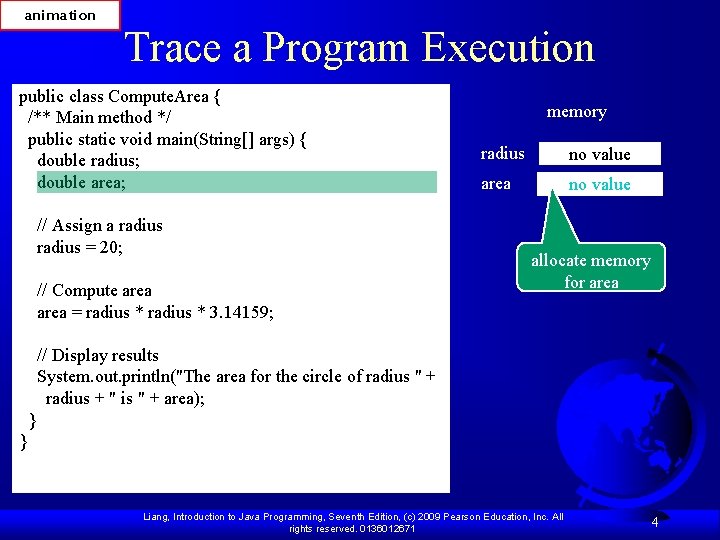
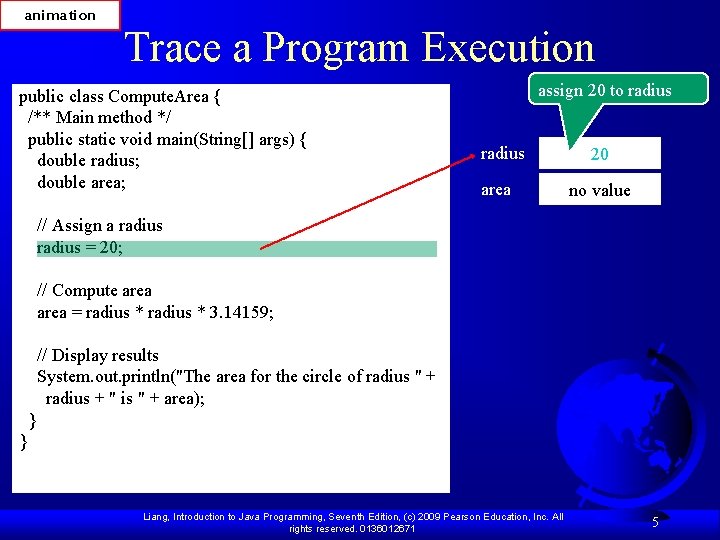
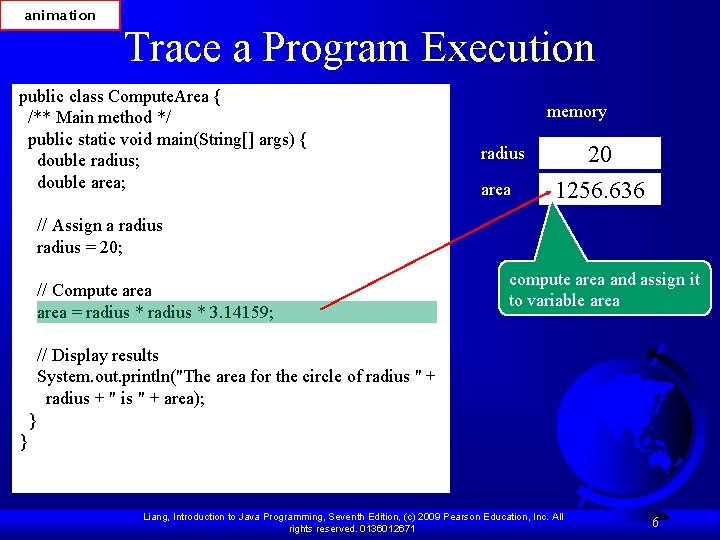
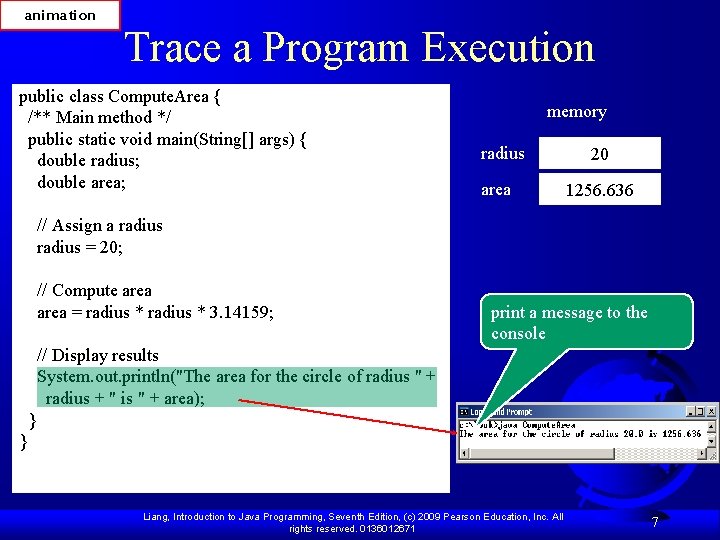
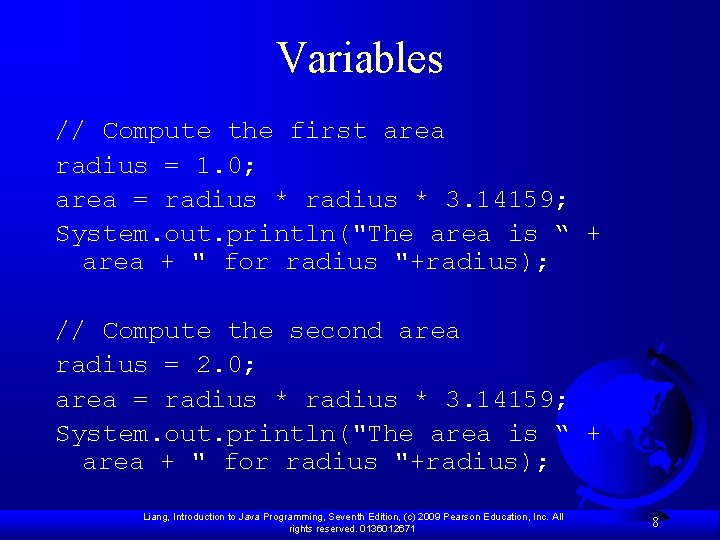
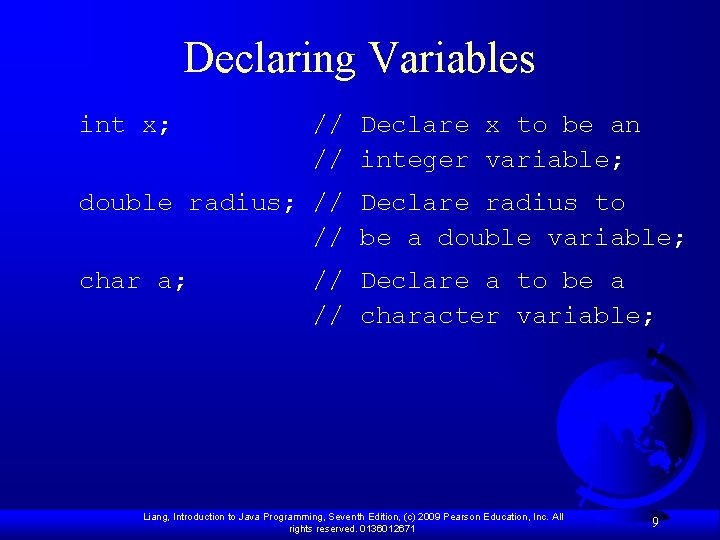
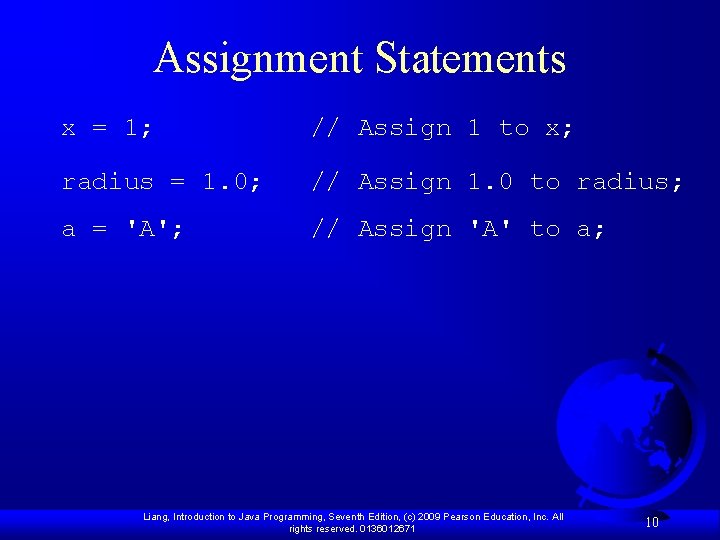
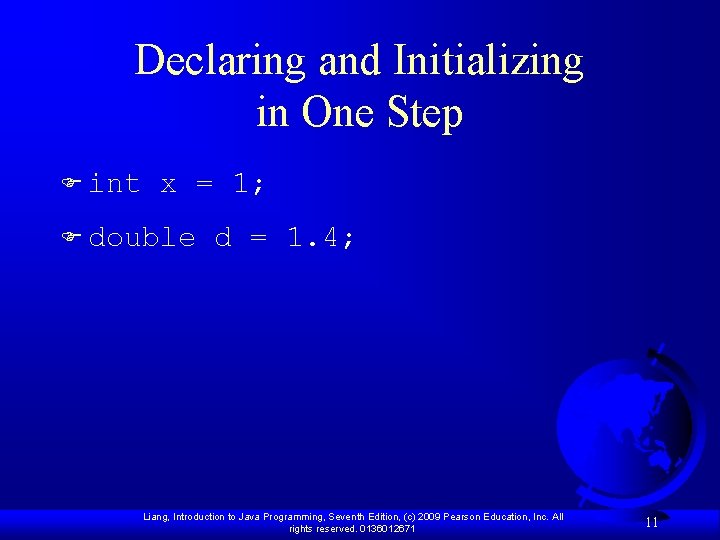
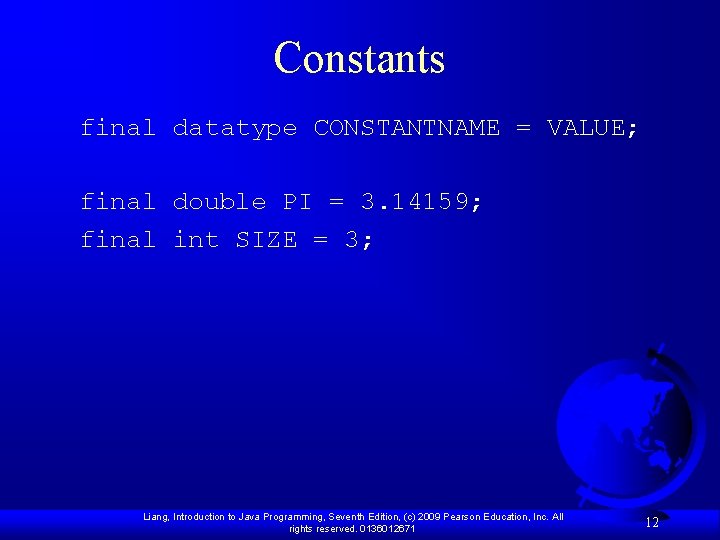
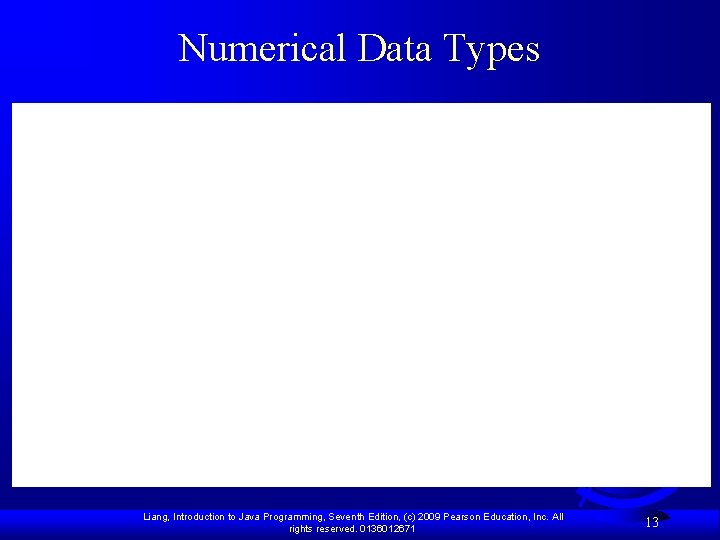
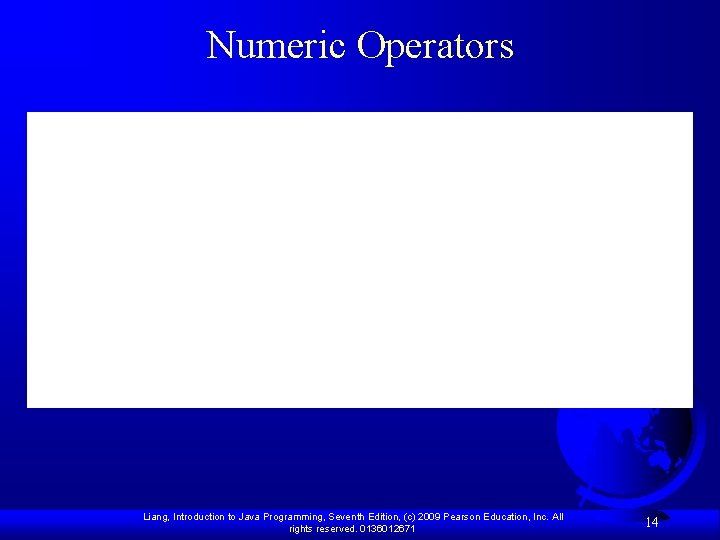
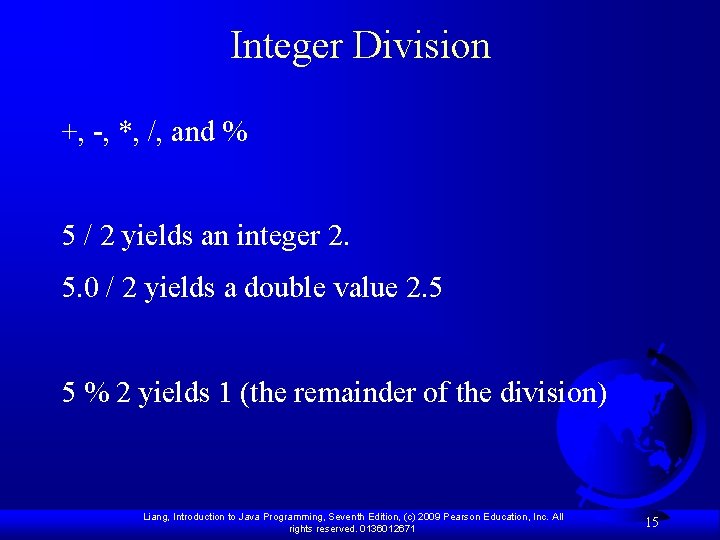
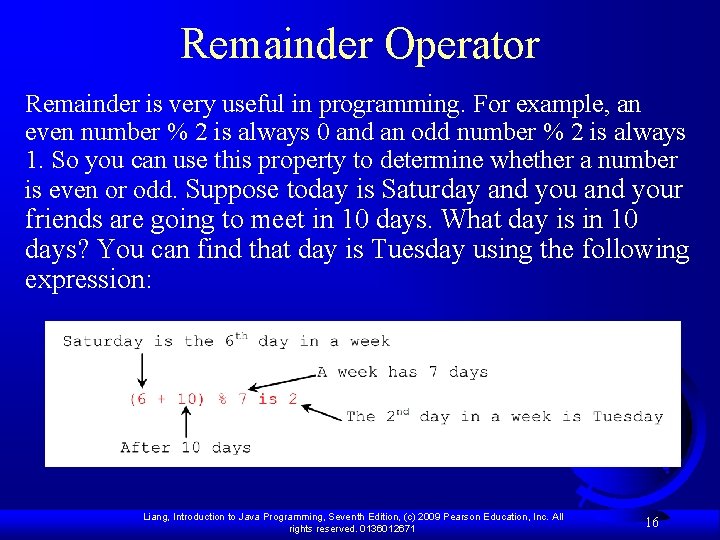
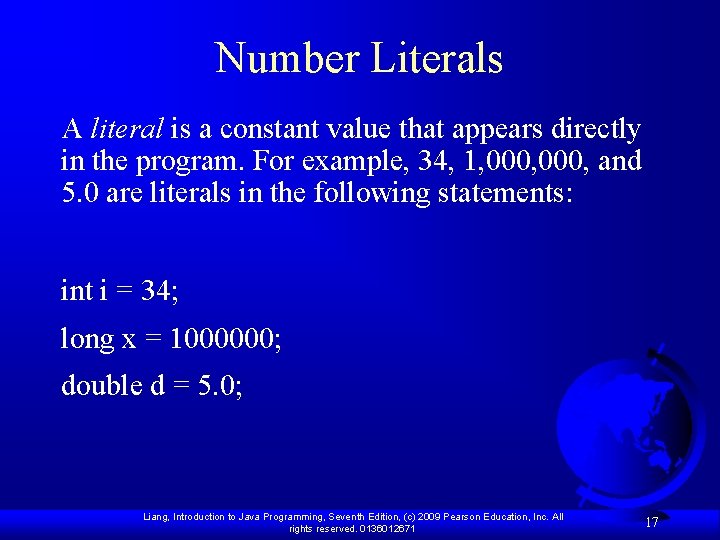
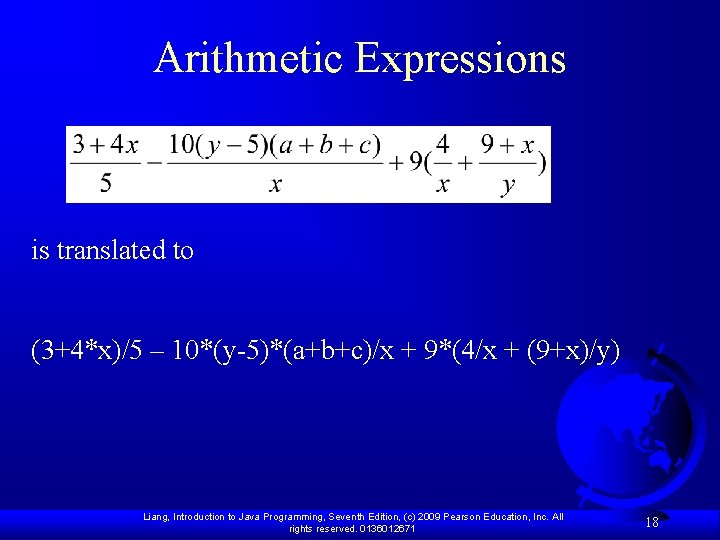
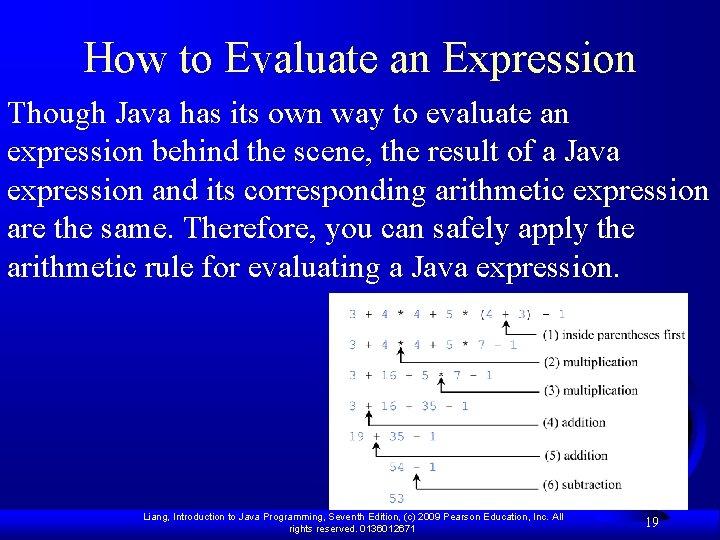
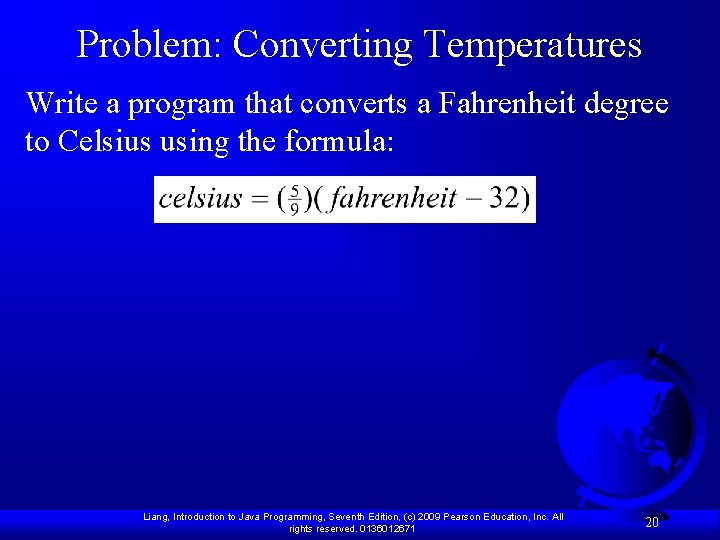
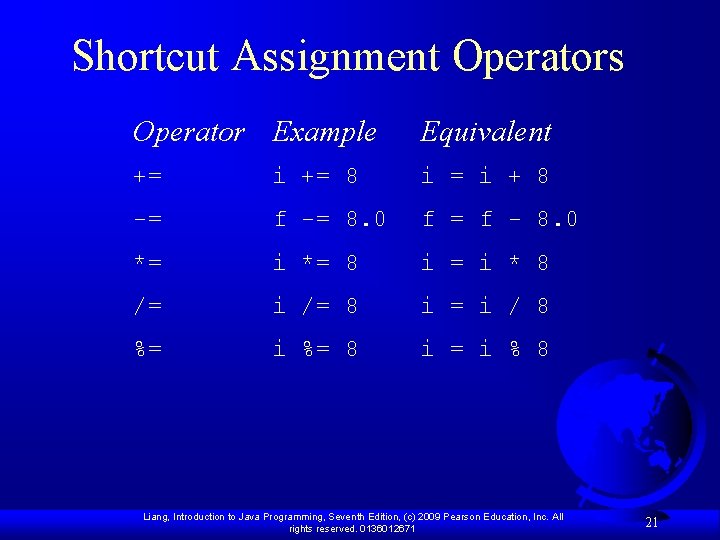
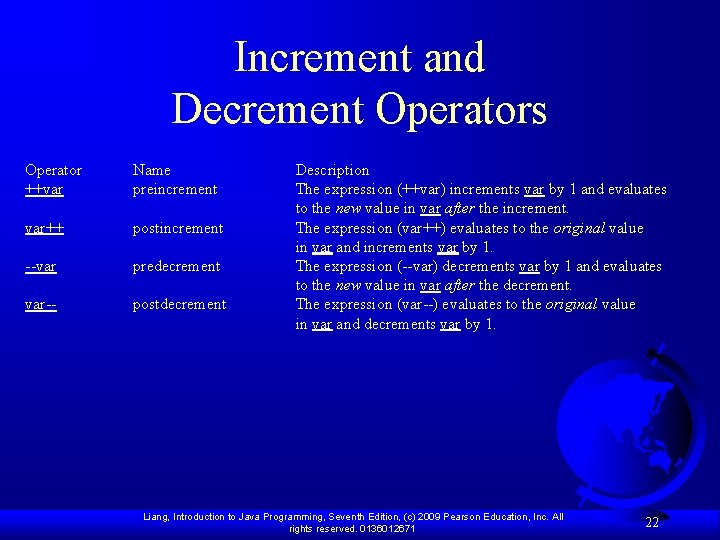
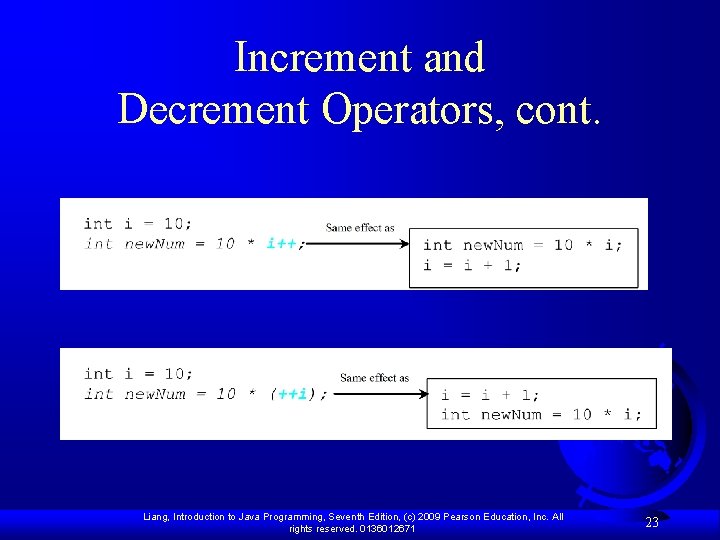
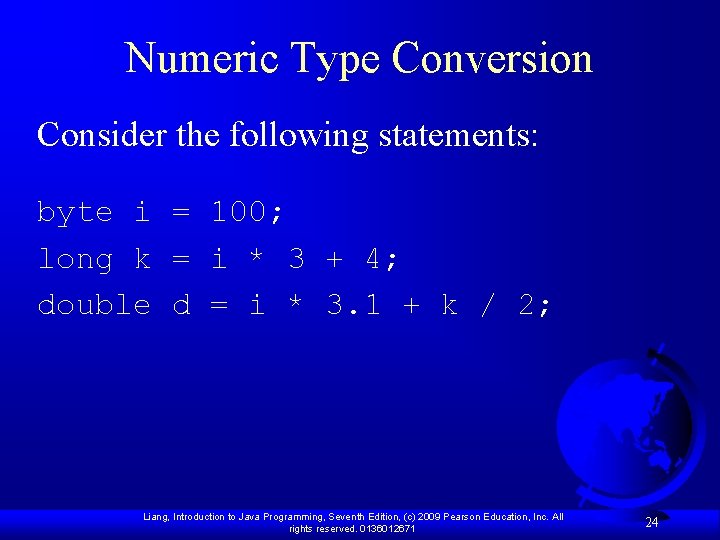
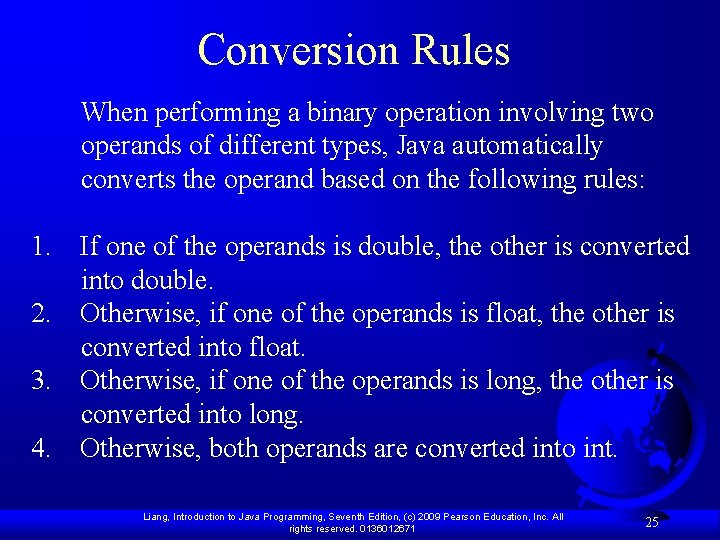
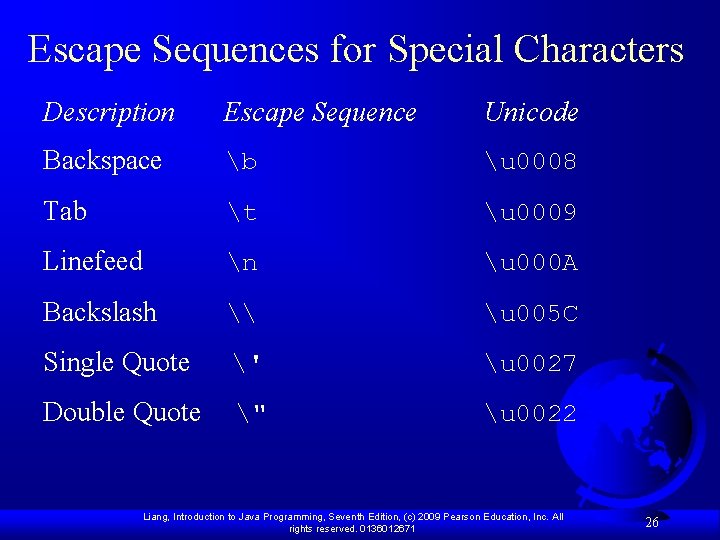
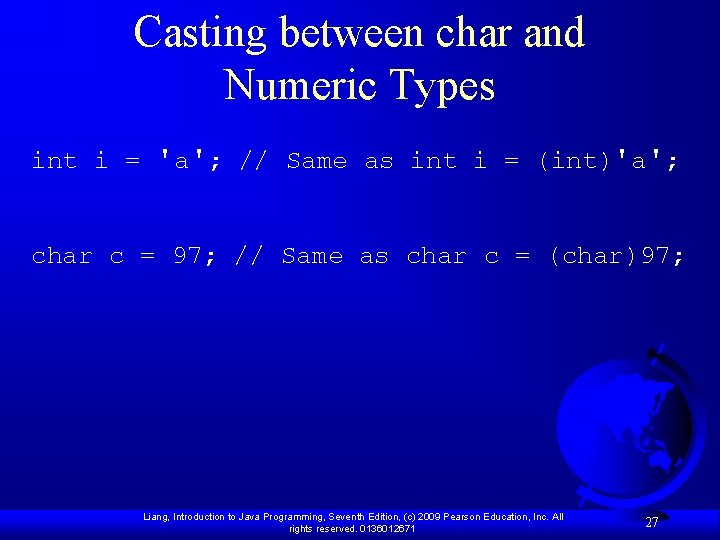
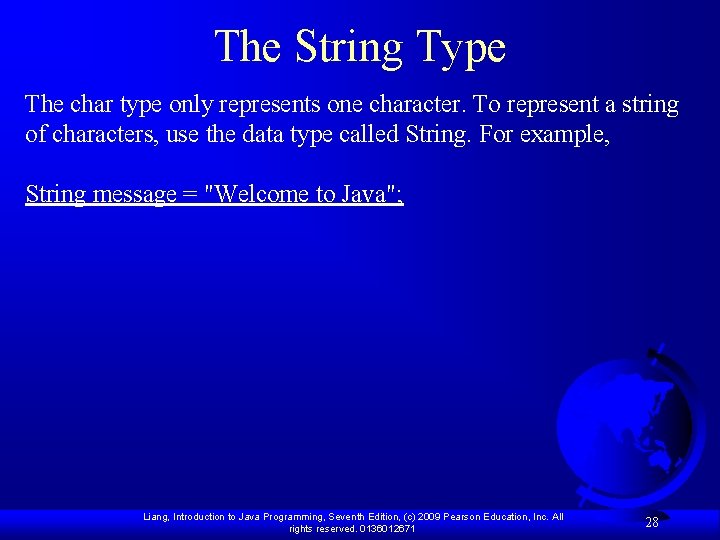
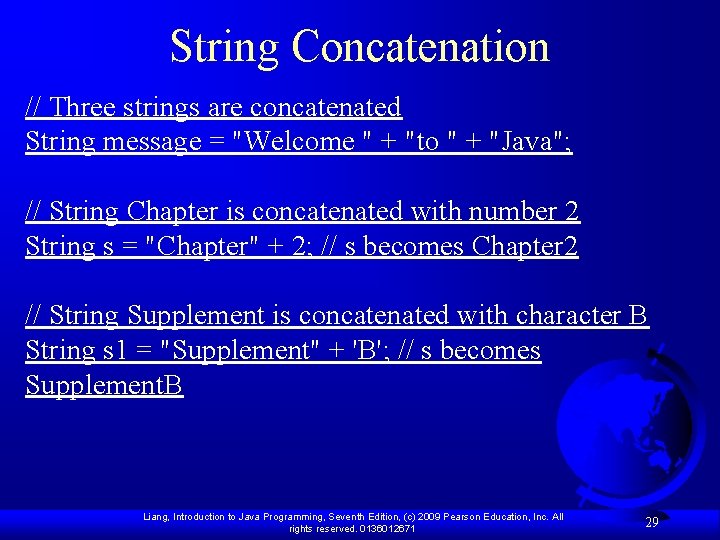
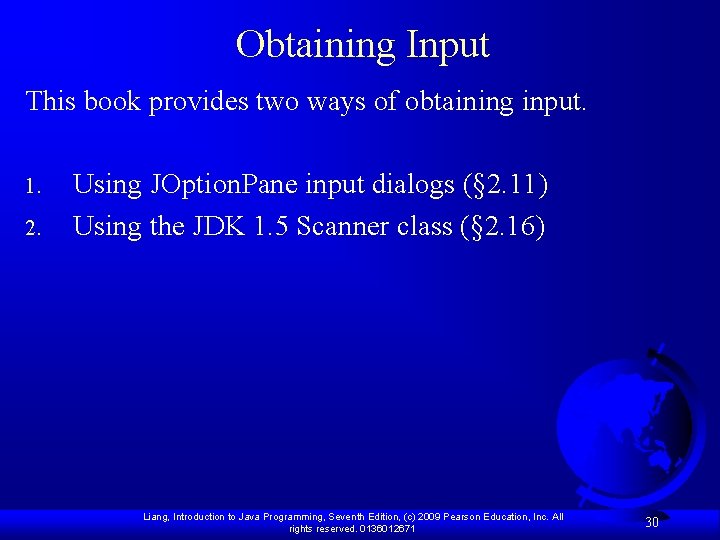
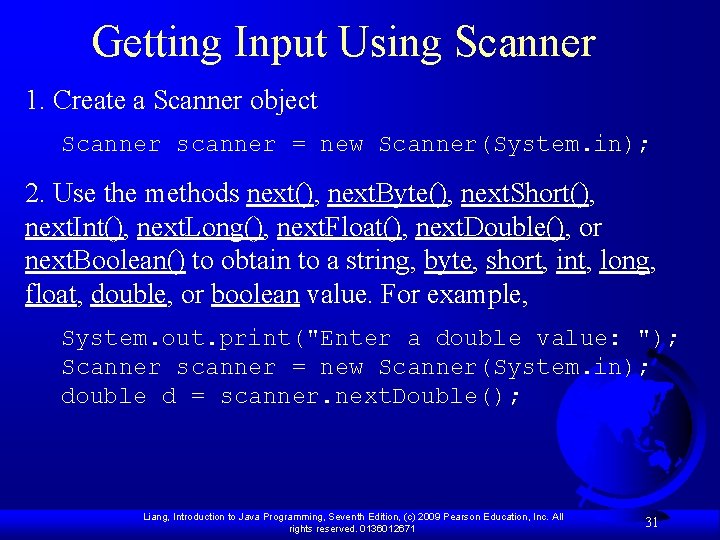
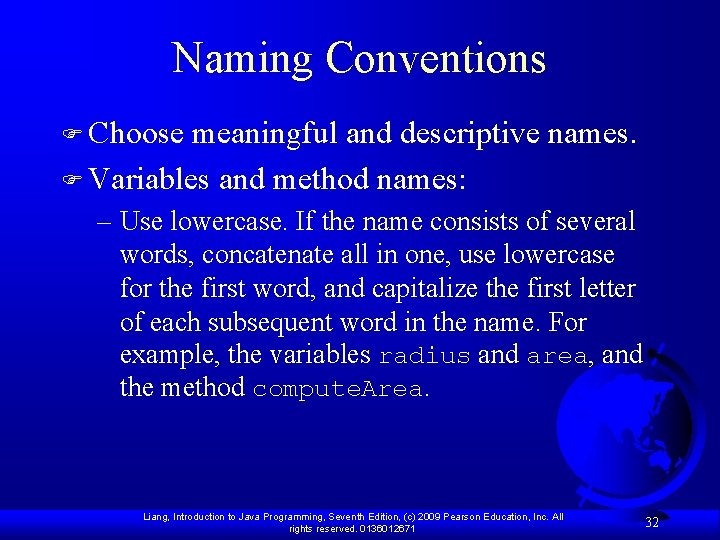
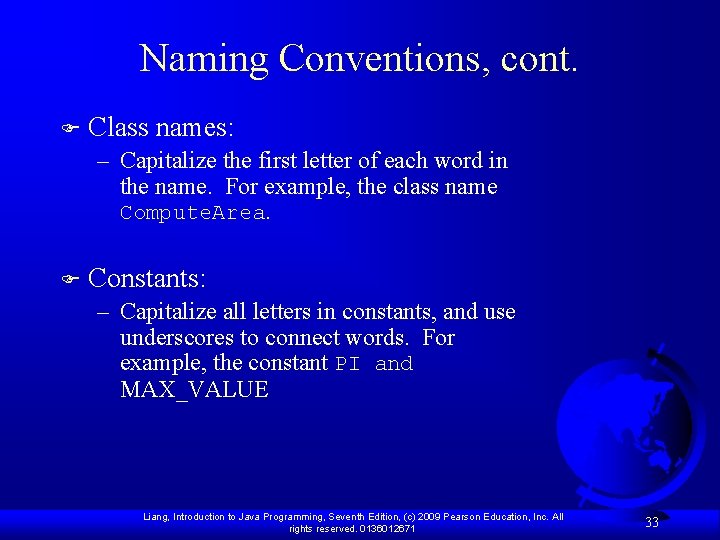
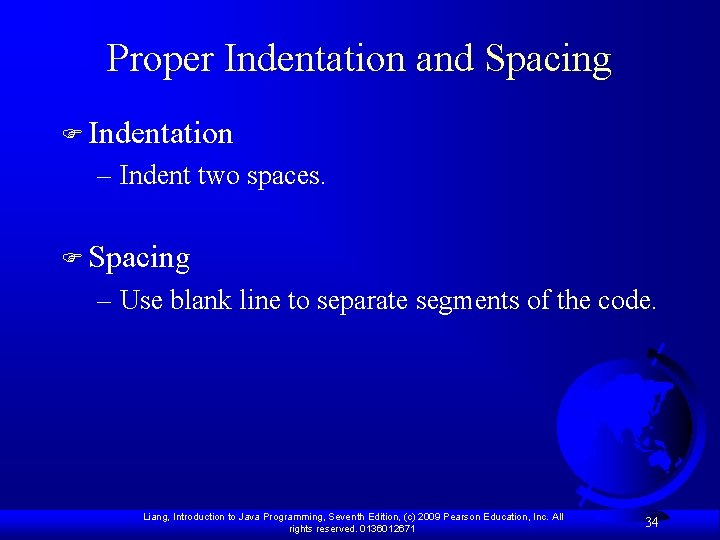
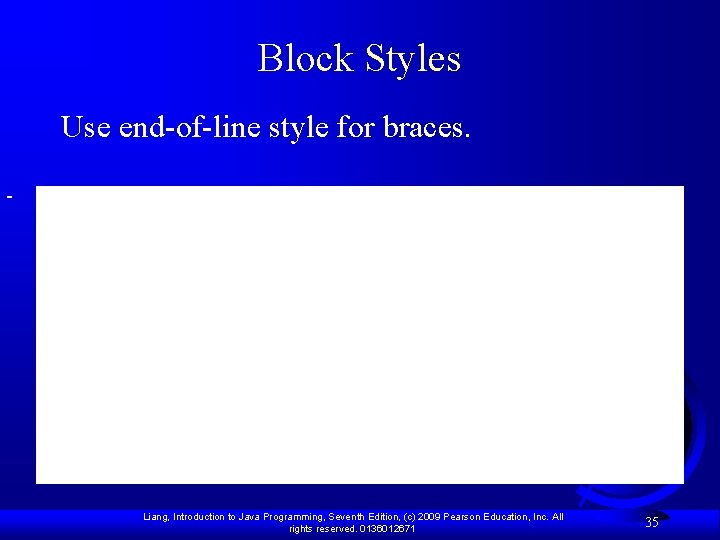
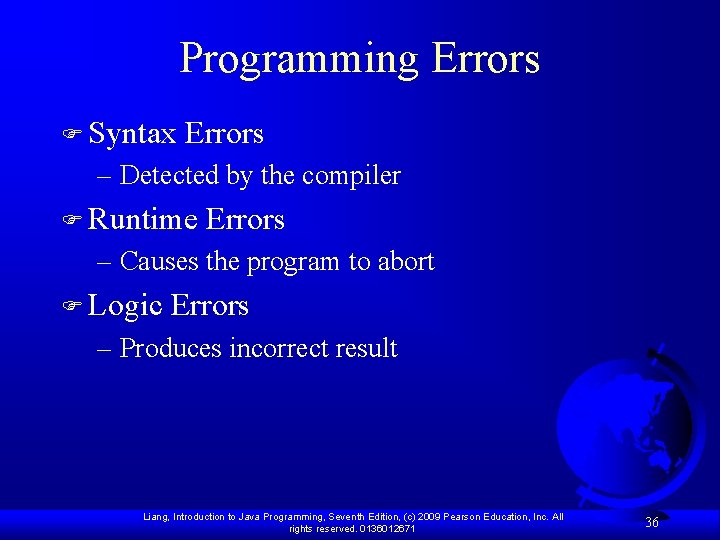
![Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) { Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/38e95ed9907c925105813a84c77451d1/image-37.jpg)
![Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) { Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/38e95ed9907c925105813a84c77451d1/image-38.jpg)
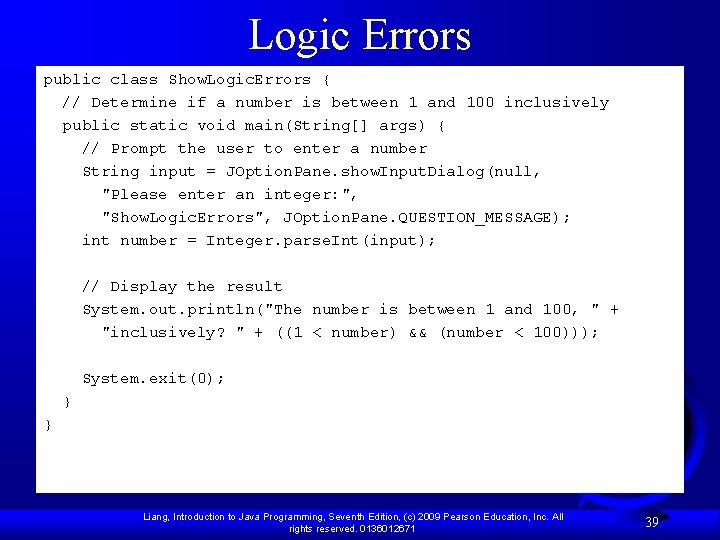
- Slides: 39
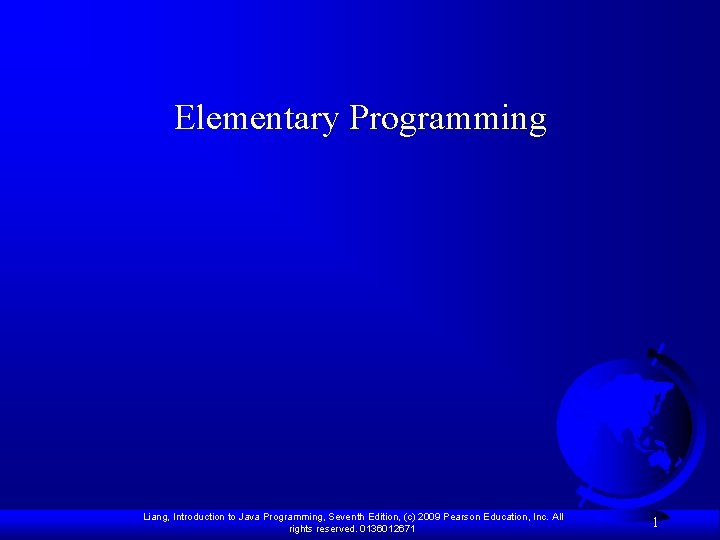
Elementary Programming Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 1
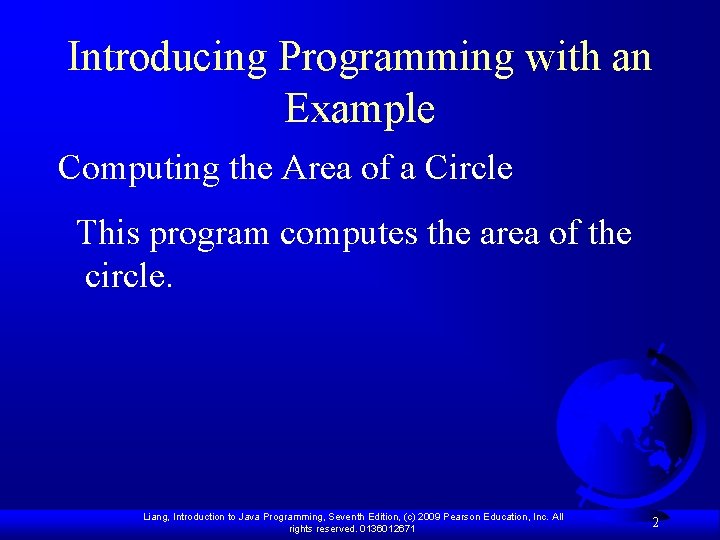
Introducing Programming with an Example Computing the Area of a Circle This program computes the area of the circle. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 2
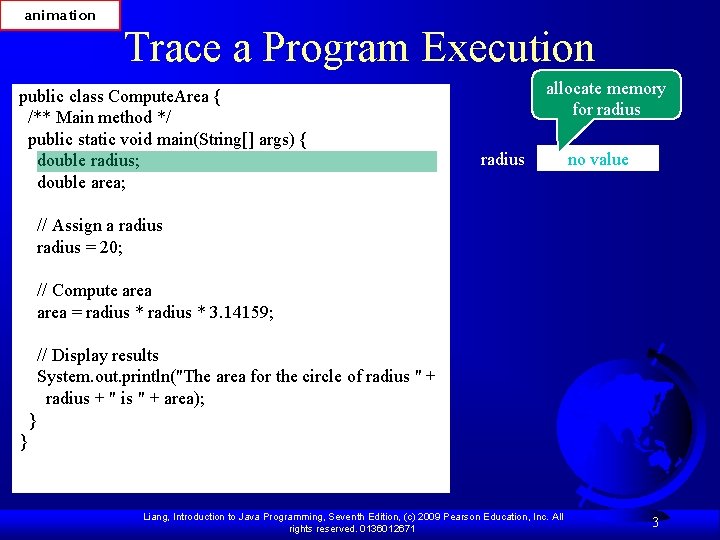
animation Trace a Program Execution public class Compute. Area { /** Main method */ public static void main(String[] args) { double radius; double area; // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } allocate memory for radius Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 no value 3
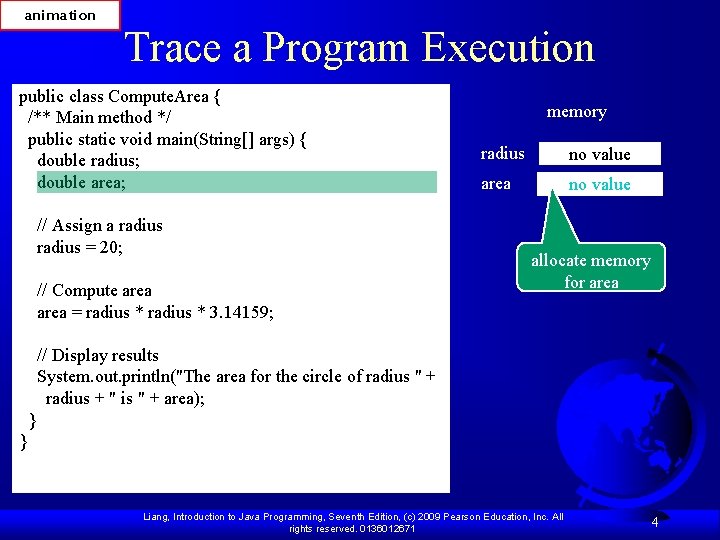
animation Trace a Program Execution public class Compute. Area { /** Main method */ public static void main(String[] args) { double radius; double area; // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } memory radius no value area no value allocate memory for area Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 4
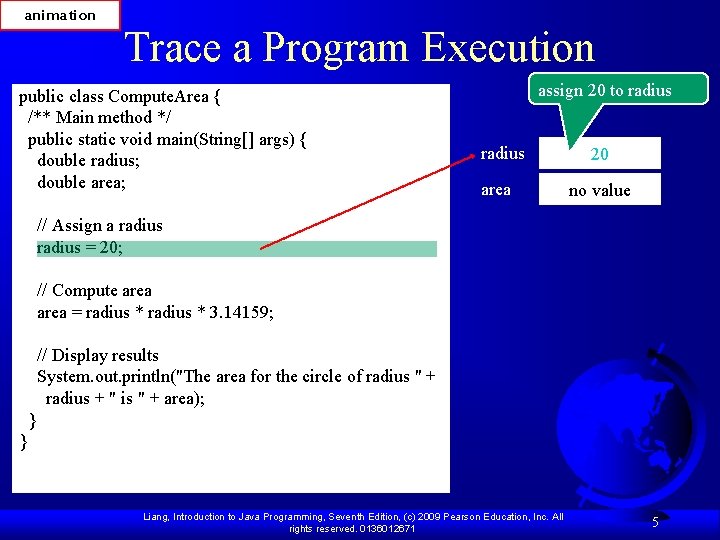
animation Trace a Program Execution public class Compute. Area { /** Main method */ public static void main(String[] args) { double radius; double area; // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } assign 20 to radius area Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 20 no value 5
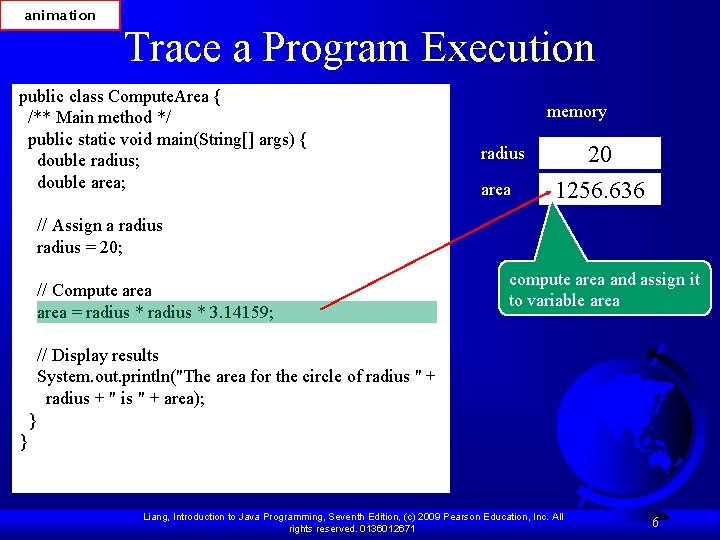
animation Trace a Program Execution public class Compute. Area { /** Main method */ public static void main(String[] args) { double radius; double area; // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } memory radius area 20 1256. 636 compute area and assign it to variable area Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 6
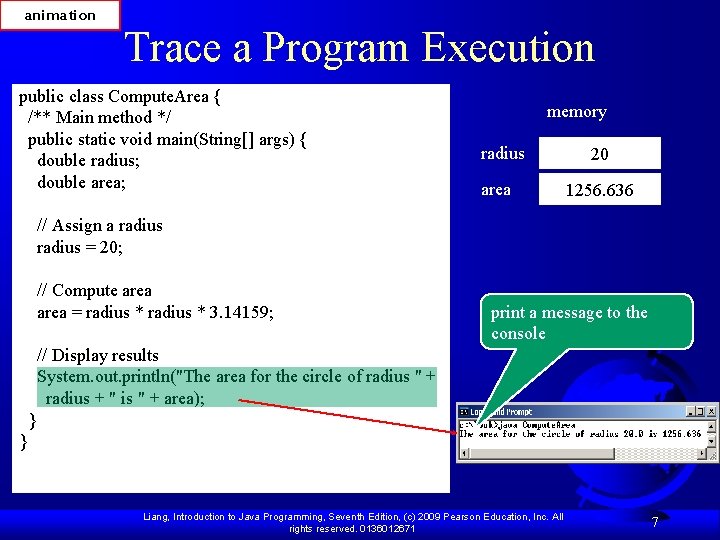
animation Trace a Program Execution public class Compute. Area { /** Main method */ public static void main(String[] args) { double radius; double area; // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } memory radius area 20 1256. 636 print a message to the console Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 7
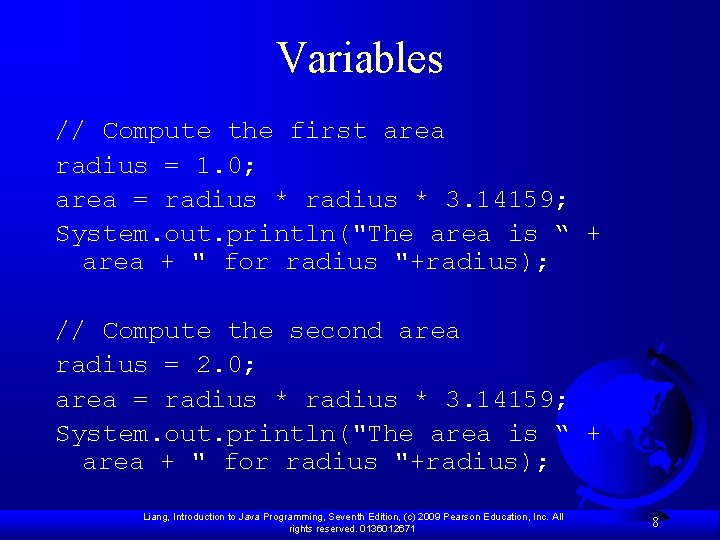
Variables // Compute the first area radius = 1. 0; area = radius * 3. 14159; System. out. println("The area is “ + area + " for radius "+radius); // Compute the second area radius = 2. 0; area = radius * 3. 14159; System. out. println("The area is “ + area + " for radius "+radius); Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 8
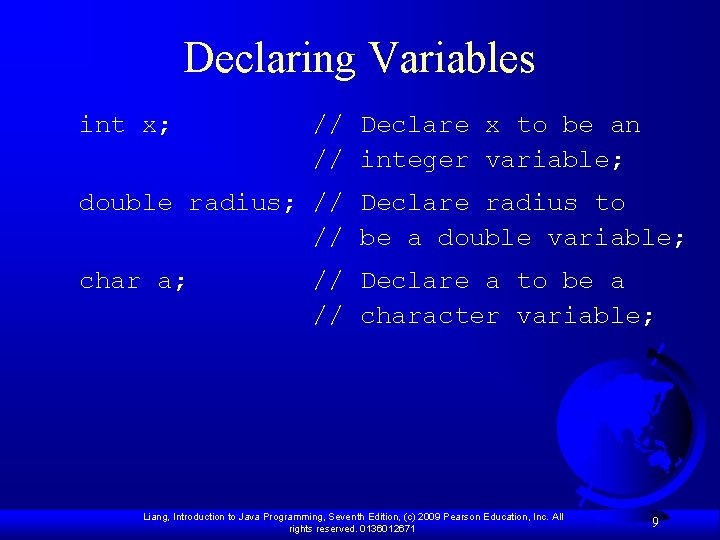
Declaring Variables int x; // Declare x to be an // integer variable; double radius; // Declare radius to // be a double variable; char a; // Declare a to be a // character variable; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 9
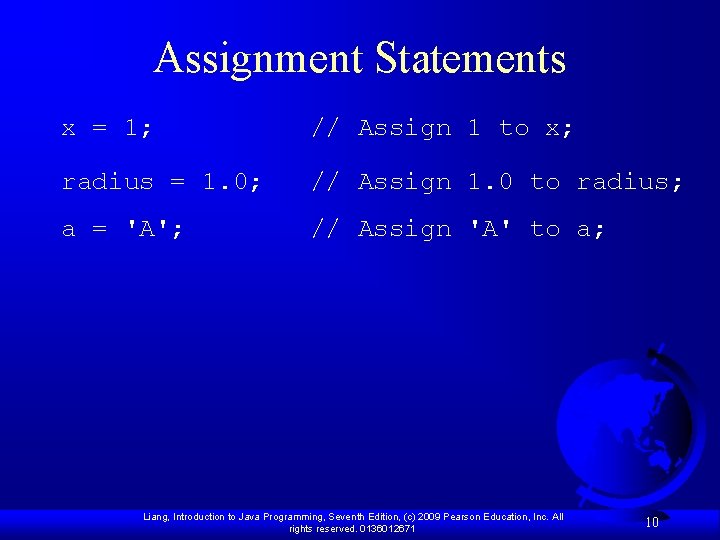
Assignment Statements x = 1; // Assign 1 to x; radius = 1. 0; // Assign 1. 0 to radius; a = 'A'; // Assign 'A' to a; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 10
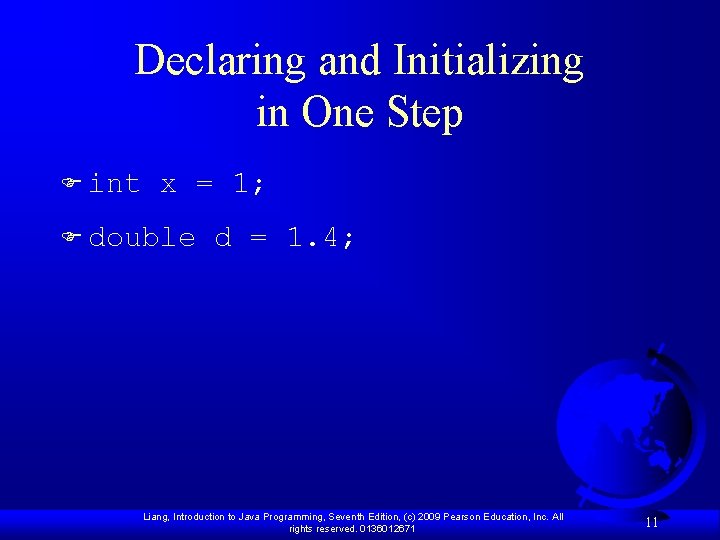
Declaring and Initializing in One Step F int x = 1; F double d = 1. 4; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 11
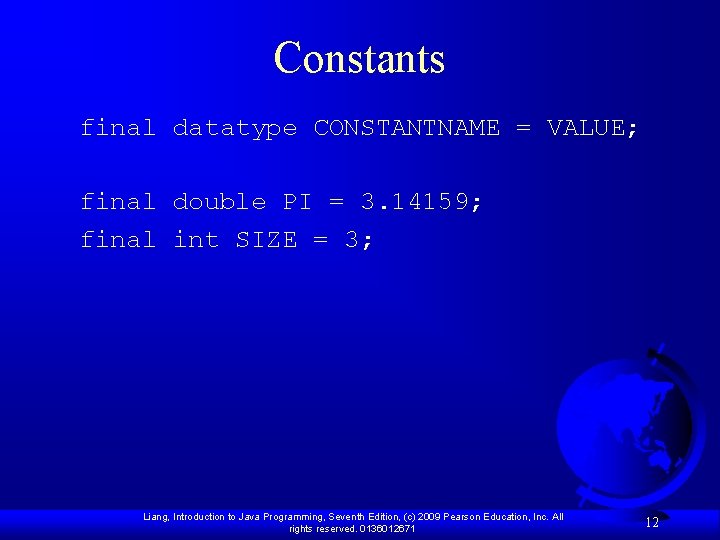
Constants final datatype CONSTANTNAME = VALUE; final double PI = 3. 14159; final int SIZE = 3; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 12
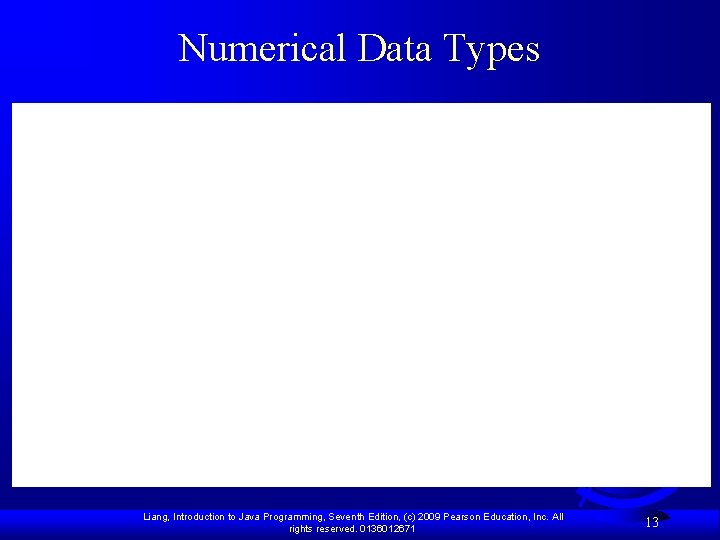
Numerical Data Types Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 13
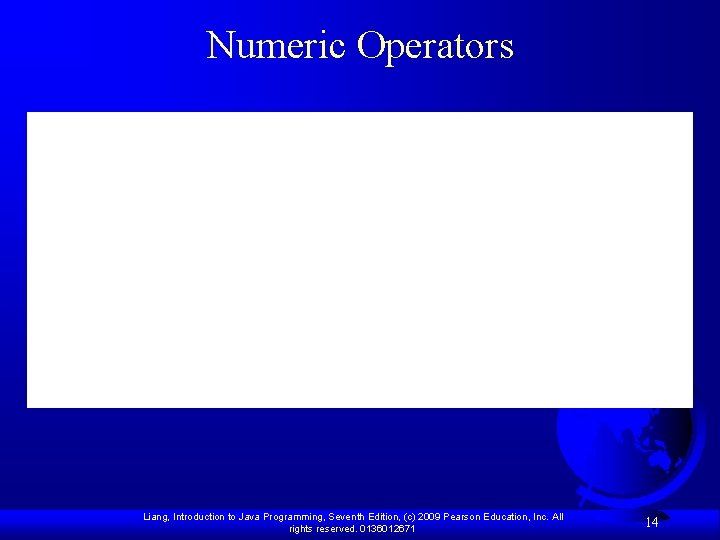
Numeric Operators Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 14
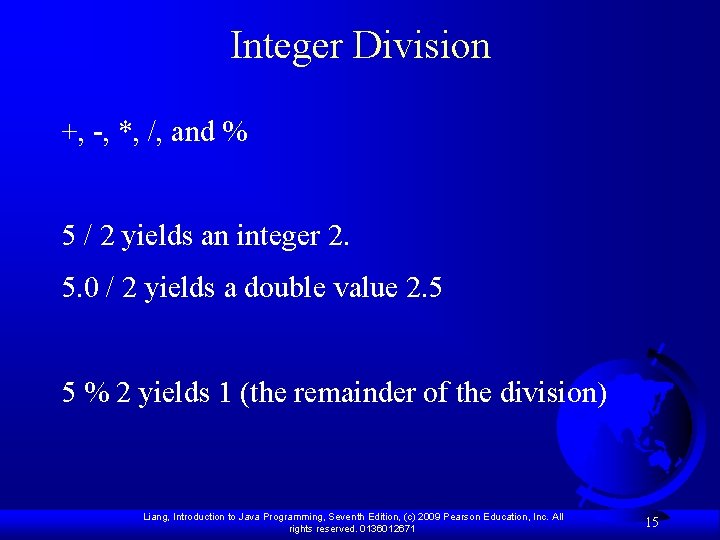
Integer Division +, -, *, /, and % 5 / 2 yields an integer 2. 5. 0 / 2 yields a double value 2. 5 5 % 2 yields 1 (the remainder of the division) Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 15
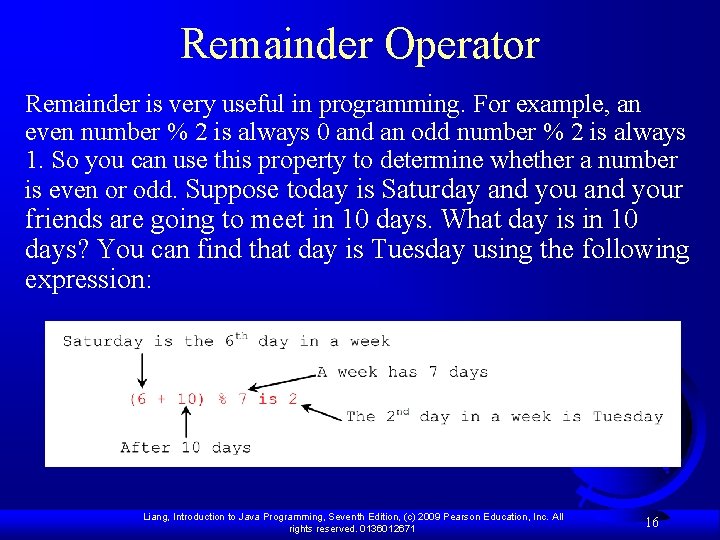
Remainder Operator Remainder is very useful in programming. For example, an even number % 2 is always 0 and an odd number % 2 is always 1. So you can use this property to determine whether a number is even or odd. Suppose today is Saturday and your friends are going to meet in 10 days. What day is in 10 days? You can find that day is Tuesday using the following expression: Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 16
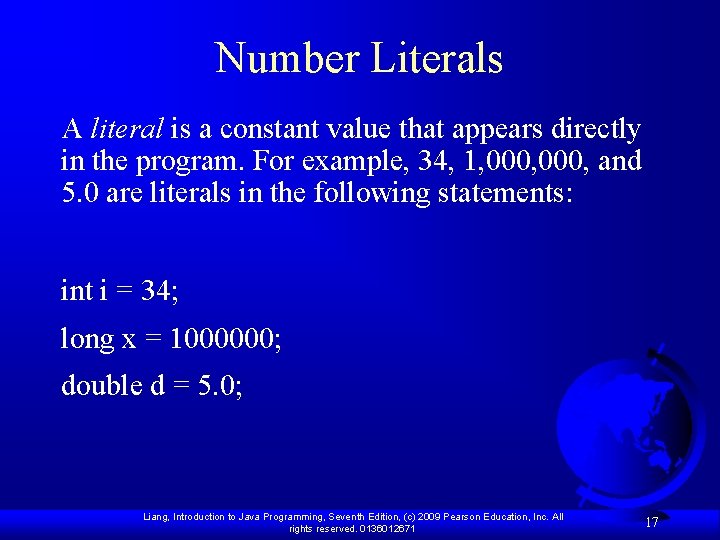
Number Literals A literal is a constant value that appears directly in the program. For example, 34, 1, 000, and 5. 0 are literals in the following statements: int i = 34; long x = 1000000; double d = 5. 0; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 17
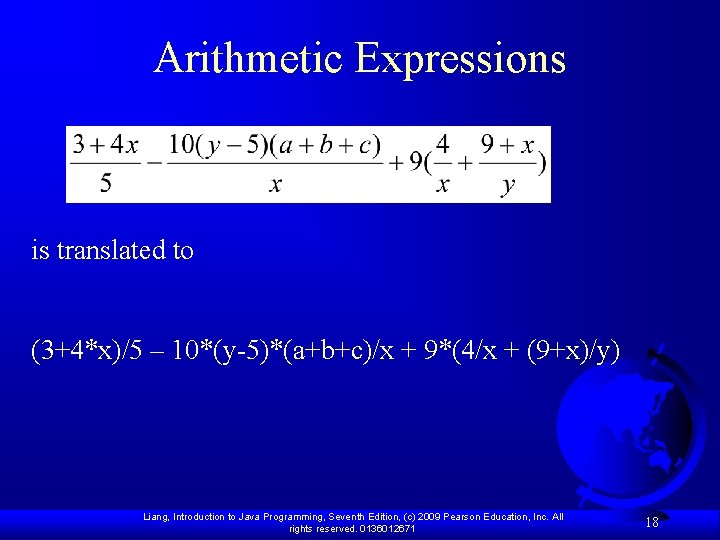
Arithmetic Expressions is translated to (3+4*x)/5 – 10*(y-5)*(a+b+c)/x + 9*(4/x + (9+x)/y) Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 18
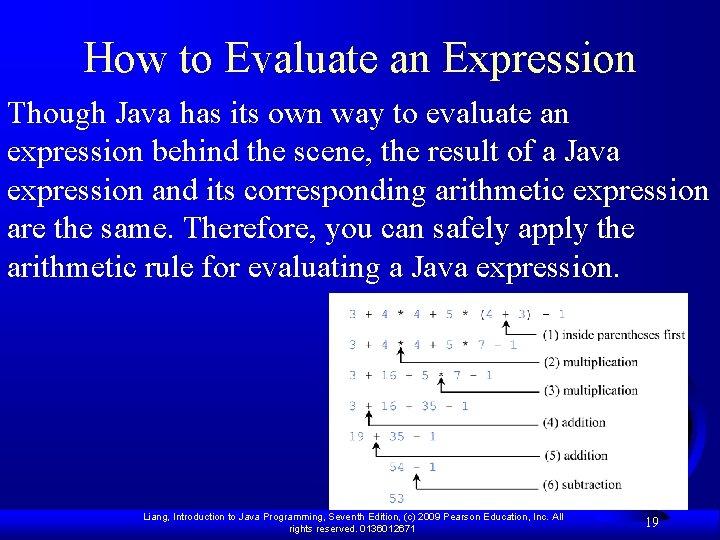
How to Evaluate an Expression Though Java has its own way to evaluate an expression behind the scene, the result of a Java expression and its corresponding arithmetic expression are the same. Therefore, you can safely apply the arithmetic rule for evaluating a Java expression. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 19
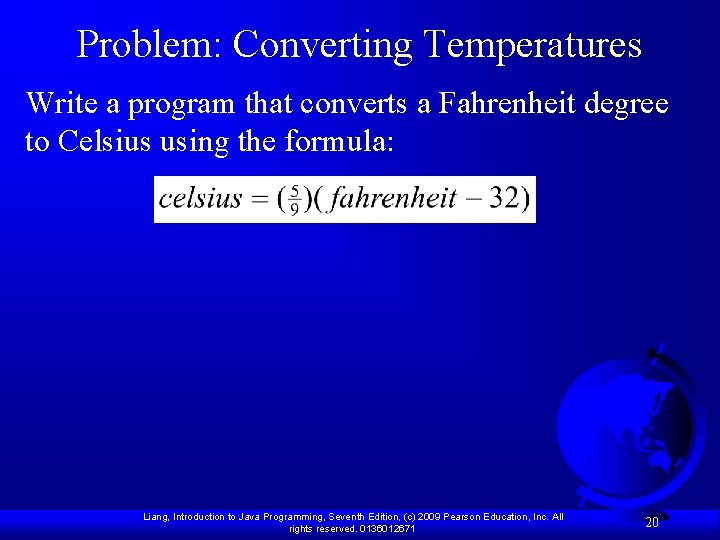
Problem: Converting Temperatures Write a program that converts a Fahrenheit degree to Celsius using the formula: Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 20
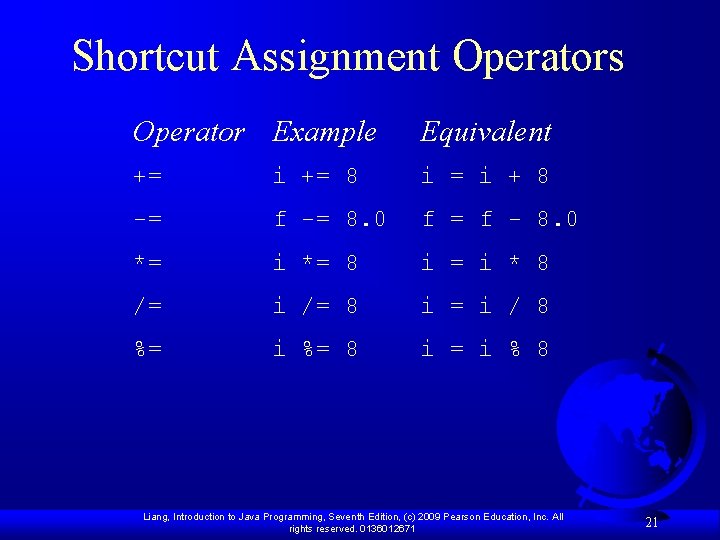
Shortcut Assignment Operators Operator Example Equivalent += i += 8 i = i + 8 -= f -= 8. 0 f = f - 8. 0 *= i *= 8 i = i * 8 /= i /= 8 i = i / 8 %= i %= 8 i = i % 8 Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 21
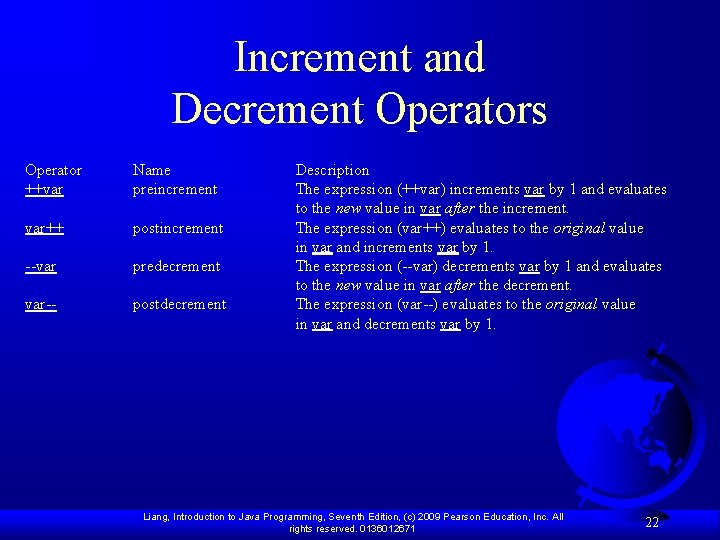
Increment and Decrement Operators Operator ++var Name preincrement var++ postincrement --var predecrement var-- postdecrement Description The expression (++var) increments var by 1 and evaluates to the new value in var after the increment. The expression (var++) evaluates to the original value in var and increments var by 1. The expression (--var) decrements var by 1 and evaluates to the new value in var after the decrement. The expression (var--) evaluates to the original value in var and decrements var by 1. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 22
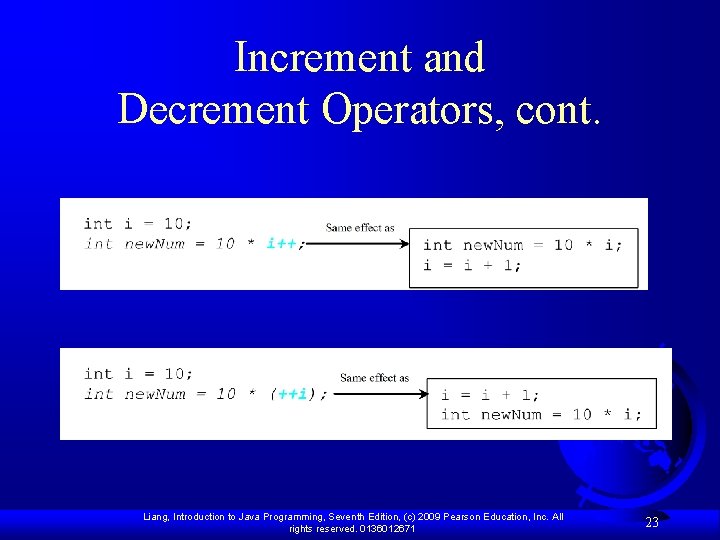
Increment and Decrement Operators, cont. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 23
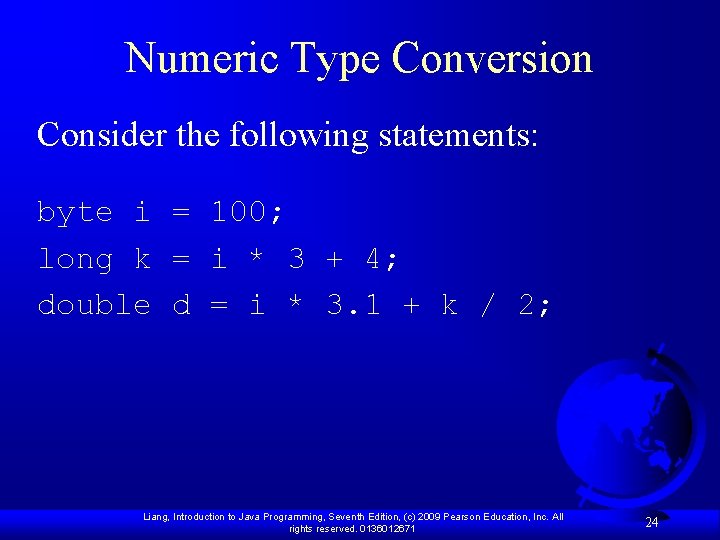
Numeric Type Conversion Consider the following statements: byte i = 100; long k = i * 3 + 4; double d = i * 3. 1 + k / 2; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 24
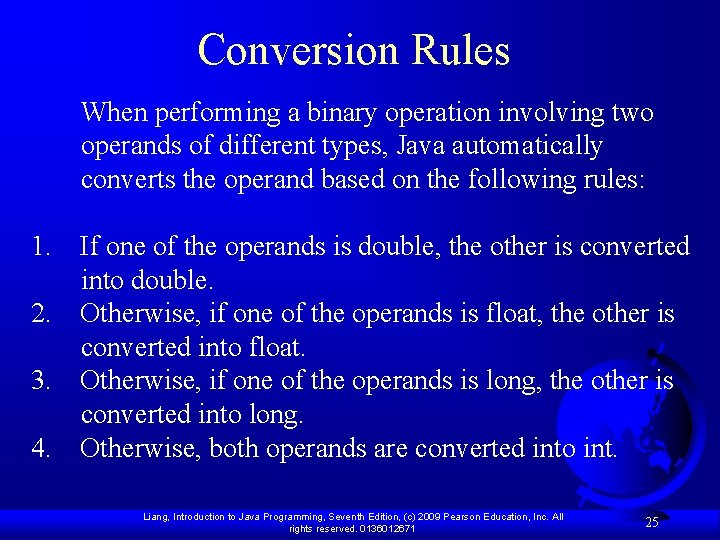
Conversion Rules When performing a binary operation involving two operands of different types, Java automatically converts the operand based on the following rules: 1. If one of the operands is double, the other is converted into double. 2. Otherwise, if one of the operands is float, the other is converted into float. 3. Otherwise, if one of the operands is long, the other is converted into long. 4. Otherwise, both operands are converted into int. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 25
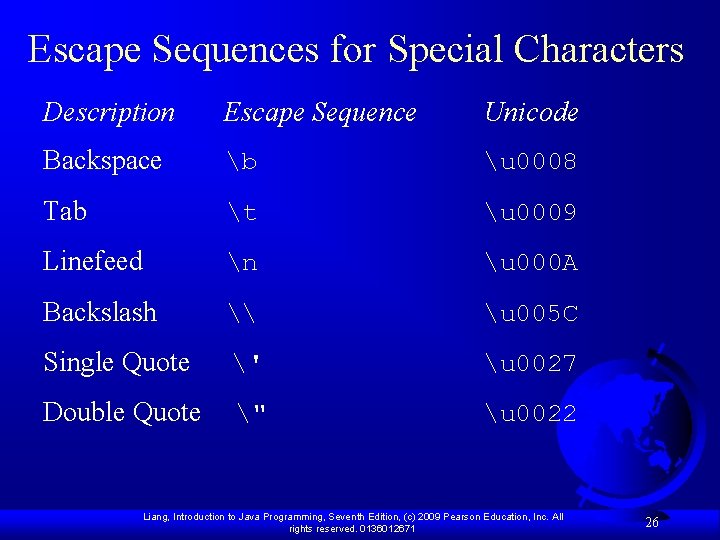
Escape Sequences for Special Characters Description Escape Sequence Unicode Backspace b u 0008 Tab t u 0009 Linefeed n u 000 A Backslash \ u 005 C Single Quote ' u 0027 Double Quote " u 0022 Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 26
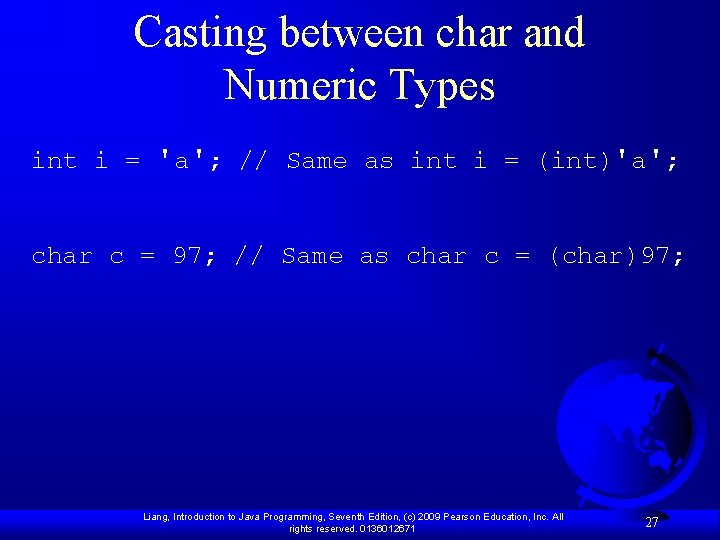
Casting between char and Numeric Types int i = 'a'; // Same as int i = (int)'a'; char c = 97; // Same as char c = (char)97; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 27
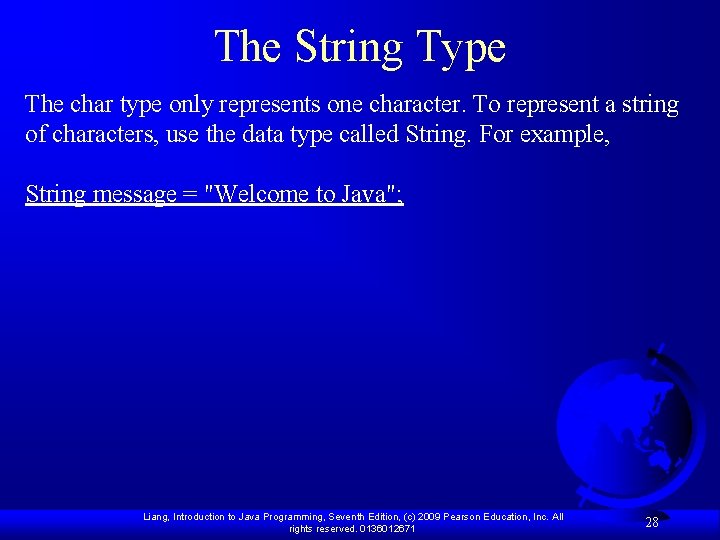
The String Type The char type only represents one character. To represent a string of characters, use the data type called String. For example, String message = "Welcome to Java"; Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 28
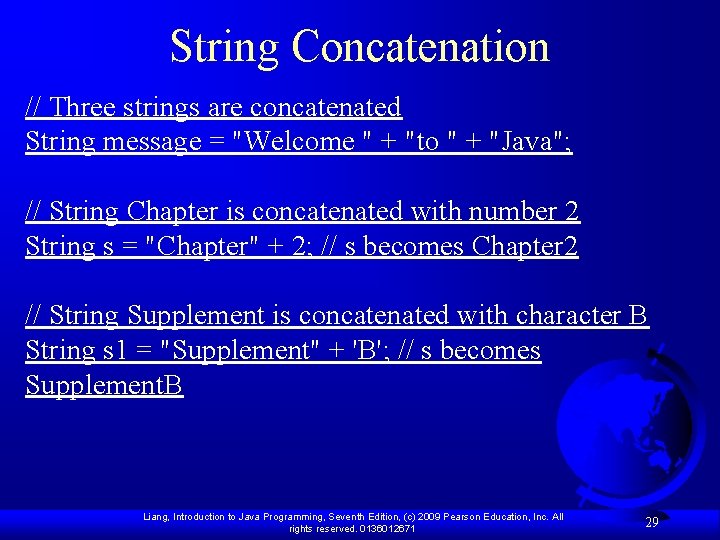
String Concatenation // Three strings are concatenated String message = "Welcome " + "to " + "Java"; // String Chapter is concatenated with number 2 String s = "Chapter" + 2; // s becomes Chapter 2 // String Supplement is concatenated with character B String s 1 = "Supplement" + 'B'; // s becomes Supplement. B Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 29
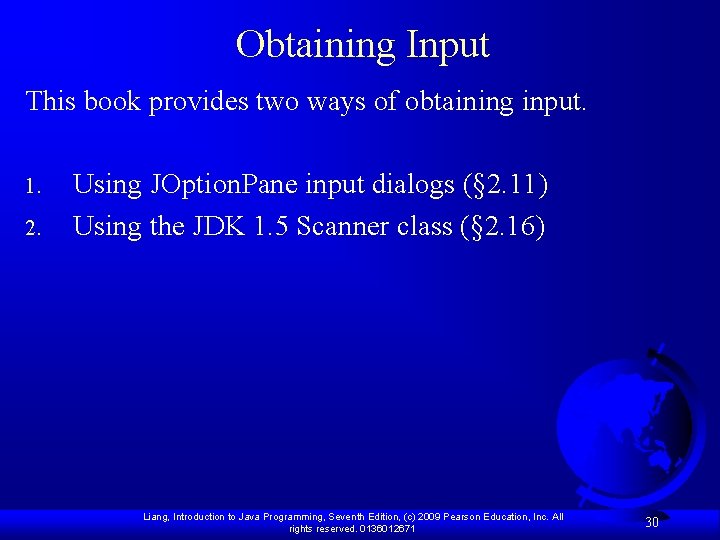
Obtaining Input This book provides two ways of obtaining input. 1. 2. Using JOption. Pane input dialogs (§ 2. 11) Using the JDK 1. 5 Scanner class (§ 2. 16) Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 30
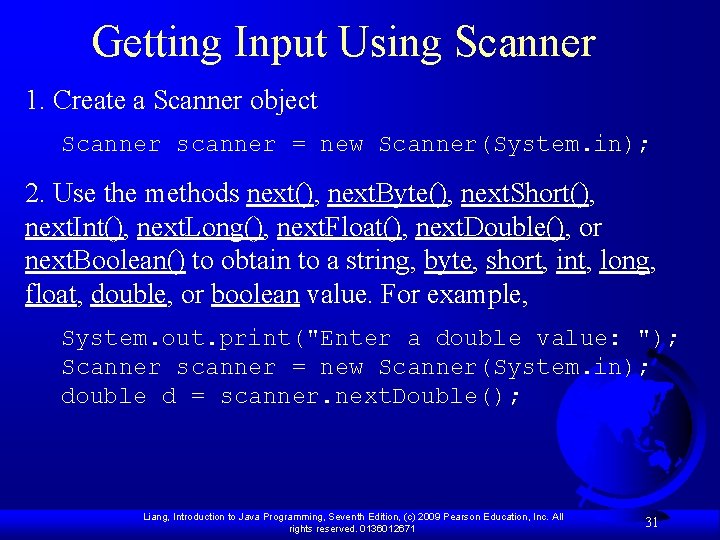
Getting Input Using Scanner 1. Create a Scanner object Scanner scanner = new Scanner(System. in); 2. Use the methods next(), next. Byte(), next. Short(), next. Int(), next. Long(), next. Float(), next. Double(), or next. Boolean() to obtain to a string, byte, short, int, long, float, double, or boolean value. For example, System. out. print("Enter a double value: "); Scanner scanner = new Scanner(System. in); double d = scanner. next. Double(); Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 31
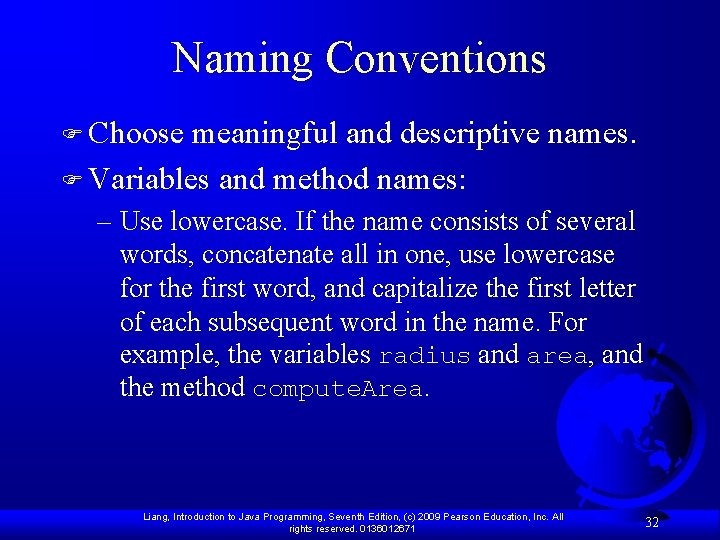
Naming Conventions F Choose meaningful and descriptive names. F Variables and method names: – Use lowercase. If the name consists of several words, concatenate all in one, use lowercase for the first word, and capitalize the first letter of each subsequent word in the name. For example, the variables radius and area, and the method compute. Area. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 32
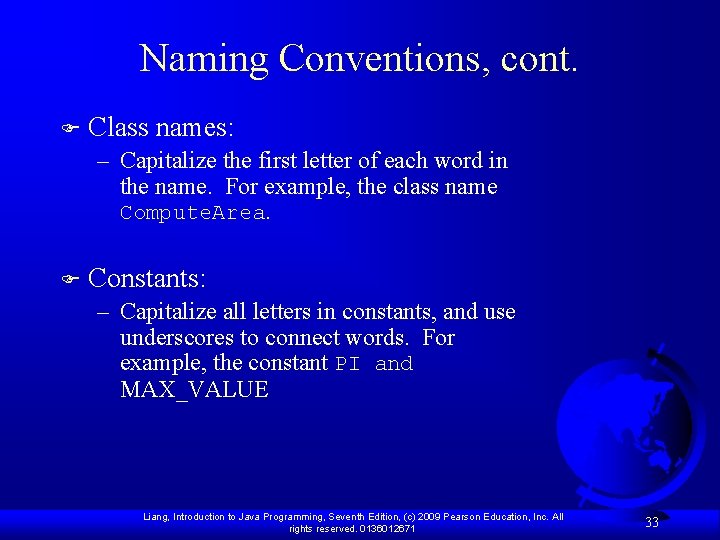
Naming Conventions, cont. F Class names: – Capitalize the first letter of each word in the name. For example, the class name Compute. Area. F Constants: – Capitalize all letters in constants, and use underscores to connect words. For example, the constant PI and MAX_VALUE Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 33
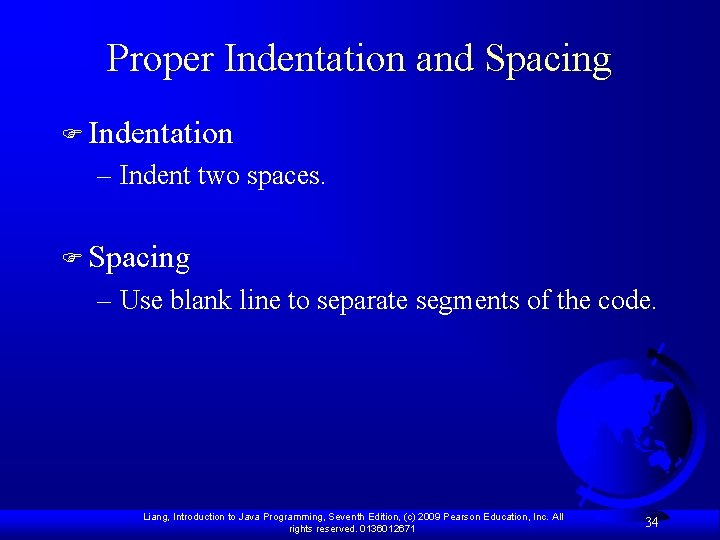
Proper Indentation and Spacing F Indentation – Indent two spaces. F Spacing – Use blank line to separate segments of the code. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 34
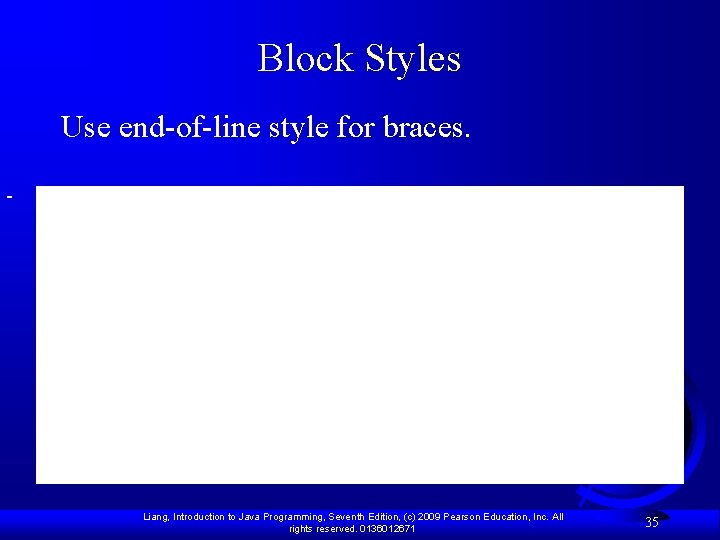
Block Styles Use end-of-line style for braces. Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 35
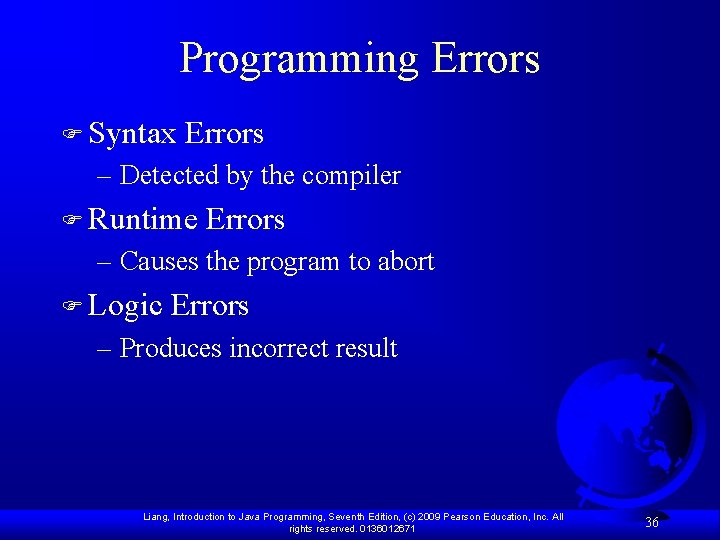
Programming Errors F Syntax Errors – Detected by the compiler F Runtime Errors – Causes the program to abort F Logic Errors – Produces incorrect result Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 36
![Syntax Errors public class Show Syntax Errors public static void mainString args Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/38e95ed9907c925105813a84c77451d1/image-37.jpg)
Syntax Errors public class Show. Syntax. Errors { public static void main(String[] args) { i = 30; System. out. println(i + 4); } } Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 37
![Runtime Errors public class Show Runtime Errors public static void mainString args Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/38e95ed9907c925105813a84c77451d1/image-38.jpg)
Runtime Errors public class Show. Runtime. Errors { public static void main(String[] args) { int i = 1 / 0; } } Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 38
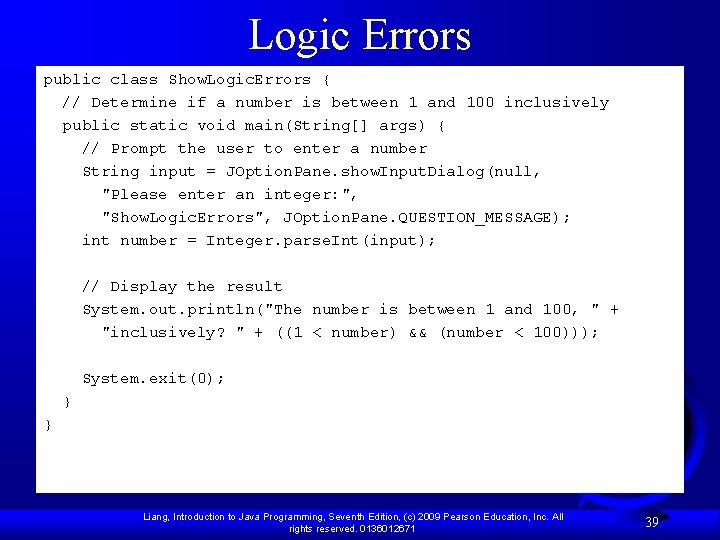
Logic Errors public class Show. Logic. Errors { // Determine if a number is between 1 and 100 inclusively public static void main(String[] args) { // Prompt the user to enter a number String input = JOption. Pane. show. Input. Dialog(null, "Please enter an integer: ", "Show. Logic. Errors", JOption. Pane. QUESTION_MESSAGE); int number = Integer. parse. Int(input); // Display the result System. out. println("The number is between 1 and 100, " + "inclusively? " + ((1 < number) && (number < 100))); System. exit(0); } } Liang, Introduction to Java Programming, Seventh Edition, (c) 2009 Pearson Education, Inc. All rights reserved. 0136012671 39
Elementary programming in java
Liang fontdemo.java
Liang fontdemo.java
Elementary programming in java
Elementary programming in java
Seventh chord inversions
Problem solving
Java an introduction to problem solving and programming
Java introduction to problem solving and programming
Introduction to java programming 10th edition quizzes
Richard seow yung liang
Zhuo shi wo li liang
Zhong hanliang
Yuanxing liang
Amber liang
Mengindeks nama toko
Randy liang
David teo choon liang
How was ma liang like
Zong-liang yang
Liang jianhui
Nba schedule maker
Chia liang cheng
Materi otk keuangan kelas 11 semester 1
Umbc mechanical engineering
Zong-liang yang
Siemens sans bold
Dr liang hao nan
Pengertian arsip dan kearsipan
Anticorps anti-nucléaire positif moucheté
Kathleen liang
Edmund liang
Cakera dwicekung
Sushil dubey intel
Diana liang faa
Foo joon liang
Shahriar 1 com assignment
Chapter 2 elementary programming
Satan from the seventh grade
Characters in the seventh man