Chapter 15 Functional Programming Languages Chapter 15 Introduction
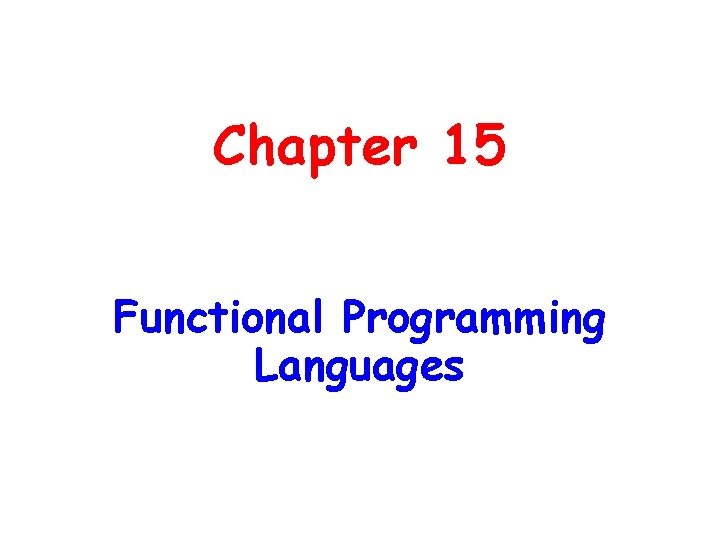
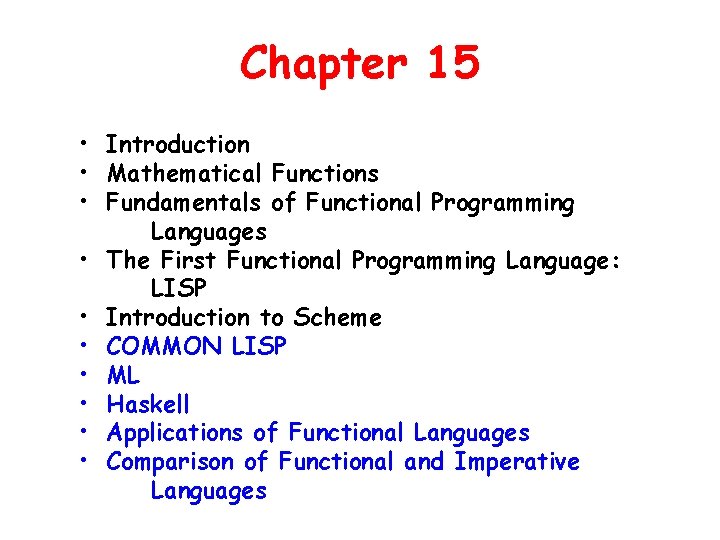
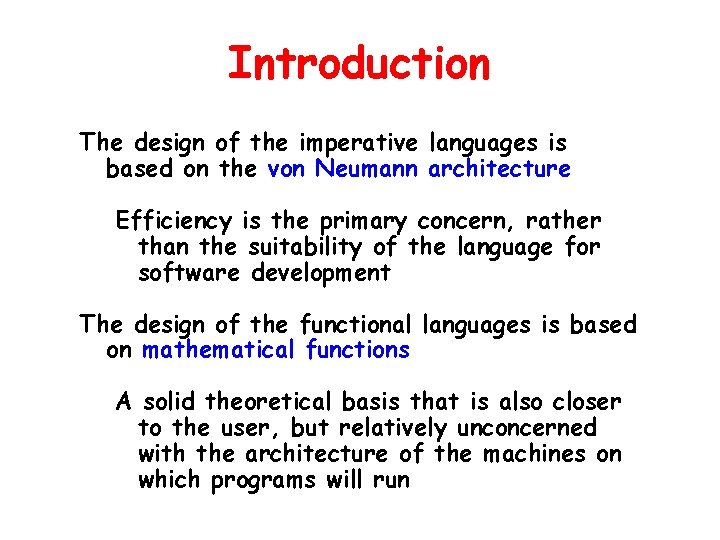
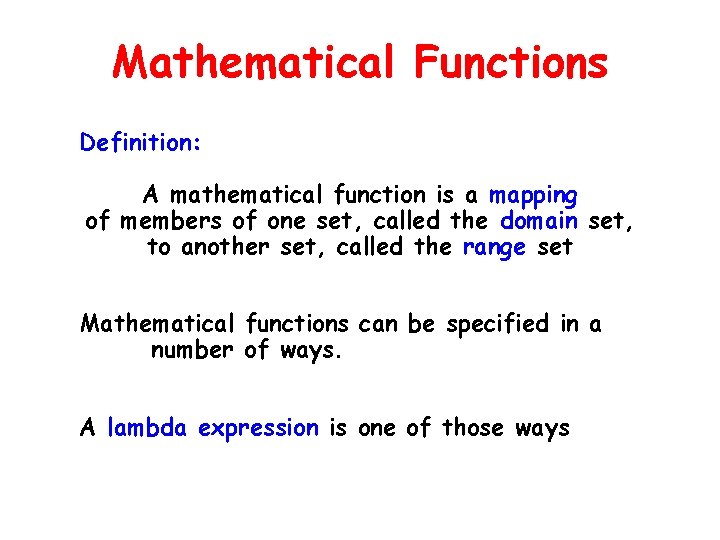
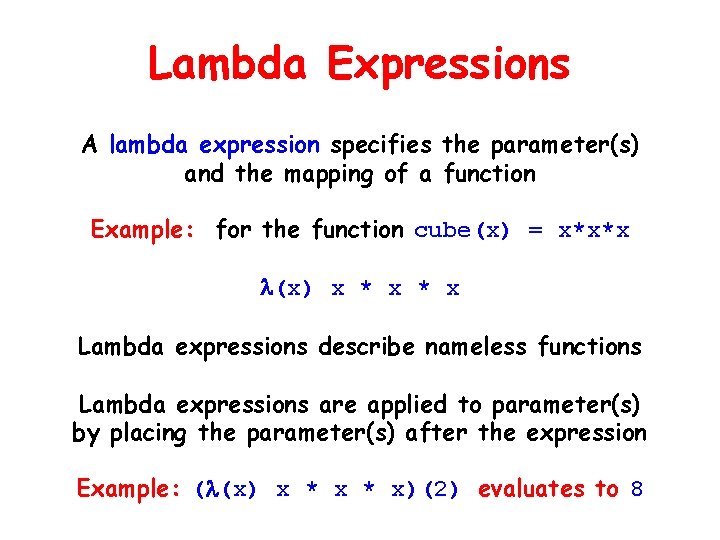
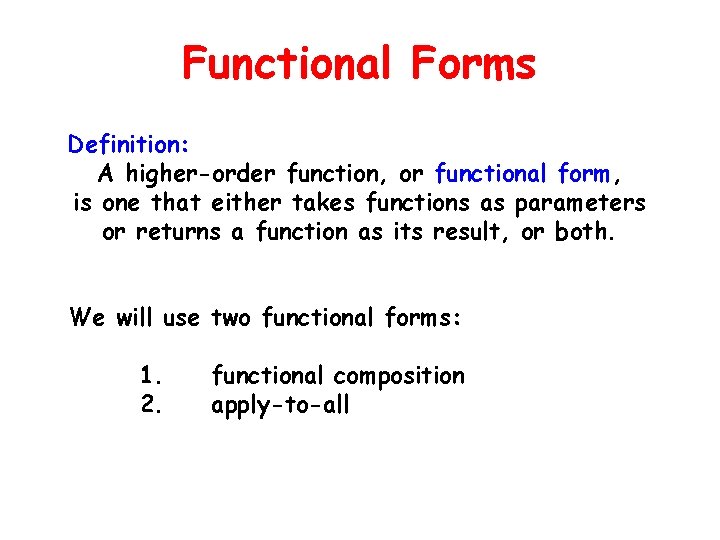
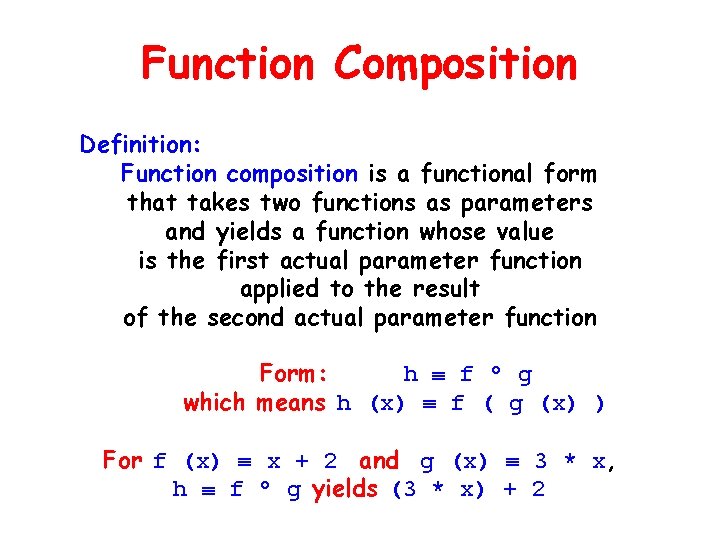
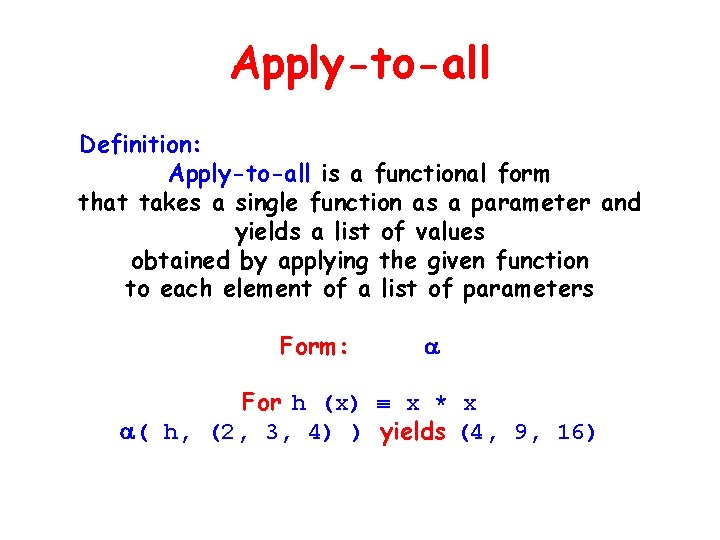
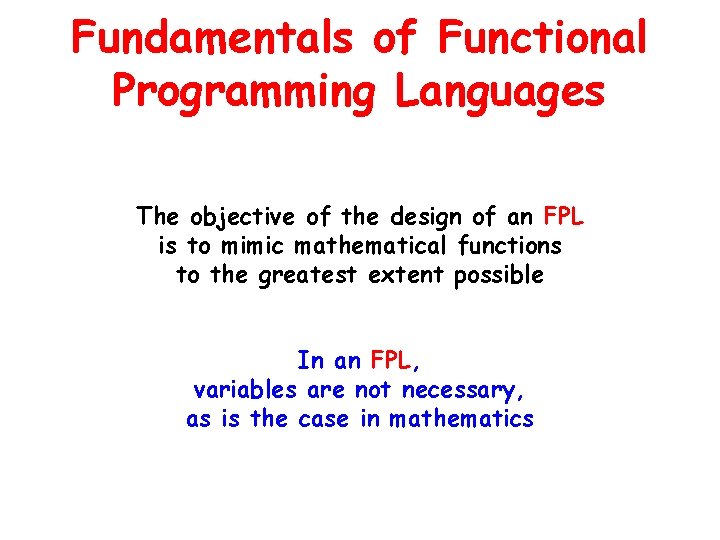
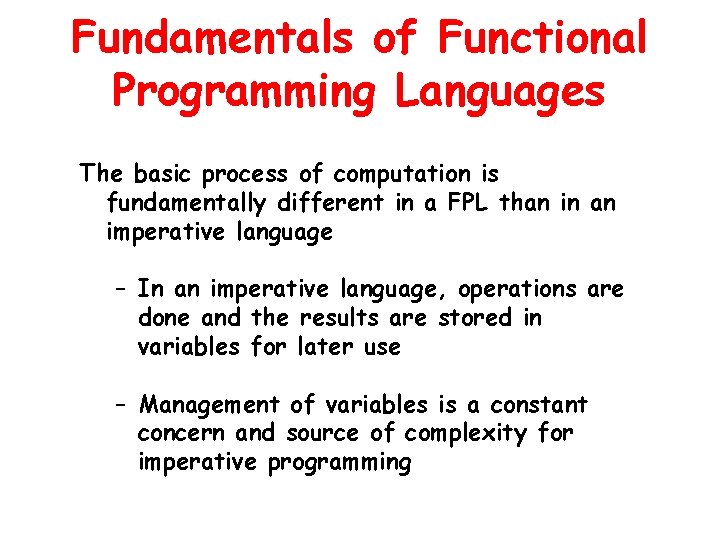
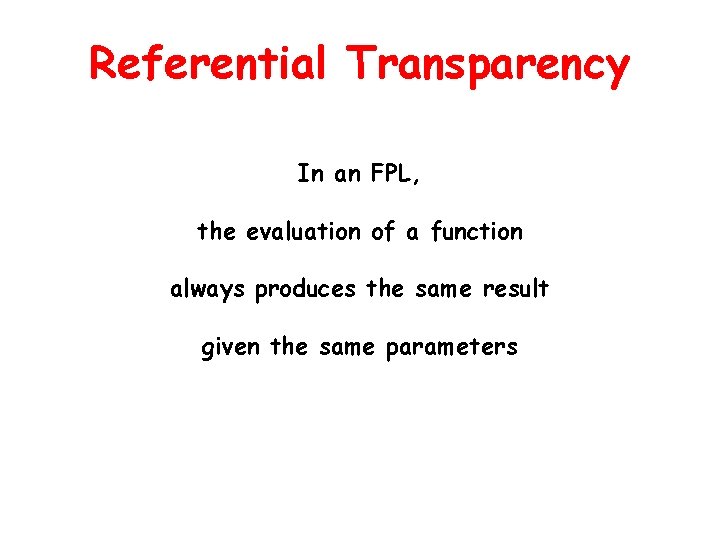
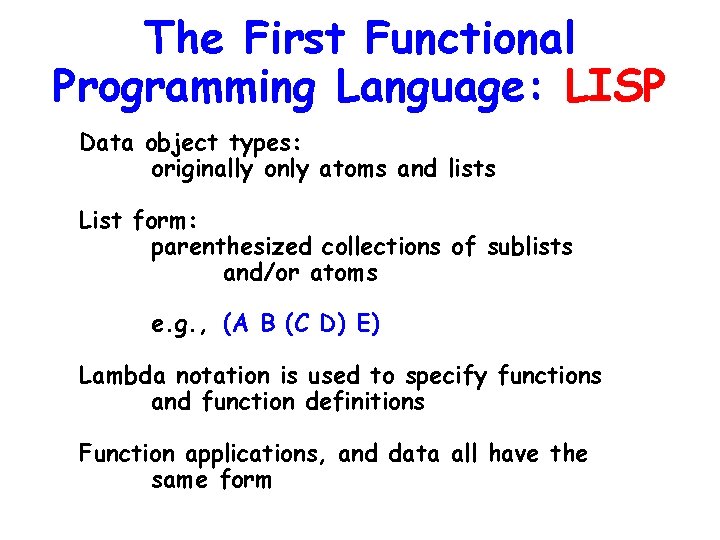
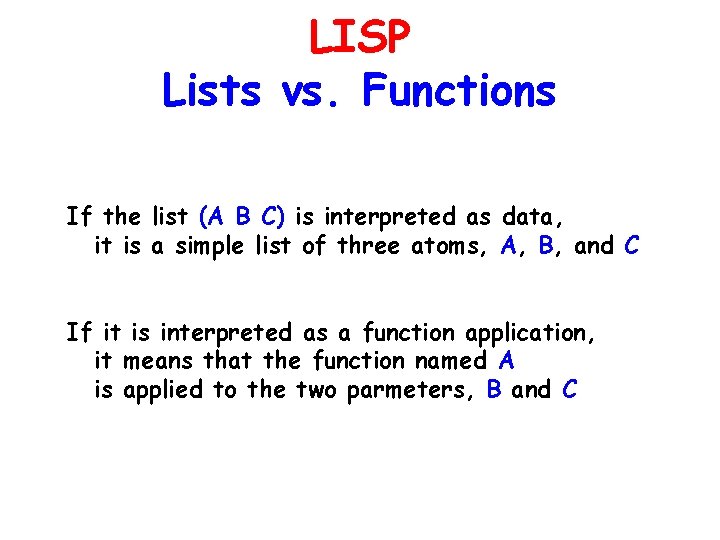
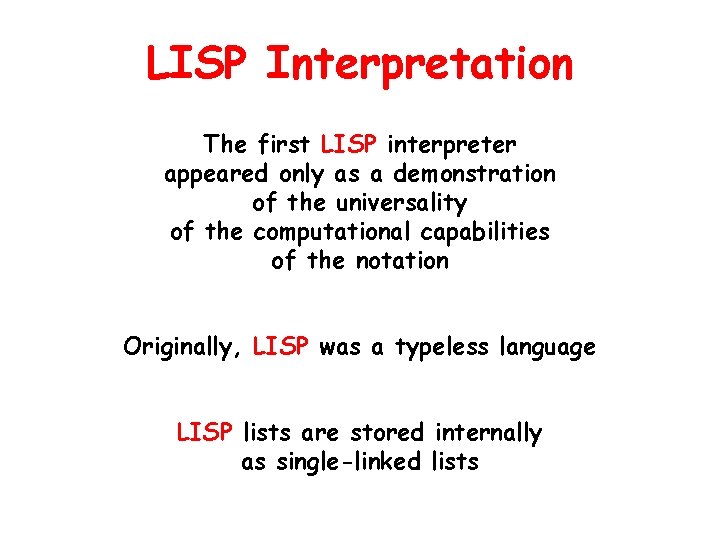
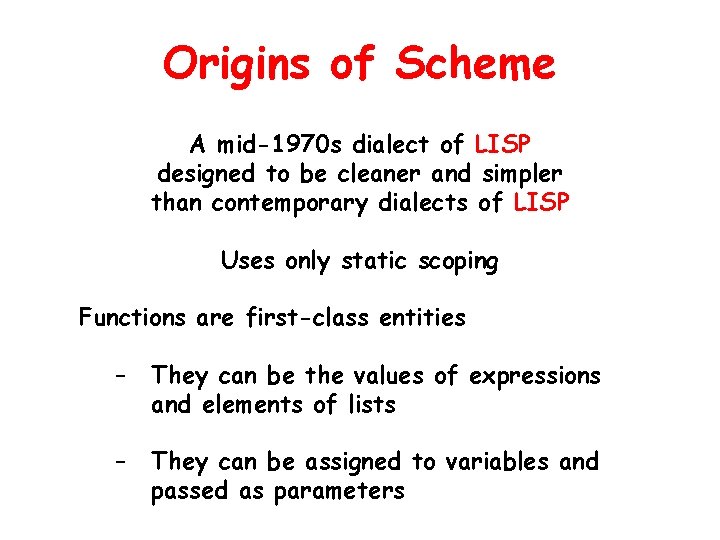
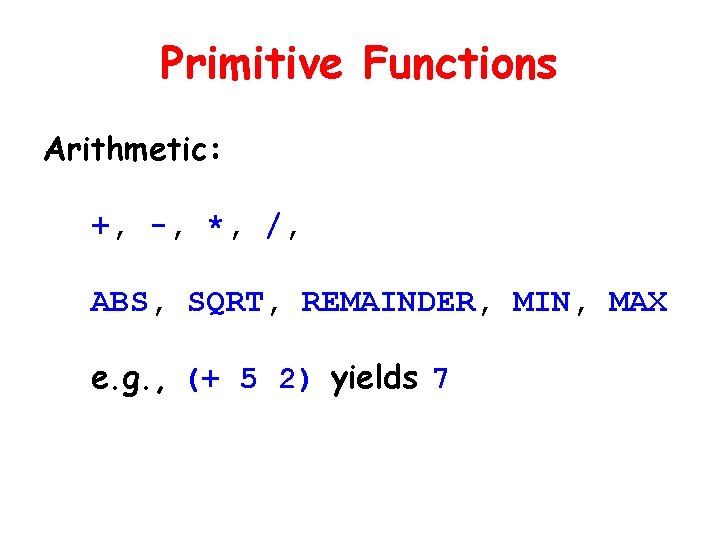
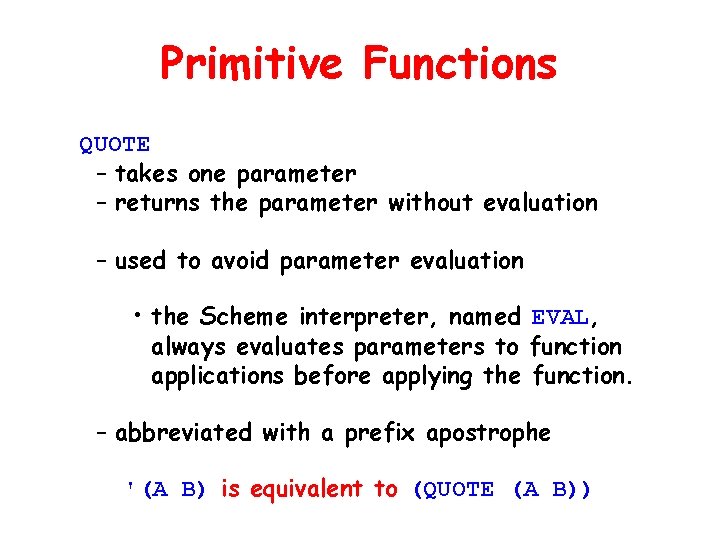
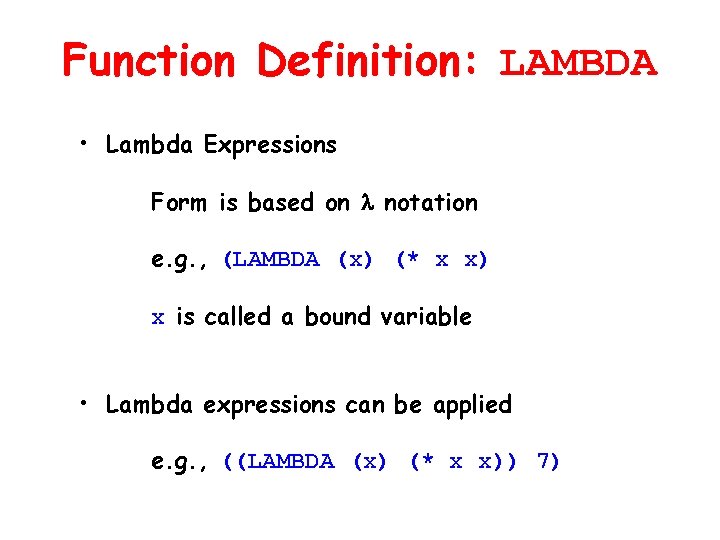
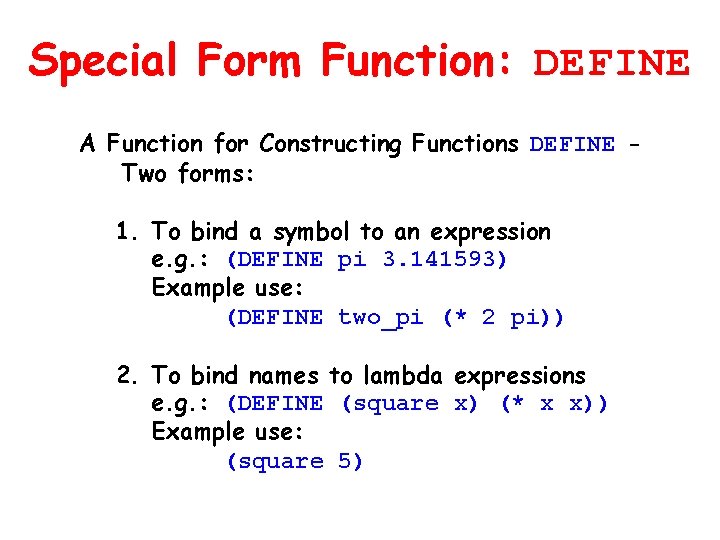
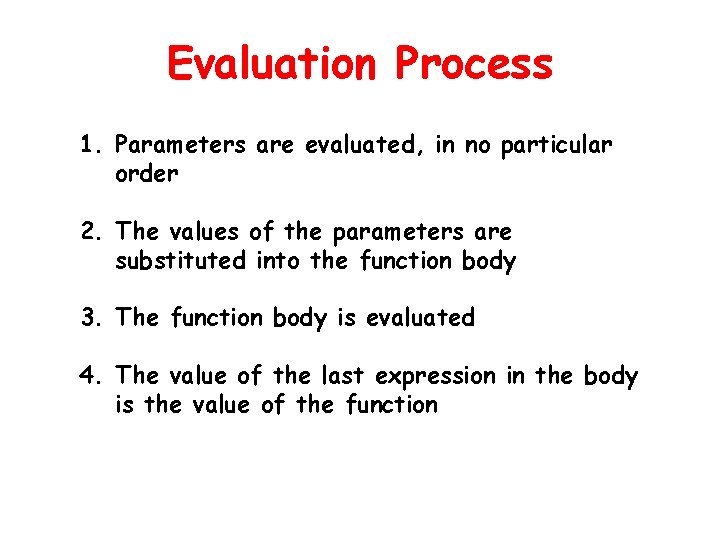
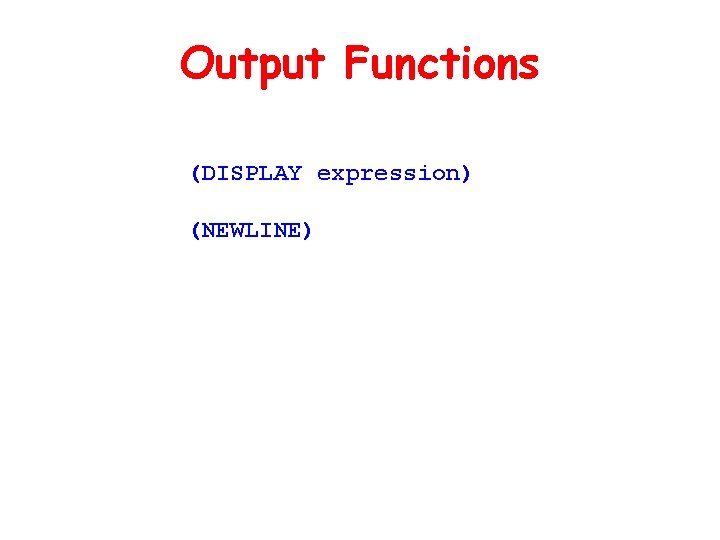
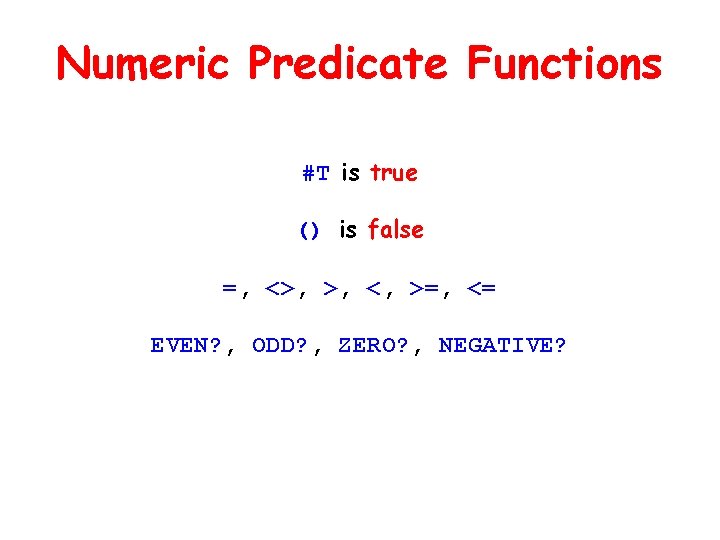
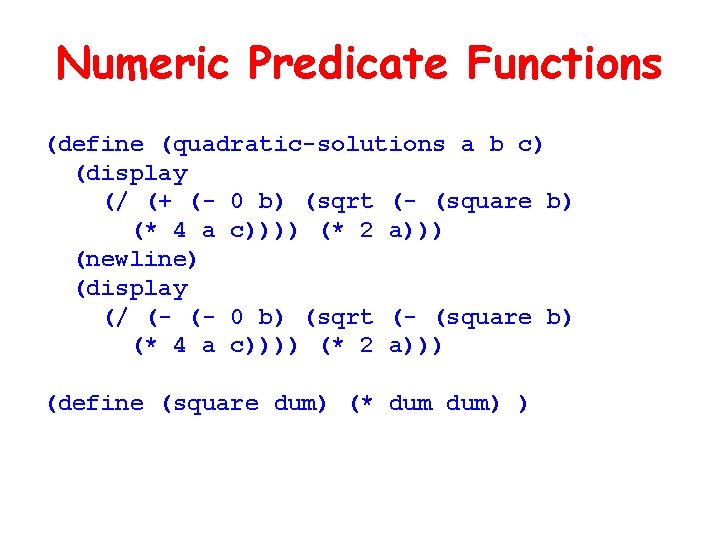
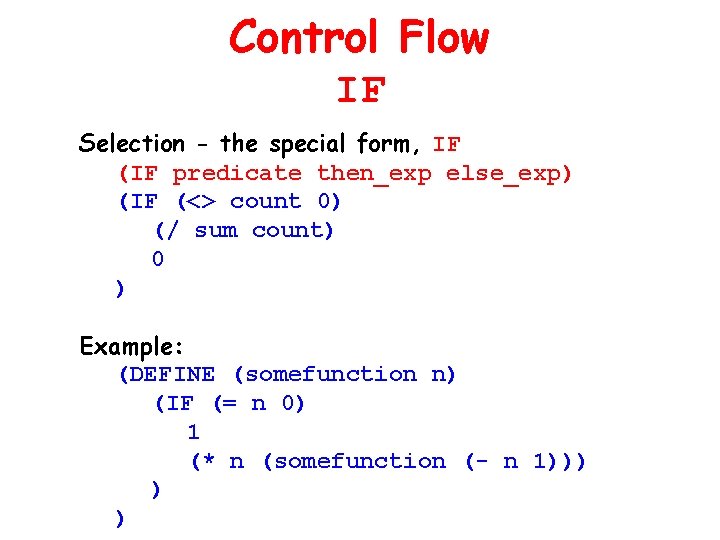
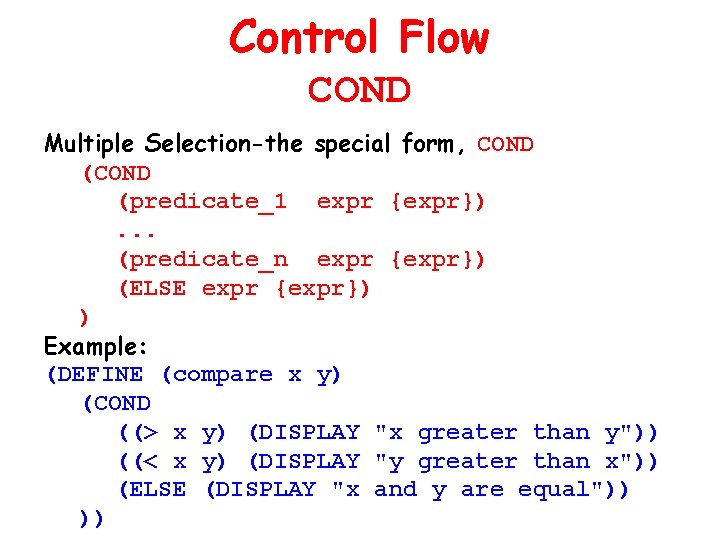
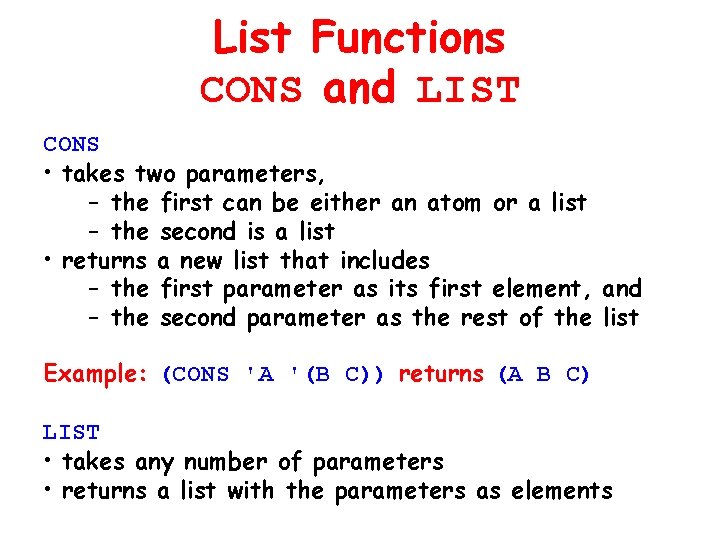
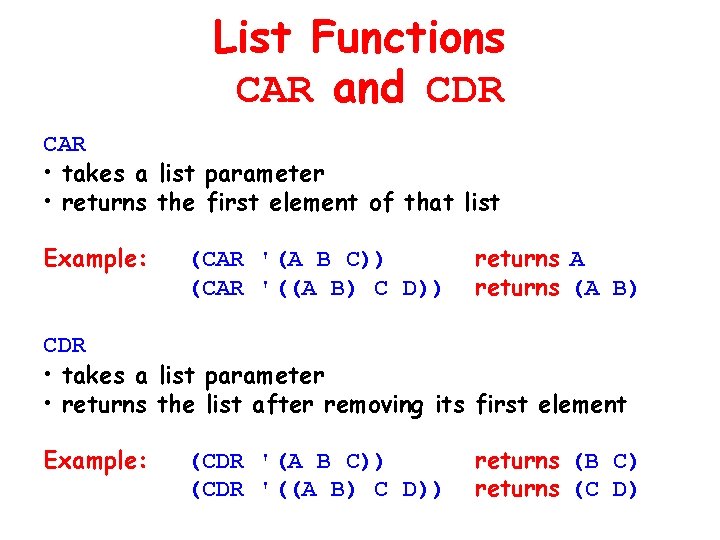
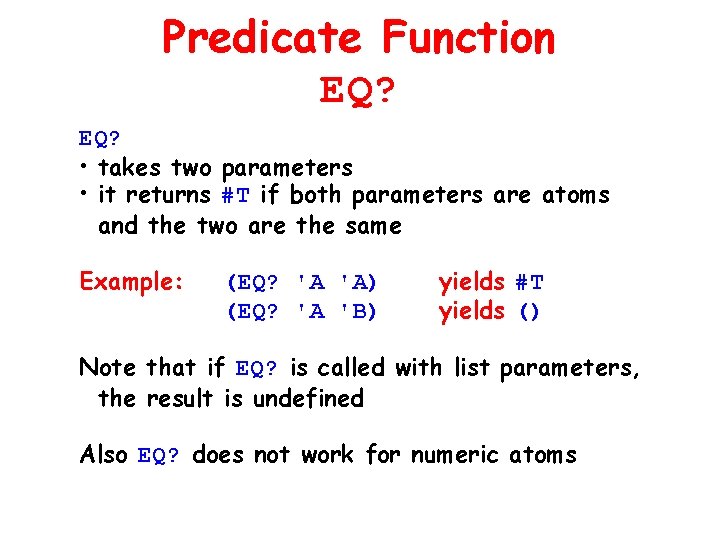
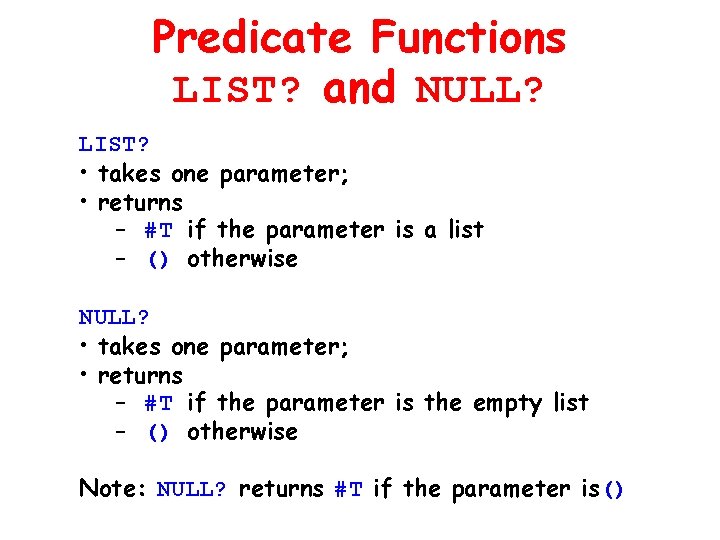
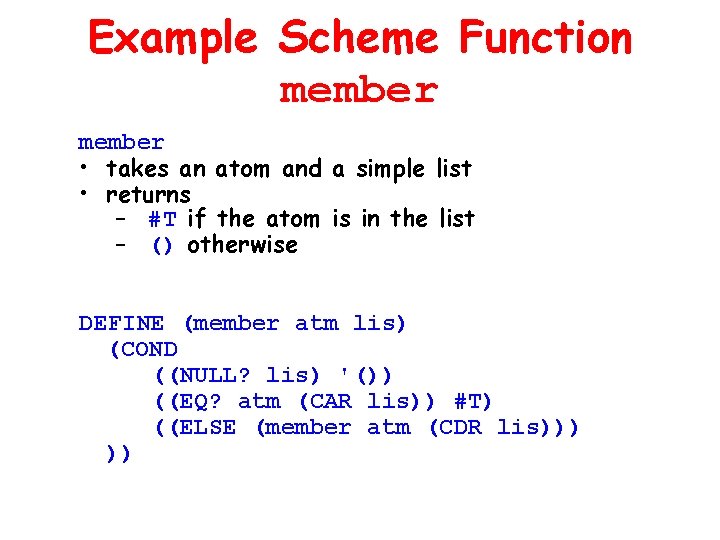
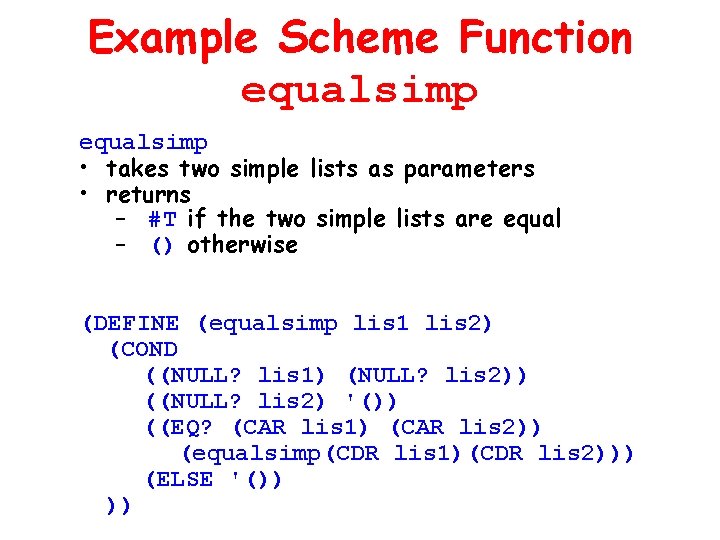
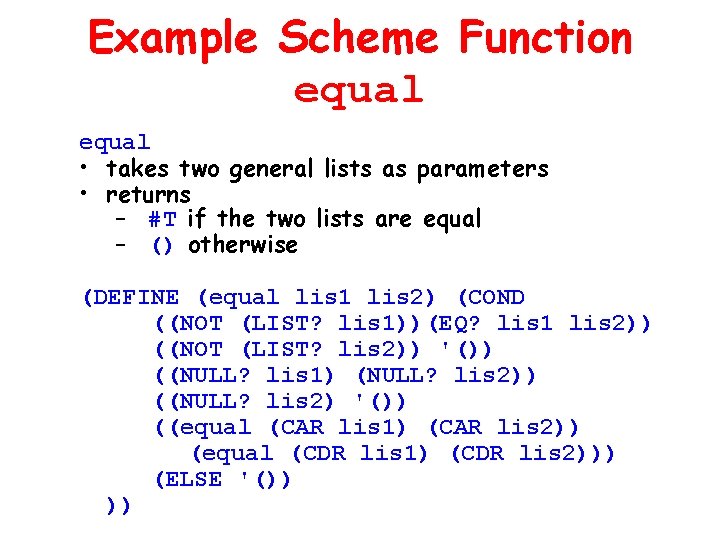
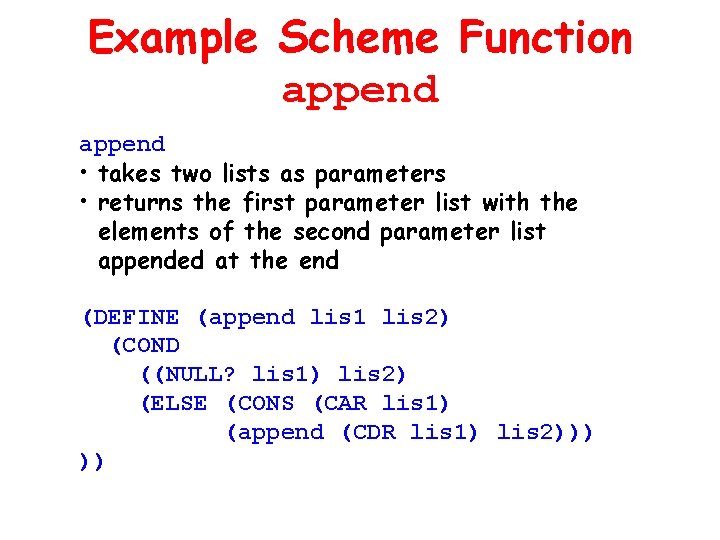
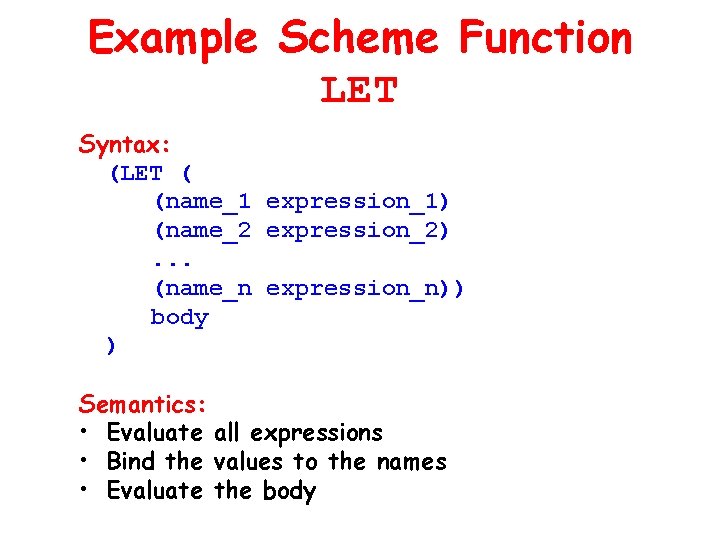
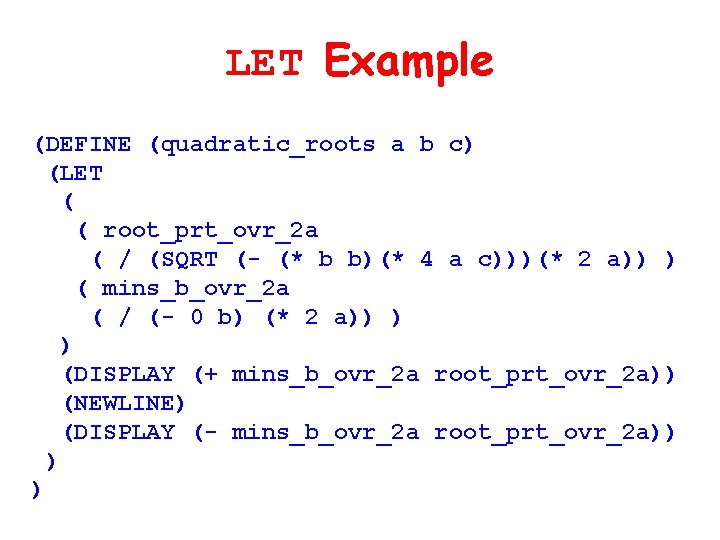
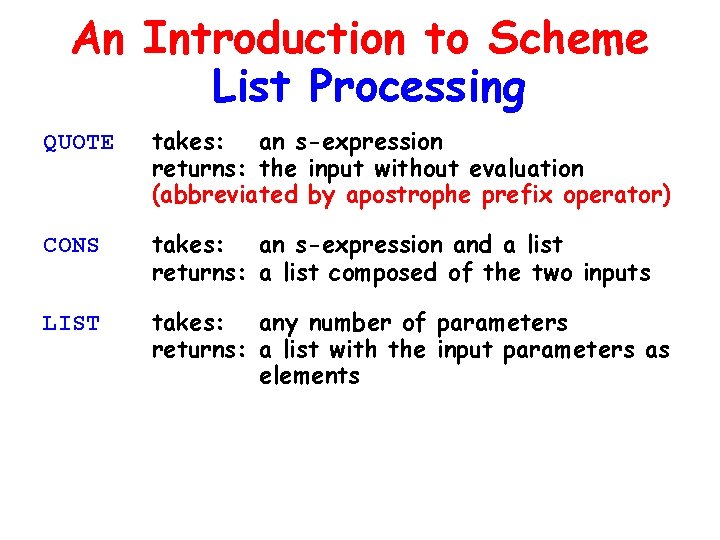
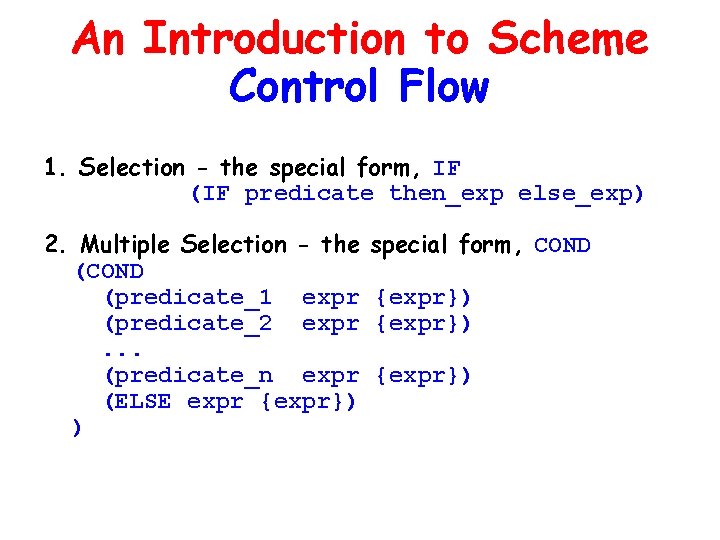
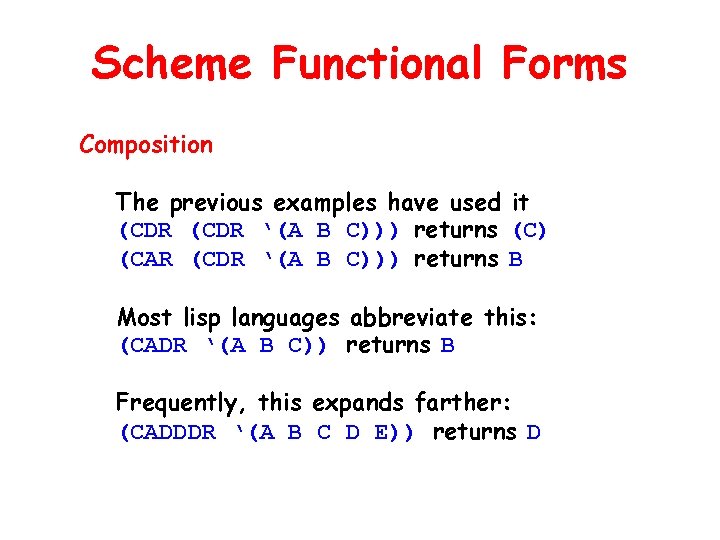
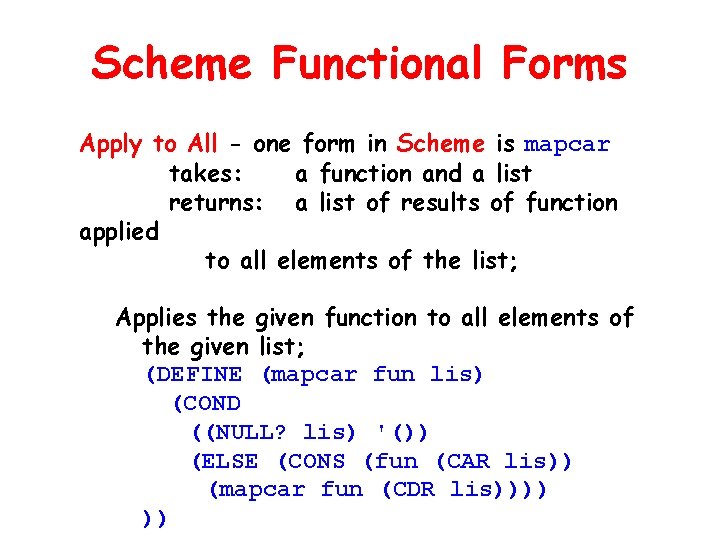
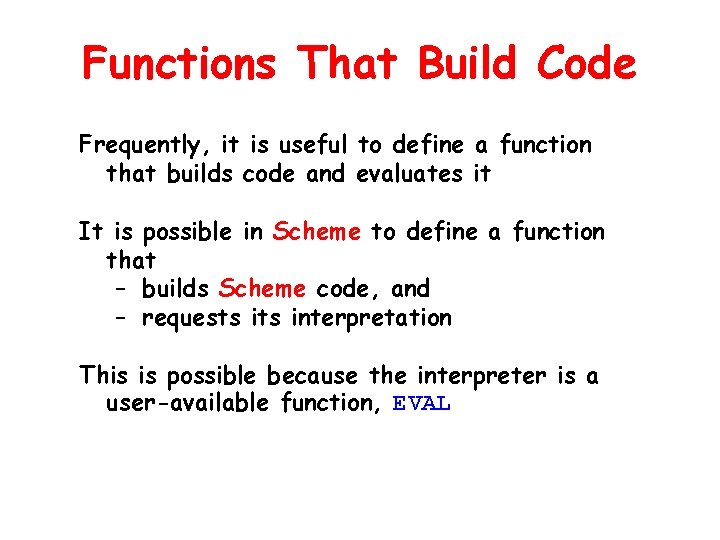
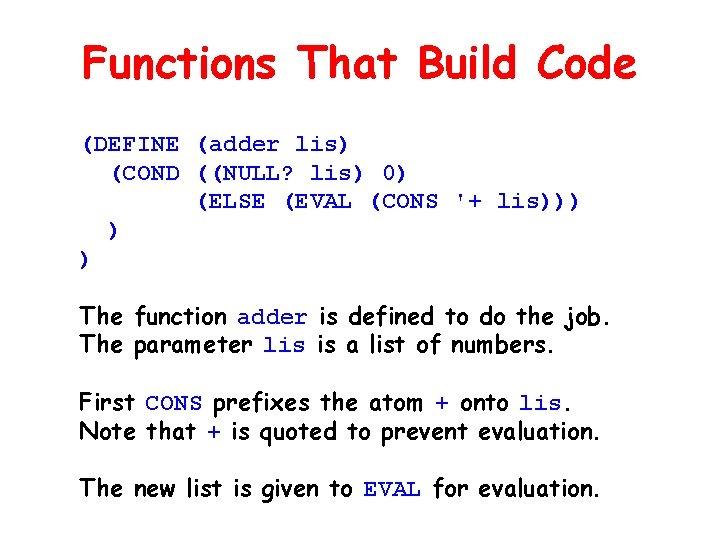
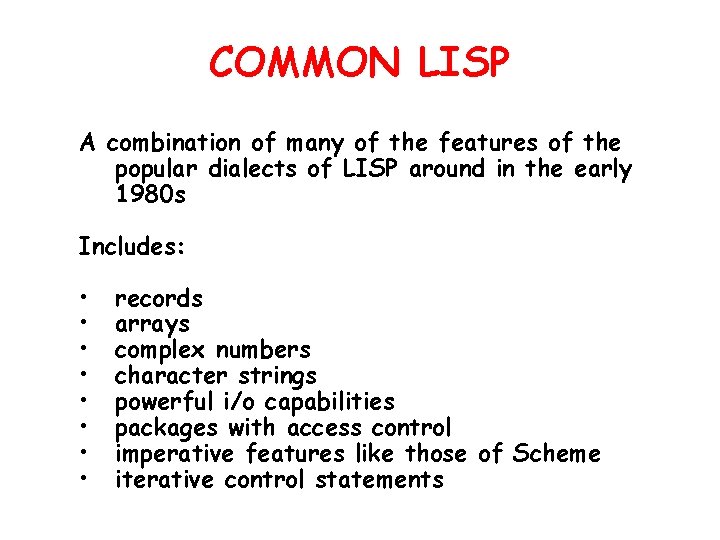
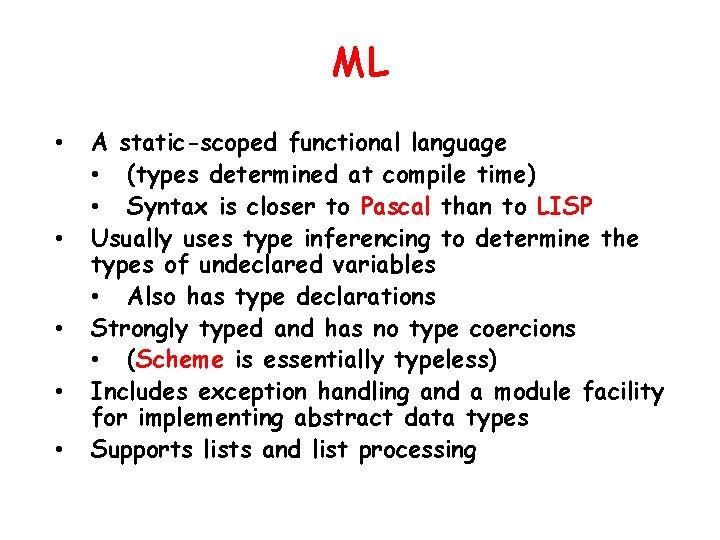
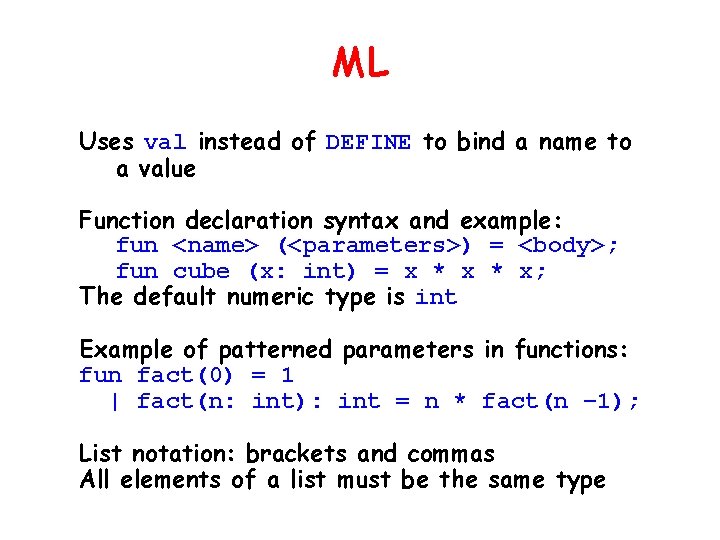
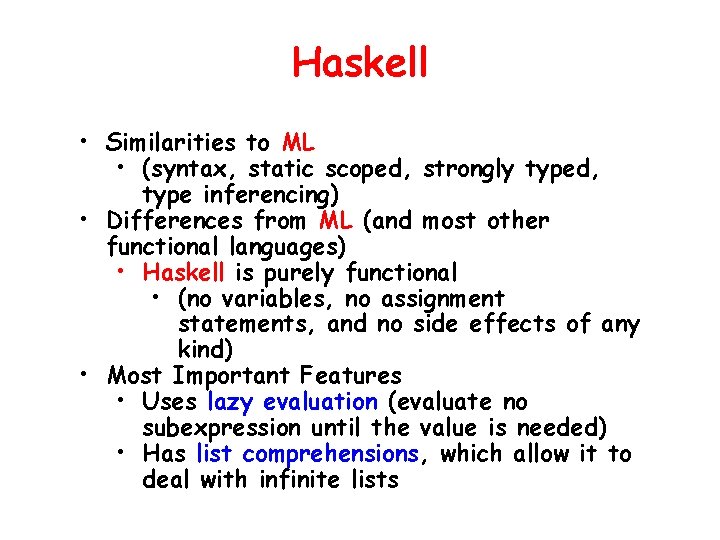
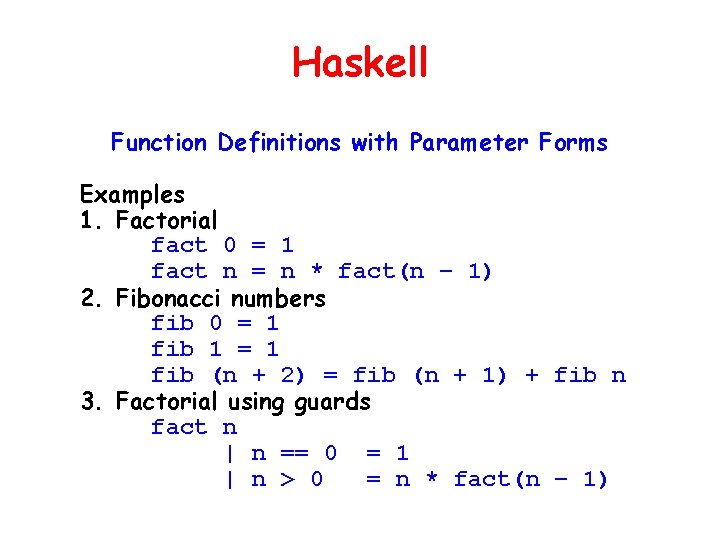
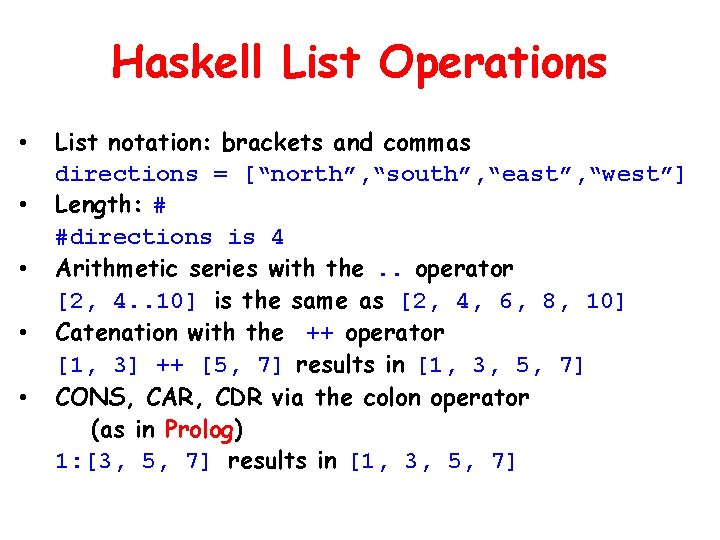
![Haskell List Operations Examples product [ ] = 1 product (a: x) = a Haskell List Operations Examples product [ ] = 1 product (a: x) = a](https://slidetodoc.com/presentation_image_h/b3a522722addcda221caf6647792a500/image-48.jpg)
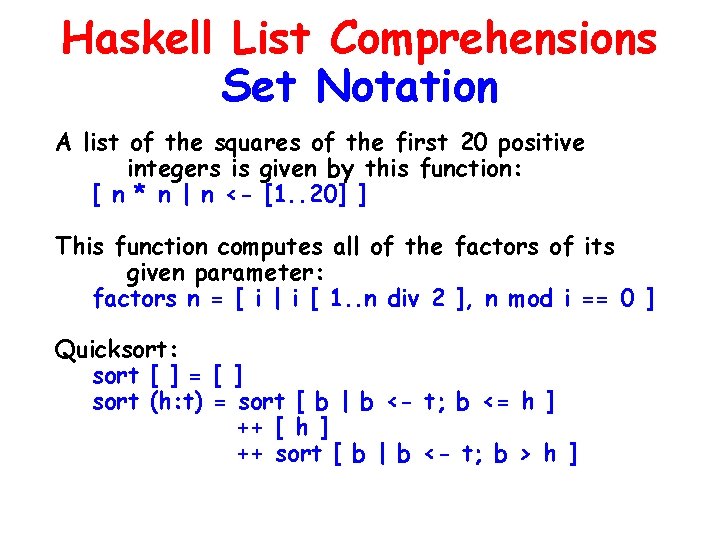
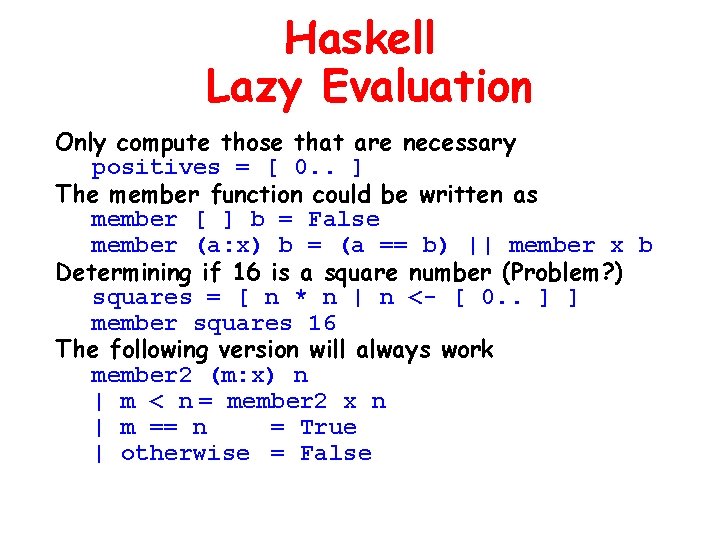
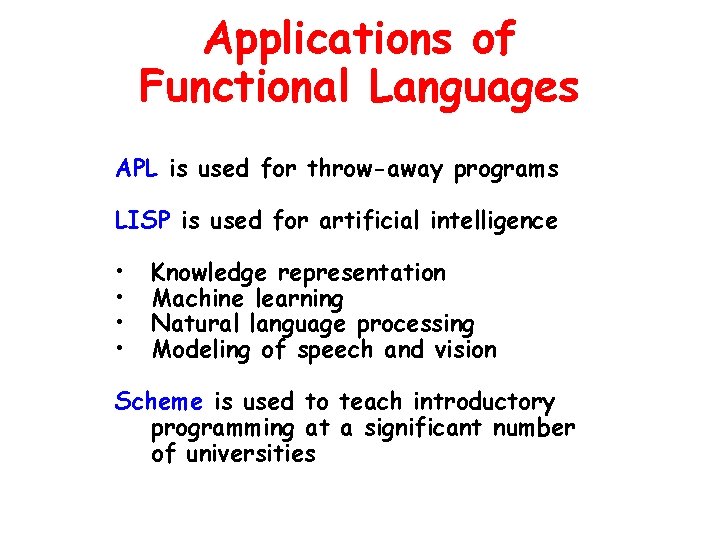
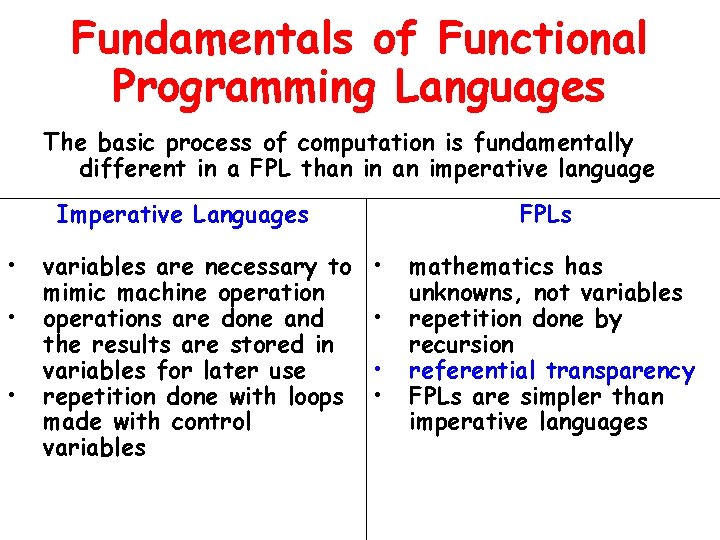
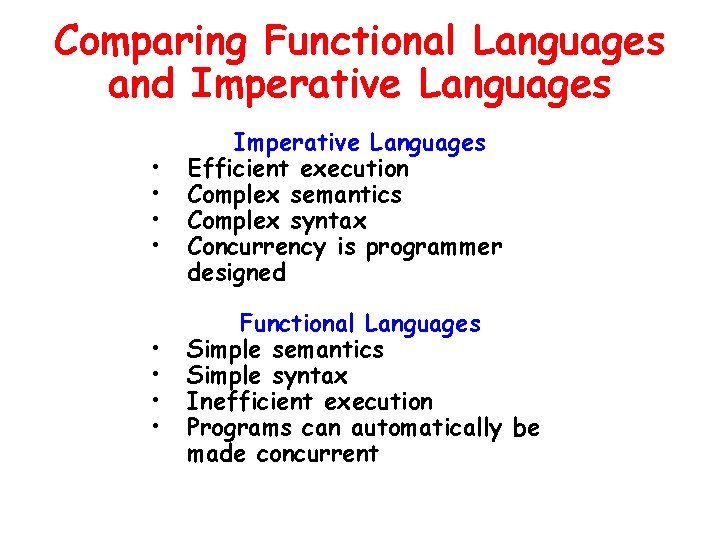
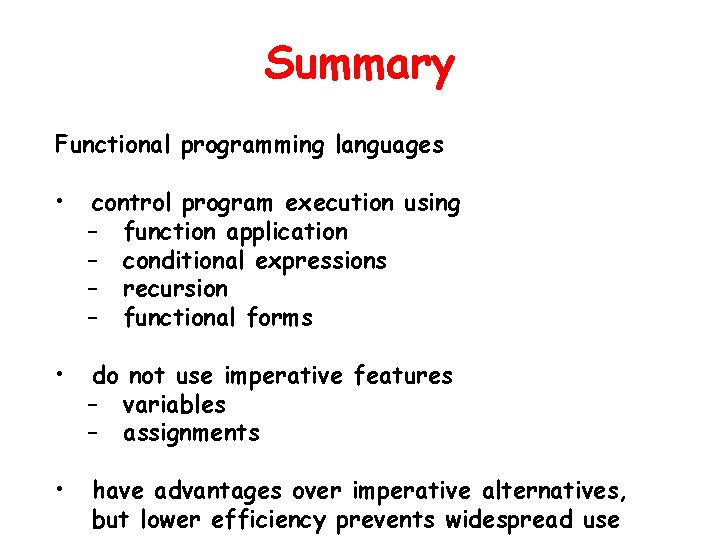
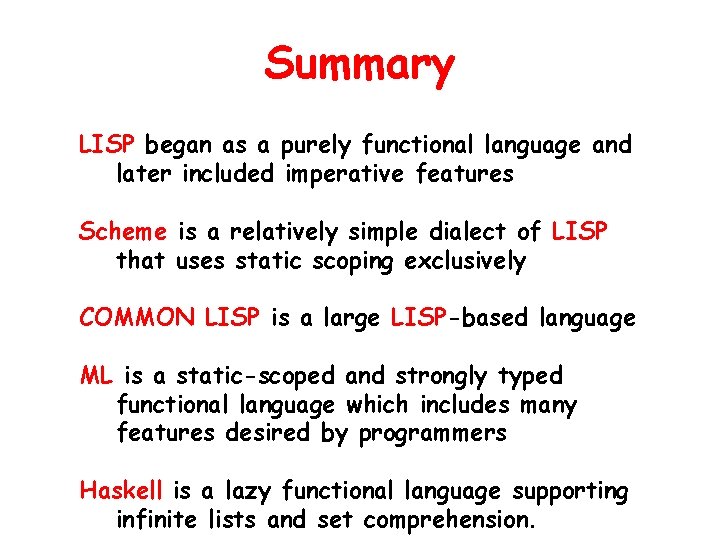
- Slides: 55
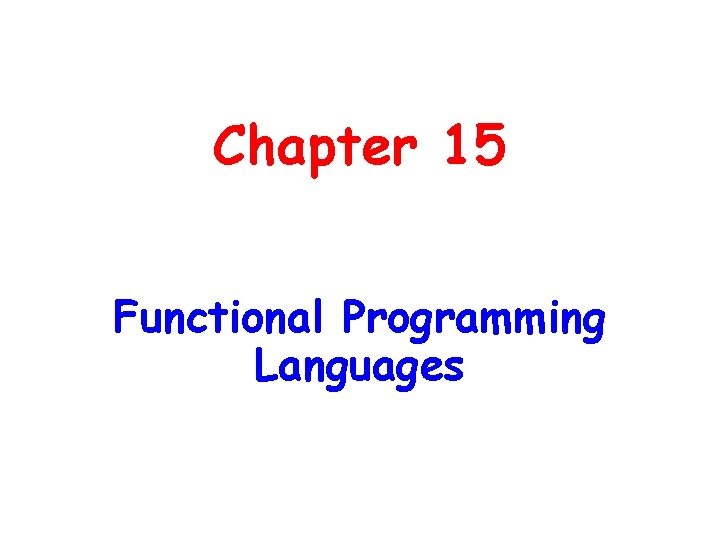
Chapter 15 Functional Programming Languages
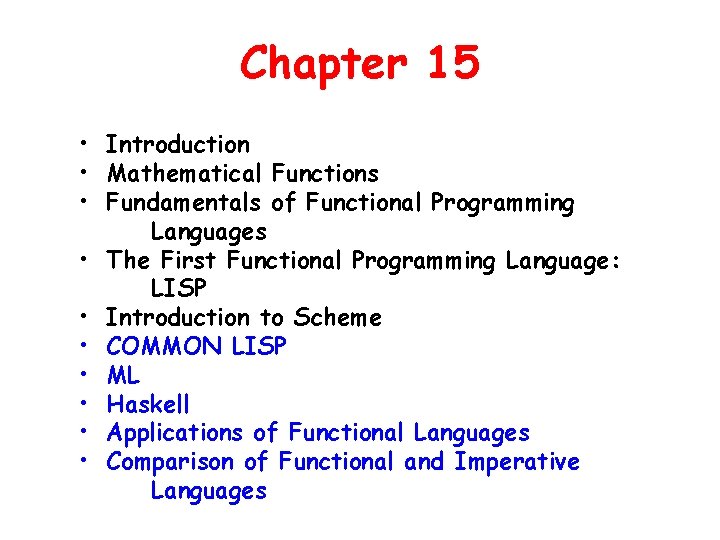
Chapter 15 • Introduction • Mathematical Functions • Fundamentals of Functional Programming Languages • The First Functional Programming Language: LISP • Introduction to Scheme • COMMON LISP • ML • Haskell • Applications of Functional Languages • Comparison of Functional and Imperative Languages
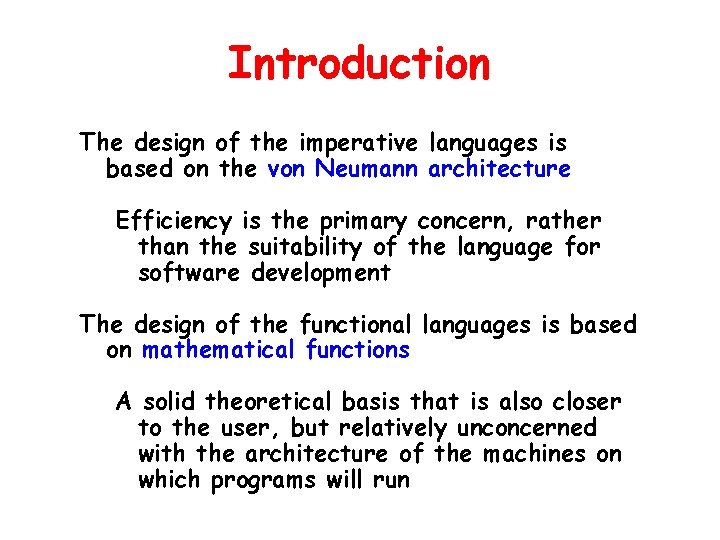
Introduction The design of the imperative languages is based on the von Neumann architecture Efficiency is the primary concern, rather than the suitability of the language for software development The design of the functional languages is based on mathematical functions A solid theoretical basis that is also closer to the user, but relatively unconcerned with the architecture of the machines on which programs will run
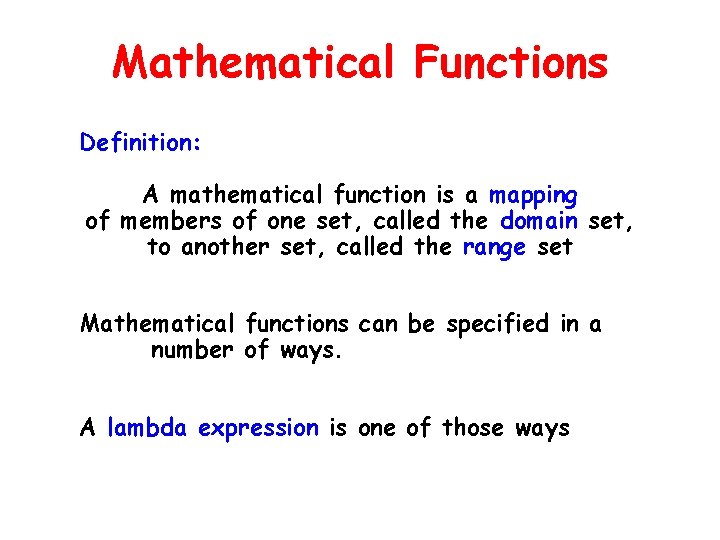
Mathematical Functions Definition: A mathematical function is a mapping of members of one set, called the domain set, to another set, called the range set Mathematical functions can be specified in a number of ways. A lambda expression is one of those ways
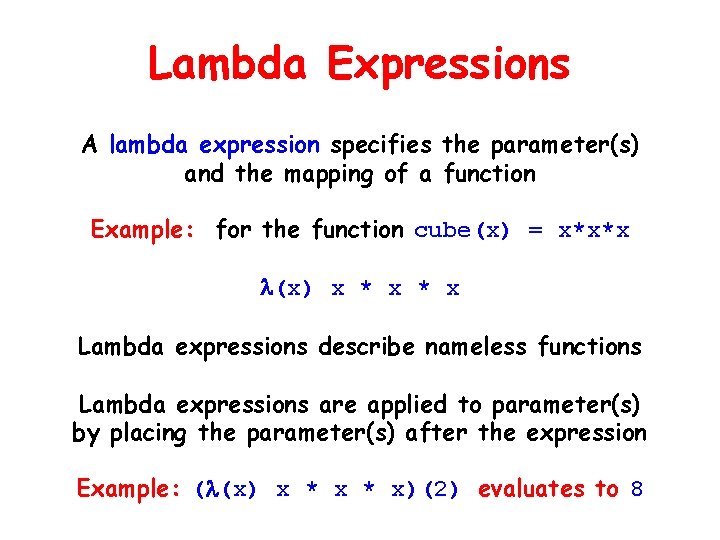
Lambda Expressions A lambda expression specifies the parameter(s) and the mapping of a function Example: for the function cube(x) = x*x*x (x) x * x Lambda expressions describe nameless functions Lambda expressions are applied to parameter(s) by placing the parameter(s) after the expression Example: ( (x) x * x)(2) evaluates to 8
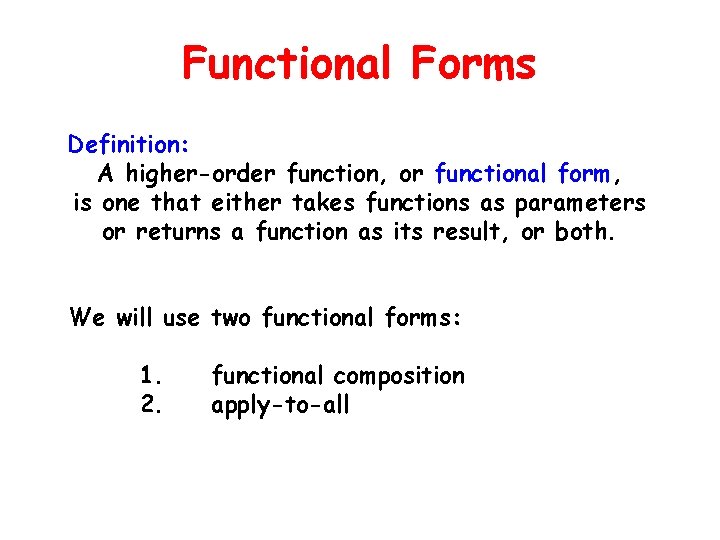
Functional Forms Definition: A higher-order function, or functional form, is one that either takes functions as parameters or returns a function as its result, or both. We will use two functional forms: 1. 2. functional composition apply-to-all
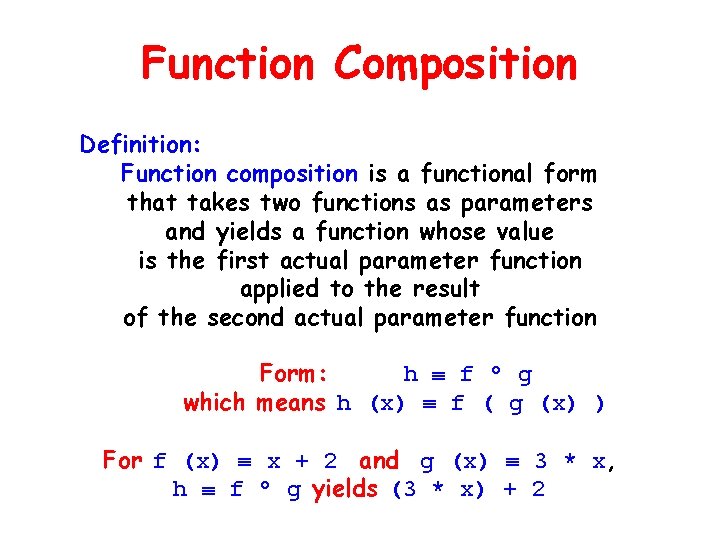
Function Composition Definition: Function composition is a functional form that takes two functions as parameters and yields a function whose value is the first actual parameter function applied to the result of the second actual parameter function Form: h f ° g which means h (x) f ( g (x) ) For f (x) x + 2 and g (x) 3 * x, h f ° g yields (3 * x) + 2
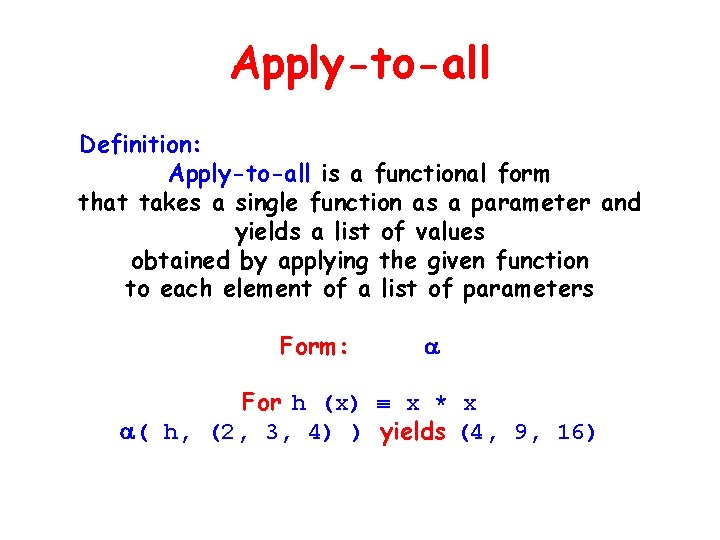
Apply-to-all Definition: Apply-to-all is a functional form that takes a single function as a parameter and yields a list of values obtained by applying the given function to each element of a list of parameters Form: For h (x) x * x ( h, (2, 3, 4) ) yields (4, 9, 16)
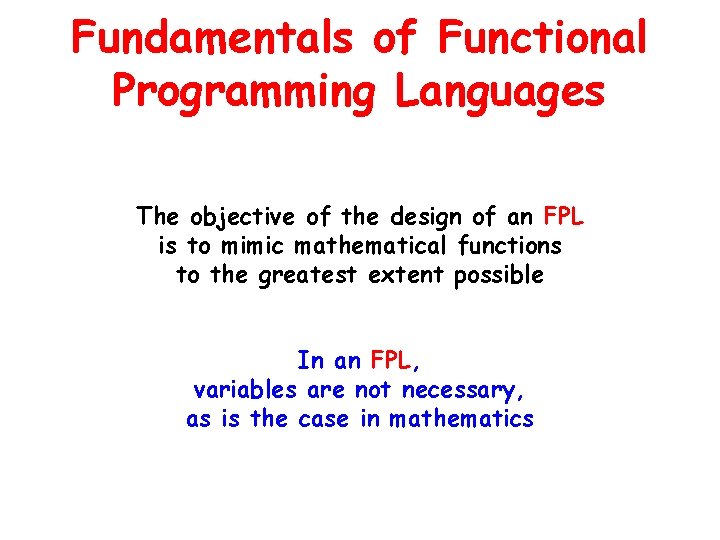
Fundamentals of Functional Programming Languages The objective of the design of an FPL is to mimic mathematical functions to the greatest extent possible In an FPL, variables are not necessary, as is the case in mathematics
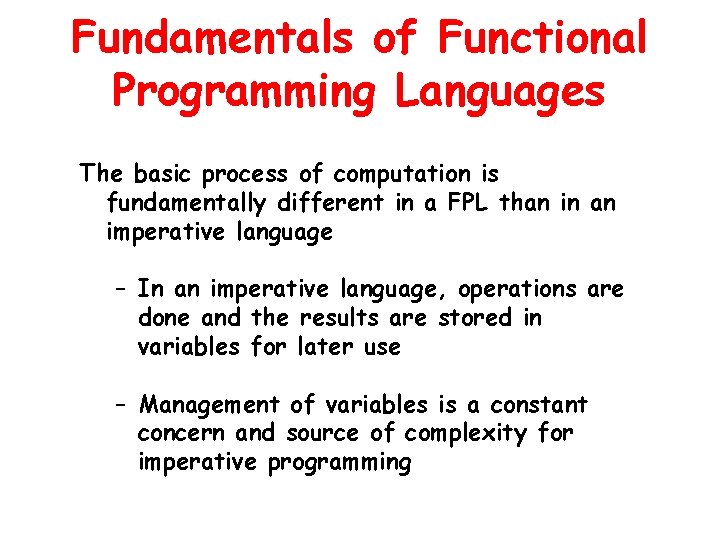
Fundamentals of Functional Programming Languages The basic process of computation is fundamentally different in a FPL than in an imperative language – In an imperative language, operations are done and the results are stored in variables for later use – Management of variables is a constant concern and source of complexity for imperative programming
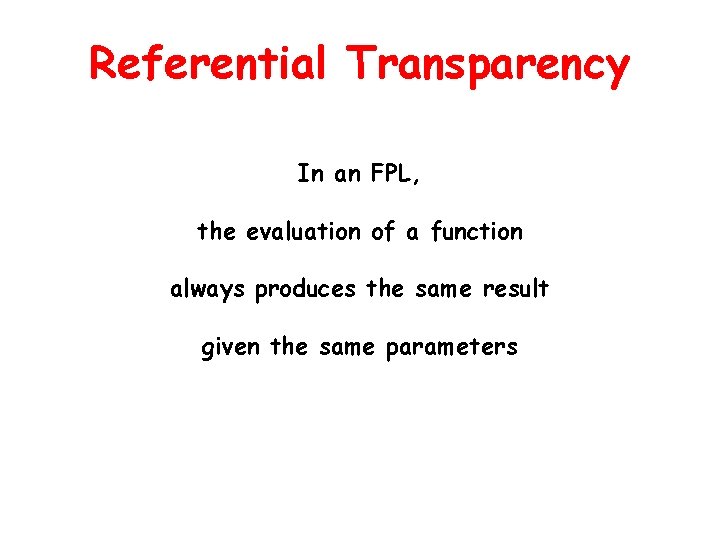
Referential Transparency In an FPL, the evaluation of a function always produces the same result given the same parameters
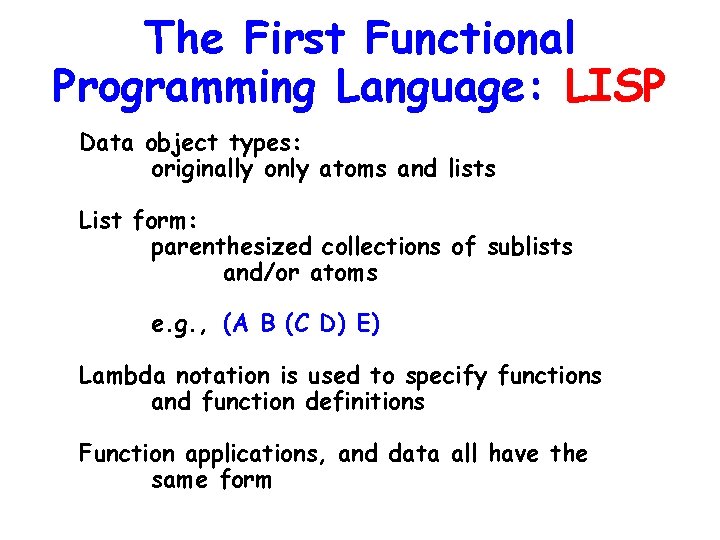
The First Functional Programming Language: LISP Data object types: originally only atoms and lists List form: parenthesized collections of sublists and/or atoms e. g. , (A B (C D) E) Lambda notation is used to specify functions and function definitions Function applications, and data all have the same form
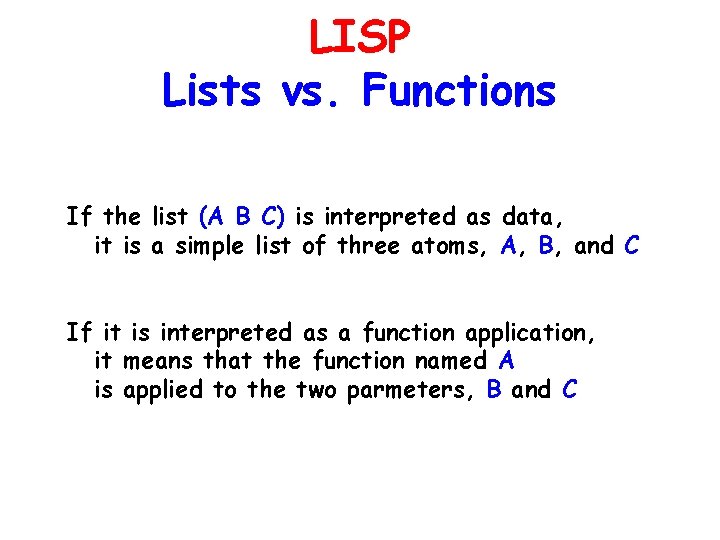
LISP Lists vs. Functions If the list (A B C) is interpreted as data, it is a simple list of three atoms, A, B, and C If it is interpreted as a function application, it means that the function named A is applied to the two parmeters, B and C
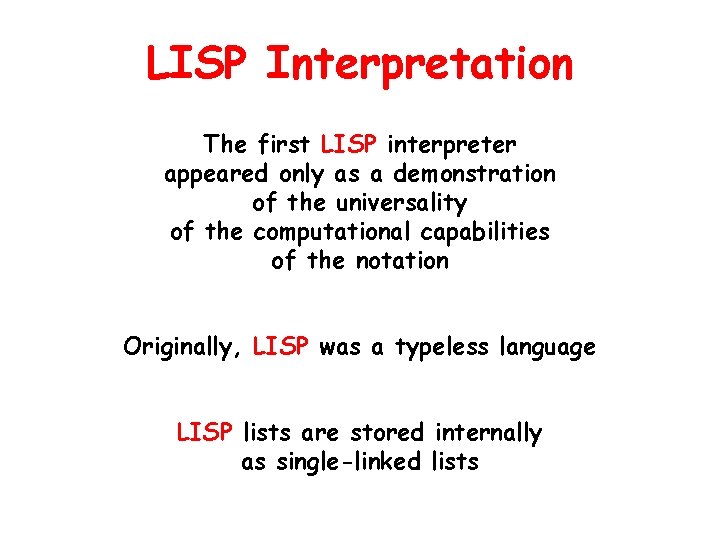
LISP Interpretation The first LISP interpreter appeared only as a demonstration of the universality of the computational capabilities of the notation Originally, LISP was a typeless language LISP lists are stored internally as single-linked lists
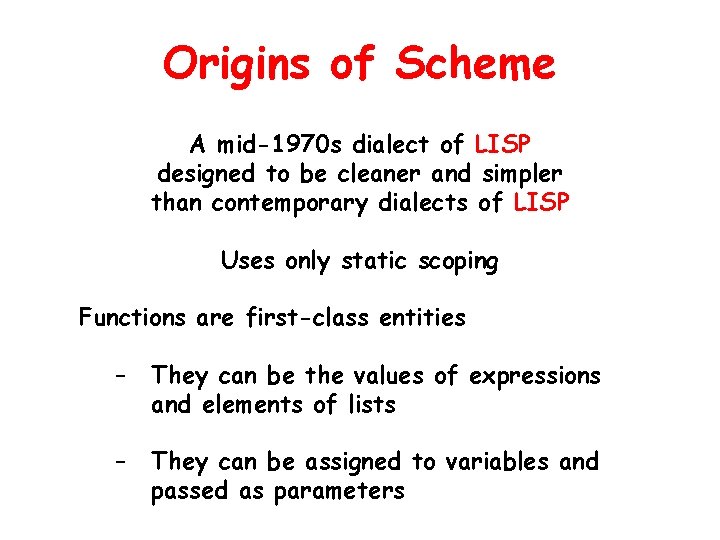
Origins of Scheme A mid-1970 s dialect of LISP designed to be cleaner and simpler than contemporary dialects of LISP Uses only static scoping Functions are first-class entities – They can be the values of expressions and elements of lists – They can be assigned to variables and passed as parameters
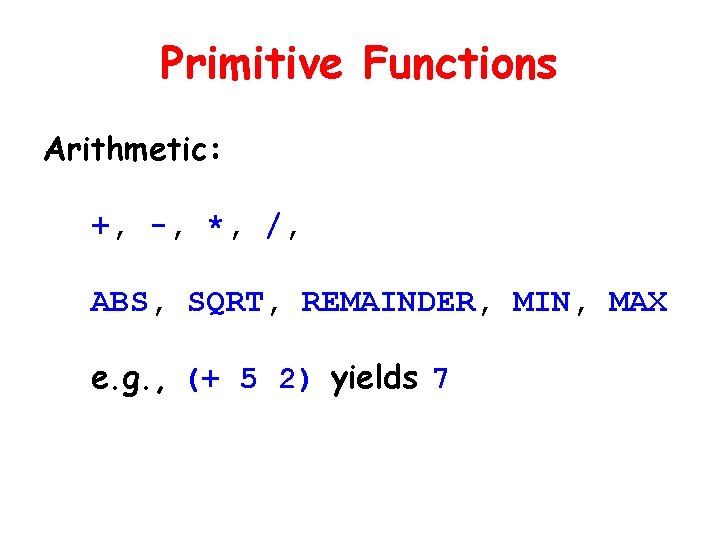
Primitive Functions Arithmetic: +, -, *, /, ABS, SQRT, REMAINDER, MIN, MAX e. g. , (+ 5 2) yields 7
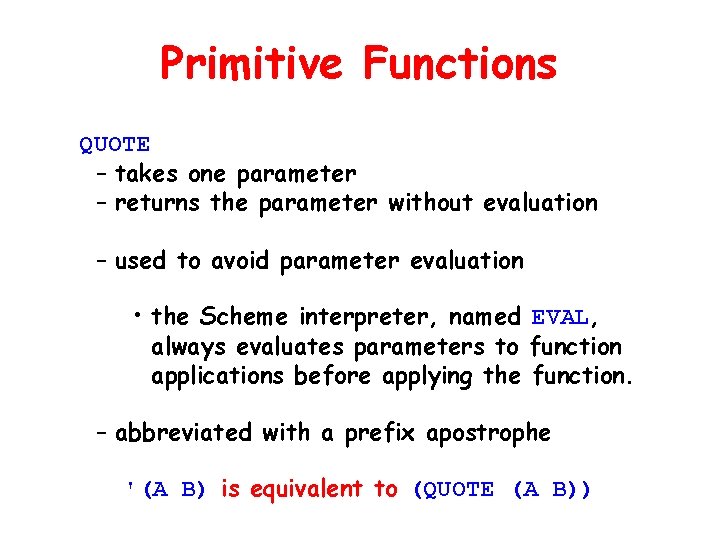
Primitive Functions QUOTE – takes one parameter – returns the parameter without evaluation – used to avoid parameter evaluation • the Scheme interpreter, named EVAL, always evaluates parameters to function applications before applying the function. – abbreviated with a prefix apostrophe '(A B) is equivalent to (QUOTE (A B))
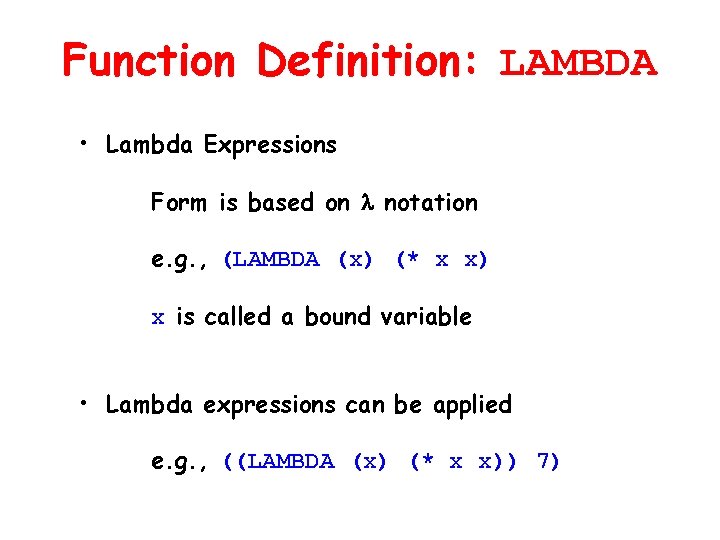
Function Definition: LAMBDA • Lambda Expressions Form is based on notation e. g. , (LAMBDA (x) (* x x) x is called a bound variable • Lambda expressions can be applied e. g. , ((LAMBDA (x) (* x x)) 7)
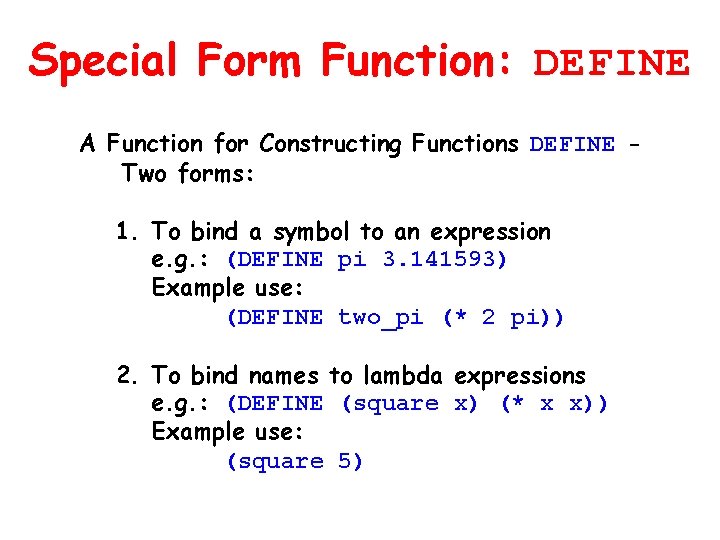
Special Form Function: DEFINE A Function for Constructing Functions DEFINE Two forms: 1. To bind a symbol to an expression e. g. : (DEFINE pi 3. 141593) Example use: (DEFINE two_pi (* 2 pi)) 2. To bind names to lambda expressions e. g. : (DEFINE (square x) (* x x)) Example use: (square 5)
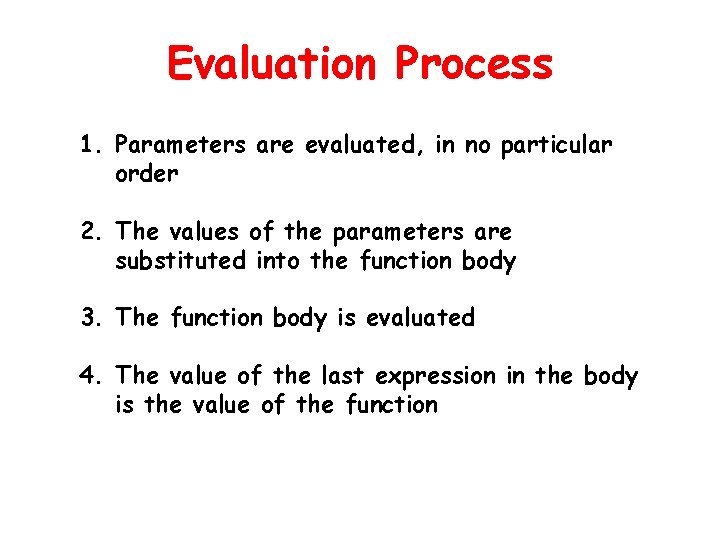
Evaluation Process 1. Parameters are evaluated, in no particular order 2. The values of the parameters are substituted into the function body 3. The function body is evaluated 4. The value of the last expression in the body is the value of the function
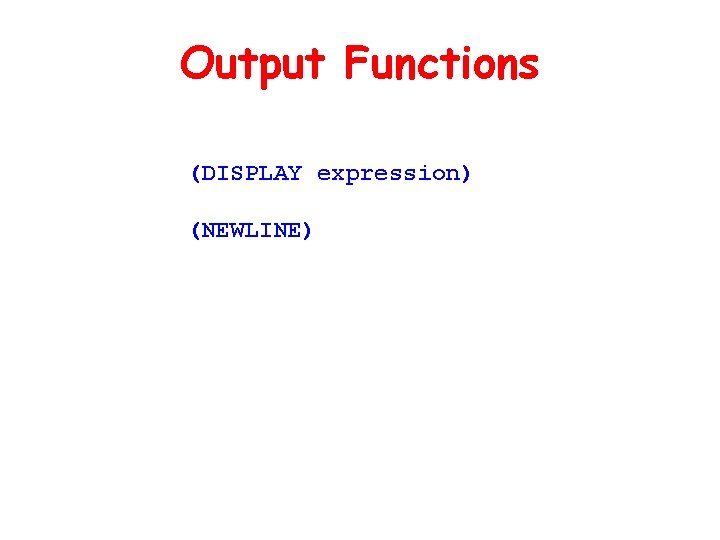
Output Functions (DISPLAY expression) (NEWLINE)
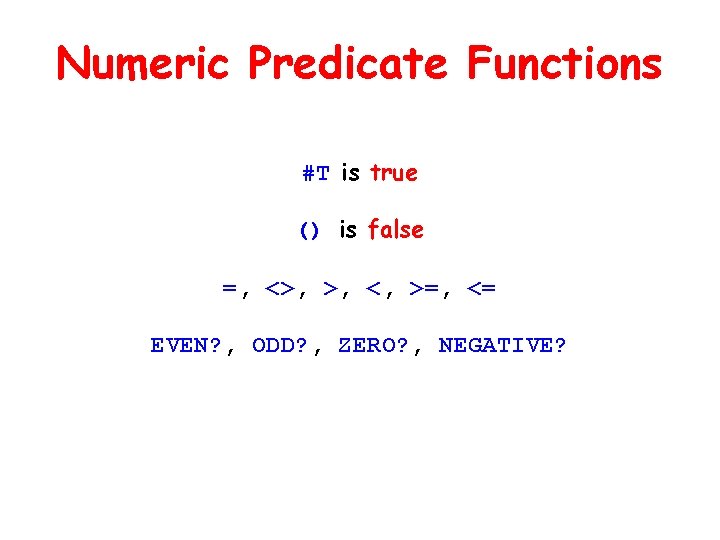
Numeric Predicate Functions #T is true () is false =, <>, >, <, >=, <= EVEN? , ODD? , ZERO? , NEGATIVE?
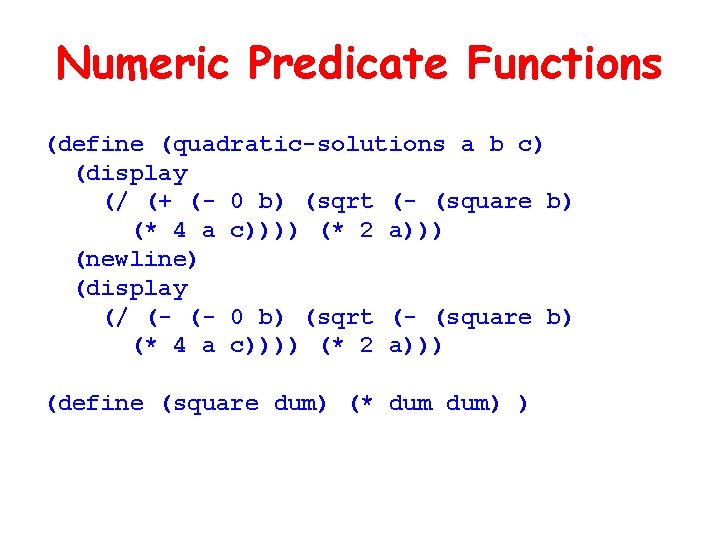
Numeric Predicate Functions (define (quadratic-solutions a b c) (display (/ (+ (- 0 b) (sqrt (- (square b) (* 4 a c)))) (* 2 a))) (newline) (display (/ (- (- 0 b) (sqrt (- (square b) (* 4 a c)))) (* 2 a))) (define (square dum) (* dum) )
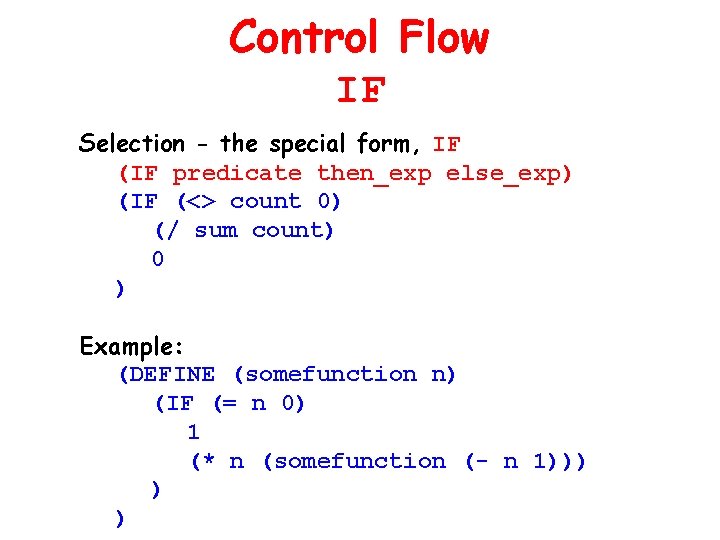
Control Flow IF Selection - the special form, IF (IF predicate then_exp else_exp) (IF (<> count 0) (/ sum count) 0 ) Example: (DEFINE (somefunction n) (IF (= n 0) 1 (* n (somefunction (- n 1))) ) )
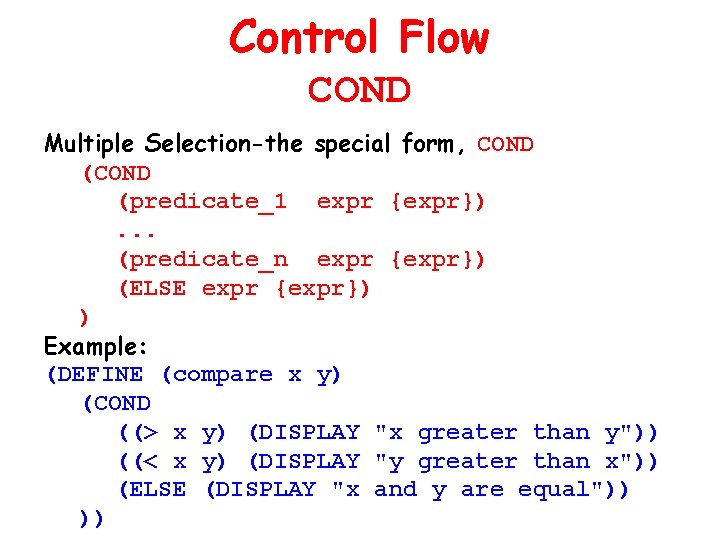
Control Flow COND Multiple Selection-the special form, COND (predicate_1 expr {expr}). . . (predicate_n expr {expr}) (ELSE expr {expr}) ) Example: (DEFINE (compare x y) (COND ((> x y) (DISPLAY "x greater than y")) ((< x y) (DISPLAY "y greater than x")) (ELSE (DISPLAY "x and y are equal")) ))
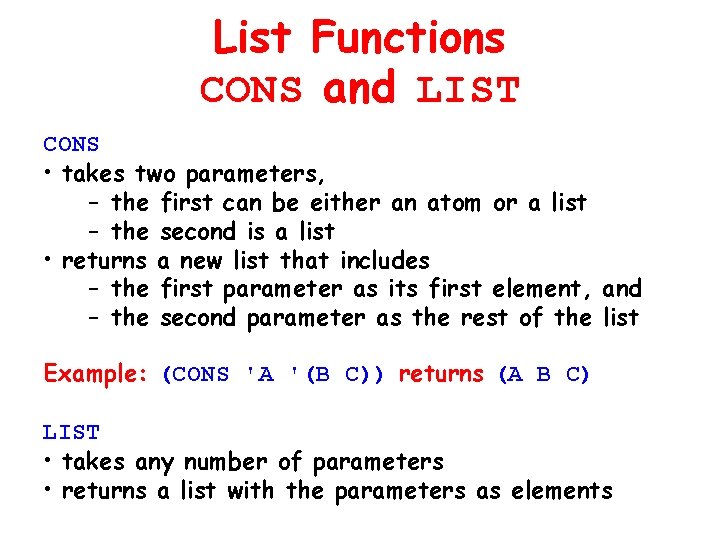
List Functions CONS and LIST CONS • takes two parameters, – the first can be either an atom or a list – the second is a list • returns a new list that includes – the first parameter as its first element, and – the second parameter as the rest of the list Example: (CONS 'A '(B C)) returns (A B C) LIST • takes any number of parameters • returns a list with the parameters as elements
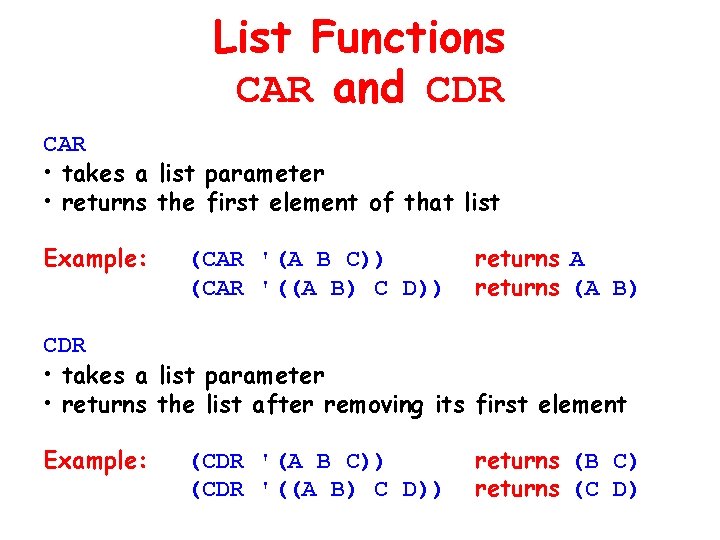
List Functions CAR and CDR CAR • takes a list parameter • returns the first element of that list Example: (CAR '(A B C)) (CAR '((A B) C D)) returns A returns (A B) CDR • takes a list parameter • returns the list after removing its first element Example: (CDR '(A B C)) (CDR '((A B) C D)) returns (B C) returns (C D)
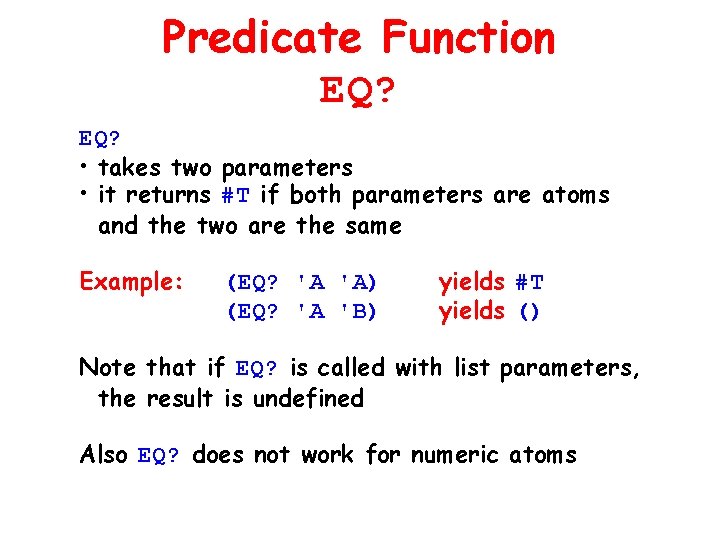
Predicate Function EQ? • takes two parameters • it returns #T if both parameters are atoms and the two are the same Example: (EQ? 'A 'A) (EQ? 'A 'B) yields #T yields () Note that if EQ? is called with list parameters, the result is undefined Also EQ? does not work for numeric atoms
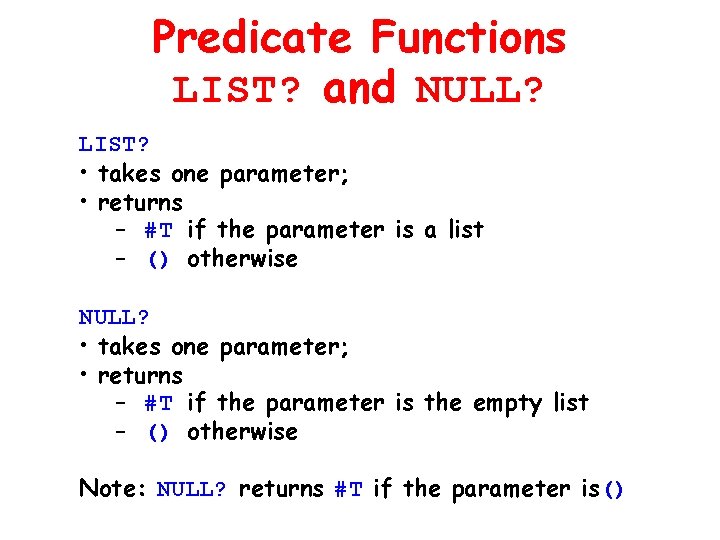
Predicate Functions LIST? and NULL? LIST? • takes one parameter; • returns – #T if the parameter is a list – () otherwise NULL? • takes one parameter; • returns – #T if the parameter is the empty list – () otherwise Note: NULL? returns #T if the parameter is()
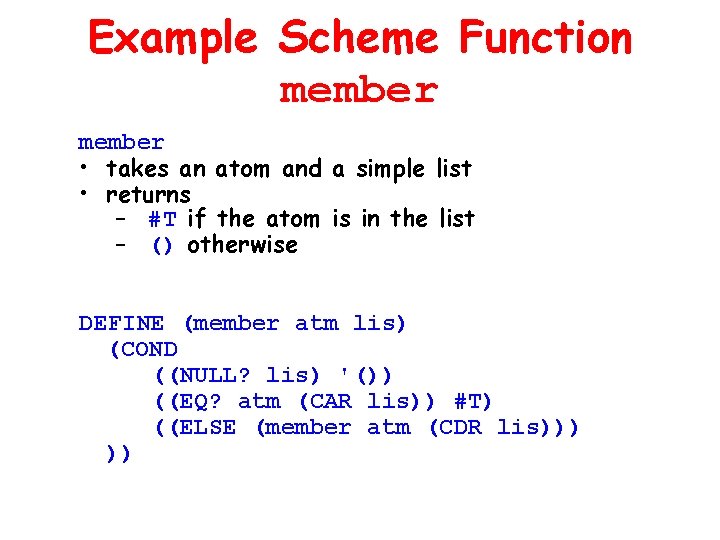
Example Scheme Function member • takes an atom and a simple list • returns – #T if the atom is in the list – () otherwise DEFINE (member atm lis) (COND ((NULL? lis) '()) ((EQ? atm (CAR lis)) #T) ((ELSE (member atm (CDR lis))) ))
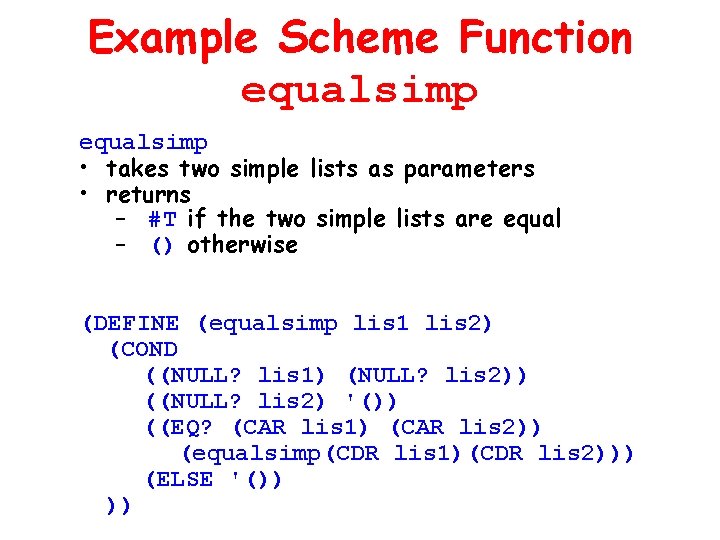
Example Scheme Function equalsimp • takes two simple lists as parameters • returns – #T if the two simple lists are equal – () otherwise (DEFINE (equalsimp lis 1 lis 2) (COND ((NULL? lis 1) (NULL? lis 2)) ((NULL? lis 2) '()) ((EQ? (CAR lis 1) (CAR lis 2)) (equalsimp(CDR lis 1)(CDR lis 2))) (ELSE '()) ))
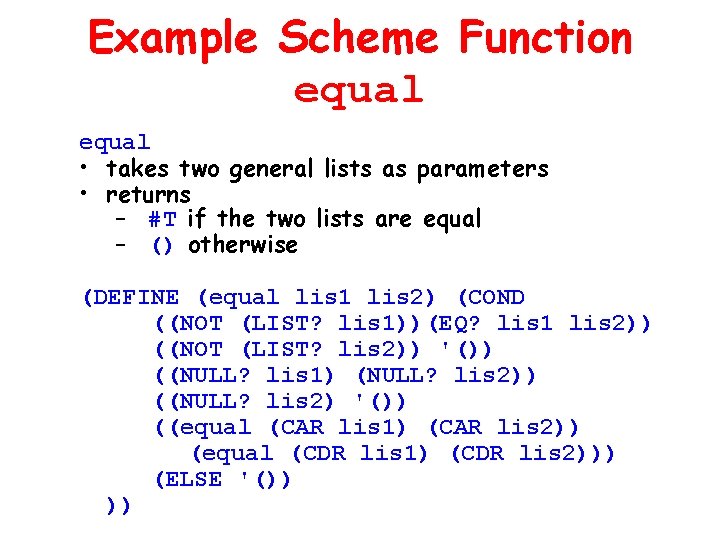
Example Scheme Function equal • takes two general lists as parameters • returns – #T if the two lists are equal – () otherwise (DEFINE (equal lis 1 lis 2) (COND ((NOT (LIST? lis 1))(EQ? lis 1 lis 2)) ((NOT (LIST? lis 2)) '()) ((NULL? lis 1) (NULL? lis 2)) ((NULL? lis 2) '()) ((equal (CAR lis 1) (CAR lis 2)) (equal (CDR lis 1) (CDR lis 2))) (ELSE '()) ))
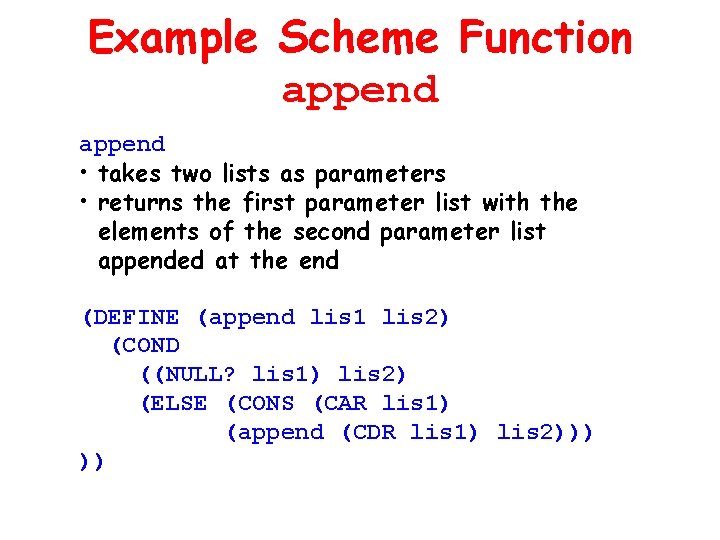
Example Scheme Function append • takes two lists as parameters • returns the first parameter list with the elements of the second parameter list appended at the end (DEFINE (append lis 1 lis 2) (COND ((NULL? lis 1) lis 2) (ELSE (CONS (CAR lis 1) (append (CDR lis 1) lis 2))) ))
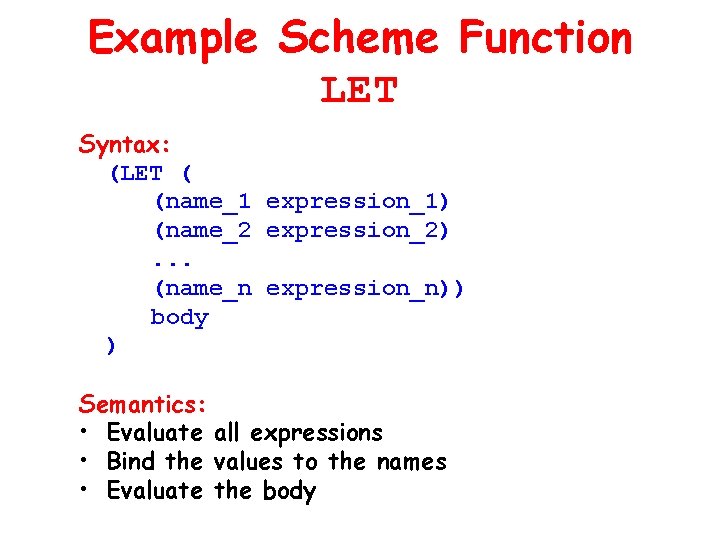
Example Scheme Function LET Syntax: (LET ( (name_1 expression_1) (name_2 expression_2). . . (name_n expression_n)) body ) Semantics: • Evaluate all expressions • Bind the values to the names • Evaluate the body
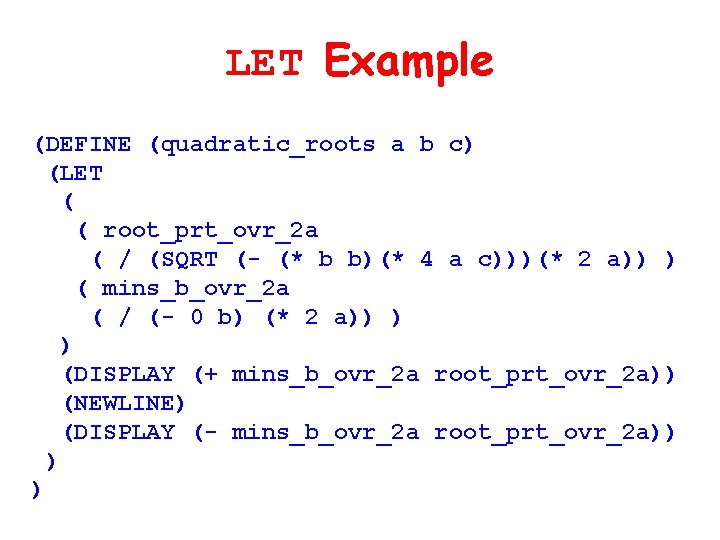
LET Example (DEFINE (quadratic_roots a b c) (LET ( ( root_prt_ovr_2 a ( / (SQRT (- (* b b)(* 4 a c)))(* 2 a)) ) ( mins_b_ovr_2 a ( / (- 0 b) (* 2 a)) ) ) (DISPLAY (+ mins_b_ovr_2 a root_prt_ovr_2 a)) (NEWLINE) (DISPLAY (- mins_b_ovr_2 a root_prt_ovr_2 a)) ) )
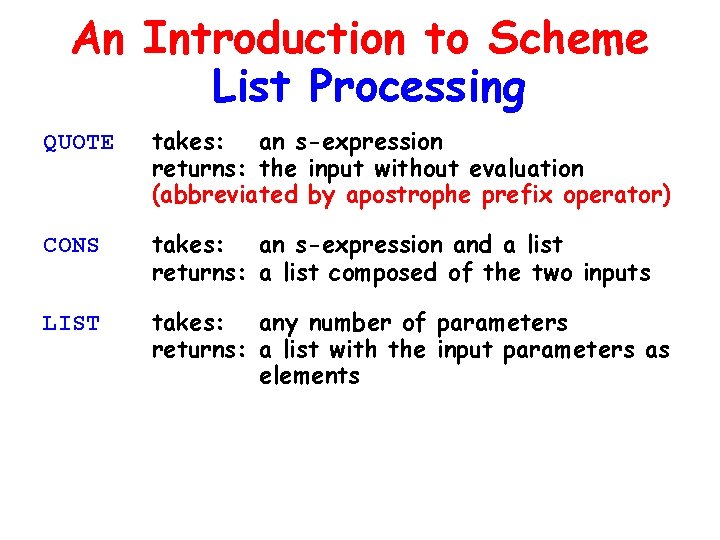
An Introduction to Scheme List Processing QUOTE takes: an s-expression returns: the input without evaluation (abbreviated by apostrophe prefix operator) CONS takes: an s-expression and a list returns: a list composed of the two inputs LIST takes: any number of parameters returns: a list with the input parameters as elements
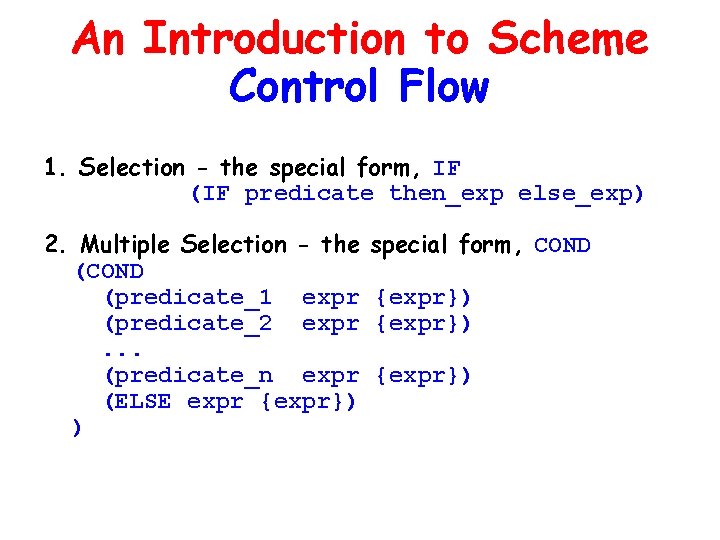
An Introduction to Scheme Control Flow 1. Selection - the special form, IF (IF predicate then_exp else_exp) 2. Multiple Selection - the (COND (predicate_1 expr (predicate_2 expr. . . (predicate_n expr (ELSE expr {expr}) ) special form, COND {expr})
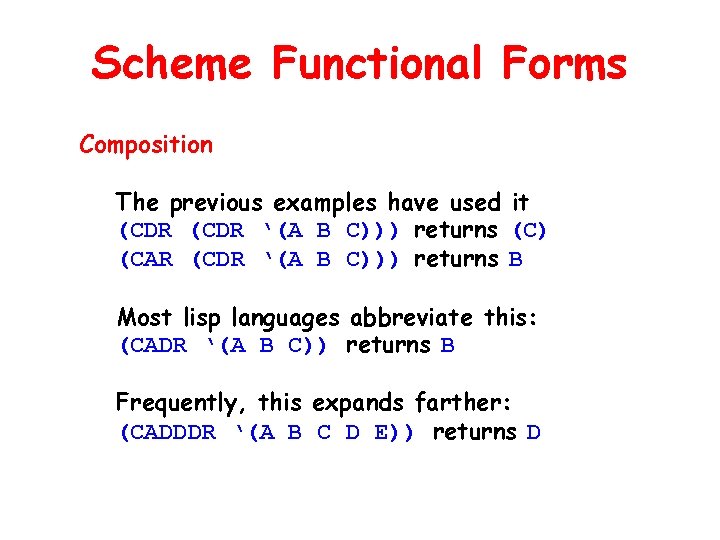
Scheme Functional Forms Composition The previous examples have used it (CDR ‘(A B C))) returns (C) (CAR (CDR ‘(A B C))) returns B Most lisp languages abbreviate this: (CADR ‘(A B C)) returns B Frequently, this expands farther: (CADDDR ‘(A B C D E)) returns D
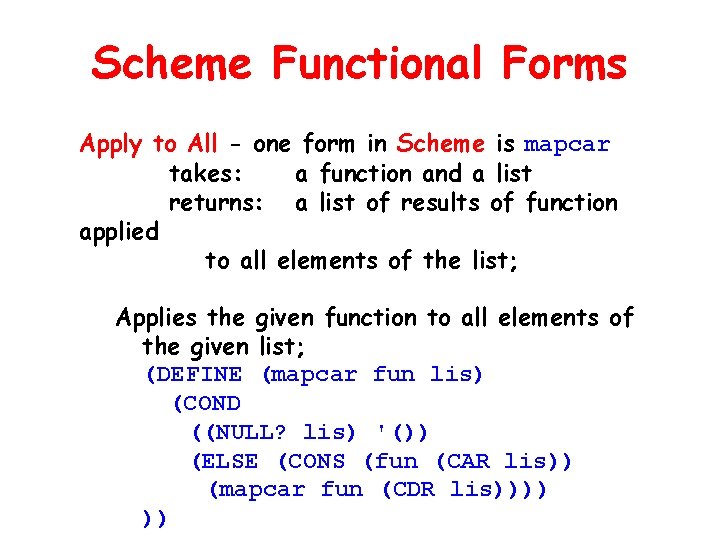
Scheme Functional Forms Apply to All - one form in Scheme is mapcar takes: a function and a list returns: a list of results of function applied to all elements of the list; Applies the given function to all elements of the given list; (DEFINE (mapcar fun lis) (COND ((NULL? lis) '()) (ELSE (CONS (fun (CAR lis)) (mapcar fun (CDR lis)))) ))
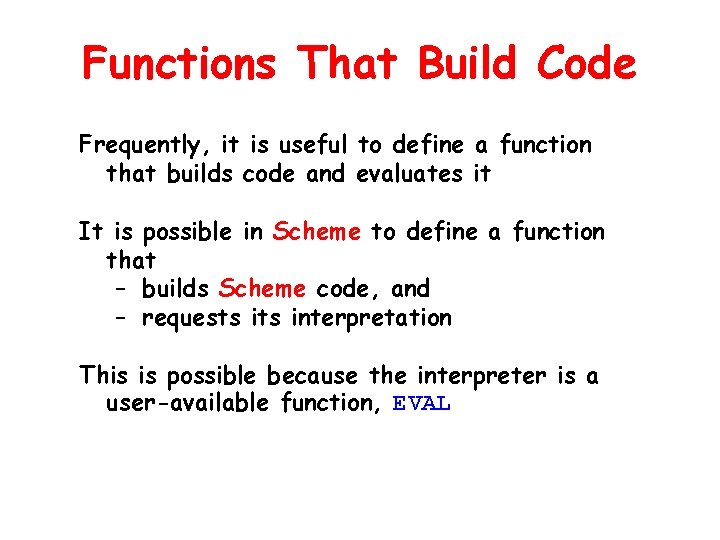
Functions That Build Code Frequently, it is useful to define a function that builds code and evaluates it It is possible in Scheme to define a function that – builds Scheme code, and – requests interpretation This is possible because the interpreter is a user-available function, EVAL
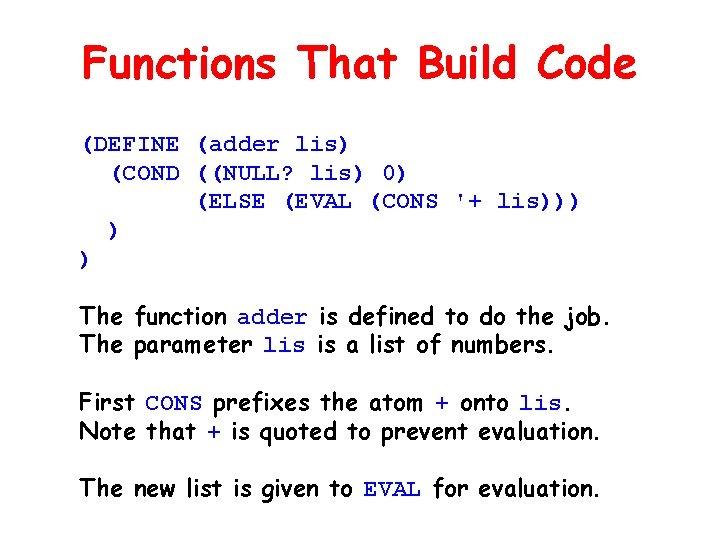
Functions That Build Code (DEFINE (adder lis) (COND ((NULL? lis) 0) (ELSE (EVAL (CONS '+ lis))) ) ) The function adder is defined to do the job. The parameter lis is a list of numbers. First CONS prefixes the atom + onto lis. Note that + is quoted to prevent evaluation. The new list is given to EVAL for evaluation.
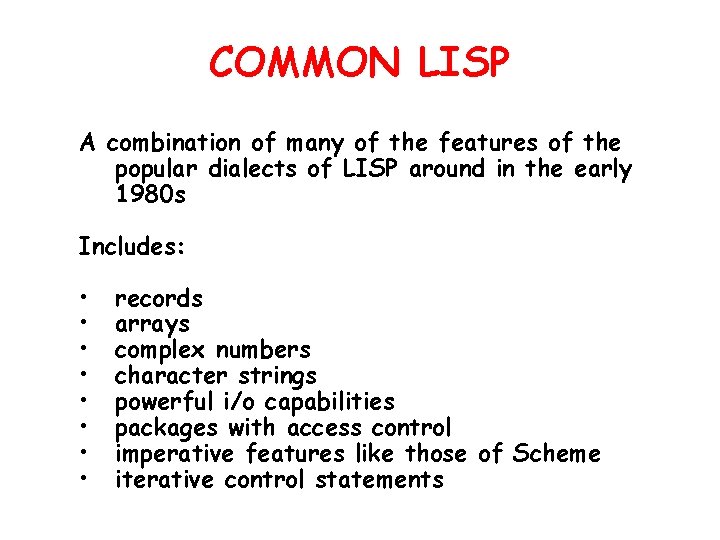
COMMON LISP A combination of many of the features of the popular dialects of LISP around in the early 1980 s Includes: • • records arrays complex numbers character strings powerful i/o capabilities packages with access control imperative features like those of Scheme iterative control statements
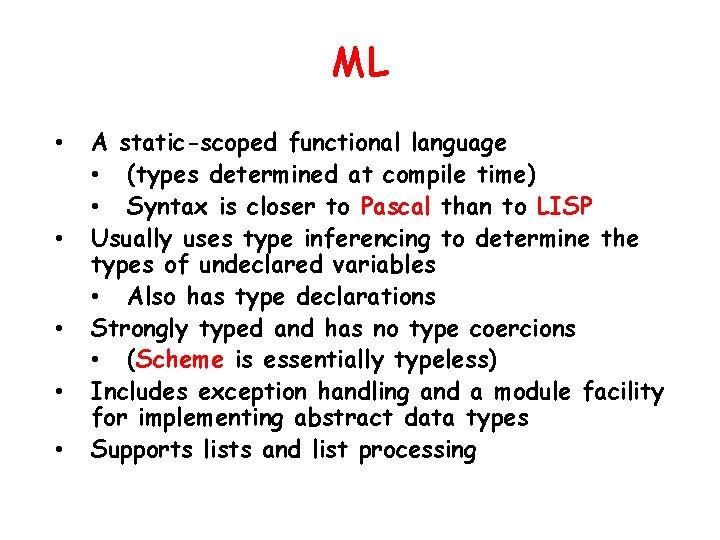
ML • • • A static-scoped functional language • (types determined at compile time) • Syntax is closer to Pascal than to LISP Usually uses type inferencing to determine the types of undeclared variables • Also has type declarations Strongly typed and has no type coercions • (Scheme is essentially typeless) Includes exception handling and a module facility for implementing abstract data types Supports lists and list processing
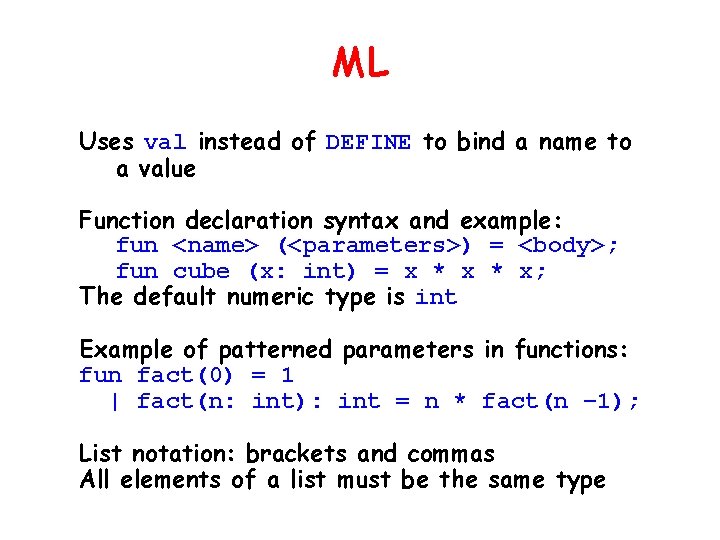
ML Uses val instead of DEFINE to bind a name to a value Function declaration syntax and example: fun <name> (<parameters>) = <body>; fun cube (x: int) = x * x; The default numeric type is int Example of patterned parameters in functions: fun fact(0) = 1 | fact(n: int): int = n * fact(n – 1); List notation: brackets and commas All elements of a list must be the same type
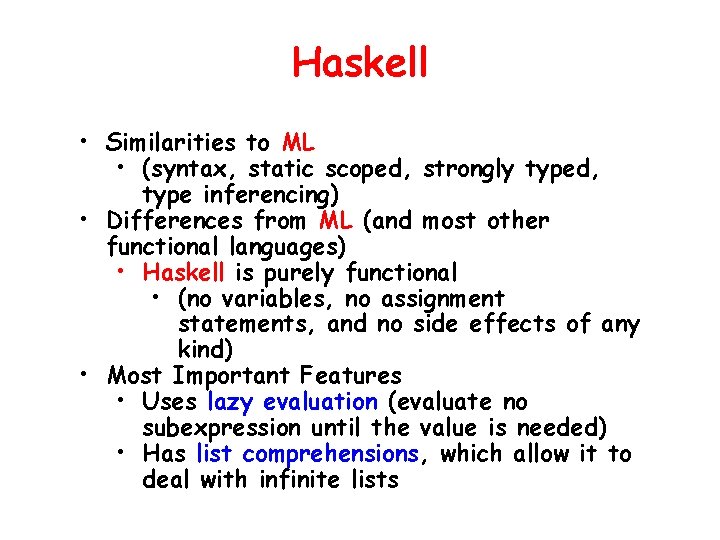
Haskell • Similarities to ML • (syntax, static scoped, strongly typed, type inferencing) • Differences from ML (and most other functional languages) • Haskell is purely functional • (no variables, no assignment statements, and no side effects of any kind) • Most Important Features • Uses lazy evaluation (evaluate no subexpression until the value is needed) • Has list comprehensions, which allow it to deal with infinite lists
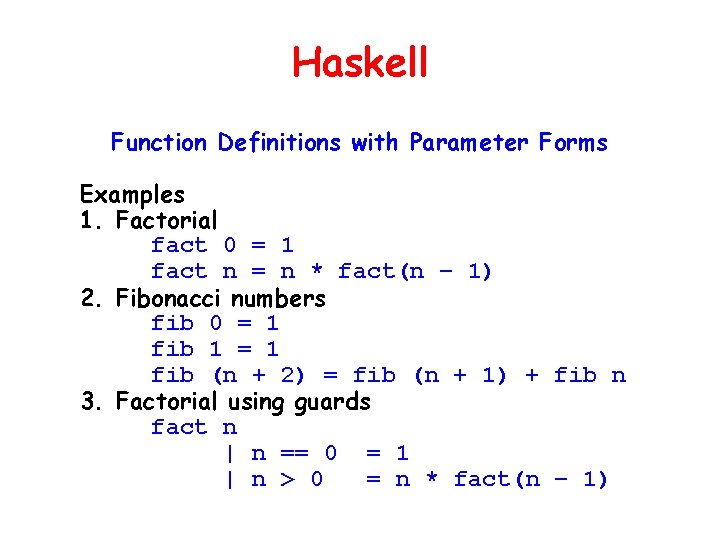
Haskell Function Definitions with Parameter Forms Examples 1. Factorial fact 0 = 1 fact n = n * fact(n – 1) 2. Fibonacci numbers fib 0 = 1 fib 1 = 1 fib (n + 2) = fib (n + 1) + fib n 3. Factorial using guards fact n | n == 0 = 1 | n > 0 = n * fact(n – 1)
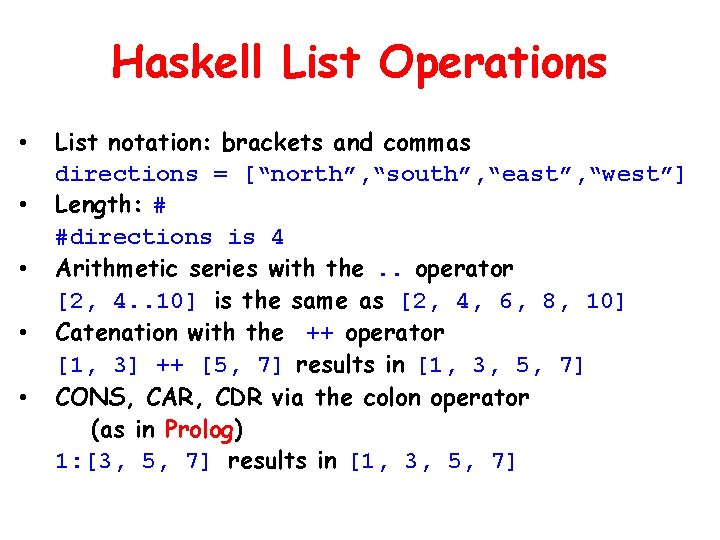
Haskell List Operations • • • List notation: brackets and commas directions = [“north”, “south”, “east”, “west”] Length: # #directions is 4 Arithmetic series with the. . operator [2, 4. . 10] is the same as [2, 4, 6, 8, 10] Catenation with the ++ operator [1, 3] ++ [5, 7] results in [1, 3, 5, 7] CONS, CAR, CDR via the colon operator (as in Prolog) 1: [3, 5, 7] results in [1, 3, 5, 7]
![Haskell List Operations Examples product 1 product a x a Haskell List Operations Examples product [ ] = 1 product (a: x) = a](https://slidetodoc.com/presentation_image_h/b3a522722addcda221caf6647792a500/image-48.jpg)
Haskell List Operations Examples product [ ] = 1 product (a: x) = a * product x fact n = product [1. . n] quadratic_root a b c = [part 1 – part 2, part 1 + part 2] where part 1 = -b/(2. 0*a) part 2 = sqrt(b^2– 4. 0*a*c)/(2. 0*a)
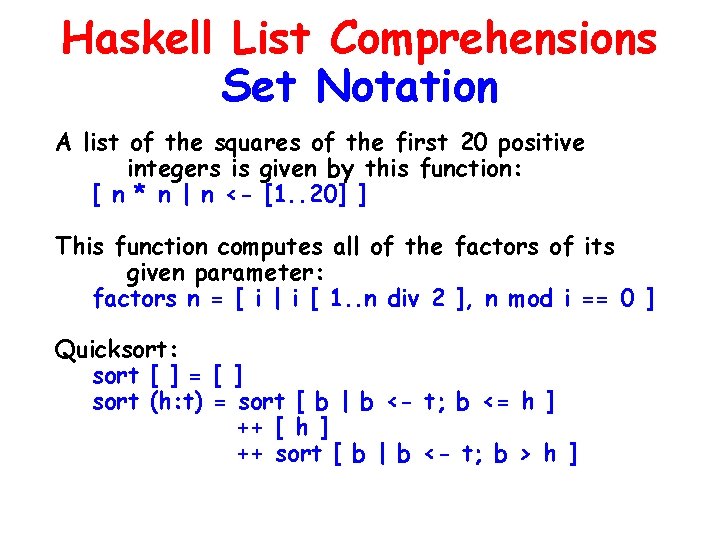
Haskell List Comprehensions Set Notation A list of the squares of the first 20 positive integers is given by this function: [ n * n | n <- [1. . 20] ] This function computes all of the factors of its given parameter: factors n = [ i | i [ 1. . n div 2 ], n mod i == 0 ] Quicksort: sort [ ] = [ ] sort (h: t) = sort [ b | b <- t; b <= h ] ++ [ h ] ++ sort [ b | b <- t; b > h ]
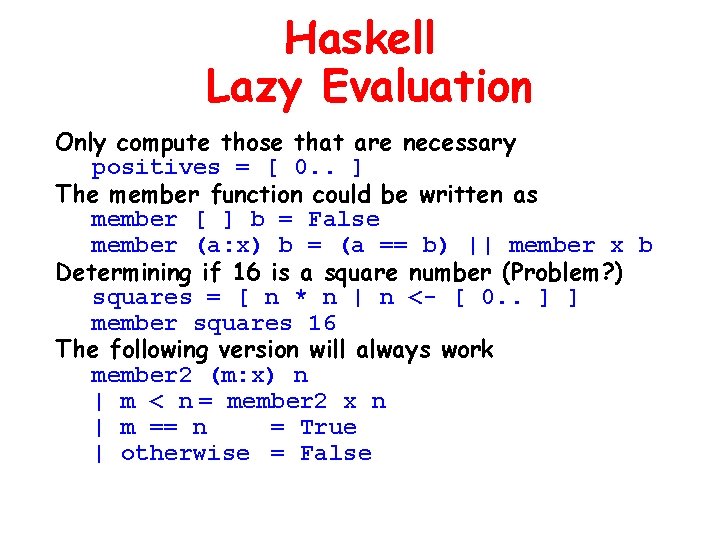
Haskell Lazy Evaluation Only compute those that are necessary positives = [ 0. . ] The member function could be written as member [ ] b = False member (a: x) b = (a == b) || member x b Determining if 16 is a square number (Problem? ) squares = [ n * n | n <- [ 0. . ] ] member squares 16 The following version will always work member 2 (m: x) n | m < n = member 2 x n | m == n = True | otherwise = False
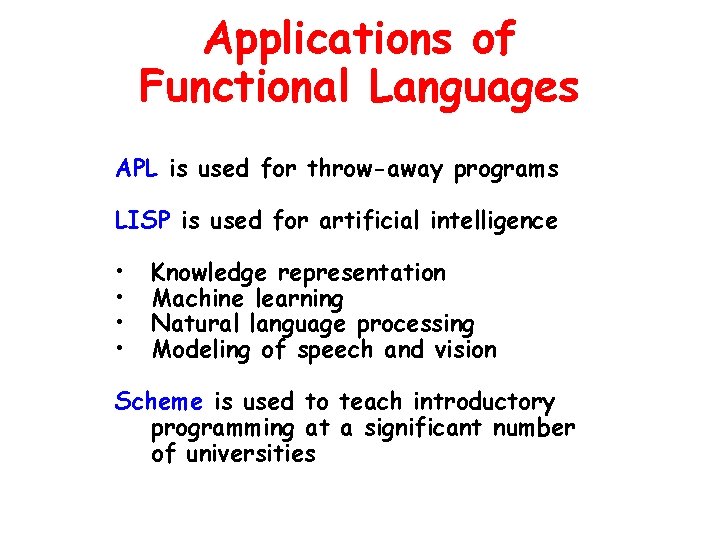
Applications of Functional Languages APL is used for throw-away programs LISP is used for artificial intelligence • • Knowledge representation Machine learning Natural language processing Modeling of speech and vision Scheme is used to teach introductory programming at a significant number of universities
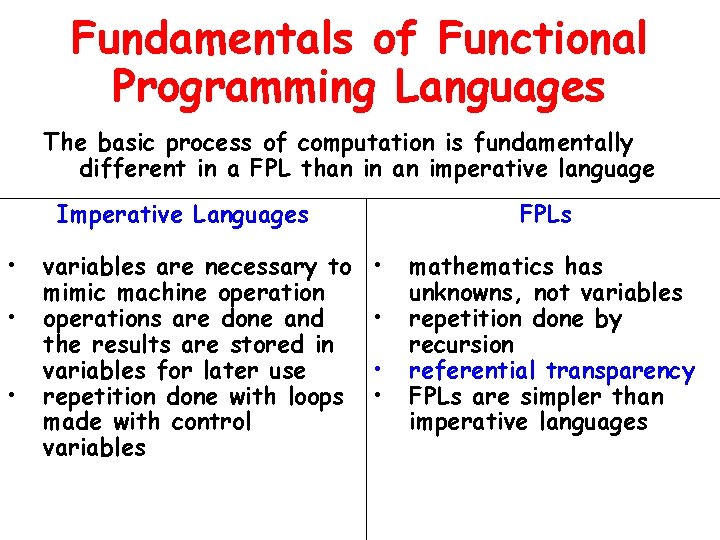
Fundamentals of Functional Programming Languages The basic process of computation is fundamentally different in a FPL than in an imperative language Imperative Languages • • • variables are necessary to mimic machine operations are done and the results are stored in variables for later use repetition done with loops made with control variables FPLs • • mathematics has unknowns, not variables repetition done by recursion referential transparency FPLs are simpler than imperative languages
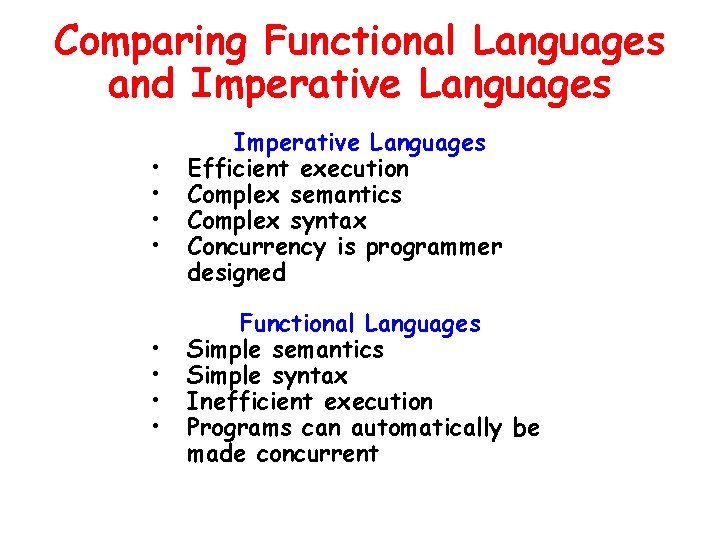
Comparing Functional Languages and Imperative Languages • • Imperative Languages Efficient execution Complex semantics Complex syntax Concurrency is programmer designed • • Functional Languages Simple semantics Simple syntax Inefficient execution Programs can automatically be made concurrent
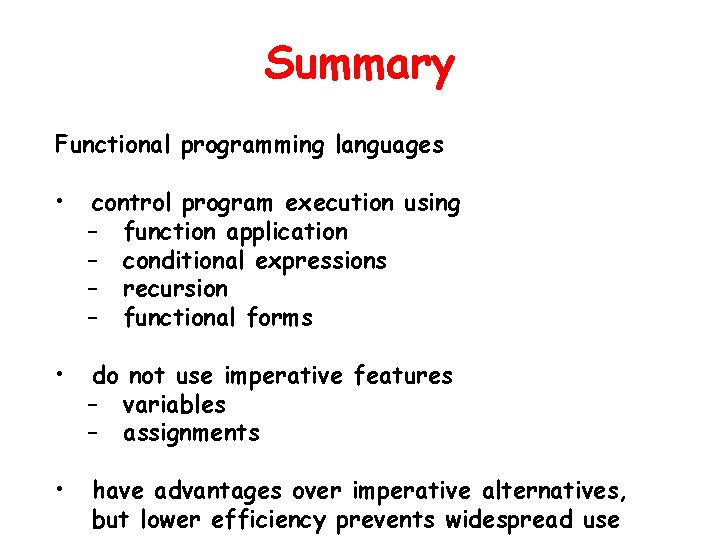
Summary Functional programming languages • control program execution using – function application – conditional expressions – recursion – functional forms • do not use imperative features – variables – assignments • have advantages over imperative alternatives, but lower efficiency prevents widespread use
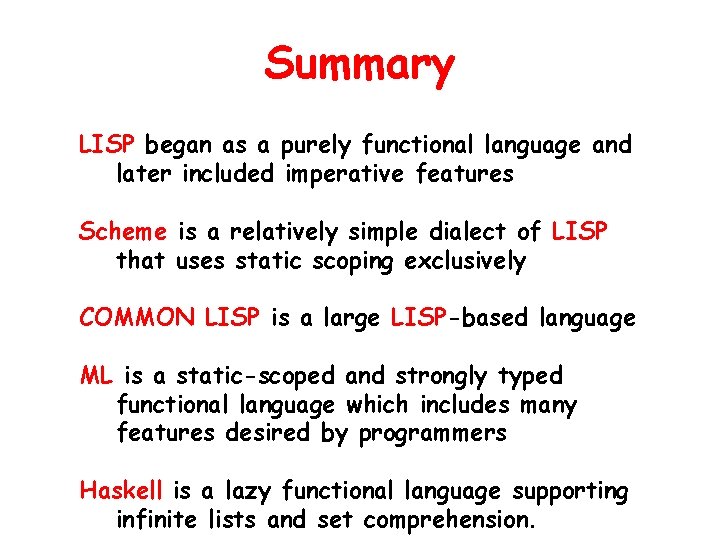
Summary LISP began as a purely functional language and later included imperative features Scheme is a relatively simple dialect of LISP that uses static scoping exclusively COMMON LISP is a large LISP-based language ML is a static-scoped and strongly typed functional language which includes many features desired by programmers Haskell is a lazy functional language supporting infinite lists and set comprehension.