Data Structures for Java William H Ford William
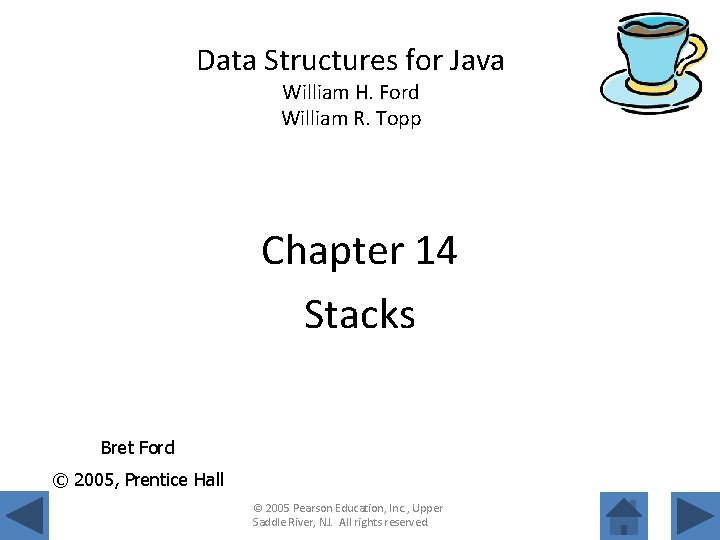
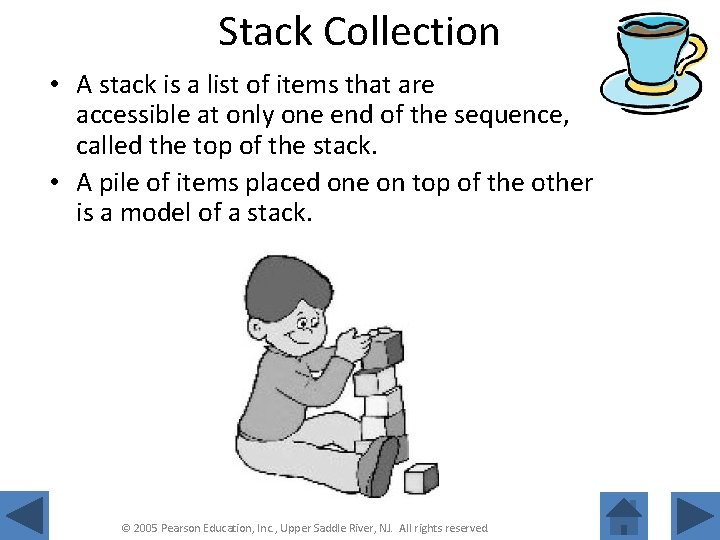
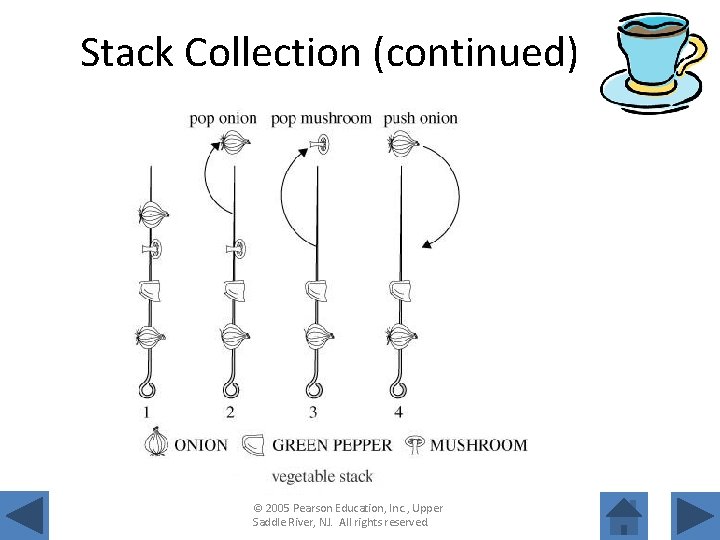
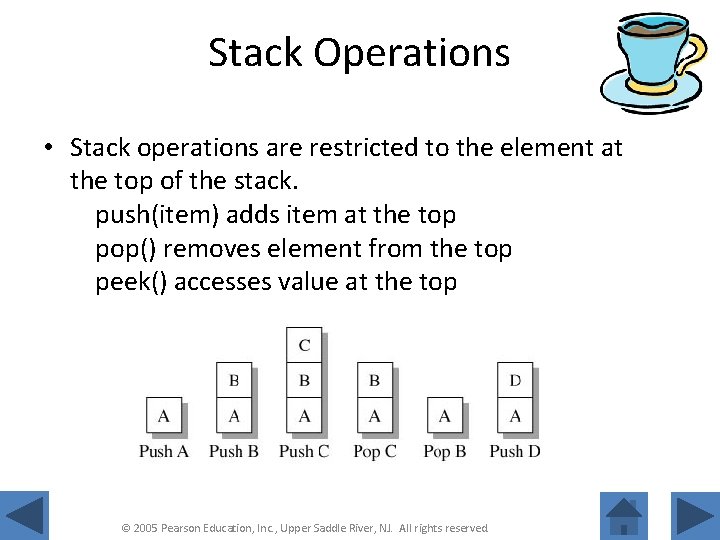
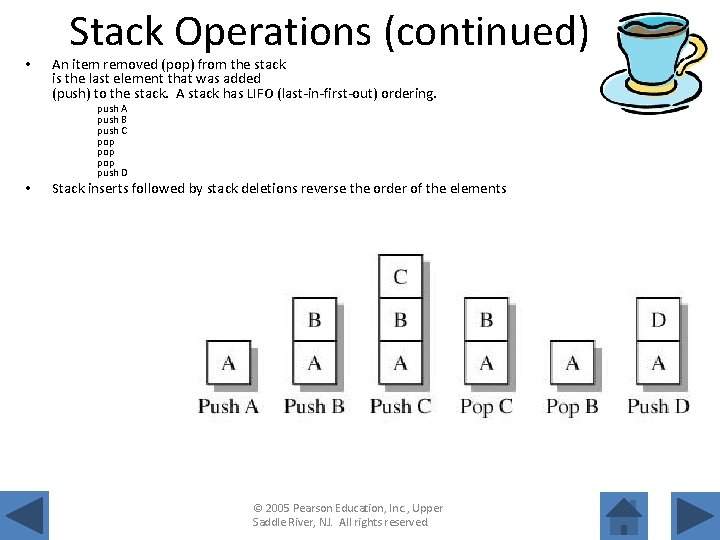
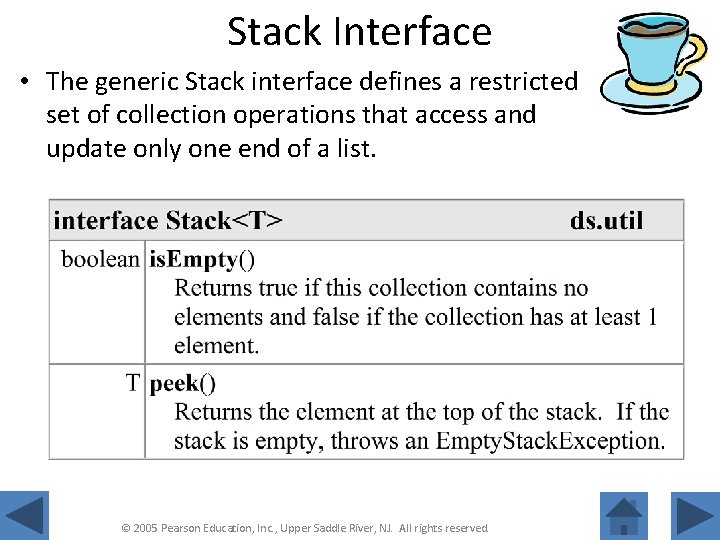
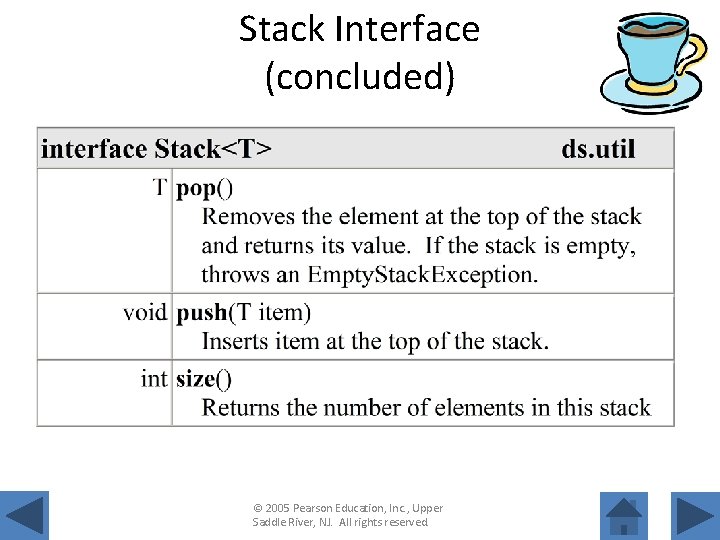
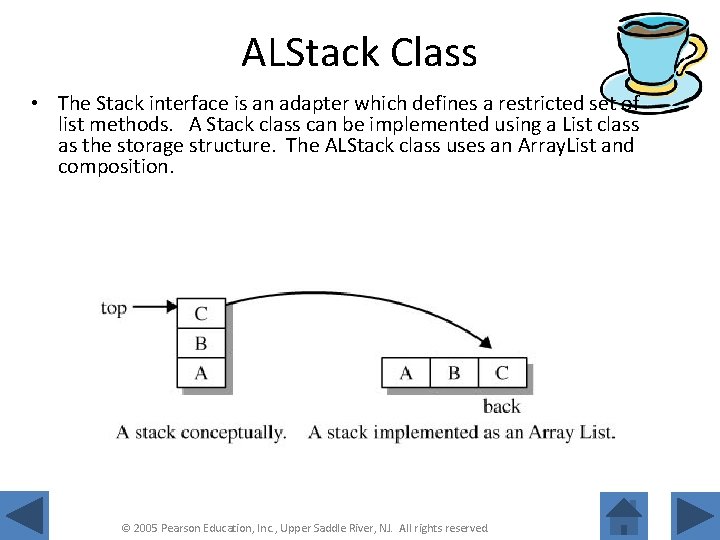
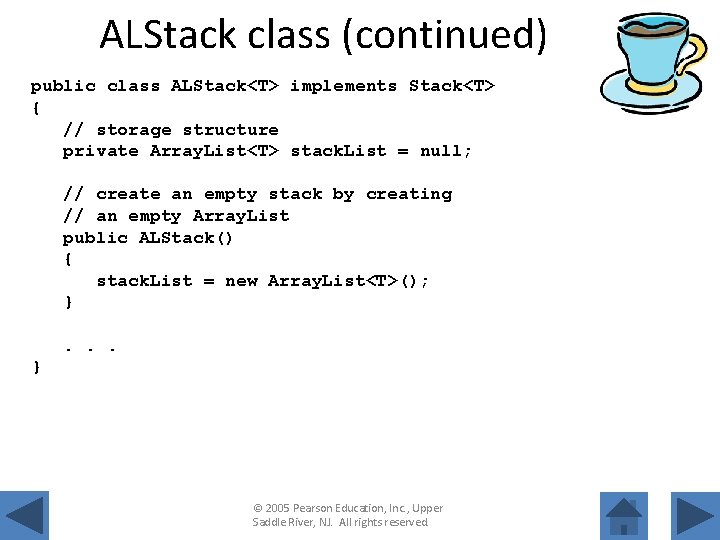
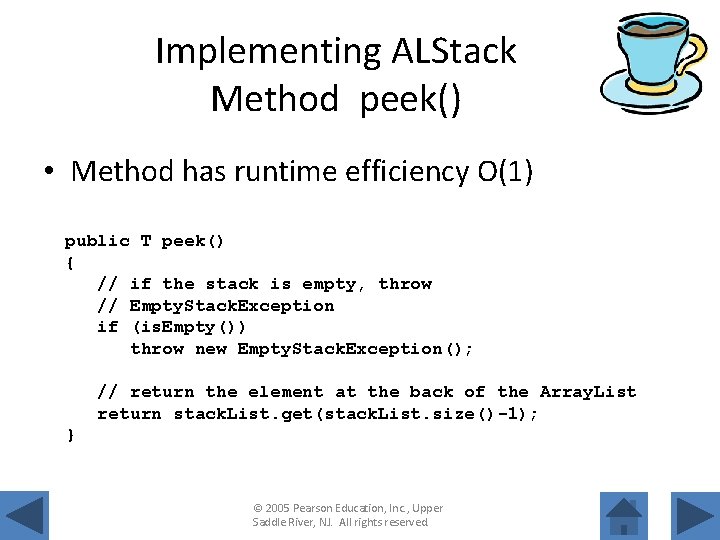
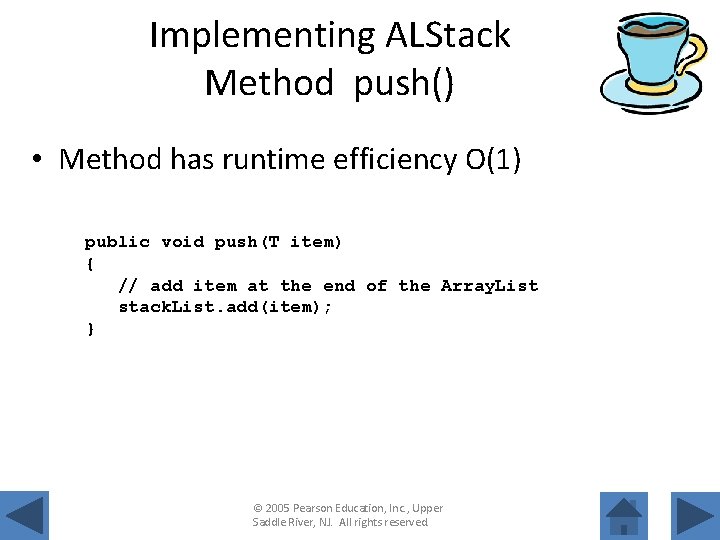
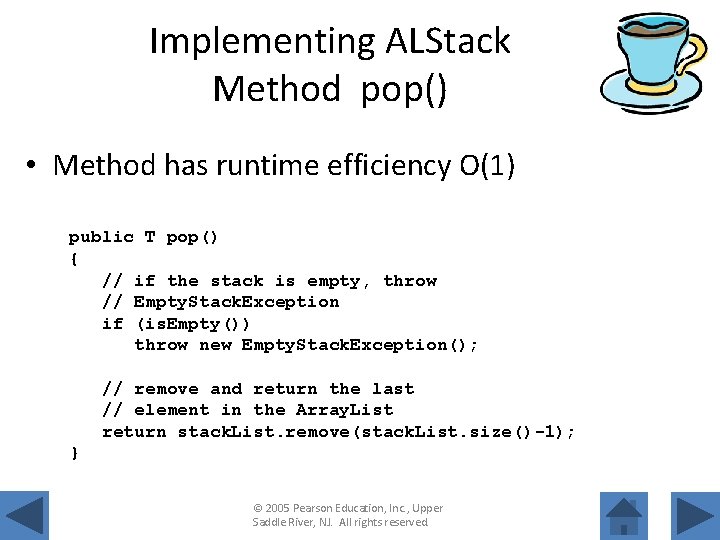
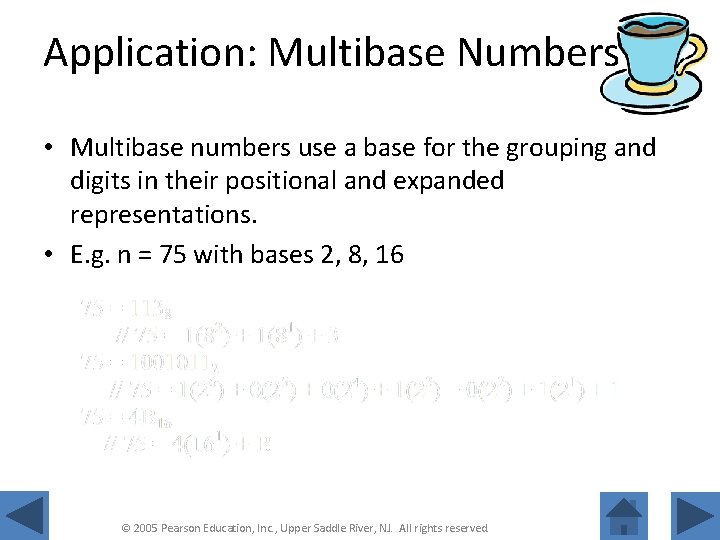
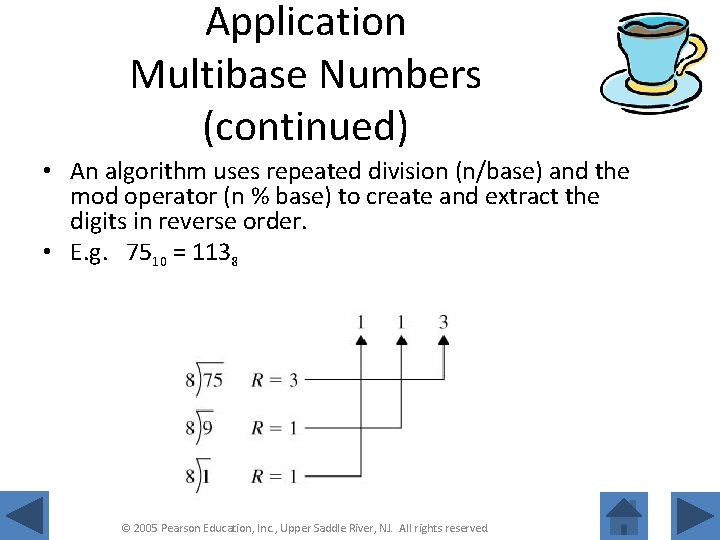
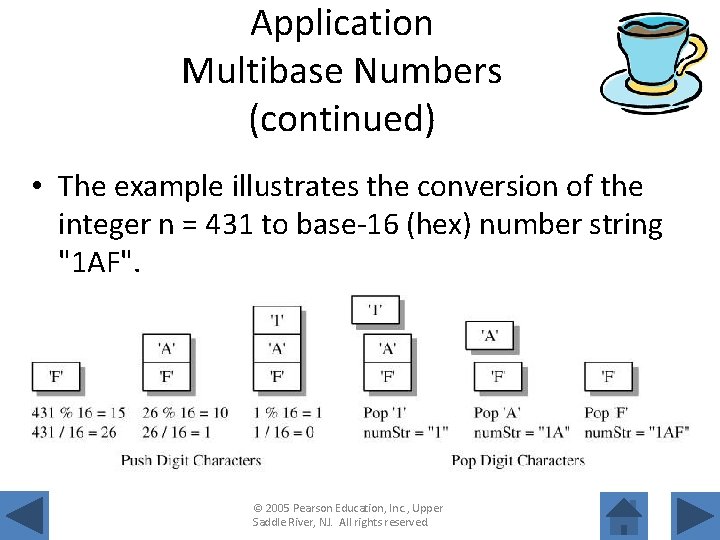
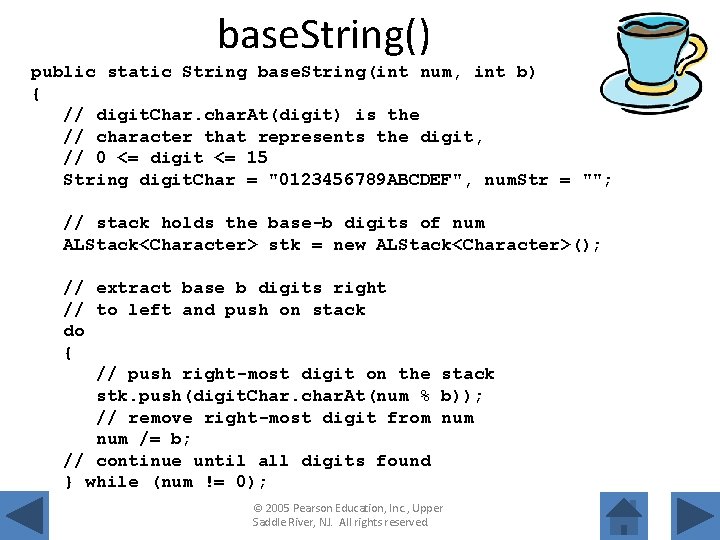
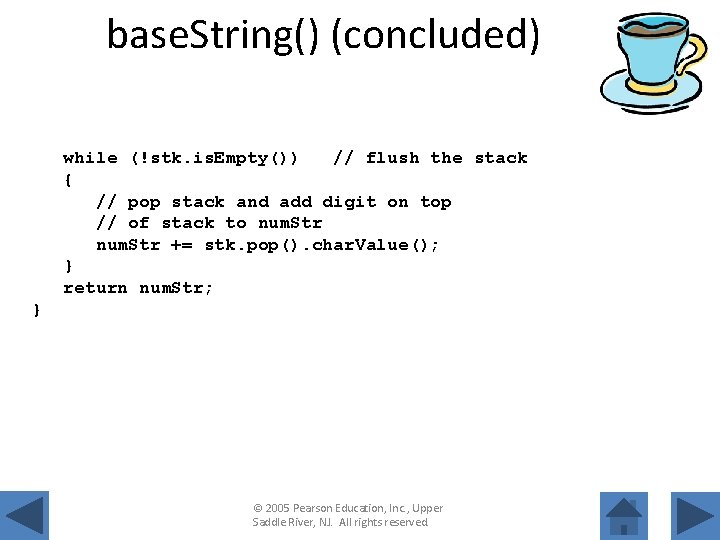
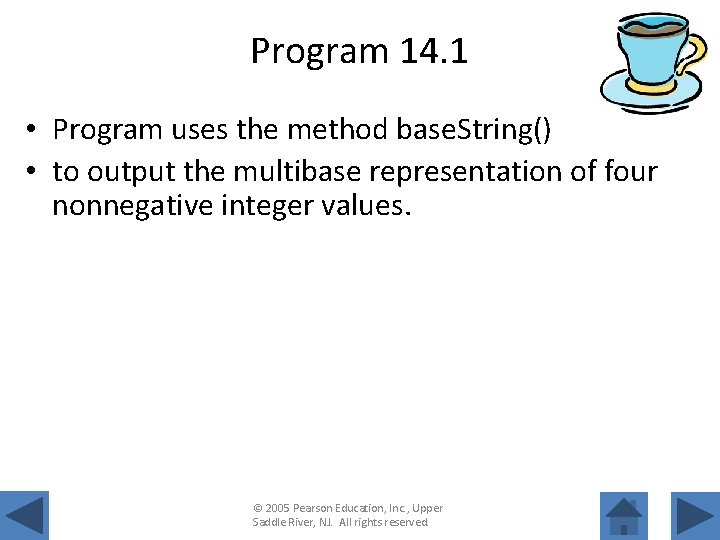
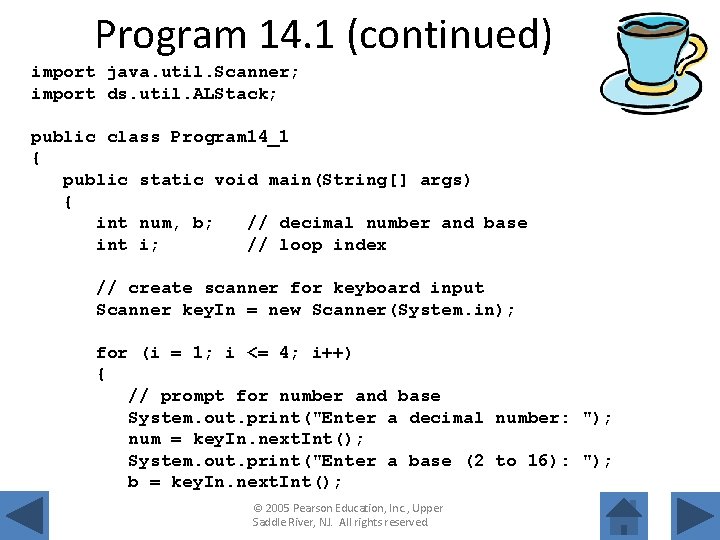
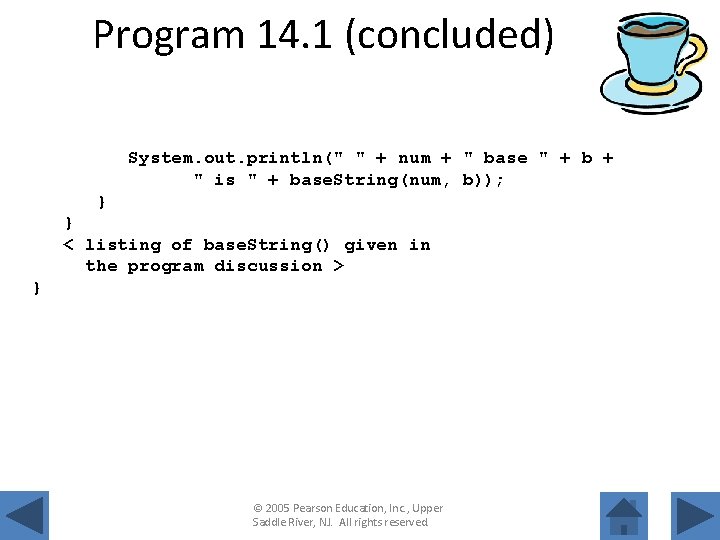
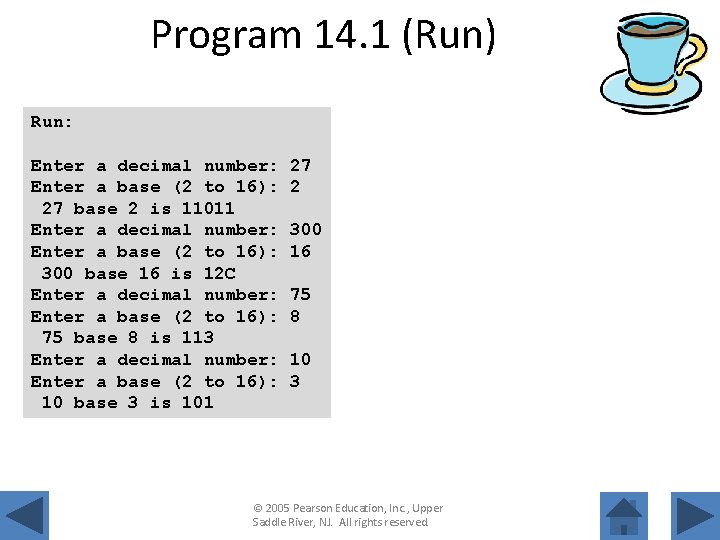
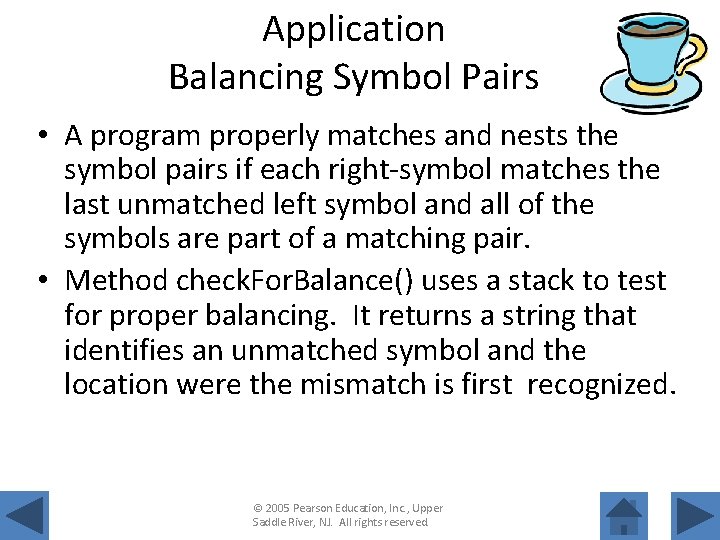
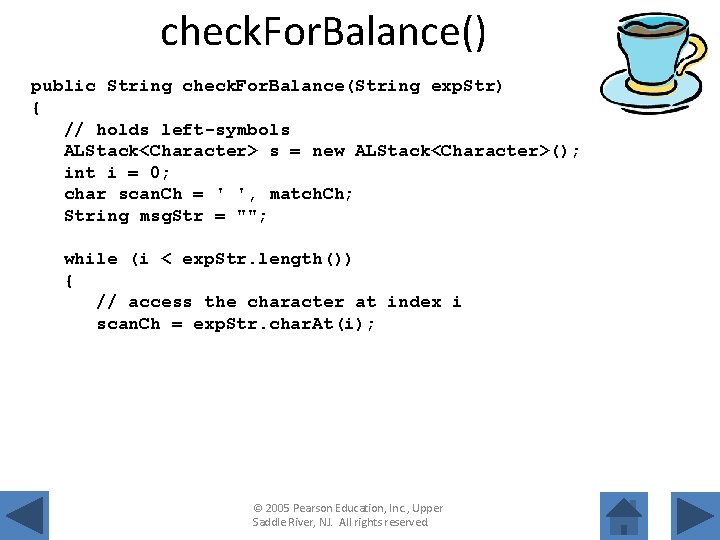
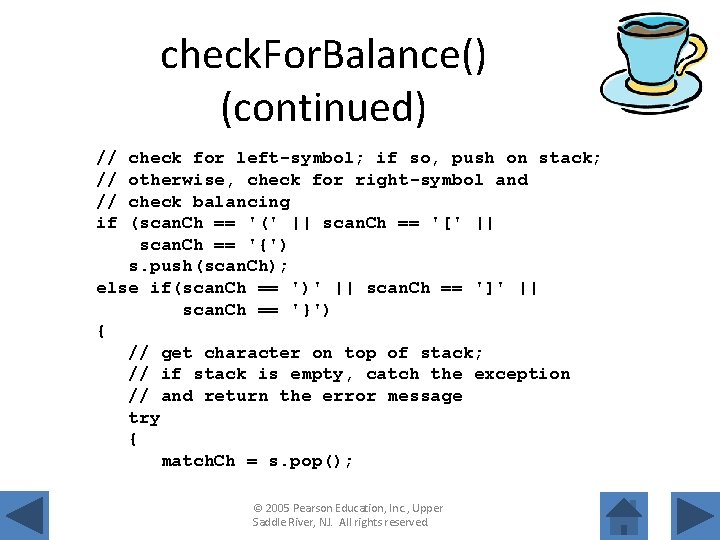
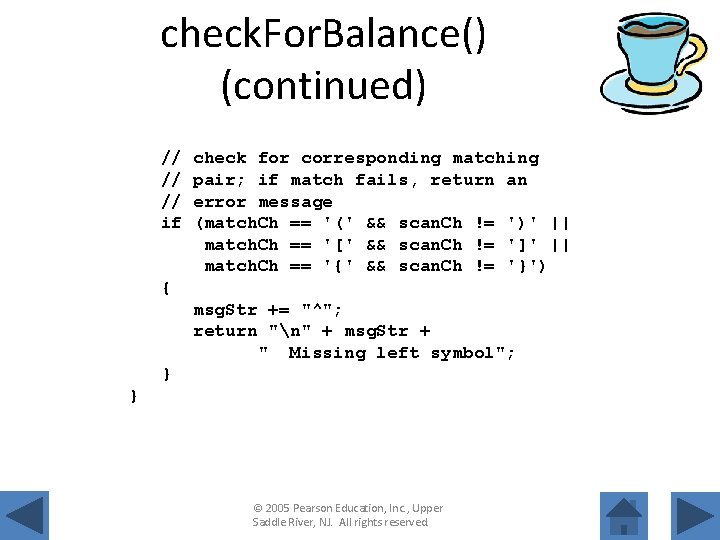
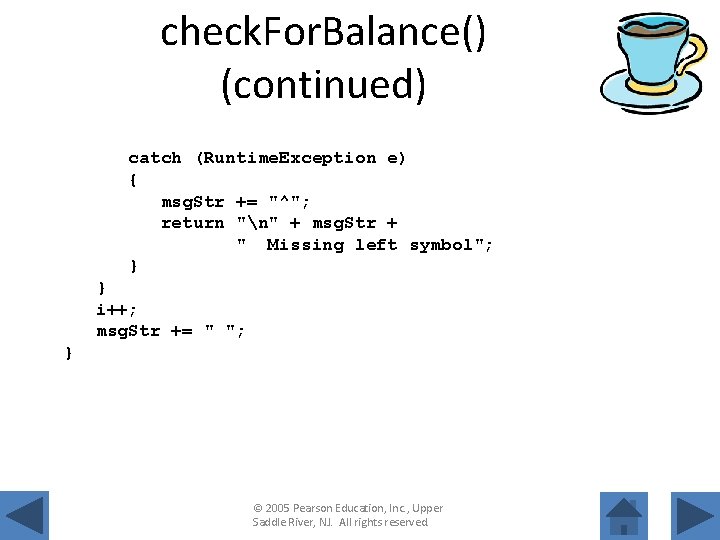
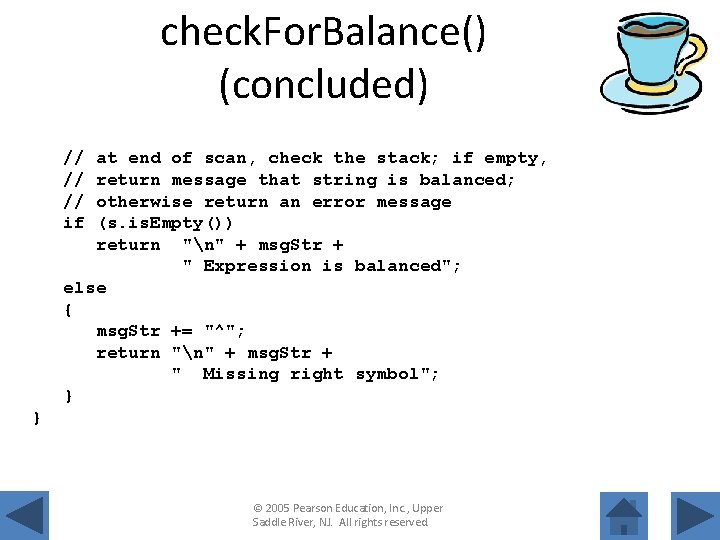
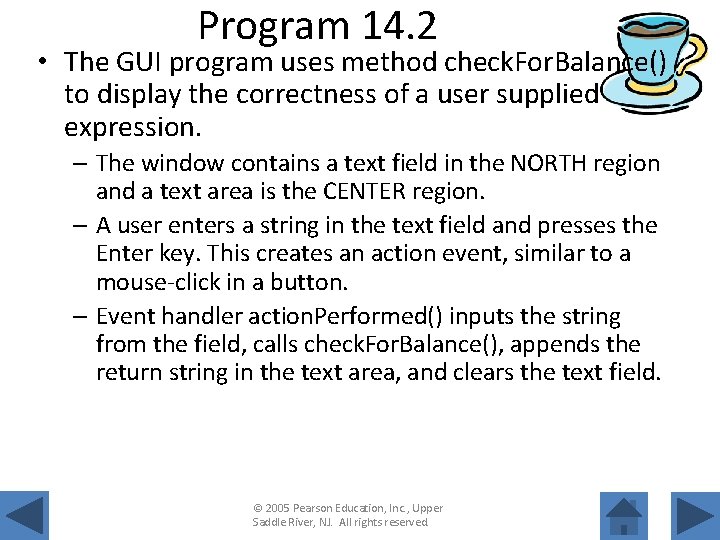
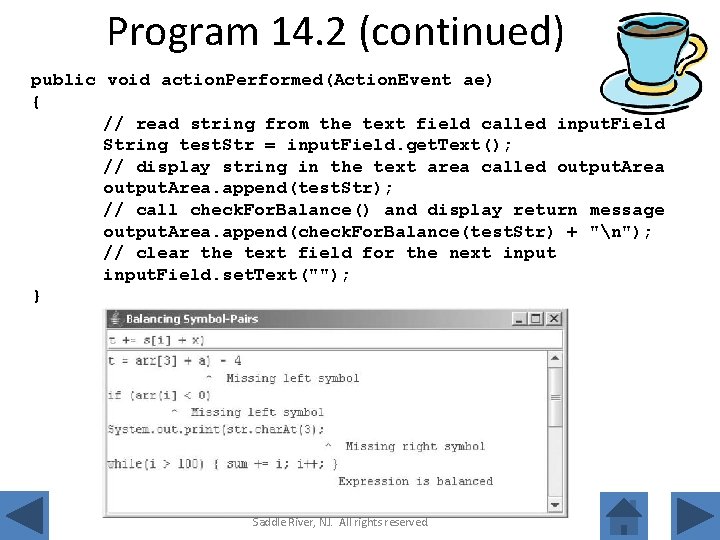
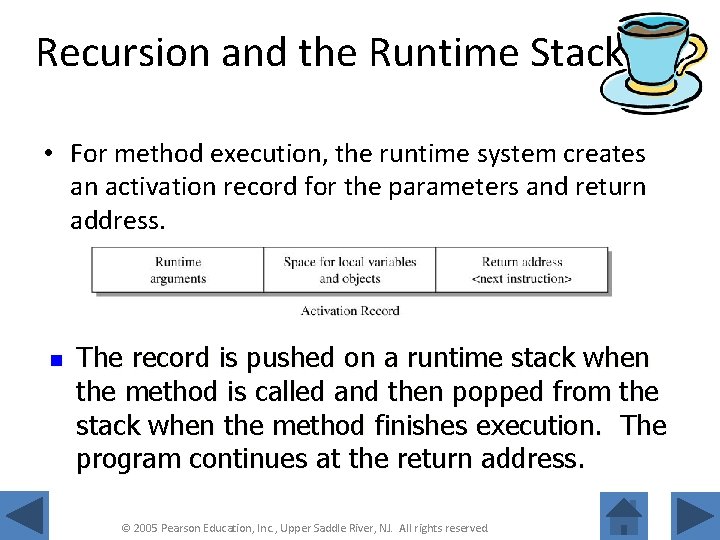
![Example: fact() • Calling fact() in main: public static void main(String[] args) { int Example: fact() • Calling fact() in main: public static void main(String[] args) { int](https://slidetodoc.com/presentation_image_h2/630ba2d0ab6d894c07c2af3124647f24/image-31.jpg)
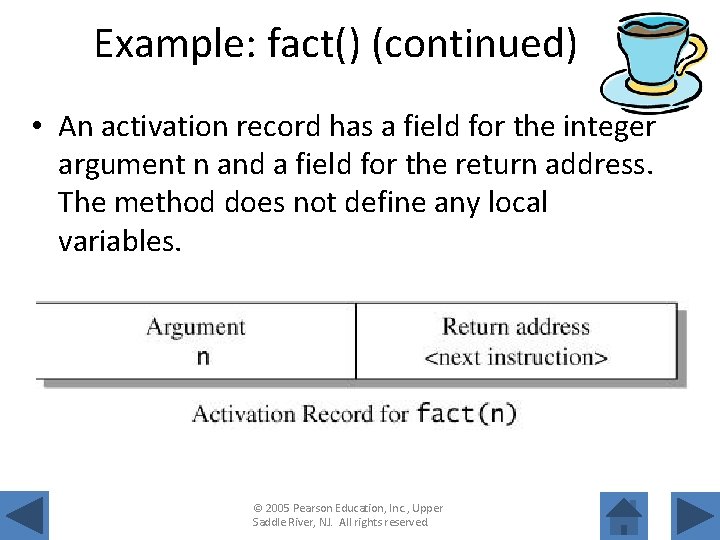
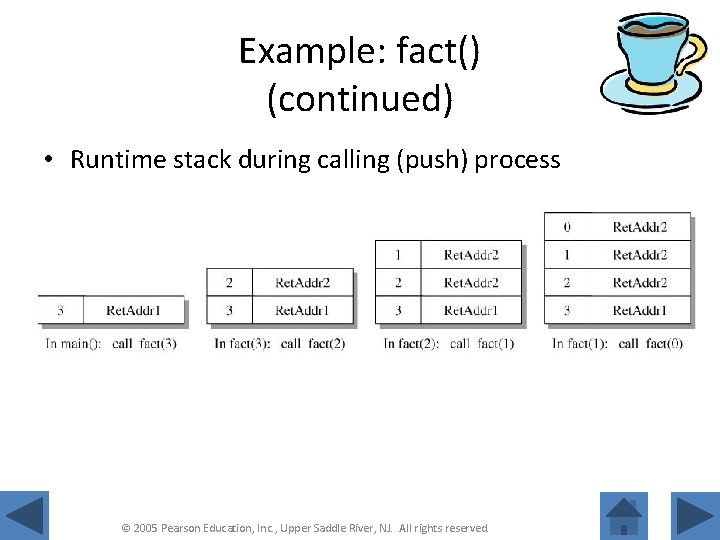
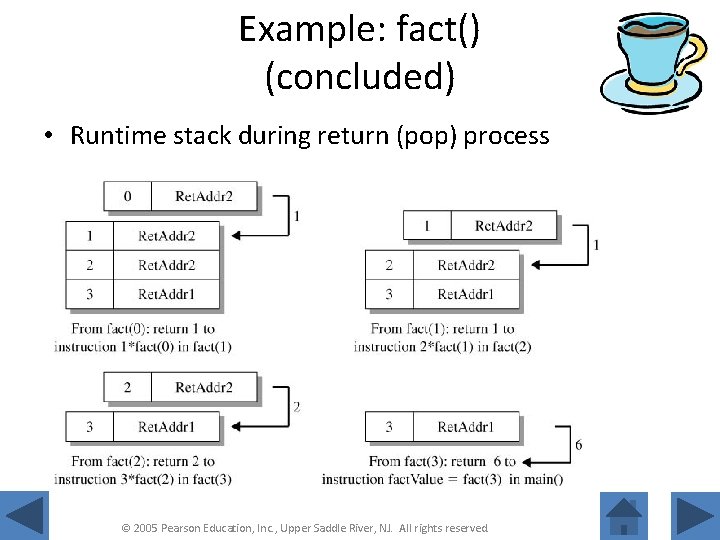
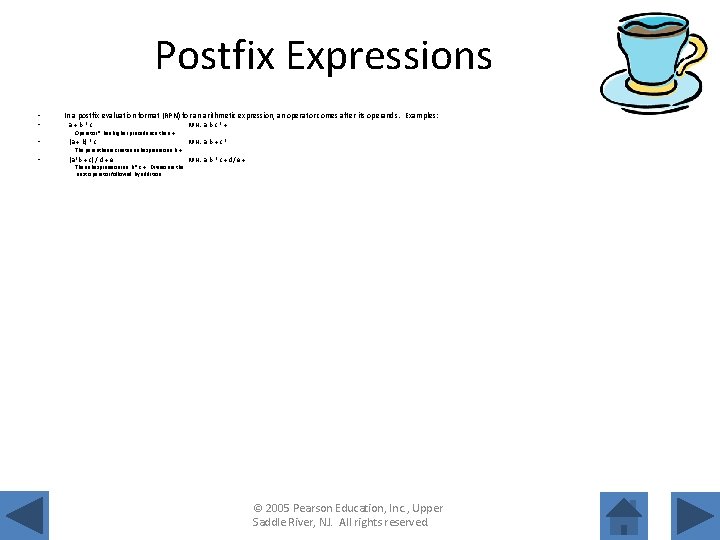
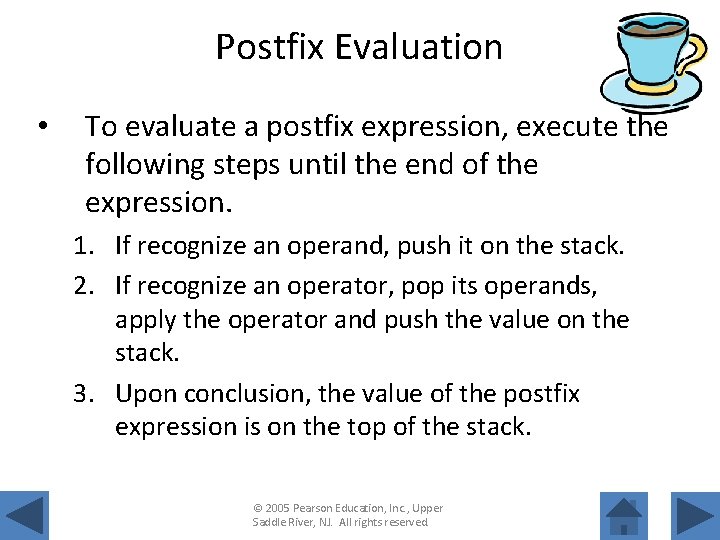
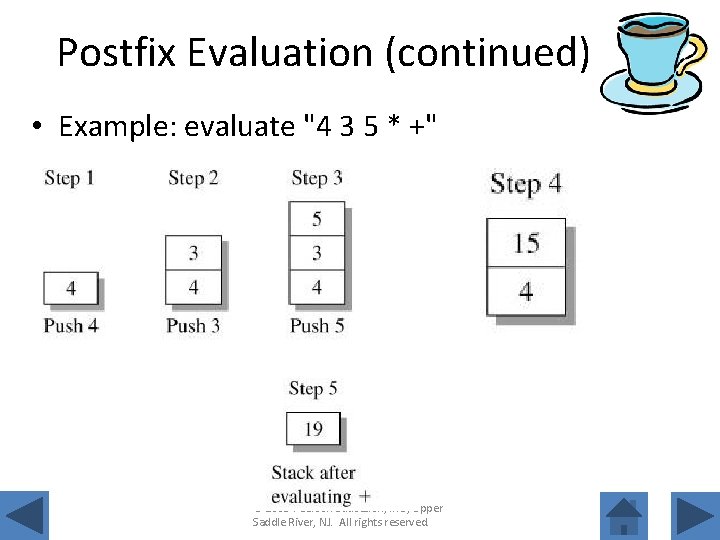
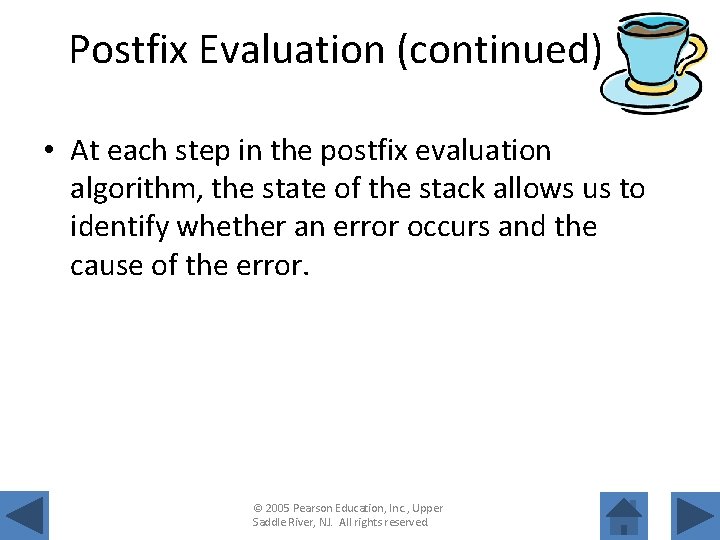
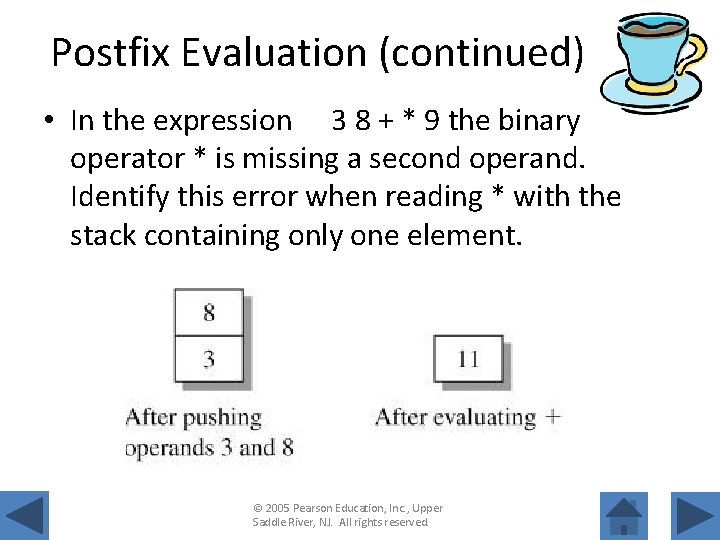
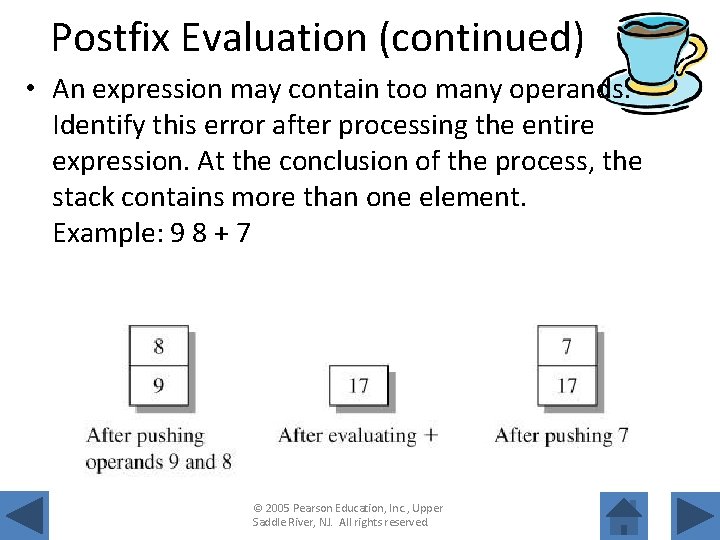
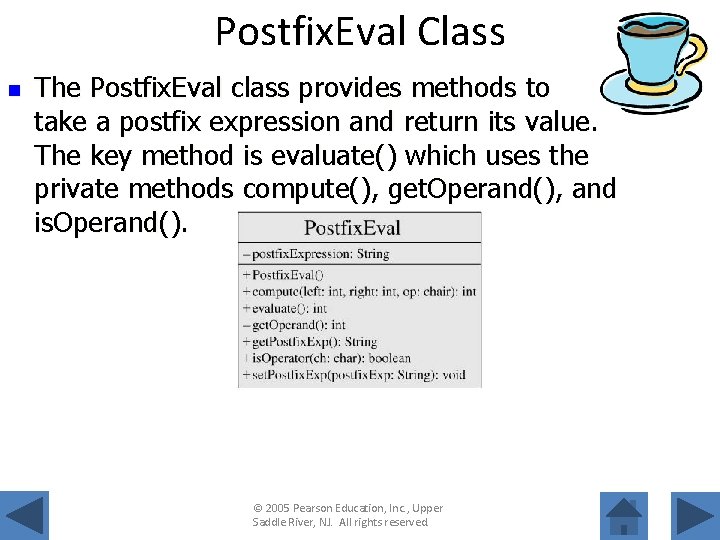
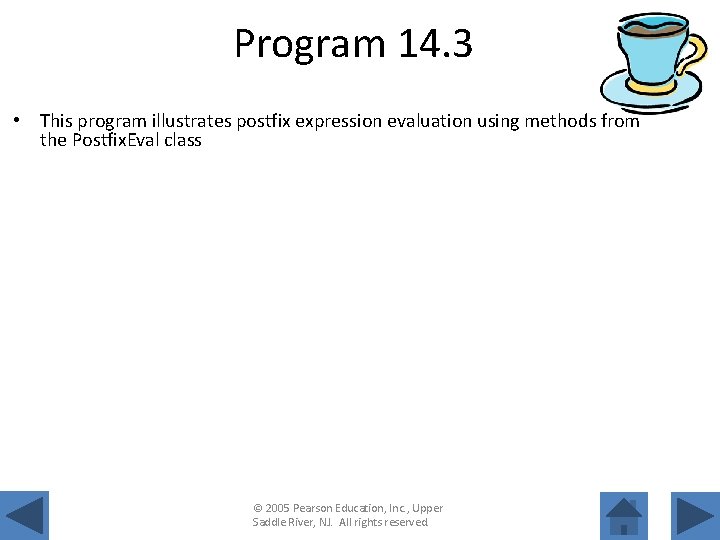
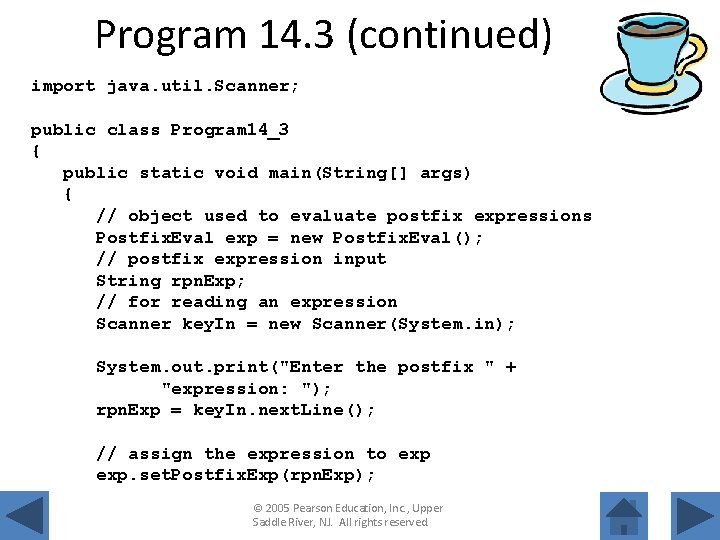
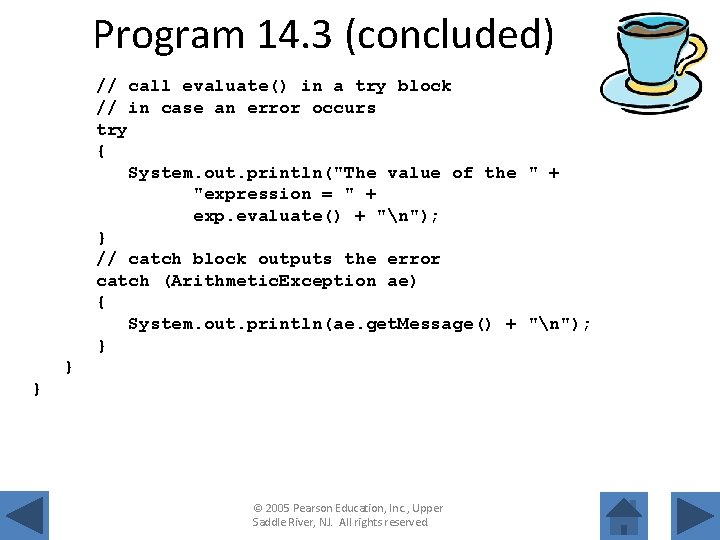
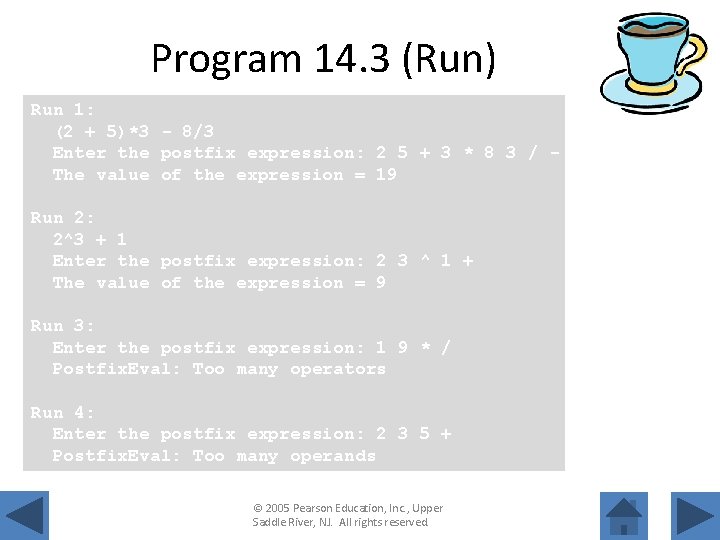
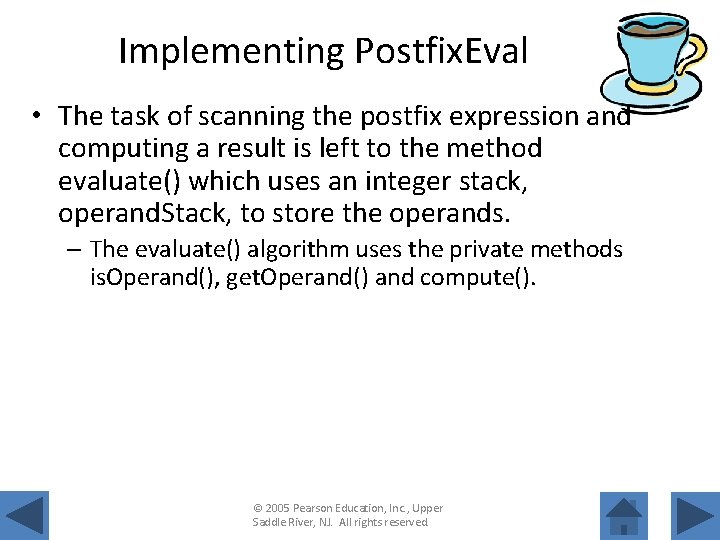
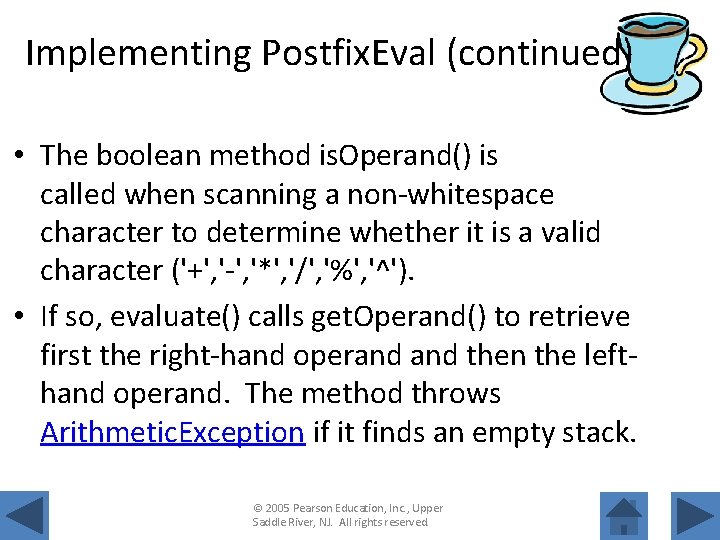
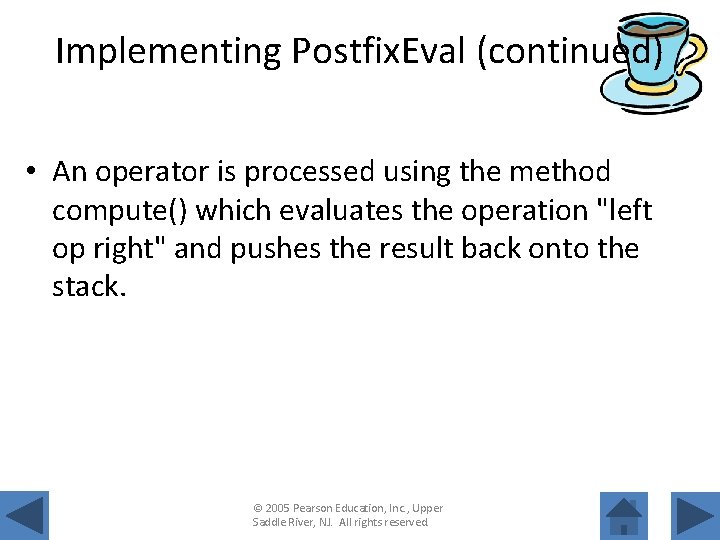
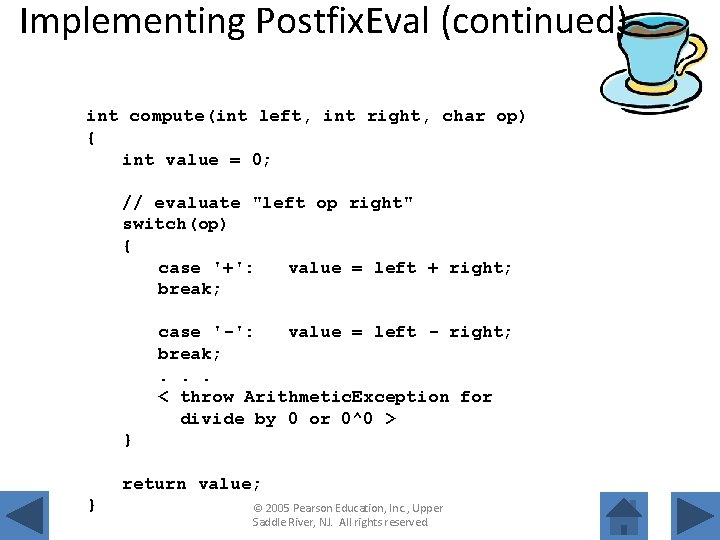
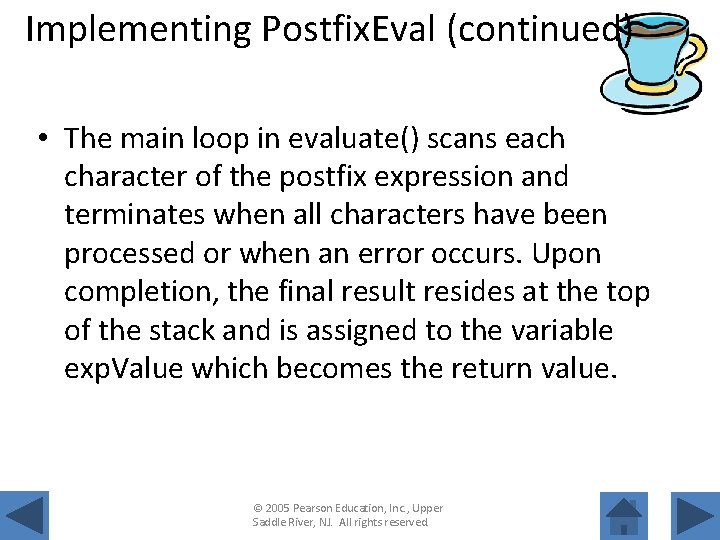
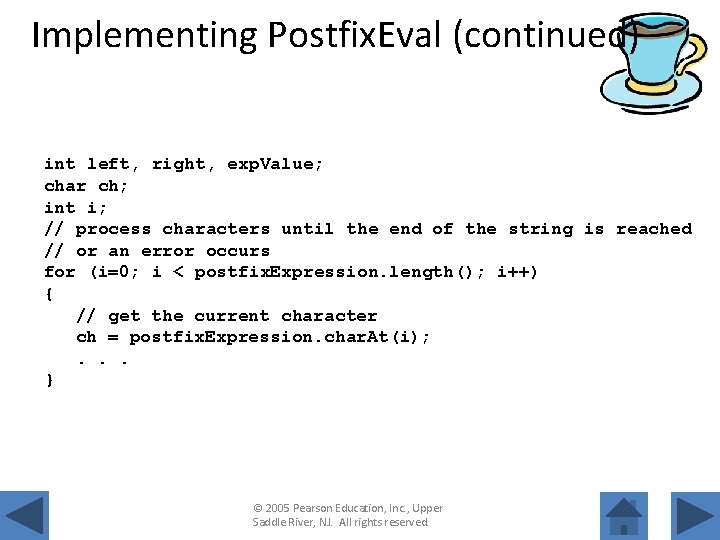
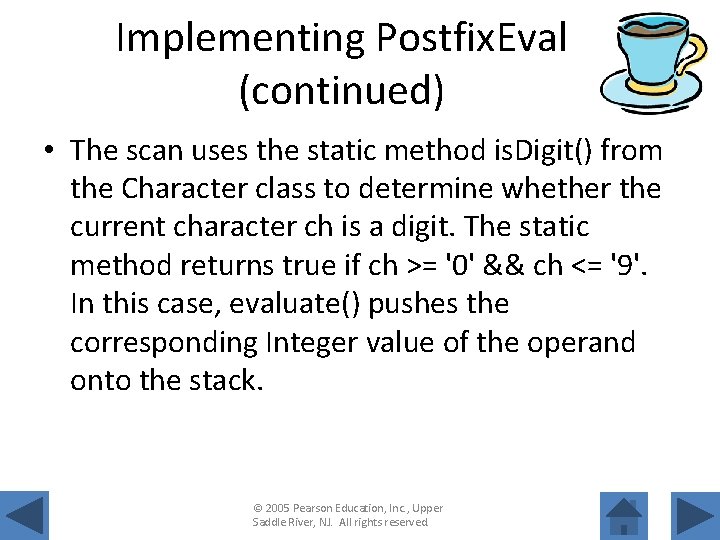
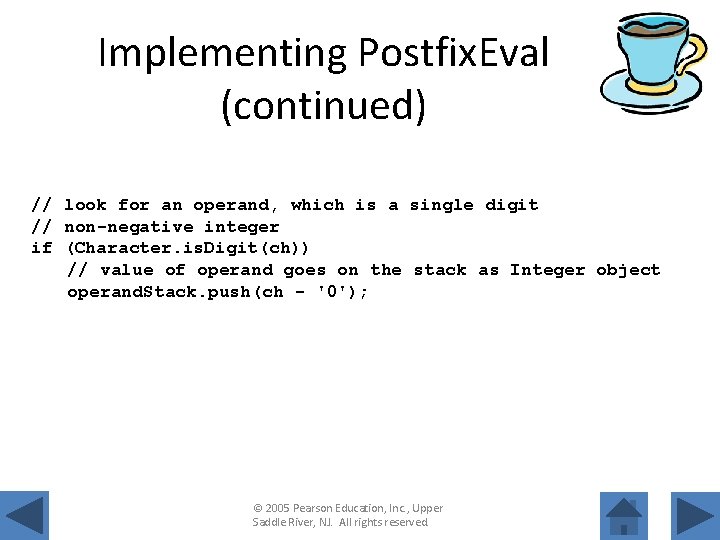
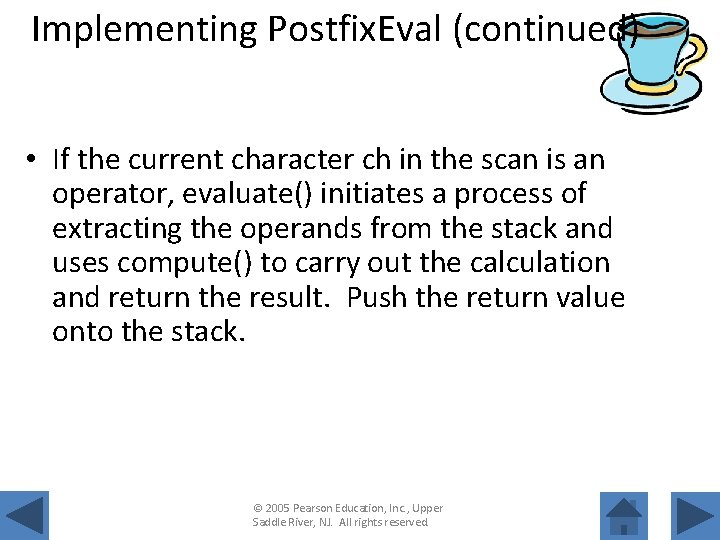
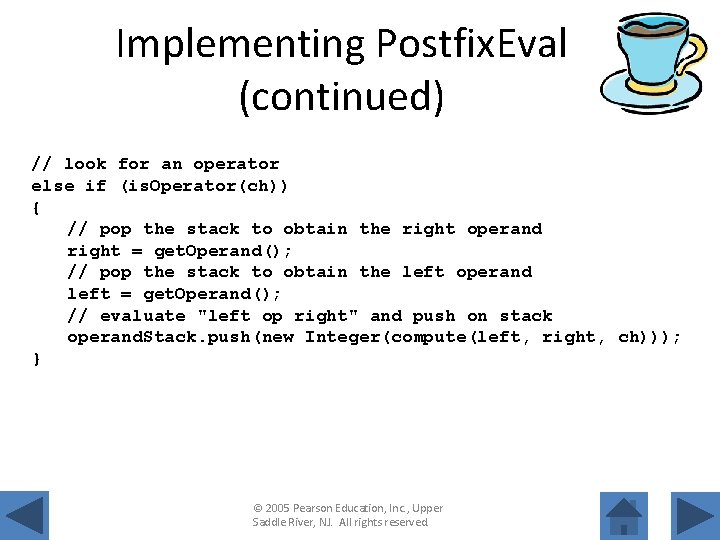
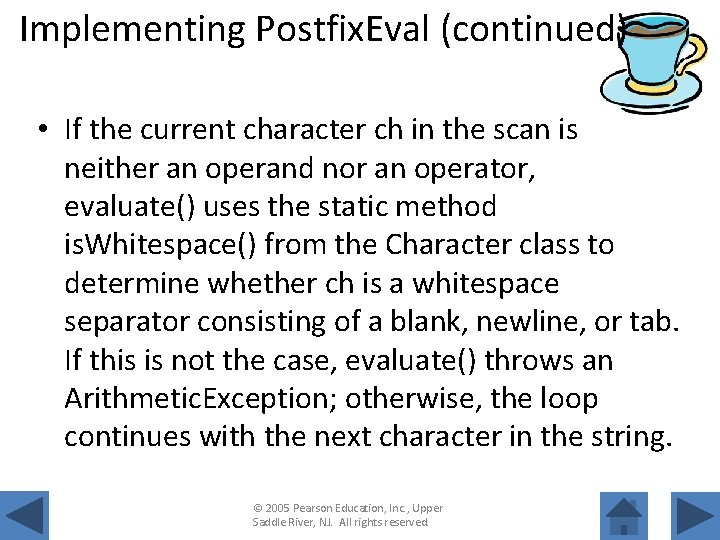
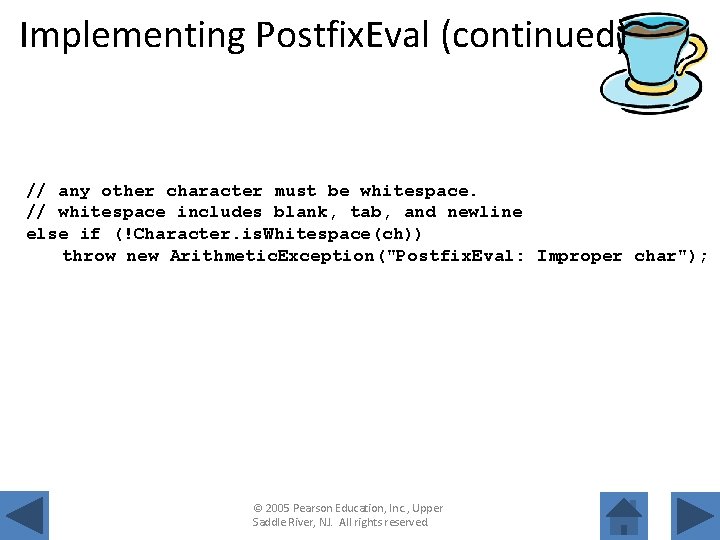
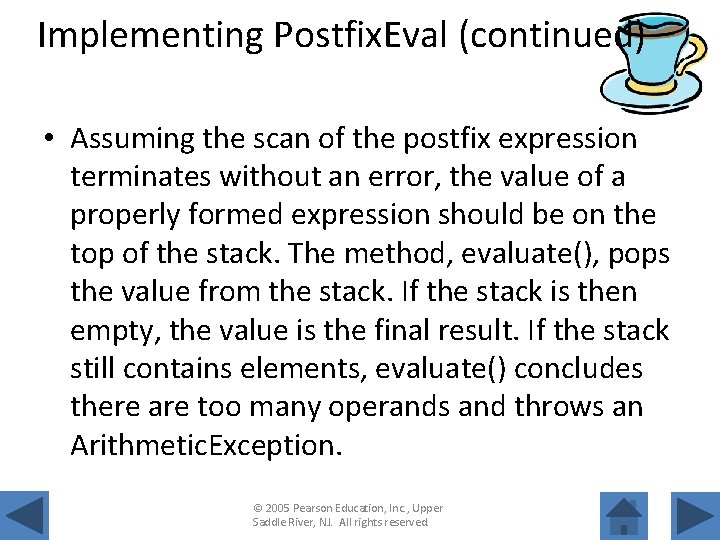
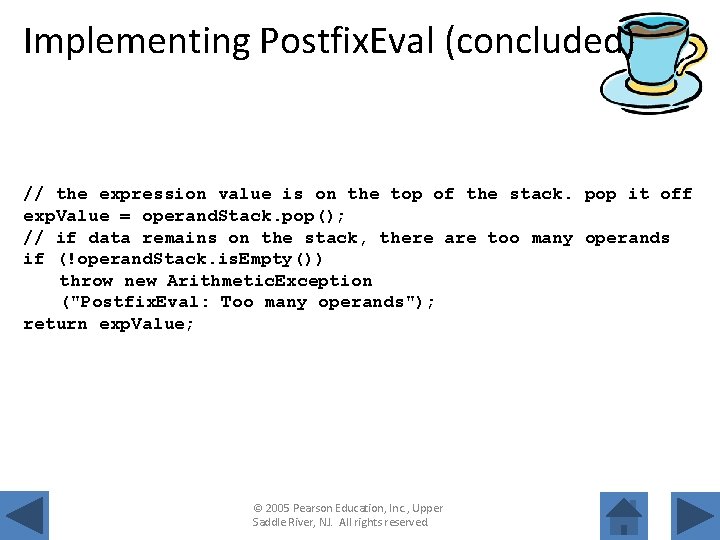
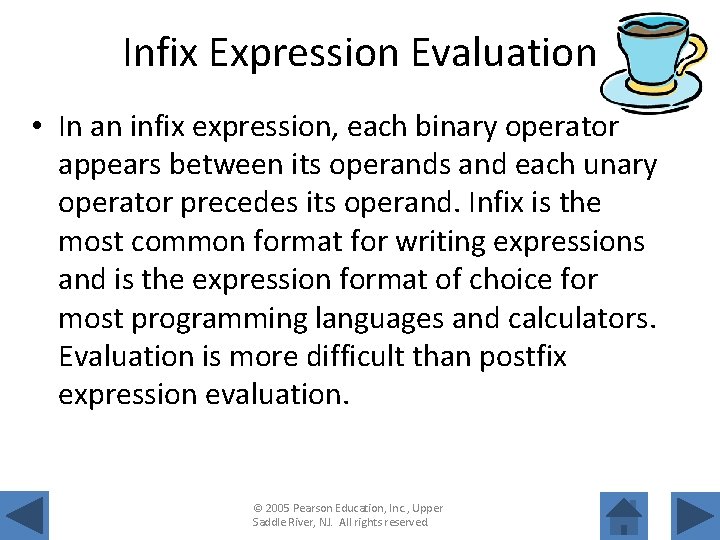
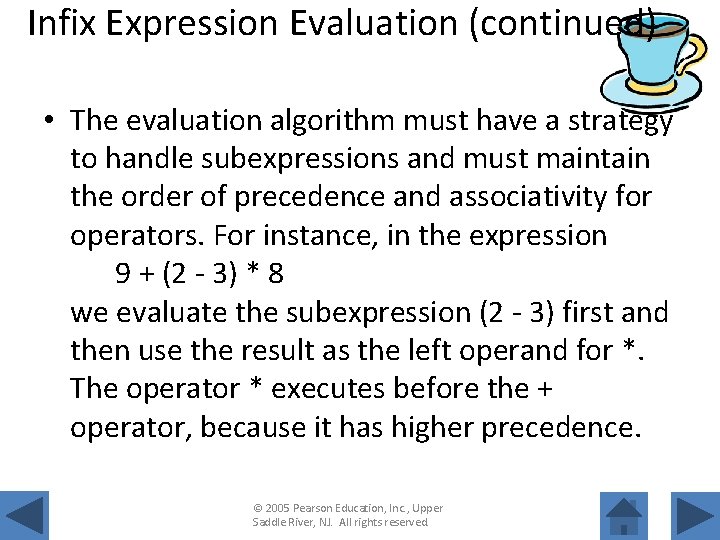
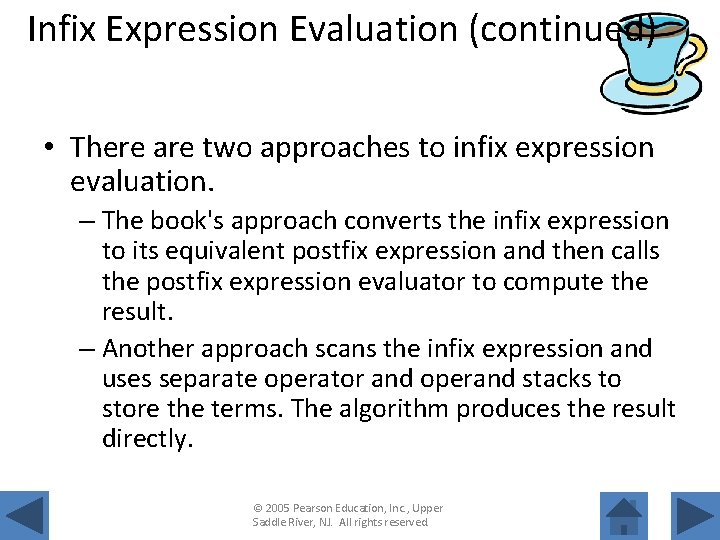
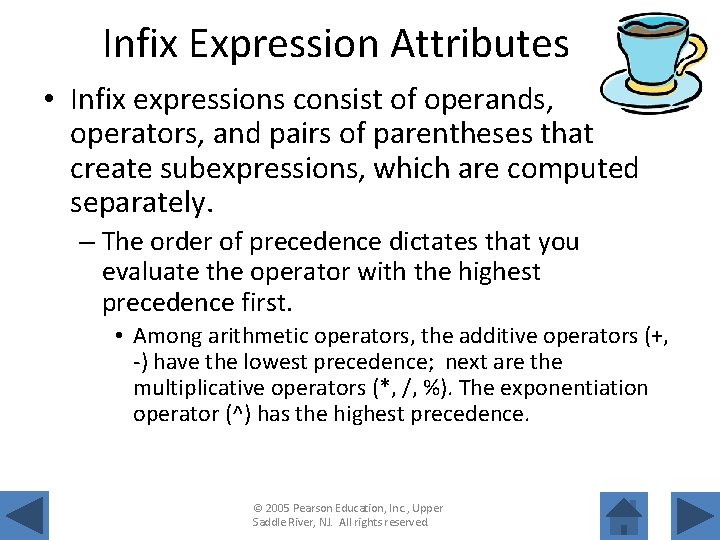
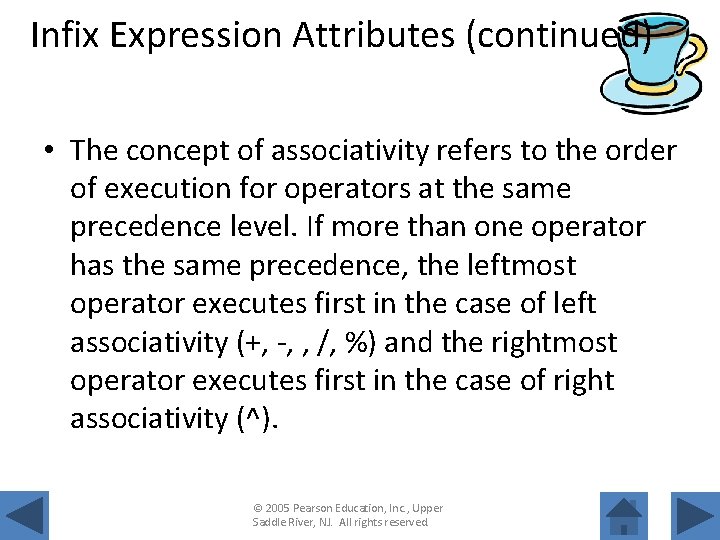
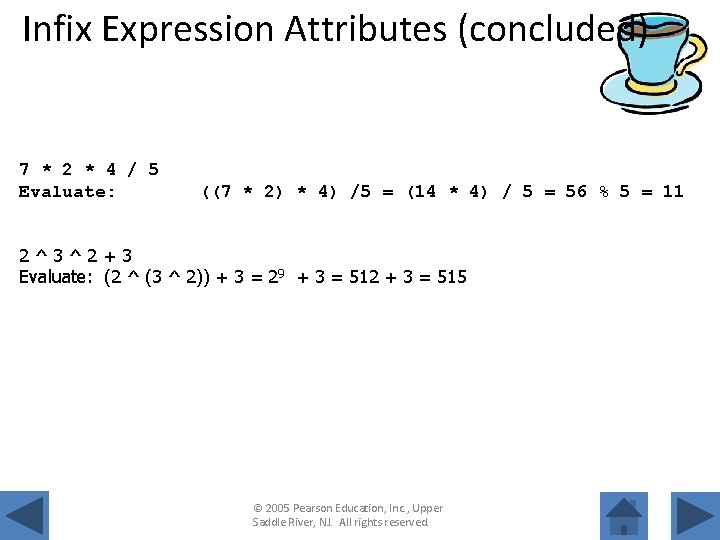
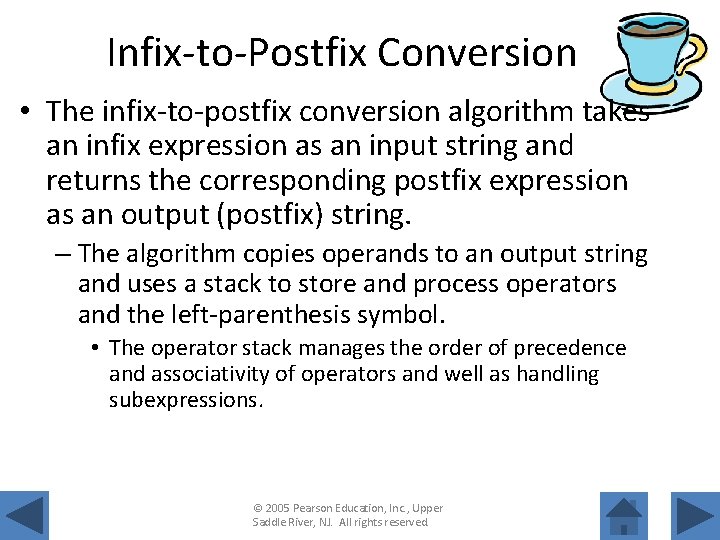
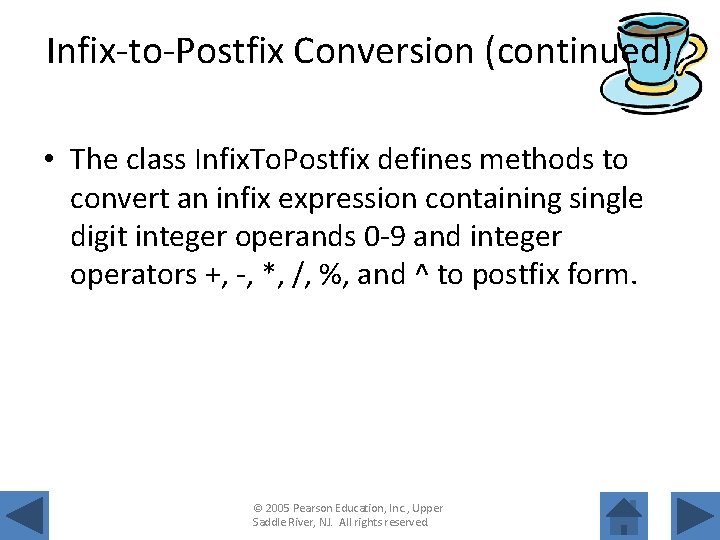
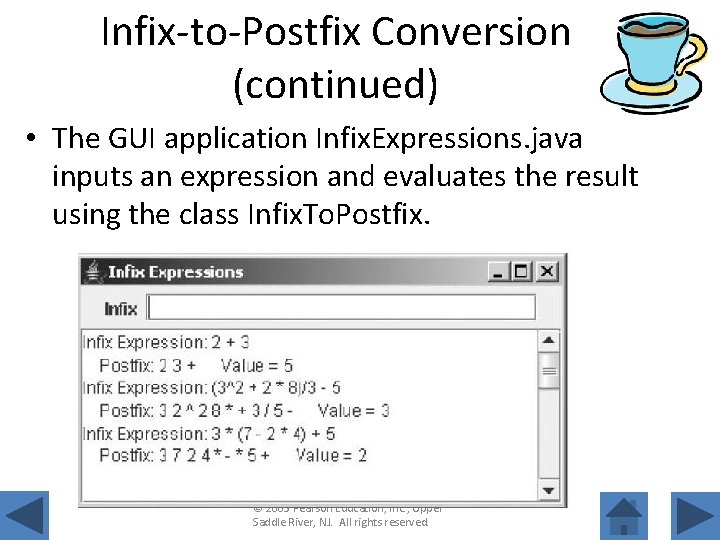
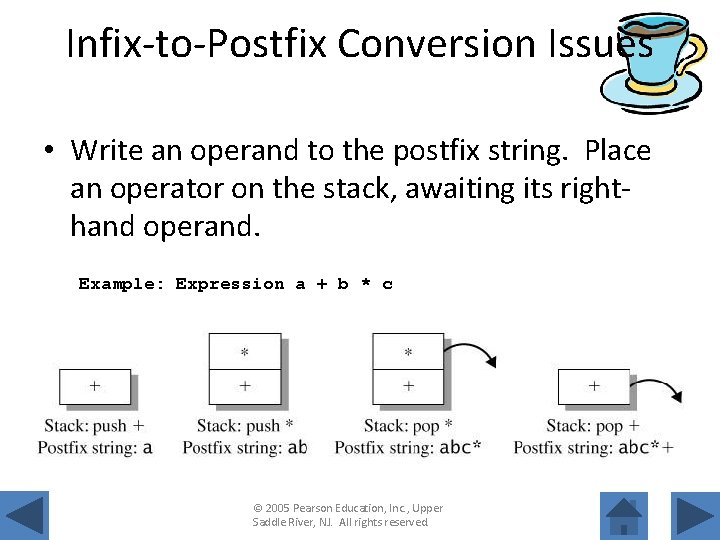
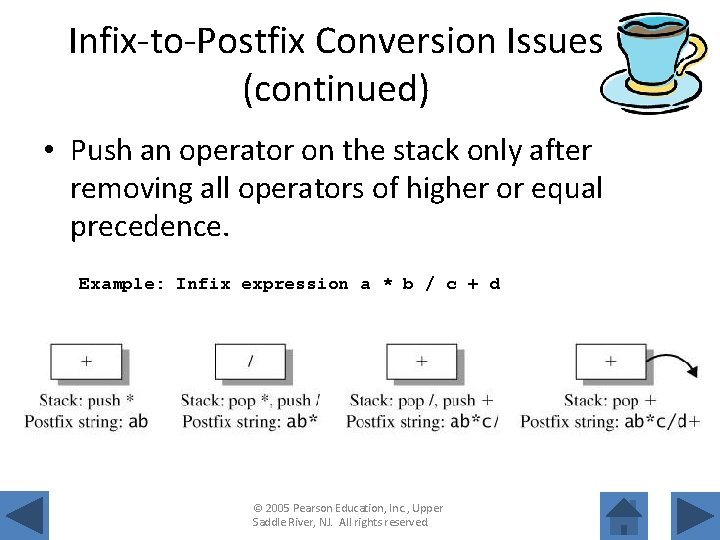
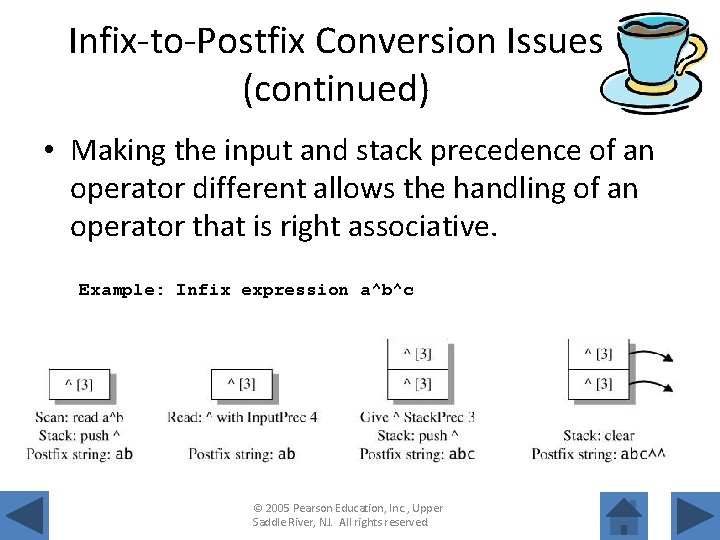
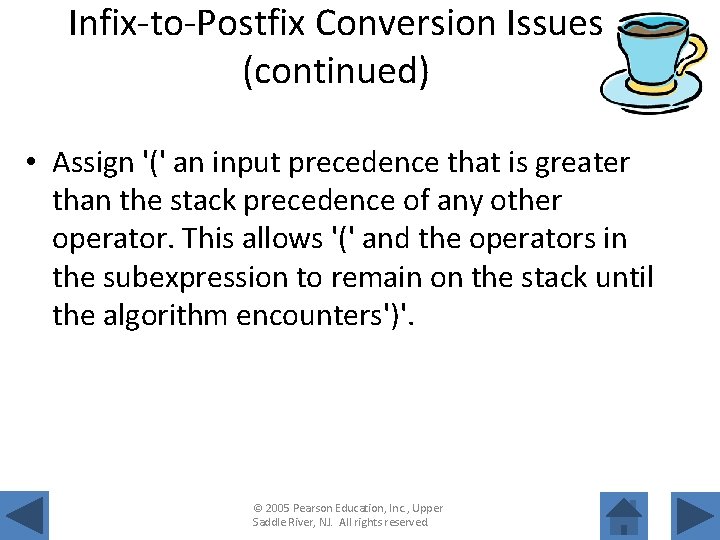
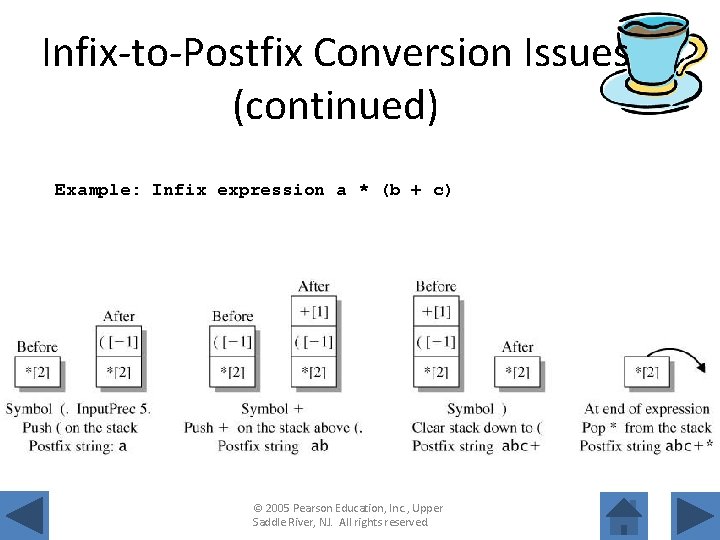
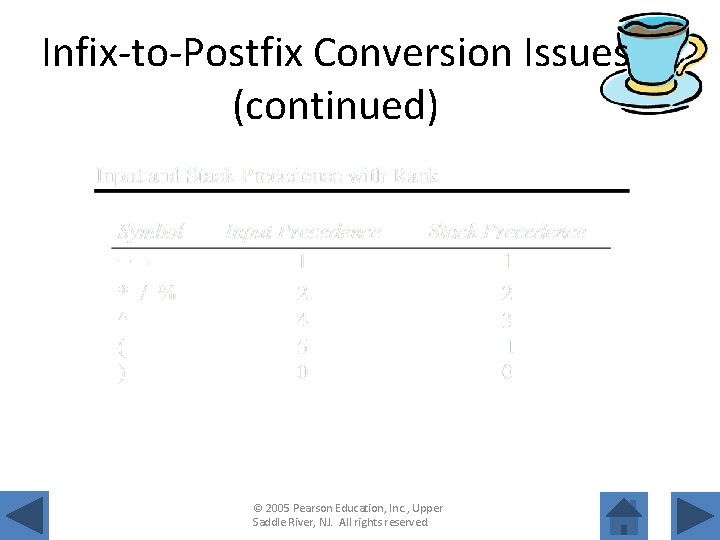
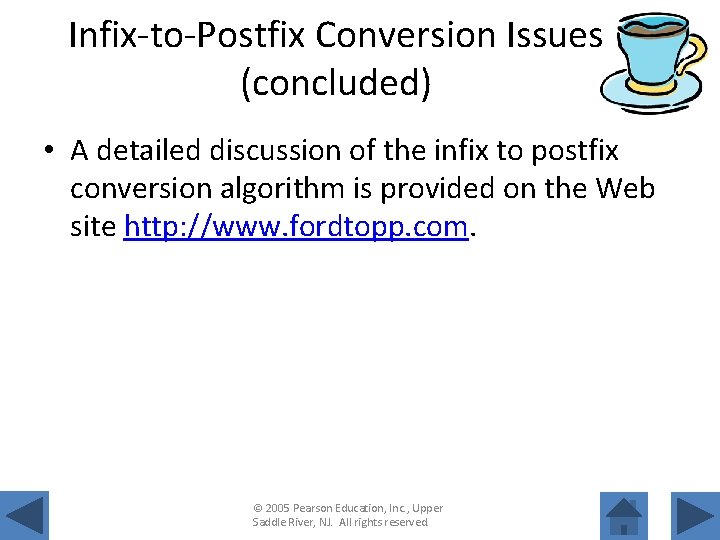
- Slides: 75
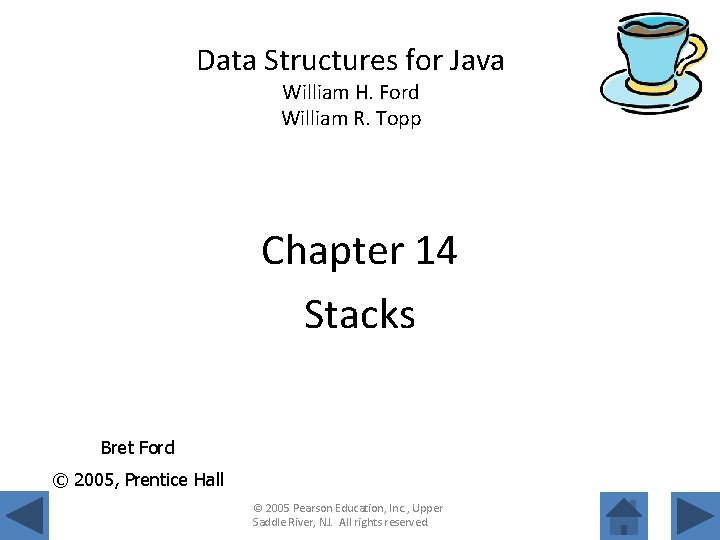
Data Structures for Java William H. Ford William R. Topp Chapter 14 Stacks Bret Ford © 2005, Prentice Hall © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
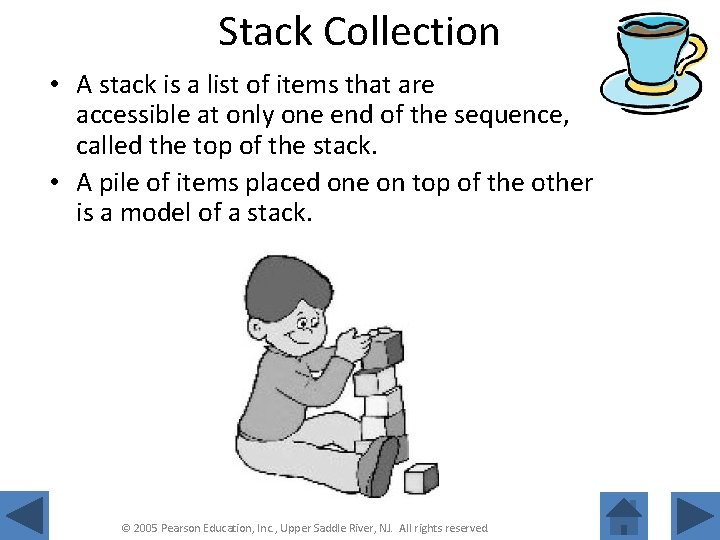
Stack Collection • A stack is a list of items that are accessible at only one end of the sequence, called the top of the stack. • A pile of items placed one on top of the other is a model of a stack. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
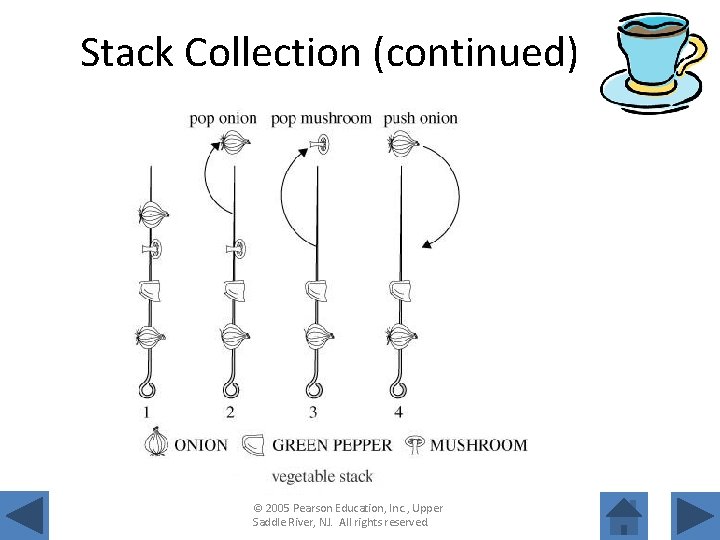
Stack Collection (continued) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
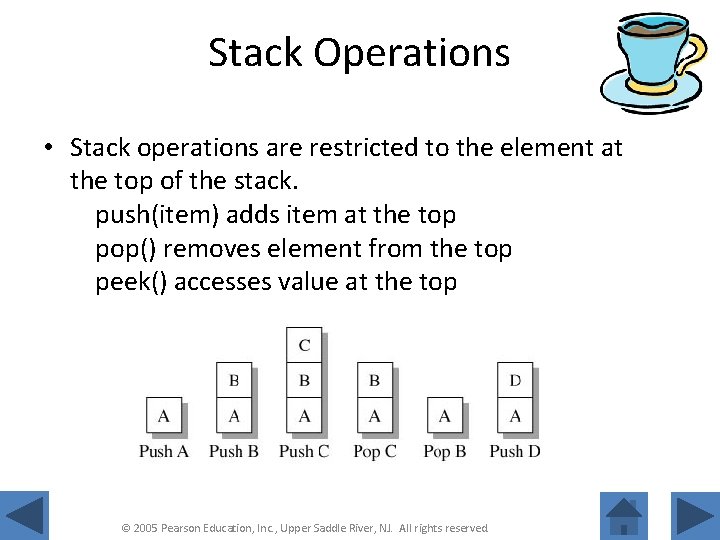
Stack Operations • Stack operations are restricted to the element at the top of the stack. push(item) adds item at the top pop() removes element from the top peek() accesses value at the top © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
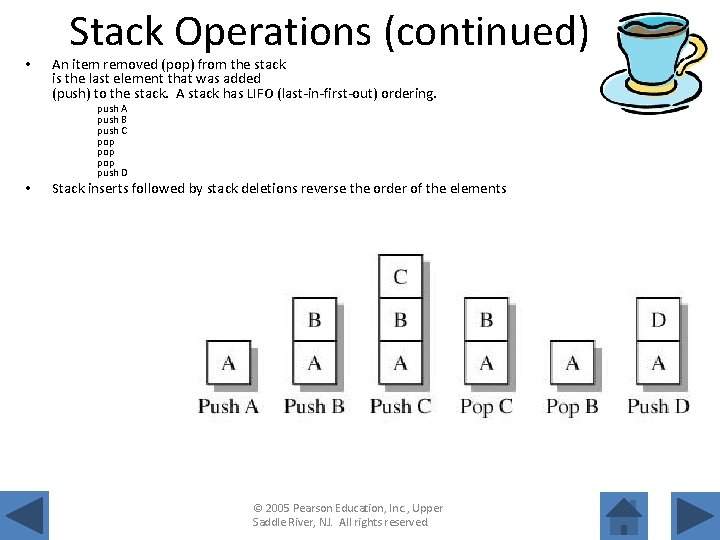
• Stack Operations (continued) An item removed (pop) from the stack is the last element that was added (push) to the stack. A stack has LIFO (last-in-first-out) ordering. push A push B push C pop pop push D • Stack inserts followed by stack deletions reverse the order of the elements © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
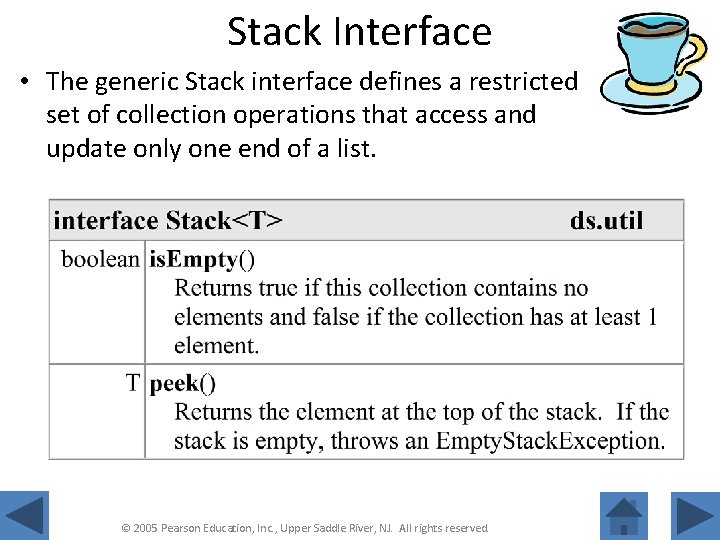
Stack Interface • The generic Stack interface defines a restricted set of collection operations that access and update only one end of a list. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
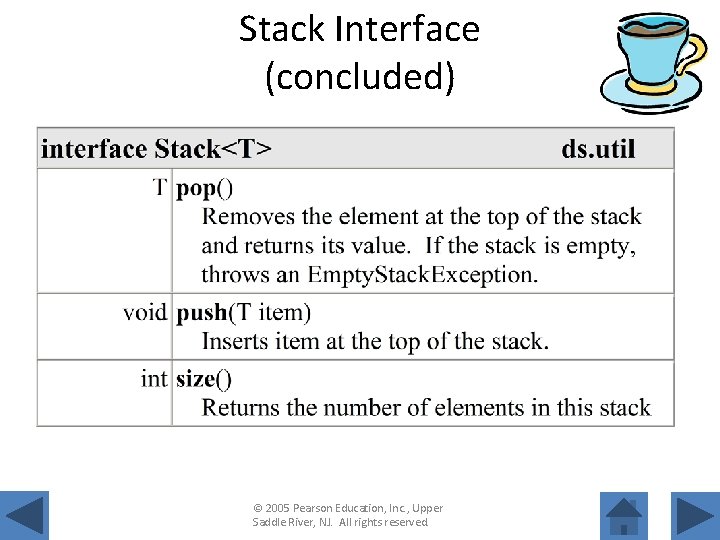
Stack Interface (concluded) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
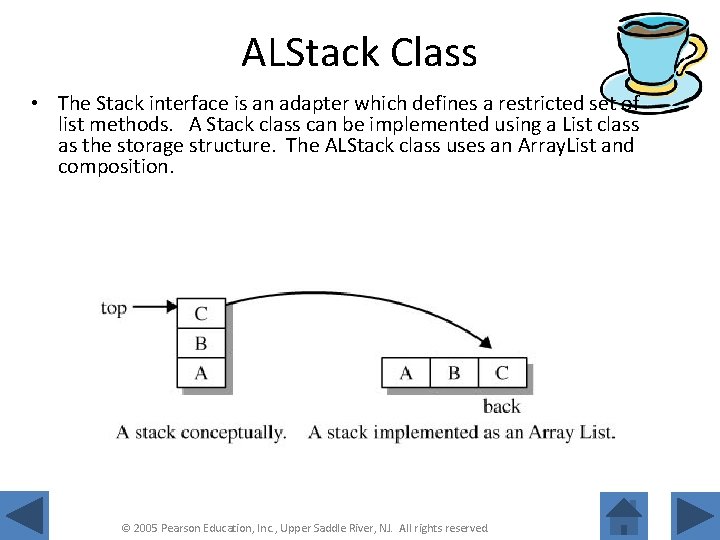
ALStack Class • The Stack interface is an adapter which defines a restricted set of list methods. A Stack class can be implemented using a List class as the storage structure. The ALStack class uses an Array. List and composition. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
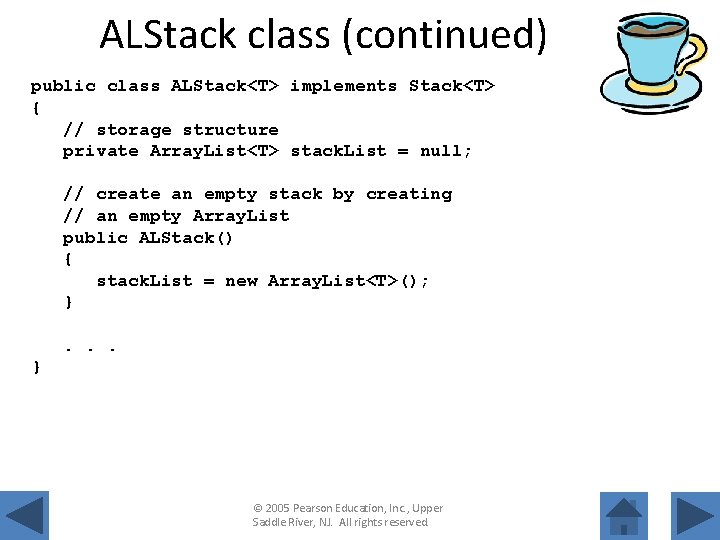
ALStack class (continued) public class ALStack<T> implements Stack<T> { // storage structure private Array. List<T> stack. List = null; // create an empty stack by creating // an empty Array. List public ALStack() { stack. List = new Array. List<T>(); }. . . } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
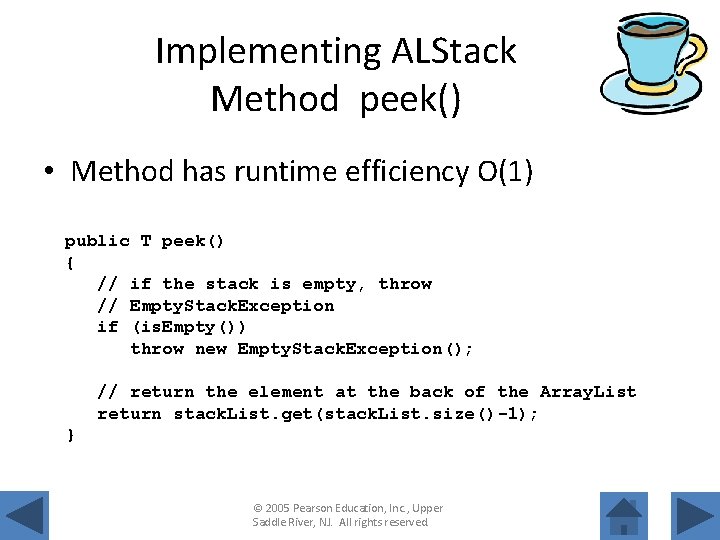
Implementing ALStack Method peek() • Method has runtime efficiency O(1) public T peek() { // if the stack is empty, throw // Empty. Stack. Exception if (is. Empty()) throw new Empty. Stack. Exception(); // return the element at the back of the Array. List return stack. List. get(stack. List. size()-1); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
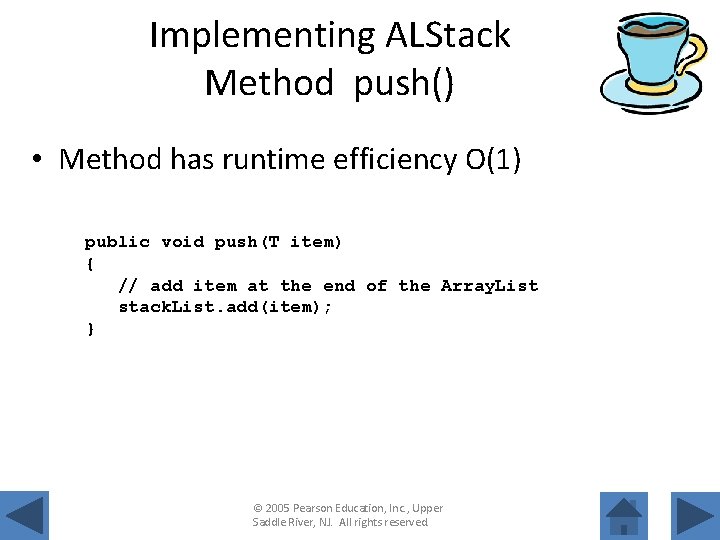
Implementing ALStack Method push() • Method has runtime efficiency O(1) public void push(T item) { // add item at the end of the Array. List stack. List. add(item); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
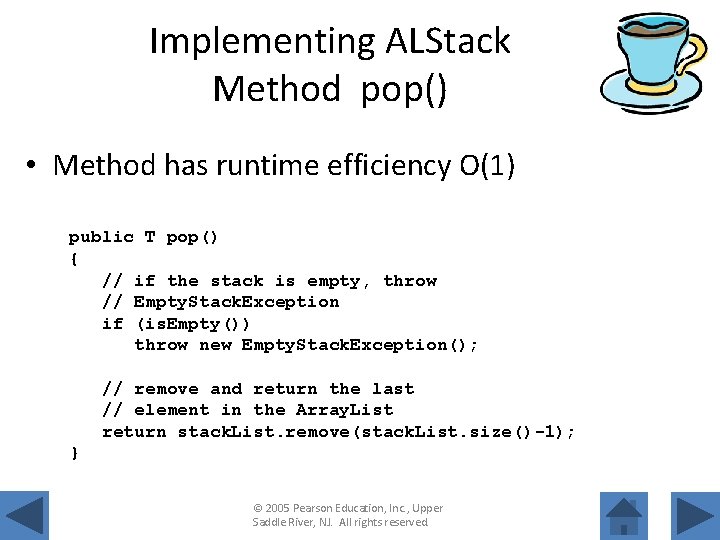
Implementing ALStack Method pop() • Method has runtime efficiency O(1) public T pop() { // if the stack is empty, throw // Empty. Stack. Exception if (is. Empty()) throw new Empty. Stack. Exception(); // remove and return the last // element in the Array. List return stack. List. remove(stack. List. size()-1); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
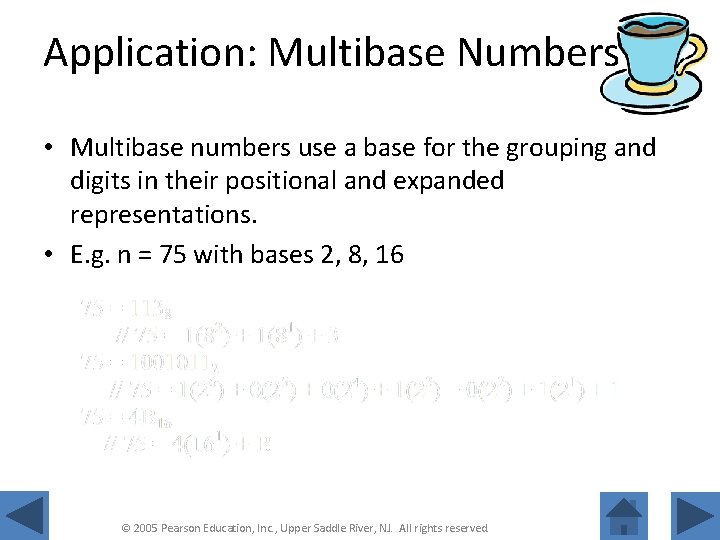
Application: Multibase Numbers • Multibase numbers use a base for the grouping and digits in their positional and expanded representations. • E. g. n = 75 with bases 2, 8, 16 © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
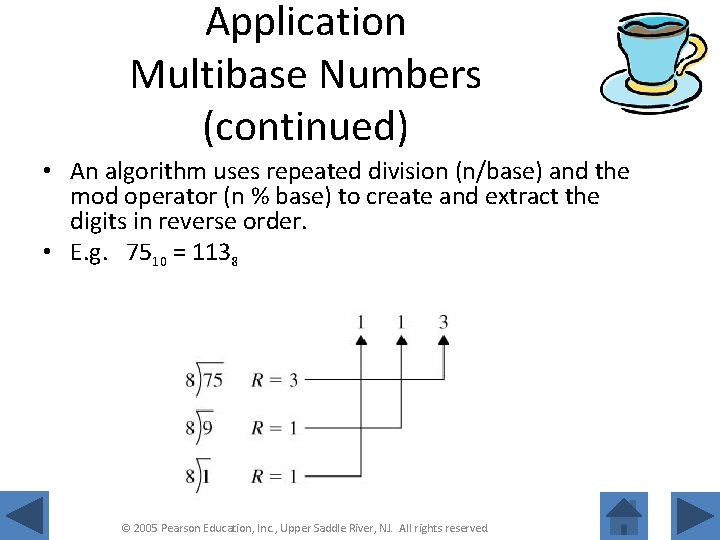
Application Multibase Numbers (continued) • An algorithm uses repeated division (n/base) and the mod operator (n % base) to create and extract the digits in reverse order. • E. g. 7510 = 1138 © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
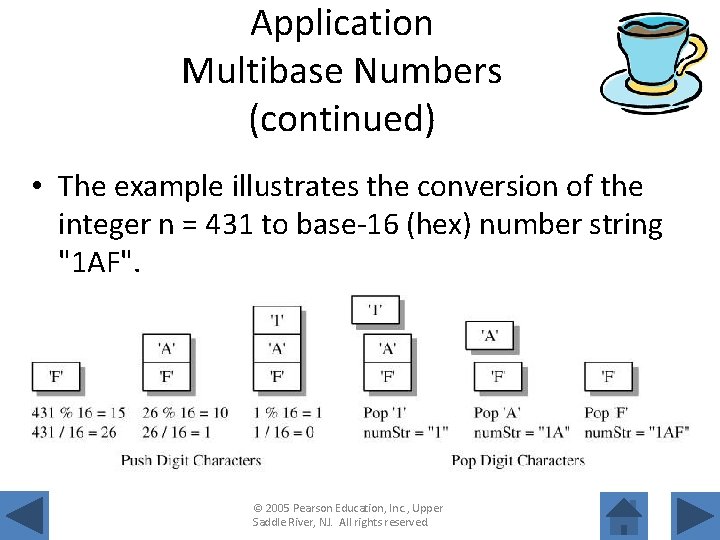
Application Multibase Numbers (continued) • The example illustrates the conversion of the integer n = 431 to base-16 (hex) number string "1 AF". © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
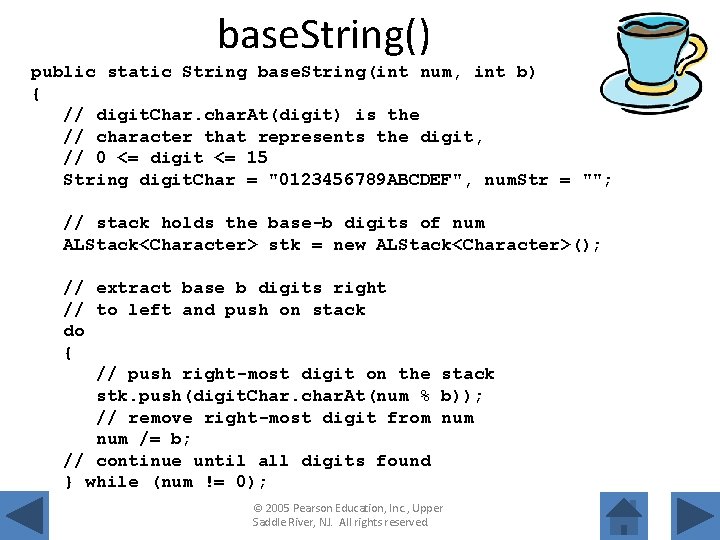
base. String() public static String base. String(int num, int b) { // digit. Char. char. At(digit) is the // character that represents the digit, // 0 <= digit <= 15 String digit. Char = "0123456789 ABCDEF", num. Str = ""; // stack holds the base-b digits of num ALStack<Character> stk = new ALStack<Character>(); // extract base b digits right // to left and push on stack do { // push right-most digit on the stack stk. push(digit. Char. char. At(num % b)); // remove right-most digit from num /= b; // continue until all digits found } while (num != 0); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
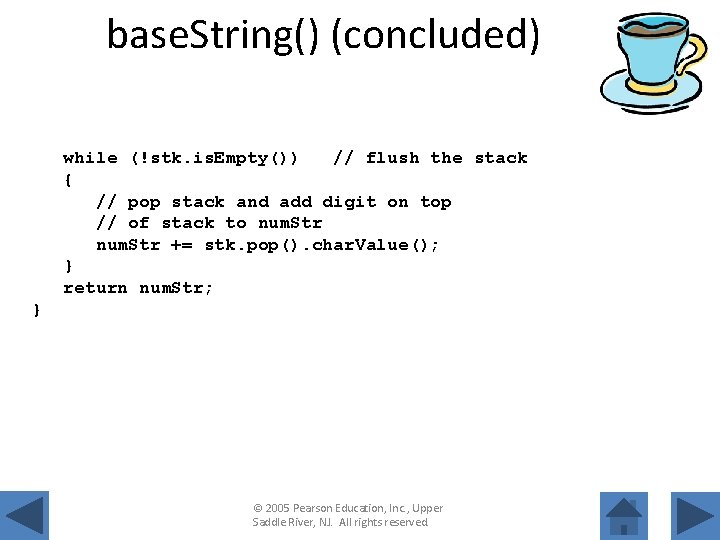
base. String() (concluded) while (!stk. is. Empty()) // flush the stack { // pop stack and add digit on top // of stack to num. Str += stk. pop(). char. Value(); } return num. Str; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
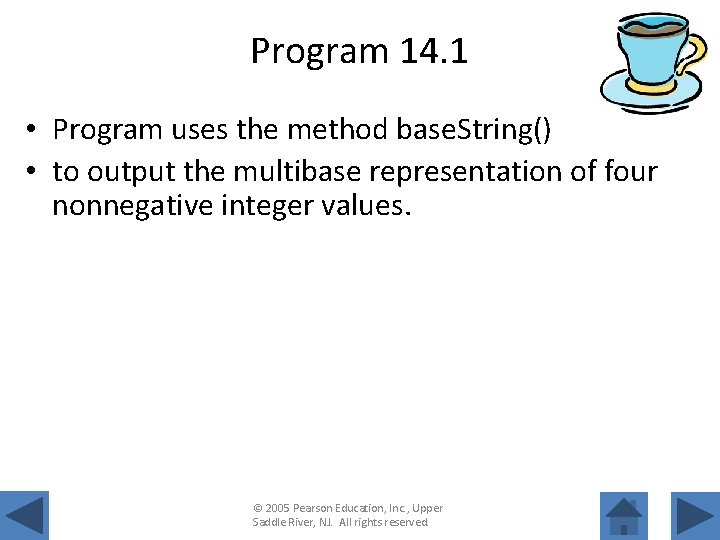
Program 14. 1 • Program uses the method base. String() • to output the multibase representation of four nonnegative integer values. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
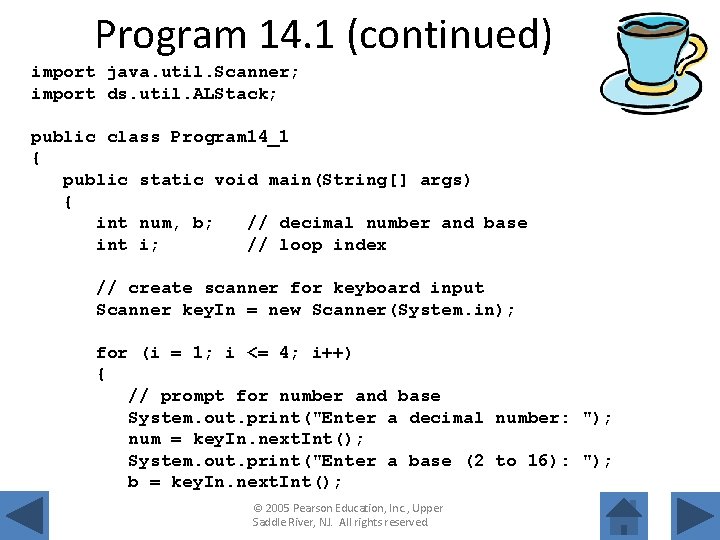
Program 14. 1 (continued) import java. util. Scanner; import ds. util. ALStack; public class Program 14_1 { public static void main(String[] args) { int num, b; // decimal number and base int i; // loop index // create scanner for keyboard input Scanner key. In = new Scanner(System. in); for (i = 1; i <= 4; i++) { // prompt for number and base System. out. print("Enter a decimal number: "); num = key. In. next. Int(); System. out. print("Enter a base (2 to 16): "); b = key. In. next. Int(); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
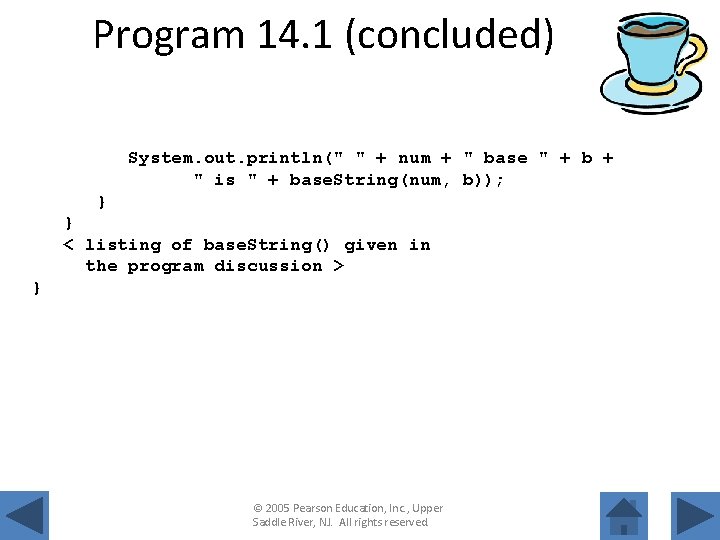
Program 14. 1 (concluded) System. out. println(" " + num + " base " + b + " is " + base. String(num, b)); } } < listing of base. String() given in the program discussion > } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
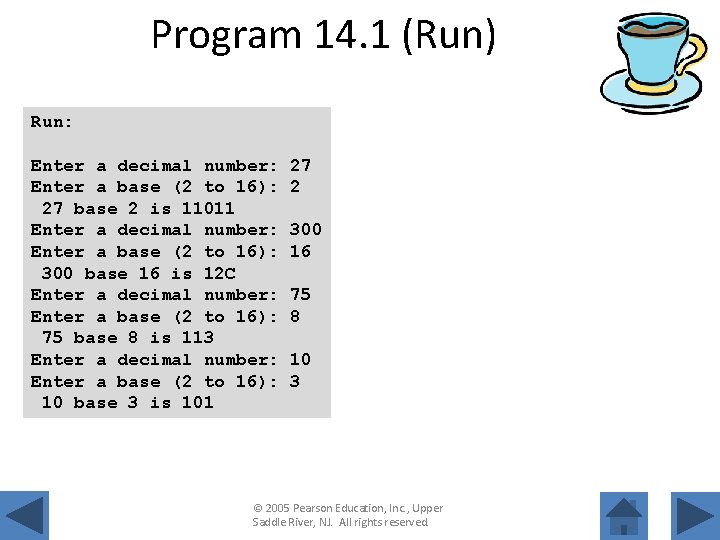
Program 14. 1 (Run) Run: Enter a decimal number: Enter a base (2 to 16): 27 base 2 is 11011 Enter a decimal number: Enter a base (2 to 16): 300 base 16 is 12 C Enter a decimal number: Enter a base (2 to 16): 75 base 8 is 113 Enter a decimal number: Enter a base (2 to 16): 10 base 3 is 101 27 2 300 16 75 8 10 3 © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
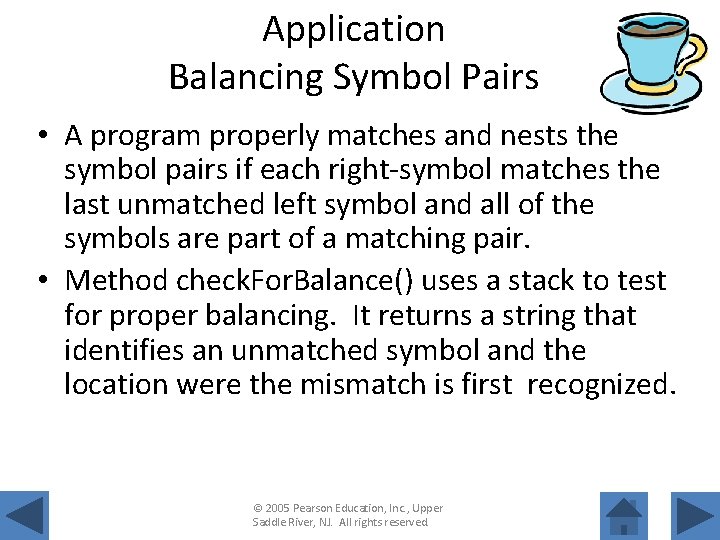
Application Balancing Symbol Pairs • A program properly matches and nests the symbol pairs if each right-symbol matches the last unmatched left symbol and all of the symbols are part of a matching pair. • Method check. For. Balance() uses a stack to test for proper balancing. It returns a string that identifies an unmatched symbol and the location were the mismatch is first recognized. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
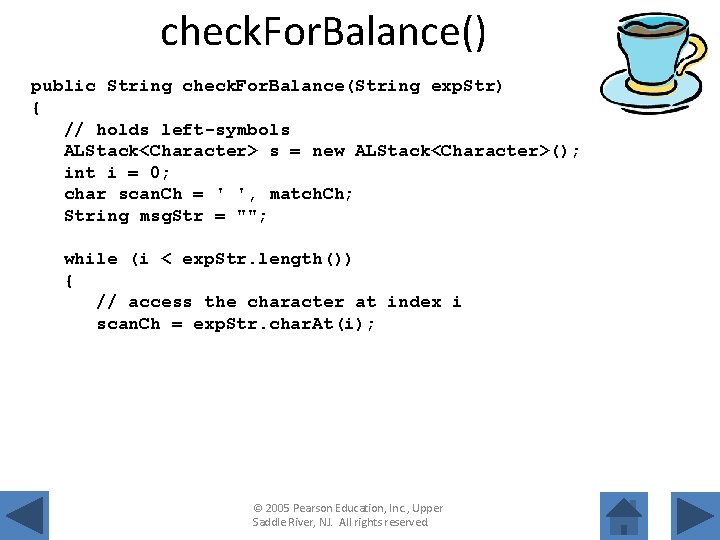
check. For. Balance() public String check. For. Balance(String exp. Str) { // holds left-symbols ALStack<Character> s = new ALStack<Character>(); int i = 0; char scan. Ch = ' ', match. Ch; String msg. Str = ""; while (i < exp. Str. length()) { // access the character at index i scan. Ch = exp. Str. char. At(i); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
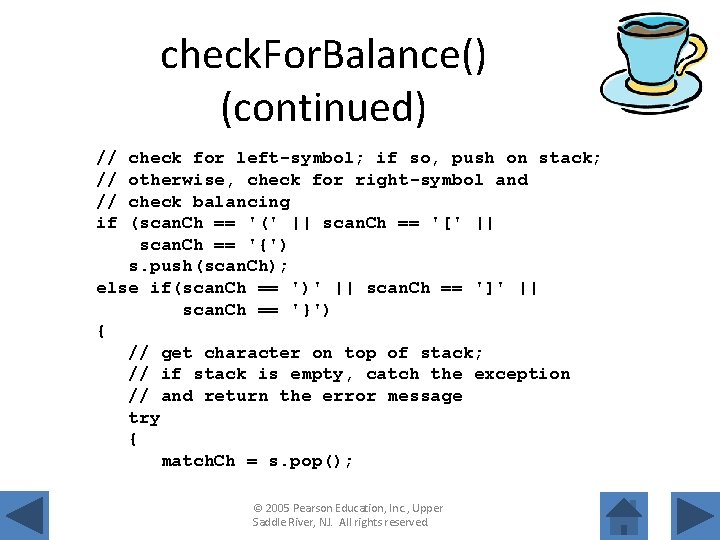
check. For. Balance() (continued) // // // if check for left-symbol; if so, push on stack; otherwise, check for right-symbol and check balancing (scan. Ch == '(' || scan. Ch == '[' || scan. Ch == '{') s. push(scan. Ch); else if(scan. Ch == ')' || scan. Ch == ']' || scan. Ch == '}') { // get character on top of stack; // if stack is empty, catch the exception // and return the error message try { match. Ch = s. pop(); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
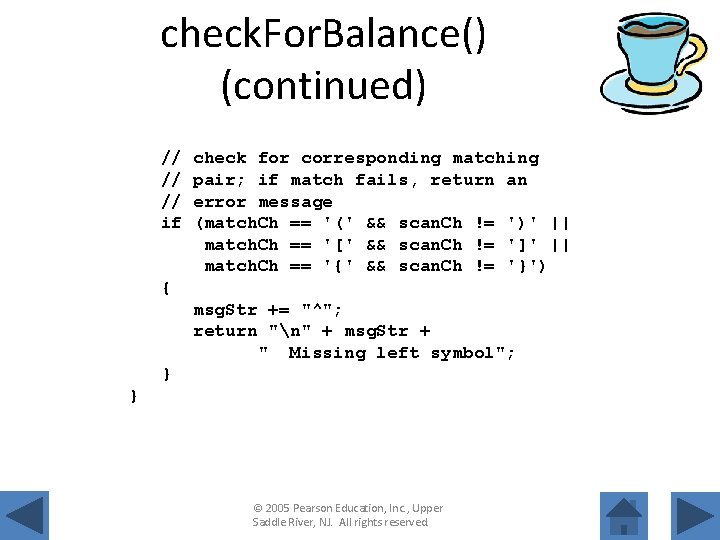
check. For. Balance() (continued) // // // if check for corresponding matching pair; if match fails, return an error message (match. Ch == '(' && scan. Ch != ')' || match. Ch == '[' && scan. Ch != ']' || match. Ch == '{' && scan. Ch != '}') { msg. Str += "^"; return "n" + msg. Str + " Missing left symbol"; } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
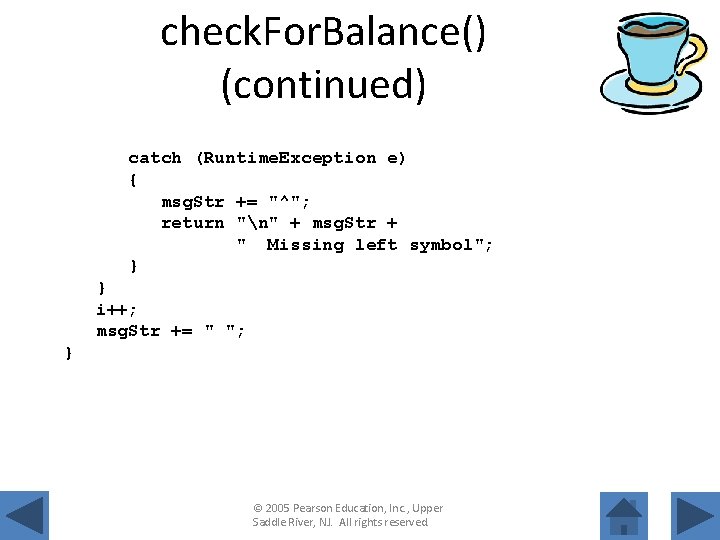
check. For. Balance() (continued) catch (Runtime. Exception e) { msg. Str += "^"; return "n" + msg. Str + " Missing left symbol"; } } i++; msg. Str += " "; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
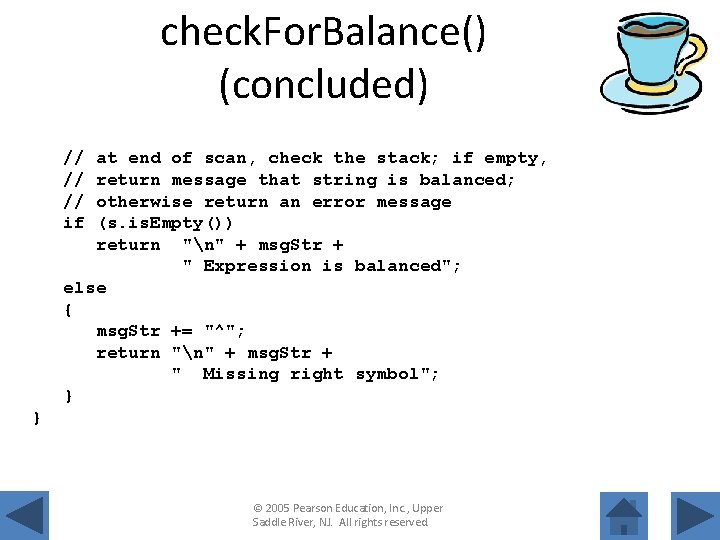
check. For. Balance() (concluded) // // // if at end of scan, check the stack; if empty, return message that string is balanced; otherwise return an error message (s. is. Empty()) return "n" + msg. Str + " Expression is balanced"; else { msg. Str += "^"; return "n" + msg. Str + " Missing right symbol"; } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
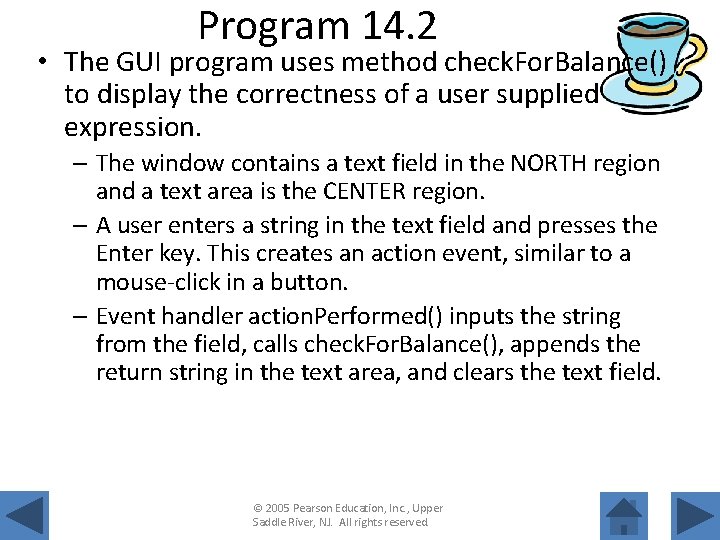
Program 14. 2 • The GUI program uses method check. For. Balance() to display the correctness of a user supplied expression. – The window contains a text field in the NORTH region and a text area is the CENTER region. – A user enters a string in the text field and presses the Enter key. This creates an action event, similar to a mouse-click in a button. – Event handler action. Performed() inputs the string from the field, calls check. For. Balance(), appends the return string in the text area, and clears the text field. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
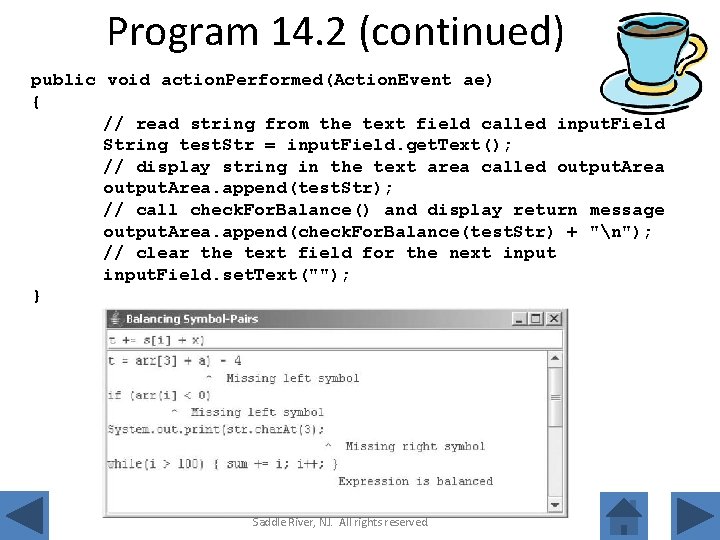
Program 14. 2 (continued) public void action. Performed(Action. Event ae) { // read string from the text field called input. Field String test. Str = input. Field. get. Text(); // display string in the text area called output. Area. append(test. Str); // call check. For. Balance() and display return message output. Area. append(check. For. Balance(test. Str) + "n"); // clear the text field for the next input. Field. set. Text(""); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
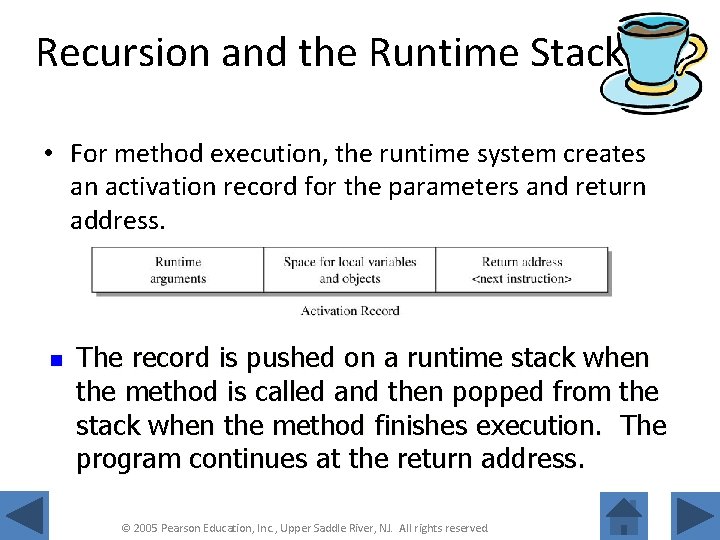
Recursion and the Runtime Stack • For method execution, the runtime system creates an activation record for the parameters and return address. n The record is pushed on a runtime stack when the method is called and then popped from the stack when the method finishes execution. The program continues at the return address. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
![Example fact Calling fact in main public static void mainString args int Example: fact() • Calling fact() in main: public static void main(String[] args) { int](https://slidetodoc.com/presentation_image_h2/630ba2d0ab6d894c07c2af3124647f24/image-31.jpg)
Example: fact() • Calling fact() in main: public static void main(String[] args) { int fact. Value; . . . fact. Value = fact(3); System. out. println("Value fact(3) = "+fact. Value); } n Recursive call in fact(): public static int fact(int n) { if (n == 0) return 1; else return n * fact(n-1); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
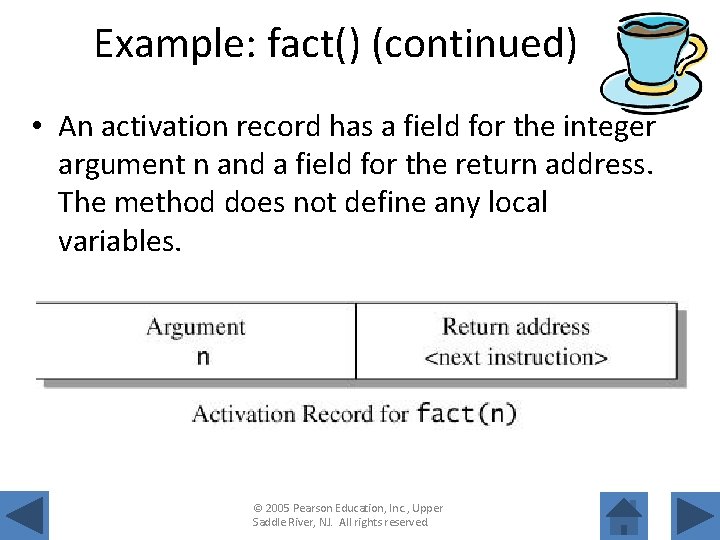
Example: fact() (continued) • An activation record has a field for the integer argument n and a field for the return address. The method does not define any local variables. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
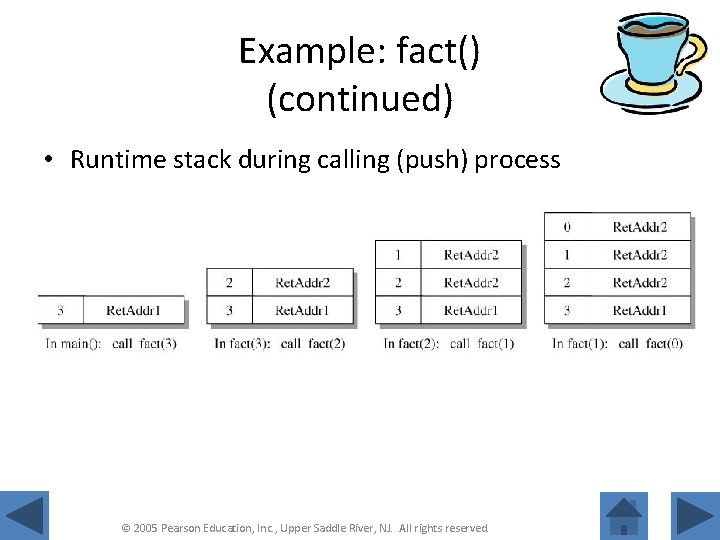
Example: fact() (continued) • Runtime stack during calling (push) process © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
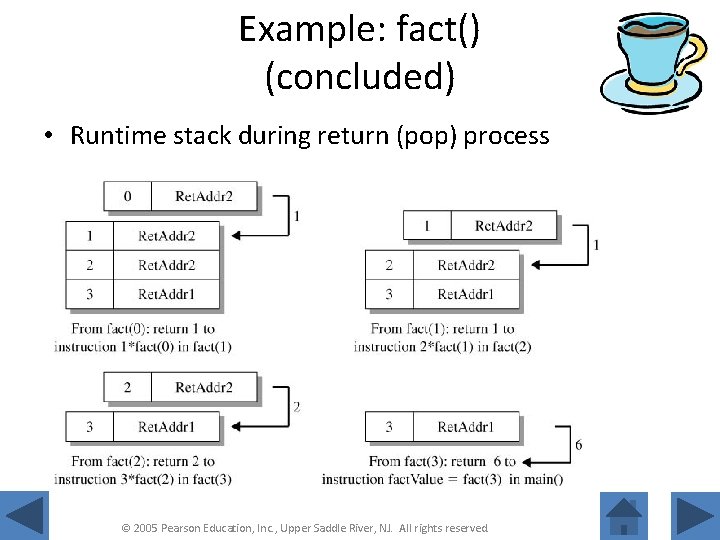
Example: fact() (concluded) • Runtime stack during return (pop) process © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
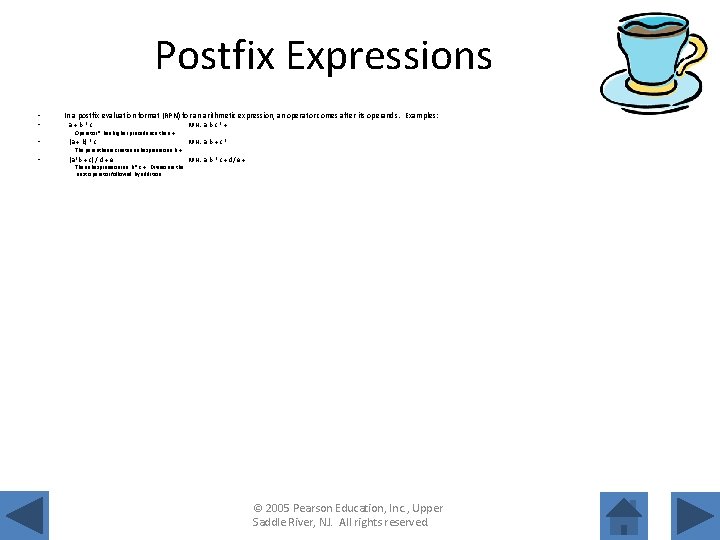
Postfix Expressions • • In a postfix evaluation format (RPN) for an arithmetic expression, an operator comes after its operands. Examples: a+b*c RPN: a b c * + Operator * has higher precedence than +. • (a + b) * c RPN: a b + c * The parenthesis creates subexpression a b + • (a*b + c) / d + e RPN: a b * c + d/ e + The subexpression is a b * c +. Division is the next operator followed by addition. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
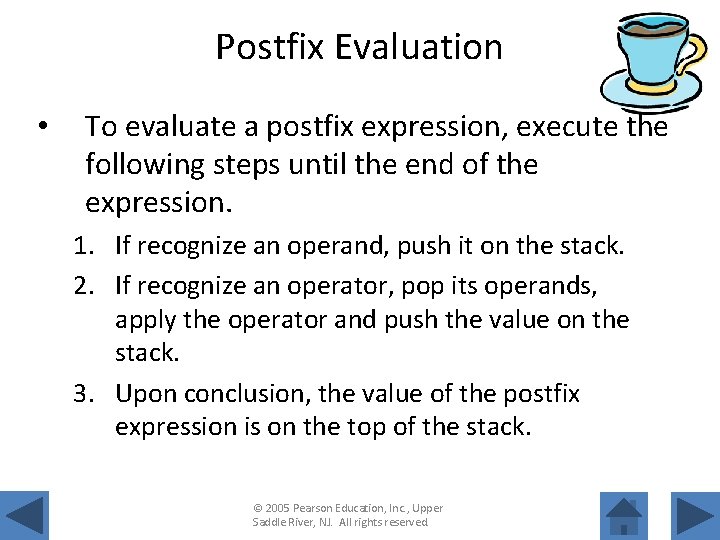
Postfix Evaluation • To evaluate a postfix expression, execute the following steps until the end of the expression. 1. If recognize an operand, push it on the stack. 2. If recognize an operator, pop its operands, apply the operator and push the value on the stack. 3. Upon conclusion, the value of the postfix expression is on the top of the stack. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
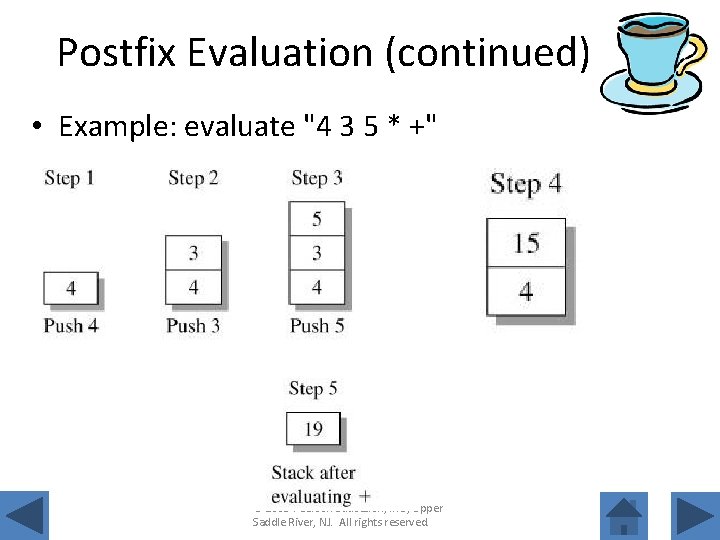
Postfix Evaluation (continued) • Example: evaluate "4 3 5 * +" © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
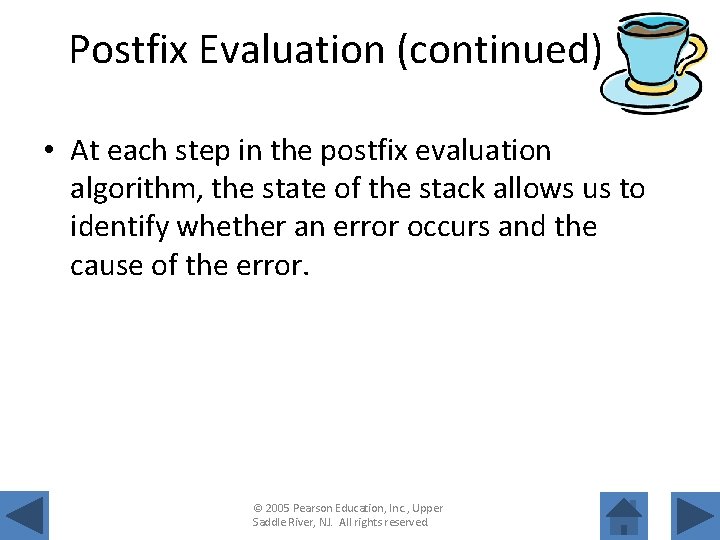
Postfix Evaluation (continued) • At each step in the postfix evaluation algorithm, the state of the stack allows us to identify whether an error occurs and the cause of the error. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
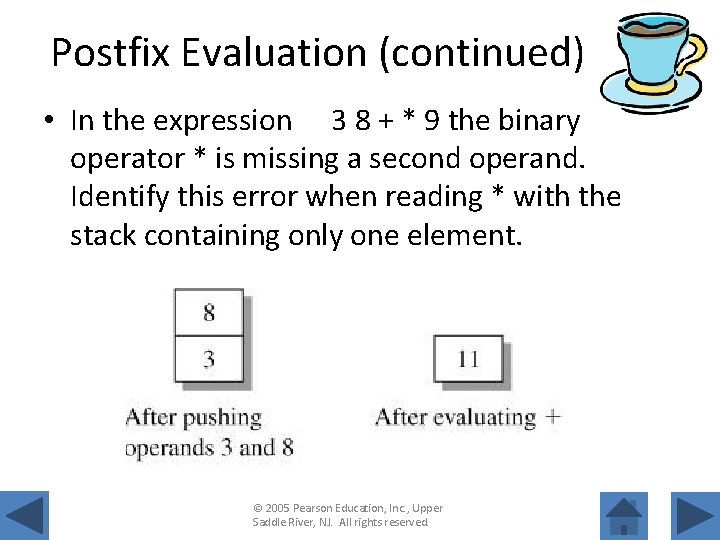
Postfix Evaluation (continued) • In the expression 3 8 + * 9 the binary operator * is missing a second operand. Identify this error when reading * with the stack containing only one element. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
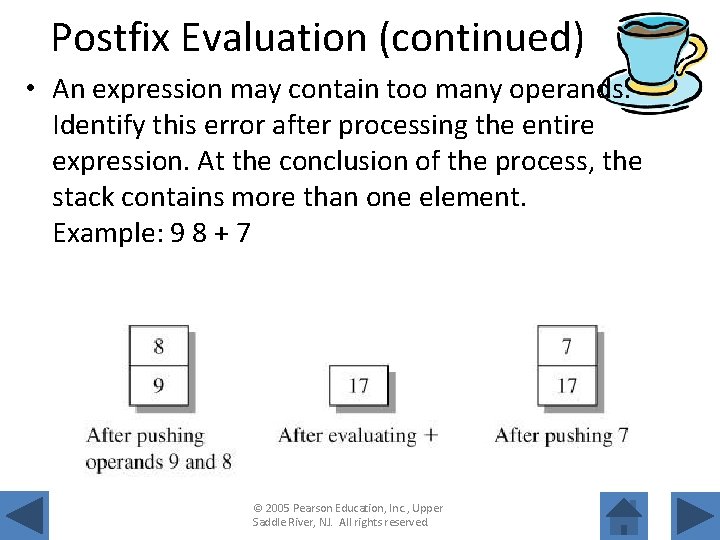
Postfix Evaluation (continued) • An expression may contain too many operands. Identify this error after processing the entire expression. At the conclusion of the process, the stack contains more than one element. Example: 9 8 + 7 © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
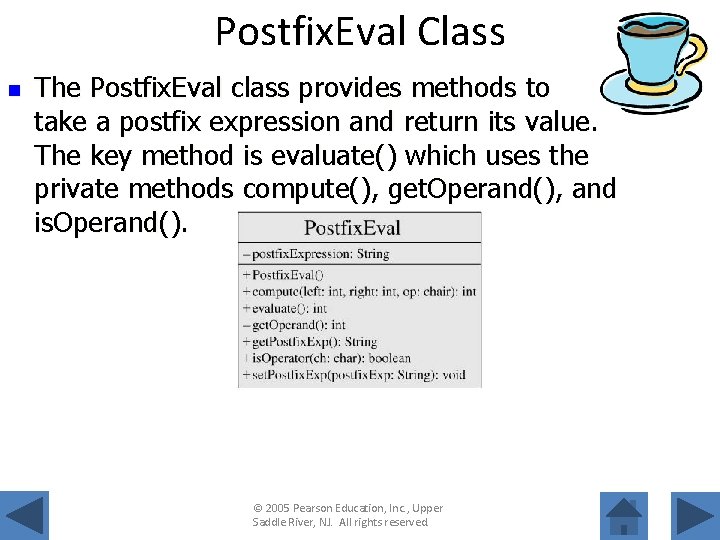
Postfix. Eval Class n The Postfix. Eval class provides methods to take a postfix expression and return its value. The key method is evaluate() which uses the private methods compute(), get. Operand(), and is. Operand(). © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
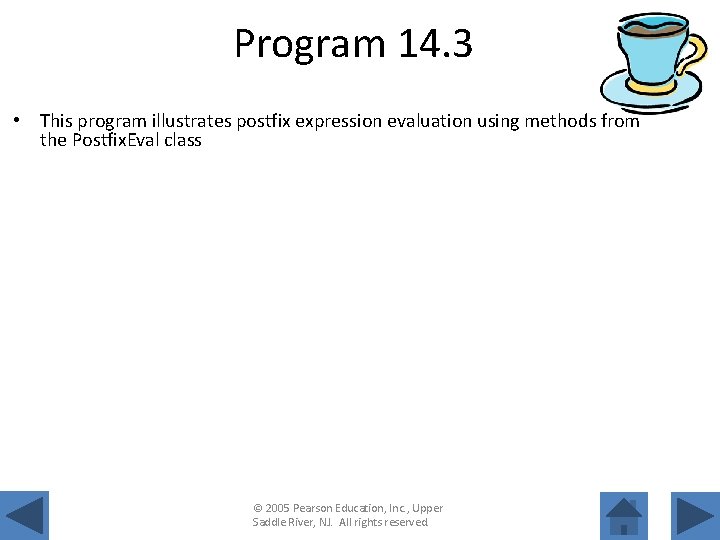
Program 14. 3 • This program illustrates postfix expression evaluation using methods from the Postfix. Eval class © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
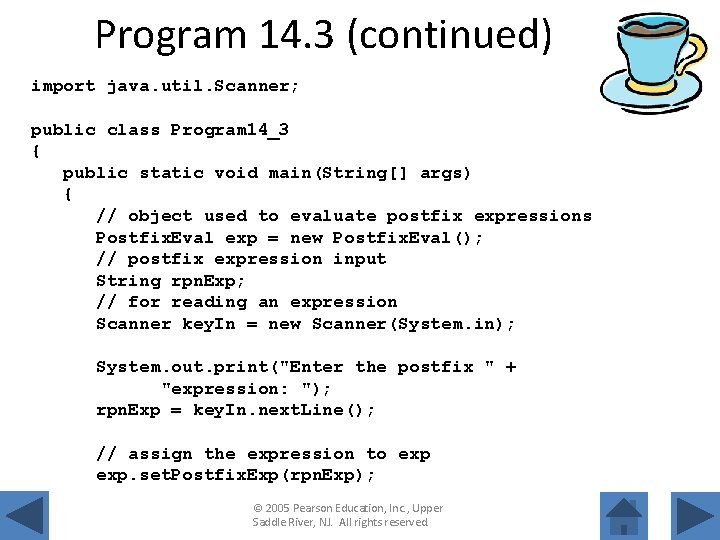
Program 14. 3 (continued) import java. util. Scanner; public class Program 14_3 { public static void main(String[] args) { // object used to evaluate postfix expressions Postfix. Eval exp = new Postfix. Eval(); // postfix expression input String rpn. Exp; // for reading an expression Scanner key. In = new Scanner(System. in); System. out. print("Enter the postfix " + "expression: "); rpn. Exp = key. In. next. Line(); // assign the expression to exp. set. Postfix. Exp(rpn. Exp); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
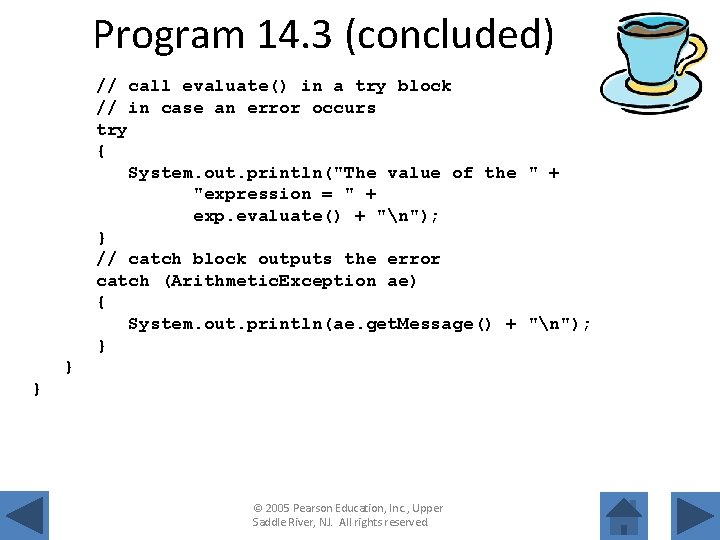
Program 14. 3 (concluded) // call evaluate() in a try block // in case an error occurs try { System. out. println("The value of the " + "expression = " + exp. evaluate() + "n"); } // catch block outputs the error catch (Arithmetic. Exception ae) { System. out. println(ae. get. Message() + "n"); } } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
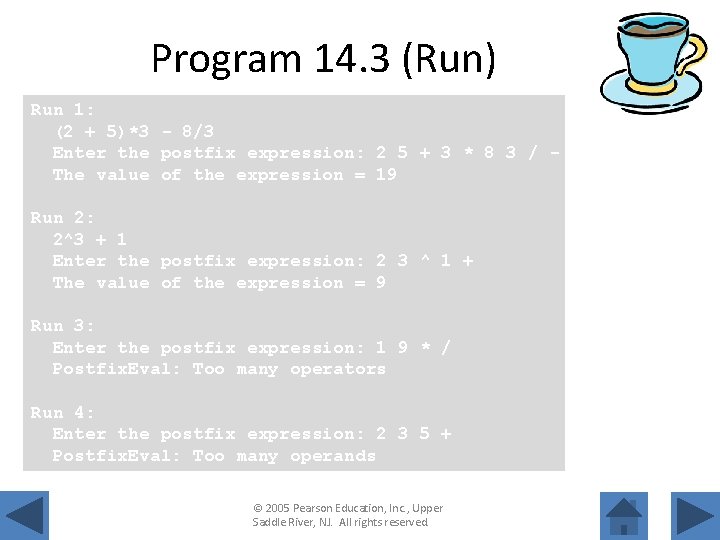
Program 14. 3 (Run) Run 1: (2 + 5)*3 - 8/3 Enter the postfix expression: 2 5 + 3 * 8 3 / The value of the expression = 19 Run 2: 2^3 + 1 Enter the postfix expression: 2 3 ^ 1 + The value of the expression = 9 Run 3: Enter the postfix expression: 1 9 * / Postfix. Eval: Too many operators Run 4: Enter the postfix expression: 2 3 5 + Postfix. Eval: Too many operands © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
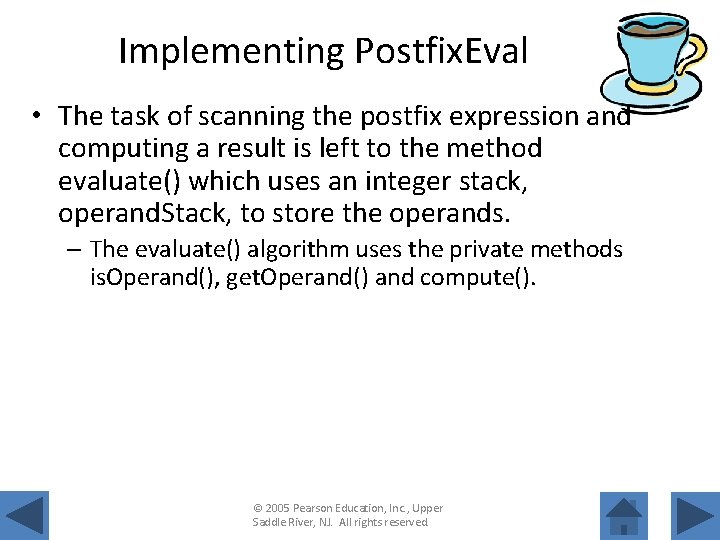
Implementing Postfix. Eval • The task of scanning the postfix expression and computing a result is left to the method evaluate() which uses an integer stack, operand. Stack, to store the operands. – The evaluate() algorithm uses the private methods is. Operand(), get. Operand() and compute(). © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
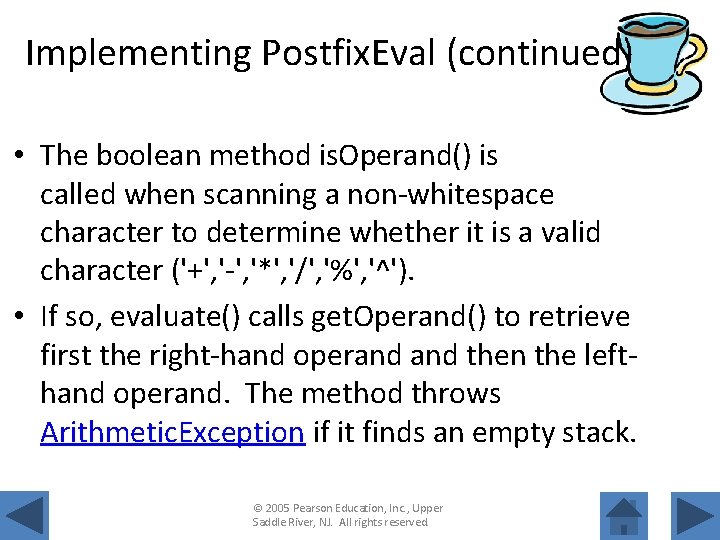
Implementing Postfix. Eval (continued) • The boolean method is. Operand() is called when scanning a non-whitespace character to determine whether it is a valid character ('+', '-', '*', '/', '%', '^'). • If so, evaluate() calls get. Operand() to retrieve first the right-hand operand then the lefthand operand. The method throws Arithmetic. Exception if it finds an empty stack. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
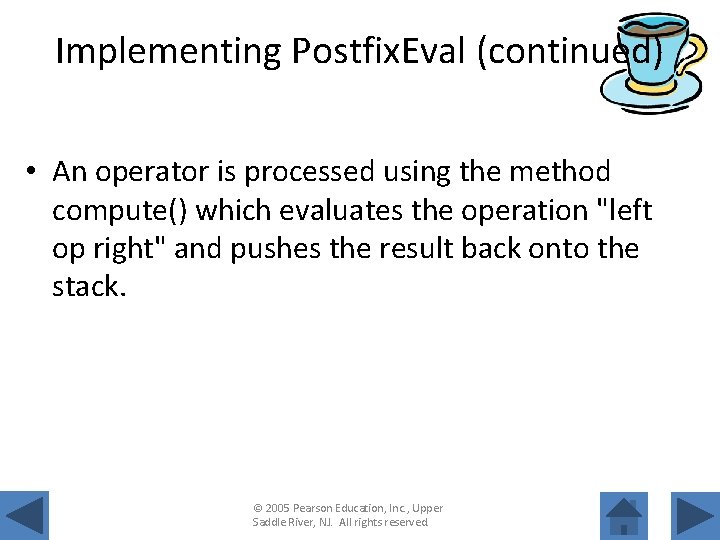
Implementing Postfix. Eval (continued) • An operator is processed using the method compute() which evaluates the operation "left op right" and pushes the result back onto the stack. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
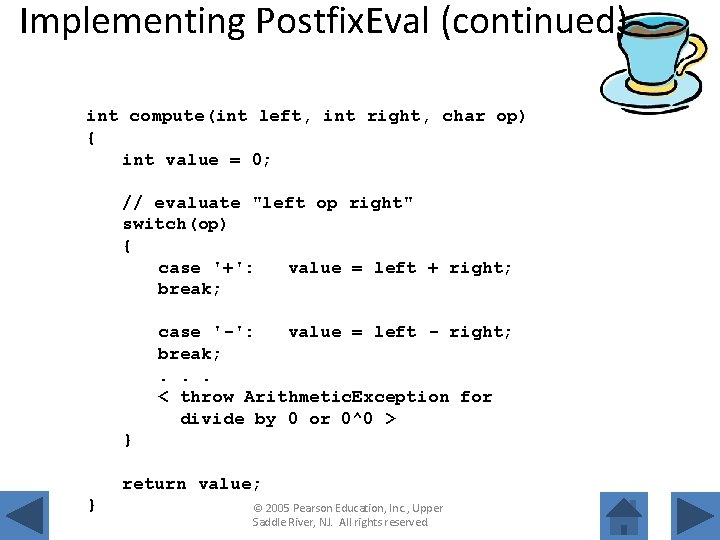
Implementing Postfix. Eval (continued) int compute(int left, int right, char op) { int value = 0; // evaluate "left op right" switch(op) { case '+': value = left + right; break; case '-': value = left - right; break; . . . < throw Arithmetic. Exception for divide by 0 or 0^0 > } return value; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
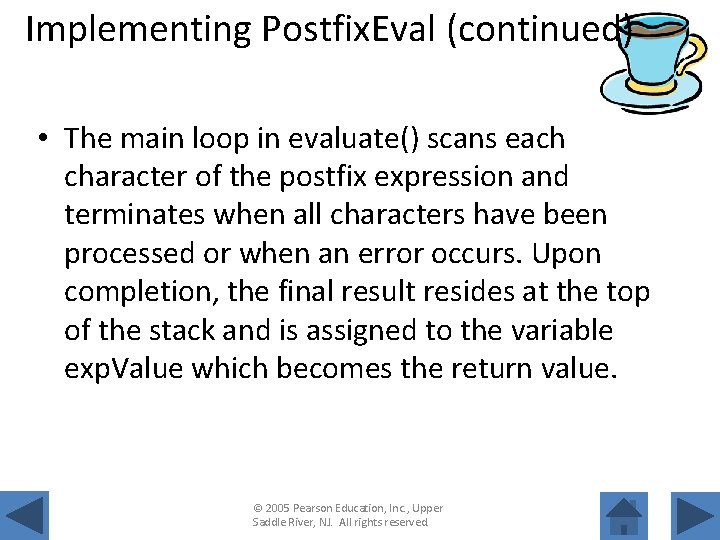
Implementing Postfix. Eval (continued) • The main loop in evaluate() scans each character of the postfix expression and terminates when all characters have been processed or when an error occurs. Upon completion, the final result resides at the top of the stack and is assigned to the variable exp. Value which becomes the return value. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
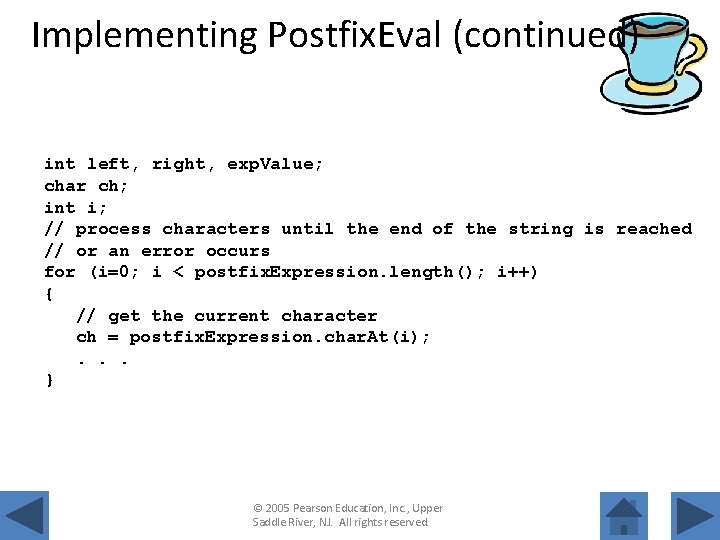
Implementing Postfix. Eval (continued) int left, right, exp. Value; char ch; int i; // process characters until the end of the string is reached // or an error occurs for (i=0; i < postfix. Expression. length(); i++) { // get the current character ch = postfix. Expression. char. At(i); . . . } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
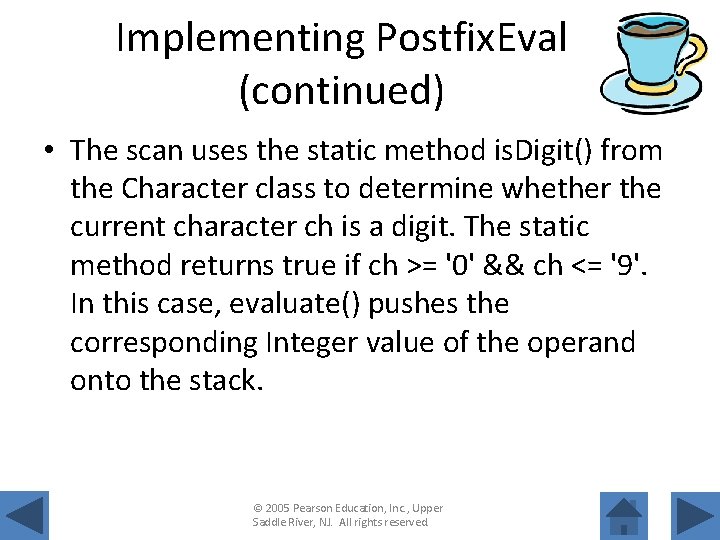
Implementing Postfix. Eval (continued) • The scan uses the static method is. Digit() from the Character class to determine whether the current character ch is a digit. The static method returns true if ch >= '0' && ch <= '9'. In this case, evaluate() pushes the corresponding Integer value of the operand onto the stack. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
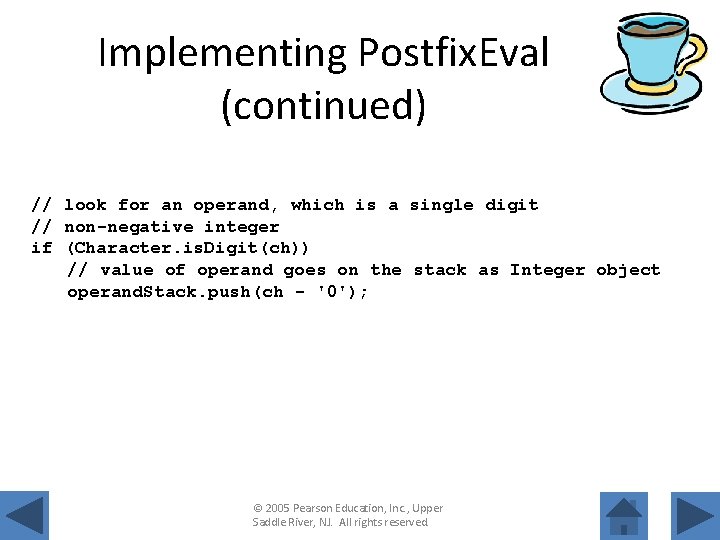
Implementing Postfix. Eval (continued) // look for an operand, which is a single digit // non-negative integer if (Character. is. Digit(ch)) // value of operand goes on the stack as Integer object operand. Stack. push(ch - '0'); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
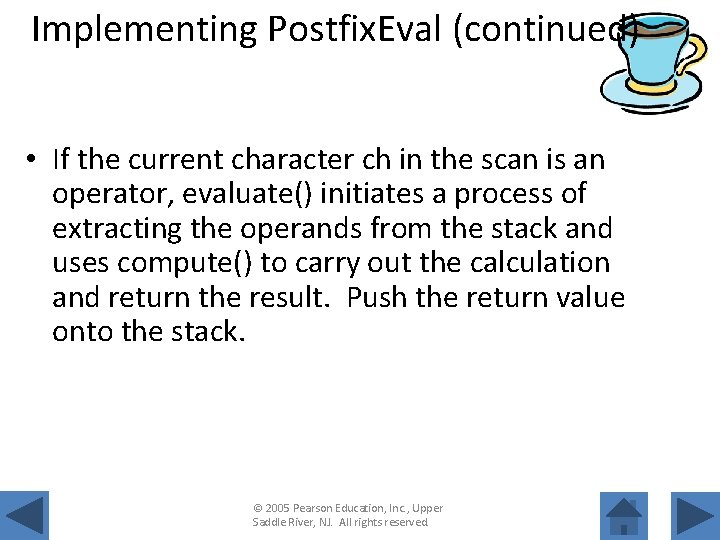
Implementing Postfix. Eval (continued) • If the current character ch in the scan is an operator, evaluate() initiates a process of extracting the operands from the stack and uses compute() to carry out the calculation and return the result. Push the return value onto the stack. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
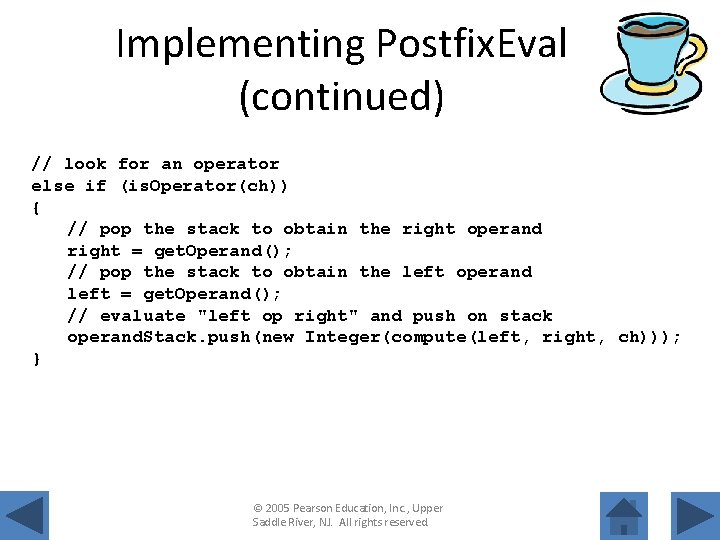
Implementing Postfix. Eval (continued) // look for an operator else if (is. Operator(ch)) { // pop the stack to obtain the right operand right = get. Operand(); // pop the stack to obtain the left operand left = get. Operand(); // evaluate "left op right" and push on stack operand. Stack. push(new Integer(compute(left, right, ch))); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
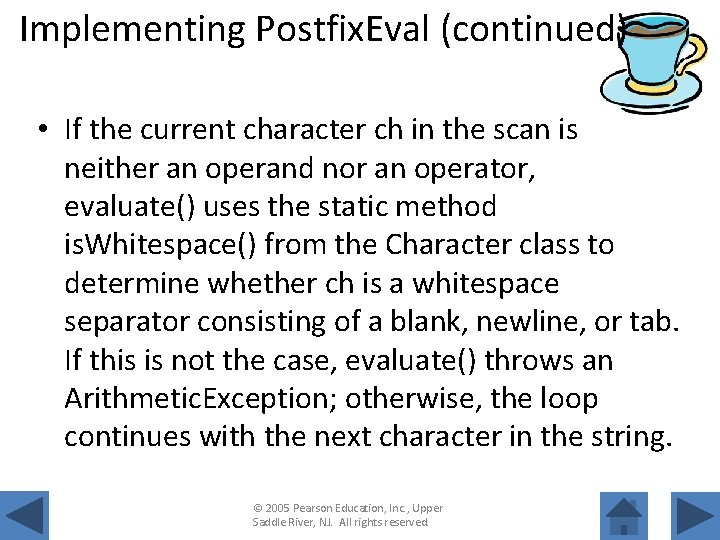
Implementing Postfix. Eval (continued) • If the current character ch in the scan is neither an operand nor an operator, evaluate() uses the static method is. Whitespace() from the Character class to determine whether ch is a whitespace separator consisting of a blank, newline, or tab. If this is not the case, evaluate() throws an Arithmetic. Exception; otherwise, the loop continues with the next character in the string. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
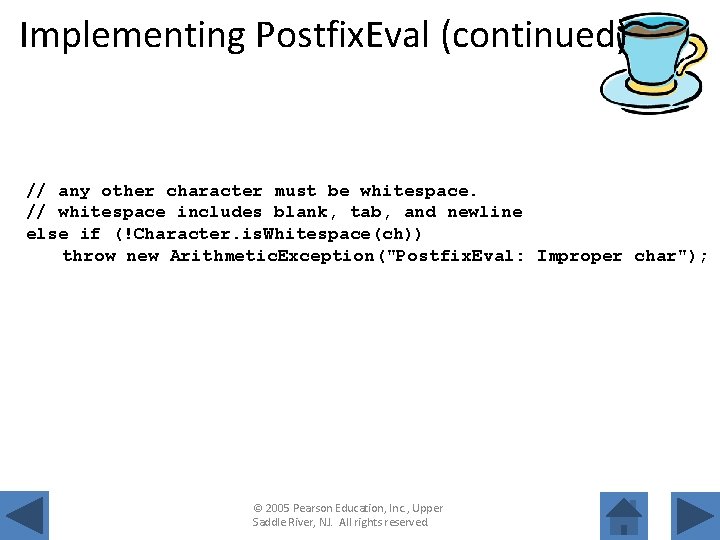
Implementing Postfix. Eval (continued) // any other character must be whitespace. // whitespace includes blank, tab, and newline else if (!Character. is. Whitespace(ch)) throw new Arithmetic. Exception("Postfix. Eval: Improper char"); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
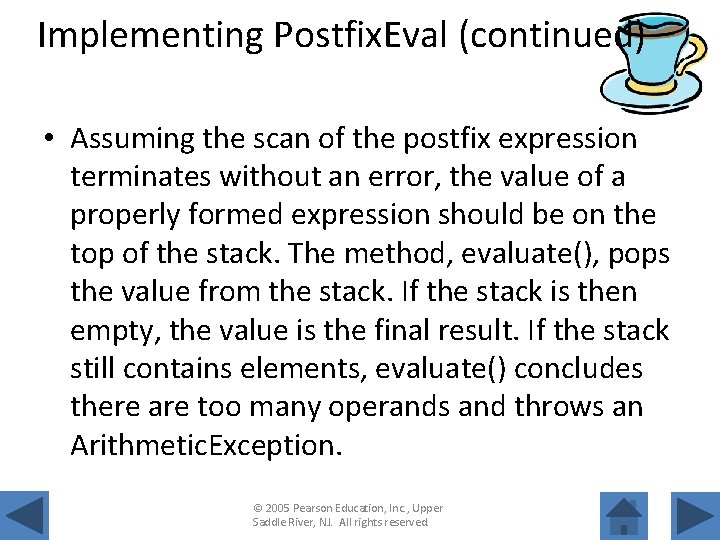
Implementing Postfix. Eval (continued) • Assuming the scan of the postfix expression terminates without an error, the value of a properly formed expression should be on the top of the stack. The method, evaluate(), pops the value from the stack. If the stack is then empty, the value is the final result. If the stack still contains elements, evaluate() concludes there are too many operands and throws an Arithmetic. Exception. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
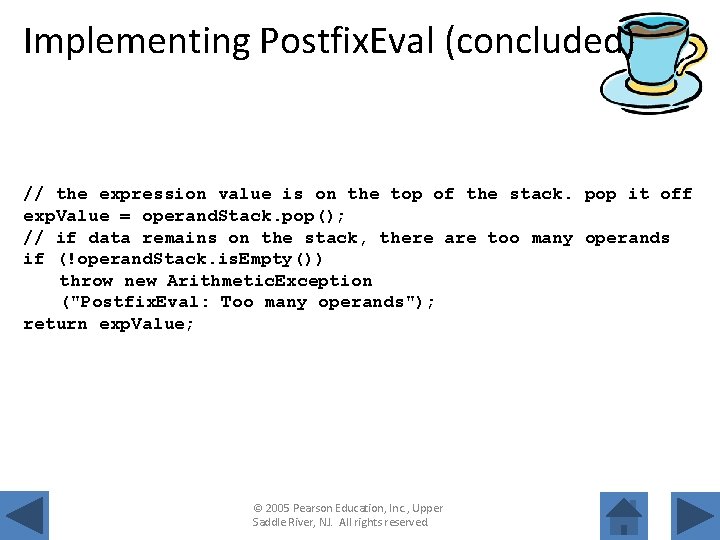
Implementing Postfix. Eval (concluded) // the expression value is on the top of the stack. pop it off exp. Value = operand. Stack. pop(); // if data remains on the stack, there are too many operands if (!operand. Stack. is. Empty()) throw new Arithmetic. Exception ("Postfix. Eval: Too many operands"); return exp. Value; © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
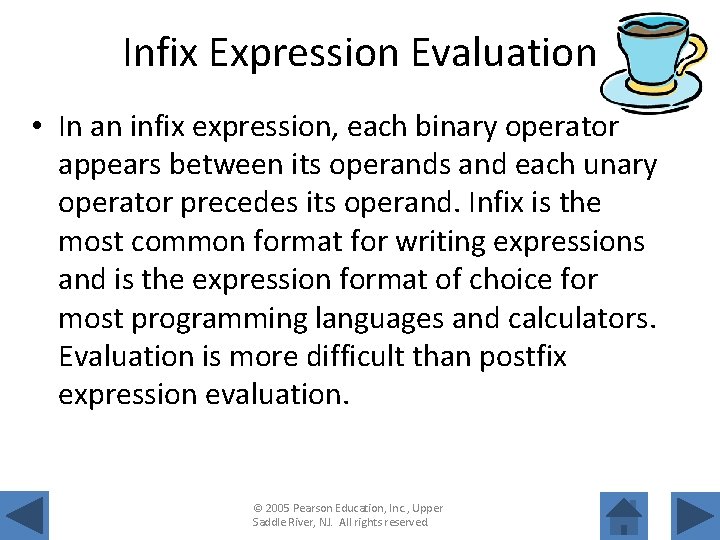
Infix Expression Evaluation • In an infix expression, each binary operator appears between its operands and each unary operator precedes its operand. Infix is the most common format for writing expressions and is the expression format of choice for most programming languages and calculators. Evaluation is more difficult than postfix expression evaluation. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
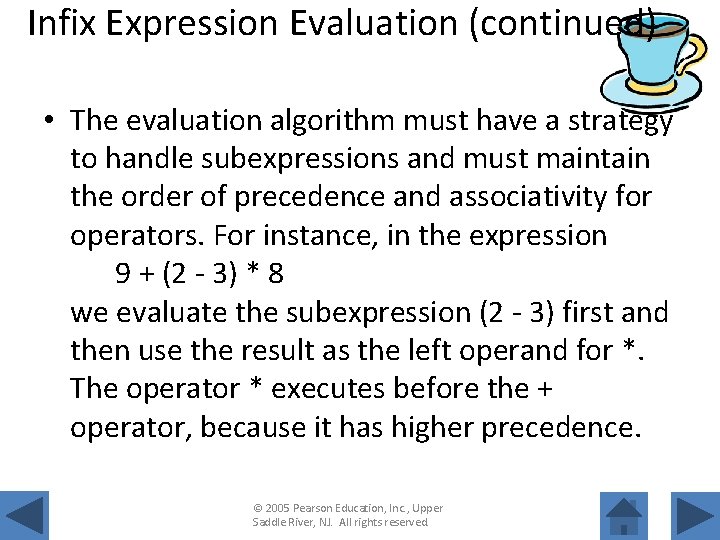
Infix Expression Evaluation (continued) • The evaluation algorithm must have a strategy to handle subexpressions and must maintain the order of precedence and associativity for operators. For instance, in the expression 9 + (2 - 3) * 8 we evaluate the subexpression (2 - 3) first and then use the result as the left operand for *. The operator * executes before the + operator, because it has higher precedence. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
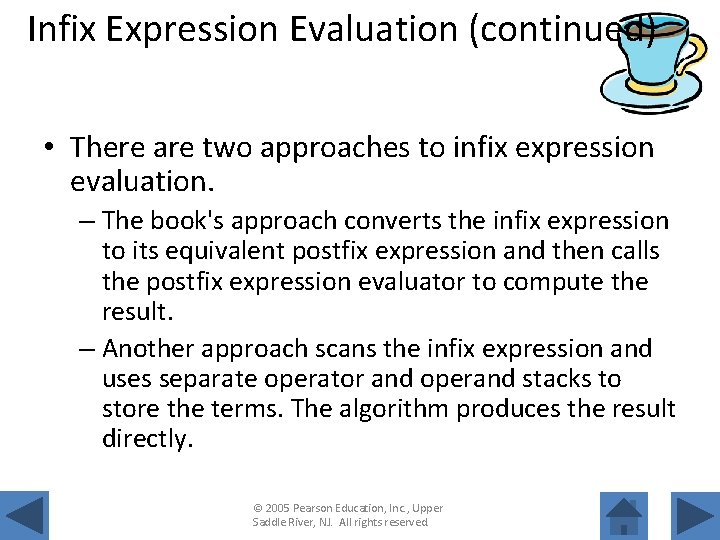
Infix Expression Evaluation (continued) • There are two approaches to infix expression evaluation. – The book's approach converts the infix expression to its equivalent postfix expression and then calls the postfix expression evaluator to compute the result. – Another approach scans the infix expression and uses separate operator and operand stacks to store the terms. The algorithm produces the result directly. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
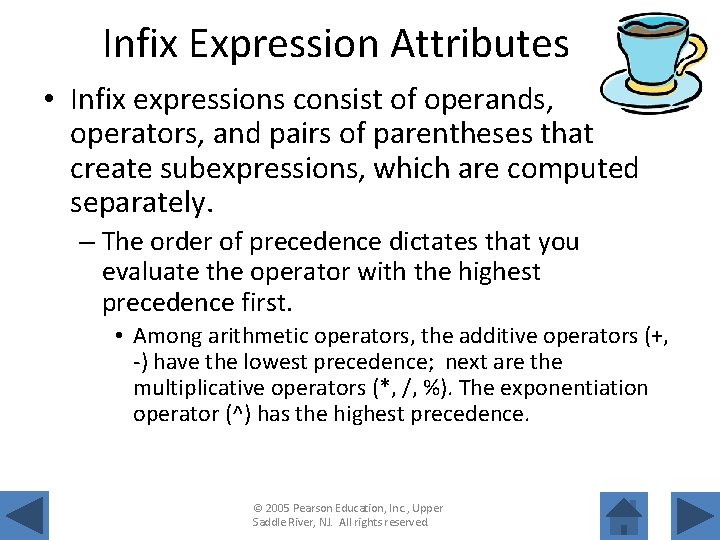
Infix Expression Attributes • Infix expressions consist of operands, operators, and pairs of parentheses that create subexpressions, which are computed separately. – The order of precedence dictates that you evaluate the operator with the highest precedence first. • Among arithmetic operators, the additive operators (+, -) have the lowest precedence; next are the multiplicative operators (*, /, %). The exponentiation operator (^) has the highest precedence. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
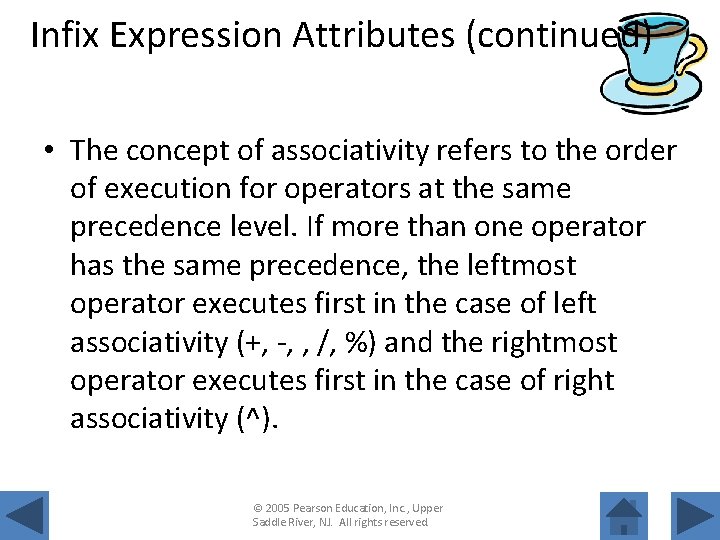
Infix Expression Attributes (continued) • The concept of associativity refers to the order of execution for operators at the same precedence level. If more than one operator has the same precedence, the leftmost operator executes first in the case of left associativity (+, -, , /, %) and the rightmost operator executes first in the case of right associativity (^). © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
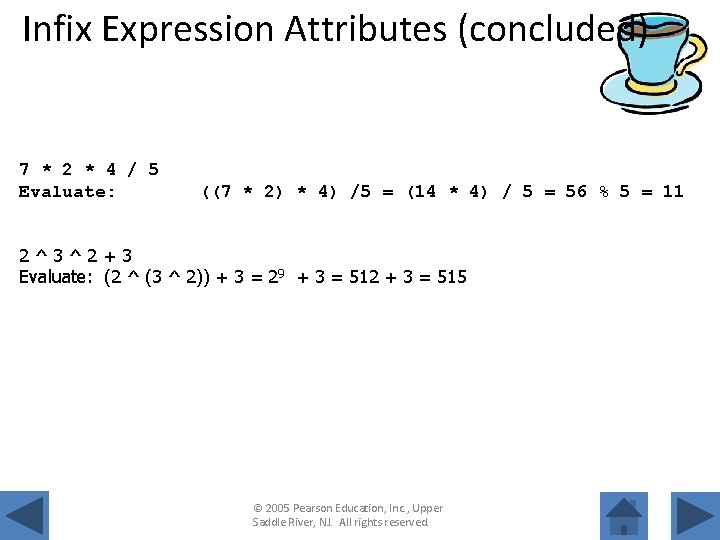
Infix Expression Attributes (concluded) 7 * 2 * 4 / 5 Evaluate: ((7 * 2) * 4) /5 = (14 * 4) / 5 = 56 % 5 = 11 2^3^2+3 Evaluate: (2 ^ (3 ^ 2)) + 3 = 29 + 3 = 512 + 3 = 515 © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
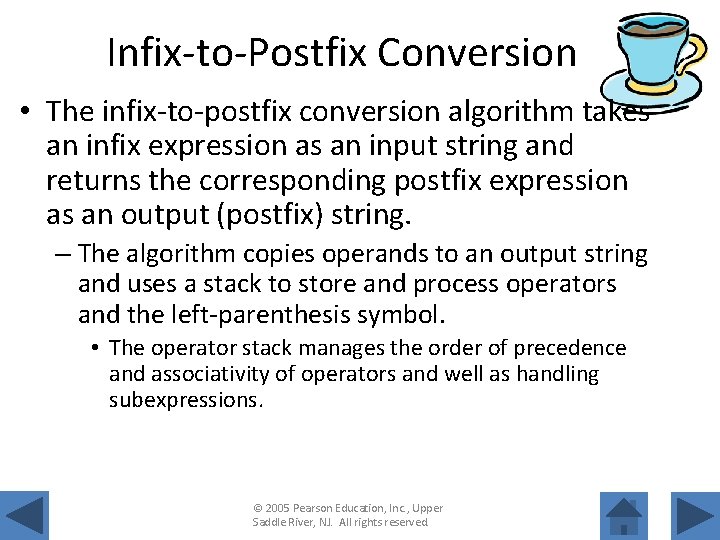
Infix-to-Postfix Conversion • The infix-to-postfix conversion algorithm takes an infix expression as an input string and returns the corresponding postfix expression as an output (postfix) string. – The algorithm copies operands to an output string and uses a stack to store and process operators and the left-parenthesis symbol. • The operator stack manages the order of precedence and associativity of operators and well as handling subexpressions. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
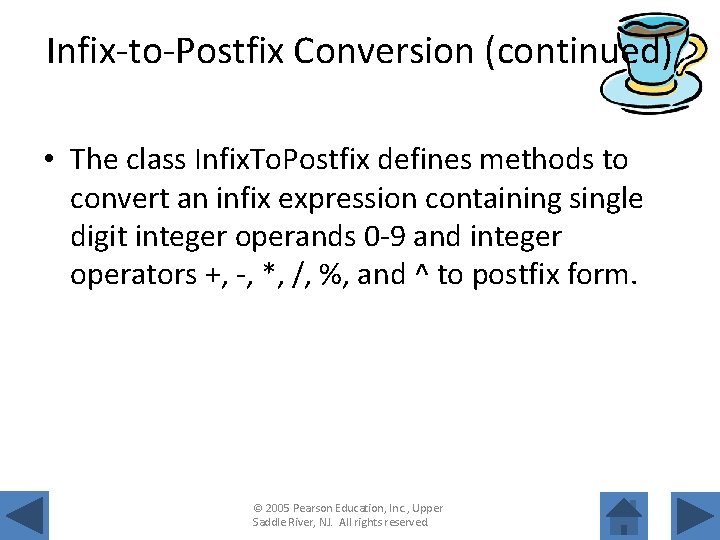
Infix-to-Postfix Conversion (continued) • The class Infix. To. Postfix defines methods to convert an infix expression containing single digit integer operands 0 -9 and integer operators +, -, *, /, %, and ^ to postfix form. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
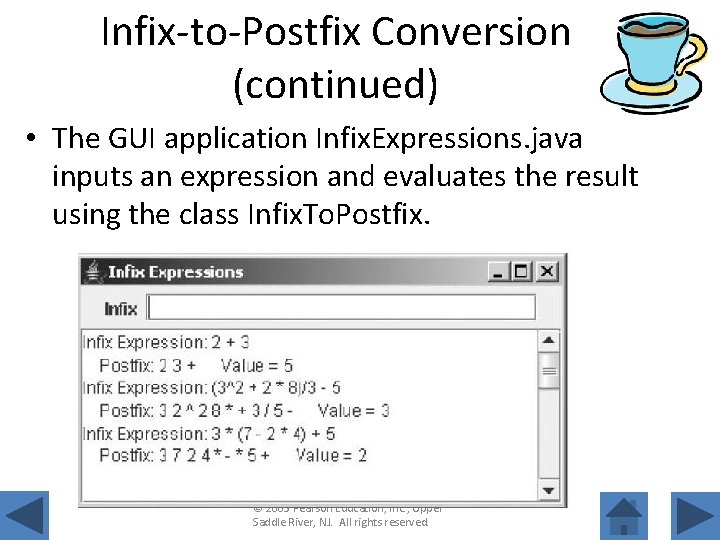
Infix-to-Postfix Conversion (continued) • The GUI application Infix. Expressions. java inputs an expression and evaluates the result using the class Infix. To. Postfix. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
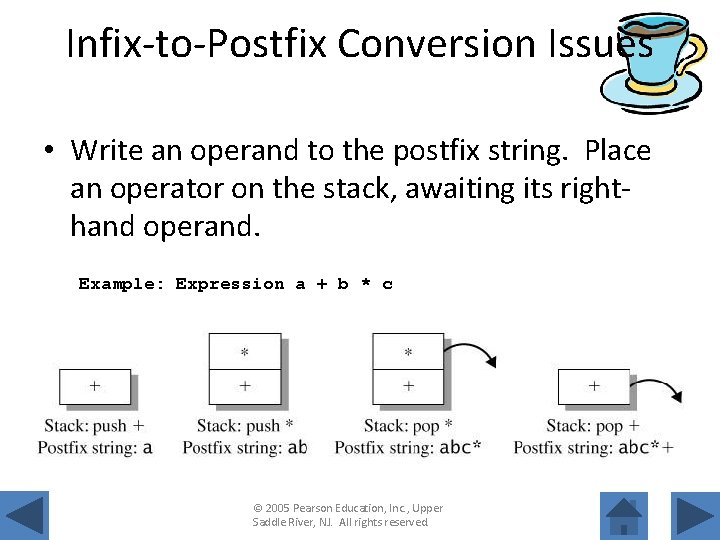
Infix-to-Postfix Conversion Issues • Write an operand to the postfix string. Place an operator on the stack, awaiting its righthand operand. Example: Expression a + b * c © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
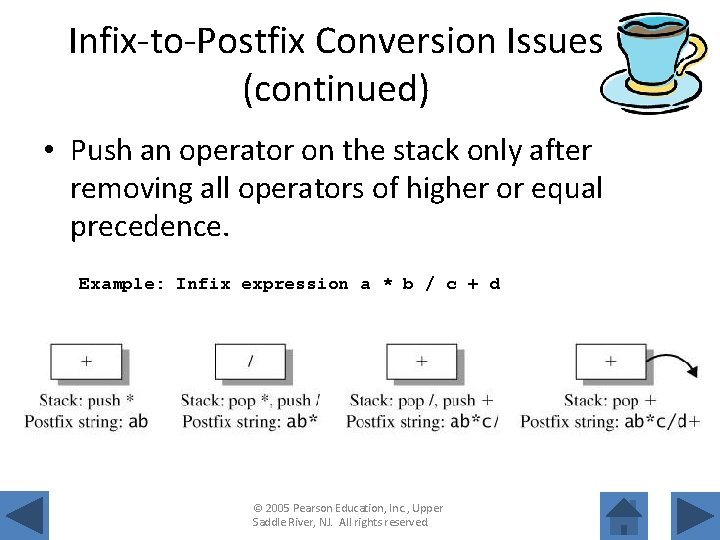
Infix-to-Postfix Conversion Issues (continued) • Push an operator on the stack only after removing all operators of higher or equal precedence. Example: Infix expression a * b / c + d © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
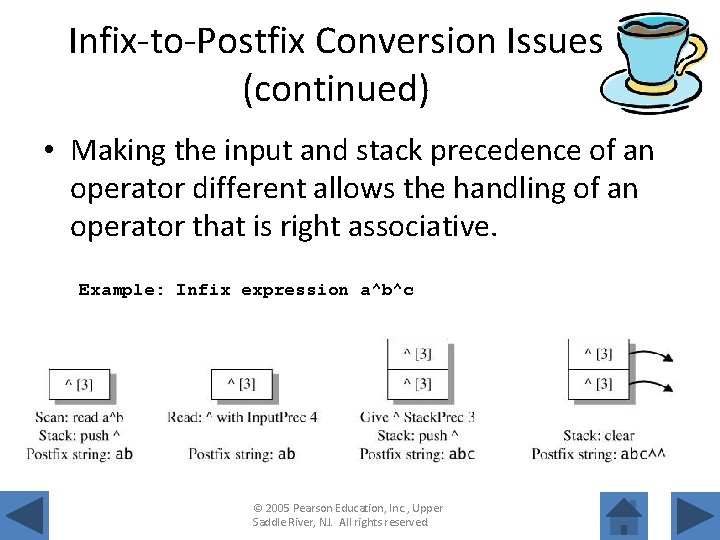
Infix-to-Postfix Conversion Issues (continued) • Making the input and stack precedence of an operator different allows the handling of an operator that is right associative. Example: Infix expression a^b^c © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
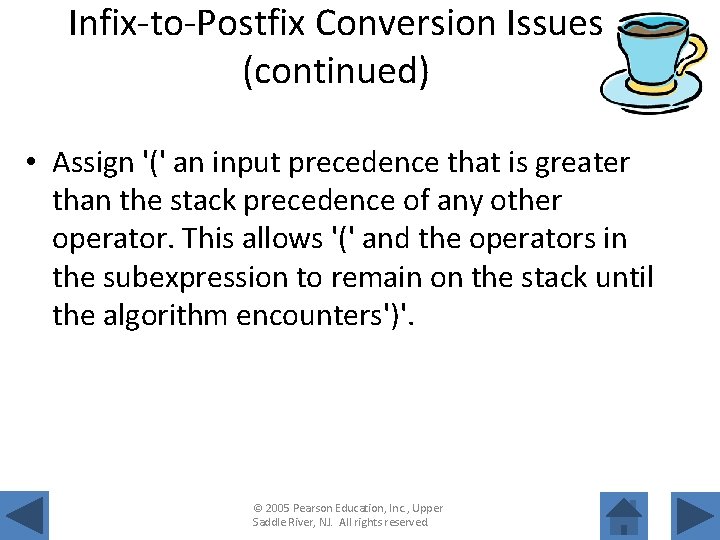
Infix-to-Postfix Conversion Issues (continued) • Assign '(' an input precedence that is greater than the stack precedence of any other operator. This allows '(' and the operators in the subexpression to remain on the stack until the algorithm encounters')'. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
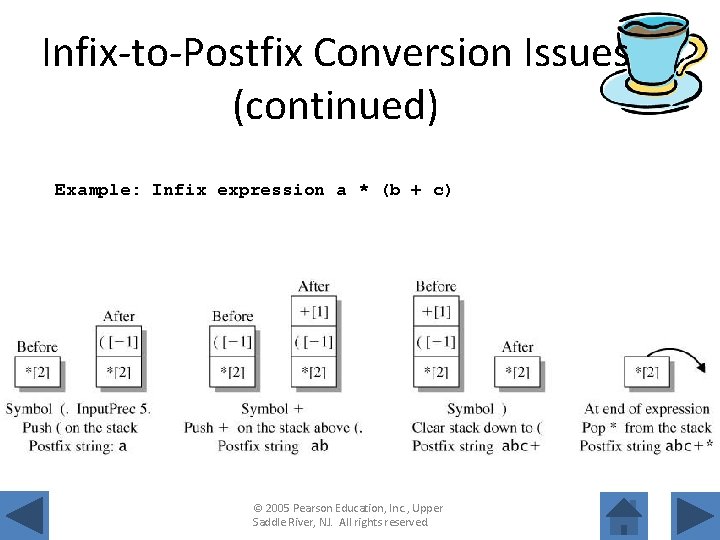
Infix-to-Postfix Conversion Issues (continued) Example: Infix expression a * (b + c) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
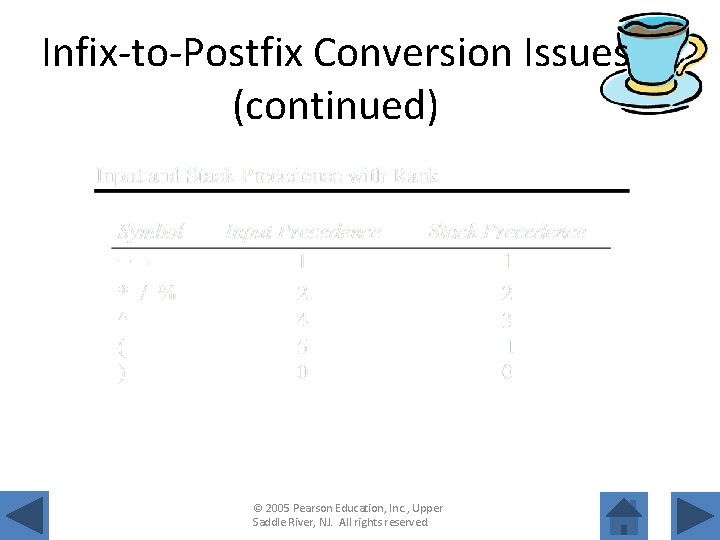
Infix-to-Postfix Conversion Issues (continued) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
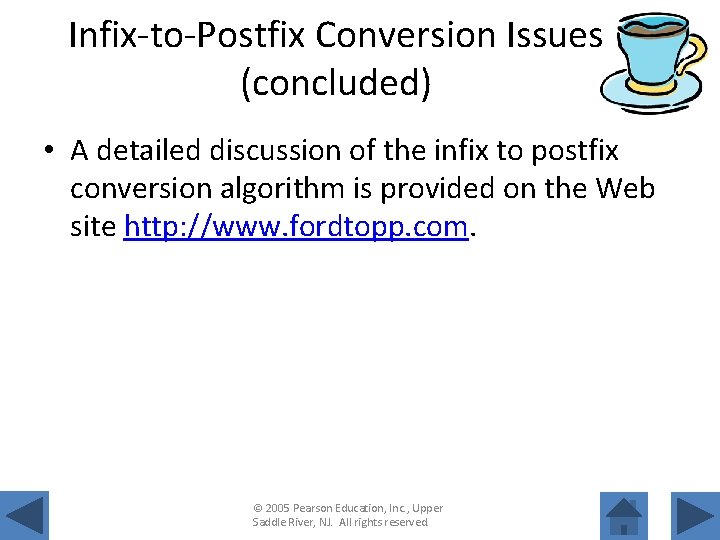
Infix-to-Postfix Conversion Issues (concluded) • A detailed discussion of the infix to postfix conversion algorithm is provided on the Web site http: //www. fordtopp. com. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
Advanced data structures in java
Dynamic data structure in java
Data structures and abstractions with java
Java data structures
Data structures in java
Data structures in java
Data structures using java
Binary search tree java
Data structures using java
Homologous structures examples
Java software structures 4th edition
Iso 22301 utbildning
Typiska novell drag
Tack för att ni lyssnade bild
Ekologiskt fotavtryck
Shingelfrisyren
En lathund för arbete med kontinuitetshantering
Särskild löneskatt för pensionskostnader
Tidbok
Anatomi organ reproduksi
Vad är densitet
Datorkunskap för nybörjare
Boverket ka
Debatt mall
Delegerande ledarstil
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Formel för lufttryck
Offentlig förvaltning
Jag har nigit för nymånens skära
Presentera för publik crossboss
Argument för teckenspråk som minoritetsspråk
Bat mitza
Klassificeringsstruktur för kommunala verksamheter
Epiteltyper
Claes martinsson
Centrum för kunskap och säkerhet
Programskede byggprocessen
Mat för unga idrottare
Verktyg för automatisering av utbetalningar
Rutin för avvikelsehantering
Smärtskolan kunskap för livet
Ministerstyre för och nackdelar
Tack för att ni har lyssnat
Referatmarkeringar
Redogör för vad psykologi är
Matematisk modellering eksempel
Tack för att ni har lyssnat
Borra hål för knoppar
Vilken grundregel finns det för tronföljden i sverige?
Relativ standardavvikelse formel
Tack för att ni har lyssnat
Steg för steg rita
Vad är verksamhetsanalys
Tobinskatten för och nackdelar
Toppslätskivling dos
Mästar lärling modellen
Egg för emanuel
Elektronik för barn
Mantel för kvinnor i antikens rom
Strategi för svensk viltförvaltning
Kung dog 1611
Humanitr
Ro i rom pax
Tack för att ni lyssnade
Multiplikation med decimaltal uppgifter
Bunden form
Inköpsprocessen steg för steg
Fuktmätningar i betong enlig rbk
Ledarskapsteorier
Skivepiteldysplasi
Myndigheten för delaktighet
Frgar
Sju principer för tillitsbaserad styrning
Läkarutlåtande för livränta
Karttecken punkthöjd