Chapter 3 1 Singly Linked List Data Structures
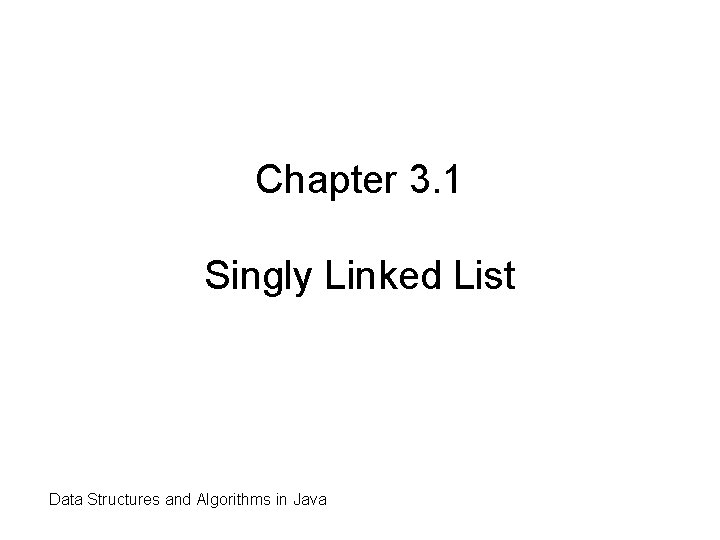
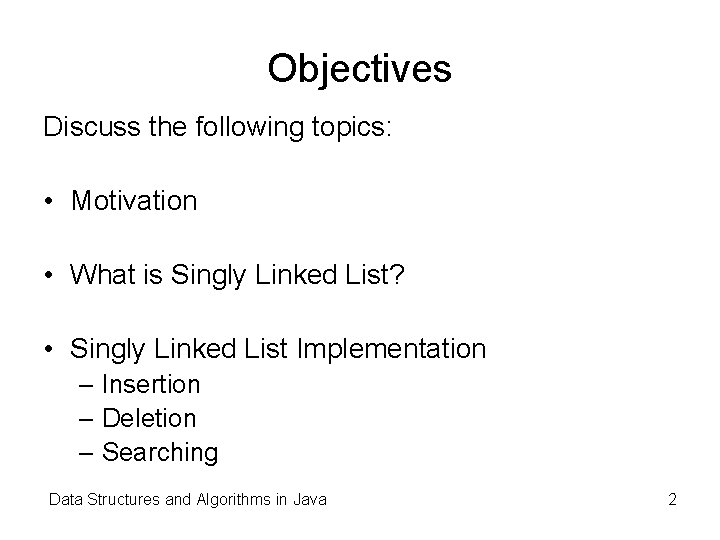
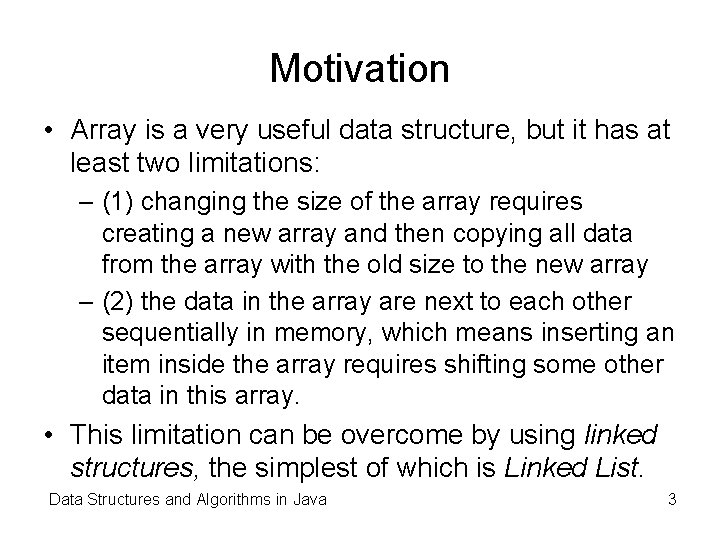
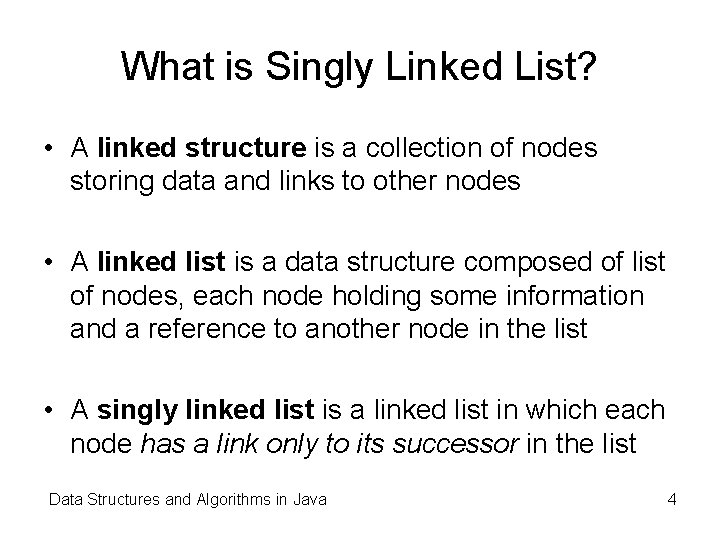
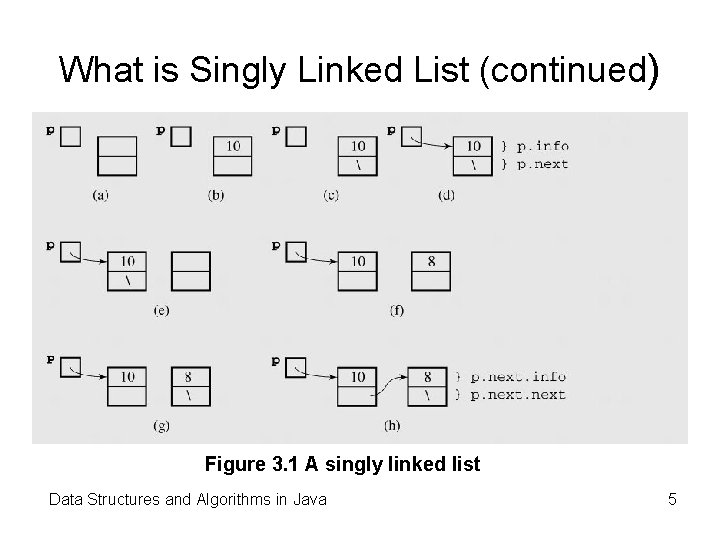
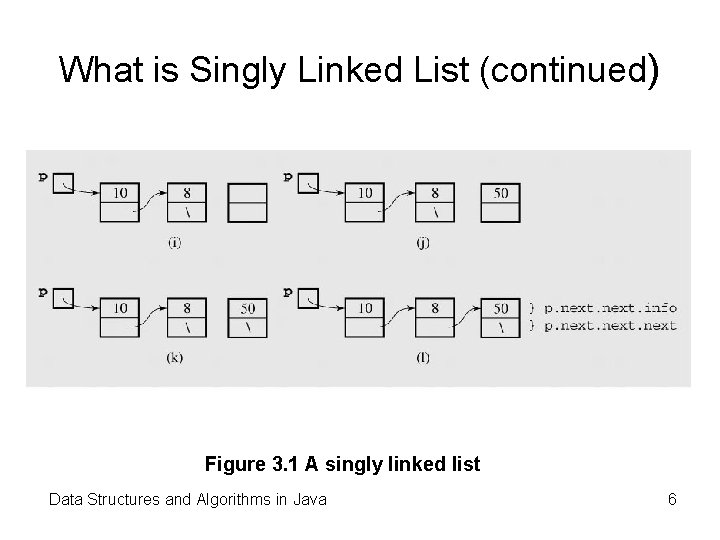
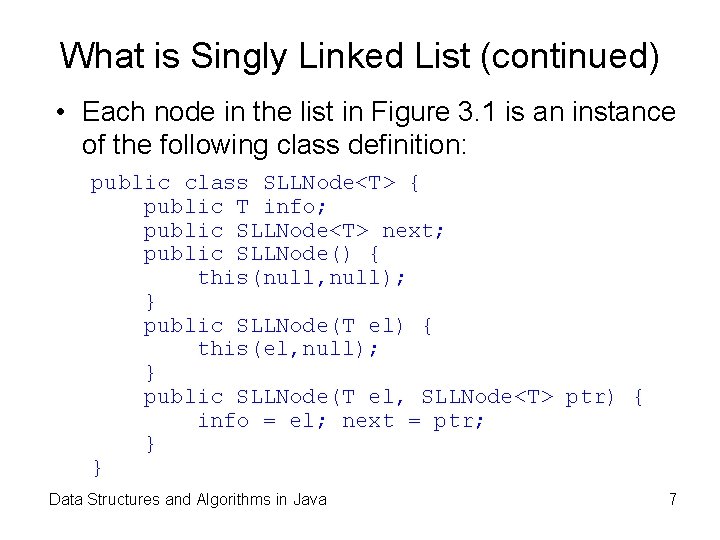
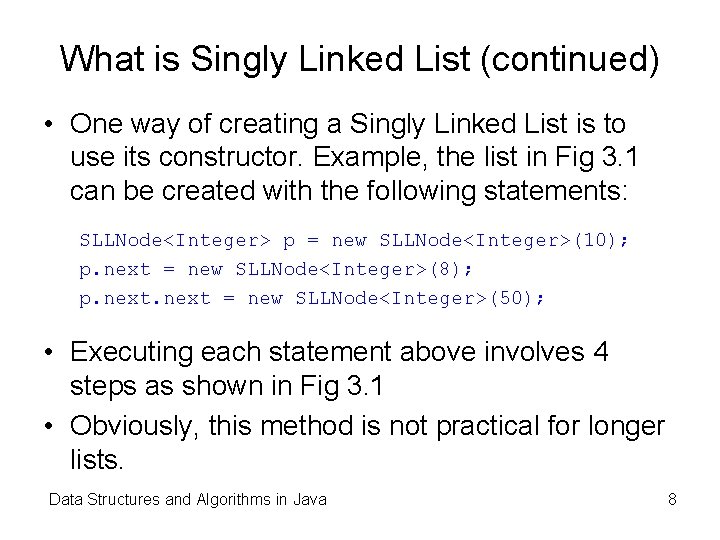
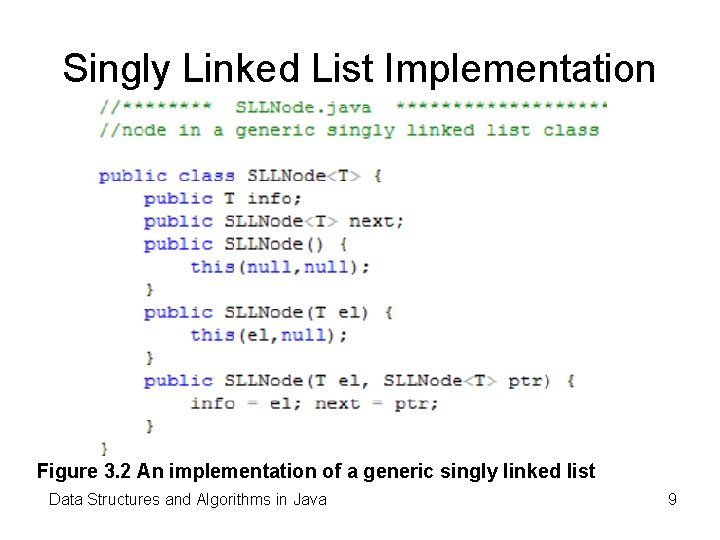
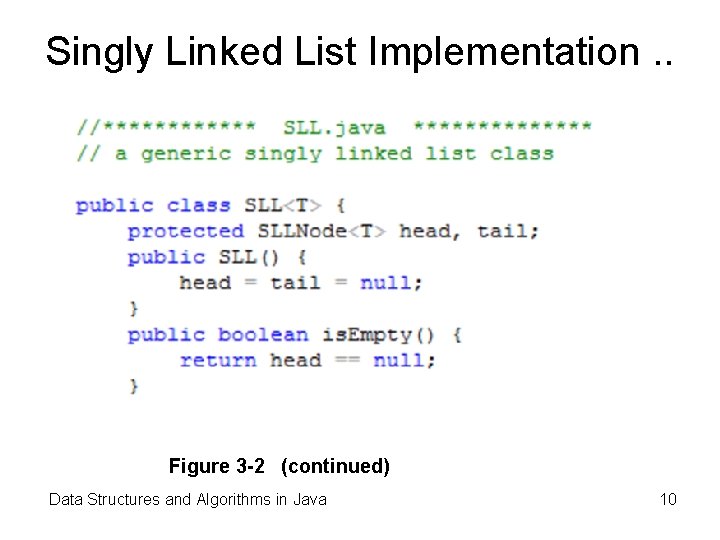
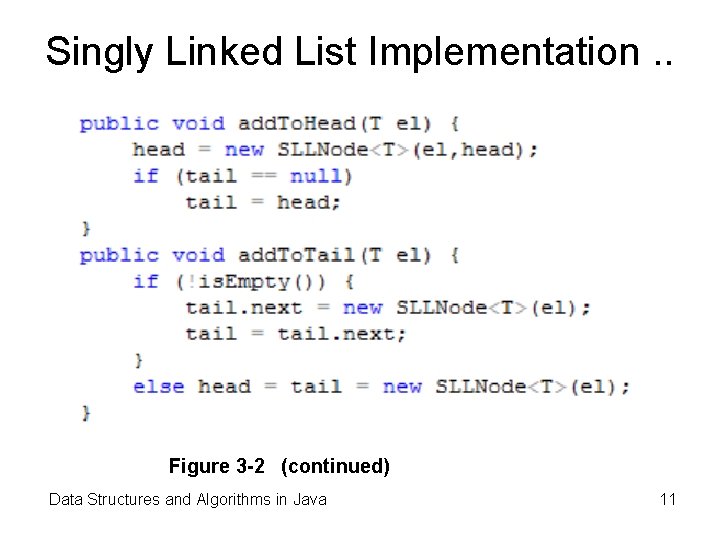
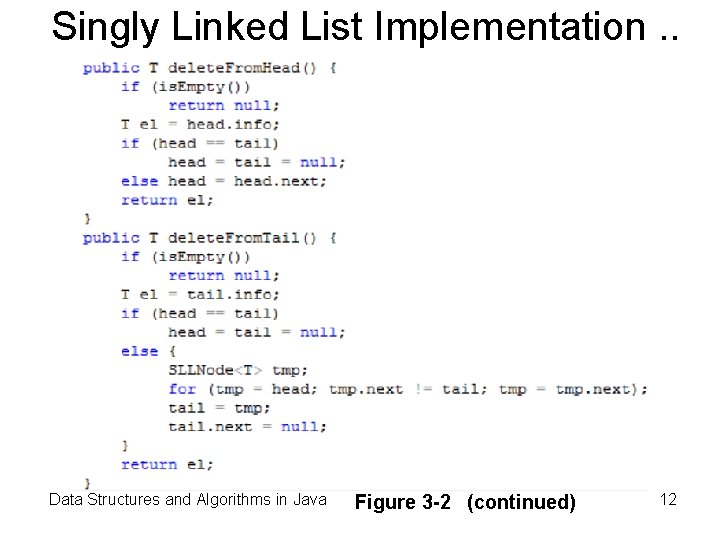
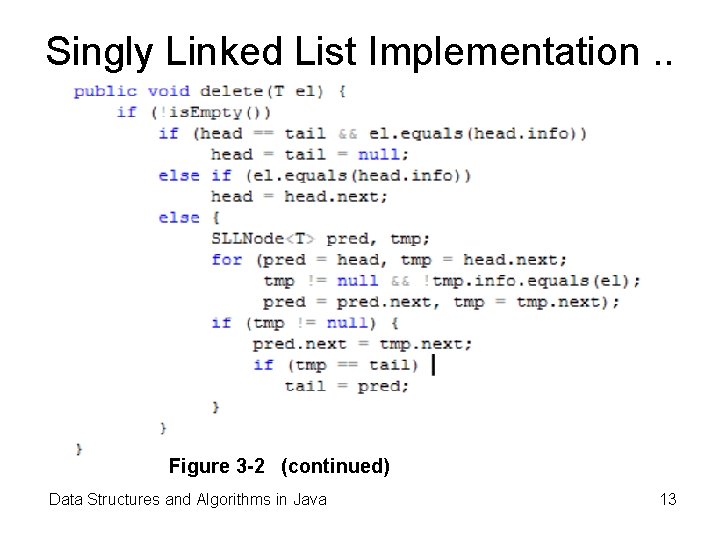
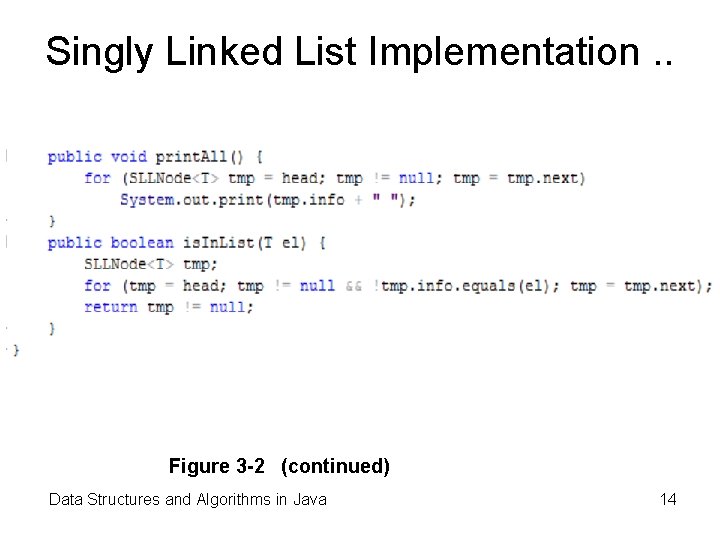
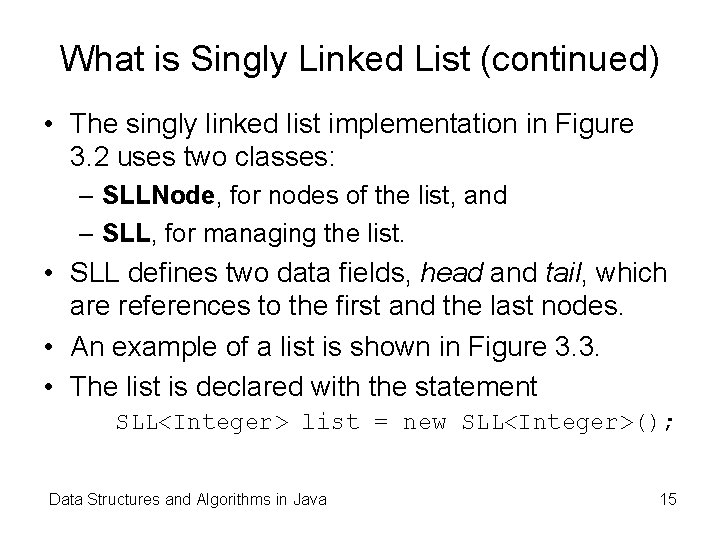
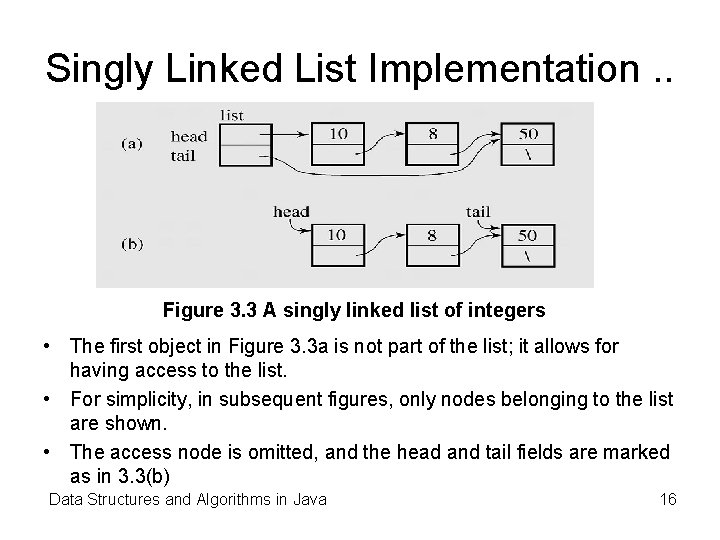
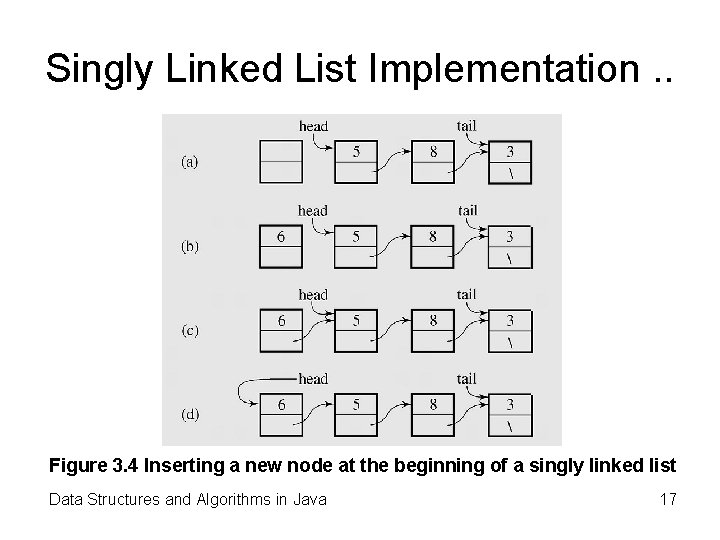
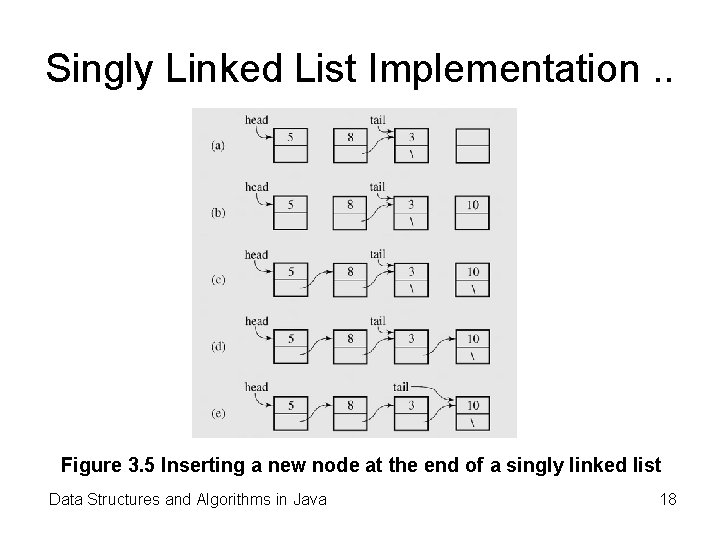
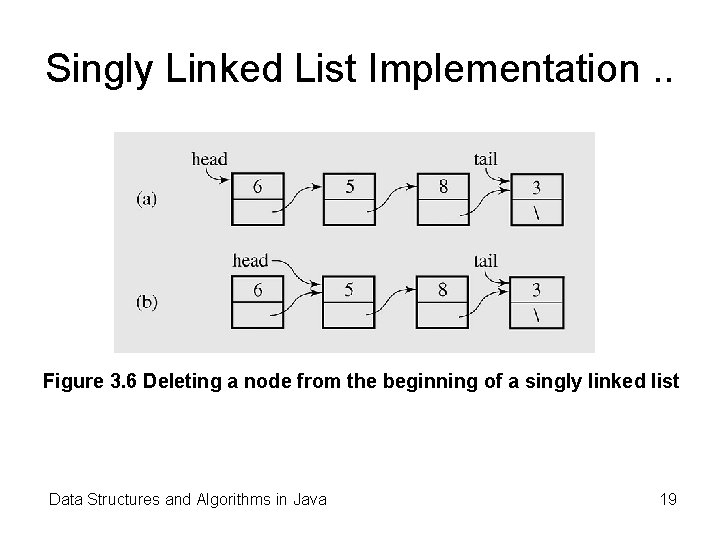
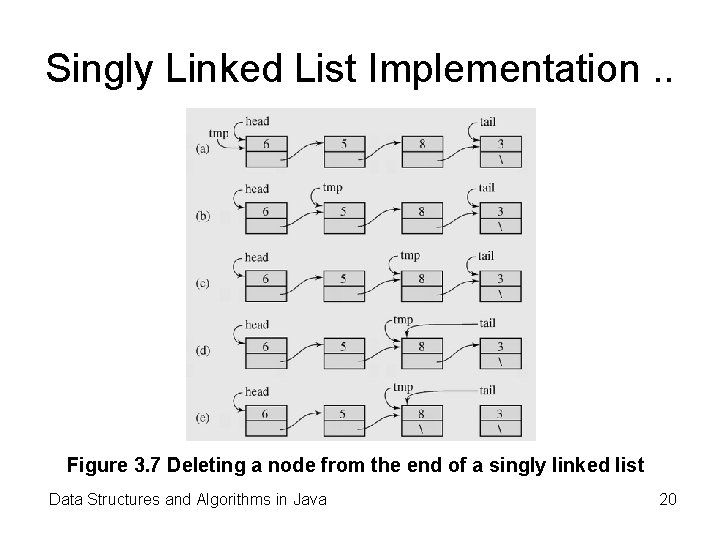
- Slides: 20
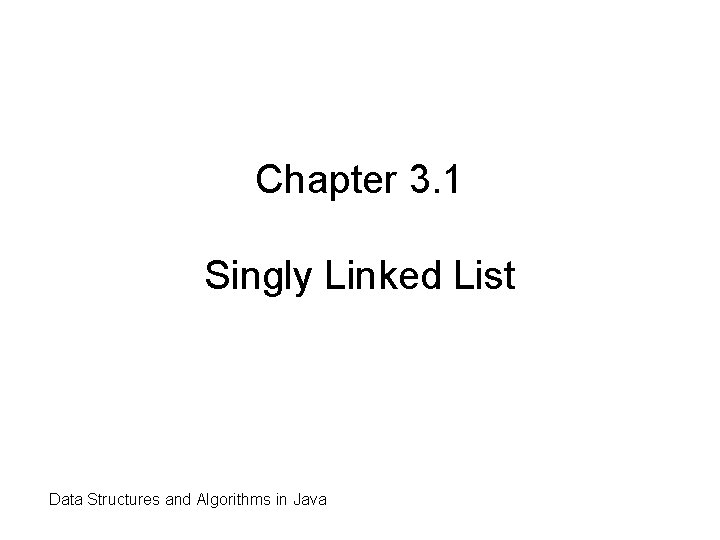
Chapter 3. 1 Singly Linked List Data Structures and Algorithms in Java
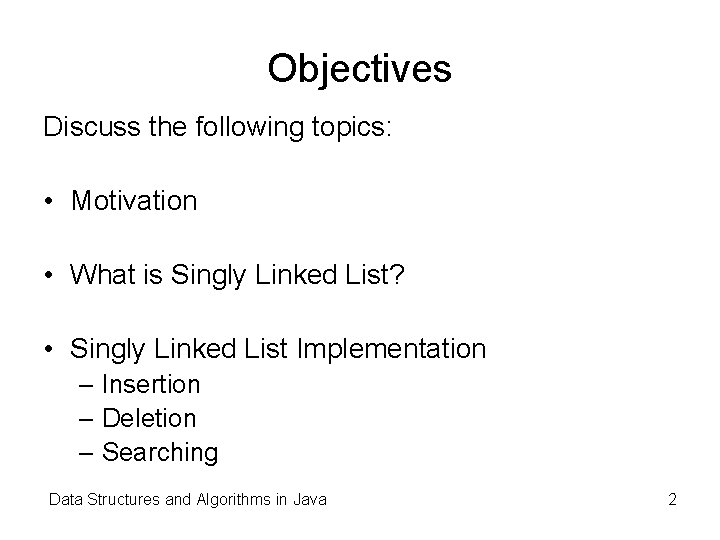
Objectives Discuss the following topics: • Motivation • What is Singly Linked List? • Singly Linked List Implementation – Insertion – Deletion – Searching Data Structures and Algorithms in Java 2
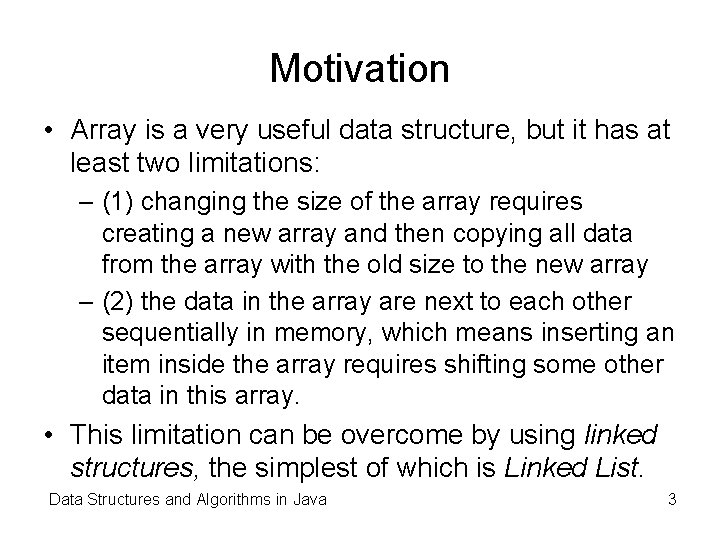
Motivation • Array is a very useful data structure, but it has at least two limitations: – (1) changing the size of the array requires creating a new array and then copying all data from the array with the old size to the new array – (2) the data in the array are next to each other sequentially in memory, which means inserting an item inside the array requires shifting some other data in this array. • This limitation can be overcome by using linked structures, the simplest of which is Linked List. Data Structures and Algorithms in Java 3
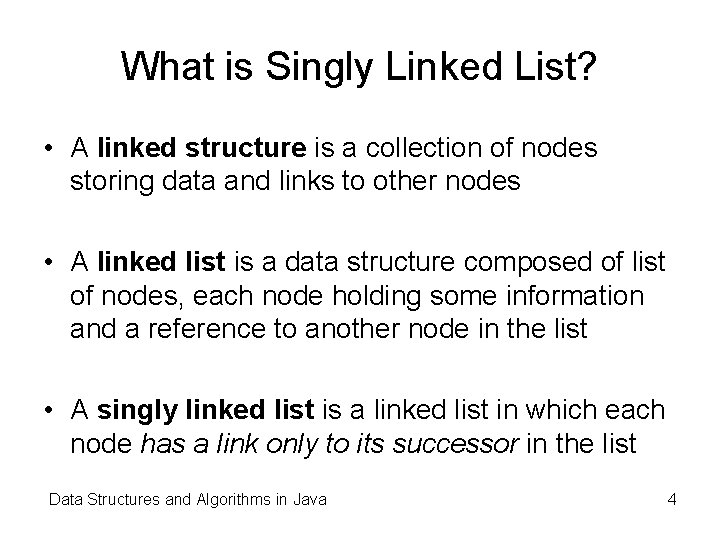
What is Singly Linked List? • A linked structure is a collection of nodes storing data and links to other nodes • A linked list is a data structure composed of list of nodes, each node holding some information and a reference to another node in the list • A singly linked list is a linked list in which each node has a link only to its successor in the list Data Structures and Algorithms in Java 4
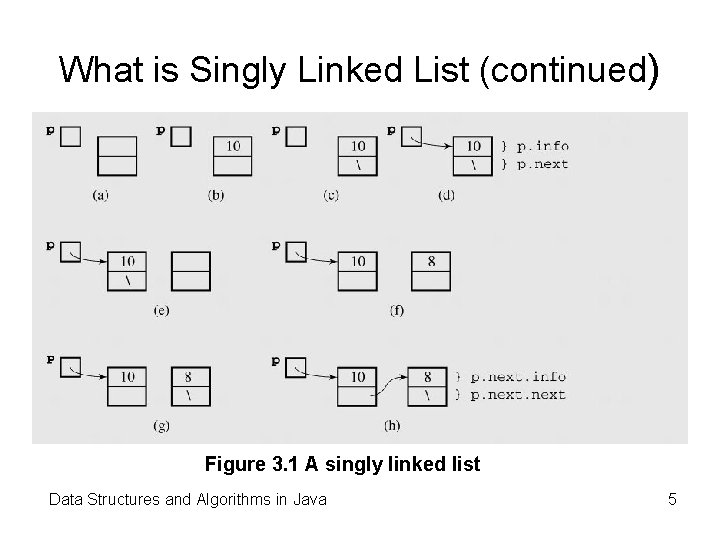
What is Singly Linked List (continued) Figure 3. 1 A singly linked list Data Structures and Algorithms in Java 5
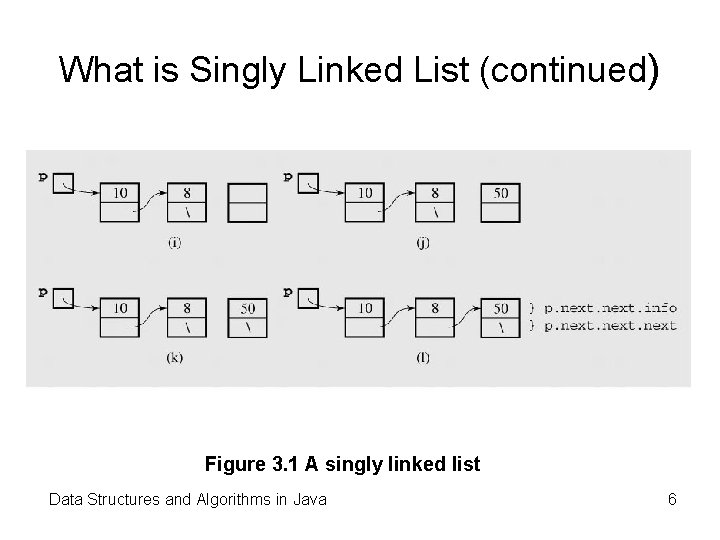
What is Singly Linked List (continued) Figure 3. 1 A singly linked list Data Structures and Algorithms in Java 6
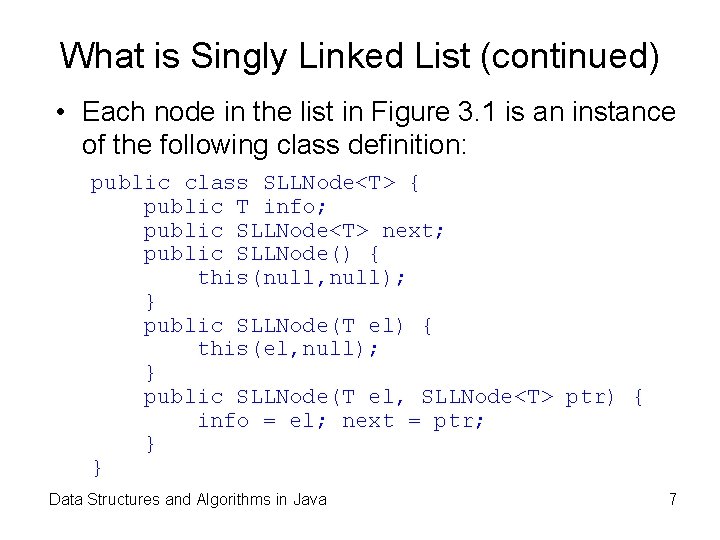
What is Singly Linked List (continued) • Each node in the list in Figure 3. 1 is an instance of the following class definition: public class SLLNode<T> { public T info; public SLLNode<T> next; public SLLNode() { this(null, null); } public SLLNode(T el) { this(el, null); } public SLLNode(T el, SLLNode<T> ptr) { info = el; next = ptr; } } Data Structures and Algorithms in Java 7
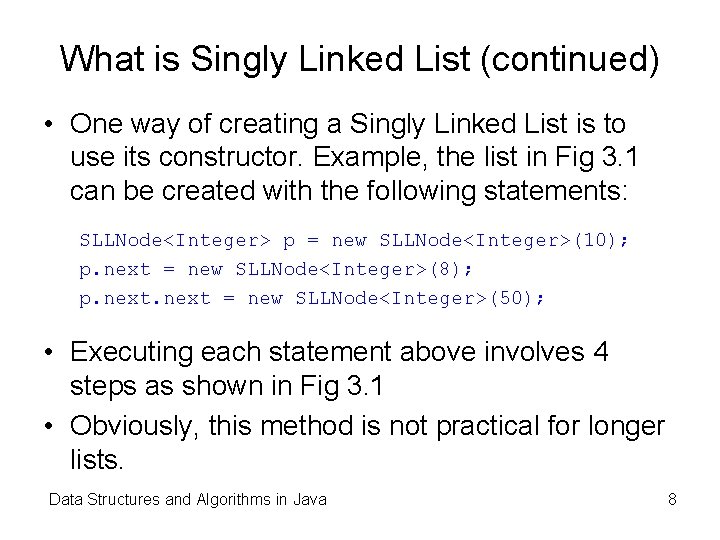
What is Singly Linked List (continued) • One way of creating a Singly Linked List is to use its constructor. Example, the list in Fig 3. 1 can be created with the following statements: SLLNode<Integer> p = new SLLNode<Integer>(10); p. next = new SLLNode<Integer>(8); p. next = new SLLNode<Integer>(50); • Executing each statement above involves 4 steps as shown in Fig 3. 1 • Obviously, this method is not practical for longer lists. Data Structures and Algorithms in Java 8
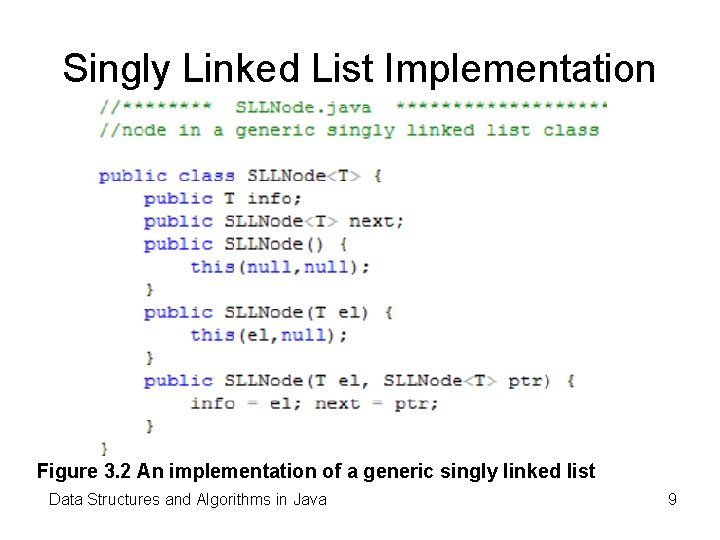
Singly Linked List Implementation Figure 3. 2 An implementation of a generic singly linked list Data Structures and Algorithms in Java 9
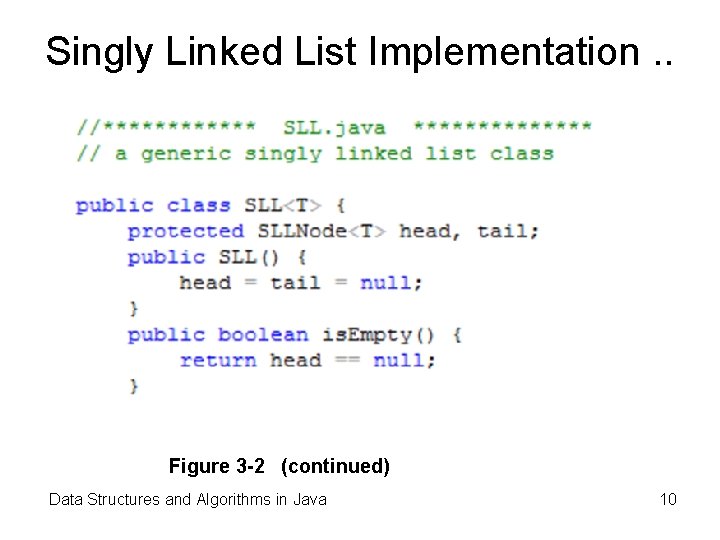
Singly Linked List Implementation. . Figure 3 -2 (continued) Data Structures and Algorithms in Java 10
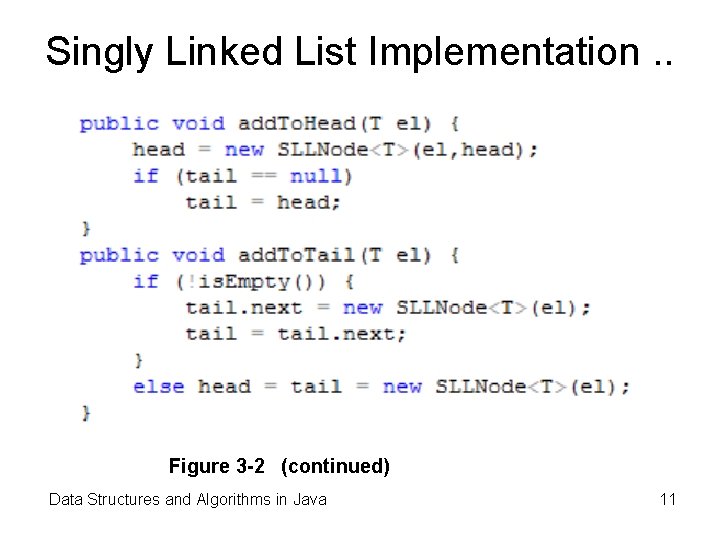
Singly Linked List Implementation. . Figure 3 -2 (continued) Data Structures and Algorithms in Java 11
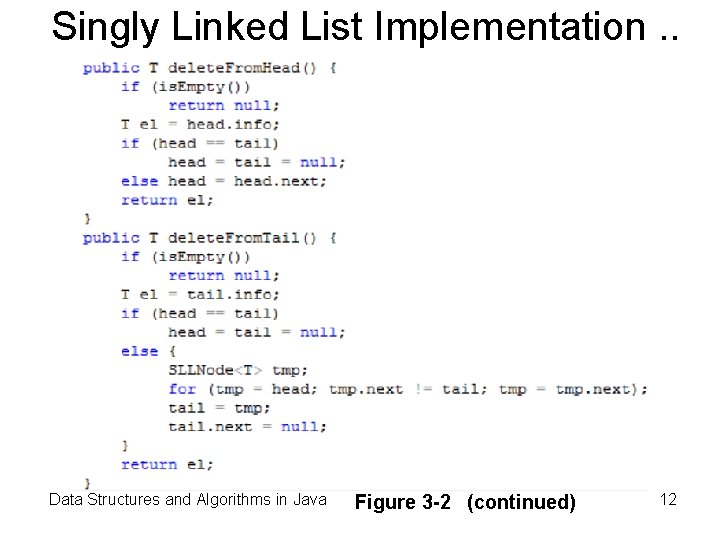
Singly Linked List Implementation. . Data Structures and Algorithms in Java Figure 3 -2 (continued) 12
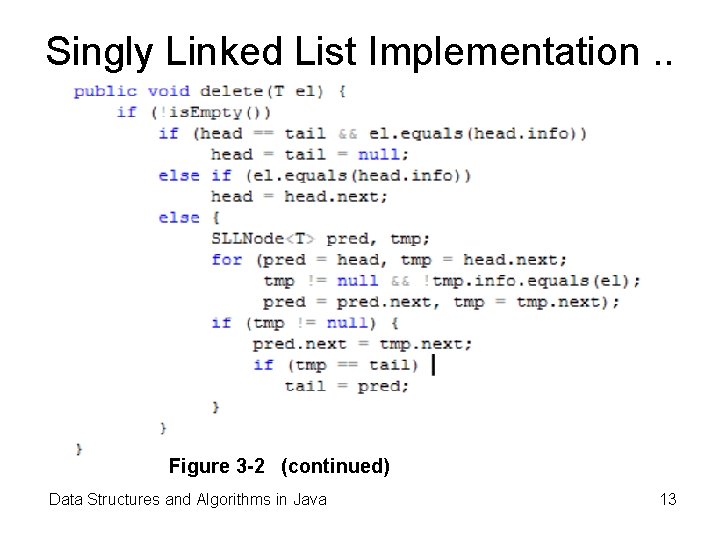
Singly Linked List Implementation. . Figure 3 -2 (continued) Data Structures and Algorithms in Java 13
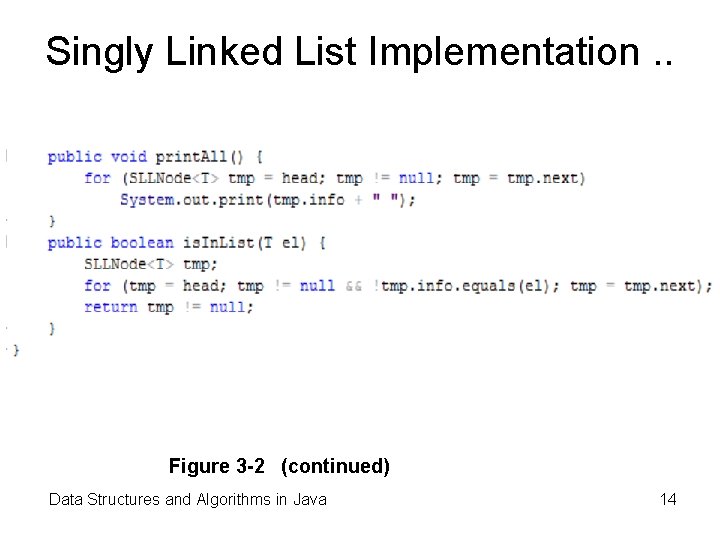
Singly Linked List Implementation. . Figure 3 -2 (continued) Data Structures and Algorithms in Java 14
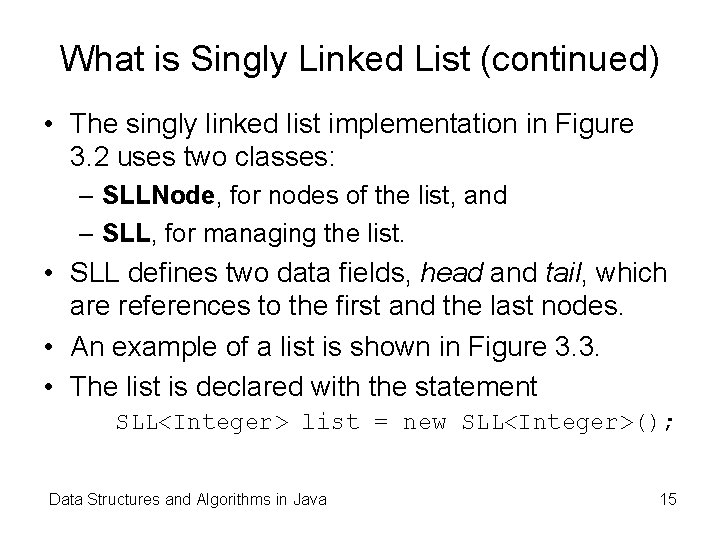
What is Singly Linked List (continued) • The singly linked list implementation in Figure 3. 2 uses two classes: – SLLNode, for nodes of the list, and – SLL, for managing the list. • SLL defines two data fields, head and tail, which are references to the first and the last nodes. • An example of a list is shown in Figure 3. 3. • The list is declared with the statement SLL<Integer> list = new SLL<Integer>(); Data Structures and Algorithms in Java 15
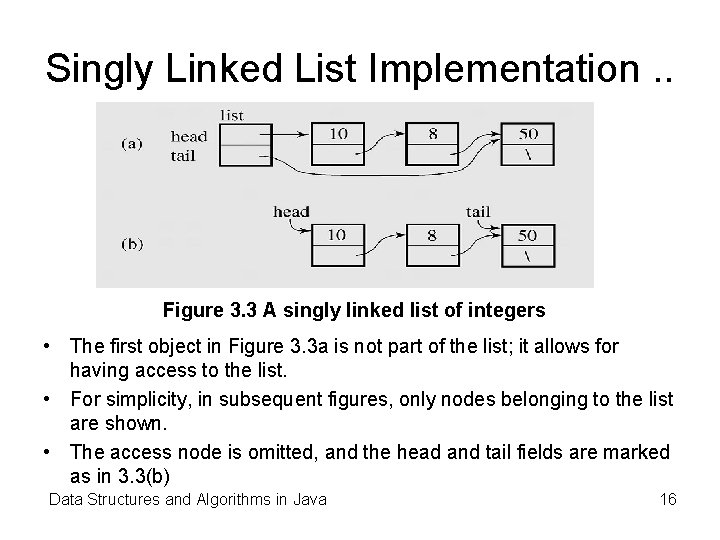
Singly Linked List Implementation. . Figure 3. 3 A singly linked list of integers • The first object in Figure 3. 3 a is not part of the list; it allows for having access to the list. • For simplicity, in subsequent figures, only nodes belonging to the list are shown. • The access node is omitted, and the head and tail fields are marked as in 3. 3(b) Data Structures and Algorithms in Java 16
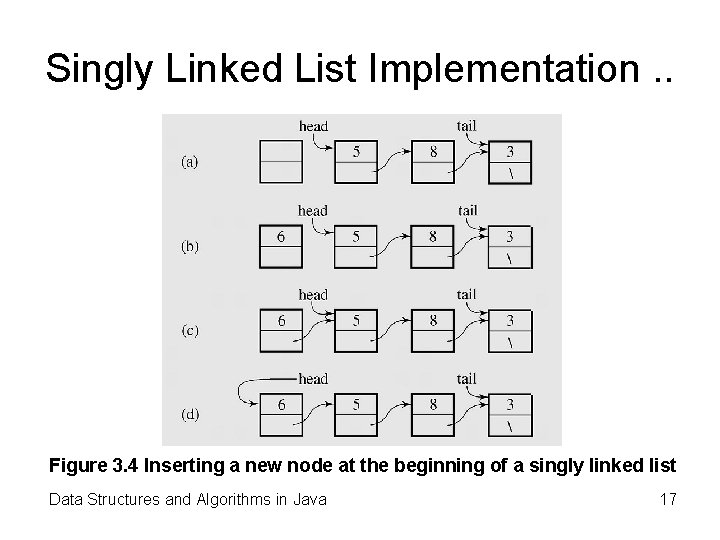
Singly Linked List Implementation. . Figure 3. 4 Inserting a new node at the beginning of a singly linked list Data Structures and Algorithms in Java 17
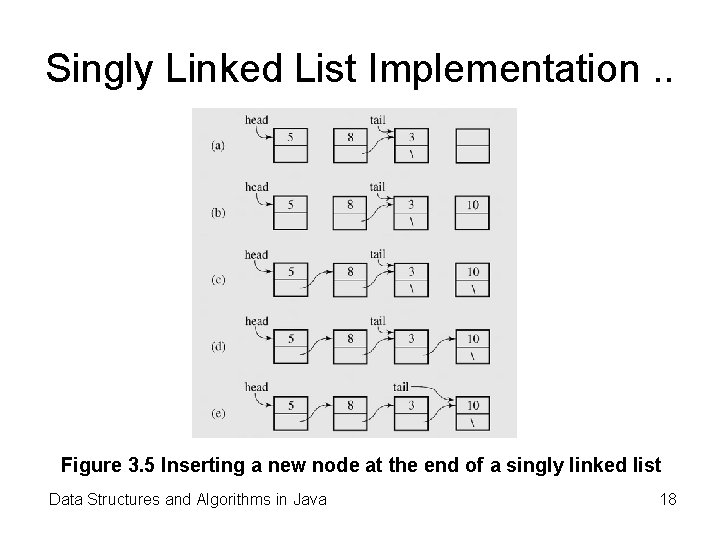
Singly Linked List Implementation. . Figure 3. 5 Inserting a new node at the end of a singly linked list Data Structures and Algorithms in Java 18
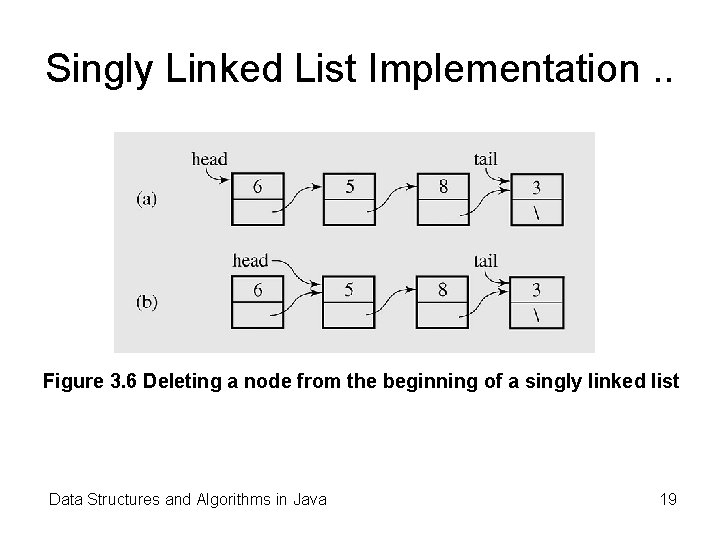
Singly Linked List Implementation. . Figure 3. 6 Deleting a node from the beginning of a singly linked list Data Structures and Algorithms in Java 19
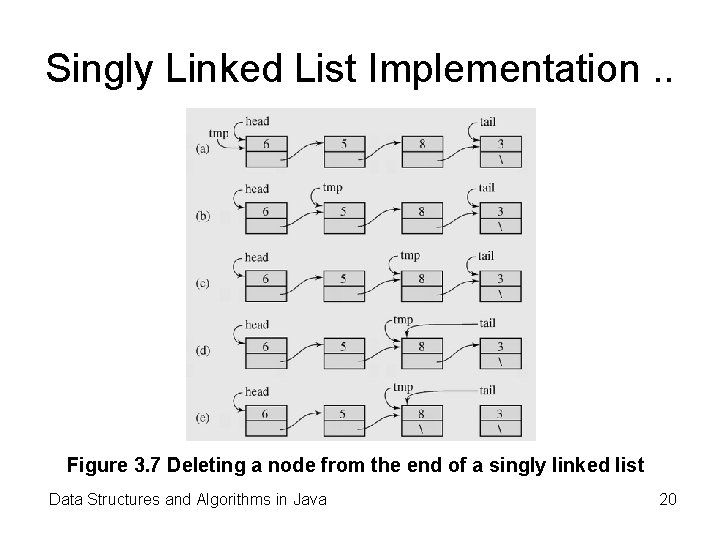
Singly Linked List Implementation. . Figure 3. 7 Deleting a node from the end of a singly linked list Data Structures and Algorithms in Java 20
Singly vs doubly linked list
Singly linked list vs doubly linked list
Java data structures
Singly vs doubly linked list
Algorithm of linked list
Polynomial addition using linked list
Disadvantages of doubly linked list
Singly linked list
Apa itu single linked list
Polynomial division using linked list in c
Singly reinforced beam
Single and double reinforcement beam
Homologous structure
Generic linked list java
Jenis linked list
Market basket analysis
Xor linked list c++
C++ list
Linked list in mips
Definition of linked list
Linked list uml