Circular Linked List Circular Linked List Circular Linked
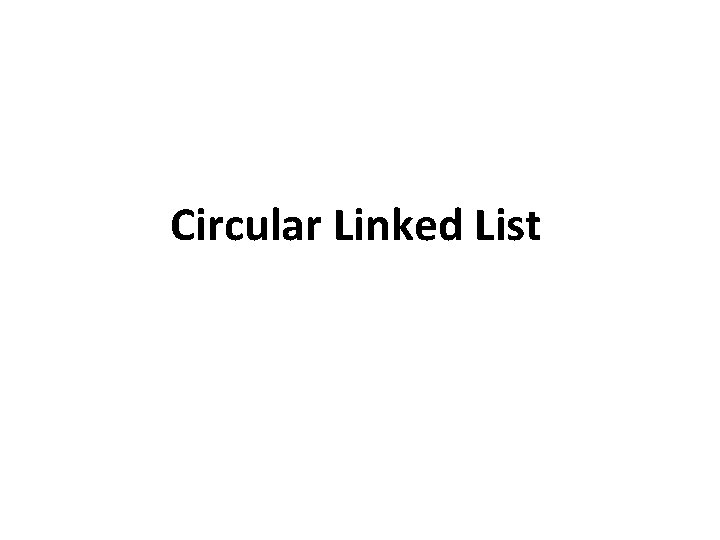
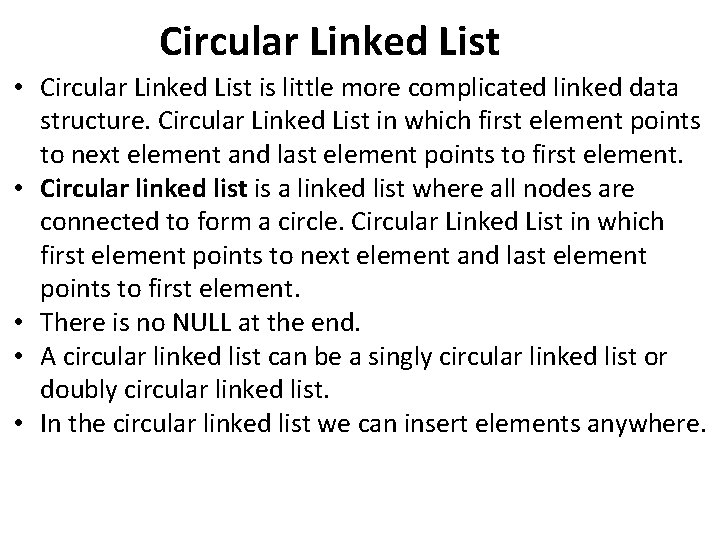
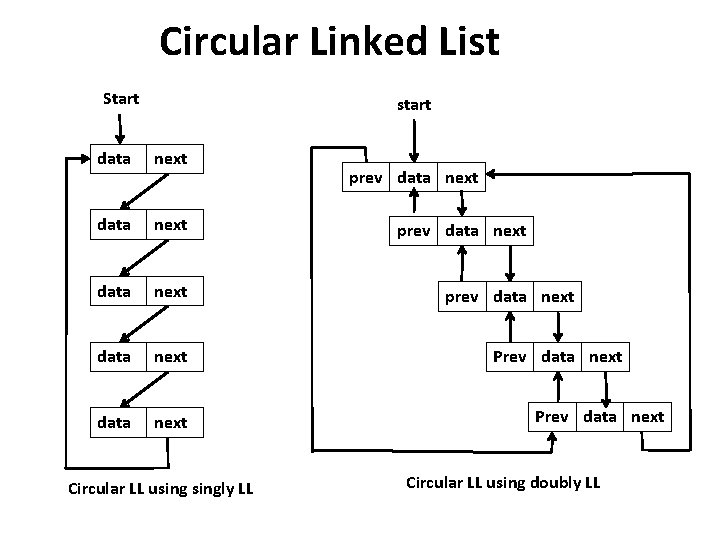
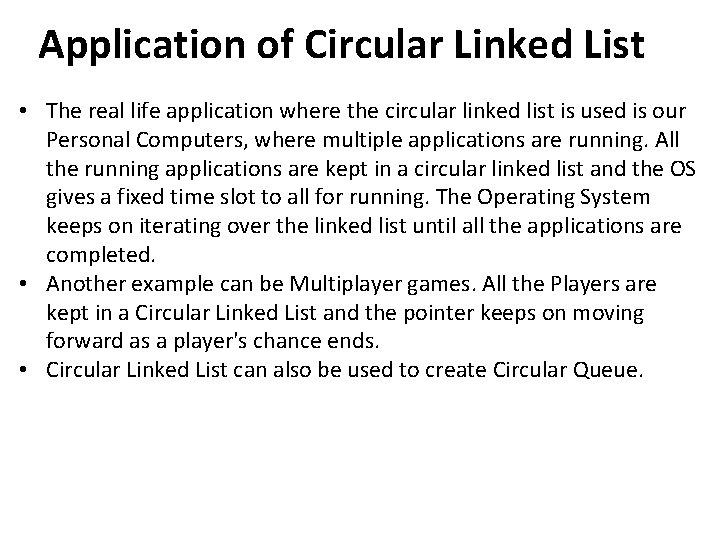
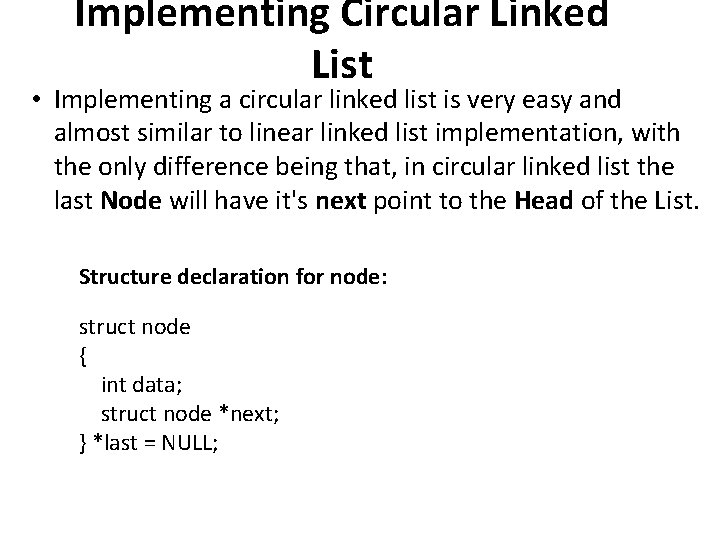
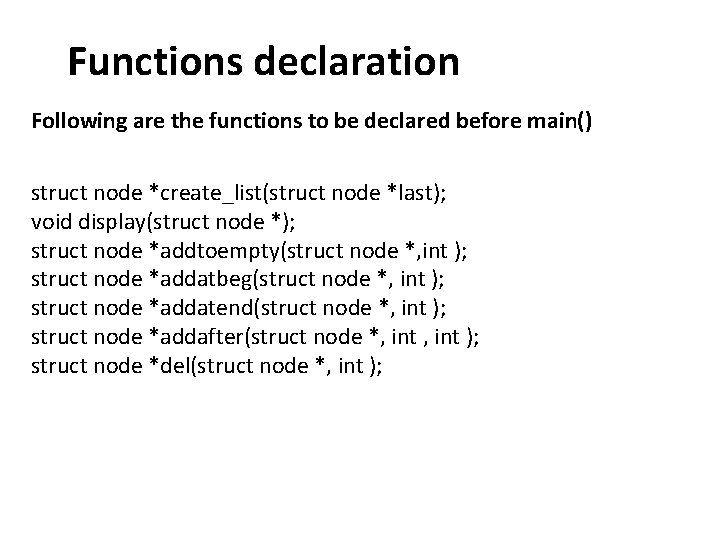
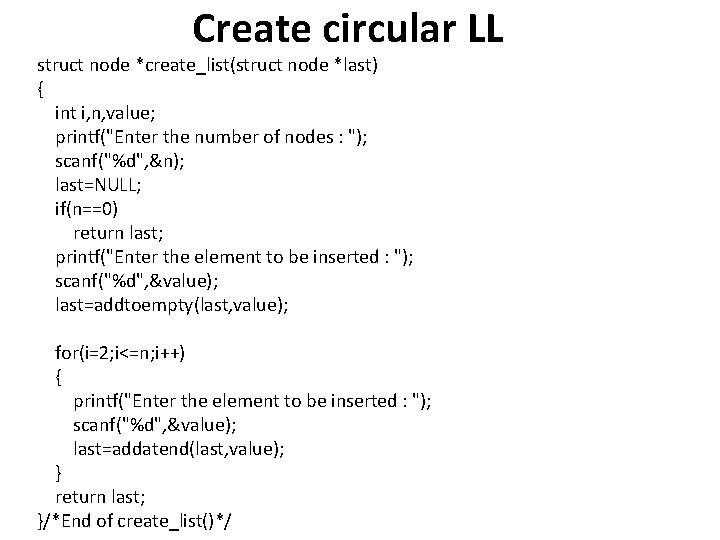
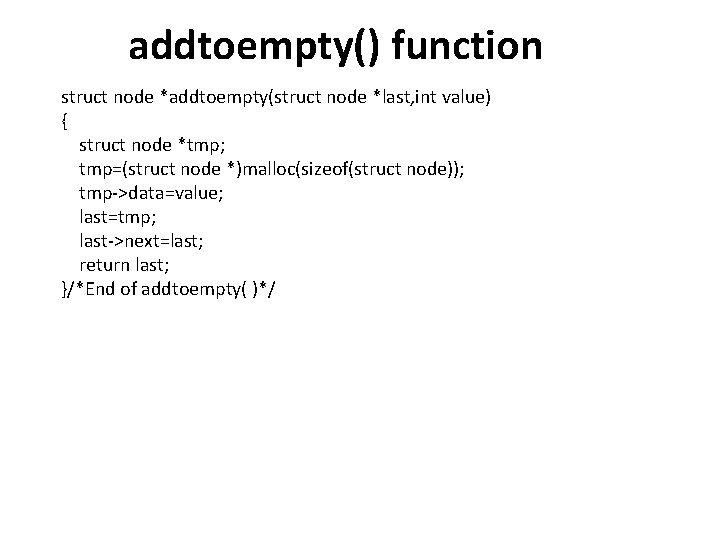
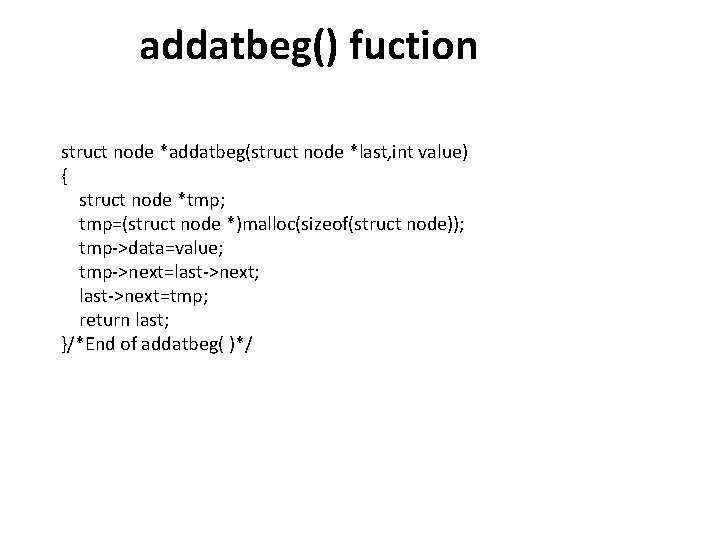
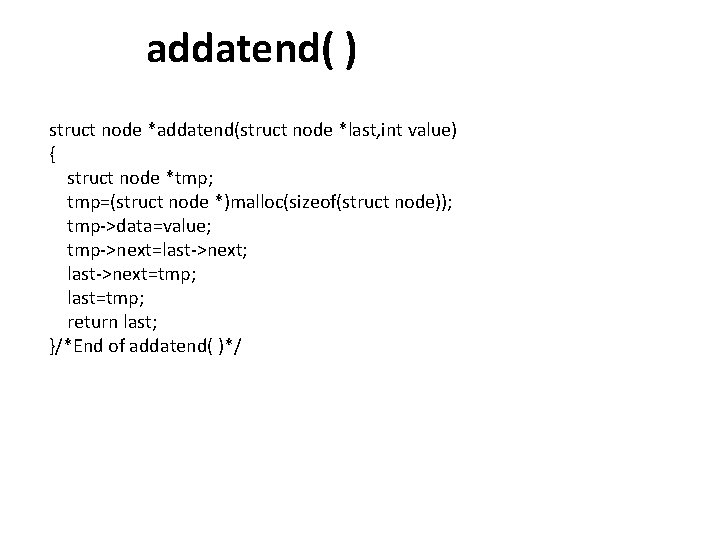
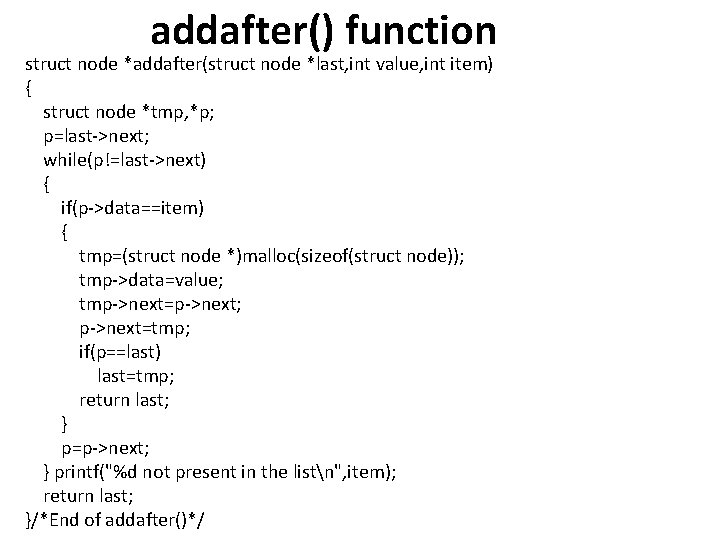
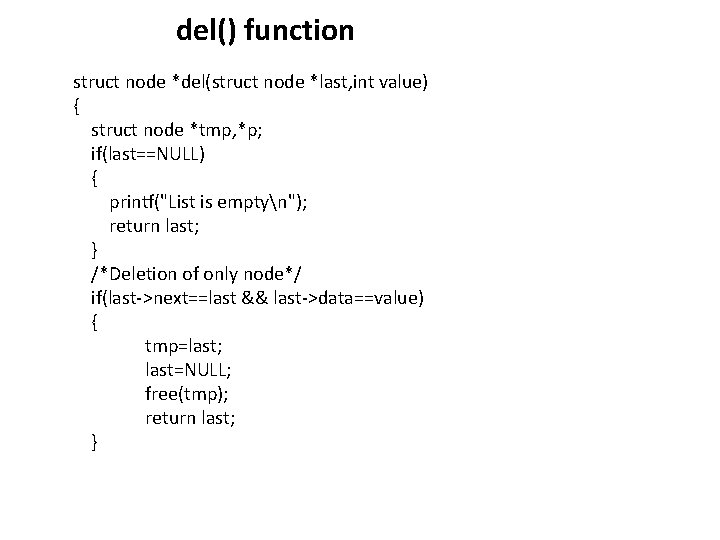
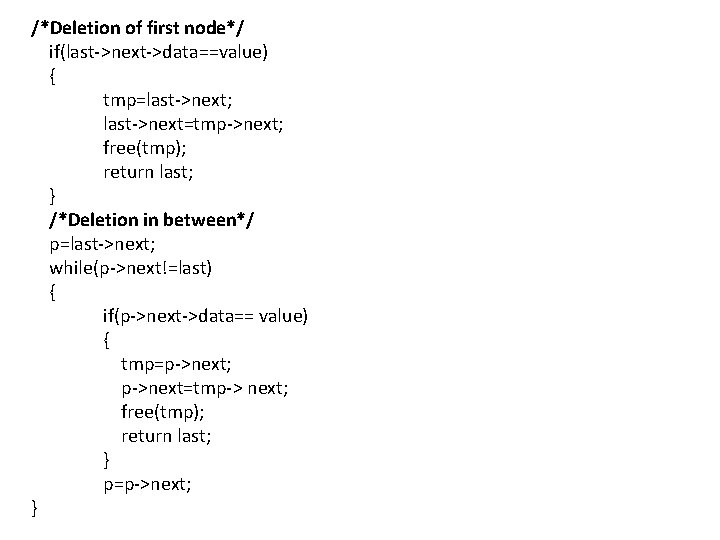
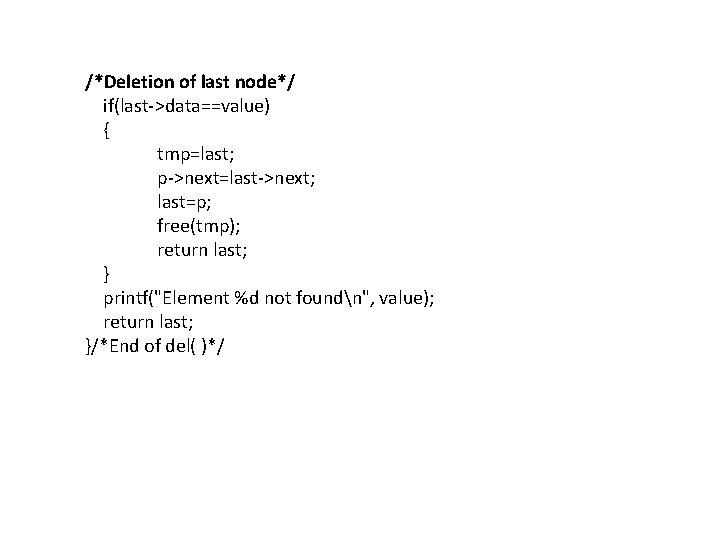
- Slides: 14
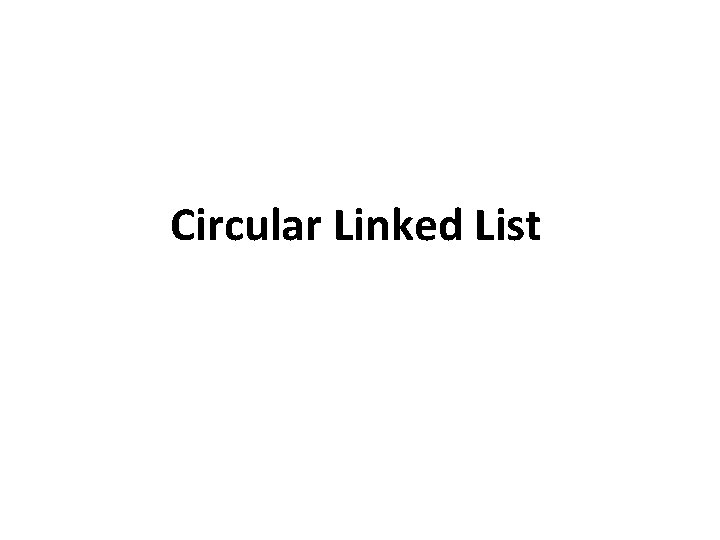
Circular Linked List
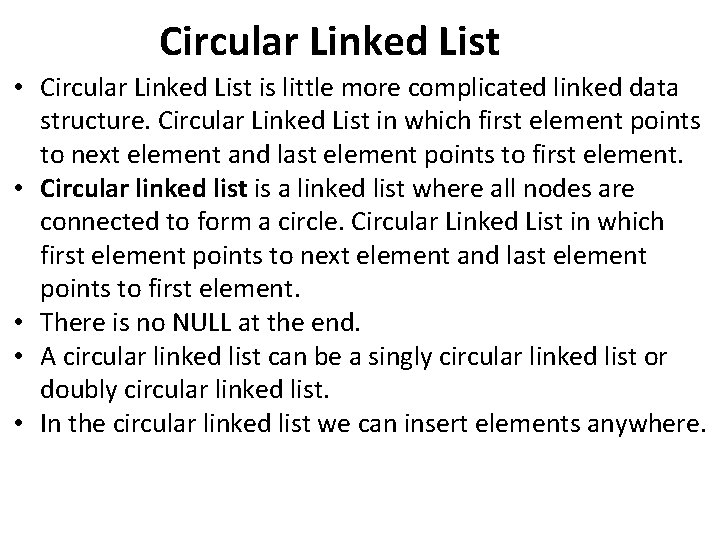
Circular Linked List • Circular Linked List is little more complicated linked data structure. Circular Linked List in which first element points to next element and last element points to first element. • Circular linked list is a linked list where all nodes are connected to form a circle. Circular Linked List in which first element points to next element and last element points to first element. • There is no NULL at the end. • A circular linked list can be a singly circular linked list or doubly circular linked list. • In the circular linked list we can insert elements anywhere.
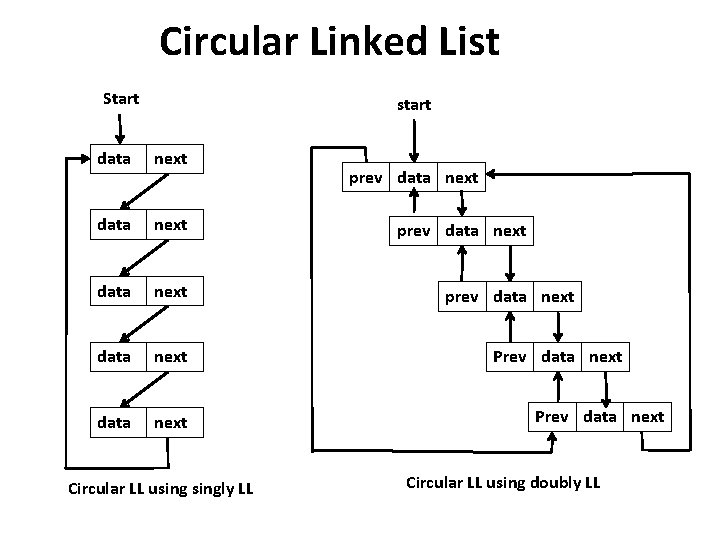
Circular Linked List Start start data next data next Circular LL usingly LL prev data next Prev data next Circular LL using doubly LL
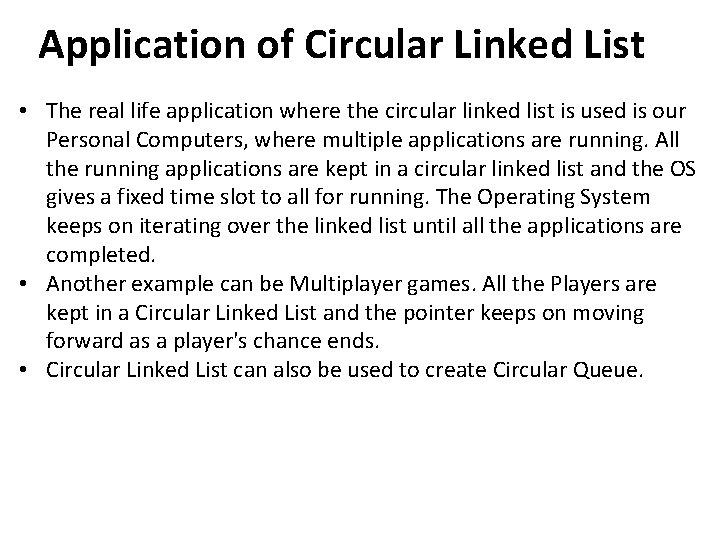
Application of Circular Linked List • The real life application where the circular linked list is used is our Personal Computers, where multiple applications are running. All the running applications are kept in a circular linked list and the OS gives a fixed time slot to all for running. The Operating System keeps on iterating over the linked list until all the applications are completed. • Another example can be Multiplayer games. All the Players are kept in a Circular Linked List and the pointer keeps on moving forward as a player's chance ends. • Circular Linked List can also be used to create Circular Queue.
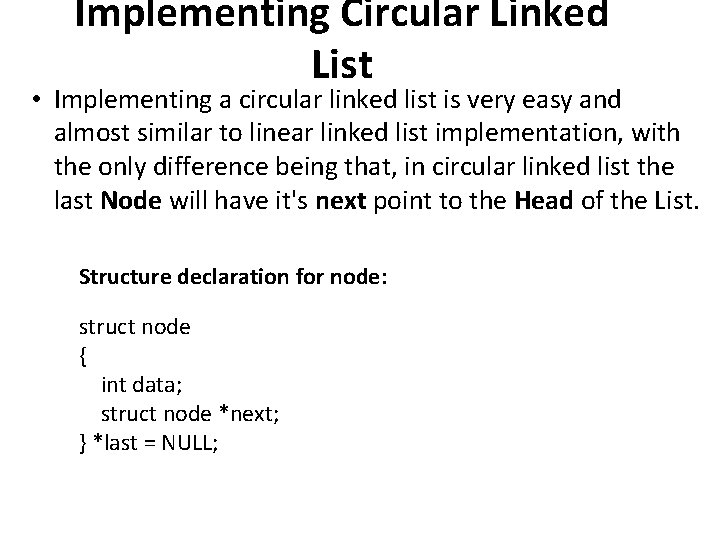
Implementing Circular Linked List • Implementing a circular linked list is very easy and almost similar to linear linked list implementation, with the only difference being that, in circular linked list the last Node will have it's next point to the Head of the List. Structure declaration for node: struct node { int data; struct node *next; } *last = NULL;
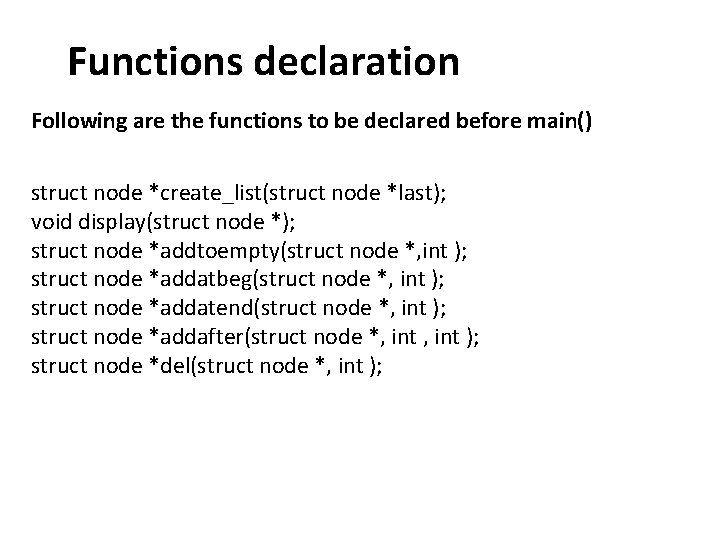
Functions declaration Following are the functions to be declared before main() struct node *create_list(struct node *last); void display(struct node *); struct node *addtoempty(struct node *, int ); struct node *addatbeg(struct node *, int ); struct node *addatend(struct node *, int ); struct node *addafter(struct node *, int ); struct node *del(struct node *, int );
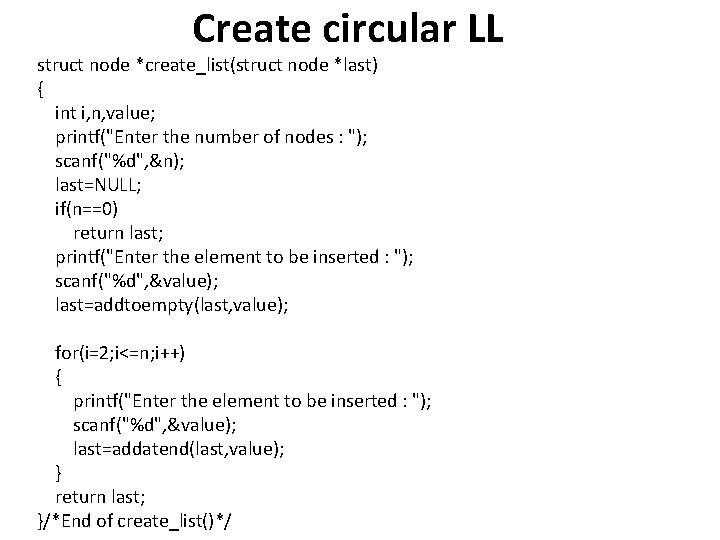
Create circular LL struct node *create_list(struct node *last) { int i, n, value; printf("Enter the number of nodes : "); scanf("%d", &n); last=NULL; if(n==0) return last; printf("Enter the element to be inserted : "); scanf("%d", &value); last=addtoempty(last, value); for(i=2; i<=n; i++) { printf("Enter the element to be inserted : "); scanf("%d", &value); last=addatend(last, value); } return last; }/*End of create_list()*/
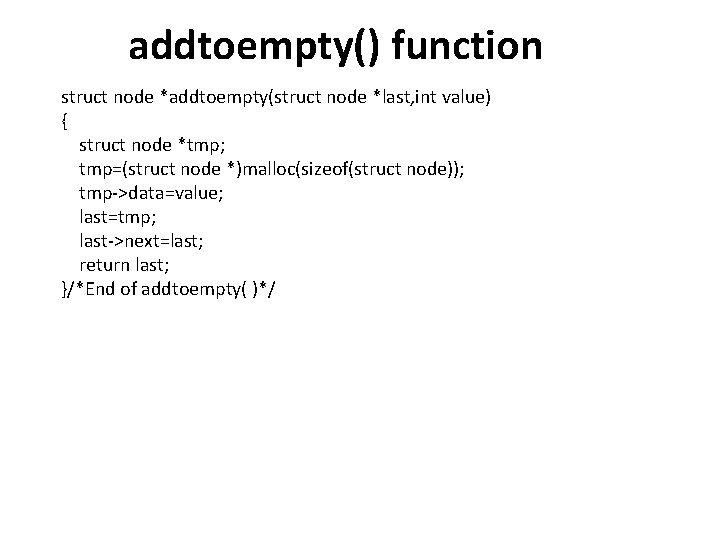
addtoempty() function struct node *addtoempty(struct node *last, int value) { struct node *tmp; tmp=(struct node *)malloc(sizeof(struct node)); tmp->data=value; last=tmp; last->next=last; return last; }/*End of addtoempty( )*/
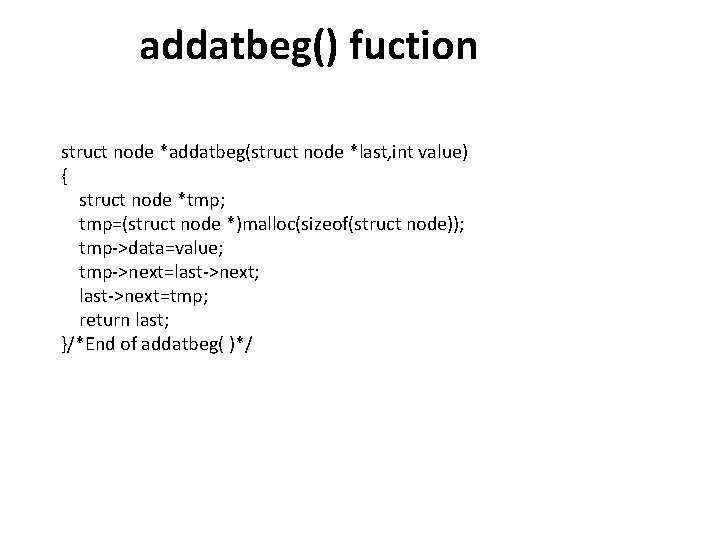
addatbeg() fuction struct node *addatbeg(struct node *last, int value) { struct node *tmp; tmp=(struct node *)malloc(sizeof(struct node)); tmp->data=value; tmp->next=last->next; last->next=tmp; return last; }/*End of addatbeg( )*/
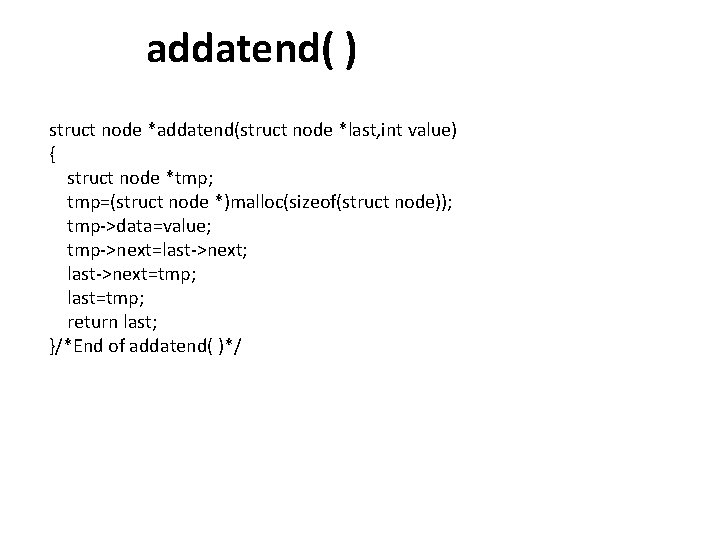
addatend( ) struct node *addatend(struct node *last, int value) { struct node *tmp; tmp=(struct node *)malloc(sizeof(struct node)); tmp->data=value; tmp->next=last->next; last->next=tmp; last=tmp; return last; }/*End of addatend( )*/
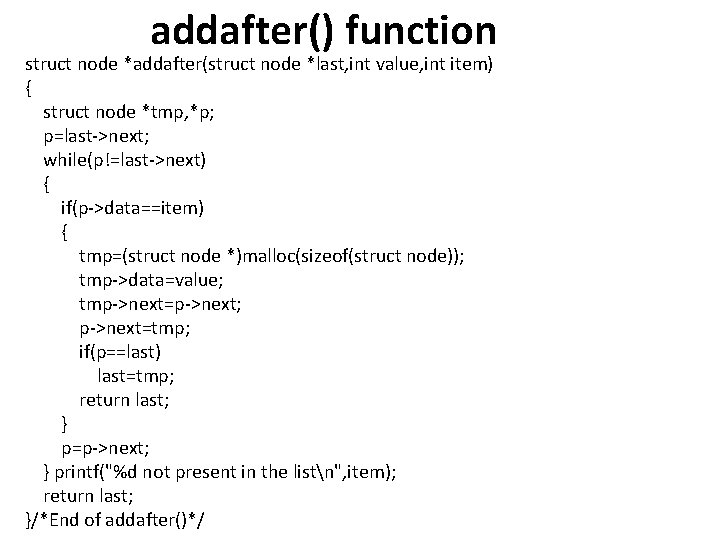
addafter() function struct node *addafter(struct node *last, int value, int item) { struct node *tmp, *p; p=last->next; while(p!=last->next) { if(p->data==item) { tmp=(struct node *)malloc(sizeof(struct node)); tmp->data=value; tmp->next=p->next; p->next=tmp; if(p==last) last=tmp; return last; } p=p->next; } printf("%d not present in the listn", item); return last; }/*End of addafter()*/
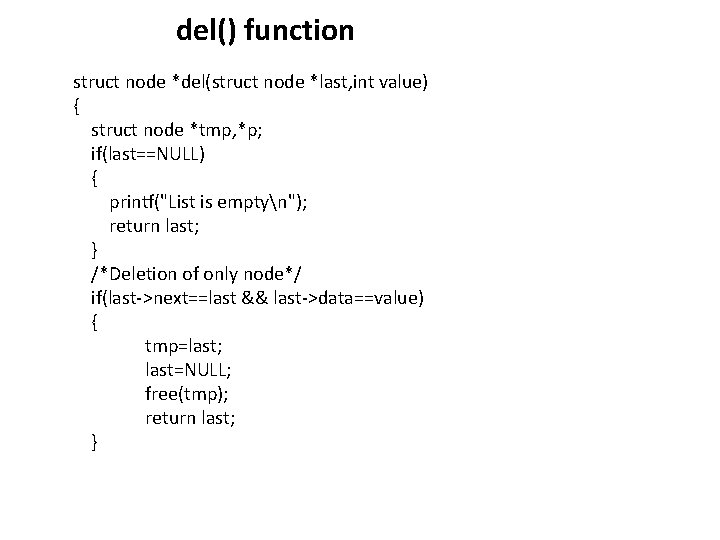
del() function struct node *del(struct node *last, int value) { struct node *tmp, *p; if(last==NULL) { printf("List is emptyn"); return last; } /*Deletion of only node*/ if(last->next==last && last->data==value) { tmp=last; last=NULL; free(tmp); return last; }
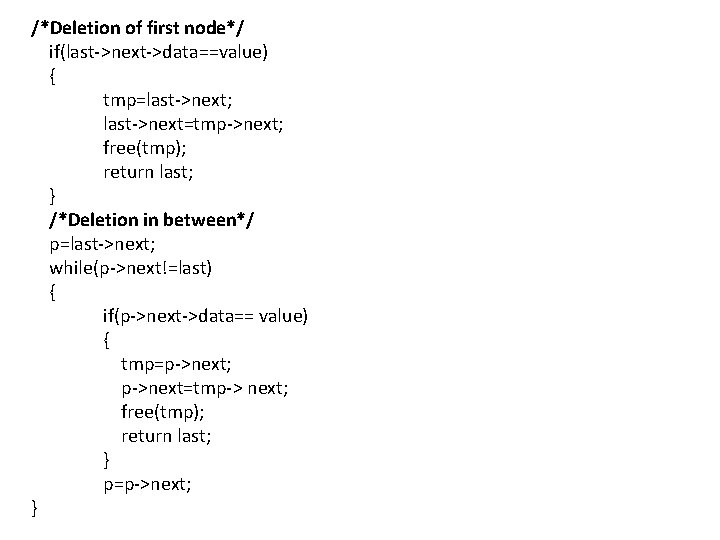
/*Deletion of first node*/ if(last->next->data==value) { tmp=last->next; last->next=tmp->next; free(tmp); return last; } /*Deletion in between*/ p=last->next; while(p->next!=last) { if(p->next->data== value) { tmp=p->next; p->next=tmp-> next; free(tmp); return last; } p=p->next; }
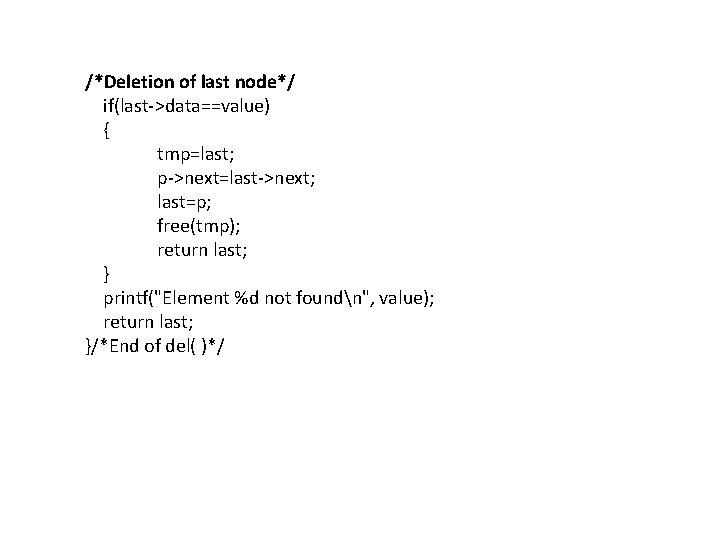
/*Deletion of last node*/ if(last->data==value) { tmp=last; p->next=last->next; last=p; free(tmp); return last; } printf("Element %d not foundn", value); return last; }/*End of del( )*/
In linked list the successive elements
Introduction to linked list
Perbedaan single linked list dan double linked list
Circular linked list with header node
Circular single linked list adalah
Disadvantages of doubly linked list
Single linked list non circular
Advantages of circular linked list
Linked list
Linked list big o
Apa yang dimaksud dengan linked list
Dynamic linked list
Xor linked list c++
Linked list pros and cons
Lock free doubly linked list