Data Structures for Java William H Ford William
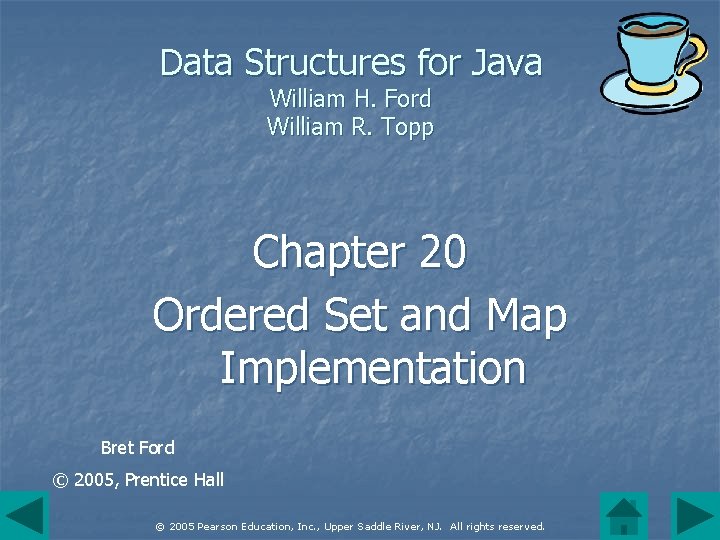
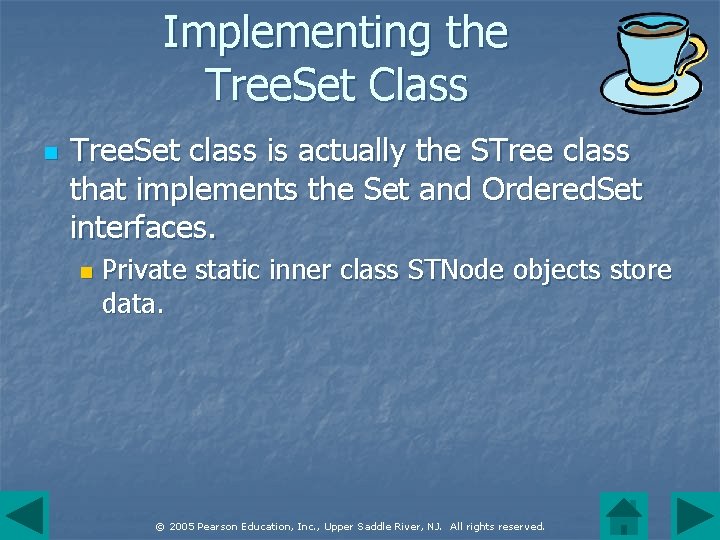
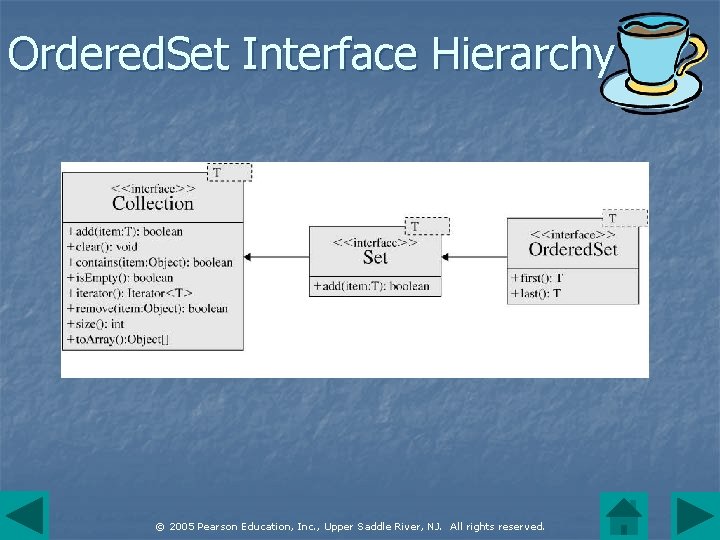
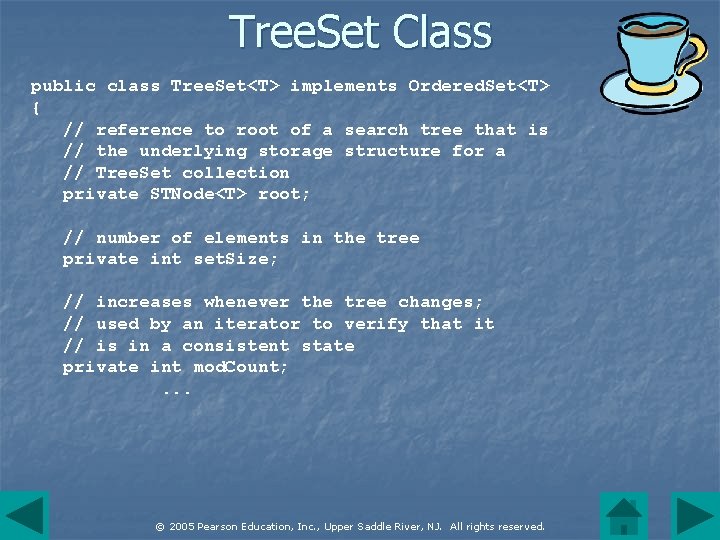
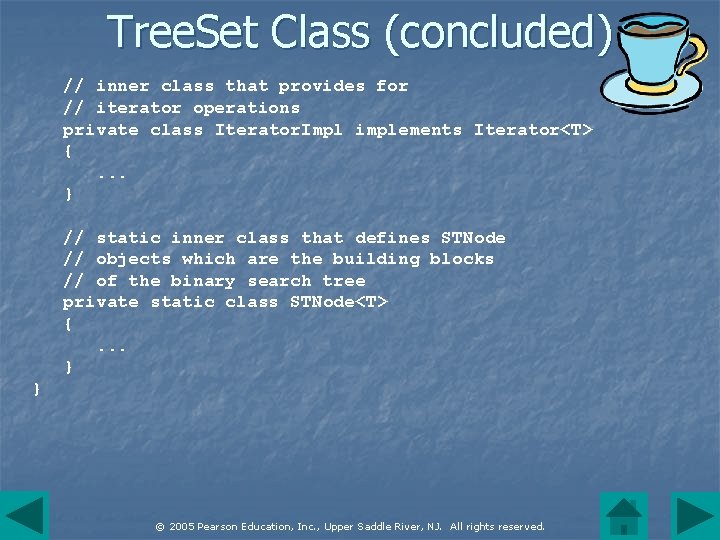
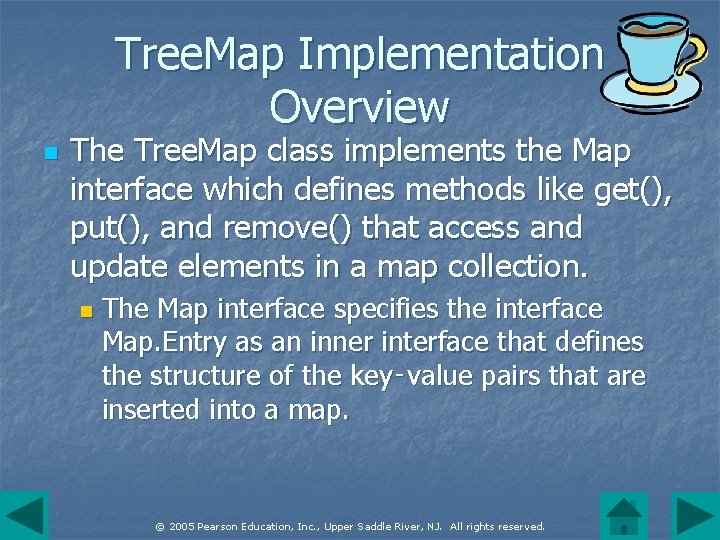
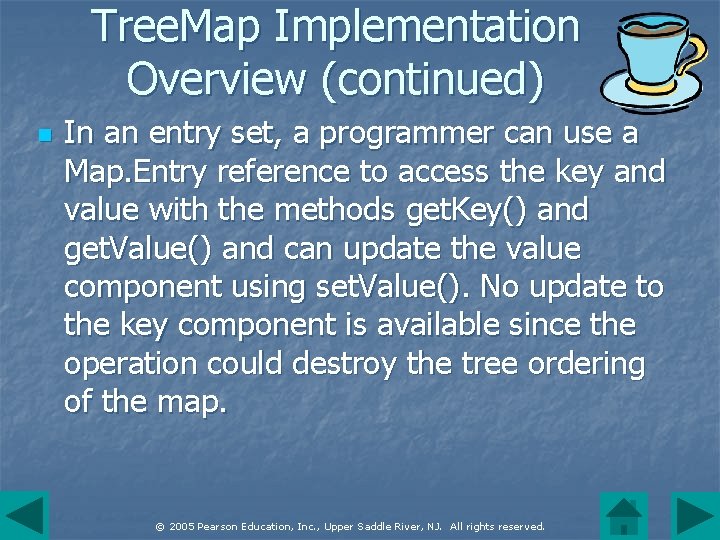
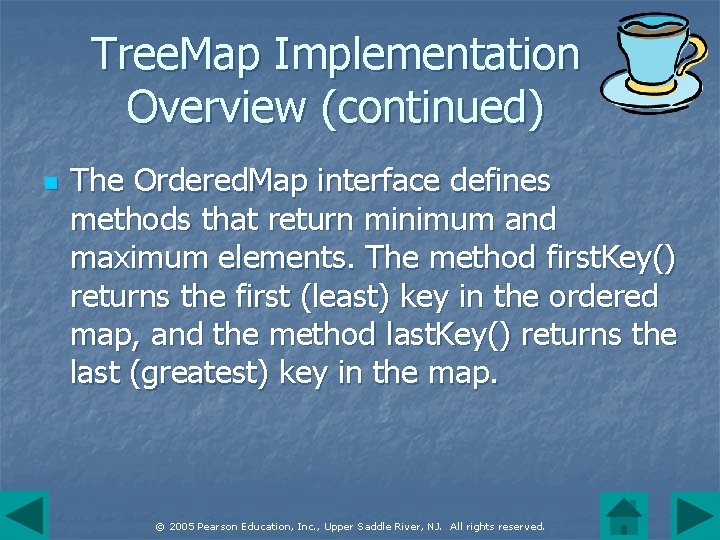
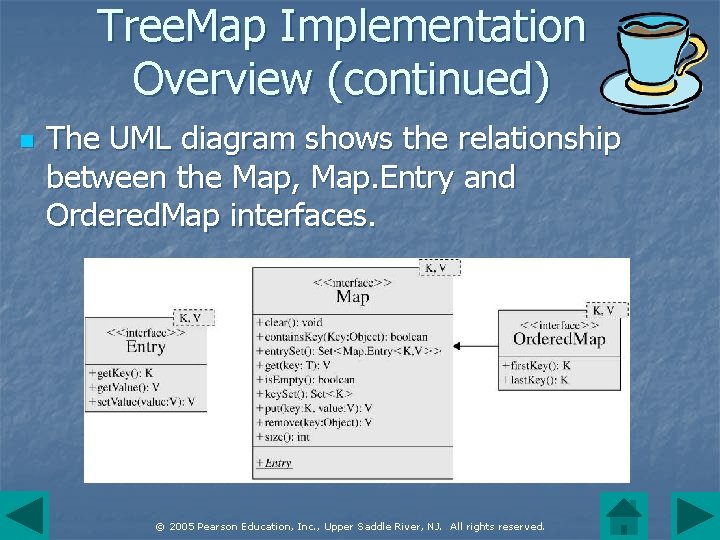
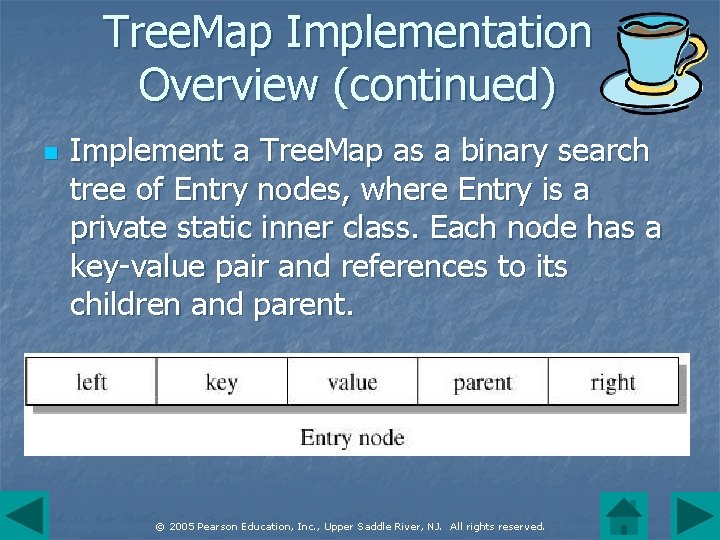
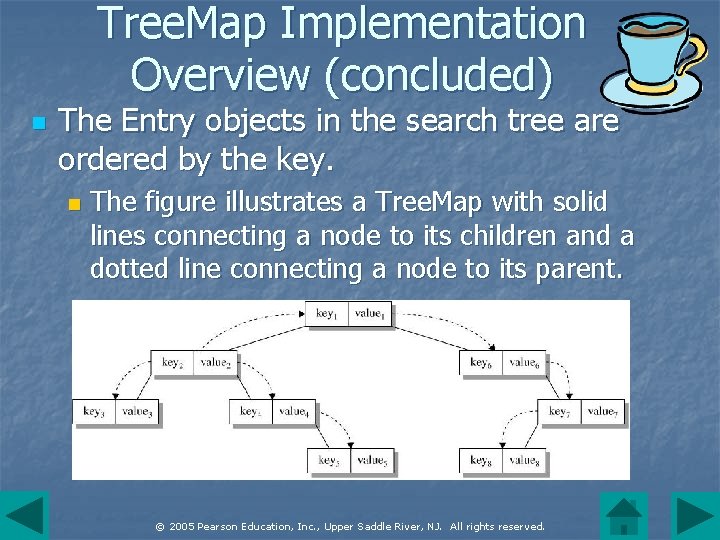
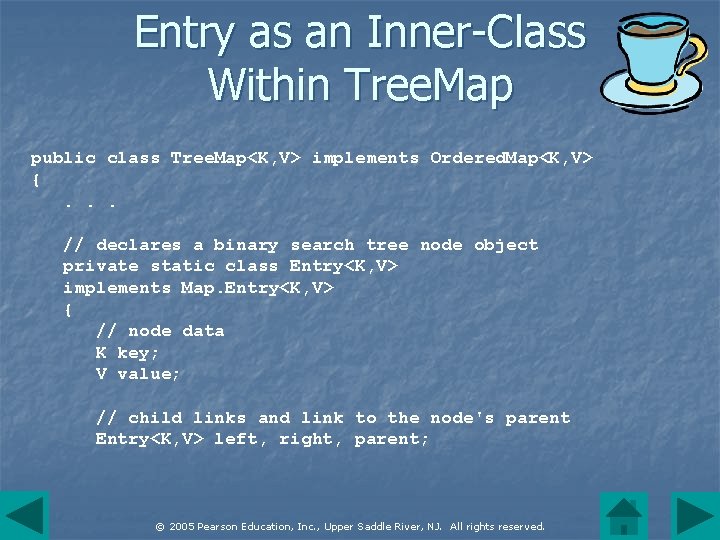
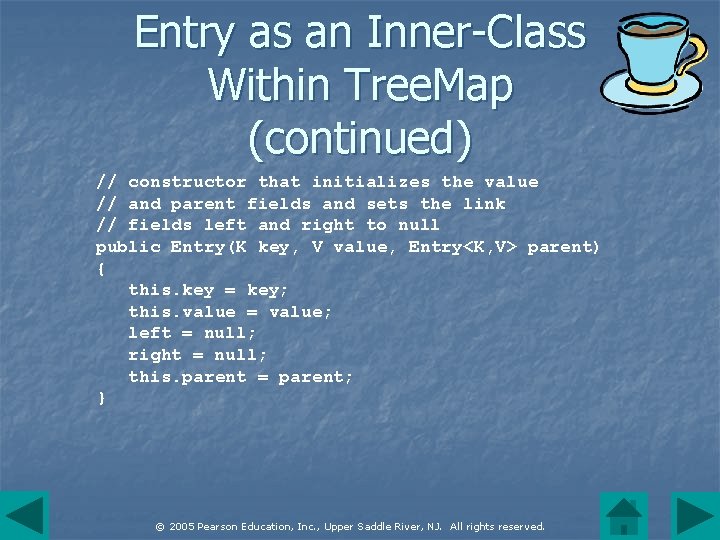
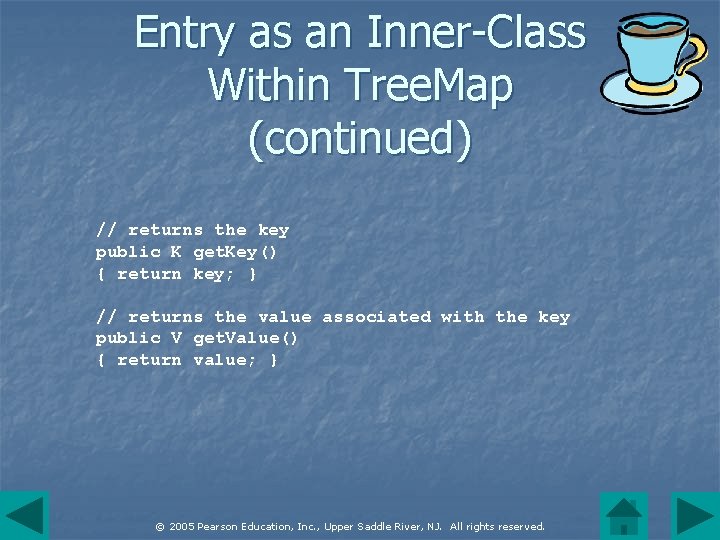
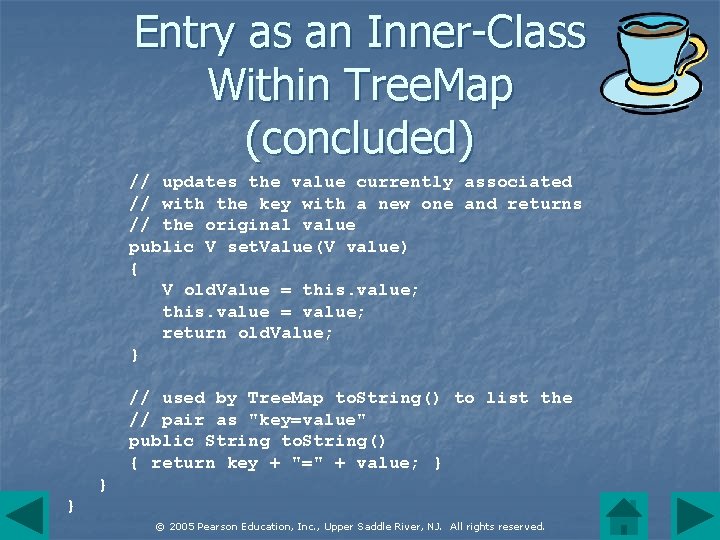
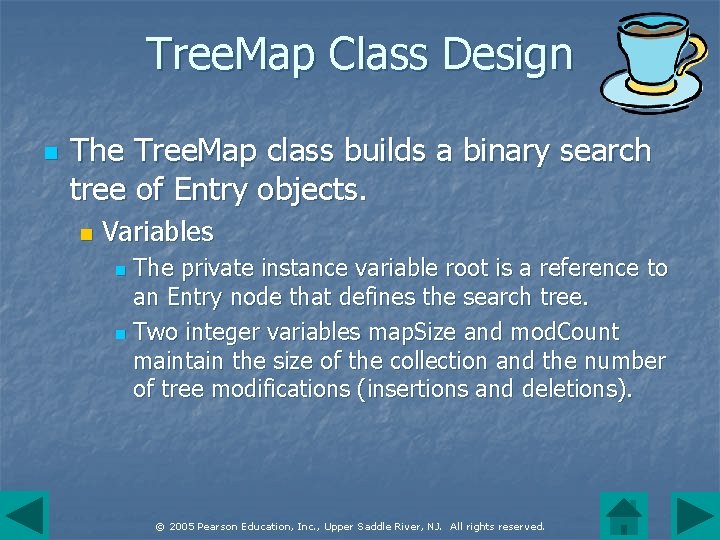
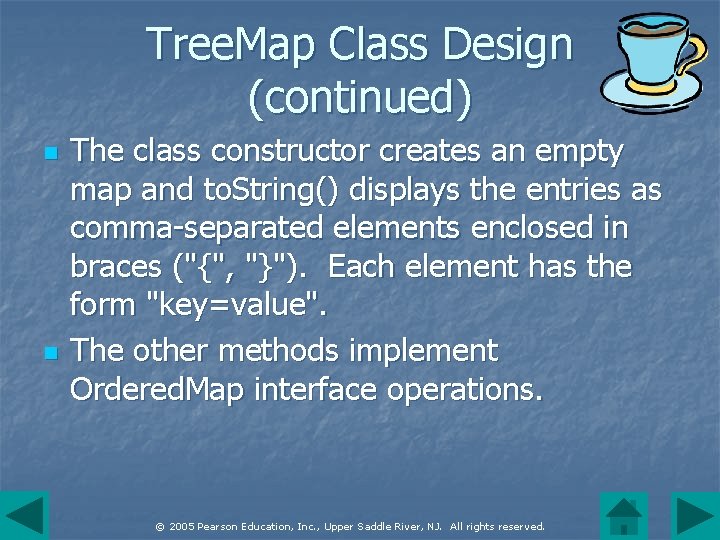
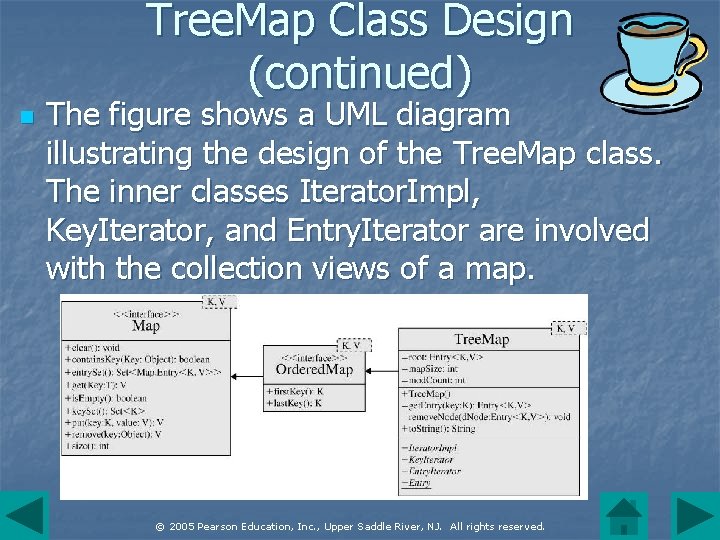
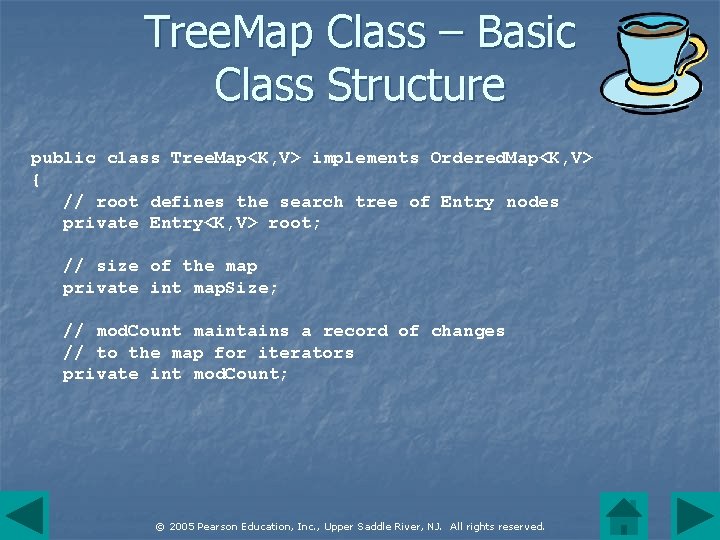
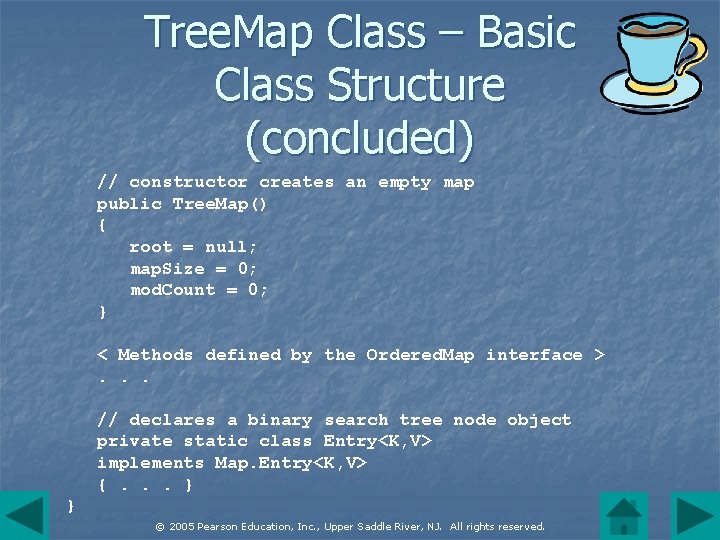
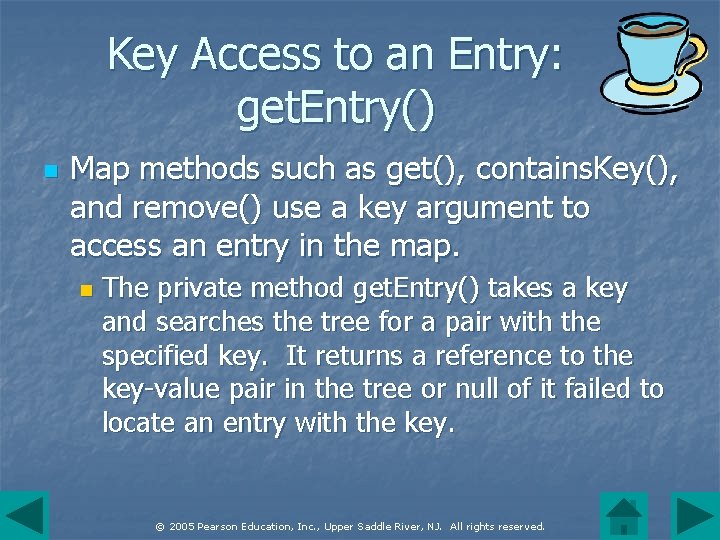
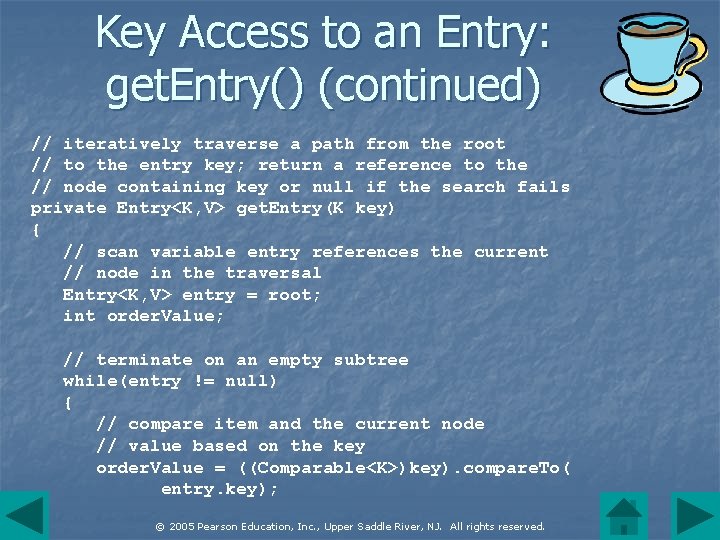
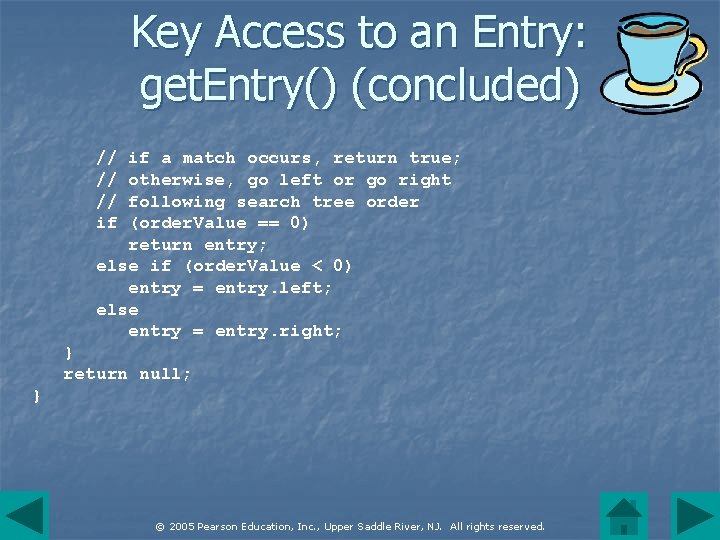
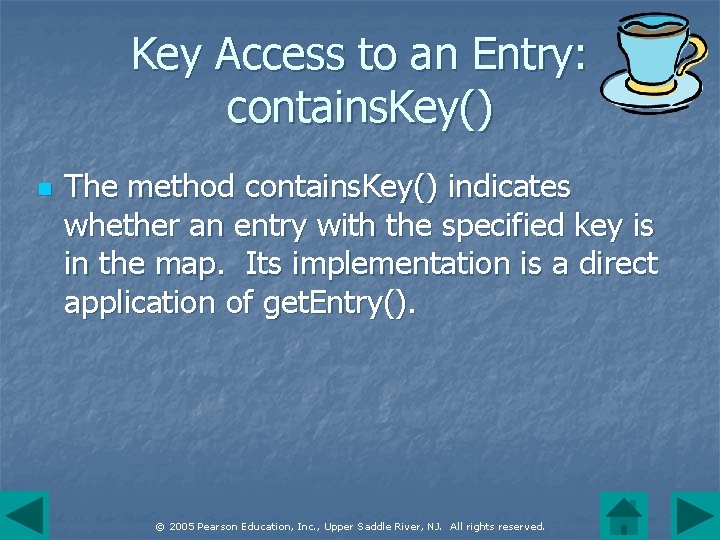
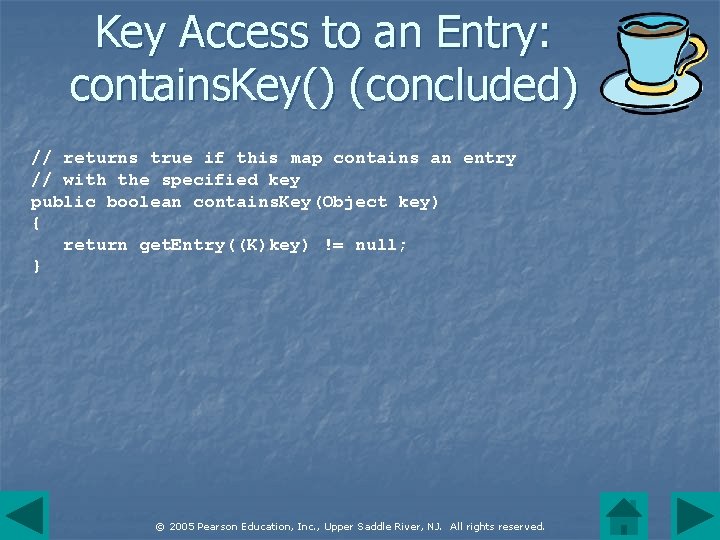
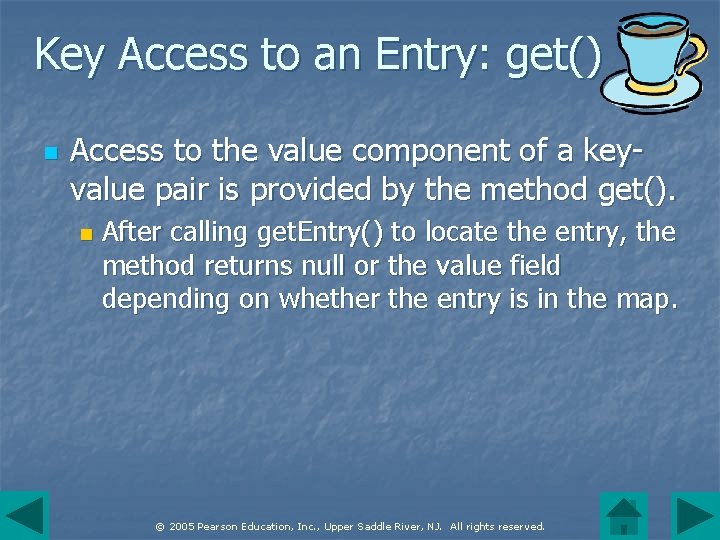
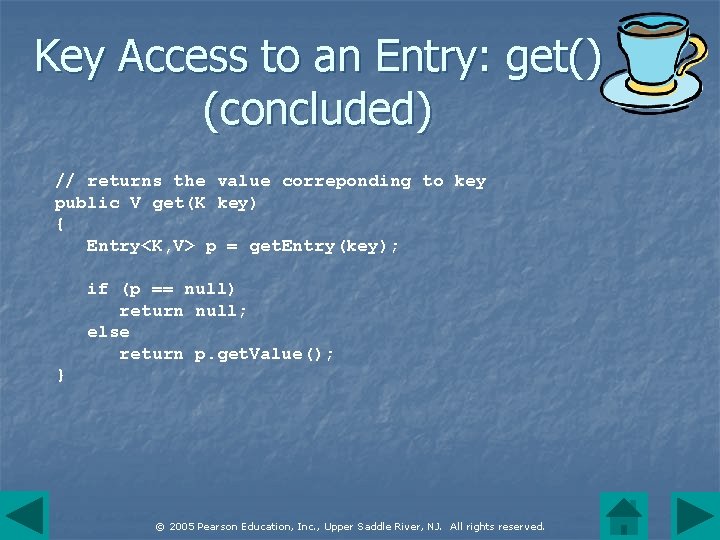
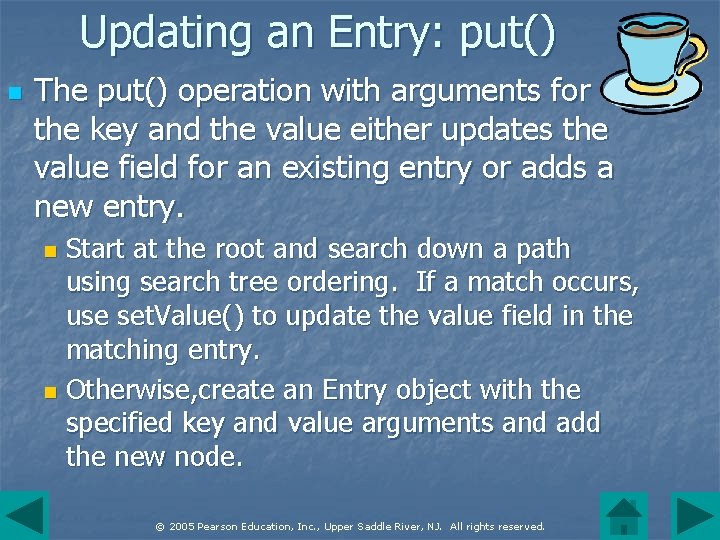
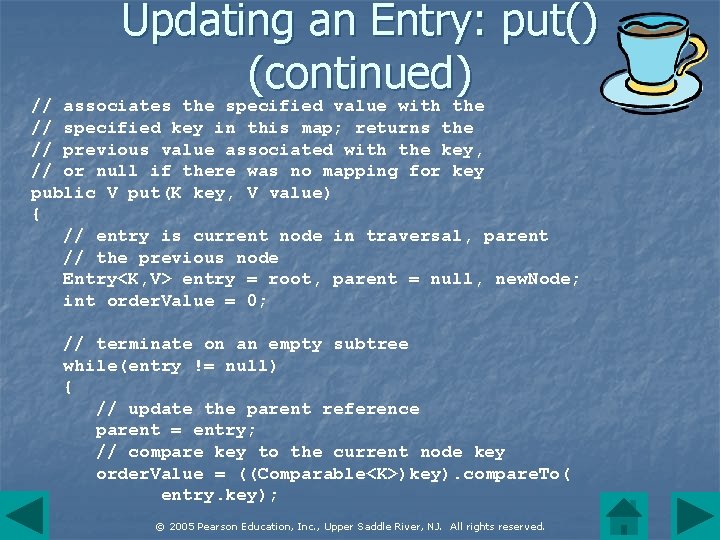
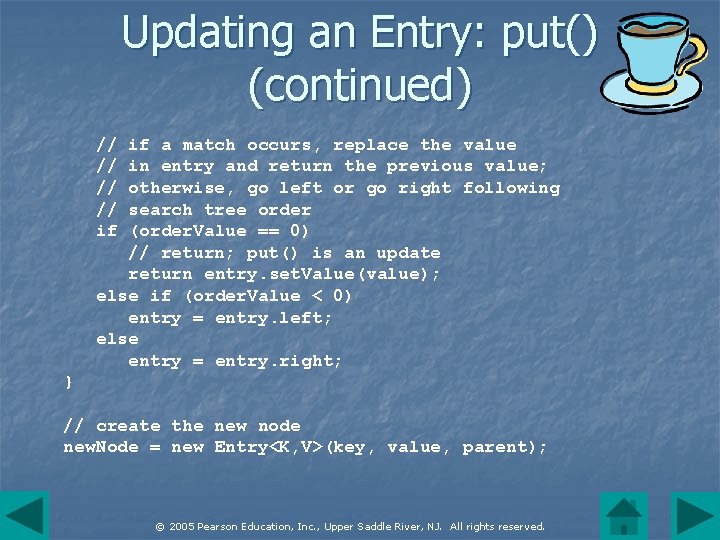
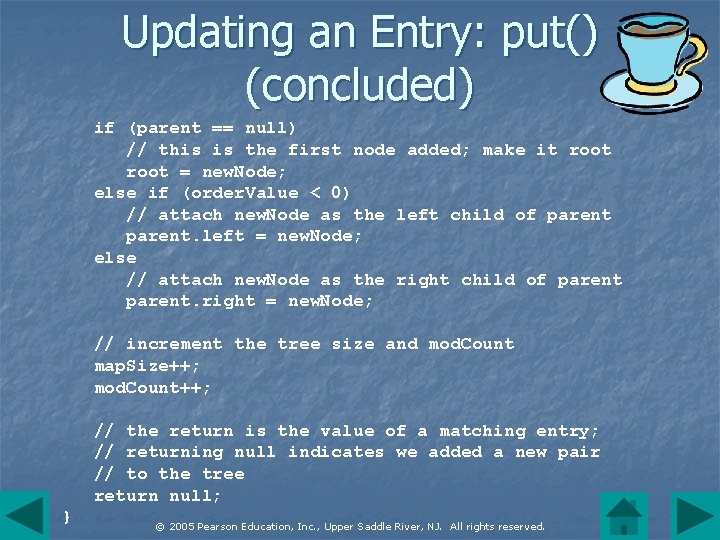
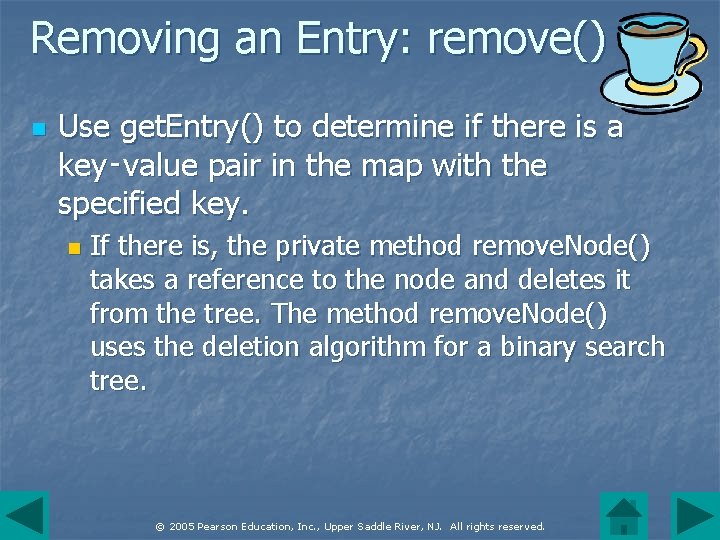
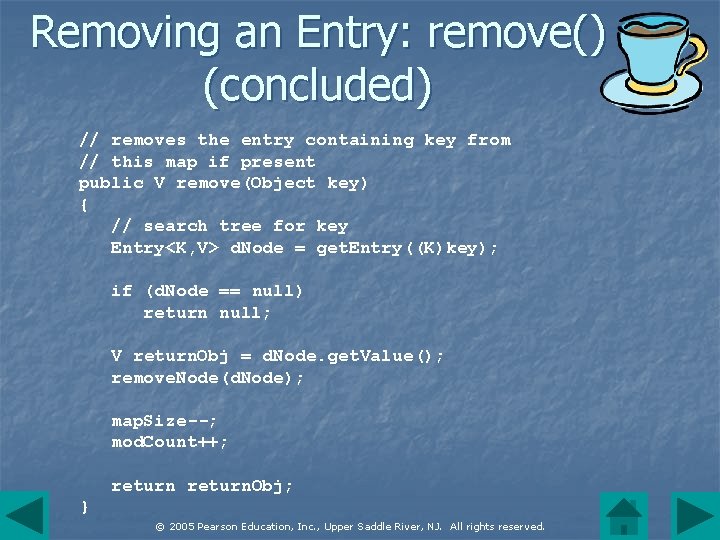
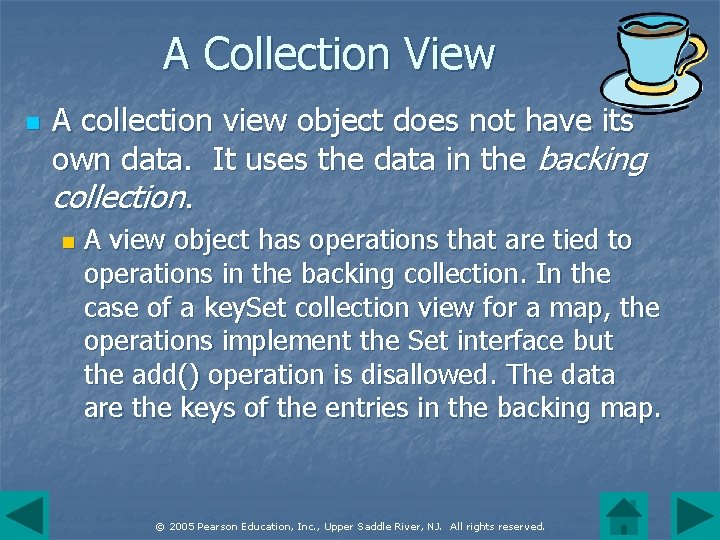
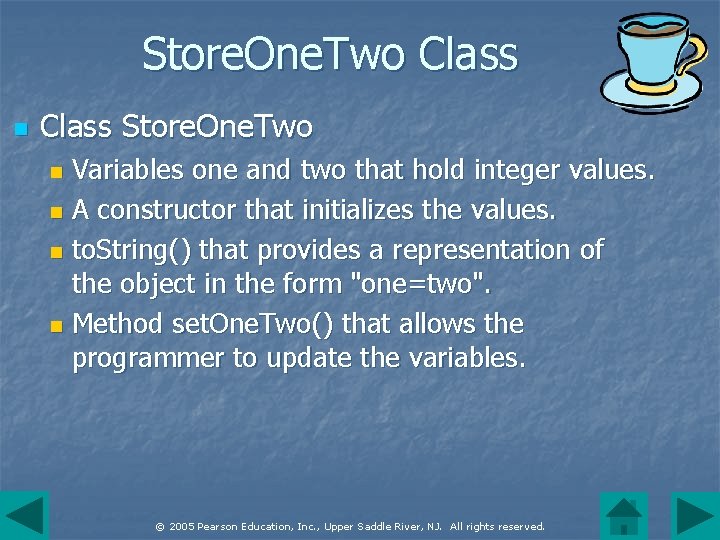
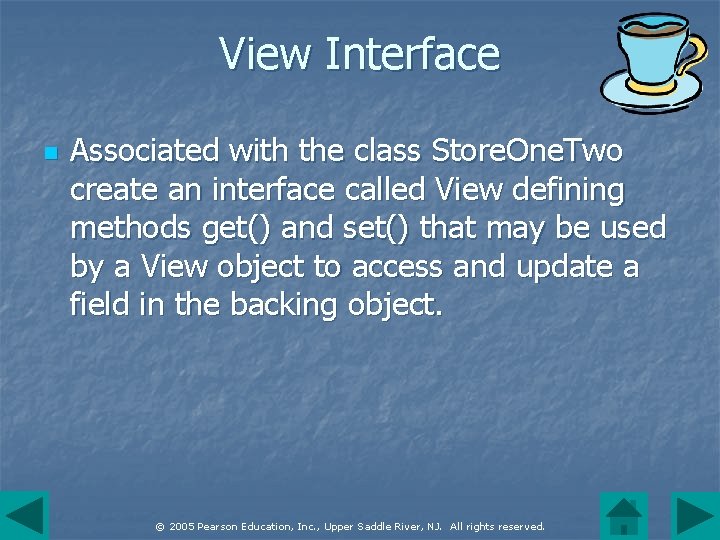
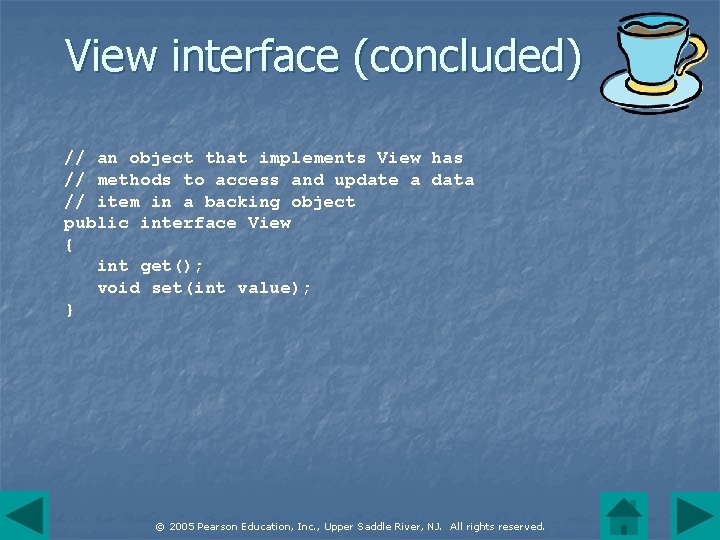
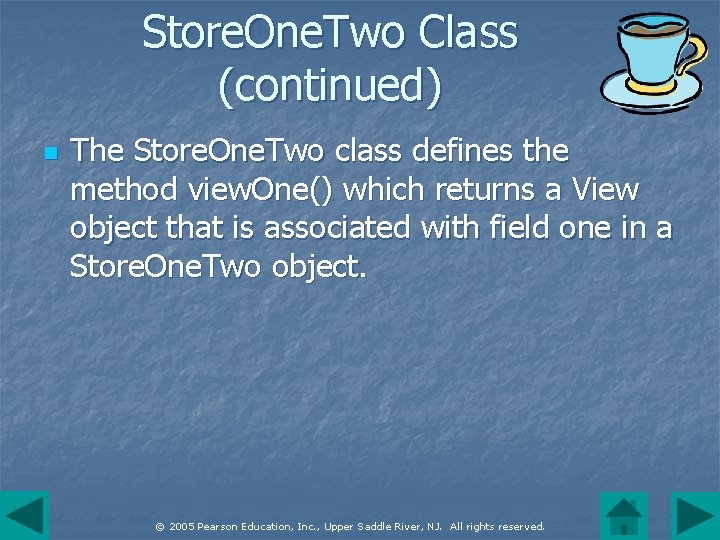
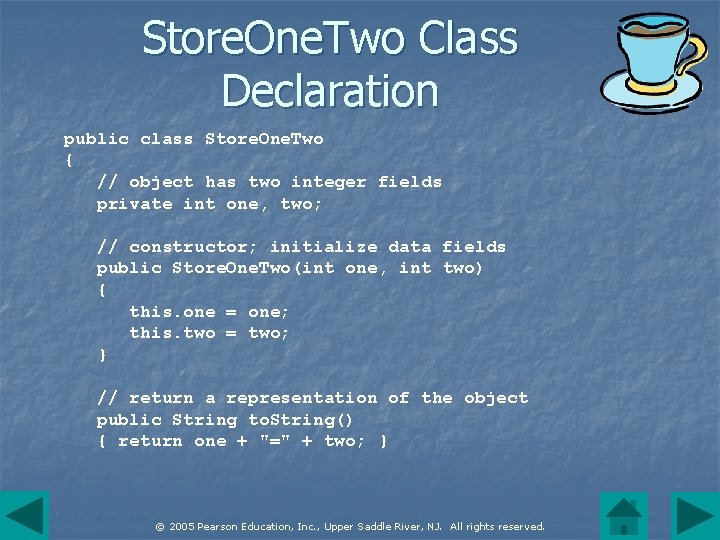
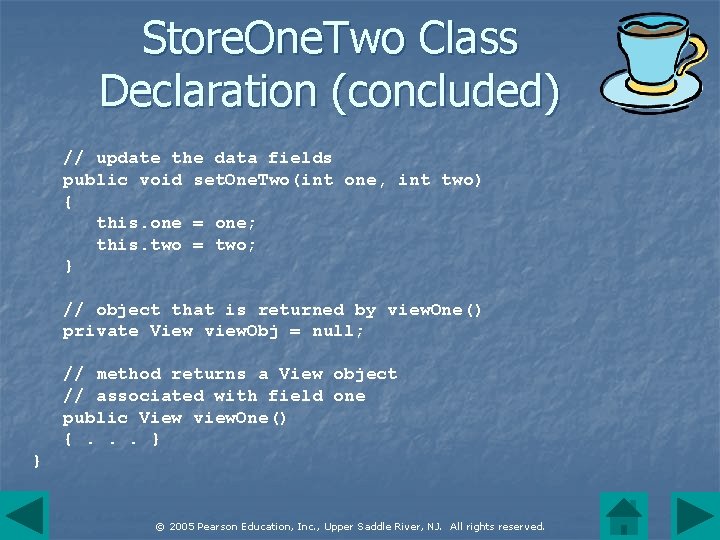
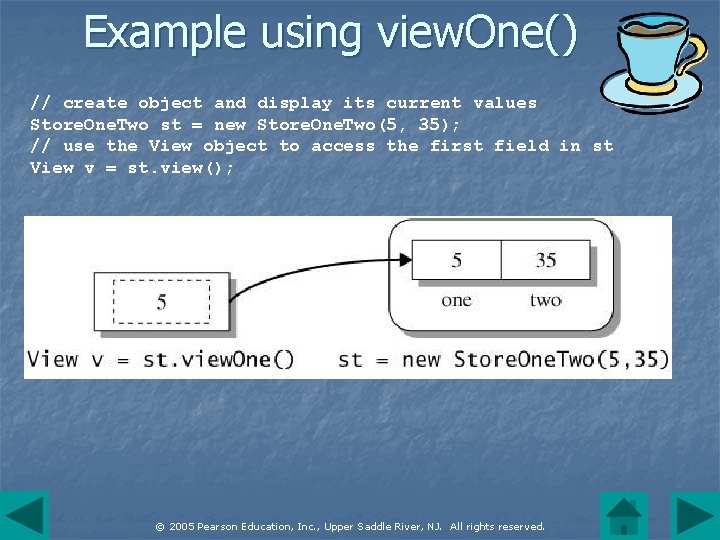
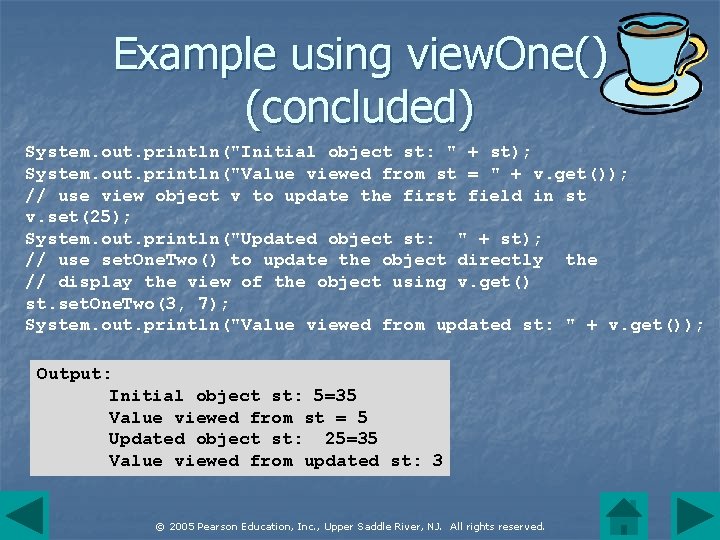
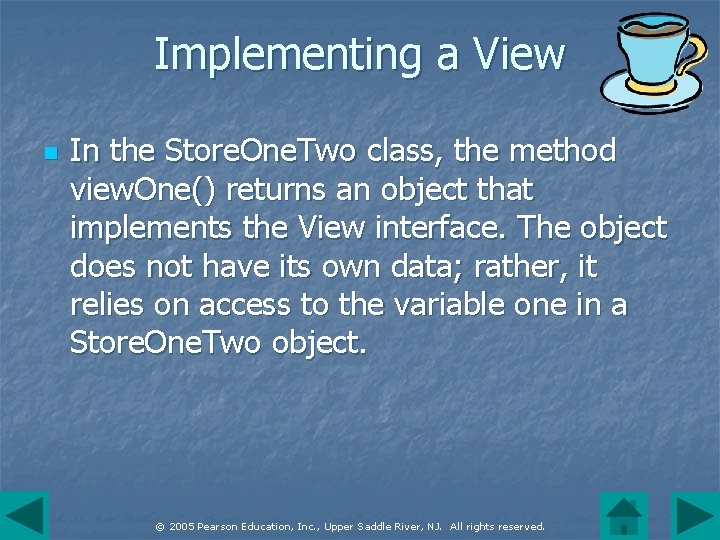
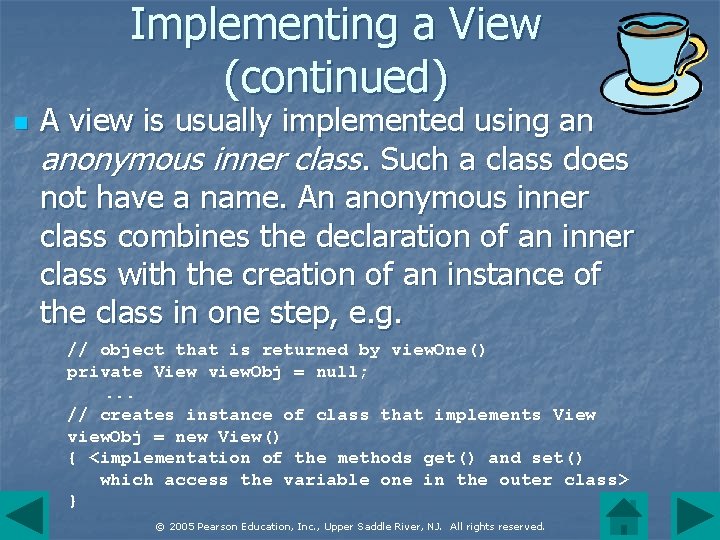
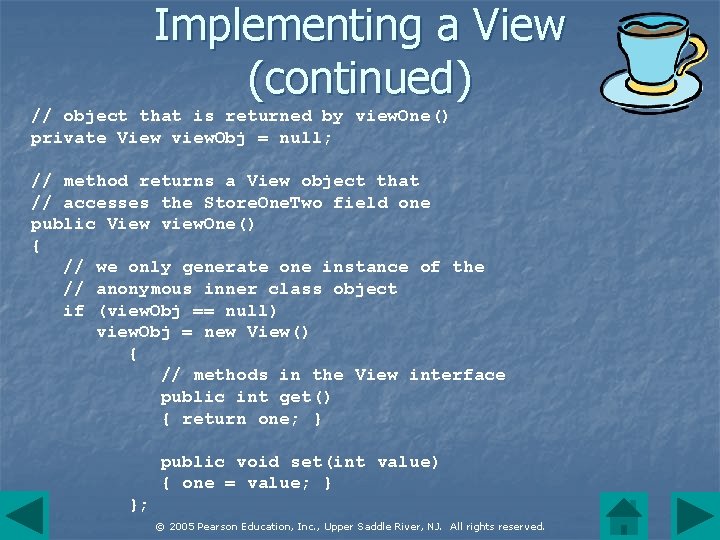
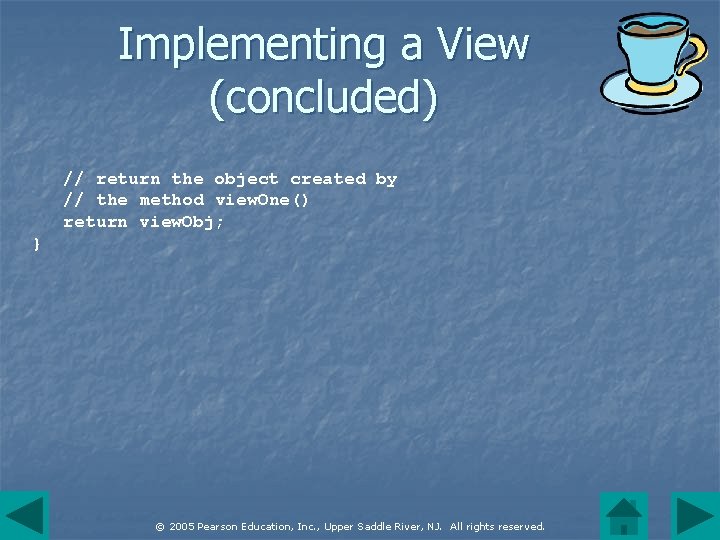
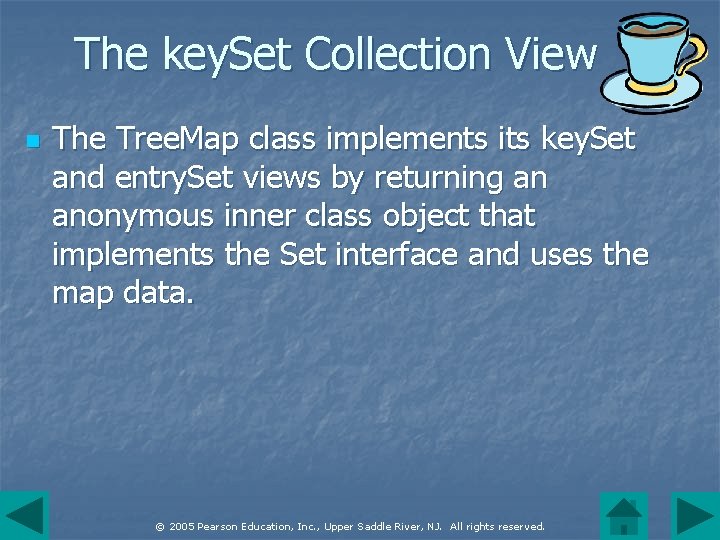
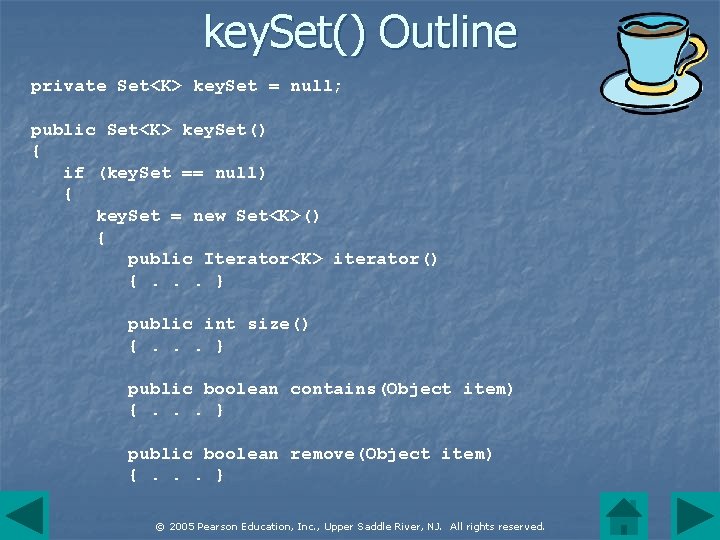
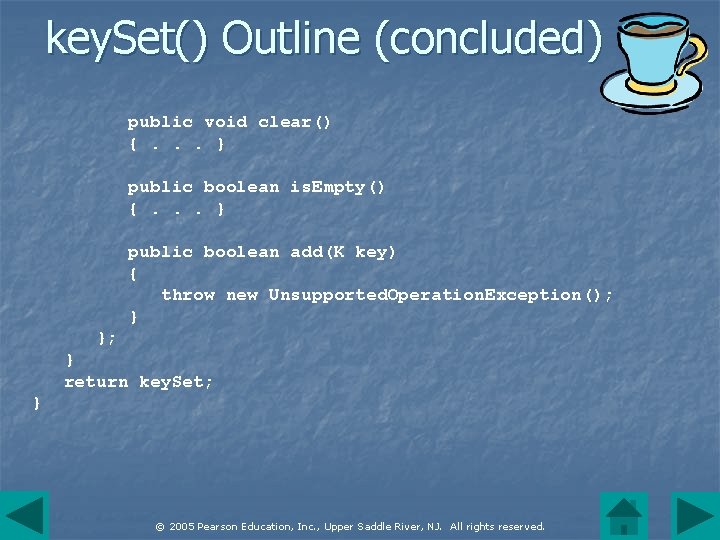
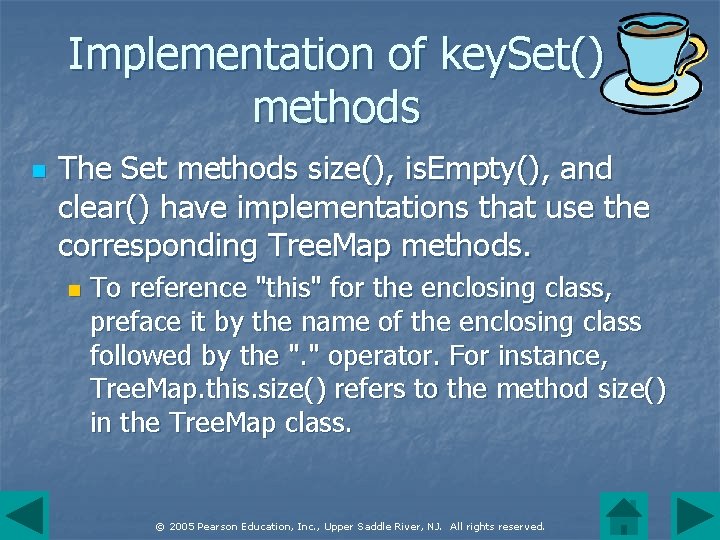
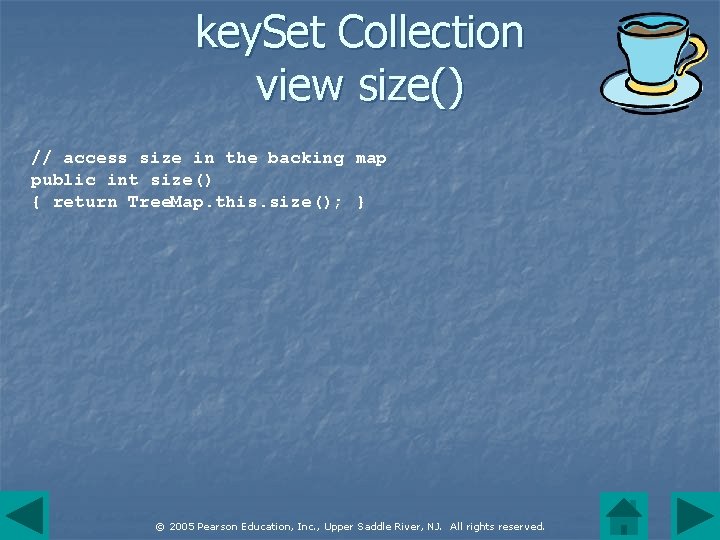
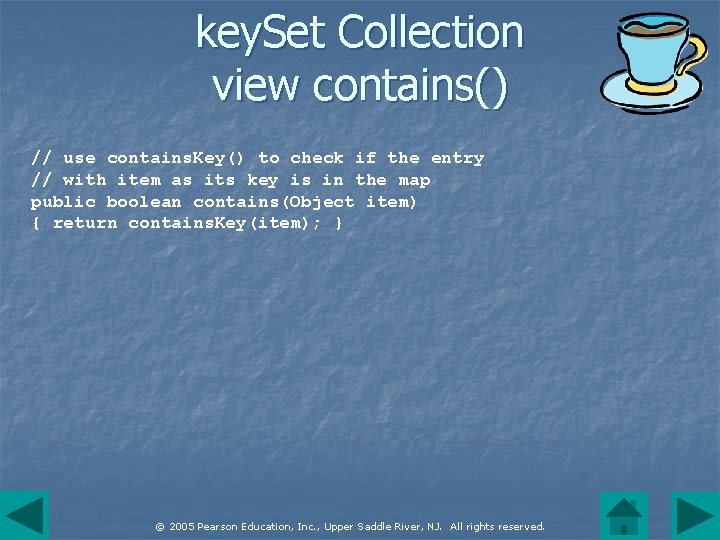
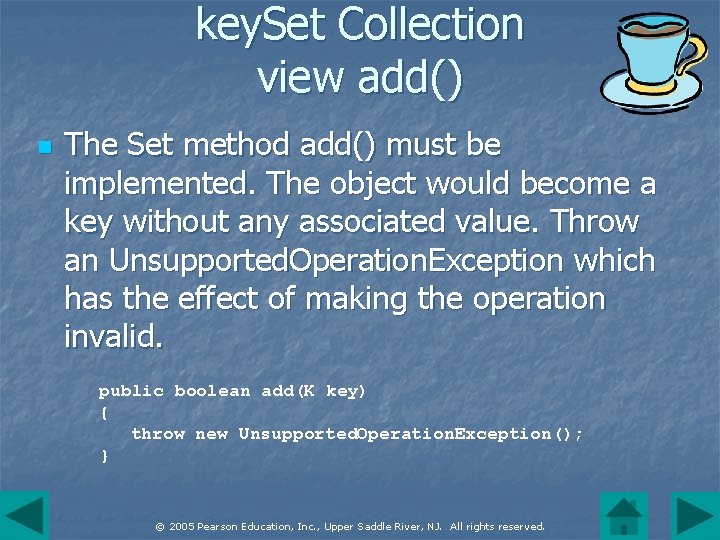
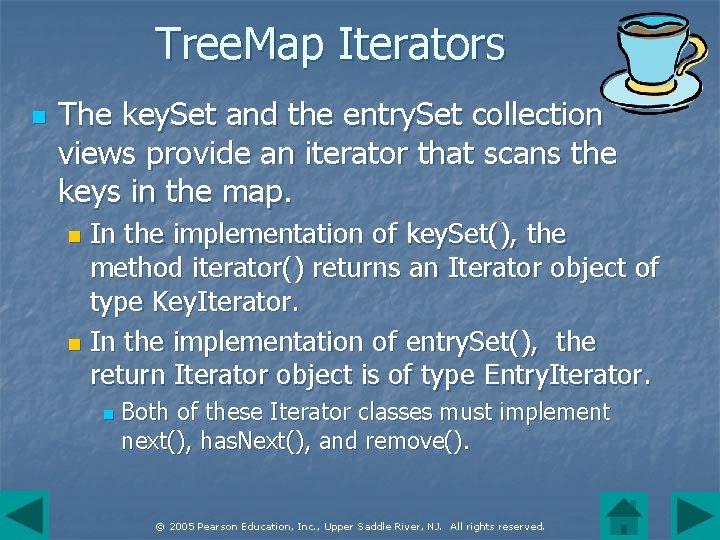
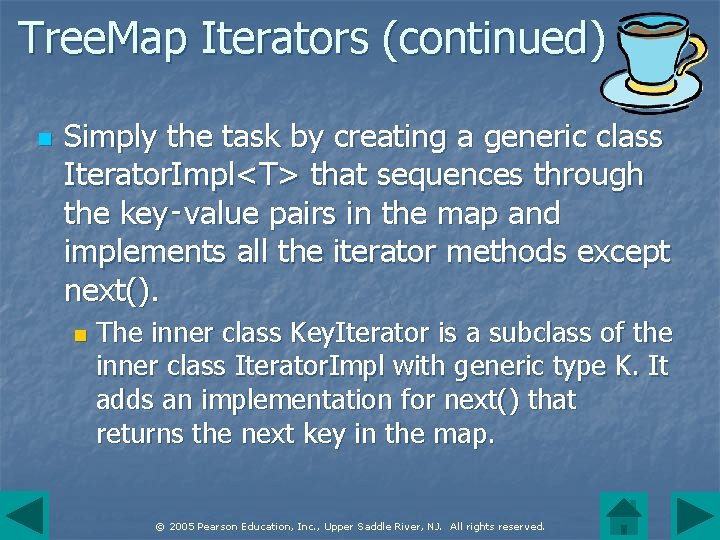
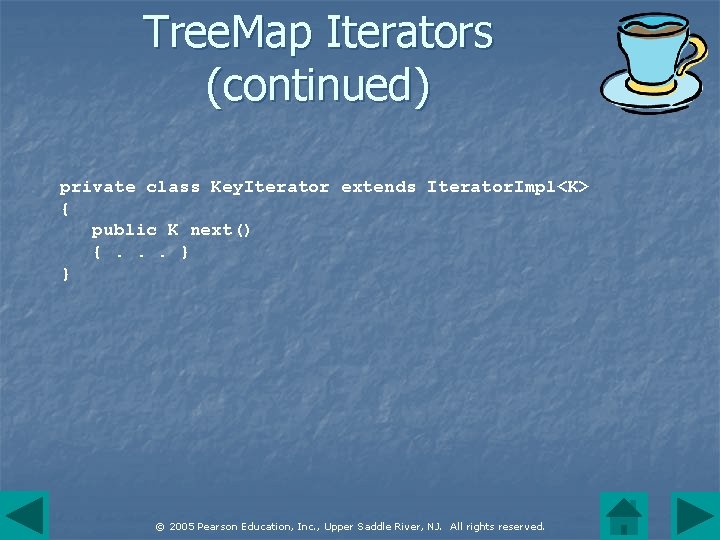
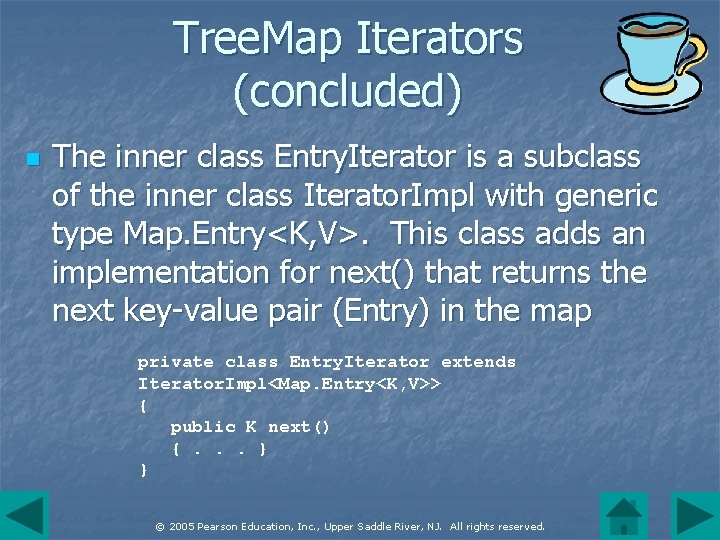
- Slides: 57
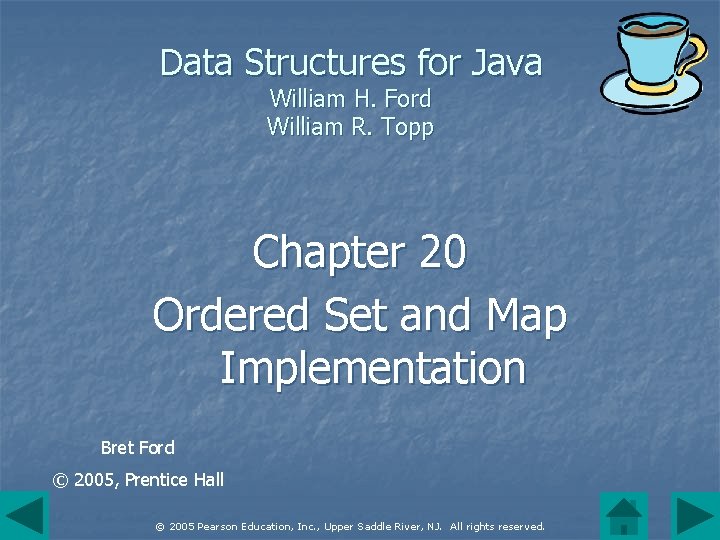
Data Structures for Java William H. Ford William R. Topp Chapter 20 Ordered Set and Map Implementation Bret Ford © 2005, Prentice Hall © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
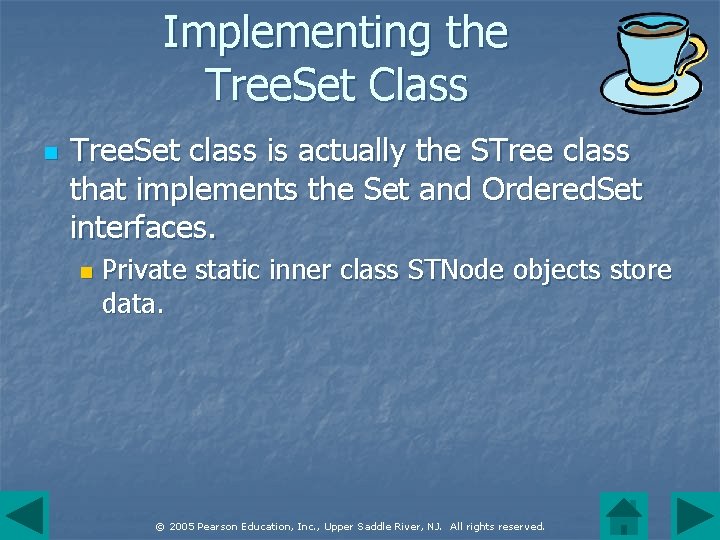
Implementing the Tree. Set Class n Tree. Set class is actually the STree class that implements the Set and Ordered. Set interfaces. n Private static inner class STNode objects store data. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
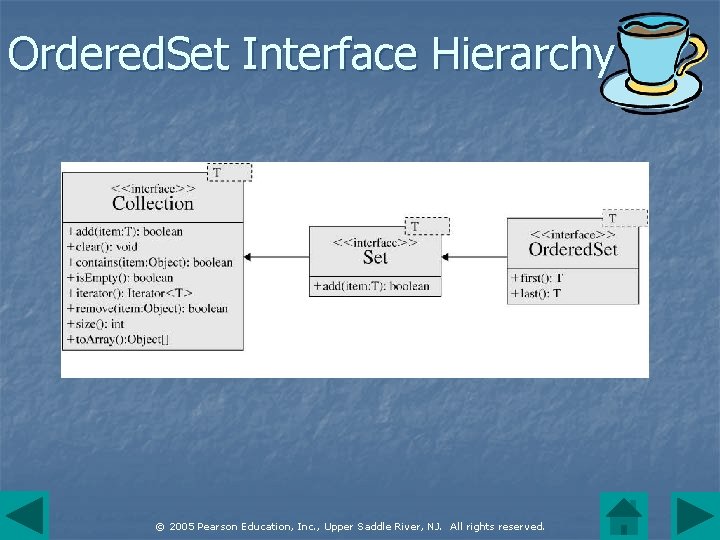
Ordered. Set Interface Hierarchy © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
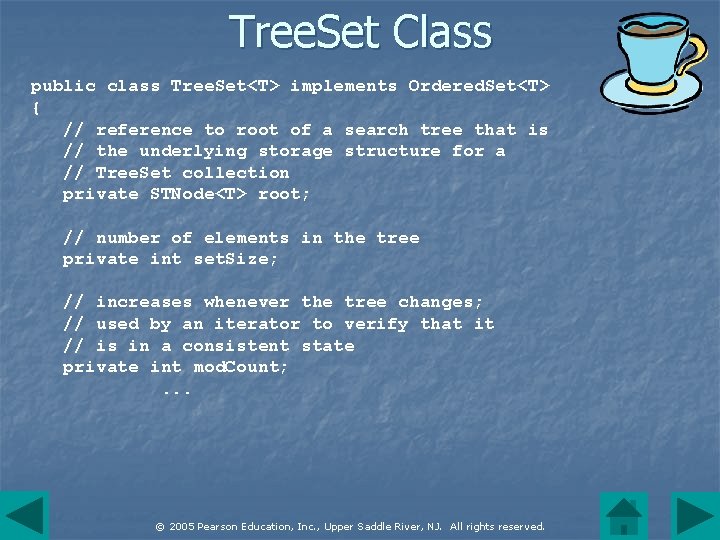
Tree. Set Class public class Tree. Set<T> implements Ordered. Set<T> { // reference to root of a search tree that is // the underlying storage structure for a // Tree. Set collection private STNode<T> root; // number of elements in the tree private int set. Size; // increases whenever the tree changes; // used by an iterator to verify that it // is in a consistent state private int mod. Count; . . . © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
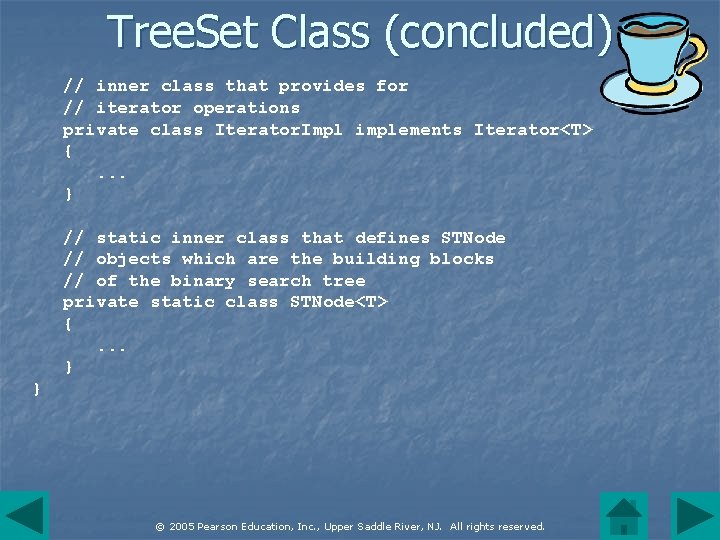
Tree. Set Class (concluded) // inner class that provides for // iterator operations private class Iterator. Impl implements Iterator<T> {. . . } // static inner class that defines STNode // objects which are the building blocks // of the binary search tree private static class STNode<T> {. . . } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
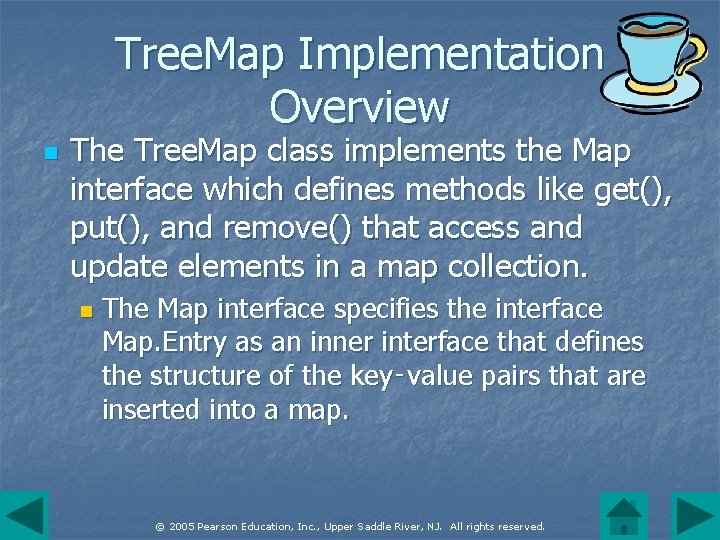
Tree. Map Implementation Overview n The Tree. Map class implements the Map interface which defines methods like get(), put(), and remove() that access and update elements in a map collection. n The Map interface specifies the interface Map. Entry as an inner interface that defines the structure of the key‑value pairs that are inserted into a map. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
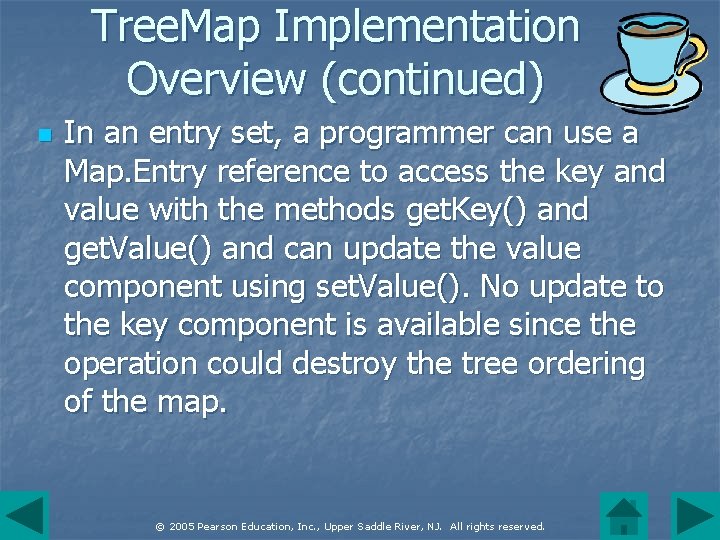
Tree. Map Implementation Overview (continued) n In an entry set, a programmer can use a Map. Entry reference to access the key and value with the methods get. Key() and get. Value() and can update the value component using set. Value(). No update to the key component is available since the operation could destroy the tree ordering of the map. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
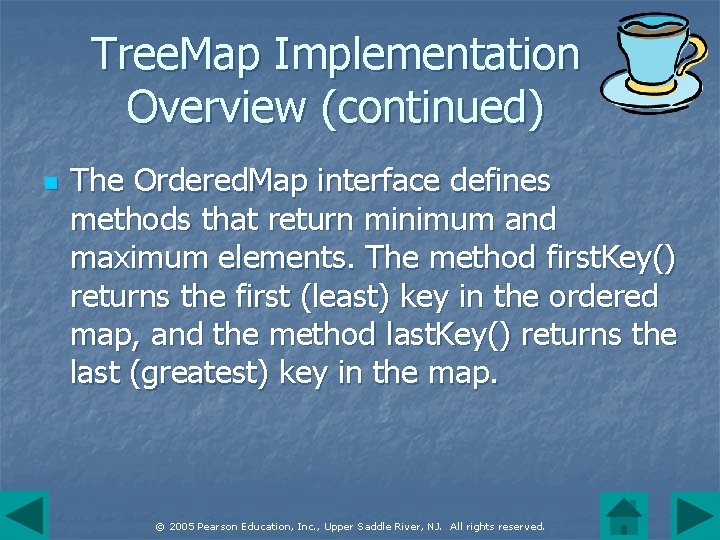
Tree. Map Implementation Overview (continued) n The Ordered. Map interface defines methods that return minimum and maximum elements. The method first. Key() returns the first (least) key in the ordered map, and the method last. Key() returns the last (greatest) key in the map. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
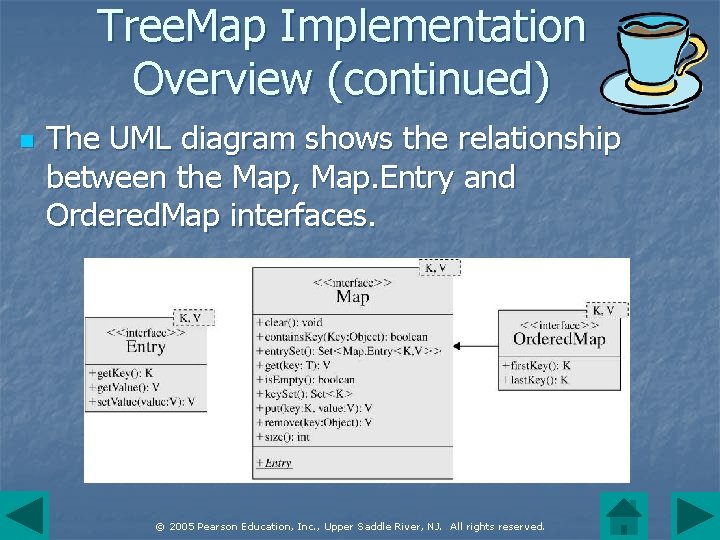
Tree. Map Implementation Overview (continued) n The UML diagram shows the relationship between the Map, Map. Entry and Ordered. Map interfaces. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
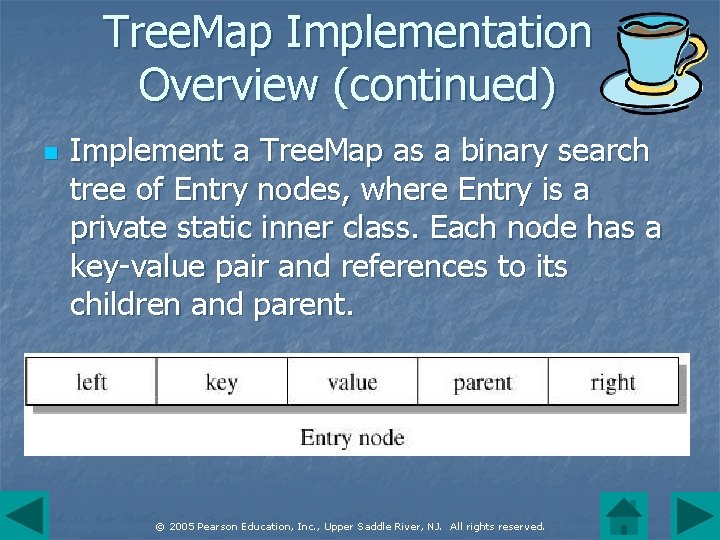
Tree. Map Implementation Overview (continued) n Implement a Tree. Map as a binary search tree of Entry nodes, where Entry is a private static inner class. Each node has a key-value pair and references to its children and parent. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
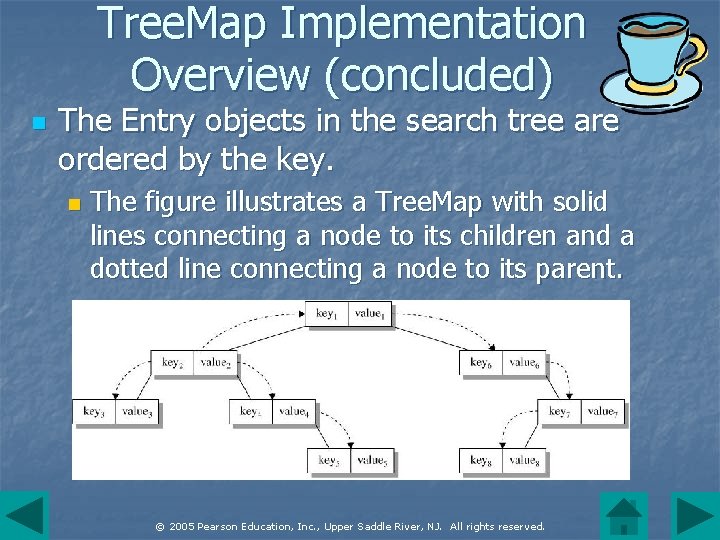
Tree. Map Implementation Overview (concluded) n The Entry objects in the search tree are ordered by the key. n The figure illustrates a Tree. Map with solid lines connecting a node to its children and a dotted line connecting a node to its parent. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
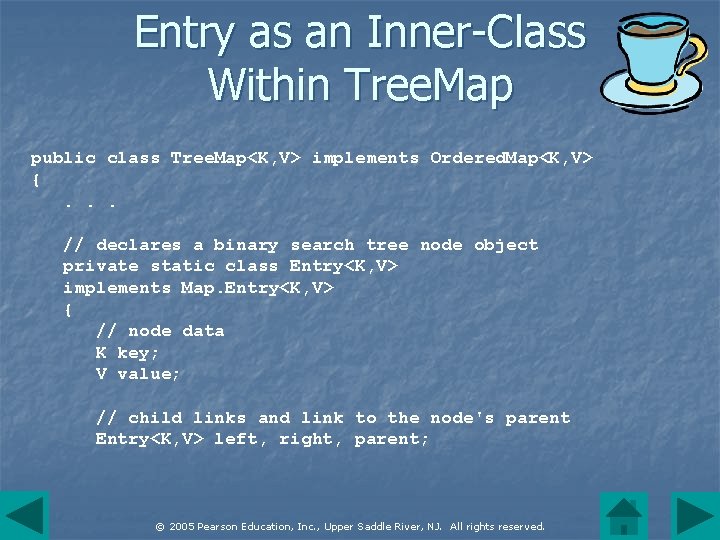
Entry as an Inner-Class Within Tree. Map public class Tree. Map<K, V> implements Ordered. Map<K, V> {. . . // declares a binary search tree node object private static class Entry<K, V> implements Map. Entry<K, V> { // node data K key; V value; // child links and link to the node's parent Entry<K, V> left, right, parent; © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
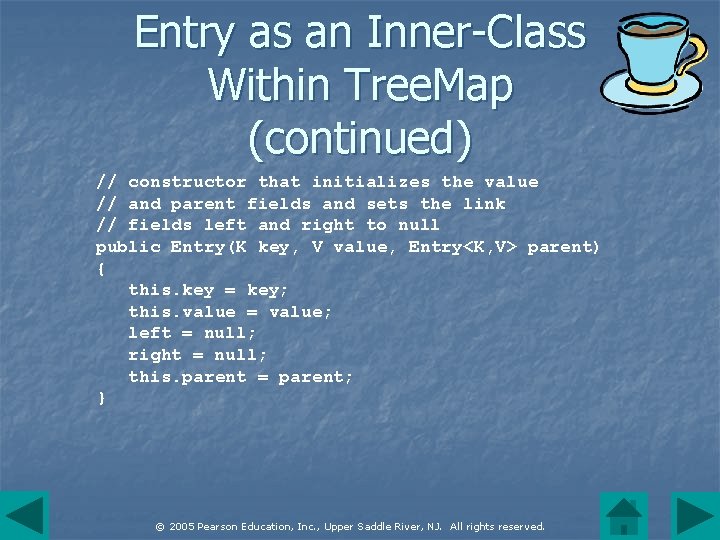
Entry as an Inner-Class Within Tree. Map (continued) // constructor that initializes the value // and parent fields and sets the link // fields left and right to null public Entry(K key, V value, Entry<K, V> parent) { this. key = key; this. value = value; left = null; right = null; this. parent = parent; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
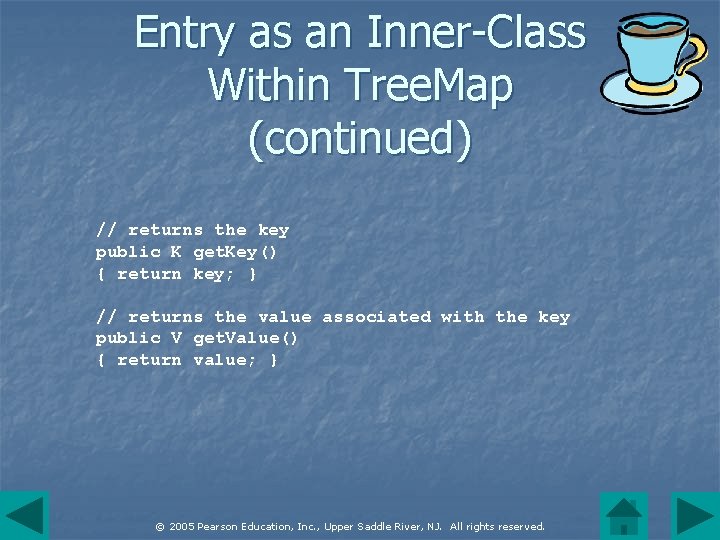
Entry as an Inner-Class Within Tree. Map (continued) // returns the key public K get. Key() { return key; } // returns the value associated with the key public V get. Value() { return value; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
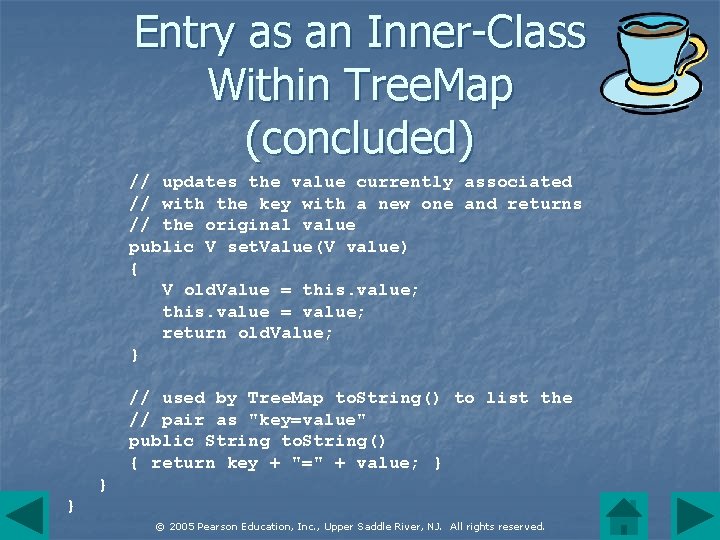
Entry as an Inner-Class Within Tree. Map (concluded) // updates the value currently associated // with the key with a new one and returns // the original value public V set. Value(V value) { V old. Value = this. value; this. value = value; return old. Value; } // used by Tree. Map to. String() to list the // pair as "key=value" public String to. String() { return key + "=" + value; } } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
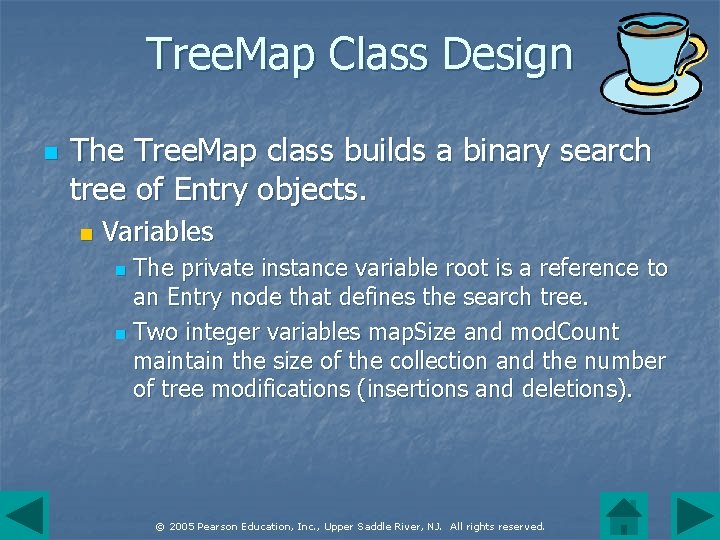
Tree. Map Class Design n The Tree. Map class builds a binary search tree of Entry objects. n Variables The private instance variable root is a reference to an Entry node that defines the search tree. n Two integer variables map. Size and mod. Count maintain the size of the collection and the number of tree modifications (insertions and deletions). n © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
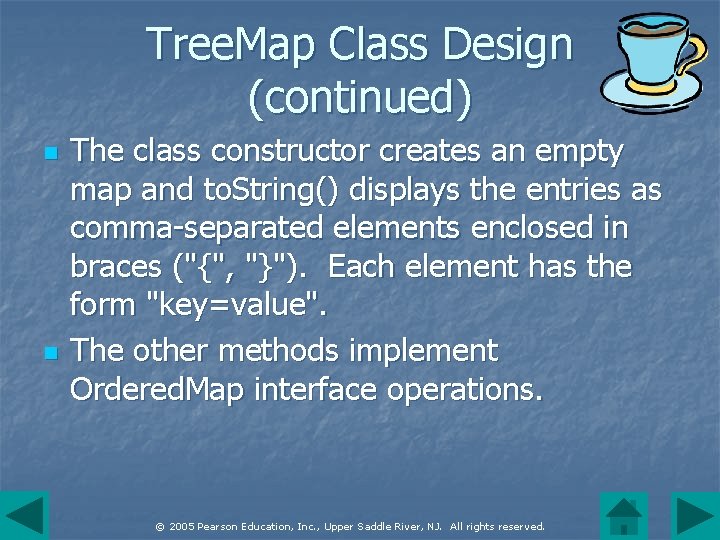
Tree. Map Class Design (continued) n n The class constructor creates an empty map and to. String() displays the entries as comma-separated elements enclosed in braces ("{", "}"). Each element has the form "key=value". The other methods implement Ordered. Map interface operations. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
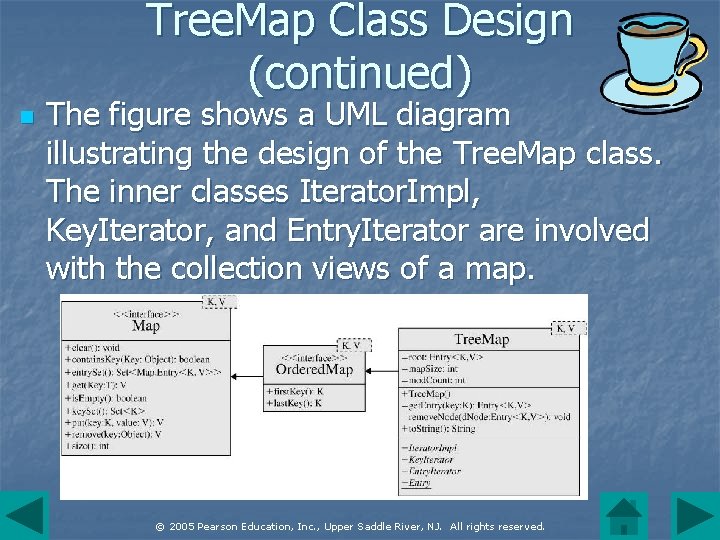
Tree. Map Class Design (continued) n The figure shows a UML diagram illustrating the design of the Tree. Map class. The inner classes Iterator. Impl, Key. Iterator, and Entry. Iterator are involved with the collection views of a map. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
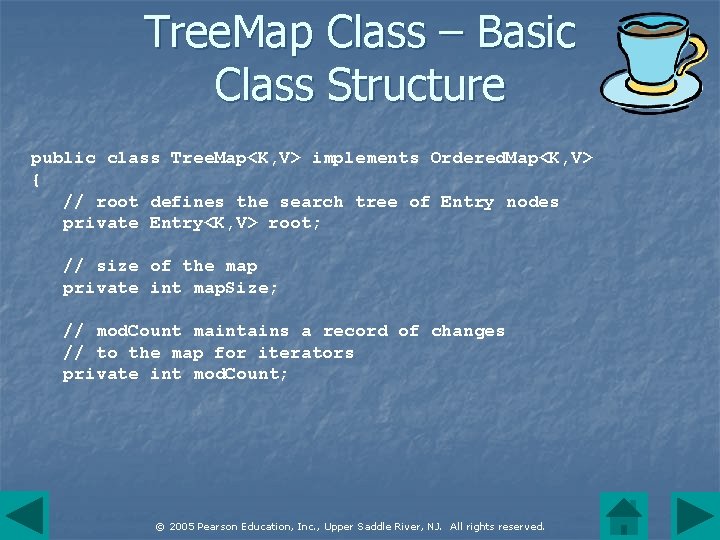
Tree. Map Class – Basic Class Structure public class Tree. Map<K, V> implements Ordered. Map<K, V> { // root defines the search tree of Entry nodes private Entry<K, V> root; // size of the map private int map. Size; // mod. Count maintains a record of changes // to the map for iterators private int mod. Count; © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
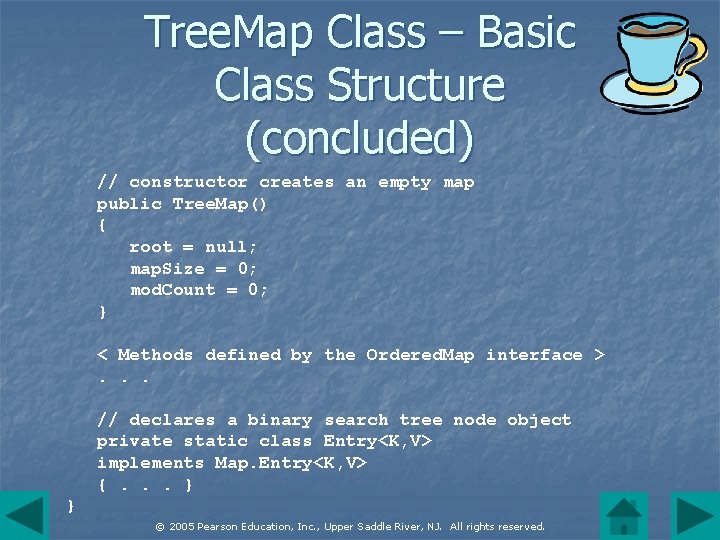
Tree. Map Class – Basic Class Structure (concluded) // constructor creates an empty map public Tree. Map() { root = null; map. Size = 0; mod. Count = 0; } < Methods defined by the Ordered. Map interface >. . . // declares a binary search tree node object private static class Entry<K, V> implements Map. Entry<K, V> {. . . } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
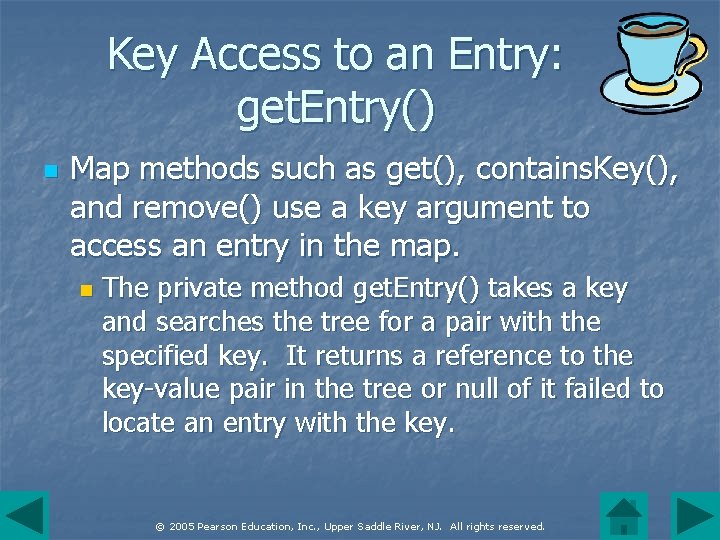
Key Access to an Entry: get. Entry() n Map methods such as get(), contains. Key(), and remove() use a key argument to access an entry in the map. n The private method get. Entry() takes a key and searches the tree for a pair with the specified key. It returns a reference to the key-value pair in the tree or null of it failed to locate an entry with the key. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
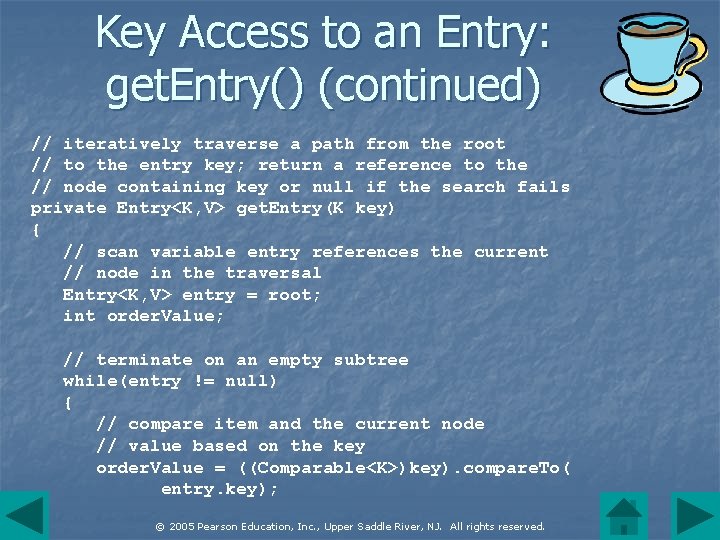
Key Access to an Entry: get. Entry() (continued) // iteratively traverse a path from the root // to the entry key; return a reference to the // node containing key or null if the search fails private Entry<K, V> get. Entry(K key) { // scan variable entry references the current // node in the traversal Entry<K, V> entry = root; int order. Value; // terminate on an empty subtree while(entry != null) { // compare item and the current node // value based on the key order. Value = ((Comparable<K>)key). compare. To( entry. key); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
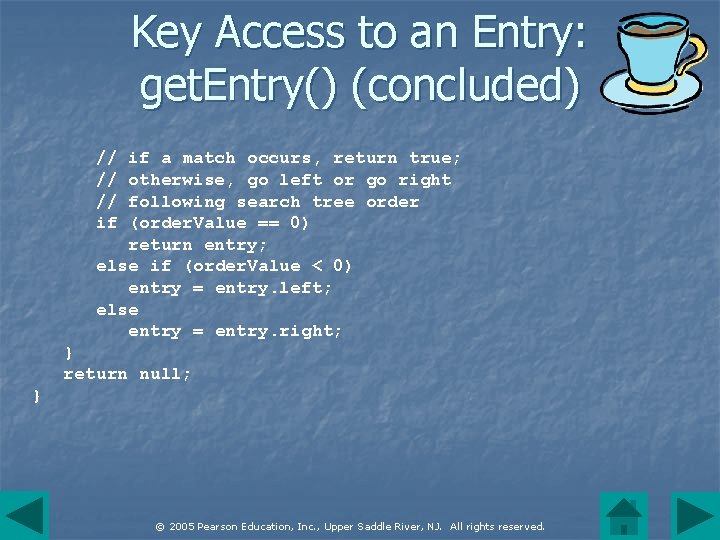
Key Access to an Entry: get. Entry() (concluded) // // // if if a match occurs, return true; otherwise, go left or go right following search tree order (order. Value == 0) return entry; else if (order. Value < 0) entry = entry. left; else entry = entry. right; } return null; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
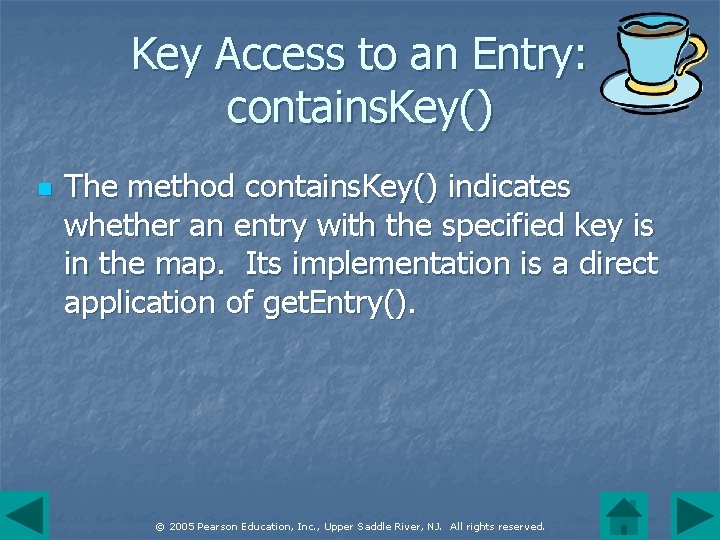
Key Access to an Entry: contains. Key() n The method contains. Key() indicates whether an entry with the specified key is in the map. Its implementation is a direct application of get. Entry(). © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
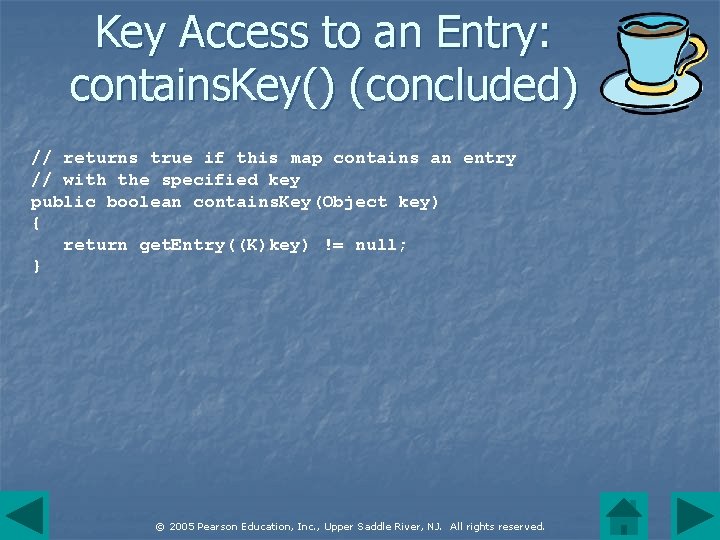
Key Access to an Entry: contains. Key() (concluded) // returns true if this map contains an entry // with the specified key public boolean contains. Key(Object key) { return get. Entry((K)key) != null; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
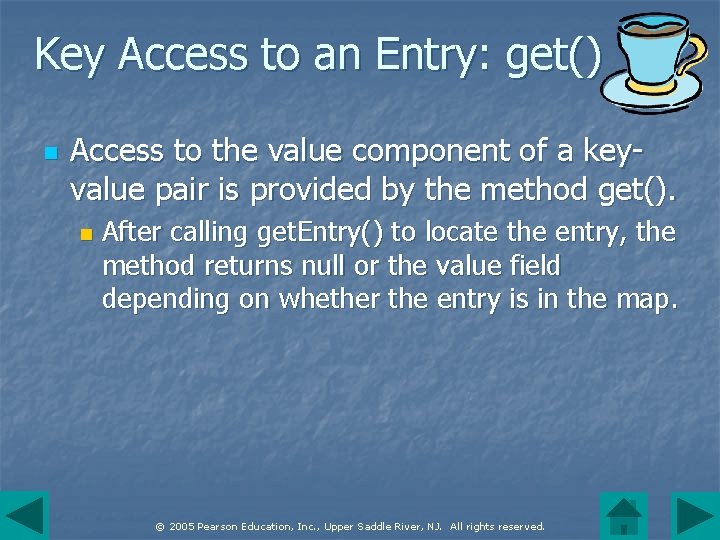
Key Access to an Entry: get() n Access to the value component of a keyvalue pair is provided by the method get(). n After calling get. Entry() to locate the entry, the method returns null or the value field depending on whether the entry is in the map. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
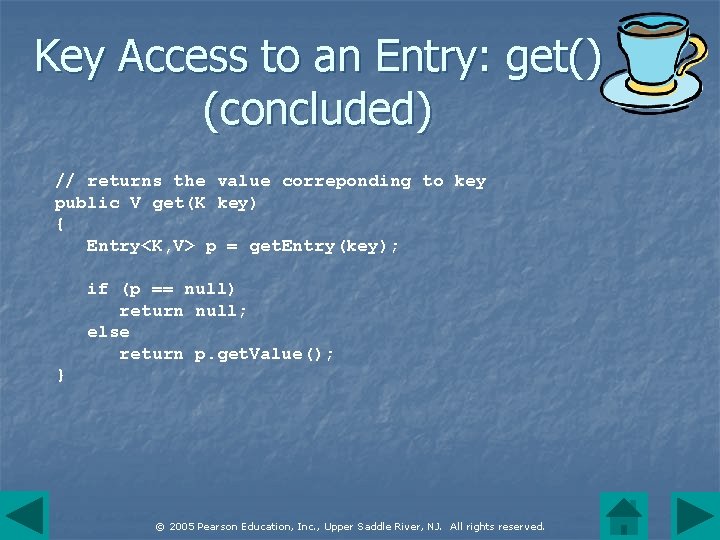
Key Access to an Entry: get() (concluded) // returns the value correponding to key public V get(K key) { Entry<K, V> p = get. Entry(key); if (p == null) return null; else return p. get. Value(); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
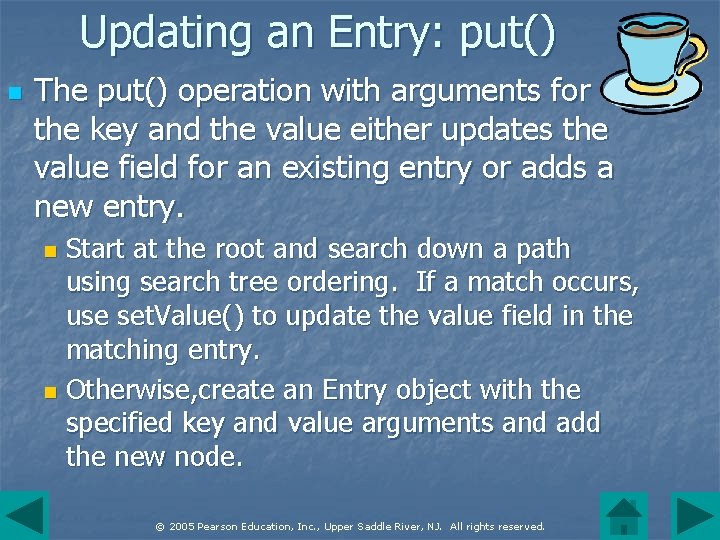
Updating an Entry: put() n The put() operation with arguments for the key and the value either updates the value field for an existing entry or adds a new entry. Start at the root and search down a path using search tree ordering. If a match occurs, use set. Value() to update the value field in the matching entry. n Otherwise, create an Entry object with the specified key and value arguments and add the new node. n © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
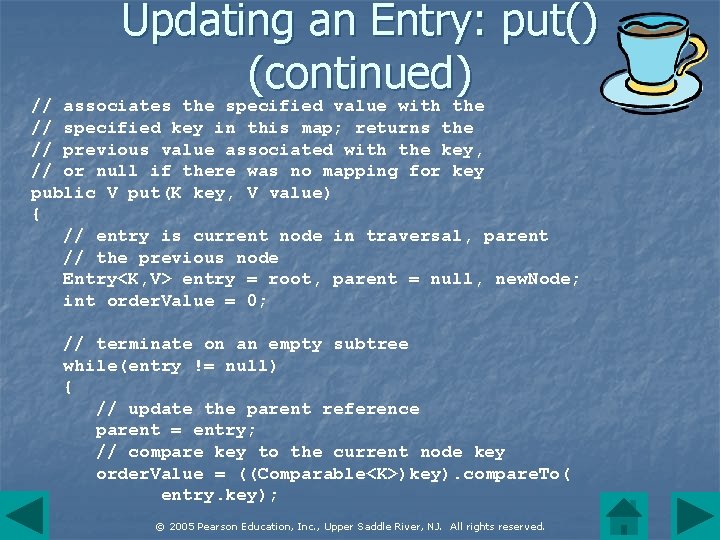
Updating an Entry: put() (continued) // associates the specified value with the // specified key in this map; returns the // previous value associated with the key, // or null if there was no mapping for key public V put(K key, V value) { // entry is current node in traversal, parent // the previous node Entry<K, V> entry = root, parent = null, new. Node; int order. Value = 0; // terminate on an empty subtree while(entry != null) { // update the parent reference parent = entry; // compare key to the current node key order. Value = ((Comparable<K>)key). compare. To( entry. key); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
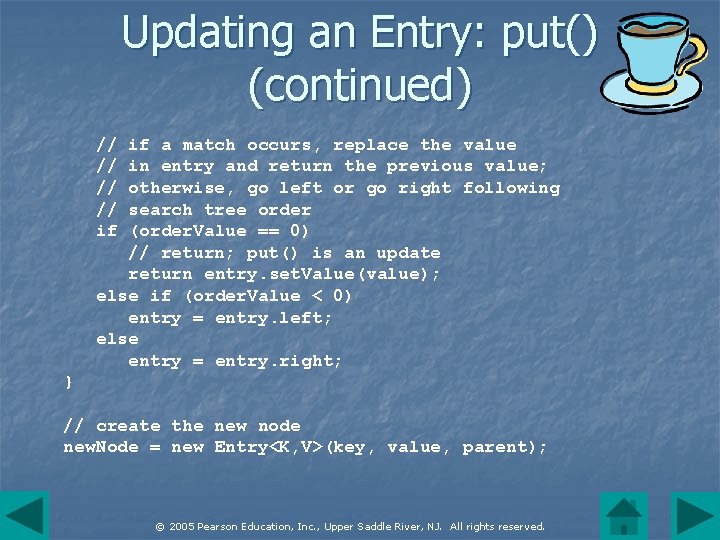
Updating an Entry: put() (continued) // // if if a match occurs, replace the value in entry and return the previous value; otherwise, go left or go right following search tree order (order. Value == 0) // return; put() is an update return entry. set. Value(value); else if (order. Value < 0) entry = entry. left; else entry = entry. right; } // create the new node new. Node = new Entry<K, V>(key, value, parent); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
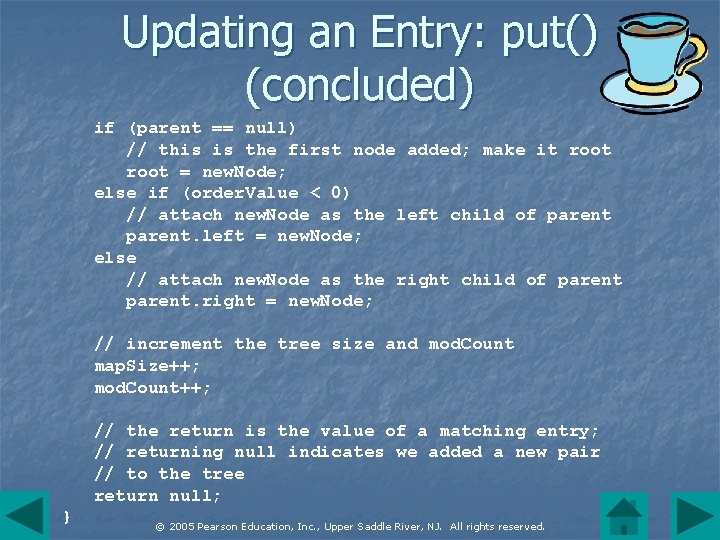
Updating an Entry: put() (concluded) if (parent == null) // this is the first node added; make it root = new. Node; else if (order. Value < 0) // attach new. Node as the left child of parent. left = new. Node; else // attach new. Node as the right child of parent. right = new. Node; // increment the tree size and mod. Count map. Size++; mod. Count++; // the return is the value of a matching entry; // returning null indicates we added a new pair // to the tree return null; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
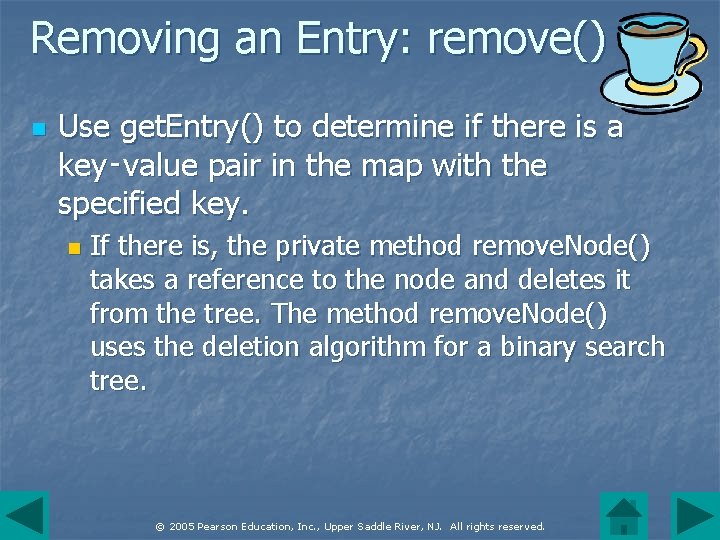
Removing an Entry: remove() n Use get. Entry() to determine if there is a key‑value pair in the map with the specified key. n If there is, the private method remove. Node() takes a reference to the node and deletes it from the tree. The method remove. Node() uses the deletion algorithm for a binary search tree. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
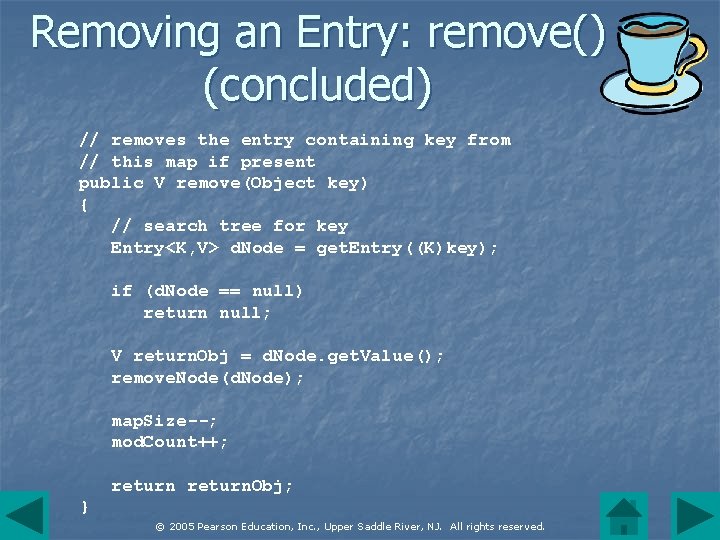
Removing an Entry: remove() (concluded) // removes the entry containing key from // this map if present public V remove(Object key) { // search tree for key Entry<K, V> d. Node = get. Entry((K)key); if (d. Node == null) return null; V return. Obj = d. Node. get. Value(); remove. Node(d. Node); map. Size--; mod. Count++; return. Obj; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
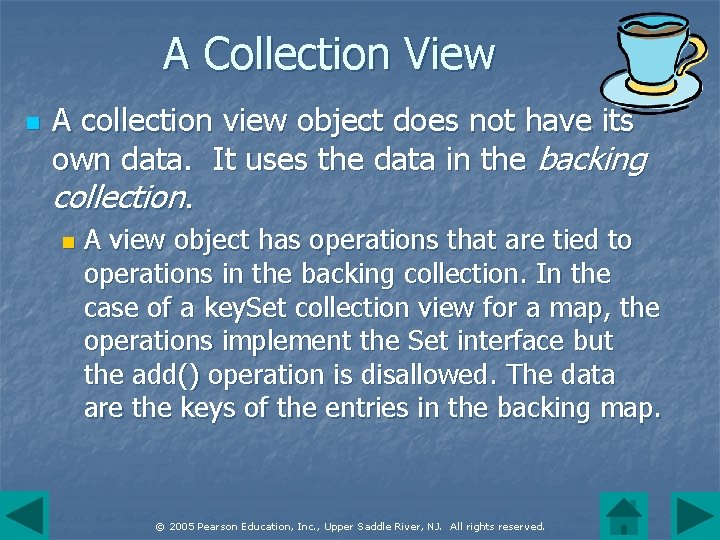
A Collection View n A collection view object does not have its own data. It uses the data in the backing collection. n A view object has operations that are tied to operations in the backing collection. In the case of a key. Set collection view for a map, the operations implement the Set interface but the add() operation is disallowed. The data are the keys of the entries in the backing map. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
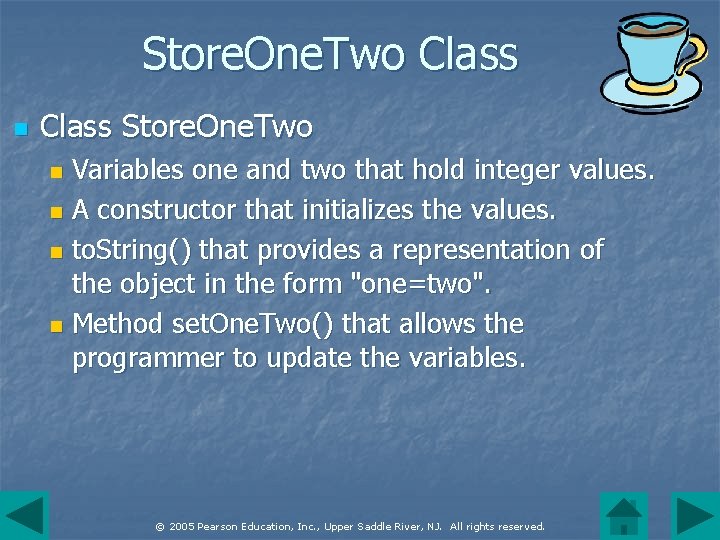
Store. One. Two Class n Class Store. One. Two Variables one and two that hold integer values. n A constructor that initializes the values. n to. String() that provides a representation of the object in the form "one=two". n Method set. One. Two() that allows the programmer to update the variables. n © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
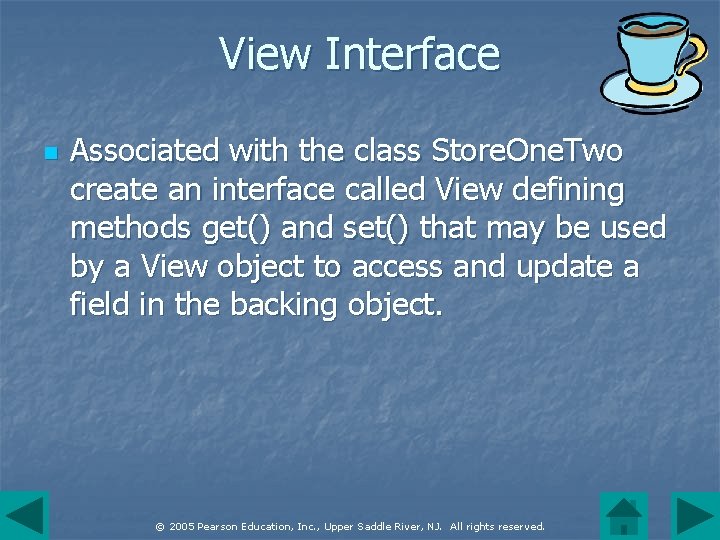
View Interface n Associated with the class Store. One. Two create an interface called View defining methods get() and set() that may be used by a View object to access and update a field in the backing object. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
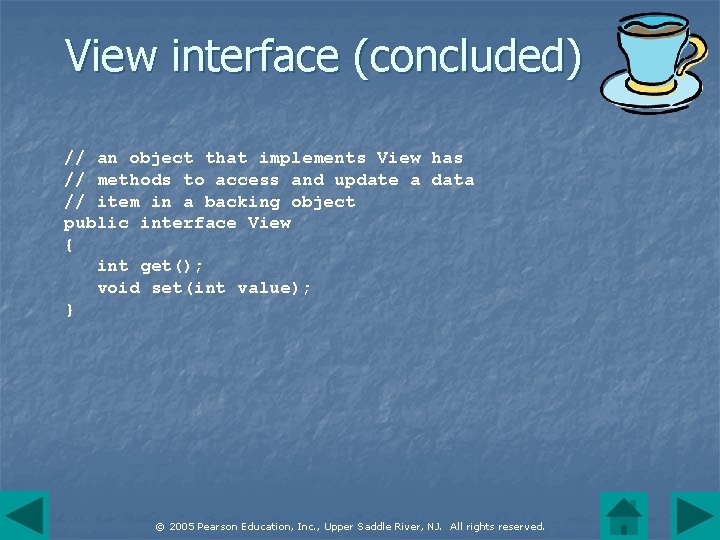
View interface (concluded) // an object that implements View has // methods to access and update a data // item in a backing object public interface View { int get(); void set(int value); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
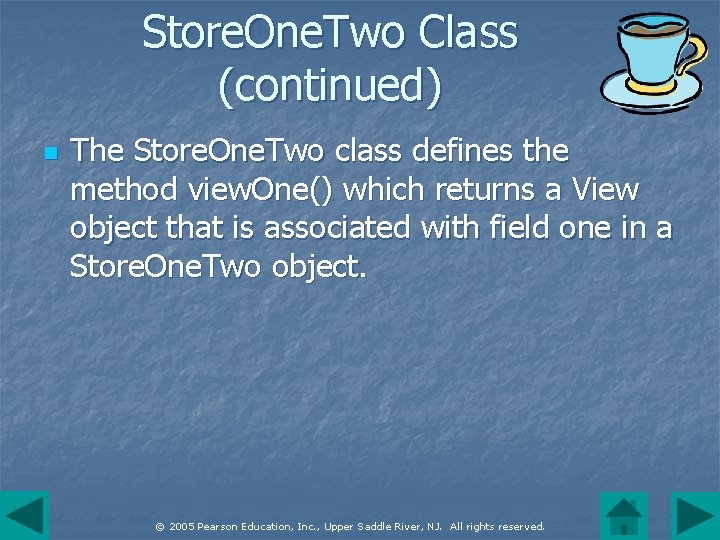
Store. One. Two Class (continued) n The Store. One. Two class defines the method view. One() which returns a View object that is associated with field one in a Store. One. Two object. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
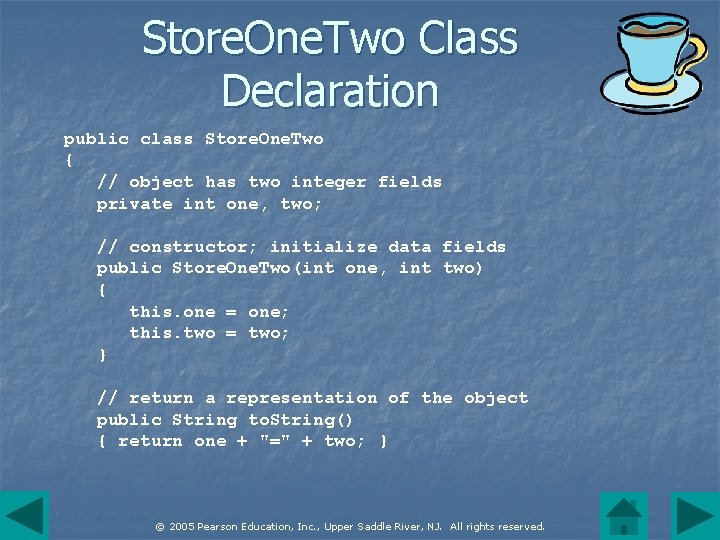
Store. One. Two Class Declaration public class Store. One. Two { // object has two integer fields private int one, two; // constructor; initialize data fields public Store. One. Two(int one, int two) { this. one = one; this. two = two; } // return a representation of the object public String to. String() { return one + "=" + two; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
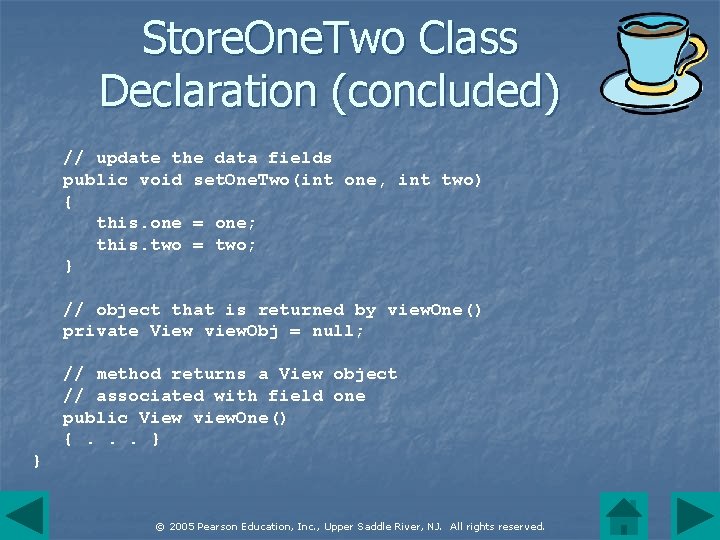
Store. One. Two Class Declaration (concluded) // update the data fields public void set. One. Two(int one, int two) { this. one = one; this. two = two; } // object that is returned by view. One() private View view. Obj = null; // method returns a View object // associated with field one public View view. One() {. . . } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
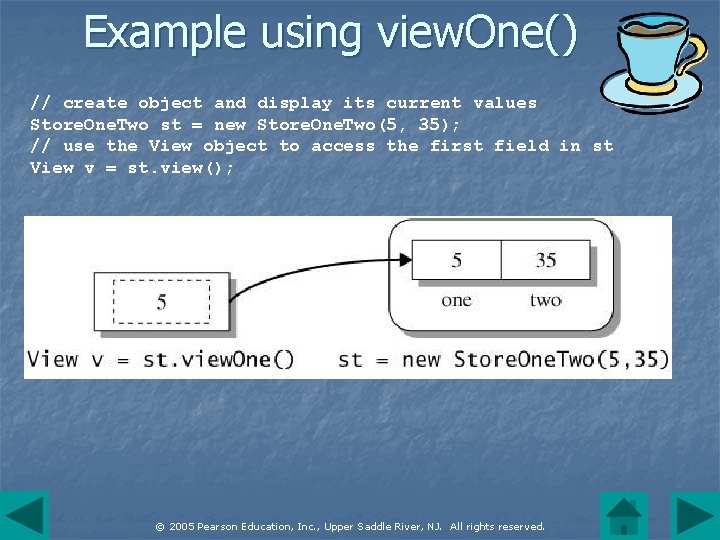
Example using view. One() // create object and display its current values Store. One. Two st = new Store. One. Two(5, 35); // use the View object to access the first field in st View v = st. view(); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
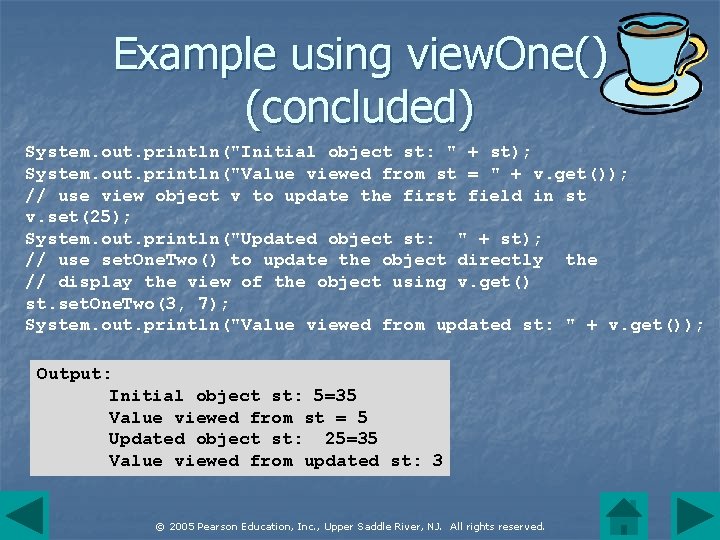
Example using view. One() (concluded) System. out. println("Initial object st: " + st); System. out. println("Value viewed from st = " + v. get()); // use view object v to update the first field in st v. set(25); System. out. println("Updated object st: " + st); // use set. One. Two() to update the object directly the // display the view of the object using v. get() st. set. One. Two(3, 7); System. out. println("Value viewed from updated st: " + v. get()); Output: Initial object st: 5=35 Value viewed from st = 5 Updated object st: 25=35 Value viewed from updated st: 3 © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
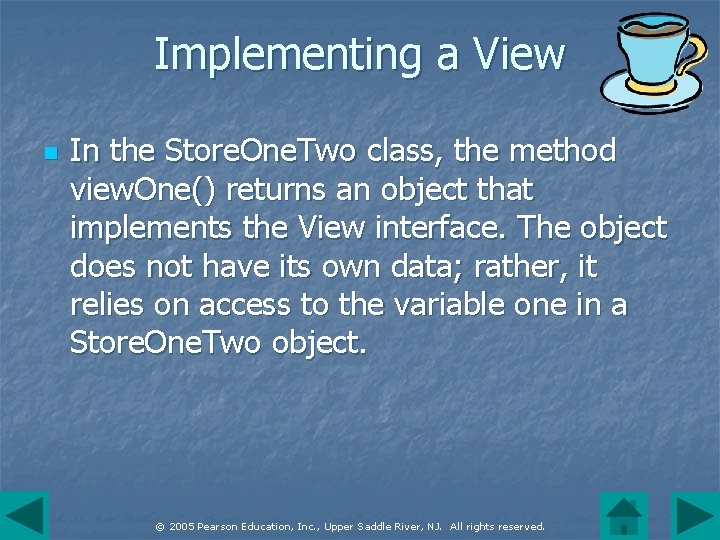
Implementing a View n In the Store. One. Two class, the method view. One() returns an object that implements the View interface. The object does not have its own data; rather, it relies on access to the variable one in a Store. One. Two object. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
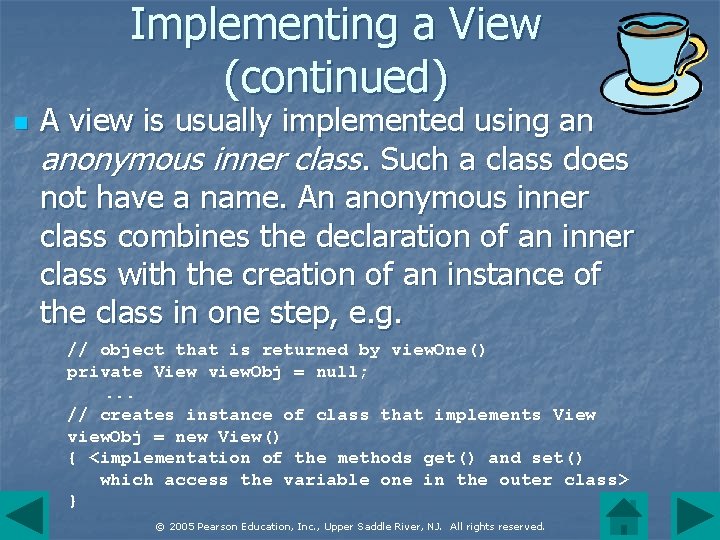
Implementing a View (continued) n A view is usually implemented using an anonymous inner class. Such a class does not have a name. An anonymous inner class combines the declaration of an inner class with the creation of an instance of the class in one step, e. g. // object that is returned by view. One() private View view. Obj = null; . . . // creates instance of class that implements View view. Obj = new View() { <implementation of the methods get() and set() which access the variable one in the outer class> } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
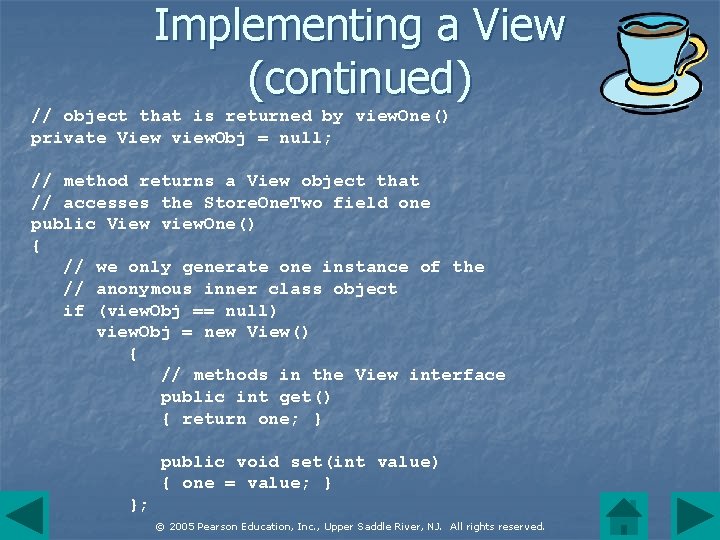
Implementing a View (continued) // object that is returned by view. One() private View view. Obj = null; // method returns a View object that // accesses the Store. One. Two field one public View view. One() { // we only generate one instance of the // anonymous inner class object if (view. Obj == null) view. Obj = new View() { // methods in the View interface public int get() { return one; } public void set(int value) { one = value; } }; © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
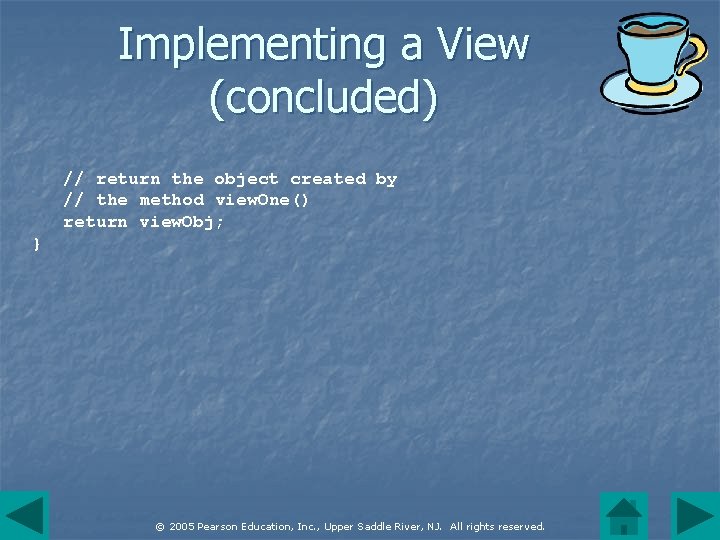
Implementing a View (concluded) // return the object created by // the method view. One() return view. Obj; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
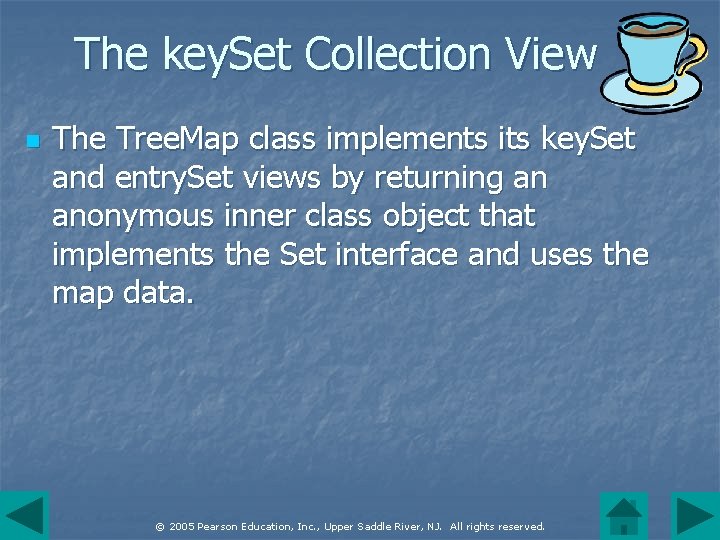
The key. Set Collection View n The Tree. Map class implements its key. Set and entry. Set views by returning an anonymous inner class object that implements the Set interface and uses the map data. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
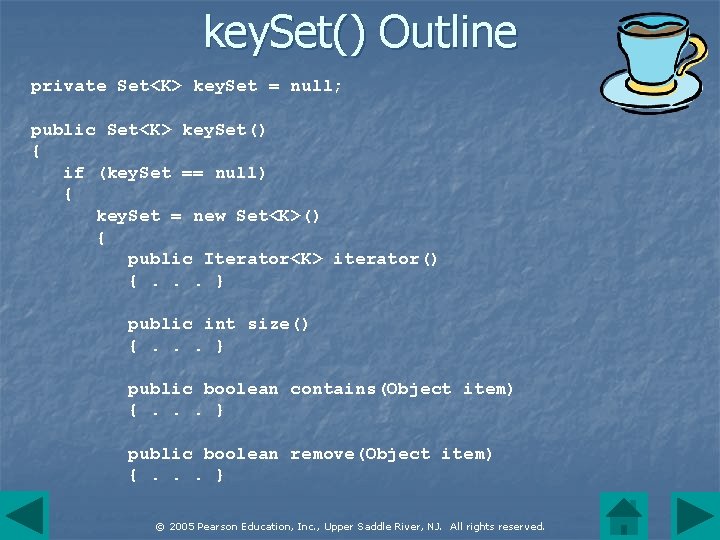
key. Set() Outline private Set<K> key. Set = null; public Set<K> key. Set() { if (key. Set == null) { key. Set = new Set<K>() { public Iterator<K> iterator() {. . . } public int size() {. . . } public boolean contains(Object item) {. . . } public boolean remove(Object item) {. . . } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
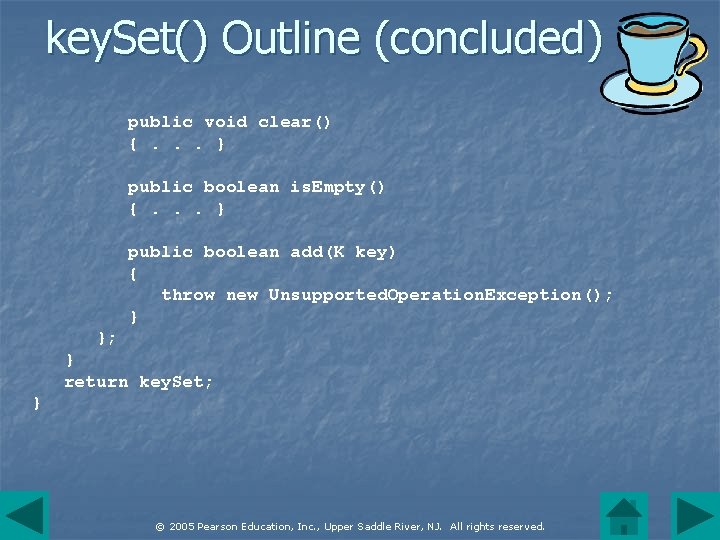
key. Set() Outline (concluded) public void clear() {. . . } public boolean is. Empty() {. . . } public boolean add(K key) { throw new Unsupported. Operation. Exception(); } }; } return key. Set; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
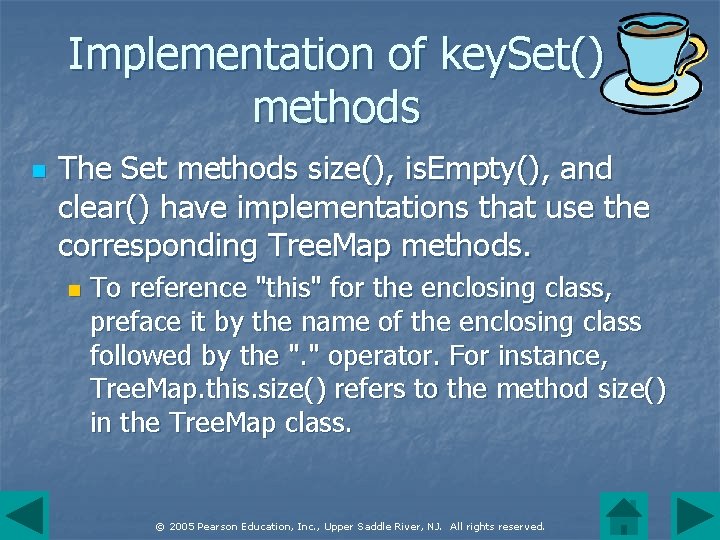
Implementation of key. Set() methods n The Set methods size(), is. Empty(), and clear() have implementations that use the corresponding Tree. Map methods. n To reference "this" for the enclosing class, preface it by the name of the enclosing class followed by the ". " operator. For instance, Tree. Map. this. size() refers to the method size() in the Tree. Map class. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
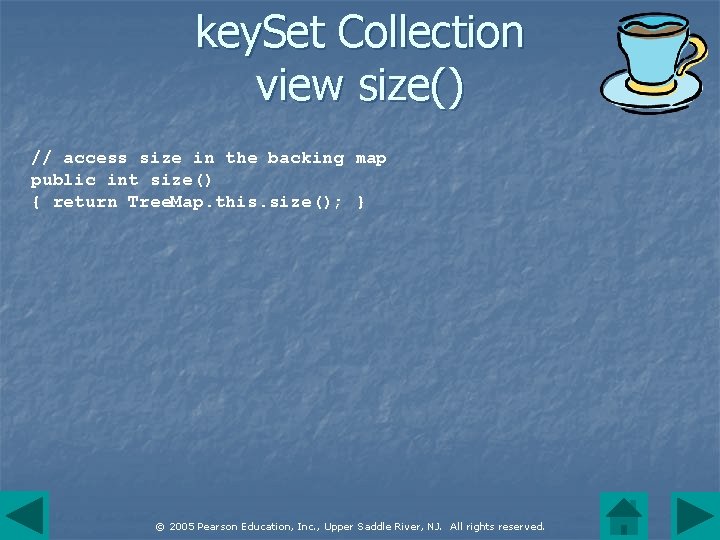
key. Set Collection view size() // access size in the backing map public int size() { return Tree. Map. this. size(); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
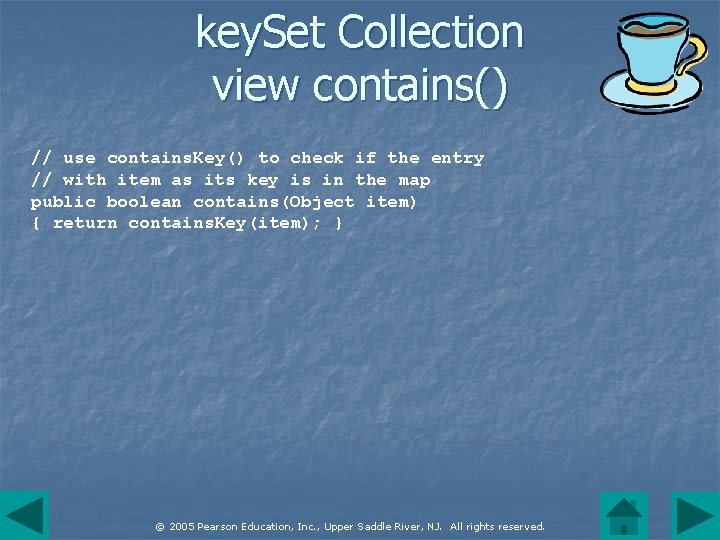
key. Set Collection view contains() // use contains. Key() to check if the entry // with item as its key is in the map public boolean contains(Object item) { return contains. Key(item); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
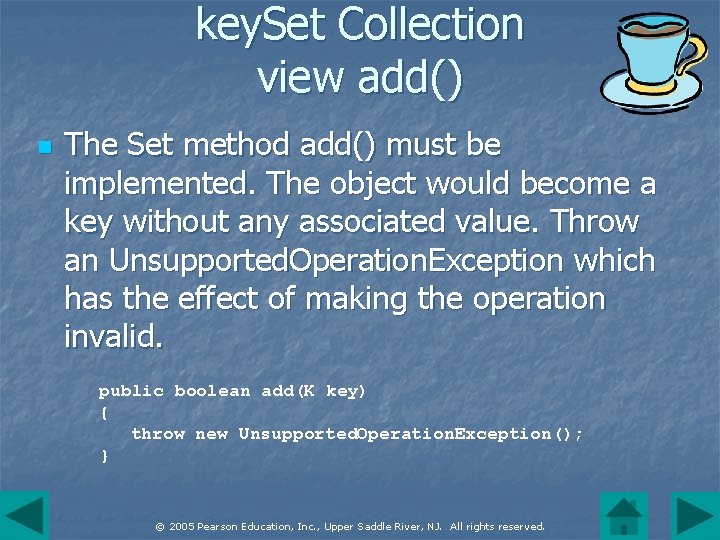
key. Set Collection view add() n The Set method add() must be implemented. The object would become a key without any associated value. Throw an Unsupported. Operation. Exception which has the effect of making the operation invalid. public boolean add(K key) { throw new Unsupported. Operation. Exception(); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
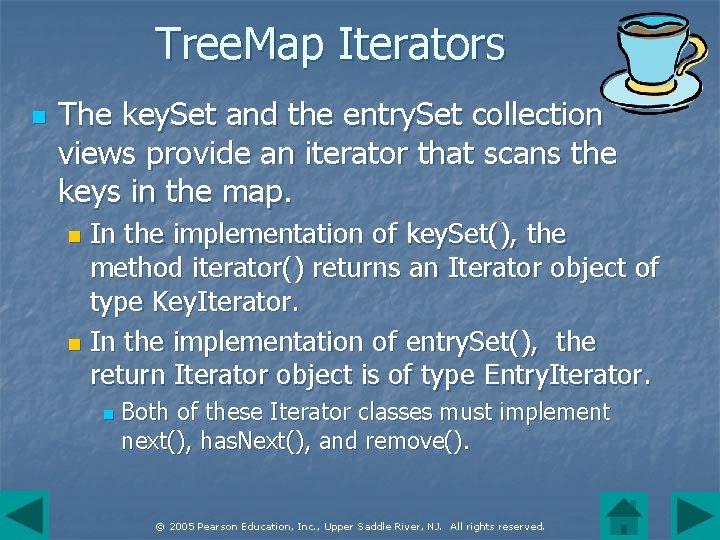
Tree. Map Iterators n The key. Set and the entry. Set collection views provide an iterator that scans the keys in the map. In the implementation of key. Set(), the method iterator() returns an Iterator object of type Key. Iterator. n In the implementation of entry. Set(), the return Iterator object is of type Entry. Iterator. n n Both of these Iterator classes must implement next(), has. Next(), and remove(). © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
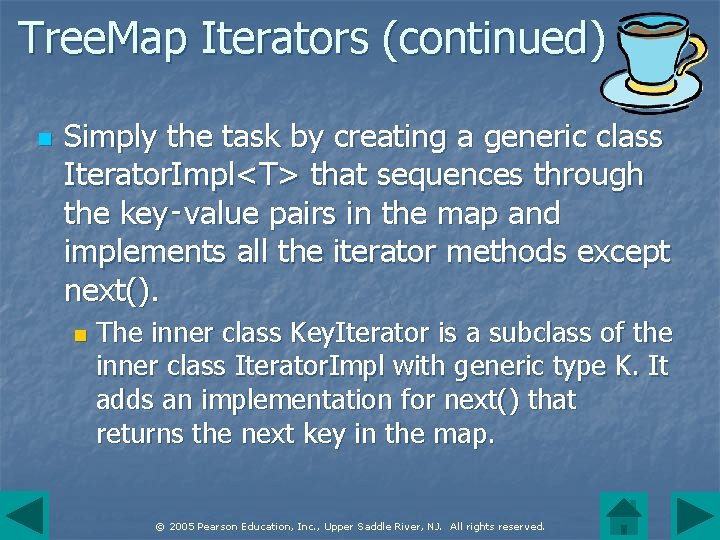
Tree. Map Iterators (continued) n Simply the task by creating a generic class Iterator. Impl<T> that sequences through the key‑value pairs in the map and implements all the iterator methods except next(). n The inner class Key. Iterator is a subclass of the inner class Iterator. Impl with generic type K. It adds an implementation for next() that returns the next key in the map. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
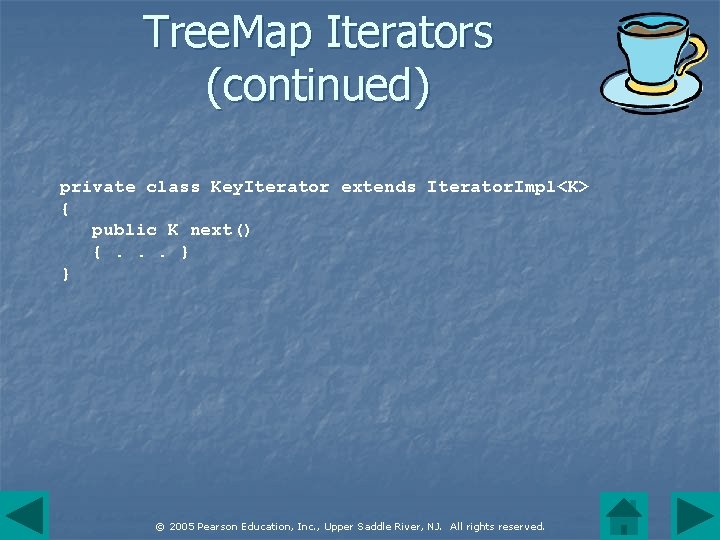
Tree. Map Iterators (continued) private class Key. Iterator extends Iterator. Impl<K> { public K next() {. . . } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
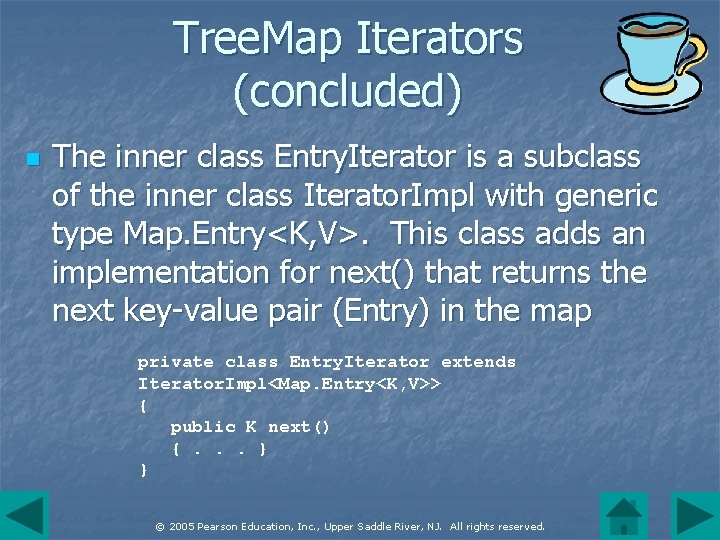
Tree. Map Iterators (concluded) n The inner class Entry. Iterator is a subclass of the inner class Iterator. Impl with generic type Map. Entry<K, V>. This class adds an implementation for next() that returns the next key-value pair (Entry) in the map private class Entry. Iterator extends Iterator. Impl<Map. Entry<K, V>> { public K next() {. . . } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
Advanced data structures in java
Java dynamic data structures
Data structures and abstractions with java
Java data structures
Data structures in java
Data structures in java
Data structures using java
Data structures using java
Data structures using java
Analogous structure
Java software structures 4th edition
Fspos vägledning för kontinuitetshantering
Typiska novell drag
Tack för att ni lyssnade bild
Ekologiskt fotavtryck
Varför kallas perioden 1918-1939 för mellankrigstiden
En lathund för arbete med kontinuitetshantering
Personalliggare bygg undantag
Vilotidsbok
Anatomi organ reproduksi
Vad är densitet
Datorkunskap för nybörjare
Stig kerman
Mall debattartikel
Delegerande ledarstil
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Lufttryck formel
Svenskt ramverk för digital samverkan
Jag har nigit för nymånens skära
Presentera för publik crossboss
Teckenspråk minoritetsspråk argument
Kanaans land
Klassificeringsstruktur för kommunala verksamheter
Luftstrupen för medicinare
Claes martinsson
Cks
Programskede byggprocessen
Mat för unga idrottare
Verktyg för automatisering av utbetalningar
Rutin för avvikelsehantering
Smärtskolan kunskap för livet
Ministerstyre för och nackdelar
Tack för att ni har lyssnat
Hur ser ett referat ut
Redogör för vad psykologi är
Matematisk modellering eksempel
Atmosfr
Borra hål för knoppar
Orubbliga rättigheter
Fr formel
Tack för att ni har lyssnat
Rita perspektiv
Vad är verksamhetsanalys
Tobinskatten för och nackdelar
Blomman för dagen drog
Gibbs reflekterande cykel
Egg för emanuel