Data Structures for Java William H Ford William
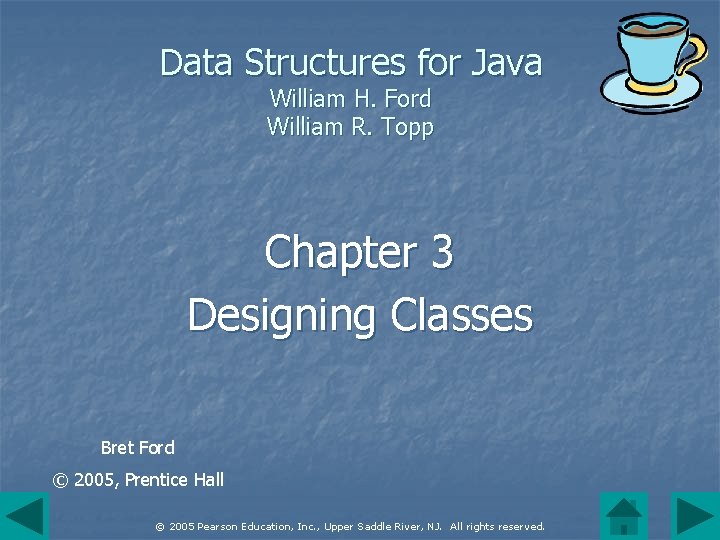
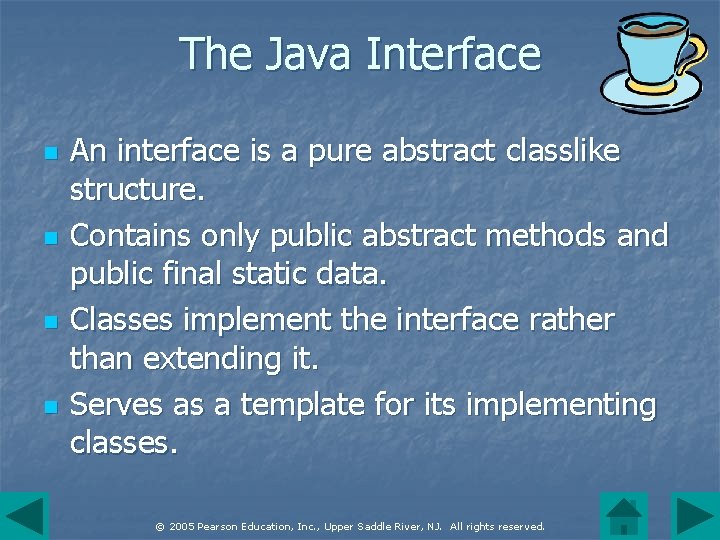
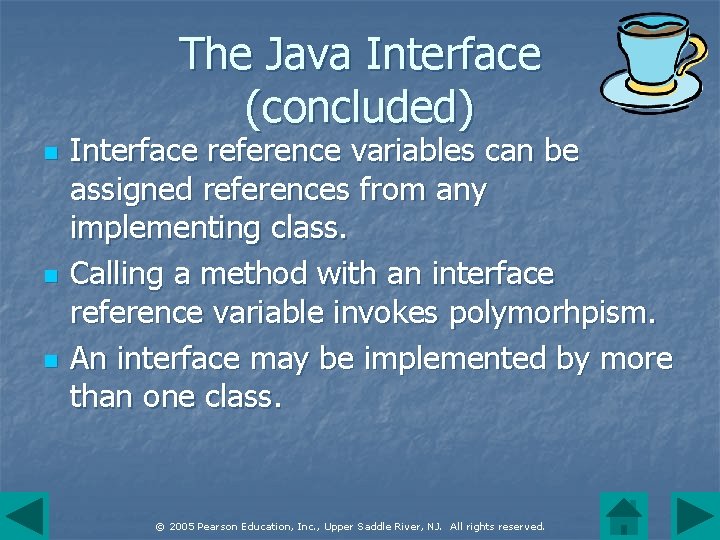
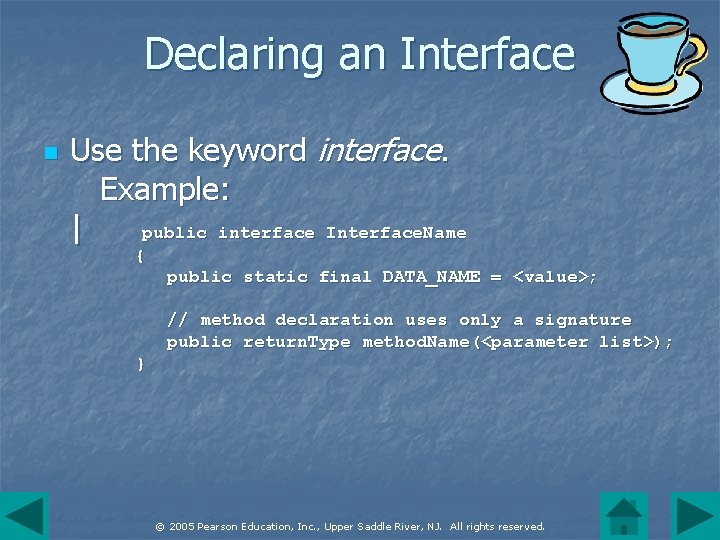
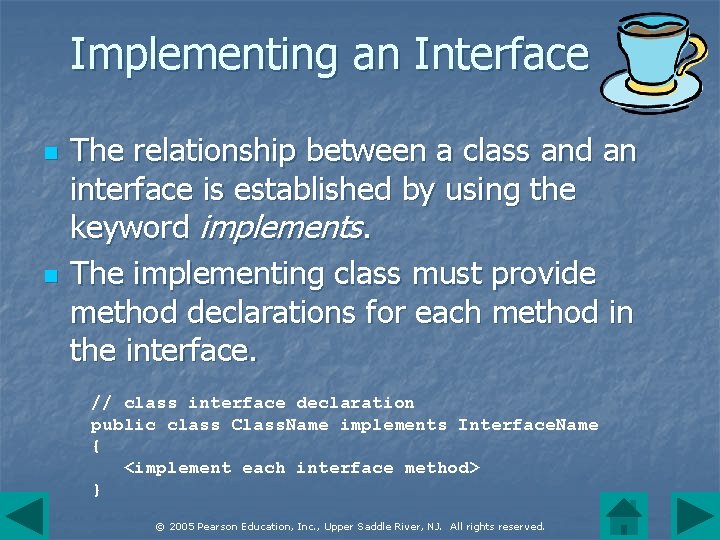
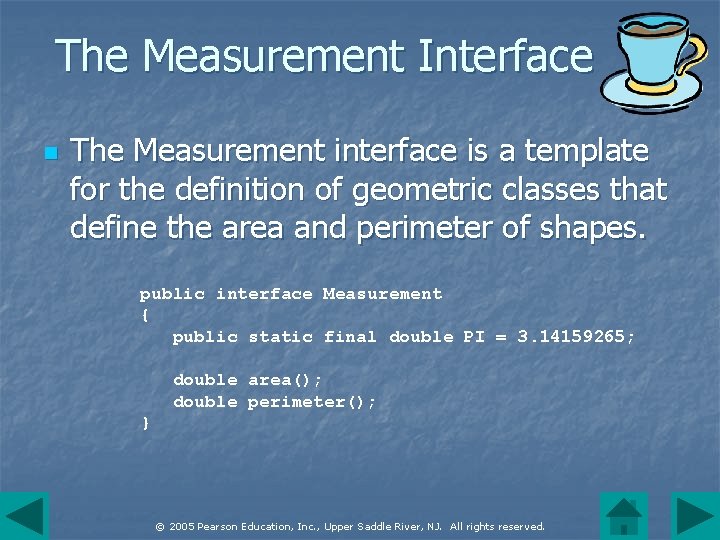
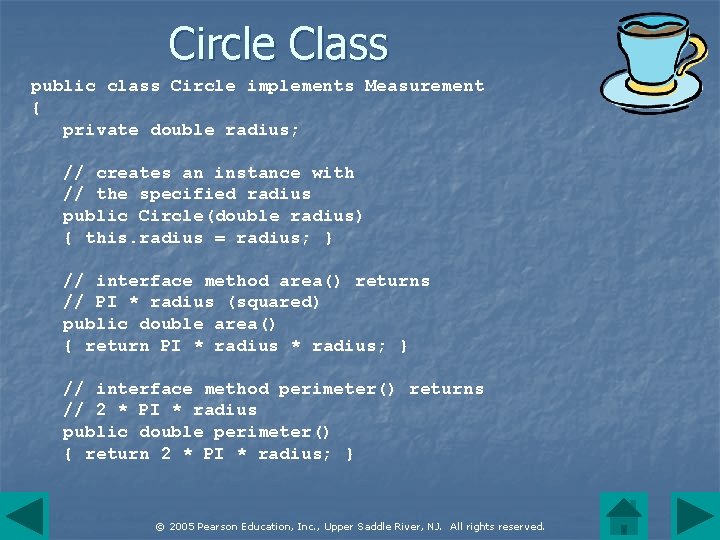
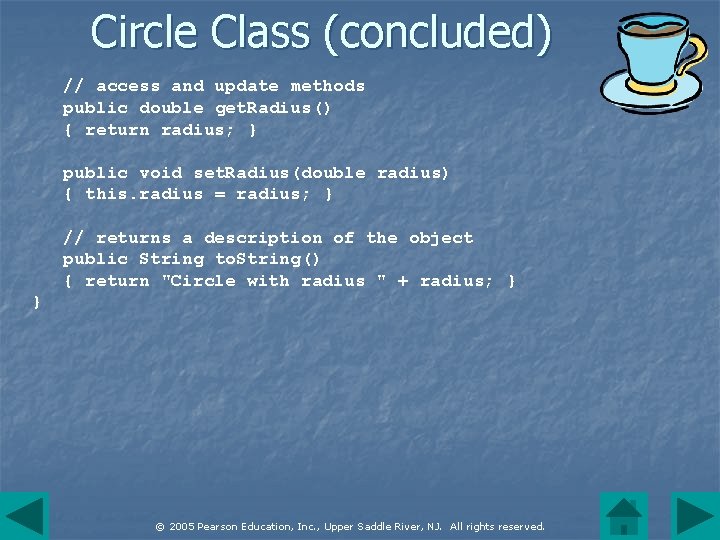
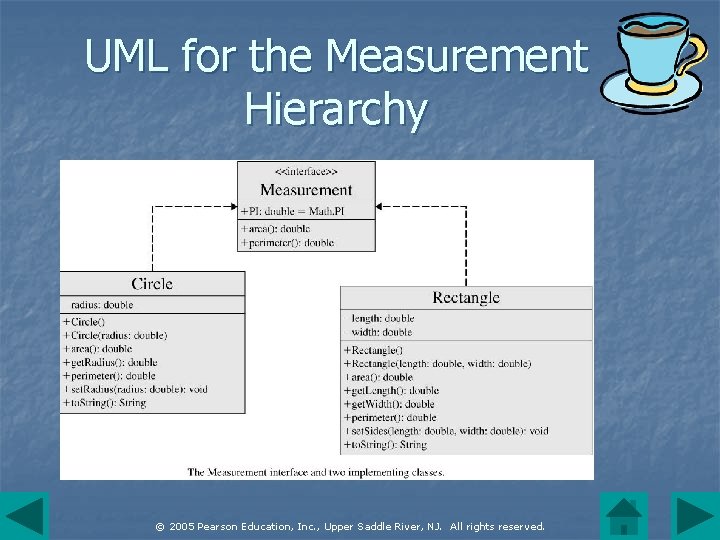
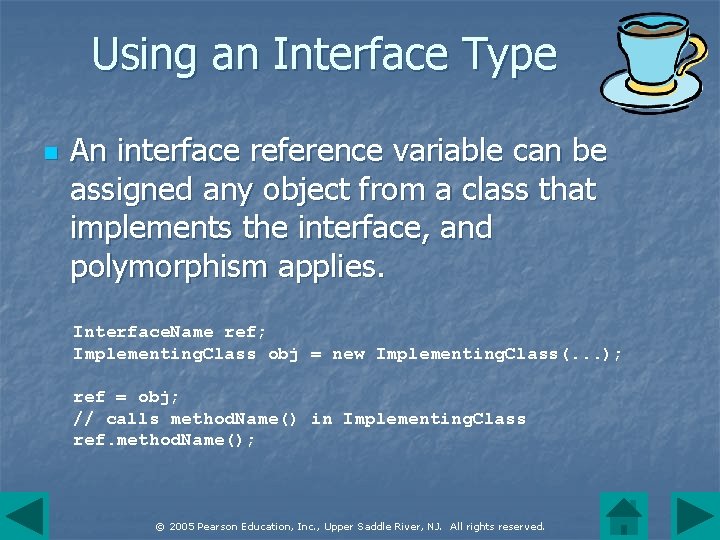
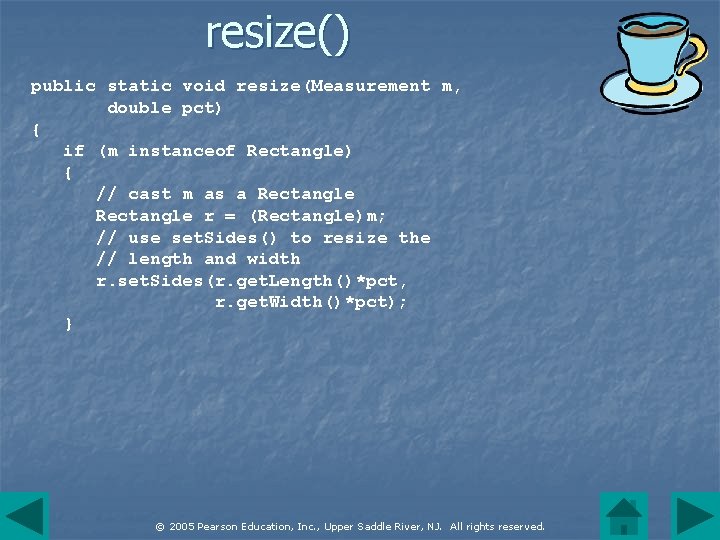
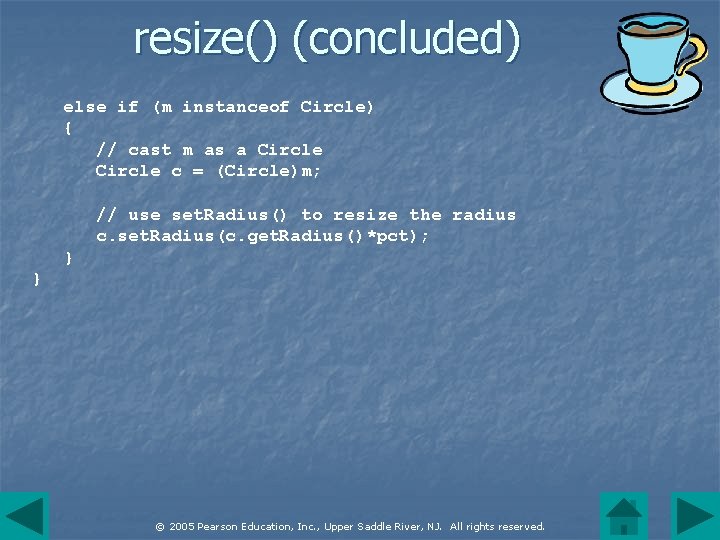
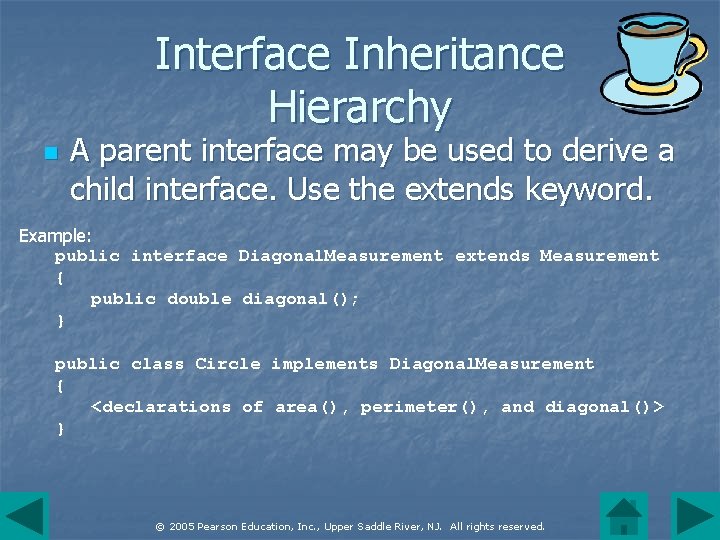
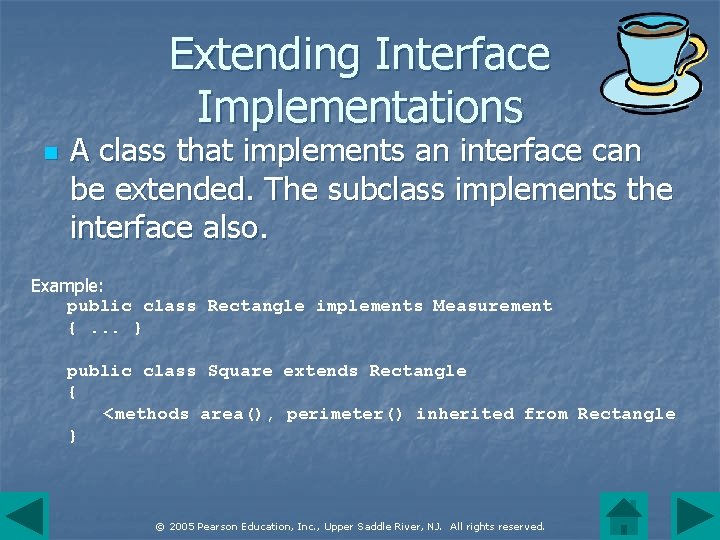
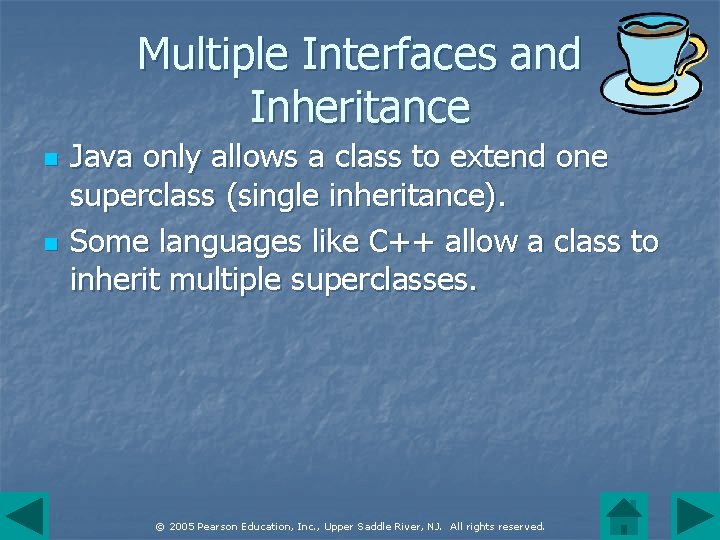
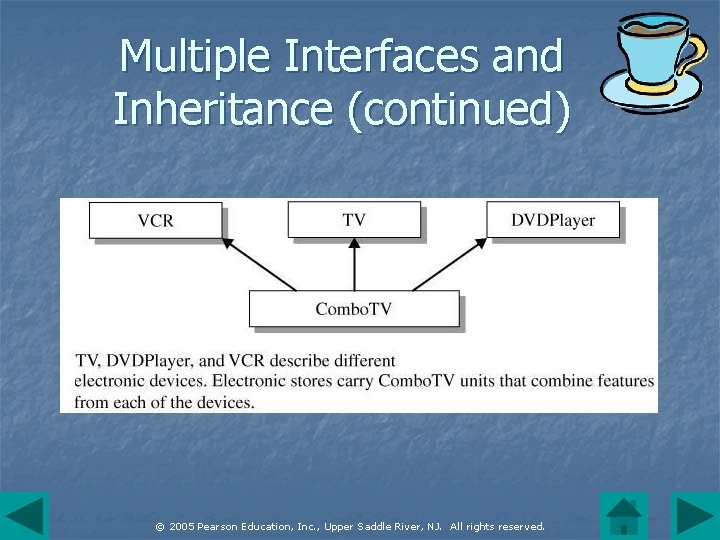
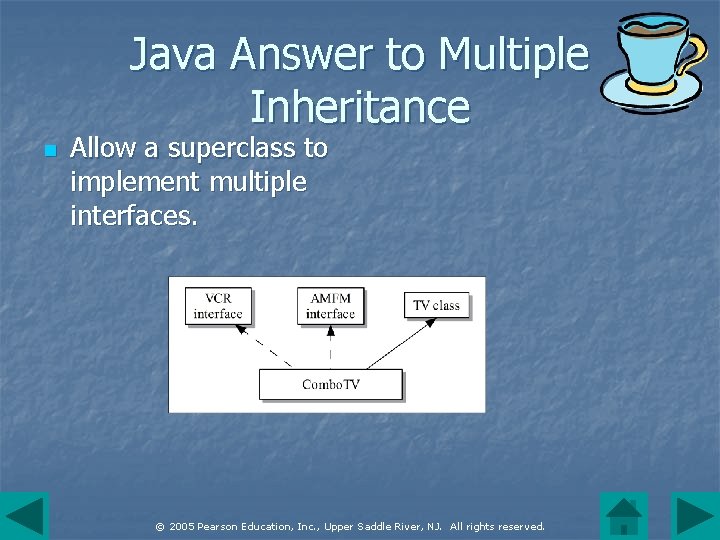
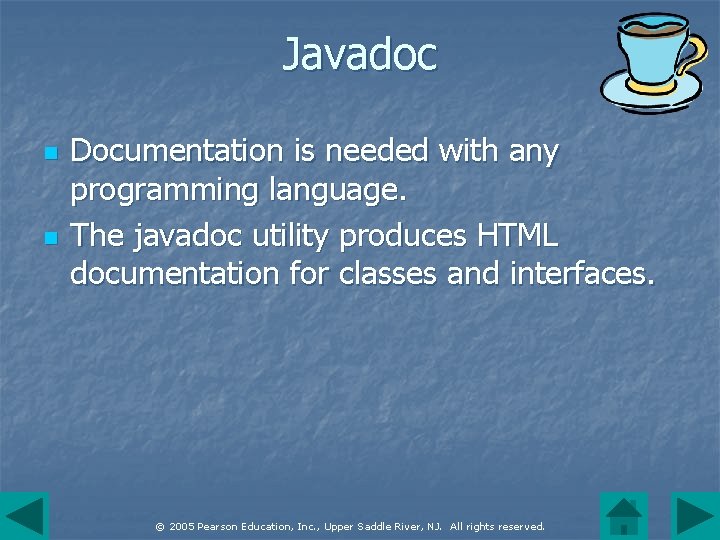
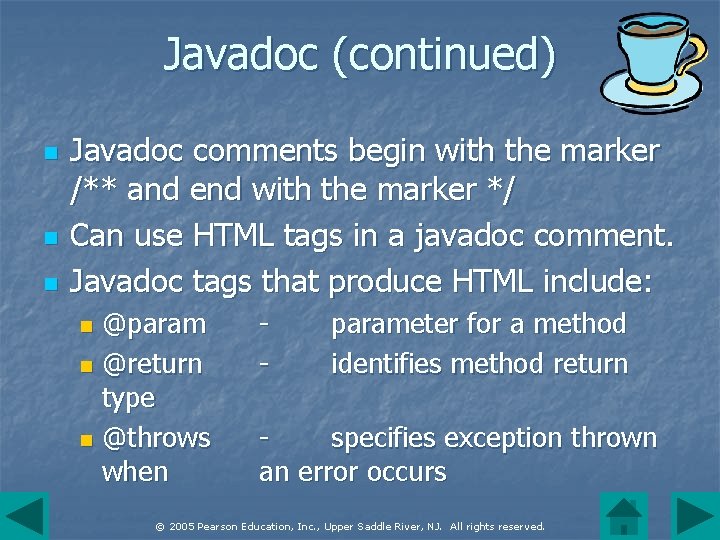
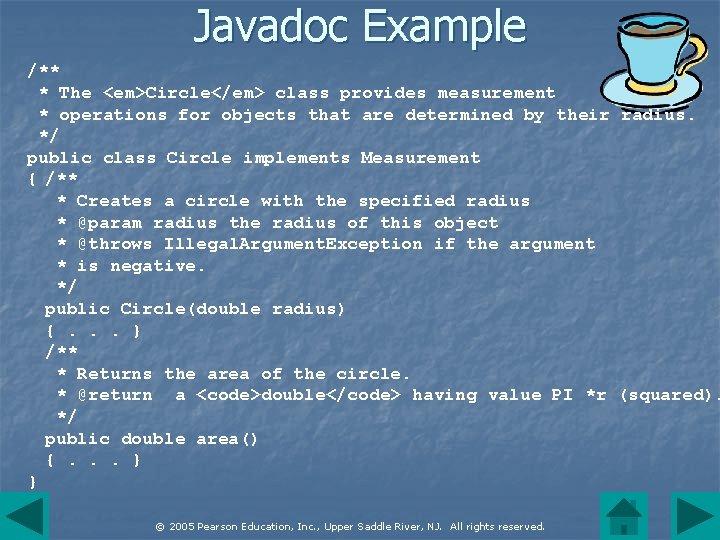
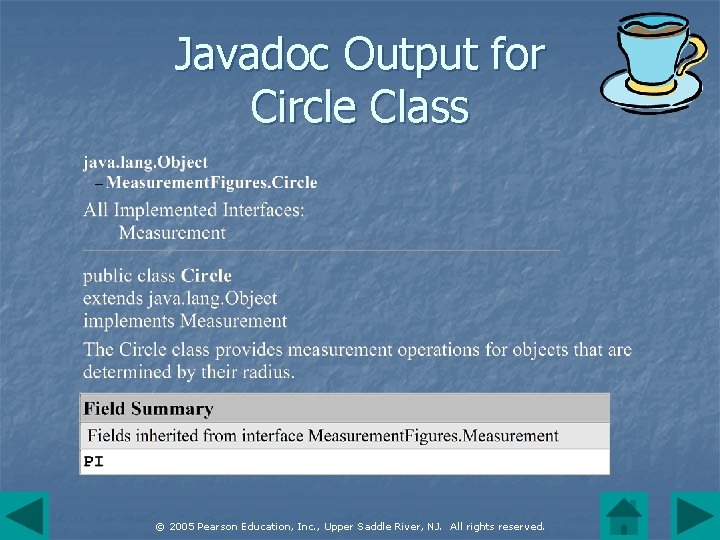
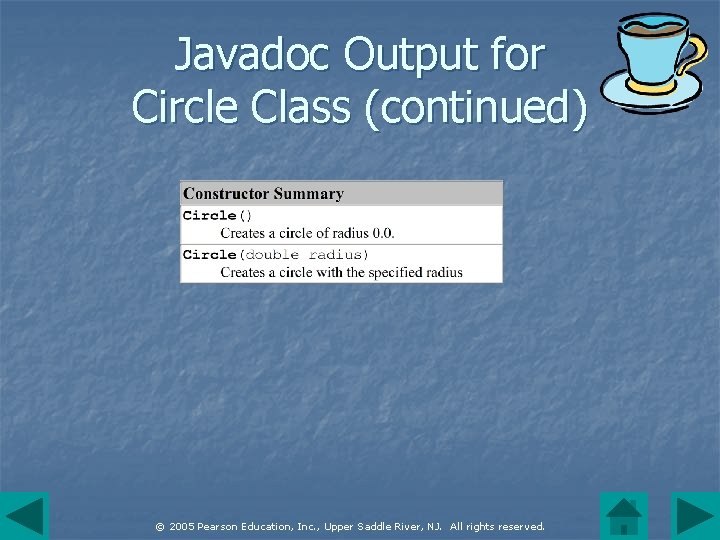
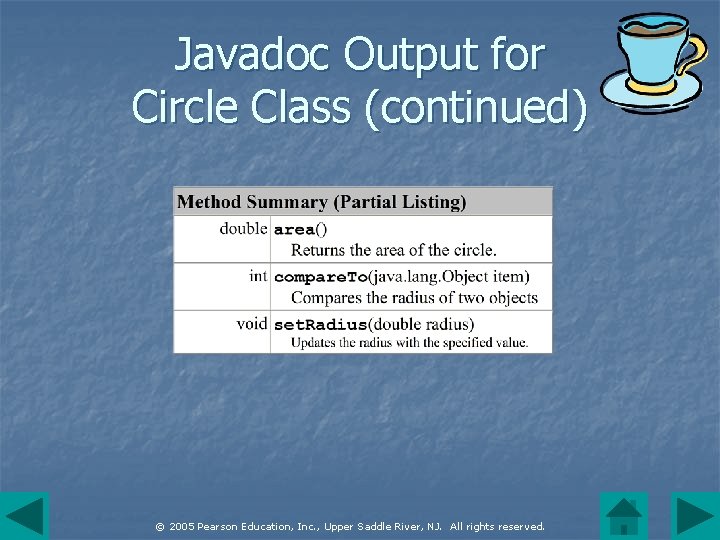
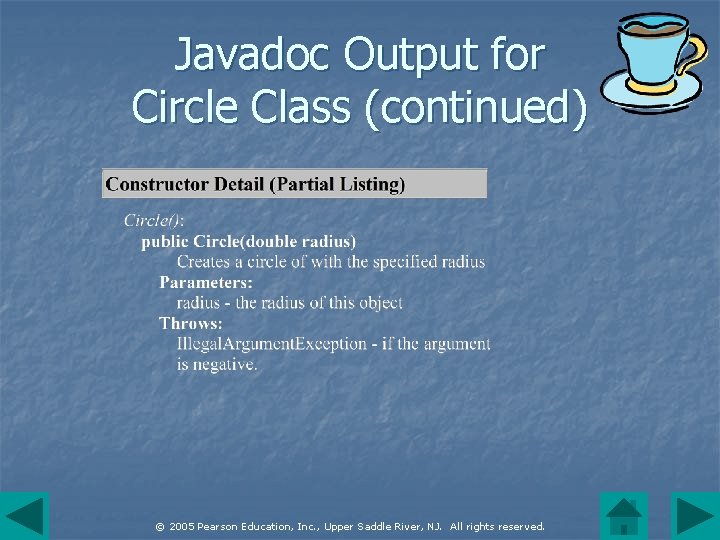
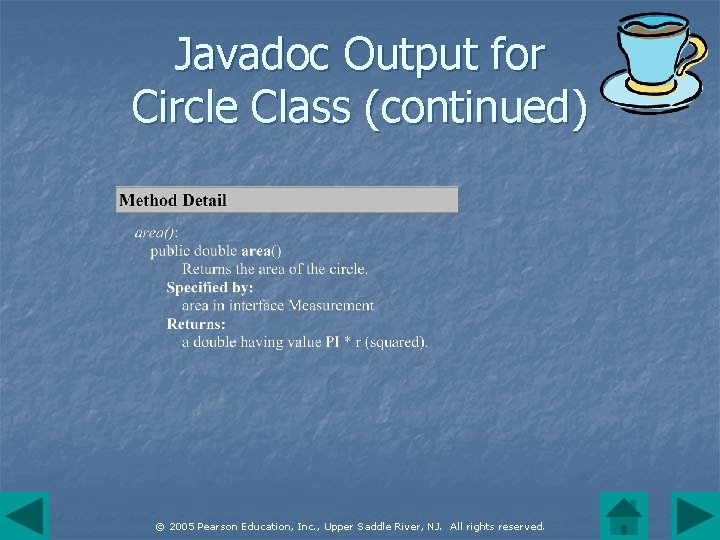
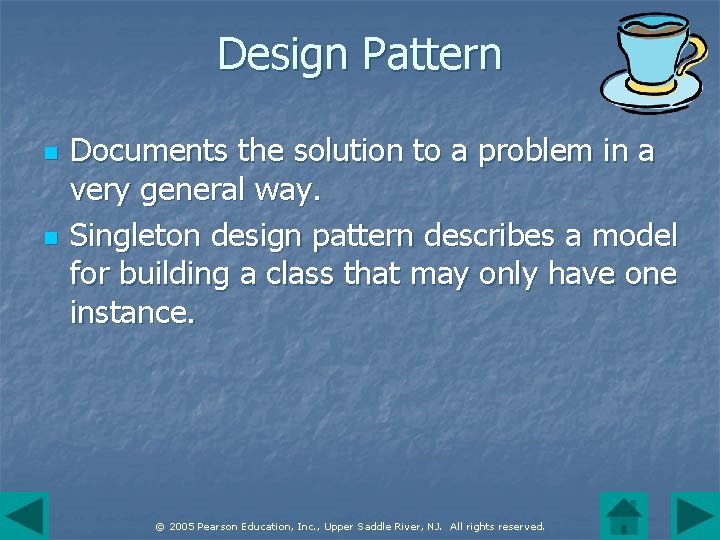
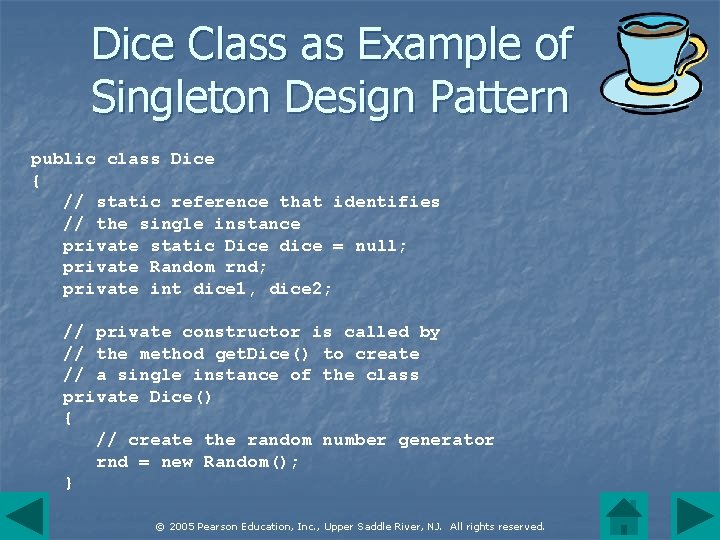
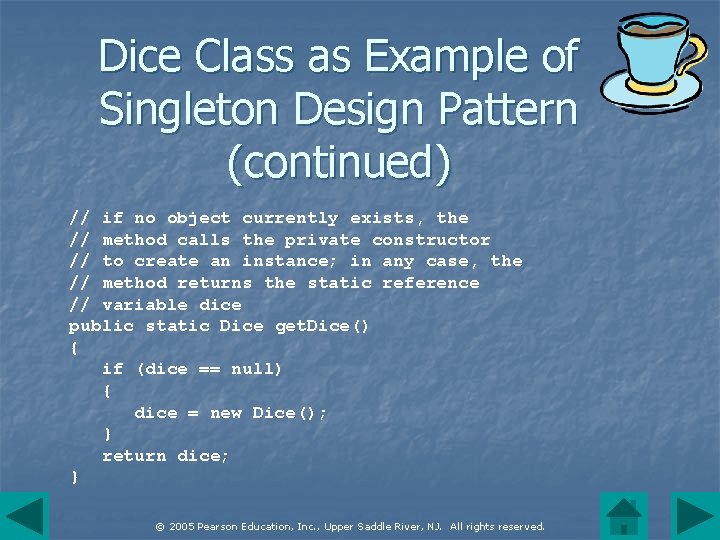
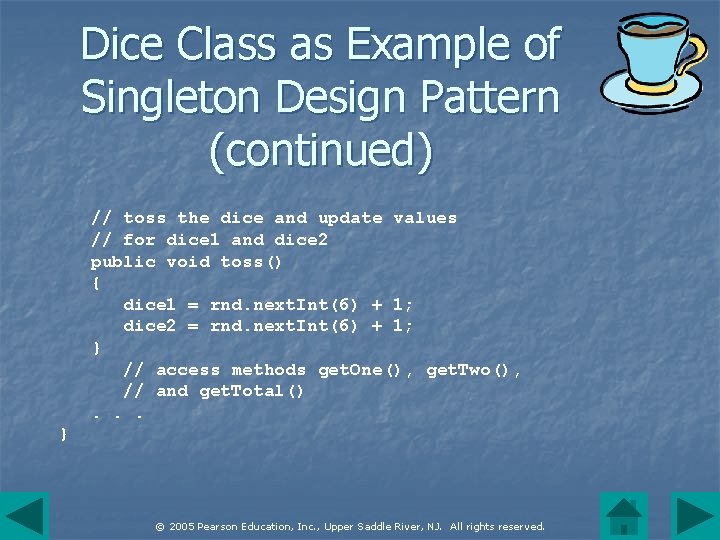
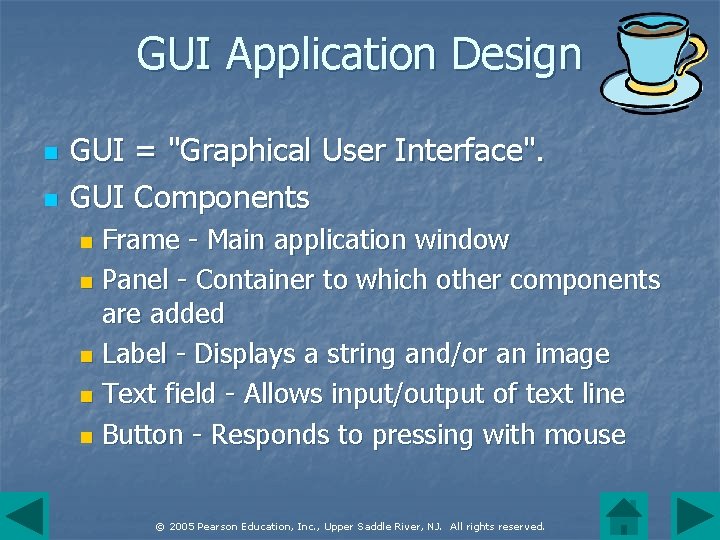
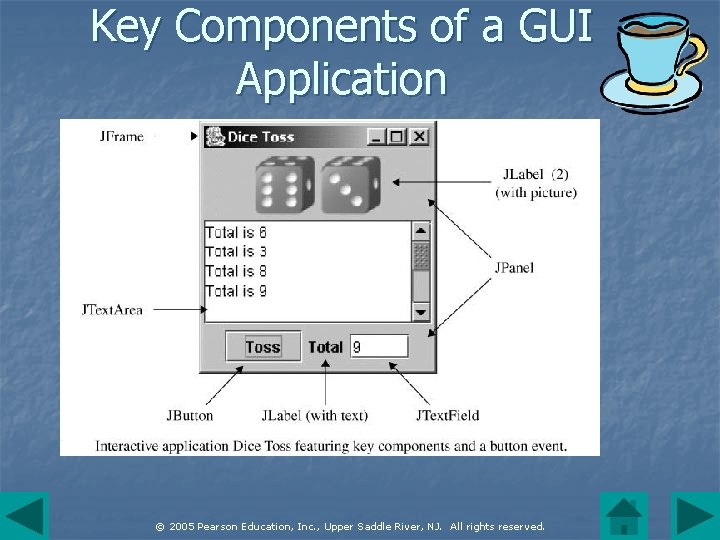
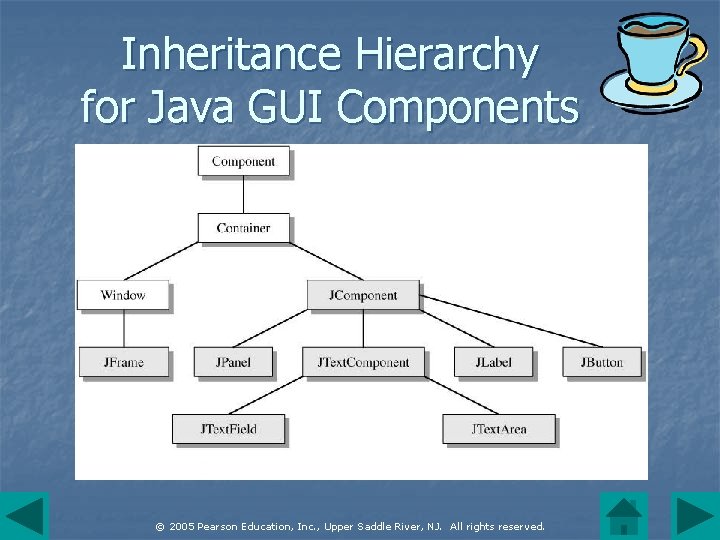
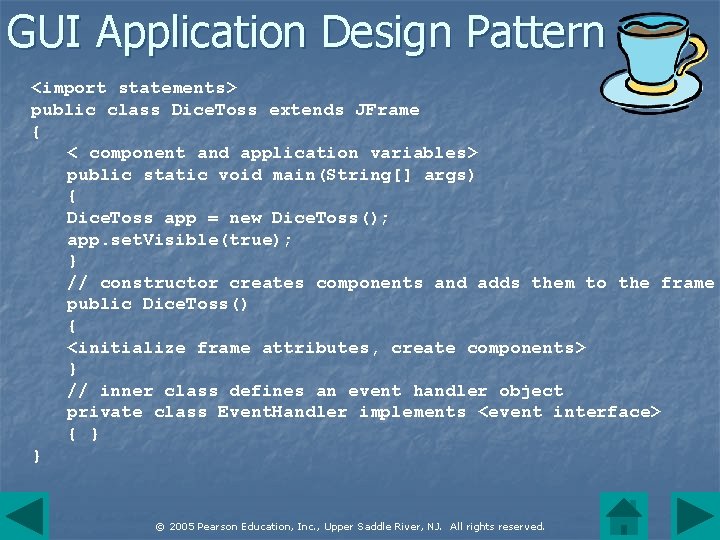
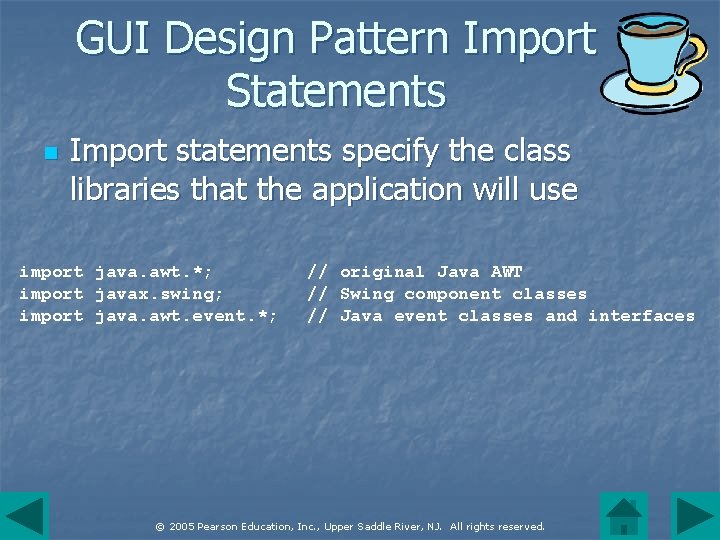
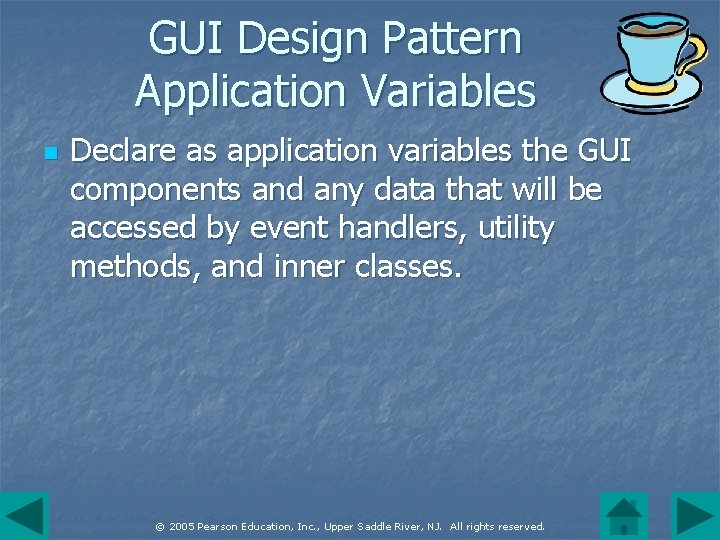
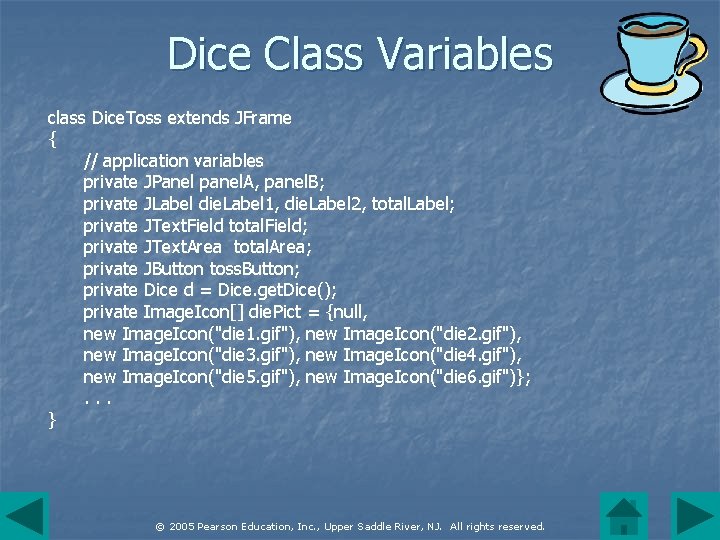
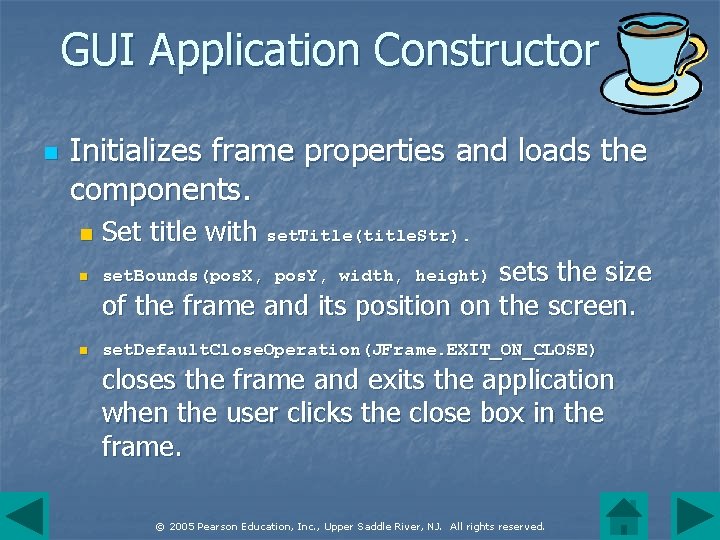
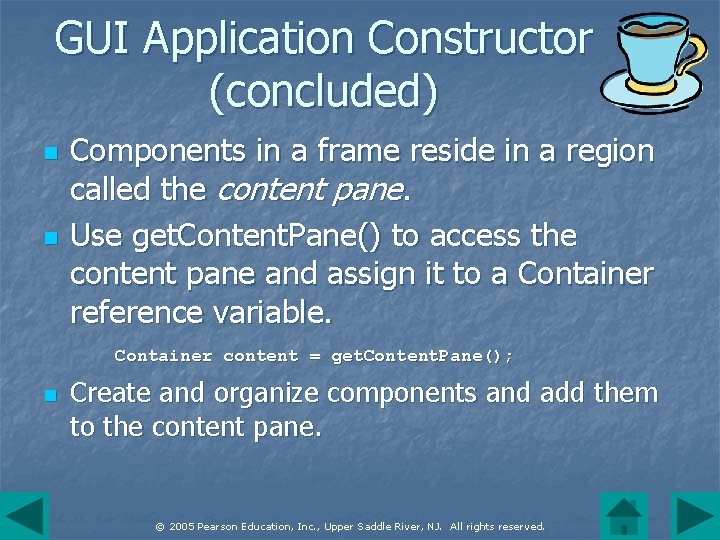
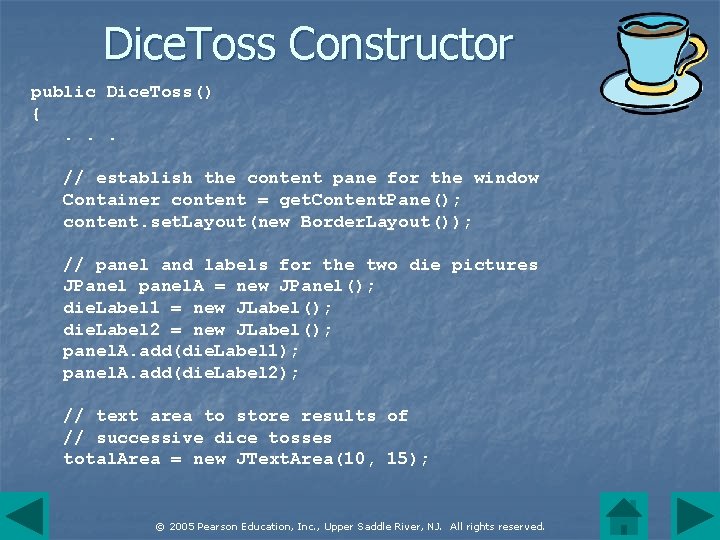
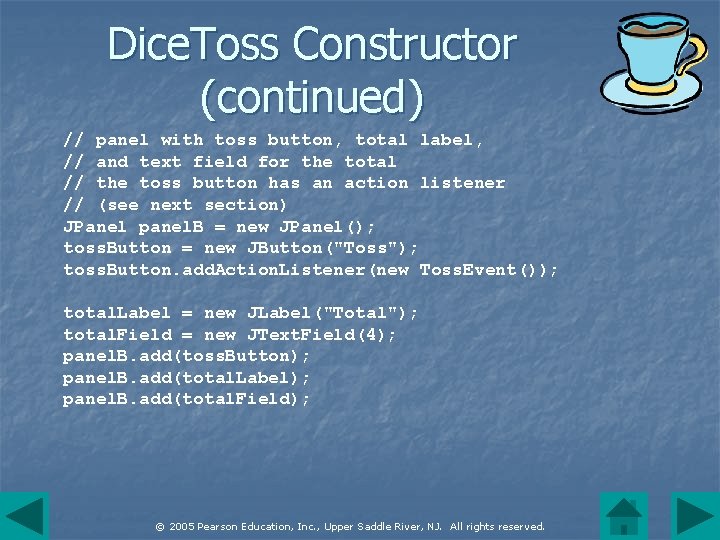
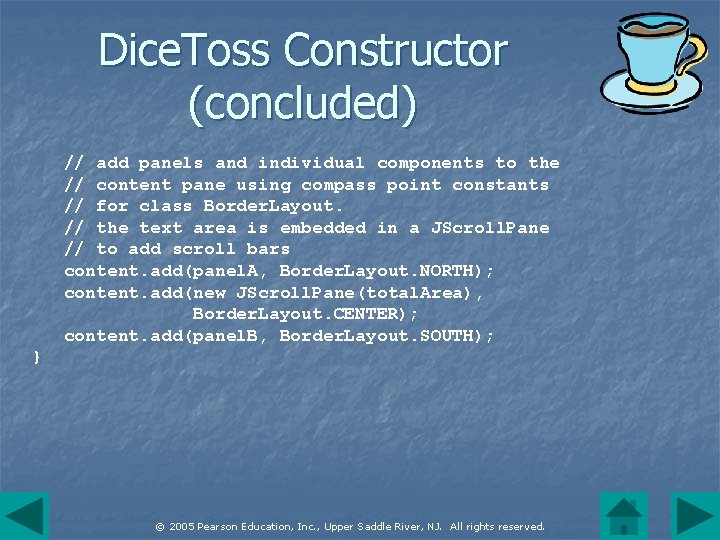
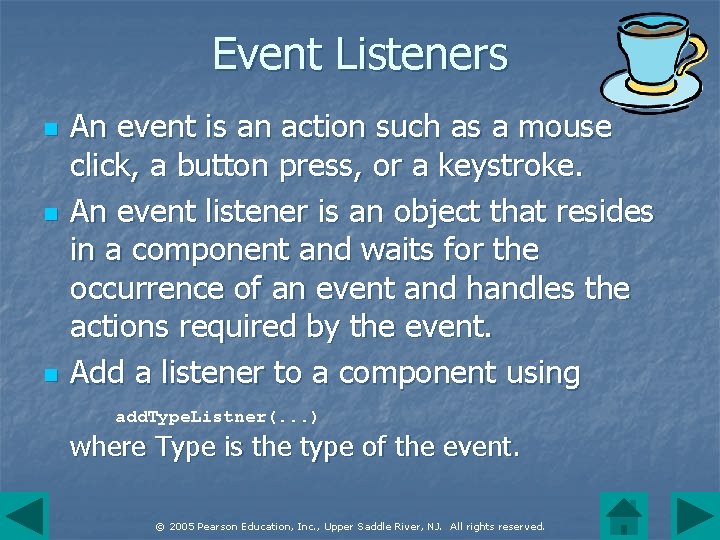
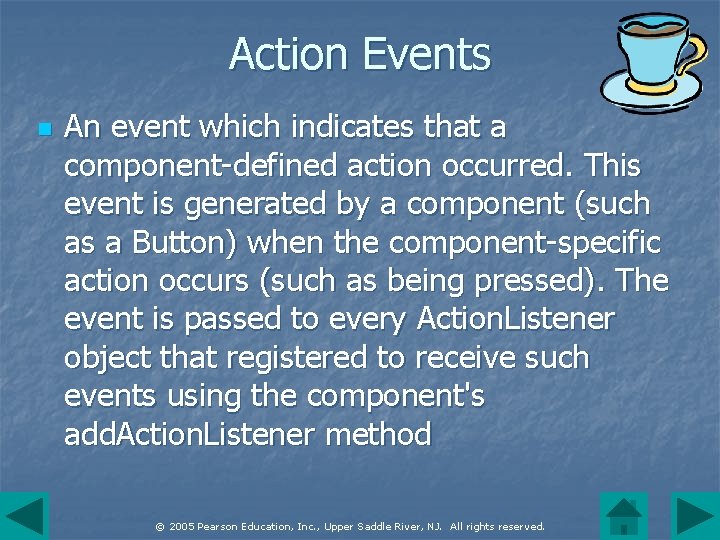
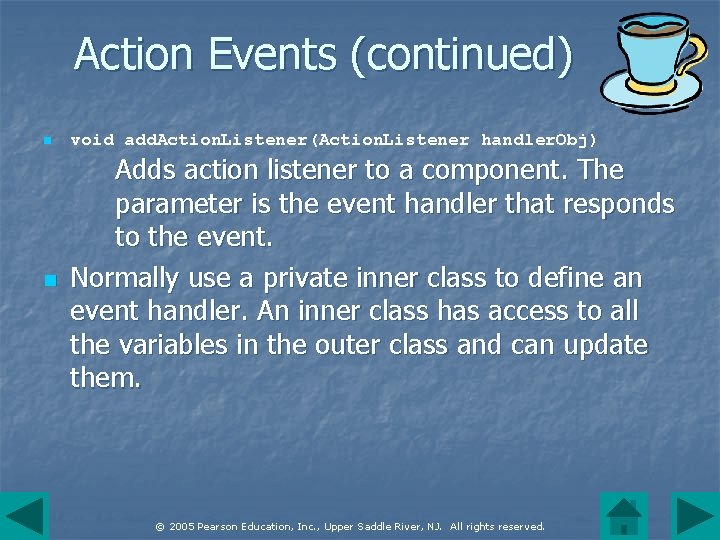
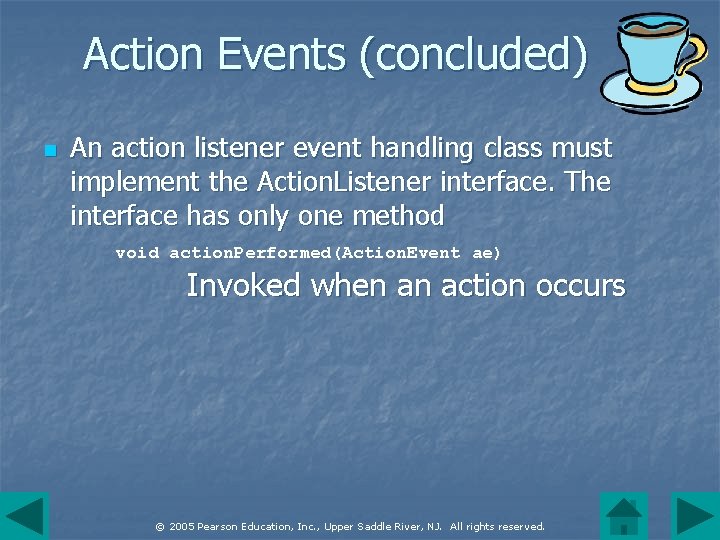
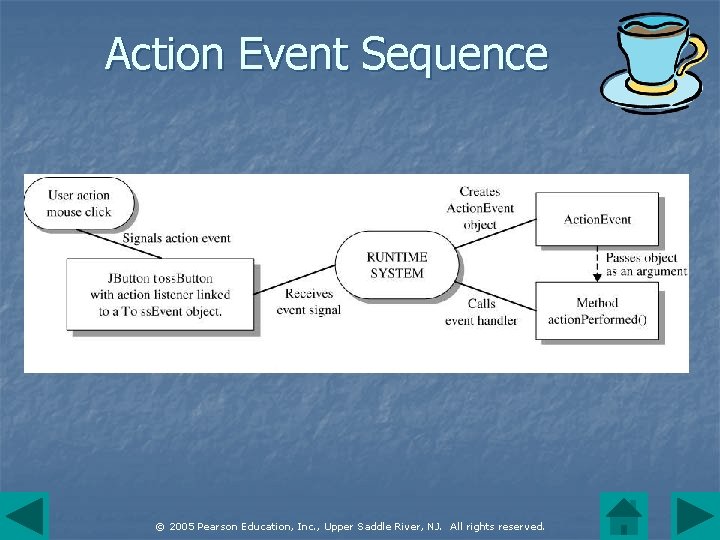
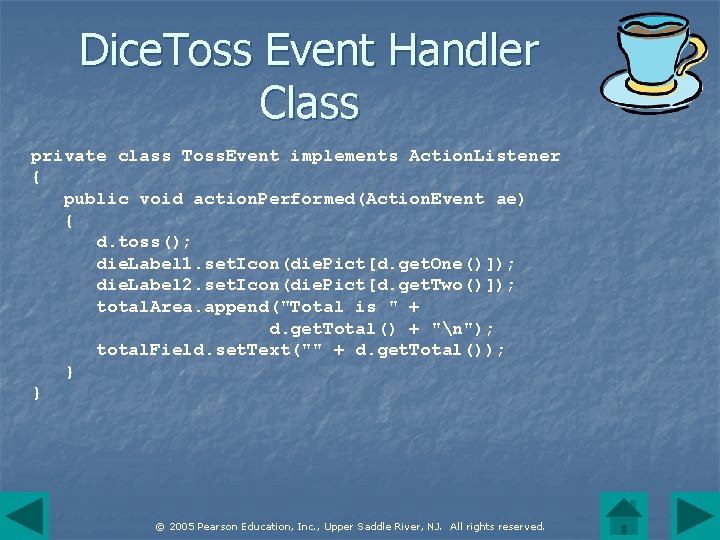
- Slides: 47
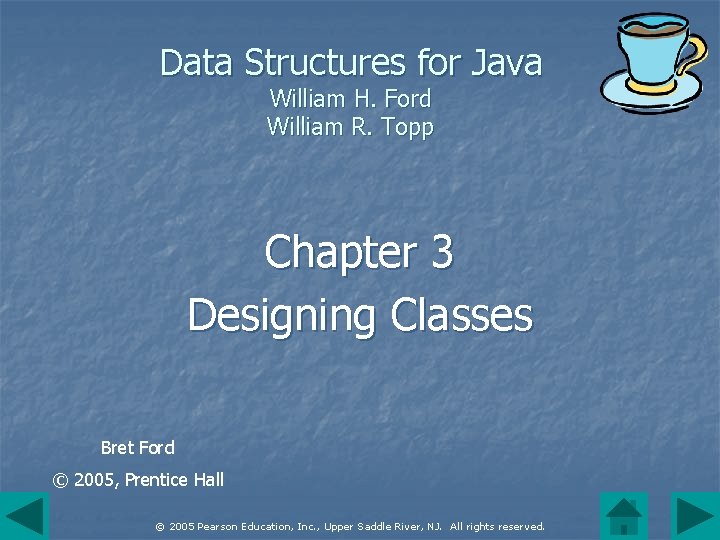
Data Structures for Java William H. Ford William R. Topp Chapter 3 Designing Classes Bret Ford © 2005, Prentice Hall © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
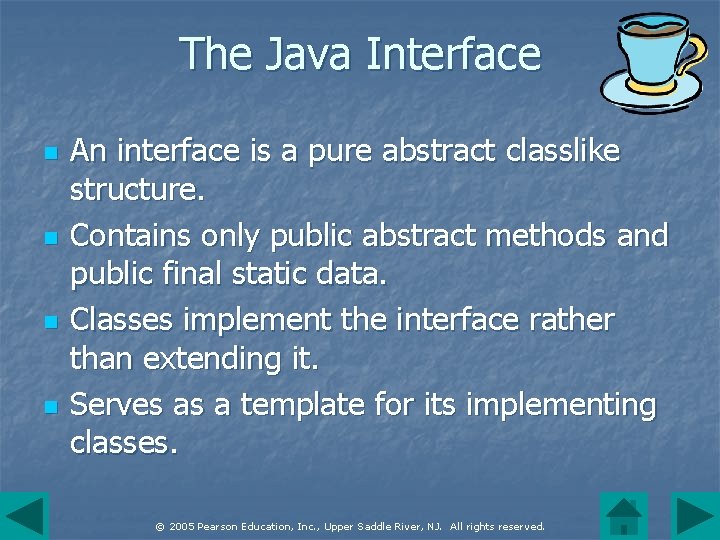
The Java Interface n n An interface is a pure abstract classlike structure. Contains only public abstract methods and public final static data. Classes implement the interface rather than extending it. Serves as a template for its implementing classes. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
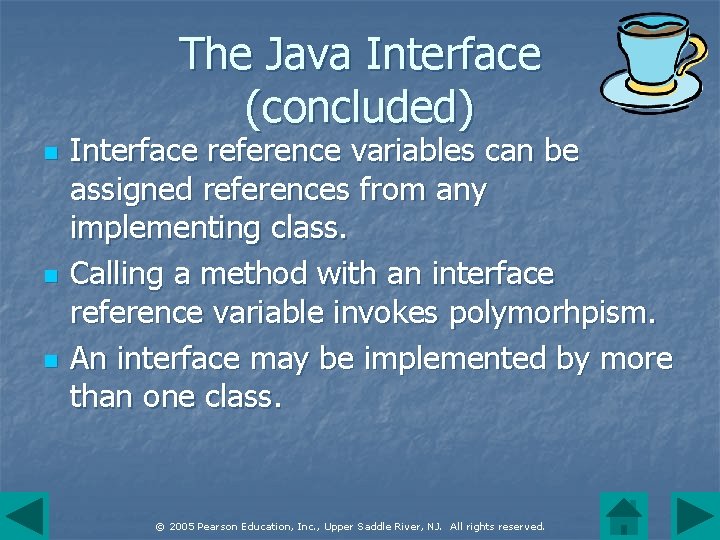
The Java Interface (concluded) n n n Interface reference variables can be assigned references from any implementing class. Calling a method with an interface reference variable invokes polymorhpism. An interface may be implemented by more than one class. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
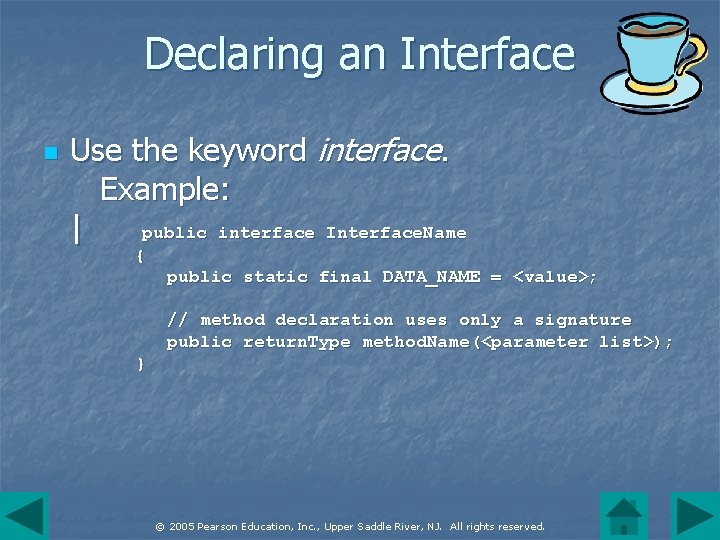
Declaring an Interface n Use the keyword interface. Example: | public interface Interface. Name { public static final DATA_NAME = <value>; // method declaration uses only a signature public return. Type method. Name(<parameter list>); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
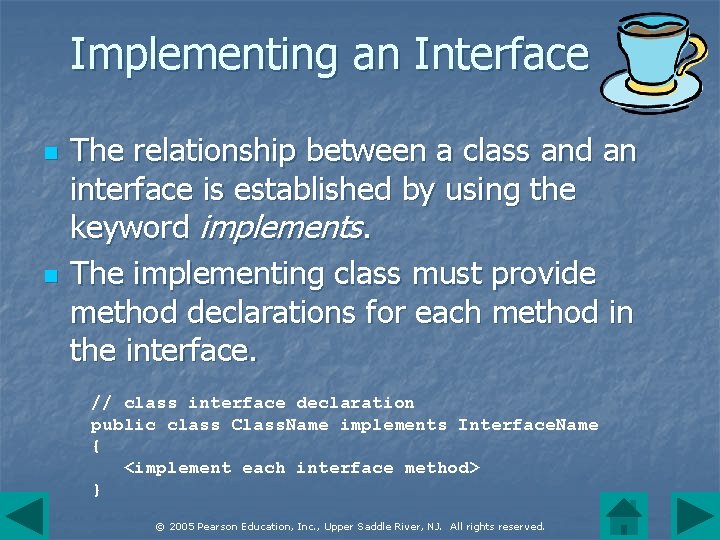
Implementing an Interface n n The relationship between a class and an interface is established by using the keyword implements. The implementing class must provide method declarations for each method in the interface. // class interface declaration public class Class. Name implements Interface. Name { <implement each interface method> } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
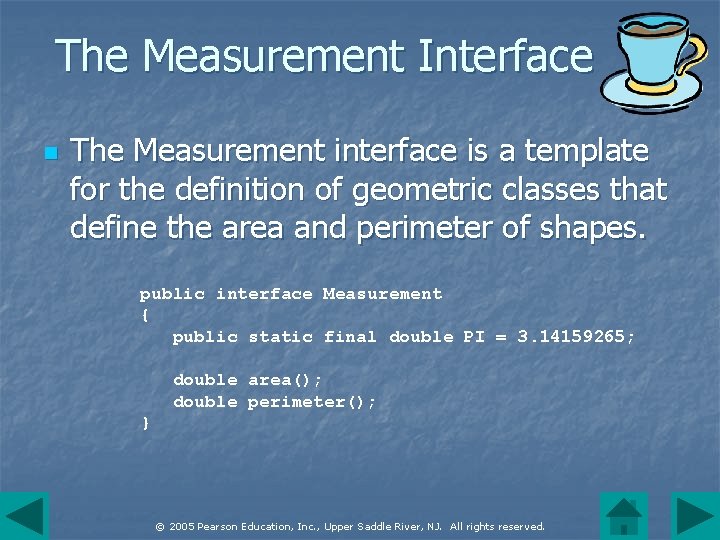
The Measurement Interface n The Measurement interface is a template for the definition of geometric classes that define the area and perimeter of shapes. public interface Measurement { public static final double PI = 3. 14159265; double area(); double perimeter(); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
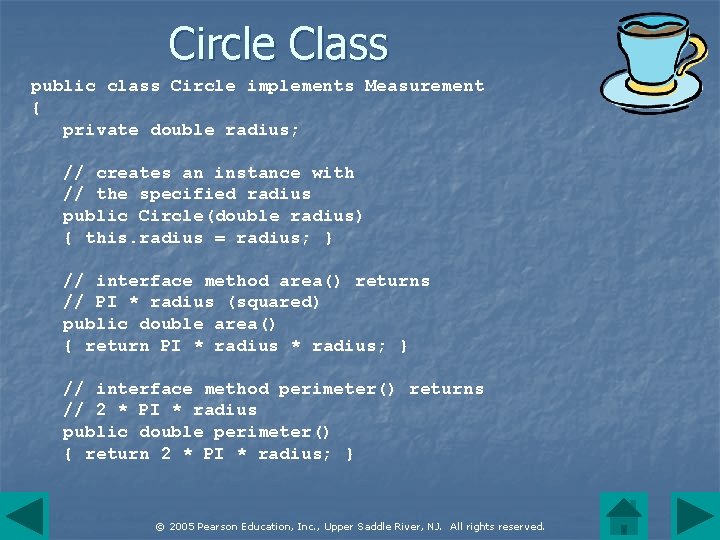
Circle Class public class Circle implements Measurement { private double radius; // creates an instance with // the specified radius public Circle(double radius) { this. radius = radius; } // interface method area() returns // PI * radius (squared) public double area() { return PI * radius; } // interface method perimeter() returns // 2 * PI * radius public double perimeter() { return 2 * PI * radius; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
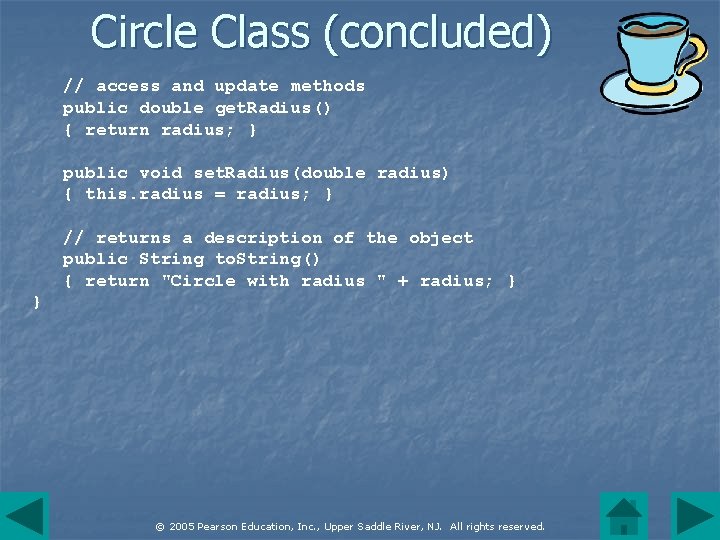
Circle Class (concluded) // access and update methods public double get. Radius() { return radius; } public void set. Radius(double radius) { this. radius = radius; } // returns a description of the object public String to. String() { return "Circle with radius " + radius; } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
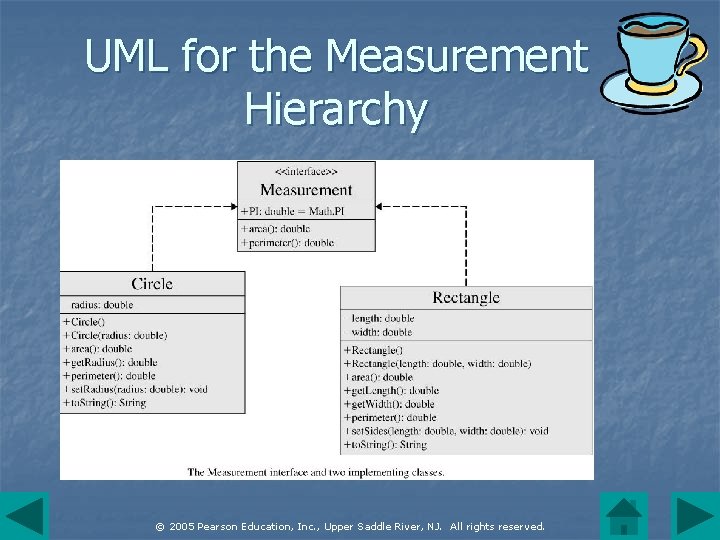
UML for the Measurement Hierarchy © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
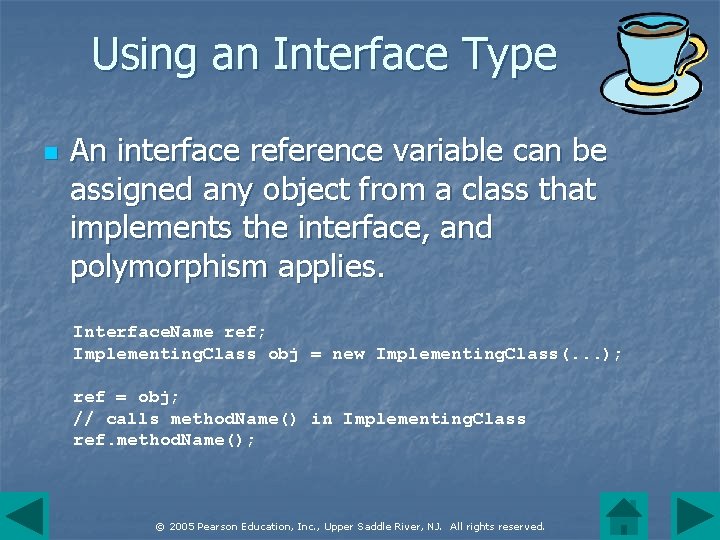
Using an Interface Type n An interface reference variable can be assigned any object from a class that implements the interface, and polymorphism applies. Interface. Name ref; Implementing. Class obj = new Implementing. Class(. . . ); ref = obj; // calls method. Name() in Implementing. Class ref. method. Name(); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
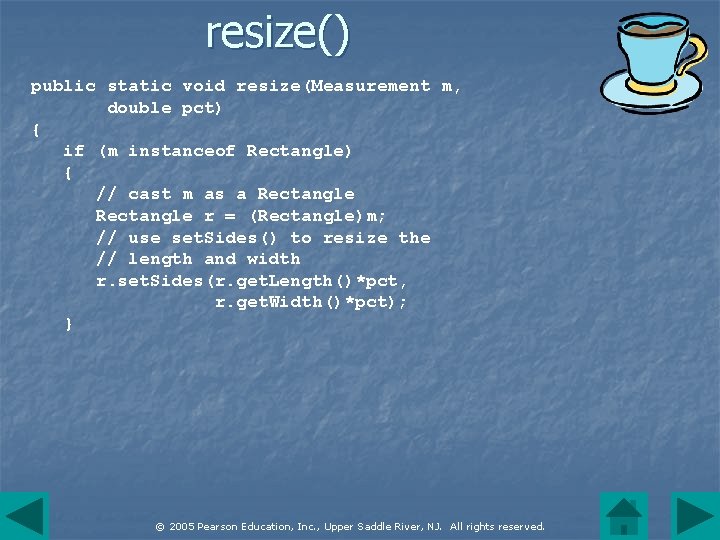
resize() public static void resize(Measurement m, double pct) { if (m instanceof Rectangle) { // cast m as a Rectangle r = (Rectangle)m; // use set. Sides() to resize the // length and width r. set. Sides(r. get. Length()*pct, r. get. Width()*pct); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
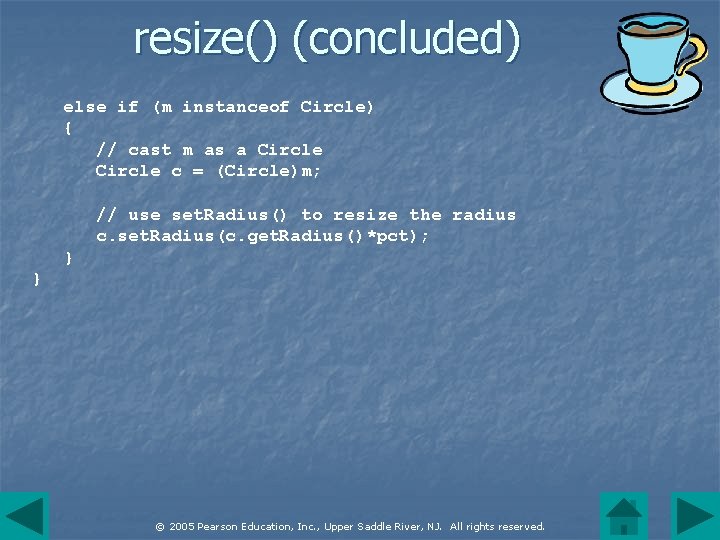
resize() (concluded) else if (m instanceof Circle) { // cast m as a Circle c = (Circle)m; // use set. Radius() to resize the radius c. set. Radius(c. get. Radius()*pct); } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
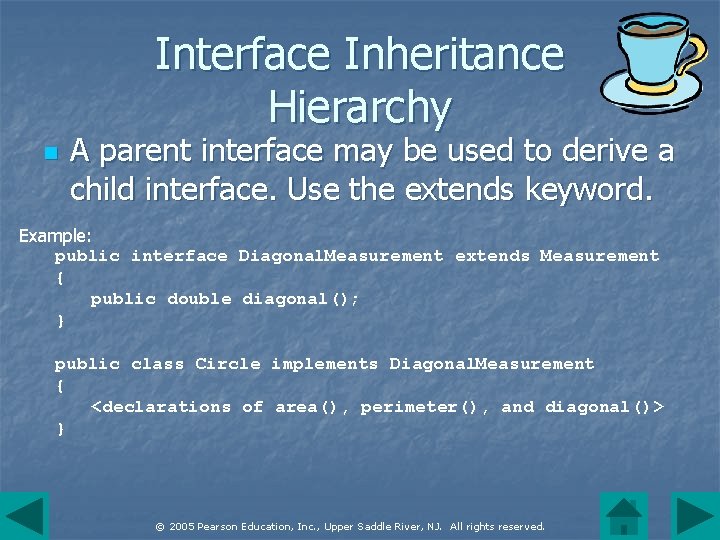
Interface Inheritance Hierarchy n A parent interface may be used to derive a child interface. Use the extends keyword. Example: public interface Diagonal. Measurement extends Measurement { public double diagonal(); } public class Circle implements Diagonal. Measurement { <declarations of area(), perimeter(), and diagonal()> } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
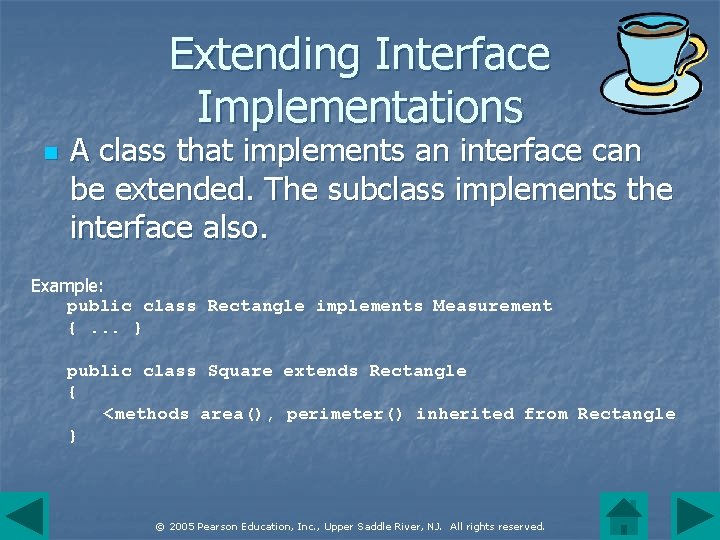
Extending Interface Implementations n A class that implements an interface can be extended. The subclass implements the interface also. Example: public class Rectangle implements Measurement {. . . } public class Square extends Rectangle { <methods area(), perimeter() inherited from Rectangle } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
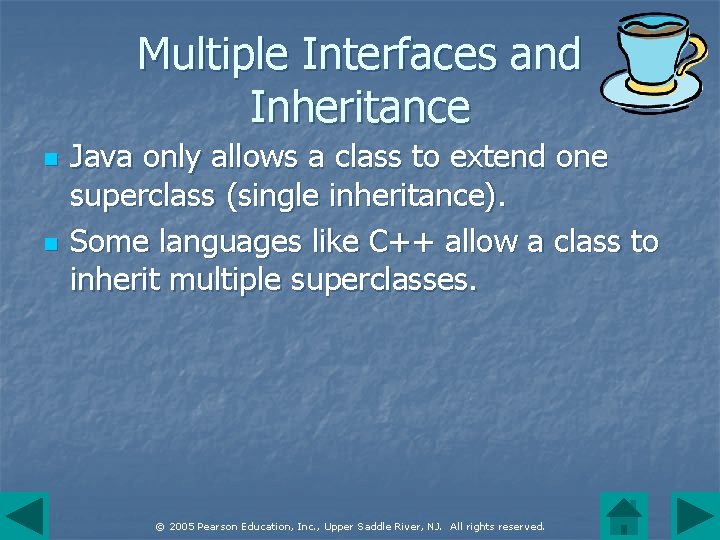
Multiple Interfaces and Inheritance n n Java only allows a class to extend one superclass (single inheritance). Some languages like C++ allow a class to inherit multiple superclasses. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
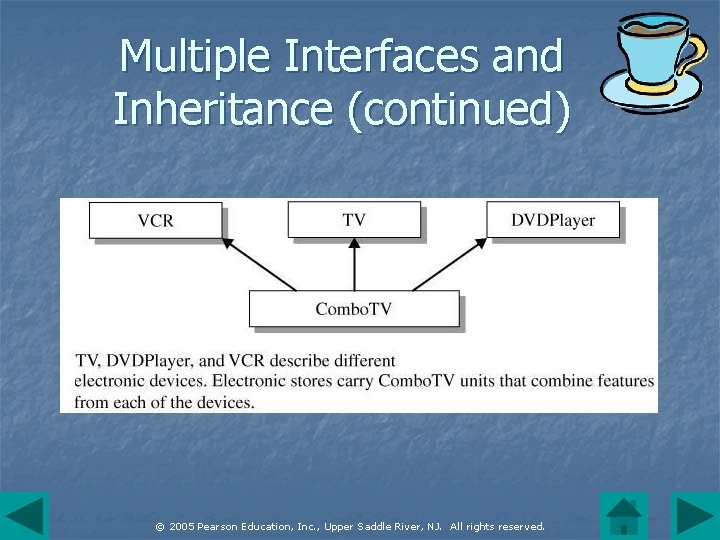
Multiple Interfaces and Inheritance (continued) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
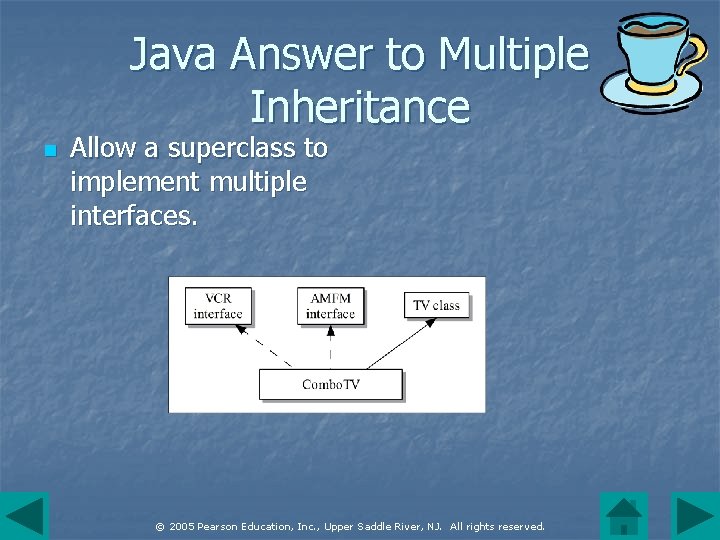
Java Answer to Multiple Inheritance n Allow a superclass to implement multiple interfaces. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
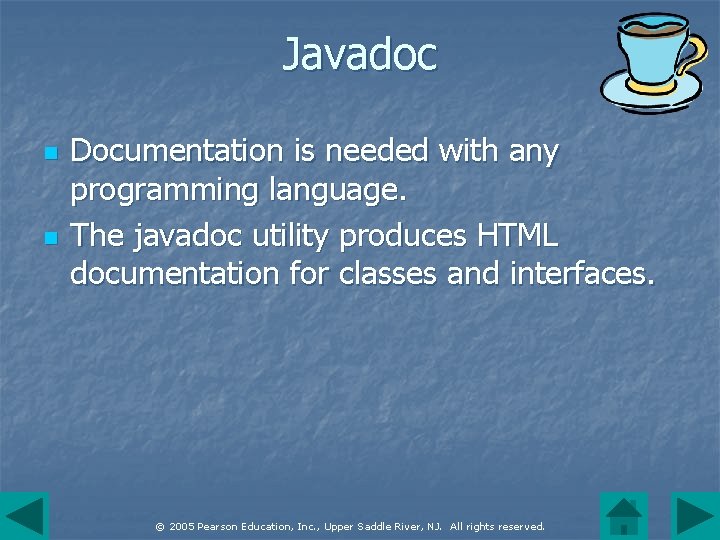
Javadoc n n Documentation is needed with any programming language. The javadoc utility produces HTML documentation for classes and interfaces. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
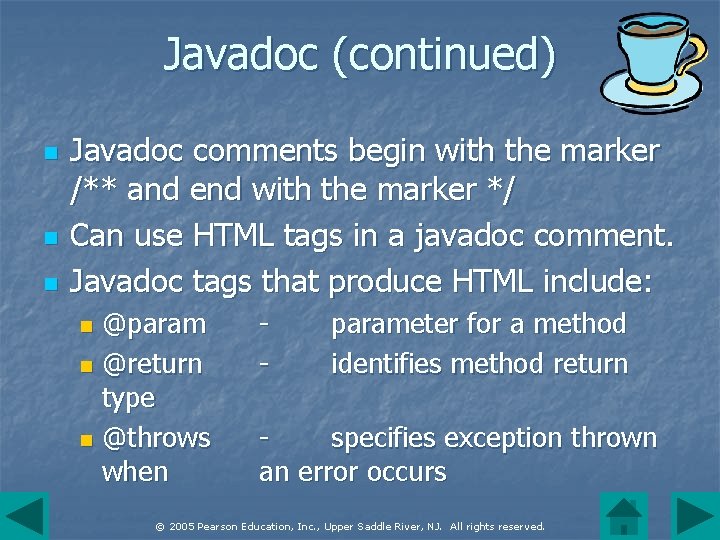
Javadoc (continued) n n n Javadoc comments begin with the marker /** and end with the marker */ Can use HTML tags in a javadoc comment. Javadoc tags that produce HTML include: @param n @return type n @throws when n - parameter for a method identifies method return specifies exception thrown an error occurs © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
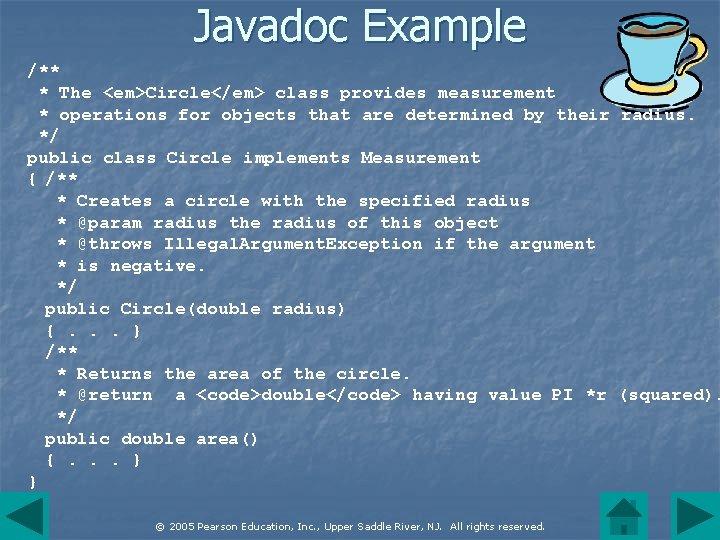
Javadoc Example /** * The <em>Circle</em> class provides measurement * operations for objects that are determined by their radius. */ public class Circle implements Measurement { /** * Creates a circle with the specified radius * @param radius the radius of this object * @throws Illegal. Argument. Exception if the argument * is negative. */ public Circle(double radius) {. . . } /** * Returns the area of the circle. * @return a <code>double</code> having value PI *r (squared). */ public double area() {. . . } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
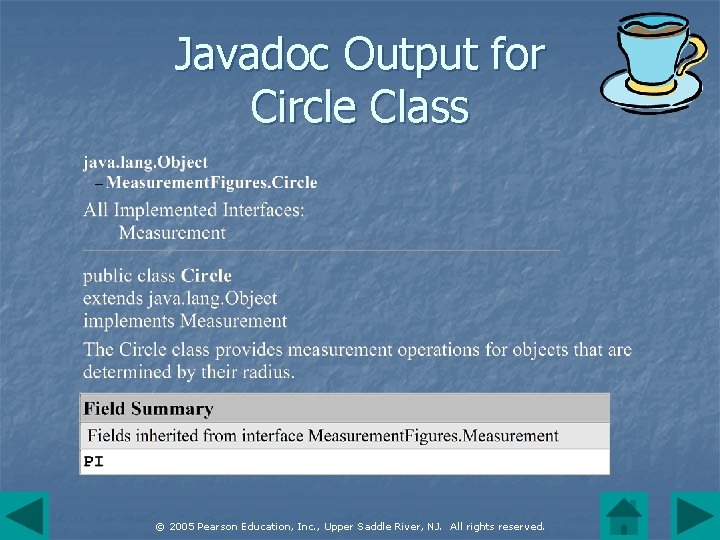
Javadoc Output for Circle Class © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
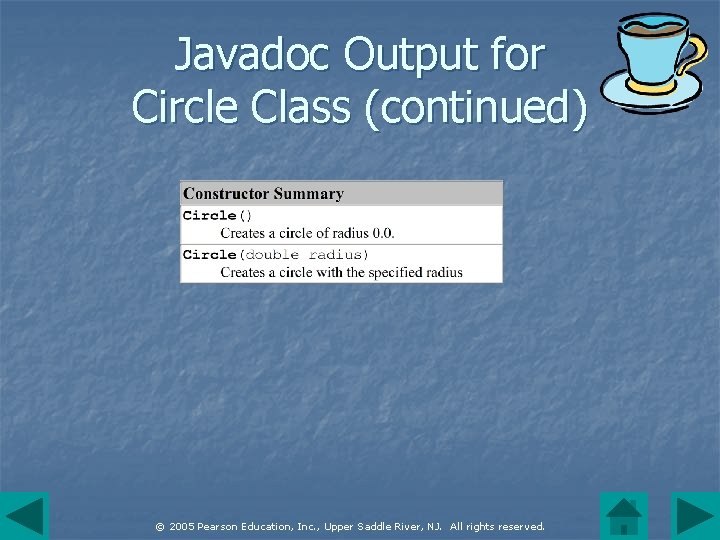
Javadoc Output for Circle Class (continued) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
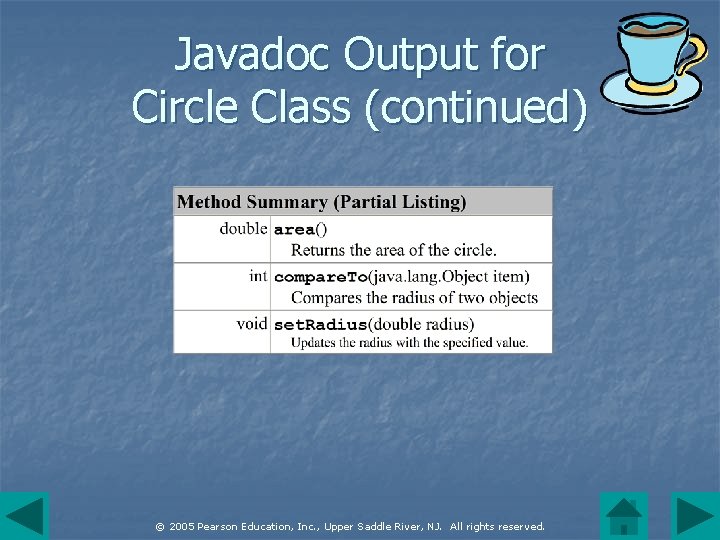
Javadoc Output for Circle Class (continued) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
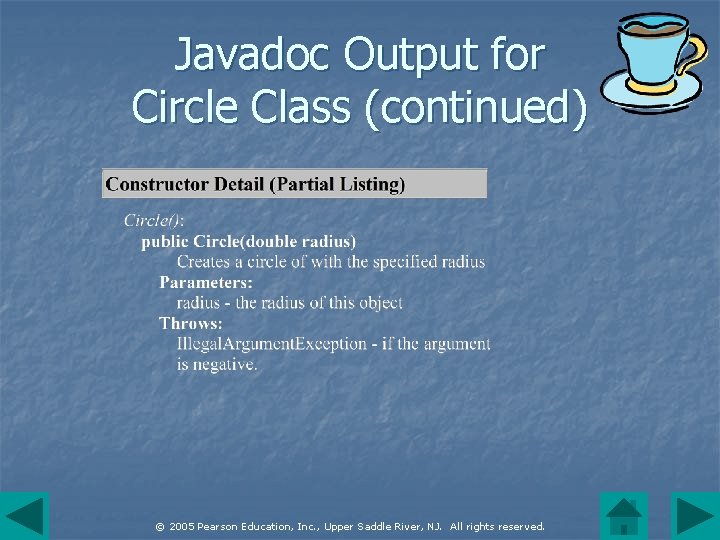
Javadoc Output for Circle Class (continued) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
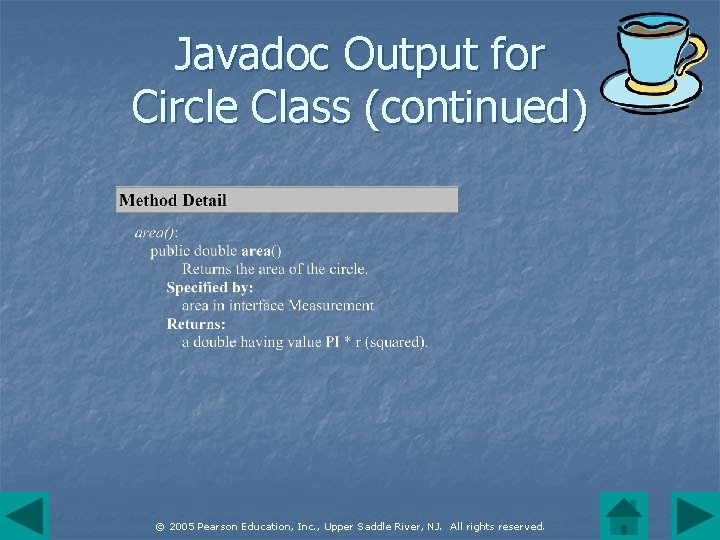
Javadoc Output for Circle Class (continued) © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
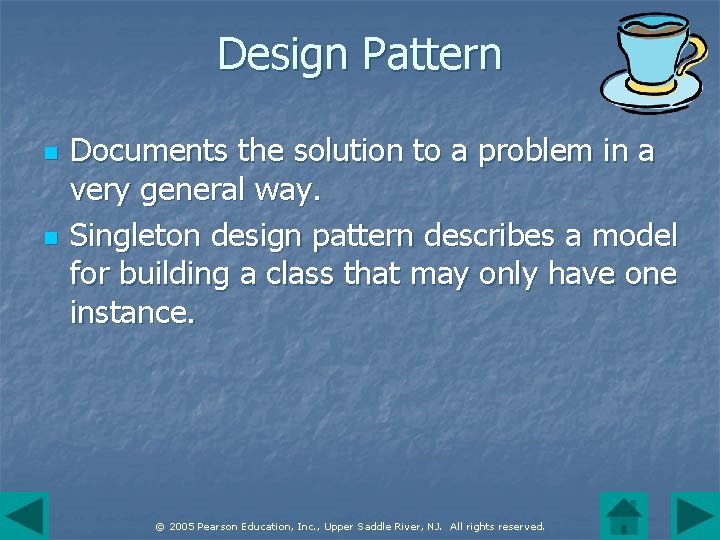
Design Pattern n n Documents the solution to a problem in a very general way. Singleton design pattern describes a model for building a class that may only have one instance. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
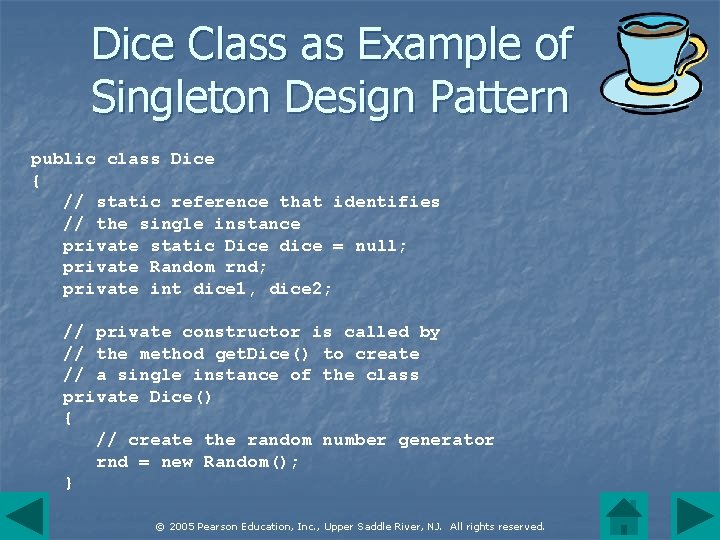
Dice Class as Example of Singleton Design Pattern public class Dice { // static reference that identifies // the single instance private static Dice dice = null; private Random rnd; private int dice 1, dice 2; // private constructor is called by // the method get. Dice() to create // a single instance of the class private Dice() { // create the random number generator rnd = new Random(); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
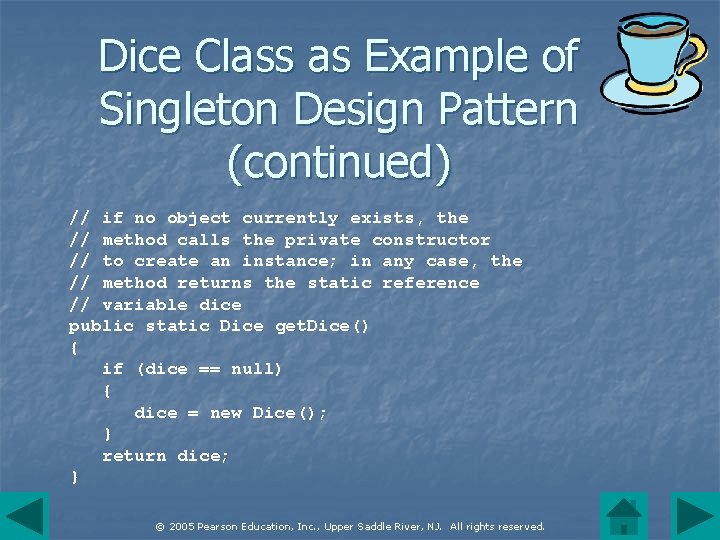
Dice Class as Example of Singleton Design Pattern (continued) // if no object currently exists, the // method calls the private constructor // to create an instance; in any case, the // method returns the static reference // variable dice public static Dice get. Dice() { if (dice == null) { dice = new Dice(); } return dice; } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
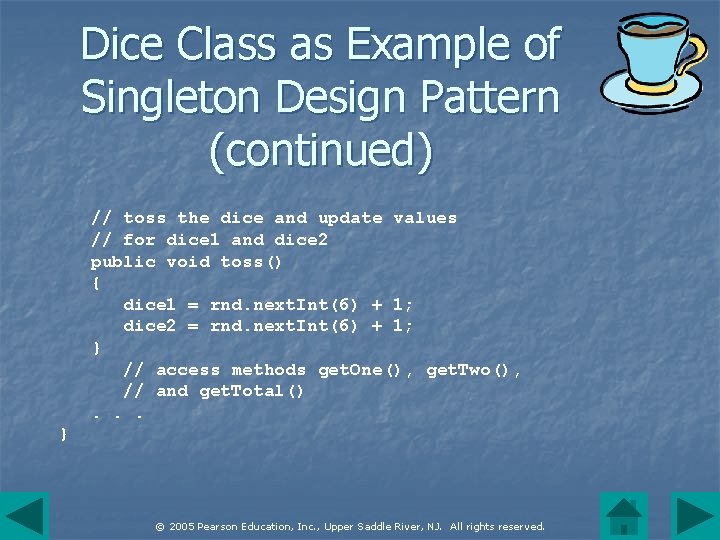
Dice Class as Example of Singleton Design Pattern (continued) // toss the dice and update values // for dice 1 and dice 2 public void toss() { dice 1 = rnd. next. Int(6) + 1; dice 2 = rnd. next. Int(6) + 1; } // access methods get. One(), get. Two(), // and get. Total(). . . } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
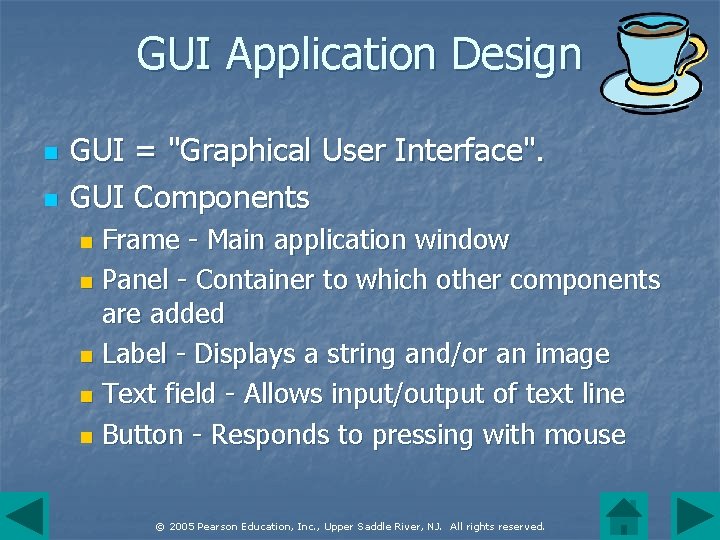
GUI Application Design n n GUI = "Graphical User Interface". GUI Components Frame - Main application window n Panel - Container to which other components are added n Label - Displays a string and/or an image n Text field - Allows input/output of text line n Button - Responds to pressing with mouse n © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
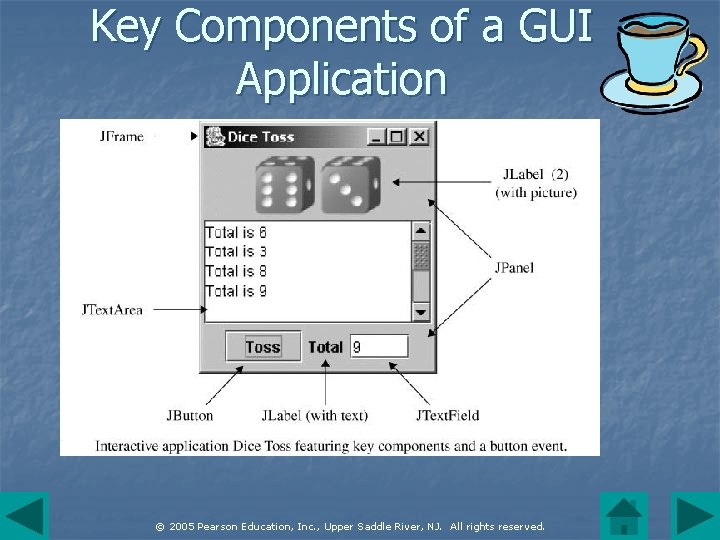
Key Components of a GUI Application © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
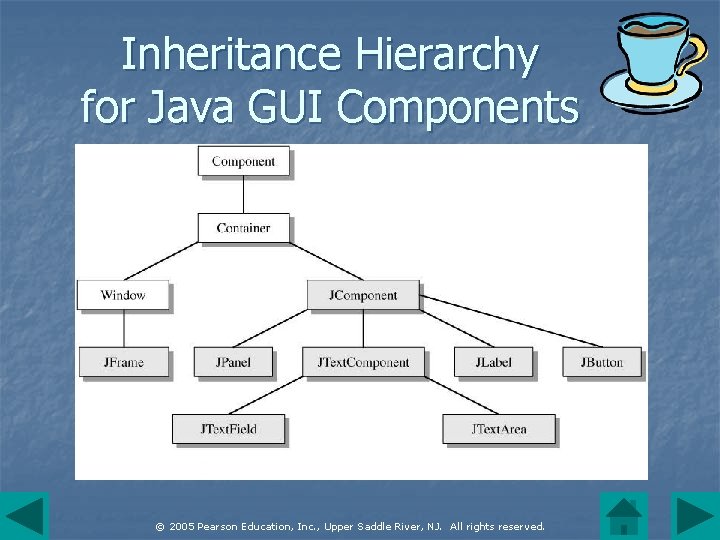
Inheritance Hierarchy for Java GUI Components © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
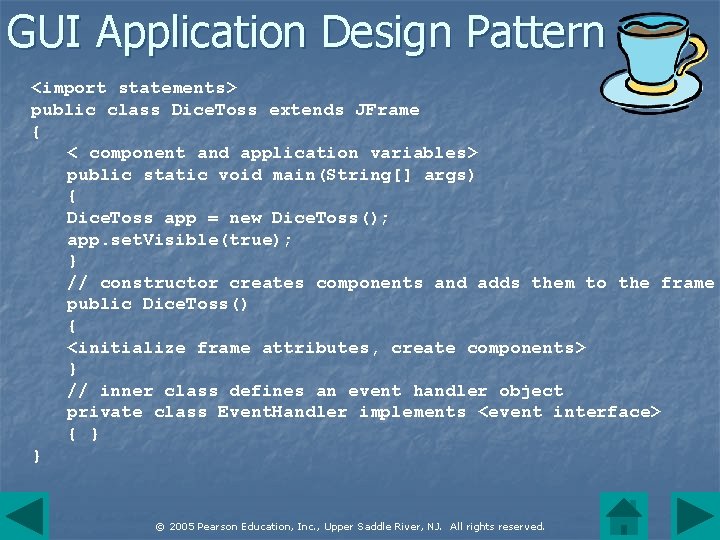
GUI Application Design Pattern <import statements> public class Dice. Toss extends JFrame { < component and application variables> public static void main(String[] args) { Dice. Toss app = new Dice. Toss(); app. set. Visible(true); } // constructor creates components and adds them to the frame public Dice. Toss() { <initialize frame attributes, create components> } // inner class defines an event handler object private class Event. Handler implements <event interface> { } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
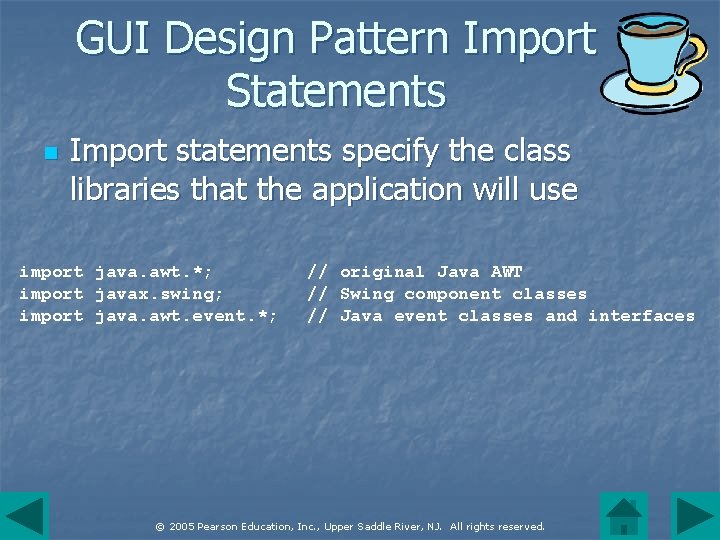
GUI Design Pattern Import Statements n Import statements specify the class libraries that the application will use import java. awt. *; import javax. swing; import java. awt. event. *; // original Java AWT // Swing component classes // Java event classes and interfaces © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
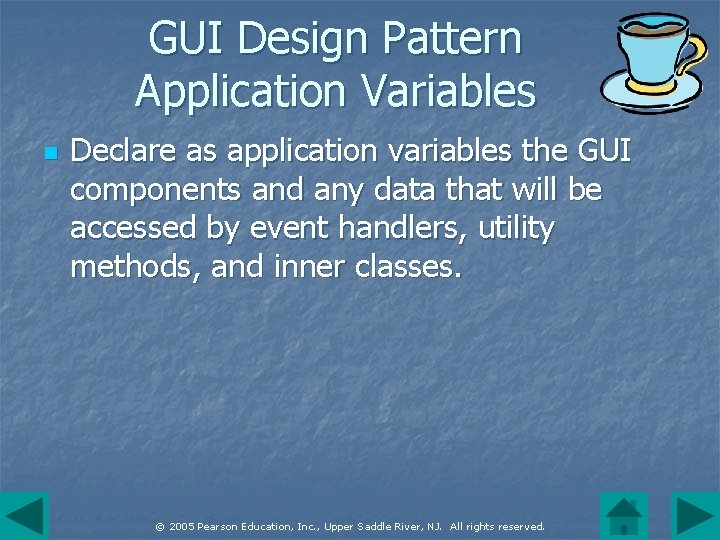
GUI Design Pattern Application Variables n Declare as application variables the GUI components and any data that will be accessed by event handlers, utility methods, and inner classes. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
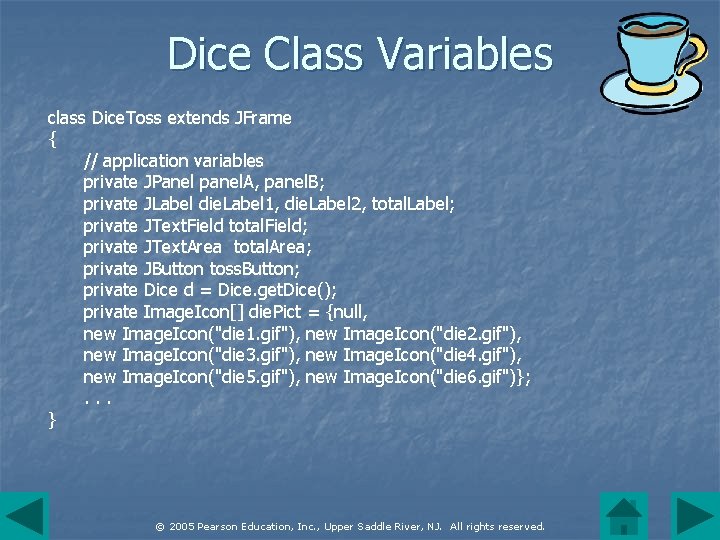
Dice Class Variables class Dice. Toss extends JFrame { // application variables private JPanel panel. A, panel. B; private JLabel die. Label 1, die. Label 2, total. Label; private JText. Field total. Field; private JText. Area total. Area; private JButton toss. Button; private Dice d = Dice. get. Dice(); private Image. Icon[] die. Pict = {null, new Image. Icon("die 1. gif"), new Image. Icon("die 2. gif"), new Image. Icon("die 3. gif"), new Image. Icon("die 4. gif"), new Image. Icon("die 5. gif"), new Image. Icon("die 6. gif")}; . . . } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
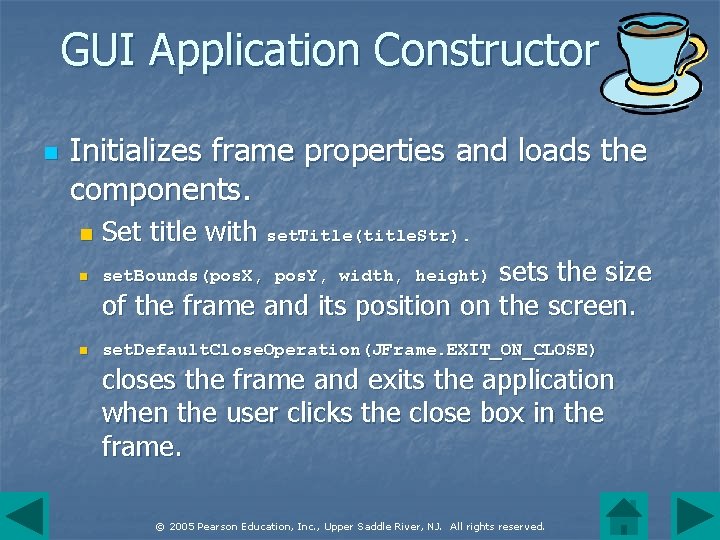
GUI Application Constructor n Initializes frame properties and loads the components. n Set title with set. Title(title. Str). n set. Bounds(pos. X, pos. Y, width, height) n set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE) sets the size of the frame and its position on the screen. closes the frame and exits the application when the user clicks the close box in the frame. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
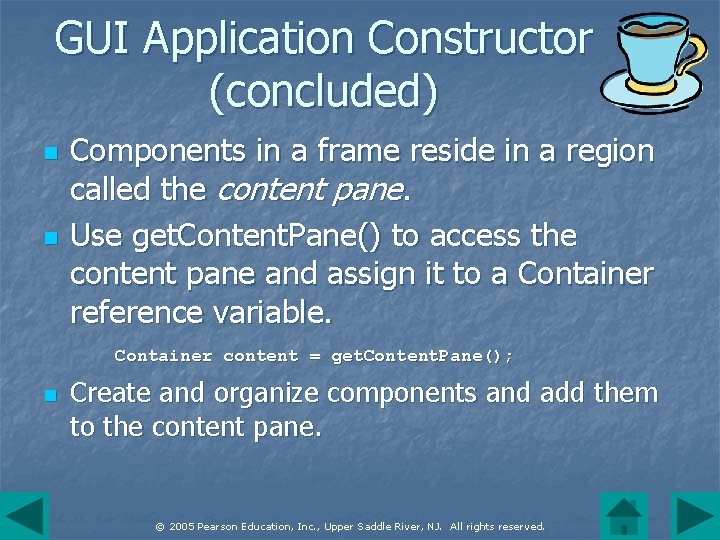
GUI Application Constructor (concluded) n n Components in a frame reside in a region called the content pane. Use get. Content. Pane() to access the content pane and assign it to a Container reference variable. Container content = get. Content. Pane(); n Create and organize components and add them to the content pane. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
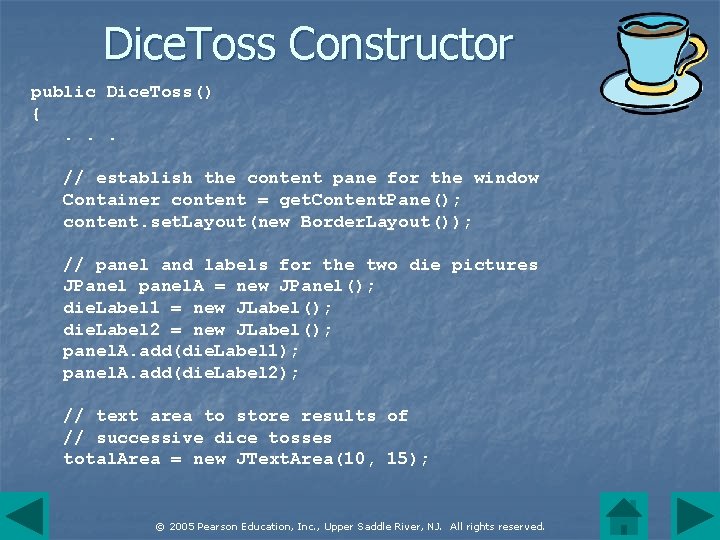
Dice. Toss Constructor public Dice. Toss() {. . . // establish the content pane for the window Container content = get. Content. Pane(); content. set. Layout(new Border. Layout()); // panel and labels for the two die pictures JPanel panel. A = new JPanel(); die. Label 1 = new JLabel(); die. Label 2 = new JLabel(); panel. A. add(die. Label 1); panel. A. add(die. Label 2); // text area to store results of // successive dice tosses total. Area = new JText. Area(10, 15); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
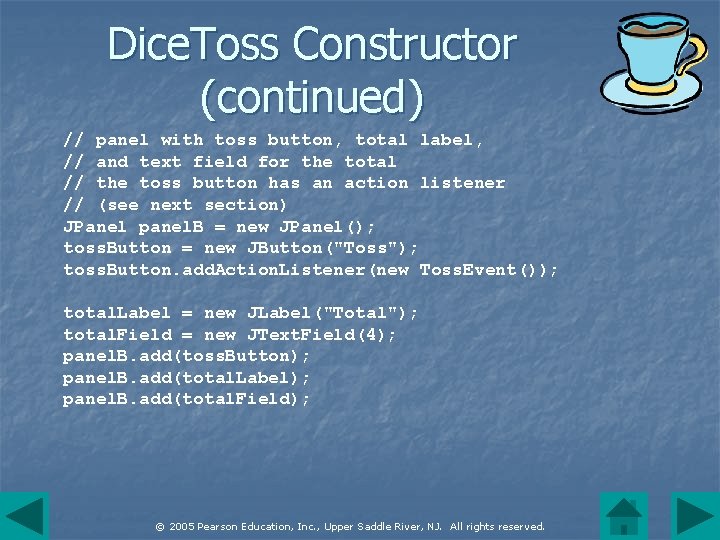
Dice. Toss Constructor (continued) // panel with toss button, total label, // and text field for the total // the toss button has an action listener // (see next section) JPanel panel. B = new JPanel(); toss. Button = new JButton("Toss"); toss. Button. add. Action. Listener(new Toss. Event()); total. Label = new JLabel("Total"); total. Field = new JText. Field(4); panel. B. add(toss. Button); panel. B. add(total. Label); panel. B. add(total. Field); © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
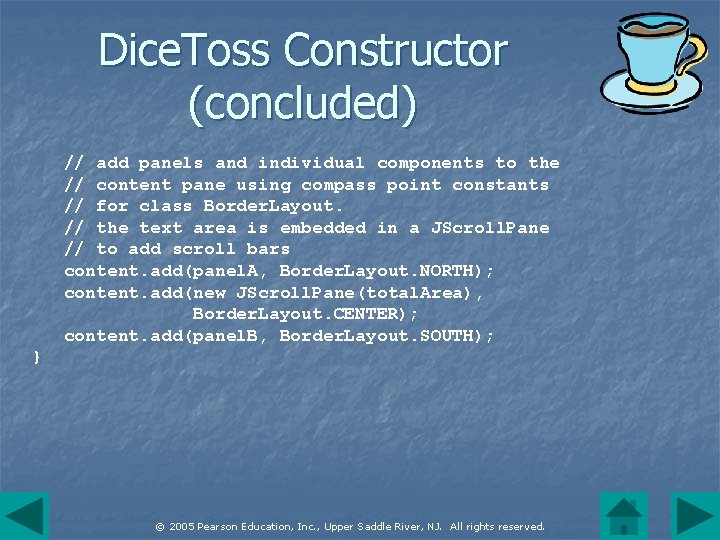
Dice. Toss Constructor (concluded) // add panels and individual components to the // content pane using compass point constants // for class Border. Layout. // the text area is embedded in a JScroll. Pane // to add scroll bars content. add(panel. A, Border. Layout. NORTH); content. add(new JScroll. Pane(total. Area), Border. Layout. CENTER); content. add(panel. B, Border. Layout. SOUTH); } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
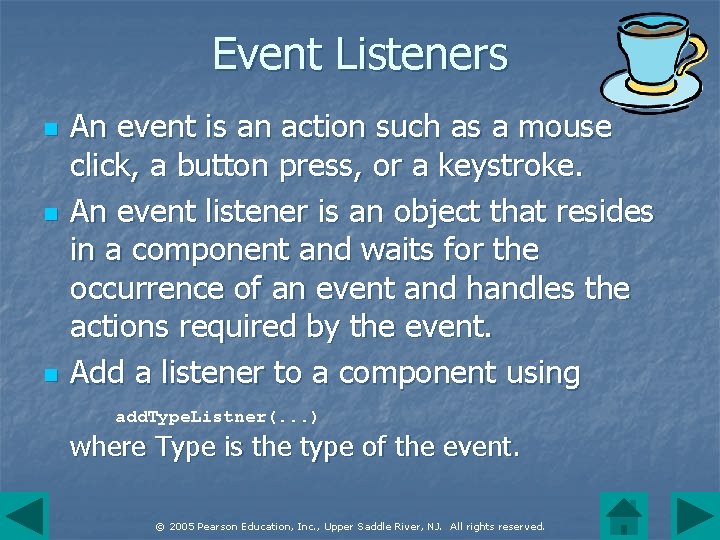
Event Listeners n n n An event is an action such as a mouse click, a button press, or a keystroke. An event listener is an object that resides in a component and waits for the occurrence of an event and handles the actions required by the event. Add a listener to a component using add. Type. Listner(. . . ) where Type is the type of the event. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
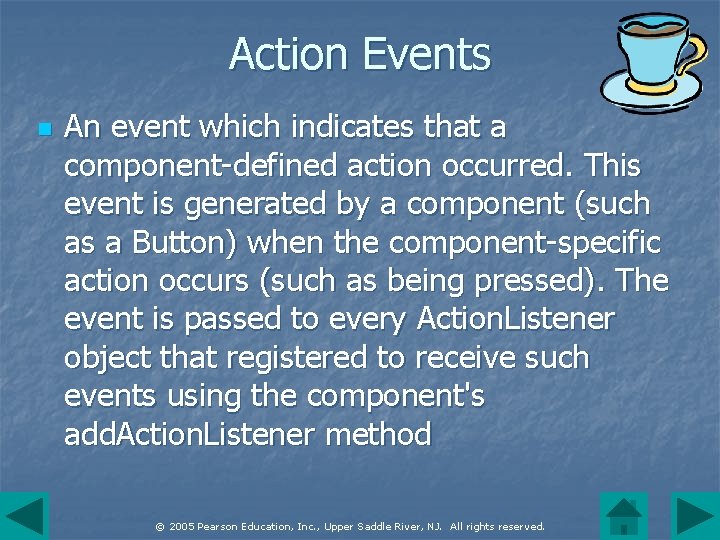
Action Events n An event which indicates that a component-defined action occurred. This event is generated by a component (such as a Button) when the component-specific action occurs (such as being pressed). The event is passed to every Action. Listener object that registered to receive such events using the component's add. Action. Listener method © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
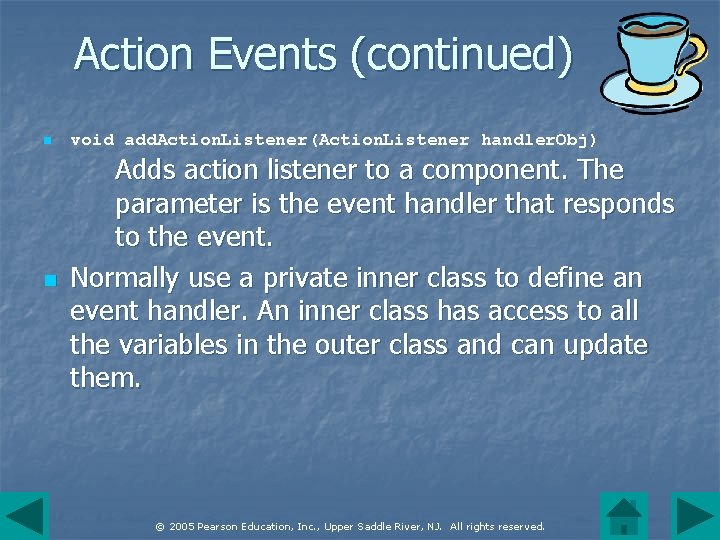
Action Events (continued) n n void add. Action. Listener(Action. Listener handler. Obj) Adds action listener to a component. The parameter is the event handler that responds to the event. Normally use a private inner class to define an event handler. An inner class has access to all the variables in the outer class and can update them. © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
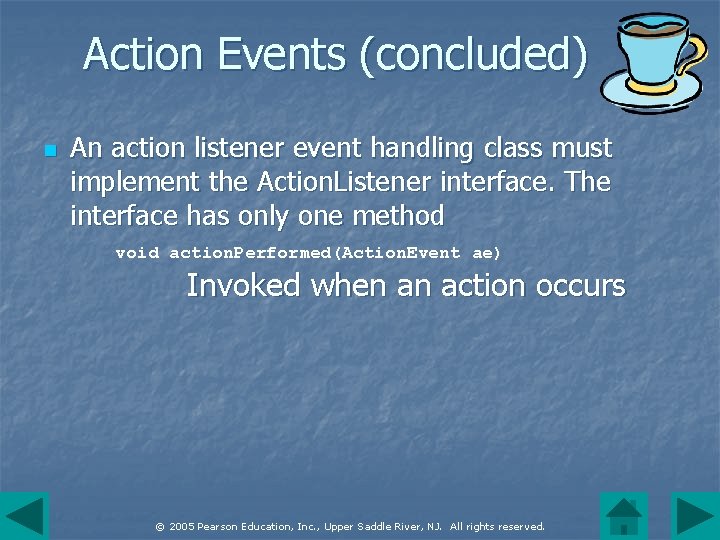
Action Events (concluded) n An action listener event handling class must implement the Action. Listener interface. The interface has only one method void action. Performed(Action. Event ae) Invoked when an action occurs © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
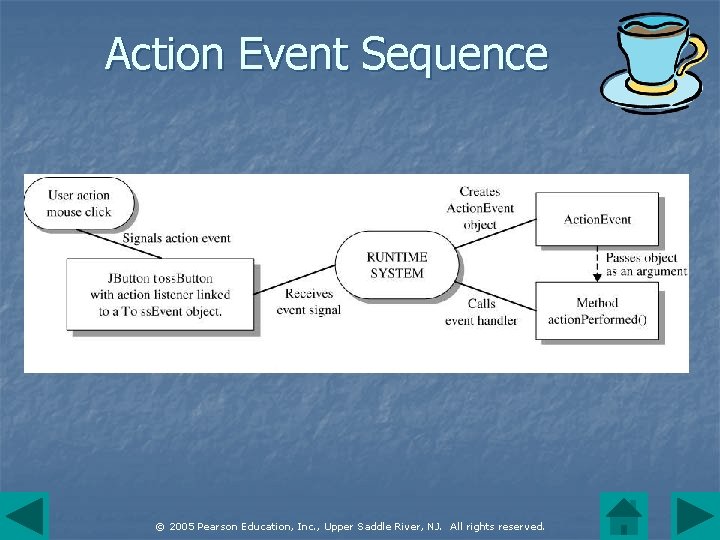
Action Event Sequence © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
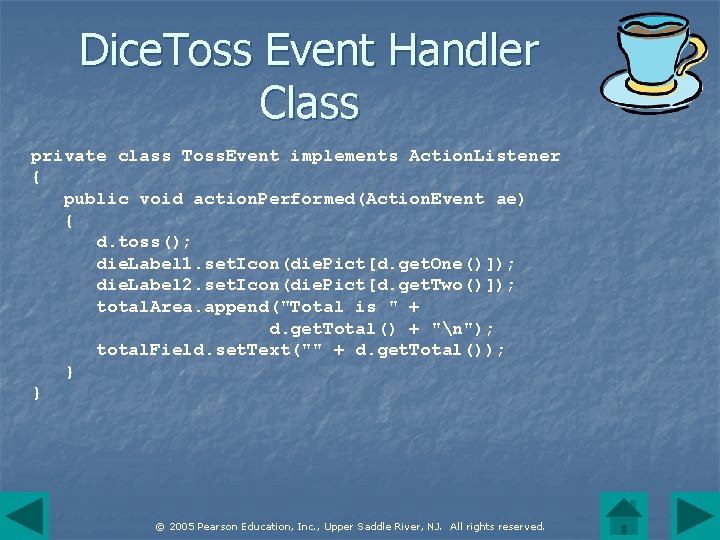
Dice. Toss Event Handler Class private class Toss. Event implements Action. Listener { public void action. Performed(Action. Event ae) { d. toss(); die. Label 1. set. Icon(die. Pict[d. get. One()]); die. Label 2. set. Icon(die. Pict[d. get. Two()]); total. Area. append("Total is " + d. get. Total() + "n"); total. Field. set. Text("" + d. get. Total()); } } © 2005 Pearson Education, Inc. , Upper Saddle River, NJ. All rights reserved.
Advanced data structures in java
Java dynamic data structures
Data structures and abstractions with java
Java data structures
Data structures in java
Data structures in java
Stacks in data structures
Binary search tree java
Data structures using java
Analogous structure
Java software structures 4th edition
Iso 22301 utbildning
Typiska drag för en novell
Nationell inriktning för artificiell intelligens
Ekologiskt fotavtryck
Shingelfrisyren
En lathund för arbete med kontinuitetshantering
Särskild löneskatt för pensionskostnader
Personlig tidbok för yrkesförare
A gastrica
Vad är densitet
Datorkunskap för nybörjare
Tack för att ni lyssnade bild
Tes debattartikel
Delegerande ledarskap
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Tryck formel
Offentlig förvaltning
Jag har nigit för nymånens skära
Presentera för publik crossboss
Jiddisch
Kanaans land
Klassificeringsstruktur för kommunala verksamheter
Mjälthilus
Claes martinsson
Centrum för kunskap och säkerhet
Verifikationsplan
Bra mat för unga idrottare
Verktyg för automatisering av utbetalningar
Rutin för avvikelsehantering
Smärtskolan kunskap för livet
Ministerstyre för och nackdelar
Tack för att ni har lyssnat
Vad är referatmarkeringar
Redogör för vad psykologi är
Stål för stötfångarsystem
Atmosfr