COSC 1306 COMPUTER SCIENCE AND PROGRAMMING JehanFranois Pris
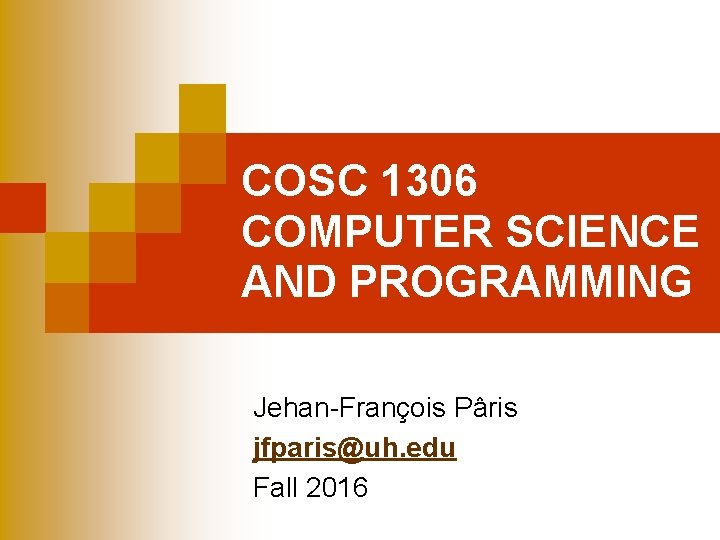
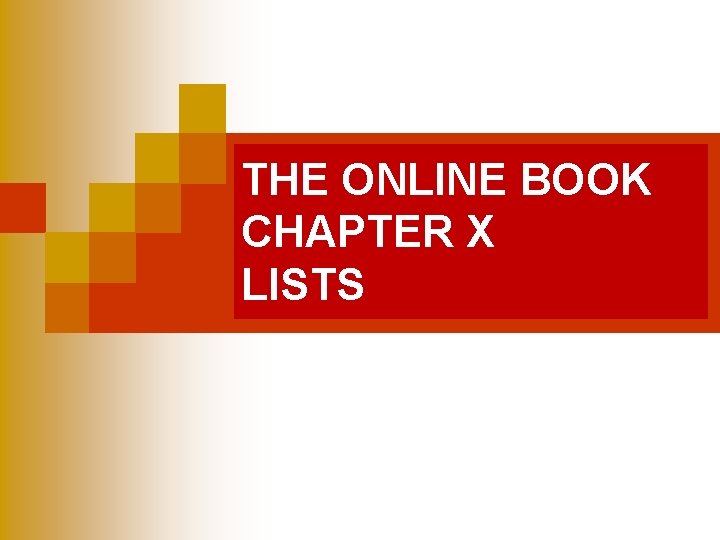
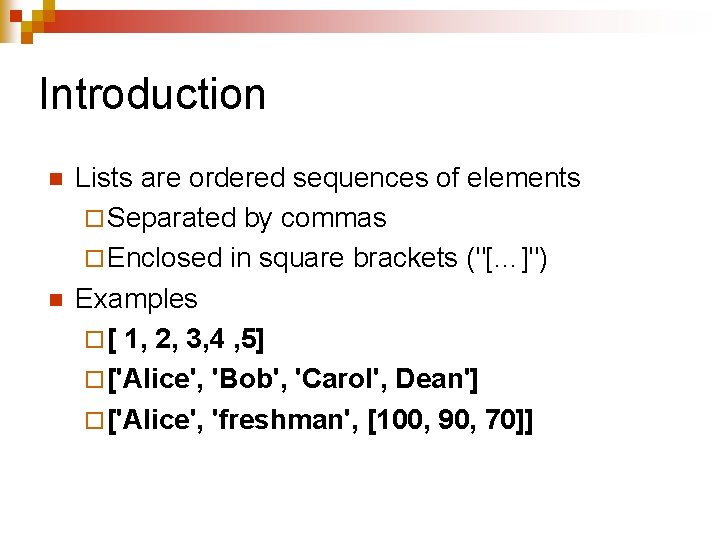
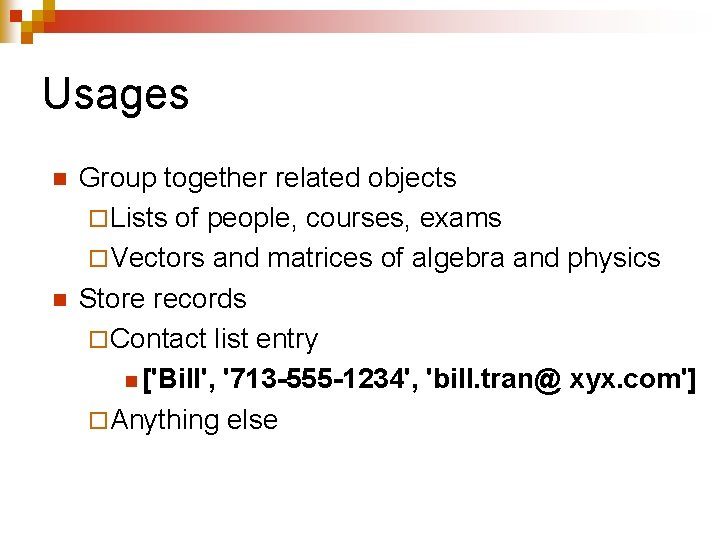
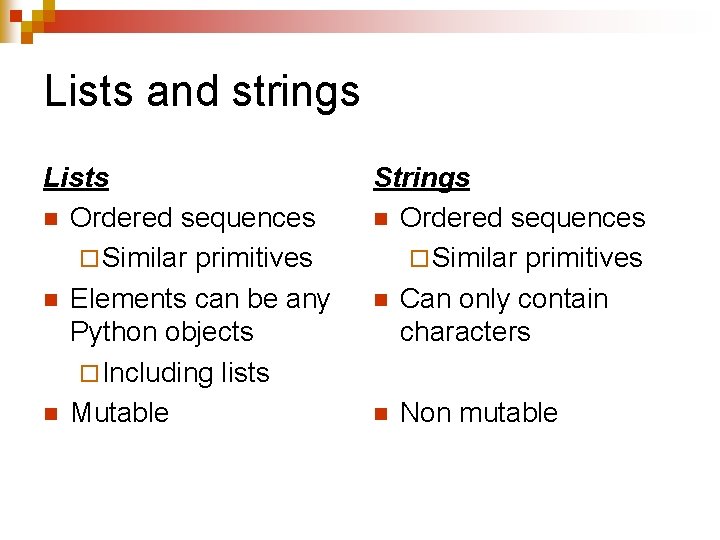
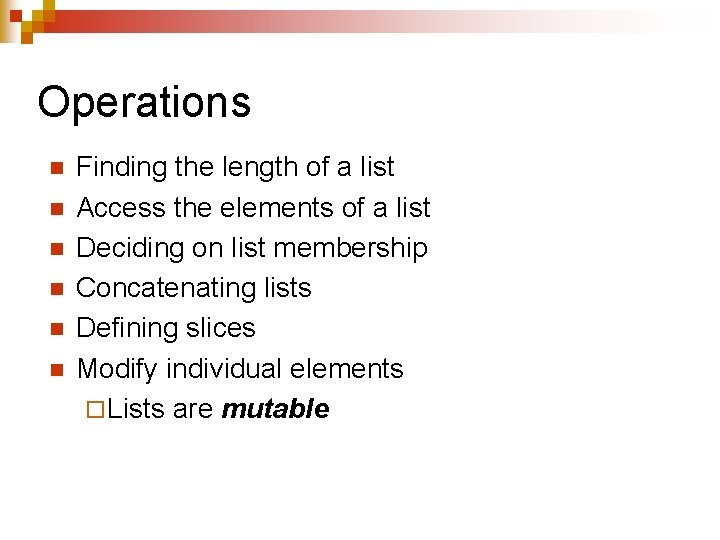
![Accessing Elements (I) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names ['Ann', 'Bob', Accessing Elements (I) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names ['Ann', 'Bob',](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-7.jpg)
![Accessing Elements (II) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[1] 'Bob' >>> Accessing Elements (II) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[1] 'Bob' >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-8.jpg)
![Finding the length of a list names = ['Ann', 'Bob', 'Carol', 'end'] >>> len(names) Finding the length of a list names = ['Ann', 'Bob', 'Carol', 'end'] >>> len(names)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-9.jpg)
![List membership n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> 'Ann' in names List membership n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> 'Ann' in names](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-10.jpg)
![An example n a = [10, 20, 30, 40, 50] a designates the whole An example n a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-11.jpg)
![List concatenation (I) >>> names = ['Alice'] + ['Bob'] >>> names ['Alice', 'Bob'] >>> List concatenation (I) >>> names = ['Alice'] + ['Bob'] >>> names ['Alice', 'Bob'] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-12.jpg)
![List concatenation (II) >>> mylist = ['Ann'] >>> mylist*3 ['Ann', 'Ann'] >>> newlist = List concatenation (II) >>> mylist = ['Ann'] >>> mylist*3 ['Ann', 'Ann'] >>> newlist =](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-13.jpg)
![List slices >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[0: 1] ['Ann'] ¨ List slices >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[0: 1] ['Ann'] ¨](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-14.jpg)
![More list slices >>> names[0: 2] ['Ann', 'Bob'] ¨ Includes names[0] and names[1] >>> More list slices >>> names[0: 2] ['Ann', 'Bob'] ¨ Includes names[0] and names[1] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-15.jpg)
![More list slices >>> names[-1: ] ['end'] n A list slice is a list More list slices >>> names[-1: ] ['end'] n A list slice is a list](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-16.jpg)
![Let us check n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[-1] 'done' Let us check n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[-1] 'done'](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-17.jpg)
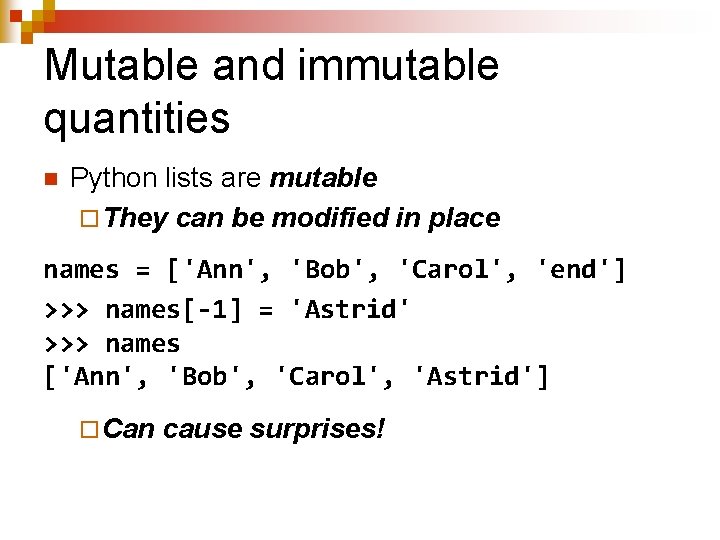
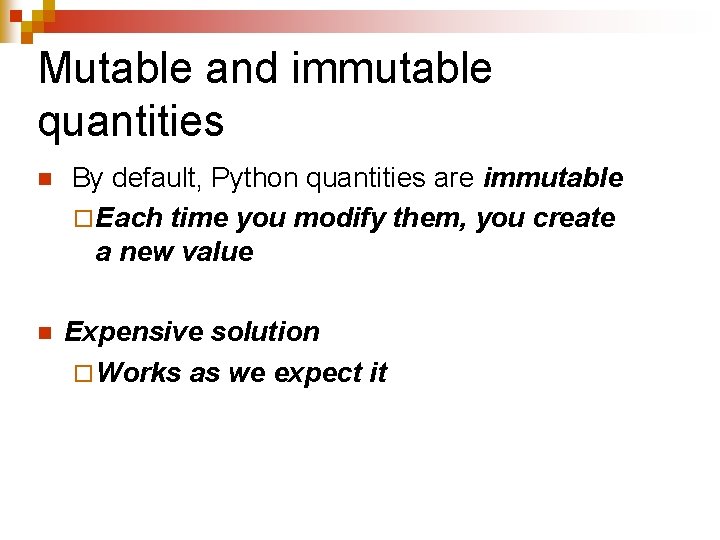
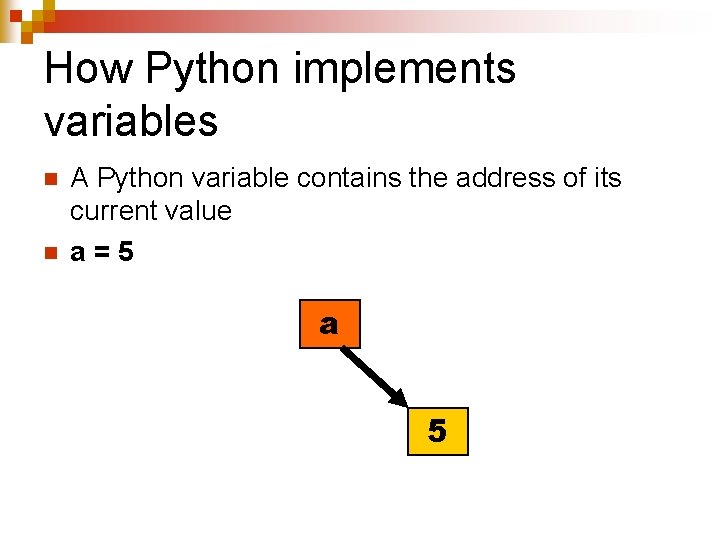
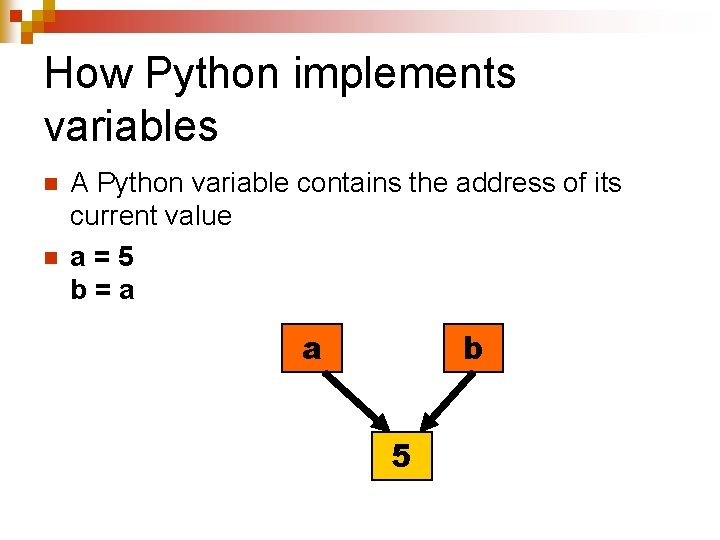
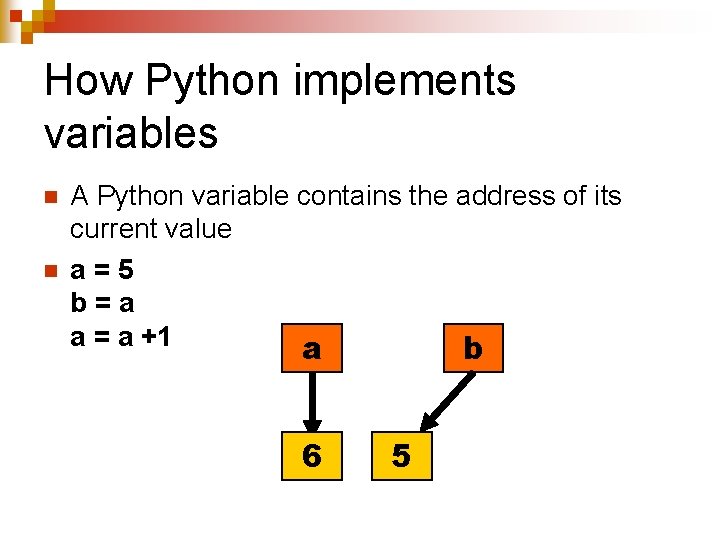
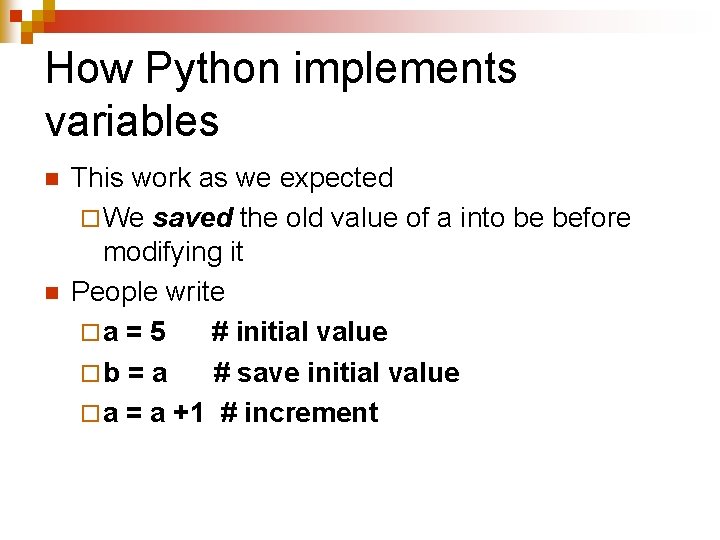
![A big surprise n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b A big surprise n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-24.jpg)
![What happened (I) n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b What happened (I) n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-25.jpg)
![What happened (II) n n n >>> a[0] = 'Lucy' >>> a ['Lucy', 'Bob', What happened (II) n n n >>> a[0] = 'Lucy' >>> a ['Lucy', 'Bob',](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-26.jpg)
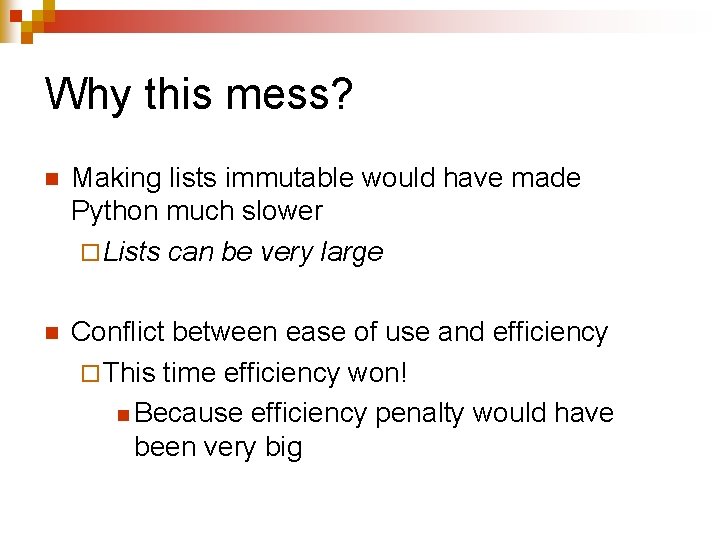
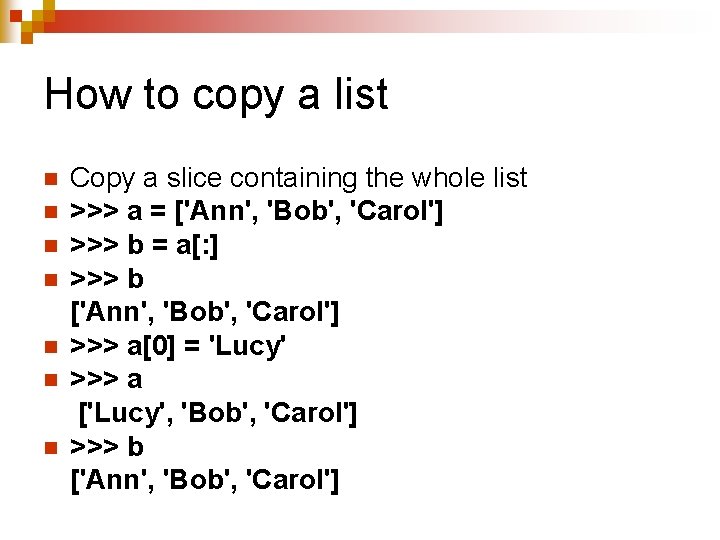
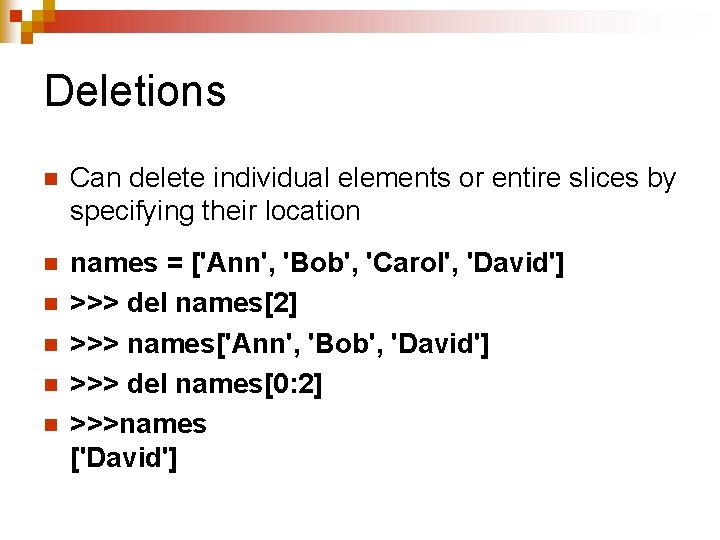
![Object and references (I) n n >>> a = ['Ann'] >>> b = ['Ann'] Object and references (I) n n >>> a = ['Ann'] >>> b = ['Ann']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-30.jpg)
![No sharing a b ['Ann'] No sharing a b ['Ann']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-31.jpg)
![Object and references (II) n n >>> a = ['Ann'] >>> b = a Object and references (II) n n >>> a = ['Ann'] >>> b = a](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-32.jpg)
![Same object is shared a b ['Ann'] Same object is shared a b ['Ann']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-33.jpg)
![Aliasing and cloning (I) n When we do ¨ >>> a = ['Ann'] ¨ Aliasing and cloning (I) n When we do ¨ >>> a = ['Ann'] ¨](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-34.jpg)
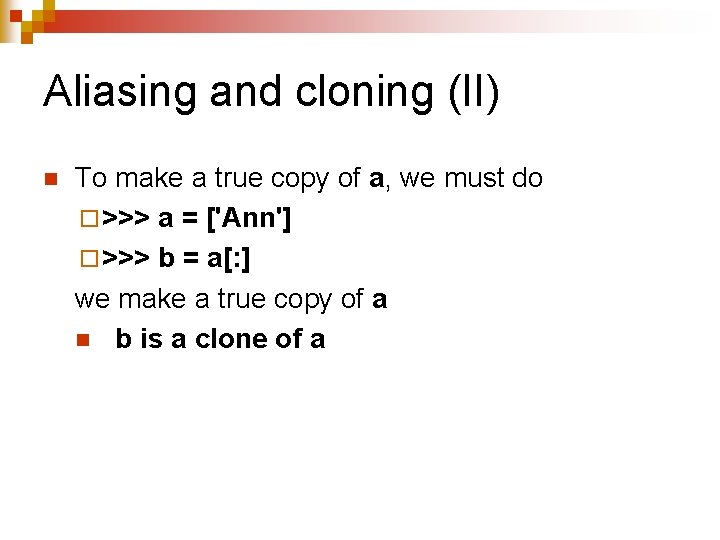
![A weird behavior n n n >>> pets = ['cats', 'dogs'] >>> oddlist =[pets]*2 A weird behavior n n n >>> pets = ['cats', 'dogs'] >>> oddlist =[pets]*2](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-36.jpg)
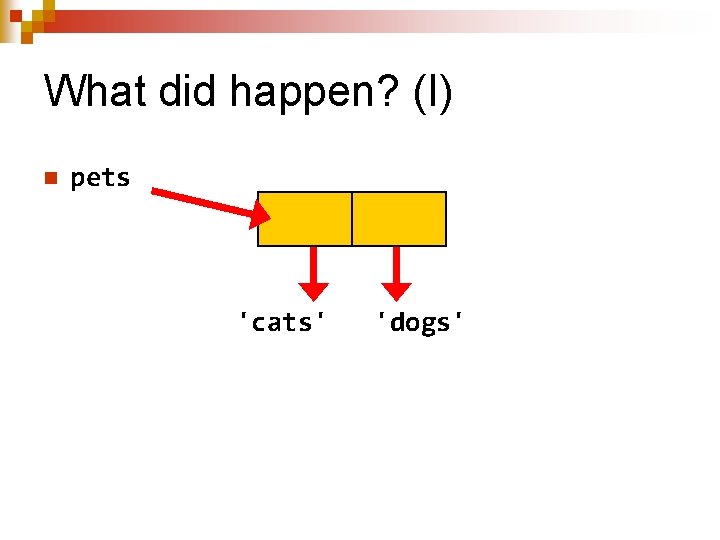
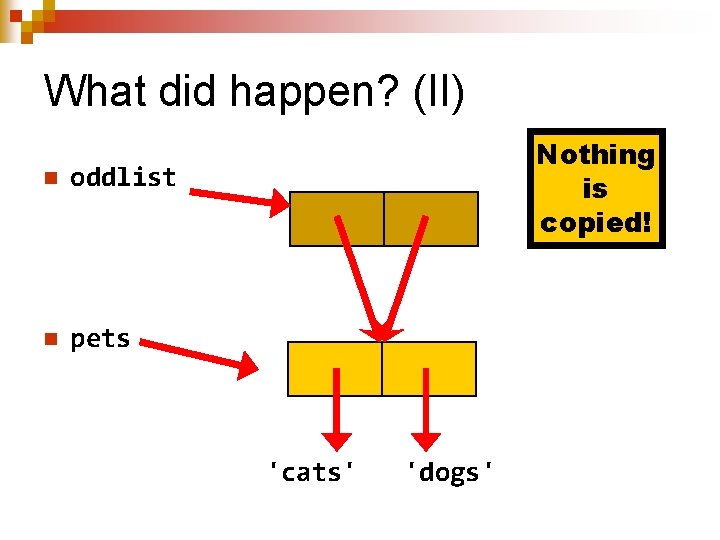
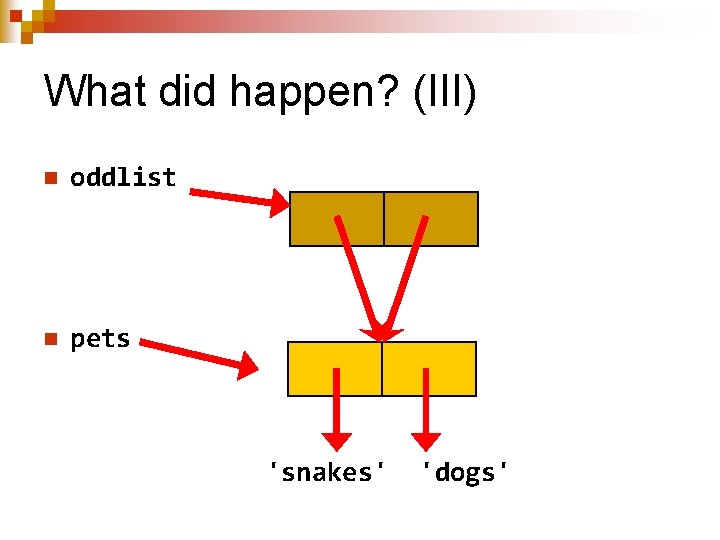
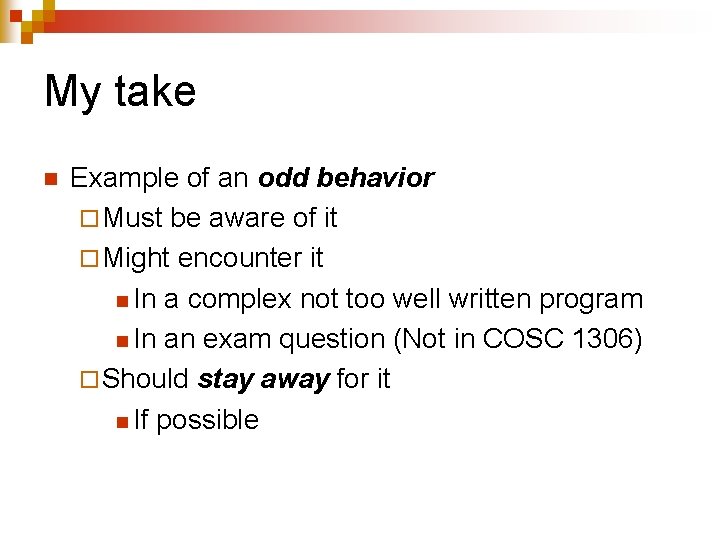
![Editing list >>> mylist = [11, 12, 13, 'done'] >>> mylist[-1] = 'finished' >>> Editing list >>> mylist = [11, 12, 13, 'done'] >>> mylist[-1] = 'finished' >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-41.jpg)
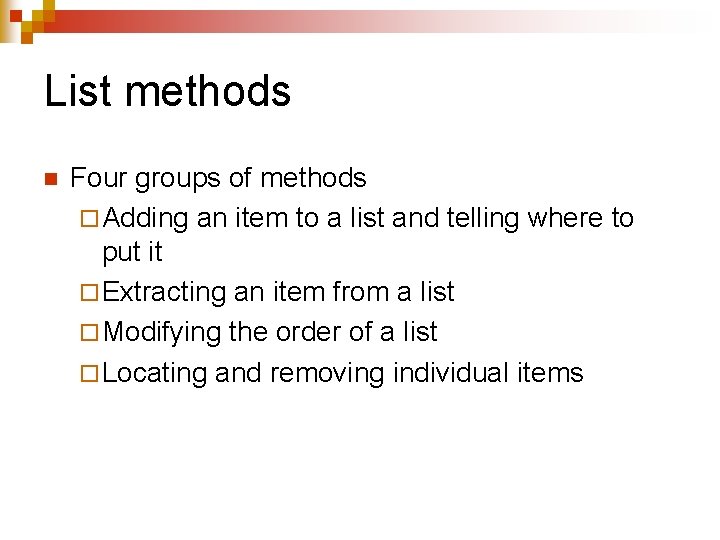
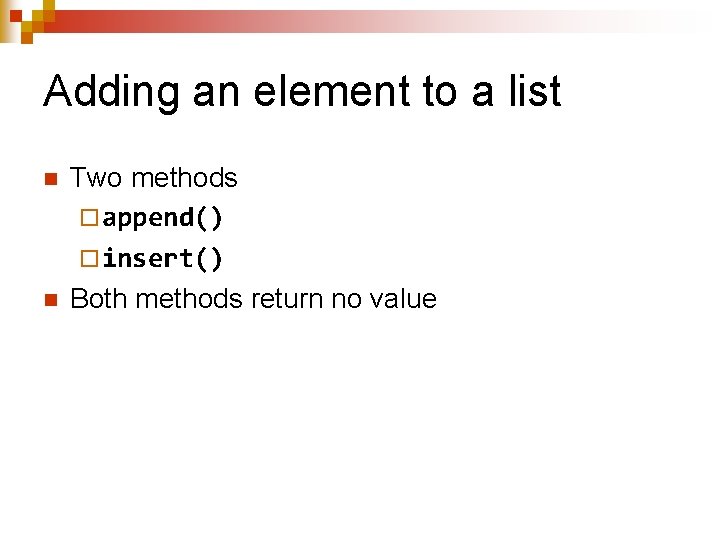
![Append(I) n n n >>> mylist = [11, 12, 13, 'finished'] >>> mylist. append('Not Append(I) n n n >>> mylist = [11, 12, 13, 'finished'] >>> mylist. append('Not](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-44.jpg)
![Append (II) >>> listoflists = [[14. 5, '1306'], [17. 5, '6360']] >>> listoflists. append([16. Append (II) >>> listoflists = [[14. 5, '1306'], [17. 5, '6360']] >>> listoflists. append([16.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-45.jpg)
![Insert (I) >>> mylist = [11, 12, 13, 'finished'] >>> mylist. insert(0, 10) #BEFORE Insert (I) >>> mylist = [11, 12, 13, 'finished'] >>> mylist. insert(0, 10) #BEFORE](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-46.jpg)
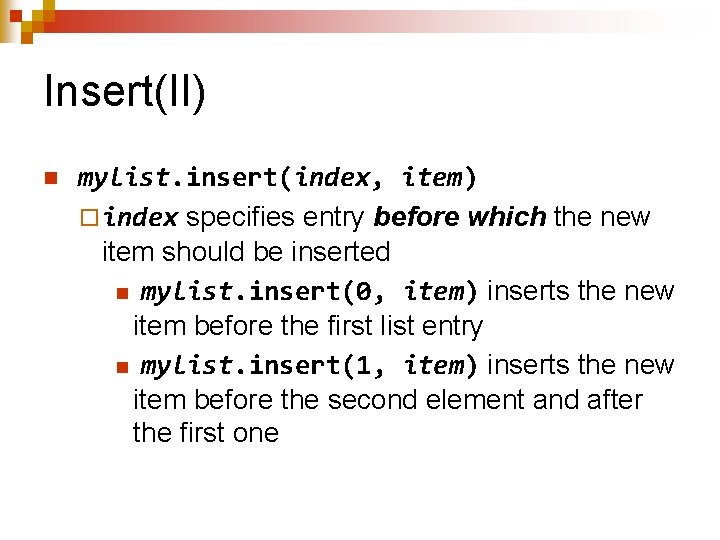
![Example (I) n a = [10, 20, 30, 40, 50] a designates the whole Example (I) n a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-48.jpg)
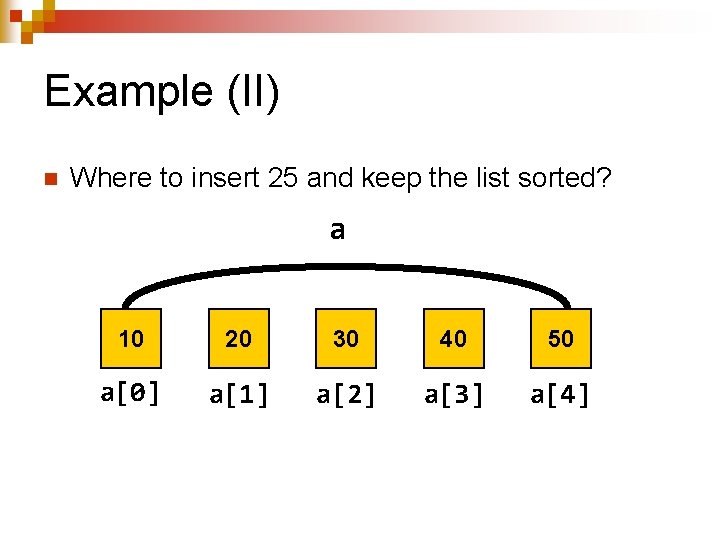
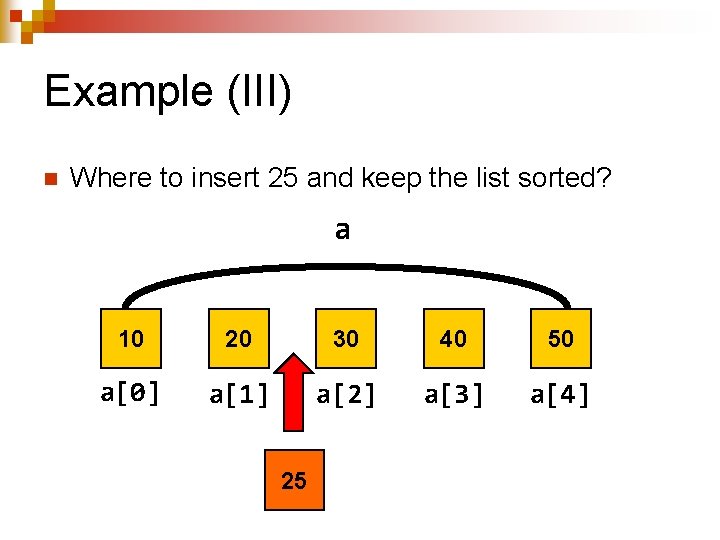
![Example (IV) n We do ¨ a. insert(2, 25) n after a[1] and before Example (IV) n We do ¨ a. insert(2, 25) n after a[1] and before](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-51.jpg)
![Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(2, Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(2,](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-52.jpg)
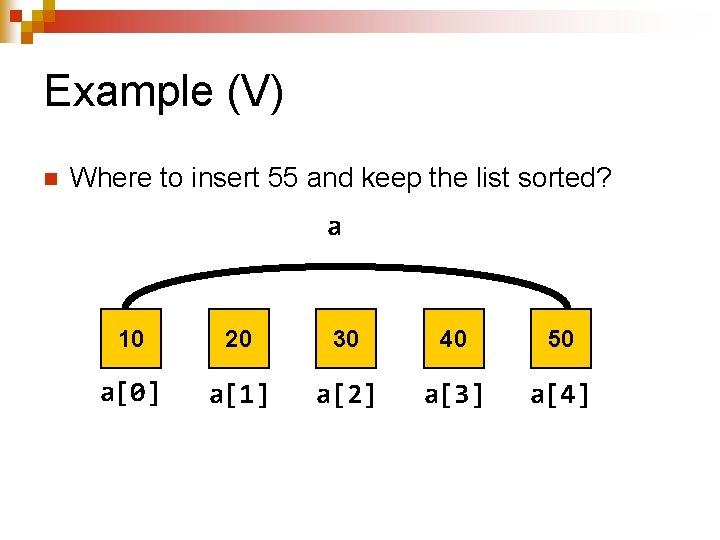
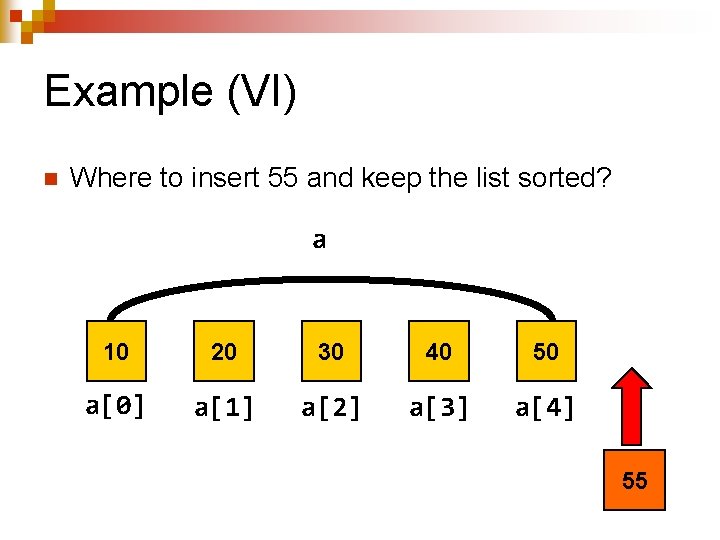
![Example (VII) n n We must insert ¨ After a[4] ¨ Before no other Example (VII) n n We must insert ¨ After a[4] ¨ Before no other](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-55.jpg)
![Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(5, Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(5,](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-56.jpg)
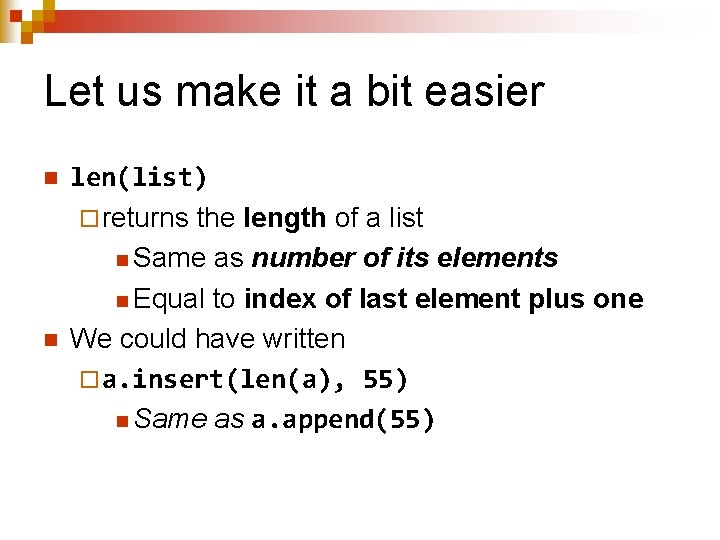
![Extracting items n n n One by one thislist. pop(i) ¨ removes thislist[i] from Extracting items n n n One by one thislist. pop(i) ¨ removes thislist[i] from](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-58.jpg)
![Examples (I) >>> mylist = [11, 22, 33, 44, 55, 66] >>> mylist. pop(0) Examples (I) >>> mylist = [11, 22, 33, 44, 55, 66] >>> mylist. pop(0)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-59.jpg)
![Examples (II) >>> mylist. pop() 66 >>> mylist [22, 33, 44, 55] >>> mylist. Examples (II) >>> mylist. pop() 66 >>> mylist [22, 33, 44, 55] >>> mylist.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-60.jpg)
![Examples (III) >>> waitlist = ['Ann', 'Cristi', 'Dean'] >>> waitlist. pop(0) 'Ann' >>> waitlist Examples (III) >>> waitlist = ['Ann', 'Cristi', 'Dean'] >>> waitlist. pop(0) 'Ann' >>> waitlist](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-61.jpg)
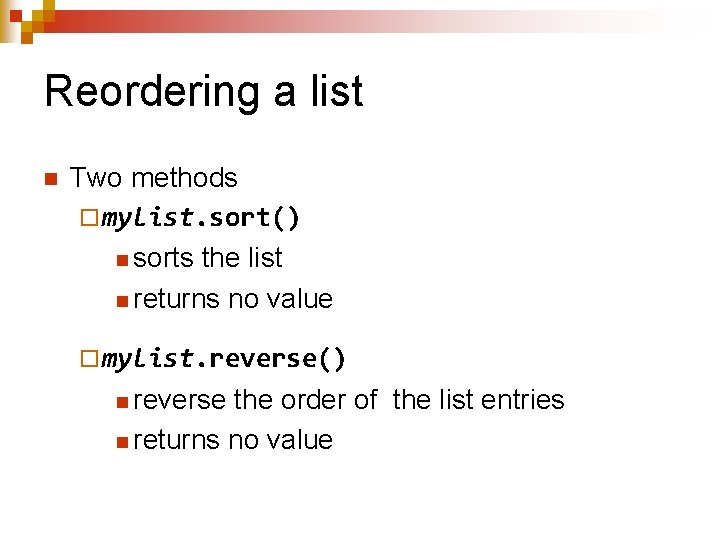
![Sorting lists >>> mylist = [11, 12, 13, 14, 'done'] >>> mylist. sort() Traceback Sorting lists >>> mylist = [11, 12, 13, 14, 'done'] >>> mylist. sort() Traceback](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-63.jpg)
![Sorting lists of strings >>> names = ['Alice', 'Carol', 'Bob'] >>> names. sort() >>> Sorting lists of strings >>> names = ['Alice', 'Carol', 'Bob'] >>> names. sort() >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-64.jpg)
![Sorting lists of numbers >>> newlist = [0, -1, +1, -2, +2] >>> newlist. Sorting lists of numbers >>> newlist = [0, -1, +1, -2, +2] >>> newlist.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-65.jpg)
![Sorting lists with sublists >>> schedule = [[14. 5, '1306'], [17. 5, '6360'], [16. Sorting lists with sublists >>> schedule = [[14. 5, '1306'], [17. 5, '6360'], [16.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-66.jpg)
![Reversing the order of a list >>> names = ['Alice', 'Bob', 'Carol'] >>> names. Reversing the order of a list >>> names = ['Alice', 'Bob', 'Carol'] >>> names.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-67.jpg)
![Sorting into a new list >>> newlist = [0, -1, +1, -2, +2] >>> Sorting into a new list >>> newlist = [0, -1, +1, -2, +2] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-68.jpg)
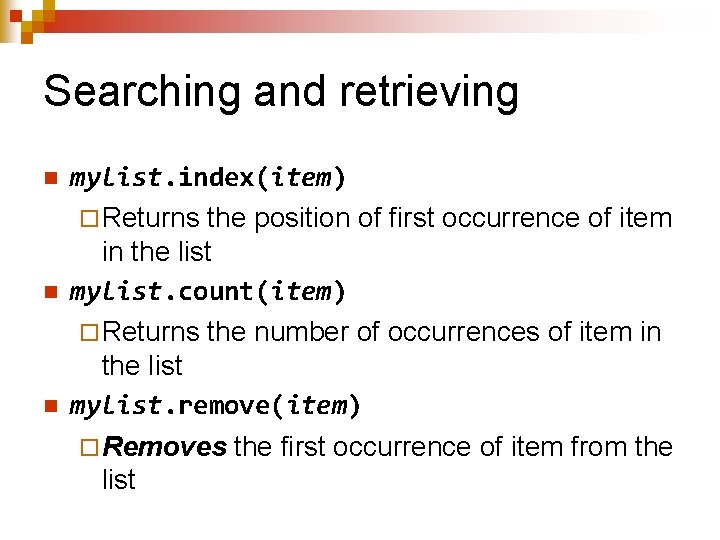
![Examples n n n n n >>> names ['Ann', 'Carol', 'Bob', 'Alice', 'Ann'] >>> Examples n n n n n >>> names ['Ann', 'Carol', 'Bob', 'Alice', 'Ann'] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-70.jpg)
![Sum: a very useful function >>> list = [10, 20] >>> sum(list) 50 >>> Sum: a very useful function >>> list = [10, 20] >>> sum(list) 50 >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-71.jpg)
![Easy averages >>> prices = [1. 899, 1. 959, 2. 029, 2. 079] >>> Easy averages >>> prices = [1. 899, 1. 959, 2. 029, 2. 079] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-72.jpg)
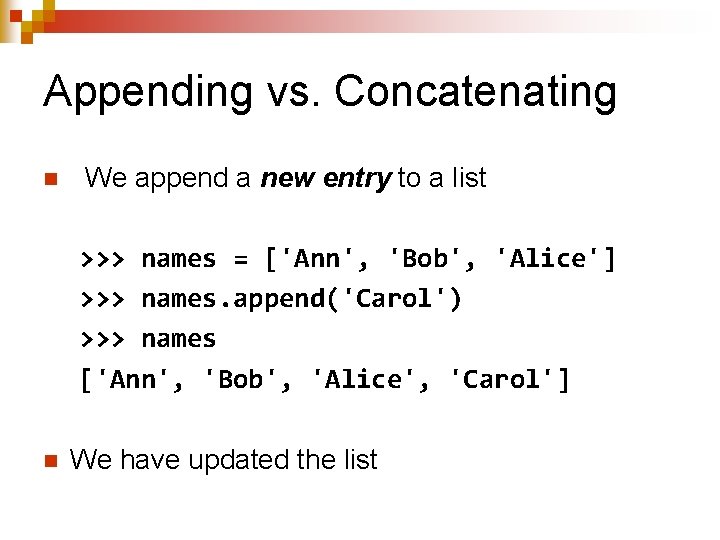
![Appending vs. Concatenating n We concatenate two lists >>> names = ['Ann', 'Bob', 'Alice'] Appending vs. Concatenating n We concatenate two lists >>> names = ['Ann', 'Bob', 'Alice']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-74.jpg)
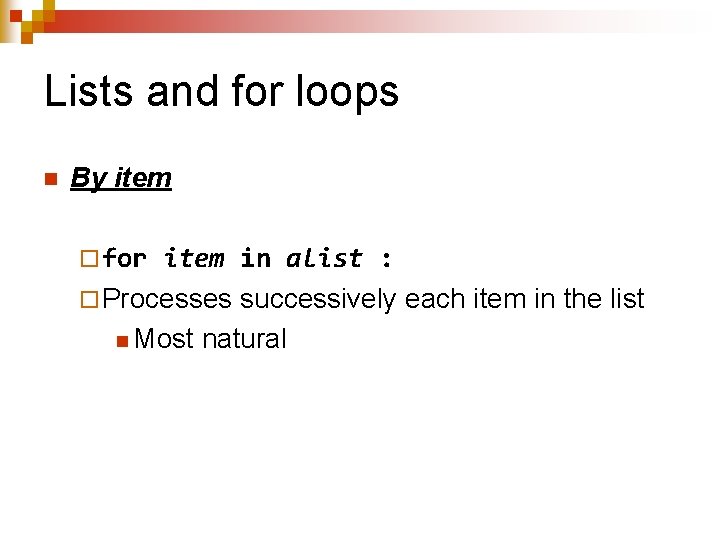
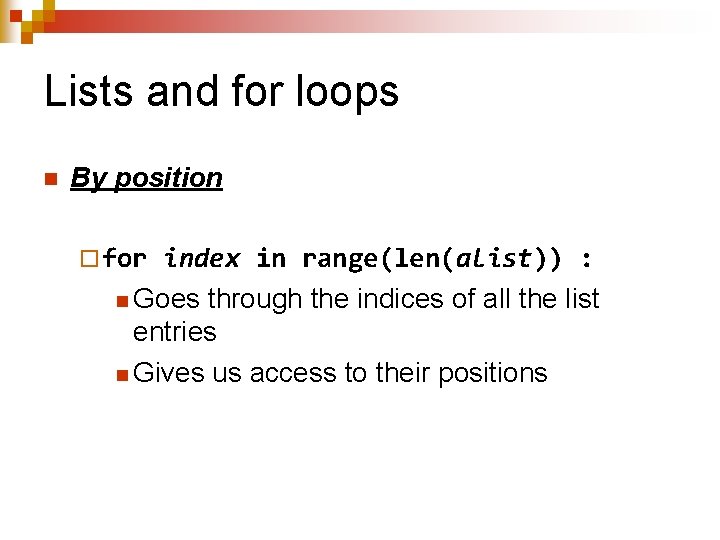
![Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for item in names : print(item) Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for item in names : print(item)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-77.jpg)
![Usage >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) : count = Usage >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) : count =](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-78.jpg)
![Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for i in range(len(names)): print(names[i]) Ann Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for i in range(len(names)): print(names[i]) Ann](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-79.jpg)
![Another countzeroes() function >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) : Another countzeroes() function >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) :](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-80.jpg)
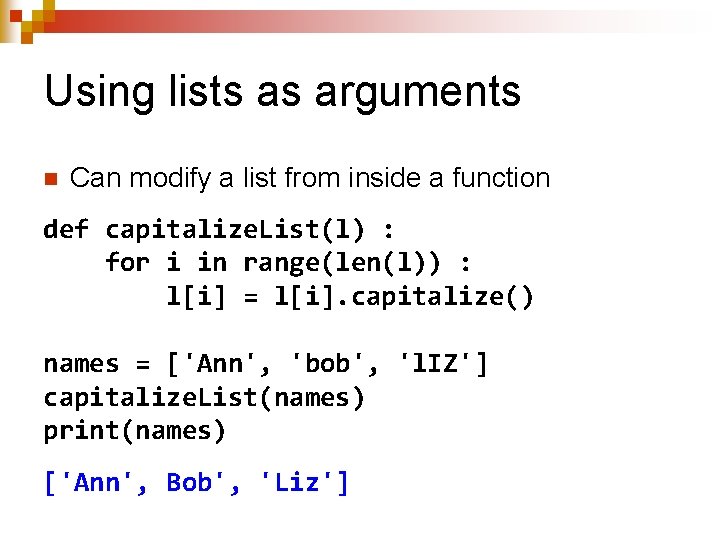
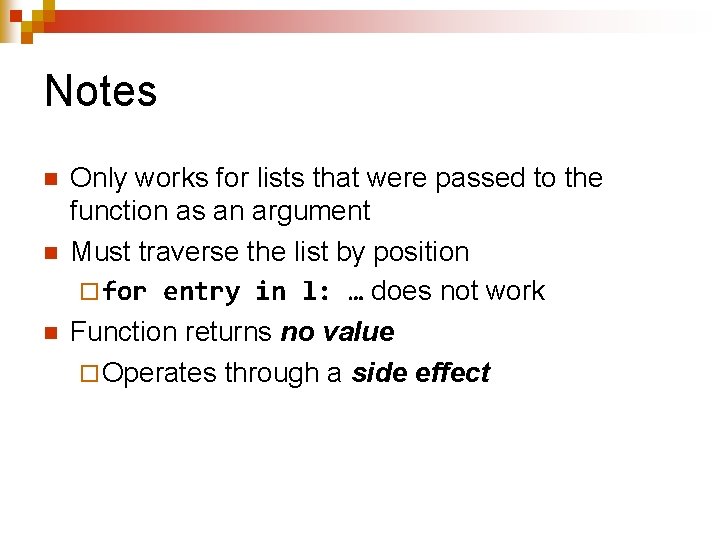
![Functions returning a list def capitalize. List(l) : newlist = [] for item in Functions returning a list def capitalize. List(l) : newlist = [] for item in](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-83.jpg)
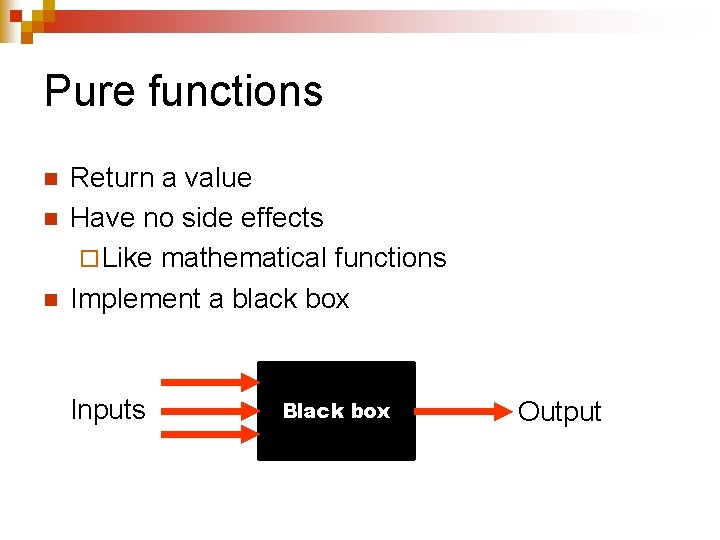
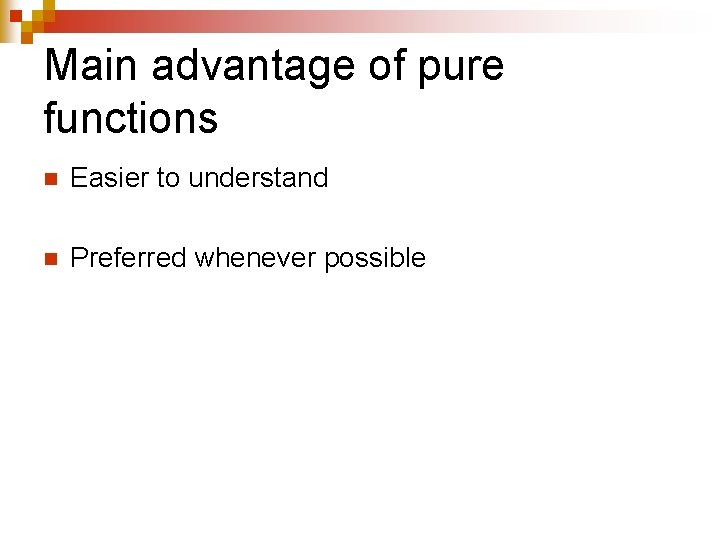
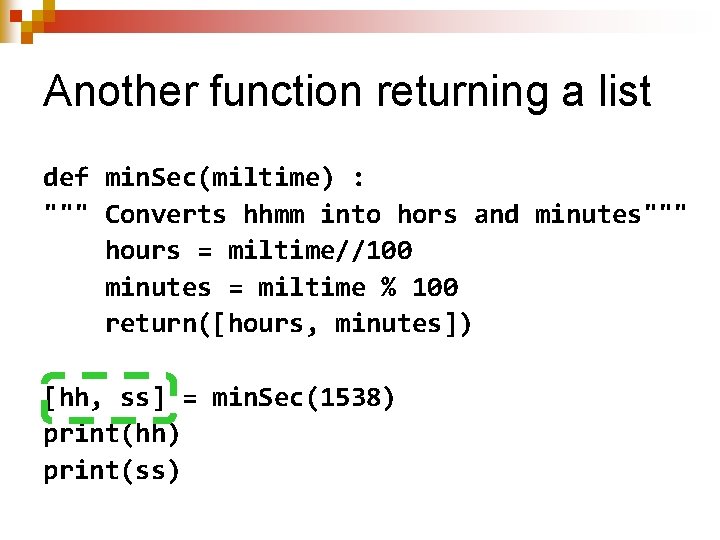
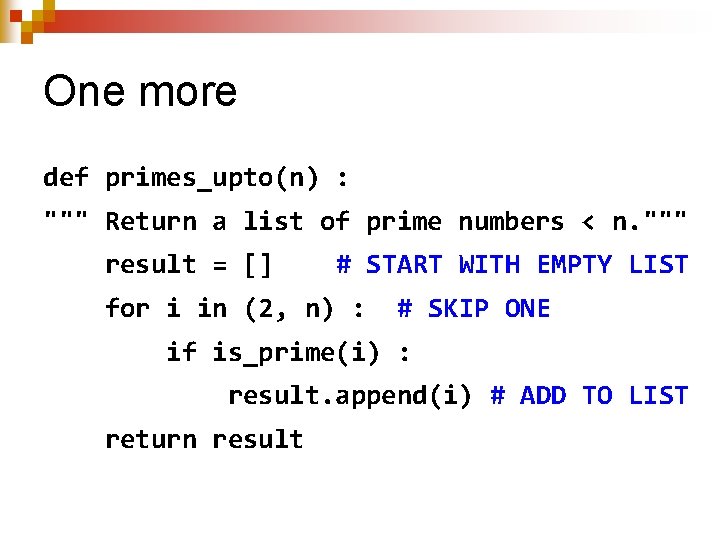
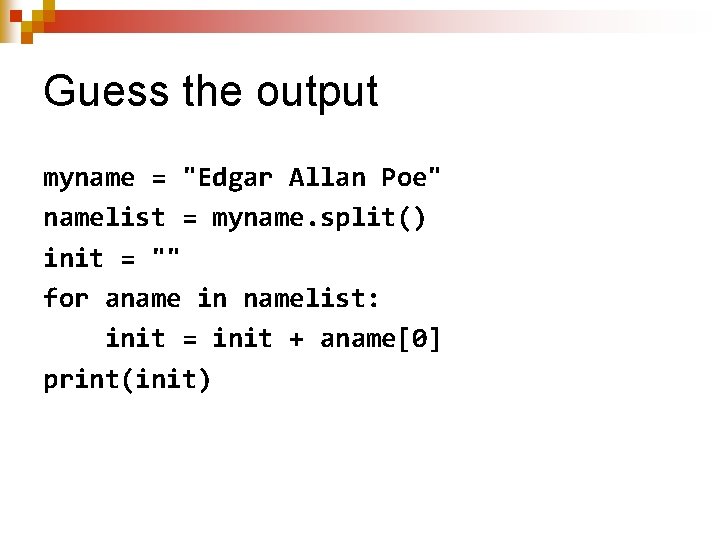
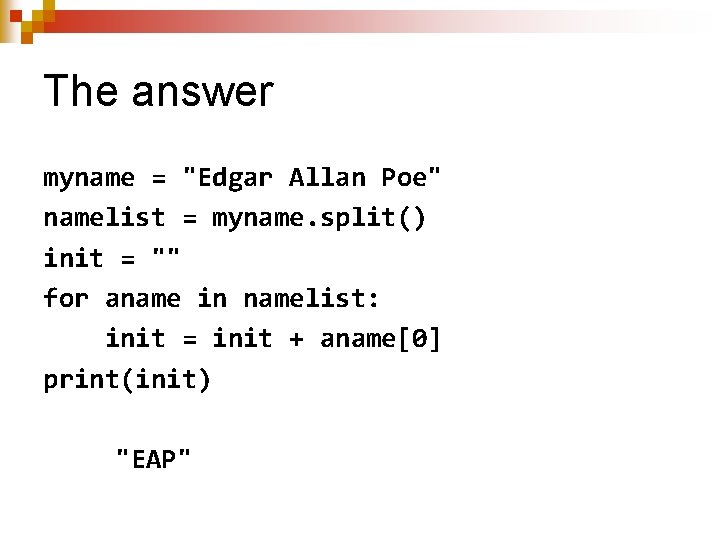
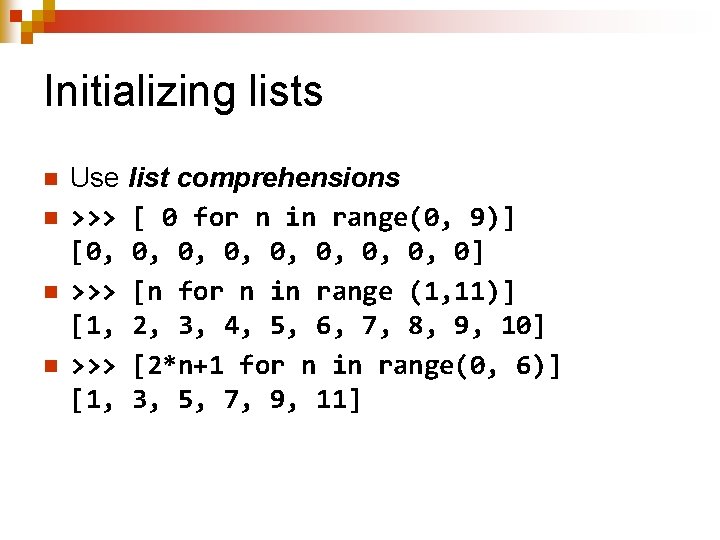
![Warning! n The for n clause is essential n [0 in range(0, 10)] [True] Warning! n The for n clause is essential n [0 in range(0, 10)] [True]](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-91.jpg)
![More comprehensions >>> [ c for c in 'Cougars'] [ 'C', 'o', 'u', 'g', More comprehensions >>> [ c for c in 'Cougars'] [ 'C', 'o', 'u', 'g',](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-92.jpg)
![More comprehensions >>> [1, first 5 = [n for n in range(1, 6)] first More comprehensions >>> [1, first 5 = [n for n in range(1, 6)] first](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-93.jpg)
![An equivalence [n*n for n in range(1, 6)] [1, 4, 9, 16, 25] is An equivalence [n*n for n in range(1, 6)] [1, 4, 9, 16, 25] is](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-94.jpg)
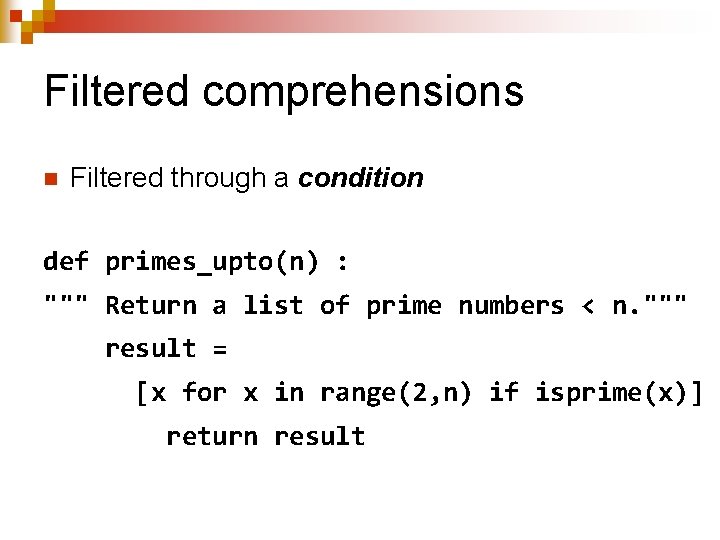
![Filtered comprehensions >>> a = [11, 22, 33, 44, 55] >>> b = [ Filtered comprehensions >>> a = [11, 22, 33, 44, 55] >>> b = [](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-96.jpg)
![More filtered comprehensions >>> s = [['Ann', 'CS'], ['Bob', 'CE'], ['Liz', 'CS']] >>> [x[0] More filtered comprehensions >>> s = [['Ann', 'CS'], ['Bob', 'CE'], ['Liz', 'CS']] >>> [x[0]](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-97.jpg)
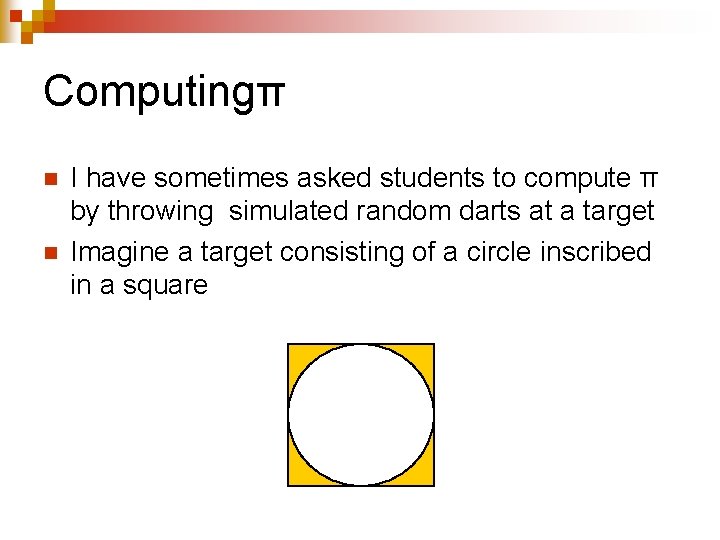
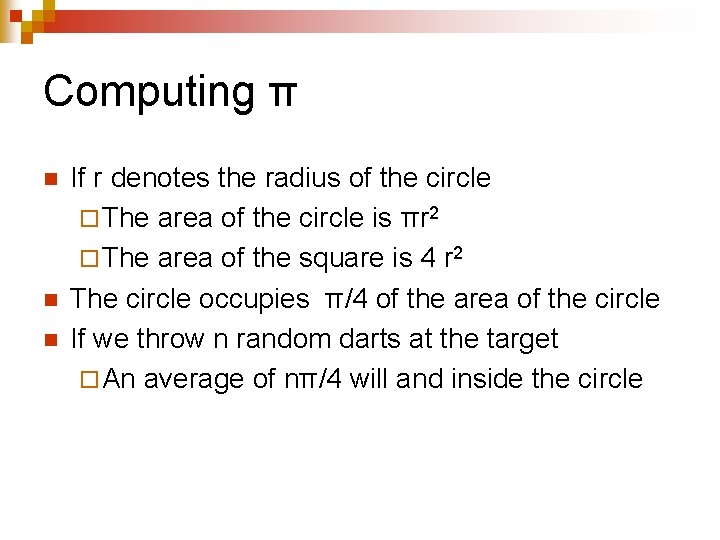
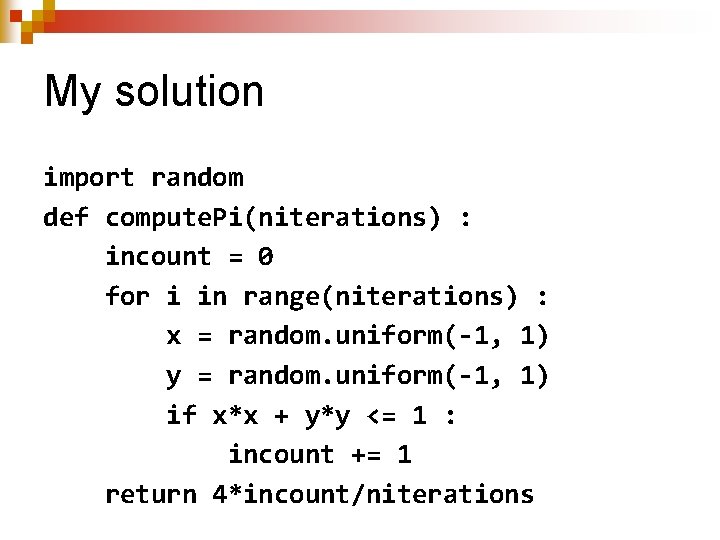
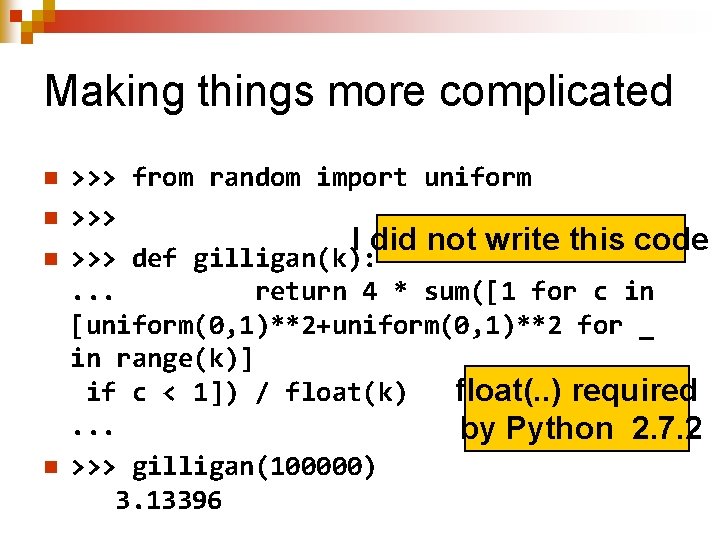
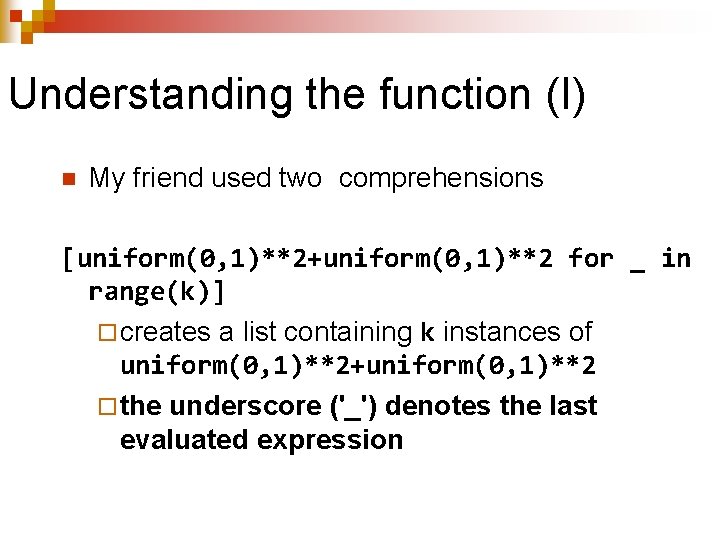
![Understanding the function (II) [1 for c in […] if c < 1] ¨ Understanding the function (II) [1 for c in […] if c < 1] ¨](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-103.jpg)
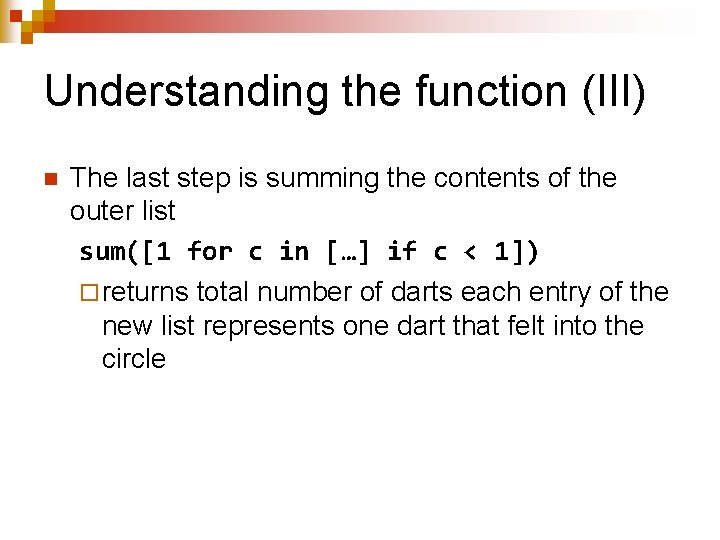
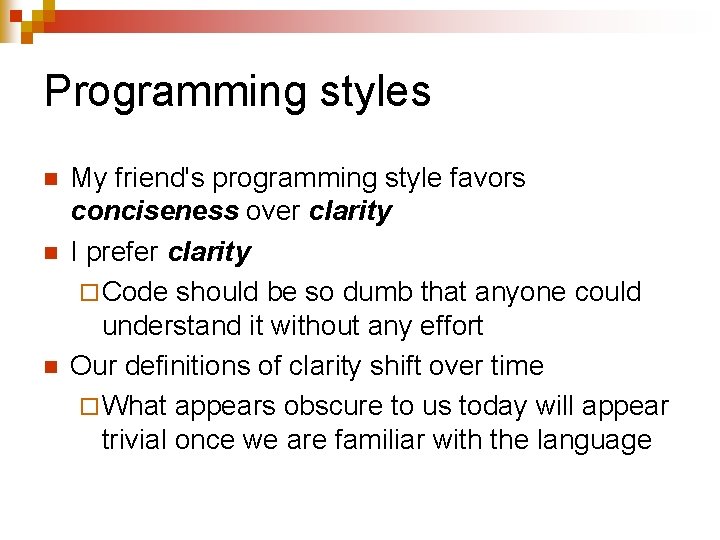
![Nested lists or lists of lists >>> nested = [['Ann', 90], ['Bob', 84]] >>> Nested lists or lists of lists >>> nested = [['Ann', 90], ['Bob', 84]] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-106.jpg)
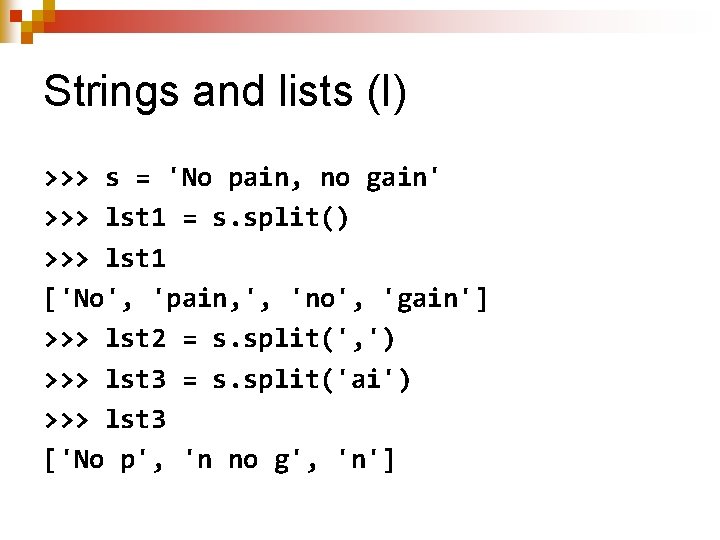
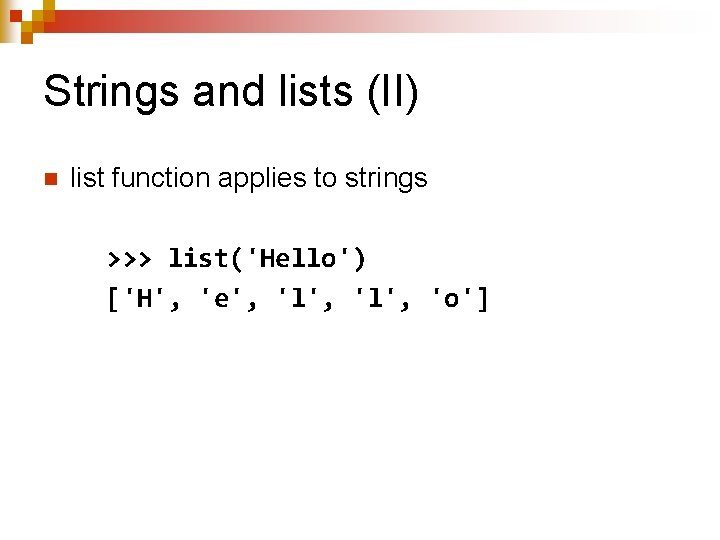
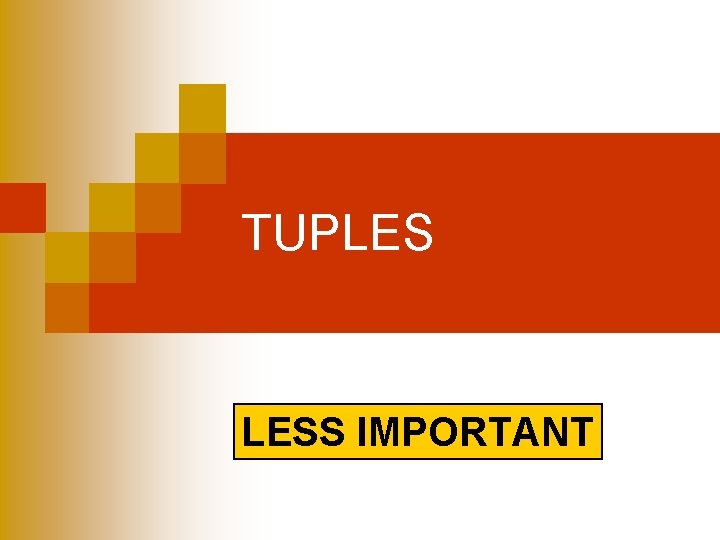
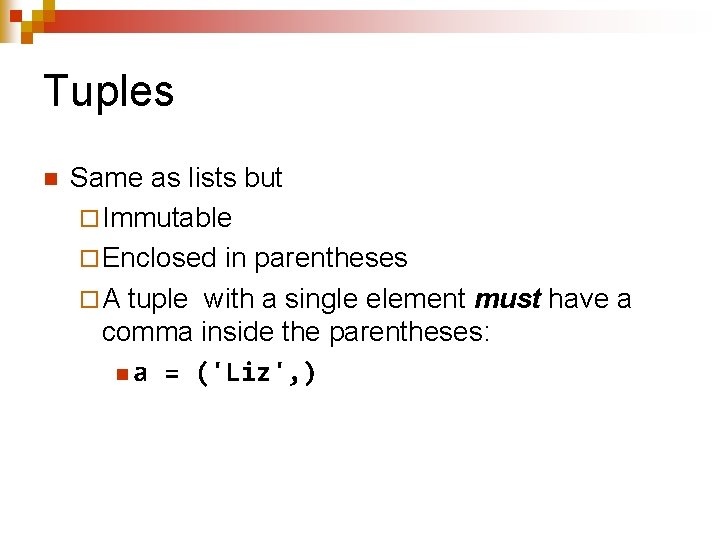
![Examples >>> mytuple = ('Ann', 'Bob', 33) >>> mytuple[0] 'Ann' >>> mytuple[-1] 33 >>> Examples >>> mytuple = ('Ann', 'Bob', 33) >>> mytuple[0] 'Ann' >>> mytuple[-1] 33 >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-111.jpg)
![Why? n No confusion possible between ['Ann'] and 'Ann' n ('Ann') is a perfectly Why? n No confusion possible between ['Ann'] and 'Ann' n ('Ann') is a perfectly](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-112.jpg)
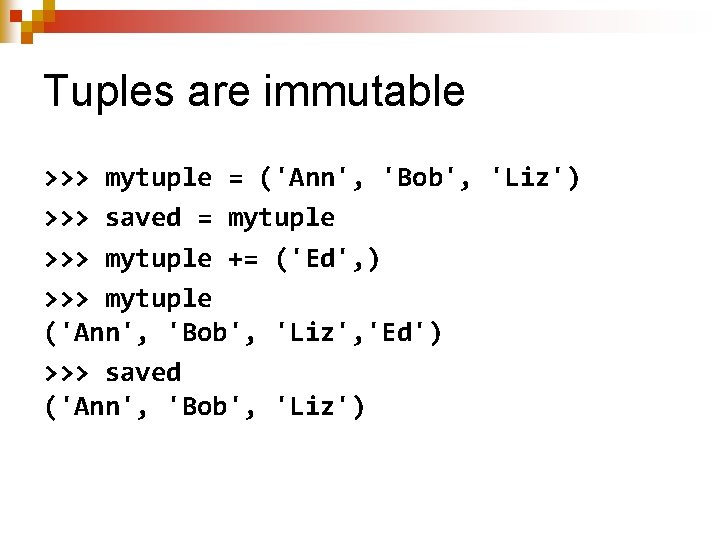
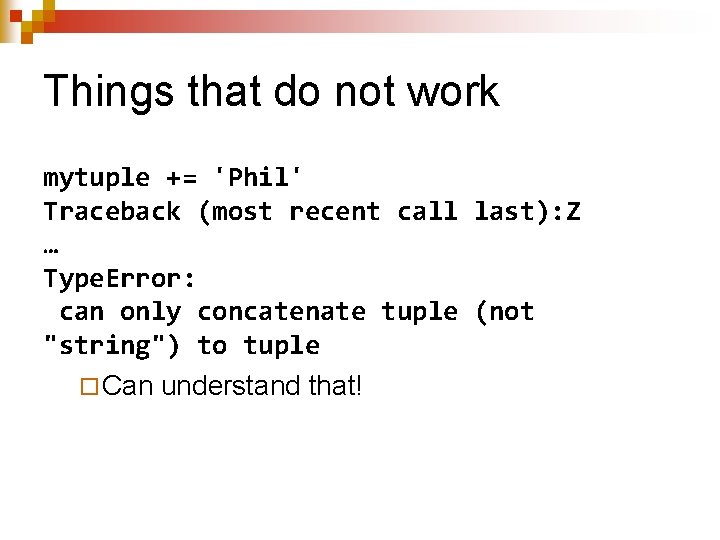
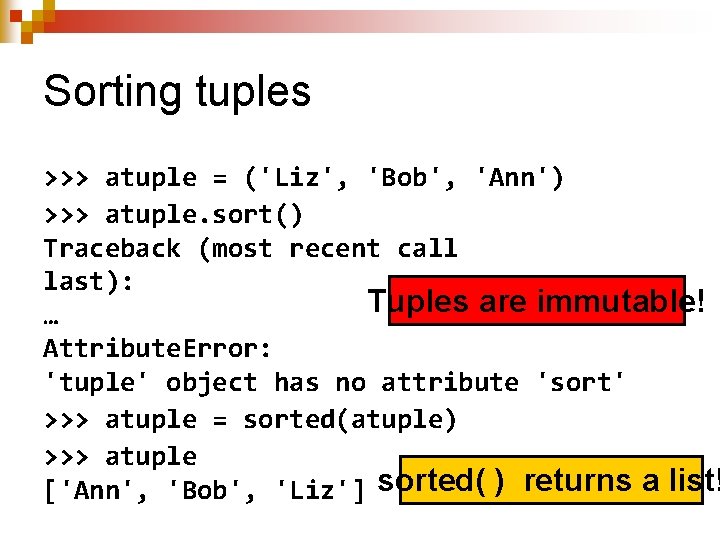
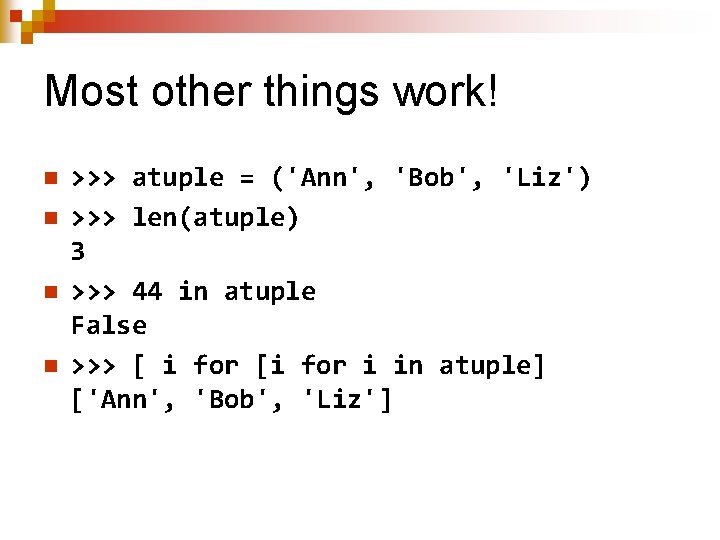
![The reverse does not work >>> alist = ['Ann', 'Bob', 'Liz'] >>> (i for The reverse does not work >>> alist = ['Ann', 'Bob', 'Liz'] >>> (i for](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-117.jpg)
![Converting sequences into tuples >>> alist = ['Ann', 'Bob', 'Liz'] >>> atuple = tuple(alist) Converting sequences into tuples >>> alist = ['Ann', 'Bob', 'Liz'] >>> atuple = tuple(alist)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-118.jpg)
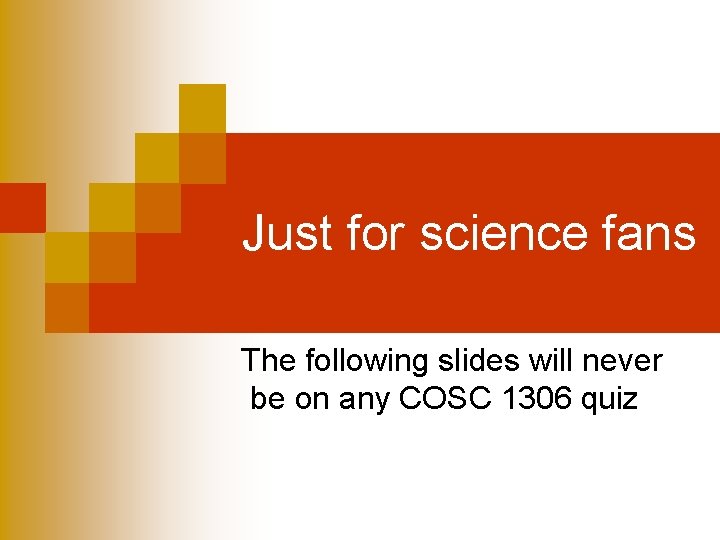
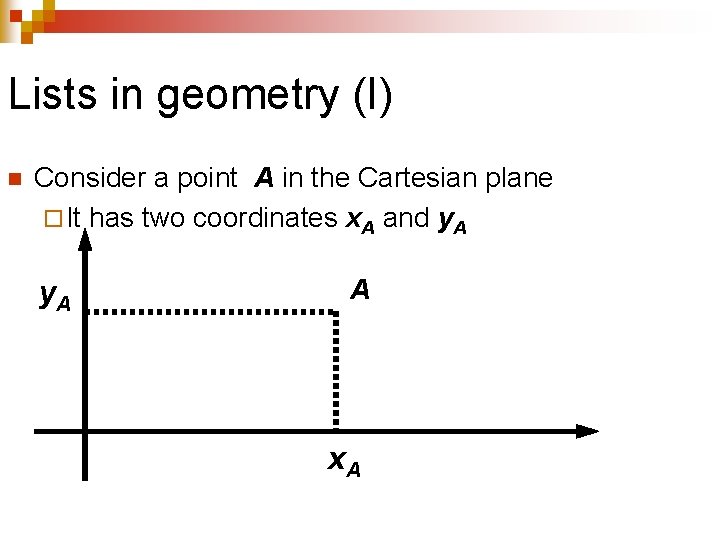
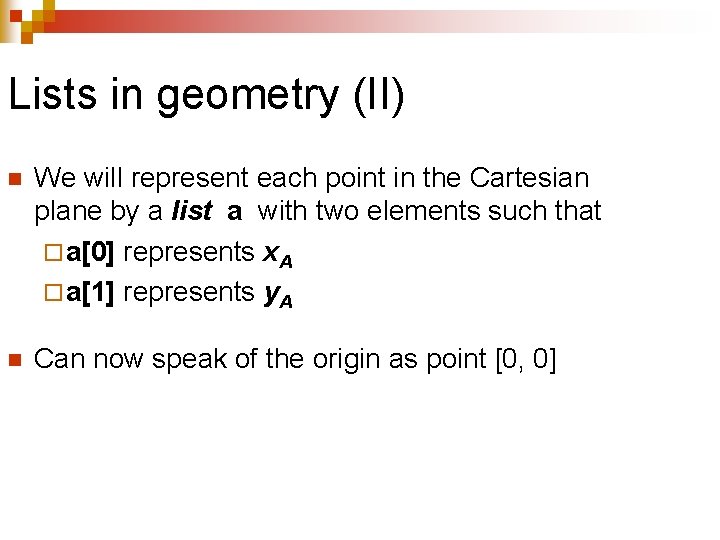
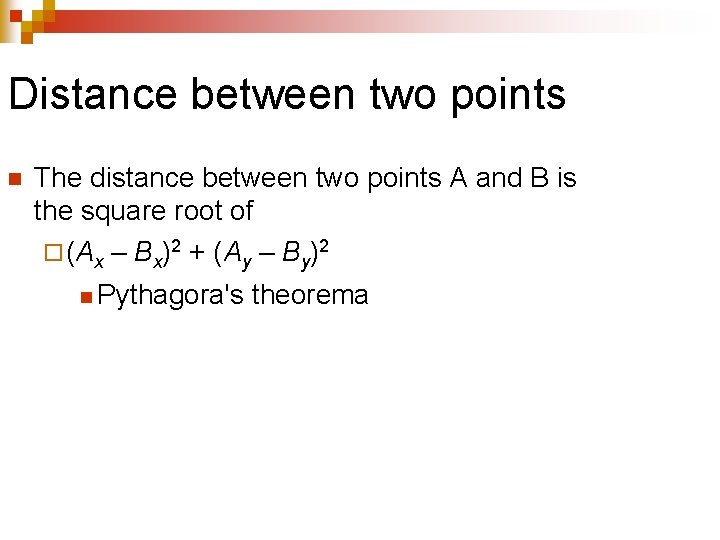
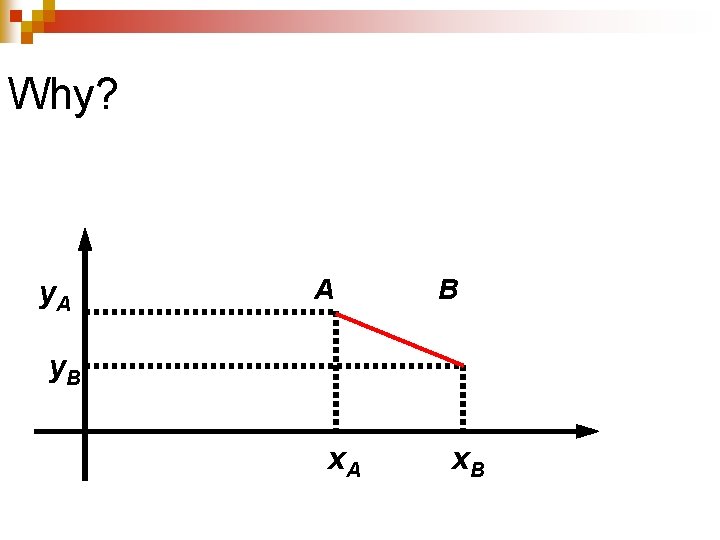
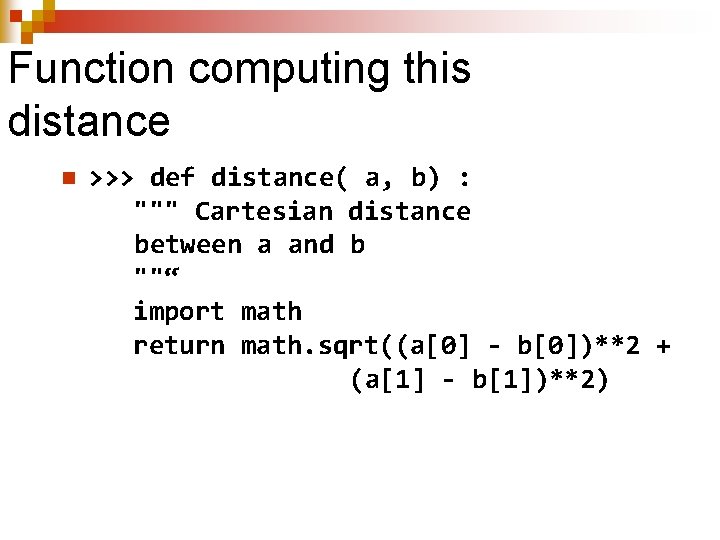
![Function computing this distance n n >>> origin = [0, 0] >>> a = Function computing this distance n n >>> origin = [0, 0] >>> a =](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-125.jpg)
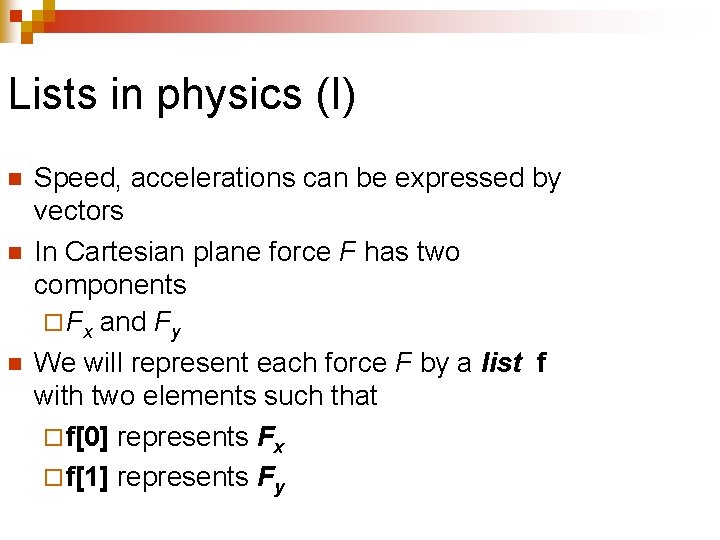
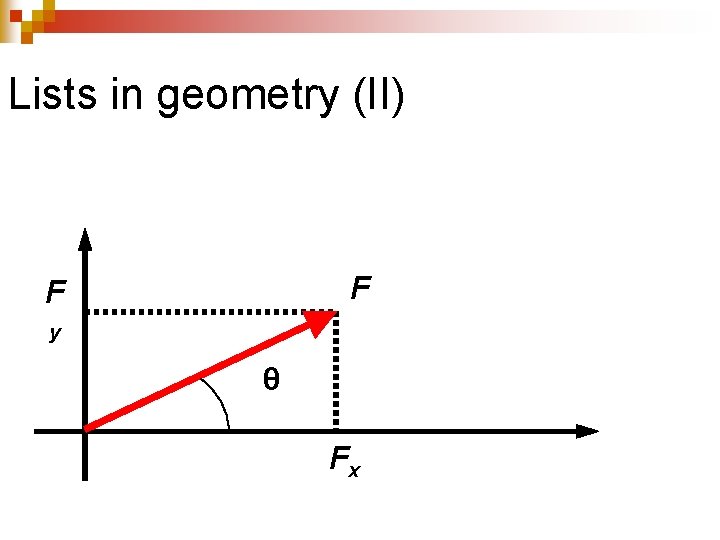
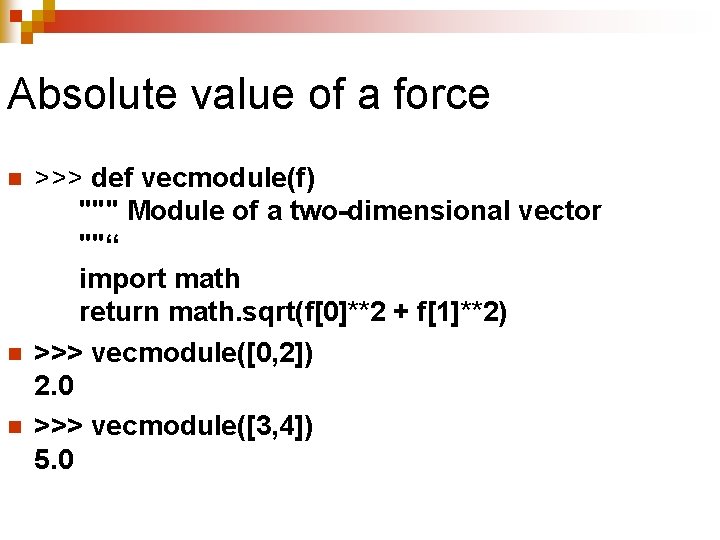
![Adding two vectors (I) n [0, 2] + [0, 3] [0, 2, 0, 3] Adding two vectors (I) n [0, 2] + [0, 3] [0, 2, 0, 3]](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-129.jpg)
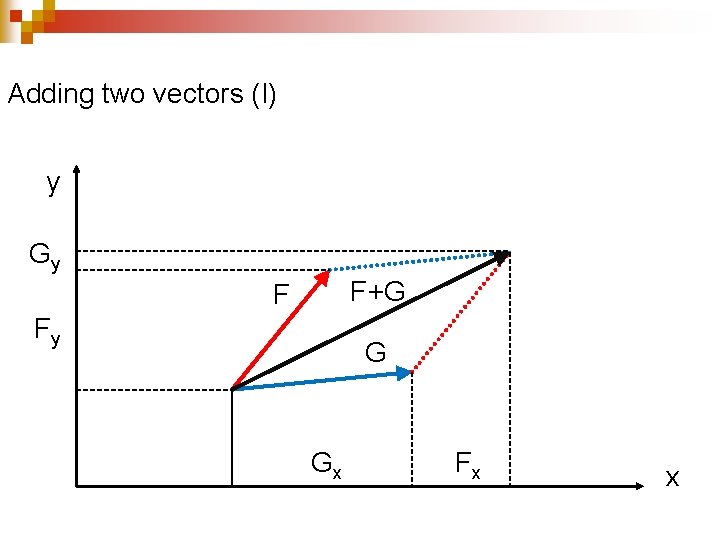
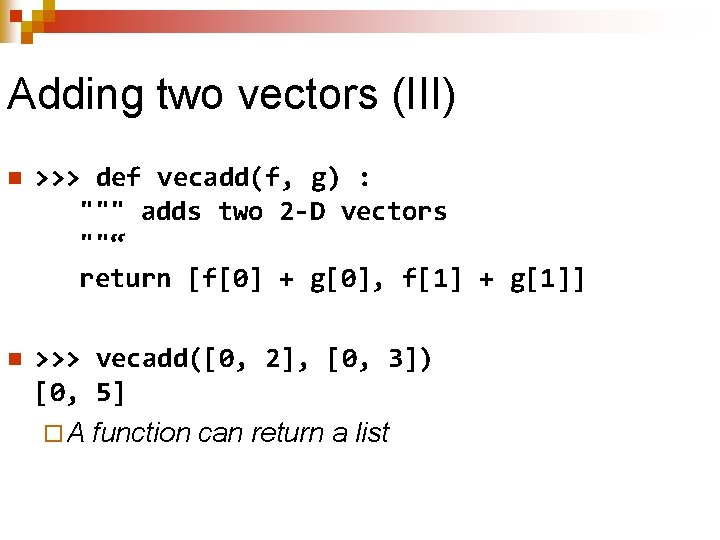
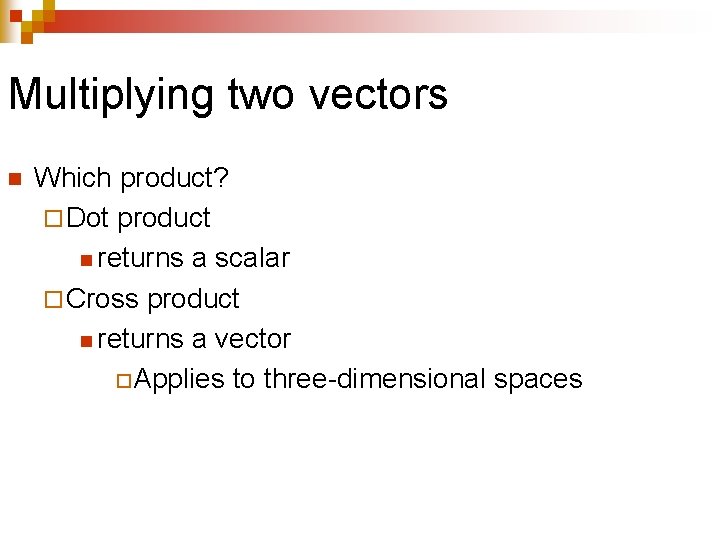
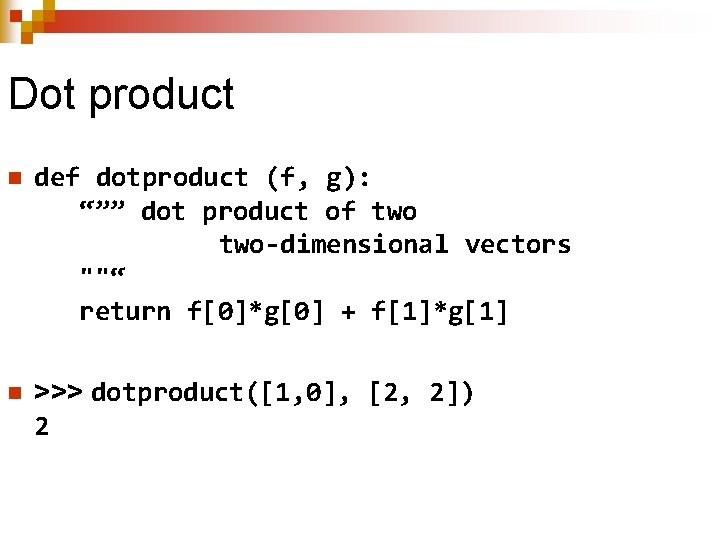
![List of lists (I) n n >>> a = [[1, 2], [3, 1]] >>> List of lists (I) n n >>> a = [[1, 2], [3, 1]] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-134.jpg)
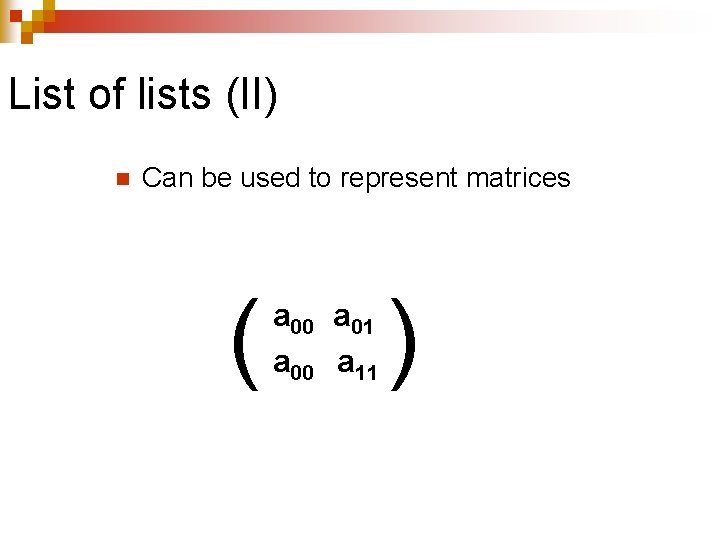
- Slides: 135
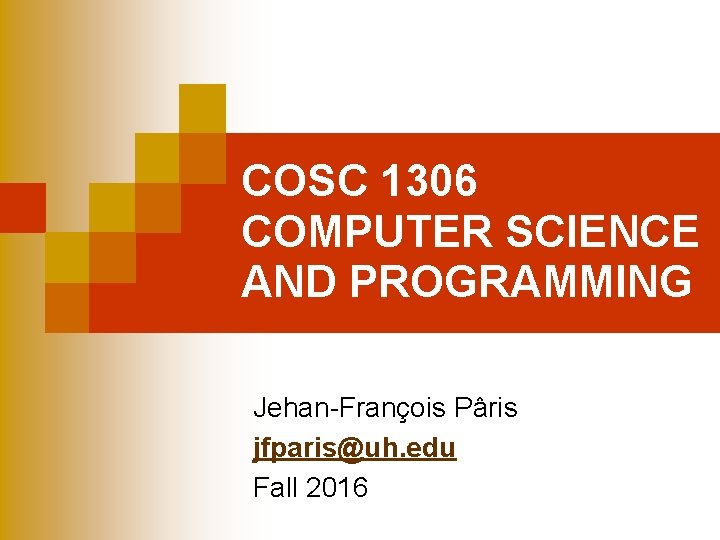
COSC 1306 COMPUTER SCIENCE AND PROGRAMMING Jehan-François Pâris jfparis@uh. edu Fall 2016
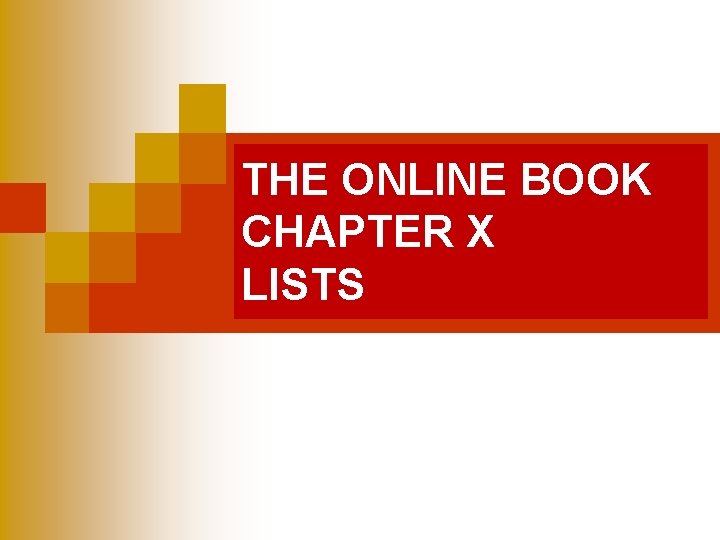
THE ONLINE BOOK CHAPTER X LISTS
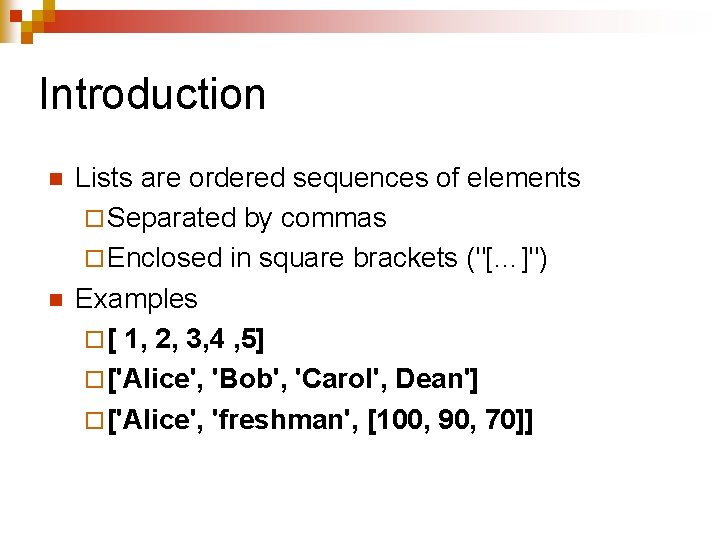
Introduction n n Lists are ordered sequences of elements ¨ Separated by commas ¨ Enclosed in square brackets ("[…]") Examples ¨ [ 1, 2, 3, 4 , 5] ¨ ['Alice', 'Bob', 'Carol', Dean'] ¨ ['Alice', 'freshman', [100, 90, 70]]
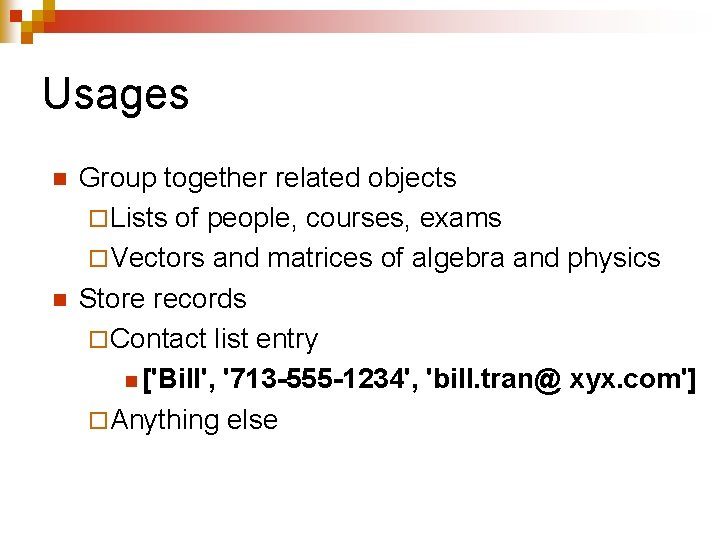
Usages n n Group together related objects ¨ Lists of people, courses, exams ¨ Vectors and matrices of algebra and physics Store records ¨ Contact list entry n ['Bill', '713 -555 -1234', 'bill. tran@ xyx. com'] ¨ Anything else
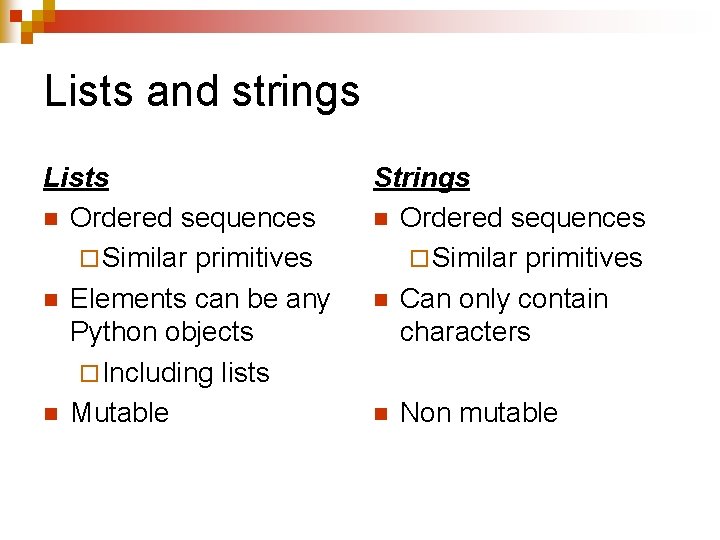
Lists and strings Lists n Ordered sequences ¨ Similar primitives n Elements can be any Python objects ¨ Including lists n Mutable Strings n Ordered sequences ¨ Similar primitives n Can only contain characters n Non mutable
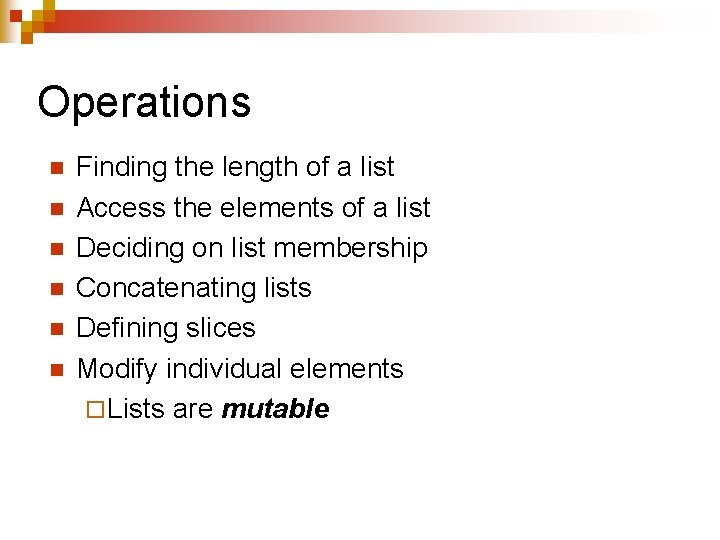
Operations n n n Finding the length of a list Access the elements of a list Deciding on list membership Concatenating lists Defining slices Modify individual elements ¨ Lists are mutable
![Accessing Elements I names Ann Bob Carol end names Ann Bob Accessing Elements (I) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names ['Ann', 'Bob',](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-7.jpg)
Accessing Elements (I) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names ['Ann', 'Bob', 'Carol', 'end'] >>> print(names) ['Ann', 'Bob', 'Carol', 'end'] >>> names[0] 'Ann' ¨ We can access individual elements
![Accessing Elements II names Ann Bob Carol end names1 Bob Accessing Elements (II) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[1] 'Bob' >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-8.jpg)
Accessing Elements (II) >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[1] 'Bob' >>> names[-1] 'end' ¨ List indexing uses the same conventions as string indexing
![Finding the length of a list names Ann Bob Carol end lennames Finding the length of a list names = ['Ann', 'Bob', 'Carol', 'end'] >>> len(names)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-9.jpg)
Finding the length of a list names = ['Ann', 'Bob', 'Carol', 'end'] >>> len(names) 4 >>>alist = ['Ann', 'Bob', 'Carol', [1, 2, 3]] >>> len(alist) 4 n New list has still four elements, the last one is list [1, 2, 3]
![List membership n n names Ann Bob Carol end Ann in names List membership n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> 'Ann' in names](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-10.jpg)
List membership n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> 'Ann' in names True >>> 'Lucy' in names False >>> 'Alice' not in names True ¨ Same operators as for strings
![An example n a 10 20 30 40 50 a designates the whole An example n a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-11.jpg)
An example n a = [10, 20, 30, 40, 50] a designates the whole list 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
![List concatenation I names Alice Bob names Alice Bob List concatenation (I) >>> names = ['Alice'] + ['Bob'] >>> names ['Alice', 'Bob'] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-12.jpg)
List concatenation (I) >>> names = ['Alice'] + ['Bob'] >>> names ['Alice', 'Bob'] >>> names =['Carol'] >>> names = names + 'Dean' … Type. Error: can only concatenate list (not "str") to list
![List concatenation II mylist Ann mylist3 Ann Ann newlist List concatenation (II) >>> mylist = ['Ann'] >>> mylist*3 ['Ann', 'Ann'] >>> newlist =](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-13.jpg)
List concatenation (II) >>> mylist = ['Ann'] >>> mylist*3 ['Ann', 'Ann'] >>> newlist = [0]*8 >>> newlist [0, 0, 0] >>> [[0]*2]*3 [[0, 0], [0, 0]]
![List slices names Ann Bob Carol end names0 1 Ann List slices >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[0: 1] ['Ann'] ¨](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-14.jpg)
List slices >>> names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[0: 1] ['Ann'] ¨ Two observations n A list slice is always a list n names[0: 1] starts with names[0] but stops before names[1]
![More list slices names0 2 Ann Bob Includes names0 and names1 More list slices >>> names[0: 2] ['Ann', 'Bob'] ¨ Includes names[0] and names[1] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-15.jpg)
More list slices >>> names[0: 2] ['Ann', 'Bob'] ¨ Includes names[0] and names[1] >>> names[0: ] ['Ann', 'Bob', 'Carol', 'end'] ¨ The whole list >>> names[1: ] ['Bob', 'Carol', 'end']
![More list slices names1 end n A list slice is a list More list slices >>> names[-1: ] ['end'] n A list slice is a list](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-16.jpg)
More list slices >>> names[-1: ] ['end'] n A list slice is a list >>> names[-1] 'end' n Not the same thing! TRAP WARNING
![Let us check n n names Ann Bob Carol end names1 done Let us check n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[-1] 'done'](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-17.jpg)
Let us check n n names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[-1] 'done' >>> names[-1: ] ['done'] >>> names[-1] == names[-1: ] False What's true for strings is not always true for lists
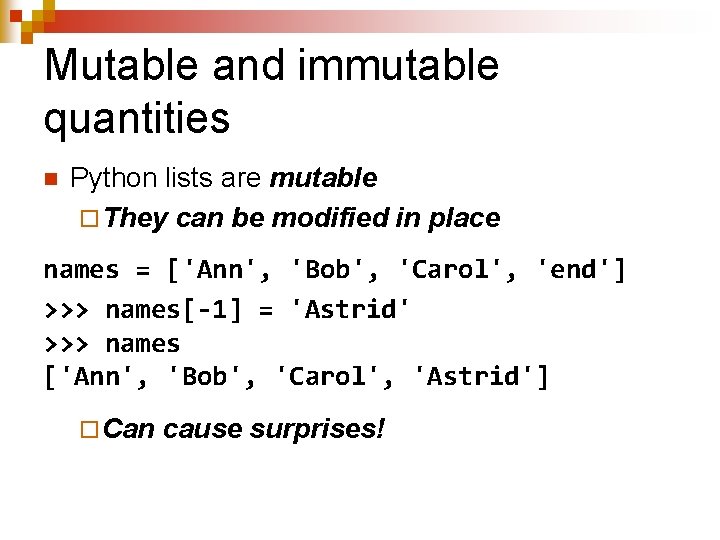
Mutable and immutable quantities n Python lists are mutable ¨ They can be modified in place names = ['Ann', 'Bob', 'Carol', 'end'] >>> names[-1] = 'Astrid' >>> names ['Ann', 'Bob', 'Carol', 'Astrid'] ¨ Can cause surprises!
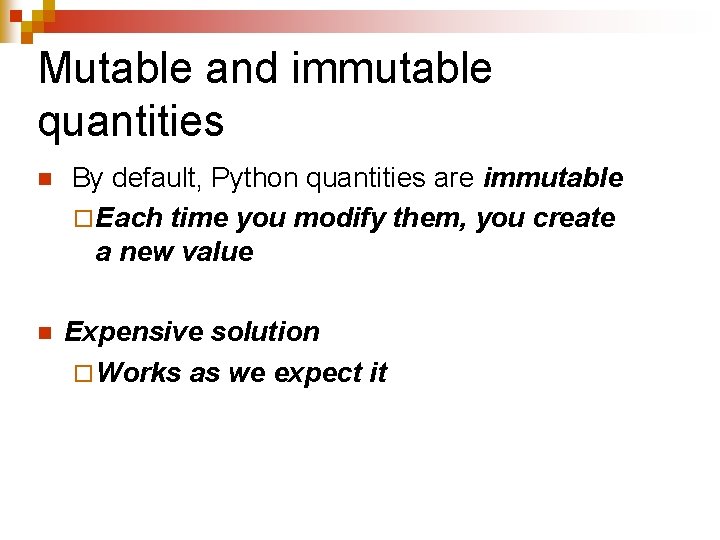
Mutable and immutable quantities n n By default, Python quantities are immutable ¨ Each time you modify them, you create a new value Expensive solution ¨ Works as we expect it
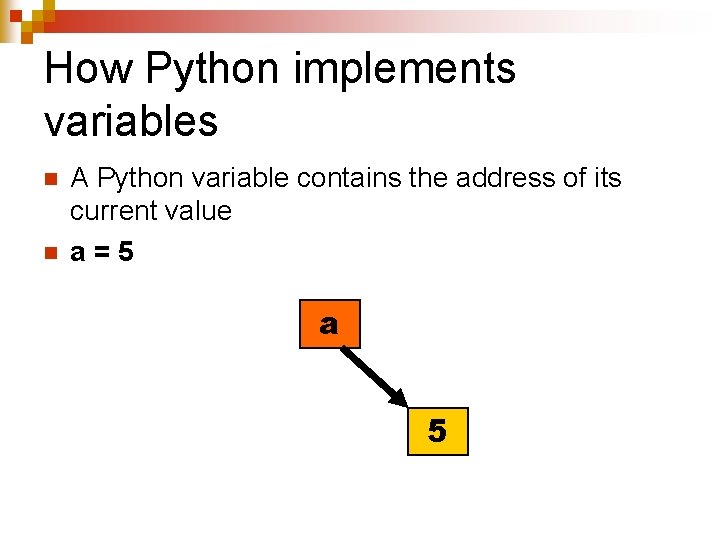
How Python implements variables n n A Python variable contains the address of its current value a=5 a 5
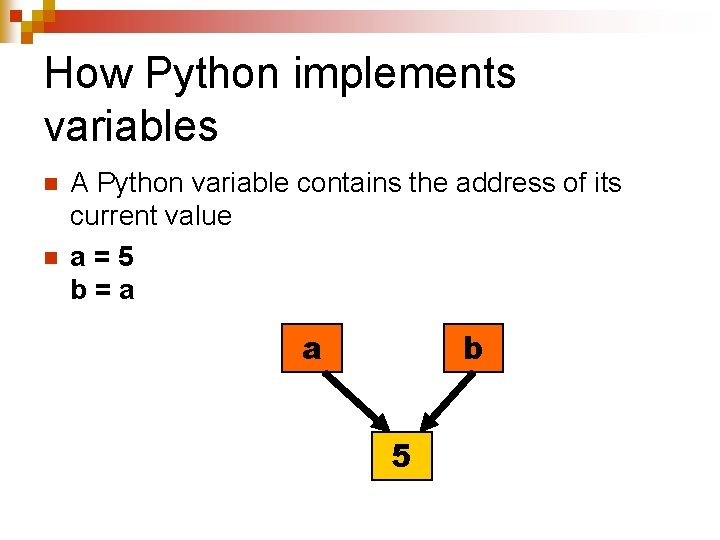
How Python implements variables n n A Python variable contains the address of its current value a=5 b=a a b 5
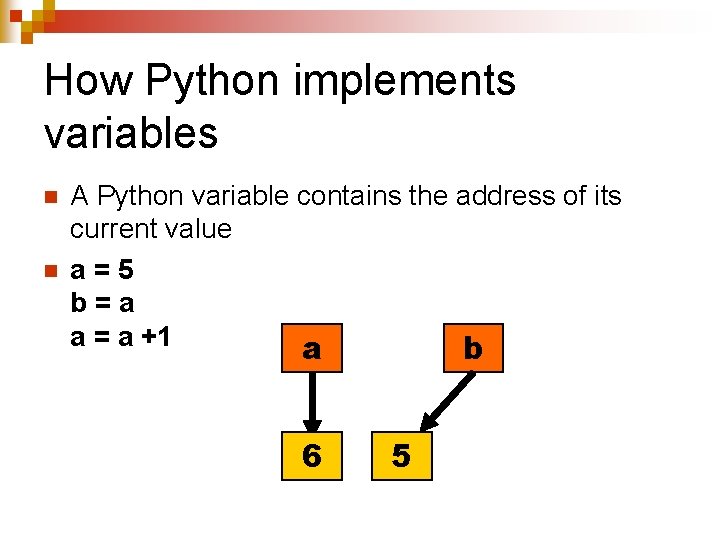
How Python implements variables n n A Python variable contains the address of its current value a=5 b=a a = a +1 a b 6 5
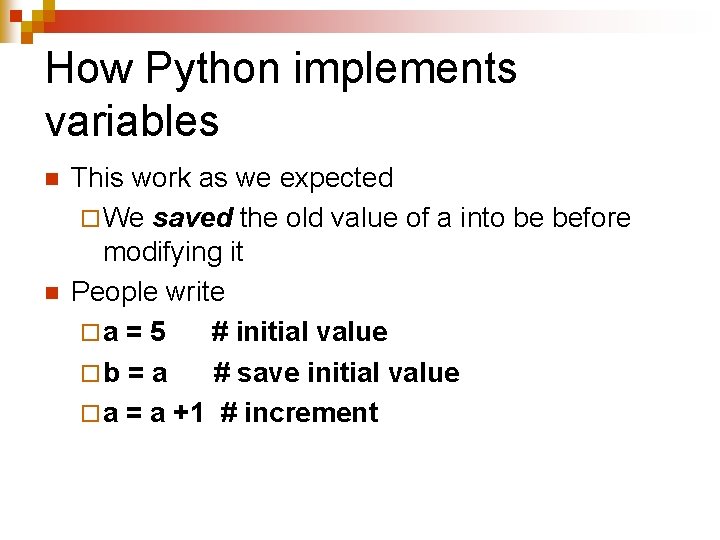
How Python implements variables n n This work as we expected ¨ We saved the old value of a into be before modifying it People write ¨a = 5 # initial value ¨b = a # save initial value ¨ a = a +1 # increment
![A big surprise n n n a Ann Bob Carol b A big surprise n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-24.jpg)
A big surprise n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b = a >>> b ['Ann', 'Bob', 'Carol'] >>> a[0] = 'Lucy' >>> a ['Lucy', 'Bob', 'Carol'] The old value of a >>> b ['Lucy', 'Bob', 'Carol'] was not saved!
![What happened I n n n a Ann Bob Carol b What happened (I) n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-25.jpg)
What happened (I) n n n >>> a = ['Ann', 'Bob', 'Carol'] >>> b = a >>> b ['Ann', 'Bob', 'Carol'] a b ['Ann', 'Bob', 'Carol']
![What happened II n n n a0 Lucy a Lucy Bob What happened (II) n n n >>> a[0] = 'Lucy' >>> a ['Lucy', 'Bob',](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-26.jpg)
What happened (II) n n n >>> a[0] = 'Lucy' >>> a ['Lucy', 'Bob', 'Carol'] >>> b ['Lucy', 'Bob', 'Carol'] a b ['Lucy', 'Bob', 'Carol']
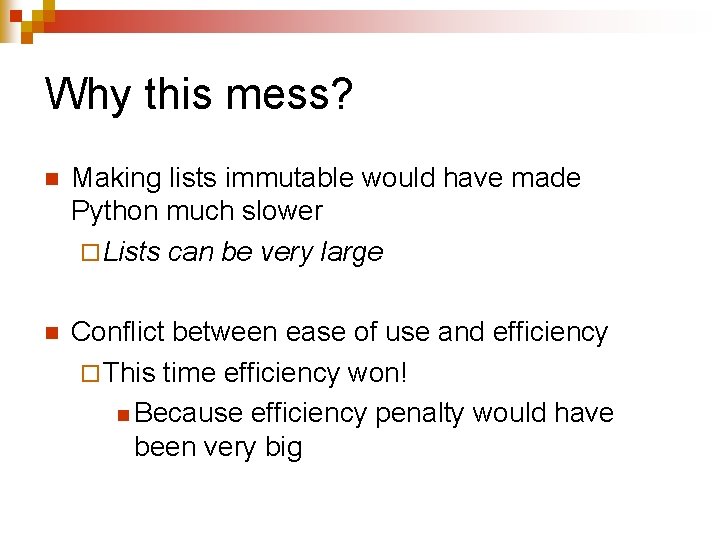
Why this mess? n Making lists immutable would have made Python much slower ¨ Lists can be very large n Conflict between ease of use and efficiency ¨ This time efficiency won! n Because efficiency penalty would have been very big
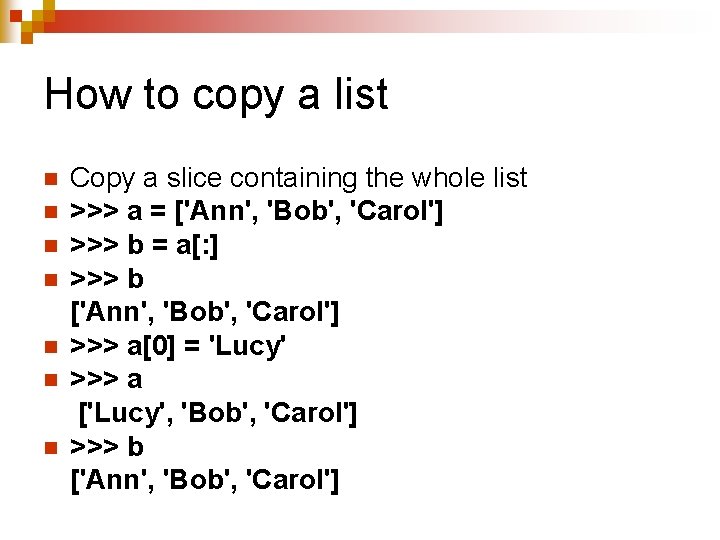
How to copy a list n n n n Copy a slice containing the whole list >>> a = ['Ann', 'Bob', 'Carol'] >>> b = a[: ] >>> b ['Ann', 'Bob', 'Carol'] >>> a[0] = 'Lucy' >>> a ['Lucy', 'Bob', 'Carol'] >>> b ['Ann', 'Bob', 'Carol']
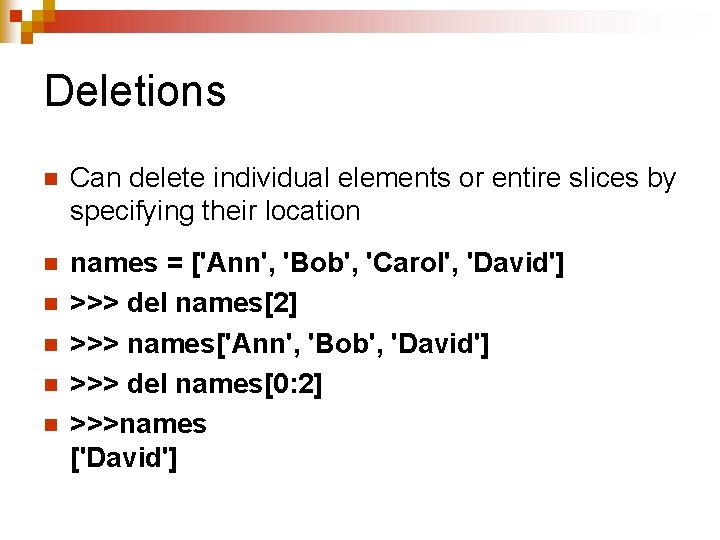
Deletions n Can delete individual elements or entire slices by specifying their location n names = ['Ann', 'Bob', 'Carol', 'David'] >>> del names[2] >>> names['Ann', 'Bob', 'David'] >>> del names[0: 2] >>>names ['David'] n n
![Object and references I n n a Ann b Ann Object and references (I) n n >>> a = ['Ann'] >>> b = ['Ann']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-30.jpg)
Object and references (I) n n >>> a = ['Ann'] >>> b = ['Ann'] >>> a == b True >>> a is b False ¨a and b point to different objects
![No sharing a b Ann No sharing a b ['Ann']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-31.jpg)
No sharing a b ['Ann']
![Object and references II n n a Ann b a Object and references (II) n n >>> a = ['Ann'] >>> b = a](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-32.jpg)
Object and references (II) n n >>> a = ['Ann'] >>> b = a >>> a == b True >>> a is b True ¨a and b now point to the same object
![Same object is shared a b Ann Same object is shared a b ['Ann']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-33.jpg)
Same object is shared a b ['Ann']
![Aliasing and cloning I n When we do a Ann Aliasing and cloning (I) n When we do ¨ >>> a = ['Ann'] ¨](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-34.jpg)
Aliasing and cloning (I) n When we do ¨ >>> a = ['Ann'] ¨ >>> b = a we do not make a copy of a, we just assign an additional variable name to the object pointed by a n b is just an alias for a
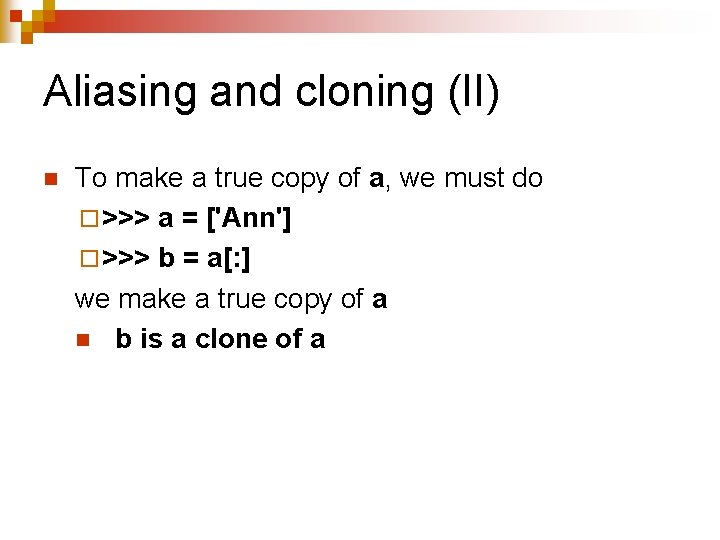
Aliasing and cloning (II) n To make a true copy of a, we must do ¨ >>> a = ['Ann'] ¨ >>> b = a[: ] we make a true copy of a n b is a clone of a
![A weird behavior n n n pets cats dogs oddlist pets2 A weird behavior n n n >>> pets = ['cats', 'dogs'] >>> oddlist =[pets]*2](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-36.jpg)
A weird behavior n n n >>> pets = ['cats', 'dogs'] >>> oddlist =[pets]*2 >>> oddlist [['cats', 'dogs'], ['cats', 'dogs']] >>> pets[0] = 'snake' >>> pets ['snake', 'dogs'] >>> oddlist [['snake', 'dogs'], ['snake', 'dogs']]
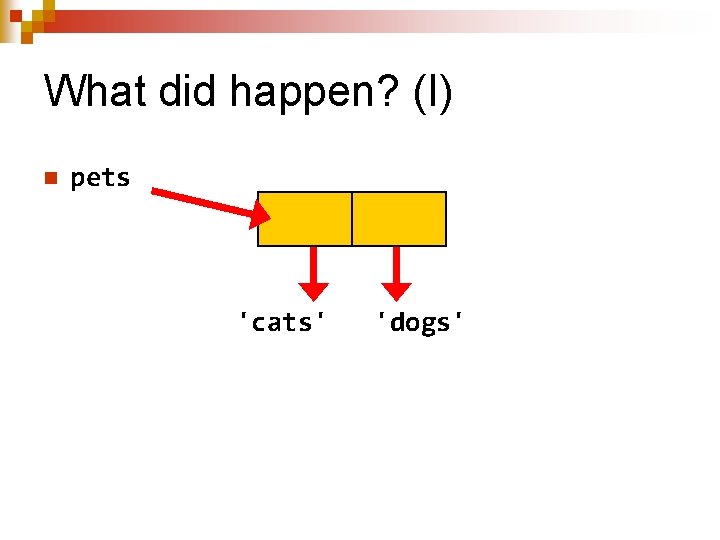
What did happen? (I) n pets 'cats' 'dogs'
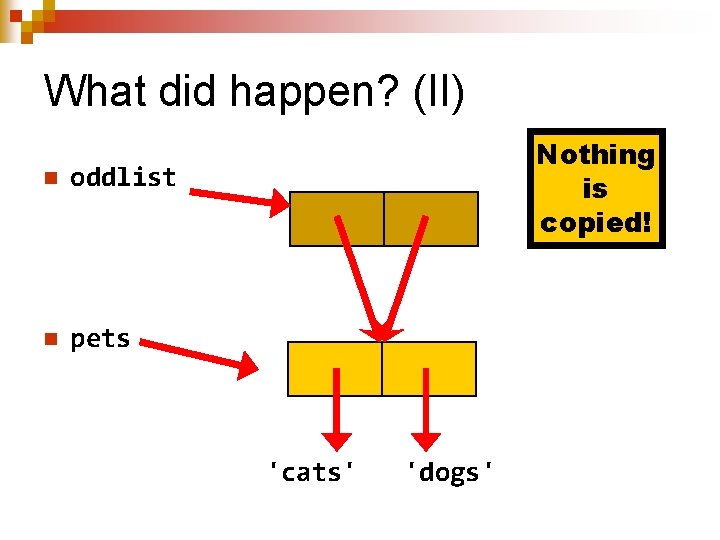
What did happen? (II) n oddlist n pets Nothing is copied! 'cats' 'dogs'
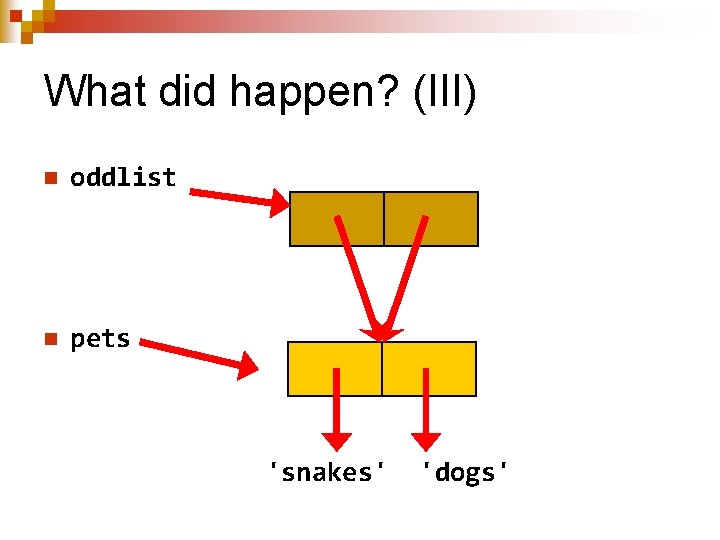
What did happen? (III) n oddlist n pets 'snakes' 'dogs'
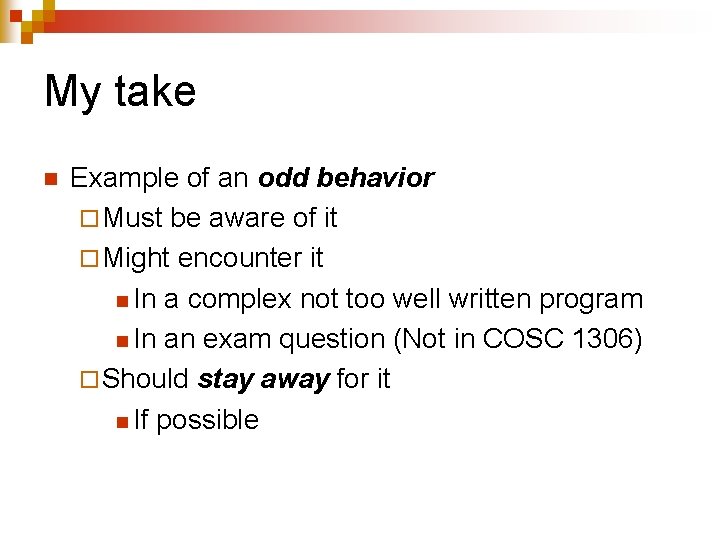
My take n Example of an odd behavior ¨ Must be aware of it ¨ Might encounter it n In a complex not too well written program n In an exam question (Not in COSC 1306) ¨ Should stay away for it n If possible
![Editing list mylist 11 12 13 done mylist1 finished Editing list >>> mylist = [11, 12, 13, 'done'] >>> mylist[-1] = 'finished' >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-41.jpg)
Editing list >>> mylist = [11, 12, 13, 'done'] >>> mylist[-1] = 'finished' >>> mylist [11, 12, 13, 'finished'] >>> mylist[0: 4] = ['XI', 'XIII'] >>> mylist ['XI', 'XIII', 'finished'] We can change the values of the existing list entries but cannot add or delete any of them
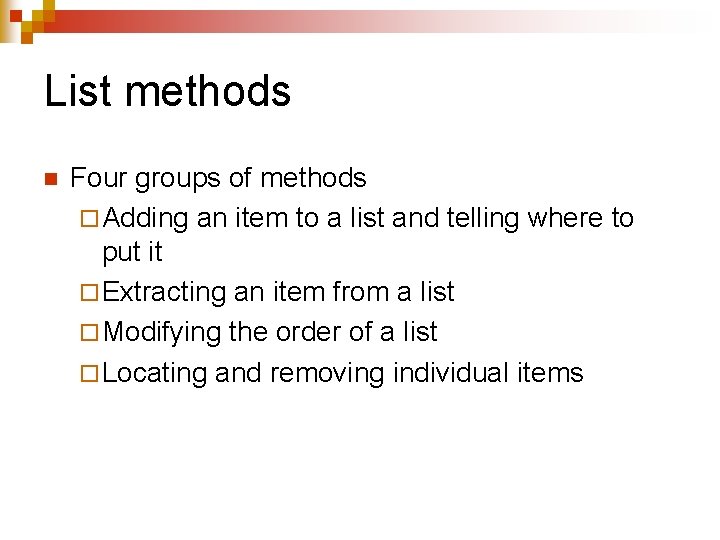
List methods n Four groups of methods ¨ Adding an item to a list and telling where to put it ¨ Extracting an item from a list ¨ Modifying the order of a list ¨ Locating and removing individual items
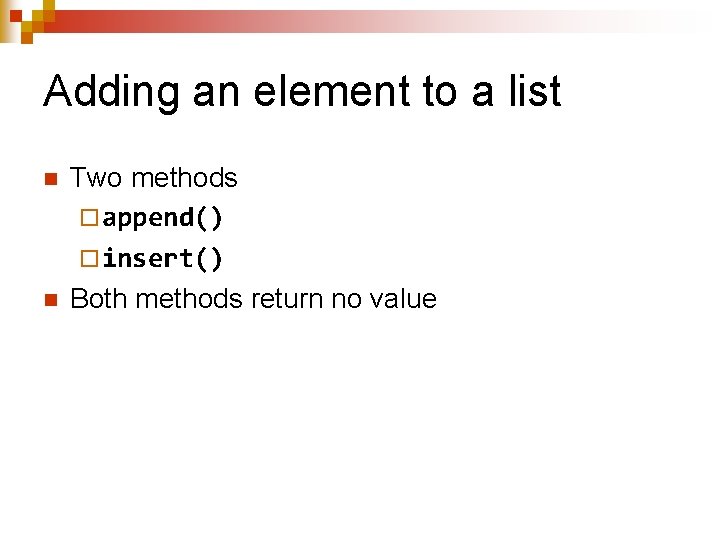
Adding an element to a list n n Two methods ¨ append() ¨ insert() Both methods return no value
![AppendI n n n mylist 11 12 13 finished mylist appendNot Append(I) n n n >>> mylist = [11, 12, 13, 'finished'] >>> mylist. append('Not](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-44.jpg)
Append(I) n n n >>> mylist = [11, 12, 13, 'finished'] >>> mylist. append('Not yet!') >>> mylist [11, 12, 13, 'finished', 'Not yet!']
![Append II listoflists 14 5 1306 17 5 6360 listoflists append16 Append (II) >>> listoflists = [[14. 5, '1306'], [17. 5, '6360']] >>> listoflists. append([16.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-45.jpg)
Append (II) >>> listoflists = [[14. 5, '1306'], [17. 5, '6360']] >>> listoflists. append([16. 5, 'TAs']) >>> listoflists [[14. 5, '1306'], [17. 5, '6360'], [16. 5, 'TAs']] Appending means adding at the end.
![Insert I mylist 11 12 13 finished mylist insert0 10 BEFORE Insert (I) >>> mylist = [11, 12, 13, 'finished'] >>> mylist. insert(0, 10) #BEFORE](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-46.jpg)
Insert (I) >>> mylist = [11, 12, 13, 'finished'] >>> mylist. insert(0, 10) #BEFORE mylist[0] >>> mylist [10, 11, 12, 13, 'finished'] >>> mylist. insert(4, 14) #BEFORE mylist[4] >>> mylist [10, 11, 12, 13, 14, 'finished']
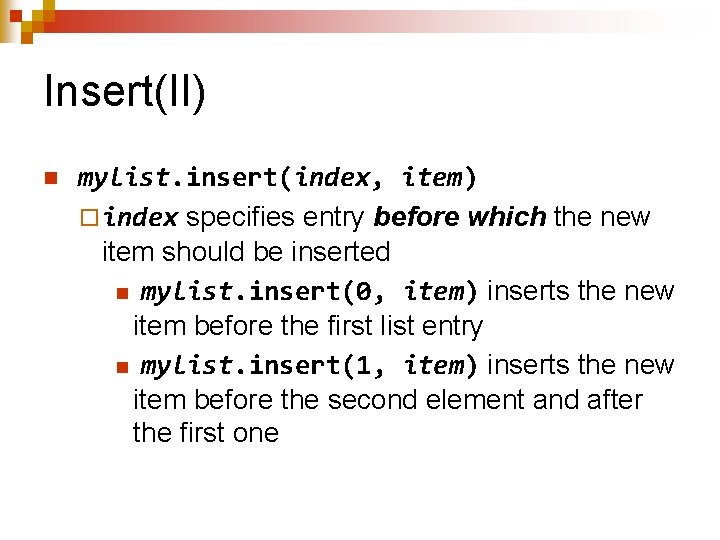
Insert(II) n mylist. insert(index, item) ¨ index specifies entry before which the new item should be inserted n mylist. insert(0, item) inserts the new item before the first list entry n mylist. insert(1, item) inserts the new item before the second element and after the first one
![Example I n a 10 20 30 40 50 a designates the whole Example (I) n a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-48.jpg)
Example (I) n a = [10, 20, 30, 40, 50] a designates the whole list 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
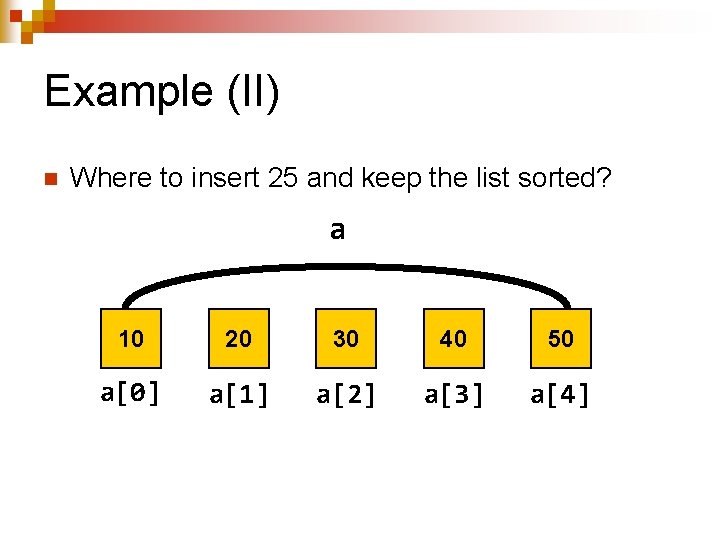
Example (II) n Where to insert 25 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
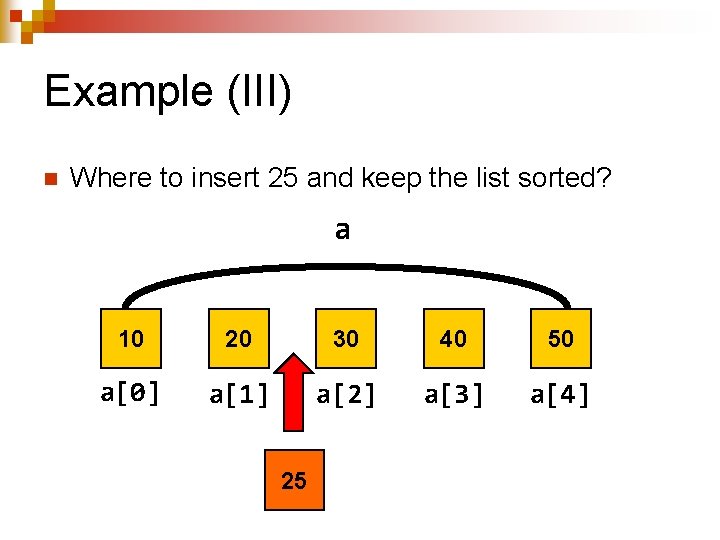
Example (III) n Where to insert 25 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4] 25
![Example IV n We do a insert2 25 n after a1 and before Example (IV) n We do ¨ a. insert(2, 25) n after a[1] and before](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-51.jpg)
Example (IV) n We do ¨ a. insert(2, 25) n after a[1] and before a[2]
![Let us check a 10 20 30 40 50 a insert2 Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(2,](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-52.jpg)
Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(2, 25) >>> a [10, 25, 30, 40, 50]
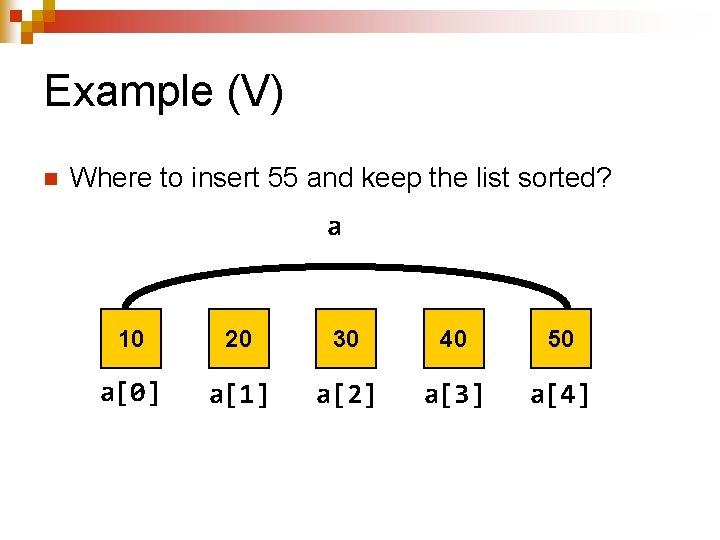
Example (V) n Where to insert 55 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
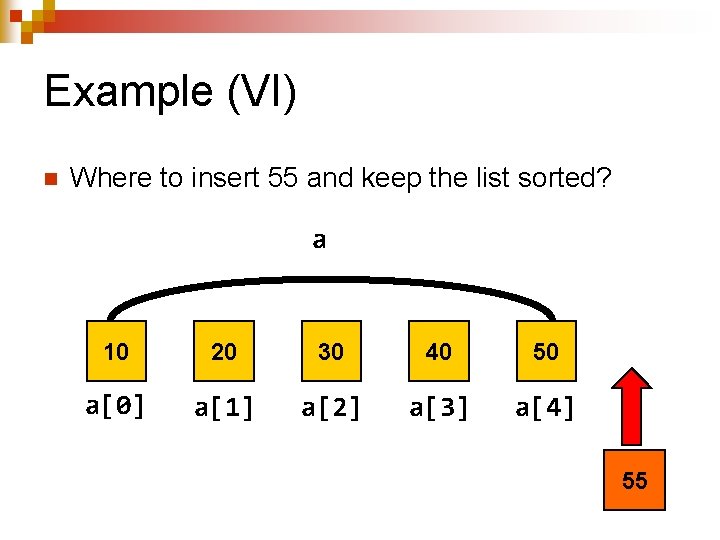
Example (VI) n Where to insert 55 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4] 55
![Example VII n n We must insert After a4 Before no other Example (VII) n n We must insert ¨ After a[4] ¨ Before no other](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-55.jpg)
Example (VII) n n We must insert ¨ After a[4] ¨ Before no other element We act as if a[5] existed ¨ a. insert(5, 55) n It works! n Same as a. append(55)
![Let us check a 10 20 30 40 50 a insert5 Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(5,](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-56.jpg)
Let us check >>> a = [10, 20, 30, 40, 50] >>> a. insert(5, 55) >>> a [10, 20, 30, 40, 55]
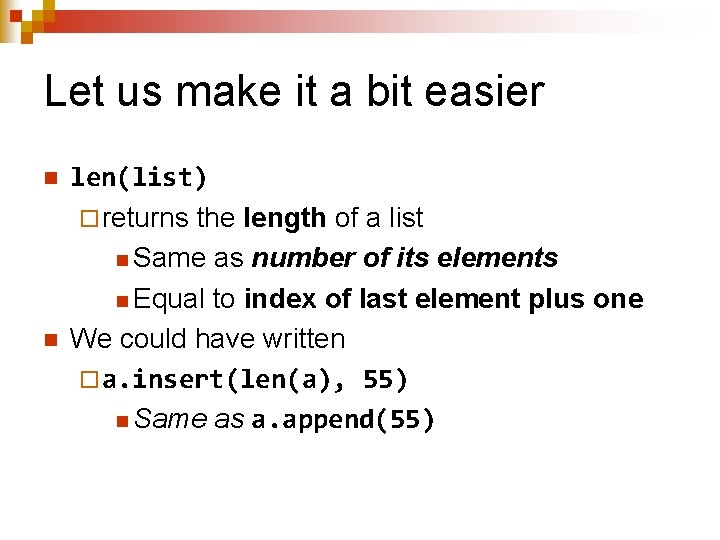
Let us make it a bit easier n n len(list) ¨ returns the length of a list n Same as number of its elements n Equal to index of last element plus one We could have written ¨ a. insert(len(a), 55) n Same as a. append(55)
![Extracting items n n n One by one thislist popi removes thislisti from Extracting items n n n One by one thislist. pop(i) ¨ removes thislist[i] from](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-58.jpg)
Extracting items n n n One by one thislist. pop(i) ¨ removes thislist[i] from thislist ¨ returns the removed entry thislist. pop( ) ¨ removes the last entry from thislist ¨ returns the removed entry
![Examples I mylist 11 22 33 44 55 66 mylist pop0 Examples (I) >>> mylist = [11, 22, 33, 44, 55, 66] >>> mylist. pop(0)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-59.jpg)
Examples (I) >>> mylist = [11, 22, 33, 44, 55, 66] >>> mylist. pop(0) 11 >>> mylist [22, 33, 44, 55, 66]
![Examples II mylist pop 66 mylist 22 33 44 55 mylist Examples (II) >>> mylist. pop() 66 >>> mylist [22, 33, 44, 55] >>> mylist.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-60.jpg)
Examples (II) >>> mylist. pop() 66 >>> mylist [22, 33, 44, 55] >>> mylist. pop(2) 44 >>> mylist [22, 33, 55]
![Examples III waitlist Ann Cristi Dean waitlist pop0 Ann waitlist Examples (III) >>> waitlist = ['Ann', 'Cristi', 'Dean'] >>> waitlist. pop(0) 'Ann' >>> waitlist](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-61.jpg)
Examples (III) >>> waitlist = ['Ann', 'Cristi', 'Dean'] >>> waitlist. pop(0) 'Ann' >>> waitlist ['Cristi', 'Dean'] >>> waitlist. append('David') >>> waitlist ['Cristi', 'Dean', 'David']
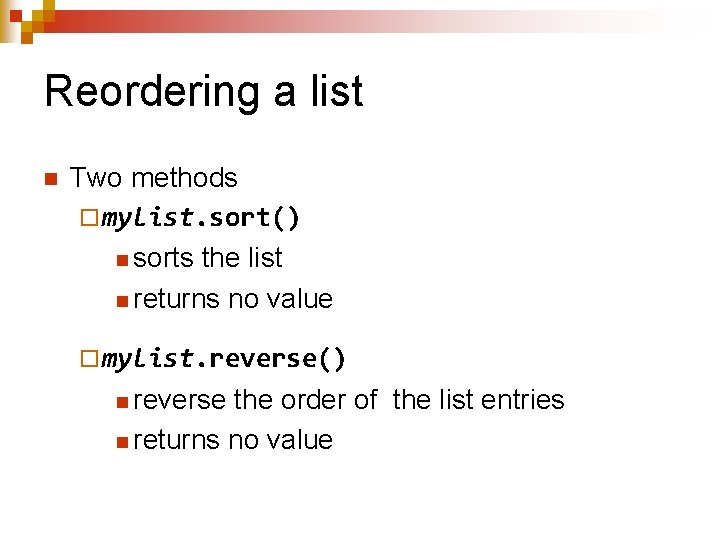
Reordering a list n Two methods ¨ mylist. sort() n sorts the list n returns no value ¨ mylist. reverse() n reverse the order of the list entries n returns no value
![Sorting lists mylist 11 12 13 14 done mylist sort Traceback Sorting lists >>> mylist = [11, 12, 13, 14, 'done'] >>> mylist. sort() Traceback](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-63.jpg)
Sorting lists >>> mylist = [11, 12, 13, 14, 'done'] >>> mylist. sort() Traceback (most recent call last): File "<pyshell#2>", line 1, in <module> mylist. sort() Type. Error: unorderable types: str() < int() ¨ Cannot compare apples and oranges
![Sorting lists of strings names Alice Carol Bob names sort Sorting lists of strings >>> names = ['Alice', 'Carol', 'Bob'] >>> names. sort() >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-64.jpg)
Sorting lists of strings >>> names = ['Alice', 'Carol', 'Bob'] >>> names. sort() >>> print(names) ['Alice', 'Bob', 'Carol'] n names. sort() is a method applied to the list names ¨ In-place sort
![Sorting lists of numbers newlist 0 1 1 2 2 newlist Sorting lists of numbers >>> newlist = [0, -1, +1, -2, +2] >>> newlist.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-65.jpg)
Sorting lists of numbers >>> newlist = [0, -1, +1, -2, +2] >>> newlist. sort() >>> print(newlist) [-2, -1, 0, 1, 2] ¨ In-place sort
![Sorting lists with sublists schedule 14 5 1306 17 5 6360 16 Sorting lists with sublists >>> schedule = [[14. 5, '1306'], [17. 5, '6360'], [16.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-66.jpg)
Sorting lists with sublists >>> schedule = [[14. 5, '1306'], [17. 5, '6360'], [16. 5, 'TA']] >>> schedule. sort() >>> schedule [[14. 5, '1306'], [16. 5, 'TA'], [17. 5, '6360']] >>>
![Reversing the order of a list names Alice Bob Carol names Reversing the order of a list >>> names = ['Alice', 'Bob', 'Carol'] >>> names.](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-67.jpg)
Reversing the order of a list >>> names = ['Alice', 'Bob', 'Carol'] >>> names. reverse() >>> names ['Carol', 'Bob', 'Alice'] n namelist. reverse() is a method applied to the list namelist
![Sorting into a new list newlist 0 1 1 2 2 Sorting into a new list >>> newlist = [0, -1, +1, -2, +2] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-68.jpg)
Sorting into a new list >>> newlist = [0, -1, +1, -2, +2] >>> sortedlist= sorted(newlist) >>> print(newlist) [0, -1, 1, -2, 2] >>> print(sortedlist) [-2, -1, 0, 1, 2] ¨ sorted(…) is a conventional Python function that returns a new list
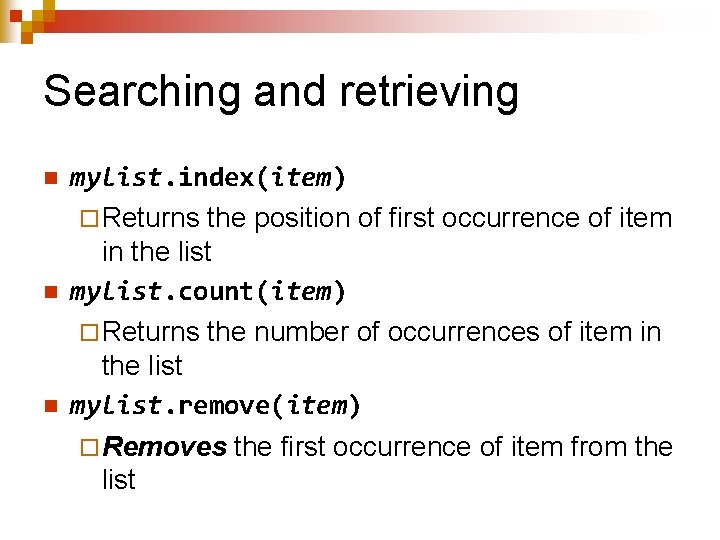
Searching and retrieving n n n mylist. index(item) ¨ Returns the position of first occurrence of item in the list mylist. count(item) ¨ Returns the number of occurrences of item in the list mylist. remove(item) ¨ Removes the first occurrence of item from the list
![Examples n n n n n names Ann Carol Bob Alice Ann Examples n n n n n >>> names ['Ann', 'Carol', 'Bob', 'Alice', 'Ann'] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-70.jpg)
Examples n n n n n >>> names ['Ann', 'Carol', 'Bob', 'Alice', 'Ann'] >>> names. index('Carol') 1 >>> names. count('Ann') 2 >>> names. remove('Ann') >>> names ['Carol', 'Bob', 'Alice', 'Ann']
![Sum a very useful function list 10 20 sumlist 50 Sum: a very useful function >>> list = [10, 20] >>> sum(list) 50 >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-71.jpg)
Sum: a very useful function >>> list = [10, 20] >>> sum(list) 50 >>> list = ['Alice', ' and ', 'Bob'] >>> sum(list) ¨ Does not work!
![Easy averages prices 1 899 1 959 2 029 2 079 Easy averages >>> prices = [1. 899, 1. 959, 2. 029, 2. 079] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-72.jpg)
Easy averages >>> prices = [1. 899, 1. 959, 2. 029, 2. 079] >>> sum(prices) 7. 9660000001 >>> len(prices) 4 >>> sum(prices)/len(prices) # GETS AVERAGE 1. 99150000003
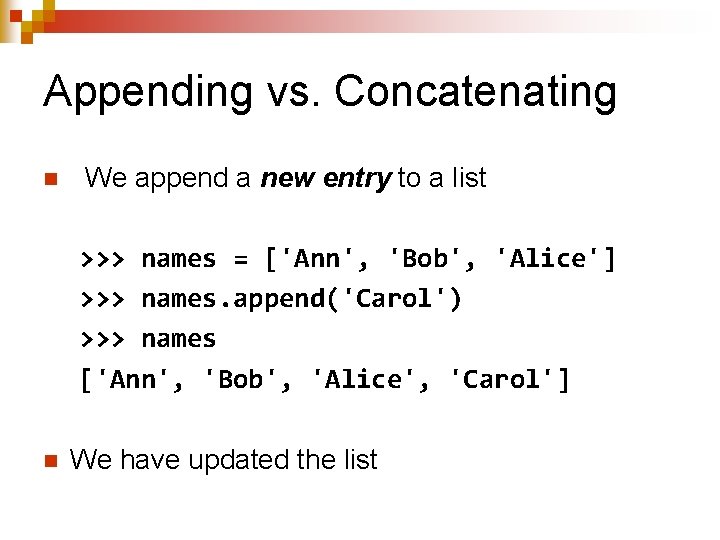
Appending vs. Concatenating n We append a new entry to a list >>> names = ['Ann', 'Bob', 'Alice'] >>> names. append('Carol') >>> names ['Ann', 'Bob', 'Alice', 'Carol'] n We have updated the list
![Appending vs Concatenating n We concatenate two lists names Ann Bob Alice Appending vs. Concatenating n We concatenate two lists >>> names = ['Ann', 'Bob', 'Alice']](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-74.jpg)
Appending vs. Concatenating n We concatenate two lists >>> names = ['Ann', 'Bob', 'Alice'] >>> names + ['Carol'] ['Ann', 'Bob', 'Alice', 'Carol'] >>> names ['Ann', 'Bob', 'Alice'] # UNCHANGED >>> names = names +['Carol'] >>> names ['Ann', 'Bob', 'Alice', 'Carol'] # OK
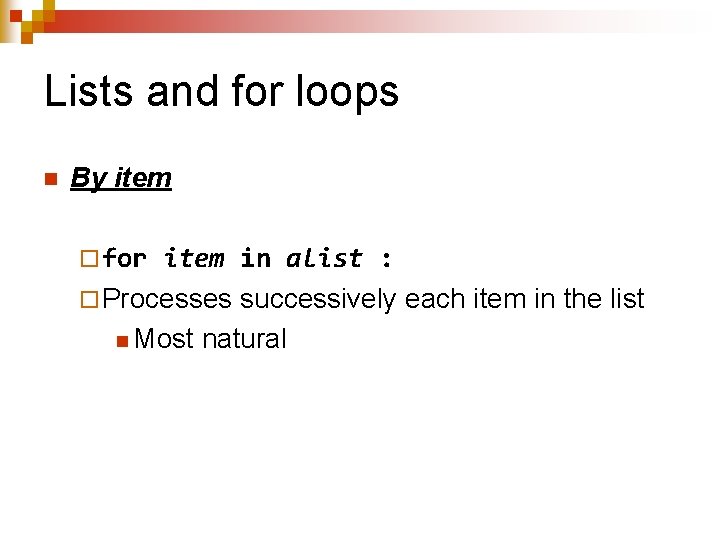
Lists and for loops n By item ¨ for item in alist : ¨ Processes successively each item in the list n Most natural
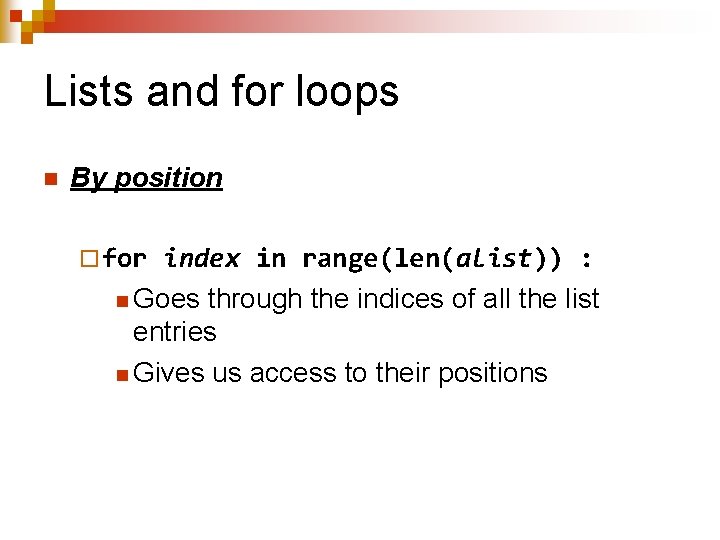
Lists and for loops n By position ¨ for index in range(len(alist)) : n Goes through the indices of all the list entries n Gives us access to their positions
![Examples names Ann Bob Alice Carol for item in names printitem Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for item in names : print(item)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-77.jpg)
Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for item in names : print(item) Ann Bob Alice Carol
![Usage scores 50 80 0 90 def countzeroesalist count Usage >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) : count =](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-78.jpg)
Usage >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) : count = 0 for item in alist : if item == 0 : count += 1 return count >>> countzeroes(scores) 1
![Examples names Ann Bob Alice Carol for i in rangelennames printnamesi Ann Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for i in range(len(names)): print(names[i]) Ann](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-79.jpg)
Examples >>> names ['Ann', 'Bob', 'Alice', 'Carol'] >>> for i in range(len(names)): print(names[i]) Ann Bob Alice Carol
![Another countzeroes function scores 50 80 0 90 def countzeroesalist Another countzeroes() function >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) :](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-80.jpg)
Another countzeroes() function >>> scores = [50, 80, 0, 90] >>> def countzeroes(alist) : count = 0 for index in range(len(scores)) : if scores[index] == 0 : count += 1 return count >>> countzeroes(scores) 1
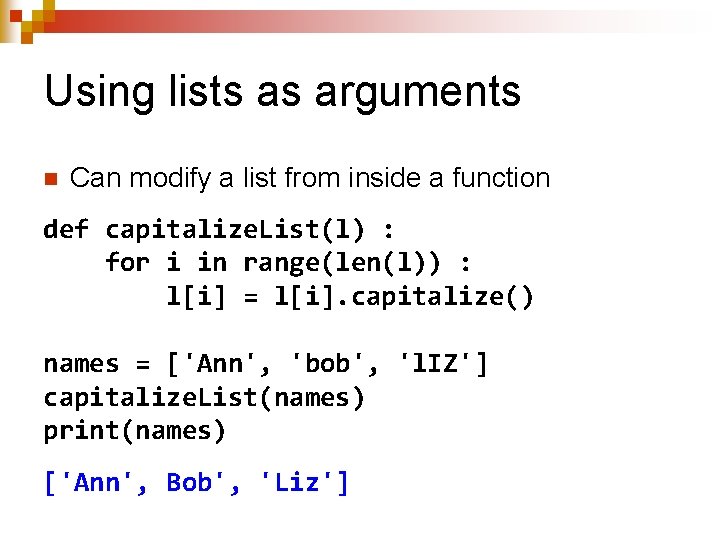
Using lists as arguments n Can modify a list from inside a function def capitalize. List(l) : for i in range(len(l)) : l[i] = l[i]. capitalize() names = ['Ann', 'bob', 'l. IZ'] capitalize. List(names) print(names) ['Ann', Bob', 'Liz']
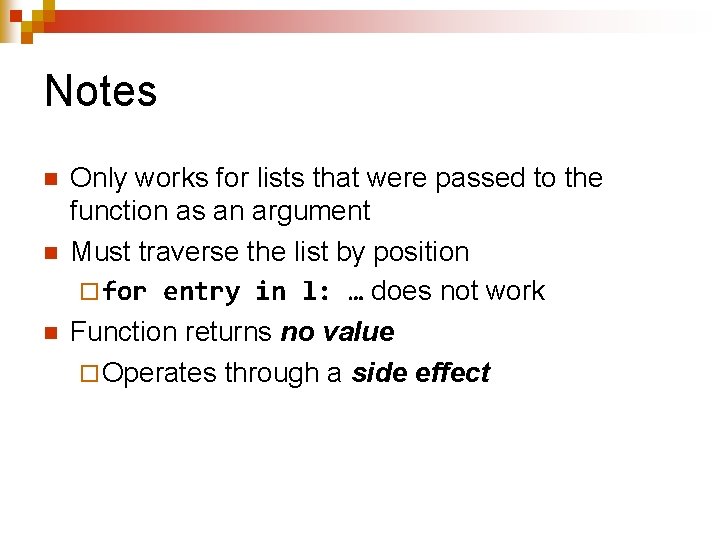
Notes n n n Only works for lists that were passed to the function as an argument Must traverse the list by position ¨ for entry in l: … does not work Function returns no value ¨ Operates through a side effect
![Functions returning a list def capitalize Listl newlist for item in Functions returning a list def capitalize. List(l) : newlist = [] for item in](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-83.jpg)
Functions returning a list def capitalize. List(l) : newlist = [] for item in l : newlist. append(item. capitalize()) return newlist names= ['ann', 'Bob', 'l. IZ'] print(capitalize. List(names)) ['Ann', Bob', 'Liz']
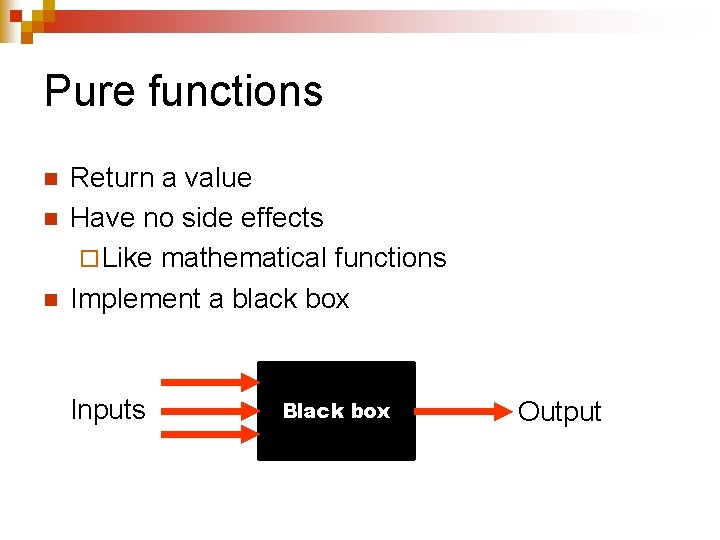
Pure functions n n n Return a value Have no side effects ¨ Like mathematical functions Implement a black box Inputs Black box Output
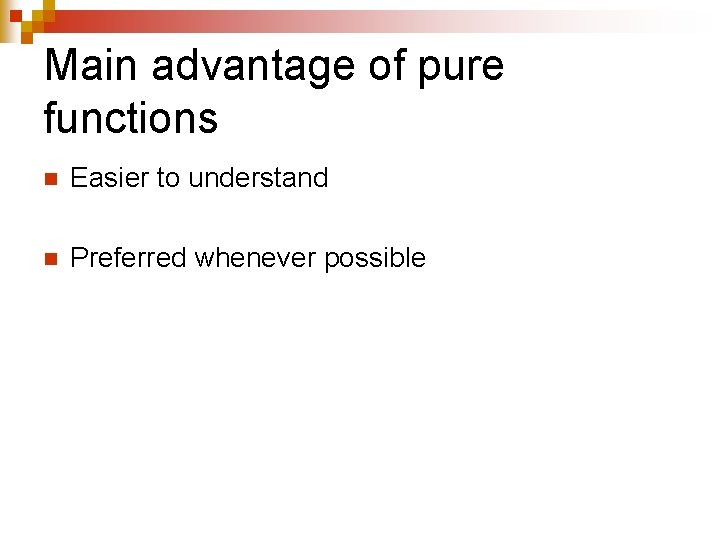
Main advantage of pure functions n Easier to understand n Preferred whenever possible
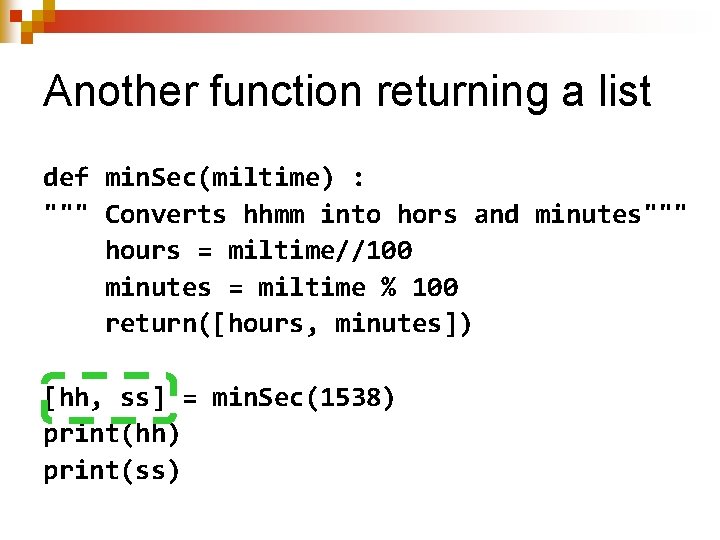
Another function returning a list def min. Sec(miltime) : """ Converts hhmm into hors and minutes""" hours = miltime//100 minutes = miltime % 100 return([hours, minutes]) [hh, ss] = min. Sec(1538) print(hh) print(ss)
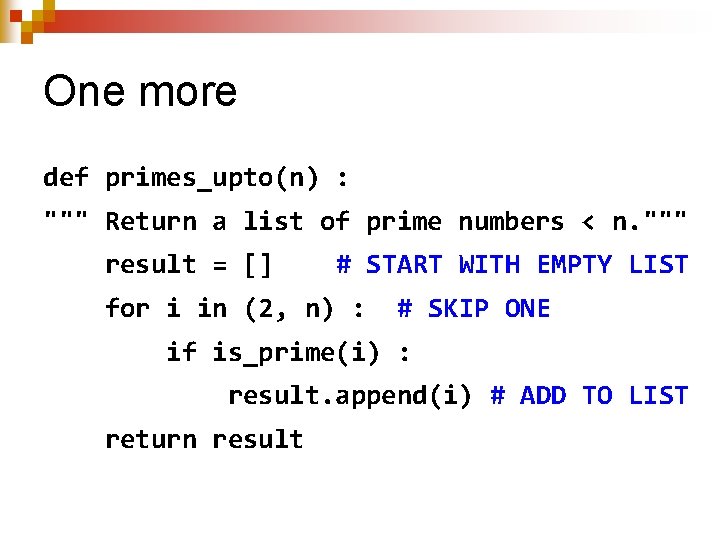
One more def primes_upto(n) : """ Return a list of prime numbers < n. """ result = [] # START WITH EMPTY LIST for i in (2, n) : # SKIP ONE if is_prime(i) : result. append(i) # ADD TO LIST return result
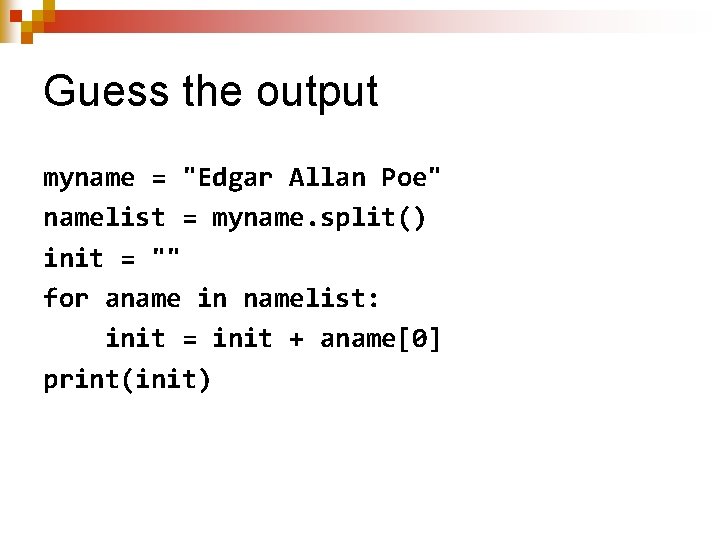
Guess the output myname = "Edgar Allan Poe" namelist = myname. split() init = "" for aname in namelist: init = init + aname[0] print(init)
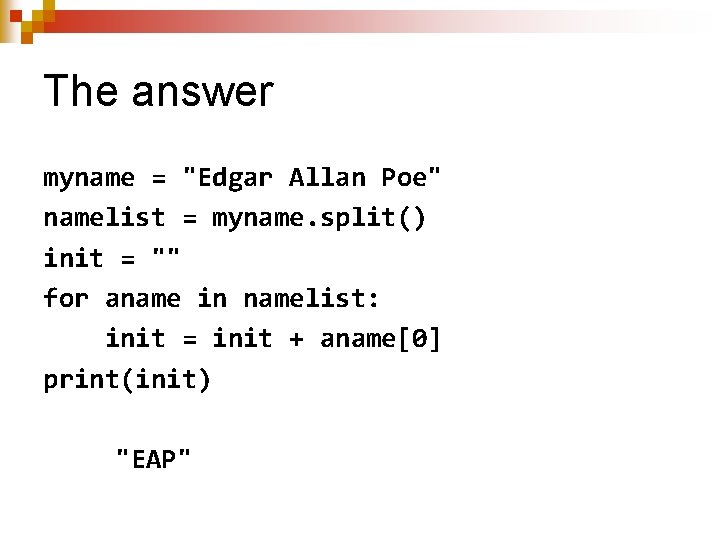
The answer myname = "Edgar Allan Poe" namelist = myname. split() init = "" for aname in namelist: init = init + aname[0] print(init) "EAP"
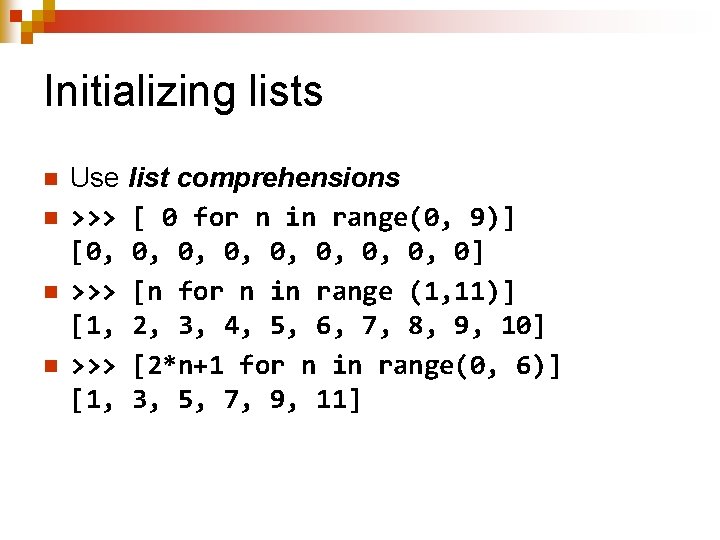
Initializing lists n n Use list comprehensions >>> [ 0 for n in range(0, 9)] [0, 0, 0] >>> [n for n in range (1, 11)] [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] >>> [2*n+1 for n in range(0, 6)] [1, 3, 5, 7, 9, 11]
![Warning n The for n clause is essential n 0 in range0 10 True Warning! n The for n clause is essential n [0 in range(0, 10)] [True]](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-91.jpg)
Warning! n The for n clause is essential n [0 in range(0, 10)] [True] ¨ Because 0 is in range(0, 10)
![More comprehensions c for c in Cougars C o u g More comprehensions >>> [ c for c in 'Cougars'] [ 'C', 'o', 'u', 'g',](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-92.jpg)
More comprehensions >>> [ c for c in 'Cougars'] [ 'C', 'o', 'u', 'g', 'a', 'r', 's'] >>> names = ['Ann', 'bob', 'liz'] >>> new = [n. capitalize() for n in names] >>> new ['Alice', 'Bob', 'Liz'] >>> names ['Alice', 'bob', 'liz']
![More comprehensions 1 first 5 n for n in range1 6 first More comprehensions >>> [1, first 5 = [n for n in range(1, 6)] first](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-93.jpg)
More comprehensions >>> [1, first 5 = [n for n in range(1, 6)] first 5 2, 3, 4, 5] first 5 squares = [n*n for n in first 5] 4, 9, 16, 25]
![An equivalence nn for n in range1 6 1 4 9 16 25 is An equivalence [n*n for n in range(1, 6)] [1, 4, 9, 16, 25] is](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-94.jpg)
An equivalence [n*n for n in range(1, 6)] [1, 4, 9, 16, 25] is same as a = [ ] for n in range(1, 6) : a. append(n*n)
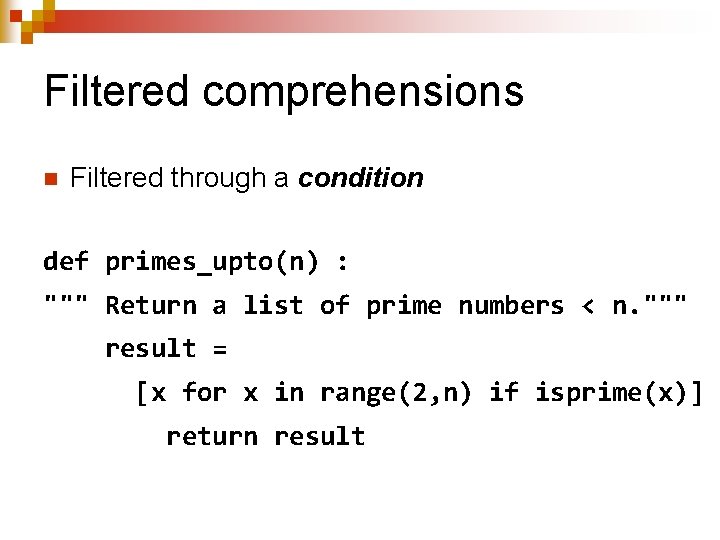
Filtered comprehensions n Filtered through a condition def primes_upto(n) : """ Return a list of prime numbers < n. """ result = [x for x in range(2, n) if isprime(x)] return result
![Filtered comprehensions a 11 22 33 44 55 b Filtered comprehensions >>> a = [11, 22, 33, 44, 55] >>> b = [](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-96.jpg)
Filtered comprehensions >>> a = [11, 22, 33, 44, 55] >>> b = [ n for n in a if n%2 == 0] >>> b [22, 44] >>> c = [n//11 for n in a if n > 20] >>> c [2, 3, 4, 5] A very powerful tool !
![More filtered comprehensions s Ann CS Bob CE Liz CS x0 More filtered comprehensions >>> s = [['Ann', 'CS'], ['Bob', 'CE'], ['Liz', 'CS']] >>> [x[0]](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-97.jpg)
More filtered comprehensions >>> s = [['Ann', 'CS'], ['Bob', 'CE'], ['Liz', 'CS']] >>> [x[0] for x in s if x[1] =='CS'] ['Ann', 'Liz'] >>> sum([1 for _ in s if _[1] =='CS']) 2
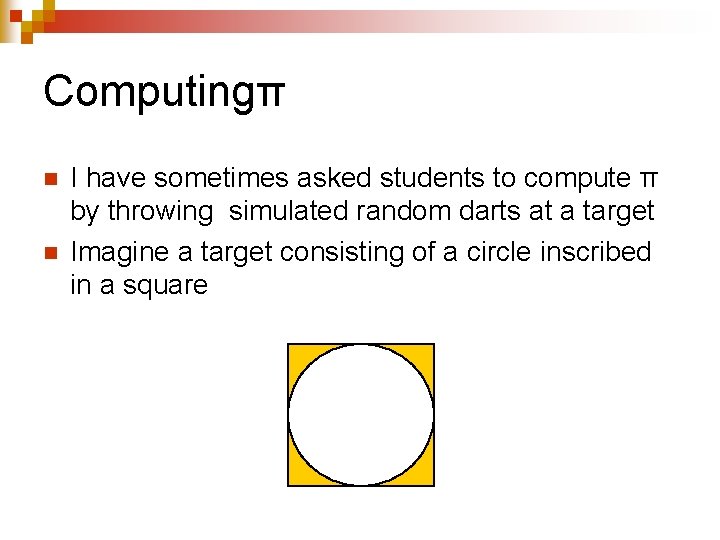
Computingπ n n I have sometimes asked students to compute π by throwing simulated random darts at a target Imagine a target consisting of a circle inscribed in a square
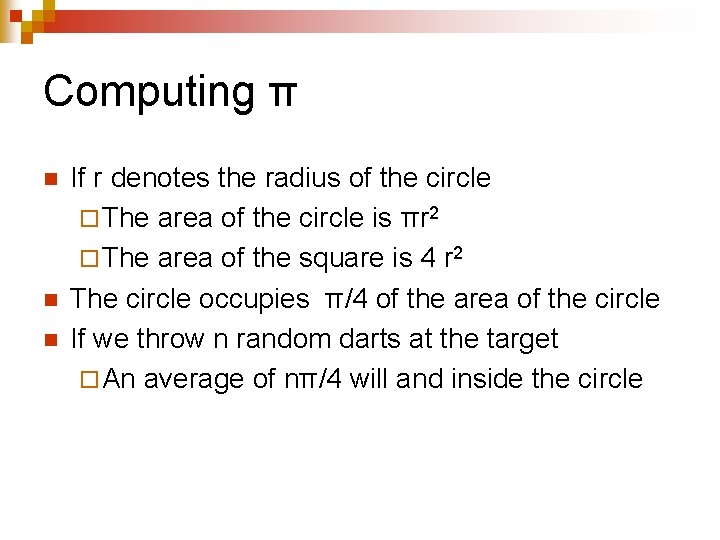
Computing π n n n If r denotes the radius of the circle ¨ The area of the circle is πr 2 ¨ The area of the square is 4 r 2 The circle occupies π/4 of the area of the circle If we throw n random darts at the target ¨ An average of nπ/4 will and inside the circle
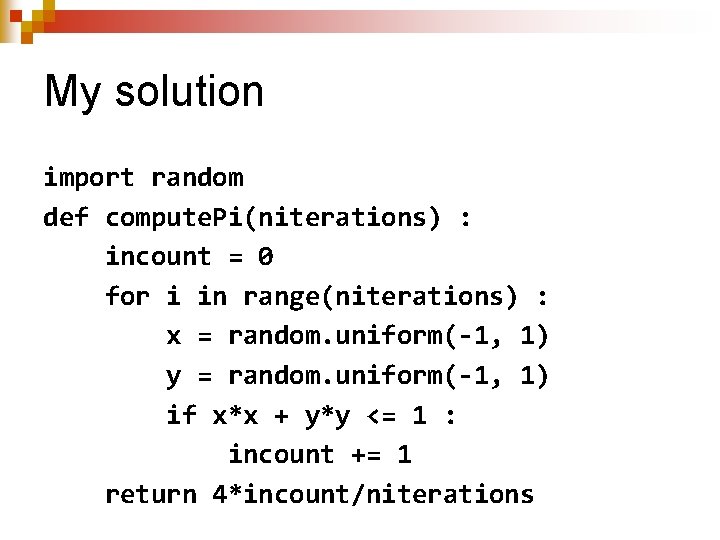
My solution import random def compute. Pi(niterations) : incount = 0 for i in range(niterations) : x = random. uniform(-1, 1) y = random. uniform(-1, 1) if x*x + y*y <= 1 : incount += 1 return 4*incount/niterations
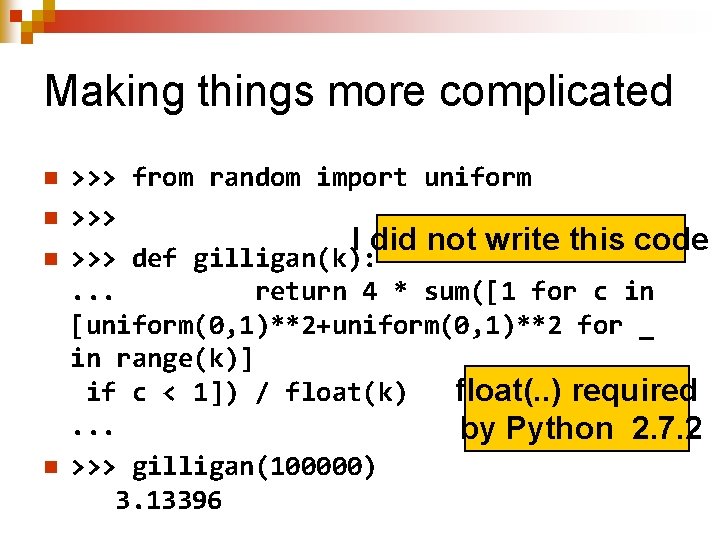
Making things more complicated n n >>> from random import uniform >>> I did not write this code >>> def gilligan(k): . . . return 4 * sum([1 for c in [uniform(0, 1)**2+uniform(0, 1)**2 for _ in range(k)] if c < 1]) / float(k) float(. . ) required. . . by Python 2. 7. 2 >>> gilligan(100000) 3. 13396
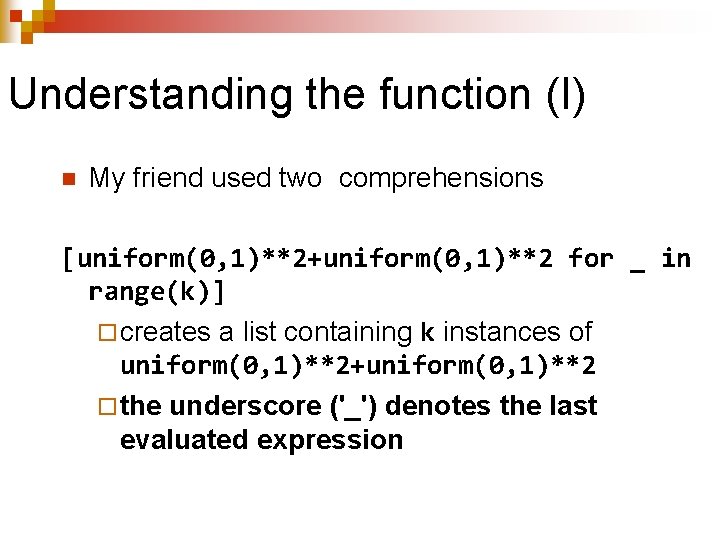
Understanding the function (I) n My friend used two comprehensions [uniform(0, 1)**2+uniform(0, 1)**2 for _ in range(k)] ¨ creates a list containing k instances of uniform(0, 1)**2+uniform(0, 1)**2 ¨ the underscore ('_') denotes the last evaluated expression
![Understanding the function II 1 for c in if c 1 Understanding the function (II) [1 for c in […] if c < 1] ¨](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-103.jpg)
Understanding the function (II) [1 for c in […] if c < 1] ¨ creates a list containing a 1 for each value in c such that c < 1 ¨ each entry of the new list represents one dart that felt into the circle
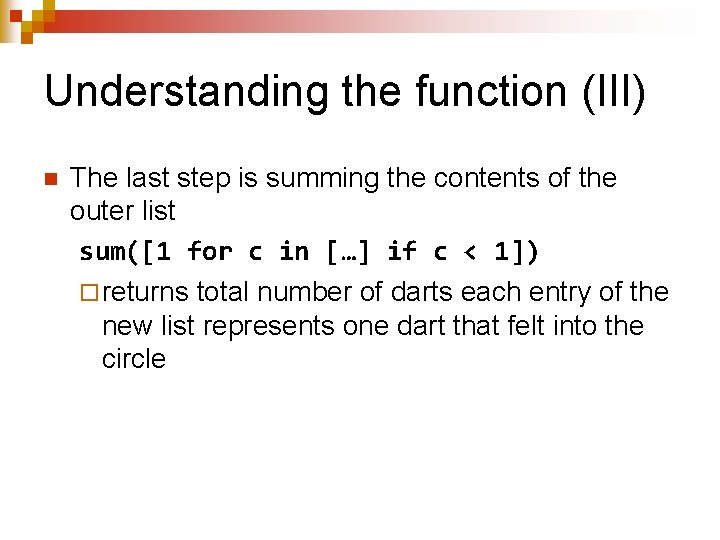
Understanding the function (III) n The last step is summing the contents of the outer list sum([1 for c in […] if c < 1]) ¨ returns total number of darts each entry of the new list represents one dart that felt into the circle
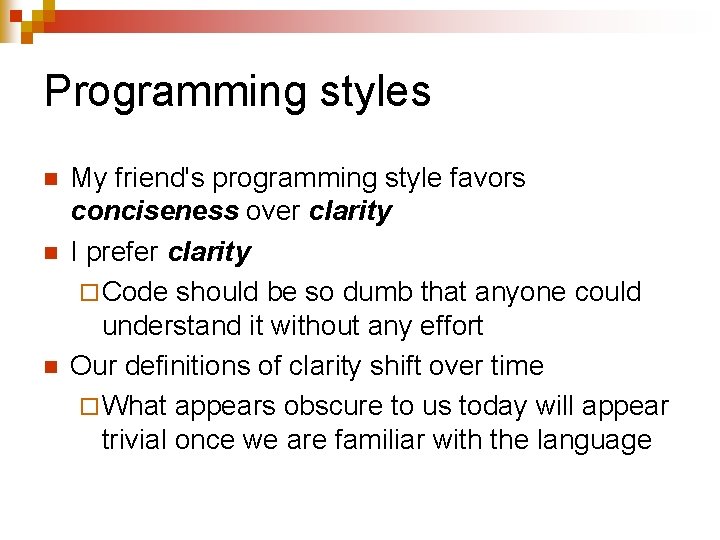
Programming styles n n n My friend's programming style favors conciseness over clarity I prefer clarity ¨ Code should be so dumb that anyone could understand it without any effort Our definitions of clarity shift over time ¨ What appears obscure to us today will appear trivial once we are familiar with the language
![Nested lists or lists of lists nested Ann 90 Bob 84 Nested lists or lists of lists >>> nested = [['Ann', 90], ['Bob', 84]] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-106.jpg)
Nested lists or lists of lists >>> nested = [['Ann', 90], ['Bob', 84]] >>> innerlist = nested[1] >>> innerlist ['Bob', 84] >>> innerlist[0] 'Bob' >>> nested[1][0] 'Bob'
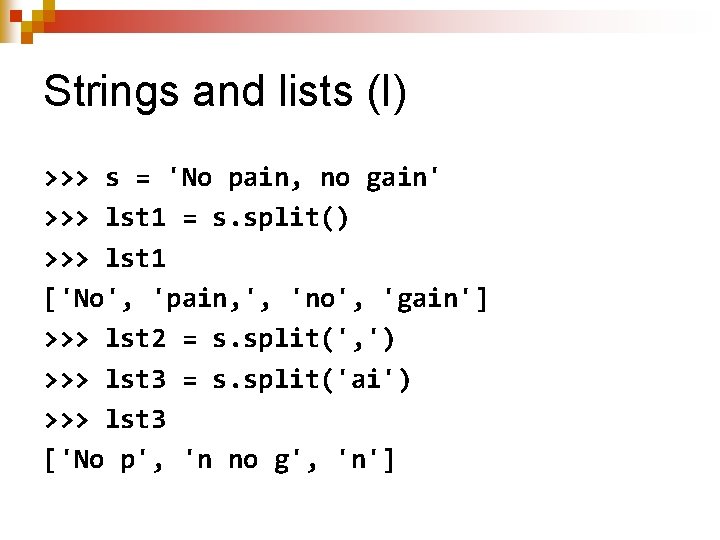
Strings and lists (I) >>> s = 'No pain, no gain' >>> lst 1 = s. split() >>> lst 1 ['No', 'pain, ', 'no', 'gain'] >>> lst 2 = s. split(', ') >>> lst 3 = s. split('ai') >>> lst 3 ['No p', 'n no g', 'n']
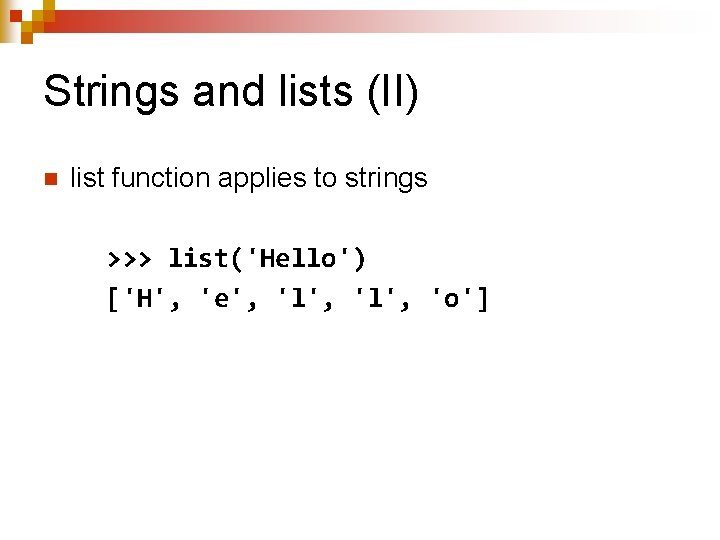
Strings and lists (II) n list function applies to strings >>> list('Hello') ['H', 'e', 'l', 'o']
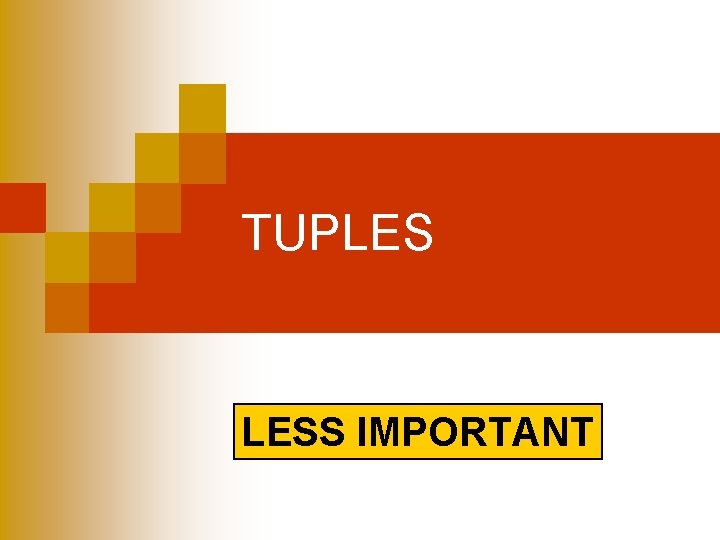
TUPLES LESS IMPORTANT
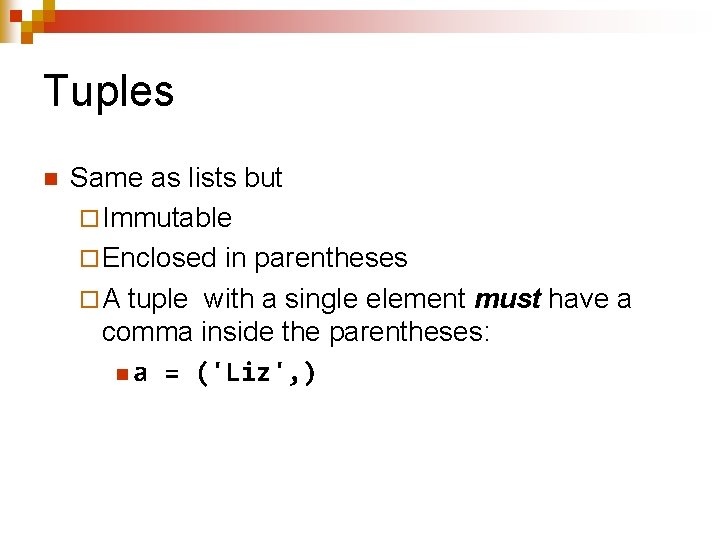
Tuples n Same as lists but ¨ Immutable ¨ Enclosed in parentheses ¨ A tuple with a single element must have a comma inside the parentheses: n a = ('Liz', )
![Examples mytuple Ann Bob 33 mytuple0 Ann mytuple1 33 Examples >>> mytuple = ('Ann', 'Bob', 33) >>> mytuple[0] 'Ann' >>> mytuple[-1] 33 >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-111.jpg)
Examples >>> mytuple = ('Ann', 'Bob', 33) >>> mytuple[0] 'Ann' >>> mytuple[-1] 33 >>> mytuple[0: 1] ('Ann', ) The comma is required!
![Why n No confusion possible between Ann and Ann n Ann is a perfectly Why? n No confusion possible between ['Ann'] and 'Ann' n ('Ann') is a perfectly](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-112.jpg)
Why? n No confusion possible between ['Ann'] and 'Ann' n ('Ann') is a perfectly acceptable expression ¨ ('Ann') without the comma is the string 'Ann' ¨ ('Ann', ) with the comma is a tuple containing the string 'Ann' n Sole dirty trick played on us by tuples!
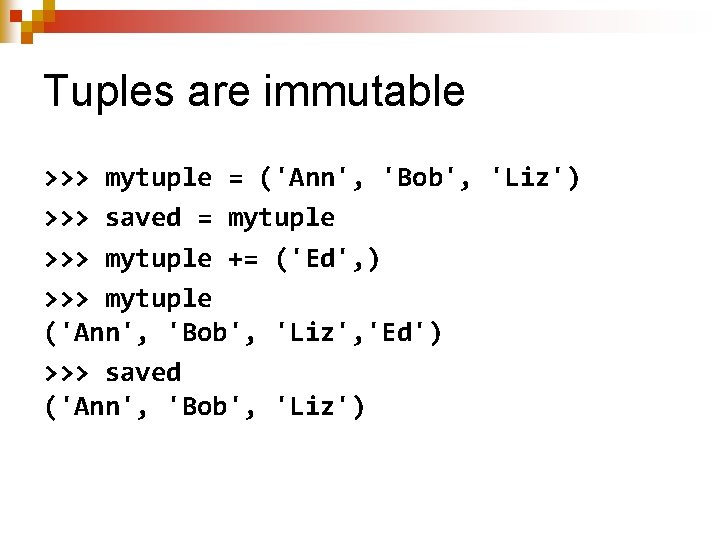
Tuples are immutable >>> mytuple = ('Ann', 'Bob', 'Liz') >>> saved = mytuple >>> mytuple += ('Ed', ) >>> mytuple ('Ann', 'Bob', 'Liz', 'Ed') >>> saved ('Ann', 'Bob', 'Liz')
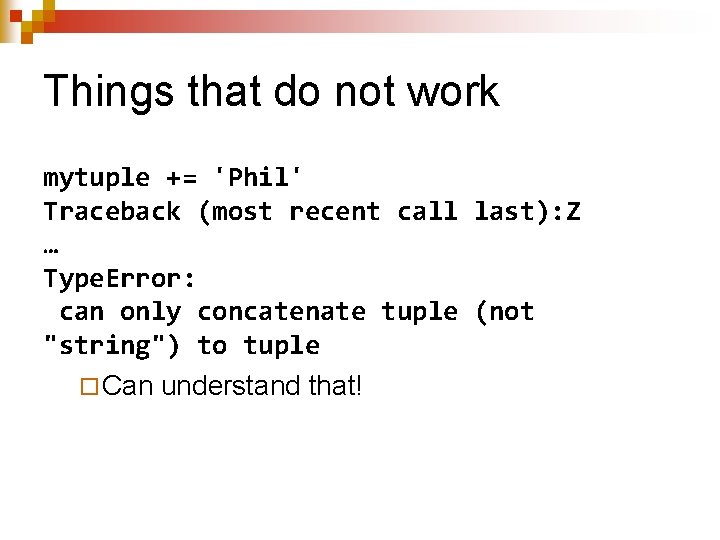
Things that do not work mytuple += 'Phil' Traceback (most recent call last): Z … Type. Error: can only concatenate tuple (not "string") to tuple ¨ Can understand that!
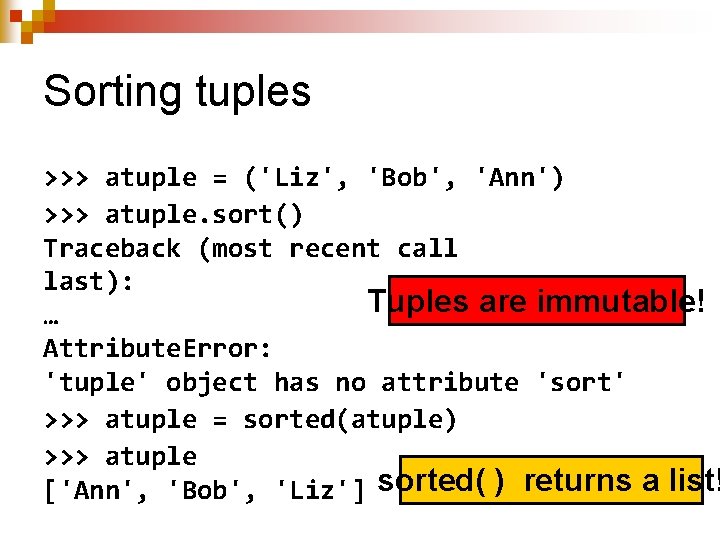
Sorting tuples >>> atuple = ('Liz', 'Bob', 'Ann') >>> atuple. sort() Traceback (most recent call last): Tuples are immutable! … Attribute. Error: 'tuple' object has no attribute 'sort' >>> atuple = sorted(atuple) >>> atuple ['Ann', 'Bob', 'Liz'] sorted( ) returns a list!
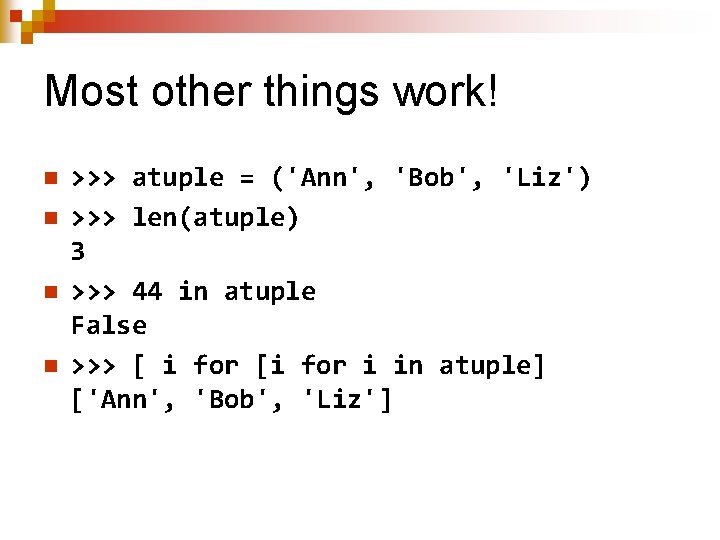
Most other things work! n n >>> atuple = ('Ann', 'Bob', 'Liz') >>> len(atuple) 3 >>> 44 in atuple False >>> [ i for [i for i in atuple] ['Ann', 'Bob', 'Liz']
![The reverse does not work alist Ann Bob Liz i for The reverse does not work >>> alist = ['Ann', 'Bob', 'Liz'] >>> (i for](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-117.jpg)
The reverse does not work >>> alist = ['Ann', 'Bob', 'Liz'] >>> (i for i in alist) <generator object <genexpr> at 0 x 02855 DA 0> ¨ Does not work!
![Converting sequences into tuples alist Ann Bob Liz atuple tuplealist Converting sequences into tuples >>> alist = ['Ann', 'Bob', 'Liz'] >>> atuple = tuple(alist)](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-118.jpg)
Converting sequences into tuples >>> alist = ['Ann', 'Bob', 'Liz'] >>> atuple = tuple(alist) >>> atuple ('Ann', 'Bob', 'Liz') >>> newtuple = tuple('Hello!') >>> newtuple ('H', 'e', 'l', 'o', '!')
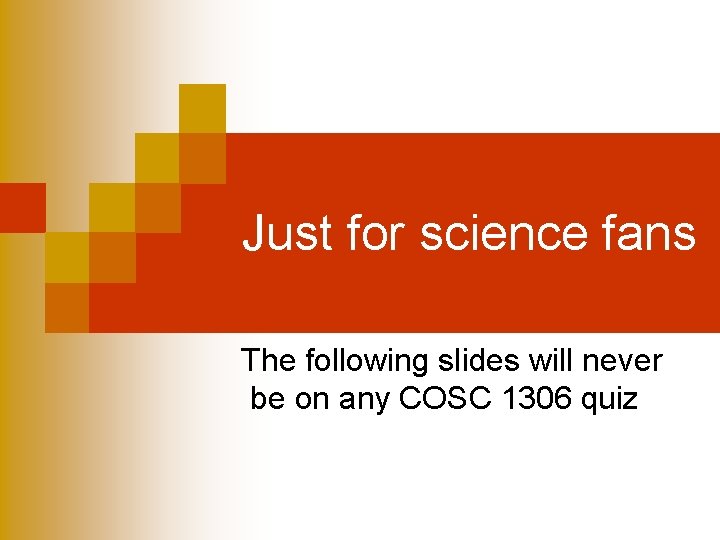
Just for science fans The following slides will never be on any COSC 1306 quiz
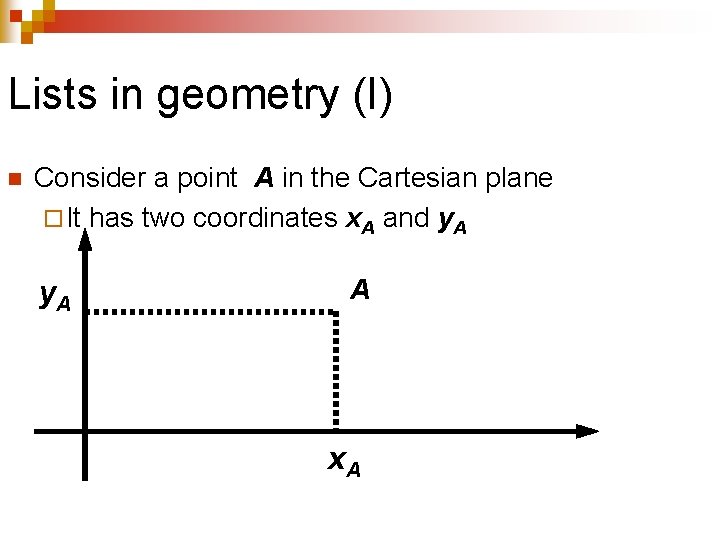
Lists in geometry (I) n Consider a point A in the Cartesian plane ¨ It has two coordinates x. A and y. A A x. A
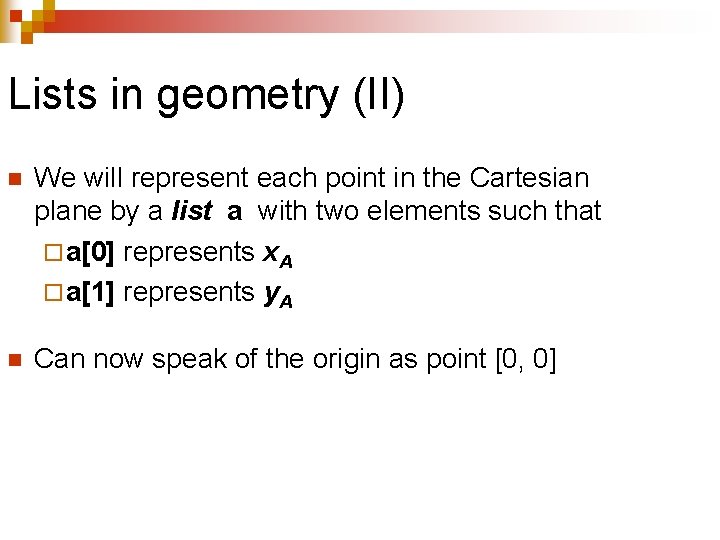
Lists in geometry (II) n We will represent each point in the Cartesian plane by a list a with two elements such that ¨ a[0] represents x. A ¨ a[1] represents y. A n Can now speak of the origin as point [0, 0]
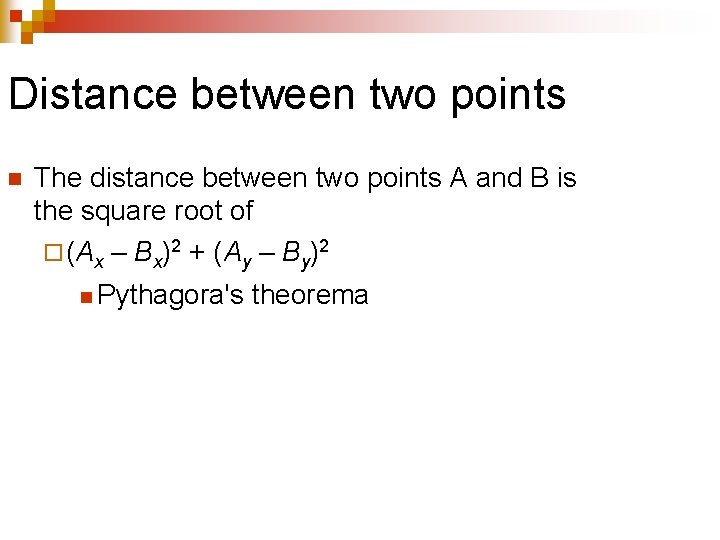
Distance between two points n The distance between two points A and B is the square root of ¨ (Ax – Bx)2 + (Ay – By)2 n Pythagora's theorema
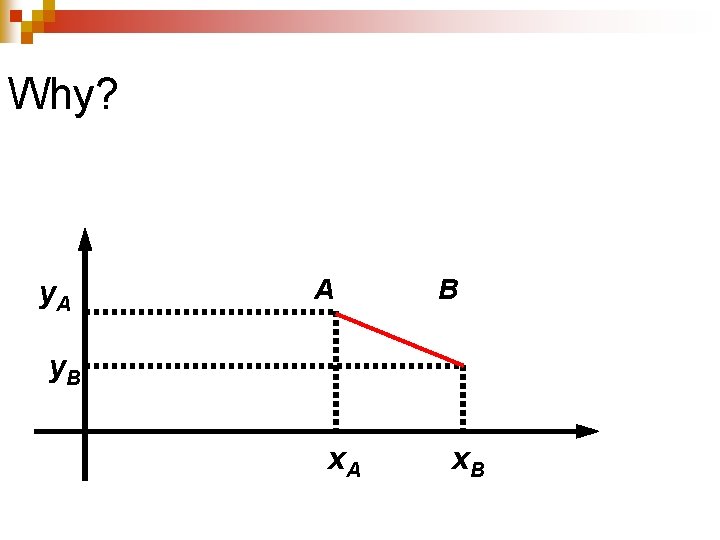
Why? y. A A B y. B x. A x. B
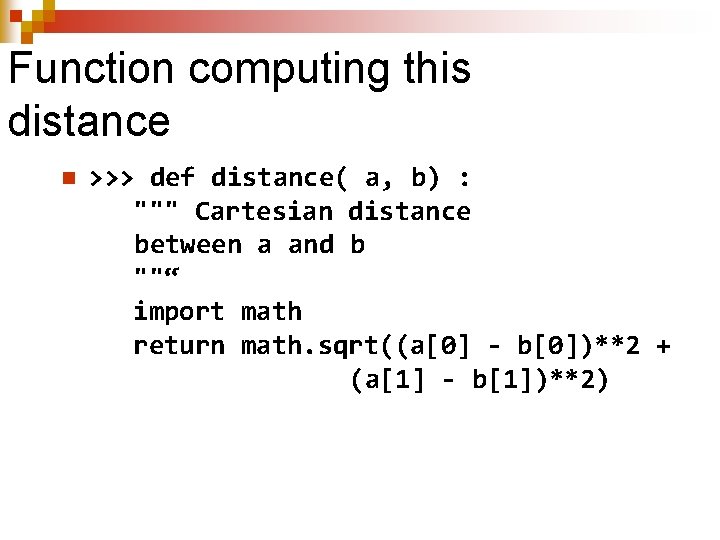
Function computing this distance n >>> def distance( a, b) : """ Cartesian distance between a and b ""“ import math return math. sqrt((a[0] - b[0])**2 + (a[1] - b[1])**2)
![Function computing this distance n n origin 0 0 a Function computing this distance n n >>> origin = [0, 0] >>> a =](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-125.jpg)
Function computing this distance n n >>> origin = [0, 0] >>> a = [1, 1] >>> distance(origin, a) 1. 4142135623730951 >>>
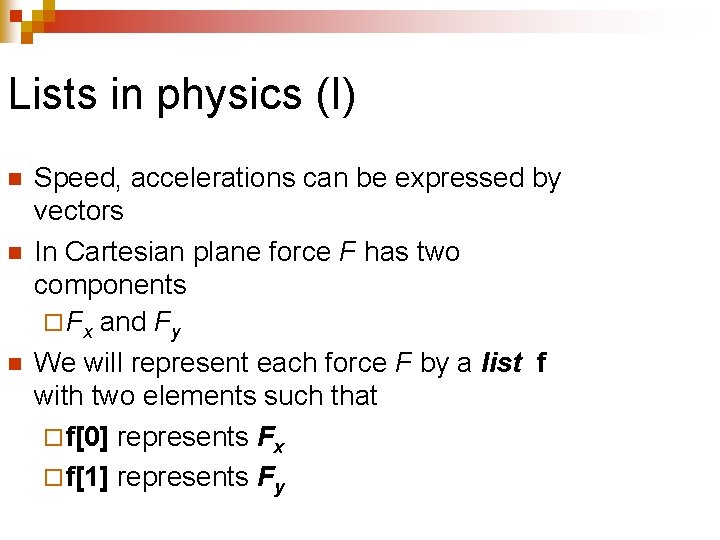
Lists in physics (I) n n n Speed, accelerations can be expressed by vectors In Cartesian plane force F has two components ¨ Fx and Fy We will represent each force F by a list f with two elements such that ¨ f[0] represents Fx ¨ f[1] represents Fy
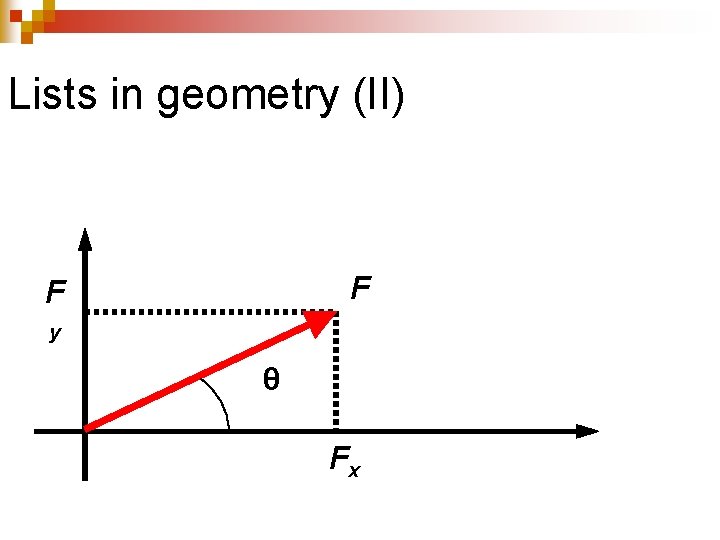
Lists in geometry (II) F F y θ Fx
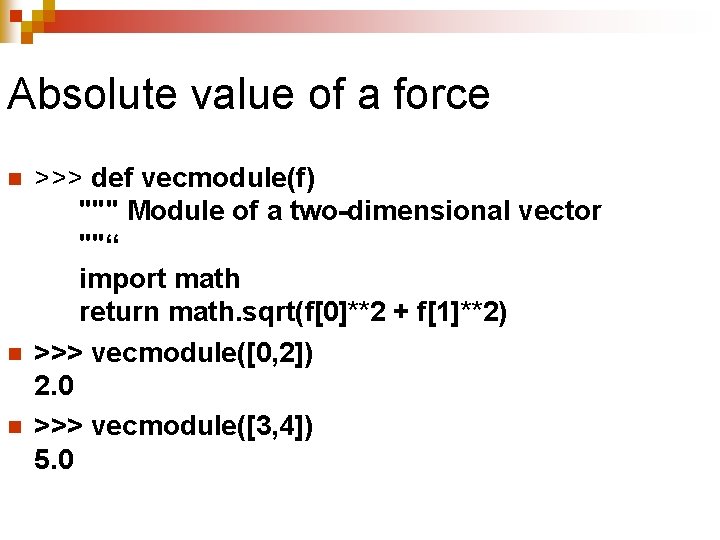
Absolute value of a force n n n >>> def vecmodule(f) """ Module of a two-dimensional vector ""“ import math return math. sqrt(f[0]**2 + f[1]**2) >>> vecmodule([0, 2]) 2. 0 >>> vecmodule([3, 4]) 5. 0
![Adding two vectors I n 0 2 0 3 0 2 0 3 Adding two vectors (I) n [0, 2] + [0, 3] [0, 2, 0, 3]](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-129.jpg)
Adding two vectors (I) n [0, 2] + [0, 3] [0, 2, 0, 3] ¨ Not what we want ¨ + operator concatenates lists
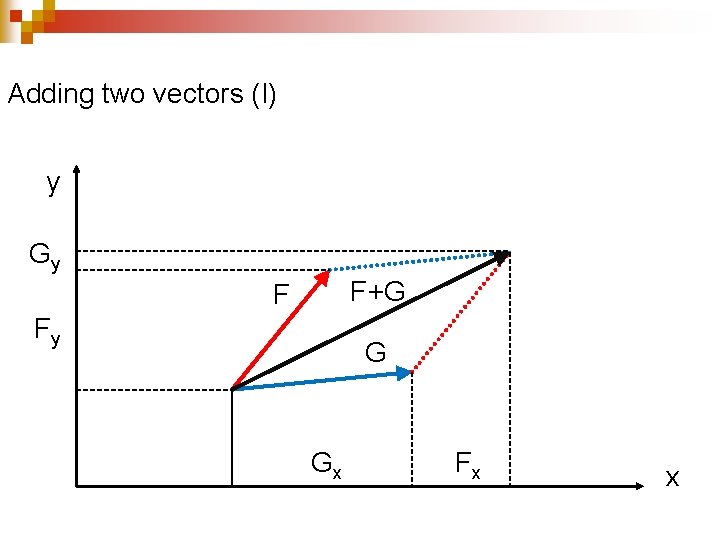
Adding two vectors (I) y Gy F+G F Fy G Gx Fx x
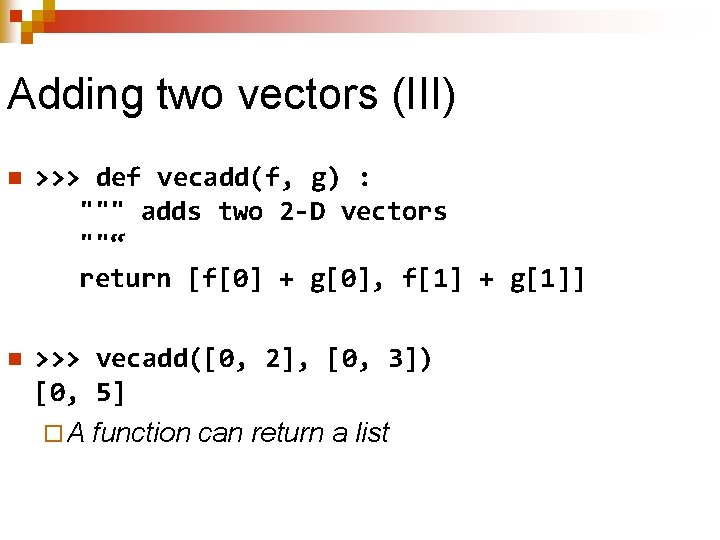
Adding two vectors (III) n >>> def vecadd(f, g) : """ adds two 2 -D vectors ""“ return [f[0] + g[0], f[1] + g[1]] n >>> vecadd([0, 2], [0, 3]) [0, 5] ¨ A function can return a list
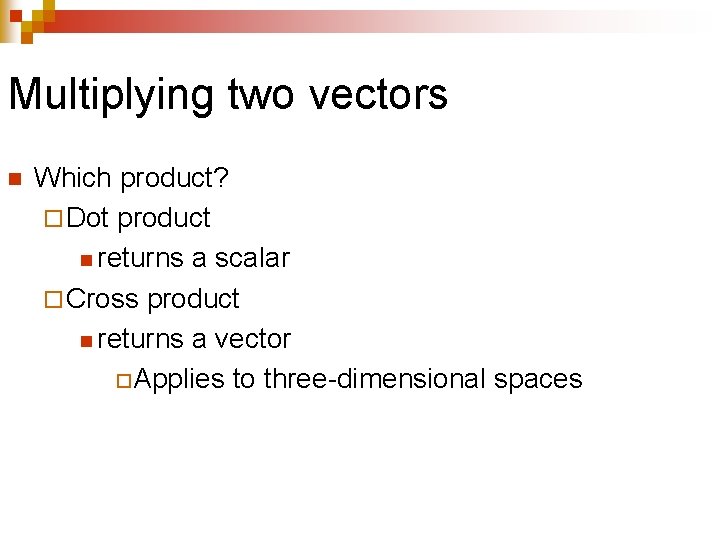
Multiplying two vectors n Which product? ¨ Dot product n returns a scalar ¨ Cross product n returns a vector ¨Applies to three-dimensional spaces
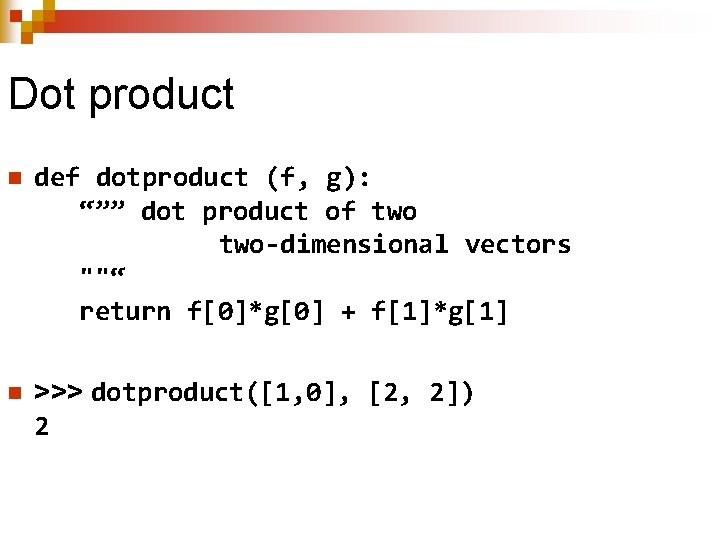
Dot product n def dotproduct (f, g): “”” dot product of two-dimensional vectors ""“ return f[0]*g[0] + f[1]*g[1] n >>> dotproduct([1, 0], [2, 2]) 2
![List of lists I n n a 1 2 3 1 List of lists (I) n n >>> a = [[1, 2], [3, 1]] >>>](https://slidetodoc.com/presentation_image_h2/4c228184b17bf1798c3ac63675153f98/image-134.jpg)
List of lists (I) n n >>> a = [[1, 2], [3, 1]] >>> a[0] [1, 2] >>> a[1][1] 1
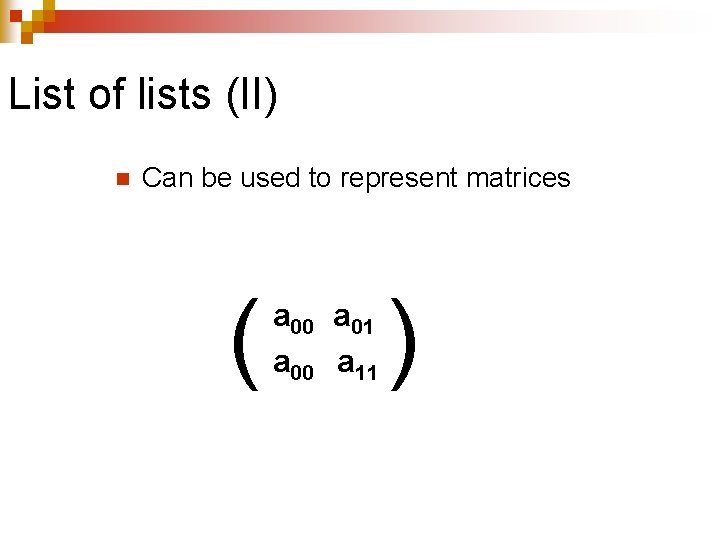
List of lists (II) n Can be used to represent matrices ( a 00 a 01 a 00 a 11 )
Cosc 1306
Cosc 1306
Cosc 1306
Ssd 1306
Music 1306
Uil computer science programming problems
Python programming an introduction to computer science
Eric’s favourite .......... is science. *
Cosc 4p61
Cosc 4p42
Cosc 3p91
Cosc 4368
Cosc 4p41
Cosc 4p41
Cosc
Cosc 3340
Cosc 320
Cosc parameters
Cosc 2p12
Cosc 4p41
Cosc 3340
8088 pinout
Cosc 121
Cosc 1p02
Cosc 2p12
Cosc 3p92
Cosc 3p92
Cosc 121
Cosc 121
Lc3 traps
Cosc 121
Cosc -cos d
Cosc 3p94
Cosc 101
Introduction to the theory of computation
Cosc 3340
Albuminkorrigerat calcium
Propofol.infusion syndrome
Sio psykolog pris
Hellefisk pris pr kg
Levetid undertak
Salgsmerkost
Pristeori
Siemens synco pris
Ipma certificering
Vektor kalkulus
Biofyringsolje pris pr liter
Målsatt pris
Ibs-attest
Kartoffelpulp pris
Udnyttelse af loftsrum
Specialforsikring
Gpu pris
Videncenter for energibesparelser i bygninger
Varemerkeregistrering pris
Slidetodoc
Ferdiggarasjer pris
Technicolor tg234 pris
Målsatt pris
Varemerkeregistrering pris
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
System programming definition
Linear vs integer programming
Definisi linear
Concepts techniques and models of computer programming
Linear programming models graphical and computer methods
What is linear programming in management science
Zj-cj simplex method
Nocti computer programming
What is nano programming
Language
Types of variables in computer programming
Programming raster display system in computer graphics
Computerite
Computer programming chapter 1
Tab sequential format
Discrete mathematics with applications susanna s. epp
Computer programming chapter 1
History of python
Computer programming with matlab
Decision making in computer programming
Computer organization
Fundamentals of computer programming syllabus
Anthropology vs sociology
Sciencefusion think central
Hard and soft science
Computer science input and output
Difference between ba and bs in computer science
Ucf college of engineering and computer science
Erik jonsson school of engineering and computer science
Computer science and engineering unr
Eecs ucla
-h#ps://microbit.org/fr/
Erik jonsson school of engineering and computer science
Erik jonsson school of engineering and computer science
Objectives of computer
What is computer organization
Input devices
Diff between computer architecture and organization
Natural vs social science
Branches of science mind map
Natural and physical science
Applied science vs pure science
Tragedy of the commons
Windcube
Iamis structures
University of phoenix computer science
How many fields in computer science
Procedural abstraction
Unsolved computer problems
University of bridgeport computer science
University of bridgeport computer science
Sequencing apcsp
Ucl computers
Ucl computer science interview
Casting computer science
Only one student failed in mathematics fol
Computer science illuminated (doc or html) file
Konsep dasar logika himpunan
Computer science yonsei university
Sat in computer science
Ib computer science topic 6
Data representation computer science
Apcs recursion
Recurrence computer science
Push down
Anticipating misuse computer science
Ocr a level computer science paper 1
Northwestern computer science department
Parse computer science
Undecidable problems in computer science
Otterbein computer science
What is iteration in computer science
How do you spell in
Polymorphism computer science