COSC 1306 COMPUTER SCIENCE AND PROGRAMMING JehanFranois Pris
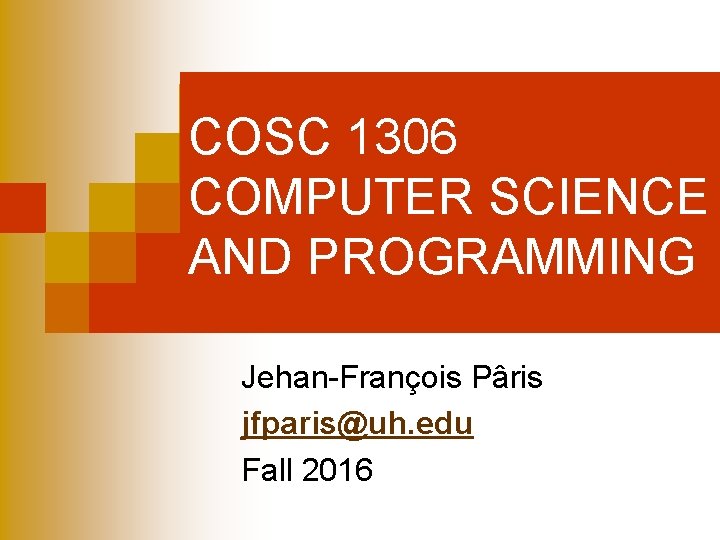
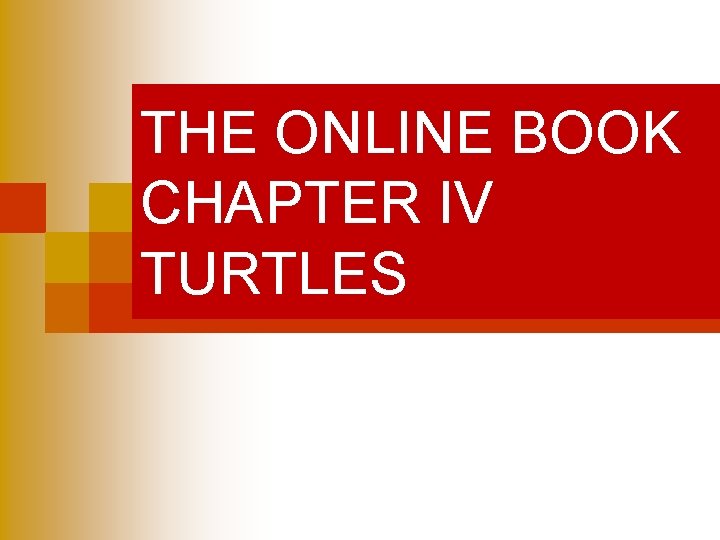
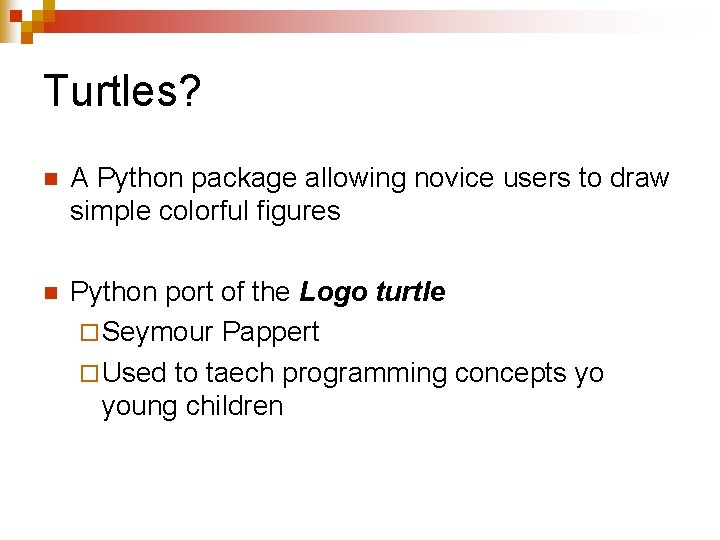
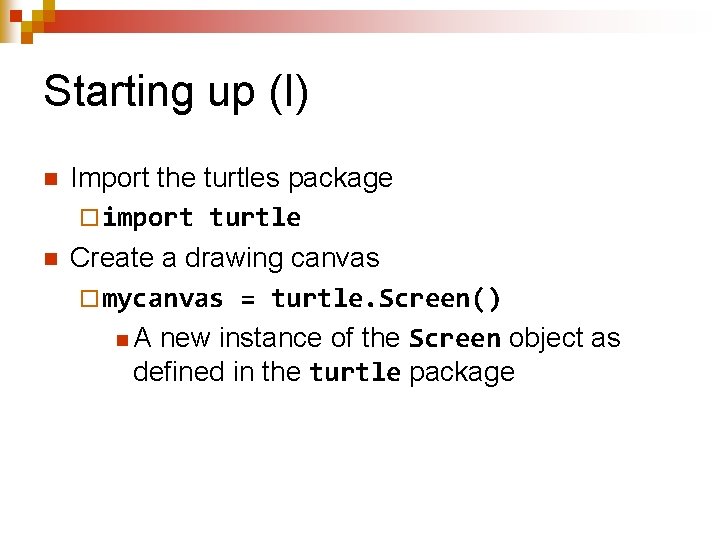
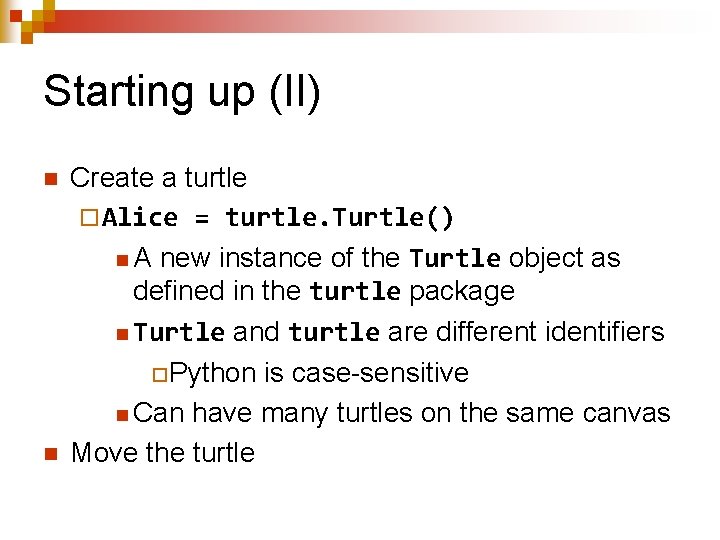
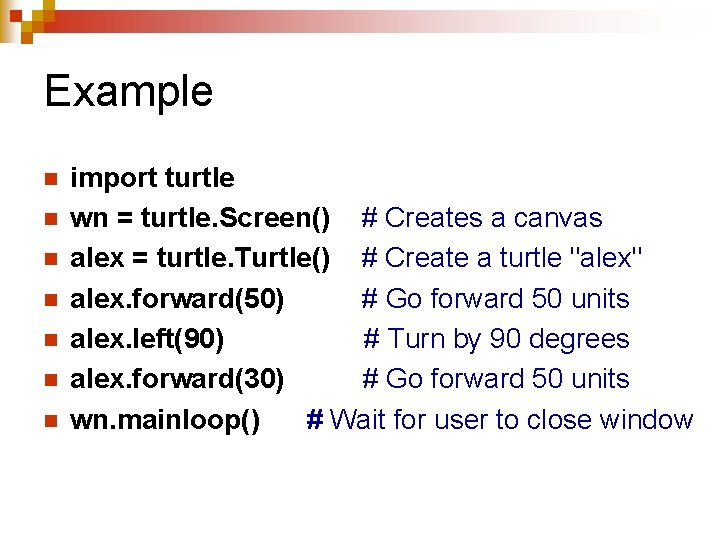
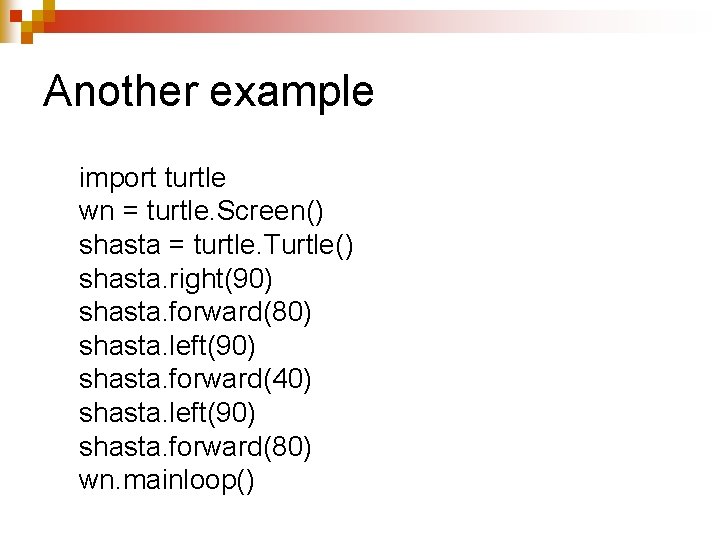
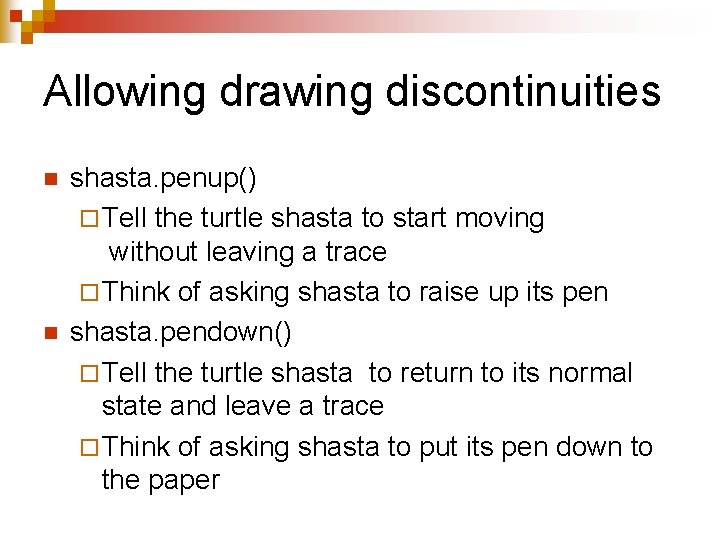
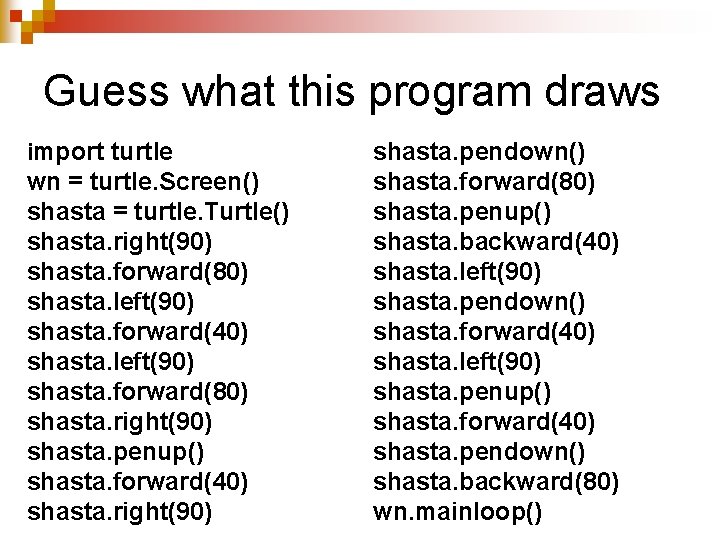
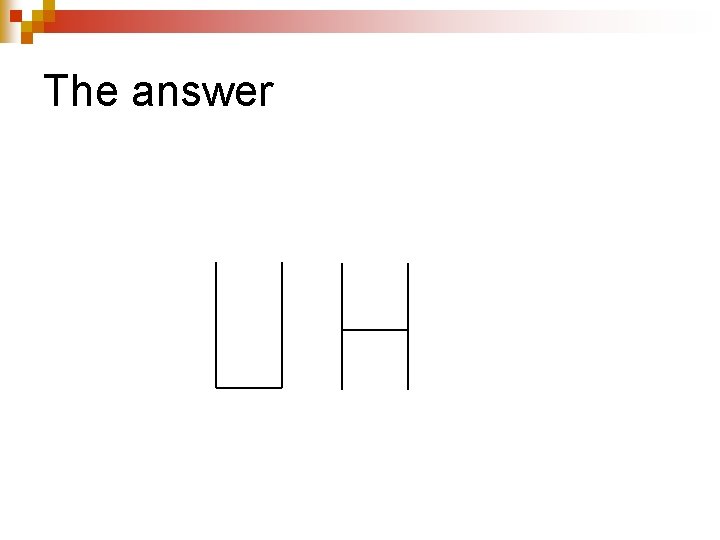
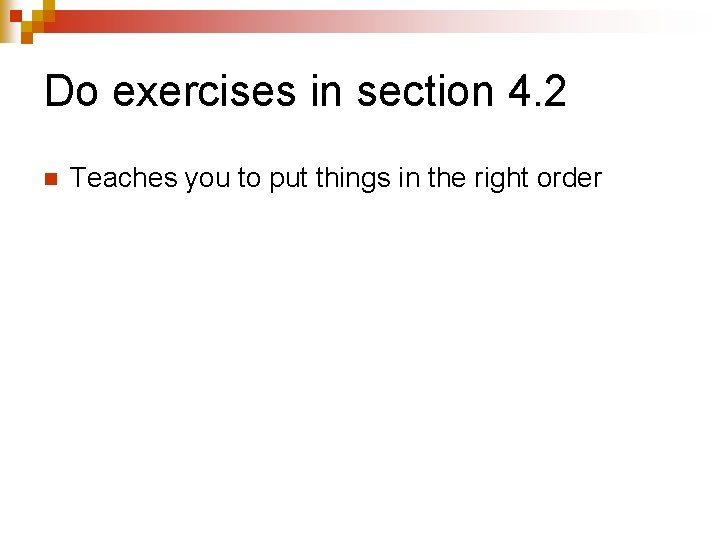
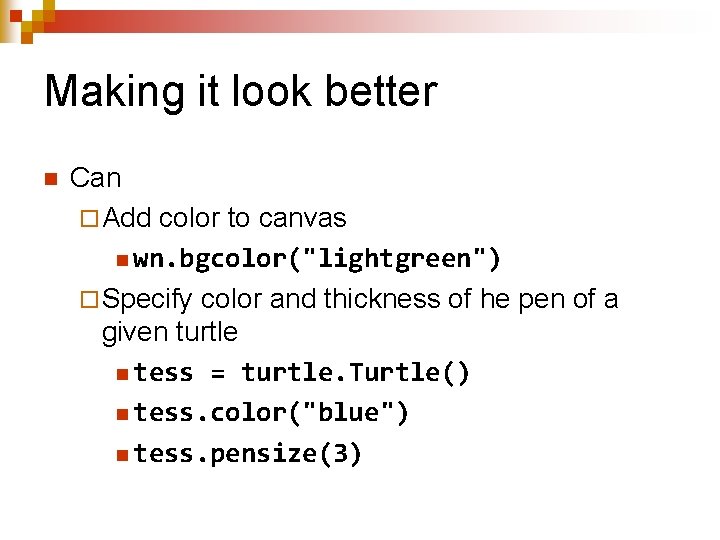
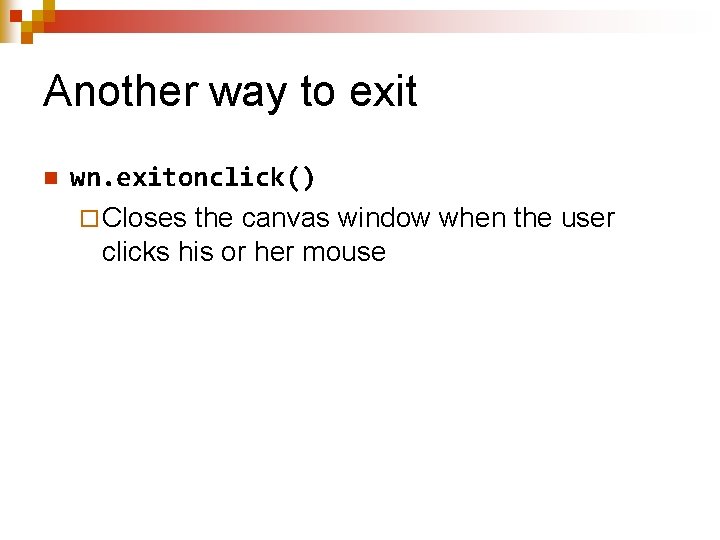
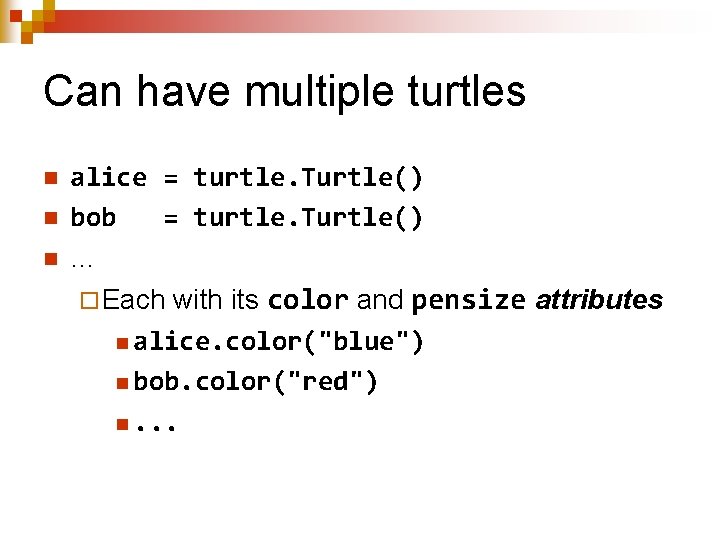
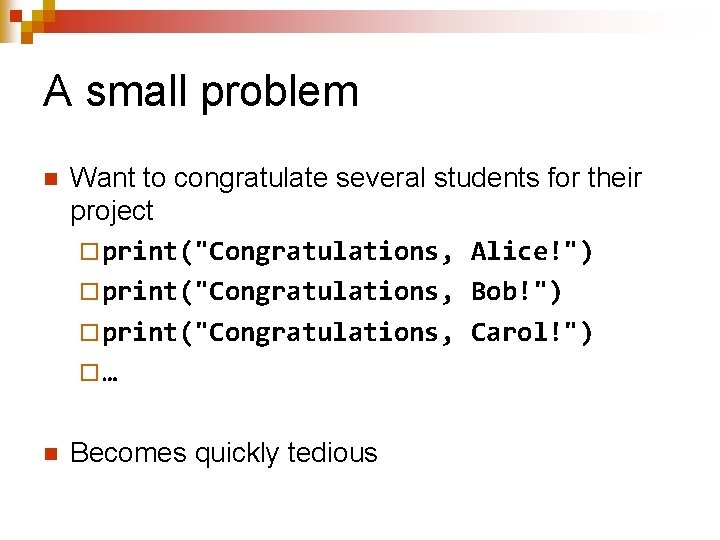
![The solution n The for loop for x in ["Alice", "Bob", "Carol"] : print("Congratulations, The solution n The for loop for x in ["Alice", "Bob", "Carol"] : print("Congratulations,](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-16.jpg)
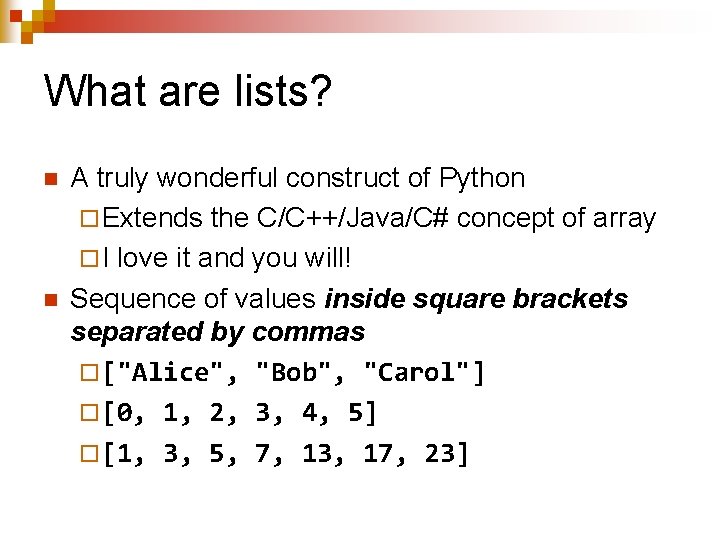
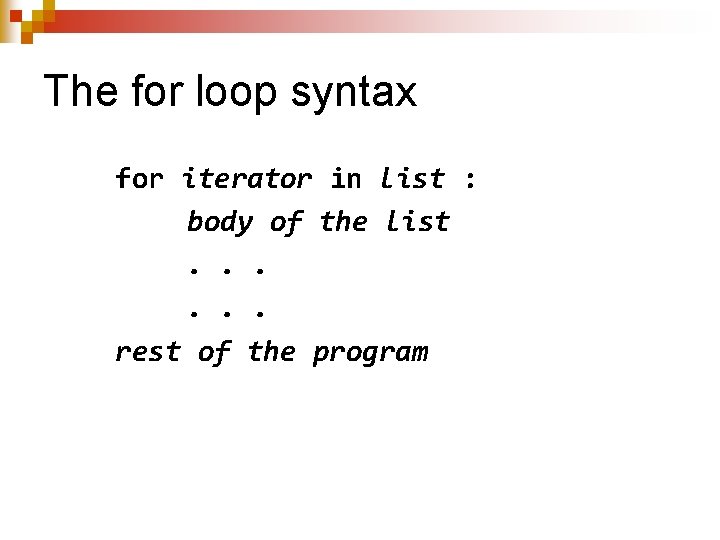
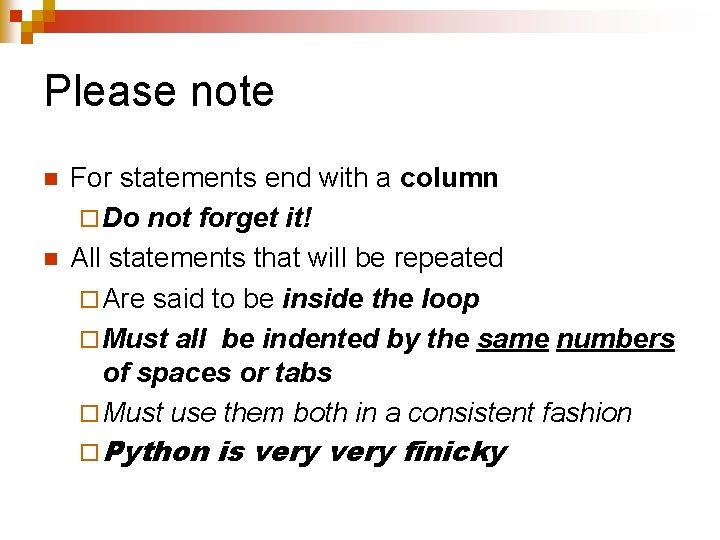
![Example (I) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!") Example (I) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!")](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-20.jpg)
![Example (II) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!") Example (II) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!")](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-21.jpg)
![Example (III) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!") Example (III) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!")](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-22.jpg)
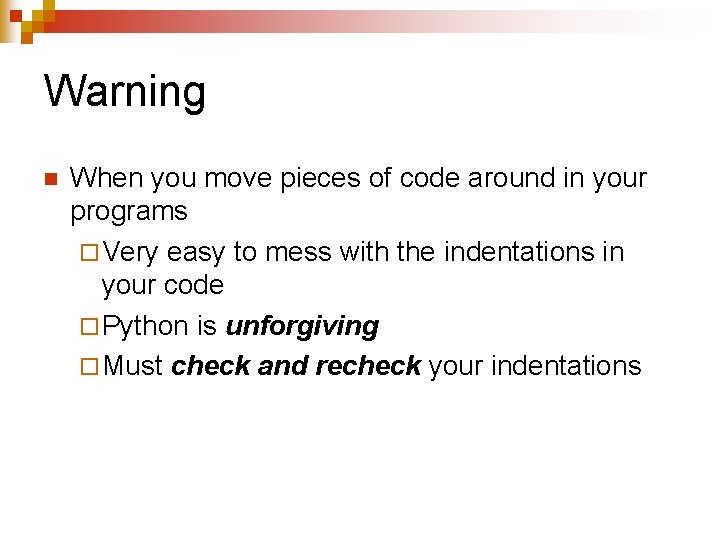
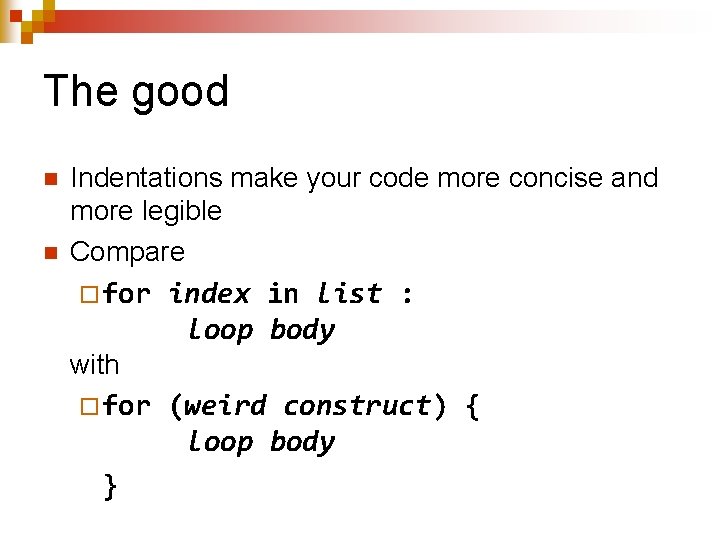
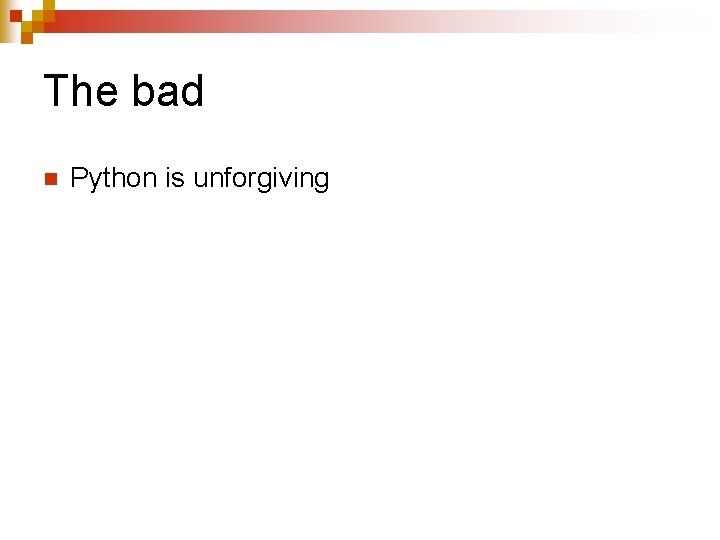
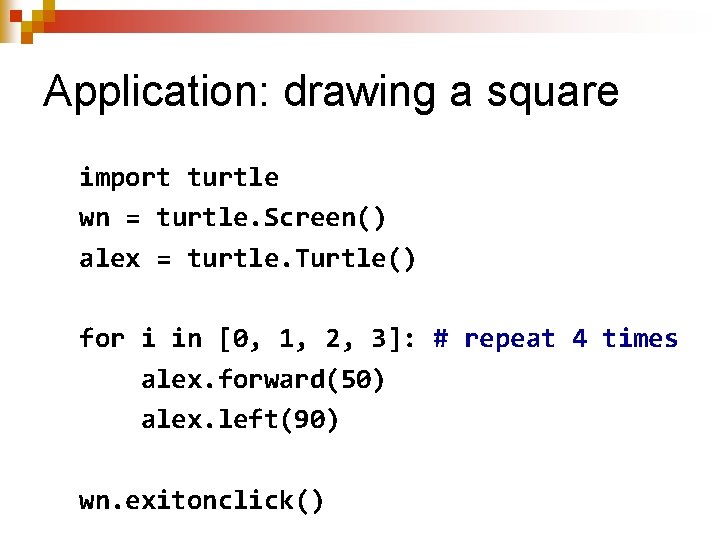
![Comments n We could have used ¨ for i in [1, 2, 3, 4]: Comments n We could have used ¨ for i in [1, 2, 3, 4]:](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-27.jpg)
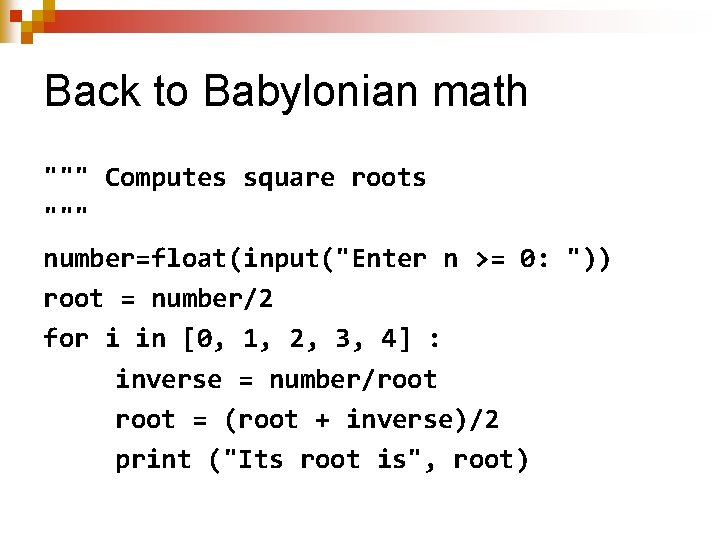
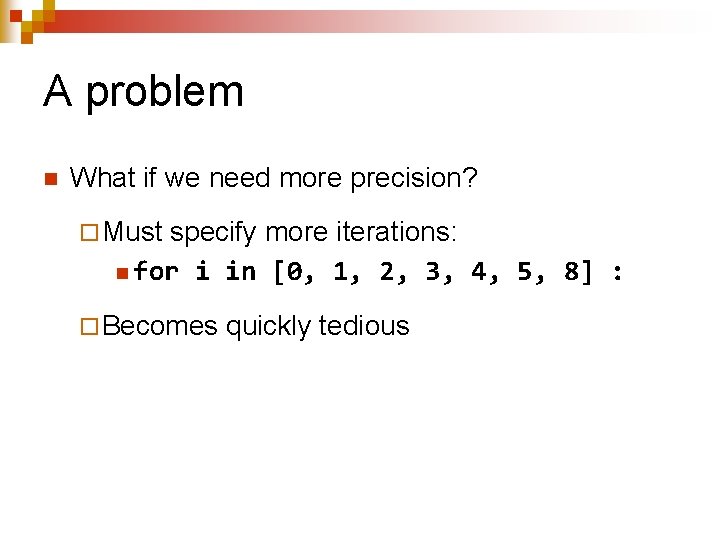
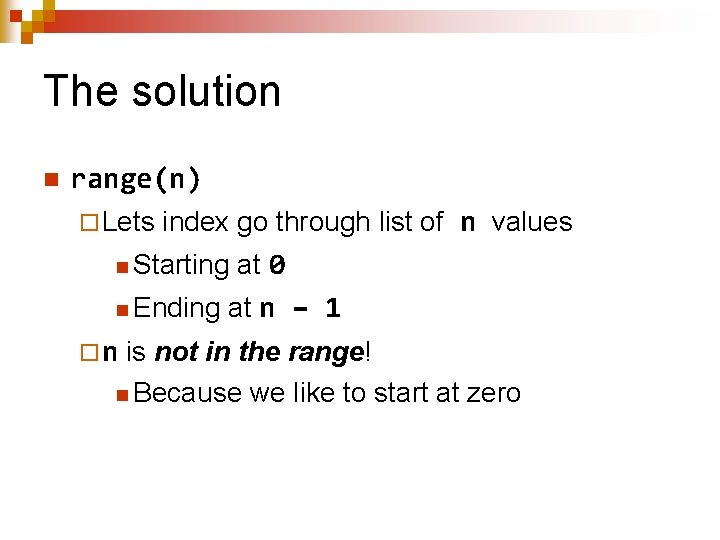
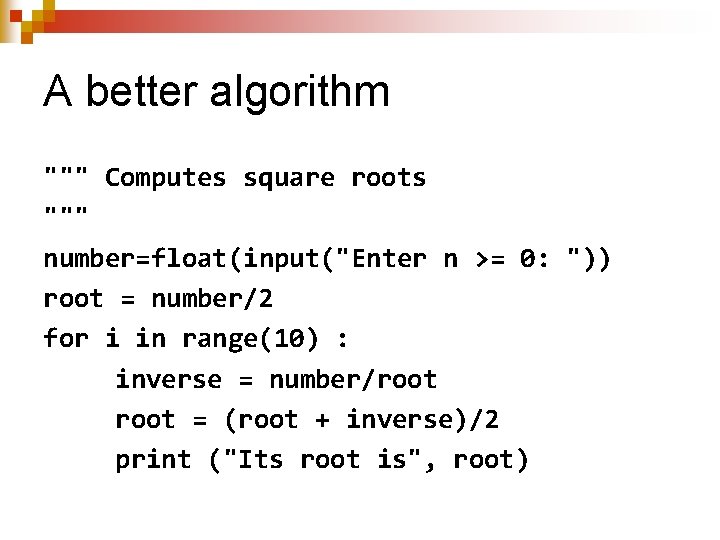
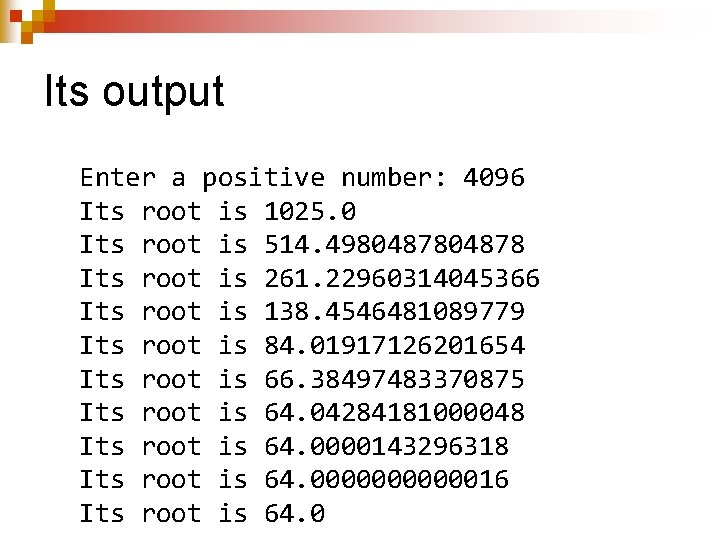
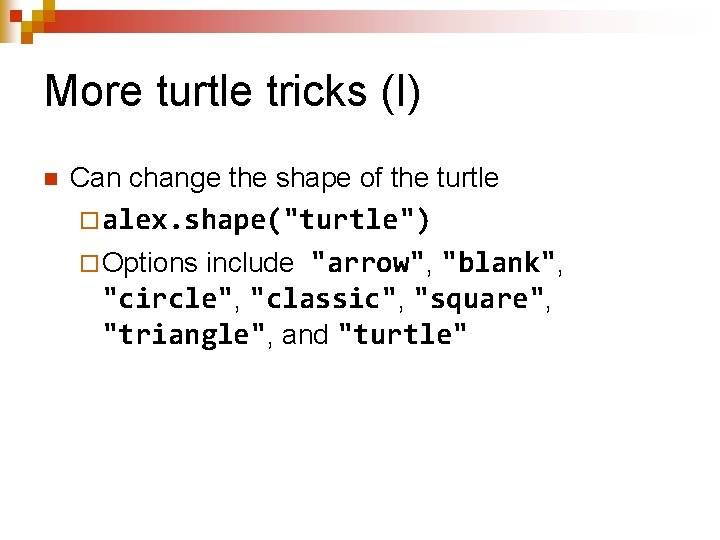
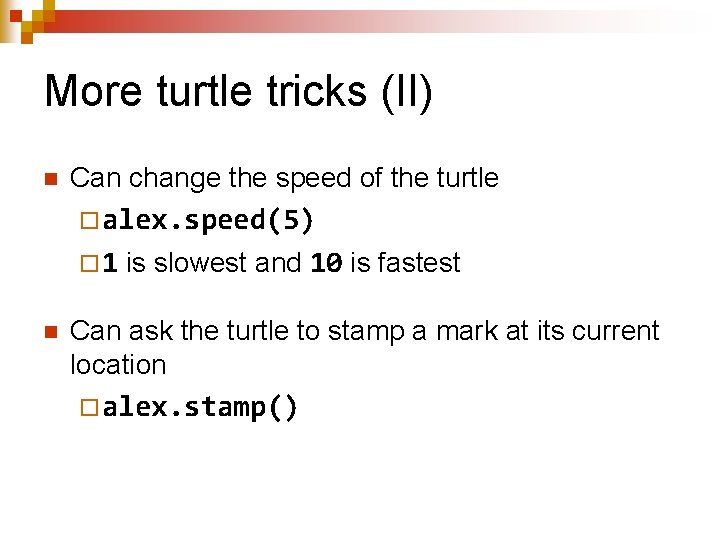
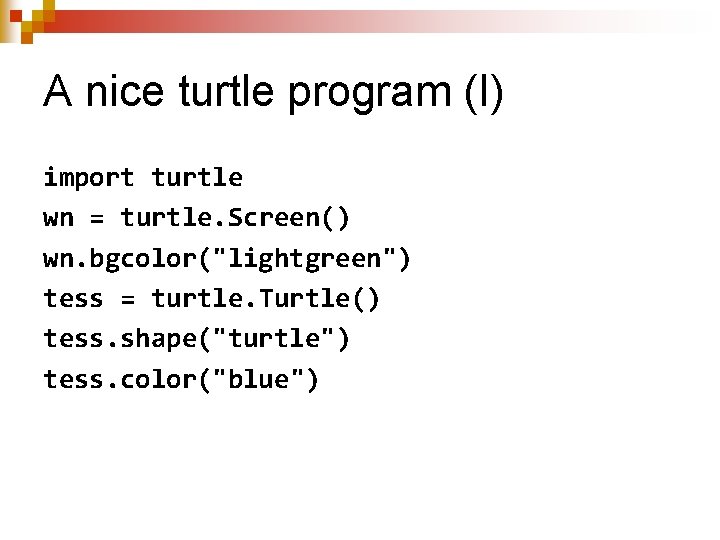
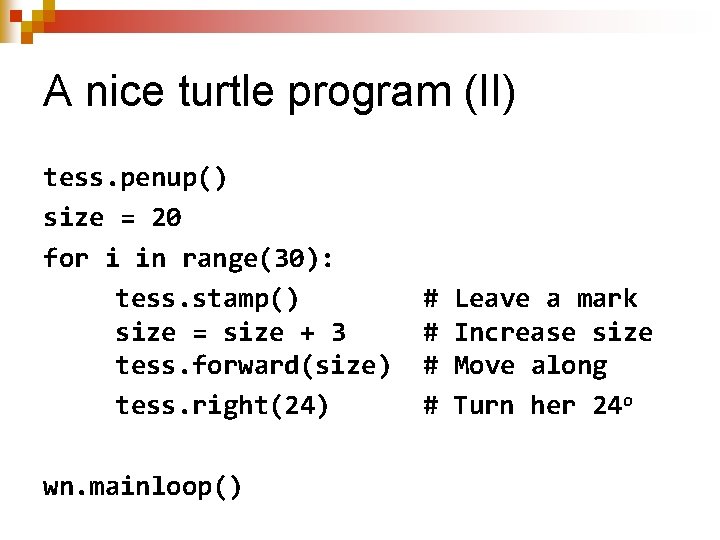
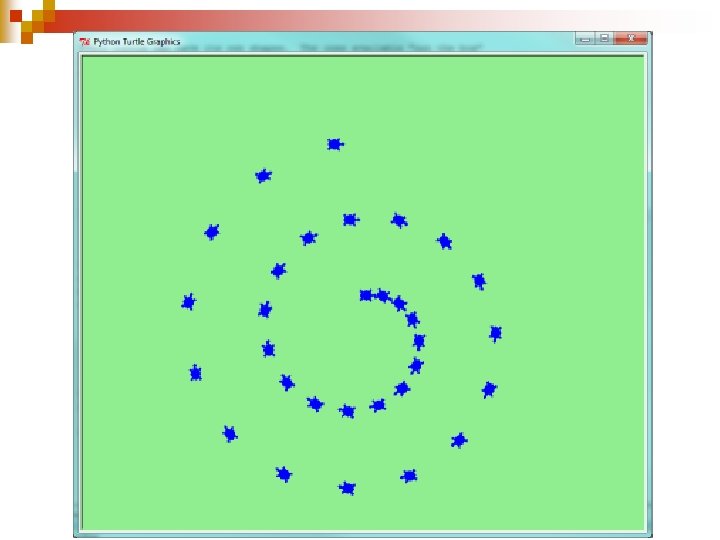
- Slides: 37
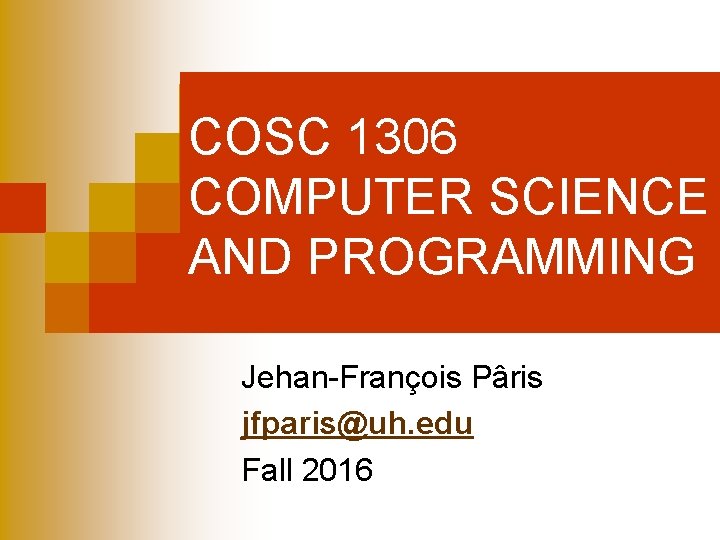
COSC 1306 COMPUTER SCIENCE AND PROGRAMMING Jehan-François Pâris jfparis@uh. edu Fall 2016
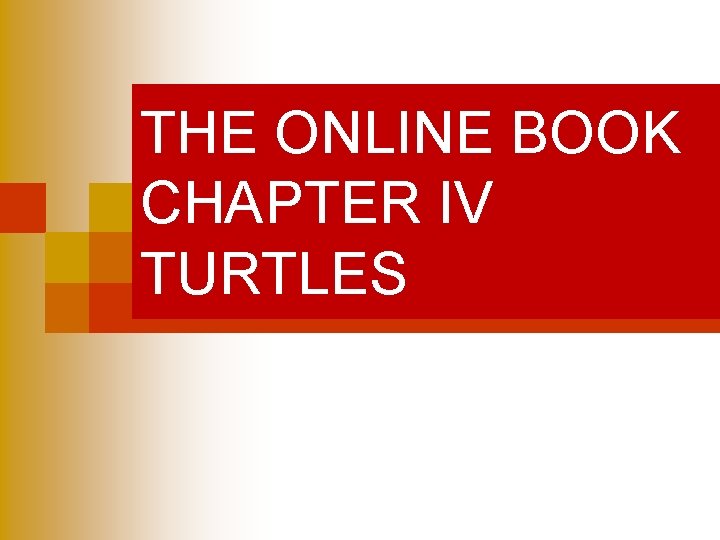
THE ONLINE BOOK CHAPTER IV TURTLES
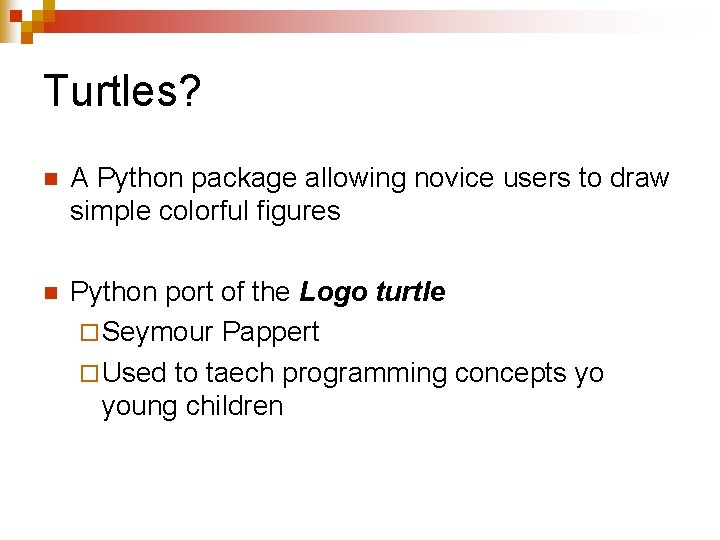
Turtles? n A Python package allowing novice users to draw simple colorful figures n Python port of the Logo turtle ¨ Seymour Pappert ¨ Used to taech programming concepts yo young children
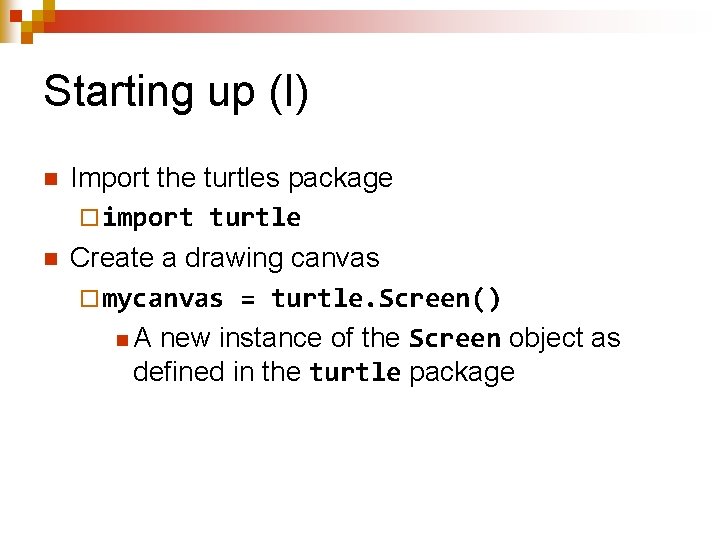
Starting up (I) n n Import the turtles package ¨ import turtle Create a drawing canvas ¨ mycanvas = turtle. Screen() n A new instance of the Screen object as defined in the turtle package
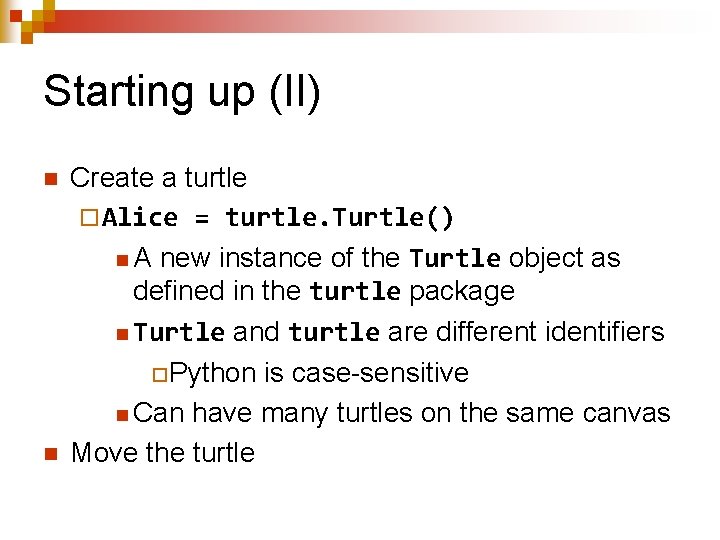
Starting up (II) n n Create a turtle ¨ Alice = turtle. Turtle() n A new instance of the Turtle object as defined in the turtle package n Turtle and turtle are different identifiers ¨Python is case-sensitive n Can have many turtles on the same canvas Move the turtle
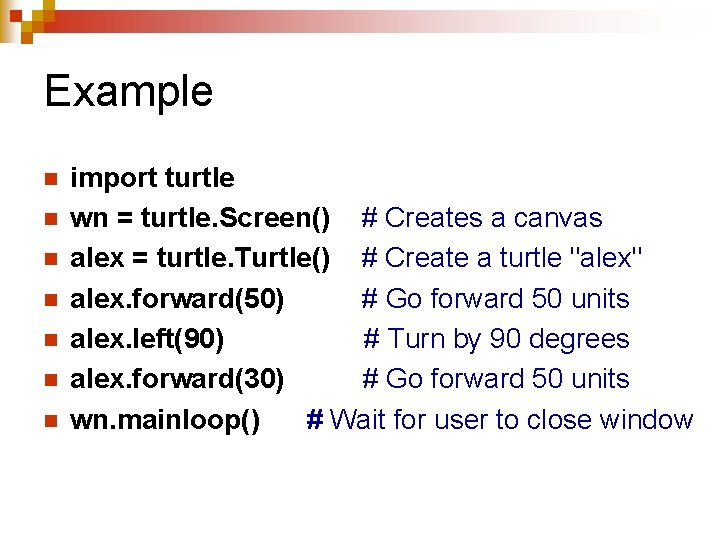
Example n n n n import turtle wn = turtle. Screen() # Creates a canvas alex = turtle. Turtle() # Create a turtle "alex" alex. forward(50) # Go forward 50 units alex. left(90) # Turn by 90 degrees alex. forward(30) # Go forward 50 units wn. mainloop() # Wait for user to close window
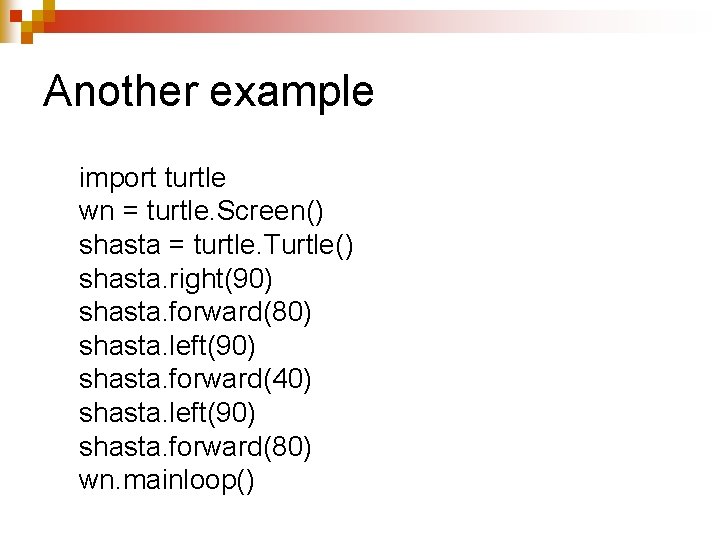
Another example import turtle wn = turtle. Screen() shasta = turtle. Turtle() shasta. right(90) shasta. forward(80) shasta. left(90) shasta. forward(40) shasta. left(90) shasta. forward(80) wn. mainloop()
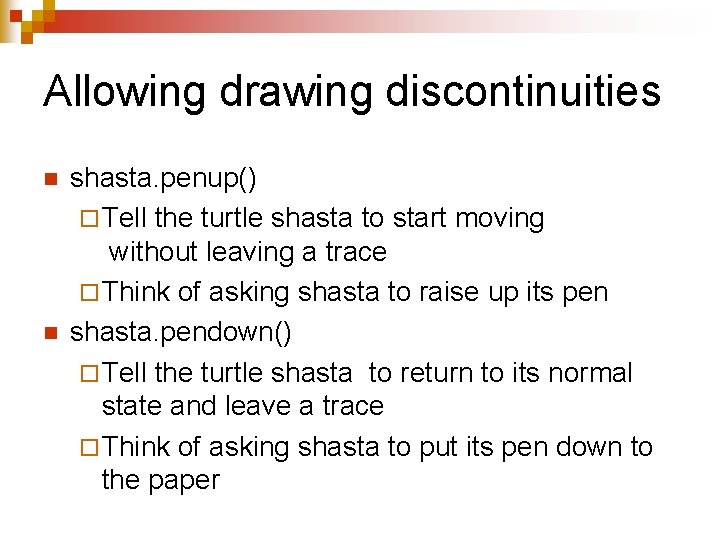
Allowing drawing discontinuities n n shasta. penup() ¨ Tell the turtle shasta to start moving without leaving a trace ¨ Think of asking shasta to raise up its pen shasta. pendown() ¨ Tell the turtle shasta to return to its normal state and leave a trace ¨ Think of asking shasta to put its pen down to the paper
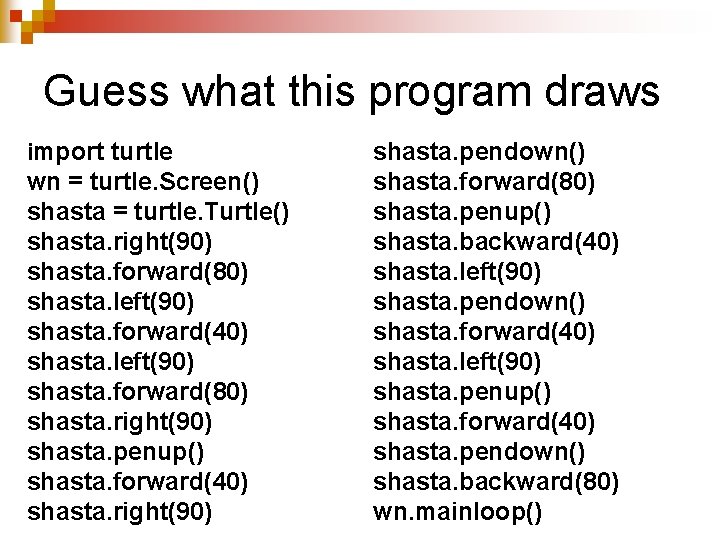
Guess what this program draws import turtle wn = turtle. Screen() shasta = turtle. Turtle() shasta. right(90) shasta. forward(80) shasta. left(90) shasta. forward(40) shasta. left(90) shasta. forward(80) shasta. right(90) shasta. penup() shasta. forward(40) shasta. right(90) shasta. pendown() shasta. forward(80) shasta. penup() shasta. backward(40) shasta. left(90) shasta. pendown() shasta. forward(40) shasta. left(90) shasta. penup() shasta. forward(40) shasta. pendown() shasta. backward(80) wn. mainloop()
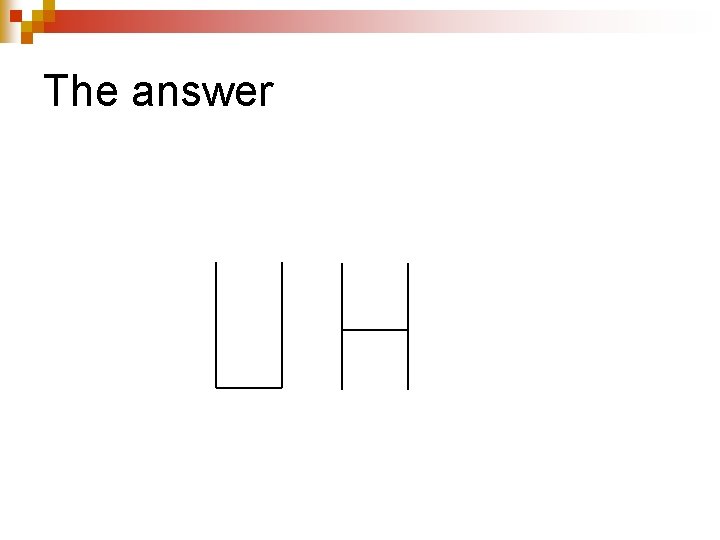
The answer
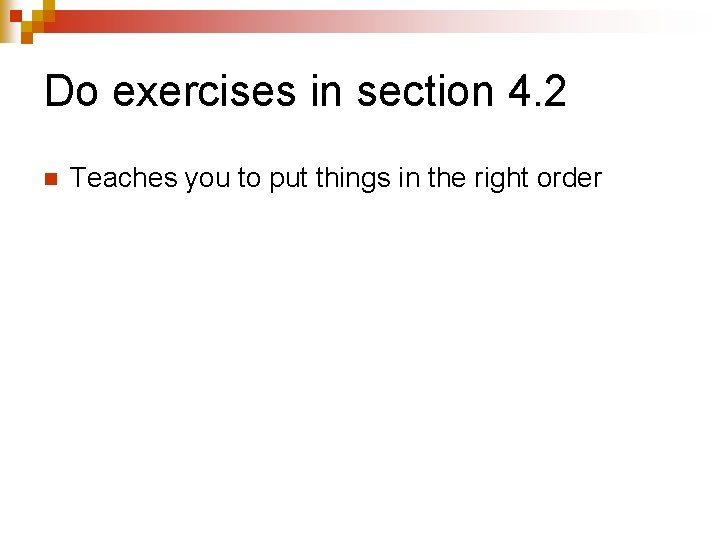
Do exercises in section 4. 2 n Teaches you to put things in the right order
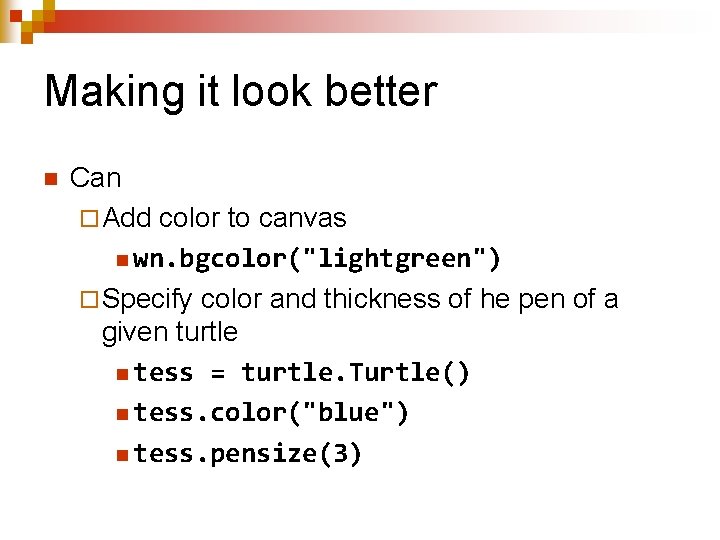
Making it look better n Can ¨ Add color to canvas n wn. bgcolor("lightgreen") ¨ Specify color and thickness of he pen of a given turtle n tess = turtle. Turtle() n tess. color("blue") n tess. pensize(3)
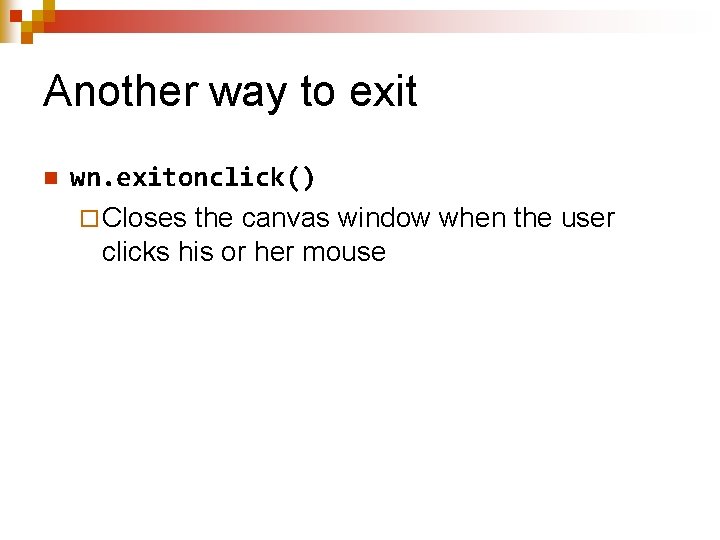
Another way to exit n wn. exitonclick() ¨ Closes the canvas window when the user clicks his or her mouse
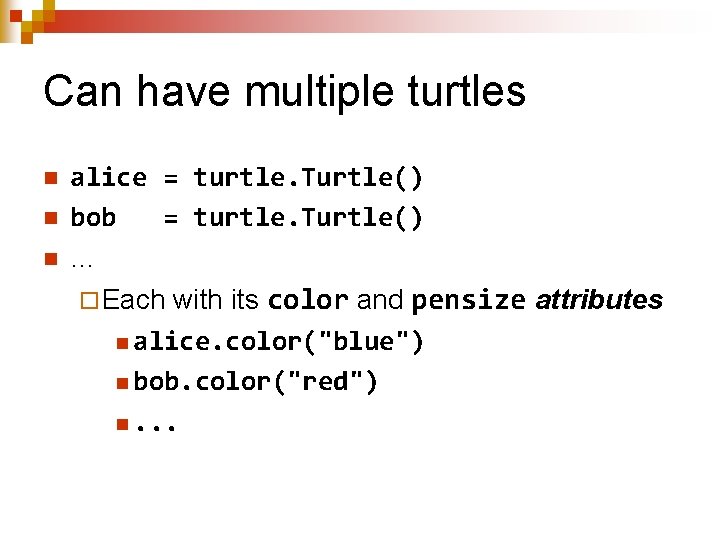
Can have multiple turtles n n n alice = turtle. Turtle() bob = turtle. Turtle() … ¨ Each with its color and pensize attributes n alice. color("blue") n bob. color("red") n. . .
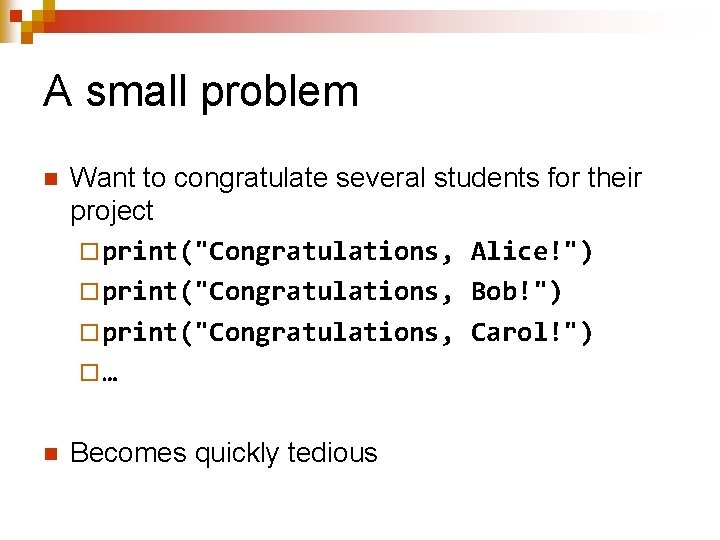
A small problem n Want to congratulate several students for their project ¨ print("Congratulations, Alice!") ¨ print("Congratulations, Bob!") ¨ print("Congratulations, Carol!") ¨… n Becomes quickly tedious
![The solution n The for loop for x in Alice Bob Carol printCongratulations The solution n The for loop for x in ["Alice", "Bob", "Carol"] : print("Congratulations,](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-16.jpg)
The solution n The for loop for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!") n Repeats the statement(s) inside the loop for all entries in the list ["Alice", "Bob", "Carol"] assigning in turn the value of each entry to x
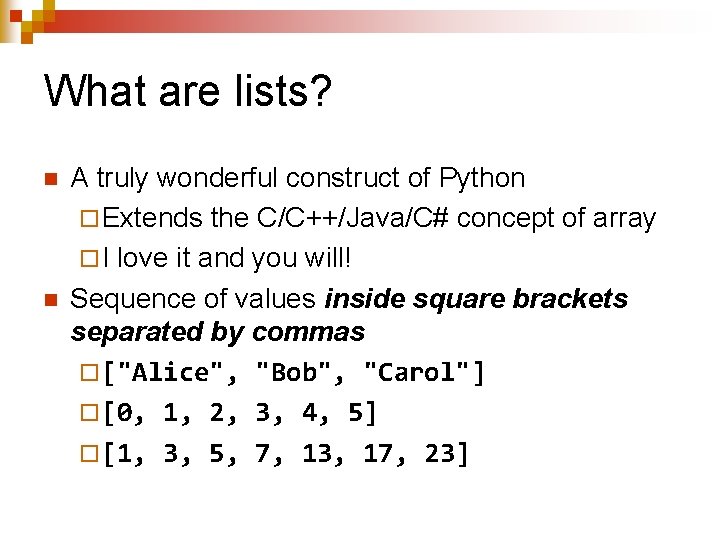
What are lists? n n A truly wonderful construct of Python ¨ Extends the C/C++/Java/C# concept of array ¨ I love it and you will! Sequence of values inside square brackets separated by commas ¨ ["Alice", "Bob", "Carol"] ¨ [0, 1, 2, 3, 4, 5] ¨ [1, 3, 5, 7, 13, 17, 23]
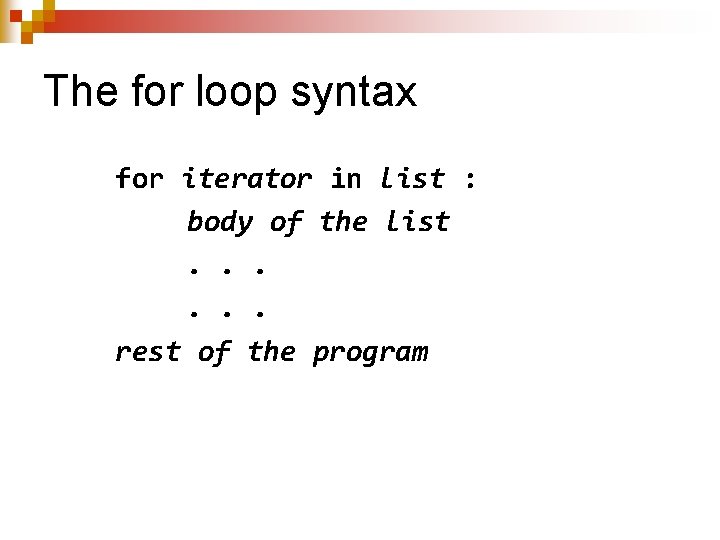
The for loop syntax for iterator in list : body of the list. . . rest of the program
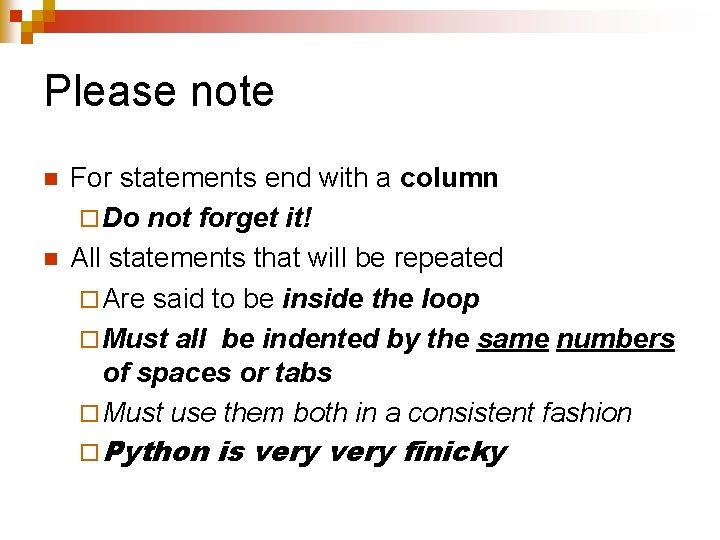
Please note n n For statements end with a column ¨ Do not forget it! All statements that will be repeated ¨ Are said to be inside the loop ¨ Must all be indented by the same numbers of spaces or tabs ¨ Must use them both in a consistent fashion ¨ Python is very finicky
![Example I for x in Alice Bob Carol printCongratulations x Example (I) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!")](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-20.jpg)
Example (I) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!") print("You did very well!") n Will print two lines of congratulations for all three entries in the list ¨ Total of six lines
![Example II for x in Alice Bob Carol printCongratulations x Example (II) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!")](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-21.jpg)
Example (II) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!") print("You did very well!") #outside n Will print a single line of congratulations for all three entries in the list then ¨ You did very well! only once ¨ Total of four lines
![Example III for x in Alice Bob Carol printCongratulations x Example (III) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!")](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-22.jpg)
Example (III) for x in ["Alice", "Bob", "Carol"] : print("Congratulations, " + x +"!") print("You did very well!") #outside n Will not print anything because the interpreter will refuse to execute the program
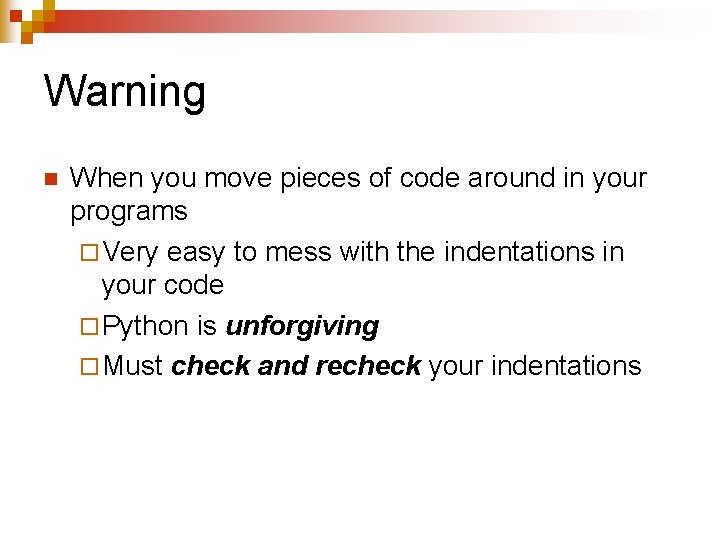
Warning n When you move pieces of code around in your programs ¨ Very easy to mess with the indentations in your code ¨ Python is unforgiving ¨ Must check and recheck your indentations
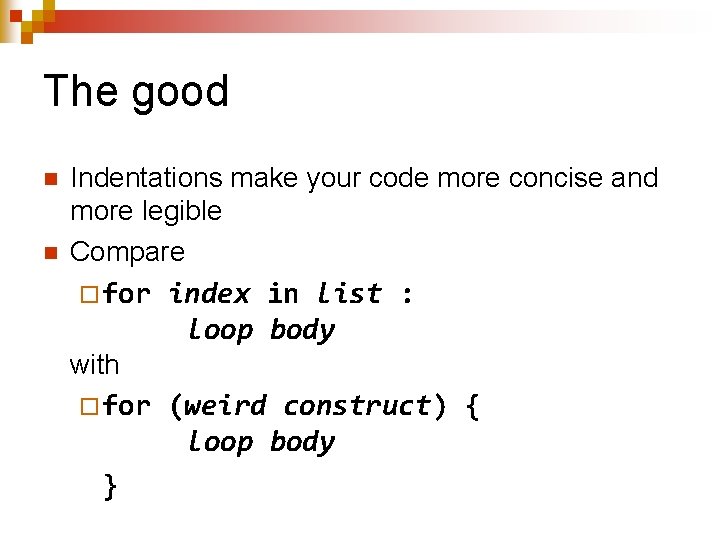
The good n n Indentations make your code more concise and more legible Compare ¨for index in list : loop body with ¨for } (weird construct) { loop body
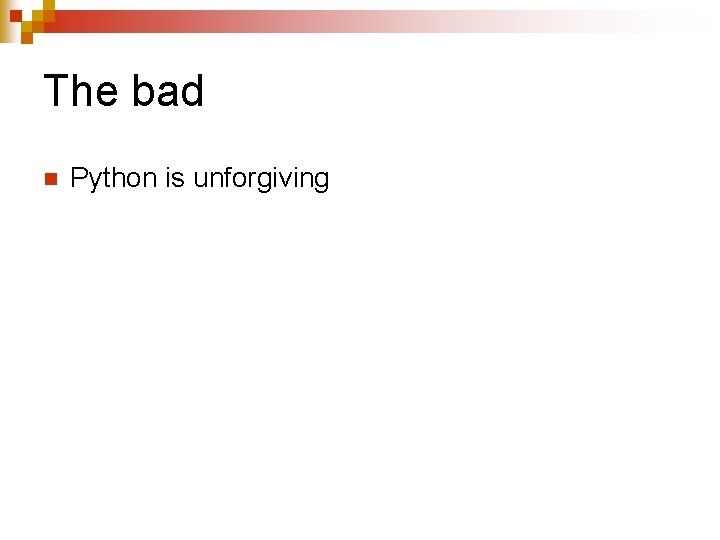
The bad n Python is unforgiving
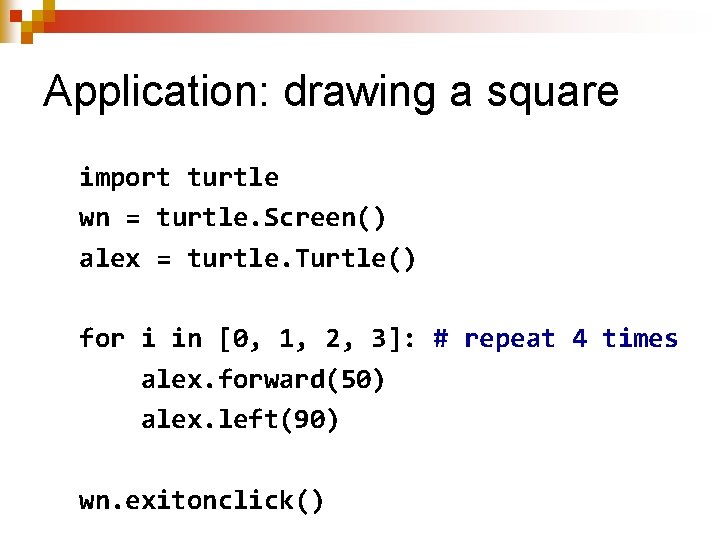
Application: drawing a square import turtle wn = turtle. Screen() alex = turtle. Turtle() for i in [0, 1, 2, 3]: # repeat 4 times alex. forward(50) alex. left(90) wn. exitonclick()
![Comments n We could have used for i in 1 2 3 4 Comments n We could have used ¨ for i in [1, 2, 3, 4]:](https://slidetodoc.com/presentation_image_h/27577d0d39011021dbfd913457cbd7b3/image-27.jpg)
Comments n We could have used ¨ for i in [1, 2, 3, 4]: as the values of i are not used inside the loop n It is just a convention to start all iterations with the zero value ¨ Unless you have a reason to do otherwise n Shared with C/C++/Java/C#/Ruby and many other similar languages ¨ Get used to it!
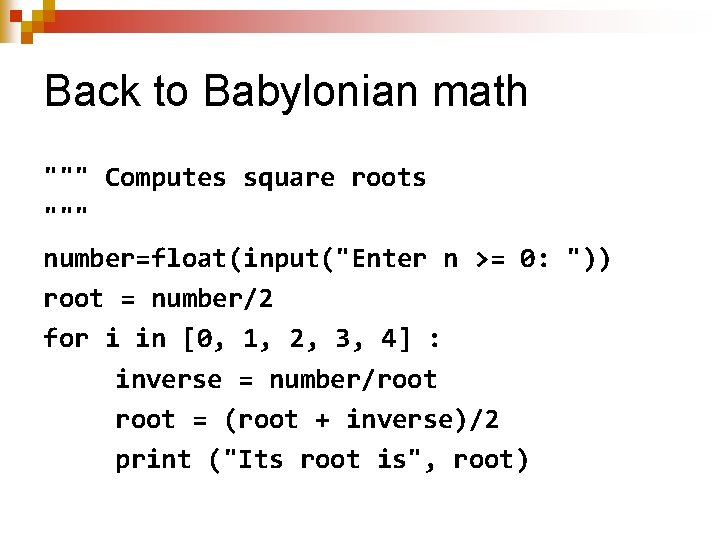
Back to Babylonian math """ Computes square roots """ number=float(input("Enter n >= 0: ")) root = number/2 for i in [0, 1, 2, 3, 4] : inverse = number/root = (root + inverse)/2 print ("Its root is", root)
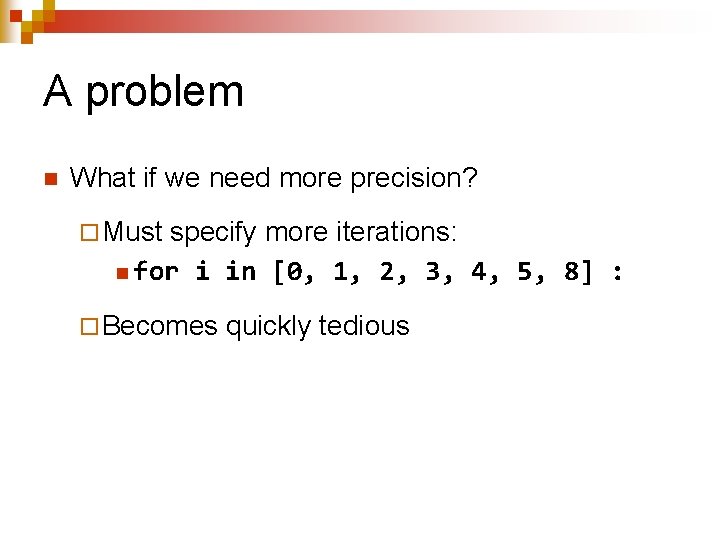
A problem n What if we need more precision? ¨ Must specify more iterations: n for i in [0, 1, 2, 3, 4, 5, 8] : ¨ Becomes quickly tedious
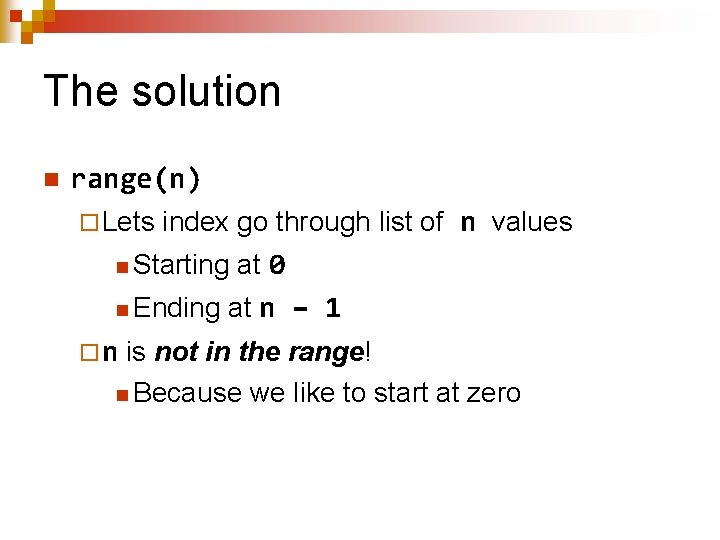
The solution n range(n) ¨ Lets index go through list of n values n Starting n Ending at 0 at n – 1 ¨n is not in the range! n Because we like to start at zero
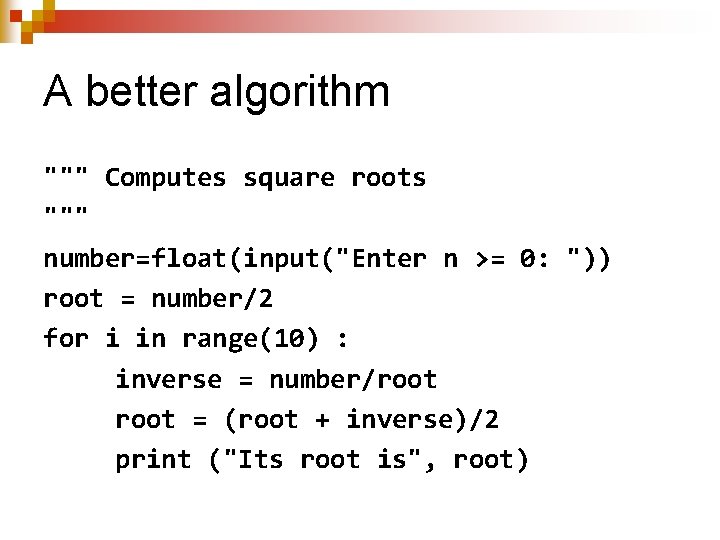
A better algorithm """ Computes square roots """ number=float(input("Enter n >= 0: ")) root = number/2 for i in range(10) : inverse = number/root = (root + inverse)/2 print ("Its root is", root)
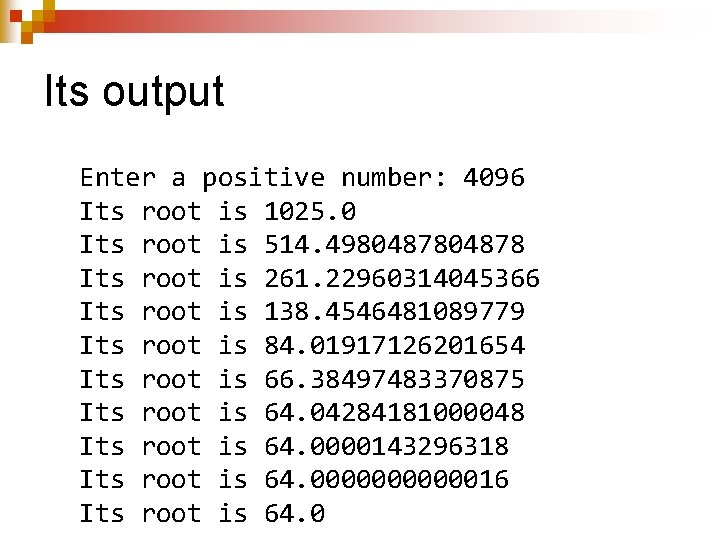
Its output Enter a positive number: 4096 Its root is 1025. 0 Its root is 514. 49804878 Its root is 261. 22960314045366 Its root is 138. 4546481089779 Its root is 84. 01917126201654 Its root is 66. 38497483370875 Its root is 64. 04284181000048 Its root is 64. 0000143296318 Its root is 64. 00000016 Its root is 64. 0
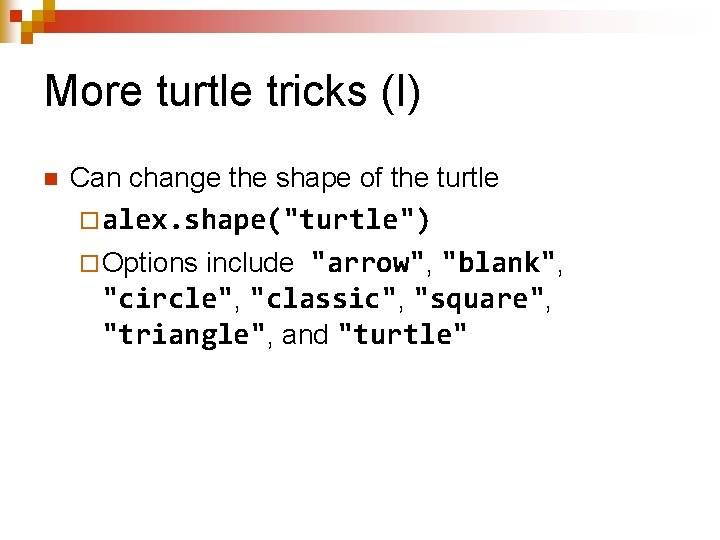
More turtle tricks (I) n Can change the shape of the turtle ¨alex. shape("turtle") include "arrow", "blank", "circle", "classic", "square", "triangle", and "turtle" ¨ Options
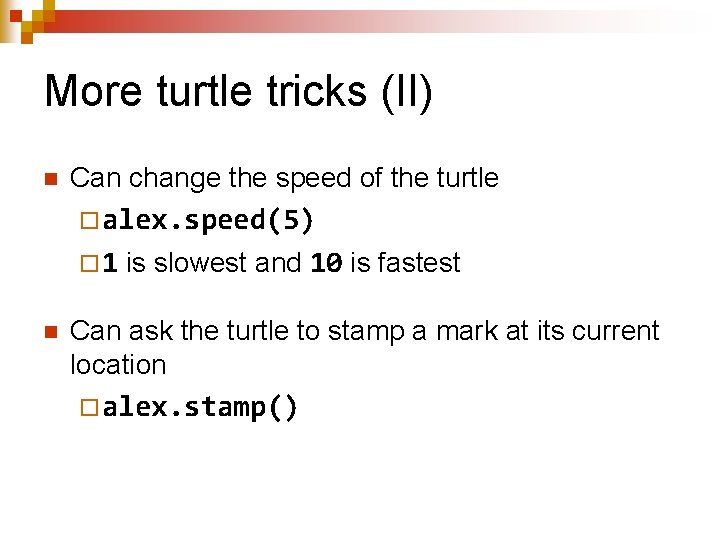
More turtle tricks (II) n Can change the speed of the turtle ¨alex. speed(5) ¨ 1 is slowest and n 10 is fastest Can ask the turtle to stamp a mark at its current location ¨alex. stamp()
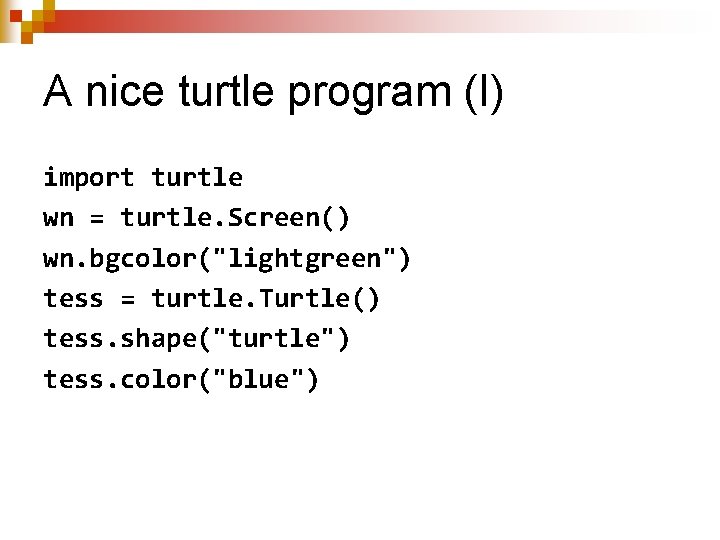
A nice turtle program (I) import turtle wn = turtle. Screen() wn. bgcolor("lightgreen") tess = turtle. Turtle() tess. shape("turtle") tess. color("blue")
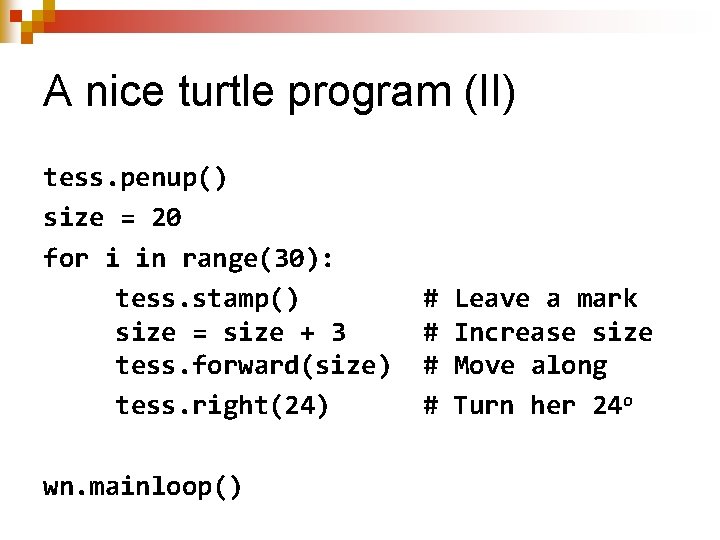
A nice turtle program (II) tess. penup() size = 20 for i in range(30): tess. stamp() size = size + 3 tess. forward(size) tess. right(24) wn. mainloop() # # Leave a mark Increase size Move along Turn her 24 o
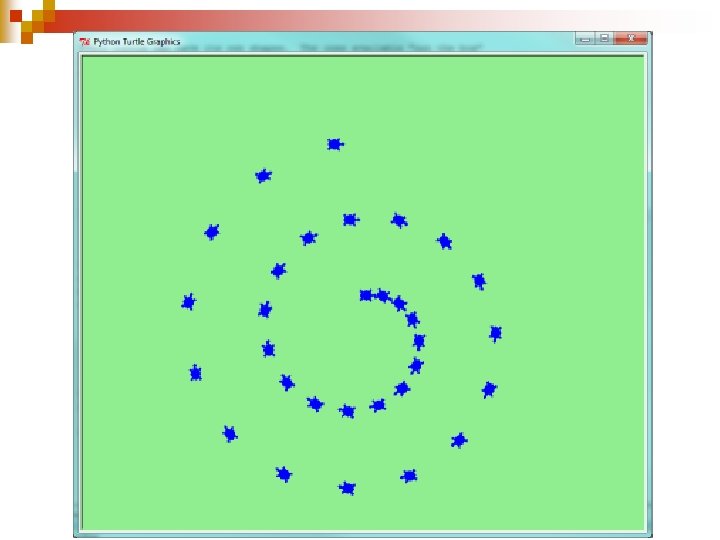