COSC 1306 COMPUTER SCIENCE AND PROGRAMMING JehanFranois Pris
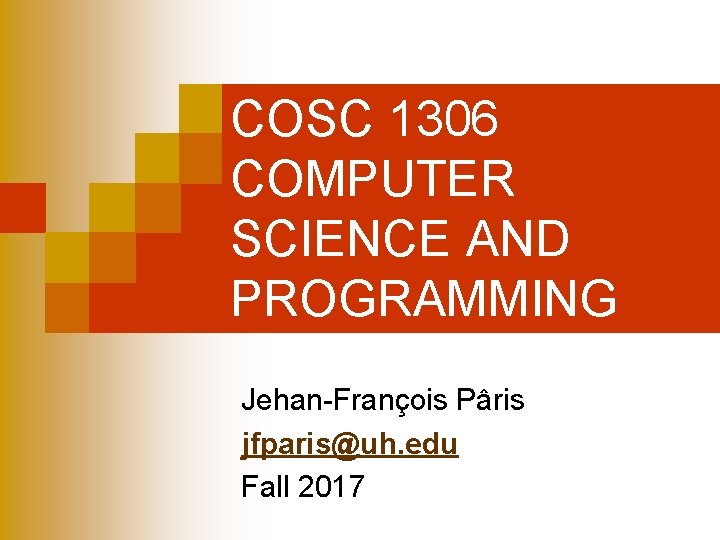
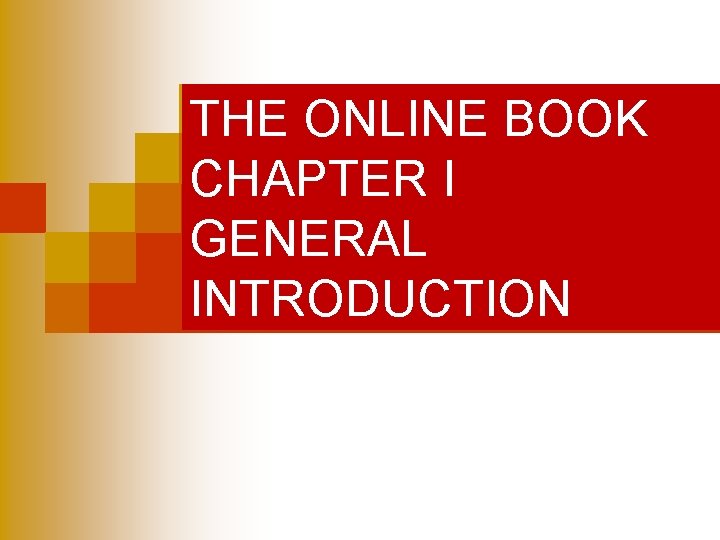
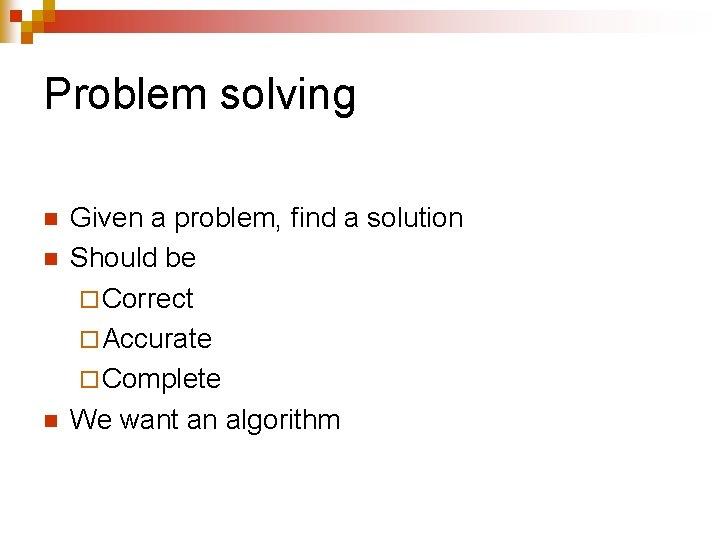
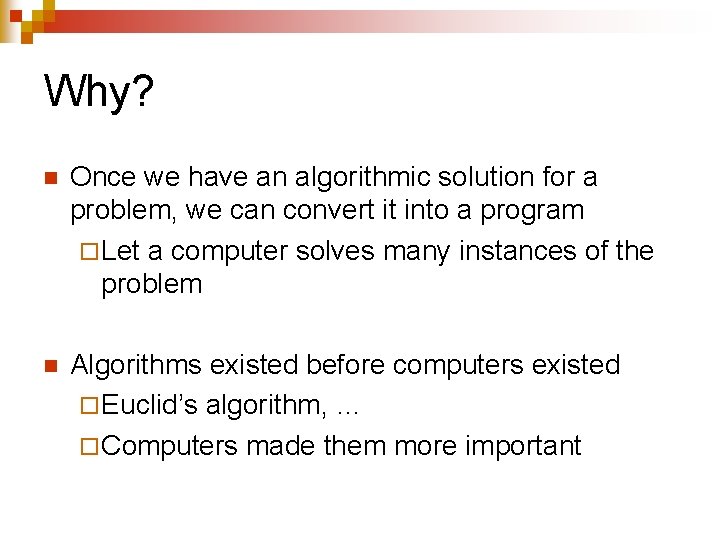
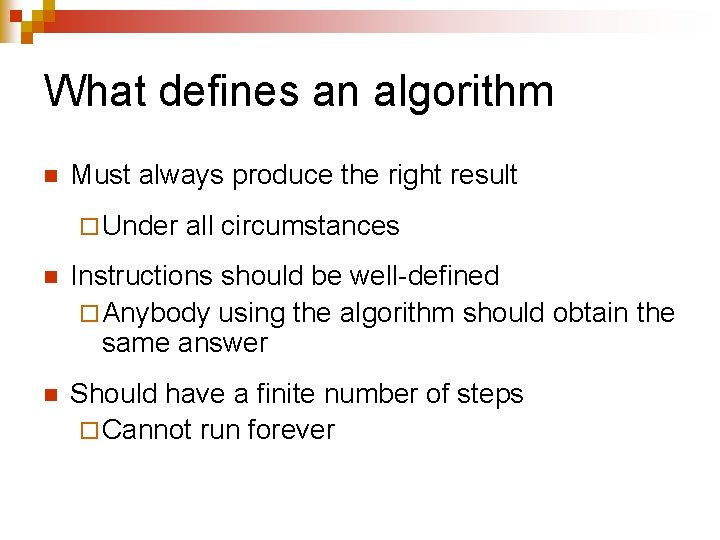
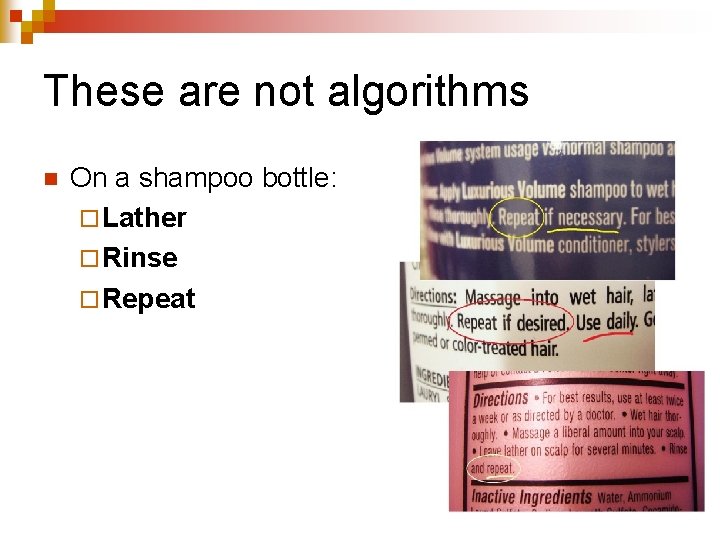
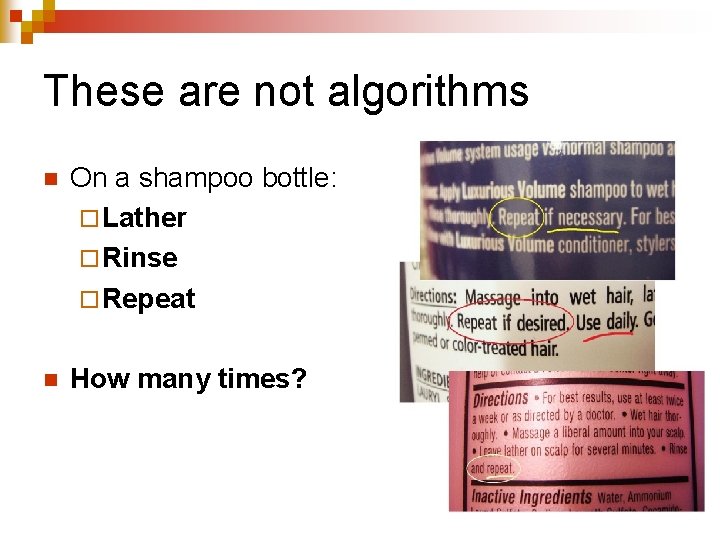
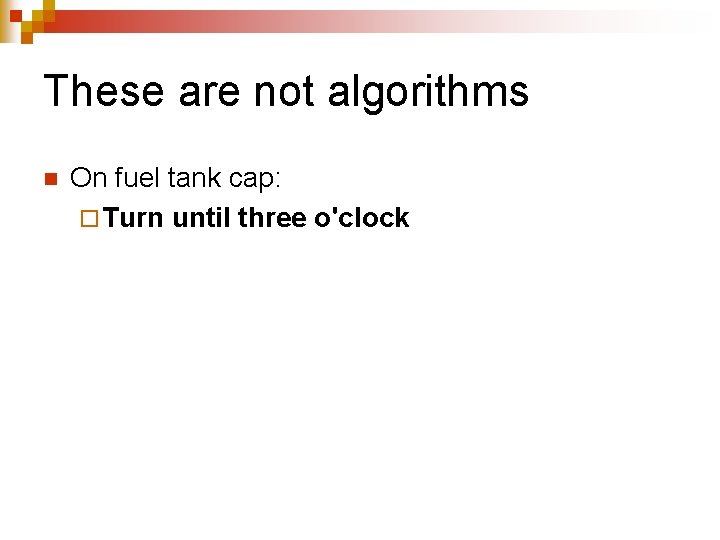
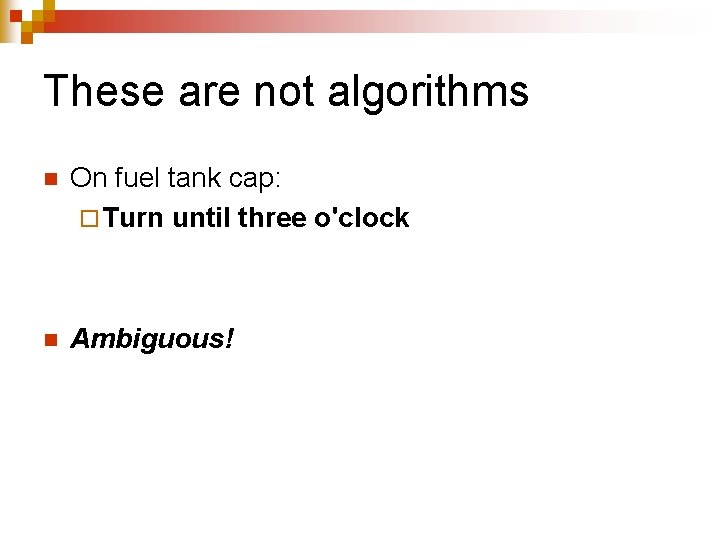
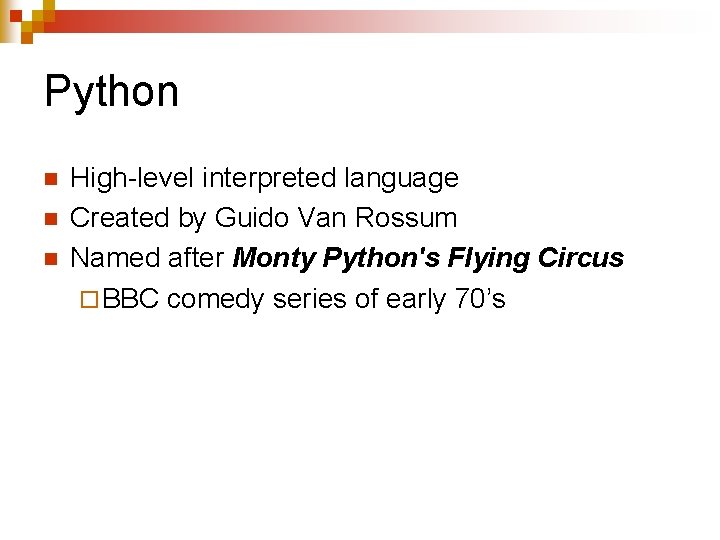
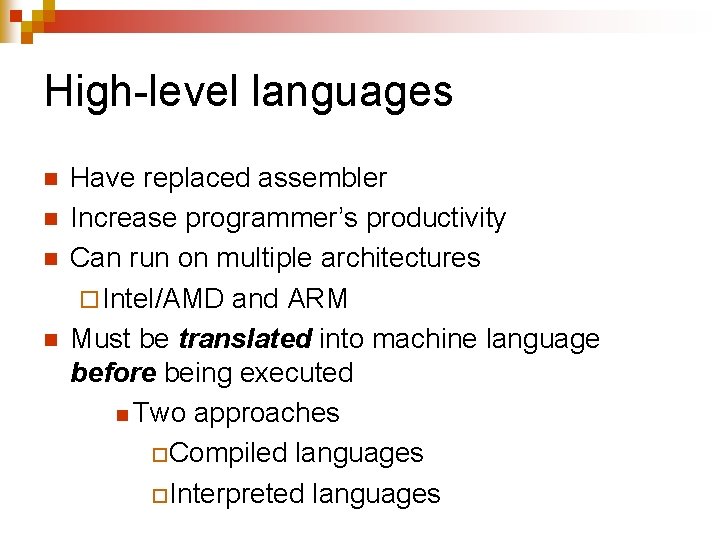
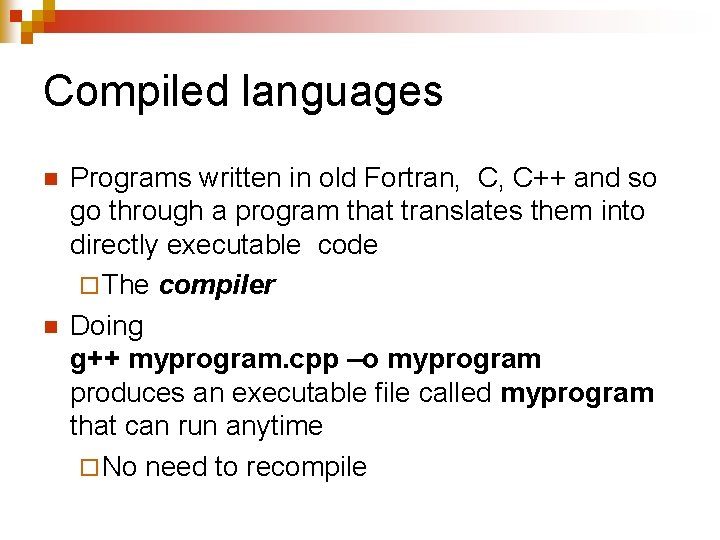
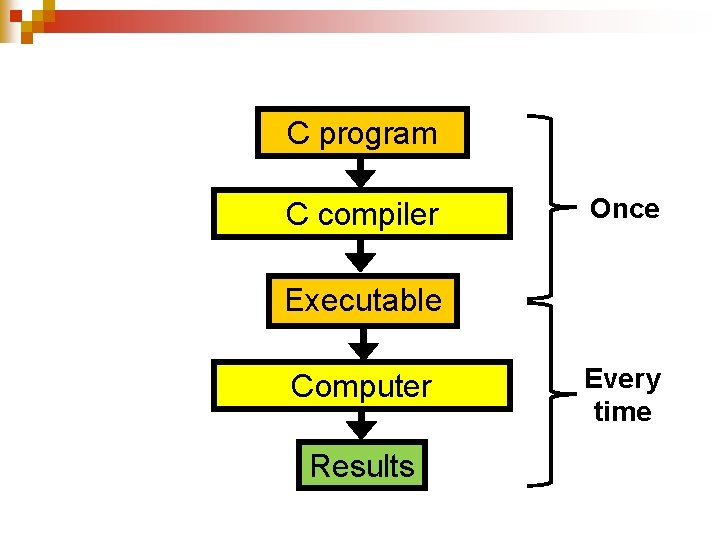
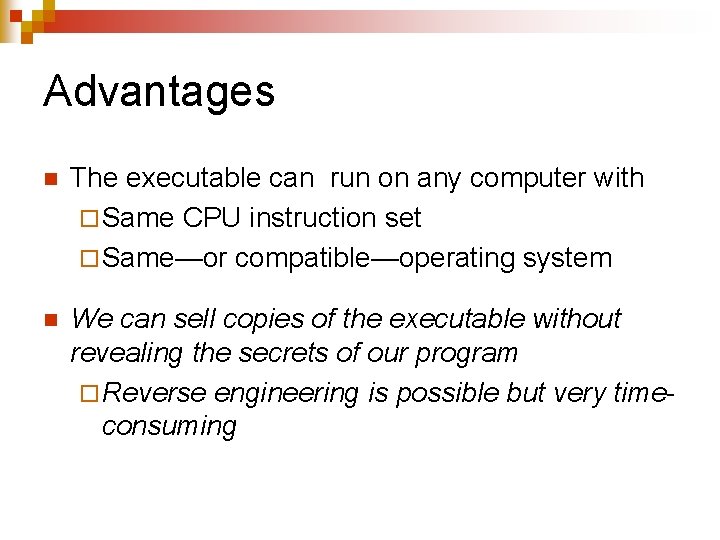
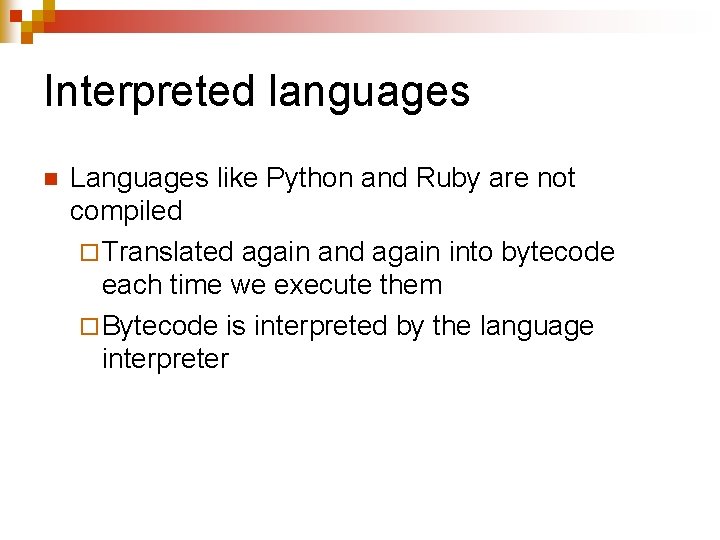
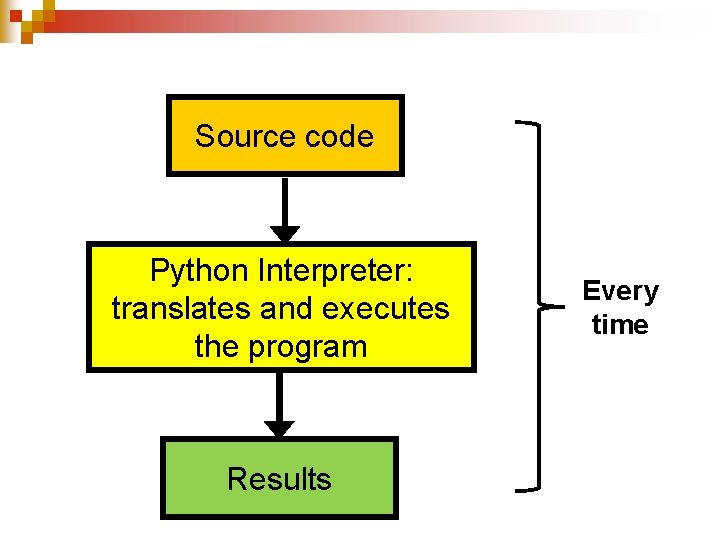
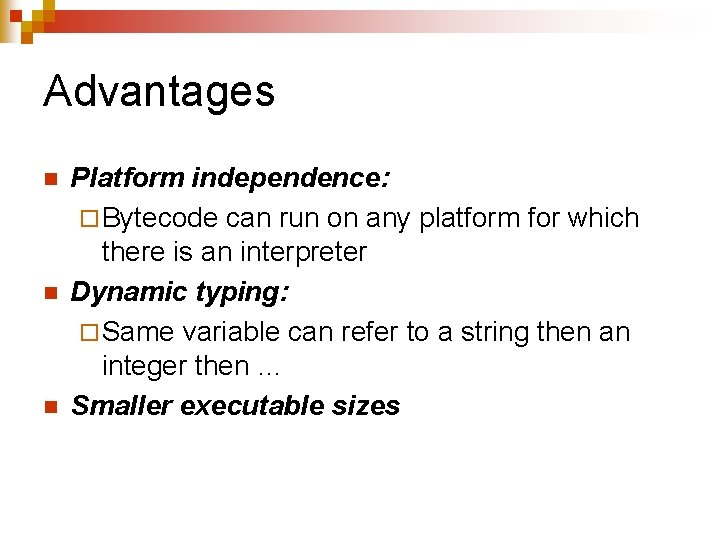
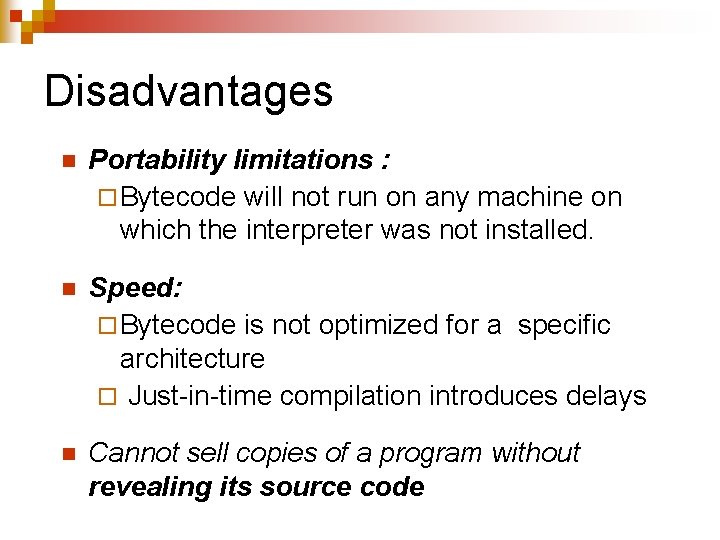
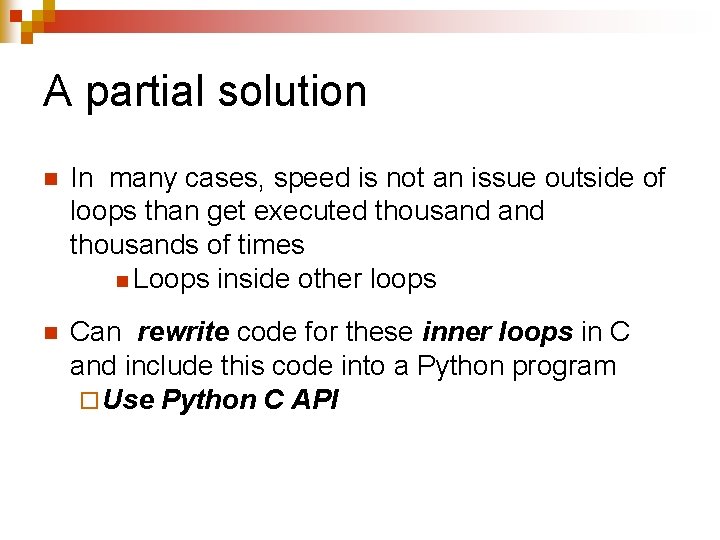
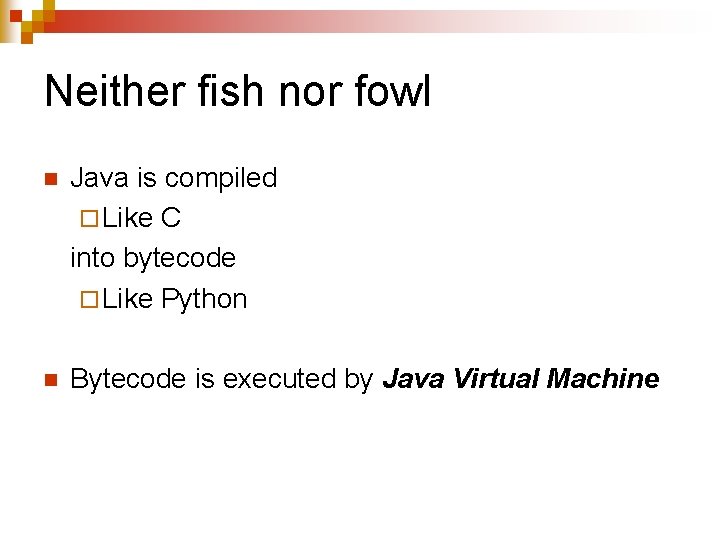
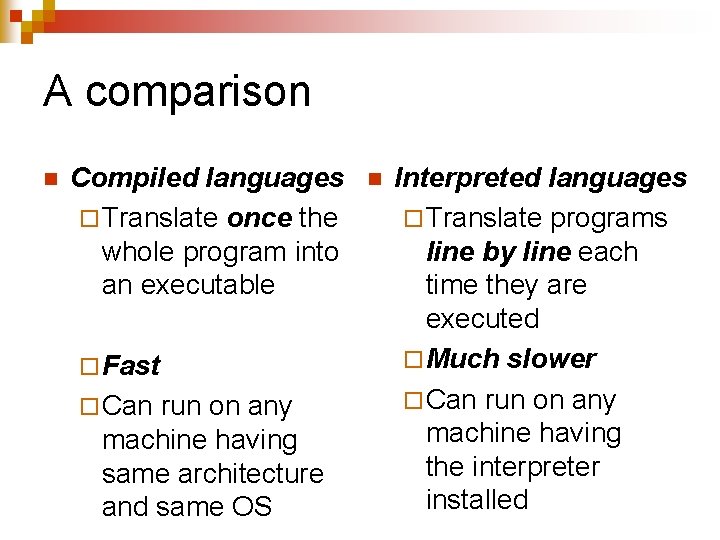
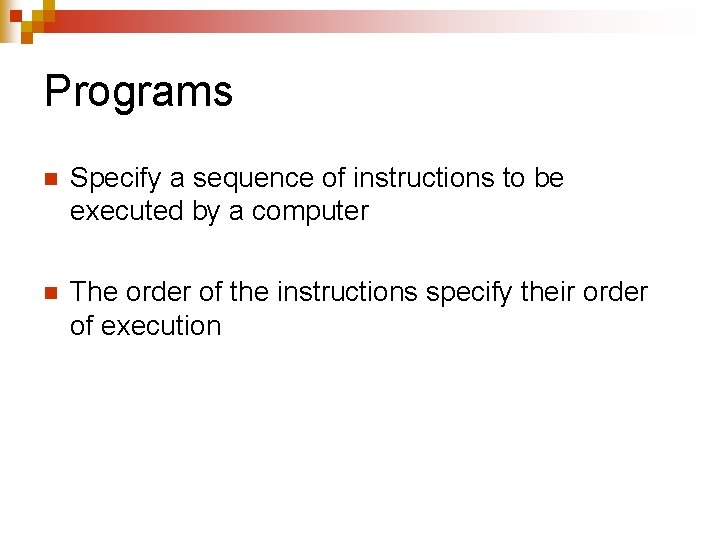
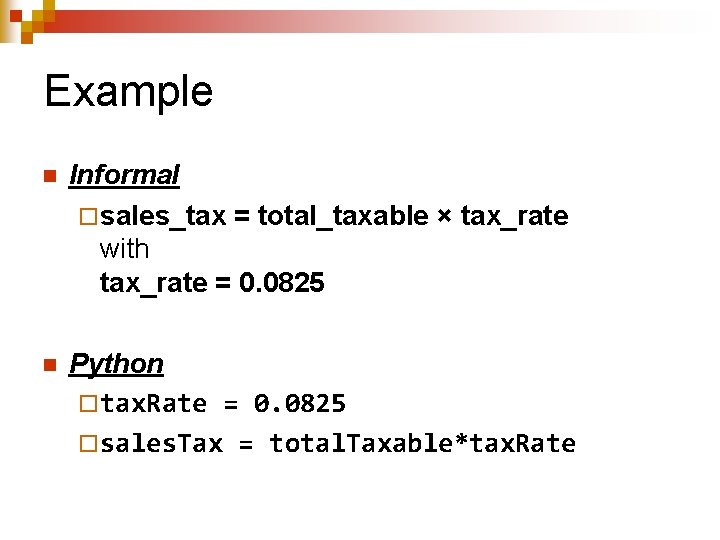
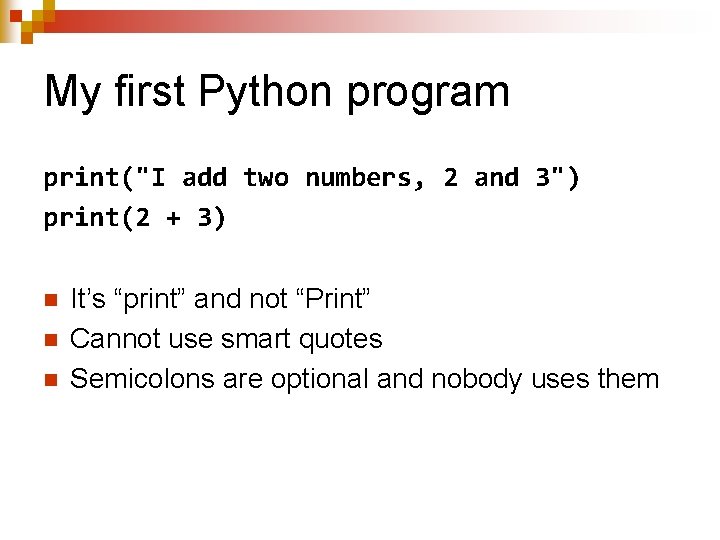
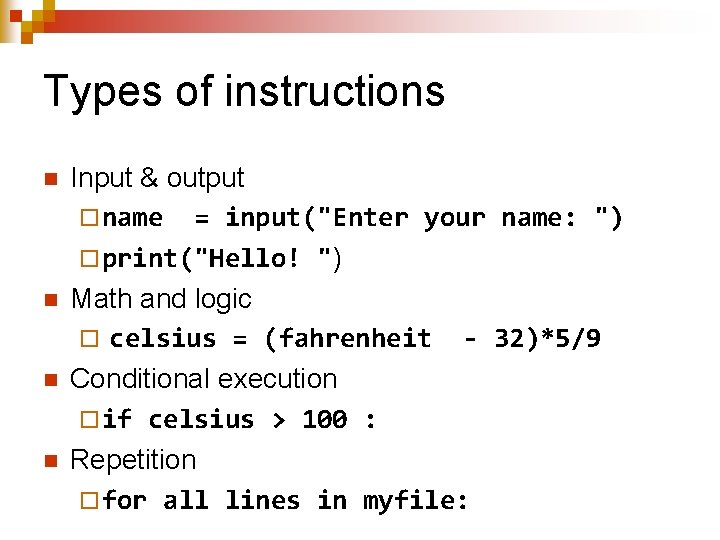
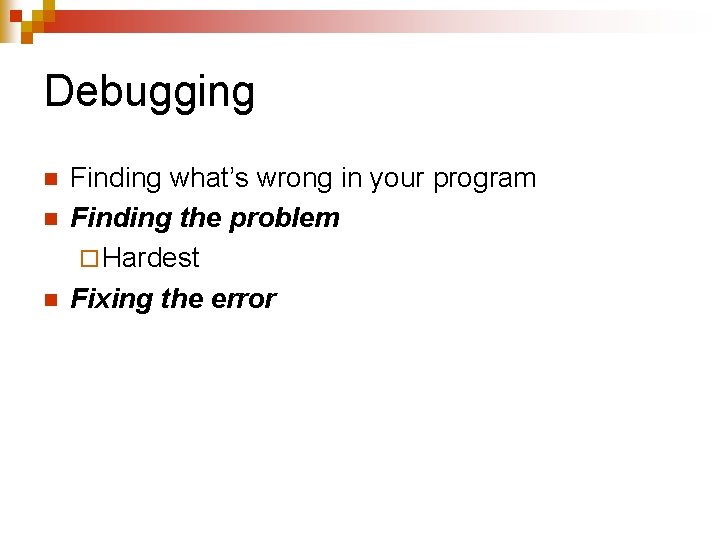
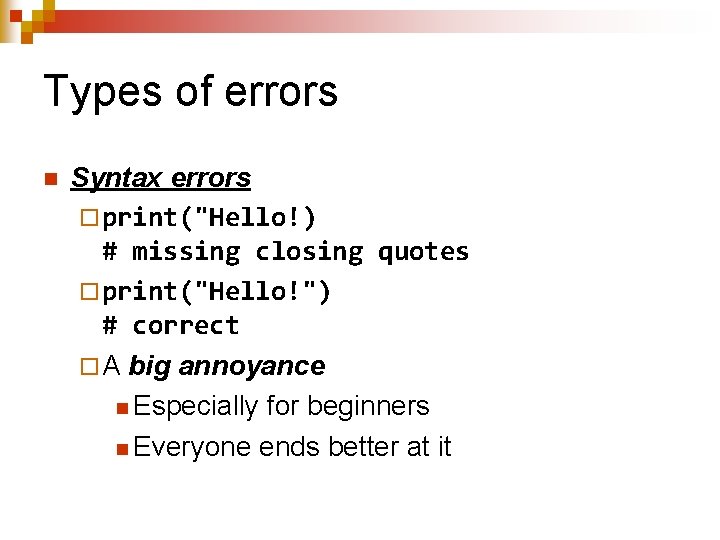
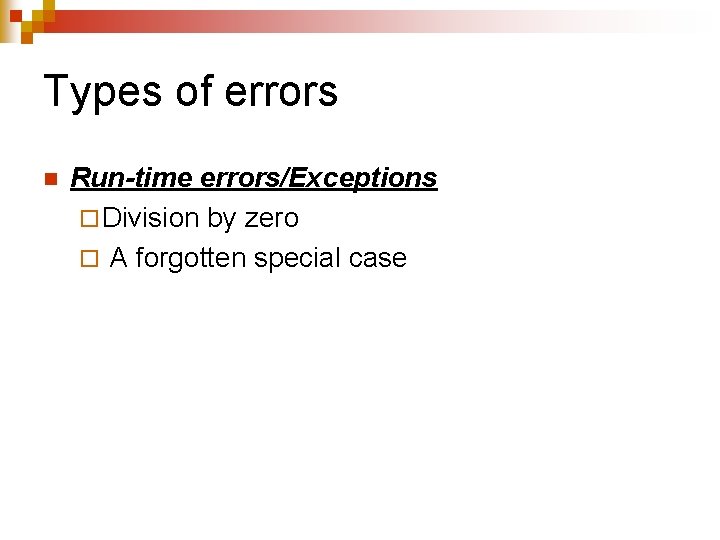
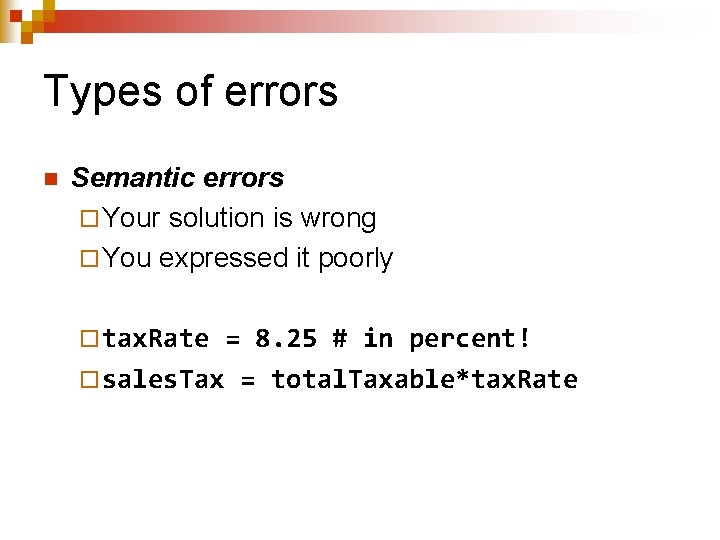
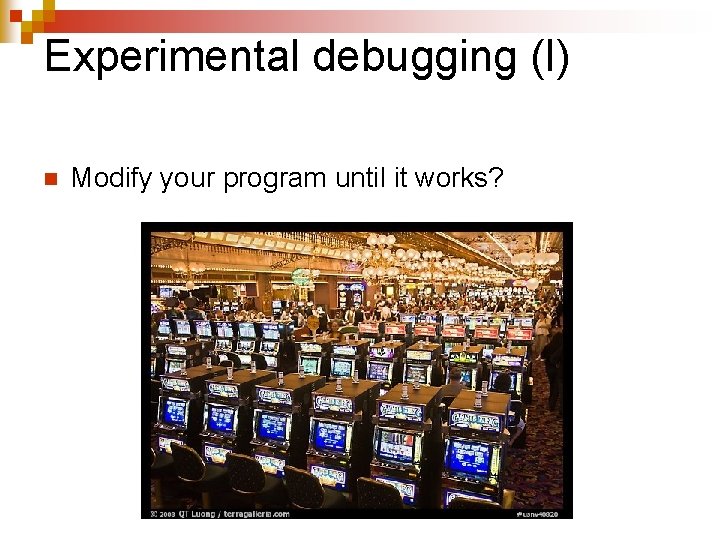
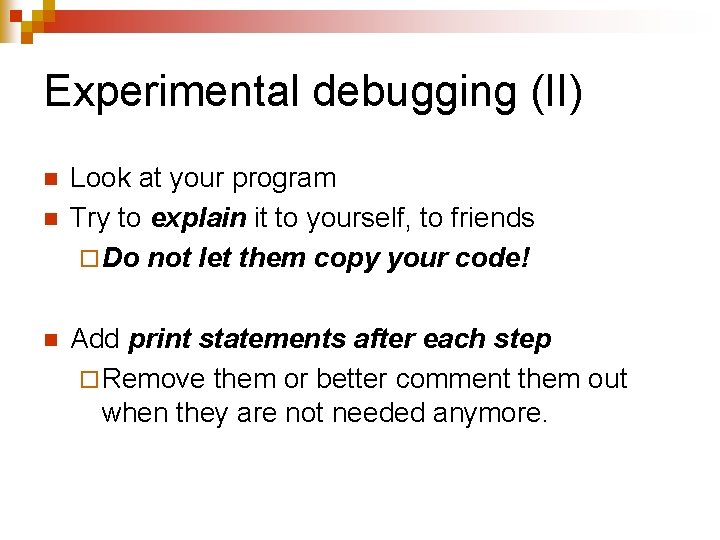
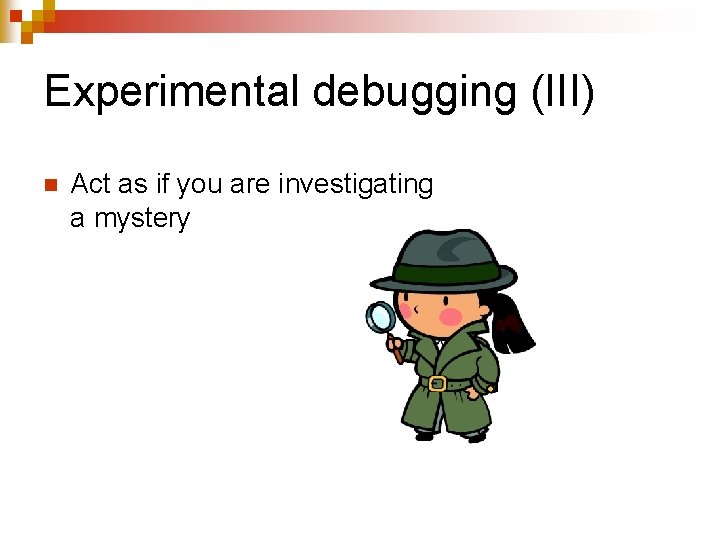
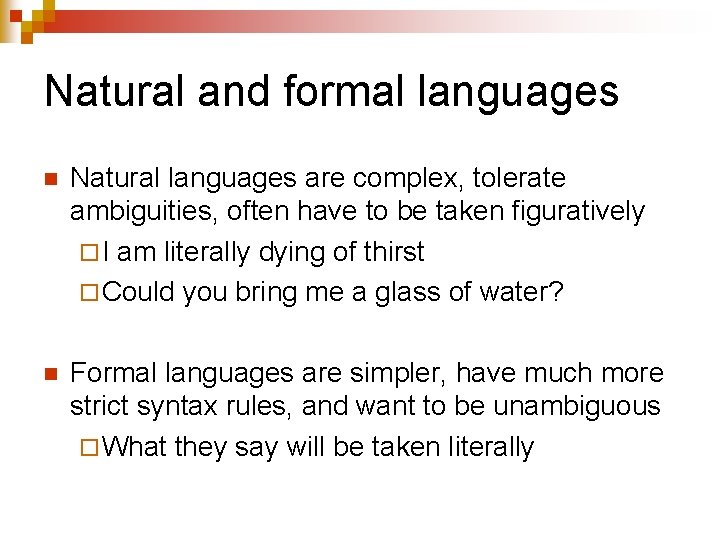
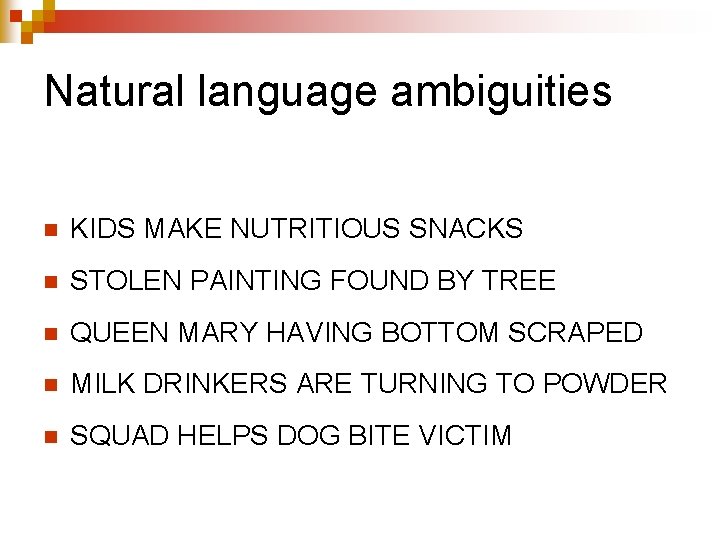
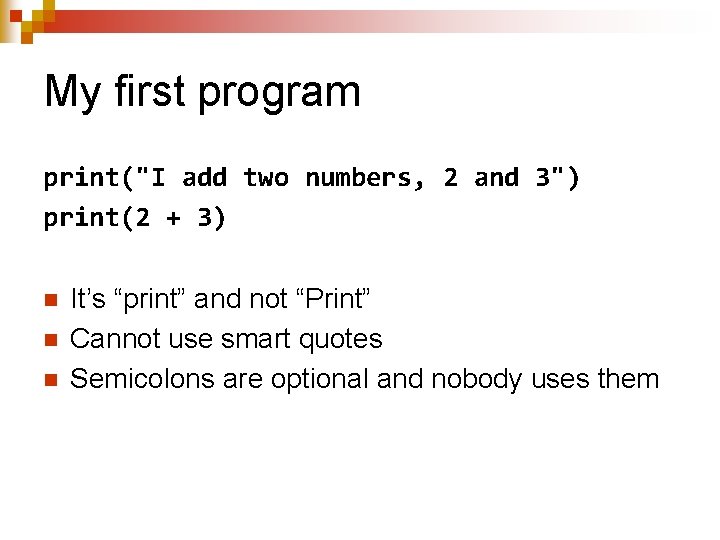
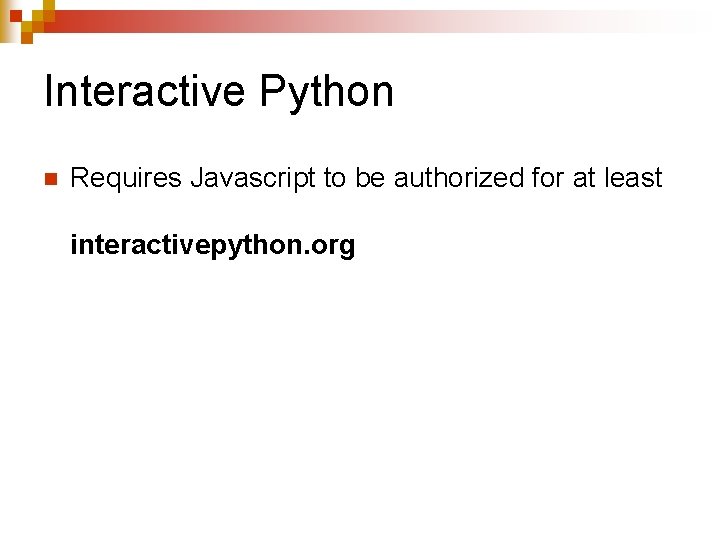
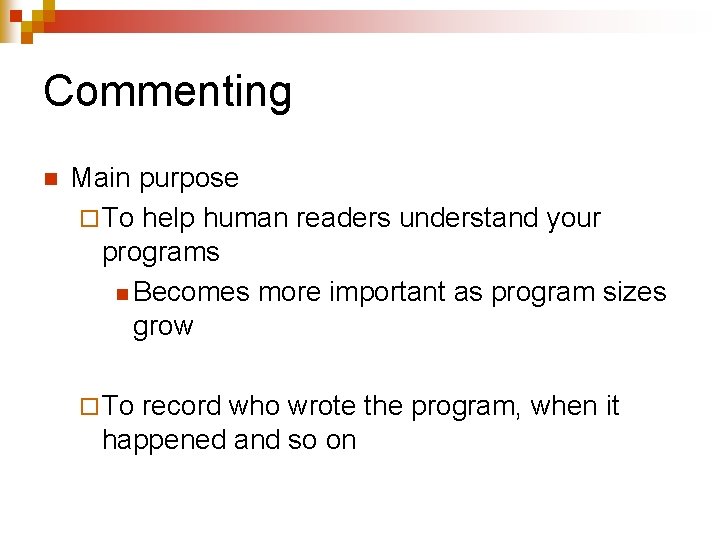
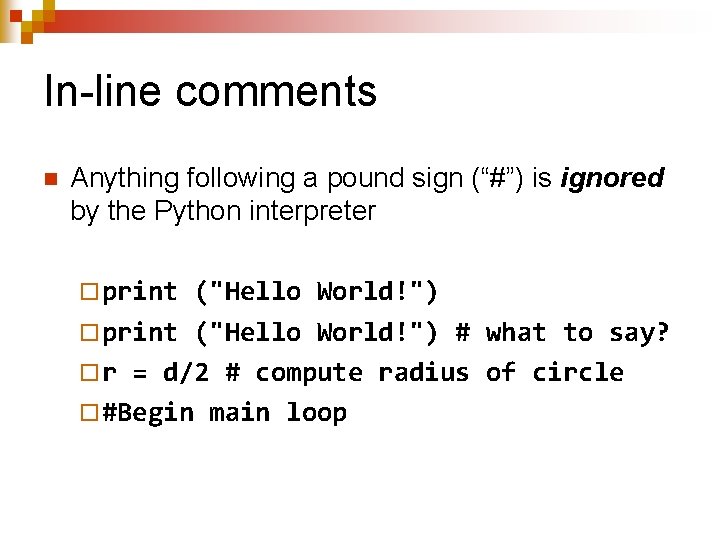
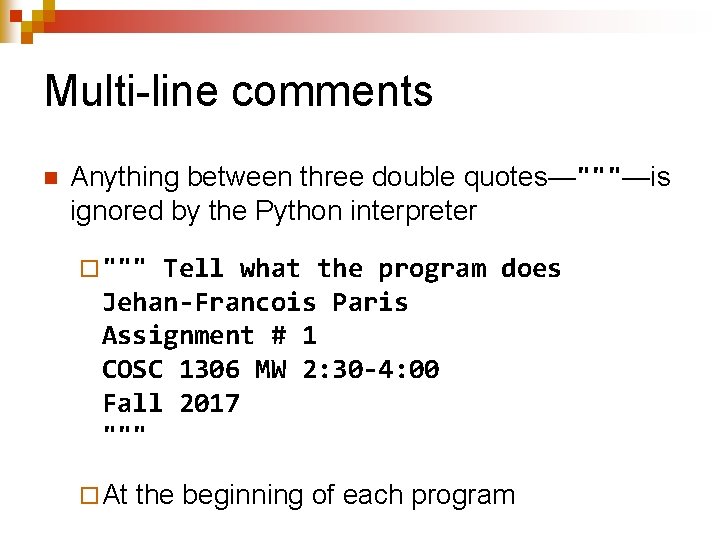
- Slides: 39
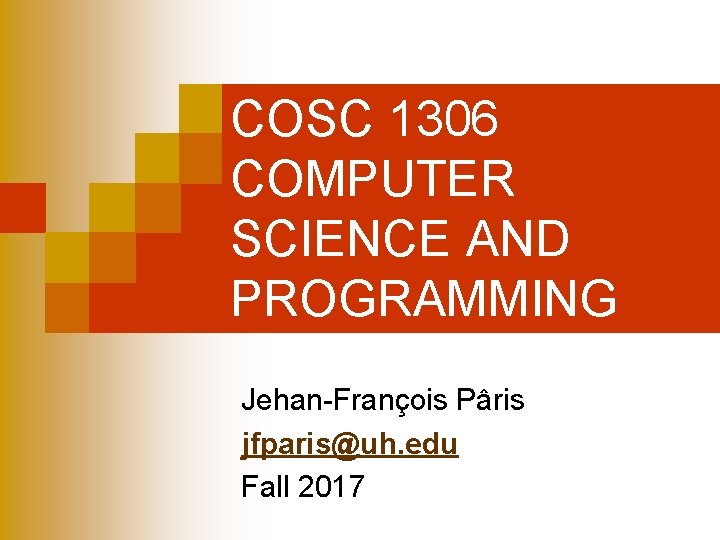
COSC 1306 COMPUTER SCIENCE AND PROGRAMMING Jehan-François Pâris jfparis@uh. edu Fall 2017
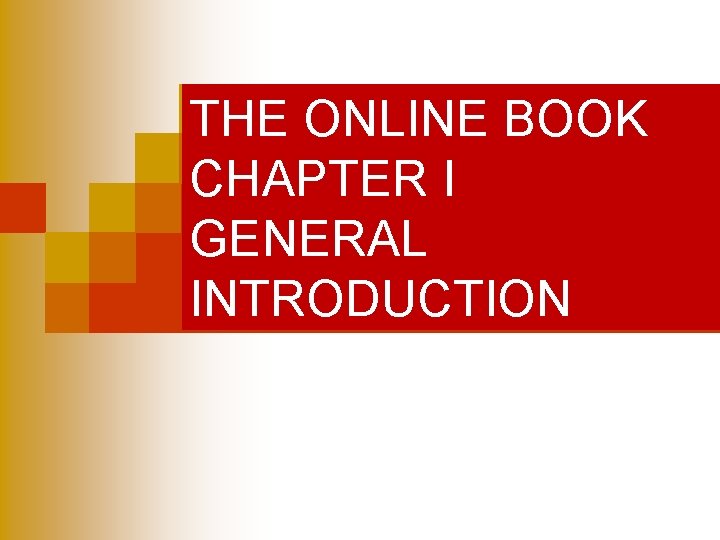
THE ONLINE BOOK CHAPTER I GENERAL INTRODUCTION
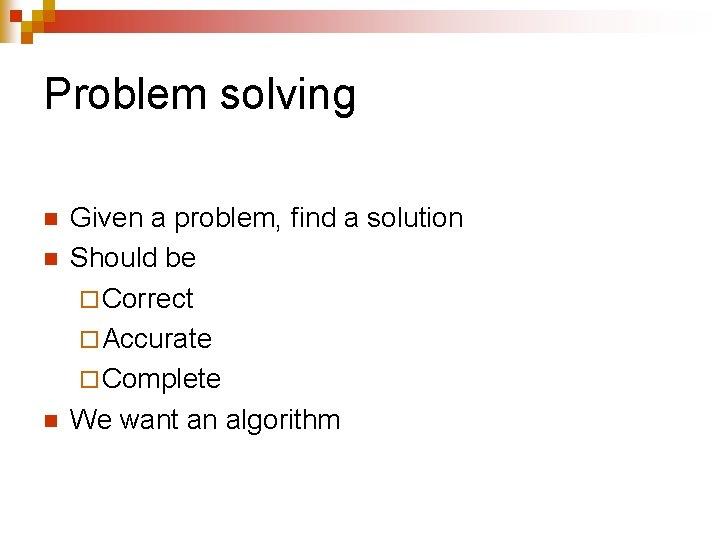
Problem solving n n n Given a problem, find a solution Should be ¨ Correct ¨ Accurate ¨ Complete We want an algorithm
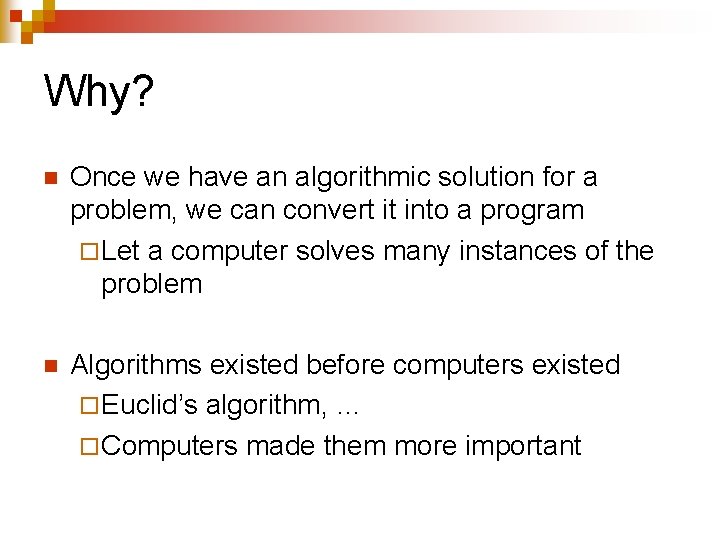
Why? n Once we have an algorithmic solution for a problem, we can convert it into a program ¨ Let a computer solves many instances of the problem n Algorithms existed before computers existed ¨ Euclid’s algorithm, … ¨ Computers made them more important
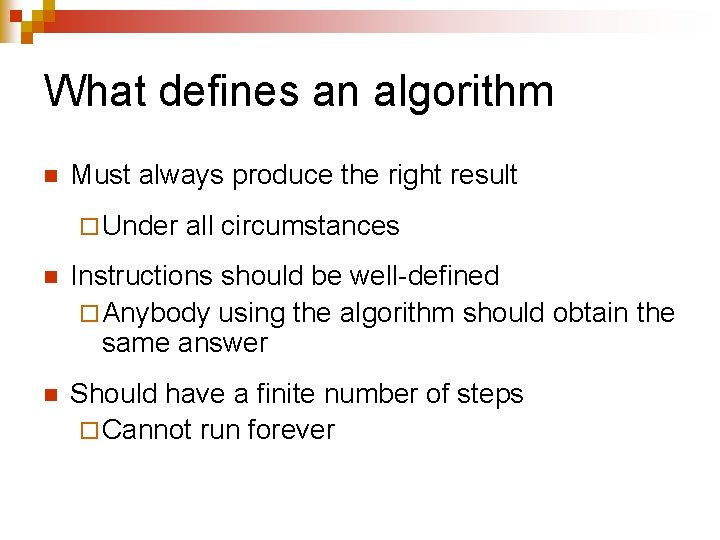
What defines an algorithm n Must always produce the right result ¨ Under all circumstances n Instructions should be well-defined ¨ Anybody using the algorithm should obtain the same answer n Should have a finite number of steps ¨ Cannot run forever
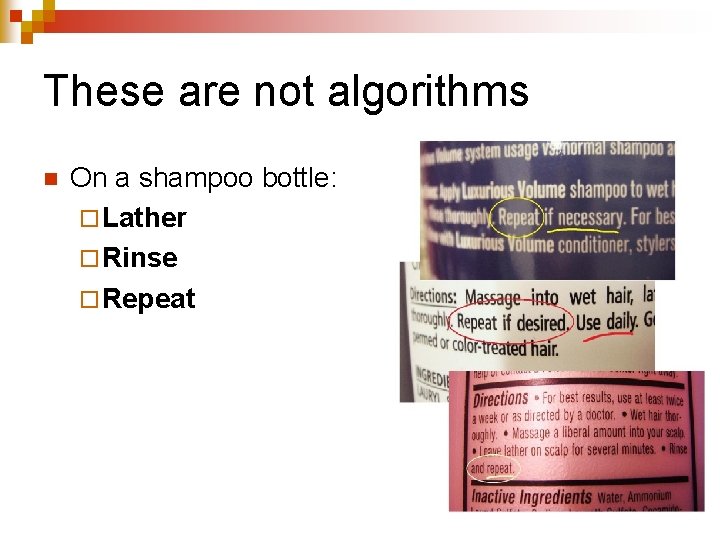
These are not algorithms n On a shampoo bottle: ¨ Lather ¨ Rinse ¨ Repeat
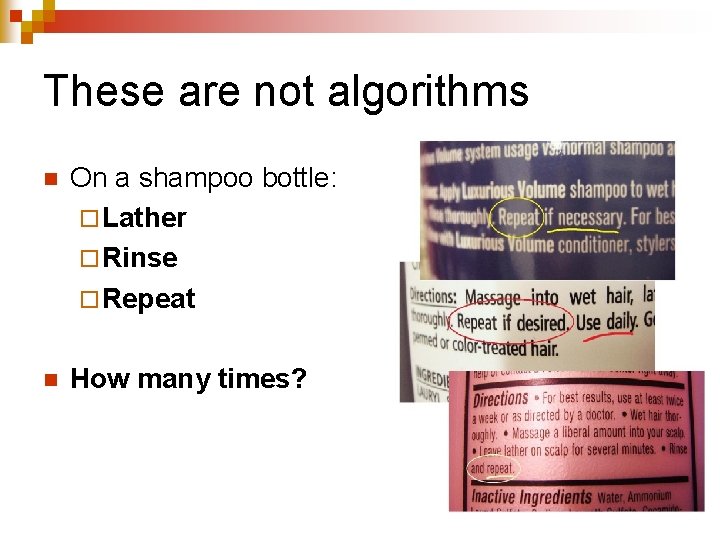
These are not algorithms n On a shampoo bottle: ¨ Lather ¨ Rinse ¨ Repeat n How many times?
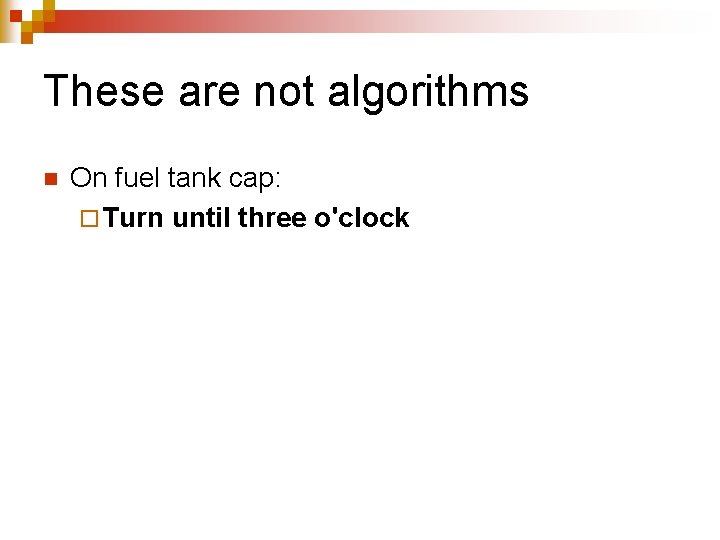
These are not algorithms n On fuel tank cap: ¨ Turn until three o'clock
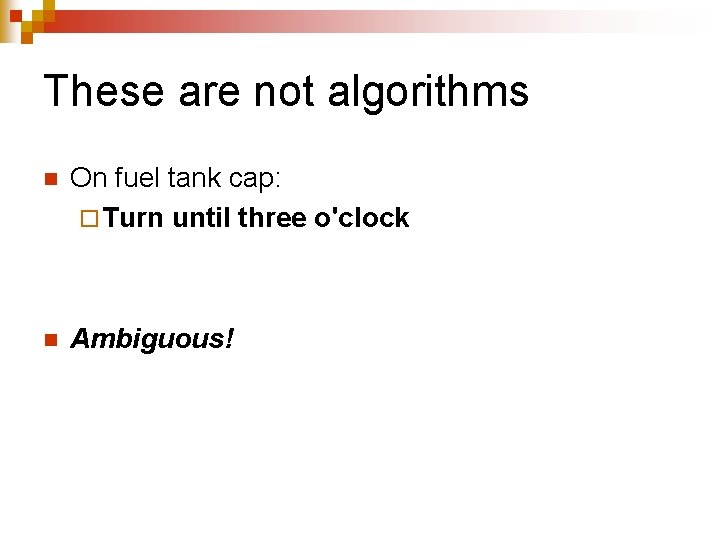
These are not algorithms n On fuel tank cap: ¨ Turn until three o'clock n Ambiguous!
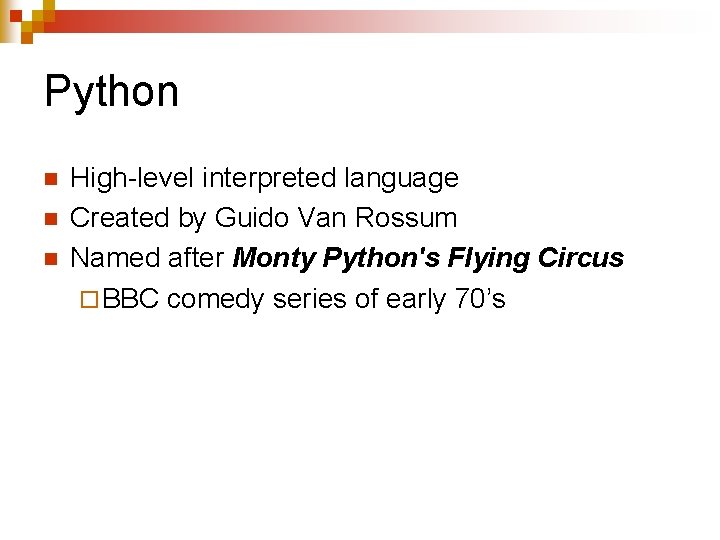
Python n High-level interpreted language Created by Guido Van Rossum Named after Monty Python's Flying Circus ¨ BBC comedy series of early 70’s
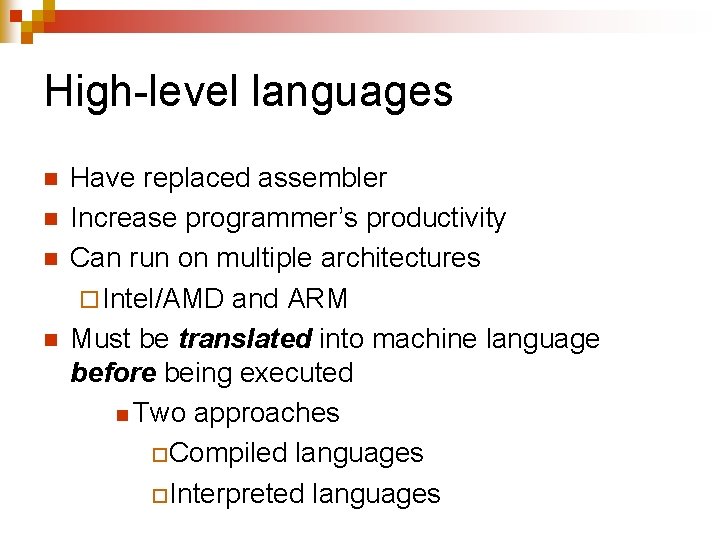
High-level languages n n Have replaced assembler Increase programmer’s productivity Can run on multiple architectures ¨ Intel/AMD and ARM Must be translated into machine language before being executed n Two approaches ¨Compiled languages ¨Interpreted languages
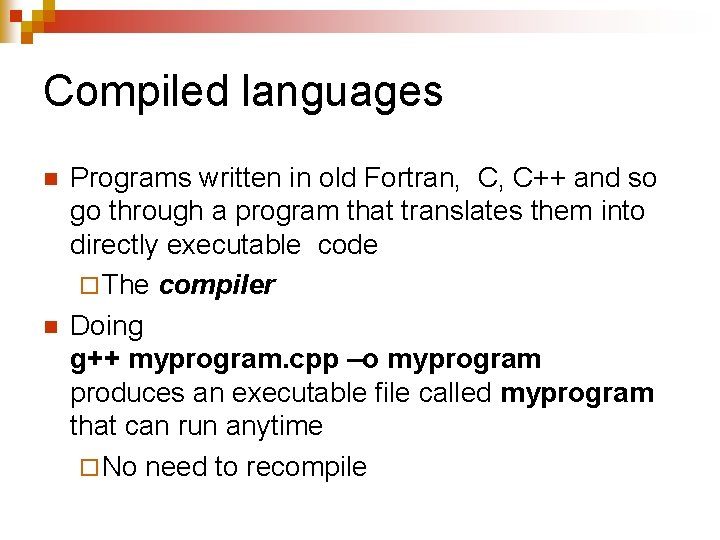
Compiled languages n n Programs written in old Fortran, C, C++ and so go through a program that translates them into directly executable code ¨ The compiler Doing g++ myprogram. cpp –o myprogram produces an executable file called myprogram that can run anytime ¨ No need to recompile
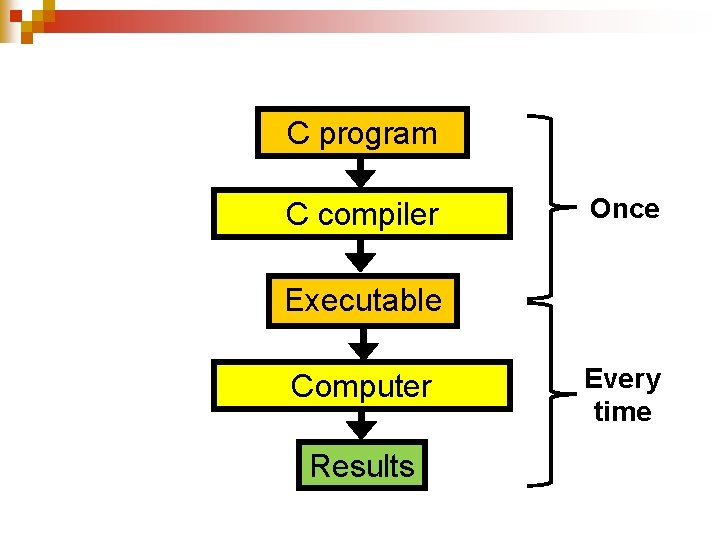
C program C compiler Once Executable Computer Results Every time
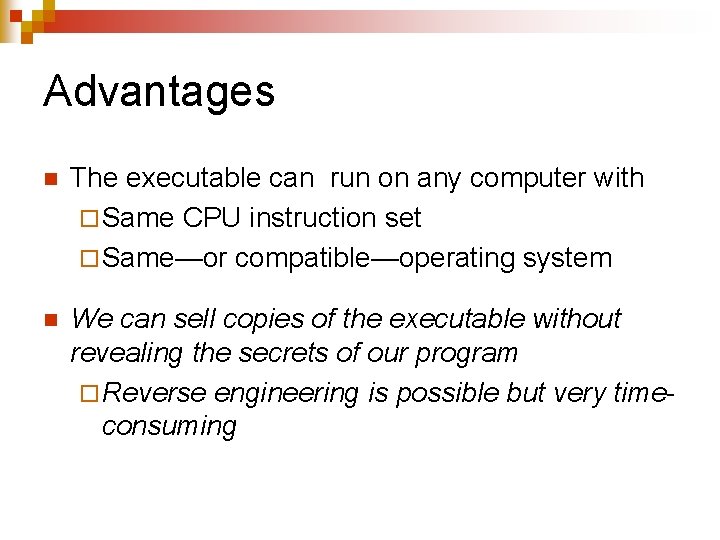
Advantages n The executable can run on any computer with ¨ Same CPU instruction set ¨ Same—or compatible—operating system n We can sell copies of the executable without revealing the secrets of our program ¨ Reverse engineering is possible but very timeconsuming
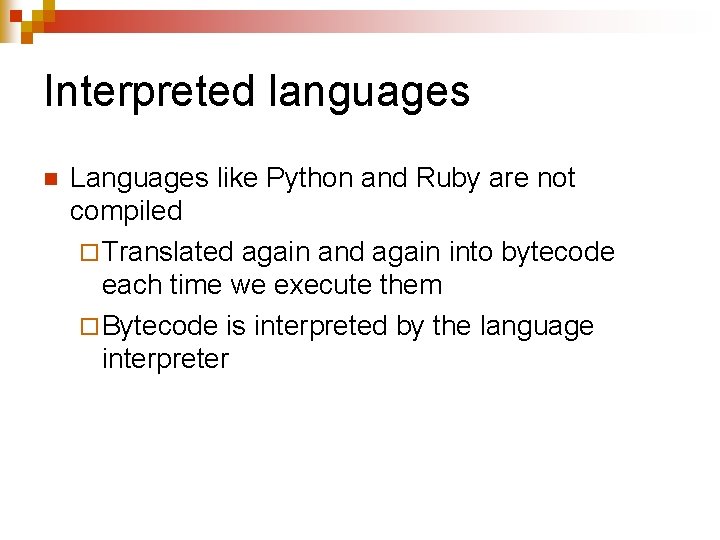
Interpreted languages n Languages like Python and Ruby are not compiled ¨ Translated again and again into bytecode each time we execute them ¨ Bytecode is interpreted by the language interpreter
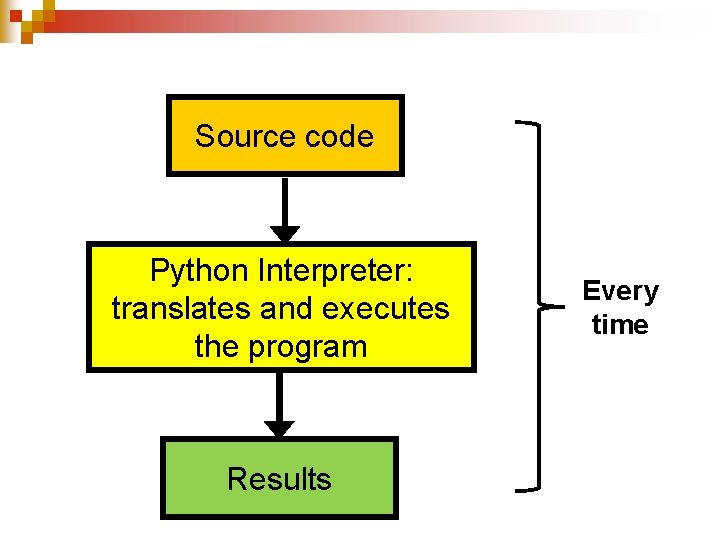
Source code Python Interpreter: translates and executes the program Results Every time
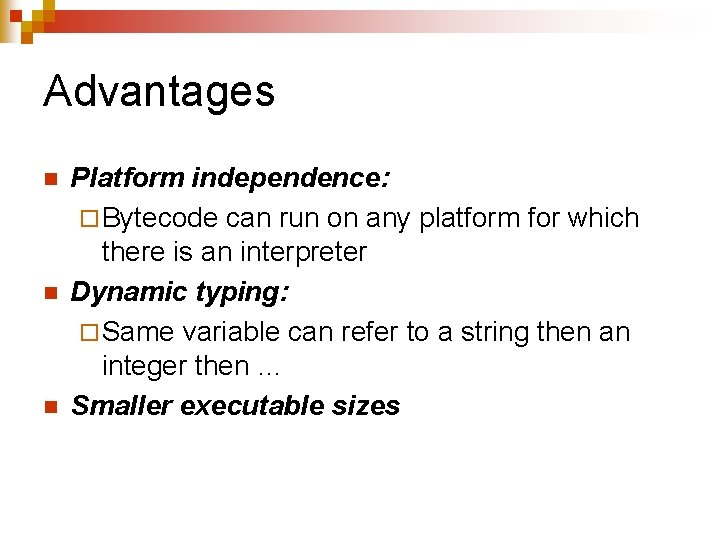
Advantages n n n Platform independence: ¨ Bytecode can run on any platform for which there is an interpreter Dynamic typing: ¨ Same variable can refer to a string then an integer then … Smaller executable sizes
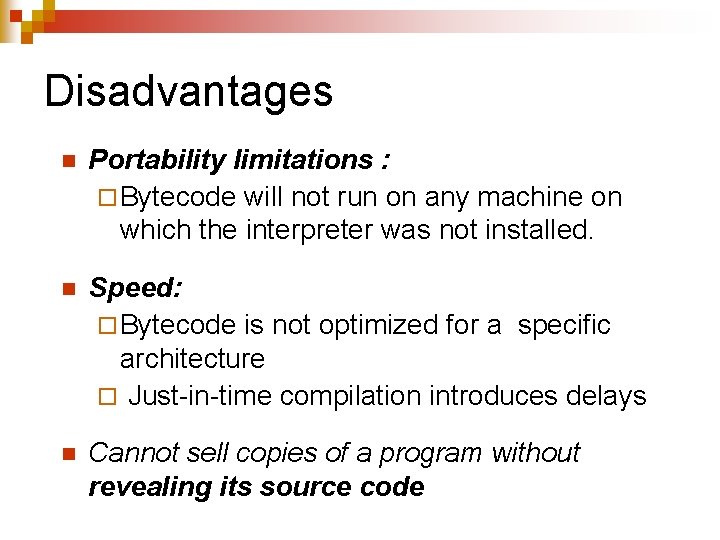
Disadvantages n Portability limitations : ¨ Bytecode will not run on any machine on which the interpreter was not installed. n Speed: ¨ Bytecode is not optimized for a specific architecture ¨ Just-in-time compilation introduces delays n Cannot sell copies of a program without revealing its source code
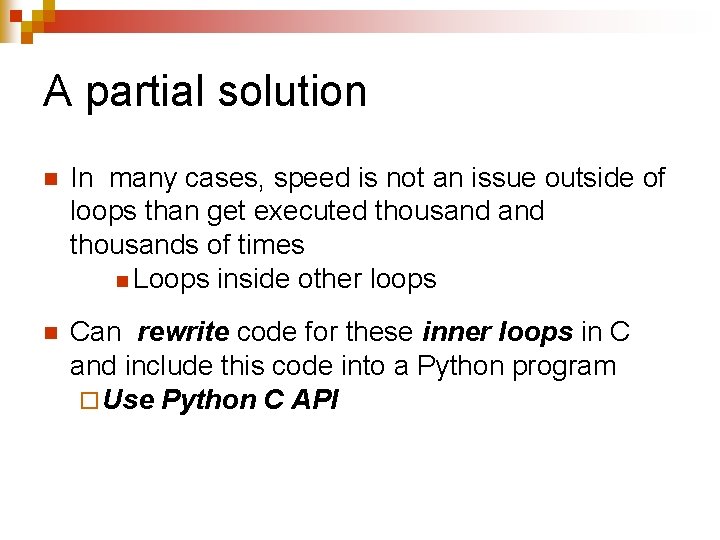
A partial solution n In many cases, speed is not an issue outside of loops than get executed thousands of times n Loops inside other loops n Can rewrite code for these inner loops in C and include this code into a Python program ¨ Use Python C API
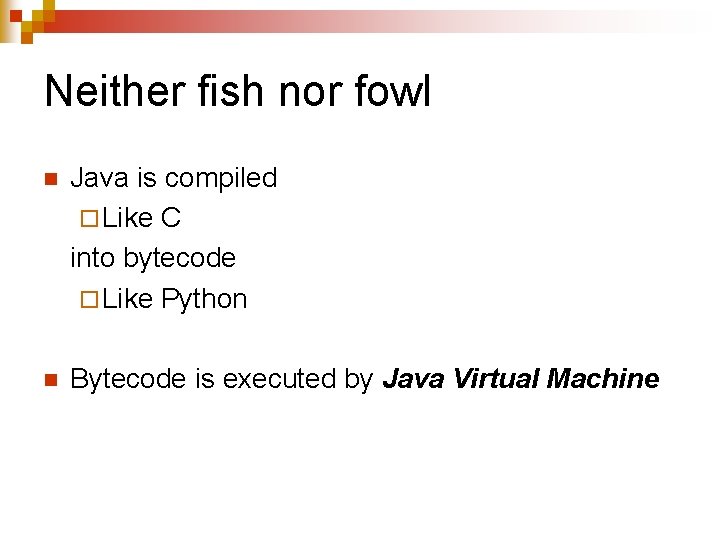
Neither fish nor fowl n Java is compiled ¨ Like C into bytecode ¨ Like Python n Bytecode is executed by Java Virtual Machine
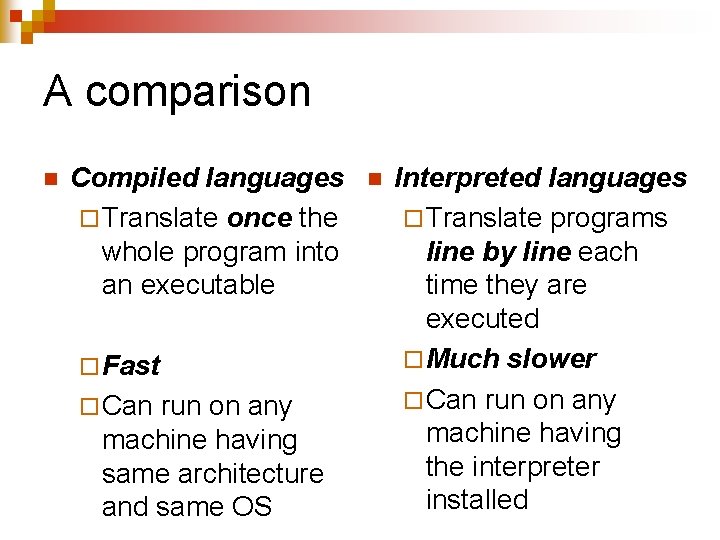
A comparison n Compiled languages ¨ Translate once the whole program into an executable ¨ Fast ¨ Can run on any machine having same architecture and same OS n Interpreted languages ¨ Translate programs line by line each time they are executed ¨ Much slower ¨ Can run on any machine having the interpreter installed
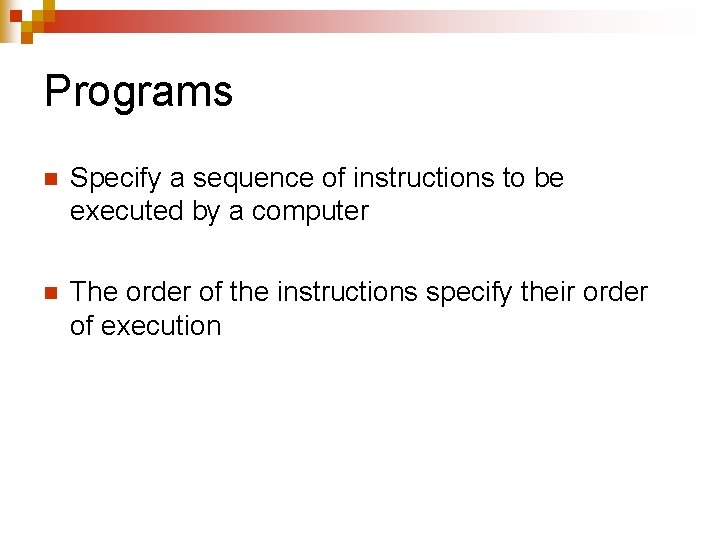
Programs n Specify a sequence of instructions to be executed by a computer n The order of the instructions specify their order of execution
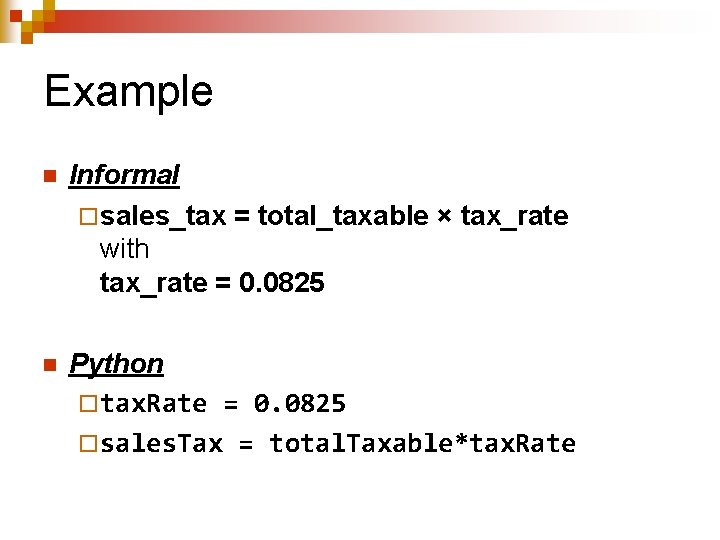
Example n Informal ¨ sales_tax = total_taxable × tax_rate with tax_rate = 0. 0825 n Python ¨ tax. Rate = 0. 0825 ¨ sales. Tax = total. Taxable*tax. Rate
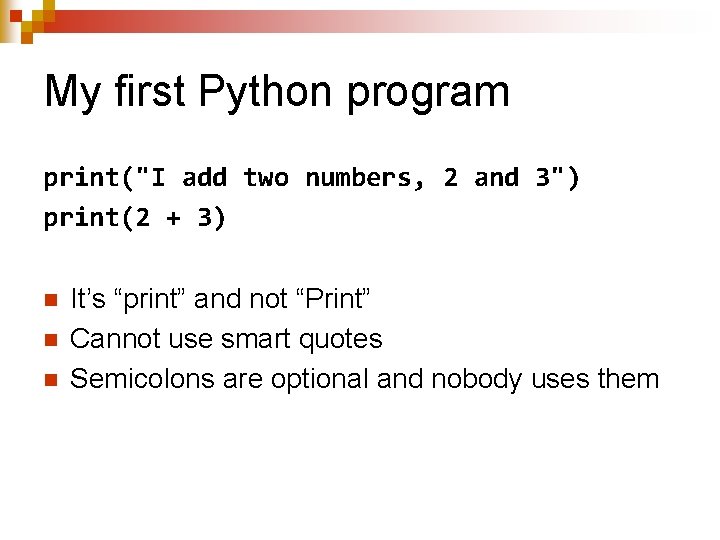
My first Python program print("I add two numbers, 2 and 3") print(2 + 3) n n n It’s “print” and not “Print” Cannot use smart quotes Semicolons are optional and nobody uses them
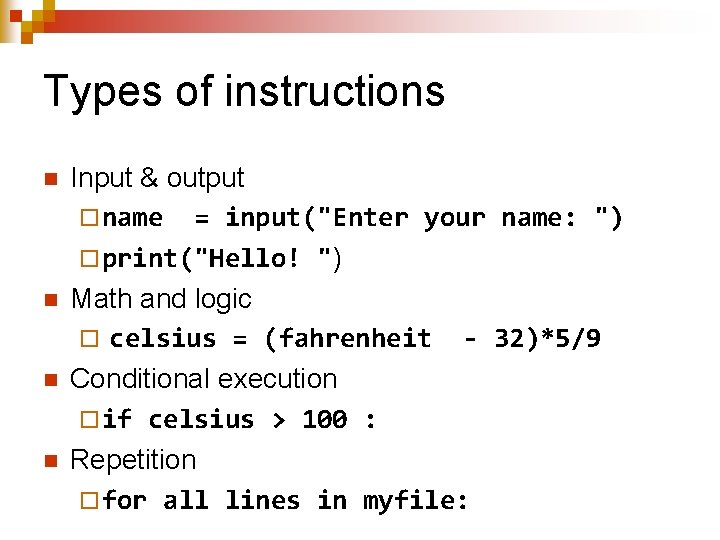
Types of instructions n n Input & output ¨ name = input("Enter your name: ") ¨ print("Hello! ") Math and logic ¨ celsius = (fahrenheit - 32)*5/9 Conditional execution ¨ if celsius > 100 : Repetition ¨ for all lines in myfile:
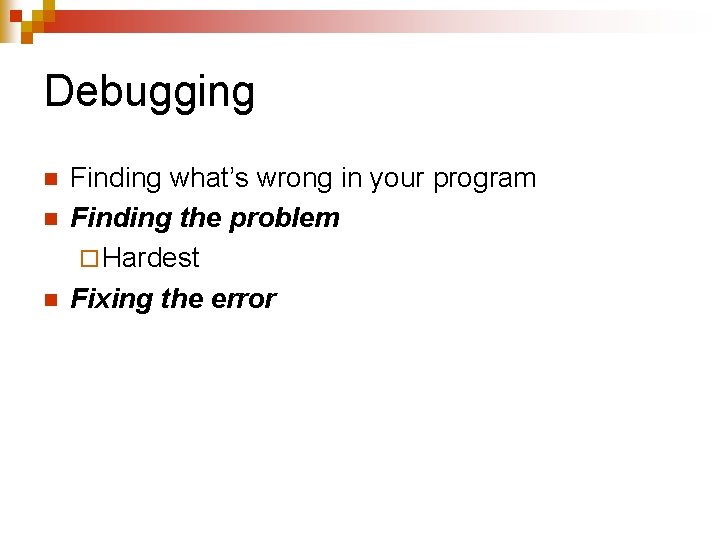
Debugging n n n Finding what’s wrong in your program Finding the problem ¨ Hardest Fixing the error
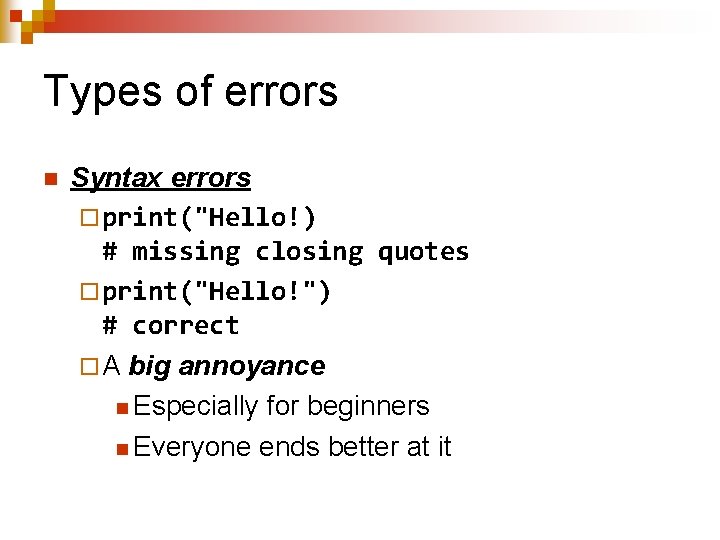
Types of errors n Syntax errors ¨ print("Hello!) # missing closing quotes ¨ print("Hello!") # correct ¨ A big annoyance n Especially for beginners n Everyone ends better at it
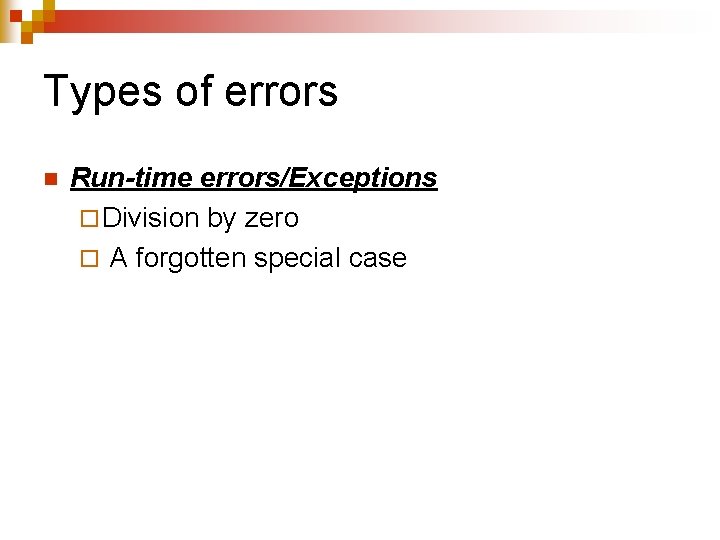
Types of errors n Run-time errors/Exceptions ¨ Division by zero ¨ A forgotten special case
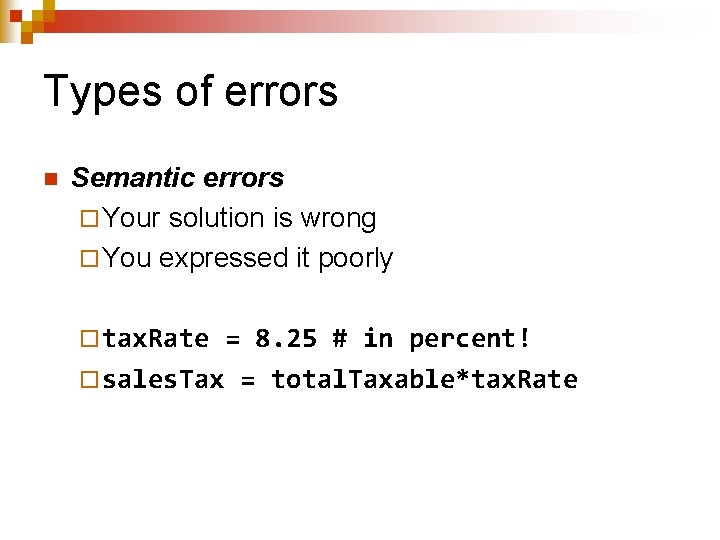
Types of errors n Semantic errors ¨ Your solution is wrong ¨ You expressed it poorly ¨ tax. Rate = 8. 25 # in percent! ¨ sales. Tax = total. Taxable*tax. Rate
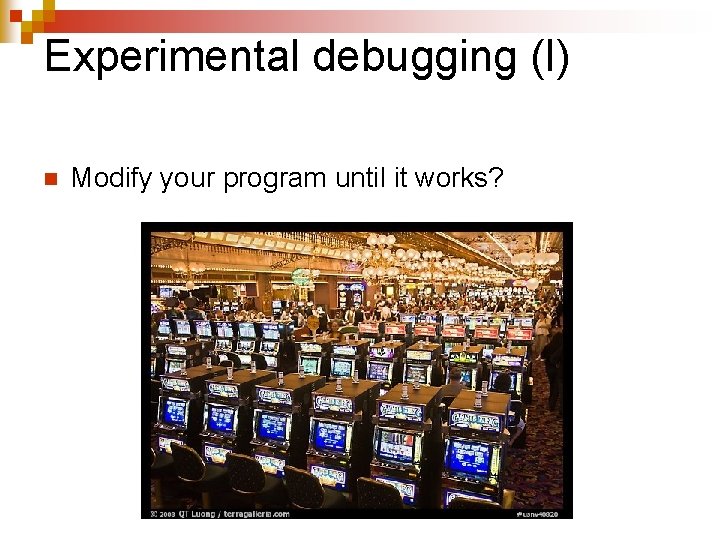
Experimental debugging (I) n Modify your program until it works?
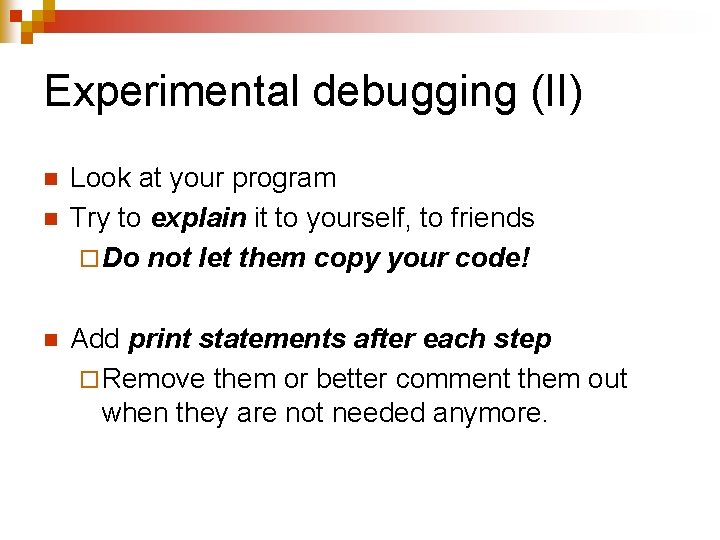
Experimental debugging (II) n n n Look at your program Try to explain it to yourself, to friends ¨ Do not let them copy your code! Add print statements after each step ¨ Remove them or better comment them out when they are not needed anymore.
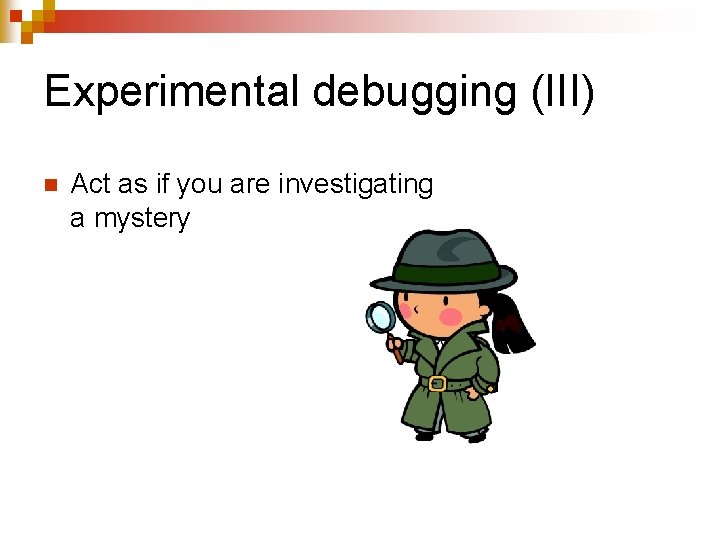
Experimental debugging (III) n Act as if you are investigating a mystery
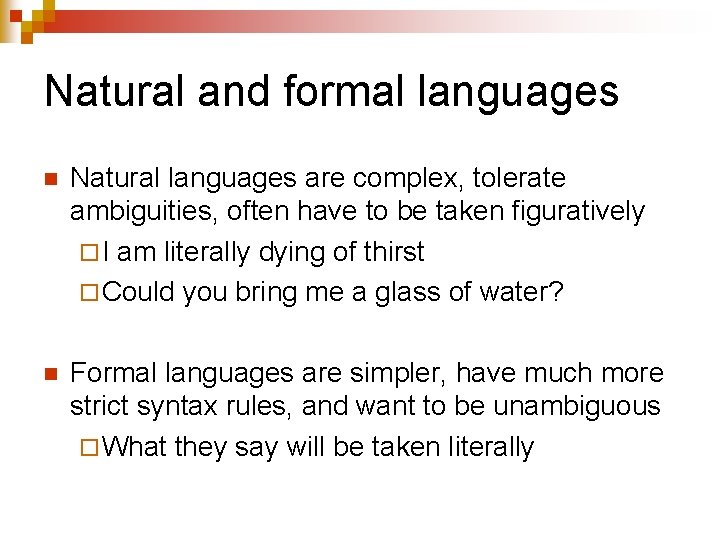
Natural and formal languages n Natural languages are complex, tolerate ambiguities, often have to be taken figuratively ¨ I am literally dying of thirst ¨ Could you bring me a glass of water? n Formal languages are simpler, have much more strict syntax rules, and want to be unambiguous ¨ What they say will be taken literally
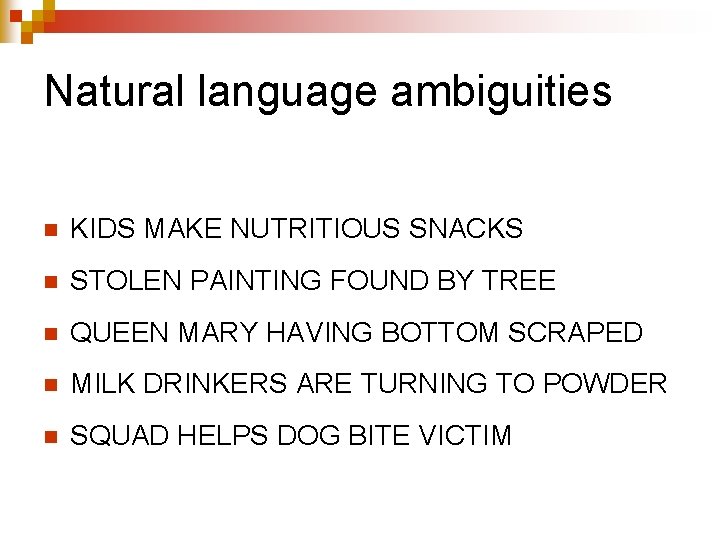
Natural language ambiguities n KIDS MAKE NUTRITIOUS SNACKS n STOLEN PAINTING FOUND BY TREE n QUEEN MARY HAVING BOTTOM SCRAPED n MILK DRINKERS ARE TURNING TO POWDER n SQUAD HELPS DOG BITE VICTIM
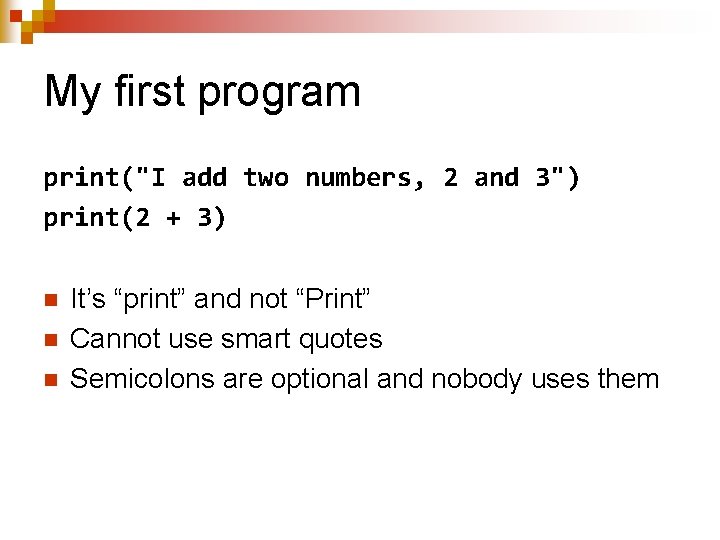
My first program print("I add two numbers, 2 and 3") print(2 + 3) n n n It’s “print” and not “Print” Cannot use smart quotes Semicolons are optional and nobody uses them
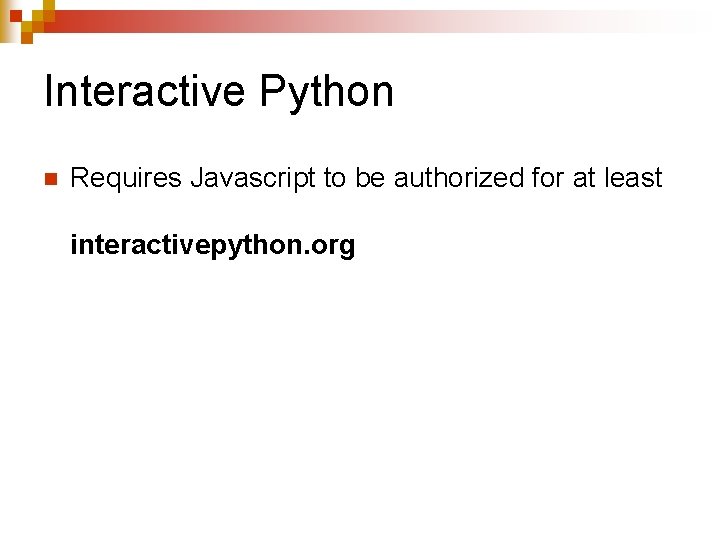
Interactive Python n Requires Javascript to be authorized for at least interactivepython. org
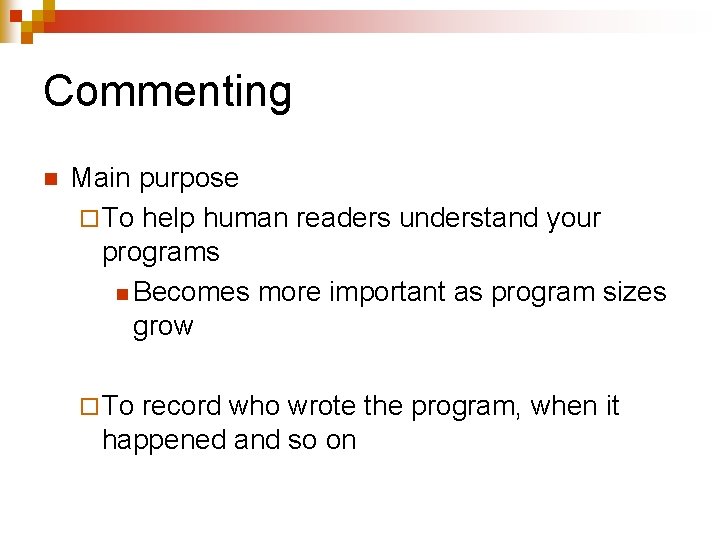
Commenting n Main purpose ¨ To help human readers understand your programs n Becomes more important as program sizes grow ¨ To record who wrote the program, when it happened and so on
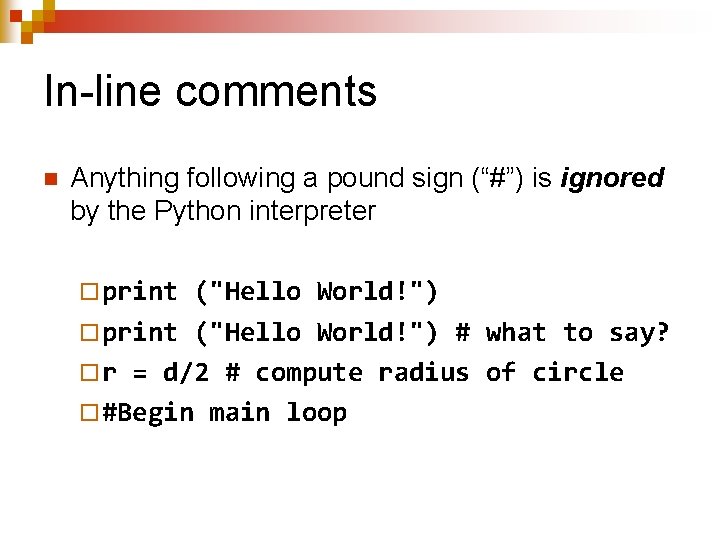
In-line comments n Anything following a pound sign (“#”) is ignored by the Python interpreter ¨ print ("Hello World!") # what to say? ¨ r = d/2 # compute radius of circle ¨ #Begin main loop
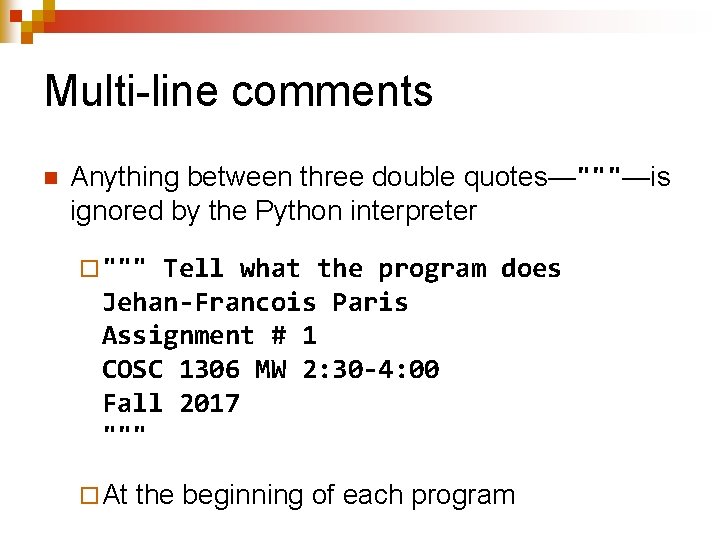
Multi-line comments n Anything between three double quotes—"""—is ignored by the Python interpreter ¨ """ Tell what the program does Jehan-Francois Paris Assignment # 1 COSC 1306 MW 2: 30 -4: 00 Fall 2017 """ ¨ At the beginning of each program
Cosc 1306
Cosc 1306
Cosc 1306
Ssd 1306
Music 1306
American computer science league practice problems
Python programming an introduction to computer science
That's my favorite
Cosc 4p61
Cosc 4p42
Cosc 3p91
Cosc 4368
Cosc 4p41
Cosc 4p41
Cosc
Cosc 3340
Cosc 320
Cosc parameters
Cosc 2p12
Adt functional programming
Cosc 3340
Cosc 3p92
Cosc 121
Cosc 1p02
Cosc 2p12
Cosc 3p92
1 bit alu
Cosc 121
Cosc 121
Trap vector table lc3
Cosc 121
Cosc -cos d
Cosc 3p94
Cosc 101
Cosc 3340
Cosc 3340
Divisun 2000 ie pris
Tiva indications
Sio helse fastlege
Preisanalyse