COSC 1306COMPUTER SCIENCE AND PROGRAMMING PYTHON LISTS JehanFranois
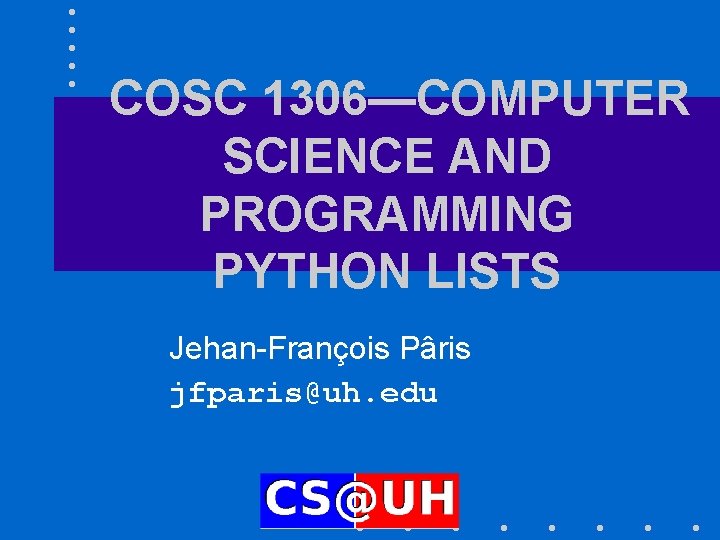
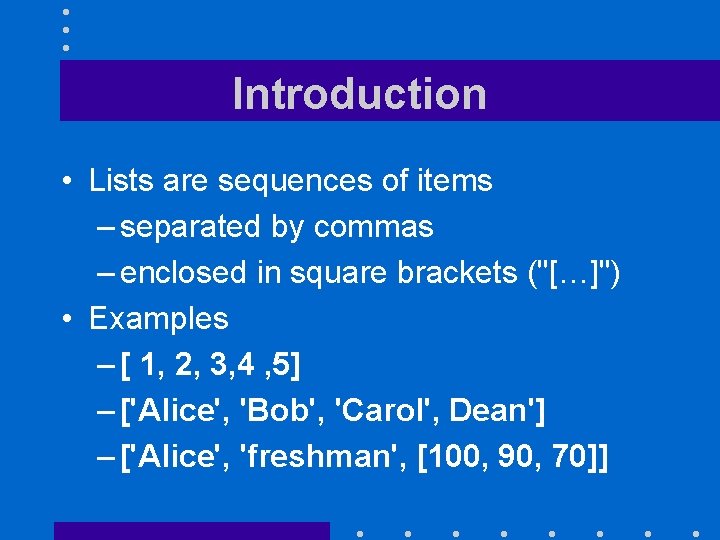
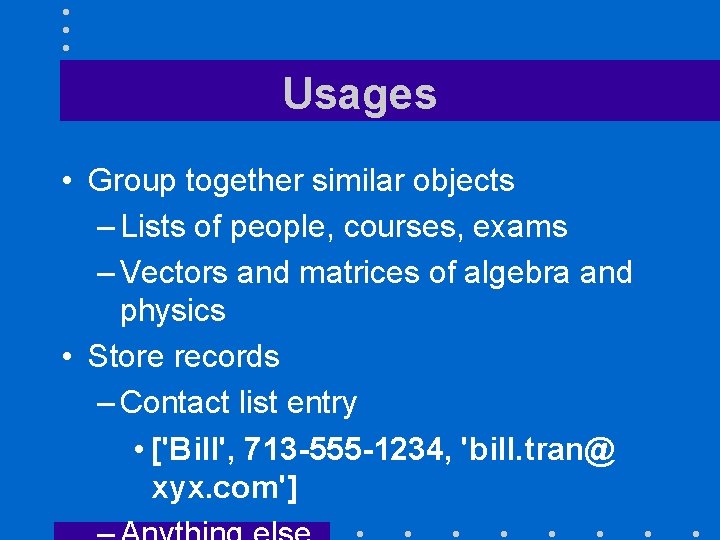
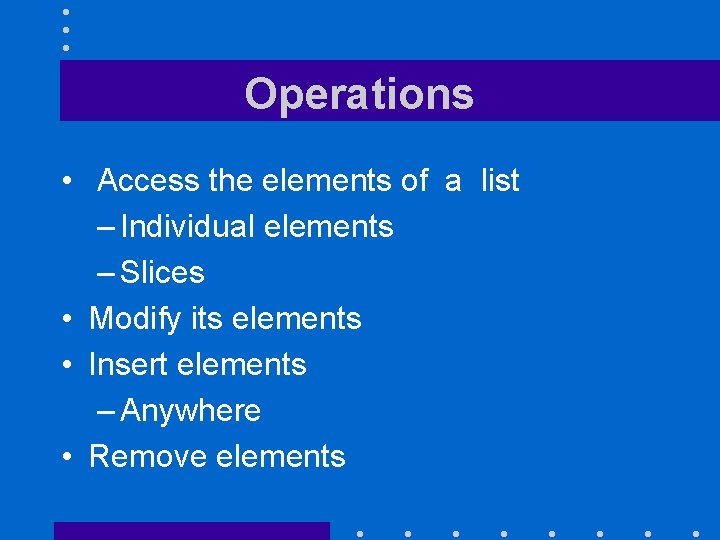
![Lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist [11, Lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist [11,](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-5.jpg)
![List slices • >>> mylist[0: 1] [11] – Two observations • A list slice List slices • >>> mylist[0: 1] [11] – Two observations • A list slice](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-6.jpg)
![More list slices • >>> mylist[0: 2] [11, 12] – Includes mylist[0] and mylist[1] More list slices • >>> mylist[0: 2] [11, 12] – Includes mylist[0] and mylist[1]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-7.jpg)
![More list slices • >>> mylist[-1: ] ['done'] • A list slice is a More list slices • >>> mylist[-1: ] ['done'] • A list slice is a](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-8.jpg)
![An example • a = [10, 20, 30, 40, 50] a designates the whole An example • a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-9.jpg)
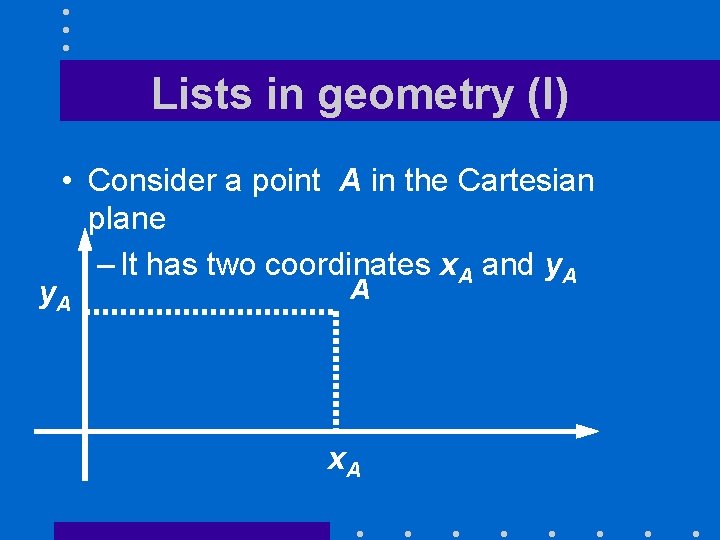
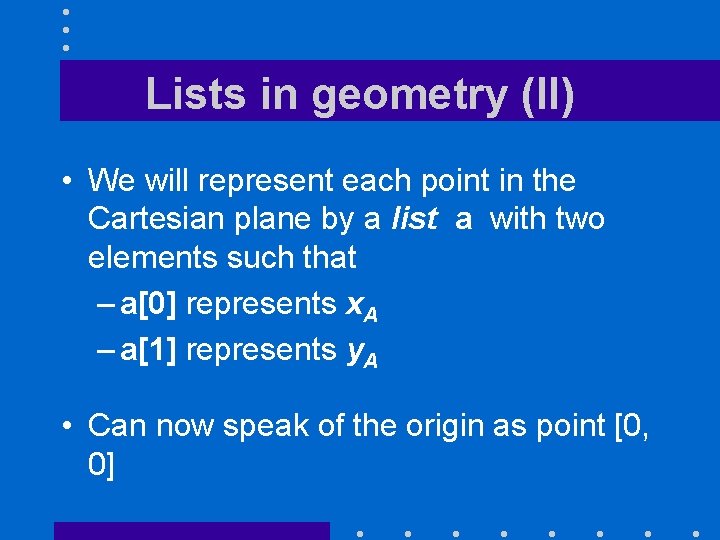
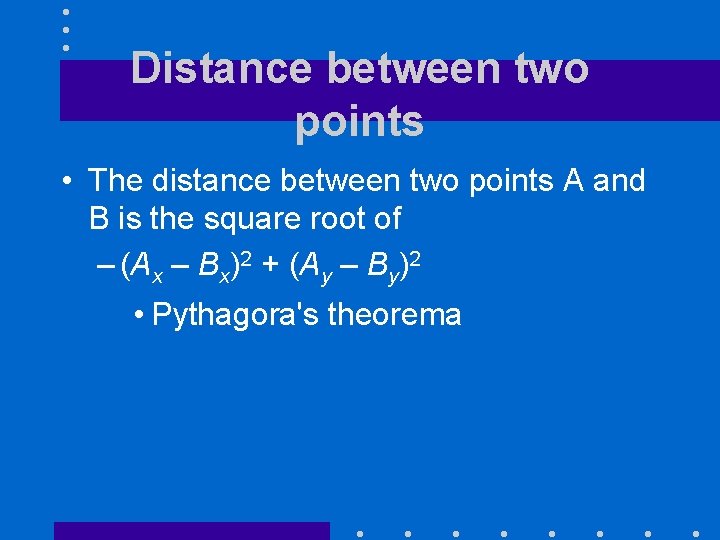
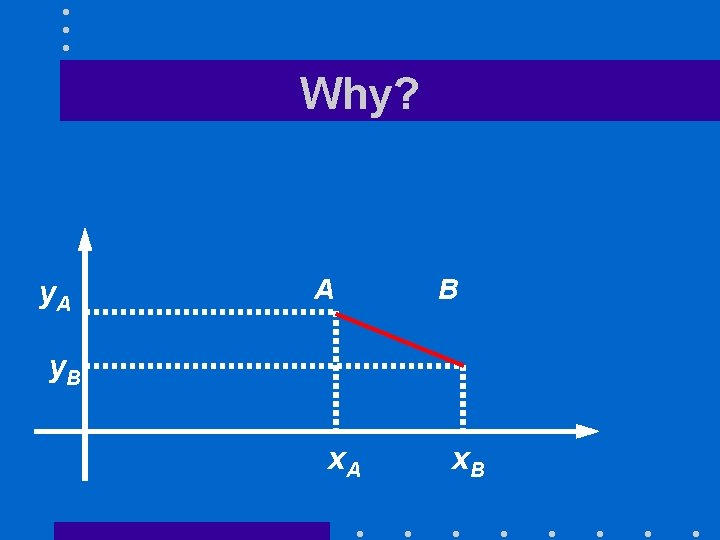
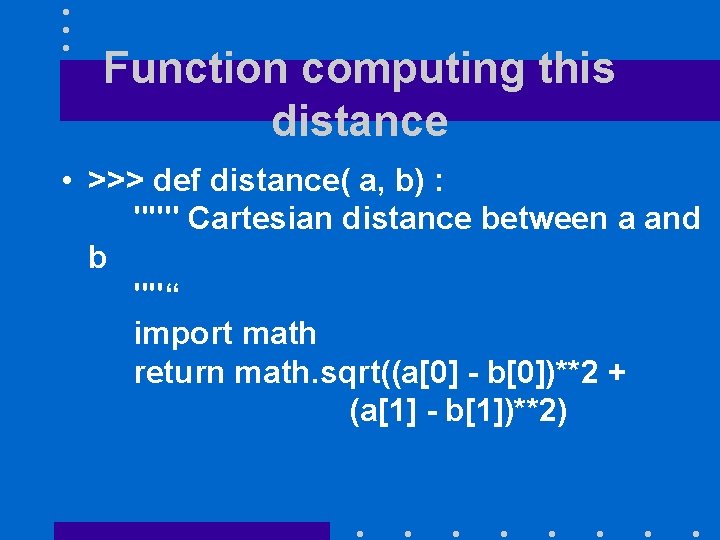
![Function computing this distance • >>> origin = [0, 0] • >>> a = Function computing this distance • >>> origin = [0, 0] • >>> a =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-15.jpg)
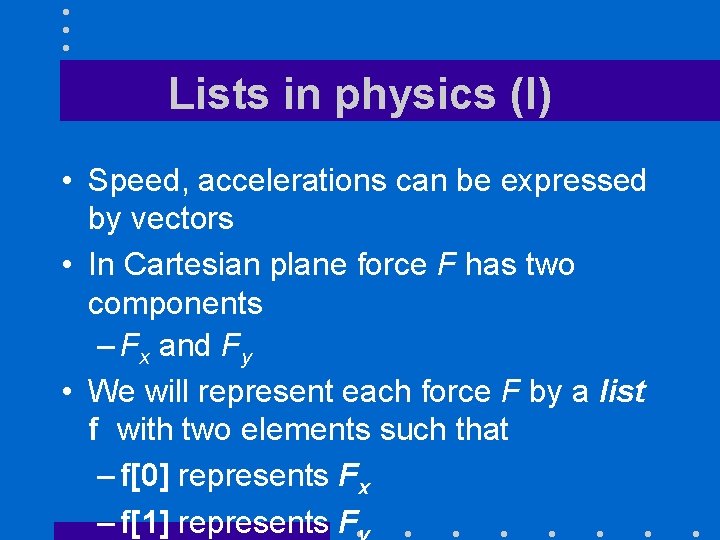
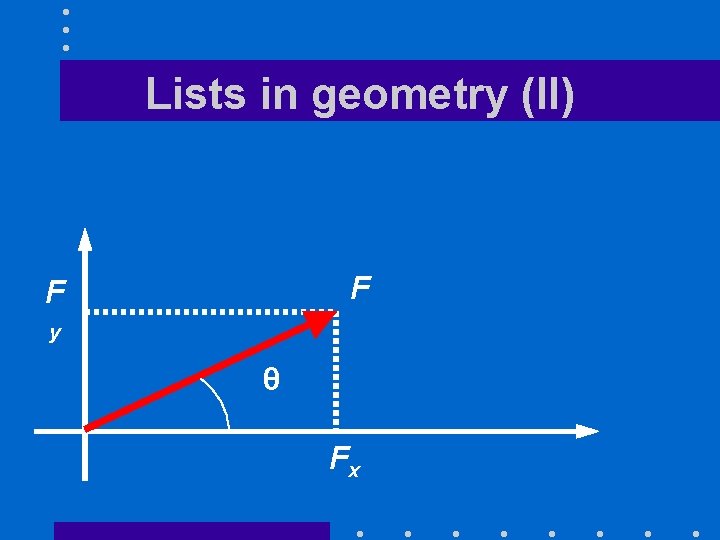
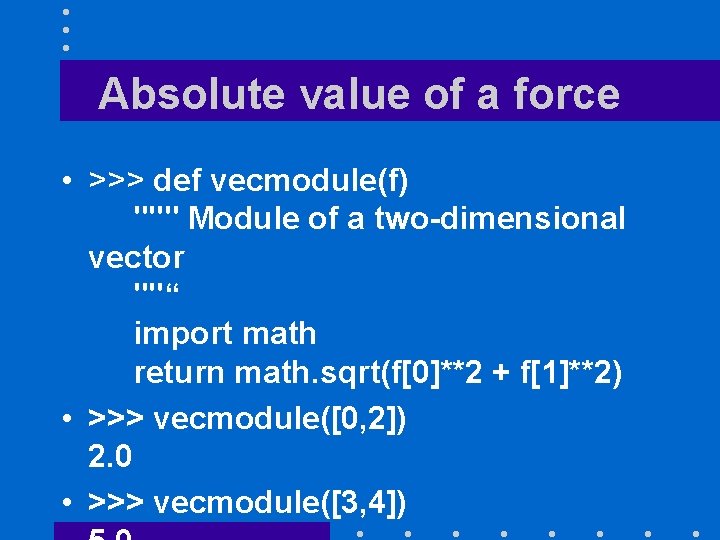
![Adding two vectors (I) • [0, 2] + [0, 3] [0, 2, 0, 3] Adding two vectors (I) • [0, 2] + [0, 3] [0, 2, 0, 3]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-19.jpg)
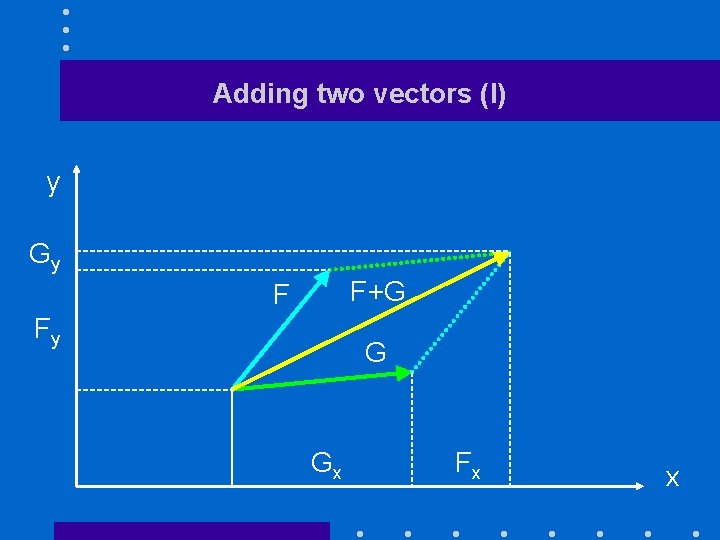
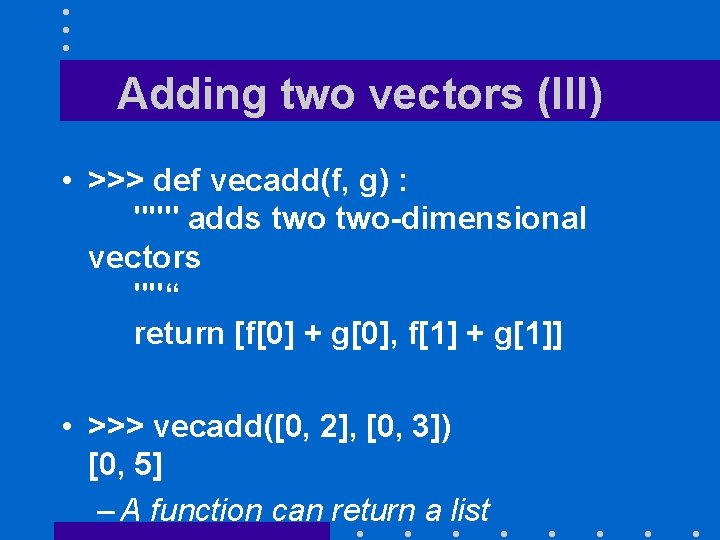
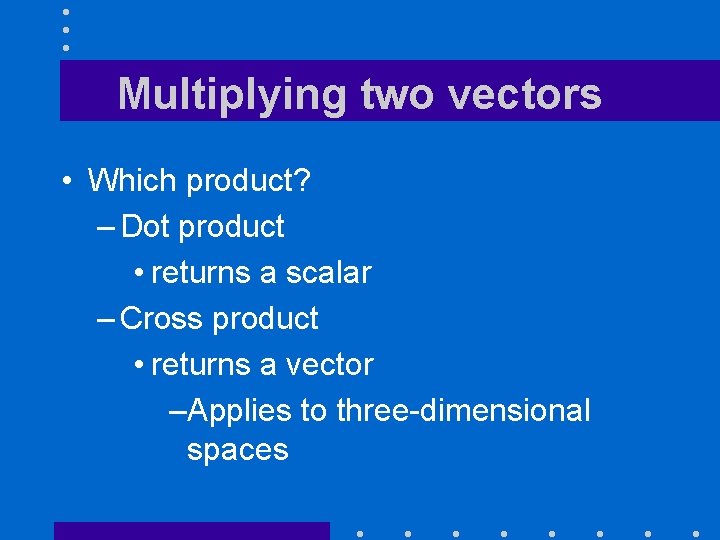
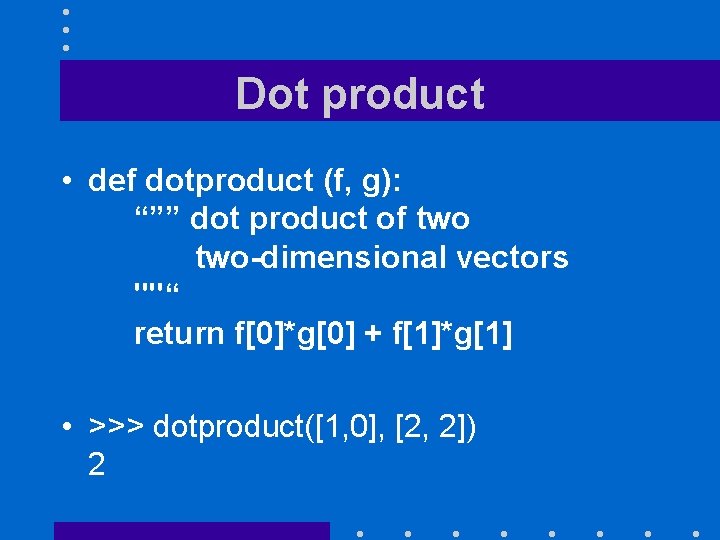
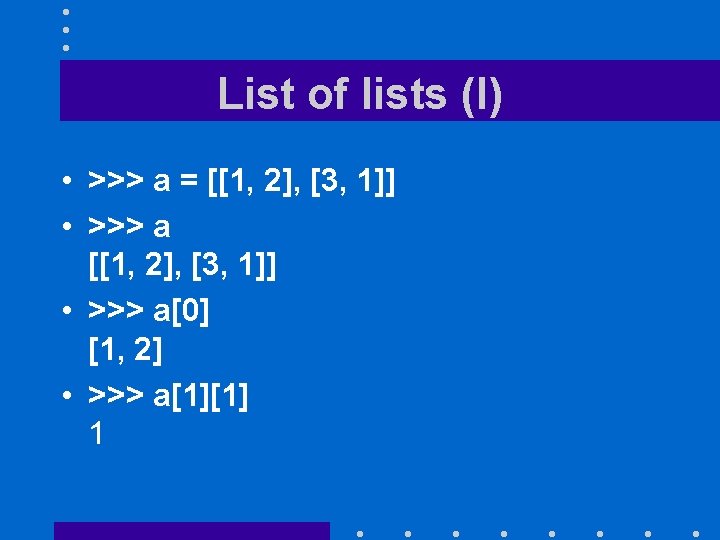
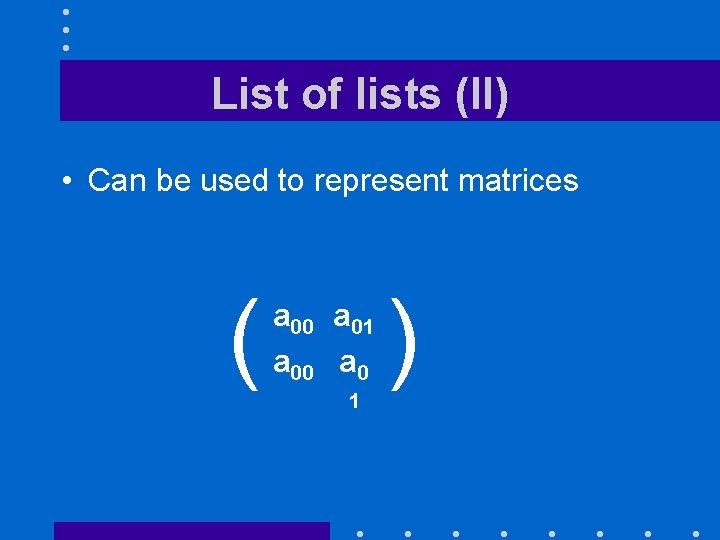
![Sorting lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist. Sorting lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist.](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-26.jpg)
![Sorting lists of strings • >>> namelist = ['Alice', 'Carol', 'Bob'] • >>> namelist. Sorting lists of strings • >>> namelist = ['Alice', 'Carol', 'Bob'] • >>> namelist.](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-27.jpg)
![Sorting lists of numbers • >>> newlist = [0, -1, +1, -2, +2] • Sorting lists of numbers • >>> newlist = [0, -1, +1, -2, +2] •](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-28.jpg)
![Sorting into a new list • >>> newlist = [0, -1, +1, -2, +2] Sorting into a new list • >>> newlist = [0, -1, +1, -2, +2]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-29.jpg)
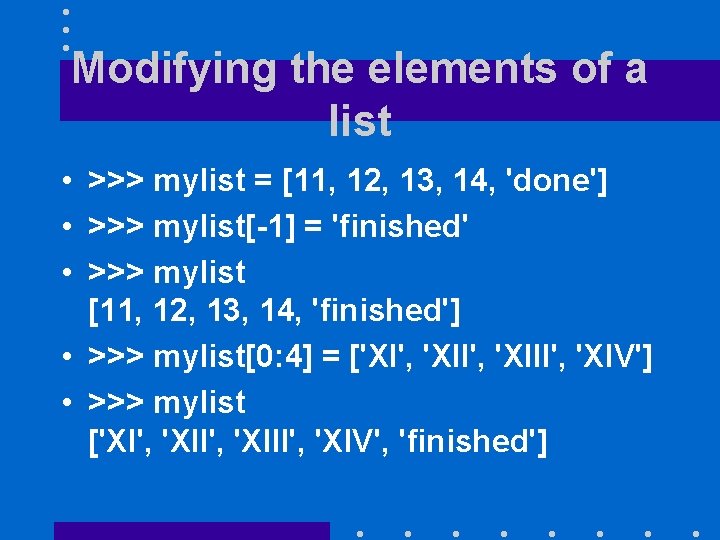
![Lists of lists (I) • >>> listoflists = [[17. 5, "1306"], [13, "6360"]] • Lists of lists (I) • >>> listoflists = [[17. 5, "1306"], [13, "6360"]] •](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-31.jpg)
![Lists of lists (II) • >>> listoflists[0][0] 13 • >>> listoflists[1][1] '1306' • >>> Lists of lists (II) • >>> listoflists[0][0] 13 • >>> listoflists[1][1] '1306' • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-32.jpg)
![Adding elements to a list • >>> mylist = [11, 12, 13, 14, 'finished'] Adding elements to a list • >>> mylist = [11, 12, 13, 14, 'finished']](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-33.jpg)
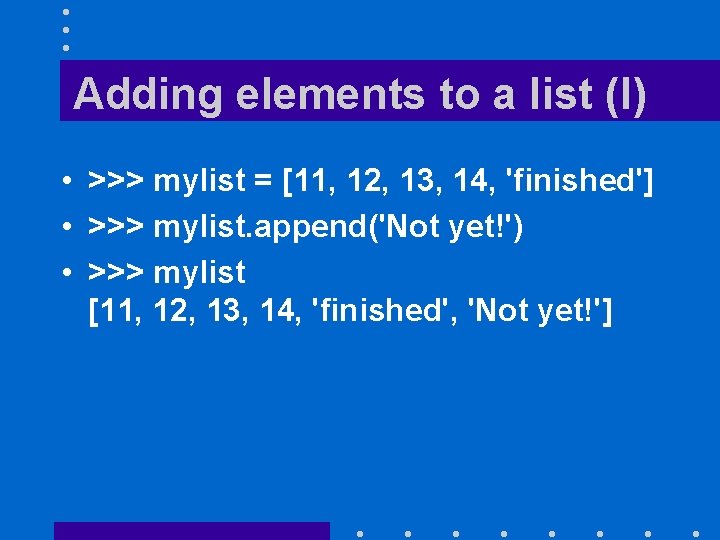
![Adding elements to a list (II) • >>> listoflists = [[13, '6360 quiz'], [17. Adding elements to a list (II) • >>> listoflists = [[13, '6360 quiz'], [17.](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-35.jpg)
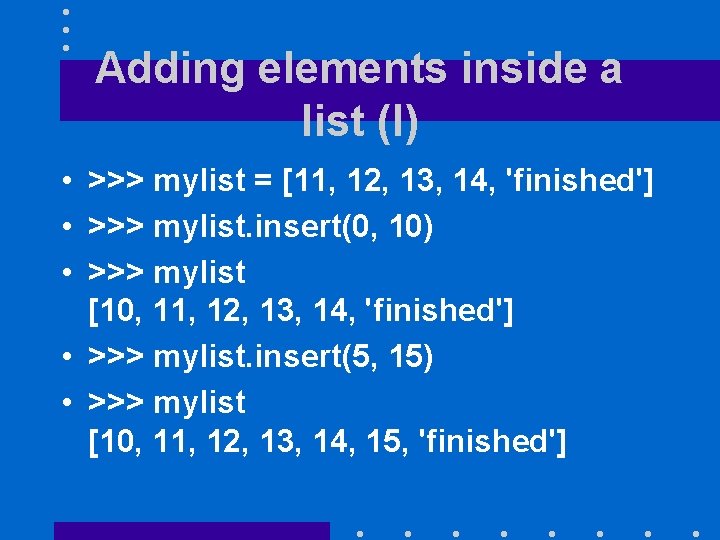
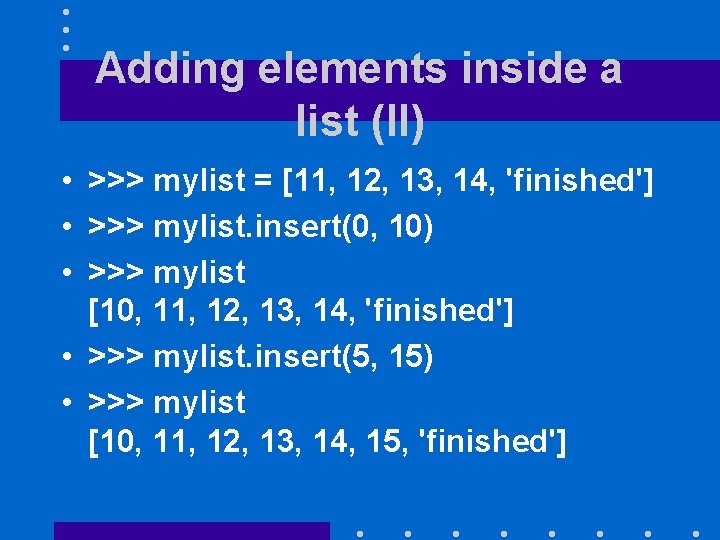
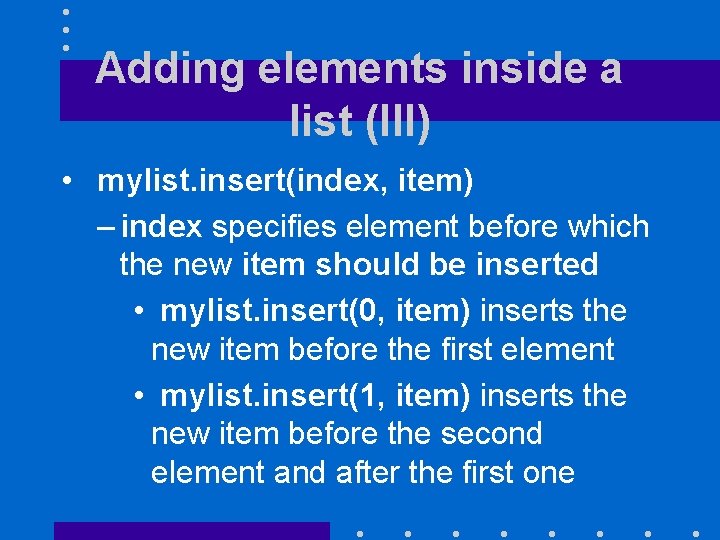
![Example (I) • a = [10, 20, 30, 40, 50] a designates the whole Example (I) • a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-39.jpg)
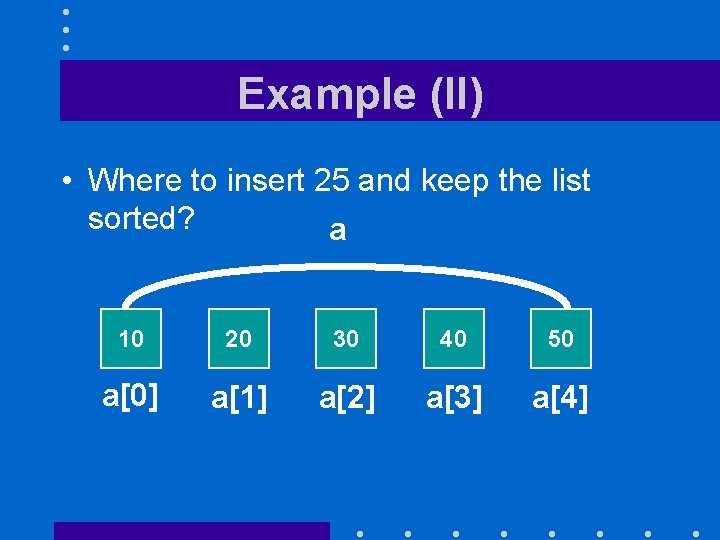
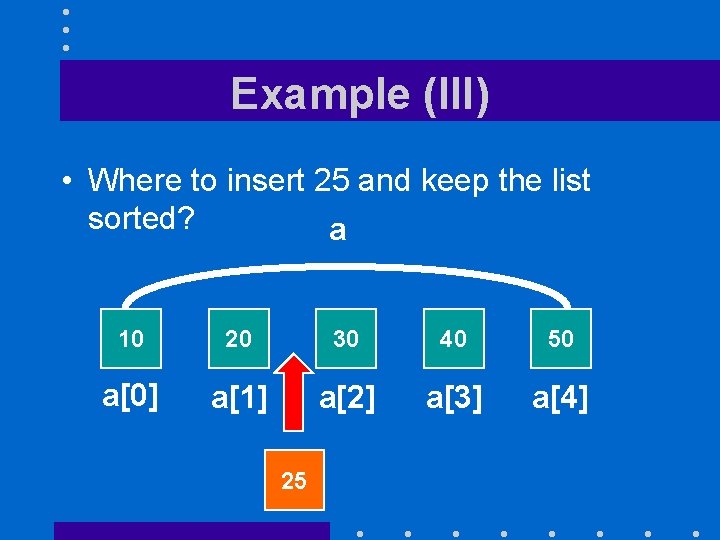
![Example (IV) • We do – a. insert(2, 25) • after a[1] and before Example (IV) • We do – a. insert(2, 25) • after a[1] and before](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-42.jpg)
![Let us check • >>> a = [10, 20, 30, 40, 50] • >>> Let us check • >>> a = [10, 20, 30, 40, 50] • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-43.jpg)
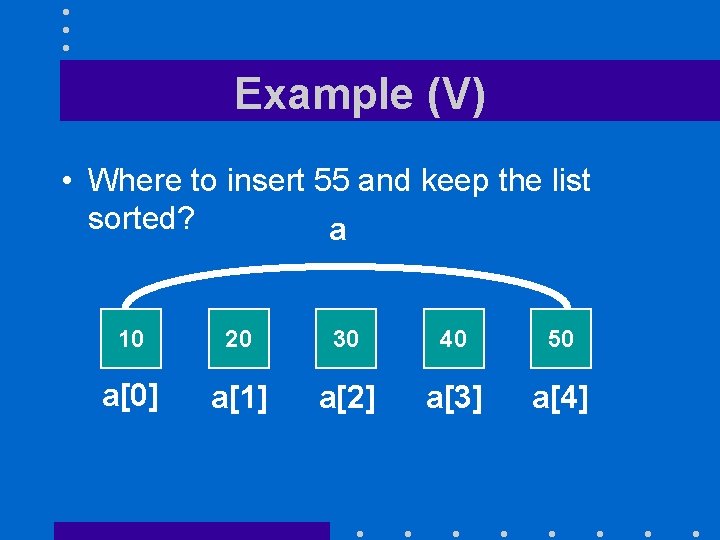
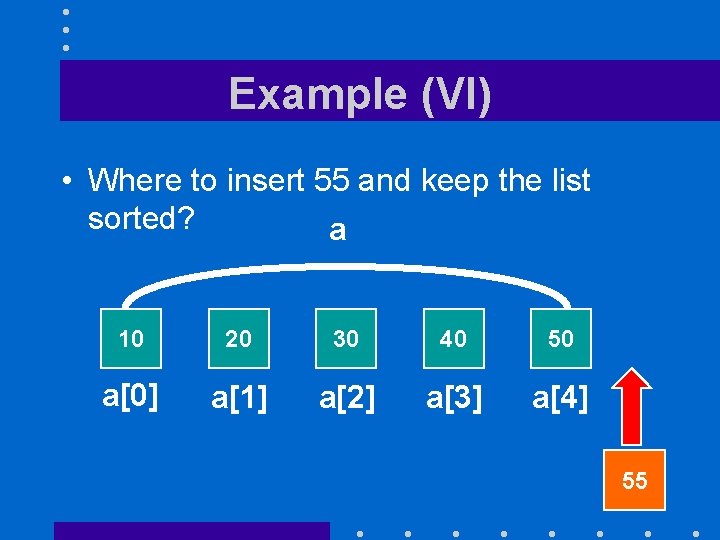
![Example (VII) • We must insert – After a[4] – Before no other element Example (VII) • We must insert – After a[4] – Before no other element](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-46.jpg)
![Let us check • >>> a = [10, 20, 30, 40, 50] • >>> Let us check • >>> a = [10, 20, 30, 40, 50] • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-47.jpg)
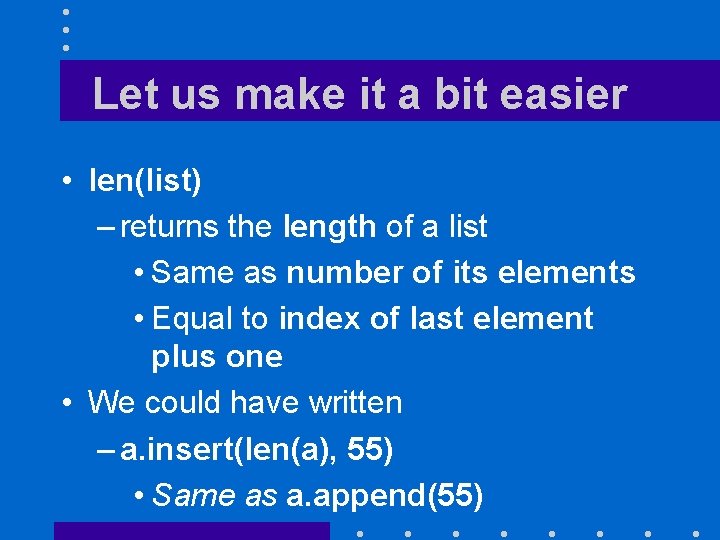
![Removing items • One by one • thislist. pop(i) – removes thislist[i] from thislist Removing items • One by one • thislist. pop(i) – removes thislist[i] from thislist](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-49.jpg)
![Examples (I) • >>> mylist = [11, 22, 33, 44, 55, 66] • >>> Examples (I) • >>> mylist = [11, 22, 33, 44, 55, 66] • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-50.jpg)
![Examples (II) • >>> mylist. pop() 66 • >>> mylist [22, 33, 44, 55] Examples (II) • >>> mylist. pop() 66 • >>> mylist [22, 33, 44, 55]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-51.jpg)
![Sum: one more useful function • >>> list = [10, 20] • >>> sum(list) Sum: one more useful function • >>> list = [10, 20] • >>> sum(list)](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-52.jpg)
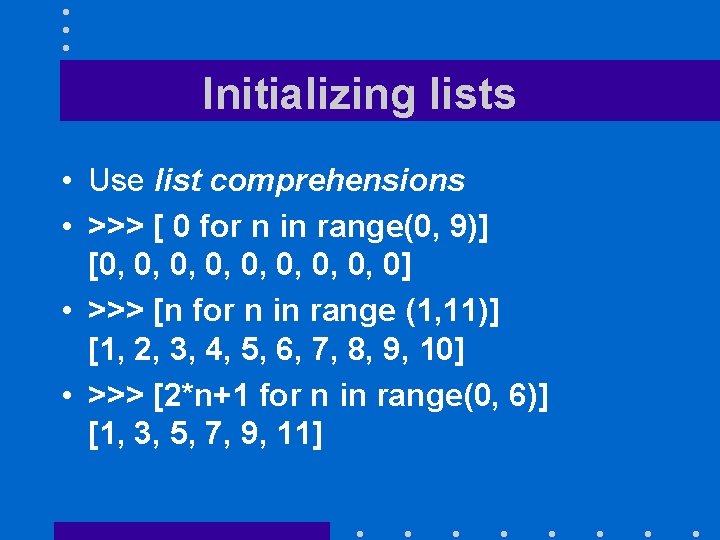
![Warning! • The for n clause is essential • [0 in range(0, 10)] [True] Warning! • The for n clause is essential • [0 in range(0, 10)] [True]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-54.jpg)
![More comprehensions • >>> [ c for c in 'COSC 1306'] ['C', 'O', 'S', More comprehensions • >>> [ c for c in 'COSC 1306'] ['C', 'O', 'S',](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-55.jpg)
![An equivalence • [2*n+1 for n in range(0, 6)] [1, 3, 5, 7, 9, An equivalence • [2*n+1 for n in range(0, 6)] [1, 3, 5, 7, 9,](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-56.jpg)
![Filtered comprehensions • >>> a = [11, 22, 33, 44, 55] • >>> b Filtered comprehensions • >>> a = [11, 22, 33, 44, 55] • >>> b](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-57.jpg)
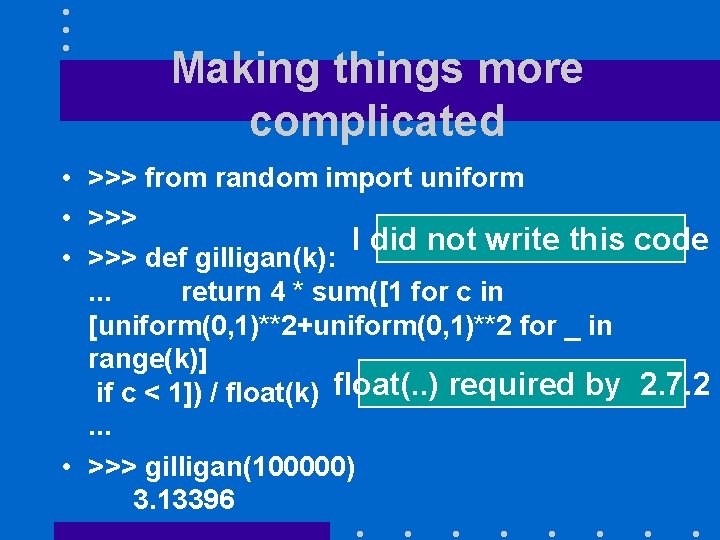
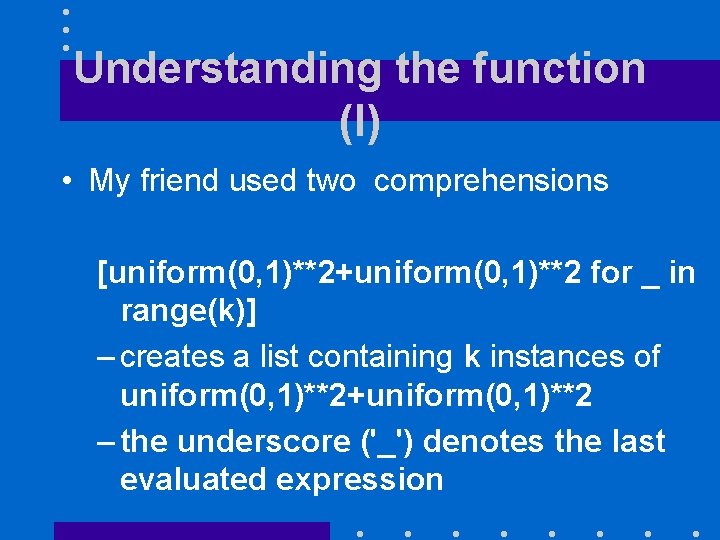
![Understanding the function (II) [1 for c in […] if c < 1] – Understanding the function (II) [1 for c in […] if c < 1] –](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-60.jpg)
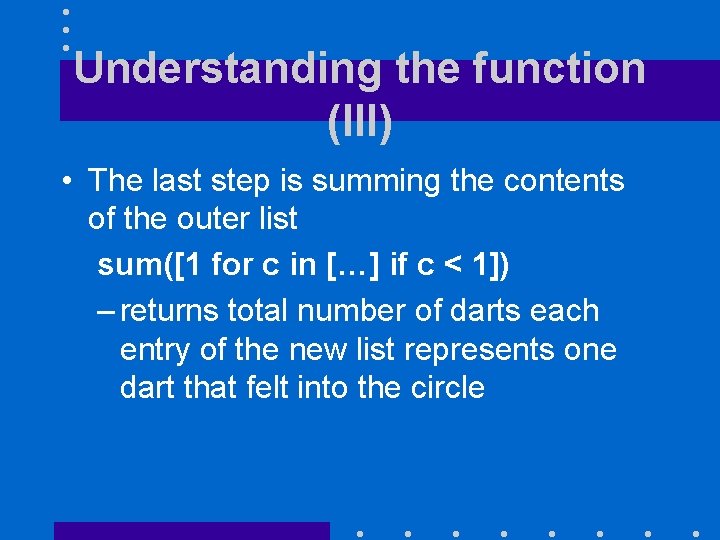
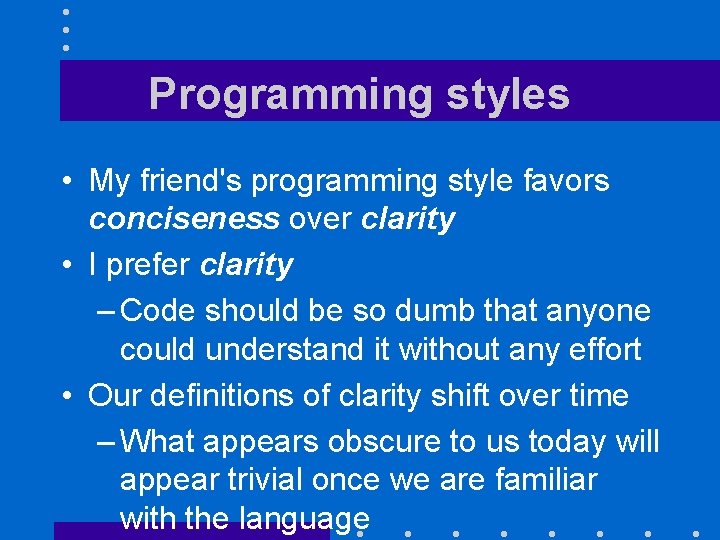
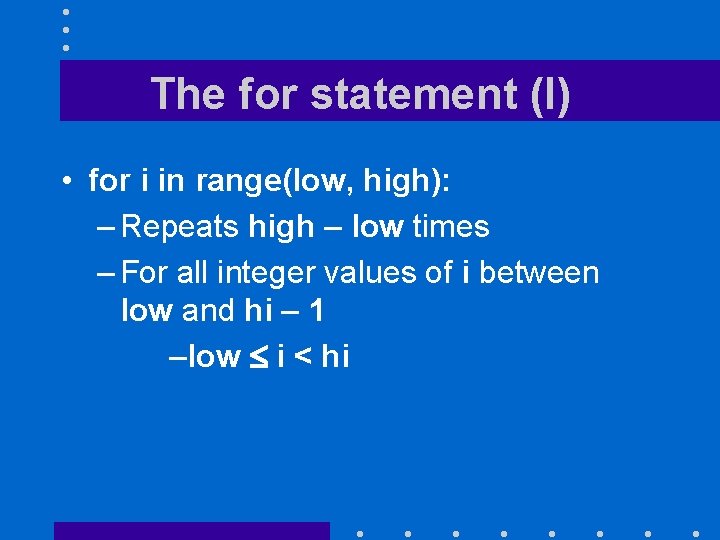
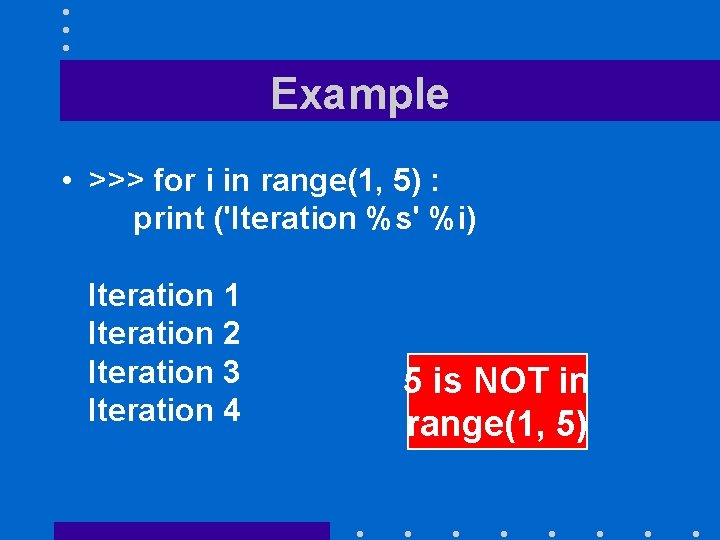
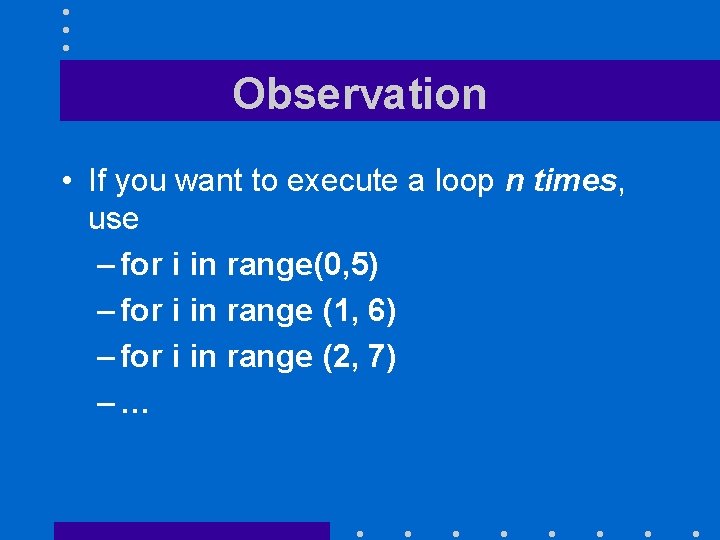
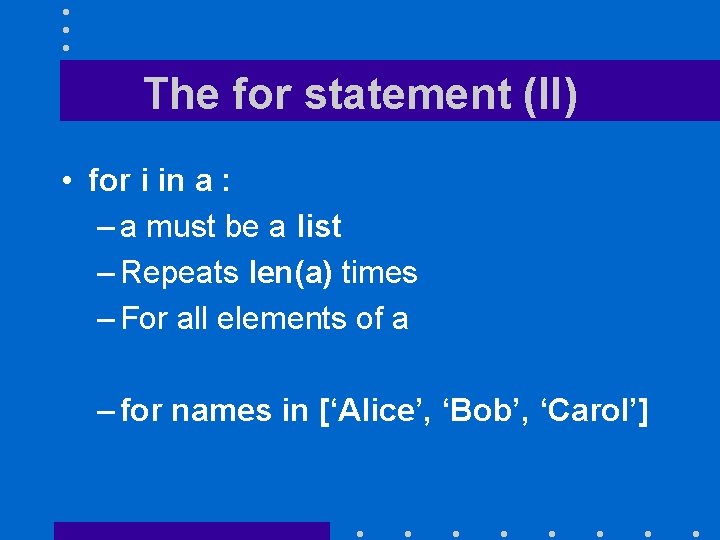
![Example (I) • >>> names = ['Alice', 'Bob', 'Carol'] • >>> for name in Example (I) • >>> names = ['Alice', 'Bob', 'Carol'] • >>> for name in](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-67.jpg)
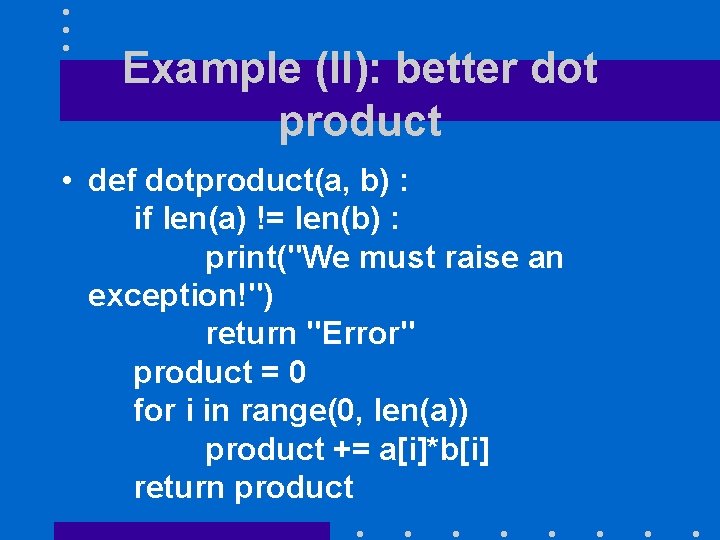
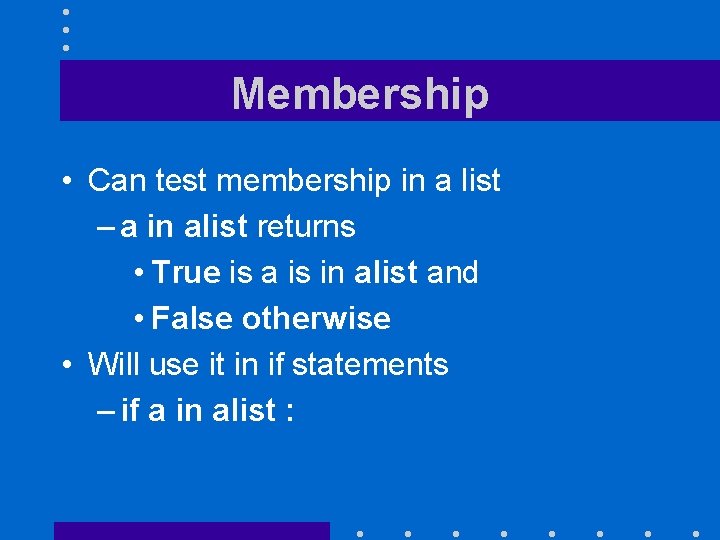
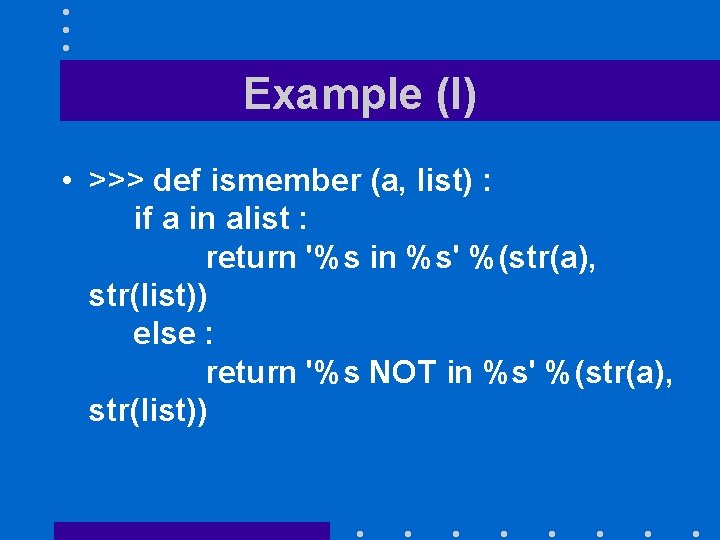
![Example (II) • >>> ismember('Bob', ['Alice', 'Carol']) "Bob NOT in ['Alice', 'Carol']" • >>> Example (II) • >>> ismember('Bob', ['Alice', 'Carol']) "Bob NOT in ['Alice', 'Carol']" • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-71.jpg)
![Copying/Saving a list • >>> a = ["Alice", "Bob", "Carol"] >>> saved = a Copying/Saving a list • >>> a = ["Alice", "Bob", "Carol"] >>> saved = a](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-72.jpg)
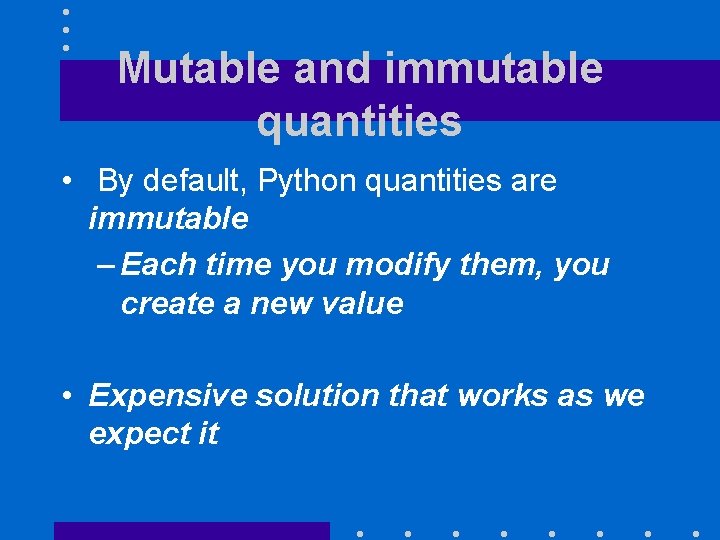
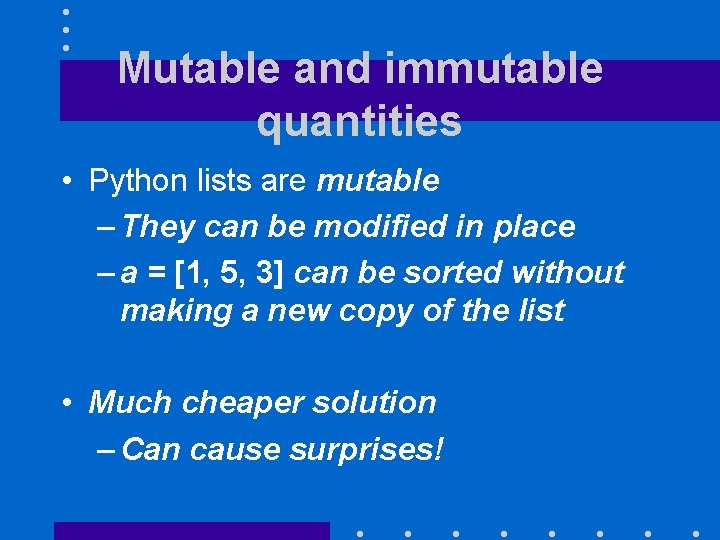
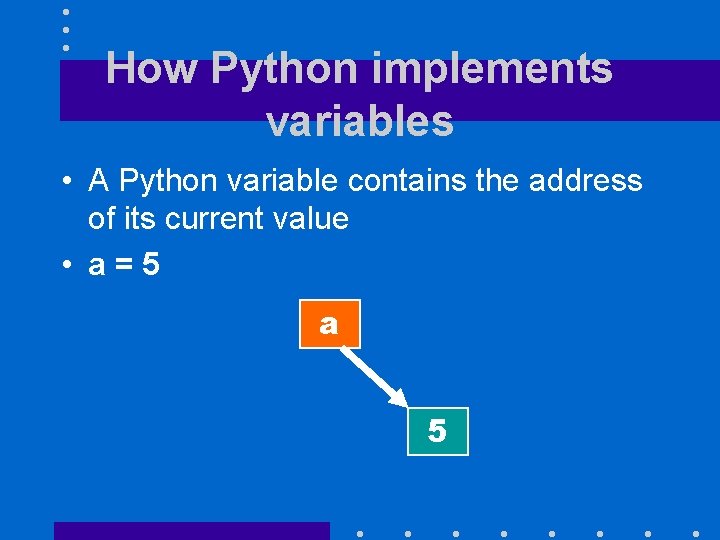
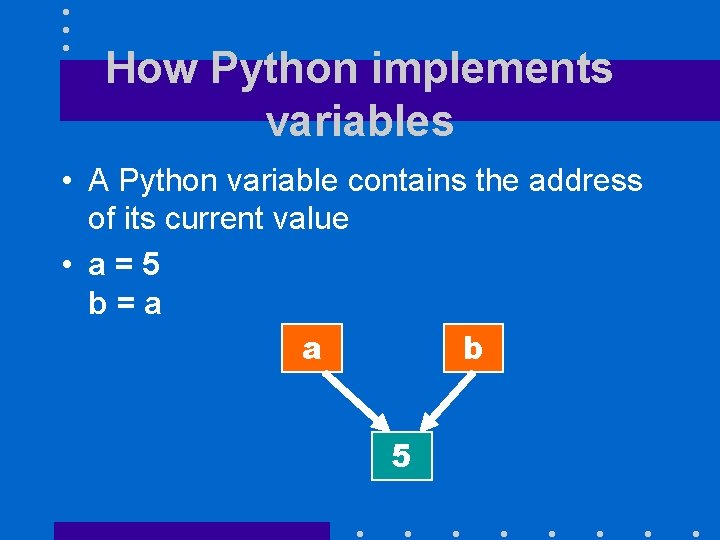
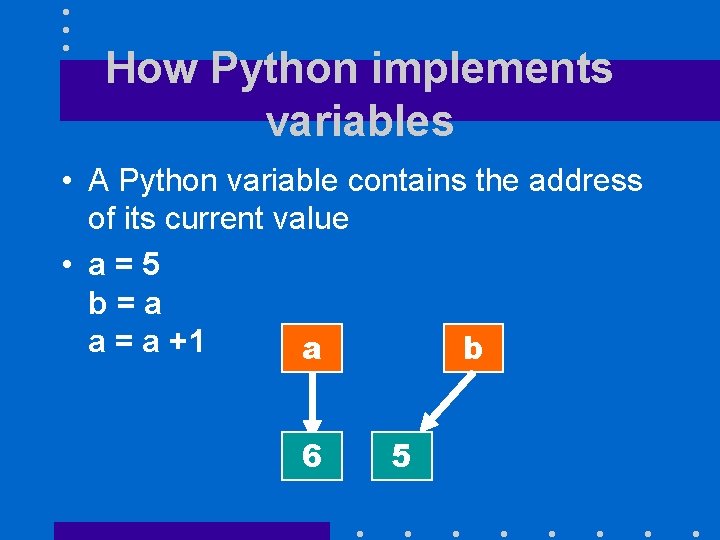
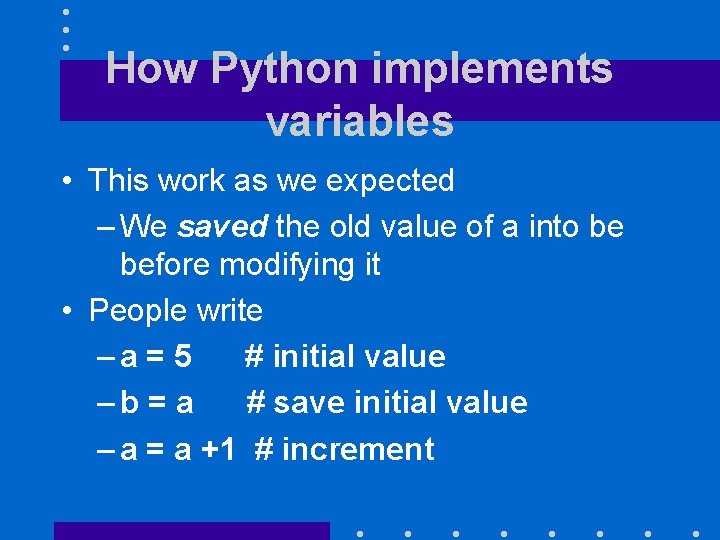
![A big surprise • >>> a = [11, 33, 22] • >>> b = A big surprise • >>> a = [11, 33, 22] • >>> b =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-79.jpg)
![What happened (I) • >>> a = [11, 33, 22] • >>> b = What happened (I) • >>> a = [11, 33, 22] • >>> b =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-80.jpg)
![What happened (II) • >>> a = [11, 33, 22] • >>> b = What happened (II) • >>> a = [11, 33, 22] • >>> b =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-81.jpg)
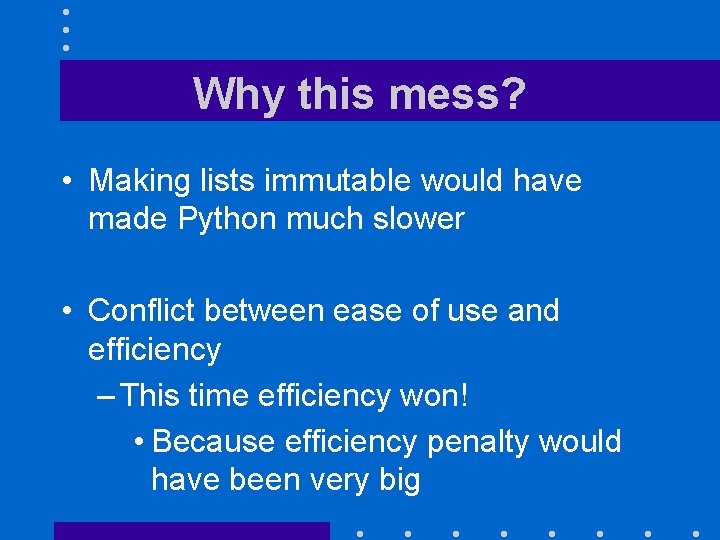
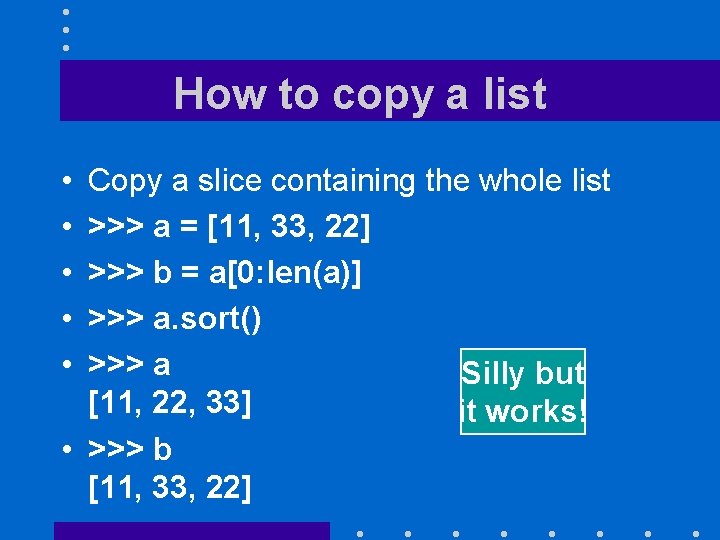
- Slides: 83
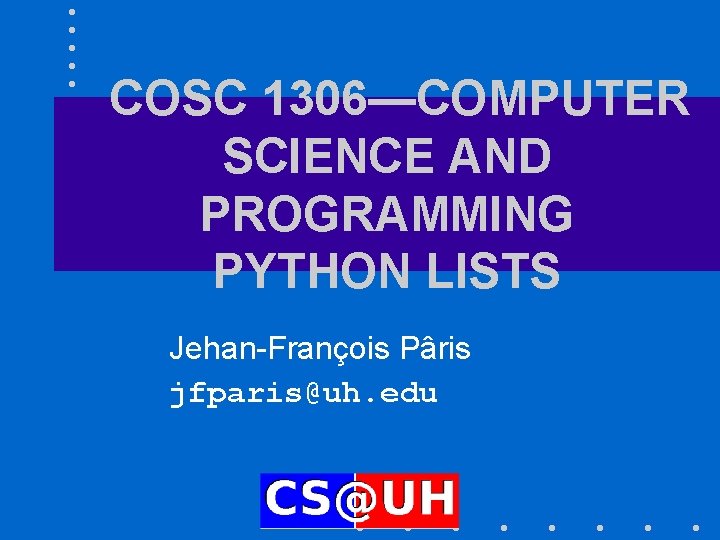
COSC 1306—COMPUTER SCIENCE AND PROGRAMMING PYTHON LISTS Jehan-François Pâris jfparis@uh. edu
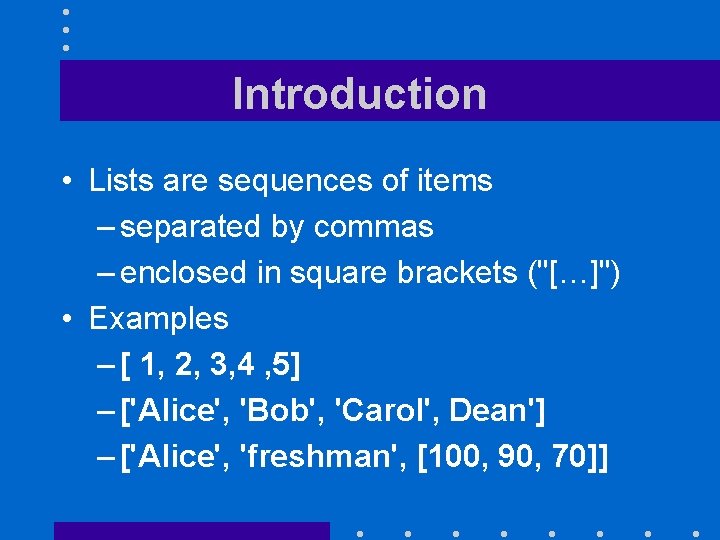
Introduction • Lists are sequences of items – separated by commas – enclosed in square brackets ("[…]") • Examples – [ 1, 2, 3, 4 , 5] – ['Alice', 'Bob', 'Carol', Dean'] – ['Alice', 'freshman', [100, 90, 70]]
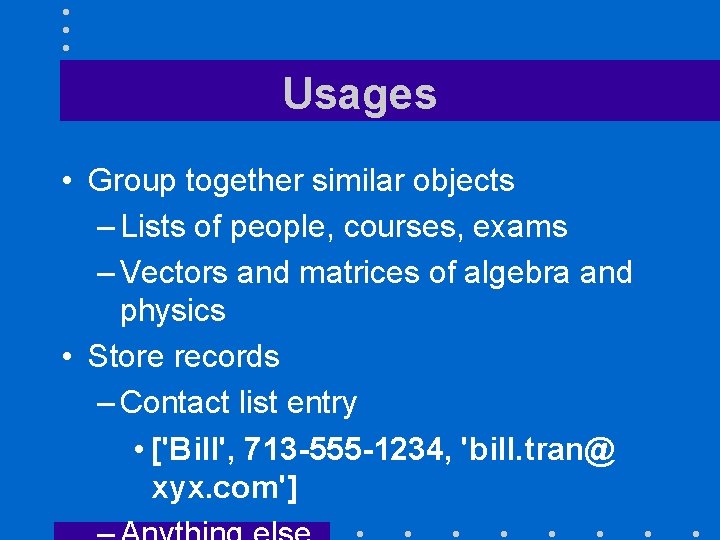
Usages • Group together similar objects – Lists of people, courses, exams – Vectors and matrices of algebra and physics • Store records – Contact list entry • ['Bill', 713 -555 -1234, 'bill. tran@ xyx. com']
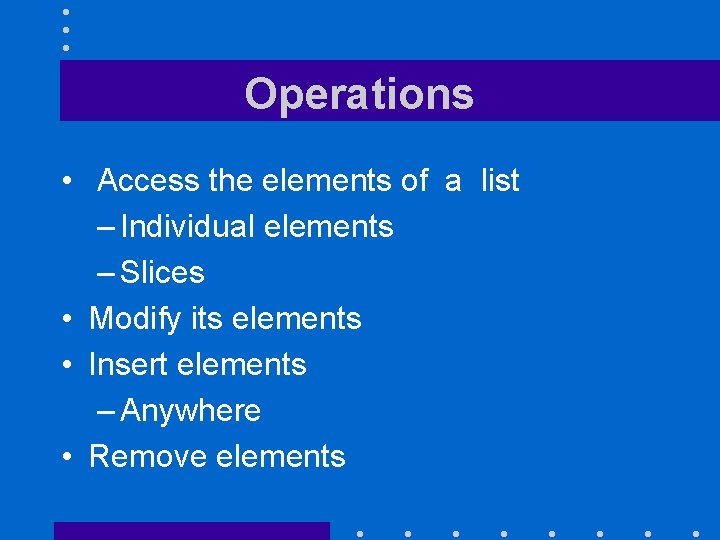
Operations • Access the elements of a list – Individual elements – Slices • Modify its elements • Insert elements – Anywhere • Remove elements
![Lists mylist 11 12 13 14 done mylist 11 Lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist [11,](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-5.jpg)
Lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist [11, 12, 13, 14, 'done'] • >>> print(mylist) [11, 12, 13, 14, 'done'] • >>> mylist[0] 11 – We can access individual elements
![List slices mylist0 1 11 Two observations A list slice List slices • >>> mylist[0: 1] [11] – Two observations • A list slice](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-6.jpg)
List slices • >>> mylist[0: 1] [11] – Two observations • A list slice is a list • mylist[0: 1] starts with list[0] but stops before mylist [1]
![More list slices mylist0 2 11 12 Includes mylist0 and mylist1 More list slices • >>> mylist[0: 2] [11, 12] – Includes mylist[0] and mylist[1]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-7.jpg)
More list slices • >>> mylist[0: 2] [11, 12] – Includes mylist[0] and mylist[1] • >>> mylist[0: ] [11, 12, 13, 14, 'done'] – The whole list • >>> mylist[1: ] [12, 13, 14, 'done']
![More list slices mylist1 done A list slice is a More list slices • >>> mylist[-1: ] ['done'] • A list slice is a](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-8.jpg)
More list slices • >>> mylist[-1: ] ['done'] • A list slice is a list • >>> mylist[-1] 'done' • Not the same thing!
![An example a 10 20 30 40 50 a designates the whole An example • a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-9.jpg)
An example • a = [10, 20, 30, 40, 50] a designates the whole list 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
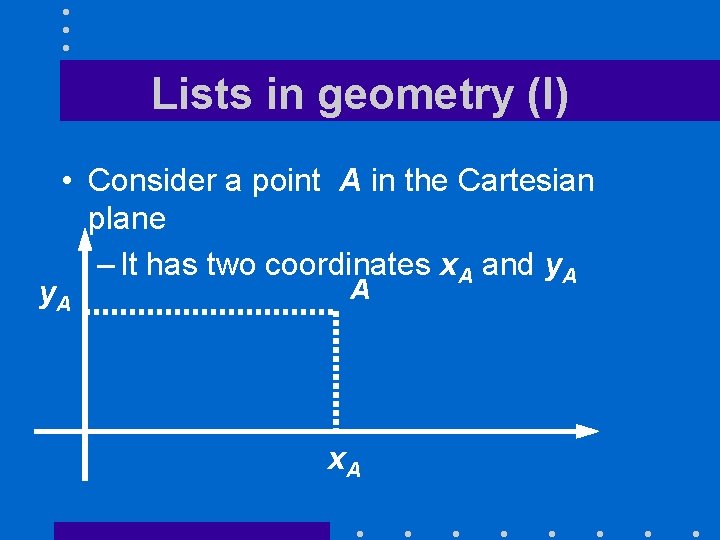
Lists in geometry (I) • Consider a point A in the Cartesian plane – It has two coordinates x. A and y. A A x. A
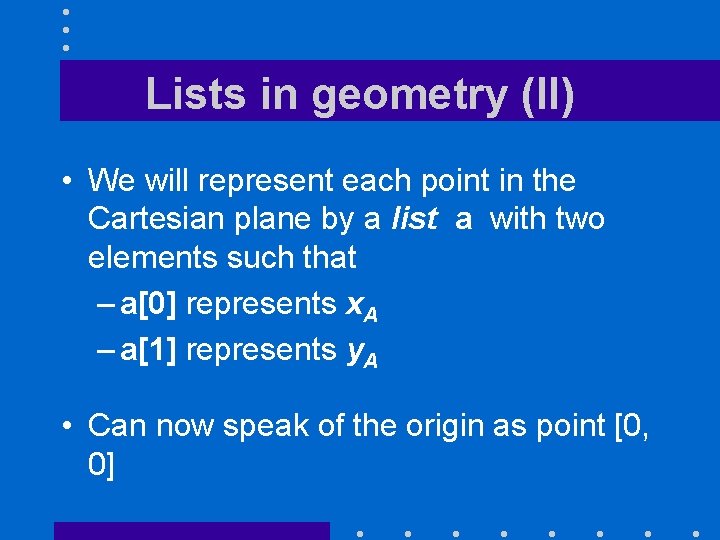
Lists in geometry (II) • We will represent each point in the Cartesian plane by a list a with two elements such that – a[0] represents x. A – a[1] represents y. A • Can now speak of the origin as point [0, 0]
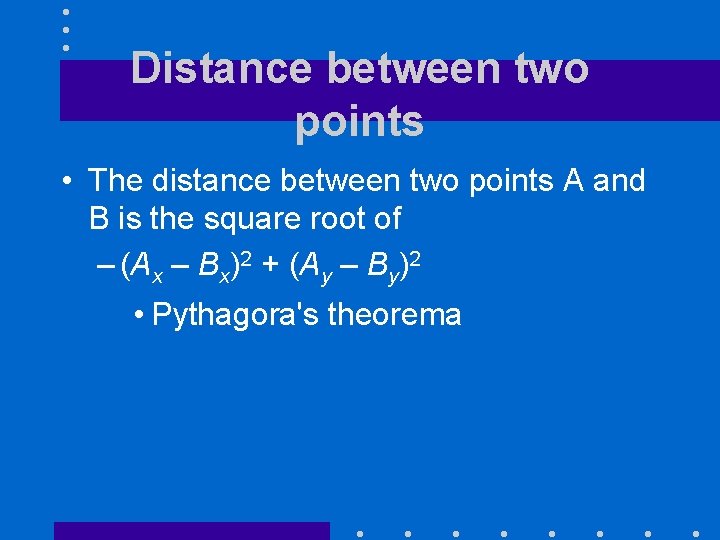
Distance between two points • The distance between two points A and B is the square root of – (Ax – Bx)2 + (Ay – By)2 • Pythagora's theorema
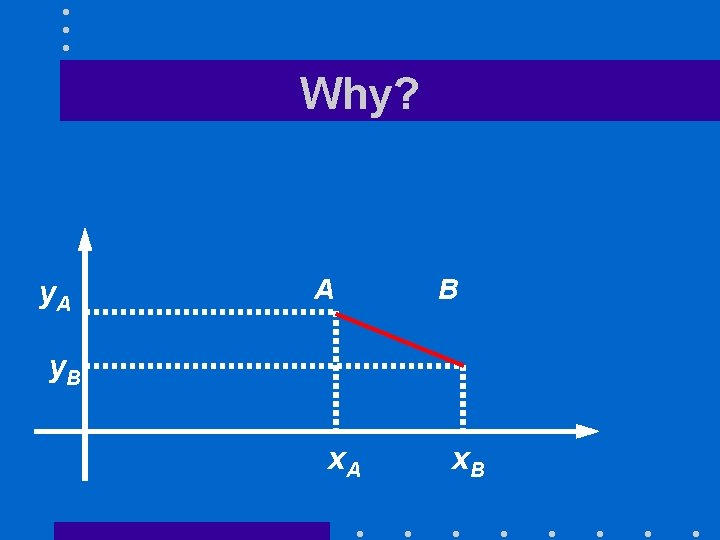
Why? y. A A B y. B x. A x. B
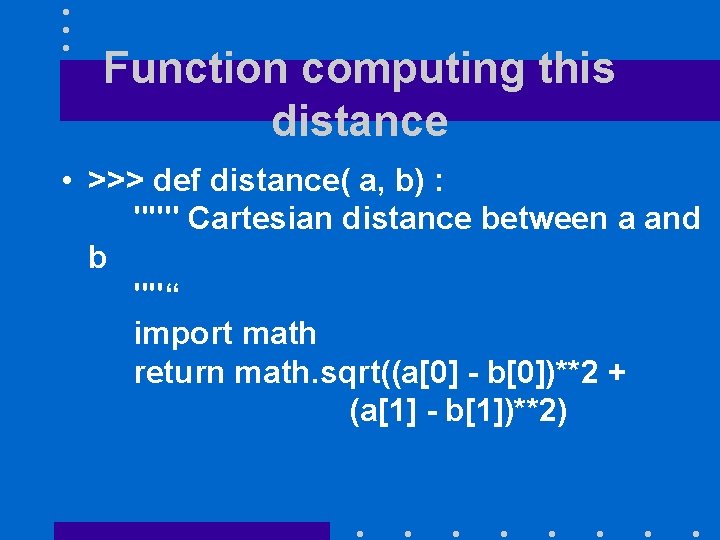
Function computing this distance • >>> def distance( a, b) : """ Cartesian distance between a and b ""“ import math return math. sqrt((a[0] - b[0])**2 + (a[1] - b[1])**2)
![Function computing this distance origin 0 0 a Function computing this distance • >>> origin = [0, 0] • >>> a =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-15.jpg)
Function computing this distance • >>> origin = [0, 0] • >>> a = [1, 1] • >>> distance(origin, a) 1. 4142135623730951 • >>>
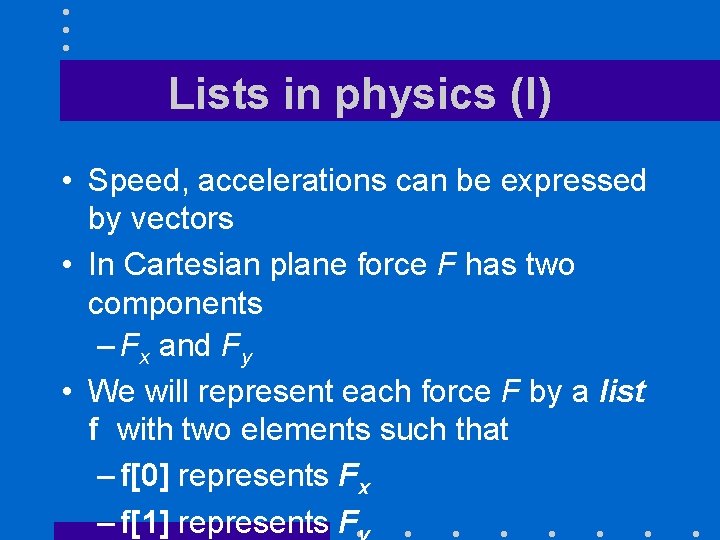
Lists in physics (I) • Speed, accelerations can be expressed by vectors • In Cartesian plane force F has two components – Fx and Fy • We will represent each force F by a list f with two elements such that – f[0] represents Fx – f[1] represents F
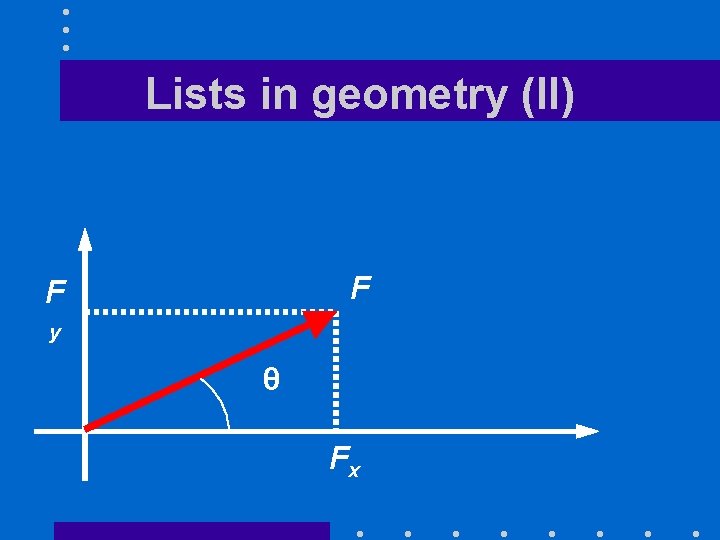
Lists in geometry (II) F F y θ Fx
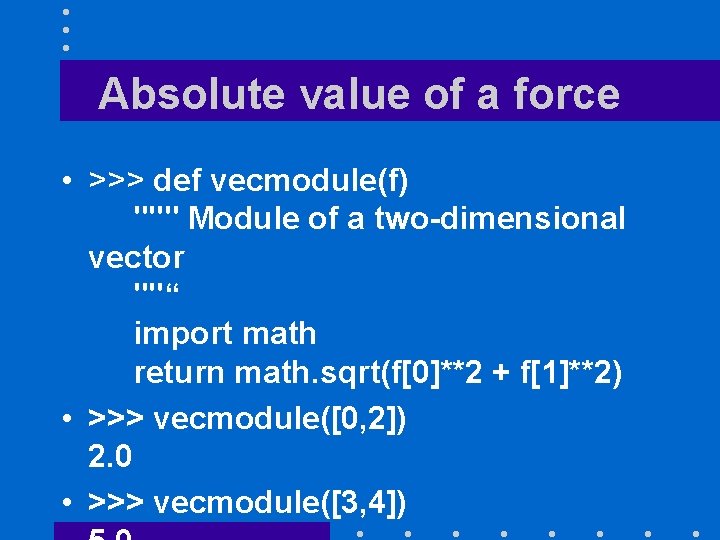
Absolute value of a force • >>> def vecmodule(f) """ Module of a two-dimensional vector ""“ import math return math. sqrt(f[0]**2 + f[1]**2) • >>> vecmodule([0, 2]) 2. 0 • >>> vecmodule([3, 4])
![Adding two vectors I 0 2 0 3 0 2 0 3 Adding two vectors (I) • [0, 2] + [0, 3] [0, 2, 0, 3]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-19.jpg)
Adding two vectors (I) • [0, 2] + [0, 3] [0, 2, 0, 3] – Not what we want – + operator concatenates lists
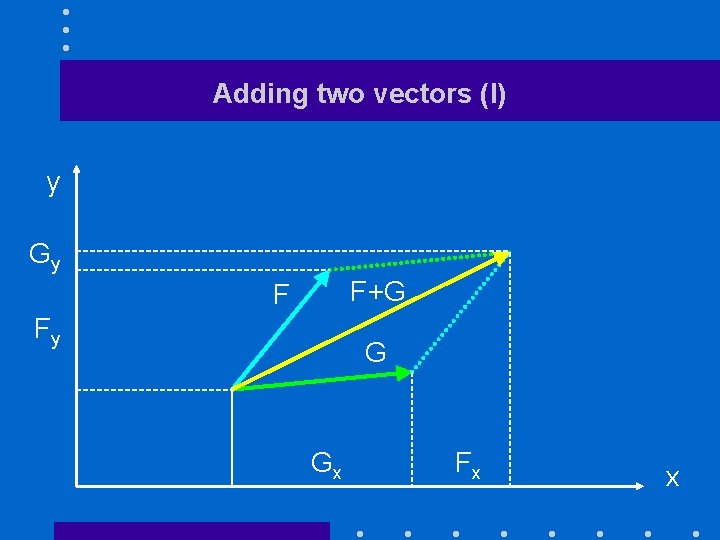
Adding two vectors (I) y Gy F+G F Fy G Gx Fx x
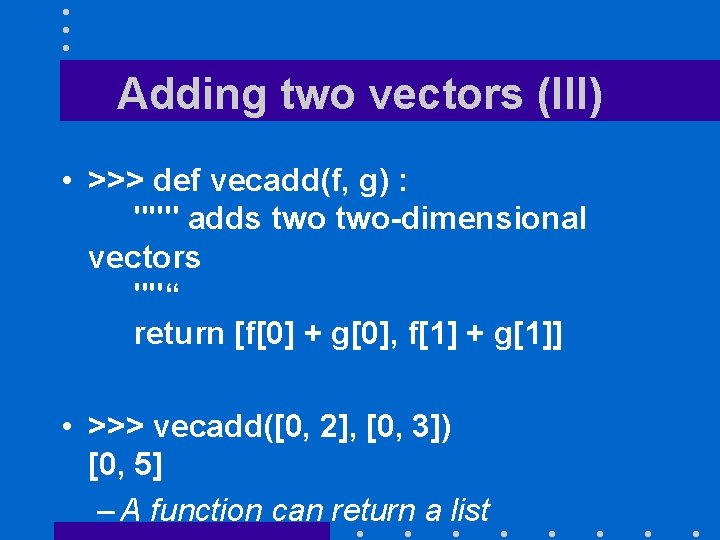
Adding two vectors (III) • >>> def vecadd(f, g) : """ adds two-dimensional vectors ""“ return [f[0] + g[0], f[1] + g[1]] • >>> vecadd([0, 2], [0, 3]) [0, 5] – A function can return a list
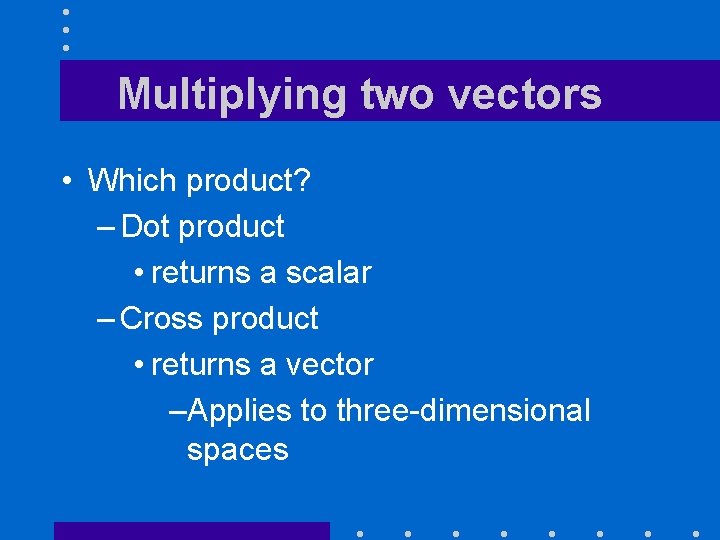
Multiplying two vectors • Which product? – Dot product • returns a scalar – Cross product • returns a vector –Applies to three-dimensional spaces
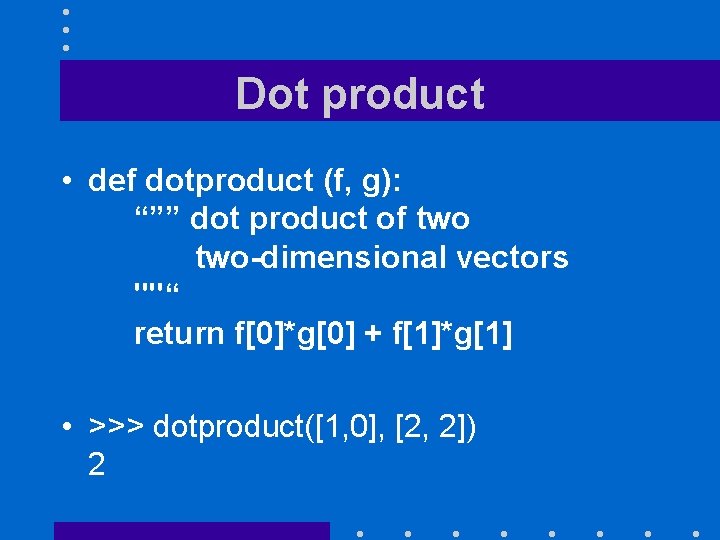
Dot product • def dotproduct (f, g): “”” dot product of two-dimensional vectors ""“ return f[0]*g[0] + f[1]*g[1] • >>> dotproduct([1, 0], [2, 2]) 2
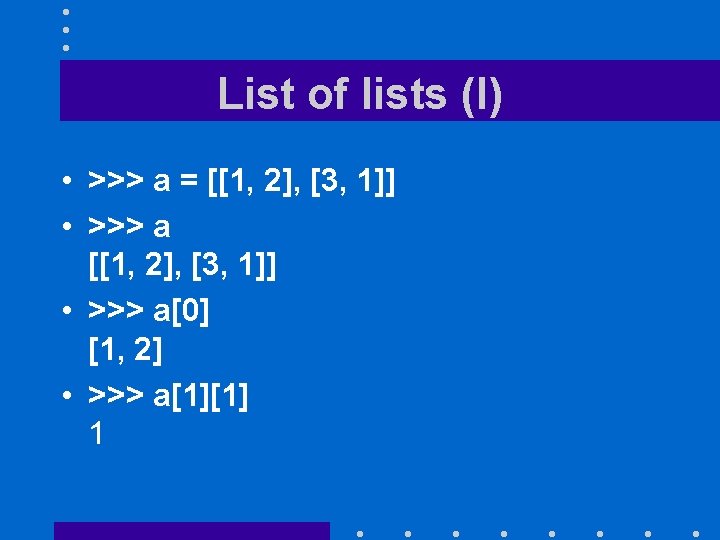
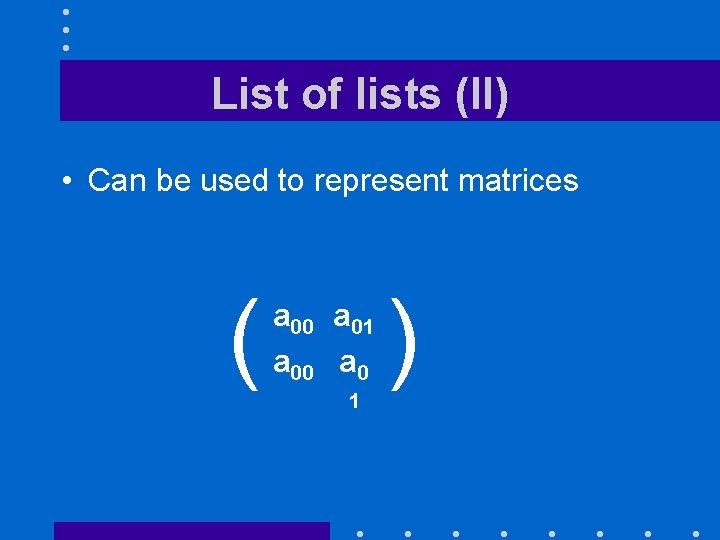
List of lists (II) • Can be used to represent matrices ( a 00 a 01 a 00 a 0 1 )
![Sorting lists mylist 11 12 13 14 done mylist Sorting lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist.](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-26.jpg)
Sorting lists • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist. sort() Traceback (most recent call last): File "<pyshell#2>", line 1, in <module> mylist. sort() Type. Error: unorderable types: str() < int() – Cannot compare apples and oranges
![Sorting lists of strings namelist Alice Carol Bob namelist Sorting lists of strings • >>> namelist = ['Alice', 'Carol', 'Bob'] • >>> namelist.](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-27.jpg)
Sorting lists of strings • >>> namelist = ['Alice', 'Carol', 'Bob'] • >>> namelist. sort() • >>> print(namelist) ['Alice', 'Bob', 'Carol'] • namelist. sort() is a method applied to the list namelist – In-place sort
![Sorting lists of numbers newlist 0 1 1 2 2 Sorting lists of numbers • >>> newlist = [0, -1, +1, -2, +2] •](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-28.jpg)
Sorting lists of numbers • >>> newlist = [0, -1, +1, -2, +2] • >>> newlist. sort() • >>> print(newlist) [-2, -1, 0, 1, 2] • In-place sort
![Sorting into a new list newlist 0 1 1 2 2 Sorting into a new list • >>> newlist = [0, -1, +1, -2, +2]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-29.jpg)
Sorting into a new list • >>> newlist = [0, -1, +1, -2, +2] • >>> sortedlist= sorted(newlist) • >>> print(newlist) [0, -1, 1, -2, 2] • >>> print(sortedlist) [-2, -1, 0, 1, 2] – sorted(…) is a conventional Python function that returns a new list
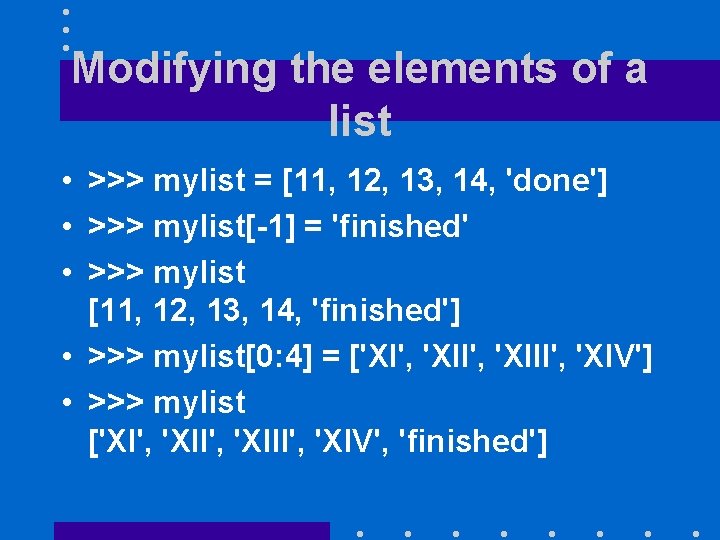
Modifying the elements of a list • >>> mylist = [11, 12, 13, 14, 'done'] • >>> mylist[-1] = 'finished' • >>> mylist [11, 12, 13, 14, 'finished'] • >>> mylist[0: 4] = ['XI', 'XIII', 'XIV'] • >>> mylist ['XI', 'XIII', 'XIV', 'finished']
![Lists of lists I listoflists 17 5 1306 13 6360 Lists of lists (I) • >>> listoflists = [[17. 5, "1306"], [13, "6360"]] •](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-31.jpg)
Lists of lists (I) • >>> listoflists = [[17. 5, "1306"], [13, "6360"]] • >>> listoflists. sort() • >>> print(listoflists) [[13, '6360'], [17. 5, '1306']] • >>> listoflists[0] [13, '6360'] • >>> listoflists[1] [17. 5, '1306']
![Lists of lists II listoflists00 13 listoflists11 1306 Lists of lists (II) • >>> listoflists[0][0] 13 • >>> listoflists[1][1] '1306' • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-32.jpg)
Lists of lists (II) • >>> listoflists[0][0] 13 • >>> listoflists[1][1] '1306' • >>> listoflists[0][1] ='6360 quiz' • >>> listoflists [[13, '6360 quiz'], [17. 5, '1306']]
![Adding elements to a list mylist 11 12 13 14 finished Adding elements to a list • >>> mylist = [11, 12, 13, 14, 'finished']](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-33.jpg)
Adding elements to a list • >>> mylist = [11, 12, 13, 14, 'finished'] • >>> mylist. append('Not yet!') • >>> mylist [11, 12, 13, 14, 'finished', 'Not yet!']
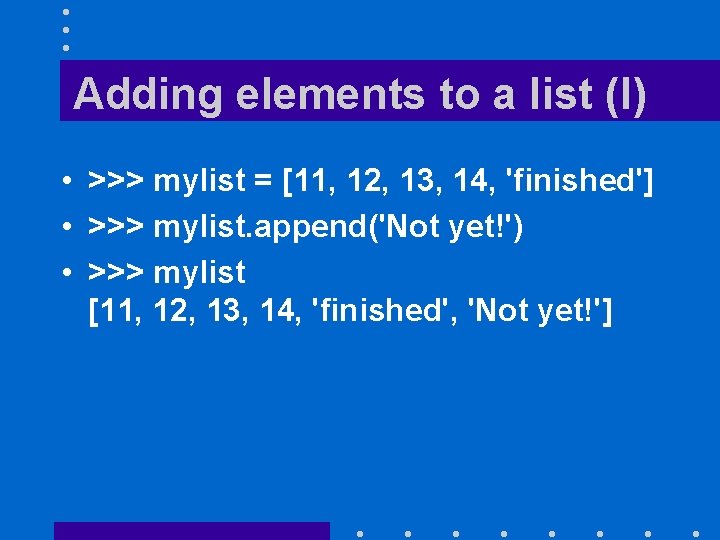
Adding elements to a list (I) • >>> mylist = [11, 12, 13, 14, 'finished'] • >>> mylist. append('Not yet!') • >>> mylist [11, 12, 13, 14, 'finished', 'Not yet!']
![Adding elements to a list II listoflists 13 6360 quiz 17 Adding elements to a list (II) • >>> listoflists = [[13, '6360 quiz'], [17.](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-35.jpg)
Adding elements to a list (II) • >>> listoflists = [[13, '6360 quiz'], [17. 5, '1306']] • >>> listoflists. append([15. 3, 'ABET']) • >>> listoflists [[13, '6360 quiz'], [17. 5, '1306'], [15. 3, 'ABET']] Appending means adding at the end.
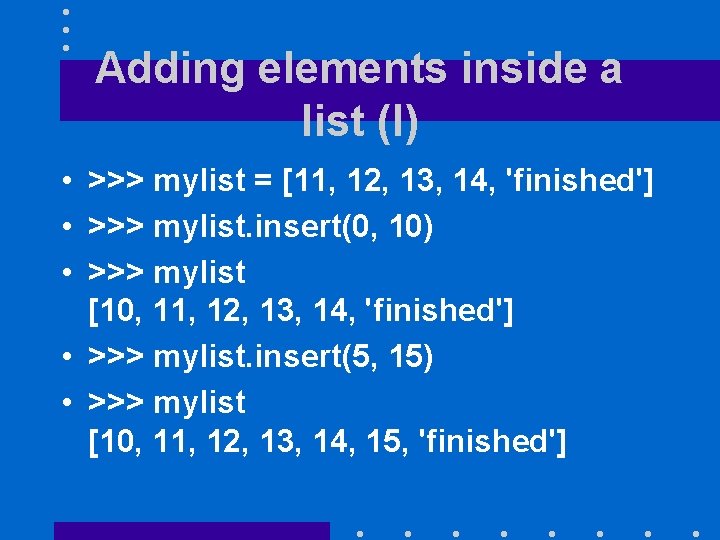
Adding elements inside a list (I) • >>> mylist = [11, 12, 13, 14, 'finished'] • >>> mylist. insert(0, 10) • >>> mylist [10, 11, 12, 13, 14, 'finished'] • >>> mylist. insert(5, 15) • >>> mylist [10, 11, 12, 13, 14, 15, 'finished']
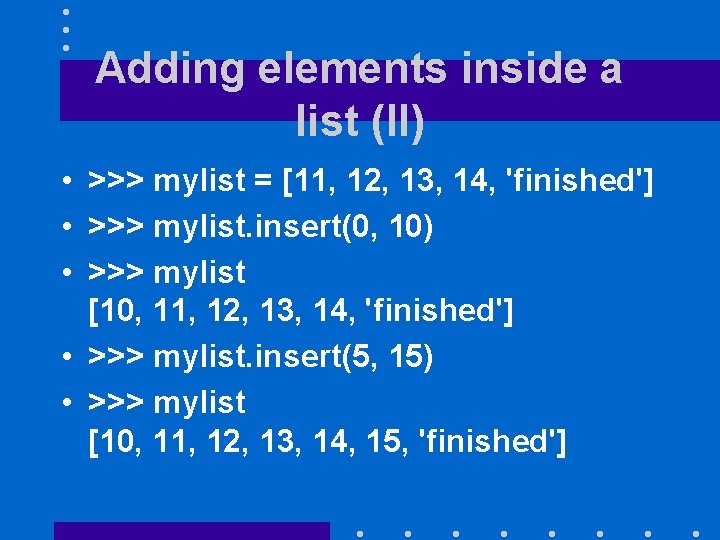
Adding elements inside a list (II) • >>> mylist = [11, 12, 13, 14, 'finished'] • >>> mylist. insert(0, 10) • >>> mylist [10, 11, 12, 13, 14, 'finished'] • >>> mylist. insert(5, 15) • >>> mylist [10, 11, 12, 13, 14, 15, 'finished']
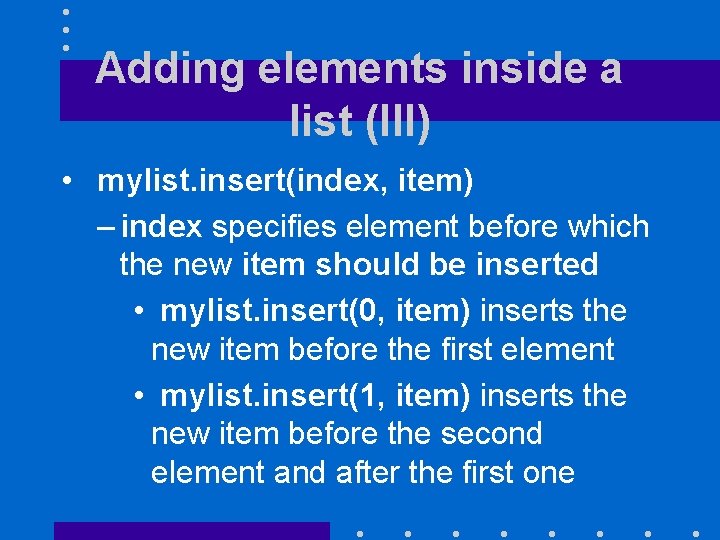
Adding elements inside a list (III) • mylist. insert(index, item) – index specifies element before which the new item should be inserted • mylist. insert(0, item) inserts the new item before the first element • mylist. insert(1, item) inserts the new item before the second element and after the first one
![Example I a 10 20 30 40 50 a designates the whole Example (I) • a = [10, 20, 30, 40, 50] a designates the whole](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-39.jpg)
Example (I) • a = [10, 20, 30, 40, 50] a designates the whole list 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
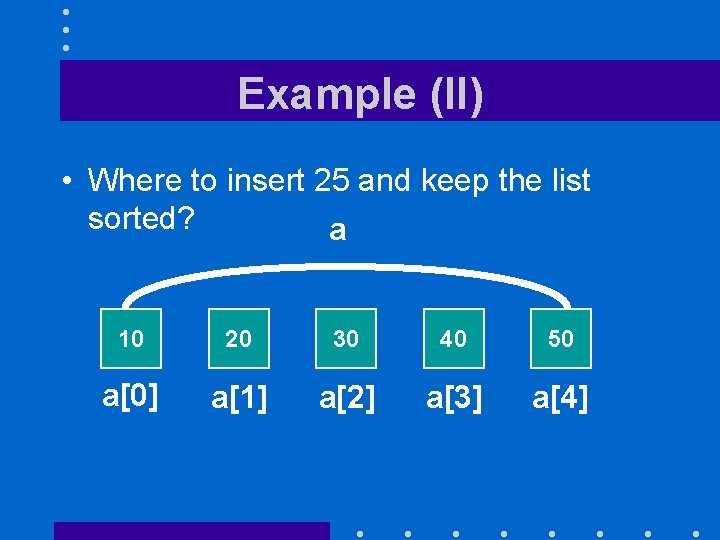
Example (II) • Where to insert 25 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
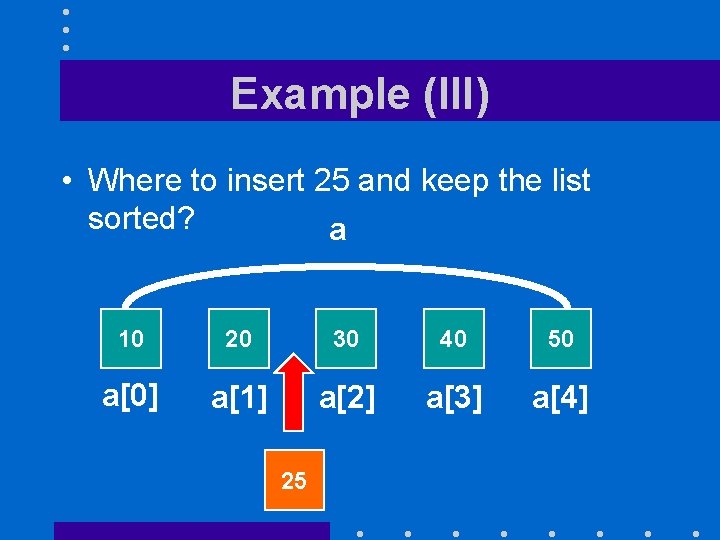
Example (III) • Where to insert 25 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4] 25
![Example IV We do a insert2 25 after a1 and before Example (IV) • We do – a. insert(2, 25) • after a[1] and before](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-42.jpg)
Example (IV) • We do – a. insert(2, 25) • after a[1] and before a[2]
![Let us check a 10 20 30 40 50 Let us check • >>> a = [10, 20, 30, 40, 50] • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-43.jpg)
Let us check • >>> a = [10, 20, 30, 40, 50] • >>> a. insert(2, 25) • >>> a [10, 25, 30, 40, 50]
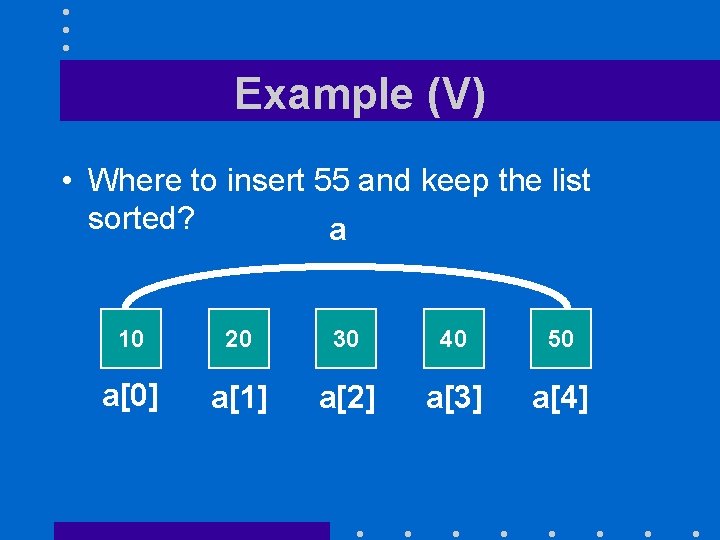
Example (V) • Where to insert 55 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4]
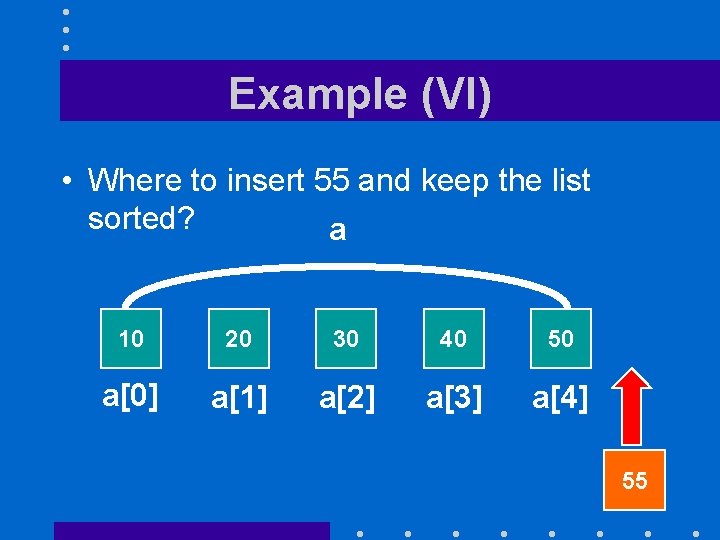
Example (VI) • Where to insert 55 and keep the list sorted? a 10 20 30 40 50 a[0] a[1] a[2] a[3] a[4] 55
![Example VII We must insert After a4 Before no other element Example (VII) • We must insert – After a[4] – Before no other element](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-46.jpg)
Example (VII) • We must insert – After a[4] – Before no other element • We act as if a[5] existed – a. insert(5, 55) • It works! • Same as a. append(55)
![Let us check a 10 20 30 40 50 Let us check • >>> a = [10, 20, 30, 40, 50] • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-47.jpg)
Let us check • >>> a = [10, 20, 30, 40, 50] • >>> a. insert(5, 55) • >>> a [10, 20, 30, 40, 55]
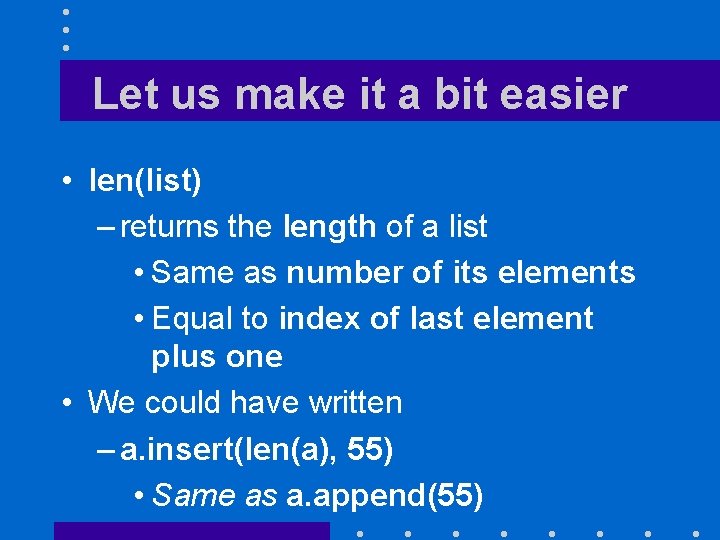
Let us make it a bit easier • len(list) – returns the length of a list • Same as number of its elements • Equal to index of last element plus one • We could have written – a. insert(len(a), 55) • Same as a. append(55)
![Removing items One by one thislist popi removes thislisti from thislist Removing items • One by one • thislist. pop(i) – removes thislist[i] from thislist](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-49.jpg)
Removing items • One by one • thislist. pop(i) – removes thislist[i] from thislist – returns the removed element • thislist. pop( ) – removes the last element from thislist – returns the removed element
![Examples I mylist 11 22 33 44 55 66 Examples (I) • >>> mylist = [11, 22, 33, 44, 55, 66] • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-50.jpg)
Examples (I) • >>> mylist = [11, 22, 33, 44, 55, 66] • >>> mylist. pop(0) 11 • >>> mylist [22, 33, 44, 55, 66]
![Examples II mylist pop 66 mylist 22 33 44 55 Examples (II) • >>> mylist. pop() 66 • >>> mylist [22, 33, 44, 55]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-51.jpg)
Examples (II) • >>> mylist. pop() 66 • >>> mylist [22, 33, 44, 55] • >>> mylist. pop(2) 44 • >>> mylist [22, 33, 55]
![Sum one more useful function list 10 20 sumlist Sum: one more useful function • >>> list = [10, 20] • >>> sum(list)](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-52.jpg)
Sum: one more useful function • >>> list = [10, 20] • >>> sum(list) 50 • >>> list = ['Alice', ' and ', 'Bob'] • >>> sum(list) – Does not work! • Would get same results with tuples
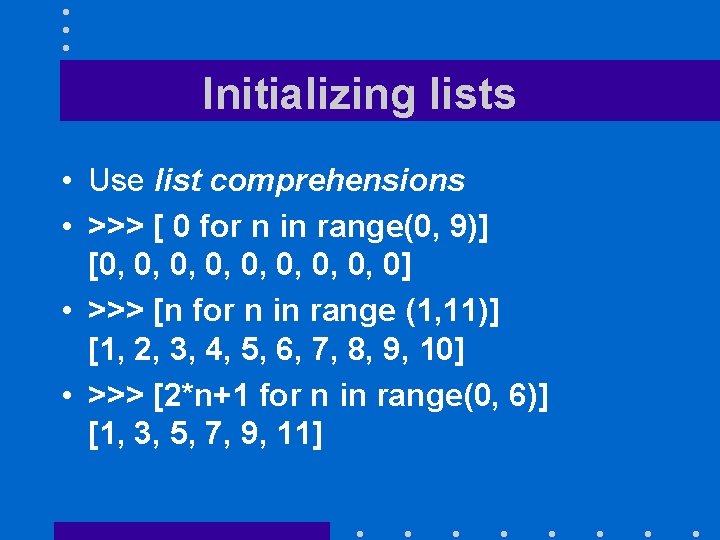
Initializing lists • Use list comprehensions • >>> [ 0 for n in range(0, 9)] [0, 0, 0] • >>> [n for n in range (1, 11)] [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] • >>> [2*n+1 for n in range(0, 6)] [1, 3, 5, 7, 9, 11]
![Warning The for n clause is essential 0 in range0 10 True Warning! • The for n clause is essential • [0 in range(0, 10)] [True]](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-54.jpg)
Warning! • The for n clause is essential • [0 in range(0, 10)] [True] – Because 0 is in range(0, 10)
![More comprehensions c for c in COSC 1306 C O S More comprehensions • >>> [ c for c in 'COSC 1306'] ['C', 'O', 'S',](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-55.jpg)
More comprehensions • >>> [ c for c in 'COSC 1306'] ['C', 'O', 'S', 'C', ' ', ‘ 1', '3', '0', '6'] • >>> names = ['Alice', 'bob', 'carol'] • >>> cap_names =[n. capitalize() for n in names] • >>> names ['Alice', 'Bob', 'Carol'] • >>> cap_names ['Alice', 'bob', 'carol']
![An equivalence 2n1 for n in range0 6 1 3 5 7 9 An equivalence • [2*n+1 for n in range(0, 6)] [1, 3, 5, 7, 9,](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-56.jpg)
An equivalence • [2*n+1 for n in range(0, 6)] [1, 3, 5, 7, 9, 11] is same as • a=[] for n in range(0, 6) : a. append(2*n + 1)
![Filtered comprehensions a 11 22 33 44 55 b Filtered comprehensions • >>> a = [11, 22, 33, 44, 55] • >>> b](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-57.jpg)
Filtered comprehensions • >>> a = [11, 22, 33, 44, 55] • >>> b = [ n for n in a if n%2 == 0] • >>> b [22, 44] • >>> c = [n//11 for n in a if n > 20] • >>> c [2, 3, 4, 5] A very powerful tool !
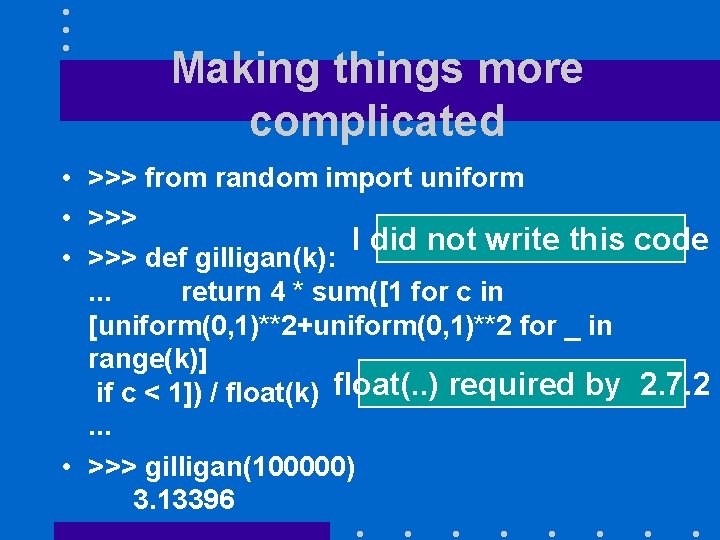
Making things more complicated • >>> from random import uniform • >>> I did not write this code • >>> def gilligan(k): . . . return 4 * sum([1 for c in [uniform(0, 1)**2+uniform(0, 1)**2 for _ in range(k)] if c < 1]) / float(k) float(. . ) required by 2. 7. 2. . . • >>> gilligan(100000) 3. 13396
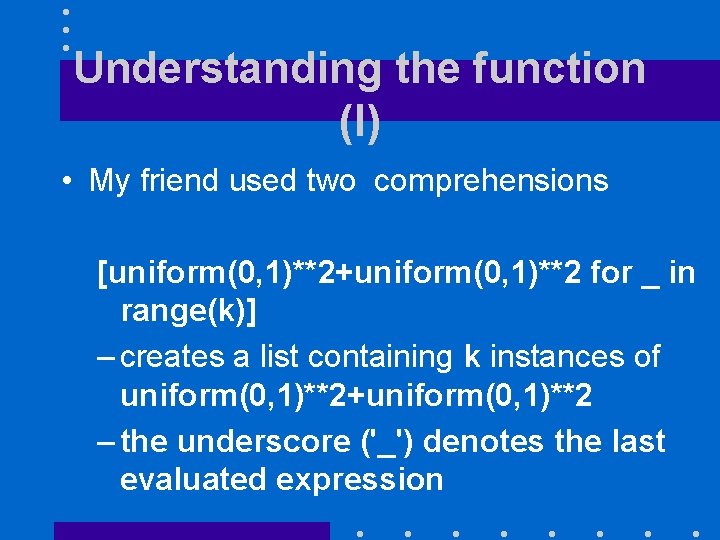
Understanding the function (I) • My friend used two comprehensions [uniform(0, 1)**2+uniform(0, 1)**2 for _ in range(k)] – creates a list containing k instances of uniform(0, 1)**2+uniform(0, 1)**2 – the underscore ('_') denotes the last evaluated expression
![Understanding the function II 1 for c in if c 1 Understanding the function (II) [1 for c in […] if c < 1] –](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-60.jpg)
Understanding the function (II) [1 for c in […] if c < 1] – creates a list containing a 1 for each value in c such that c < 1 – each entry of the new list represents one dart that felt into the circle
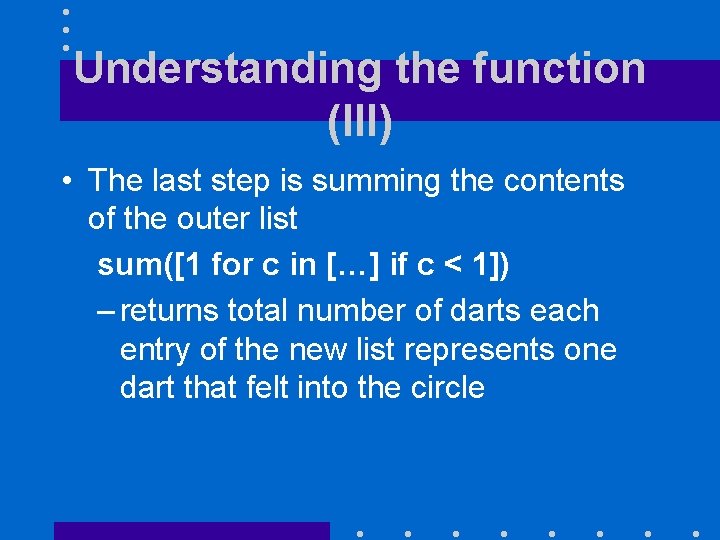
Understanding the function (III) • The last step is summing the contents of the outer list sum([1 for c in […] if c < 1]) – returns total number of darts each entry of the new list represents one dart that felt into the circle
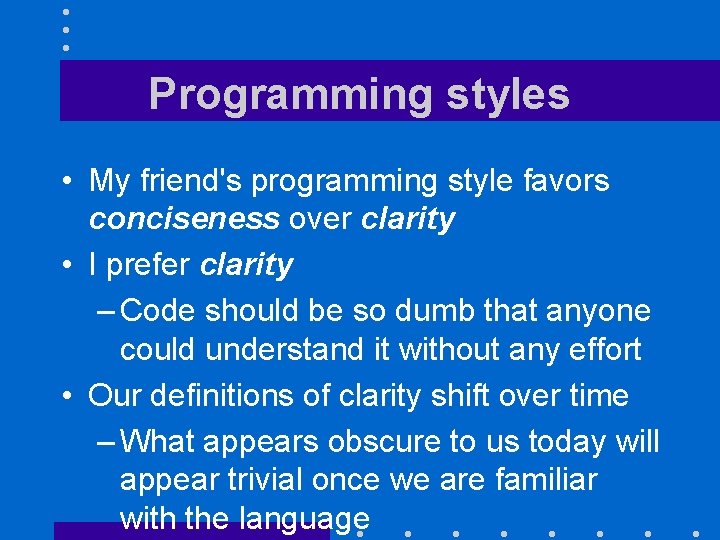
Programming styles • My friend's programming style favors conciseness over clarity • I prefer clarity – Code should be so dumb that anyone could understand it without any effort • Our definitions of clarity shift over time – What appears obscure to us today will appear trivial once we are familiar with the language
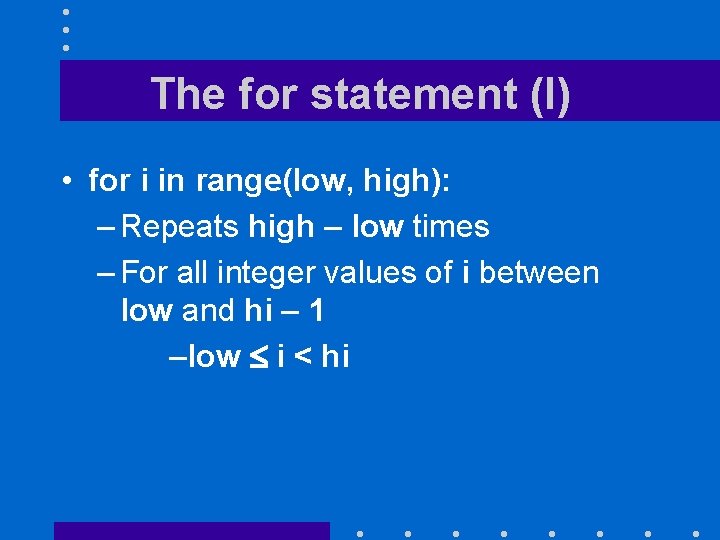
The for statement (I) • for i in range(low, high): – Repeats high – low times – For all integer values of i between low and hi – 1 –low i < hi
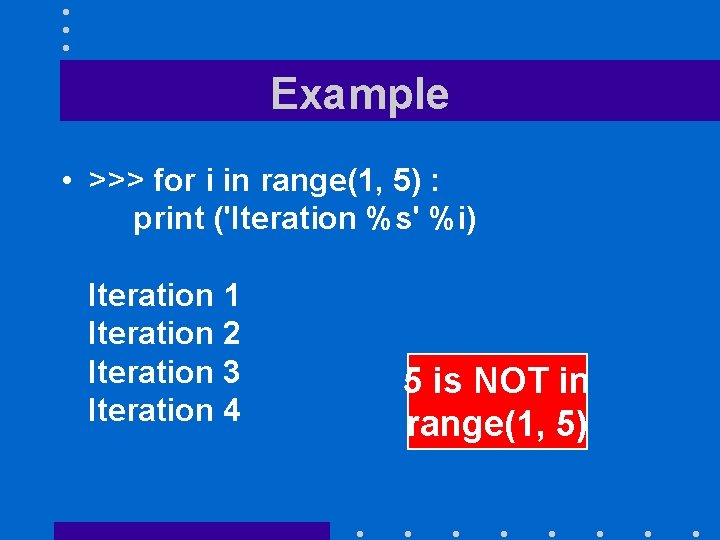
Example • >>> for i in range(1, 5) : print ('Iteration %s' %i) Iteration 1 Iteration 2 Iteration 3 Iteration 4 5 is NOT in range(1, 5)
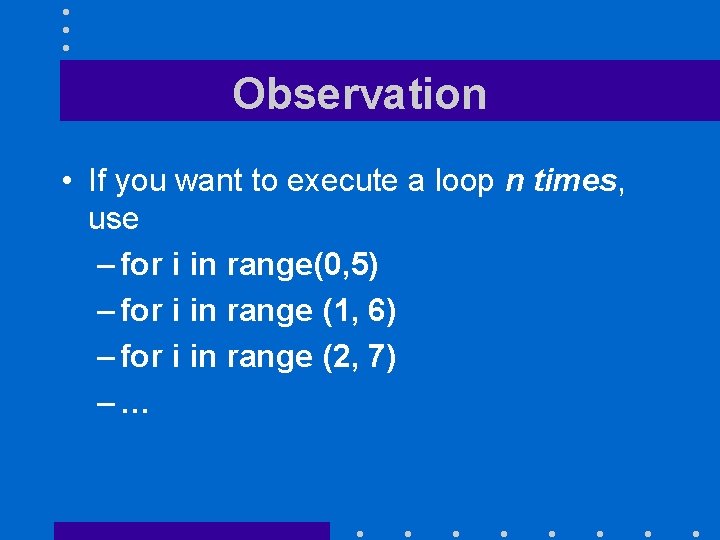
Observation • If you want to execute a loop n times, use – for i in range(0, 5) – for i in range (1, 6) – for i in range (2, 7) –…
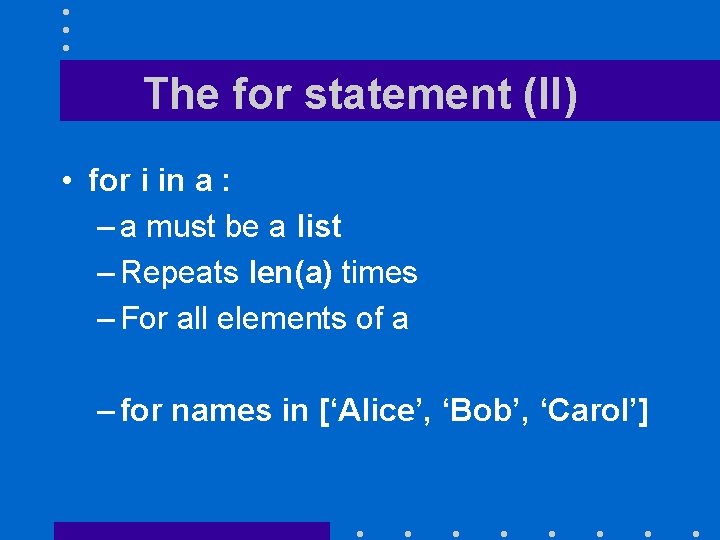
The for statement (II) • for i in a : – a must be a list – Repeats len(a) times – For all elements of a – for names in [‘Alice’, ‘Bob’, ‘Carol’]
![Example I names Alice Bob Carol for name in Example (I) • >>> names = ['Alice', 'Bob', 'Carol'] • >>> for name in](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-67.jpg)
Example (I) • >>> names = ['Alice', 'Bob', 'Carol'] • >>> for name in names : print('Hi ' + name + '!') Hi Alice! Hi Bob! Hi Carol! • >>>
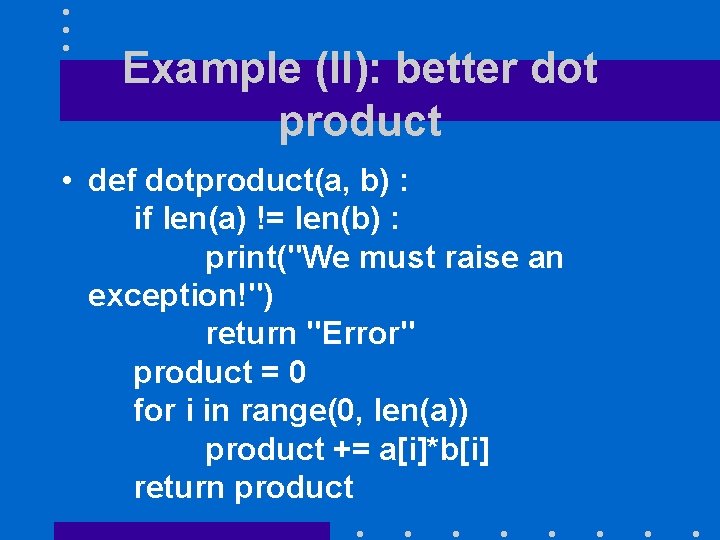
Example (II): better dot product • def dotproduct(a, b) : if len(a) != len(b) : print("We must raise an exception!") return "Error" product = 0 for i in range(0, len(a)) product += a[i]*b[i] return product
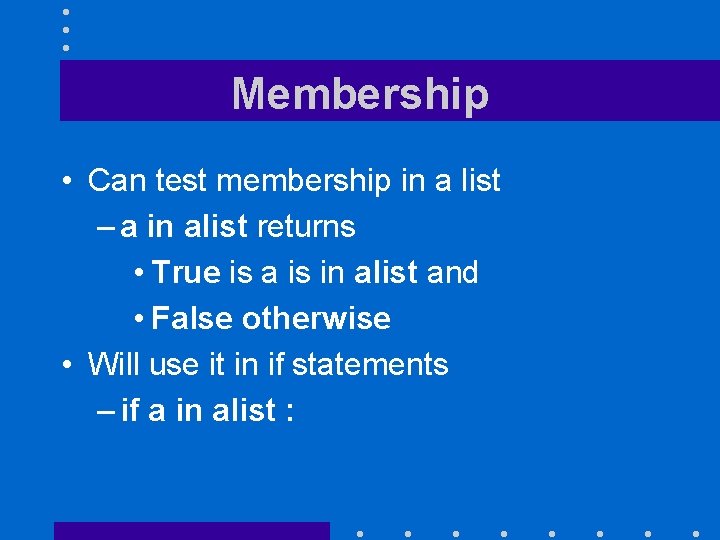
Membership • Can test membership in a list – a in alist returns • True is a is in alist and • False otherwise • Will use it in if statements – if a in alist :
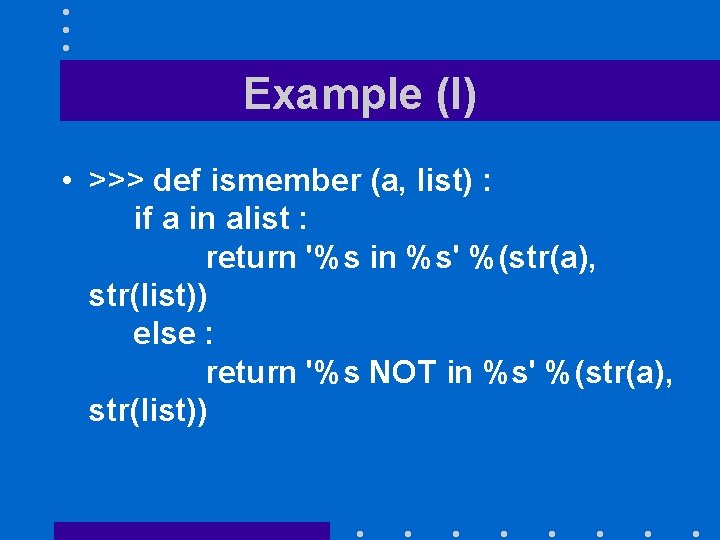
Example (I) • >>> def ismember (a, list) : if a in alist : return '%s in %s' %(str(a), str(list)) else : return '%s NOT in %s' %(str(a), str(list))
![Example II ismemberBob Alice Carol Bob NOT in Alice Carol Example (II) • >>> ismember('Bob', ['Alice', 'Carol']) "Bob NOT in ['Alice', 'Carol']" • >>>](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-71.jpg)
Example (II) • >>> ismember('Bob', ['Alice', 'Carol']) "Bob NOT in ['Alice', 'Carol']" • >>> ismember('Alice', ['Alice', 'Carol']) "Alice in ['Alice', 'Carol']"
![CopyingSaving a list a Alice Bob Carol saved a Copying/Saving a list • >>> a = ["Alice", "Bob", "Carol"] >>> saved = a](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-72.jpg)
Copying/Saving a list • >>> a = ["Alice", "Bob", "Carol"] >>> saved = a >>> a. pop(0) 'Alice' >>> a ['Bob', 'Carol'] >>> saved ['Bob', 'Carol'] We did not save anything!
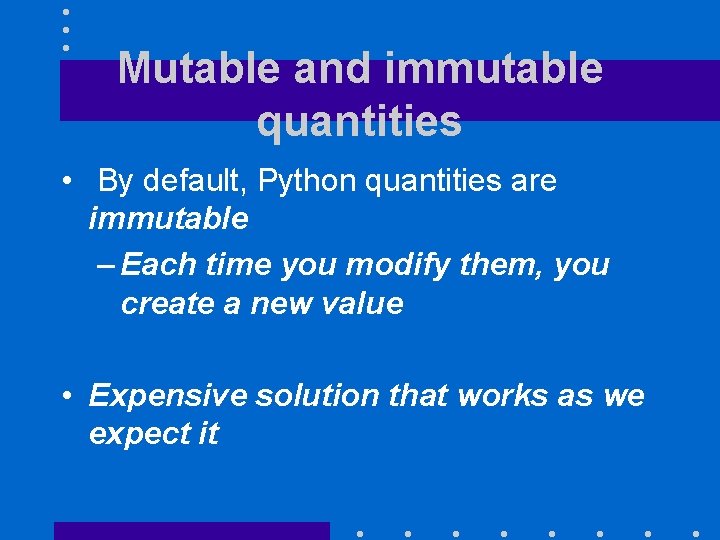
Mutable and immutable quantities • By default, Python quantities are immutable – Each time you modify them, you create a new value • Expensive solution that works as we expect it
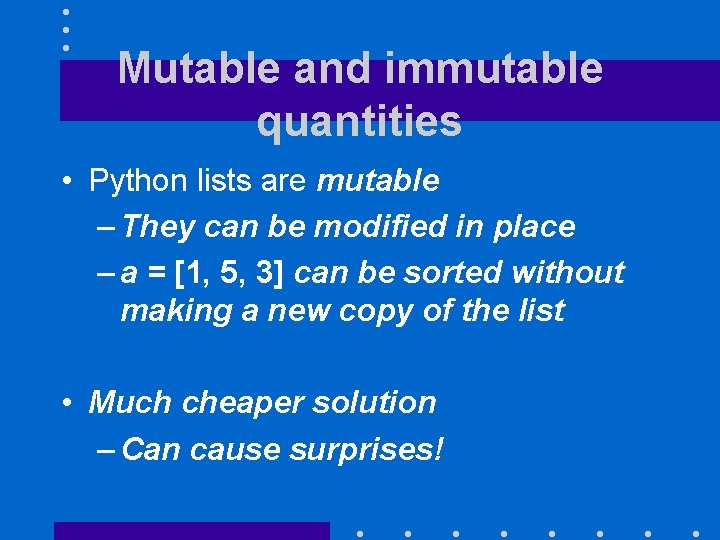
Mutable and immutable quantities • Python lists are mutable – They can be modified in place – a = [1, 5, 3] can be sorted without making a new copy of the list • Much cheaper solution – Can cause surprises!
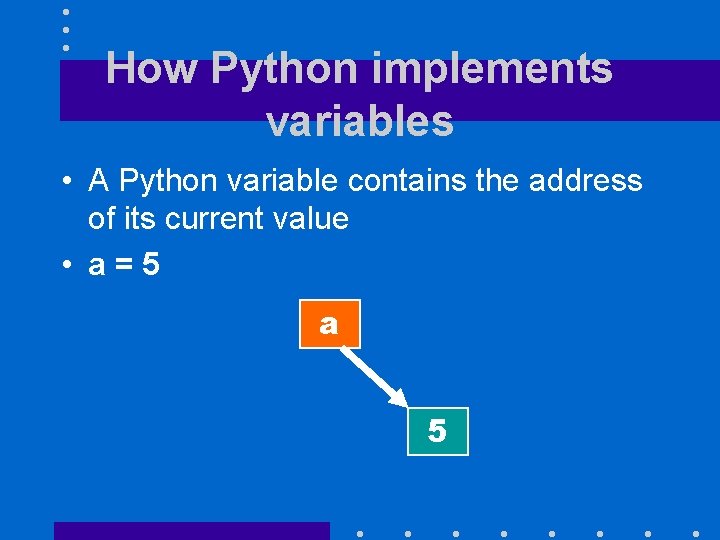
How Python implements variables • A Python variable contains the address of its current value • a=5 a 5
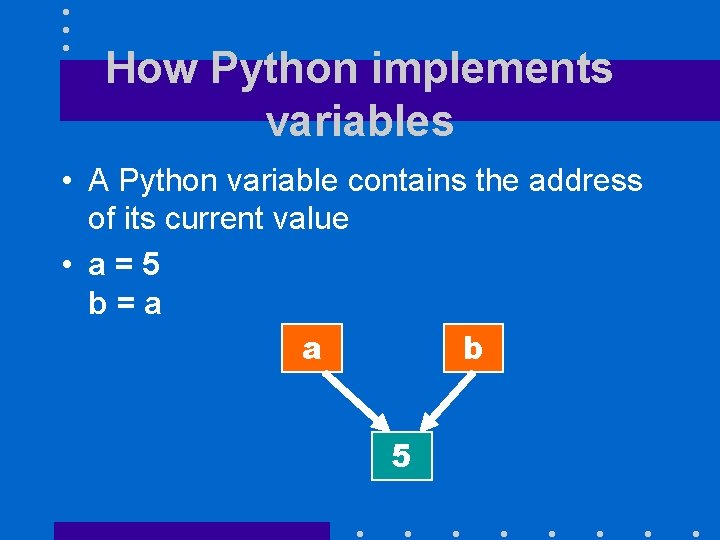
How Python implements variables • A Python variable contains the address of its current value • a=5 b=a a b 5
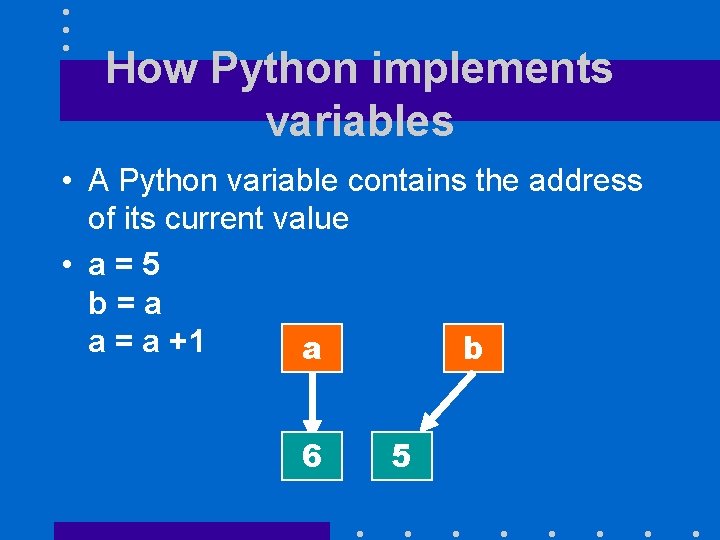
How Python implements variables • A Python variable contains the address of its current value • a=5 b=a a = a +1 a b 6 5
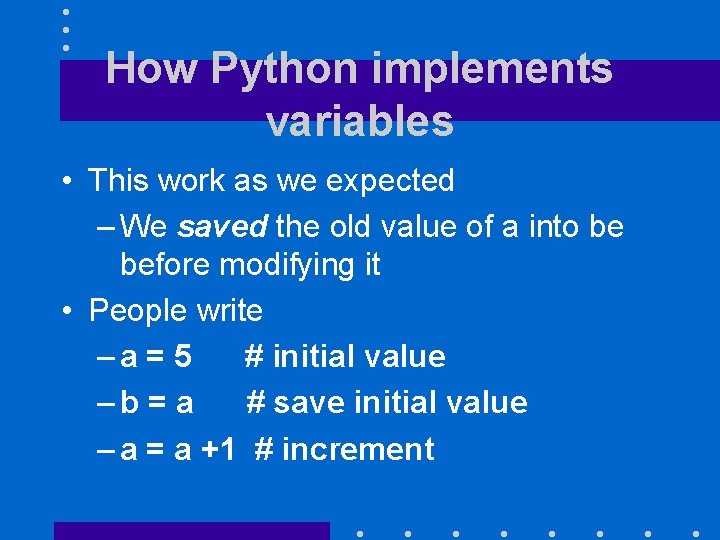
How Python implements variables • This work as we expected – We saved the old value of a into be before modifying it • People write –a = 5 # initial value –b = a # save initial value – a = a +1 # increment
![A big surprise a 11 33 22 b A big surprise • >>> a = [11, 33, 22] • >>> b =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-79.jpg)
A big surprise • >>> a = [11, 33, 22] • >>> b = a • >>> b [11, 33, 22] • >>> a. sort() • >>> a [11, 22, 33] • >>> b [11, 22, 33] The old value of a was not saved!
![What happened I a 11 33 22 b What happened (I) • >>> a = [11, 33, 22] • >>> b =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-80.jpg)
What happened (I) • >>> a = [11, 33, 22] • >>> b = a • >>> b [11, 33, 22] a b [11, 33, 22]
![What happened II a 11 33 22 b What happened (II) • >>> a = [11, 33, 22] • >>> b =](https://slidetodoc.com/presentation_image_h2/5af833c526414af6af4c37a8ffed8063/image-81.jpg)
What happened (II) • >>> a = [11, 33, 22] • >>> b = a • >>> b [11, 33, 22] • >>> a. sort() • >>> a [11, 22, 33] • >>> b [11, 22, 33] a b [11, 22, 33]
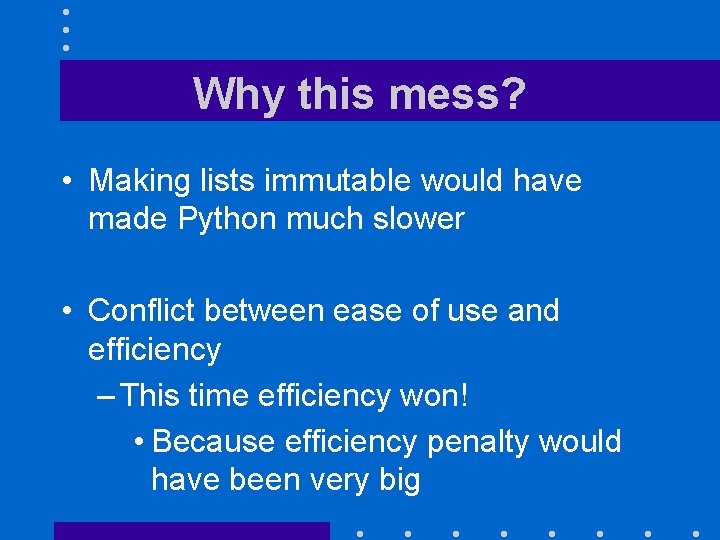
Why this mess? • Making lists immutable would have made Python much slower • Conflict between ease of use and efficiency – This time efficiency won! • Because efficiency penalty would have been very big
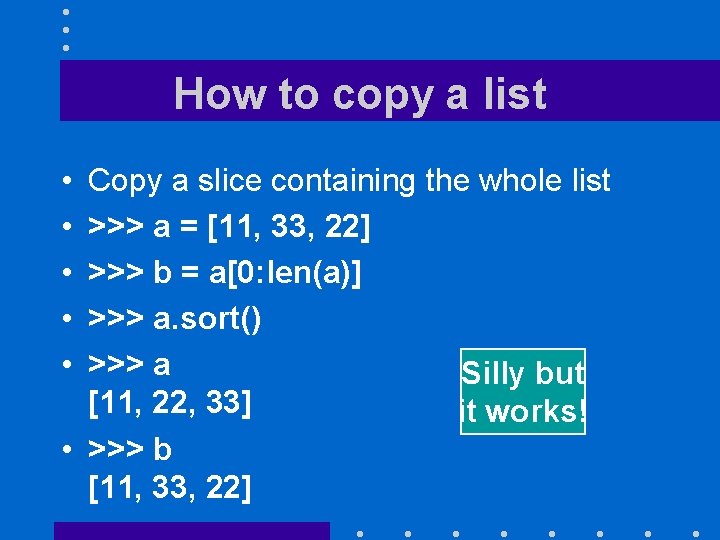
How to copy a list • • • Copy a slice containing the whole list >>> a = [11, 33, 22] >>> b = a[0: len(a)] >>> a. sort() >>> a Silly but [11, 22, 33] it works! • >>> b [11, 33, 22]
Concatinates
Lists of tuples python
Python list methods
Python programming an introduction to computer science
My subject is
Rapid gui programming with python and qt
Cosc 4p61
Cosc 4p42
Cosc 3p91
Cosc 1306
Cosc 1306
Cosc 4368
Cosc 4p41
Cosc 4p41
Cosc
Cosc 3340
Cosc 320
Cosc parameters
Cosc 2p12
Cosc 4p41
Cosc 3340
68030 pinout
Cosc 121
Cosc 1p02
Cosc 2p12
Cosc 3p92
1 bit alu
Cosc 121
Cosc 121
Cosc 121
Cosc 121
Cosc -cos d
Cosc 1306
Cosc 3p94
Cosc101
Is etm recognizable
Cosc 3340
Procedural python
Python chapter 5 programming exercises
Second order cone programming python
Python programming in context
Constraint programming python
Python programming in context
Python cgi programming examples
Python audio programming
String python
Programming essentials in python
Python blackjack oop
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
What is system program
Integer programming vs linear programming
Definisi linear
What is semicolon
Lesson 3 lists practice
Sound waves spelling word lists
Swst spelling test
Year 7 spelling words
Resource list edinburgh
Lisp lists
Java types of lists
Words their way 2nd grade
Read write inc spelling
Prolog list of lists
Parallel structure example
Mexica tribute lists ap world history
Wish lists year
Political lists new
Qri word lists
No nonsense spelling strategies
Wish lists year
"new bookmarking lists 2018"
"new bookmarking lists 2018"
Political wish lists
Ei wirds
Ucl library reading lists
Swot analysis between nike and adidas
Blockly lists
Management science linear programming problems
American computer science league practice problems
Simplex method in management science
Rapid change
Sciencefusion think central
Hard science and soft science