Sharif University of Technology C Programming Languages Lecturer
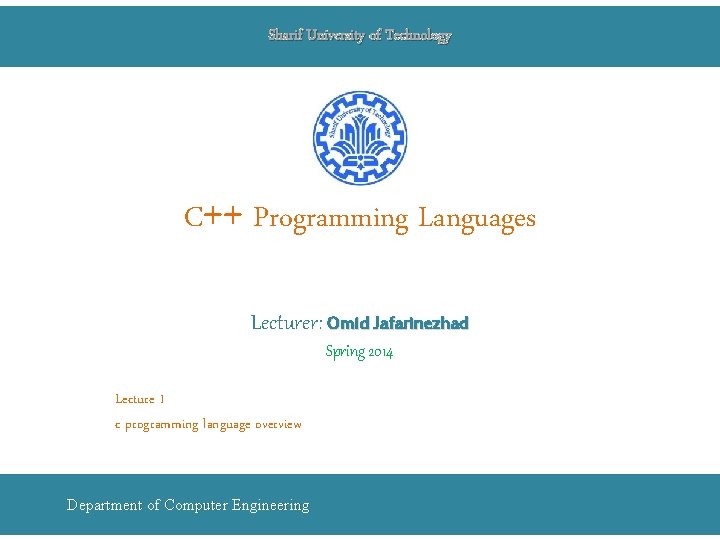
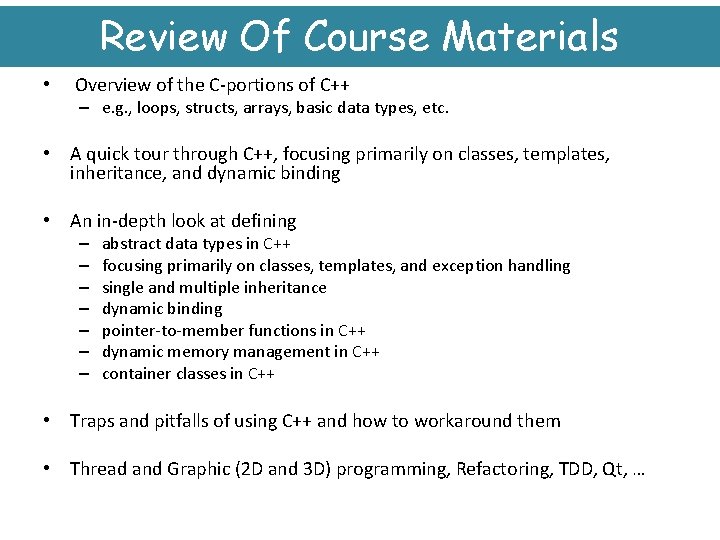
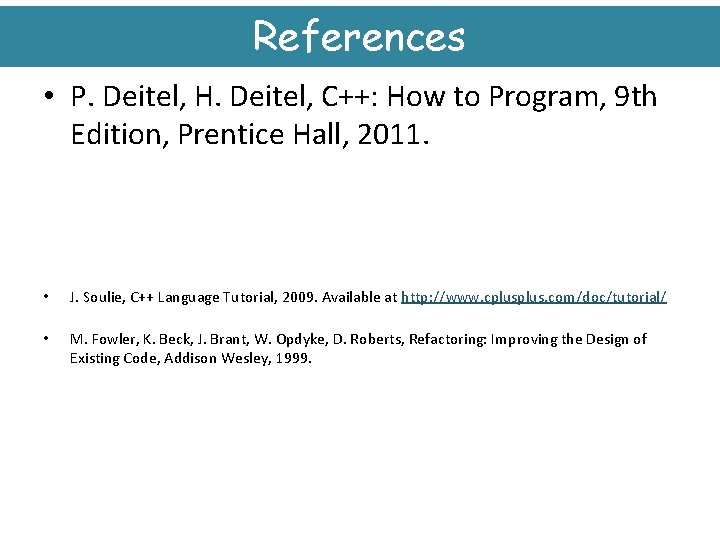
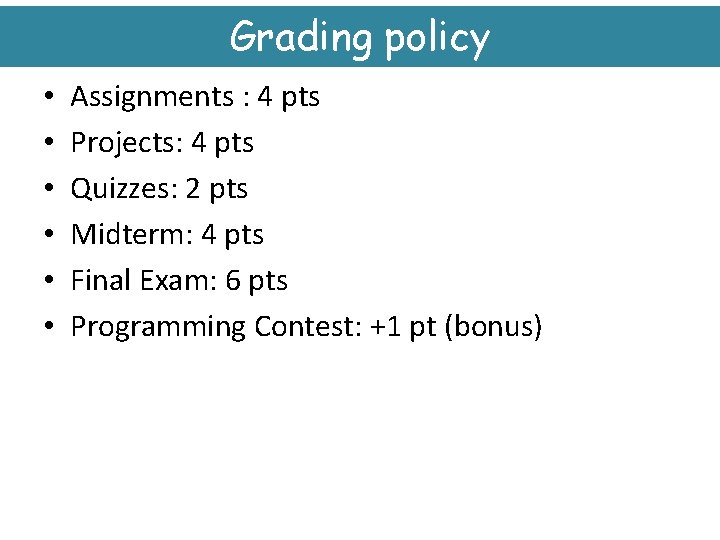
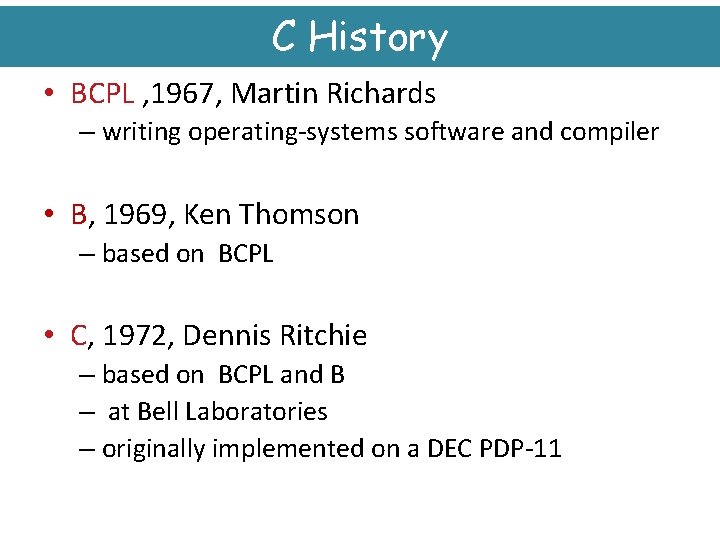
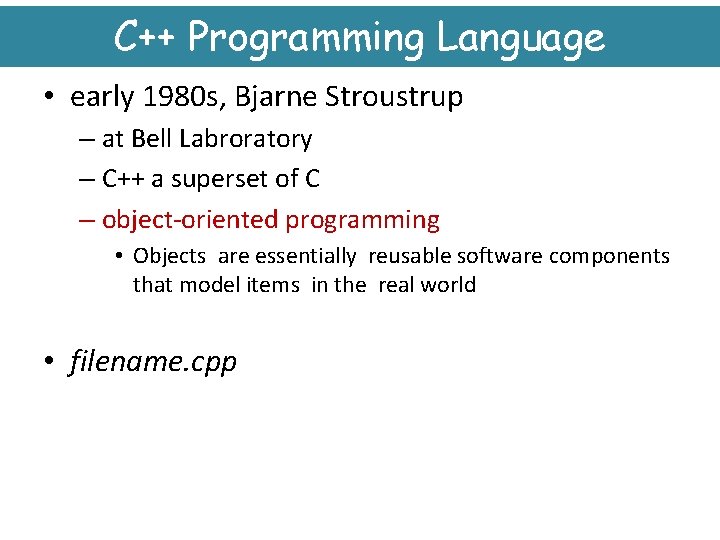
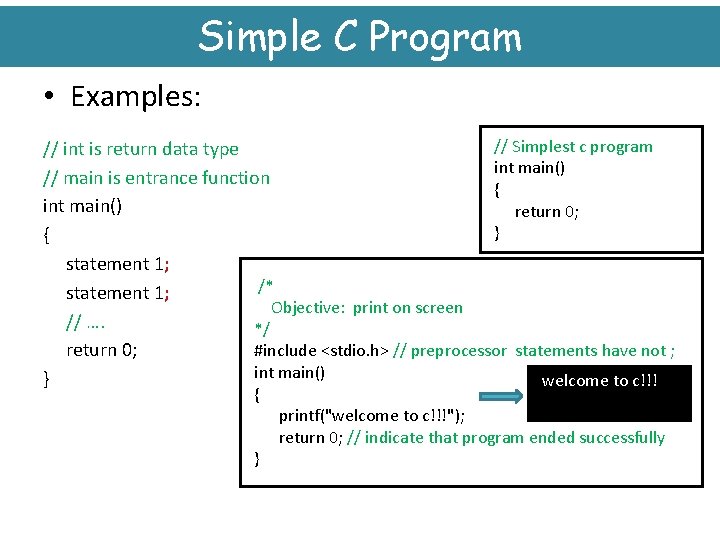
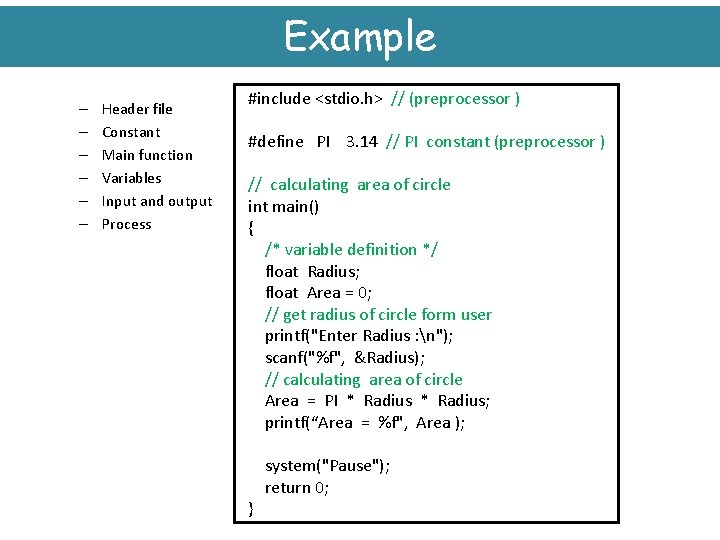
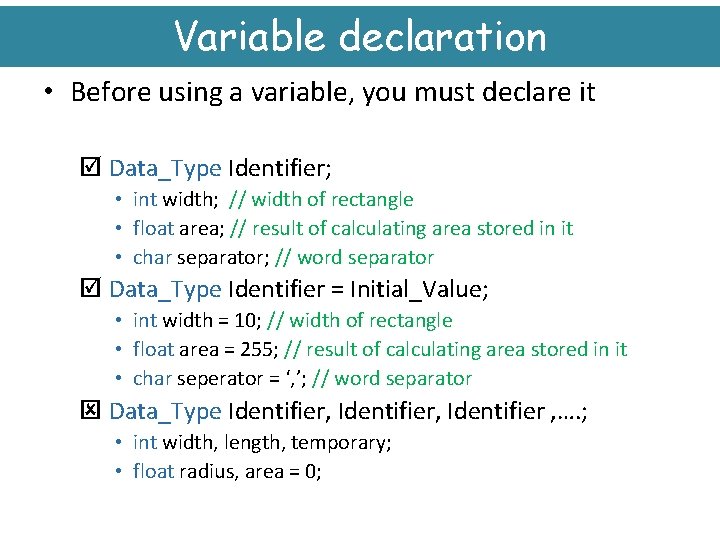
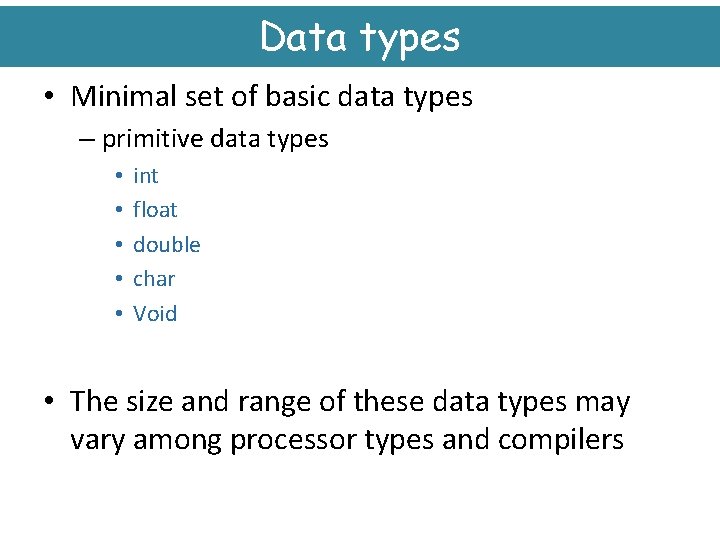
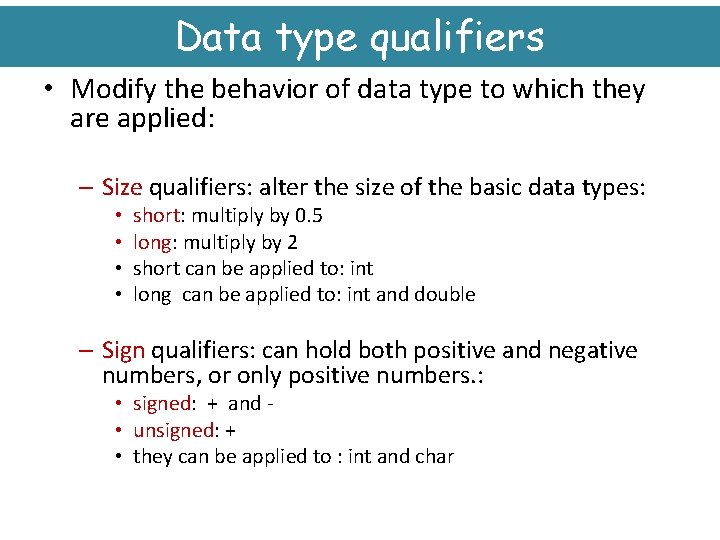
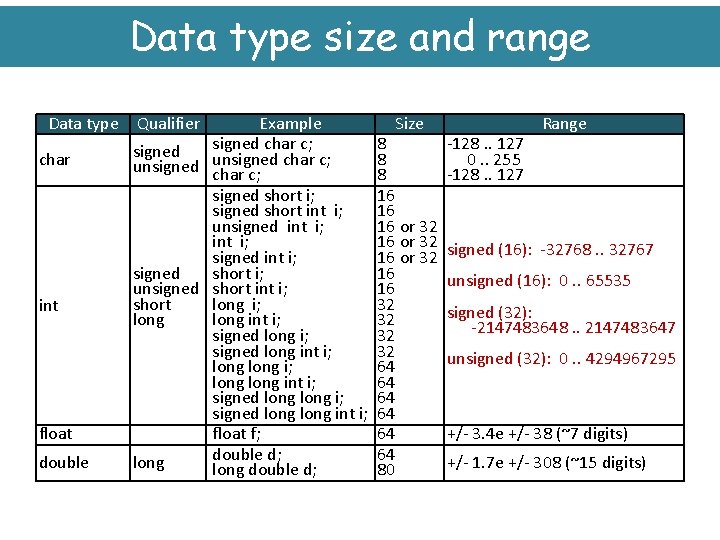
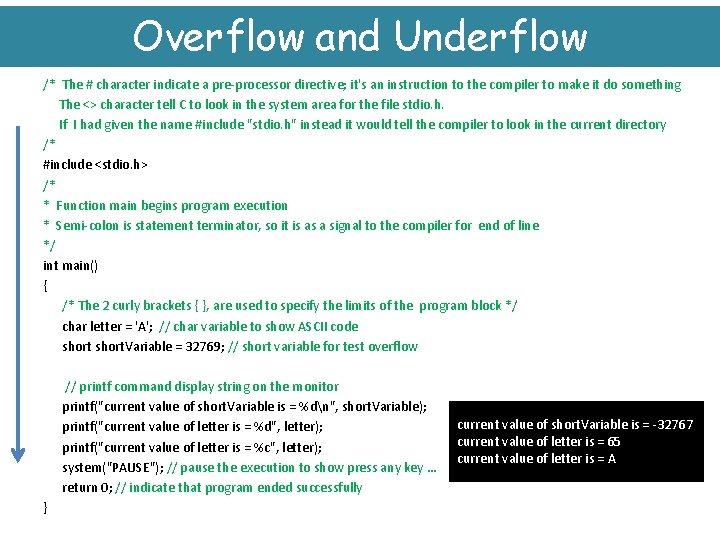
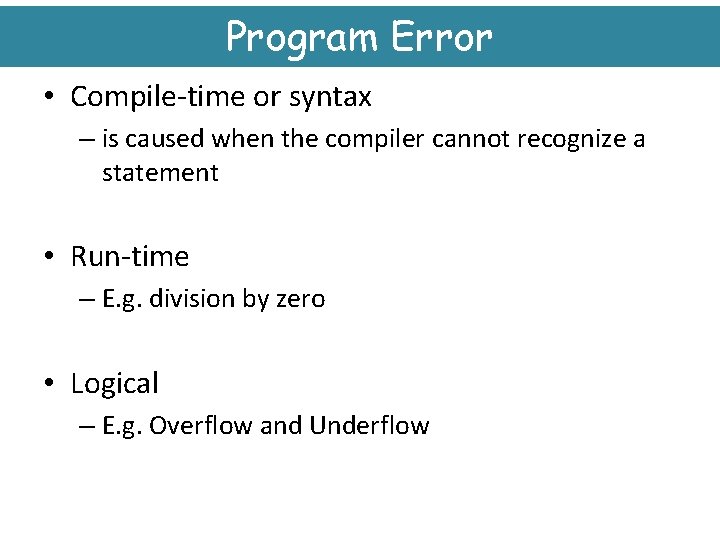
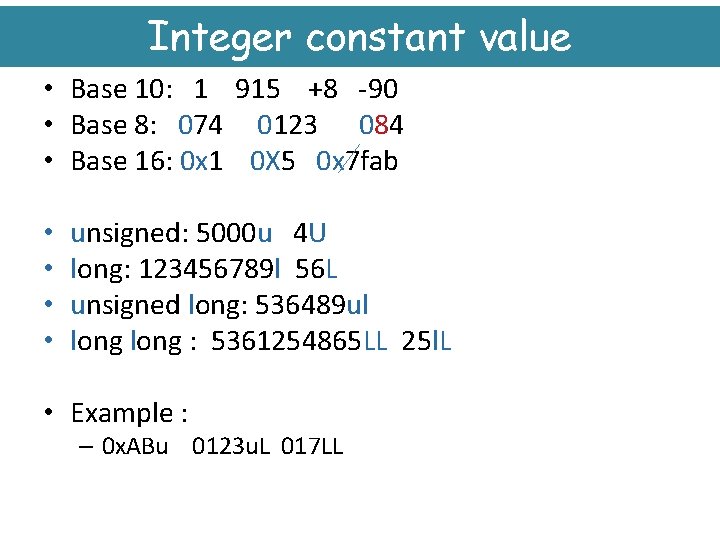
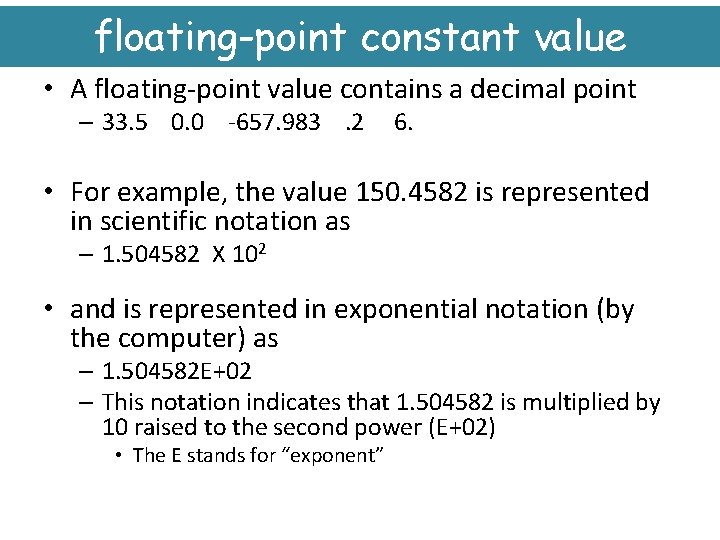
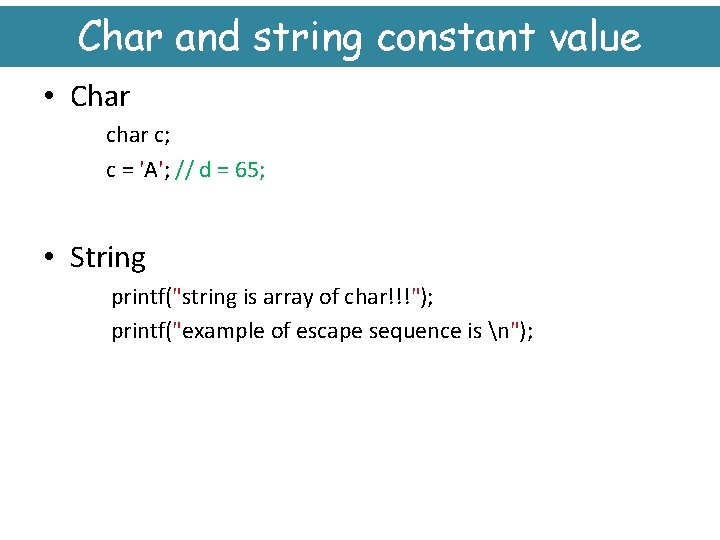
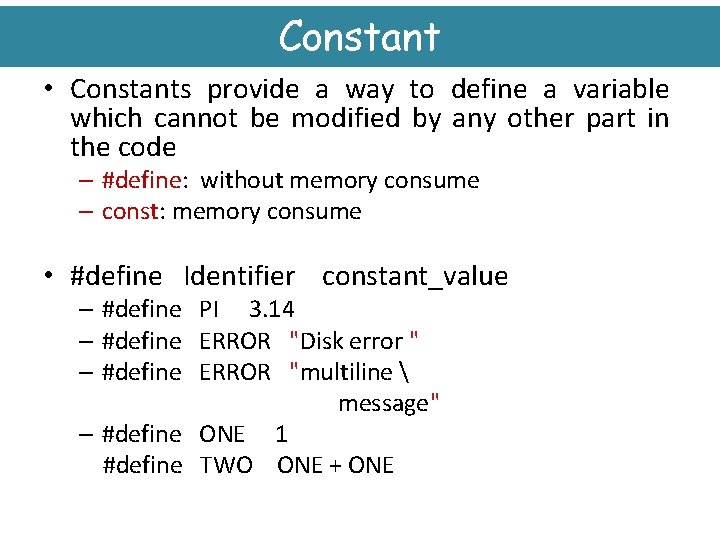
![Constant • const [Data_Type] Identifier = constant_value; – const p = 3; // const Constant • const [Data_Type] Identifier = constant_value; – const p = 3; // const](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-19.jpg)
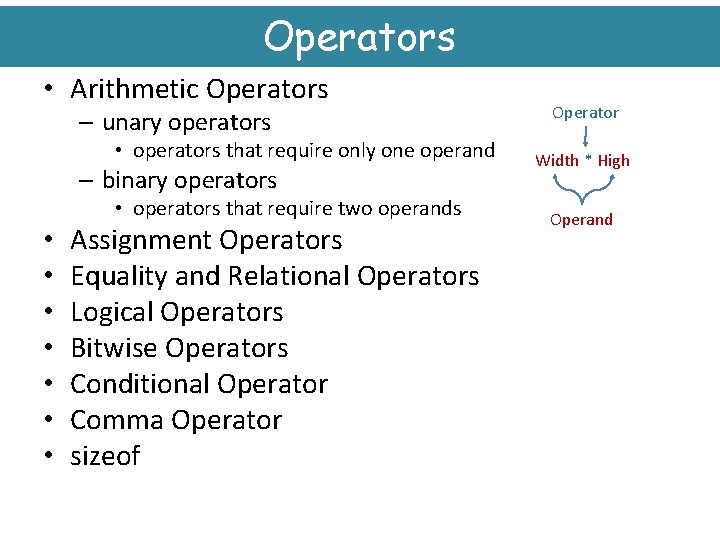
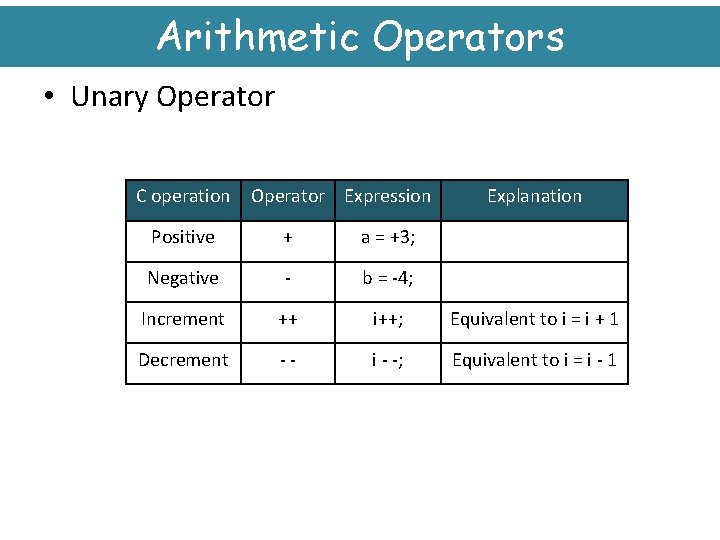
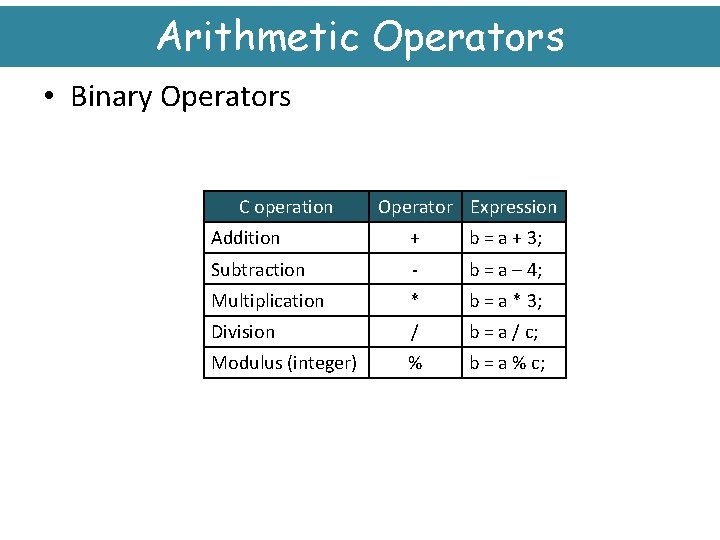
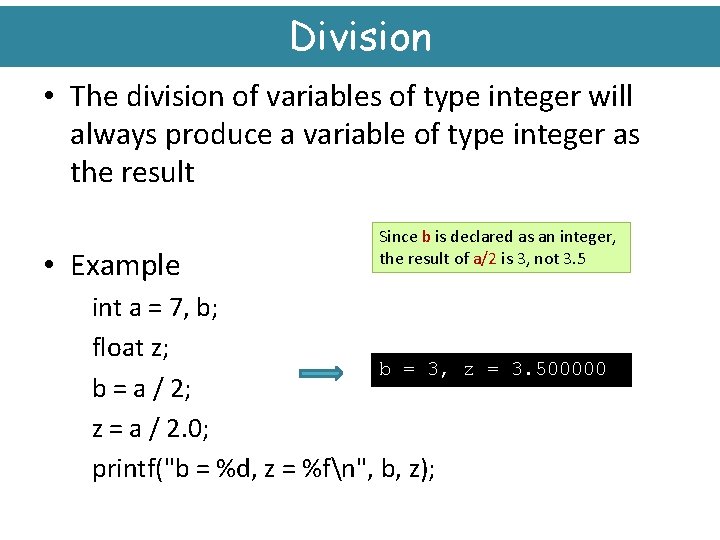
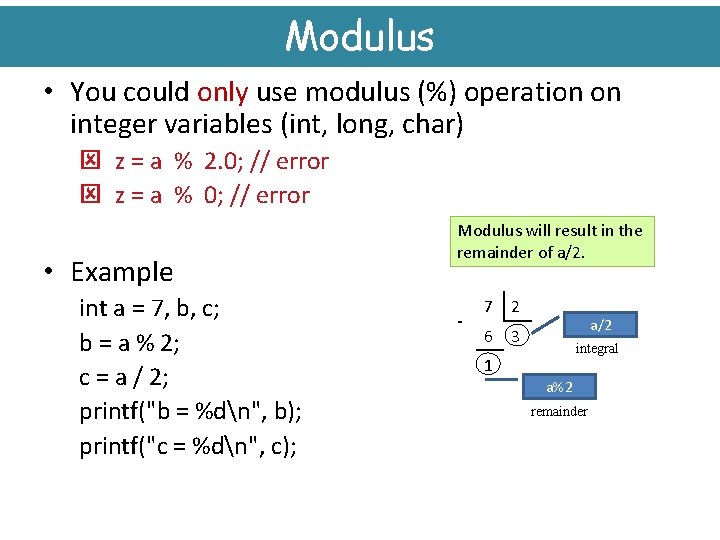
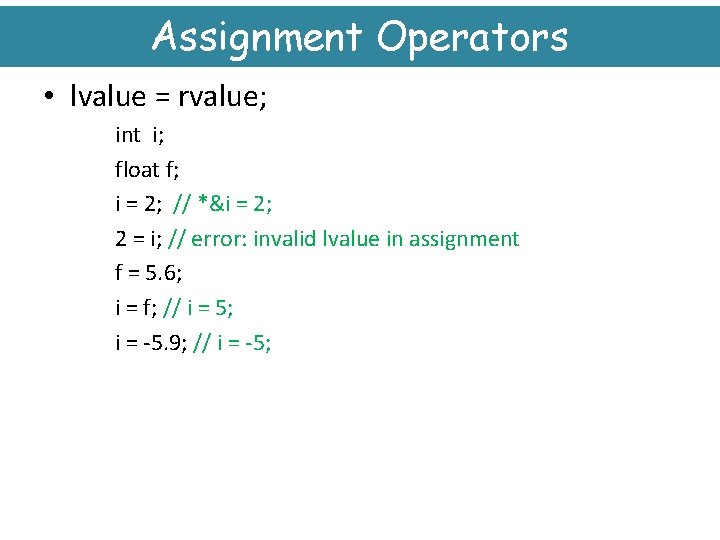
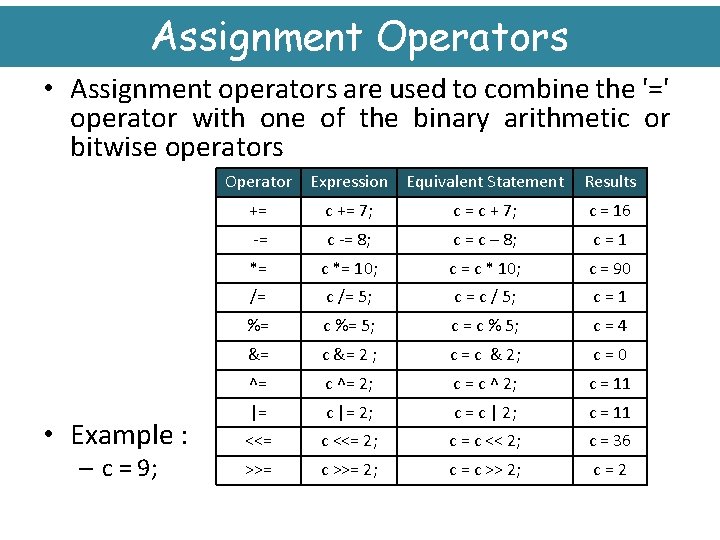
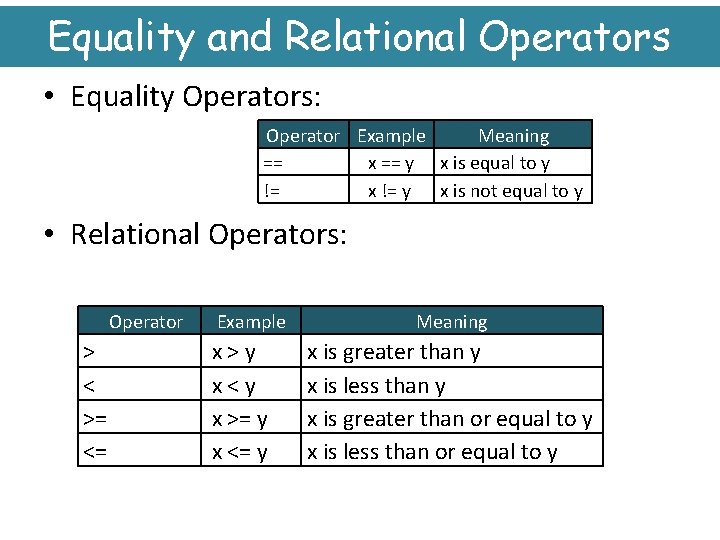
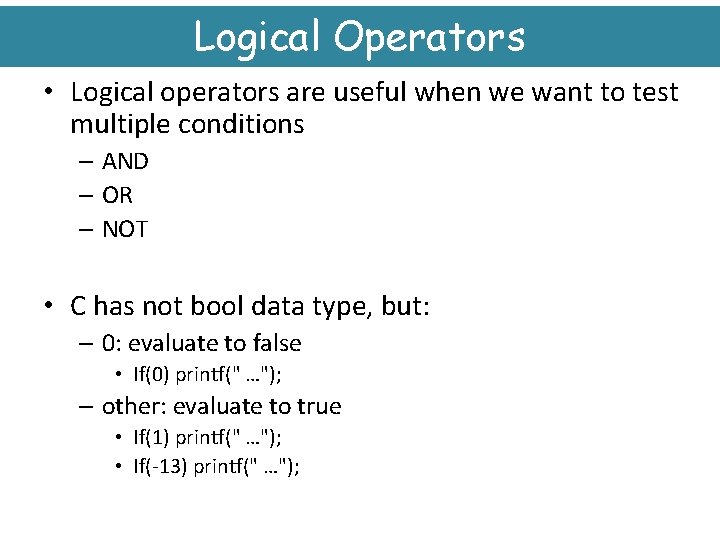
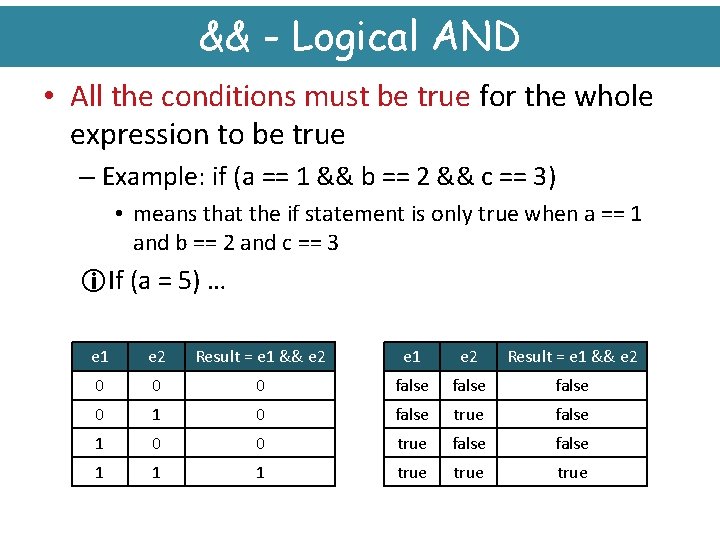
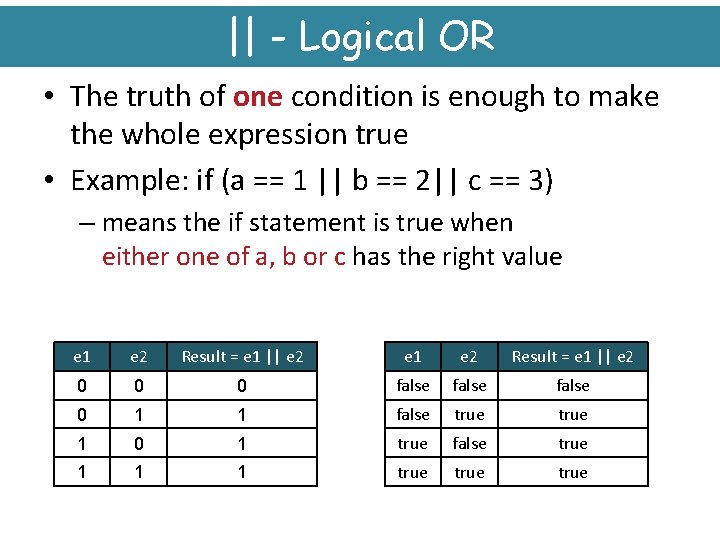
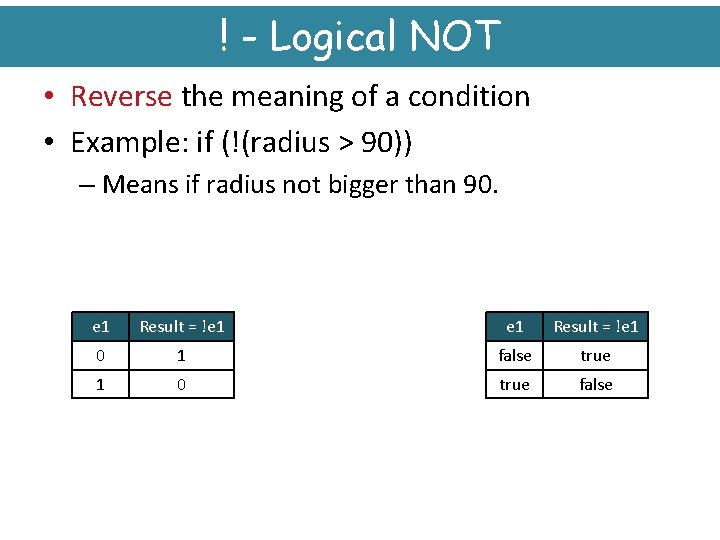
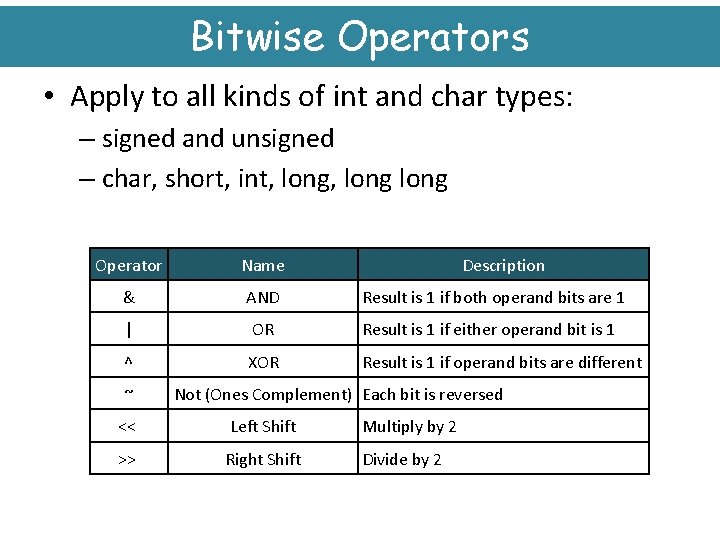
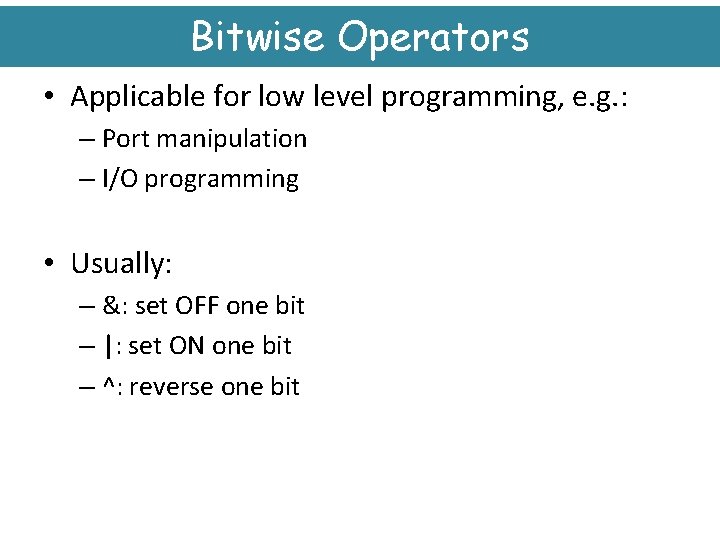
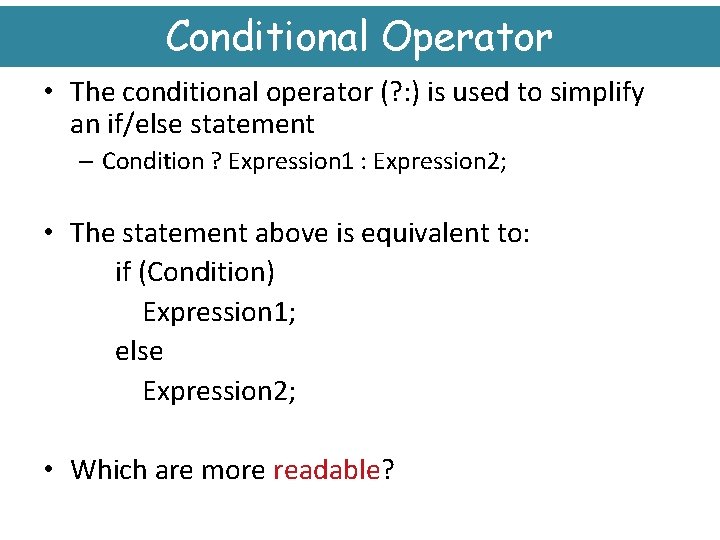
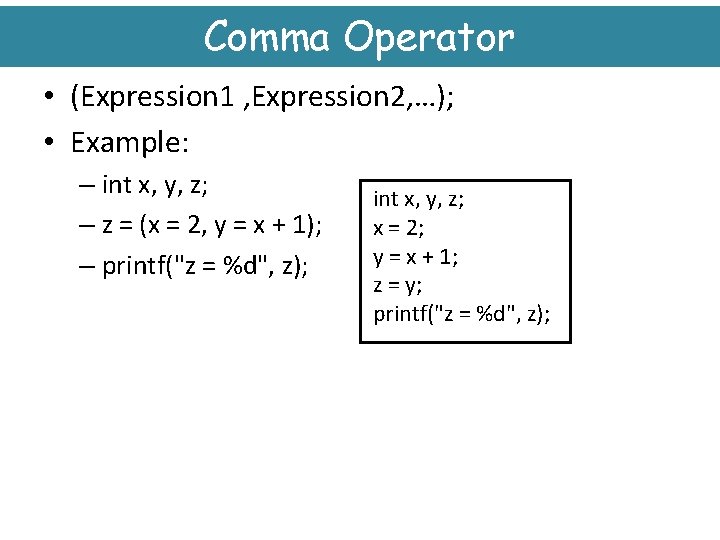
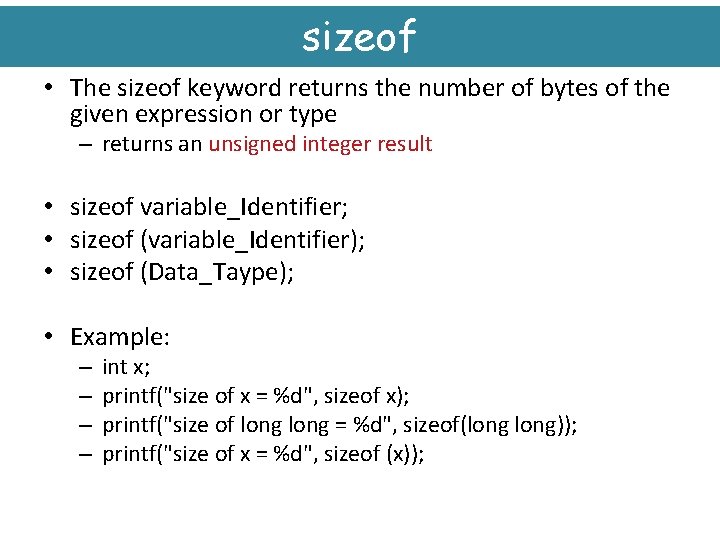
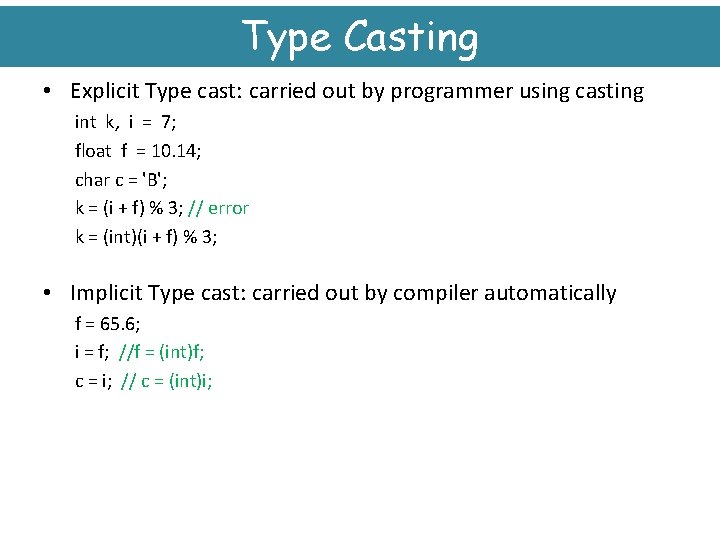
![Precedence Rules Primary Expression Operators () []. -> Unary Operators * & + left-to-right Precedence Rules Primary Expression Operators () []. -> Unary Operators * & + left-to-right](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-38.jpg)
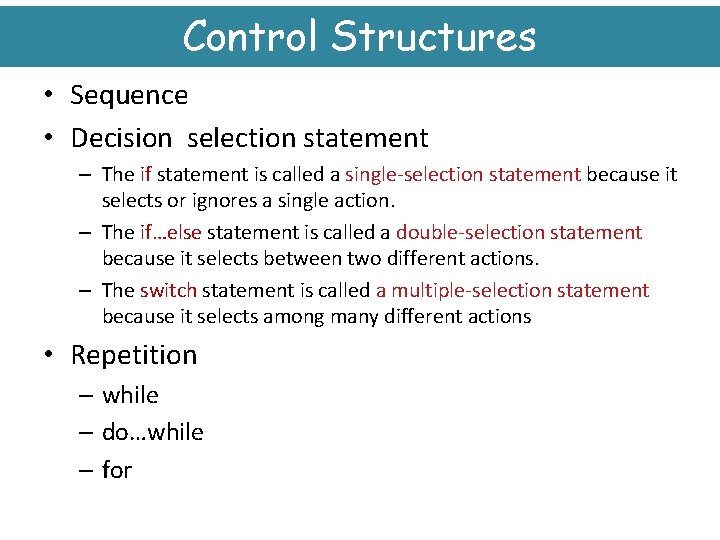
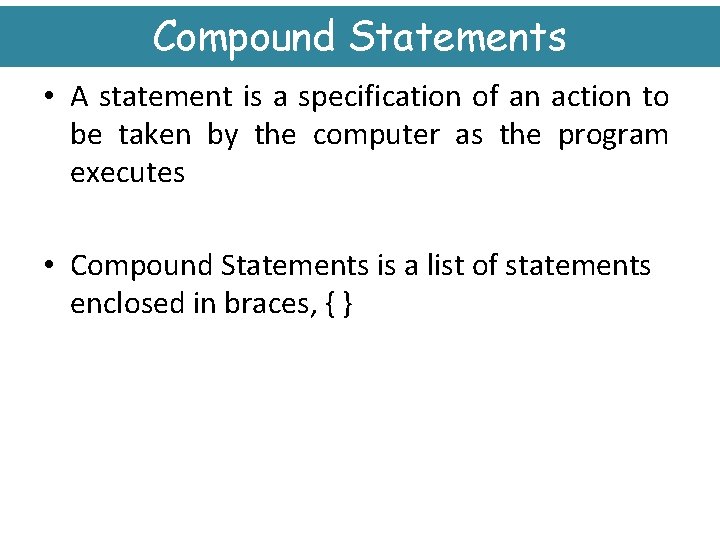
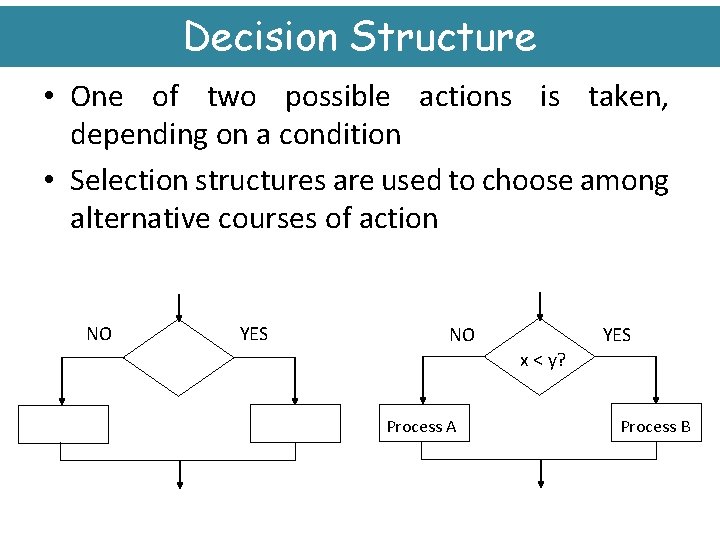
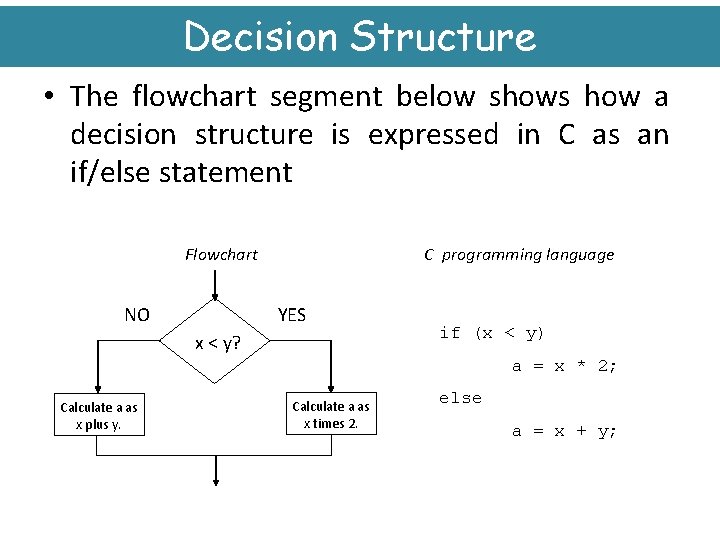
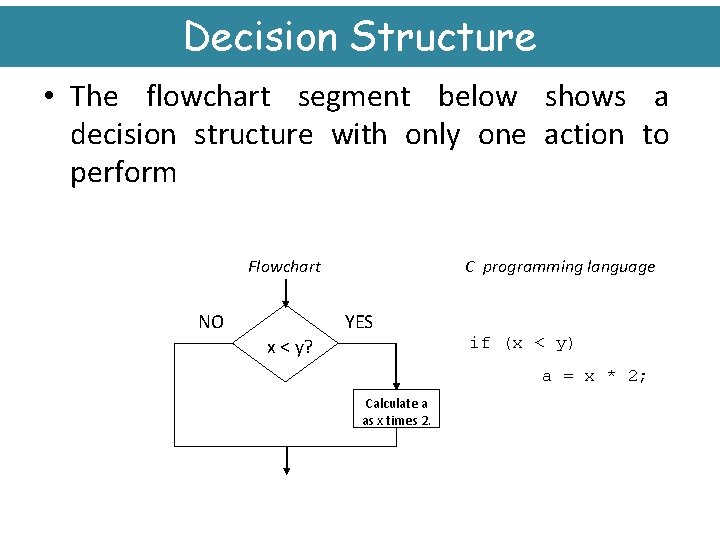
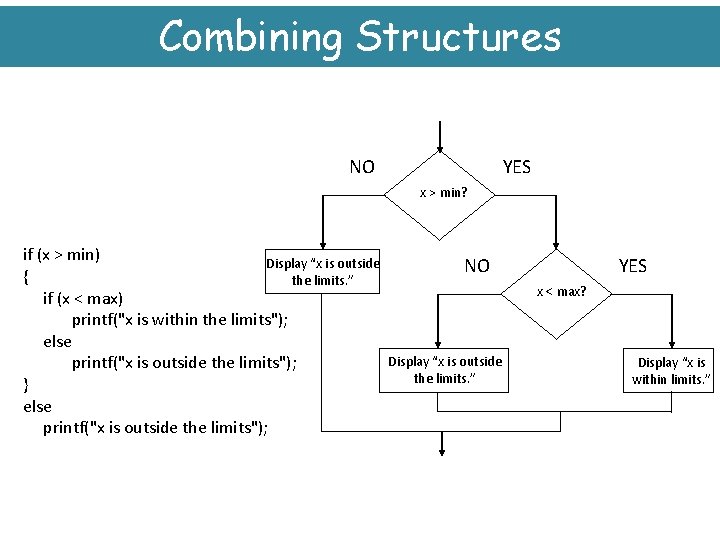
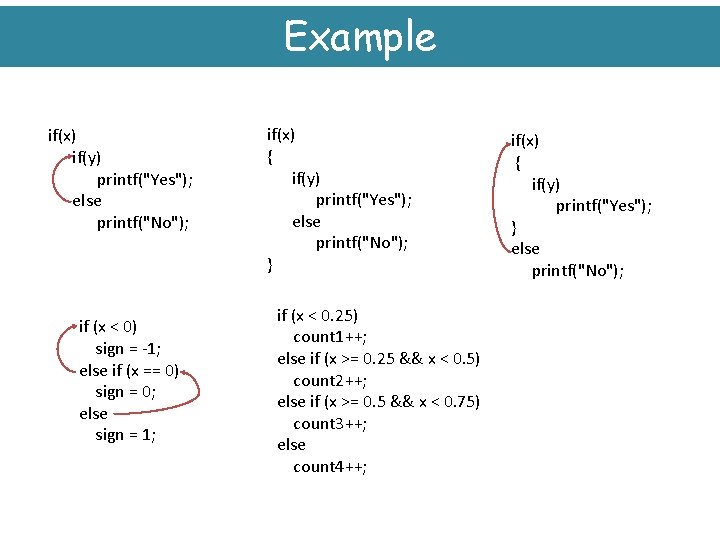
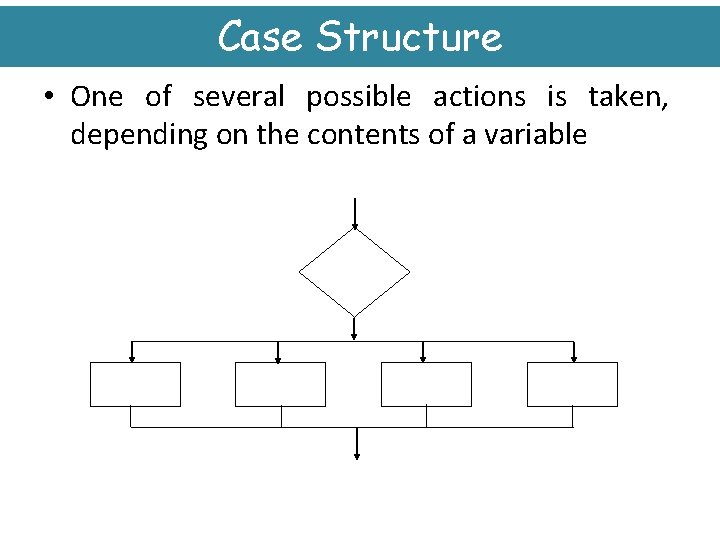
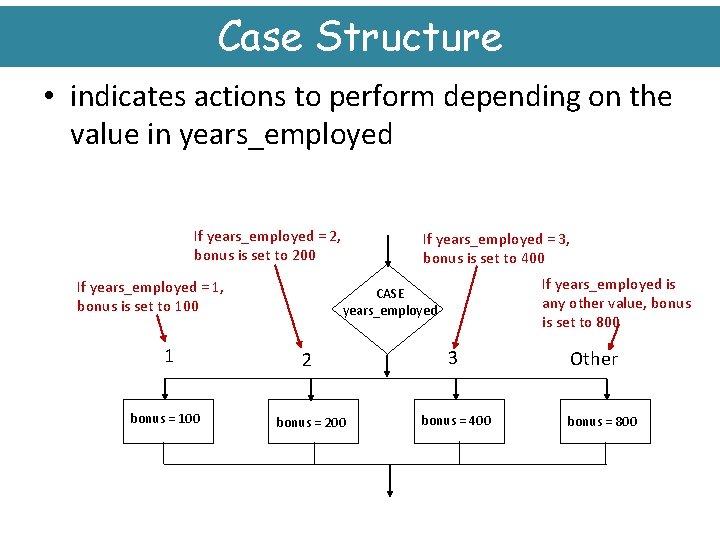
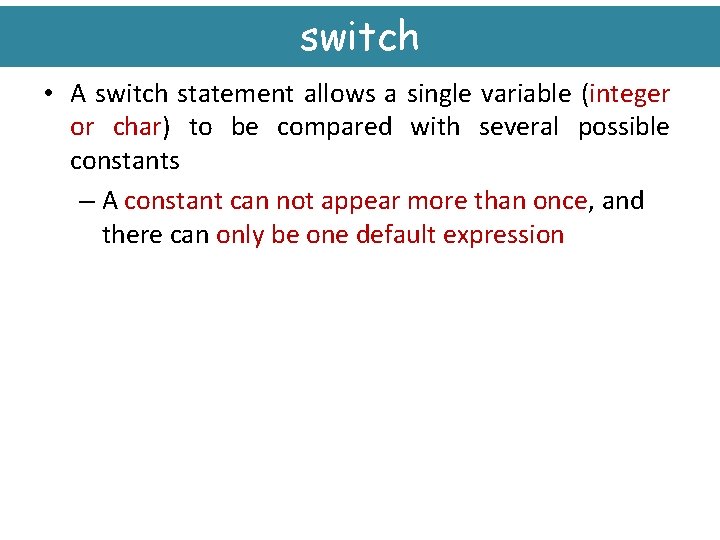
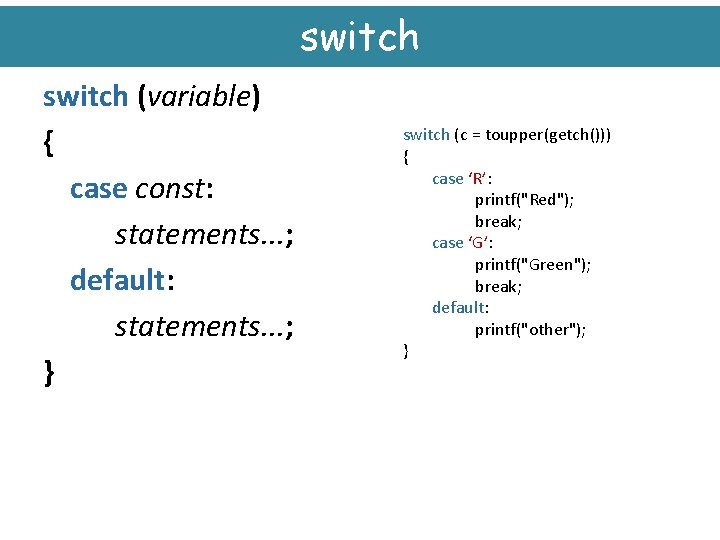
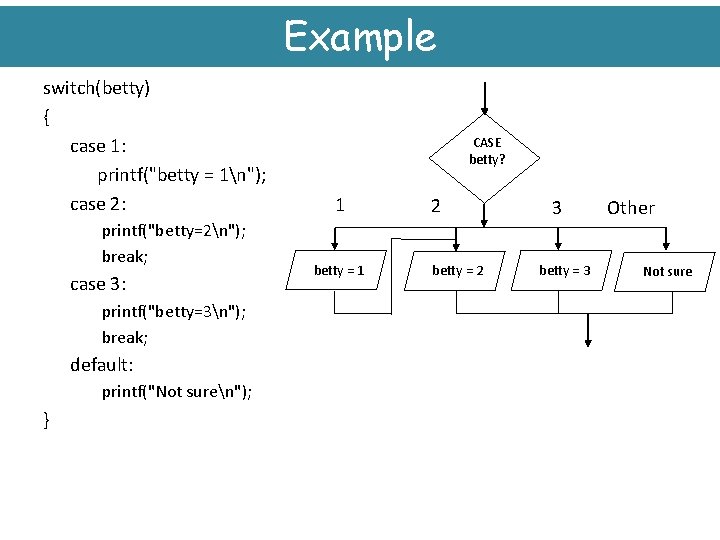
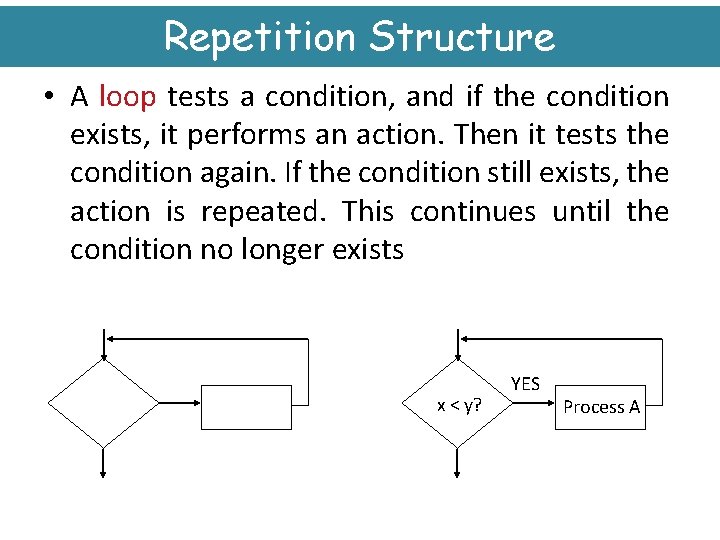
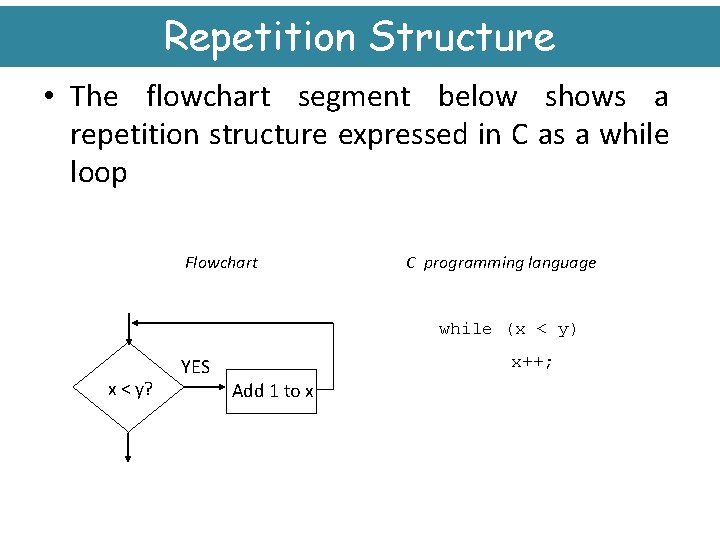
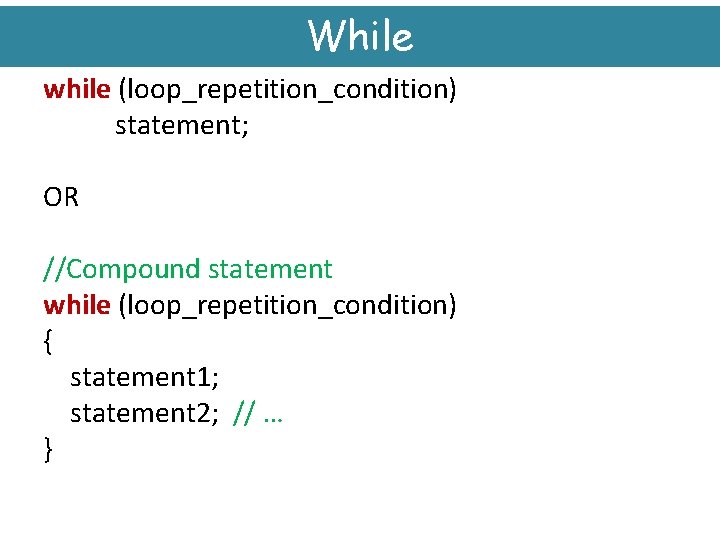
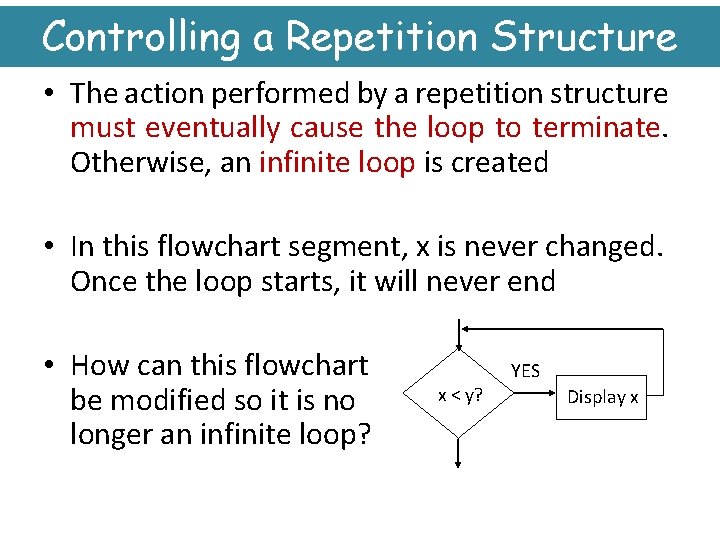
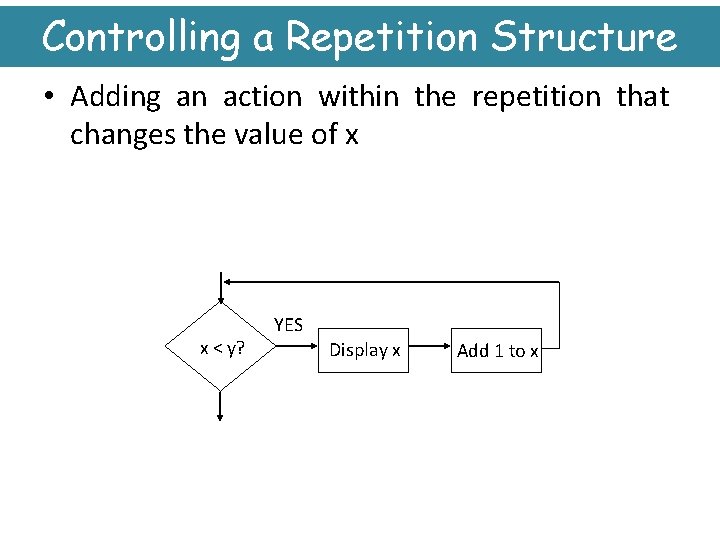
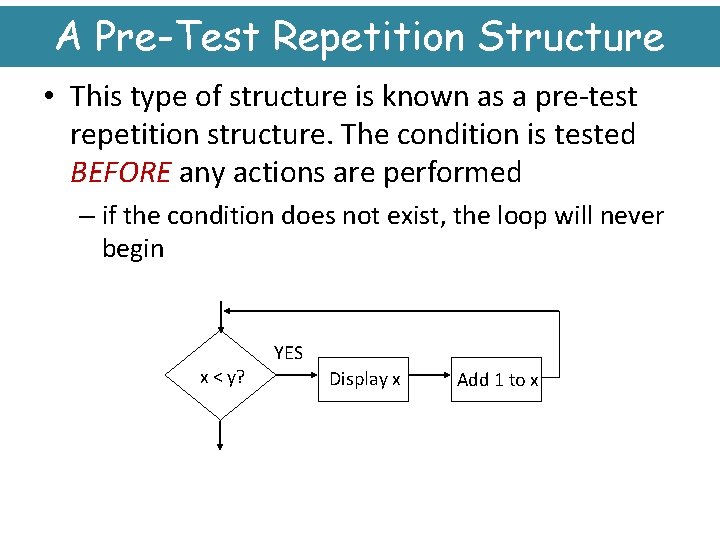
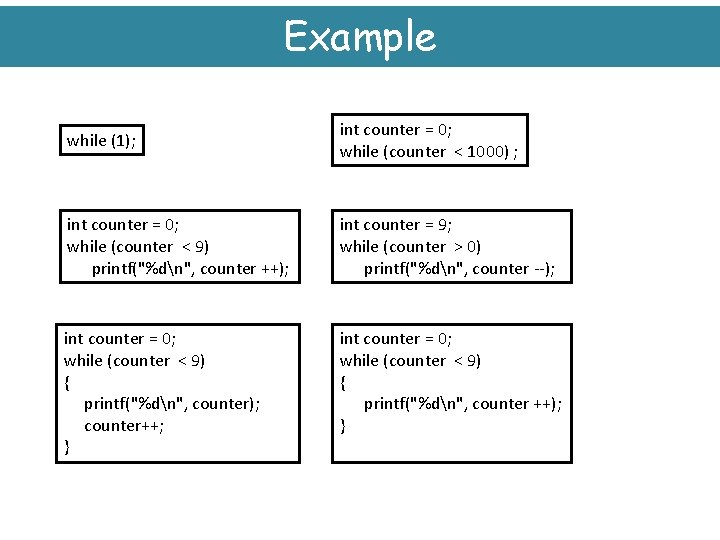
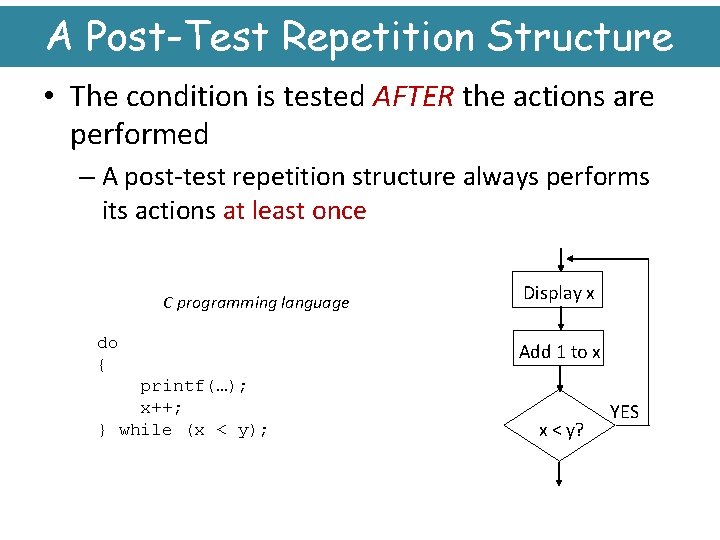
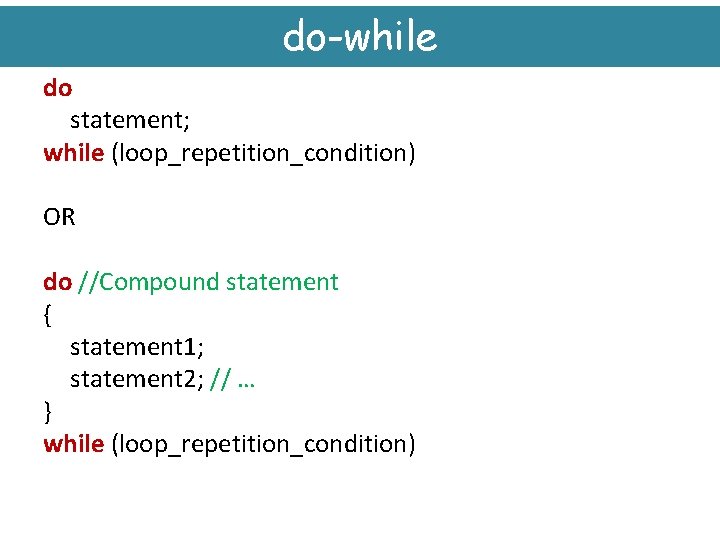
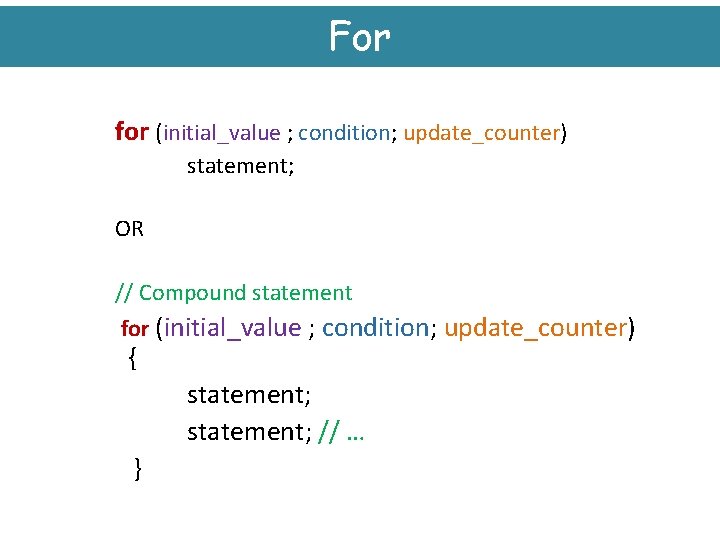
![Array • Generic declaration: typename variablename[size]; – – – typename is any type variablename Array • Generic declaration: typename variablename[size]; – – – typename is any type variablename](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-61.jpg)
![Array Representation int A[3]; 0 x 1008 A[2] 0 x 1004 A[1] 0 x Array Representation int A[3]; 0 x 1008 A[2] 0 x 1004 A[1] 0 x](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-62.jpg)
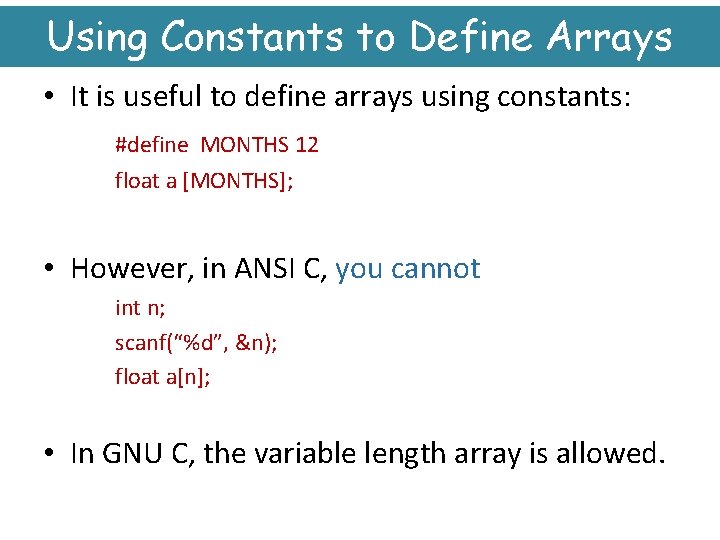
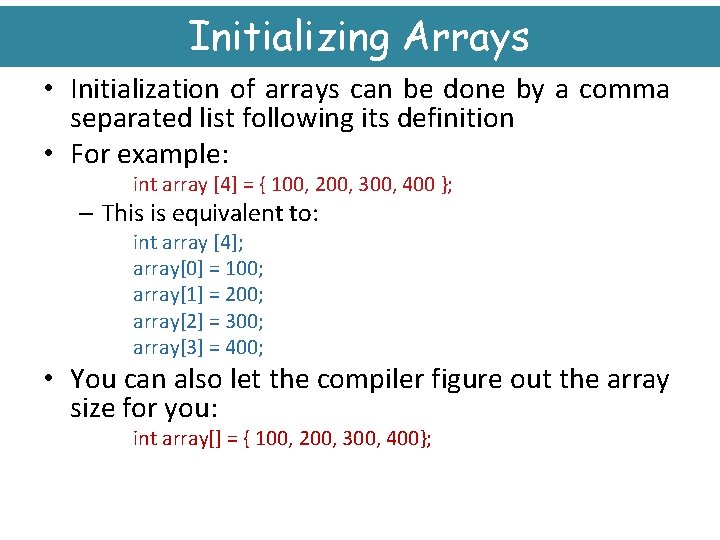
![Initializing Arrays • For example: int array [4] = { 100, 200 }; • Initializing Arrays • For example: int array [4] = { 100, 200 }; •](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-65.jpg)
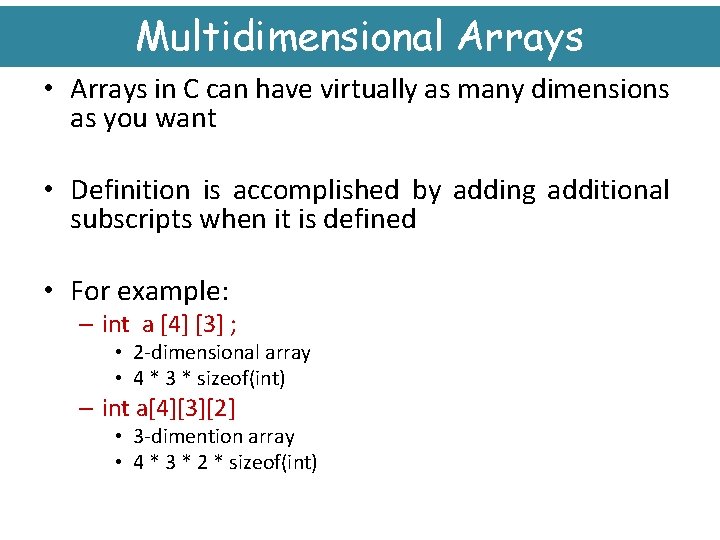
![Multidimensional Arrays Representation a[0][0] a[0][1] a[0][2] a[0][3] Row 0 Row 1 Row 2 Column Multidimensional Arrays Representation a[0][0] a[0][1] a[0][2] a[0][3] Row 0 Row 1 Row 2 Column](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-67.jpg)
![Initializing Multidimensional Arrays • The following initializes a[4][3]: int a[4] [3] = { {1, Initializing Multidimensional Arrays • The following initializes a[4][3]: int a[4] [3] = { {1,](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-68.jpg)
![Examples • Initialization – Example: • short b[ 2 ] = { { 1, Examples • Initialization – Example: • short b[ 2 ] = { { 1,](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-69.jpg)
![Examples int a[10]; // Ok int a[2. 5]; // Syntax error int a[-5]; // Examples int a[10]; // Ok int a[2. 5]; // Syntax error int a[-5]; //](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-70.jpg)
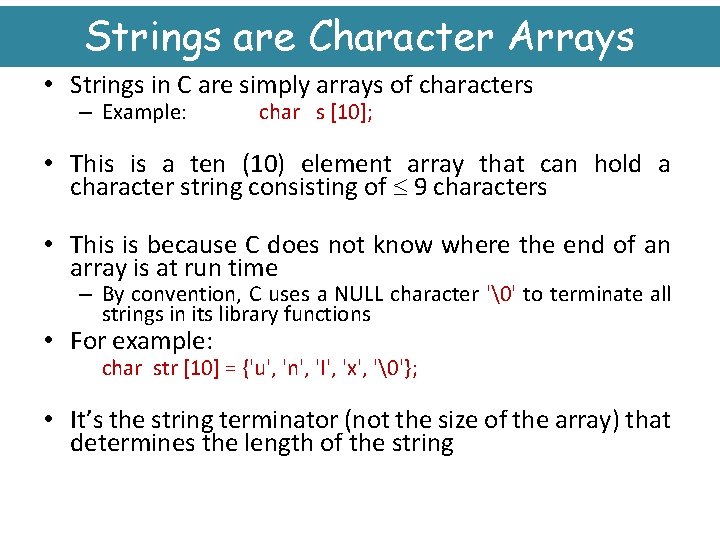
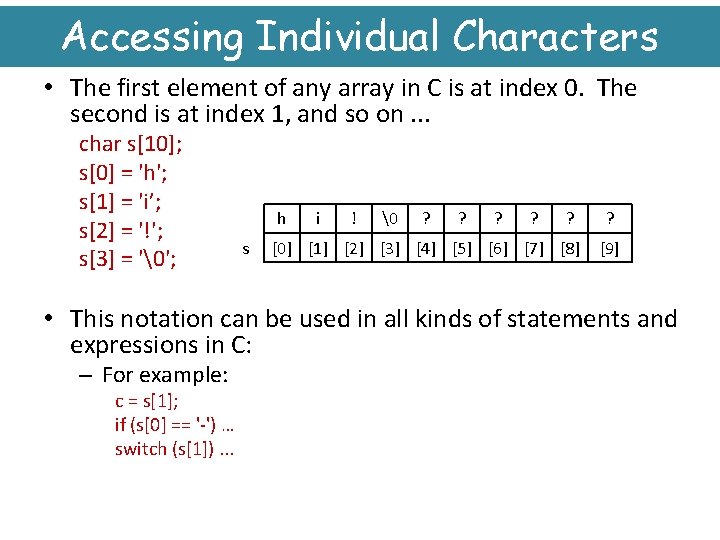
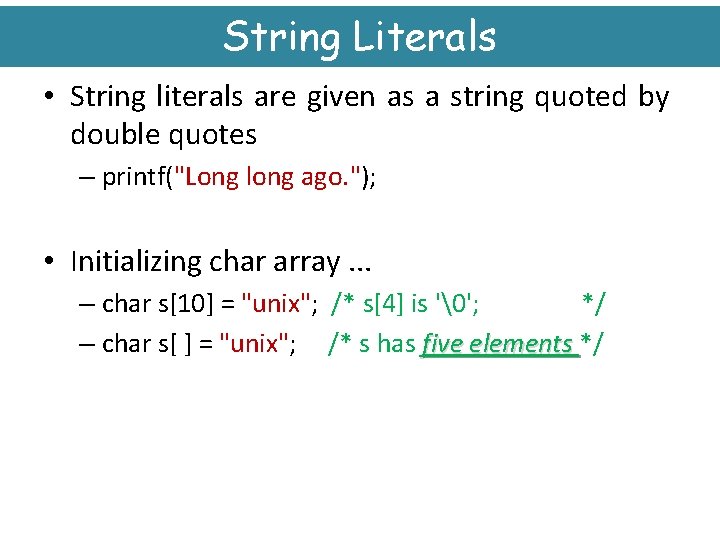
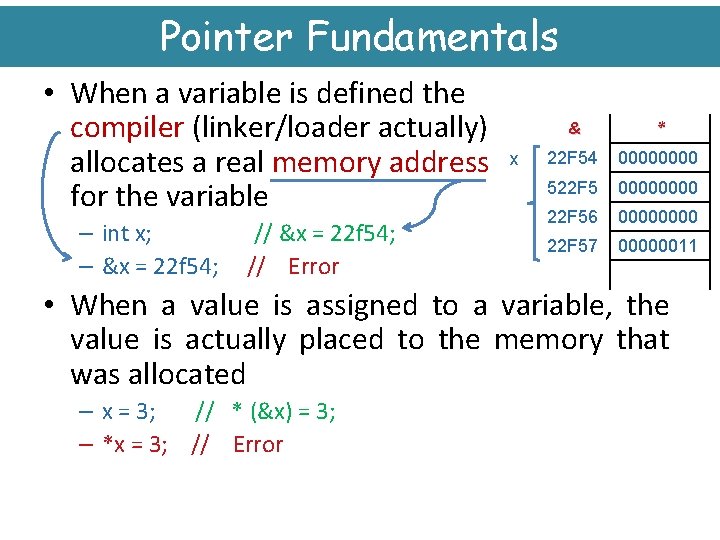
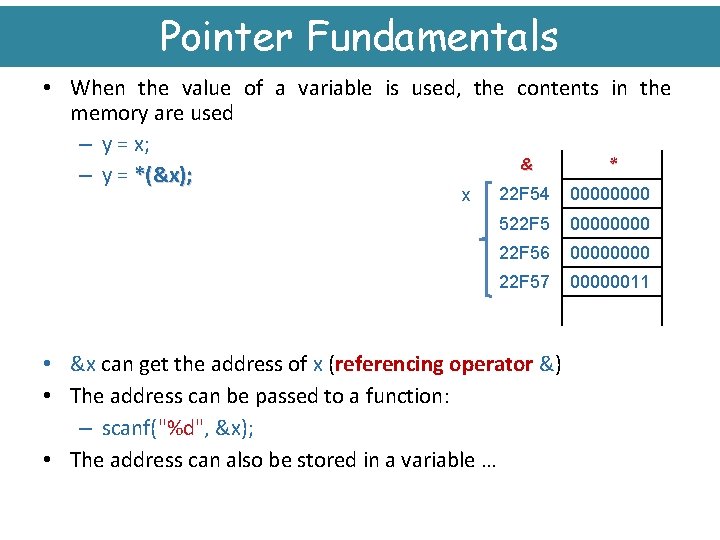
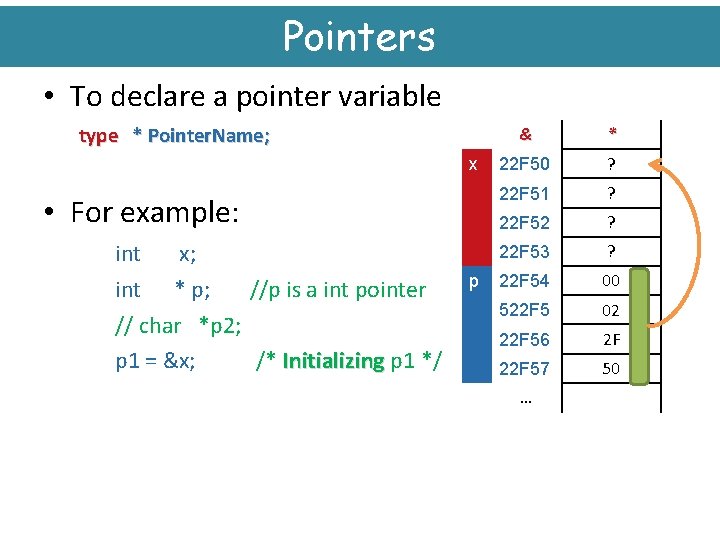
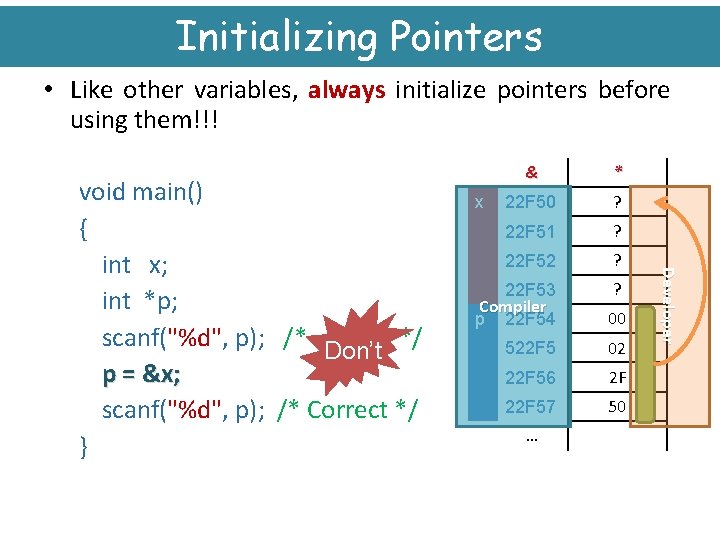
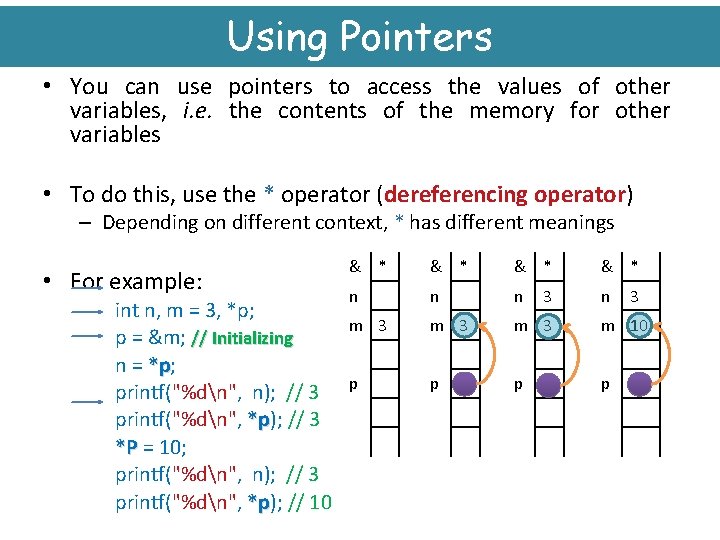
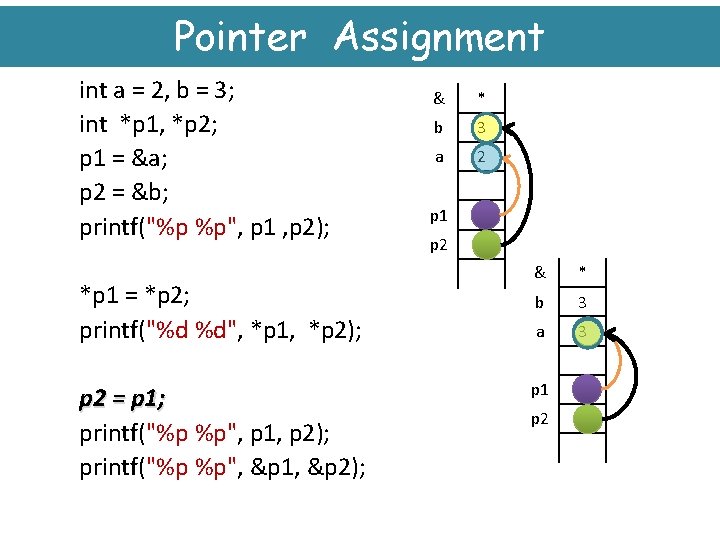
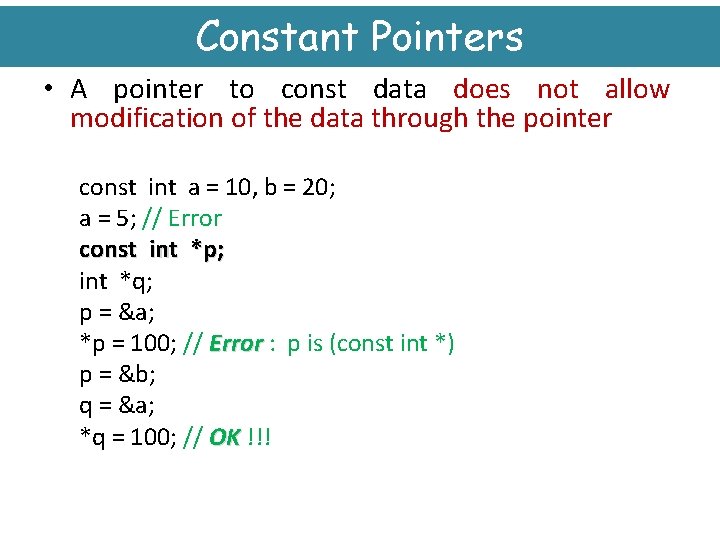
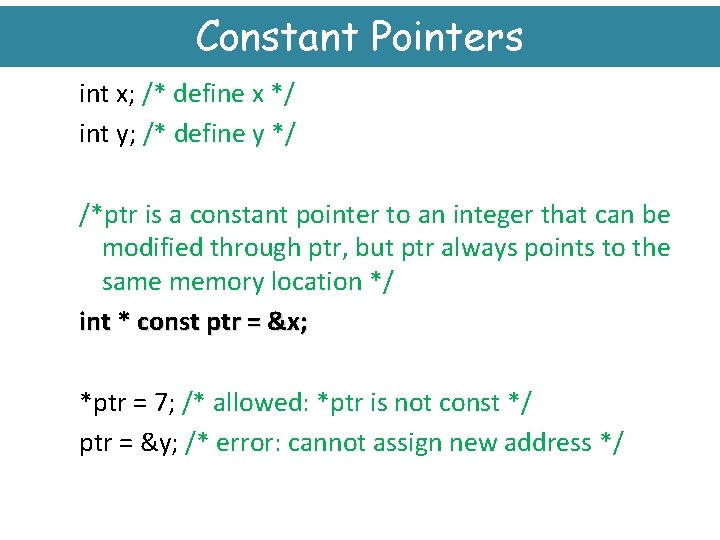
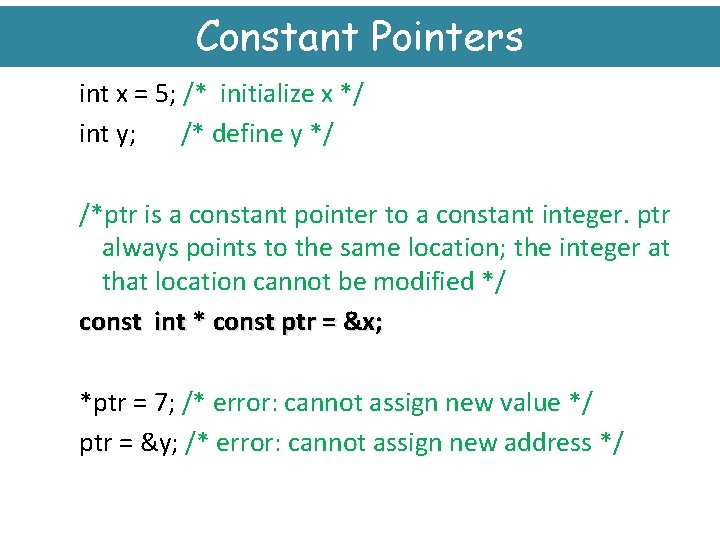
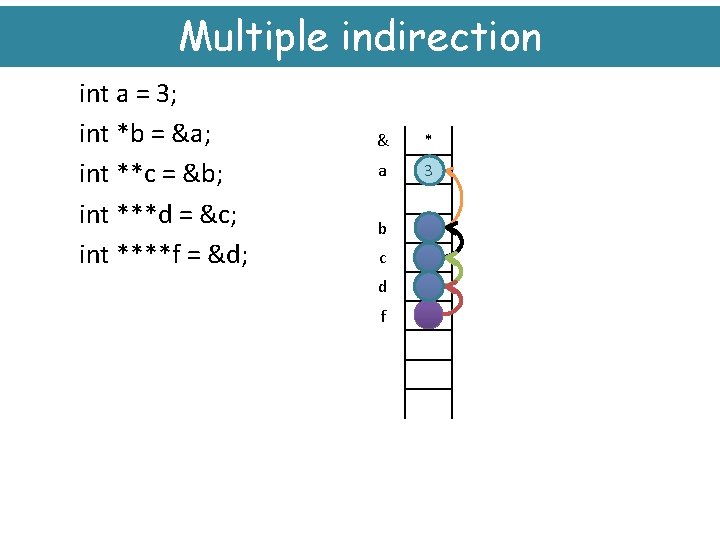
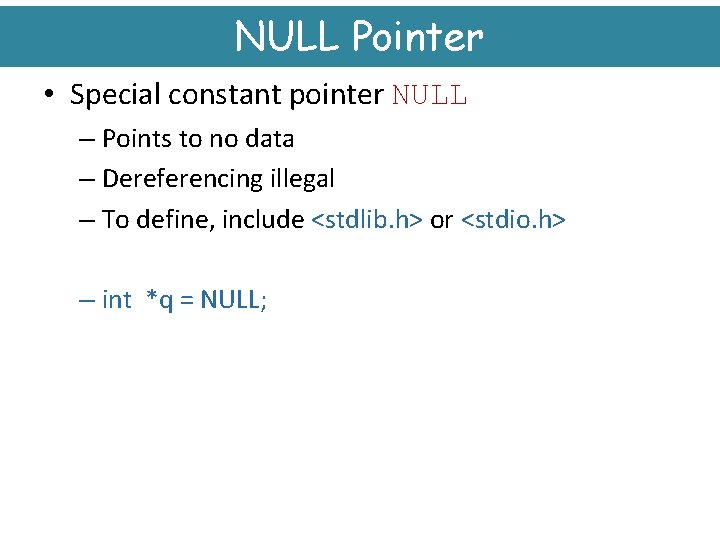
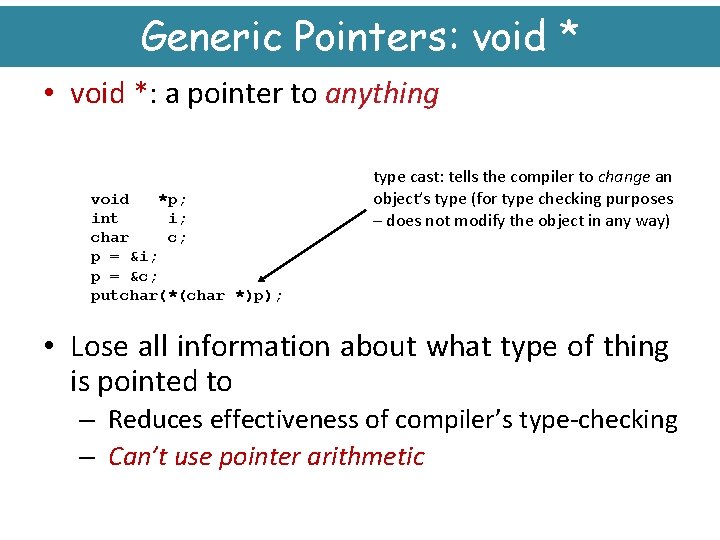
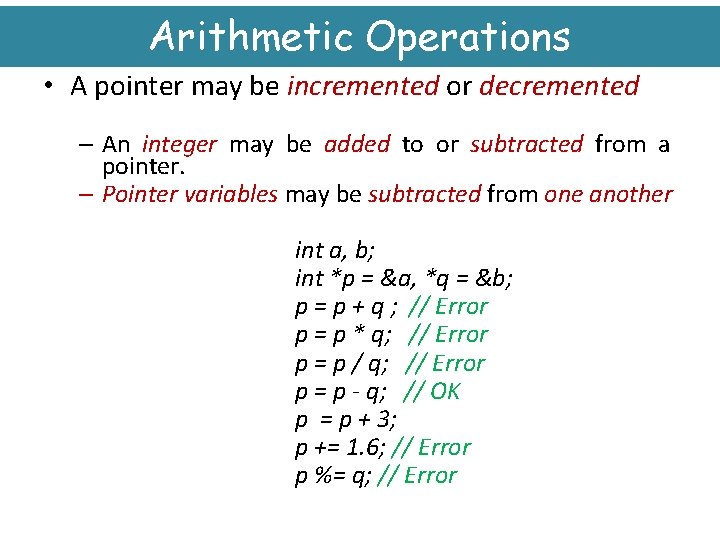
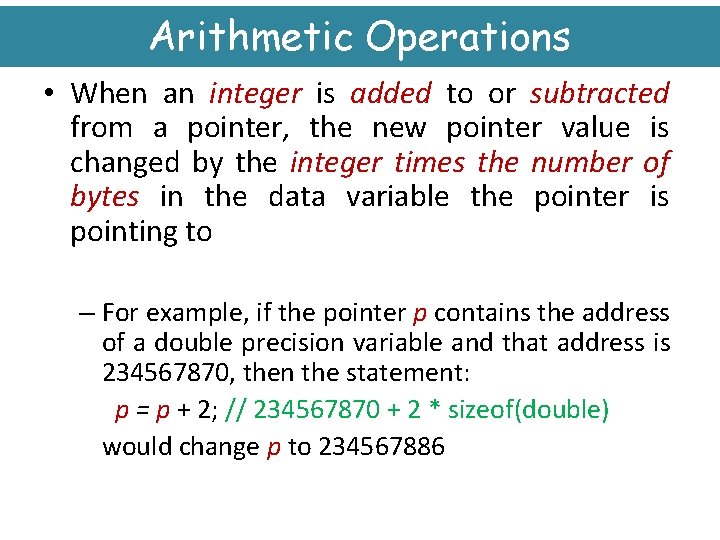
![Logical Operations • Pointers can be used in comparisons int a[10], *p, *q , Logical Operations • Pointers can be used in comparisons int a[10], *p, *q ,](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-88.jpg)
![Pointers and Arrays Array pointer to the initial (0 th) array element a &a[0] Pointers and Arrays Array pointer to the initial (0 th) array element a &a[0]](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-89.jpg)
![Pointers and Arrays Array pointer to the initial (0 th) array element a &a[0] Pointers and Arrays Array pointer to the initial (0 th) array element a &a[0]](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-90.jpg)
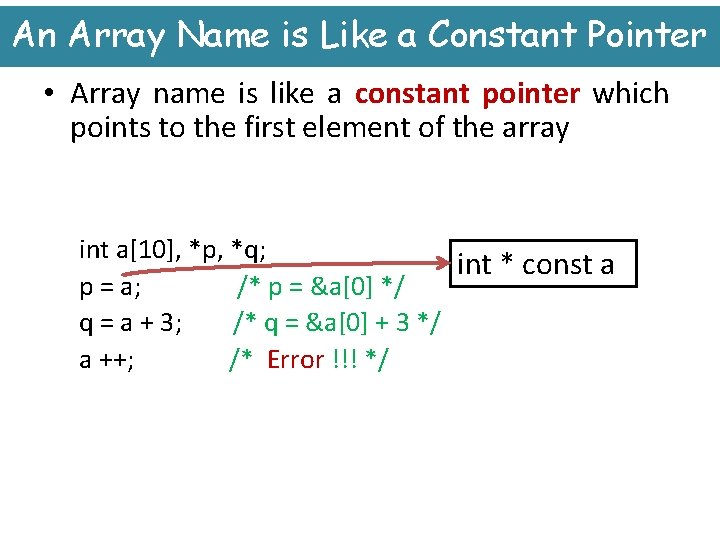
![Example int a[10], i; int *p = a; // int *p = &a[0]; for Example int a[10], i; int *p = a; // int *p = &a[0]; for](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-92.jpg)
![An example int a[10], *p, *q; p = &a[2]; q = p + 3; An example int a[10], *p, *q; p = &a[2]; q = p + 3;](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-93.jpg)
![An Example int a[10], *p; a++; //Error a--; // Error a += 3; //Error An Example int a[10], *p; a++; //Error a--; // Error a += 3; //Error](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-94.jpg)
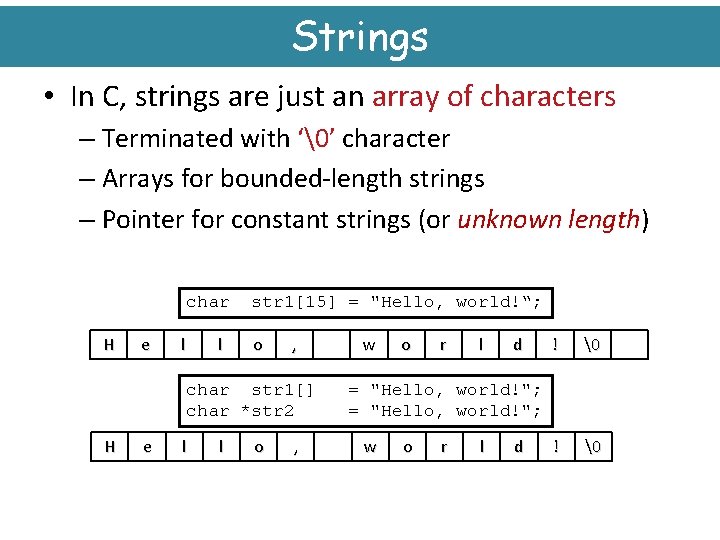
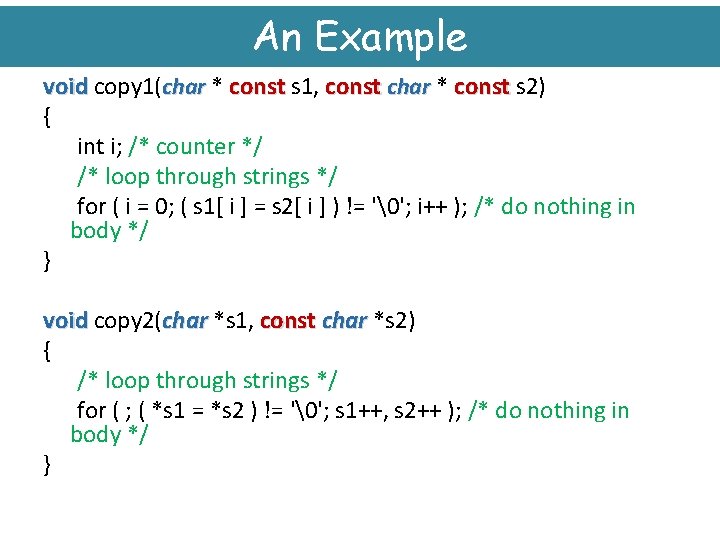
![Multi-Dimensional Arrays int a[row][col]; a[row][col] *(*(a + row) + col) scanf(" %d ", &a[0][0]) Multi-Dimensional Arrays int a[row][col]; a[row][col] *(*(a + row) + col) scanf(" %d ", &a[0][0])](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-97.jpg)
![Array of Pointers char *suit[ 4 ] = { "Hearts", "Diamonds", "Clubs", "Spades" }; Array of Pointers char *suit[ 4 ] = { "Hearts", "Diamonds", "Clubs", "Spades" };](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-98.jpg)
![Array of Pointers int a=1, b=2, c=3, d=4; int *k[4] = {&a, &b, &c, Array of Pointers int a=1, b=2, c=3, d=4; int *k[4] = {&a, &b, &c,](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-99.jpg)
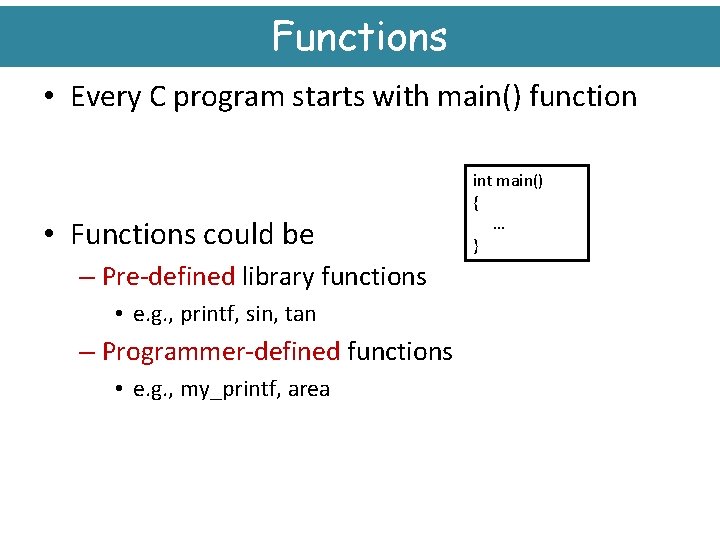
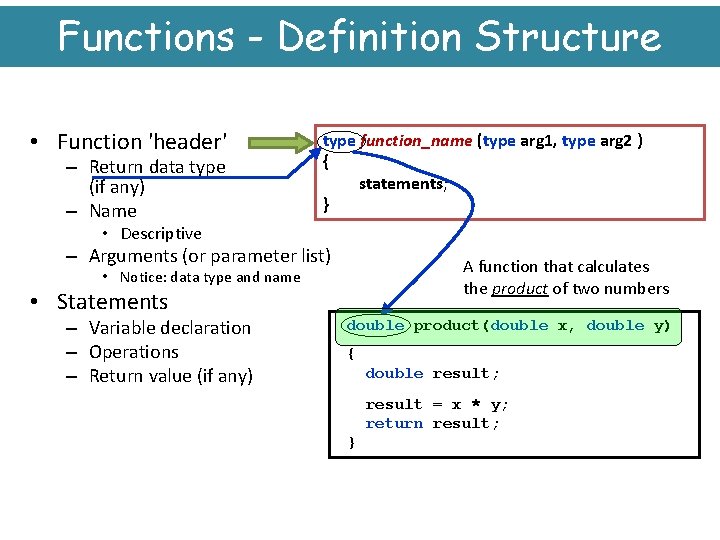
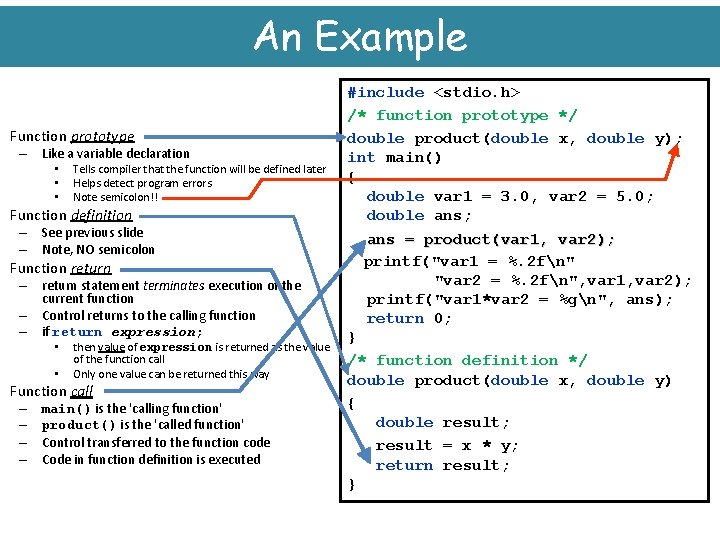
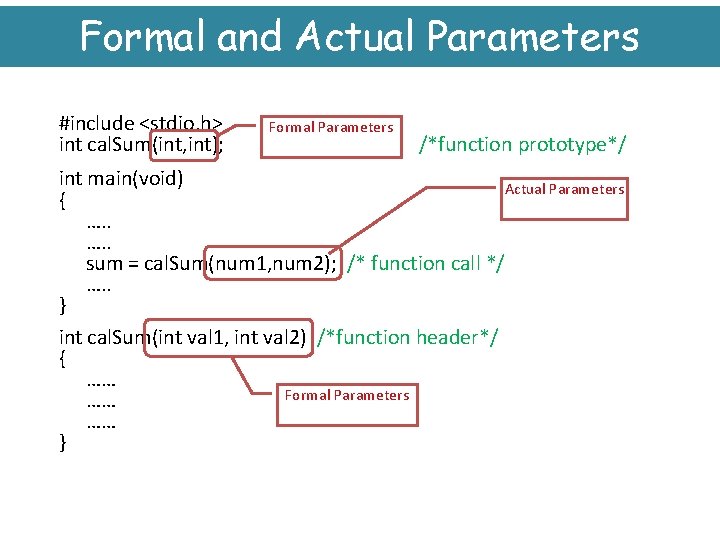
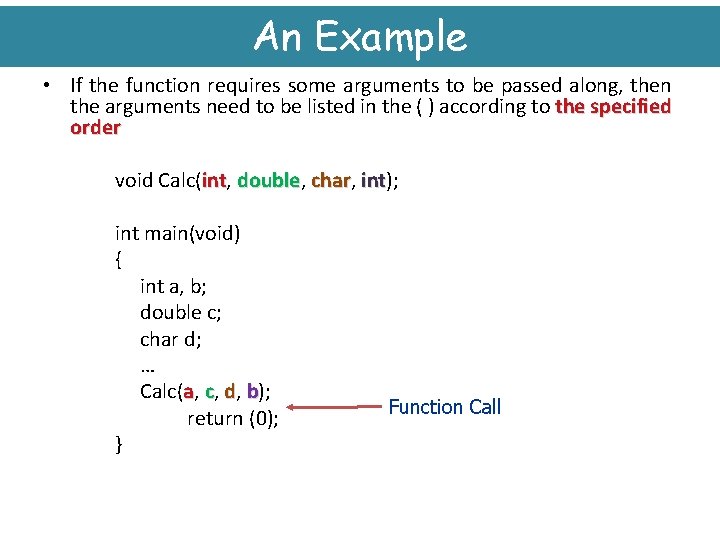
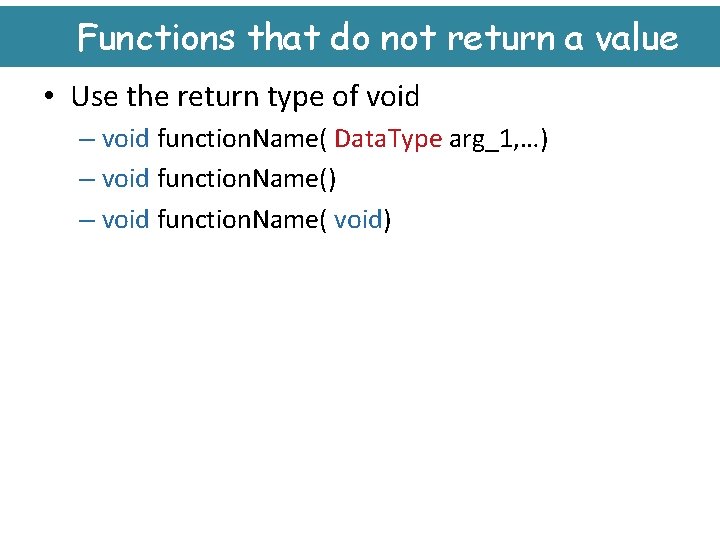
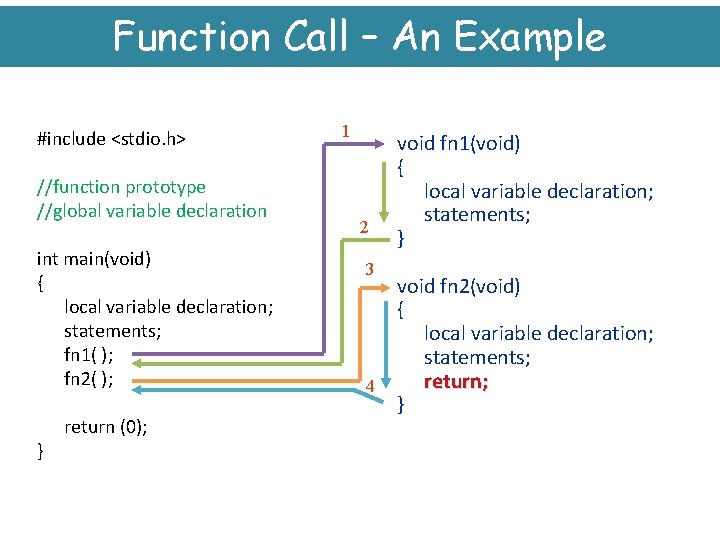
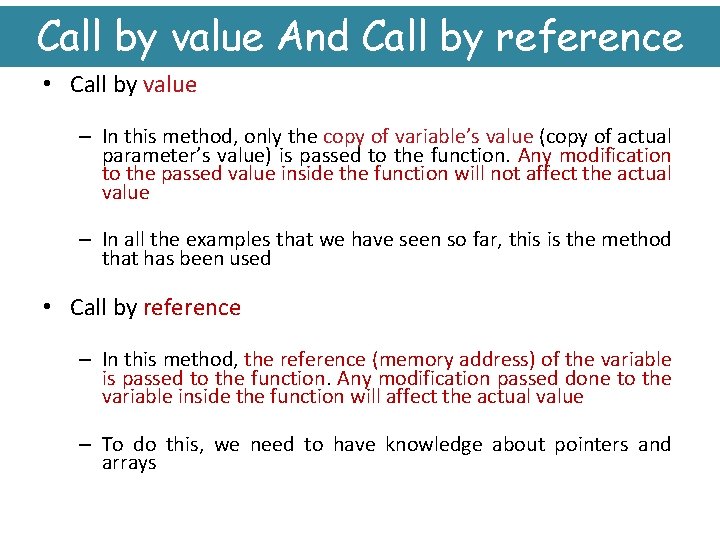
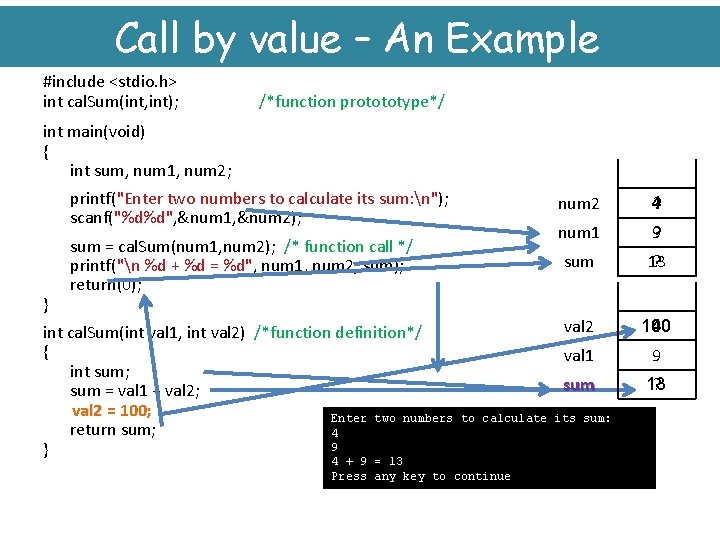
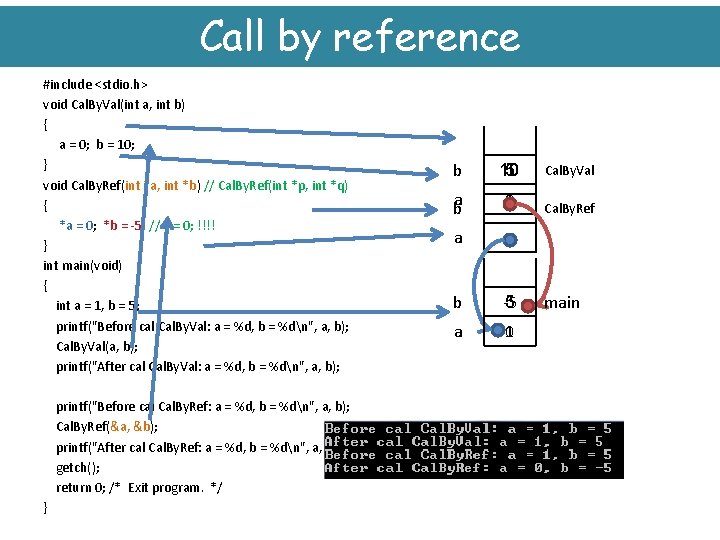
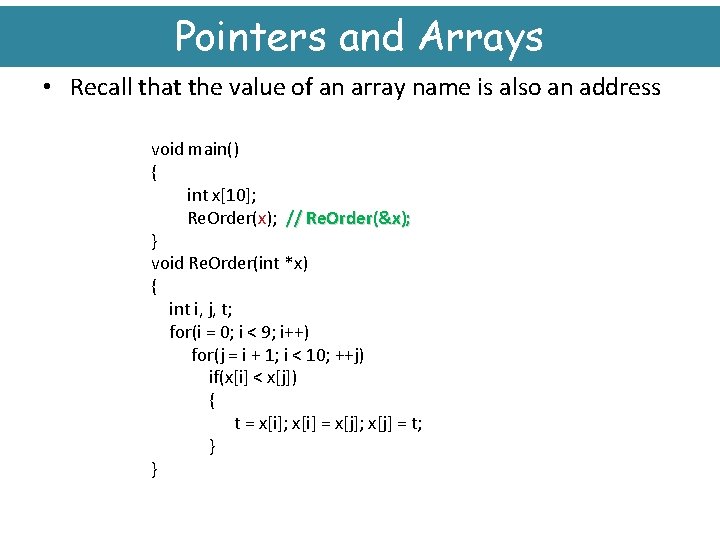
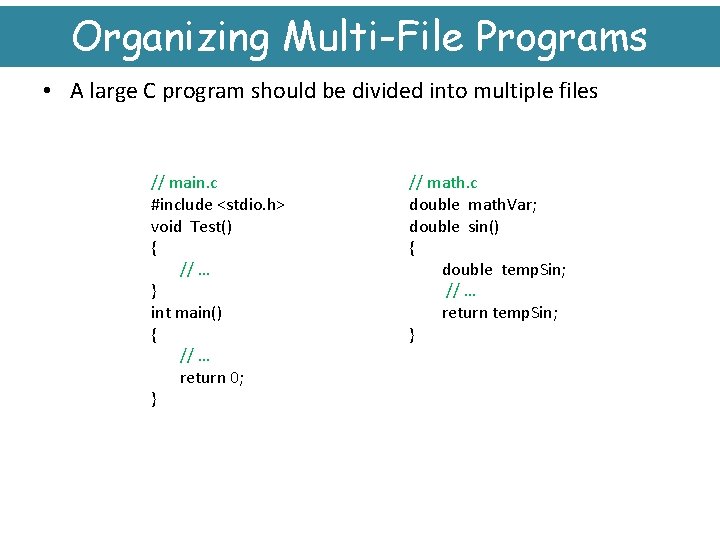
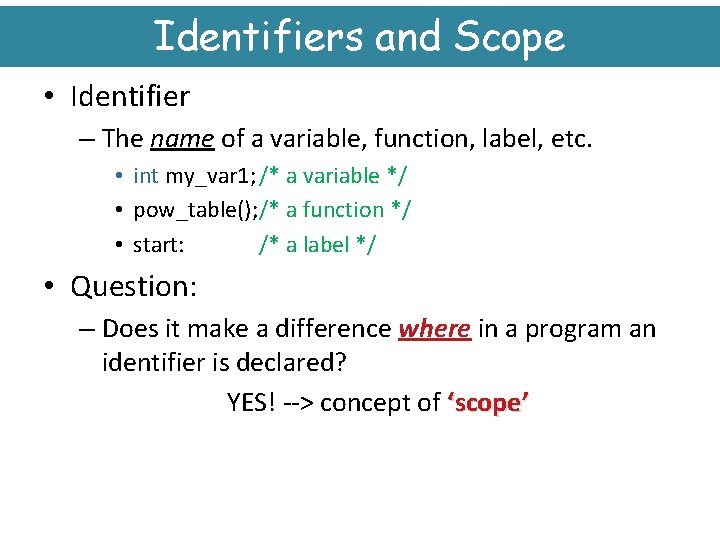
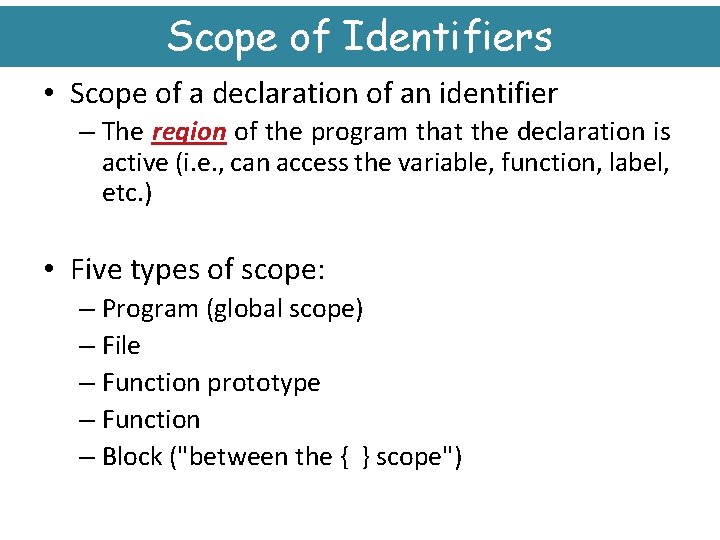
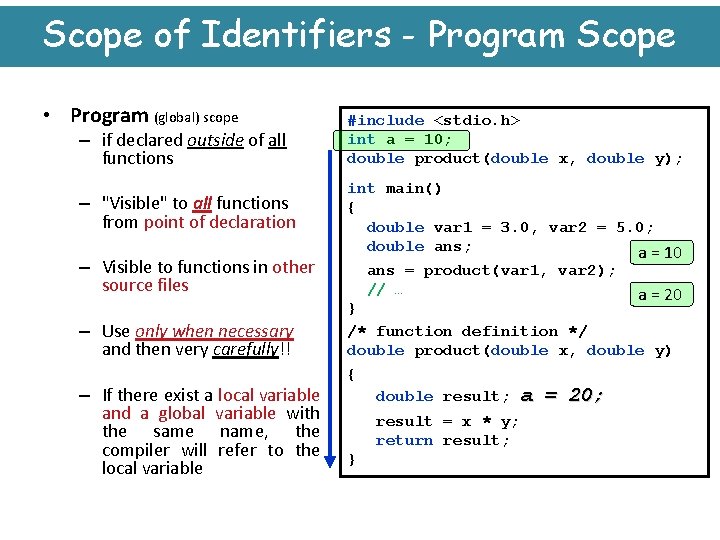
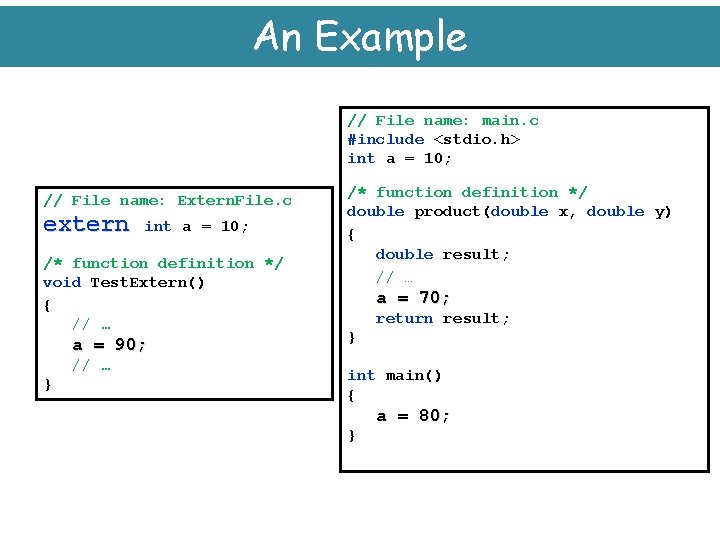
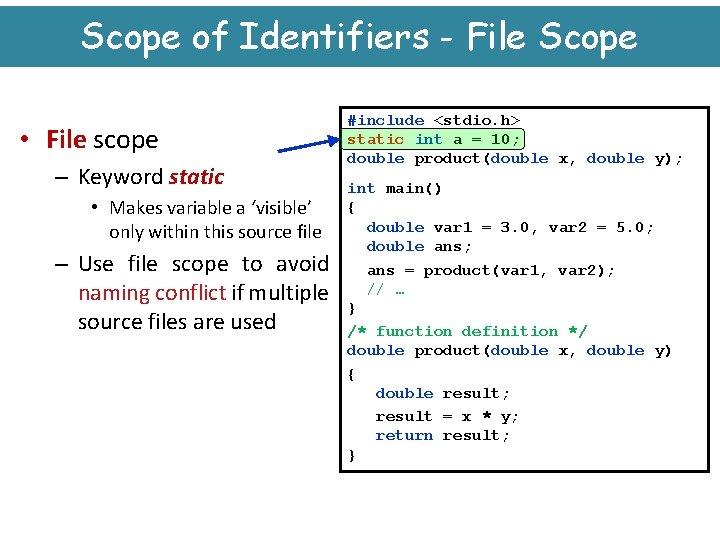
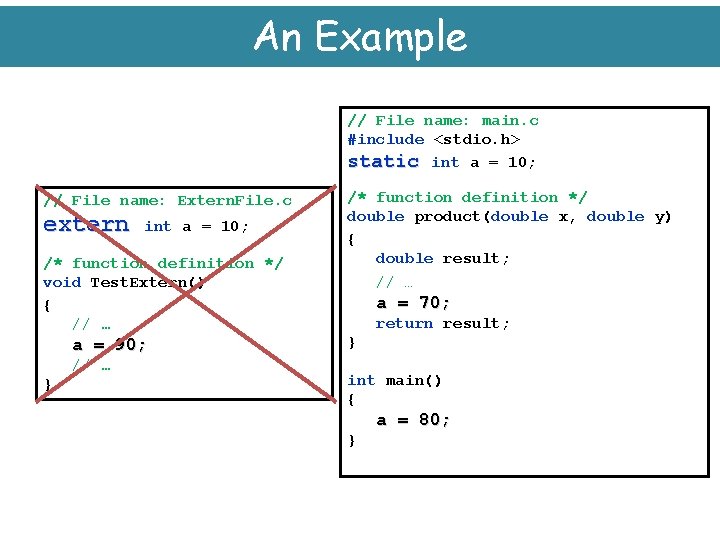
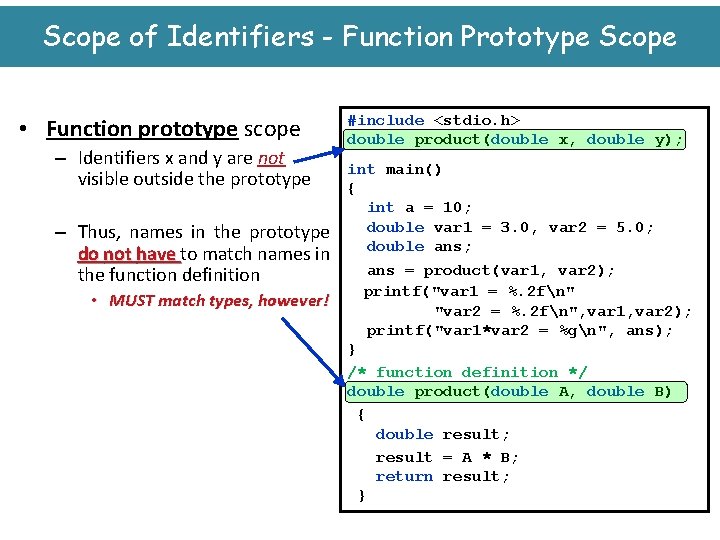
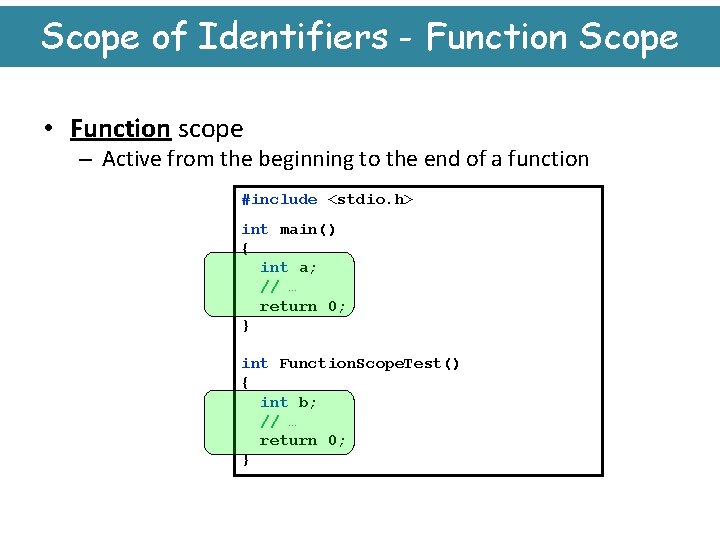
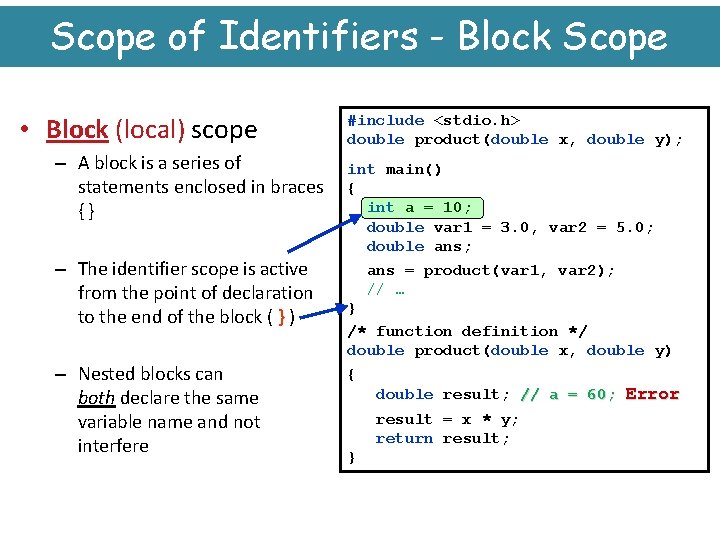
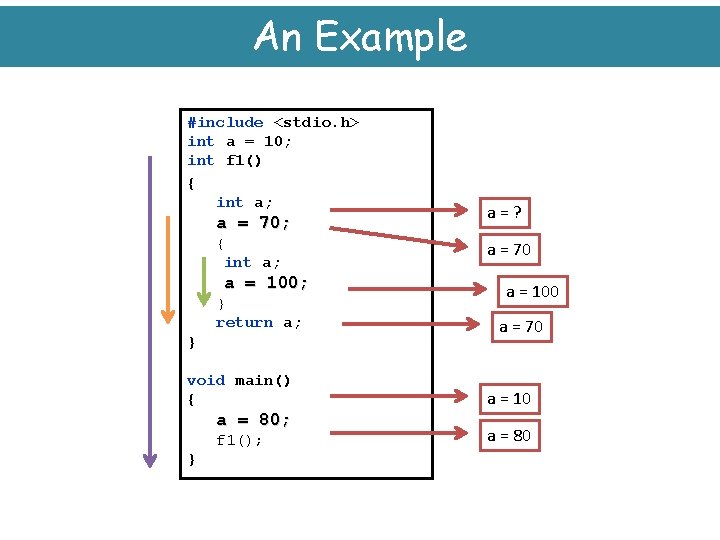
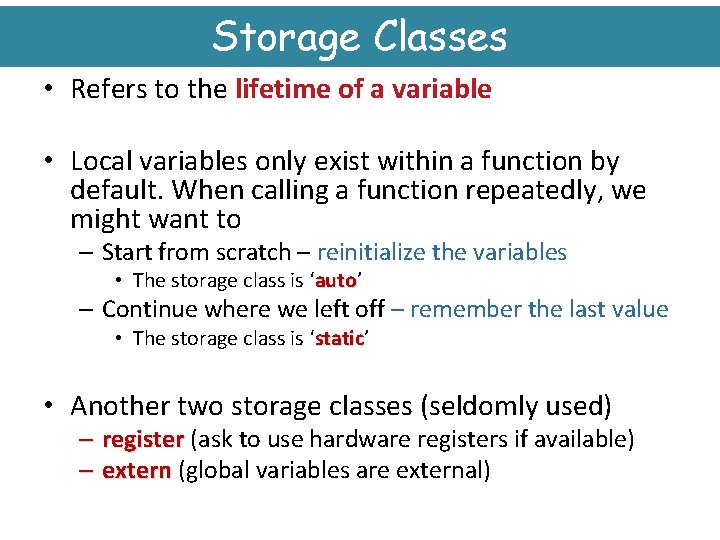
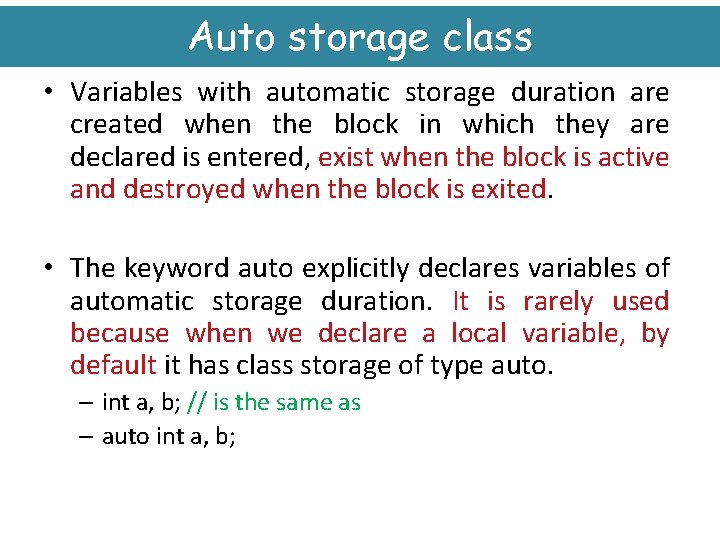
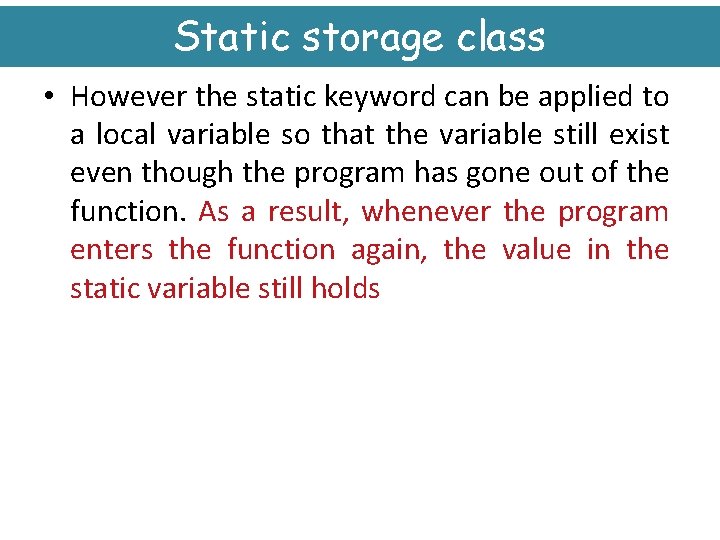
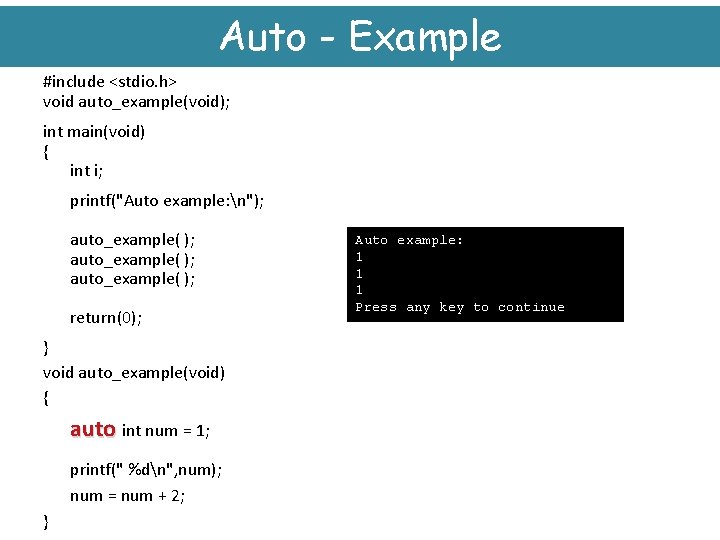
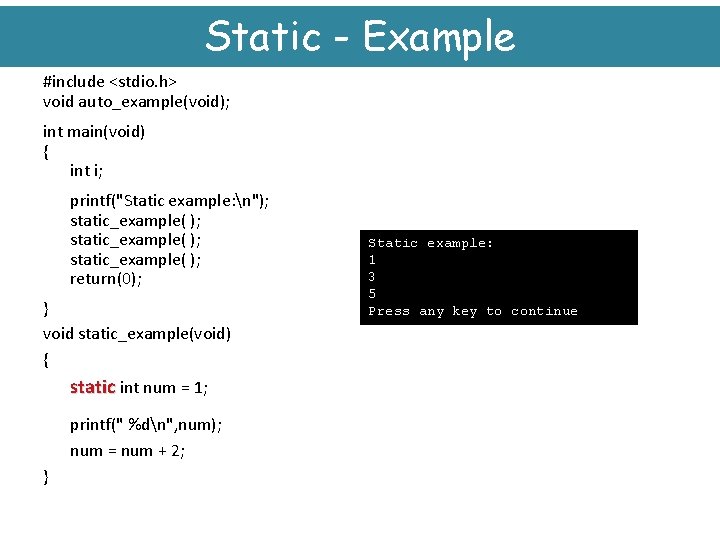
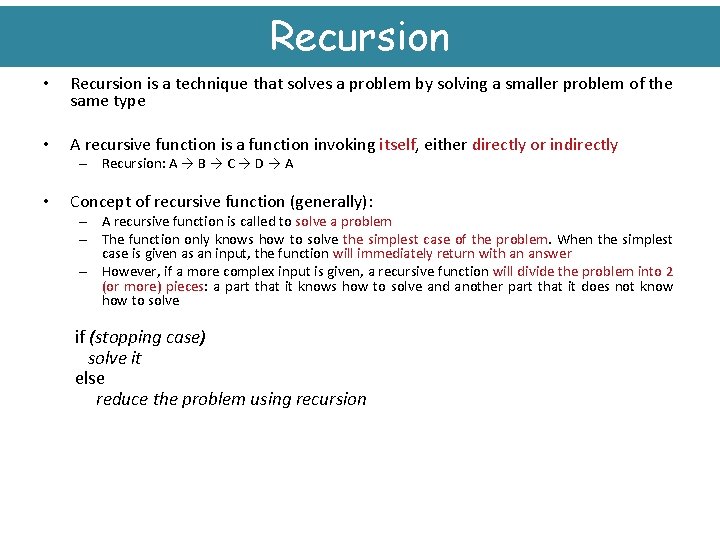
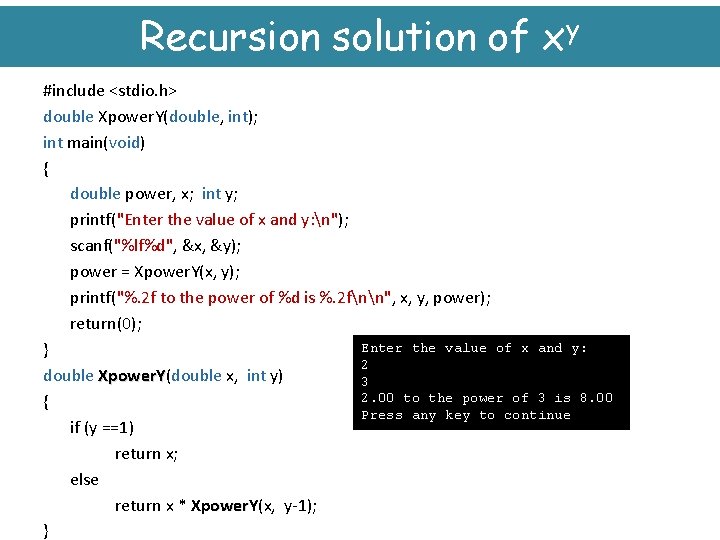
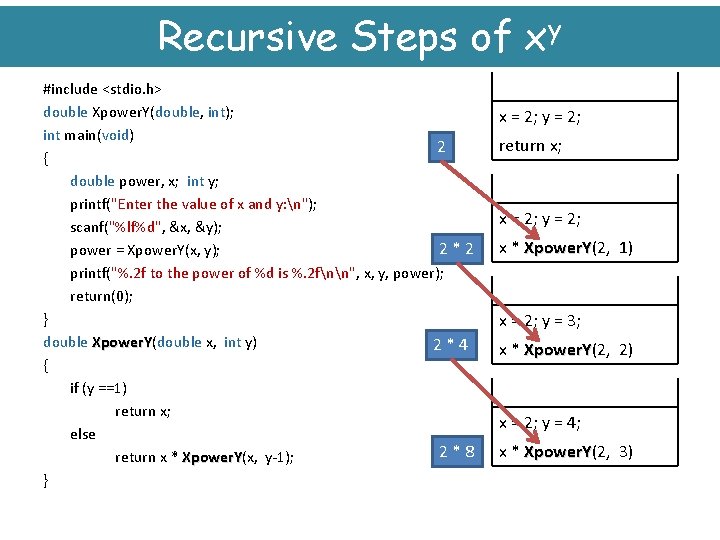
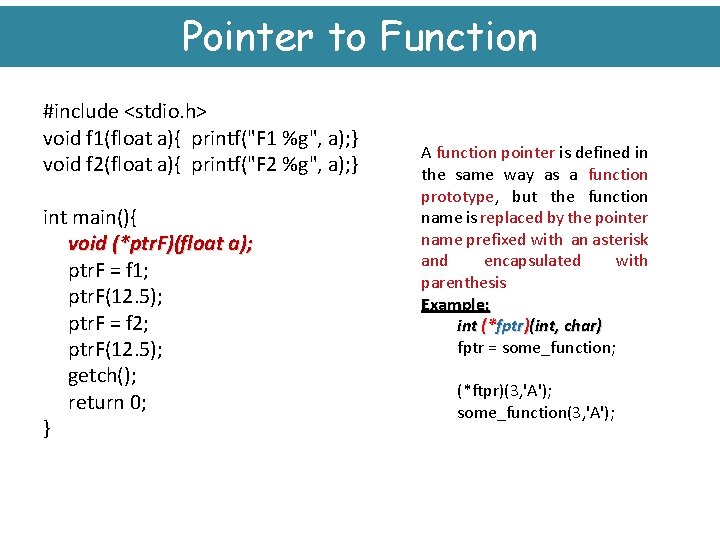
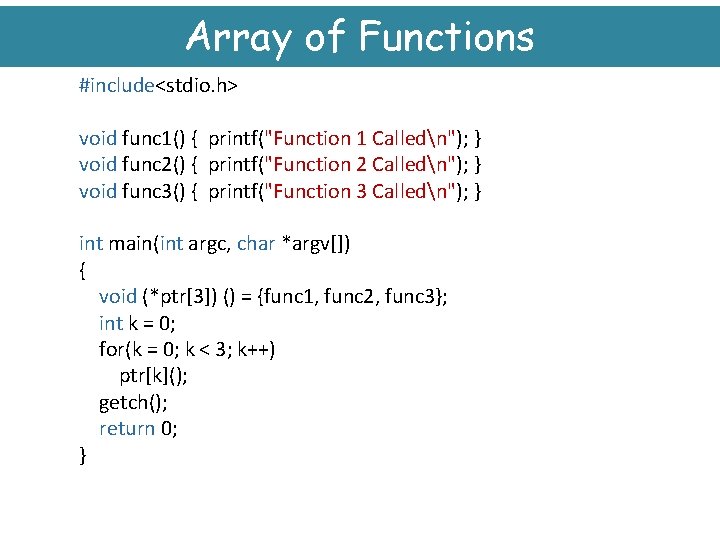
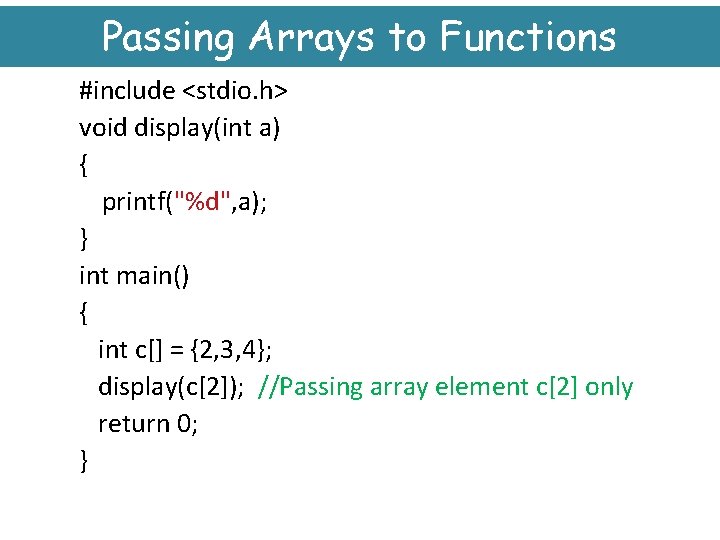
![Passing Arrays to Functions #include <stdio. h> float average(float a[], int count); // float Passing Arrays to Functions #include <stdio. h> float average(float a[], int count); // float](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-133.jpg)
![Passing Arrays to Functions #include <stdio. h> void f 1(float *a) { a[1] = Passing Arrays to Functions #include <stdio. h> void f 1(float *a) { a[1] =](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-134.jpg)
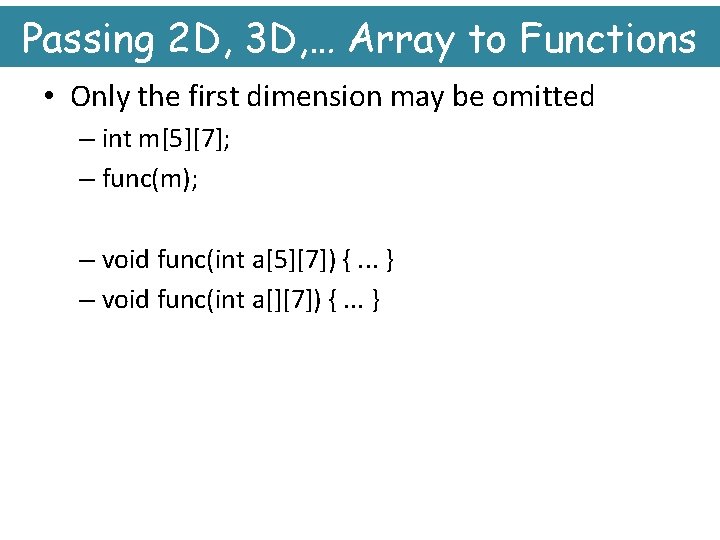
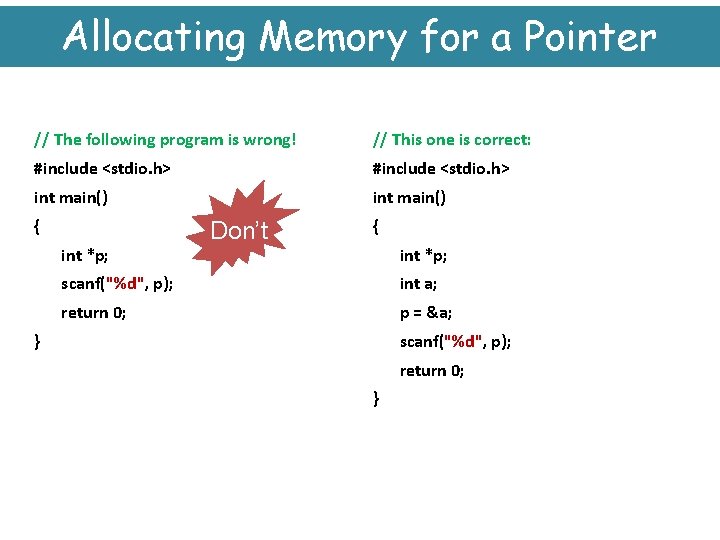
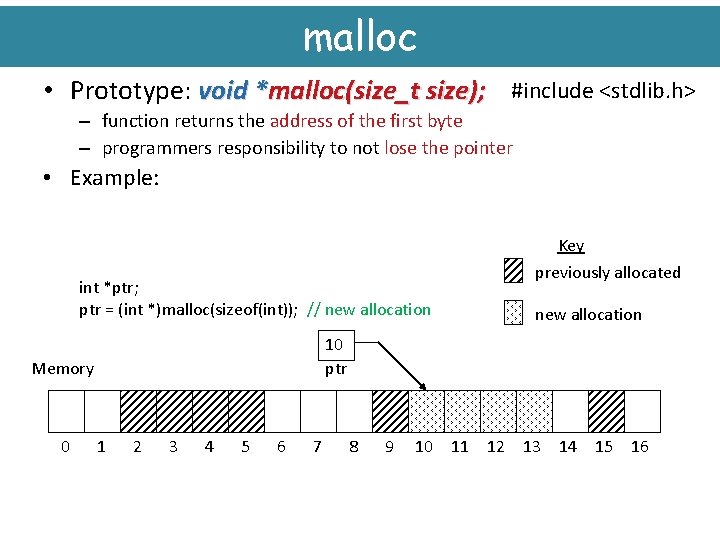
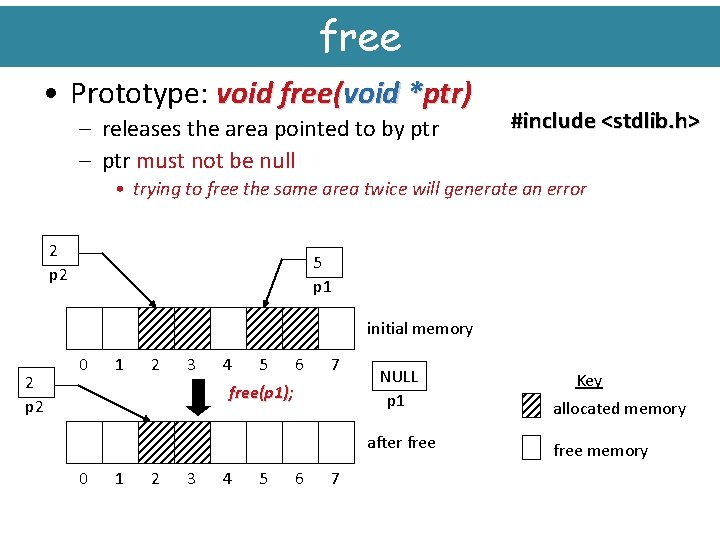
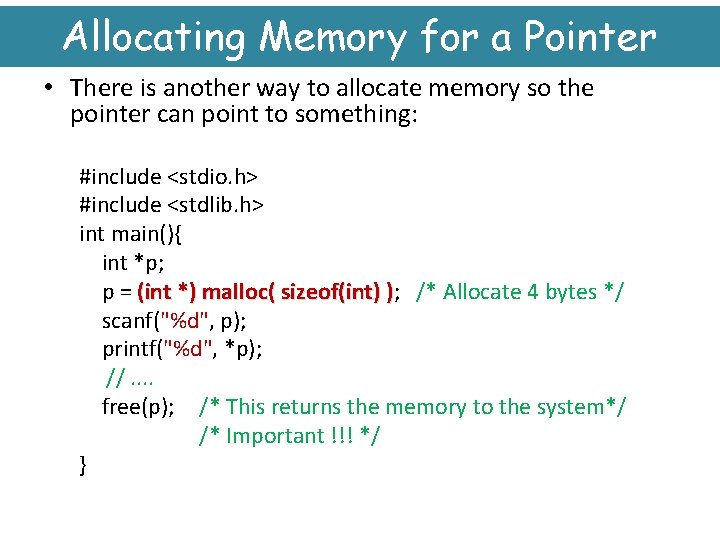
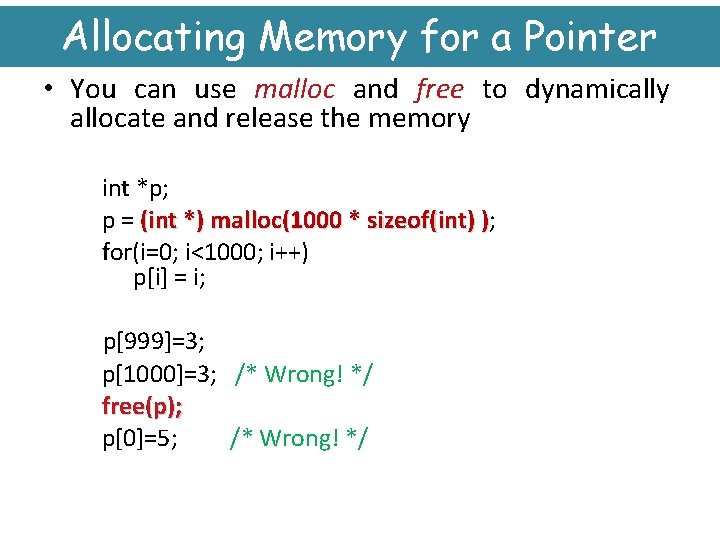
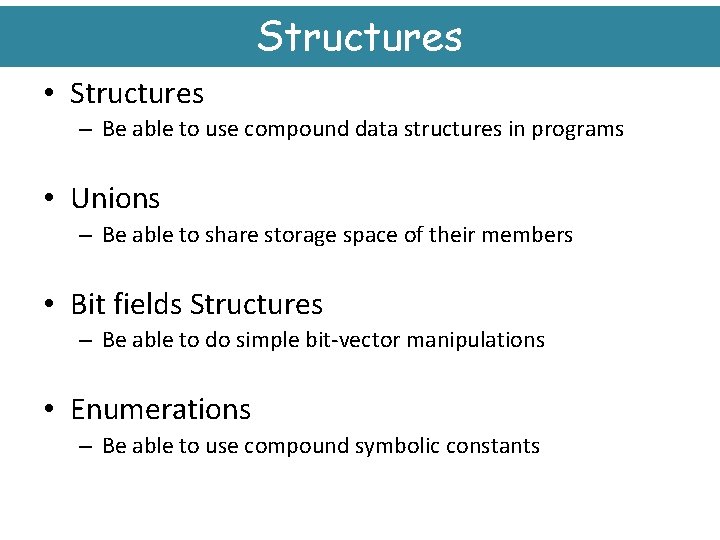
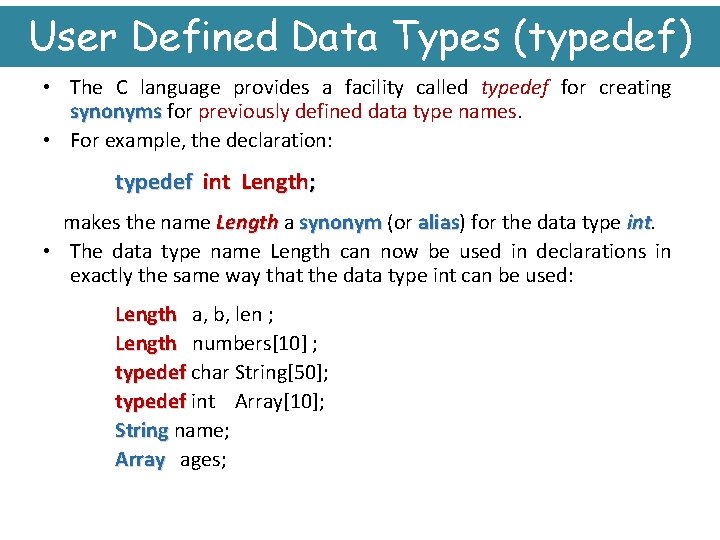
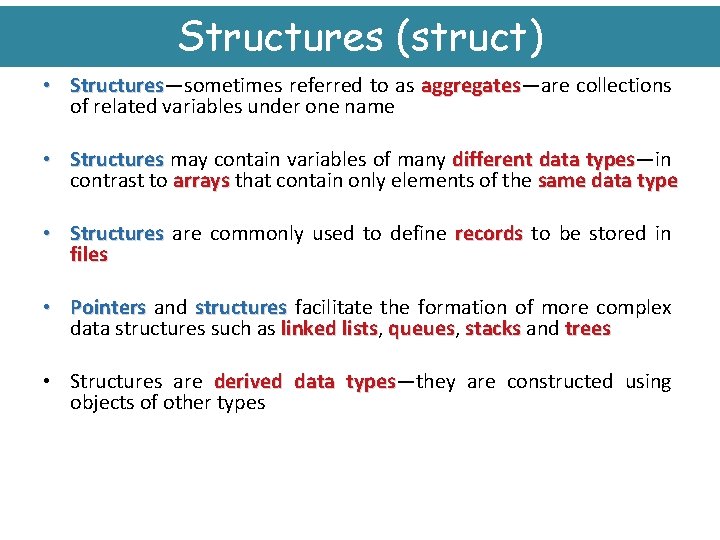
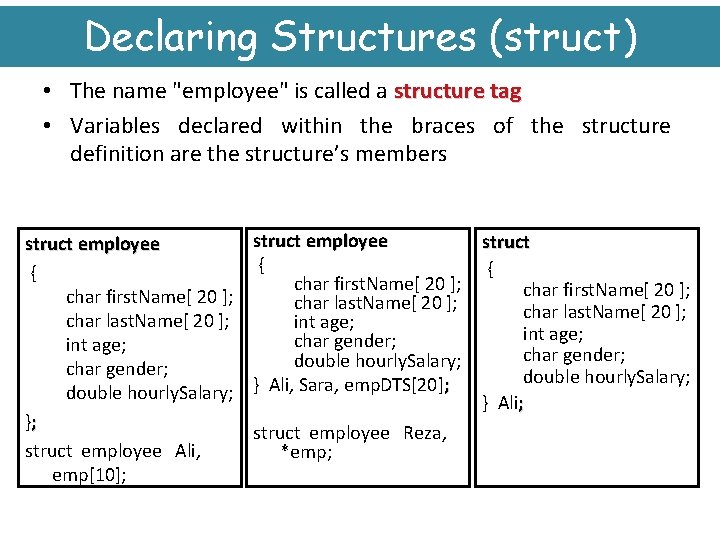
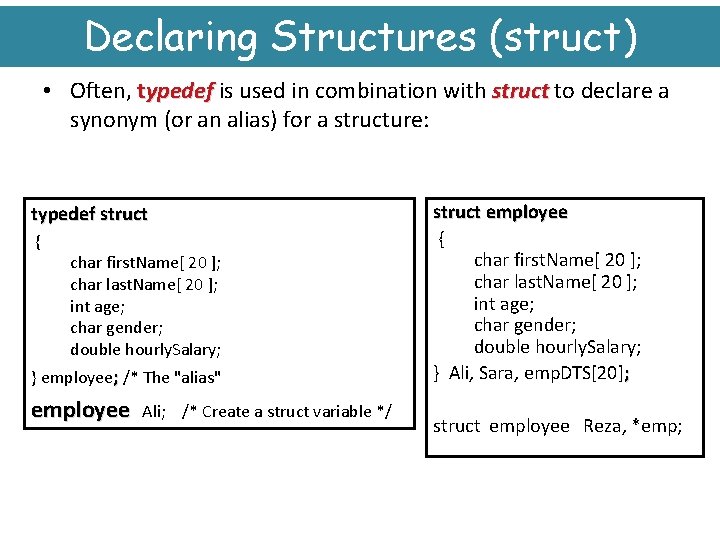
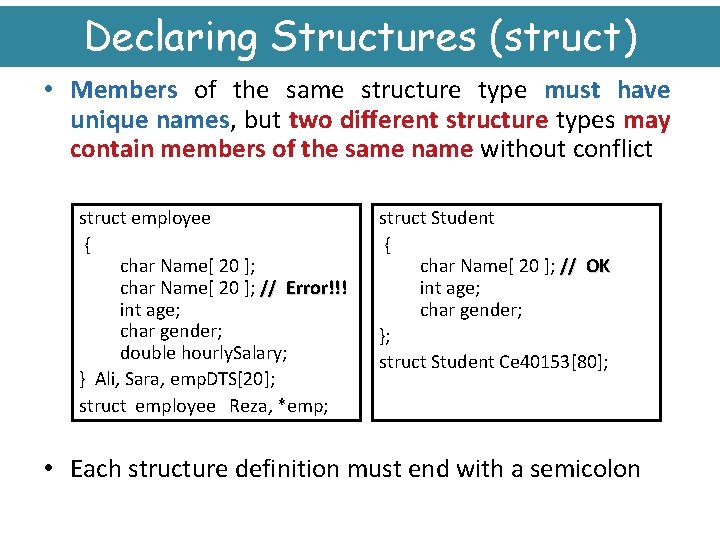
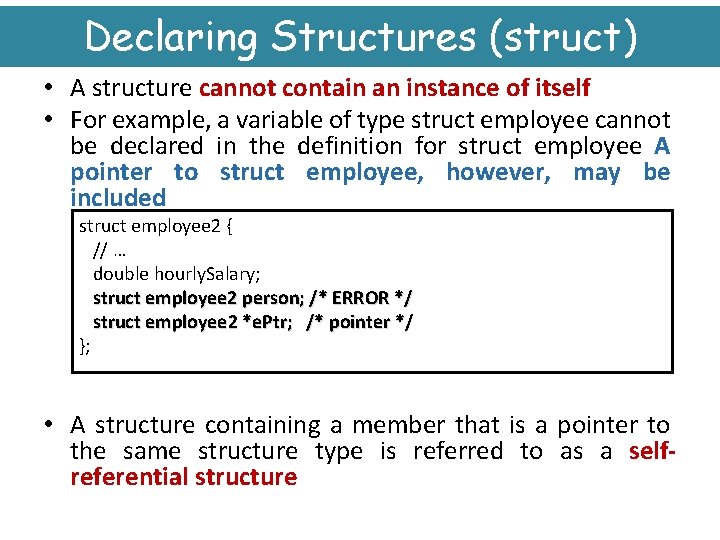
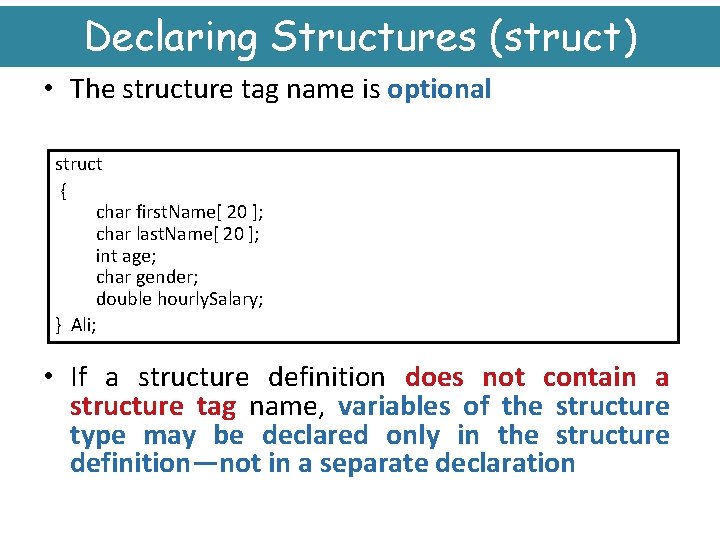
![Memory layout struct COST { int amount; char currency_type[2]; } struct PART { char Memory layout struct COST { int amount; char currency_type[2]; } struct PART { char](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-149.jpg)
![Memory layout struct COST { int amount; char currency_type[2]; } struct PART { } Memory layout struct COST { int amount; char currency_type[2]; } struct PART { }](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-150.jpg)
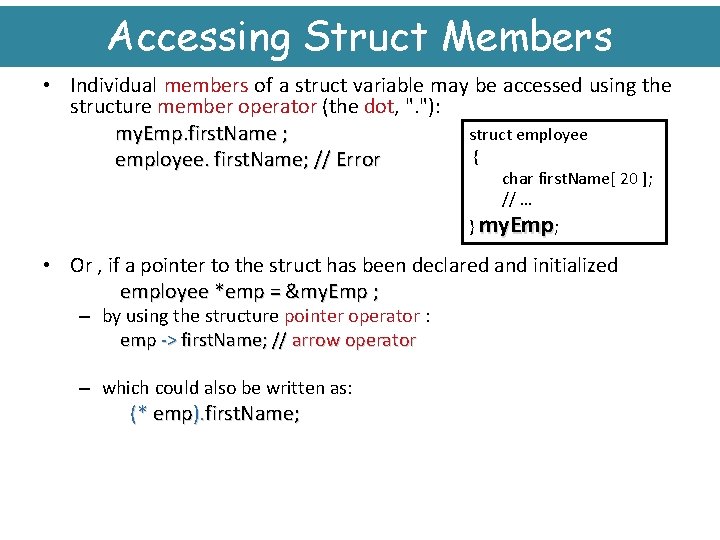
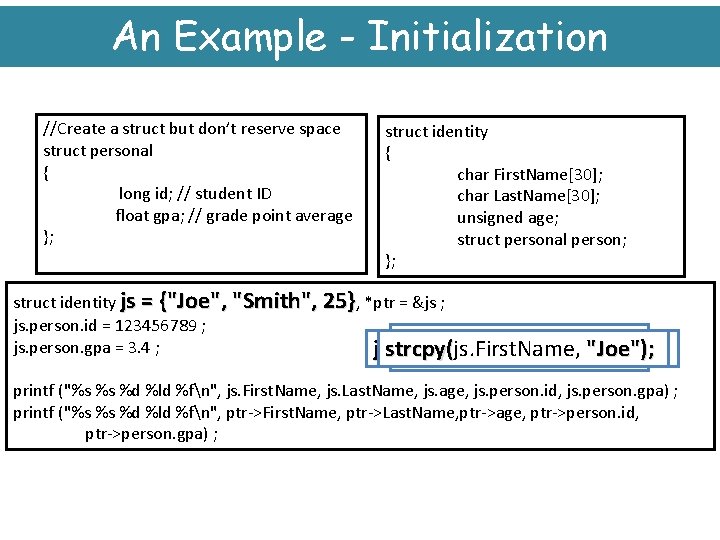
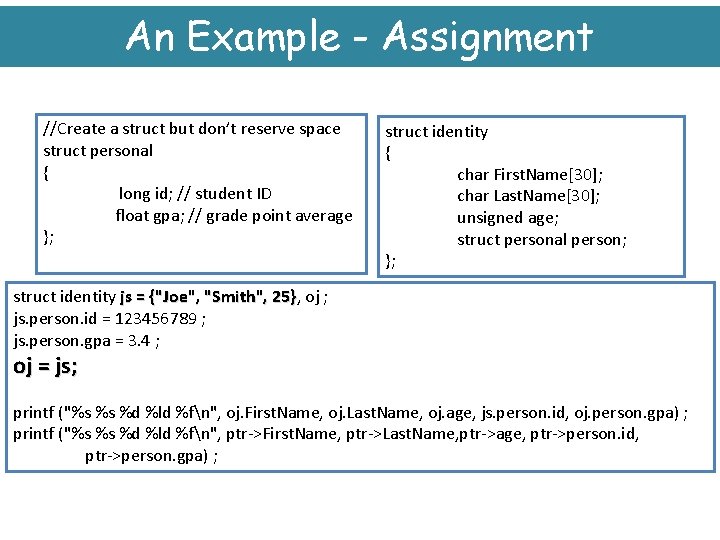
![Arrays of Structures struct identity sharif. C 40153[80] = {"omid", "Jafarinezhad", 14, 9140153, 20, Arrays of Structures struct identity sharif. C 40153[80] = {"omid", "Jafarinezhad", 14, 9140153, 20,](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-154.jpg)
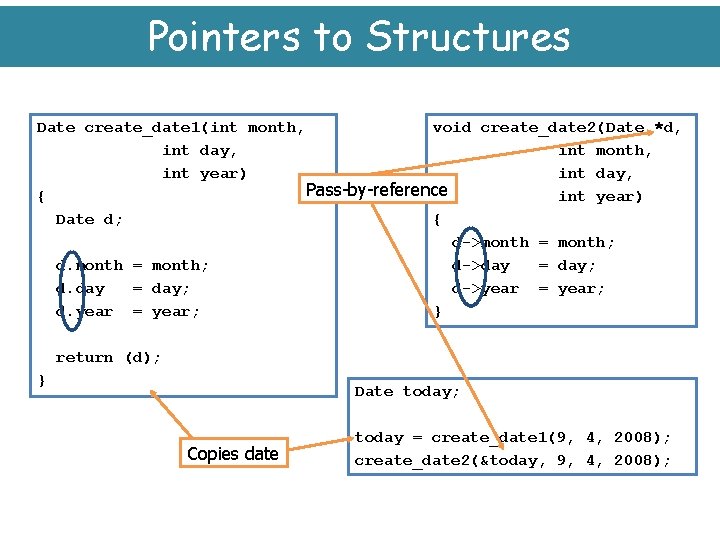
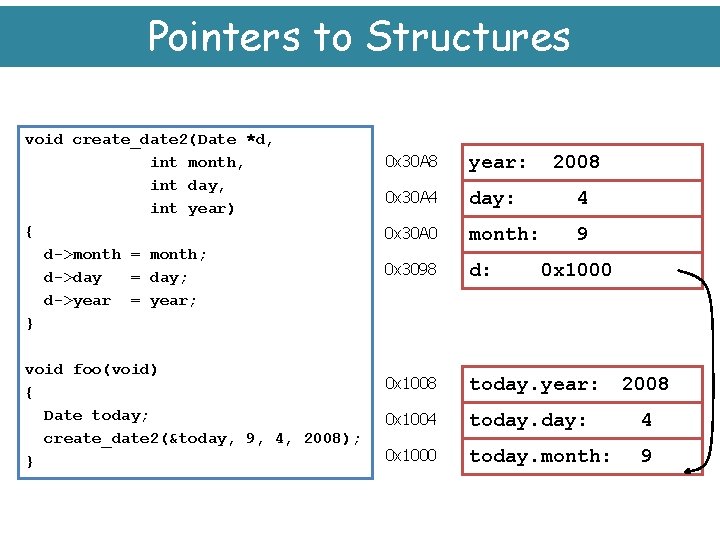
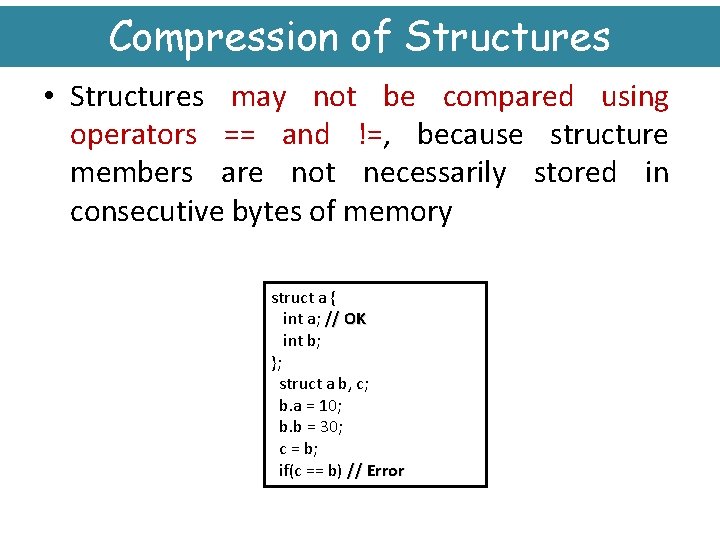
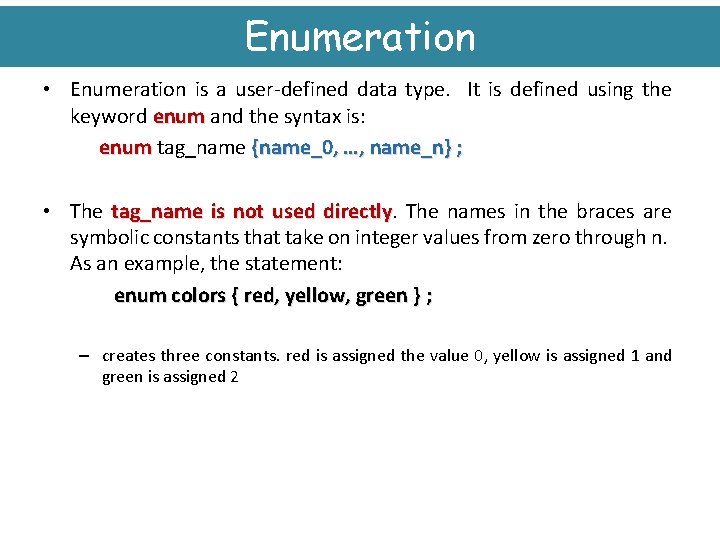
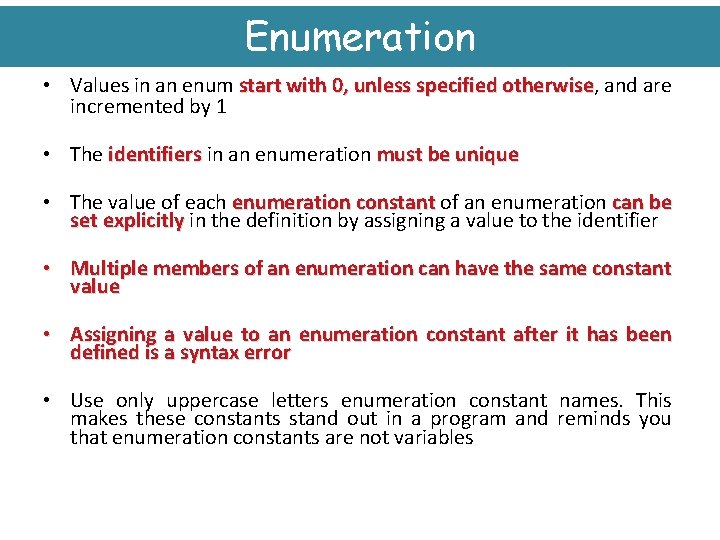
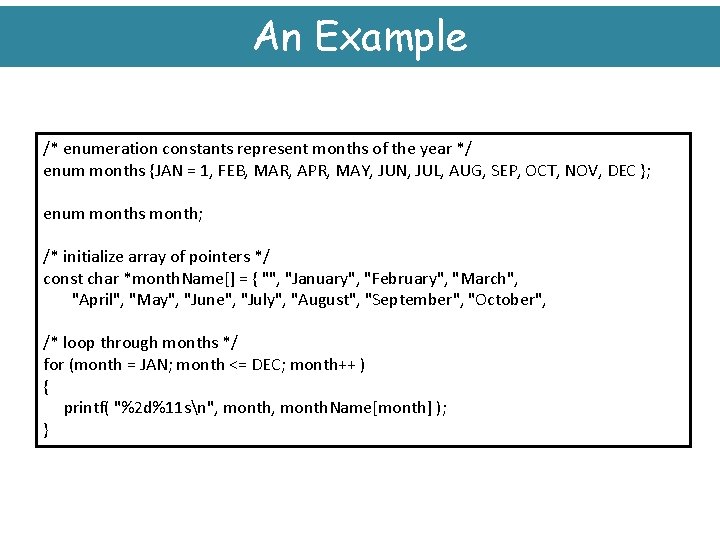
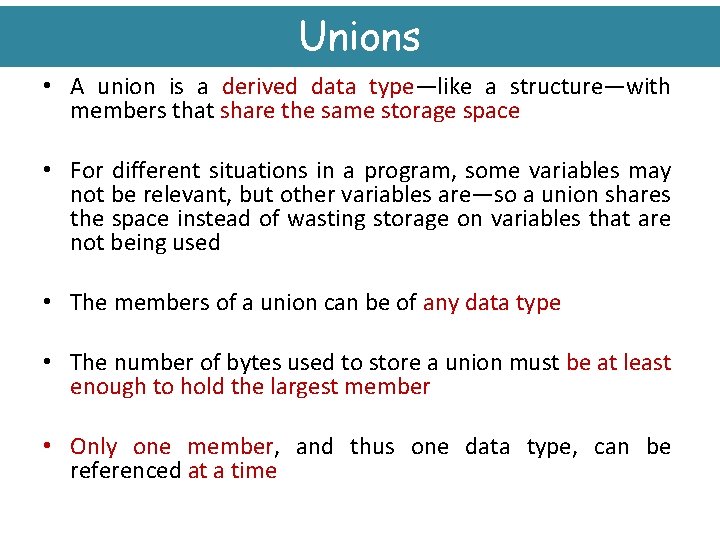
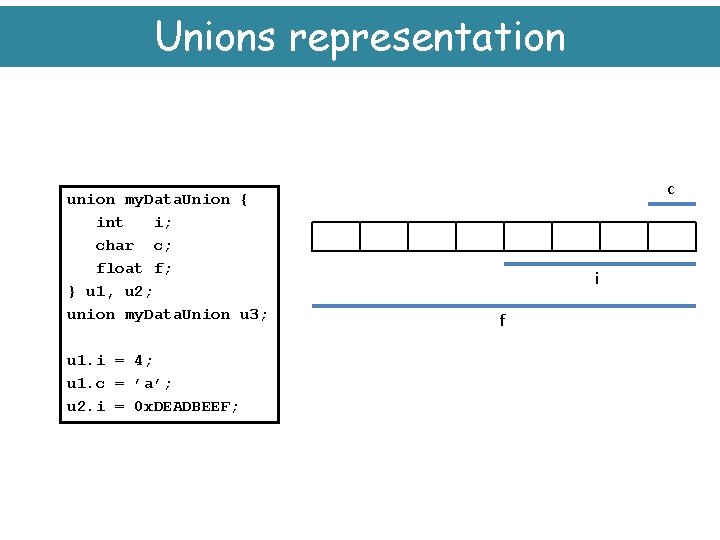
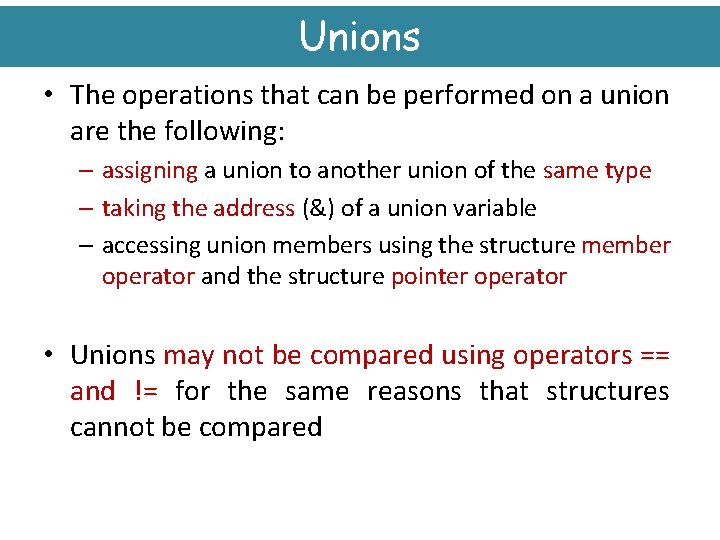
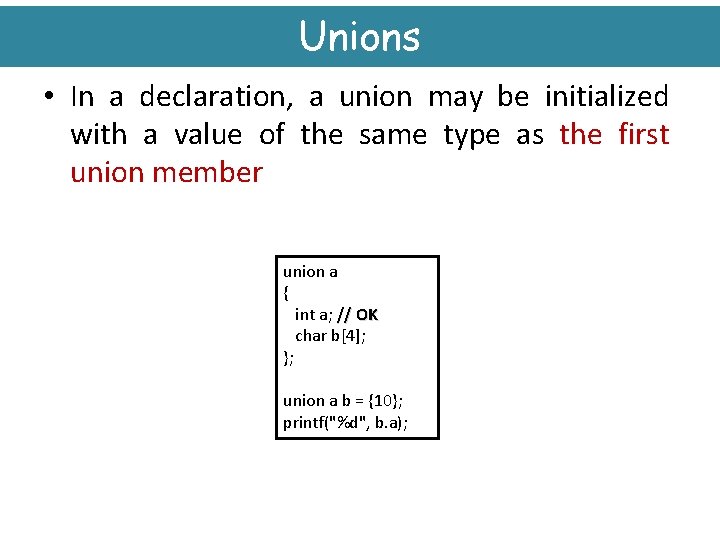
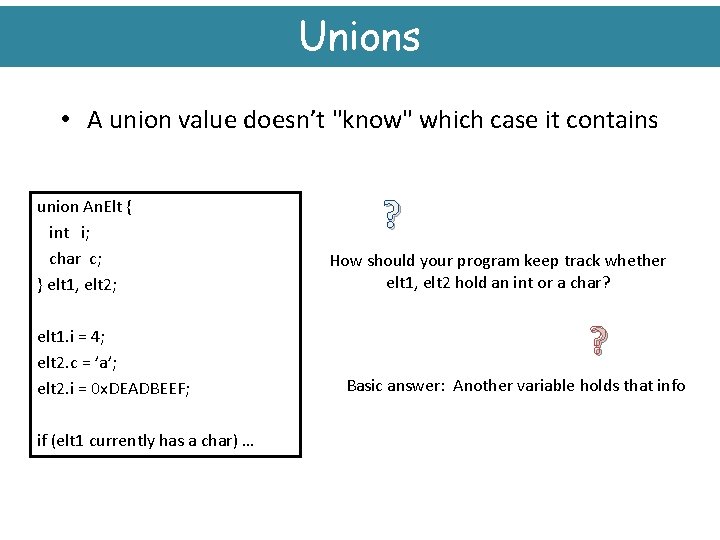
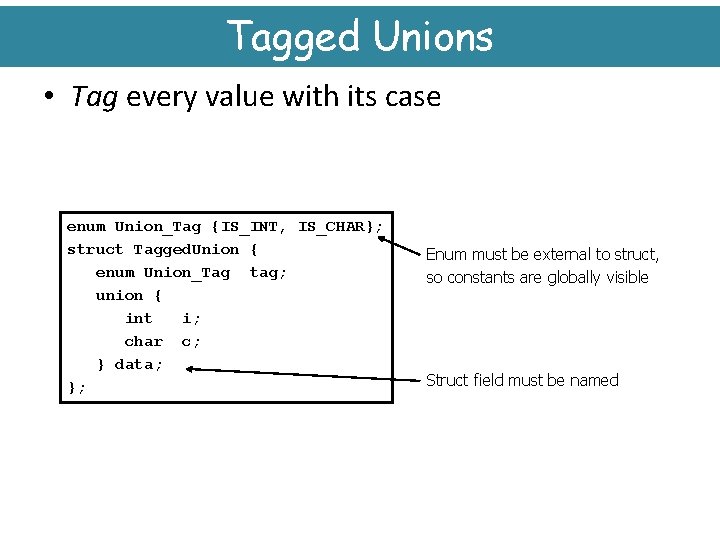
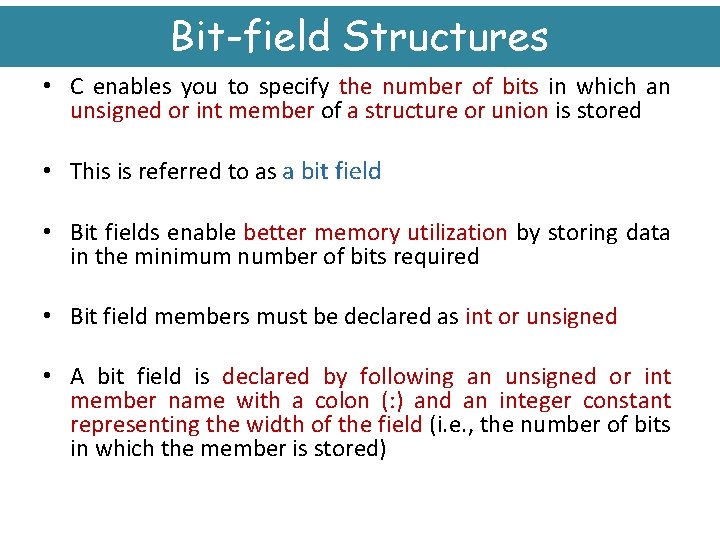
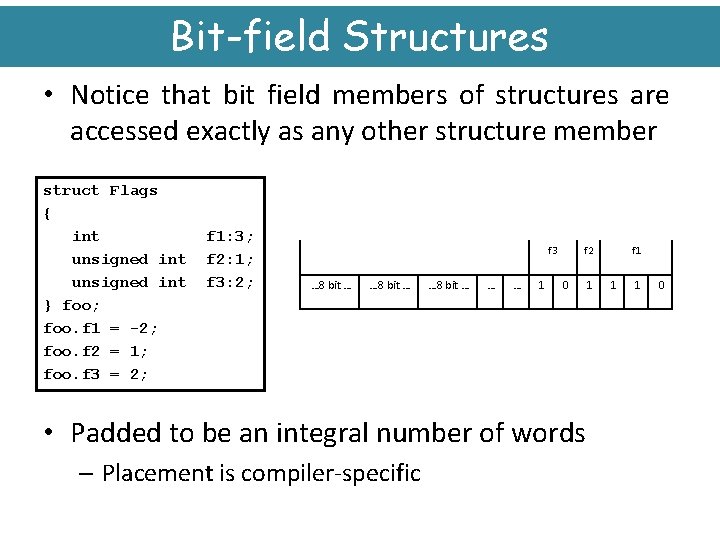
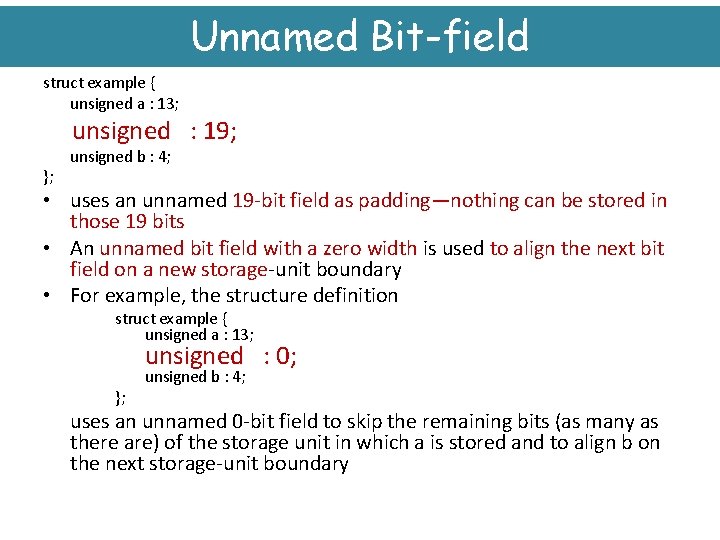
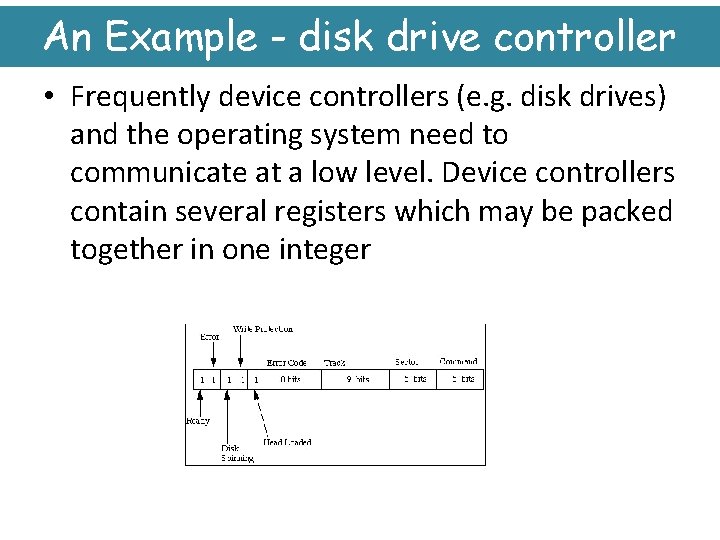
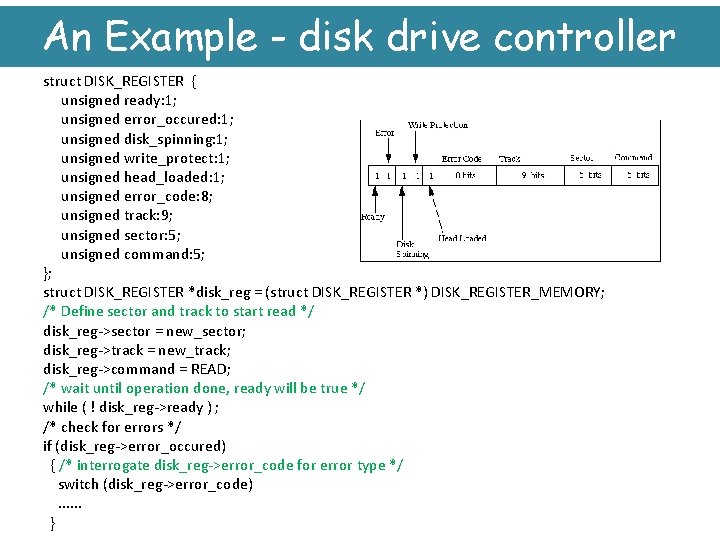
- Slides: 171
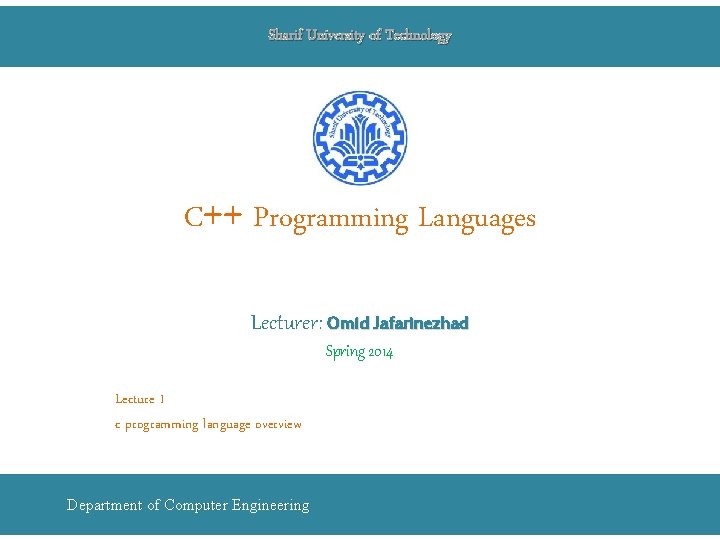
Sharif University of Technology C++ Programming Languages Lecturer: Omid Jafarinezhad Spring 2014 Lecture 1 c programming language overview Department of Computer Engineering
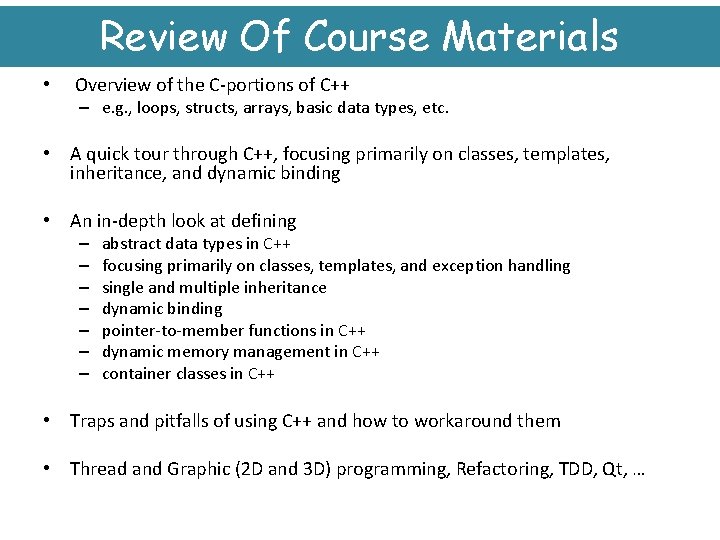
Review Of Course Materials • Overview of the C-portions of C++ – e. g. , loops, structs, arrays, basic data types, etc. • A quick tour through C++, focusing primarily on classes, templates, inheritance, and dynamic binding • An in-depth look at defining – – – – abstract data types in C++ focusing primarily on classes, templates, and exception handling single and multiple inheritance dynamic binding pointer-to-member functions in C++ dynamic memory management in C++ container classes in C++ • Traps and pitfalls of using C++ and how to workaround them • Thread and Graphic (2 D and 3 D) programming, Refactoring, TDD, Qt, …
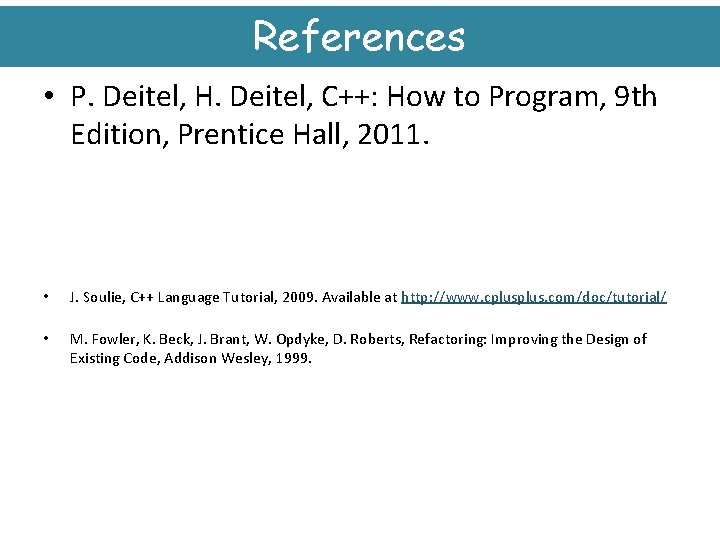
References • P. Deitel, H. Deitel, C++: How to Program, 9 th Edition, Prentice Hall, 2011. • J. Soulie, C++ Language Tutorial, 2009. Available at http: //www. cplus. com/doc/tutorial/ • M. Fowler, K. Beck, J. Brant, W. Opdyke, D. Roberts, Refactoring: Improving the Design of Existing Code, Addison Wesley, 1999.
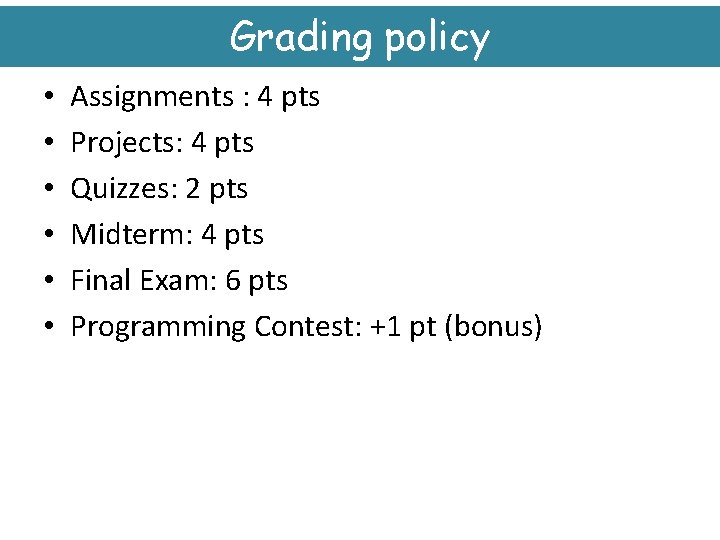
Grading policy • • • Assignments : 4 pts Projects: 4 pts Quizzes: 2 pts Midterm: 4 pts Final Exam: 6 pts Programming Contest: +1 pt (bonus)
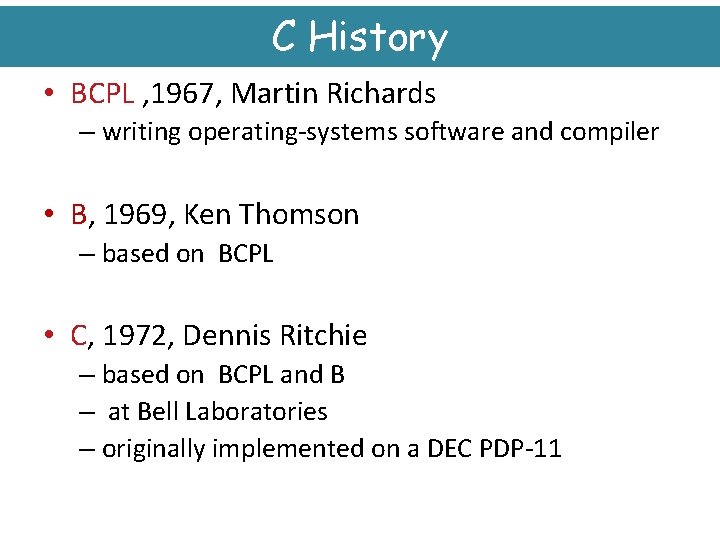
C History • BCPL , 1967, Martin Richards – writing operating-systems software and compiler • B, 1969, Ken Thomson – based on BCPL • C, 1972, Dennis Ritchie – based on BCPL and B – at Bell Laboratories – originally implemented on a DEC PDP-11
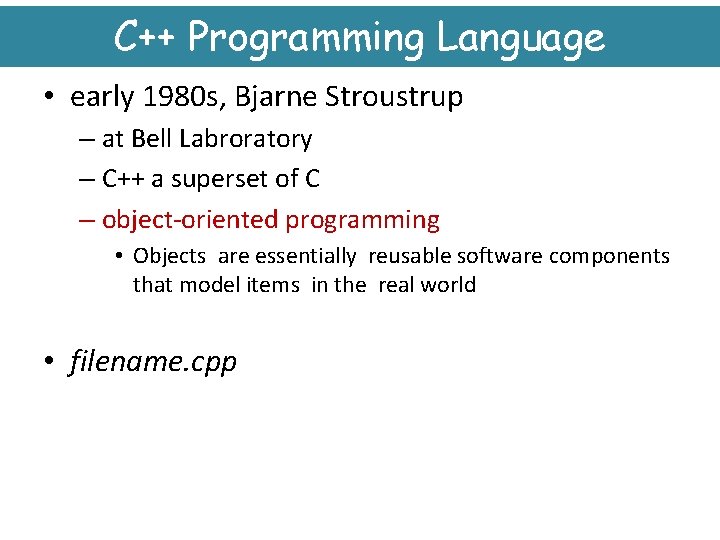
C++ Programming Language • early 1980 s, Bjarne Stroustrup – at Bell Labroratory – C++ a superset of C – object-oriented programming • Objects are essentially reusable software components that model items in the real world • filename. cpp
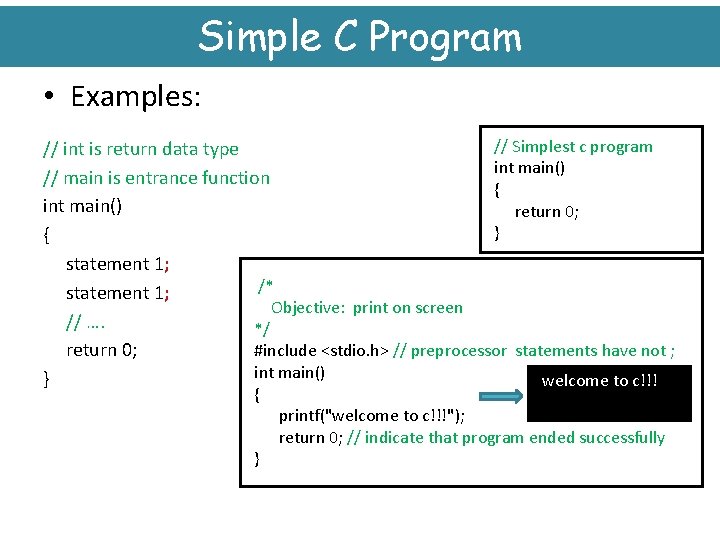
Simple C Program • Examples: // Simplest c program // int is return data type int main() // main is entrance function { int main() return 0; } { statement 1; /* statement 1; Objective: print on screen // …. */ return 0; #include <stdio. h> // preprocessor statements have not ; int main() } welcome to c!!! { } printf("welcome to c!!!"); return 0; // indicate that program ended successfully
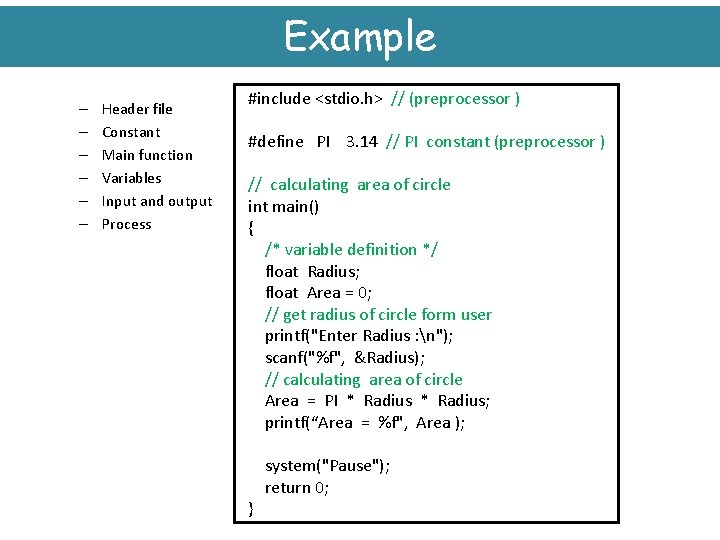
Example – – – Header file Constant Main function Variables Input and output Process #include <stdio. h> // (preprocessor ) #define PI 3. 14 // PI constant (preprocessor ) // calculating area of circle int main() { /* variable definition */ float Radius; float Area = 0; // get radius of circle form user printf("Enter Radius : n"); scanf("%f", &Radius); // calculating area of circle Area = PI * Radius; printf(“Area = %f", Area ); } system("Pause"); return 0;
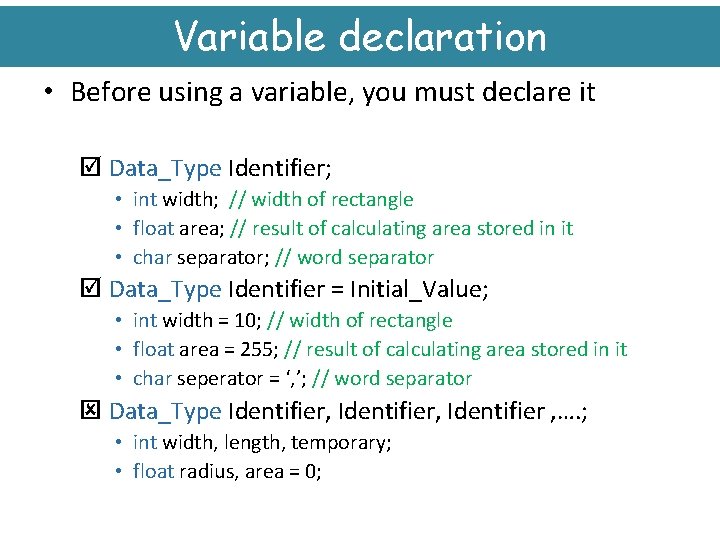
Variable declaration • Before using a variable, you must declare it þ Data_Type Identifier; • int width; // width of rectangle • float area; // result of calculating area stored in it • char separator; // word separator þ Data_Type Identifier = Initial_Value; • int width = 10; // width of rectangle • float area = 255; // result of calculating area stored in it • char seperator = ‘, ’; // word separator Data_Type Identifier, Identifier , …. ; • int width, length, temporary; • float radius, area = 0;
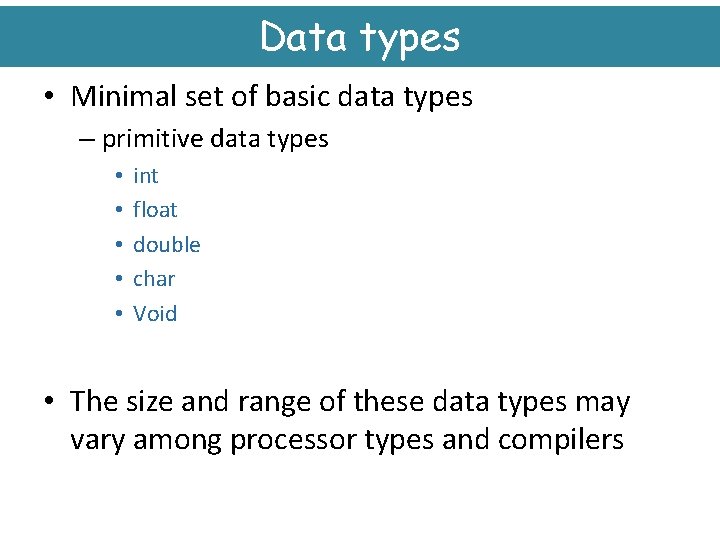
Data types • Minimal set of basic data types – primitive data types • • • int float double char Void • The size and range of these data types may vary among processor types and compilers
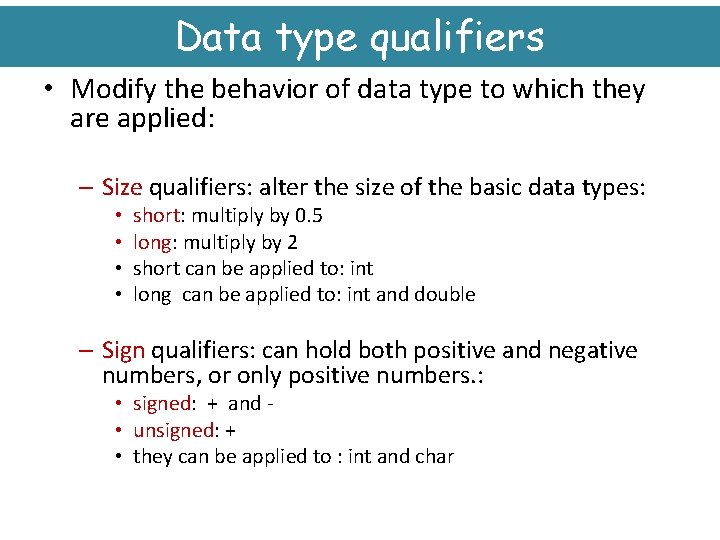
Data type qualifiers • Modify the behavior of data type to which they are applied: – Size qualifiers: alter the size of the basic data types: • • short: multiply by 0. 5 long: multiply by 2 short can be applied to: int long can be applied to: int and double – Sign qualifiers: can hold both positive and negative numbers, or only positive numbers. : • signed: + and • unsigned: + • they can be applied to : int and char
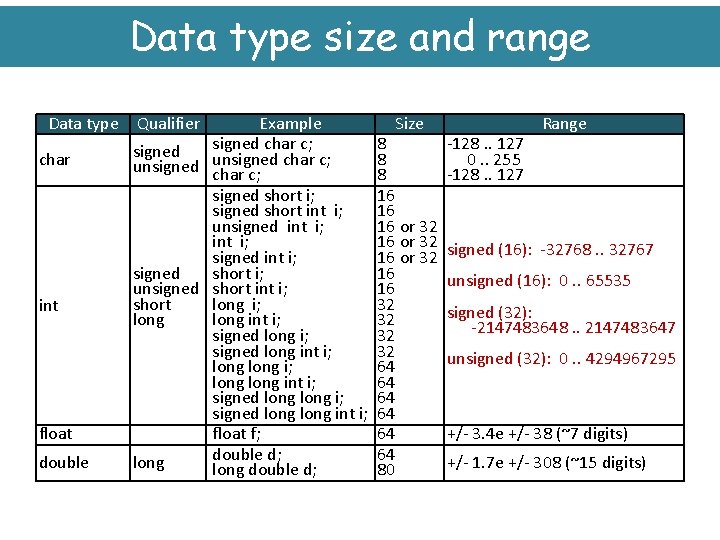
Data type size and range Data type Qualifier char int float double Example signed char c; unsigned char c; signed short int i; unsigned int i; short i; signed unsigned short int i; long i; short long int i; long signed long i; signed long int i; long int i; signed long int i; float f; double d; long double d; Size 8 8 8 16 16 16 or 32 16 16 32 32 64 64 64 80 -128. . 127 0. . 255 -128. . 127 Range signed (16): -32768. . 32767 unsigned (16): 0. . 65535 signed (32): -2147483648. . 2147483647 unsigned (32): 0. . 4294967295 +/- 3. 4 e +/- 38 (~7 digits) +/- 1. 7 e +/- 308 (~15 digits)
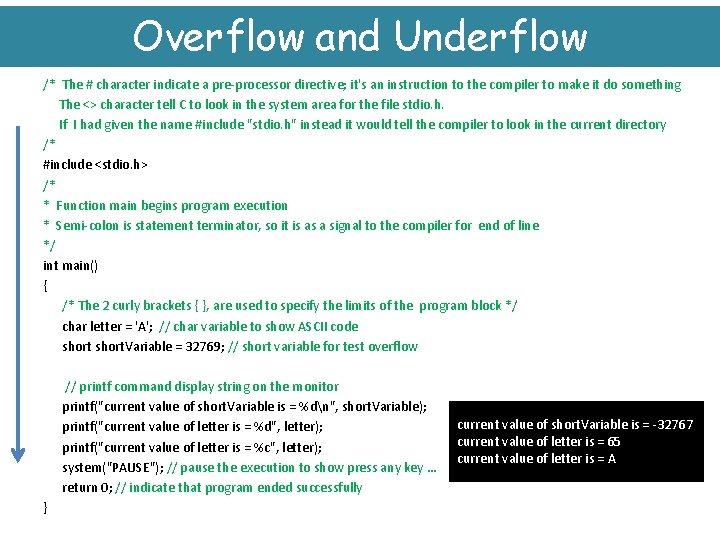
Overflow and Underflow /* The # character indicate a pre-processor directive; it's an instruction to the compiler to make it do something The <> character tell C to look in the system area for the file stdio. h. If I had given the name #include "stdio. h" instead it would tell the compiler to look in the current directory /* #include <stdio. h> /* * Function main begins program execution * Semi-colon is statement terminator, so it is as a signal to the compiler for end of line */ int main() { /* The 2 curly brackets { }, are used to specify the limits of the program block */ char letter = 'A'; // char variable to show ASCII code short. Variable = 32769; // short variable for test overflow // printf command display string on the monitor printf("current value of short. Variable is = %dn", short. Variable); printf("current value of letter is = %d", letter); printf("current value of letter is = %c", letter); system("PAUSE"); // pause the execution to show press any key … return 0; // indicate that program ended successfully } current value of short. Variable is = -32767 current value of letter is = 65 current value of letter is = A
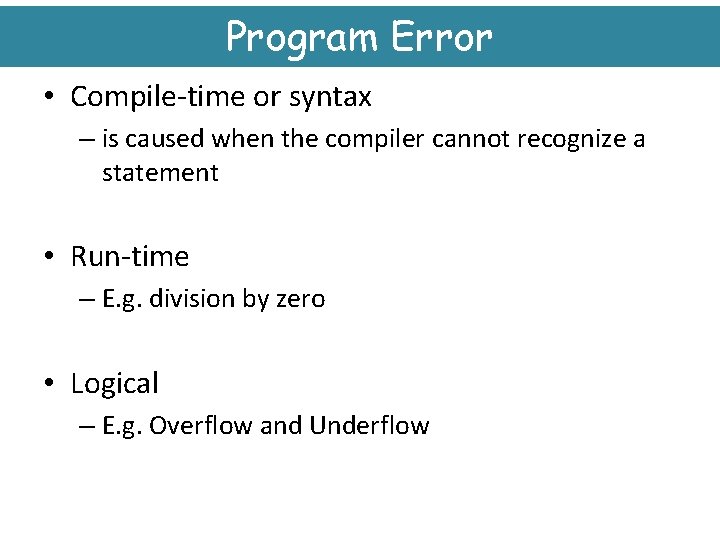
Program Error • Compile-time or syntax – is caused when the compiler cannot recognize a statement • Run-time – E. g. division by zero • Logical – E. g. Overflow and Underflow
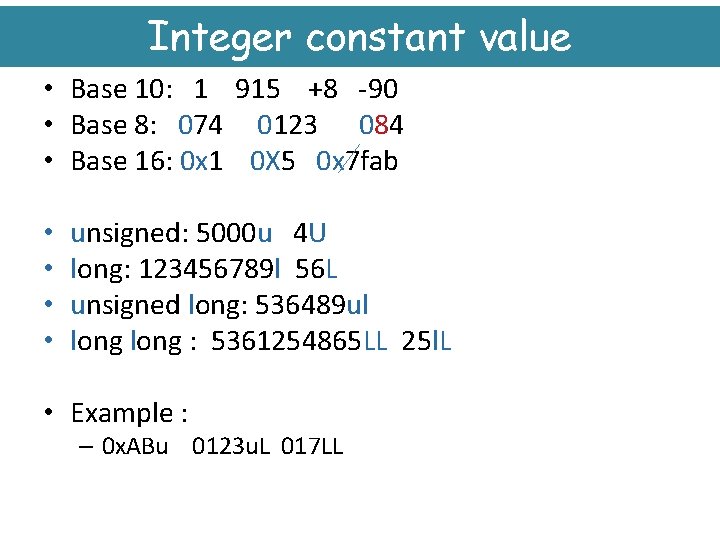
Integer constant value • Base 10: 1 915 +8 -90 • Base 8: 074 0123 084 • Base 16: 0 x 1 0 X 5 0 x 7 fab • • unsigned: 5000 u 4 U long: 123456789 l 56 L unsigned long: 536489 ul long : 5361254865 LL 25 l. L • Example : – 0 x. ABu 0123 u. L 017 LL
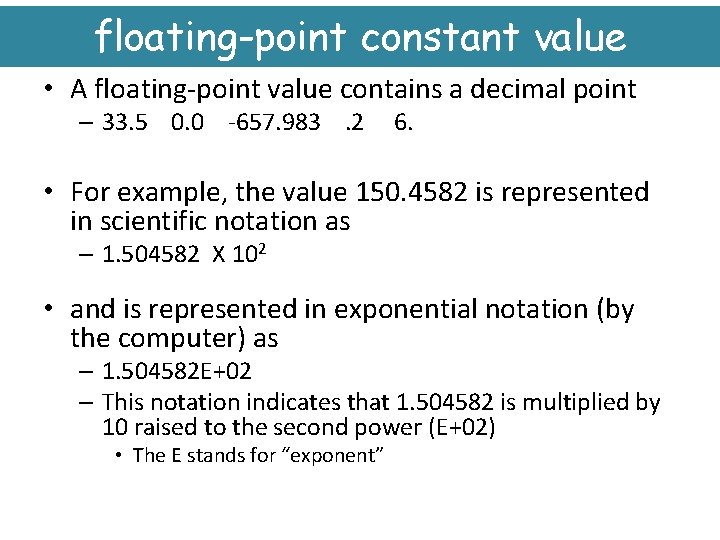
floating-point constant value • A floating-point value contains a decimal point – 33. 5 0. 0 -657. 983. 2 6. • For example, the value 150. 4582 is represented in scientific notation as – 1. 504582 X 102 • and is represented in exponential notation (by the computer) as – 1. 504582 E+02 – This notation indicates that 1. 504582 is multiplied by 10 raised to the second power (E+02) • The E stands for “exponent”
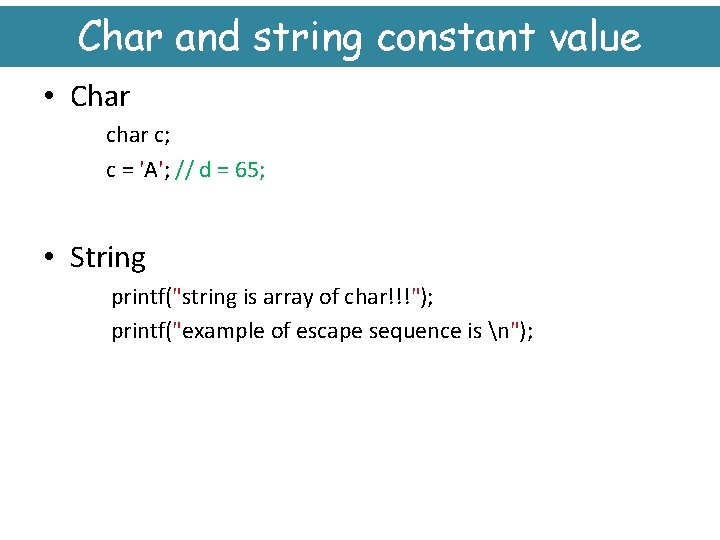
Char and string constant value • Char c; c = 'A'; // d = 65; • String printf("string is array of char!!!"); printf("example of escape sequence is n");
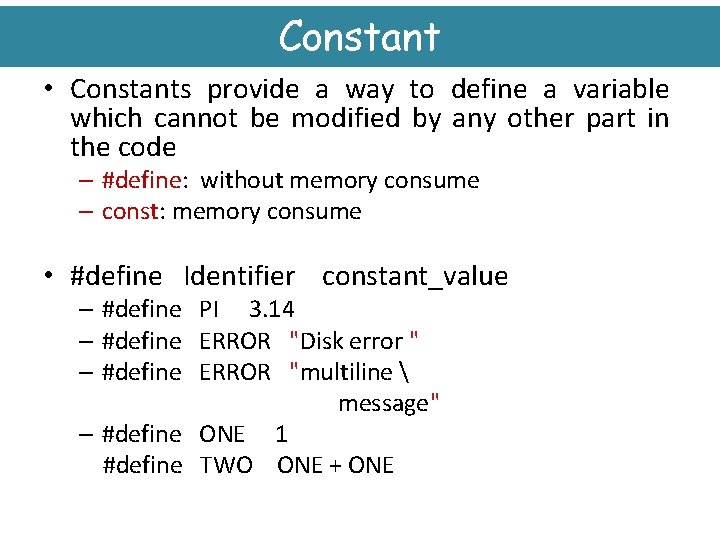
Constant • Constants provide a way to define a variable which cannot be modified by any other part in the code – #define: without memory consume – const: memory consume • #define Identifier constant_value – #define PI 3. 14 – #define ERROR "Disk error " – #define ERROR "multiline message" – #define ONE 1 #define TWO ONE + ONE
![Constant const DataType Identifier constantvalue const p 3 const Constant • const [Data_Type] Identifier = constant_value; – const p = 3; // const](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-19.jpg)
Constant • const [Data_Type] Identifier = constant_value; – const p = 3; // const int p = 3; – const p; p = 3. 14; // compile error – const p = 3. 14; // p = 3 because default is int – const float p = 3. 14;
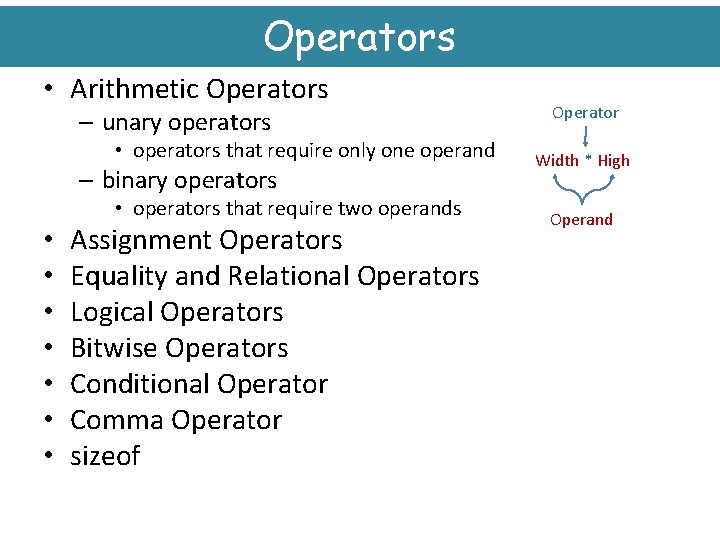
Operators • Arithmetic Operators – unary operators • operators that require only one operand – binary operators • operators that require two operands • • Assignment Operators Equality and Relational Operators Logical Operators Bitwise Operators Conditional Operator Comma Operator sizeof Operator Width * High Operand
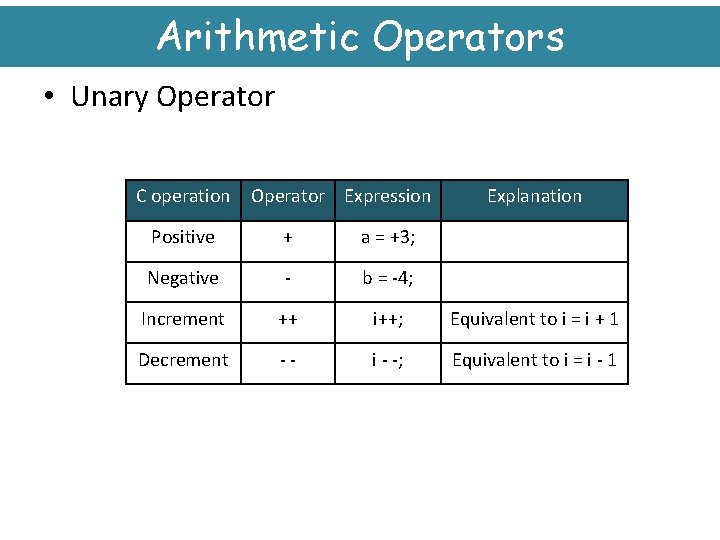
Arithmetic Operators • Unary Operator C operation Operator Expression Explanation Positive + a = +3; Negative - b = -4; Increment ++ i++; Equivalent to i = i + 1 Decrement -- i - -; Equivalent to i = i - 1
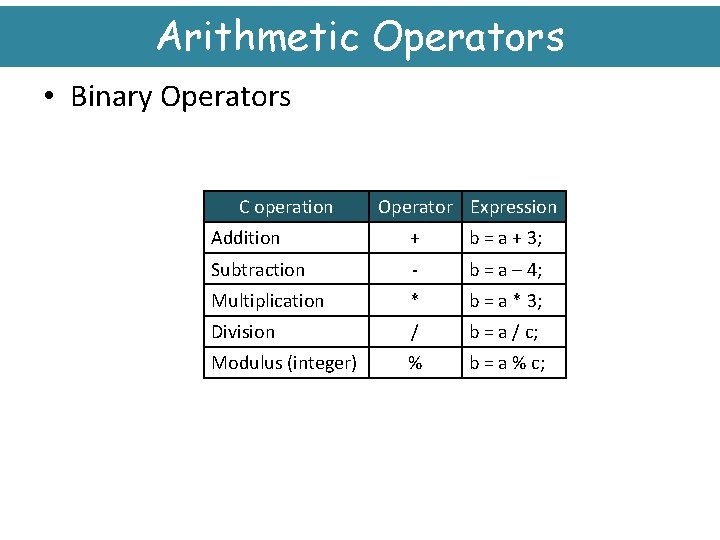
Arithmetic Operators • Binary Operators C operation Operator Expression Addition + b = a + 3; Subtraction - b = a – 4; Multiplication * b = a * 3; Division / b = a / c; Modulus (integer) % b = a % c;
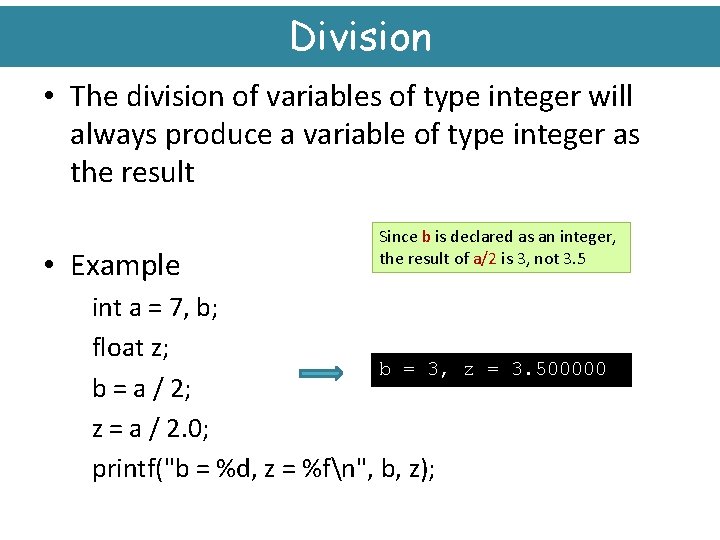
Division • The division of variables of type integer will always produce a variable of type integer as the result • Example Since b is declared as an integer, the result of a/2 is 3, not 3. 5 int a = 7, b; float z; b = 3, b = a / 2; z = a / 2. 0; printf("b = %d, z = %fn", b, z); z = 3. 500000
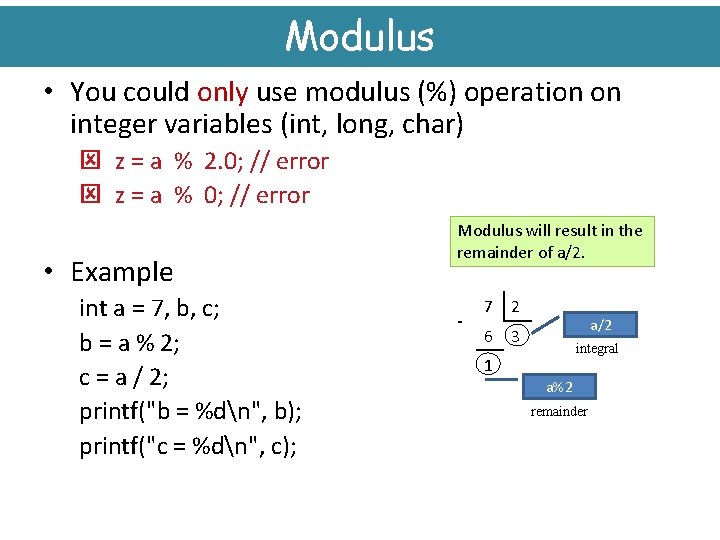
Modulus • You could only use modulus (%) operation on integer variables (int, long, char) z = a % 2. 0; // error z = a % 0; // error • Example int a = 7, b, c; b = a % 2; c = a / 2; printf("b = %dn", b); printf("c = %dn", c); Modulus will result in the remainder of a/2. - 7 2 6 3 a/2 integral 1 a%2 remainder
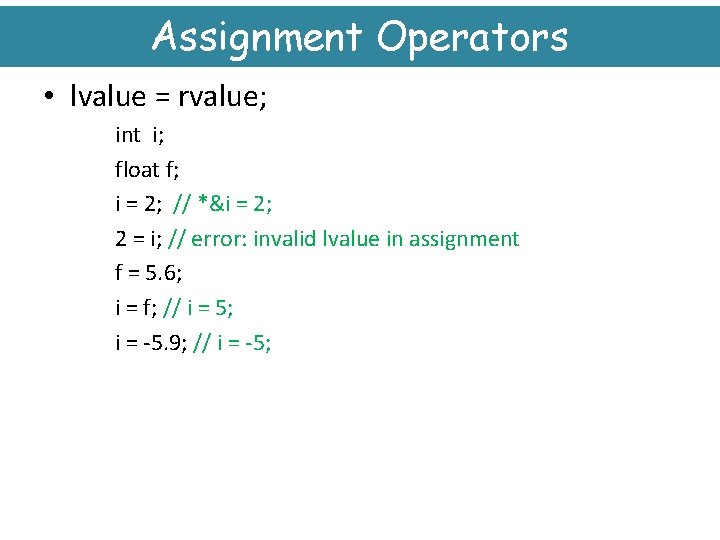
Assignment Operators • lvalue = rvalue; int i; float f; i = 2; // *&i = 2; 2 = i; // error: invalid lvalue in assignment f = 5. 6; i = f; // i = 5; i = -5. 9; // i = -5;
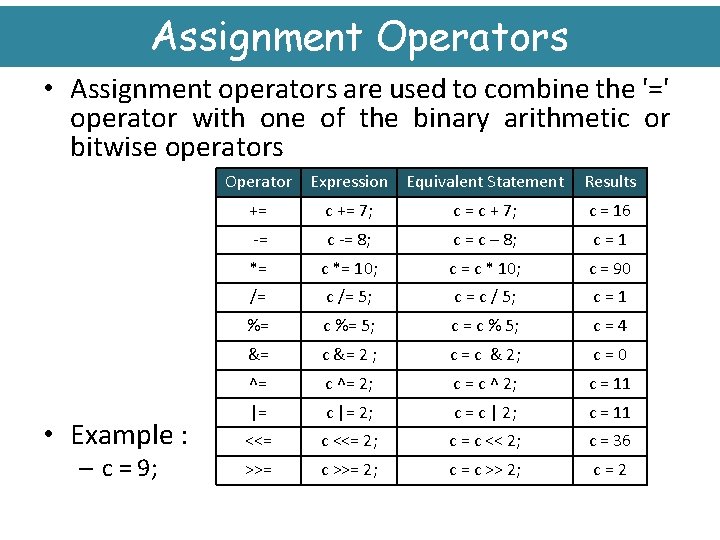
Assignment Operators • Assignment operators are used to combine the '=' operator with one of the binary arithmetic or bitwise operators Operator • Example : – c = 9; Expression Equivalent Statement Results += c += 7; c = c + 7; c = 16 -= c -= 8; c = c – 8; c=1 *= c *= 10; c = c * 10; c = 90 /= c /= 5; c = c / 5; c=1 %= c %= 5; c = c % 5; c=4 &= c &= 2 ; c = c & 2; c=0 ^= c ^= 2; c = c ^ 2; c = 11 |= c |= 2; c = c | 2; c = 11 <<= c <<= 2; c = c << 2; c = 36 >>= c >>= 2; c = c >> 2; c=2
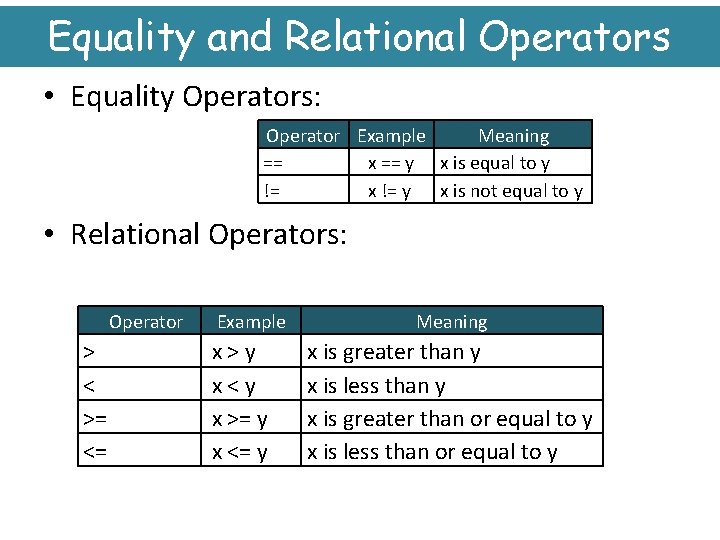
Equality and Relational Operators • Equality Operators: Operator Example Meaning == x == y x is equal to y != x != y x is not equal to y • Relational Operators: Operator > < >= <= Example x>y x<y x >= y x <= y Meaning x is greater than y x is less than y x is greater than or equal to y x is less than or equal to y
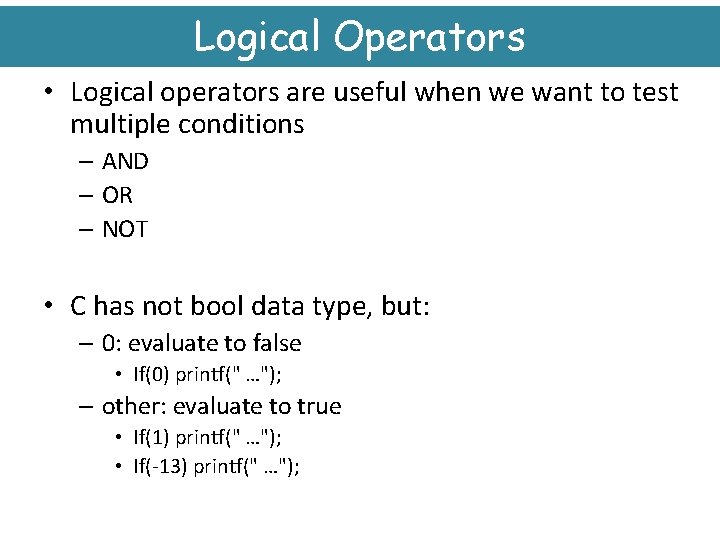
Logical Operators • Logical operators are useful when we want to test multiple conditions – AND – OR – NOT • C has not bool data type, but: – 0: evaluate to false • If(0) printf(" …"); – other: evaluate to true • If(1) printf(" …"); • If(-13) printf(" …");
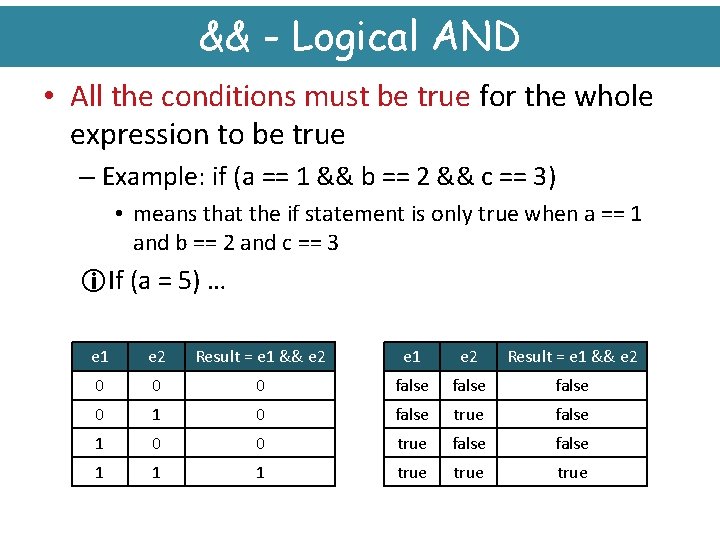
&& - Logical AND • All the conditions must be true for the whole expression to be true – Example: if (a == 1 && b == 2 && c == 3) • means that the if statement is only true when a == 1 and b == 2 and c == 3 If (a = 5) … e 1 e 2 Result = e 1 && e 2 0 0 0 false 0 1 0 false true false 1 0 0 true false 1 1 1 true
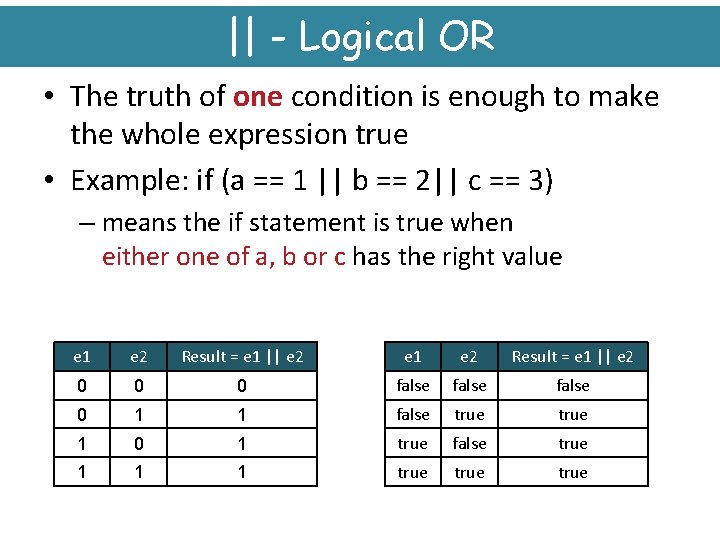
|| - Logical OR • The truth of one condition is enough to make the whole expression true • Example: if (a == 1 || b == 2|| c == 3) – means the if statement is true when either one of a, b or c has the right value e 1 e 2 Result = e 1 || e 2 0 0 0 false 0 1 1 false true 1 0 1 true false true 1 1 1 true
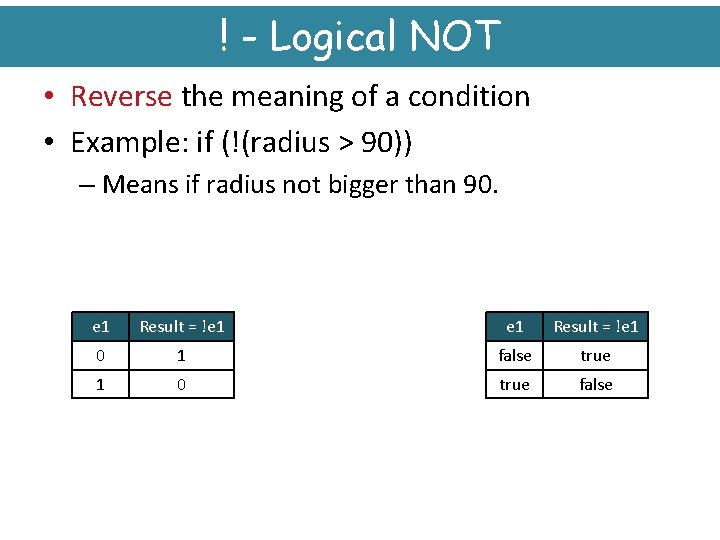
! - Logical NOT • Reverse the meaning of a condition • Example: if (!(radius > 90)) – Means if radius not bigger than 90. e 1 Result = !e 1 0 1 false true 1 0 true false
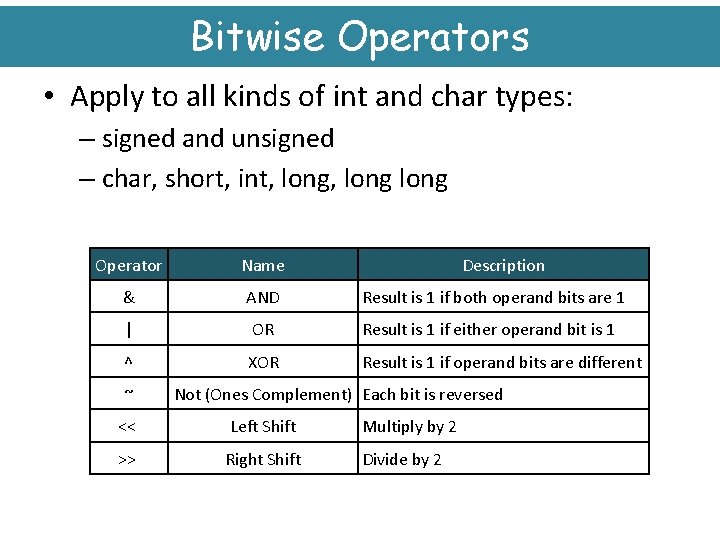
Bitwise Operators • Apply to all kinds of int and char types: – signed and unsigned – char, short, int, long Operator Name & AND Result is 1 if both operand bits are 1 | OR Result is 1 if either operand bit is 1 ^ XOR Result is 1 if operand bits are different ~ Description Not (Ones Complement) Each bit is reversed << Left Shift Multiply by 2 >> Right Shift Divide by 2
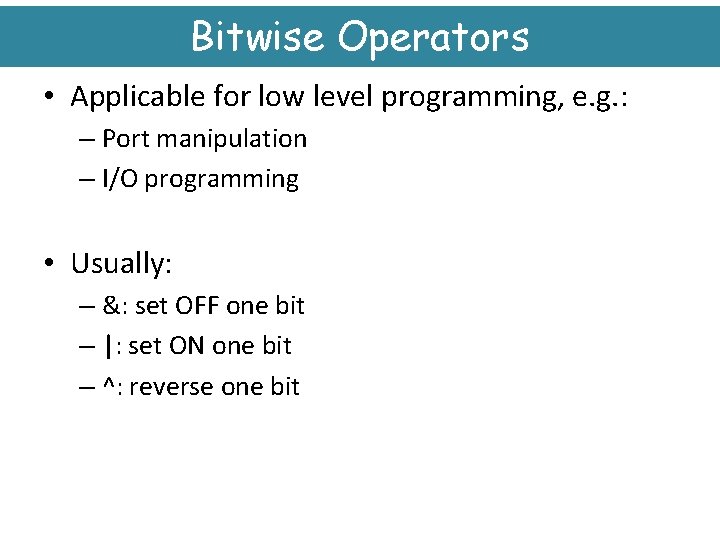
Bitwise Operators • Applicable for low level programming, e. g. : – Port manipulation – I/O programming • Usually: – &: set OFF one bit – |: set ON one bit – ^: reverse one bit
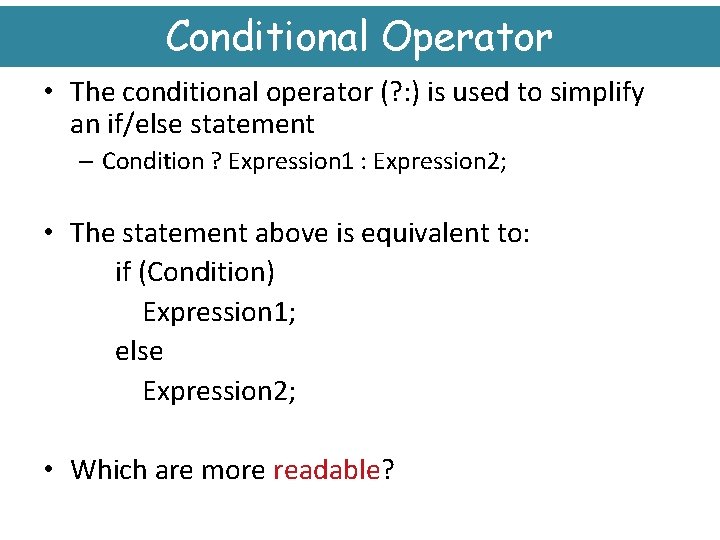
Conditional Operator • The conditional operator (? : ) is used to simplify an if/else statement – Condition ? Expression 1 : Expression 2; • The statement above is equivalent to: if (Condition) Expression 1; else Expression 2; • Which are more readable?
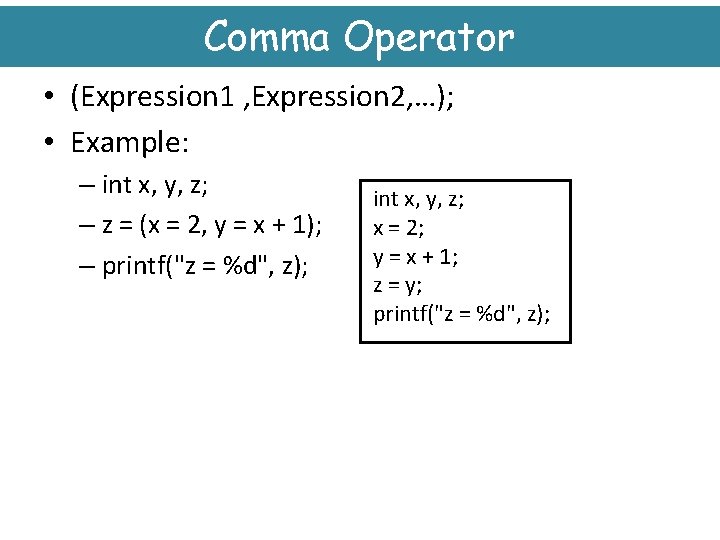
Comma Operator • (Expression 1 , Expression 2, …); • Example: – int x, y, z; – z = (x = 2, y = x + 1); – printf("z = %d", z); int x, y, z; x = 2; y = x + 1; z = y; printf("z = %d", z);
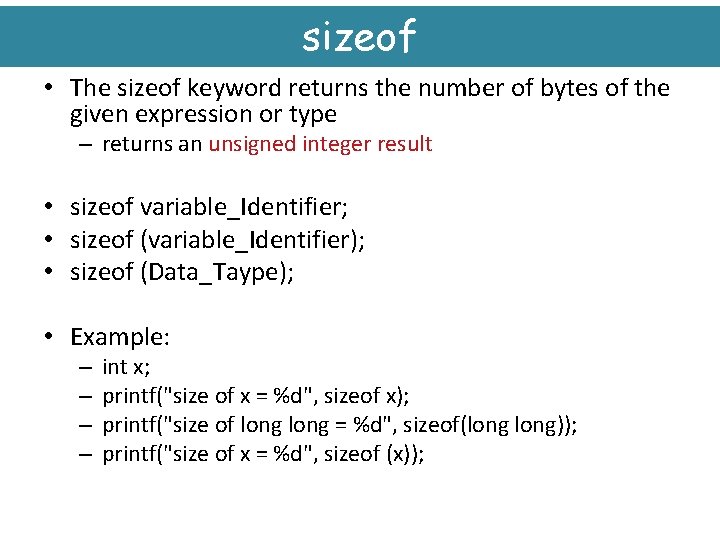
sizeof • The sizeof keyword returns the number of bytes of the given expression or type – returns an unsigned integer result • sizeof variable_Identifier; • sizeof (variable_Identifier); • sizeof (Data_Taype); • Example: – – int x; printf("size of x = %d", sizeof x); printf("size of long = %d", sizeof(long)); printf("size of x = %d", sizeof (x));
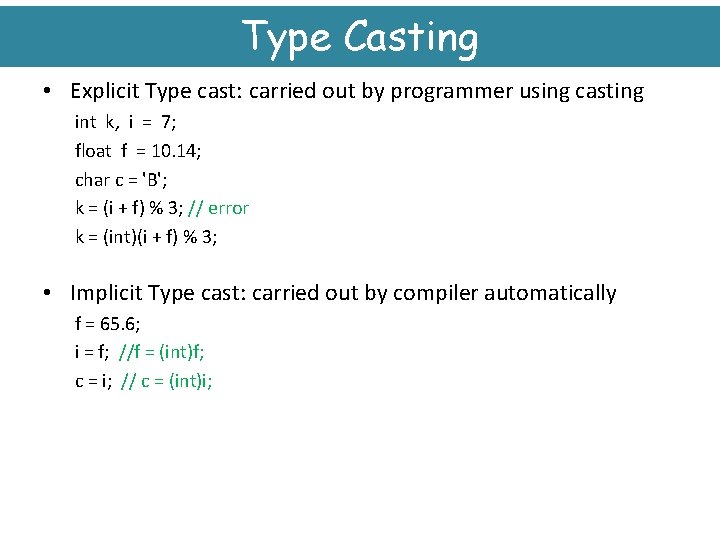
Type Casting • Explicit Type cast: carried out by programmer using casting int k, i = 7; float f = 10. 14; char c = 'B'; k = (i + f) % 3; // error k = (int)(i + f) % 3; • Implicit Type cast: carried out by compiler automatically f = 65. 6; i = f; //f = (int)f; c = i; // c = (int)i;
![Precedence Rules Primary Expression Operators Unary Operators lefttoright Precedence Rules Primary Expression Operators () []. -> Unary Operators * & + left-to-right](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-38.jpg)
Precedence Rules Primary Expression Operators () []. -> Unary Operators * & + left-to-right - ! ~ ++expr --expr (typecast) sizeof right-to-left * / % + >> << < > <= >= Binary Operators == != left-to-right & ^ | && || Ternary Operator ? : right-to-left Assignment Operators = += -= *= /= %= >>= <<= &= ^= |= right-to-left Post increment expr++ - Comma , expr-- left-to-right
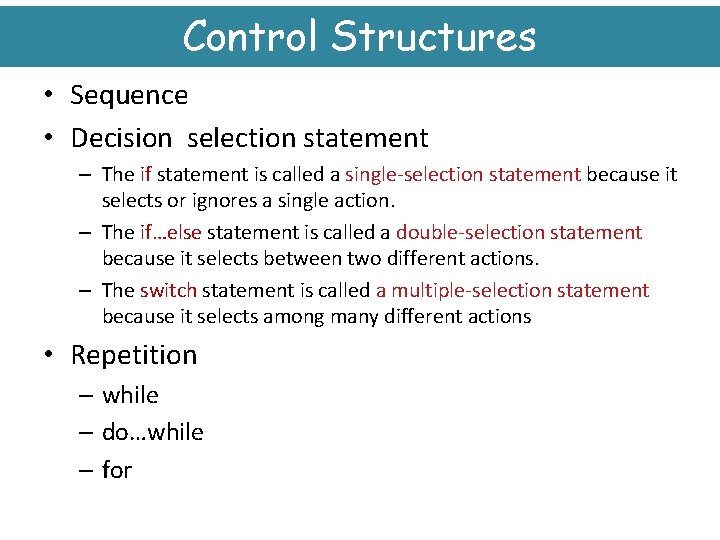
Control Structures • Sequence • Decision selection statement – The if statement is called a single-selection statement because it selects or ignores a single action. – The if…else statement is called a double-selection statement because it selects between two different actions. – The switch statement is called a multiple-selection statement because it selects among many different actions • Repetition – while – do…while – for
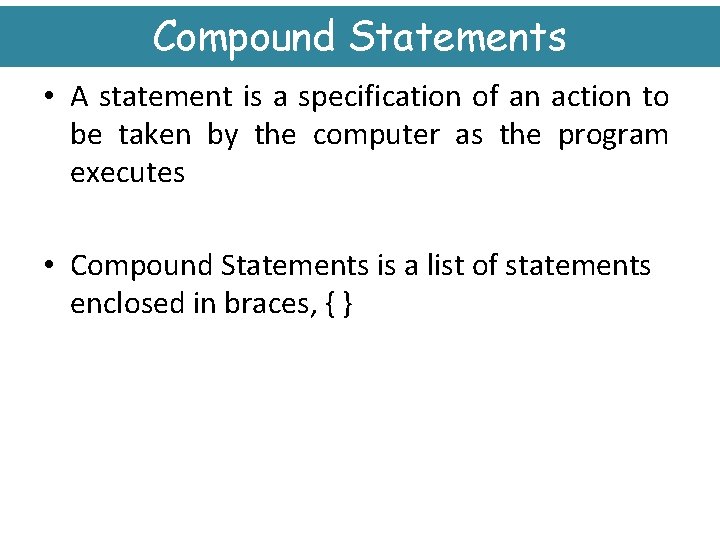
Compound Statements • A statement is a specification of an action to be taken by the computer as the program executes • Compound Statements is a list of statements enclosed in braces, { }
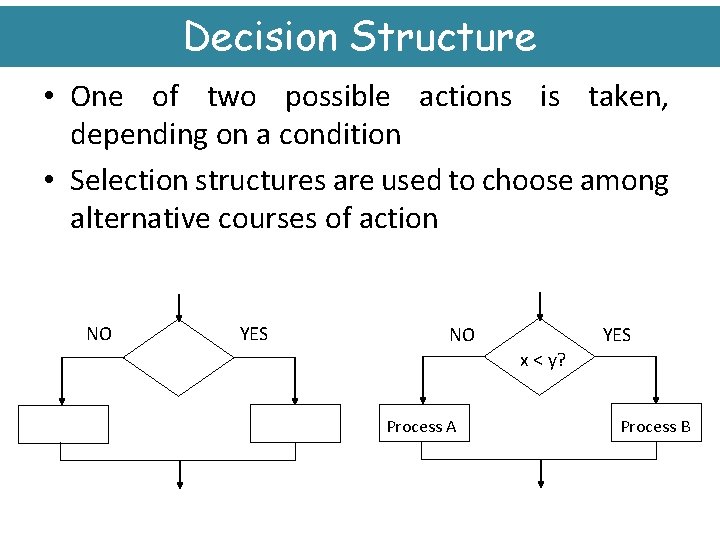
Decision Structure • One of two possible actions is taken, depending on a condition • Selection structures are used to choose among alternative courses of action NO YES x < y? Process A Process B
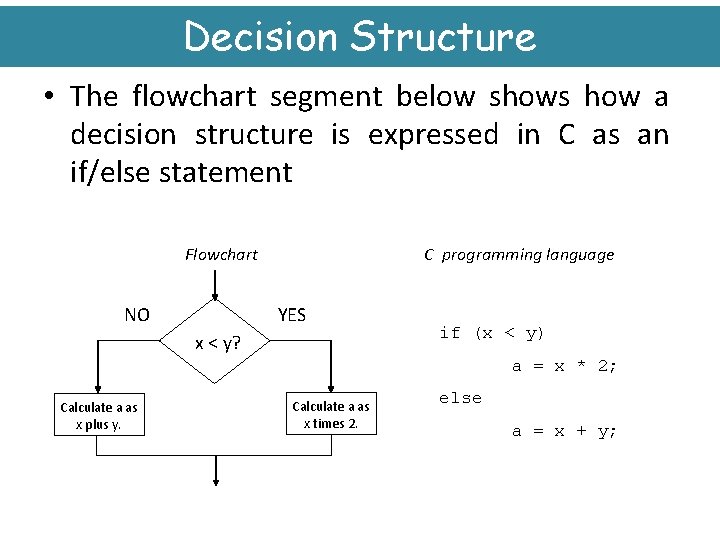
Decision Structure • The flowchart segment below shows how a decision structure is expressed in C as an if/else statement Flowchart NO C programming language YES x < y? if (x < y) a = x * 2; Calculate a as x plus y. Calculate a as x times 2. else a = x + y;
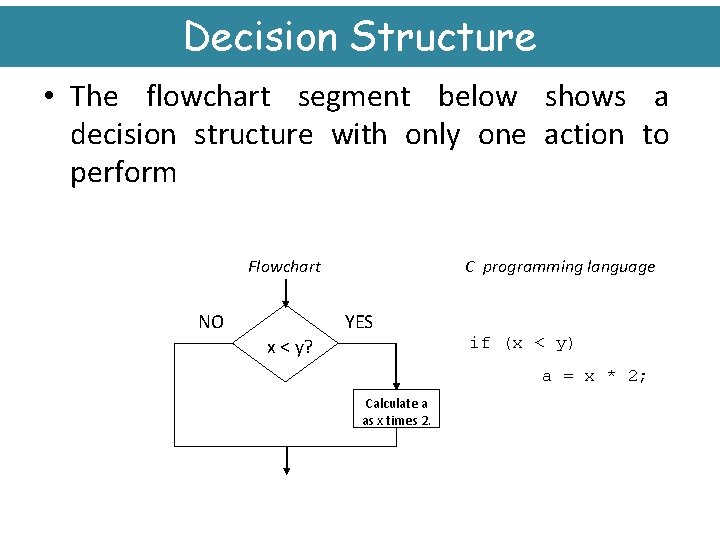
Decision Structure • The flowchart segment below shows a decision structure with only one action to perform C programming language Flowchart NO YES if (x < y) x < y? a = x * 2; Calculate a as x times 2.
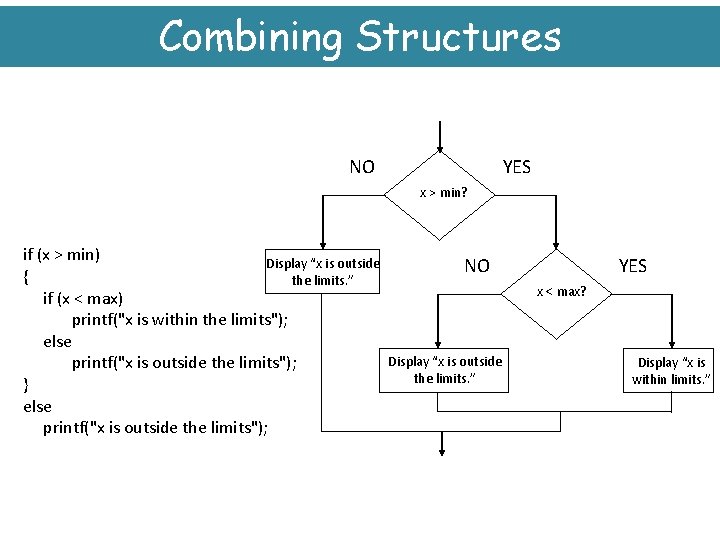
Combining Structures NO YES x > min? if (x > min) Display “x is outside { the limits. ” if (x < max) printf("x is within the limits"); else printf("x is outside the limits"); } else printf("x is outside the limits"); NO YES x < max? Display “x is outside the limits. ” Display “x is within limits. ”
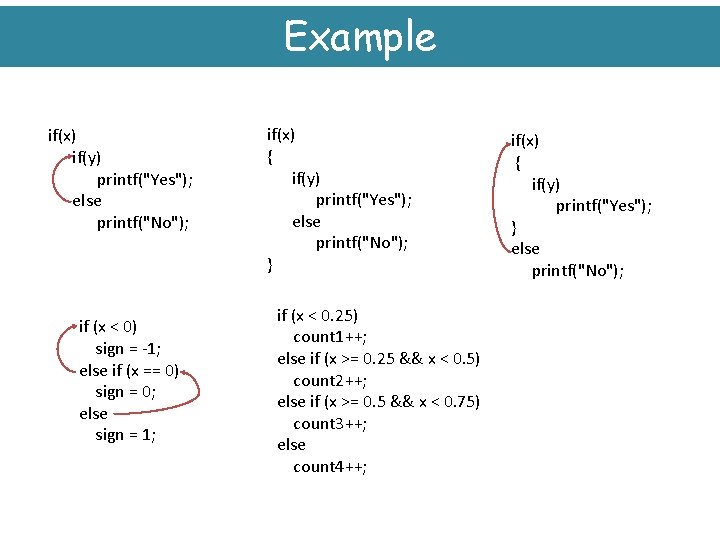
Example if(x) if(y) printf("Yes"); else printf("No"); if (x < 0) sign = -1; else if (x == 0) sign = 0; else sign = 1; if(x) { if(y) printf("Yes"); else printf("No"); } if (x < 0. 25) count 1++; else if (x >= 0. 25 && x < 0. 5) count 2++; else if (x >= 0. 5 && x < 0. 75) count 3++; else count 4++; if(x) { if(y) printf("Yes"); } else printf("No");
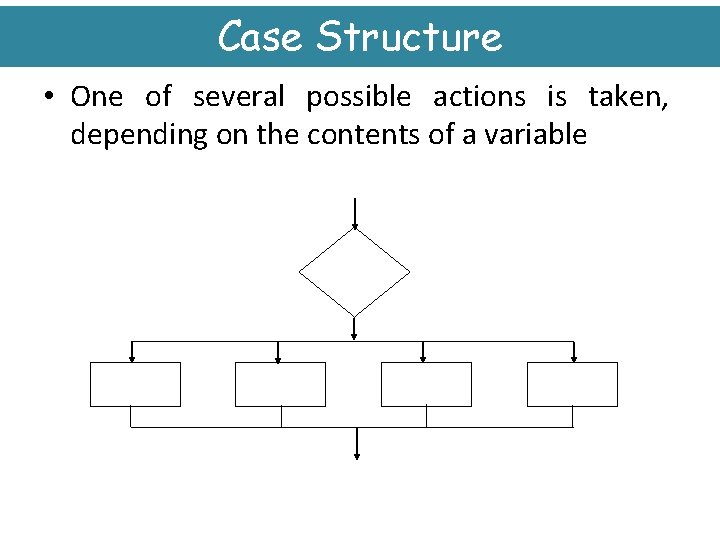
Case Structure • One of several possible actions is taken, depending on the contents of a variable
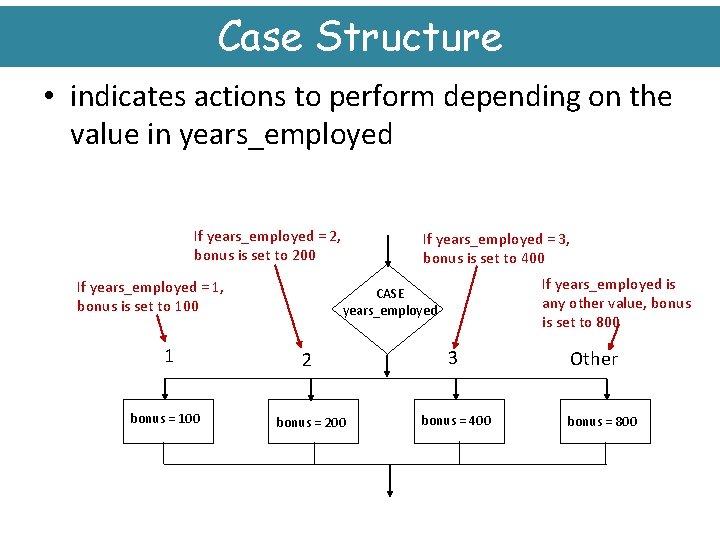
Case Structure • indicates actions to perform depending on the value in years_employed If years_employed = 2, bonus is set to 200 If years_employed = 1, bonus is set to 100 1 bonus = 100 If years_employed = 3, bonus is set to 400 If years_employed is any other value, bonus is set to 800 CASE years_employed 2 3 bonus = 200 bonus = 400 Other bonus = 800
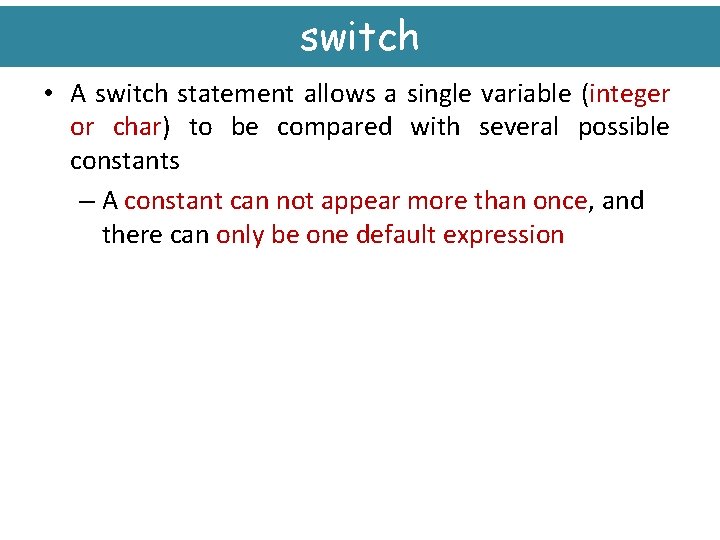
switch • A switch statement allows a single variable (integer or char) to be compared with several possible constants – A constant can not appear more than once, and there can only be one default expression
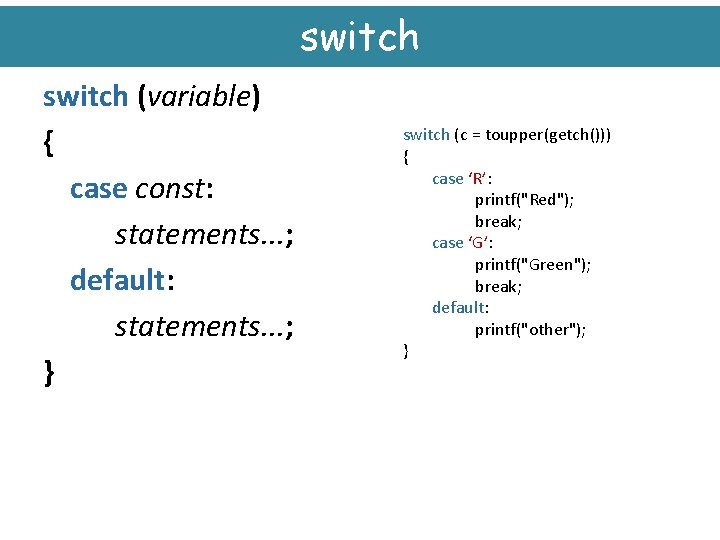
switch (variable) { case const: statements. . . ; default: statements. . . ; } switch (c = toupper(getch())) { case ‘R’: printf("Red"); break; case ‘G’: printf("Green"); break; default: printf("other"); }
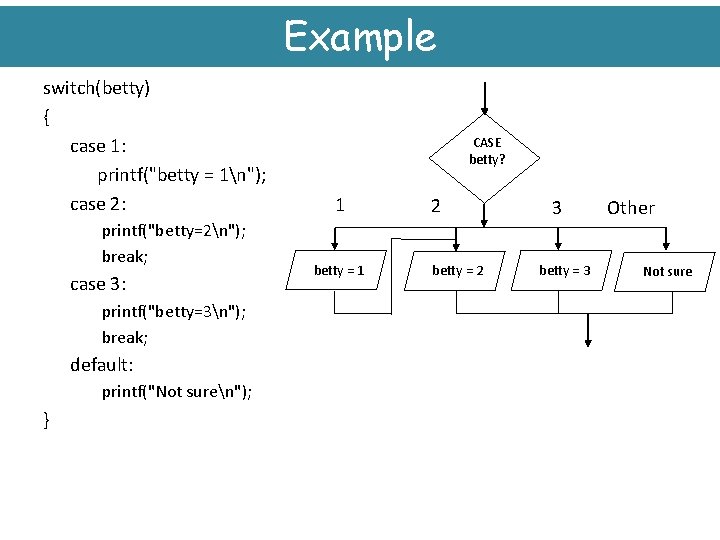
Example switch(betty) { case 1: printf("betty = 1n"); case 2: printf("betty=2n"); break; case 3: printf("betty=3n"); break; default: printf("Not suren"); } CASE betty? 1 betty = 1 2 betty = 2 3 betty = 3 Other Not sure
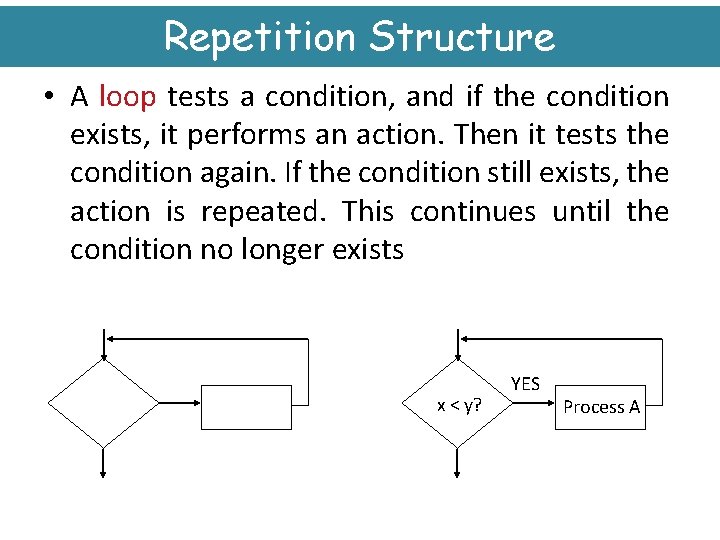
Repetition Structure • A loop tests a condition, and if the condition exists, it performs an action. Then it tests the condition again. If the condition still exists, the action is repeated. This continues until the condition no longer exists x < y? YES Process A
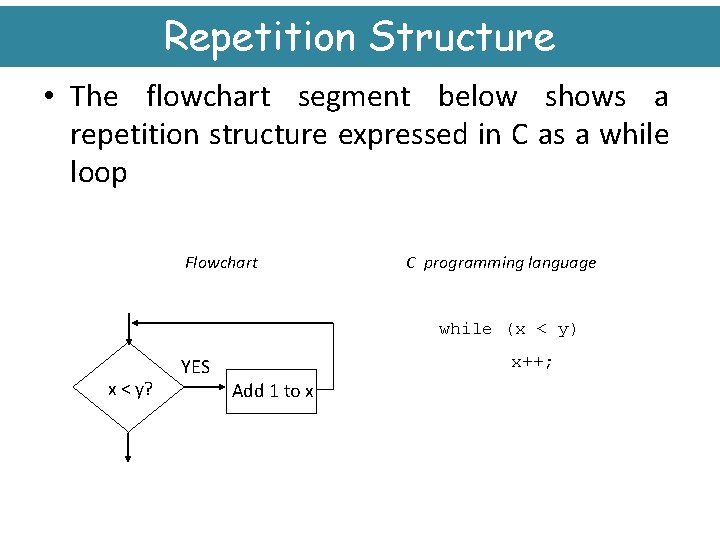
Repetition Structure • The flowchart segment below shows a repetition structure expressed in C as a while loop Flowchart C programming language while (x < y) x < y? YES x++; Add 1 to x
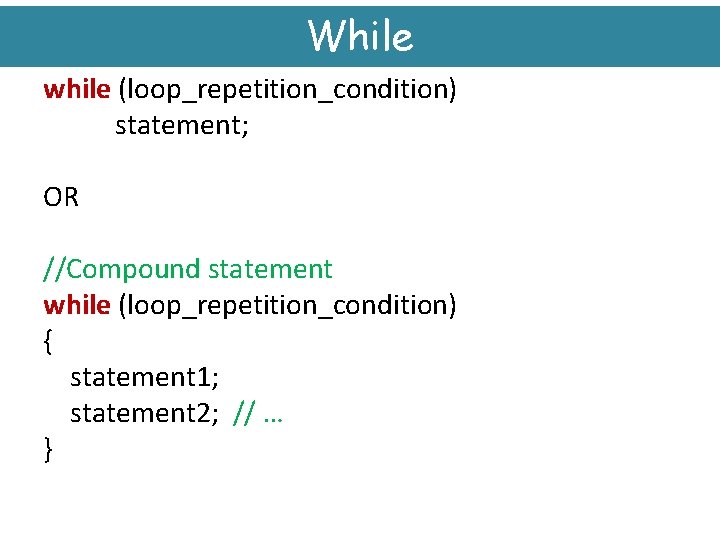
While while (loop_repetition_condition) statement; OR //Compound statement while (loop_repetition_condition) { statement 1; statement 2; // … }
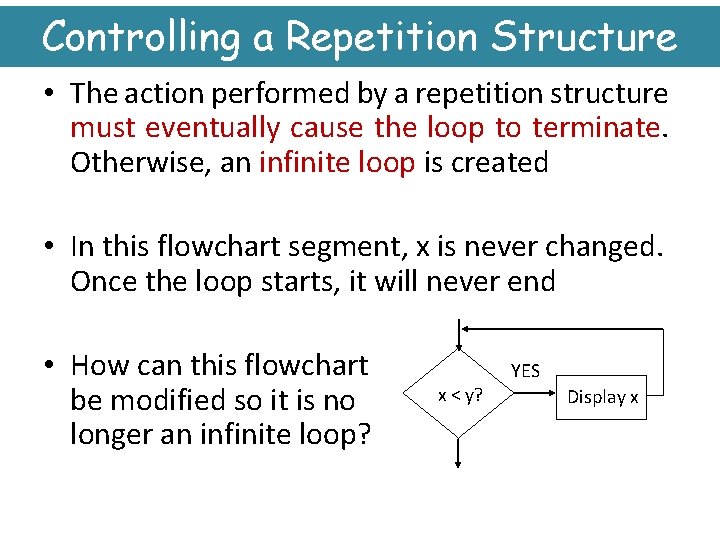
Controlling a Repetition Structure • The action performed by a repetition structure must eventually cause the loop to terminate. Otherwise, an infinite loop is created • In this flowchart segment, x is never changed. Once the loop starts, it will never end • How can this flowchart be modified so it is no longer an infinite loop? x < y? YES Display x
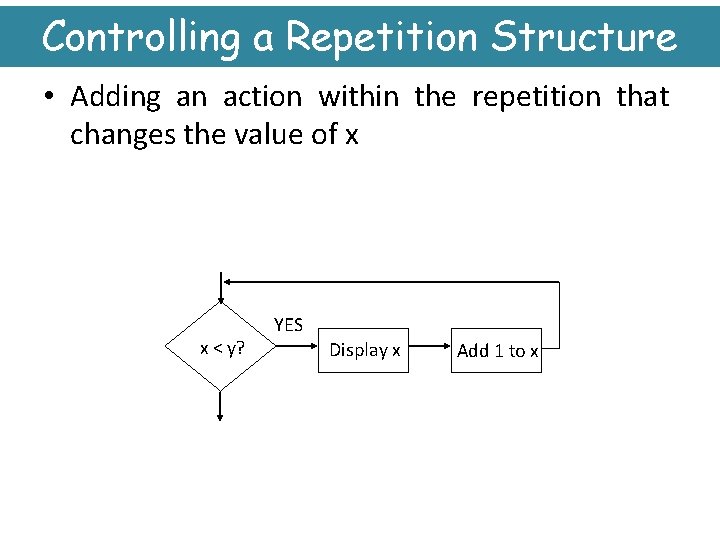
Controlling a Repetition Structure • Adding an action within the repetition that changes the value of x x < y? YES Display x Add 1 to x
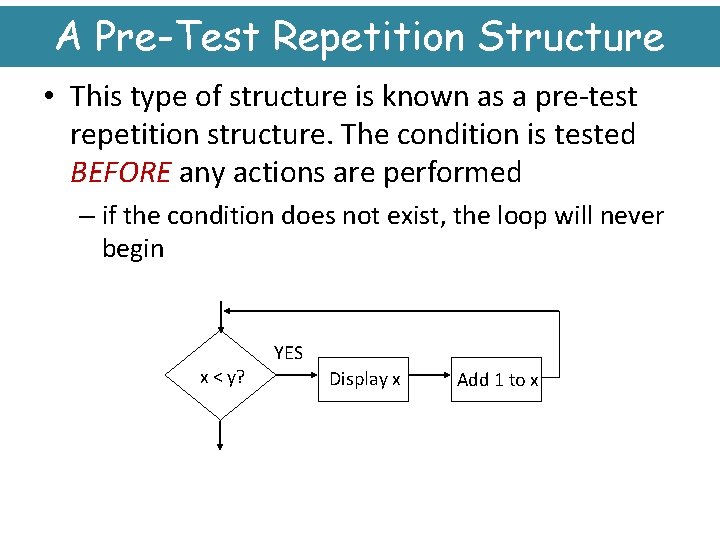
A Pre-Test Repetition Structure • This type of structure is known as a pre-test repetition structure. The condition is tested BEFORE any actions are performed – if the condition does not exist, the loop will never begin x < y? YES Display x Add 1 to x
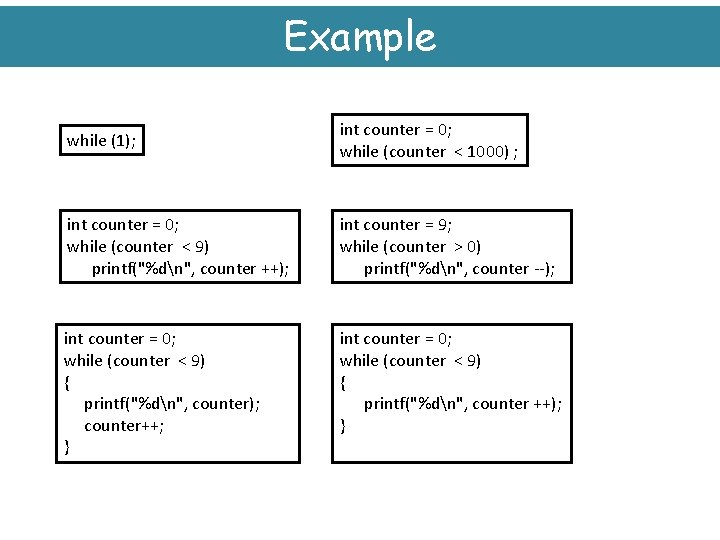
Example while (1); int counter = 0; while (counter < 1000) ; int counter = 0; while (counter < 9) printf("%dn", counter ++); int counter = 9; while (counter > 0) printf("%dn", counter --); int counter = 0; while (counter < 9) { printf("%dn", counter); counter++; } int counter = 0; while (counter < 9) { printf("%dn", counter ++); }
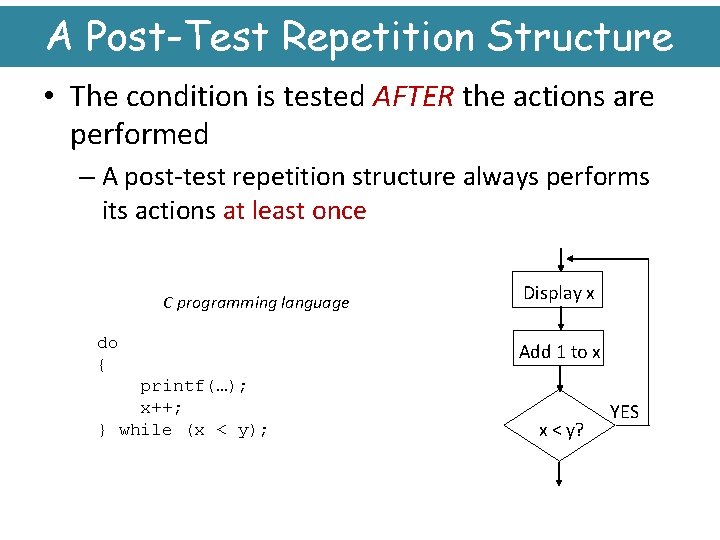
A Post-Test Repetition Structure • The condition is tested AFTER the actions are performed – A post-test repetition structure always performs its actions at least once C programming language do { printf(…); x++; } while (x < y); Display x Add 1 to x x < y? YES
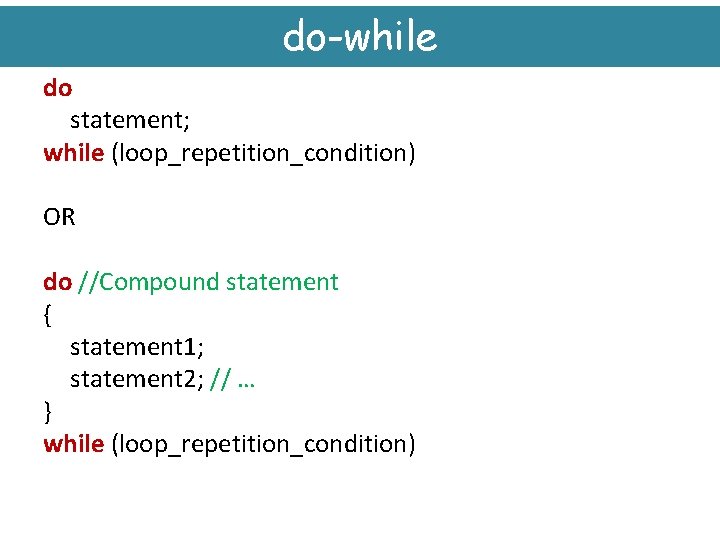
do-while do statement; while (loop_repetition_condition) OR do //Compound statement { statement 1; statement 2; // … } while (loop_repetition_condition)
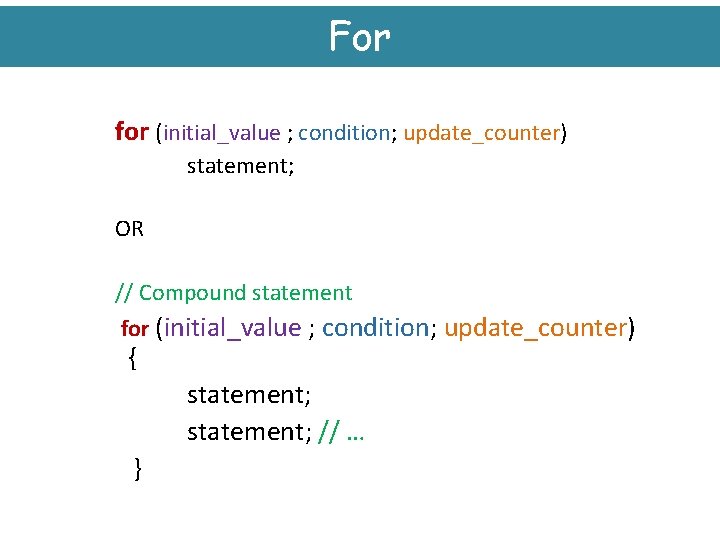
For for (initial_value ; condition; update_counter) statement; OR // Compound statement for (initial_value ; condition; update_counter) { statement; // … }
![Array Generic declaration typename variablenamesize typename is any type variablename Array • Generic declaration: typename variablename[size]; – – – typename is any type variablename](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-61.jpg)
Array • Generic declaration: typename variablename[size]; – – – typename is any type variablename is any legal variable name size is a number the compiler can figure out For example : int a[10]; Defines an array of ints with subscripts ranging from 0 to 9 There are 10*sizeof(int) bytes of memory reserved for this array. 0 a 1 2 3 4 5 6 7 8 10 – You can use a[0]=10; x=a[2]; a[3]=a[2]; etc. – You can use scanf("%d", &a[3]); 9
![Array Representation int A3 0 x 1008 A2 0 x 1004 A1 0 x Array Representation int A[3]; 0 x 1008 A[2] 0 x 1004 A[1] 0 x](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-62.jpg)
Array Representation int A[3]; 0 x 1008 A[2] 0 x 1004 A[1] 0 x 1000 A[0] All elements of same type – homogenous Last element (index size - 1) First element (index 0) A[-1] array[0] = 3; array[2] = 4; array[10] = 5; array[-1] = 6; No bounds checking! 3 * 4 = 12 sizeof(A)? sizeof(A[0]) = sizeof(A[1]) = sizeof(A[2])? 4
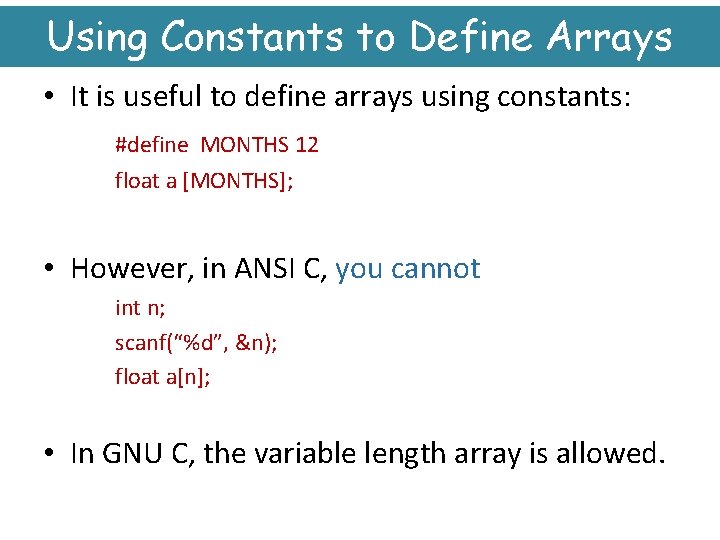
Using Constants to Define Arrays • It is useful to define arrays using constants: #define MONTHS 12 float a [MONTHS]; • However, in ANSI C, you cannot int n; scanf(“%d”, &n); float a[n]; • In GNU C, the variable length array is allowed.
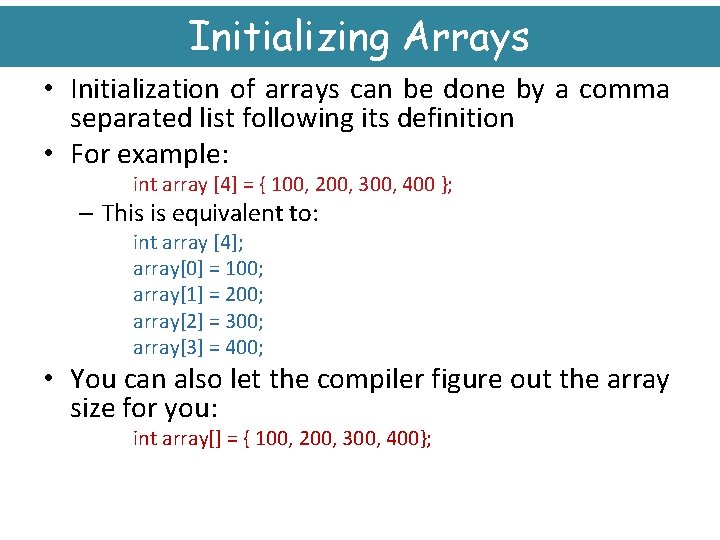
Initializing Arrays • Initialization of arrays can be done by a comma separated list following its definition • For example: int array [4] = { 100, 200, 300, 400 }; – This is equivalent to: int array [4]; array[0] = 100; array[1] = 200; array[2] = 300; array[3] = 400; • You can also let the compiler figure out the array size for you: int array[] = { 100, 200, 300, 400};
![Initializing Arrays For example int array 4 100 200 Initializing Arrays • For example: int array [4] = { 100, 200 }; •](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-65.jpg)
Initializing Arrays • For example: int array [4] = { 100, 200 }; • Also can be done by int array [4] = { 100, 200, 0, 0 }; – This is equivalent to int array [4]; array[0] = 100; array[1] = 200; array[2] = 0; array[3] = 0;
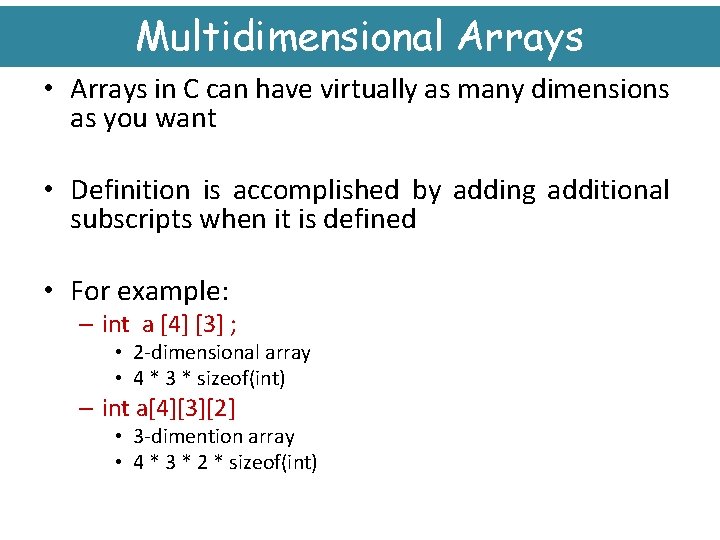
Multidimensional Arrays • Arrays in C can have virtually as many dimensions as you want • Definition is accomplished by adding additional subscripts when it is defined • For example: – int a [4] [3] ; • 2 -dimensional array • 4 * 3 * sizeof(int) – int a[4][3][2] • 3 -dimention array • 4 * 3 * 2 * sizeof(int)
![Multidimensional Arrays Representation a00 a01 a02 a03 Row 0 Row 1 Row 2 Column Multidimensional Arrays Representation a[0][0] a[0][1] a[0][2] a[0][3] Row 0 Row 1 Row 2 Column](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-67.jpg)
Multidimensional Arrays Representation a[0][0] a[0][1] a[0][2] a[0][3] Row 0 Row 1 Row 2 Column 0 Column 1 Column 2 Column 3 a[ 0 ][ 1 ] a[ 0 ][ 2 ] a[ 0 ][ 3 ] a[ 1 ][ 0 ] a[ 1 ][ 2 ] a[ 1 ][ 3 ] a[ 2 ][ 0 ] a[ 2 ][ 1 ] a[ 2 ][ 3 ] a[1][0] a[1][1] a[1][2] Column subscript Array name Row subscript a[1][3] a[2][0] a[2][1] a[2][2] a[2][3] int a[n][m] ; &a[i][j] = [(m * i) + j] * (sizeof(int)) + &a[0]
![Initializing Multidimensional Arrays The following initializes a43 int a4 3 1 Initializing Multidimensional Arrays • The following initializes a[4][3]: int a[4] [3] = { {1,](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-68.jpg)
Initializing Multidimensional Arrays • The following initializes a[4][3]: int a[4] [3] = { {1, 2, 3} , {4, 5, 6} , {7, 8, 9} , {10, 11, 12} }; • Also can be done by: int a[4] [3] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 }; – is equivalent to a[0][0] = 1; a[0][1] = 2; a[0][2] = 3; a[1][0] = 4; . . . a[3][2] = 12;
![Examples Initialization Example short b 2 1 Examples • Initialization – Example: • short b[ 2 ] = { { 1,](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-69.jpg)
Examples • Initialization – Example: • short b[ 2 ] = { { 1, 2 }, { 3, 4 } }; 1 2 3 4 – If not enough, unspecified elements set to zero • short b[ 2 ] = { { 1 }, { 3, 4 } }; 1 0 3 4
![Examples int a10 Ok int a2 5 Syntax error int a5 Examples int a[10]; // Ok int a[2. 5]; // Syntax error int a[-5]; //](https://slidetodoc.com/presentation_image_h2/4afc69848ccfd3e3cd3683119ac74fd1/image-70.jpg)
Examples int a[10]; // Ok int a[2. 5]; // Syntax error int a[-5]; // Syntax error int a[0]; // Logical error a[3. 6] = 10; // Syntax error or a[3] = 10; a[3. 2] = 10; // Syntax error or a[3] = 10; int b[2][3] = { {1, 2}, {3, 4}, {5, 6} }; // Syntax error
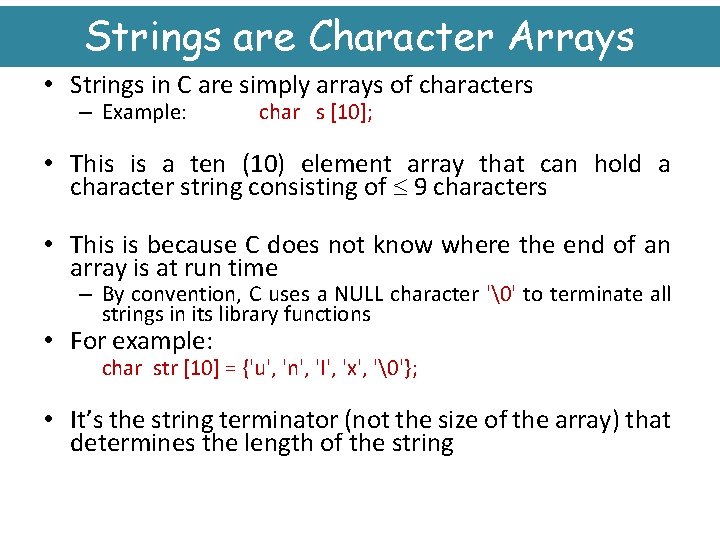
Strings are Character Arrays • Strings in C are simply arrays of characters – Example: char s [10]; • This is a ten (10) element array that can hold a character string consisting of 9 characters • This is because C does not know where the end of an array is at run time – By convention, C uses a NULL character '