Sharif University of Technology C Programming Languages Lecturer
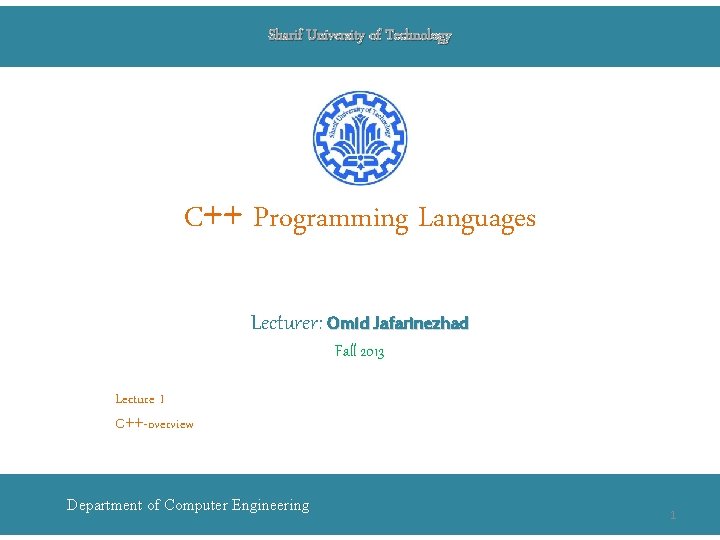
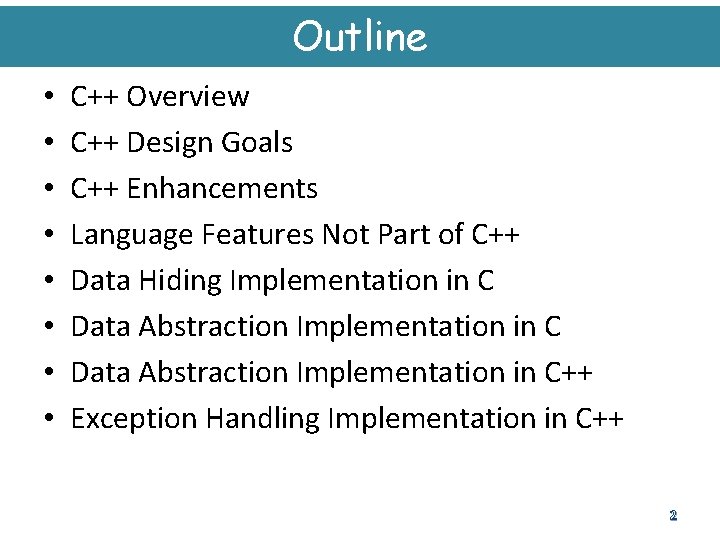
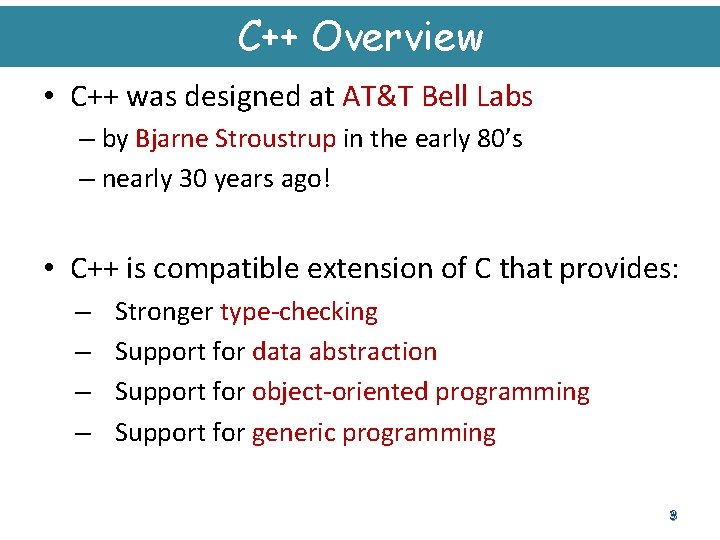
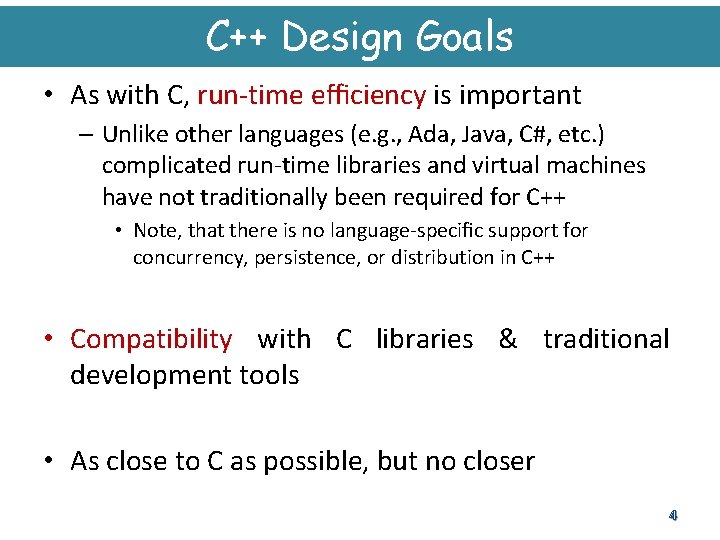
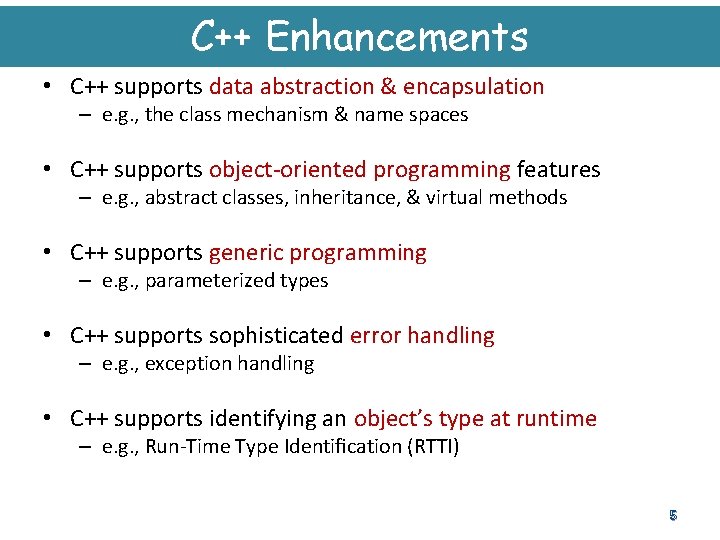
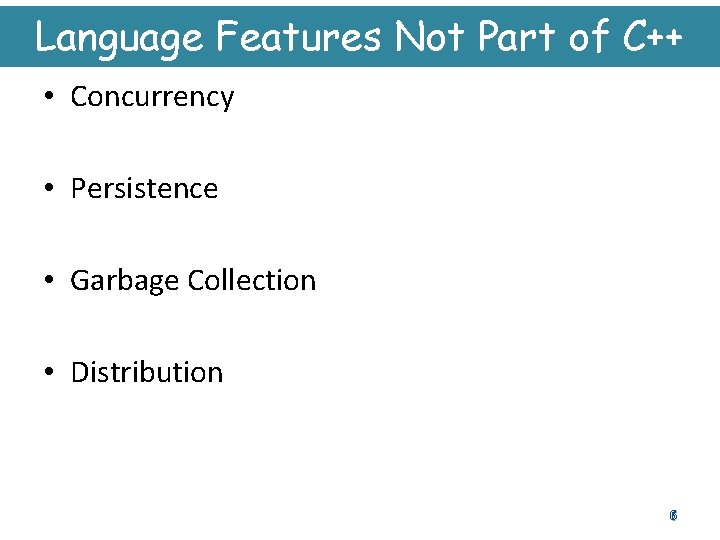
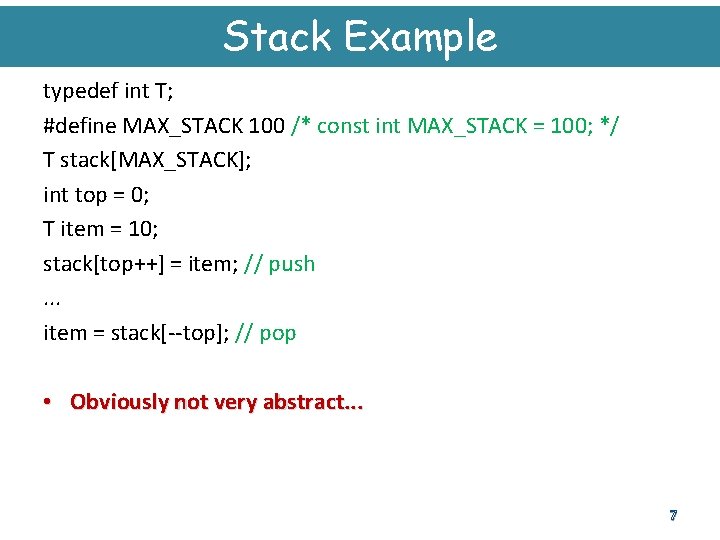
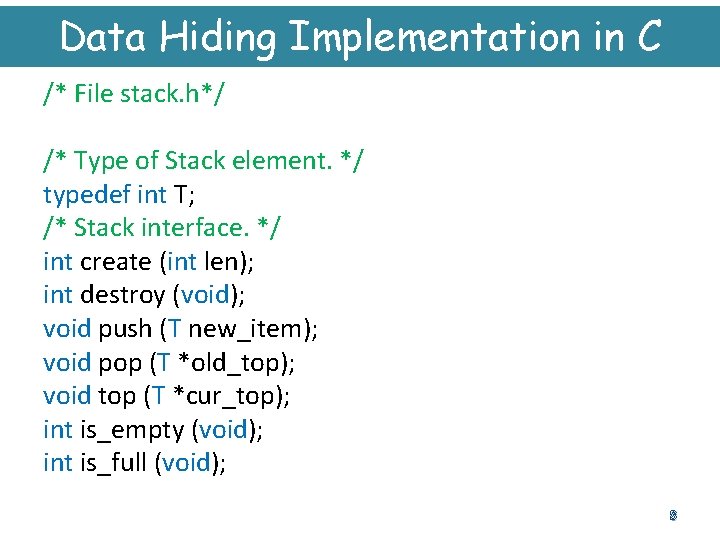
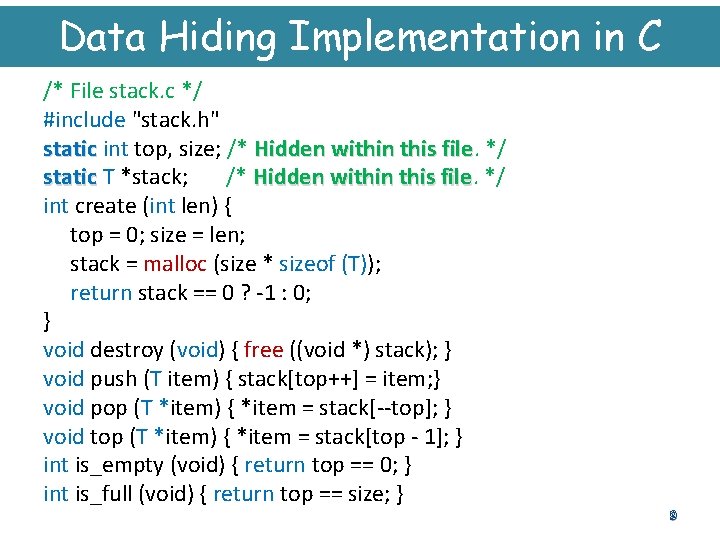
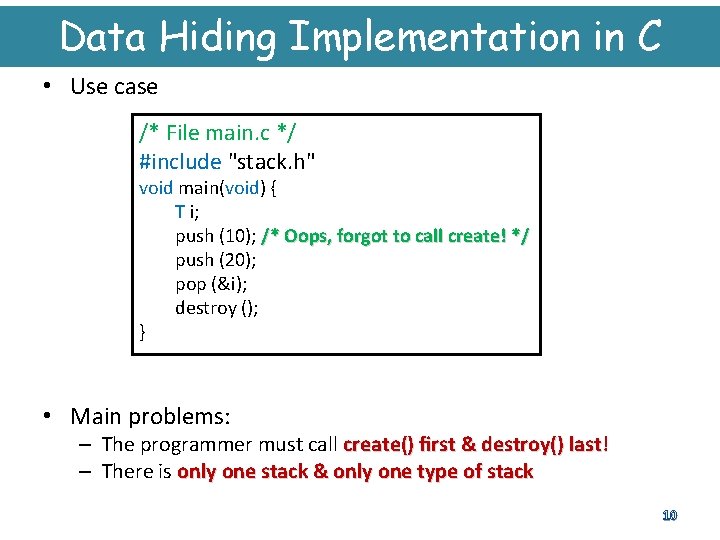
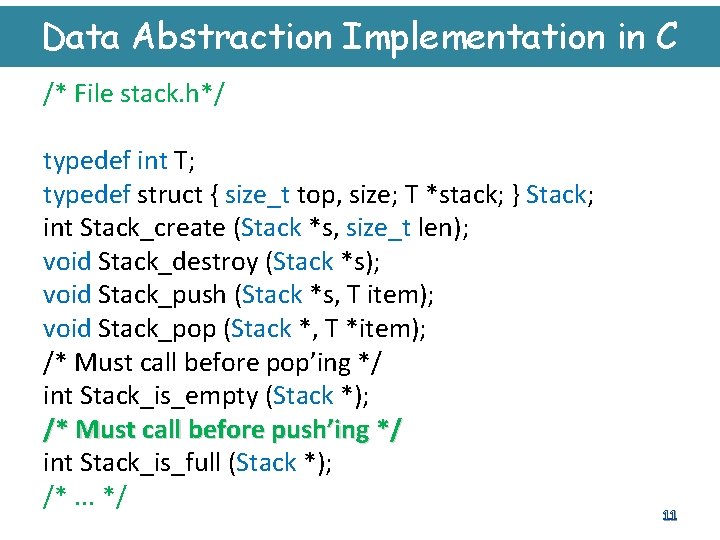
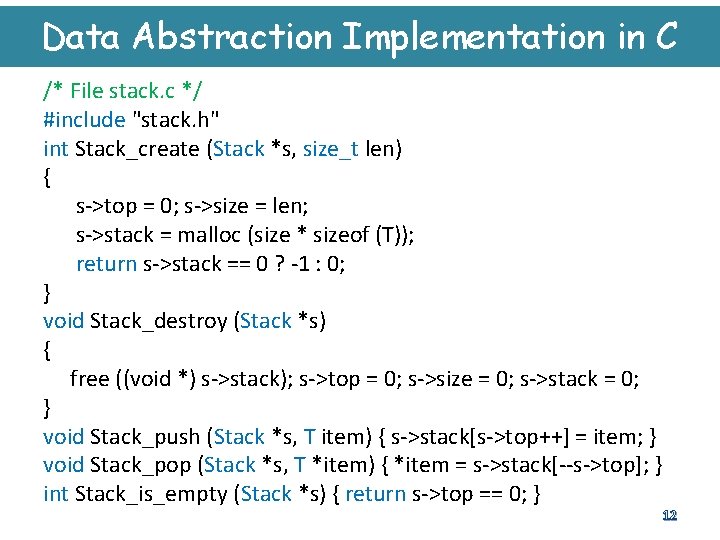
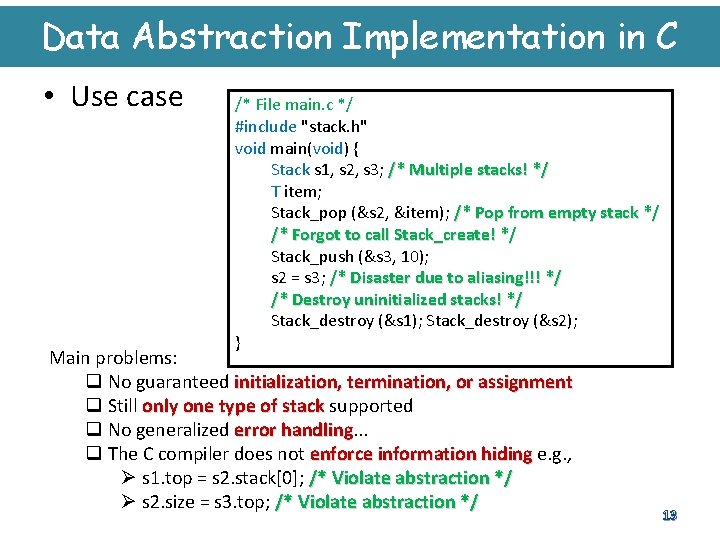
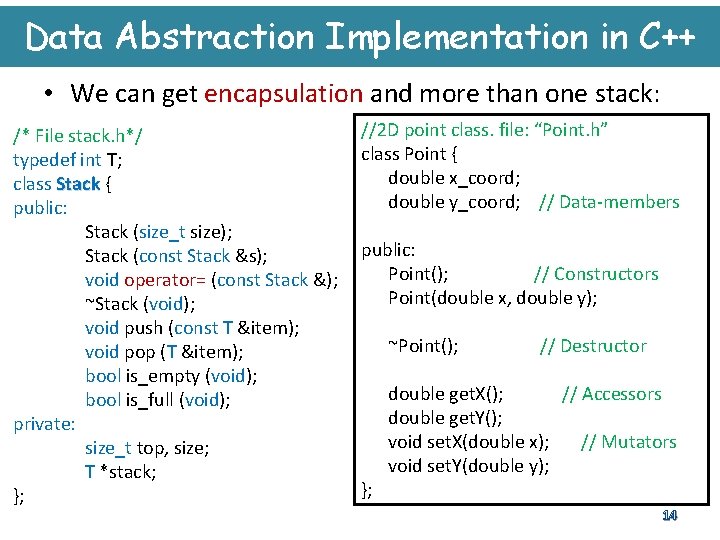
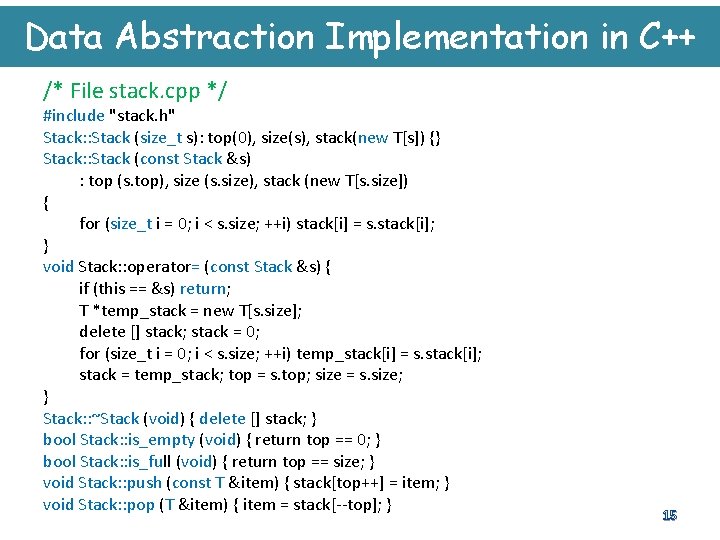
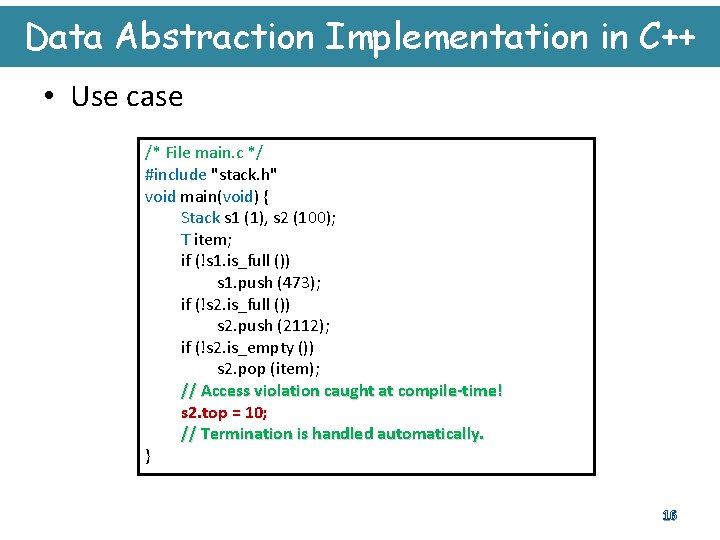
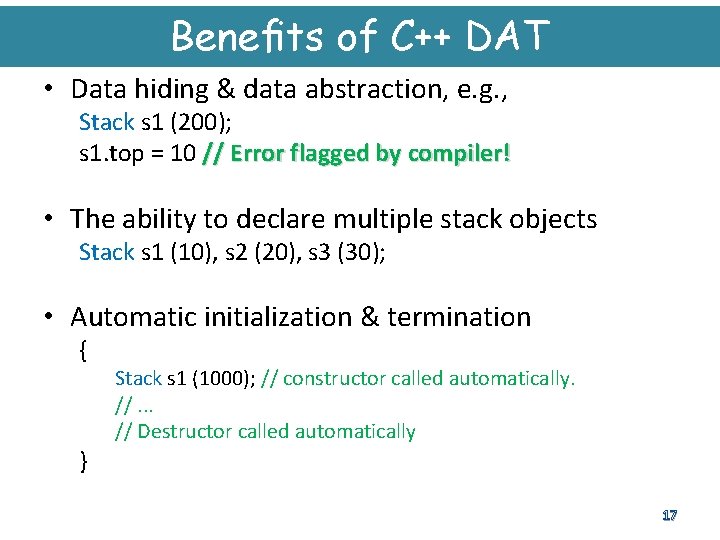
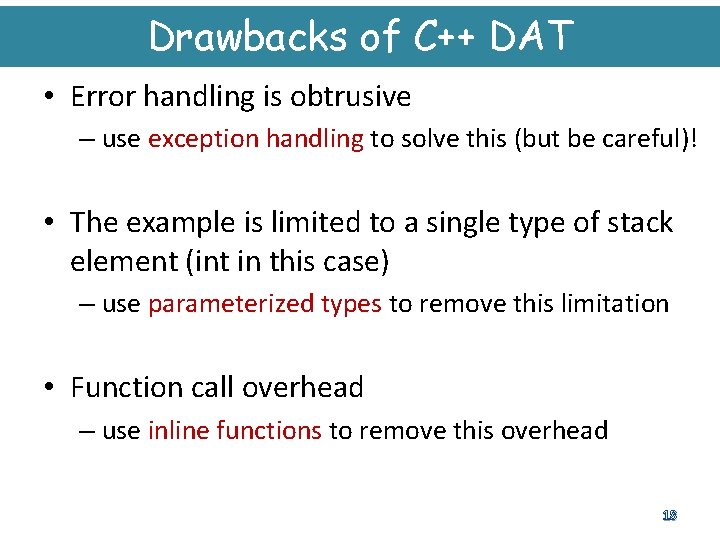
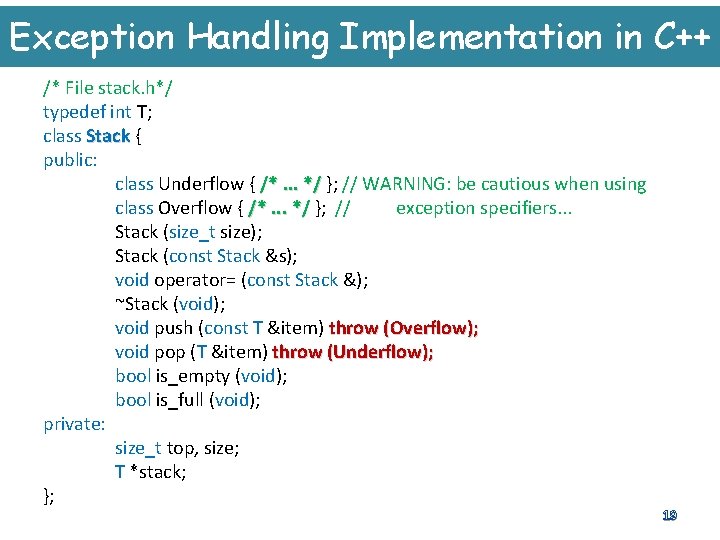
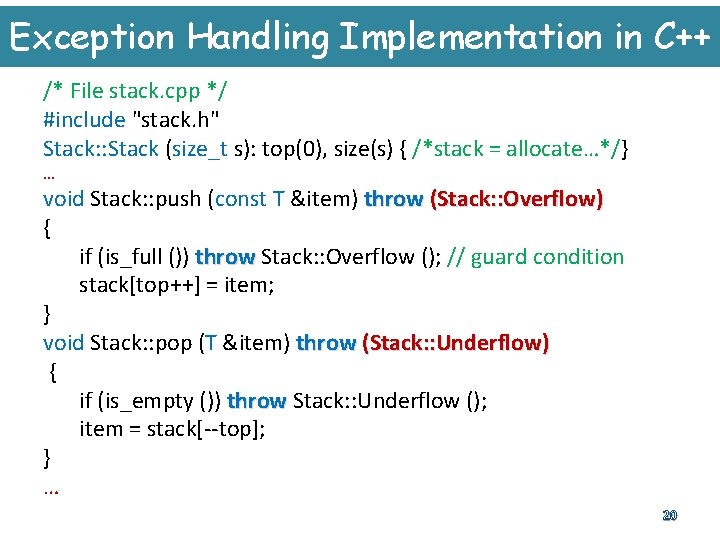
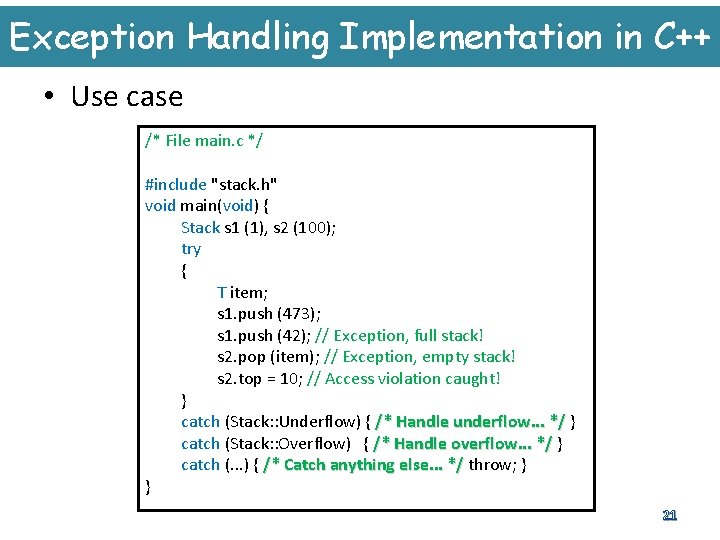
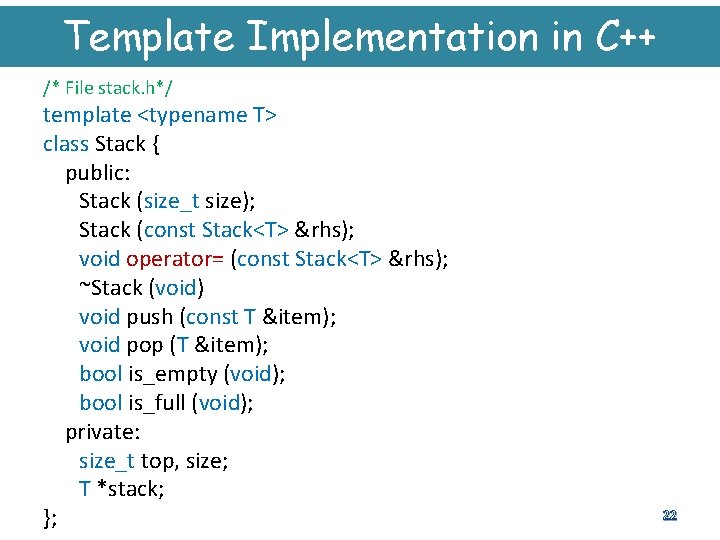
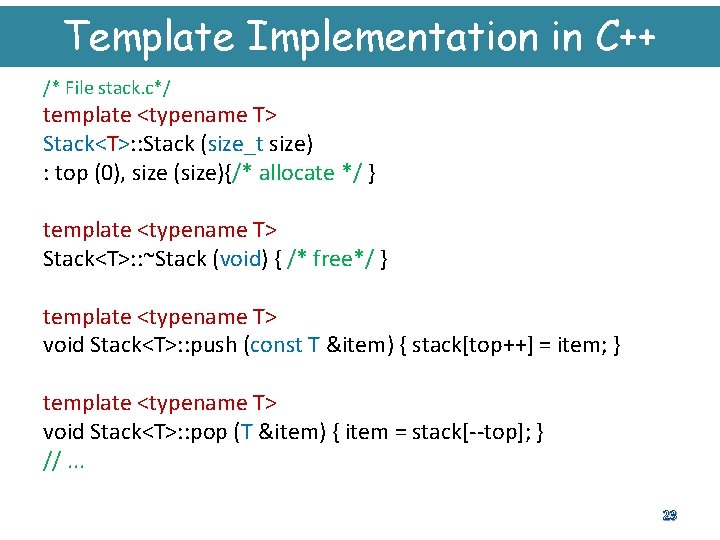
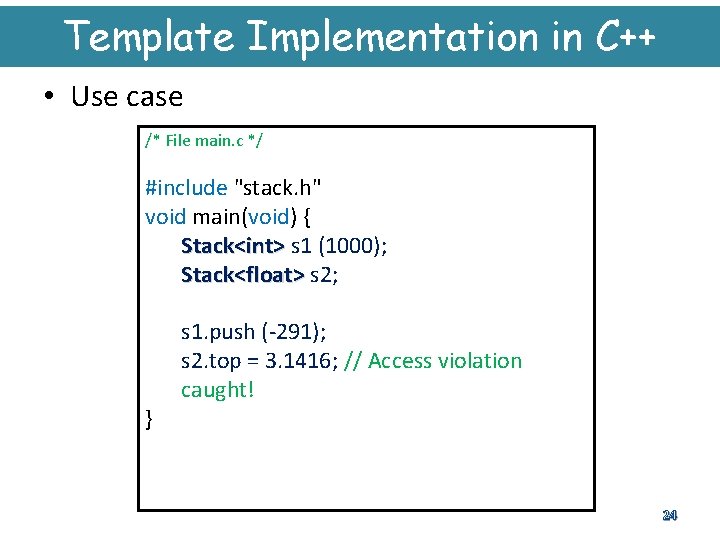
- Slides: 24
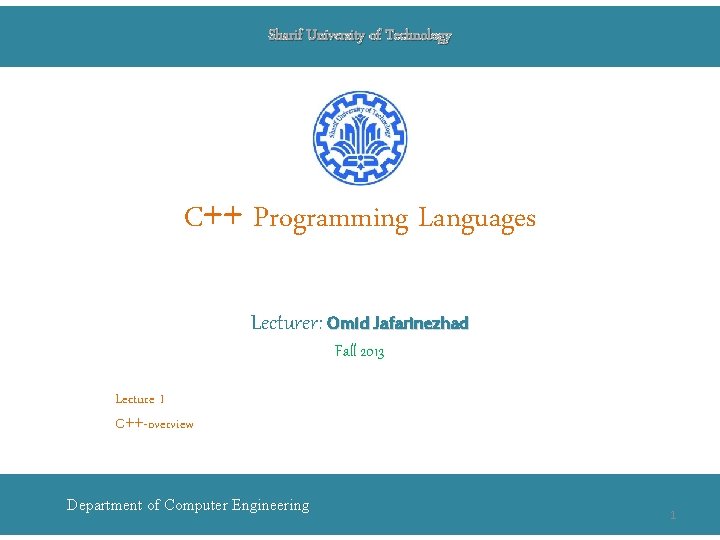
Sharif University of Technology C++ Programming Languages Lecturer: Omid Jafarinezhad Fall 2013 Lecture 1 C++-overview Department of Computer Engineering 1
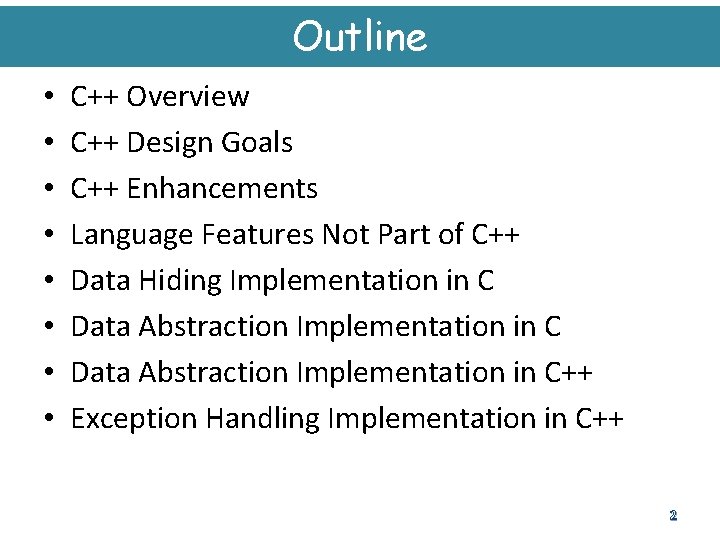
Outline • • C++ Overview C++ Design Goals C++ Enhancements Language Features Not Part of C++ Data Hiding Implementation in C Data Abstraction Implementation in C++ Exception Handling Implementation in C++ 2
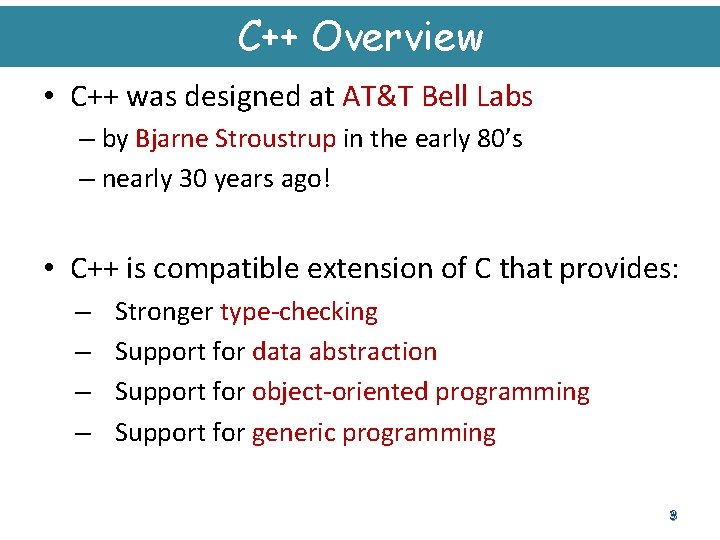
C++ Overview • C++ was designed at AT&T Bell Labs – by Bjarne Stroustrup in the early 80’s – nearly 30 years ago! • C++ is compatible extension of C that provides: – – Stronger type-checking Support for data abstraction Support for object-oriented programming Support for generic programming 3
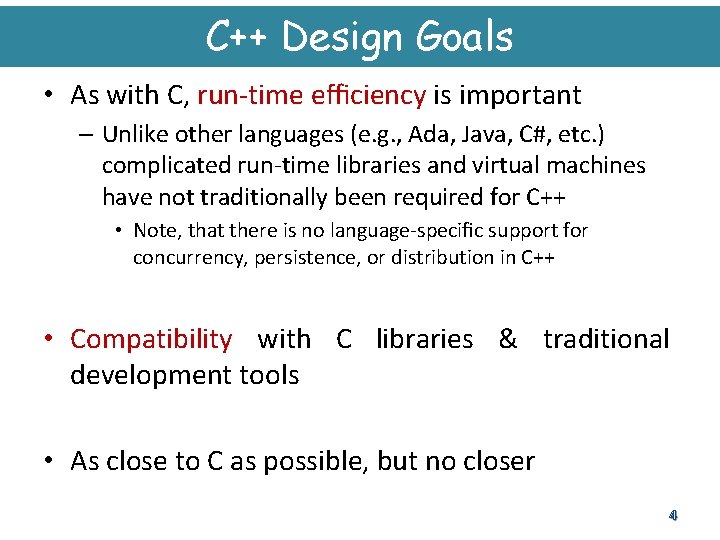
C++ Design Goals • As with C, run-time efficiency is important – Unlike other languages (e. g. , Ada, Java, C#, etc. ) complicated run-time libraries and virtual machines have not traditionally been required for C++ • Note, that there is no language-specific support for concurrency, persistence, or distribution in C++ • Compatibility with C libraries & traditional development tools • As close to C as possible, but no closer 4
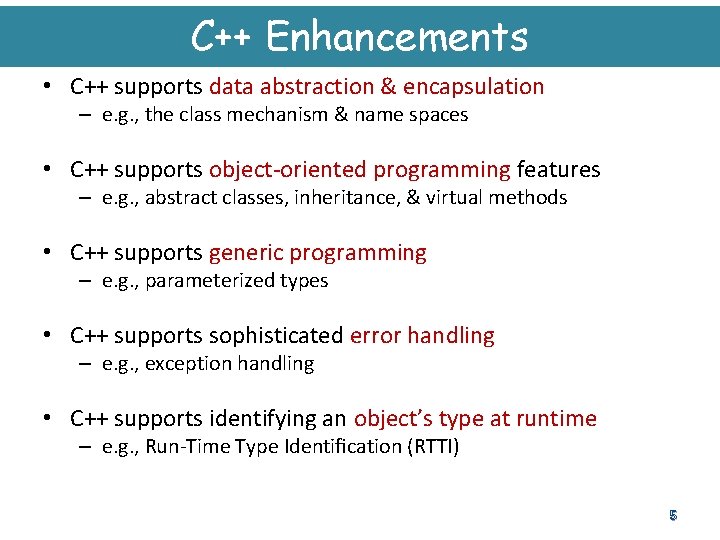
C++ Enhancements • C++ supports data abstraction & encapsulation – e. g. , the class mechanism & name spaces • C++ supports object-oriented programming features – e. g. , abstract classes, inheritance, & virtual methods • C++ supports generic programming – e. g. , parameterized types • C++ supports sophisticated error handling – e. g. , exception handling • C++ supports identifying an object’s type at runtime – e. g. , Run-Time Type Identification (RTTI) 5
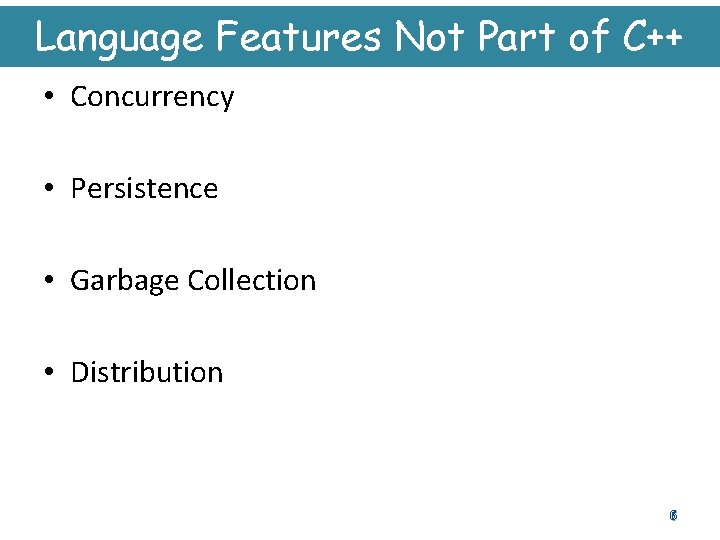
Language Features Not Part of C++ • Concurrency • Persistence • Garbage Collection • Distribution 6
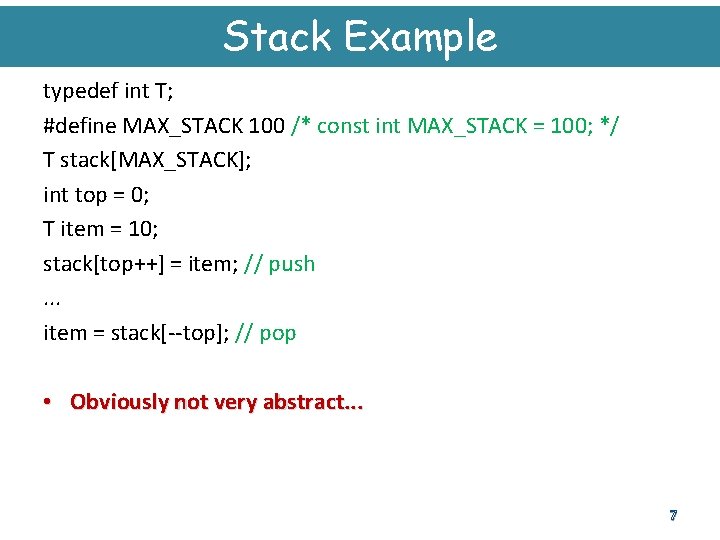
Stack Example typedef int T; #define MAX_STACK 100 /* const int MAX_STACK = 100; */ T stack[MAX_STACK]; int top = 0; T item = 10; stack[top++] = item; // push. . . item = stack[--top]; // pop • Obviously not very abstract. . . 7
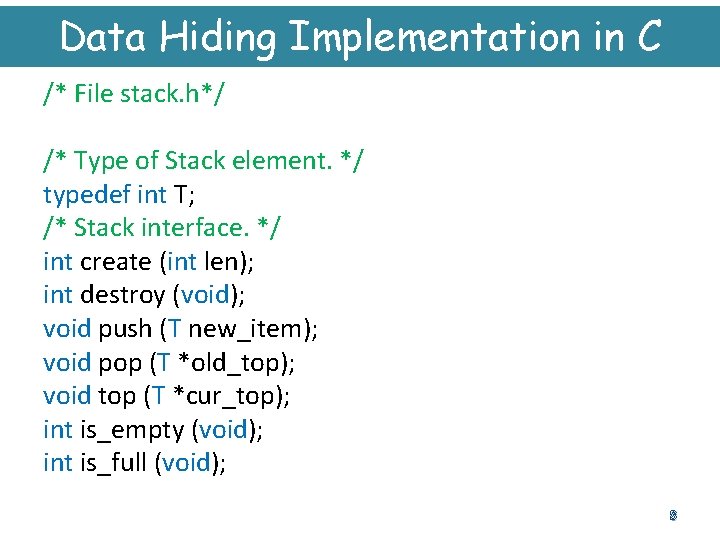
Data Hiding Implementation in C /* File stack. h*/ /* Type of Stack element. */ typedef int T; /* Stack interface. */ int create (int len); int destroy (void); void push (T new_item); void pop (T *old_top); void top (T *cur_top); int is_empty (void); int is_full (void); 8
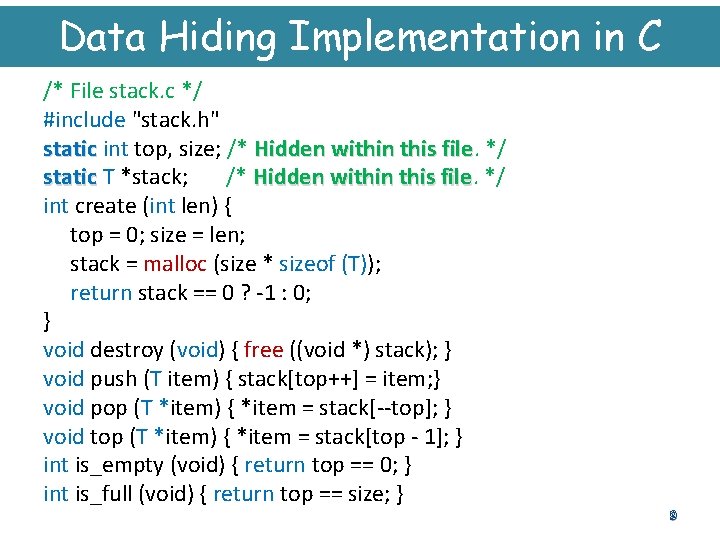
Data Hiding Implementation in C /* File stack. c */ #include "stack. h" static int top, size; /* Hidden within this file */ static T *stack; /* Hidden within this file */ int create (int len) { top = 0; size = len; stack = malloc (size * sizeof (T)); return stack == 0 ? -1 : 0; } void destroy (void) { free ((void *) stack); } void push (T item) { stack[top++] = item; } void pop (T *item) { *item = stack[--top]; } void top (T *item) { *item = stack[top - 1]; } int is_empty (void) { return top == 0; } int is_full (void) { return top == size; } 9
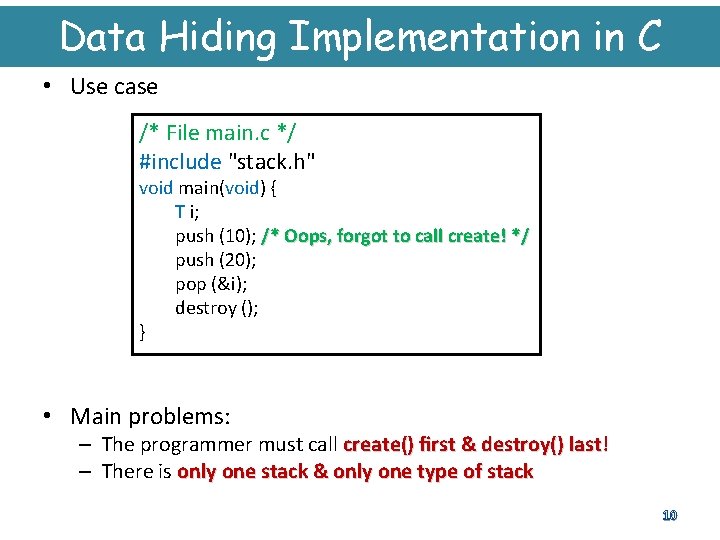
Data Hiding Implementation in C • Use case /* File main. c */ #include "stack. h" void main(void) { T i; push (10); /* Oops, forgot to call create! */ push (20); pop (&i); destroy (); } • Main problems: – The programmer must call create() first & destroy() last! – There is only one stack & only one type of stack 10
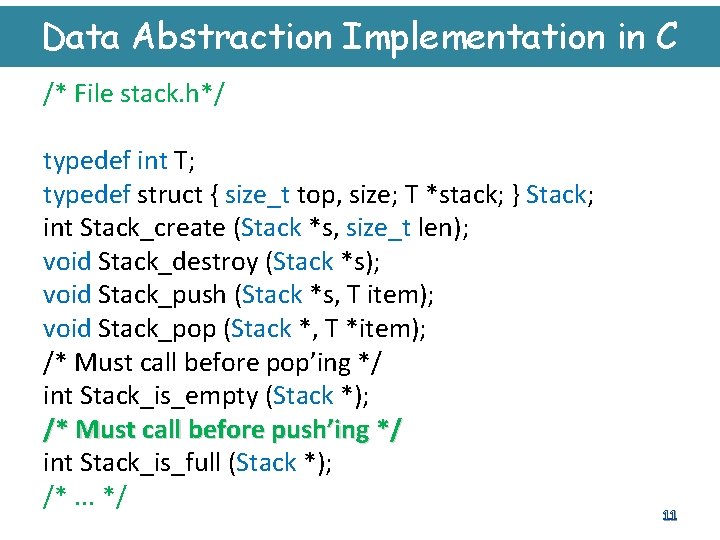
Data Abstraction Implementation in C /* File stack. h*/ typedef int T; typedef struct { size_t top, size; T *stack; } Stack; int Stack_create (Stack *s, size_t len); void Stack_destroy (Stack *s); void Stack_push (Stack *s, T item); void Stack_pop (Stack *, T *item); /* Must call before pop’ing */ int Stack_is_empty (Stack *); /* Must call before push’ing */ int Stack_is_full (Stack *); /*. . . */ 11
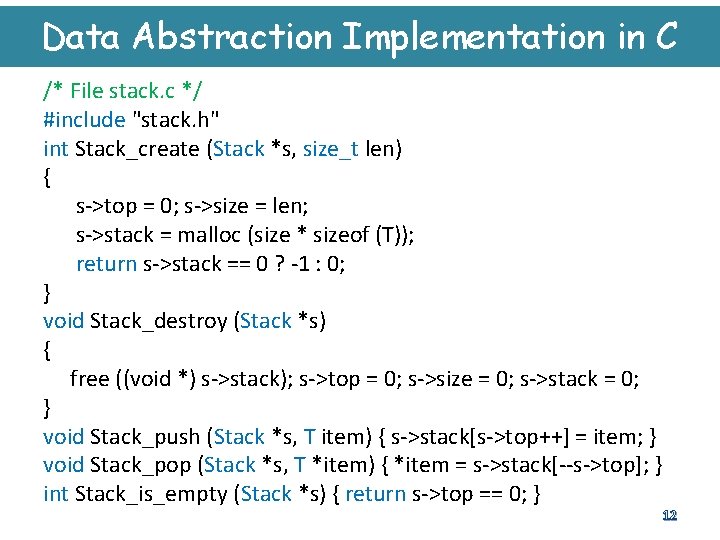
Data Abstraction Implementation in C /* File stack. c */ #include "stack. h" int Stack_create (Stack *s, size_t len) { s->top = 0; s->size = len; s->stack = malloc (size * sizeof (T)); return s->stack == 0 ? -1 : 0; } void Stack_destroy (Stack *s) { free ((void *) s->stack); s->top = 0; s->size = 0; s->stack = 0; } void Stack_push (Stack *s, T item) { s->stack[s->top++] = item; } void Stack_pop (Stack *s, T *item) { *item = s->stack[--s->top]; } int Stack_is_empty (Stack *s) { return s->top == 0; } 12
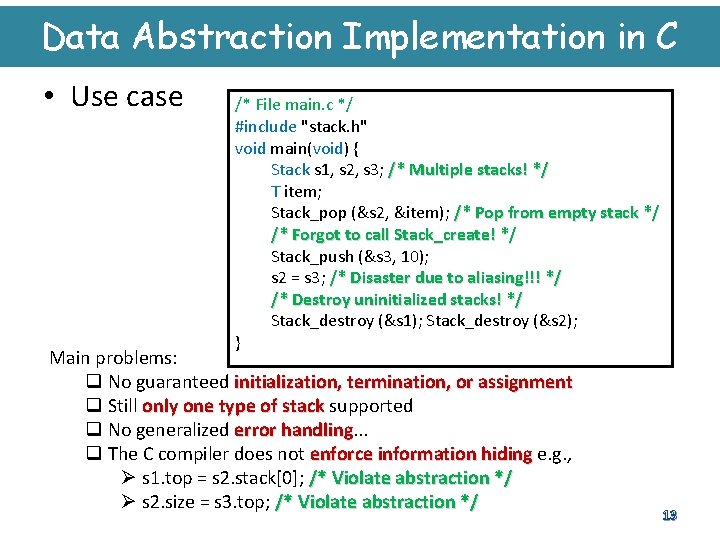
Data Abstraction Implementation in C • Use case /* File main. c */ #include "stack. h" void main(void) { Stack s 1, s 2, s 3; /* Multiple stacks! */ T item; Stack_pop (&s 2, &item); /* Pop from empty stack */ /* Forgot to call Stack_create! */ Stack_push (&s 3, 10); s 2 = s 3; /* Disaster due to aliasing!!! */ /* Destroy uninitialized stacks! */ Stack_destroy (&s 1); Stack_destroy (&s 2); } Main problems: q No guaranteed initialization, termination, or assignment q Still only one type of stack supported q No generalized error handling. . . handling q The C compiler does not enforce information hiding e. g. , Ø s 1. top = s 2. stack[0]; /* Violate abstraction */ Ø s 2. size = s 3. top; /* Violate abstraction */ 13
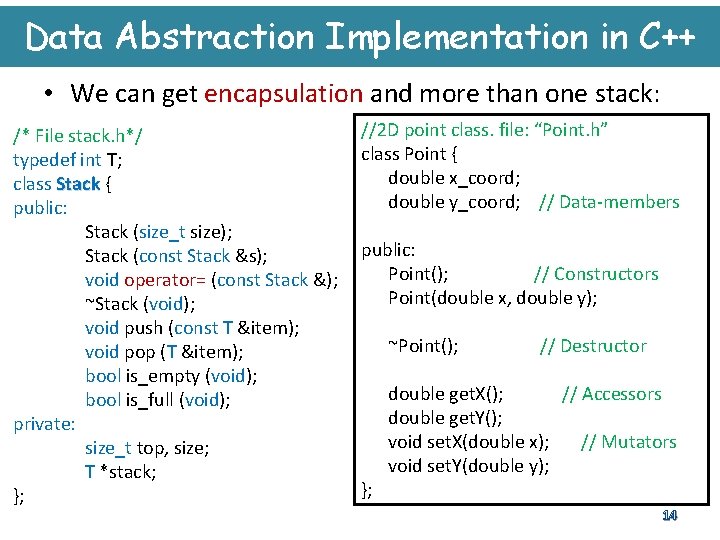
Data Abstraction Implementation in C++ • We can get encapsulation and more than one stack: /* File stack. h*/ typedef int T; class Stack { public: Stack (size_t size); Stack (const Stack &s); void operator= (const Stack &); ~Stack (void); void push (const T &item); void pop (T &item); bool is_empty (void); bool is_full (void); private: size_t top, size; T *stack; }; //2 D point class. file: “Point. h” class Point { double x_coord; double y_coord; // Data-members public: Point(); // Constructors Point(double x, double y); ~Point(); }; // Destructor double get. X(); // Accessors double get. Y(); void set. X(double x); // Mutators void set. Y(double y); 14
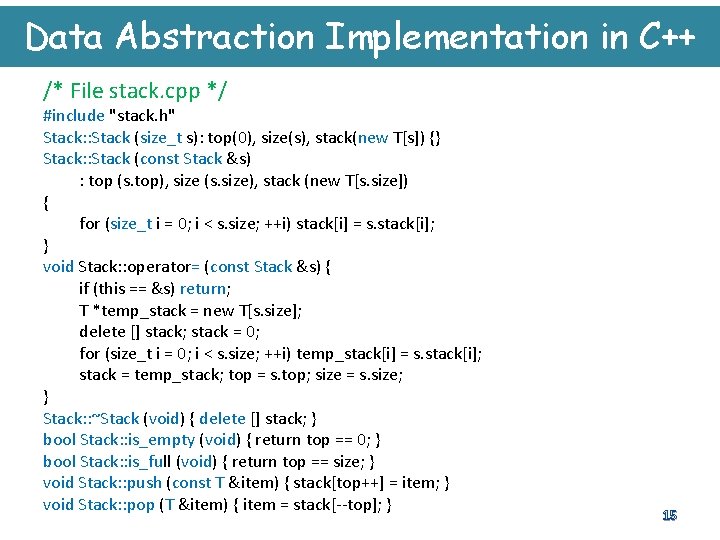
Data Abstraction Implementation in C++ /* File stack. cpp */ #include "stack. h" Stack: : Stack (size_t s): top(0), size(s), stack(new T[s]) {} Stack: : Stack (const Stack &s) : top (s. top), size (s. size), stack (new T[s. size]) { for (size_t i = 0; i < s. size; ++i) stack[i] = s. stack[i]; } void Stack: : operator= (const Stack &s) { if (this == &s) return; T *temp_stack = new T[s. size]; delete [] stack; stack = 0; for (size_t i = 0; i < s. size; ++i) temp_stack[i] = s. stack[i]; stack = temp_stack; top = s. top; size = s. size; } Stack: : ~Stack (void) { delete [] stack; } bool Stack: : is_empty (void) { return top == 0; } bool Stack: : is_full (void) { return top == size; } void Stack: : push (const T &item) { stack[top++] = item; } void Stack: : pop (T &item) { item = stack[--top]; } 15
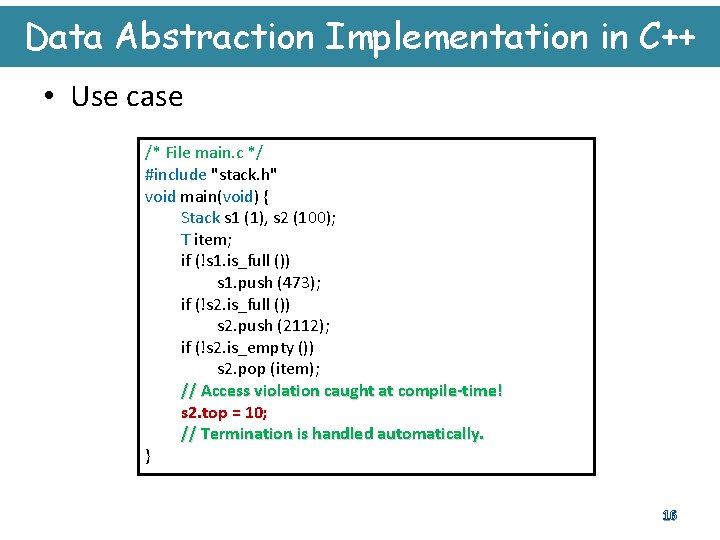
Data Abstraction Implementation in C++ • Use case /* File main. c */ #include "stack. h" void main(void) { Stack s 1 (1), s 2 (100); T item; if (!s 1. is_full ()) s 1. push (473); if (!s 2. is_full ()) s 2. push (2112); if (!s 2. is_empty ()) s 2. pop (item); // Access violation caught at compile-time! s 2. top = 10; // Termination is handled automatically. } 16
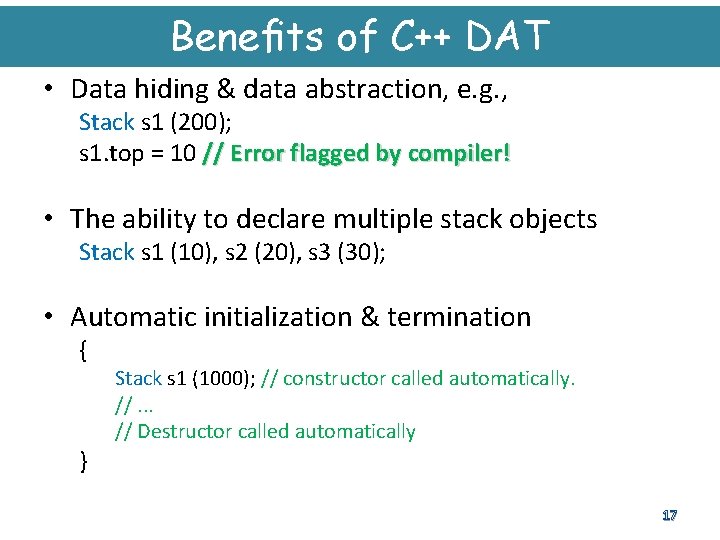
Benefits of C++ DAT • Data hiding & data abstraction, e. g. , Stack s 1 (200); s 1. top = 10 // Error flagged by compiler! • The ability to declare multiple stack objects Stack s 1 (10), s 2 (20), s 3 (30); • Automatic initialization & termination { } Stack s 1 (1000); // constructor called automatically. //. . . // Destructor called automatically 17
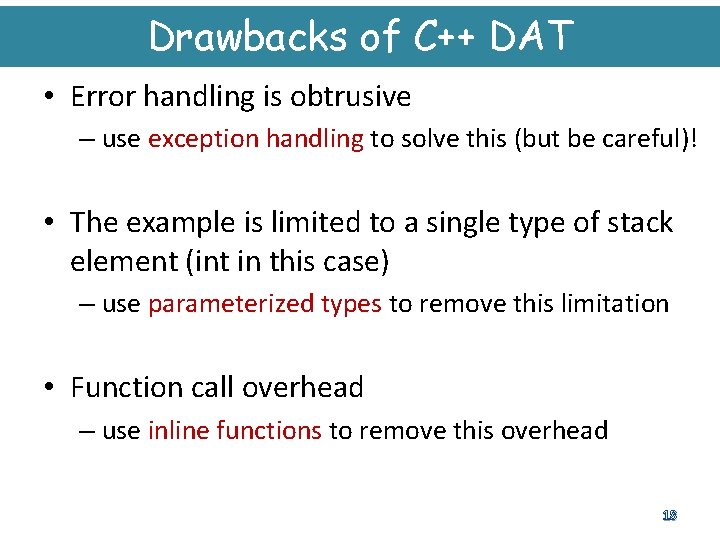
Drawbacks of C++ DAT • Error handling is obtrusive – use exception handling to solve this (but be careful)! • The example is limited to a single type of stack element (int in this case) – use parameterized types to remove this limitation • Function call overhead – use inline functions to remove this overhead 18
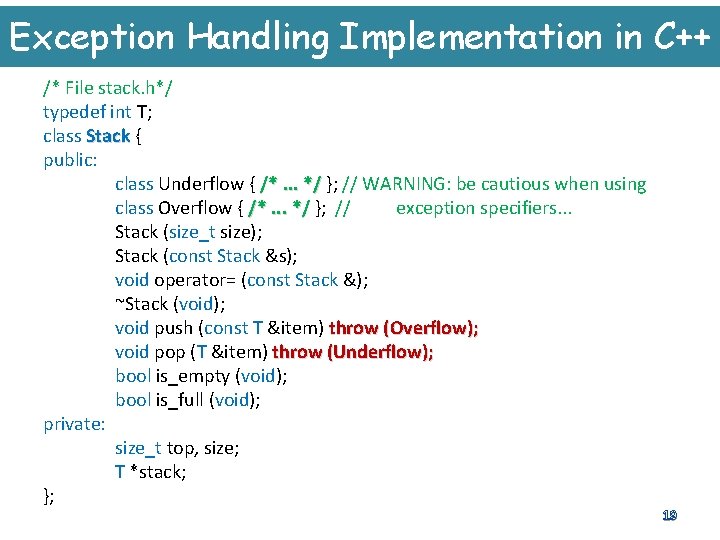
Exception Handling Implementation in C++ /* File stack. h*/ typedef int T; class Stack { public: class Underflow { /*. . . */ }; // WARNING: be cautious when using class Overflow { /*. . . */ }; // exception specifiers. . . Stack (size_t size); Stack (const Stack &s); void operator= (const Stack &); ~Stack (void); void push (const T &item) throw (Overflow); void pop (T &item) throw (Underflow); bool is_empty (void); bool is_full (void); private: size_t top, size; T *stack; }; 19
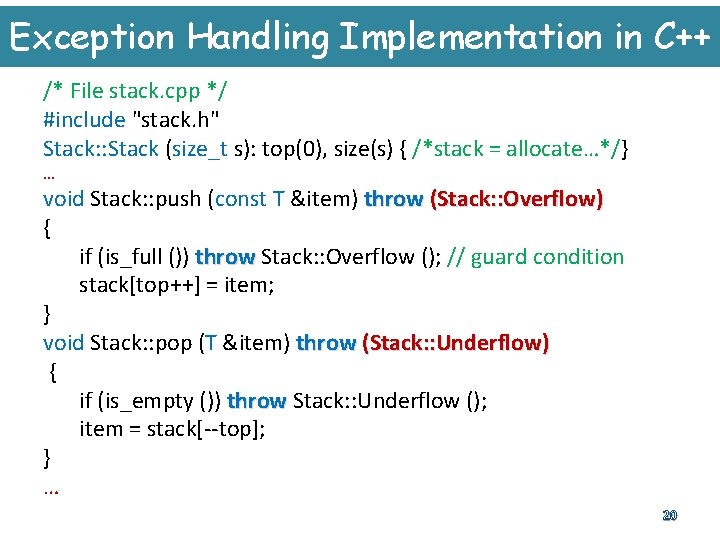
Exception Handling Implementation in C++ /* File stack. cpp */ #include "stack. h" Stack: : Stack (size_t s): top(0), size(s) { /*stack = allocate…*/} … void Stack: : push (const T &item) throw (Stack: : Overflow) { if (is_full ()) throw Stack: : Overflow (); // guard condition stack[top++] = item; } void Stack: : pop (T &item) throw (Stack: : Underflow) { if (is_empty ()) throw Stack: : Underflow (); item = stack[--top]; } … 20
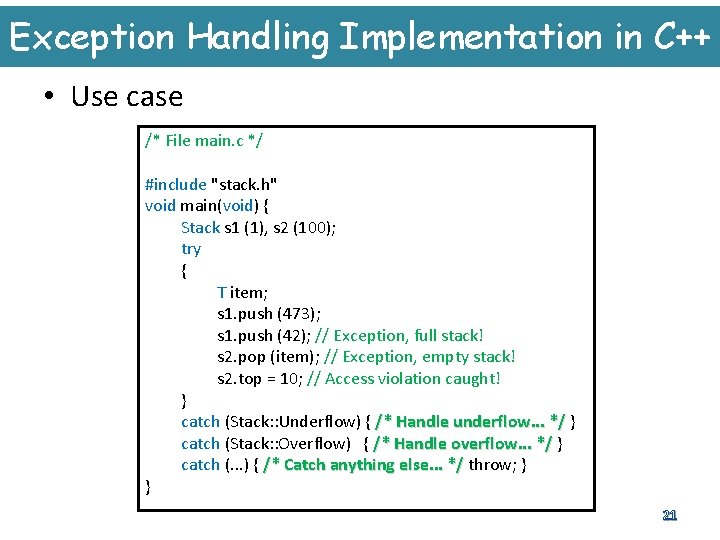
Exception Handling Implementation in C++ • Use case /* File main. c */ #include "stack. h" void main(void) { Stack s 1 (1), s 2 (100); try { T item; s 1. push (473); s 1. push (42); // Exception, full stack! s 2. pop (item); // Exception, empty stack! s 2. top = 10; // Access violation caught! } catch (Stack: : Underflow) { /* Handle underflow. . . */ } catch (Stack: : Overflow) { /* Handle overflow. . . */ } catch (. . . ) { /* Catch anything else. . . */ throw; } } 21
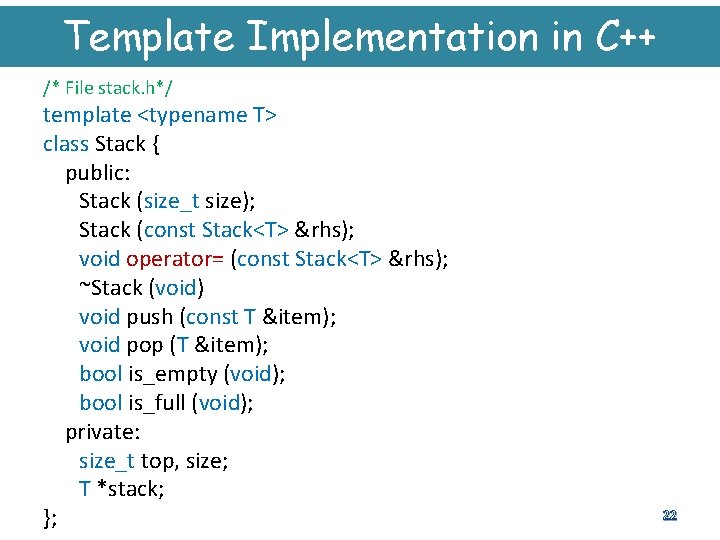
Template Implementation in C++ /* File stack. h*/ template <typename T> class Stack { public: Stack (size_t size); Stack (const Stack<T> &rhs); void operator= (const Stack<T> &rhs); ~Stack (void) void push (const T &item); void pop (T &item); bool is_empty (void); bool is_full (void); private: size_t top, size; T *stack; }; 22
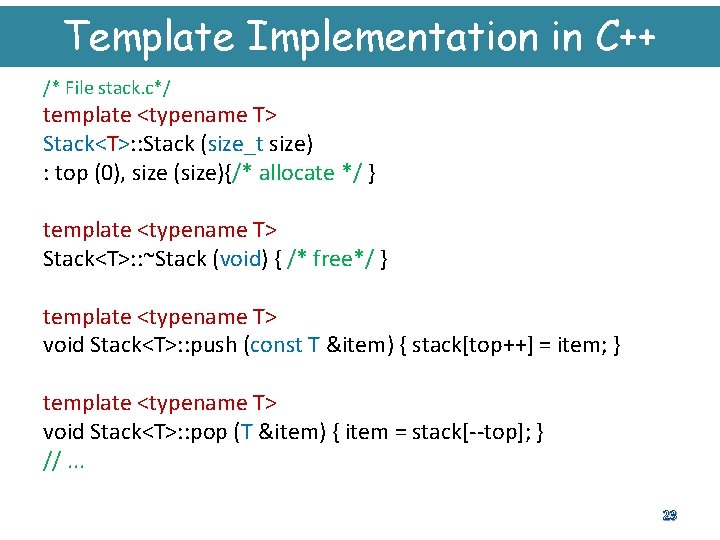
Template Implementation in C++ /* File stack. c*/ template <typename T> Stack<T>: : Stack (size_t size) : top (0), size (size){/* allocate */ } template <typename T> Stack<T>: : ~Stack (void) { /* free*/ } template <typename T> void Stack<T>: : push (const T &item) { stack[top++] = item; } template <typename T> void Stack<T>: : pop (T &item) { item = stack[--top]; } //. . . 23
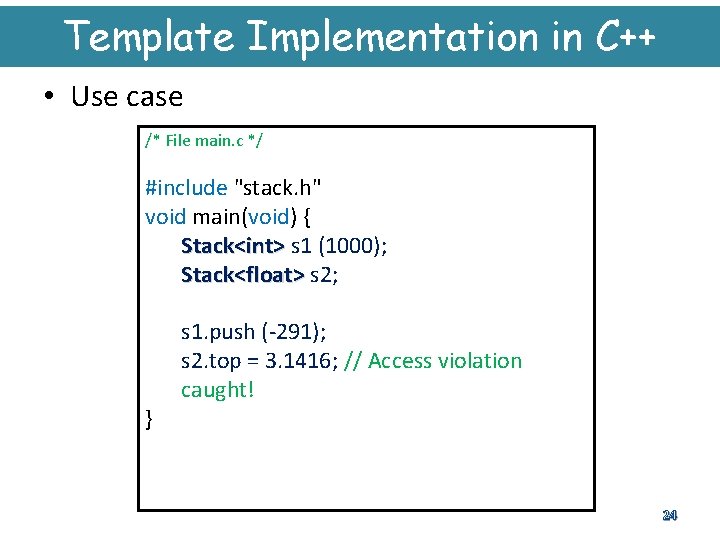
Template Implementation in C++ • Use case /* File main. c */ #include "stack. h" void main(void) { Stack<int> s 1 (1000); Stack<float> s 2; } s 1. push (-291); s 2. top = 3. 1416; // Access violation caught! 24
Lecturer's name
Sharif university of technology
Muhammad nawaz sharif university of agriculture
Physician associate lecturer
Spe distinguished lecturer
Good morning students
Photography lecturer
Lecturer in charge
Designation lecturer
Designation of lecturer
Why himalayan rivers are pernnial in nature
Lecturer name
Pearson lecturer resources
Spe distinguished lecturer
Lector vs lecturer
Lecturer in charge
Cfa lecturer handbook
Lecturer asad ali
Real-time systems and programming languages
Cs 421 uiuc
Thread dalam java
Programming languages levels
Introduction to programming languages
Plc programming languages
Joey paquet