Sharif University of Technology C Programming Languages Lecturer
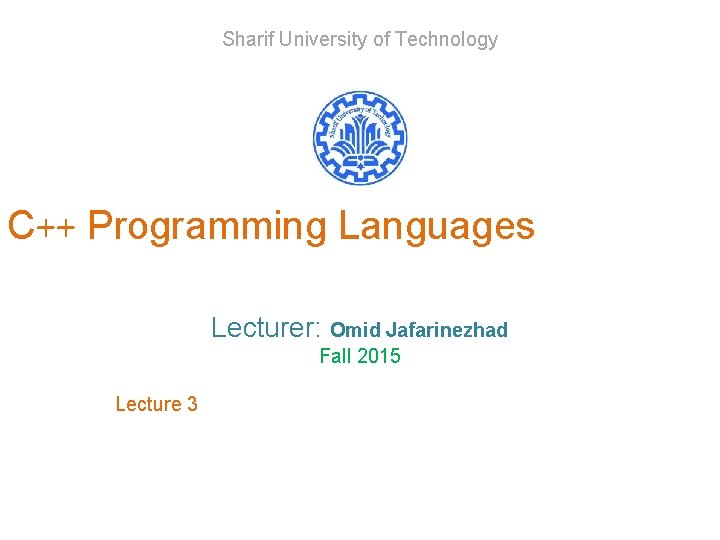
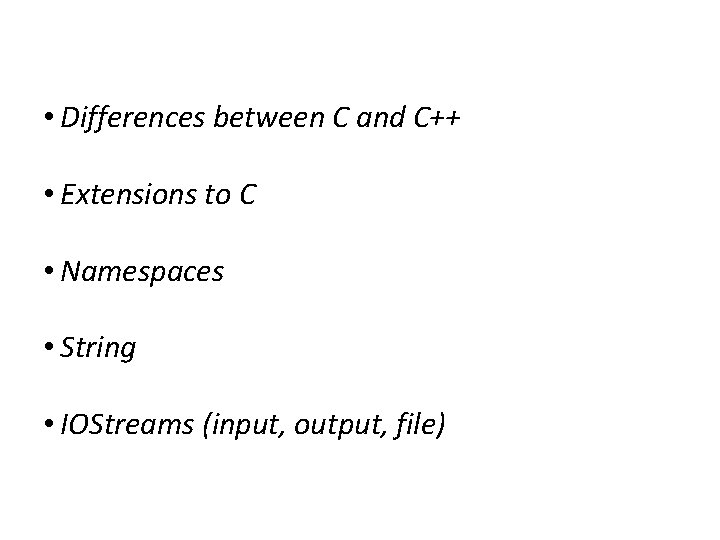
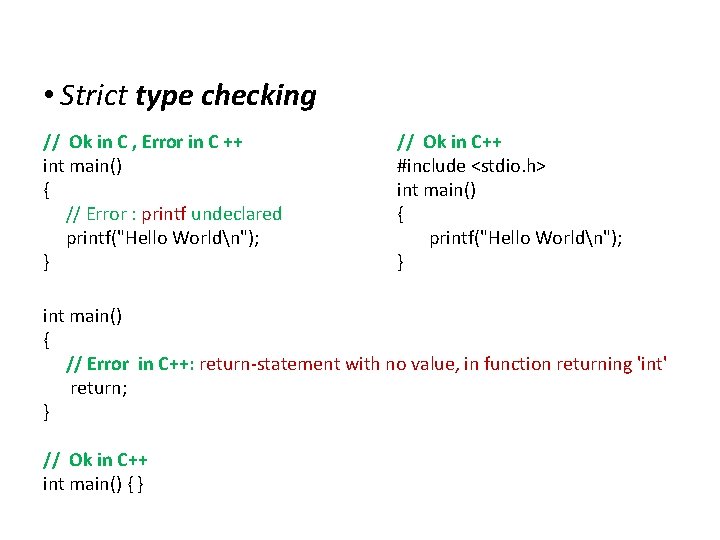
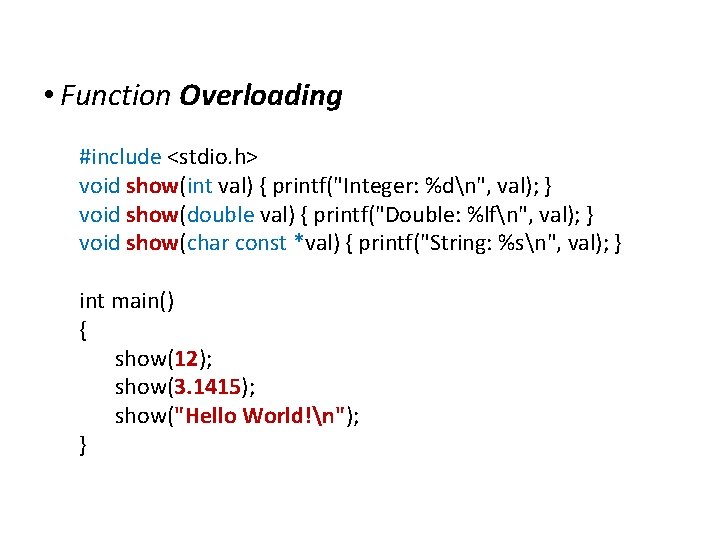
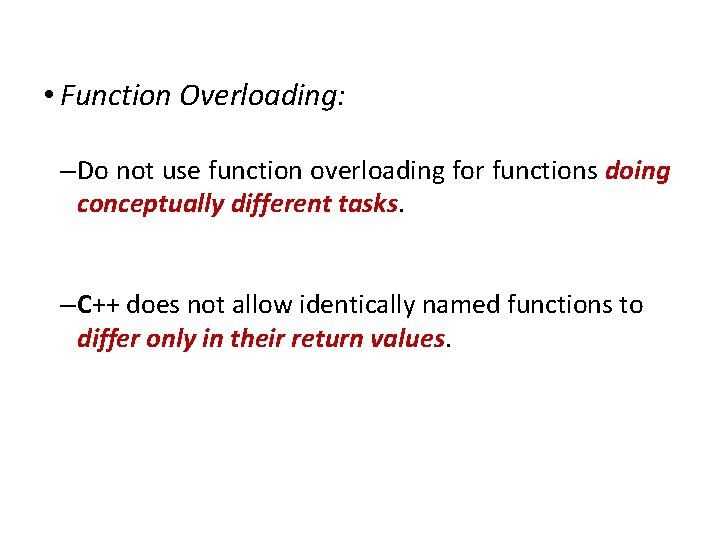
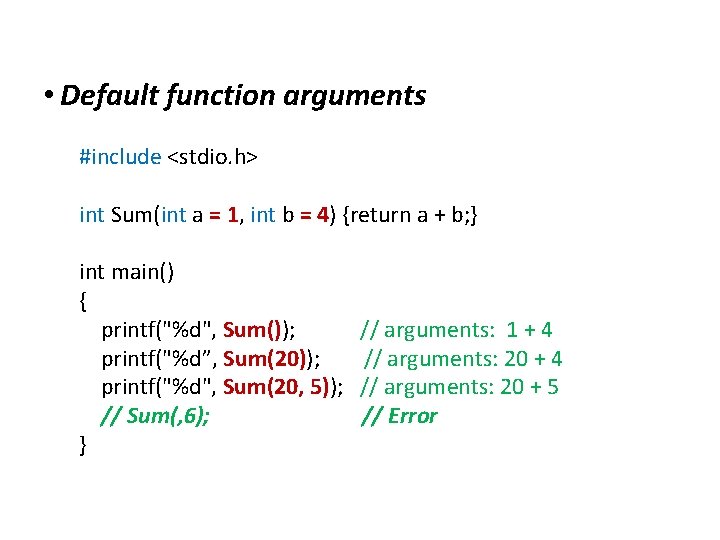
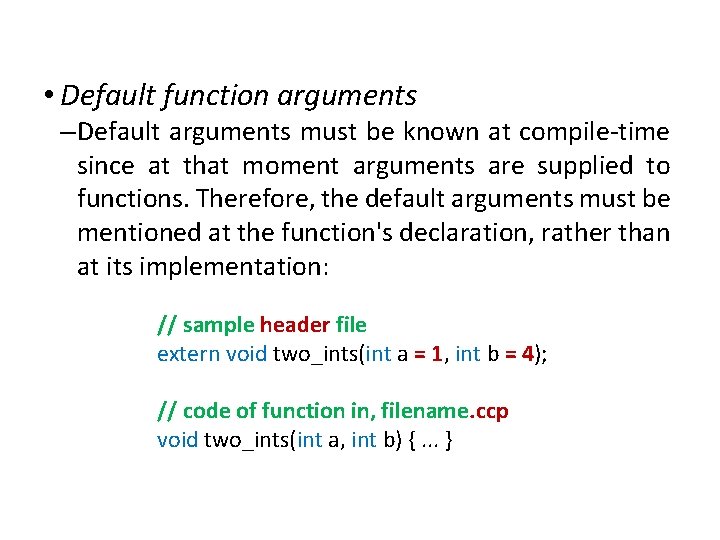
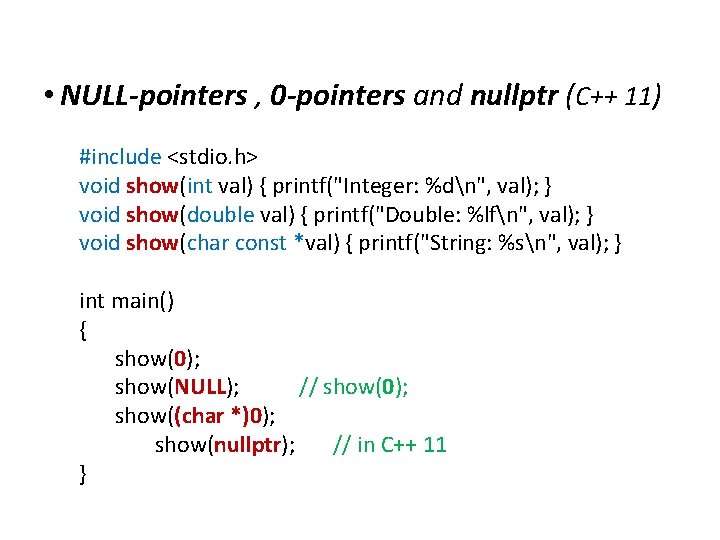
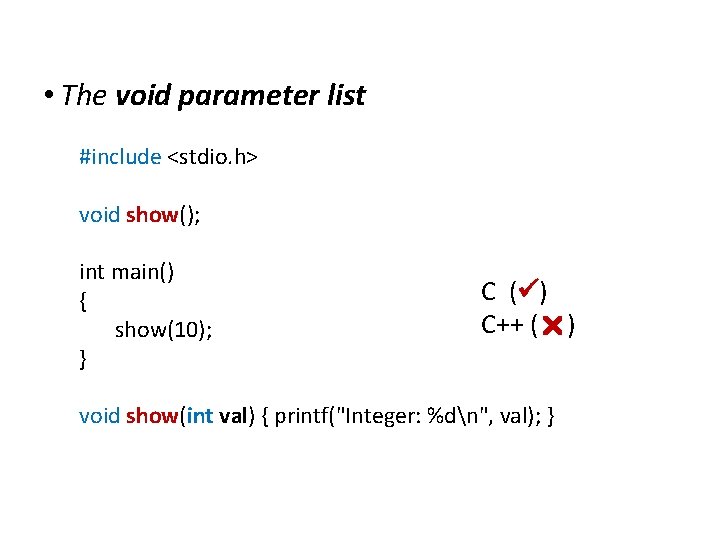
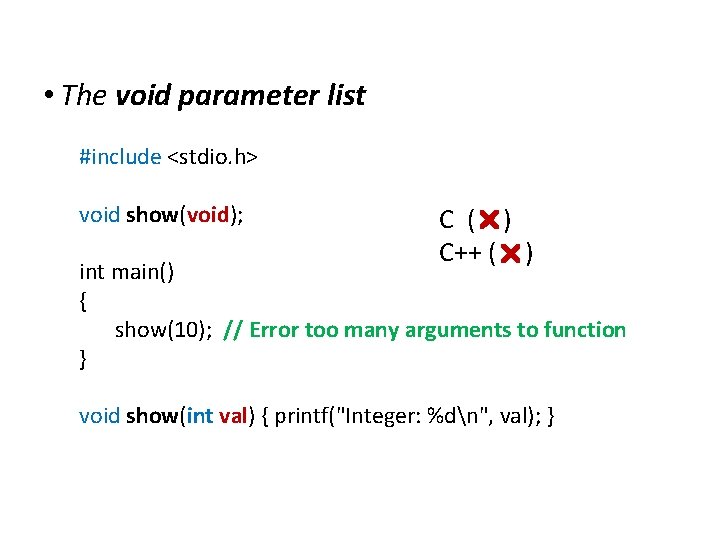
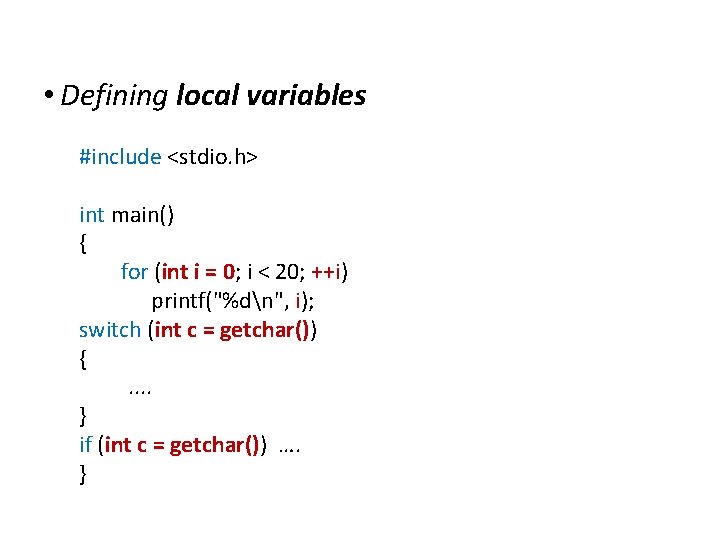
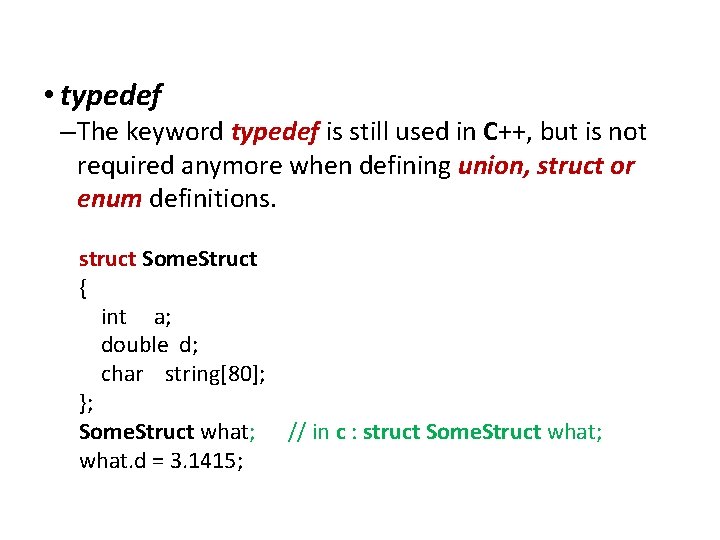
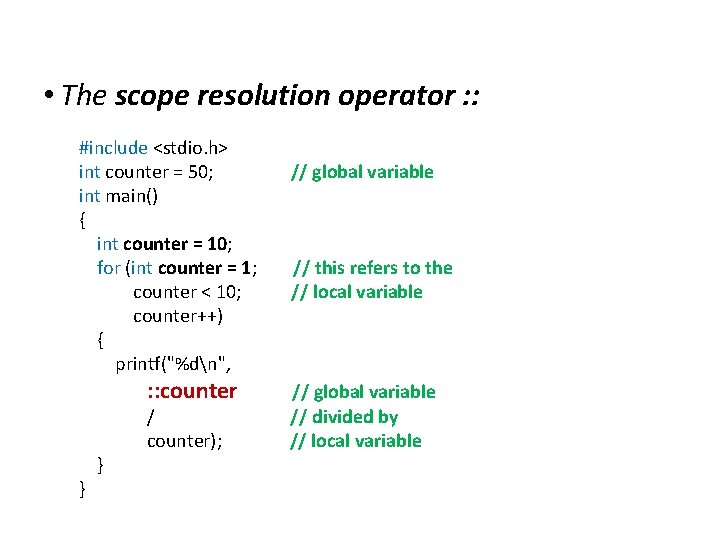
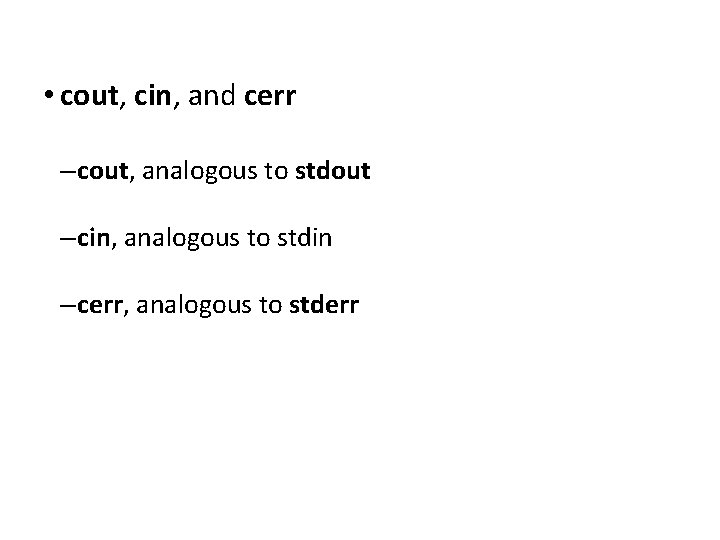
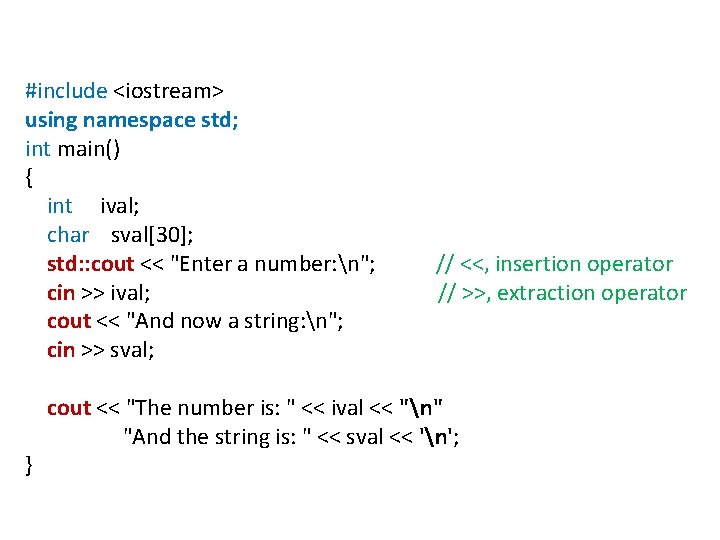
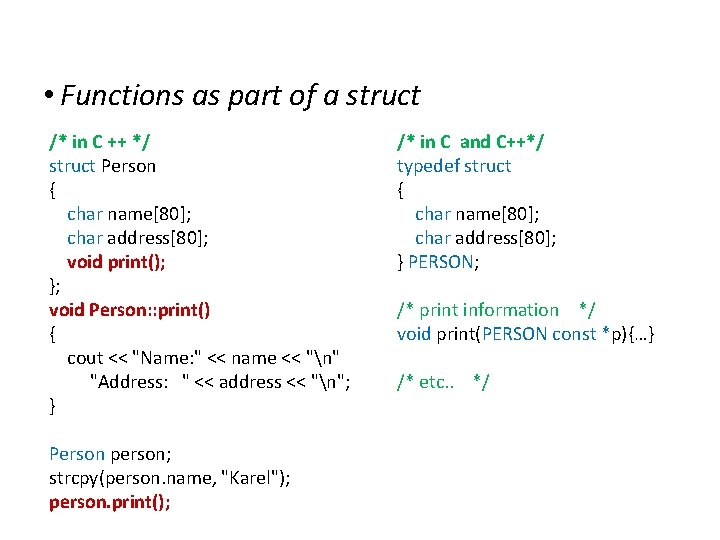
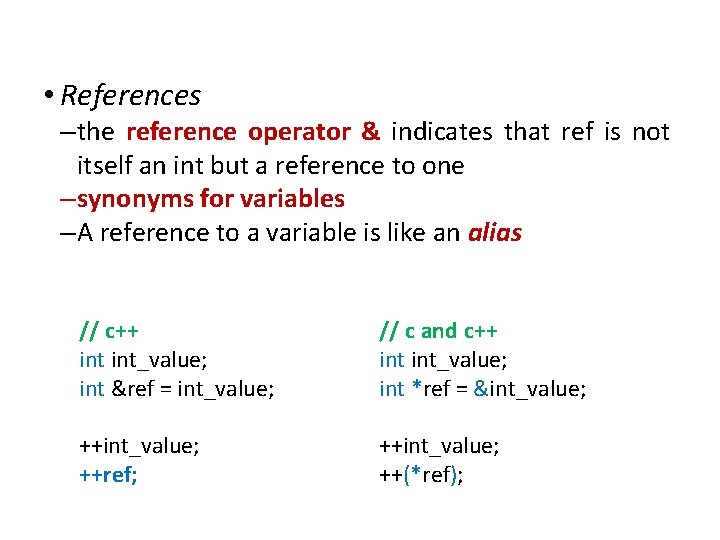
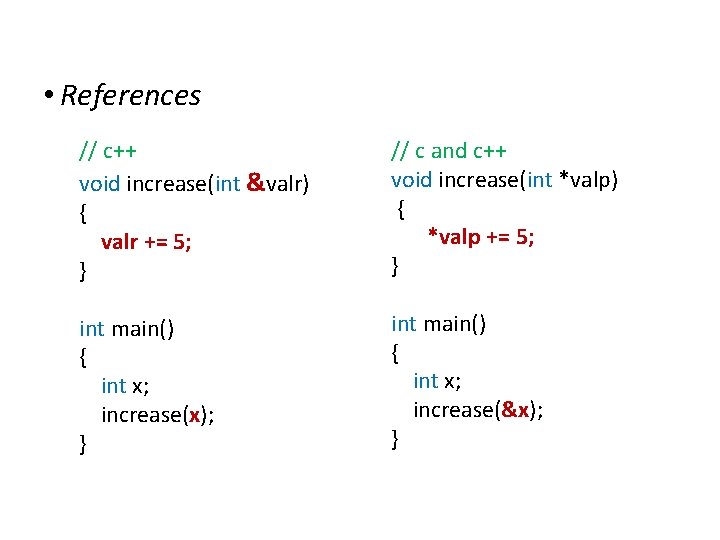
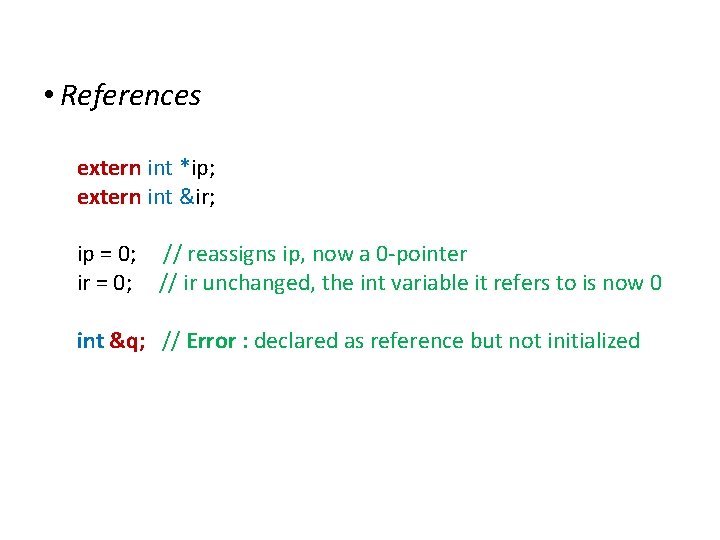
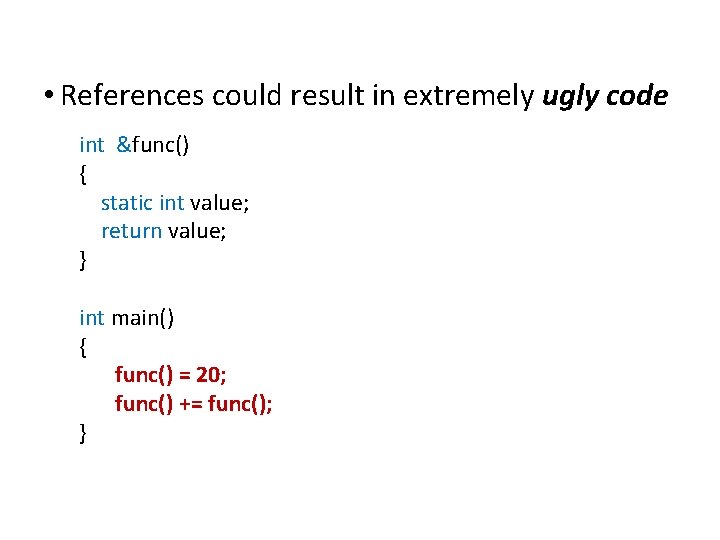
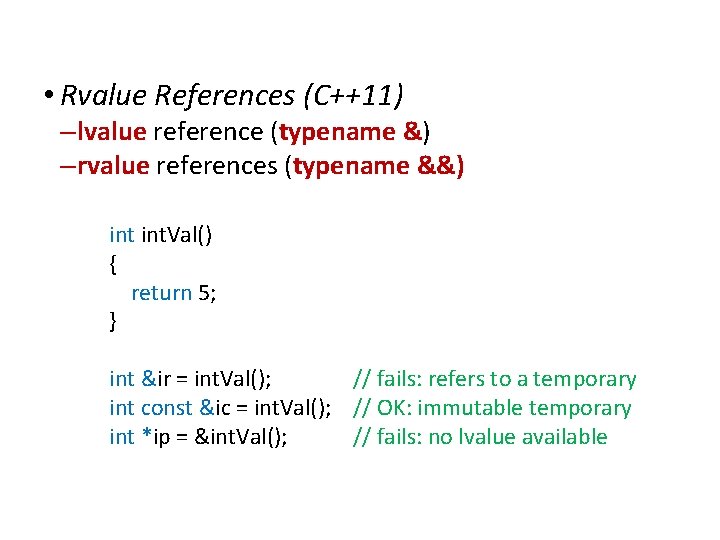
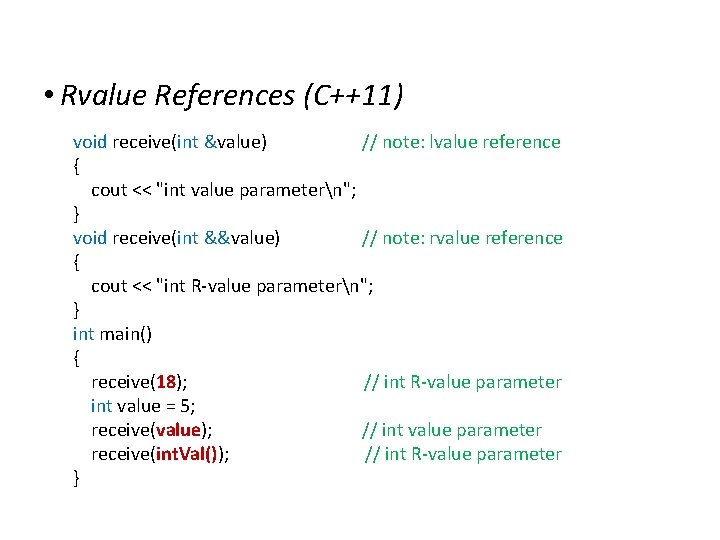
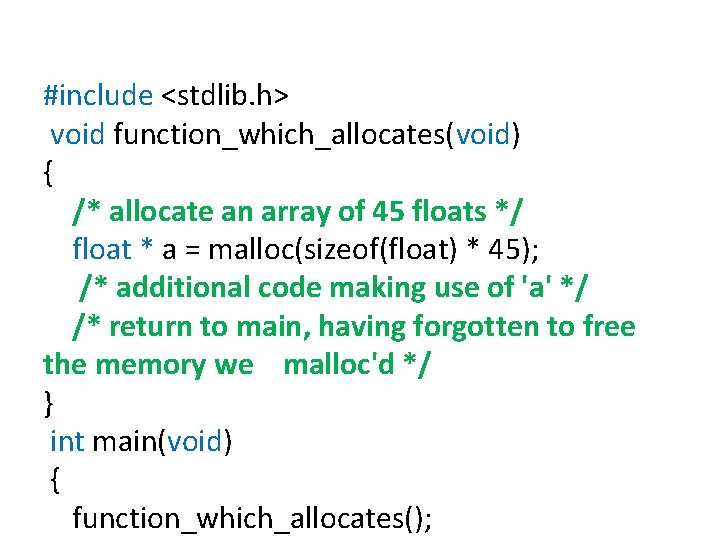
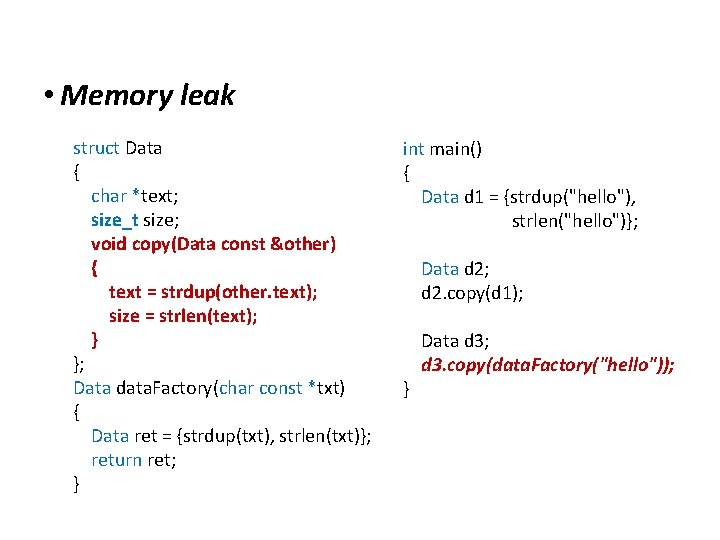
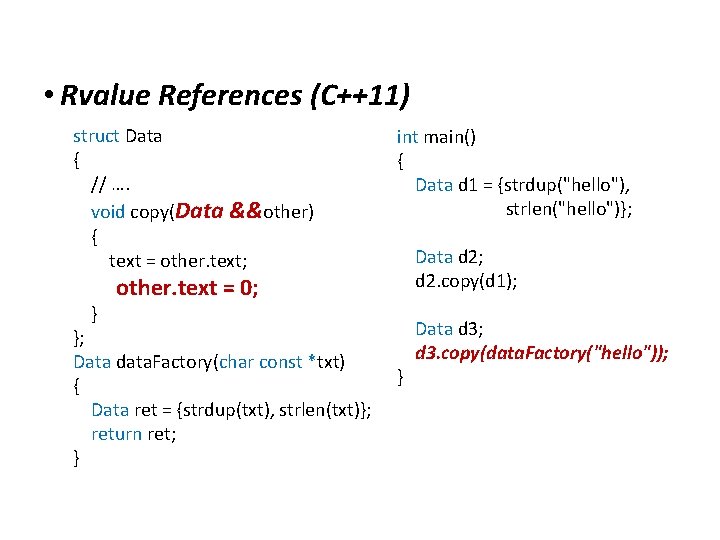
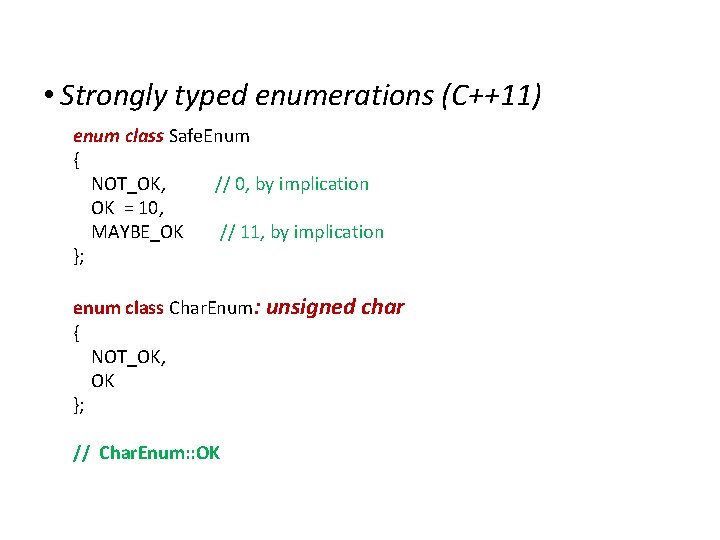
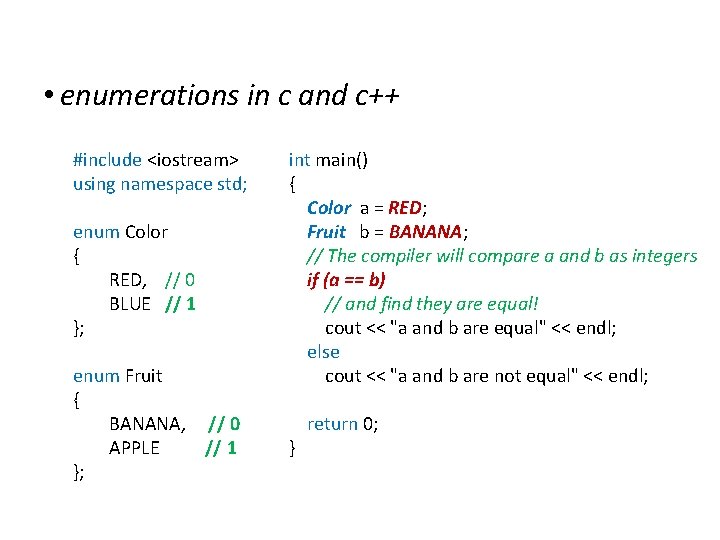
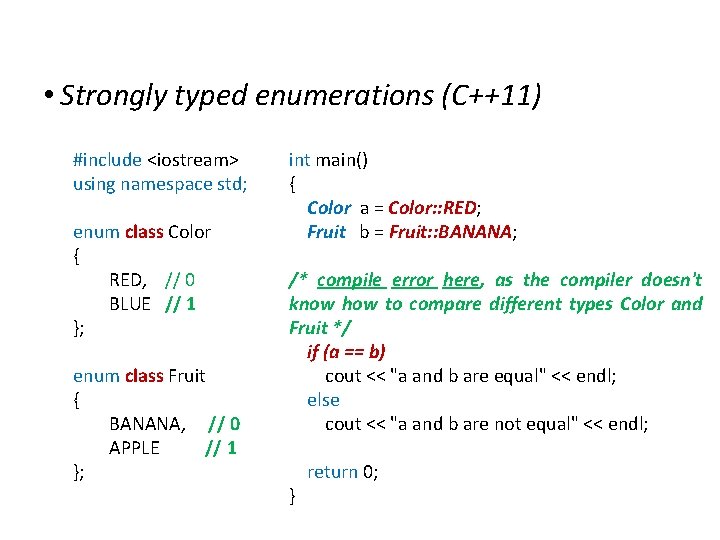
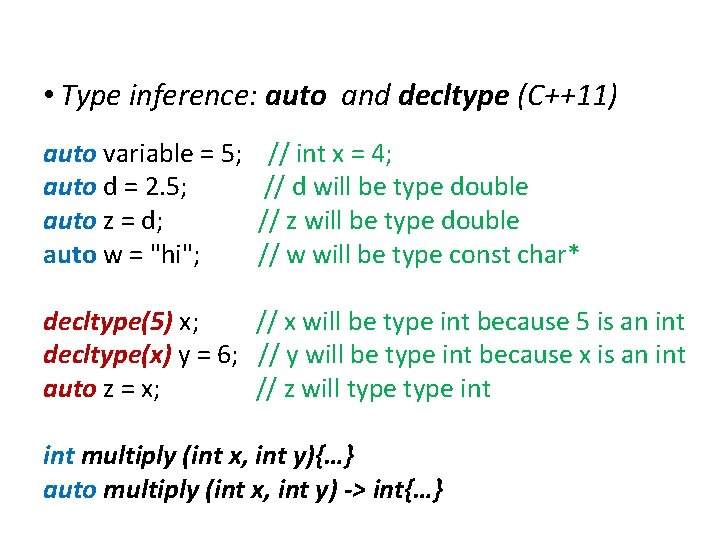
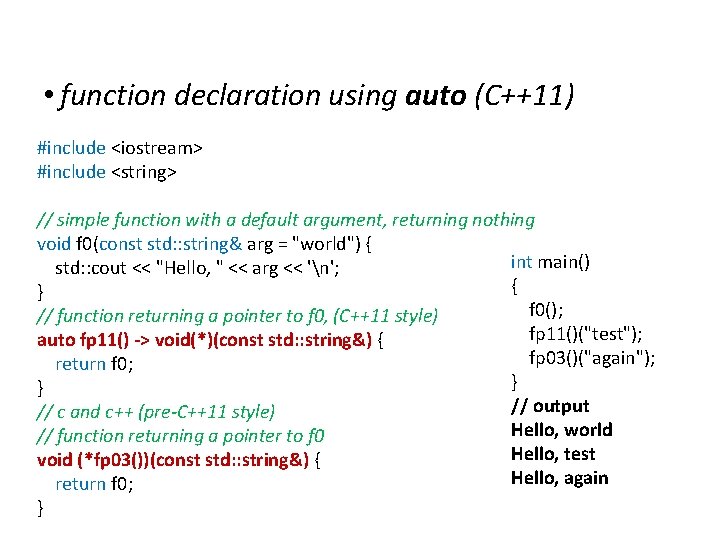
![Extensions to C • Range-based for-loops (C++11) struct Big. Struct { double array[100]; int Extensions to C • Range-based for-loops (C++11) struct Big. Struct { double array[100]; int](https://slidetodoc.com/presentation_image_h/9338e8fb76f47ecbf126ab6c6b08158c/image-31.jpg)
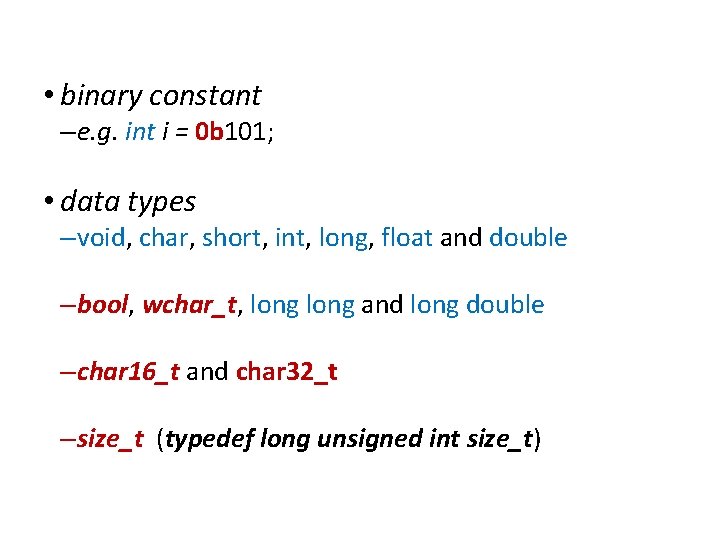
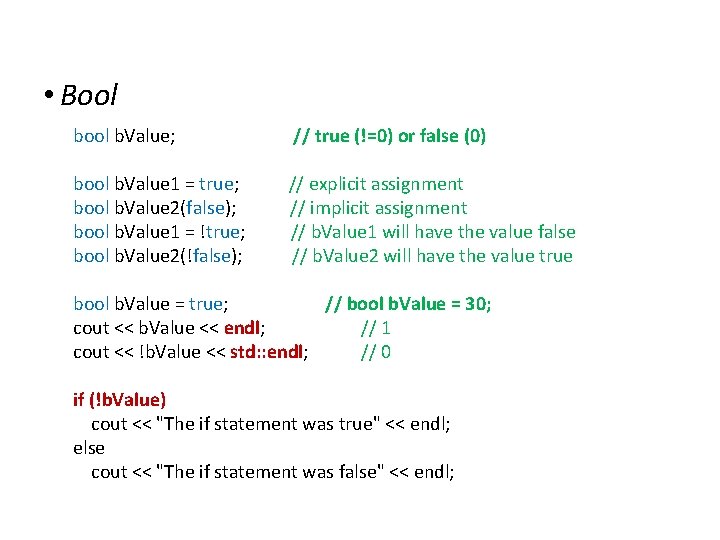
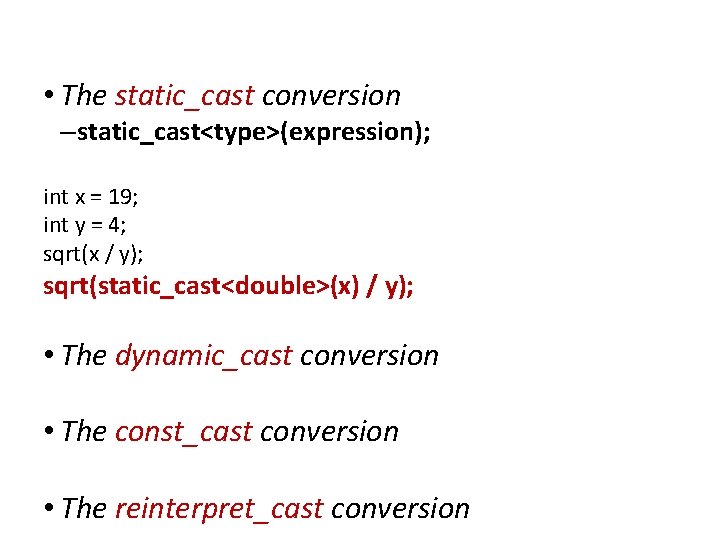
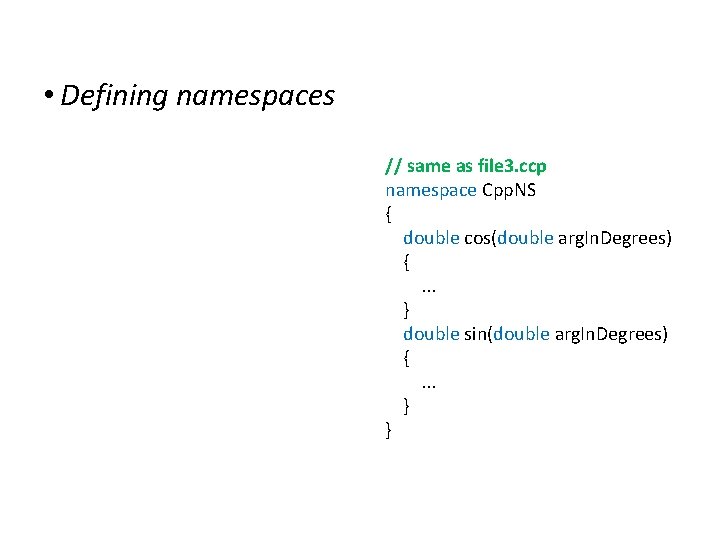
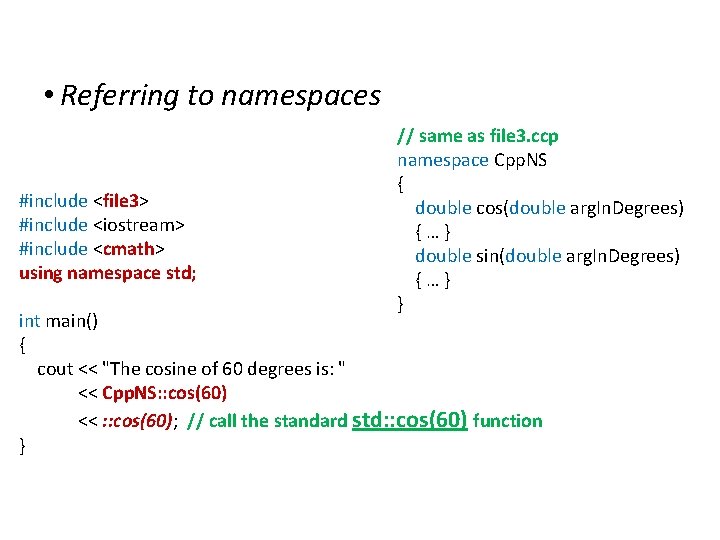
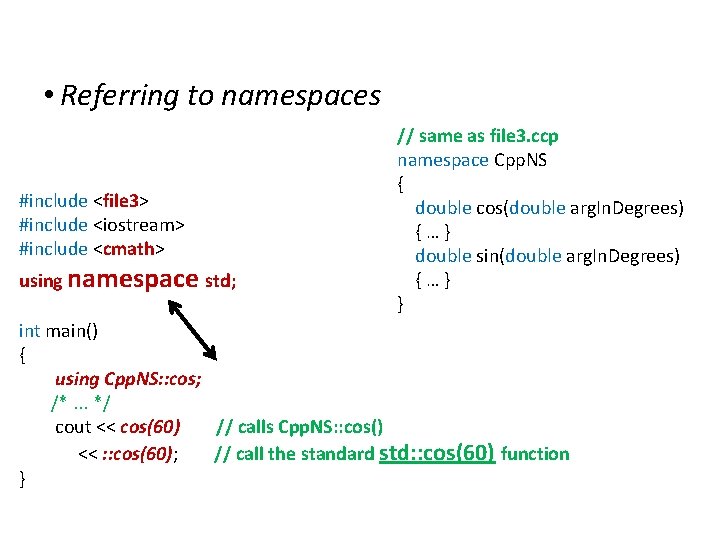
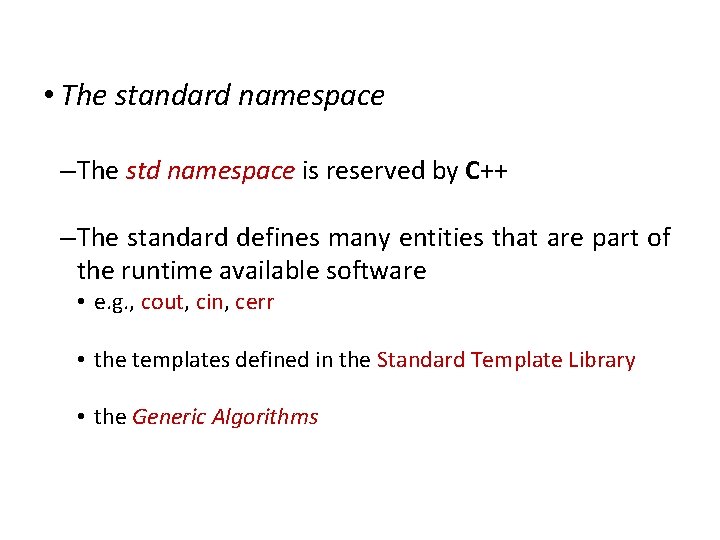
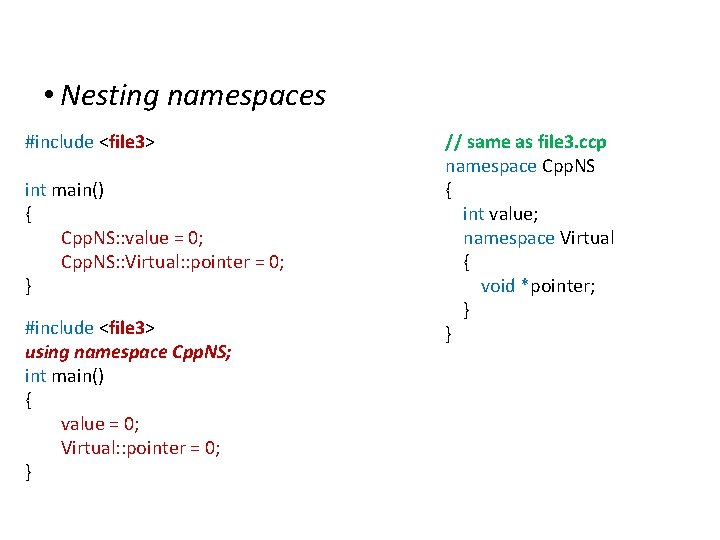
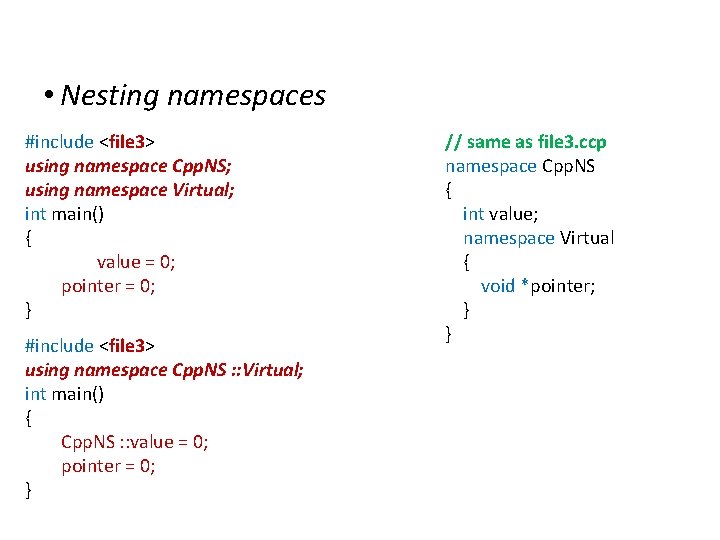
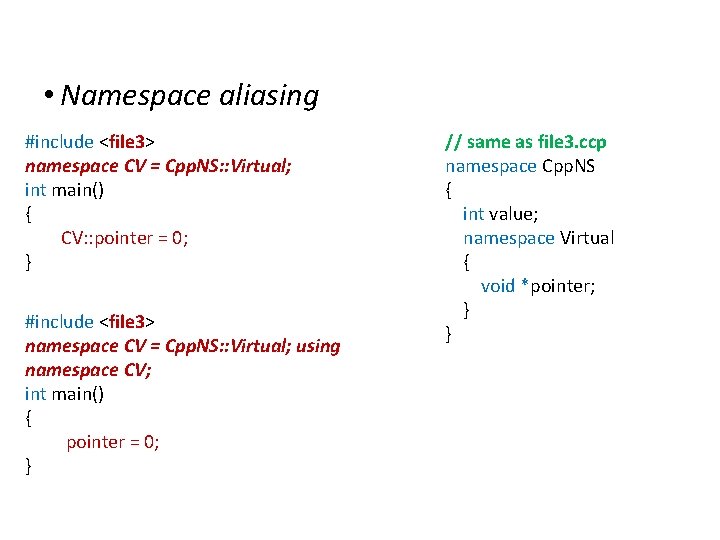
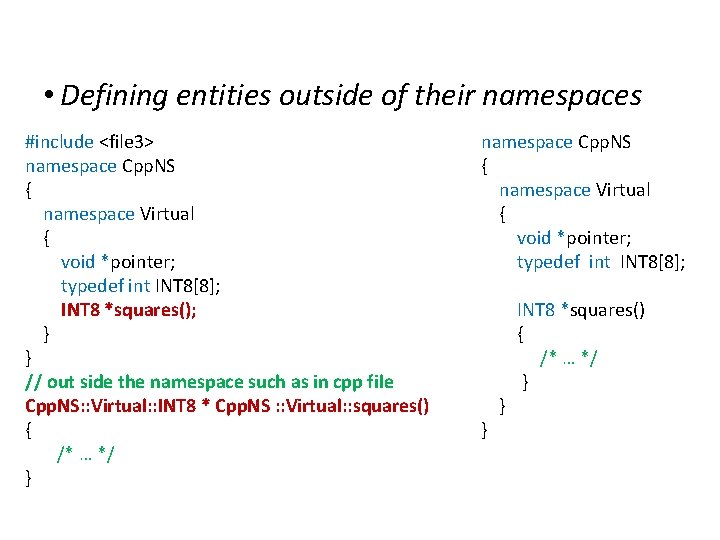
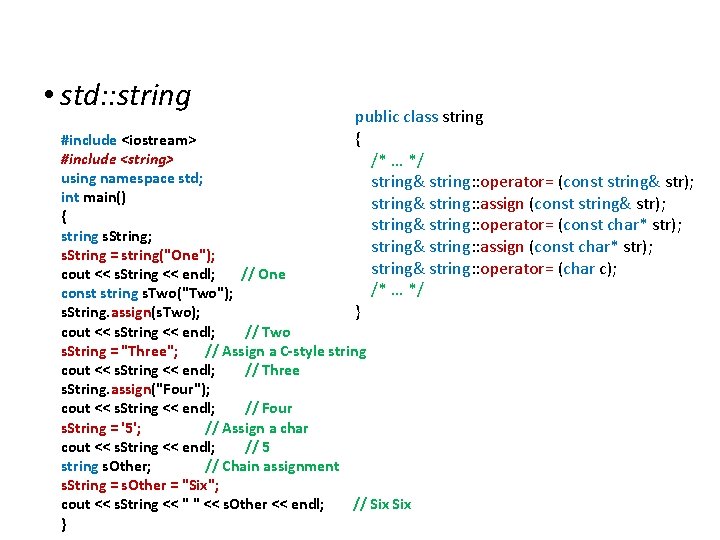
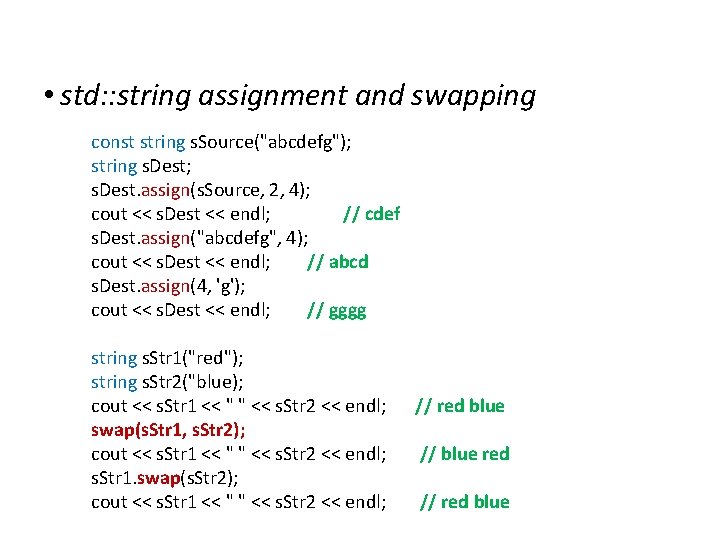
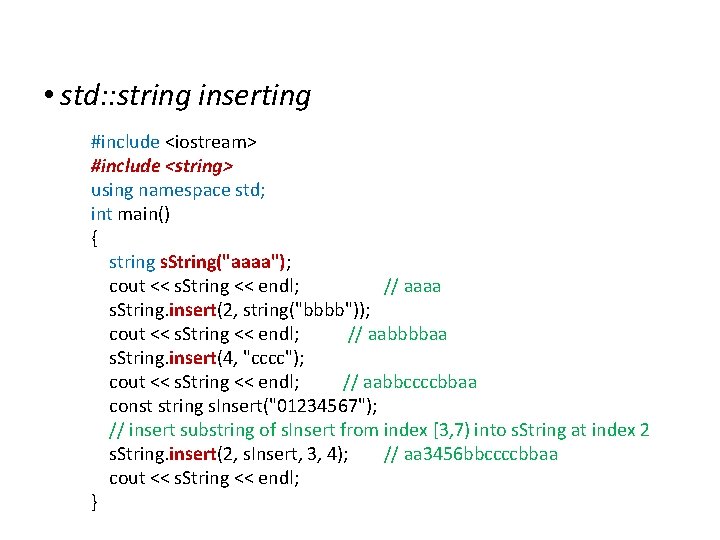
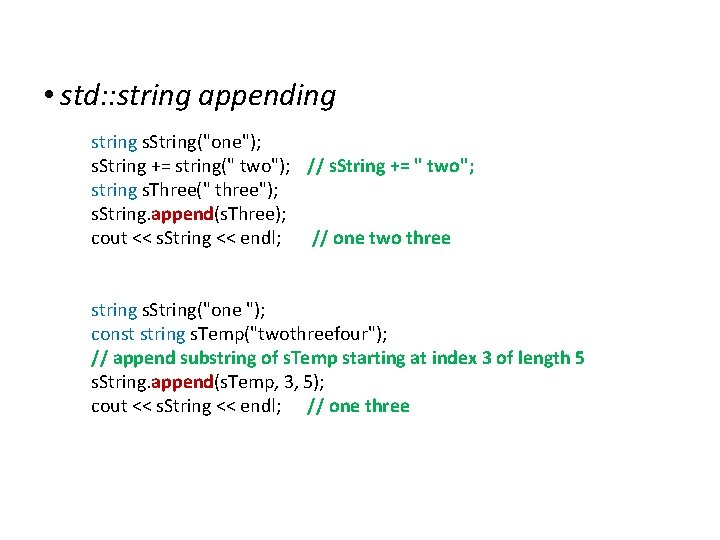
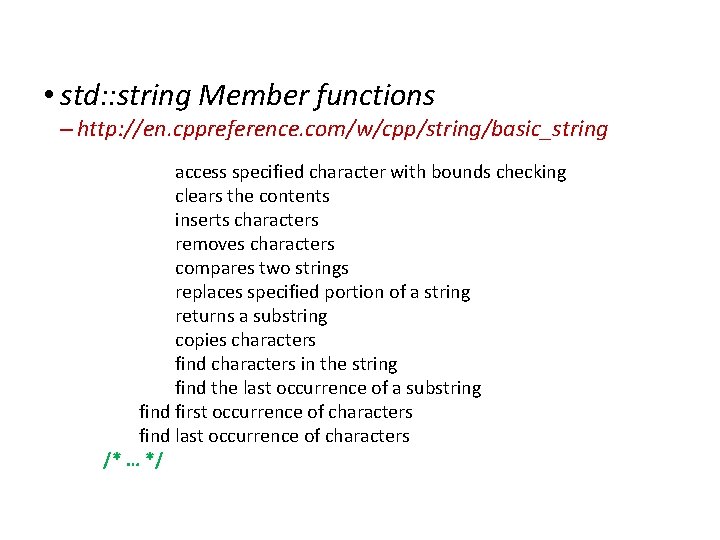
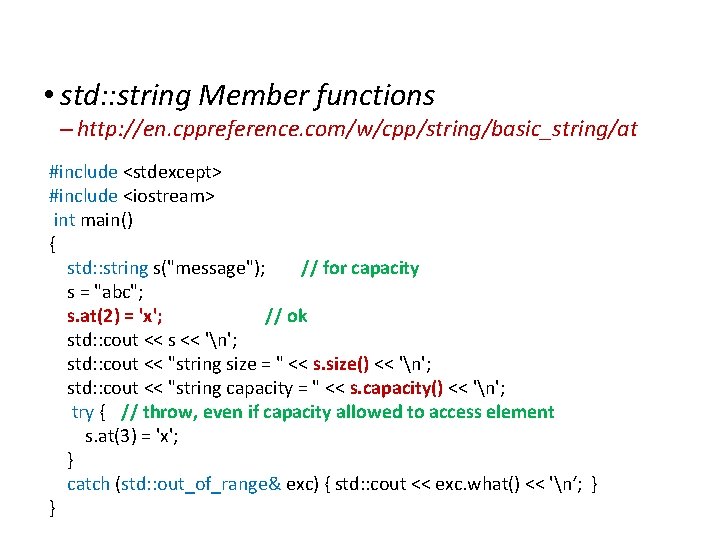
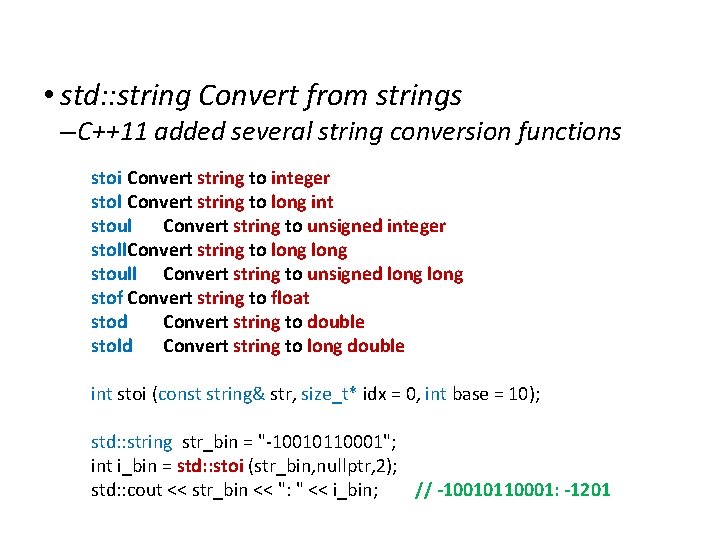
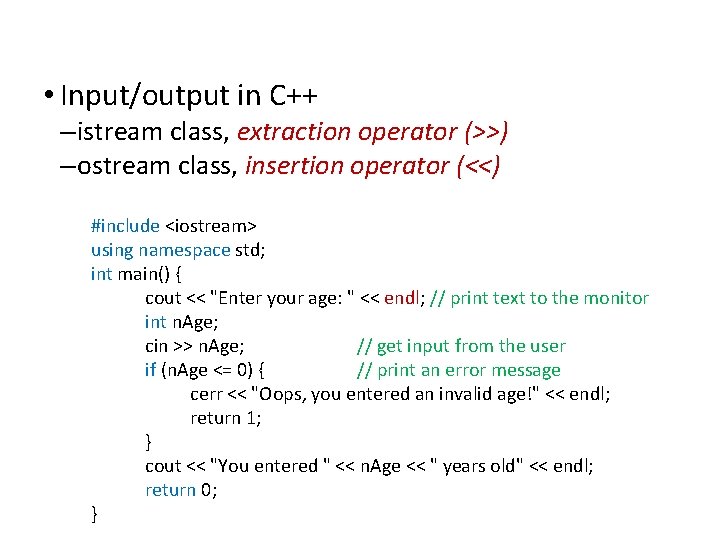
![IOStreams • extraction operator (>>) char buf[10]; cin >> buf; // what happens if IOStreams • extraction operator (>>) char buf[10]; cin >> buf; // what happens if](https://slidetodoc.com/presentation_image_h/9338e8fb76f47ecbf126ab6c6b08158c/image-51.jpg)
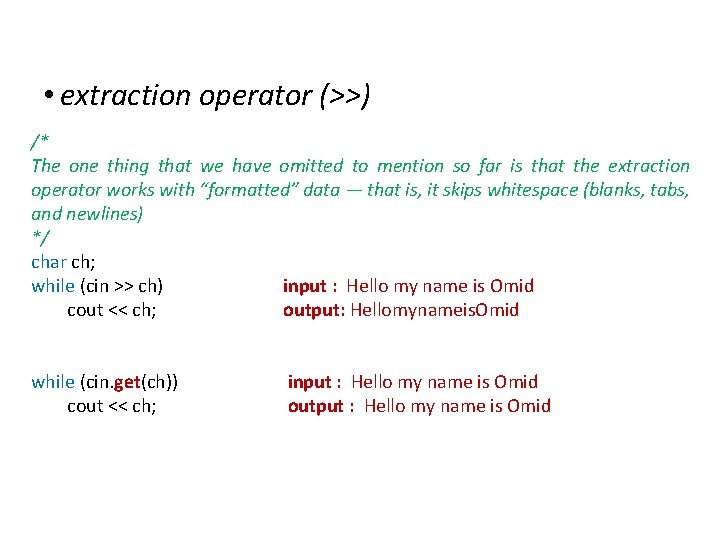
![IOStreams • extraction operator (>>) char str. Buf[11]; // only read the first 10 IOStreams • extraction operator (>>) char str. Buf[11]; // only read the first 10](https://slidetodoc.com/presentation_image_h/9338e8fb76f47ecbf126ab6c6b08158c/image-53.jpg)
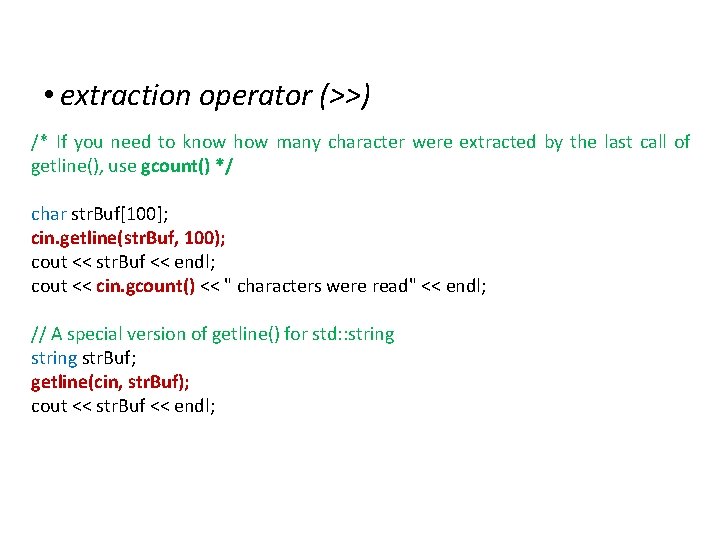
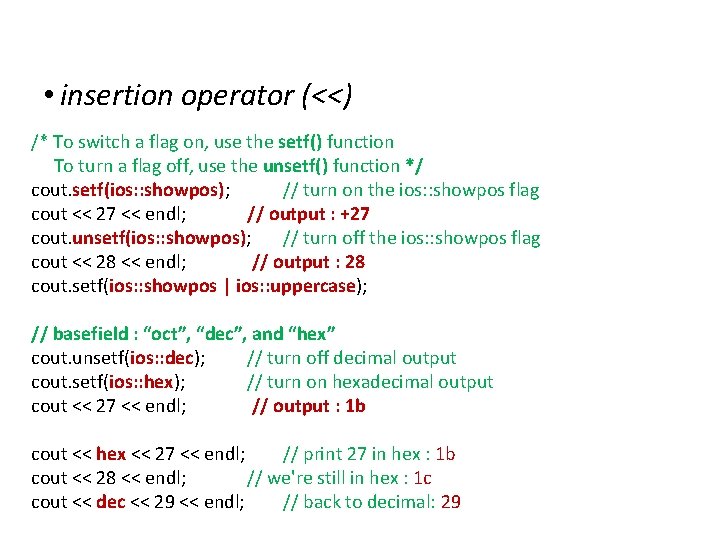
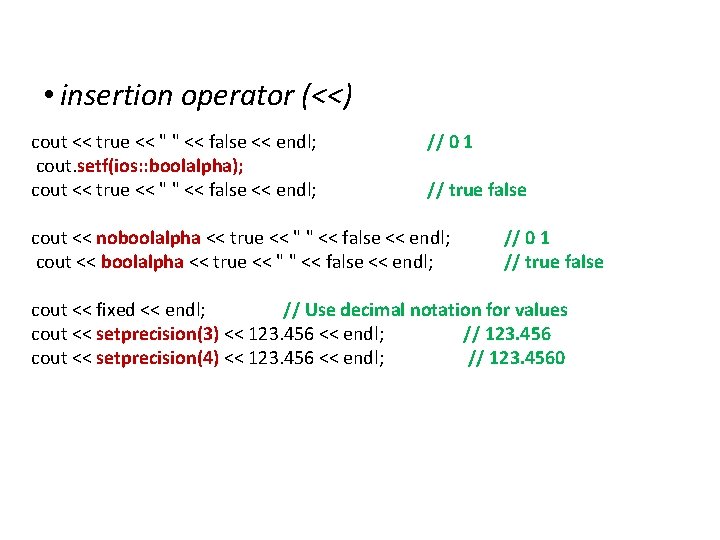
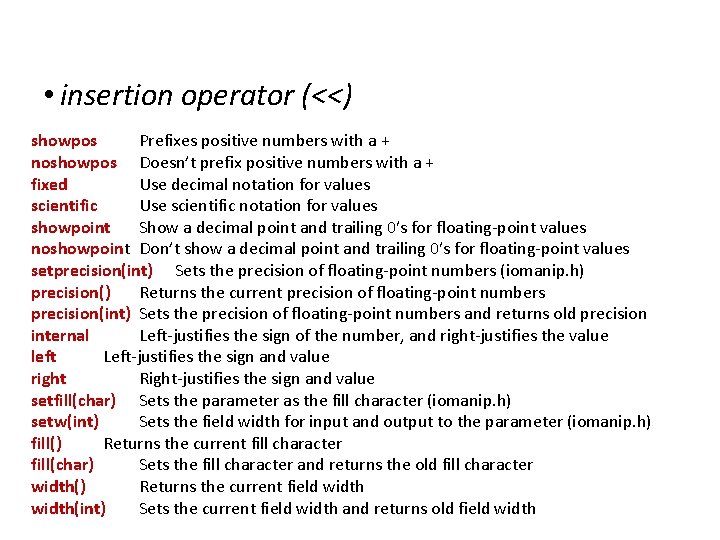
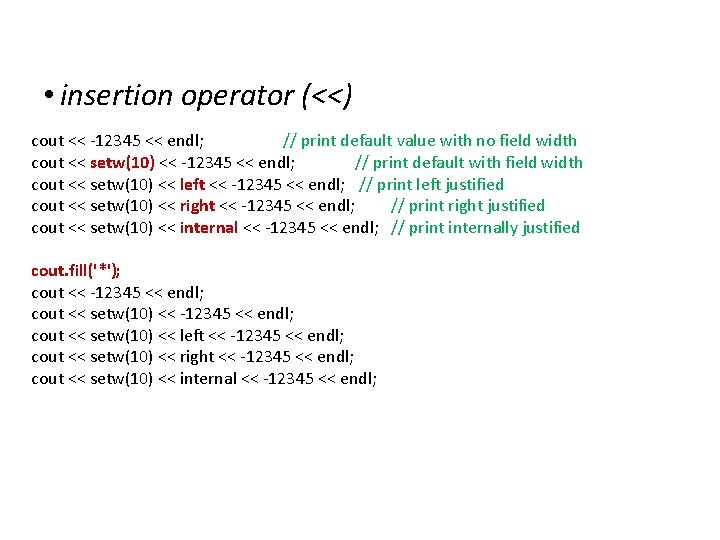
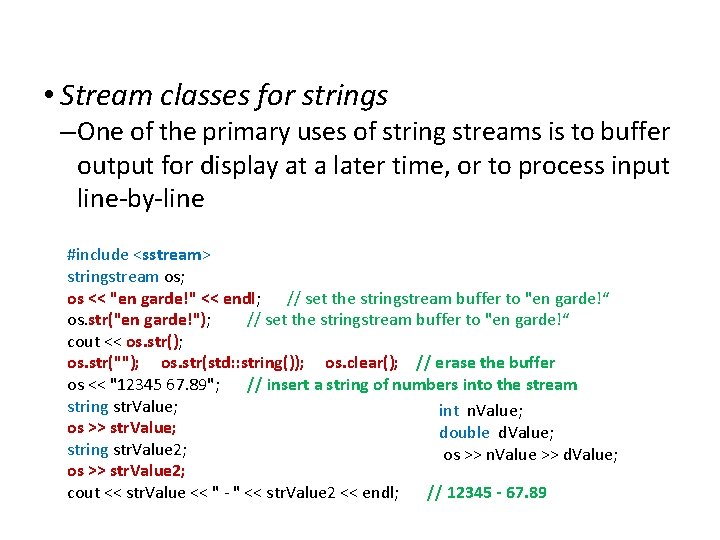
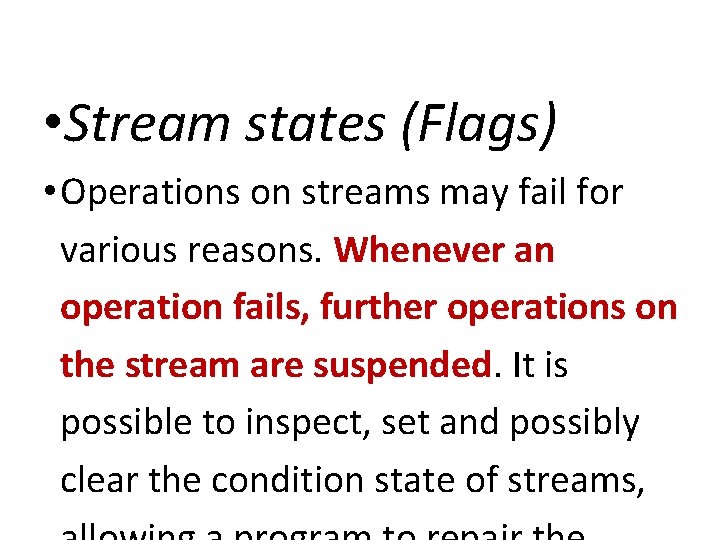
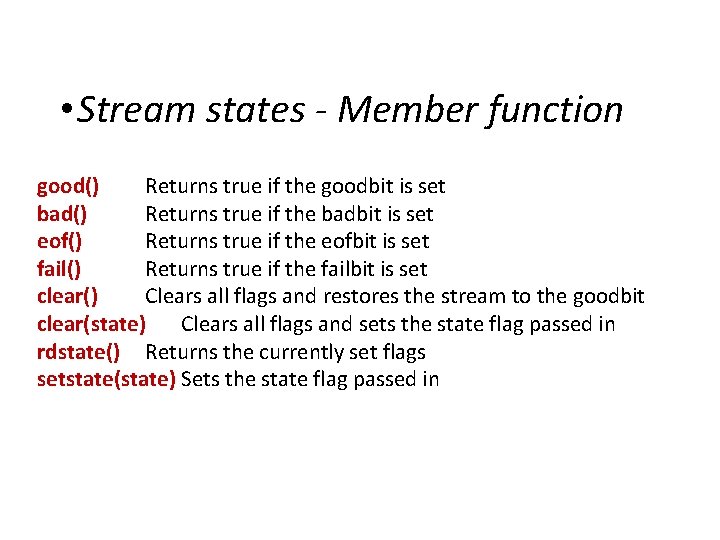
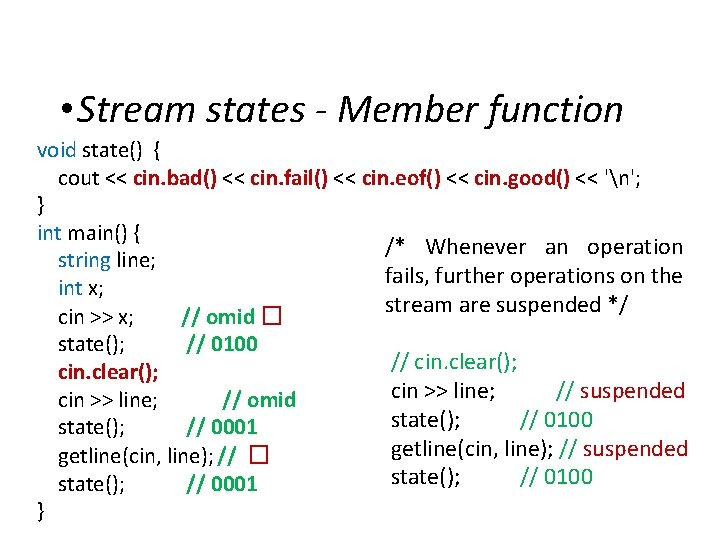
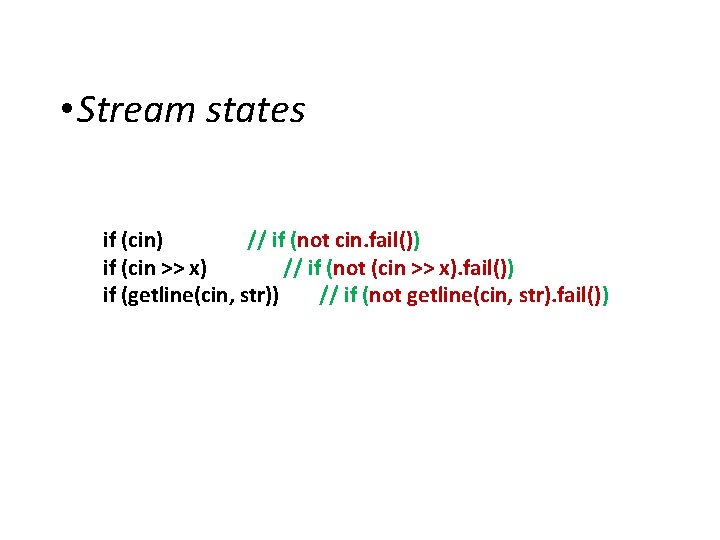
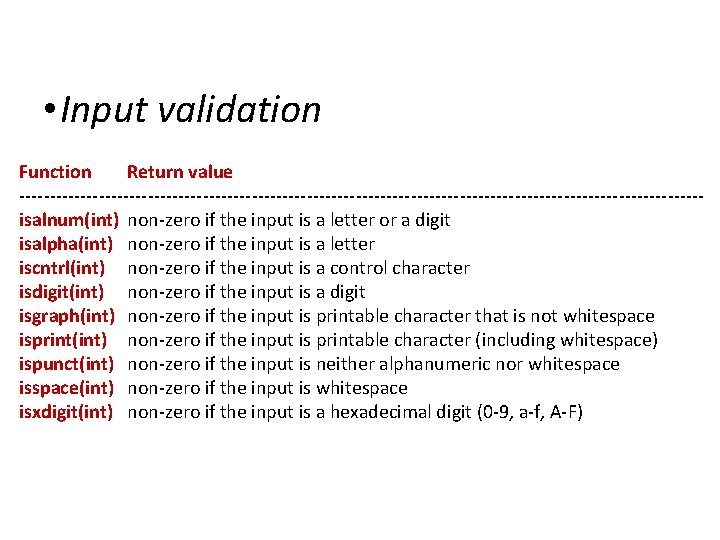
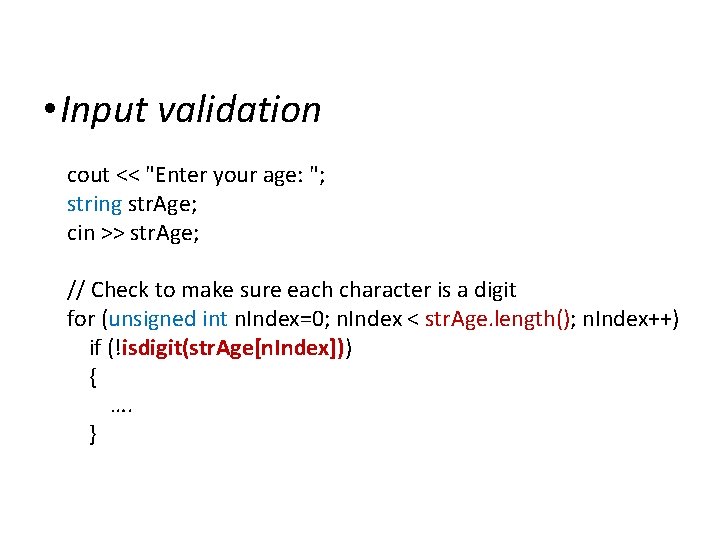
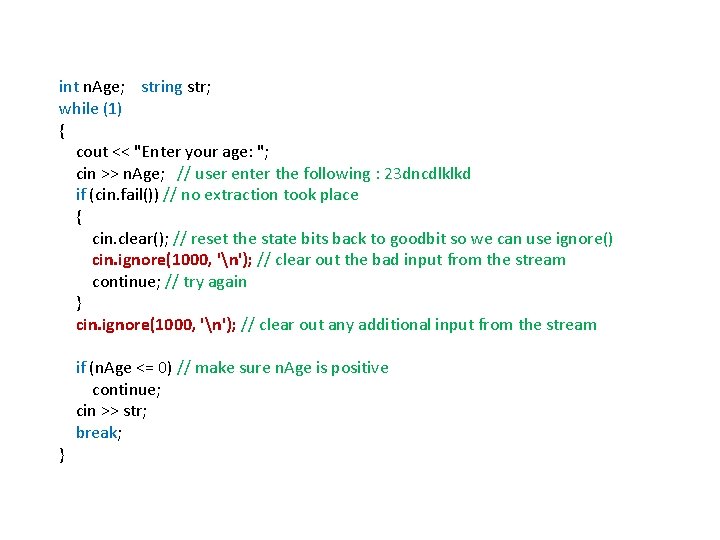
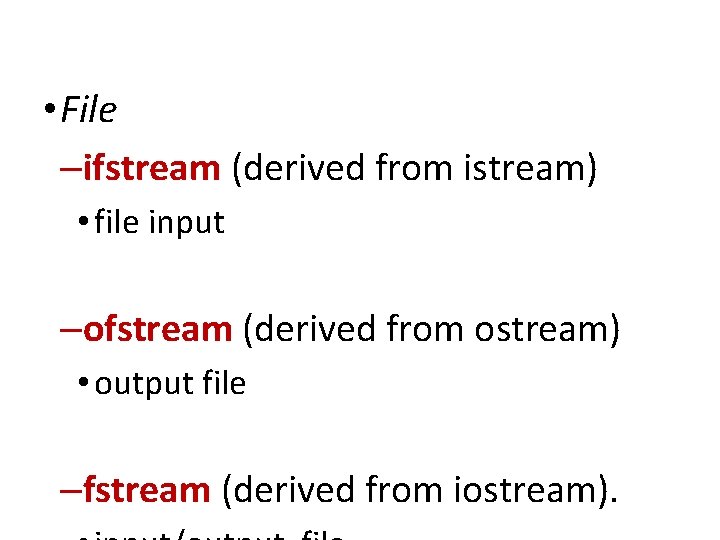
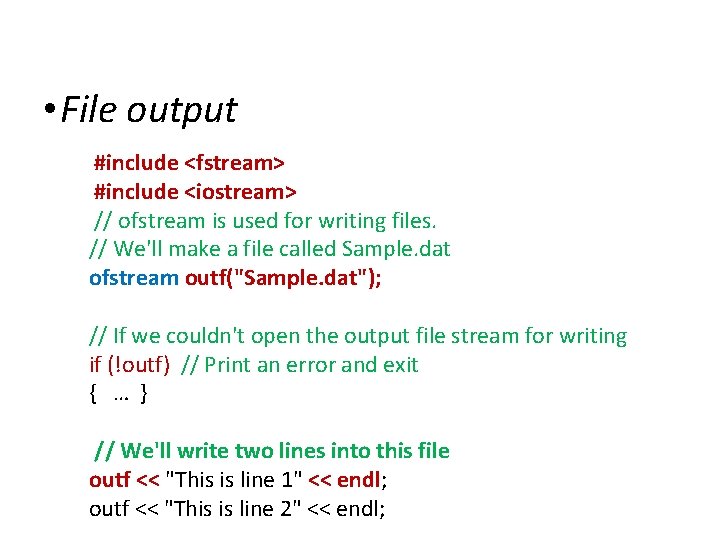
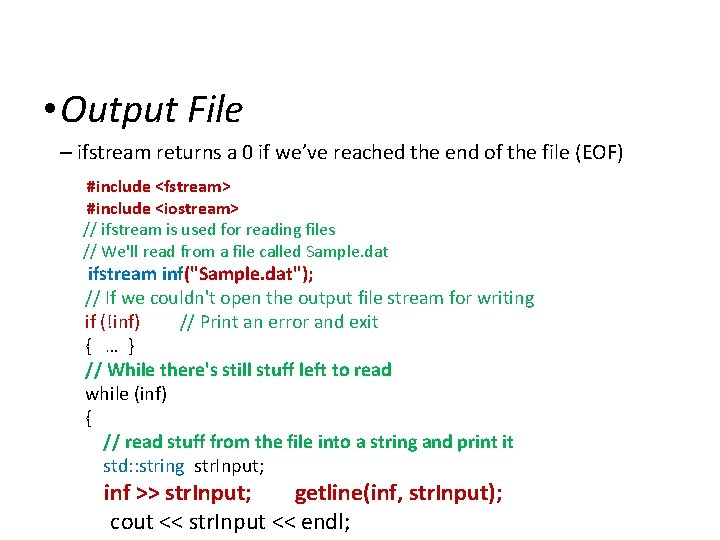
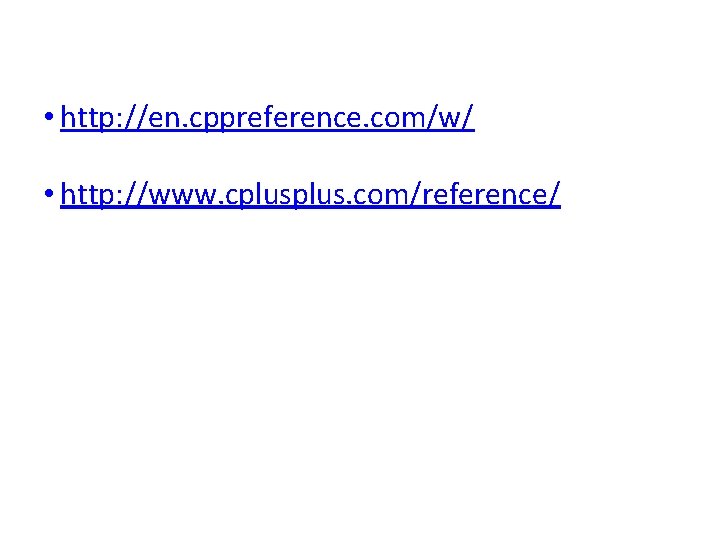
- Slides: 70
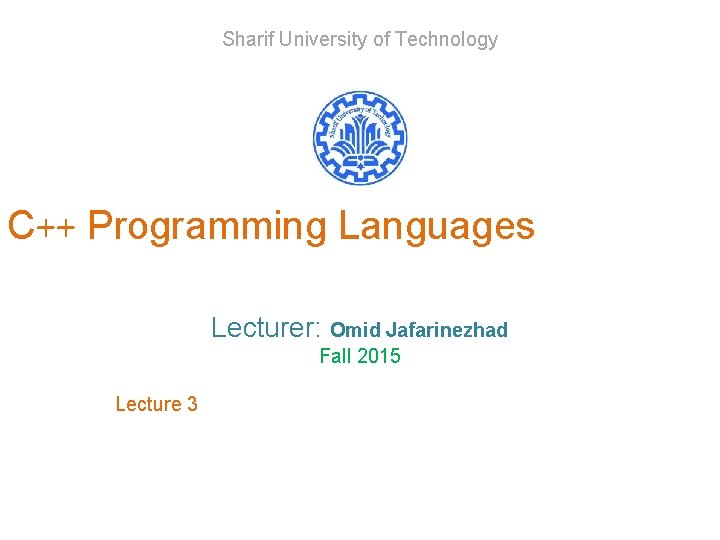
Sharif University of Technology C++ Programming Languages Lecturer: Omid Jafarinezhad Fall 2015 Lecture 3 Department of Computer Engineering
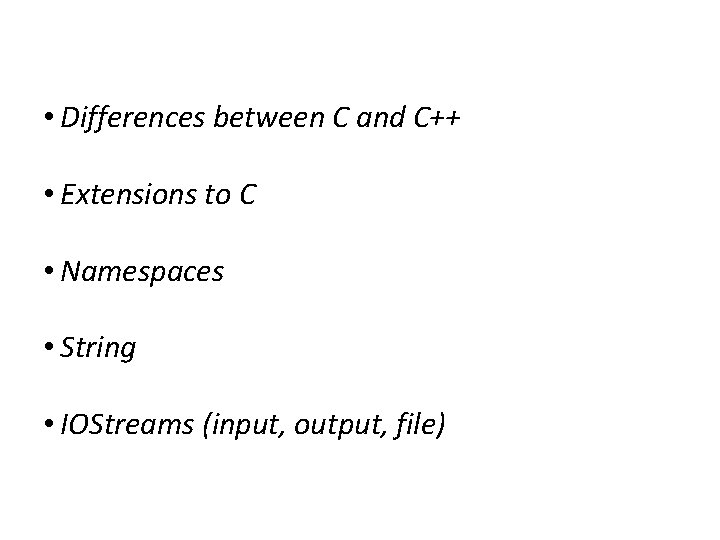
Outline • Differences between C and C++ • Extensions to C • Namespaces • String • IOStreams (input, output, file)
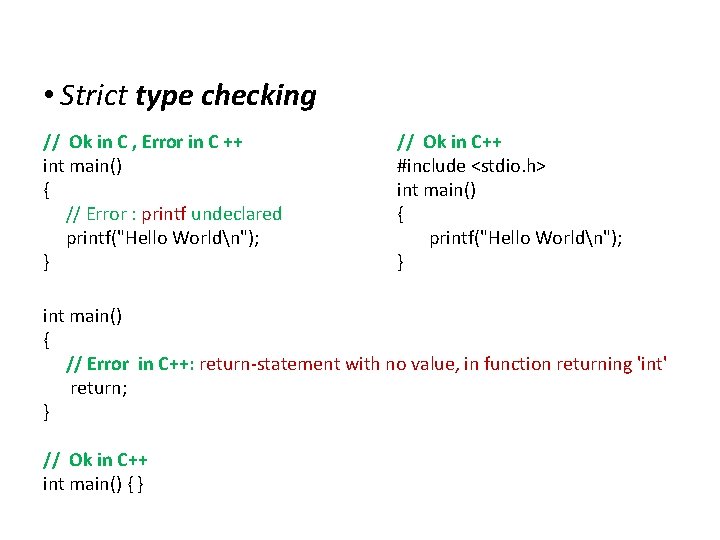
Differences between C and C++ • Strict type checking // Ok in C , Error in C ++ int main() { // Error : printf undeclared printf("Hello Worldn"); } // Ok in C++ #include <stdio. h> int main() { printf("Hello Worldn"); } int main() { // Error in C++: return-statement with no value, in function returning 'int' return; } // Ok in C++ int main() { }
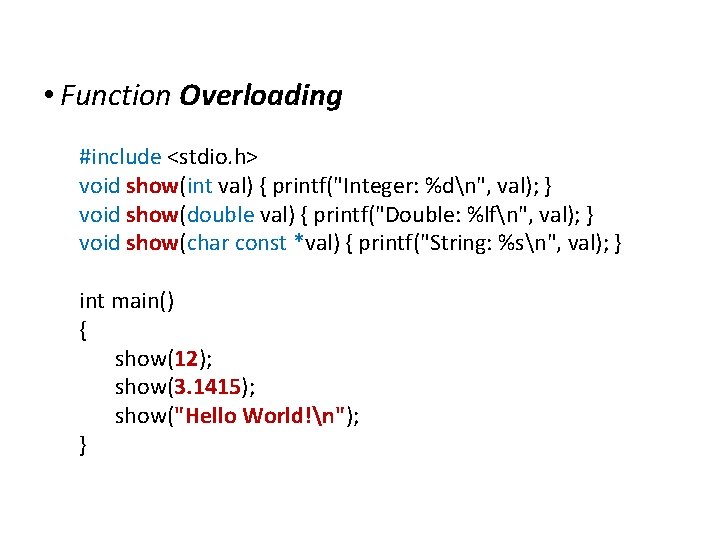
Extensions to C • Function Overloading #include <stdio. h> void show(int val) { printf("Integer: %dn", val); } void show(double val) { printf("Double: %lfn", val); } void show(char const *val) { printf("String: %sn", val); } int main() { show(12); show(3. 1415); show("Hello World!n"); }
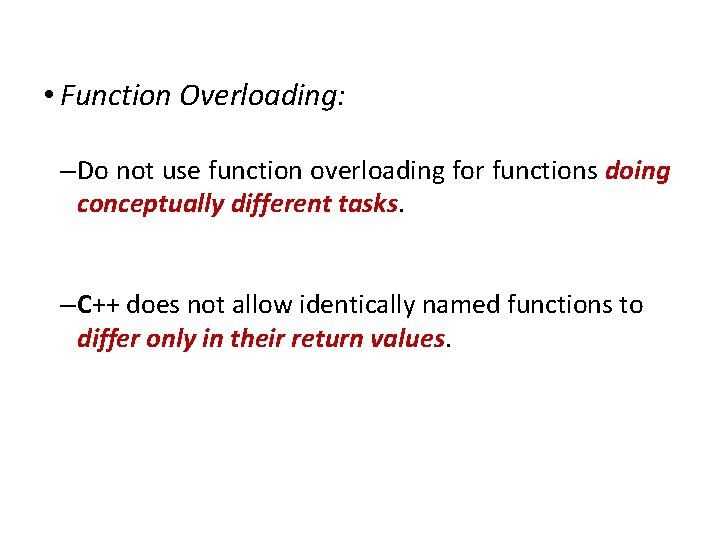
Extensions to C • Function Overloading: – Do not use function overloading for functions doing conceptually different tasks. – C++ does not allow identically named functions to differ only in their return values.
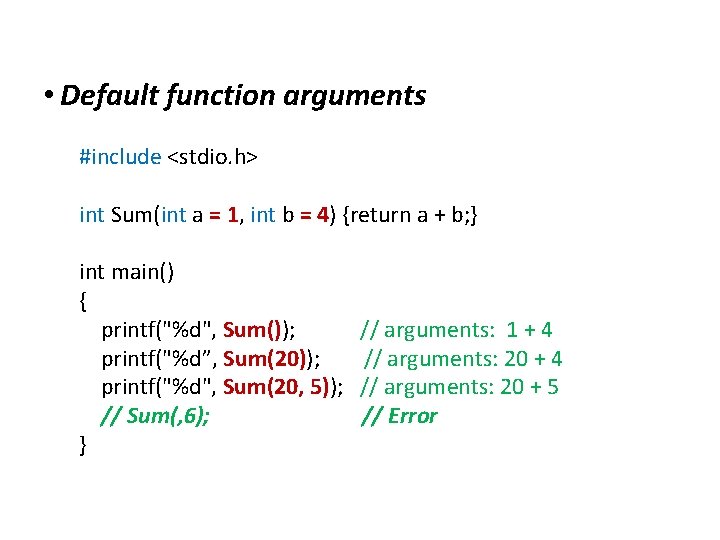
Extensions to C • Default function arguments #include <stdio. h> int Sum(int a = 1, int b = 4) {return a + b; } int main() { printf("%d", Sum()); // arguments: 1 + 4 printf("%d”, Sum(20)); // arguments: 20 + 4 printf("%d", Sum(20, 5)); // arguments: 20 + 5 // Sum(, 6); // Error }
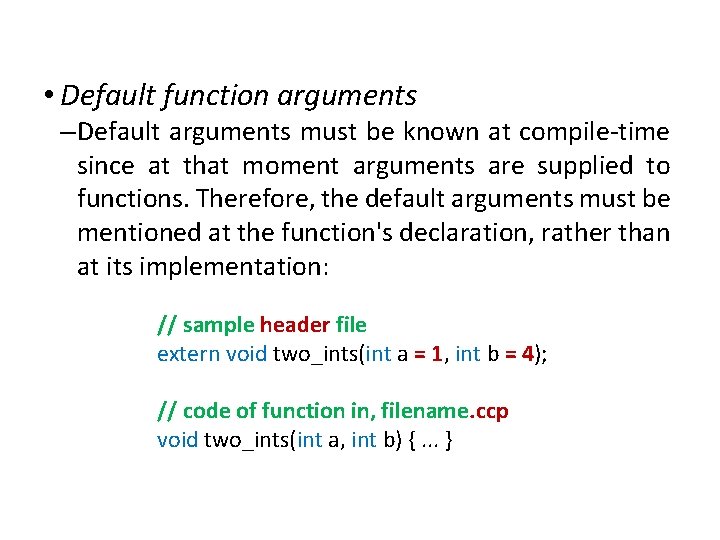
Extensions to C • Default function arguments – Default arguments must be known at compile-time since at that moment arguments are supplied to functions. Therefore, the default arguments must be mentioned at the function's declaration, rather than at its implementation: // sample header file extern void two_ints(int a = 1, int b = 4); // code of function in, filename. ccp void two_ints(int a, int b) {. . . }
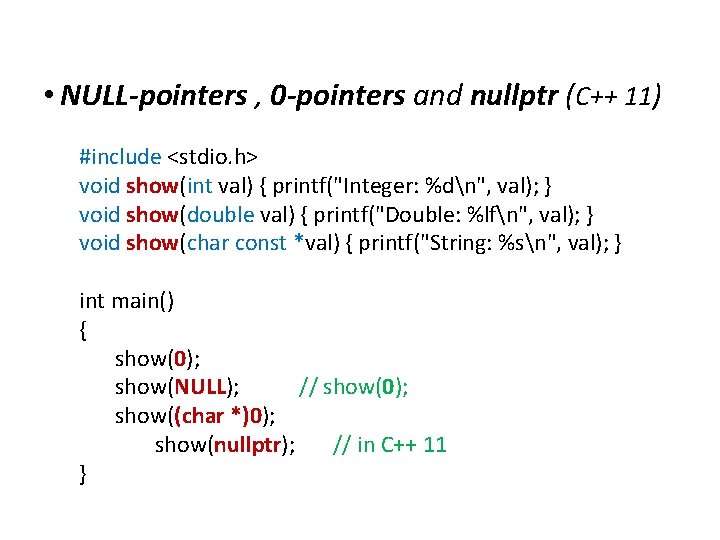
Differences between C and C++ • NULL-pointers , 0 -pointers and nullptr (C++ 11) #include <stdio. h> void show(int val) { printf("Integer: %dn", val); } void show(double val) { printf("Double: %lfn", val); } void show(char const *val) { printf("String: %sn", val); } int main() { show(0); show(NULL); // show(0); show((char *)0); show(nullptr); // in C++ 11 }
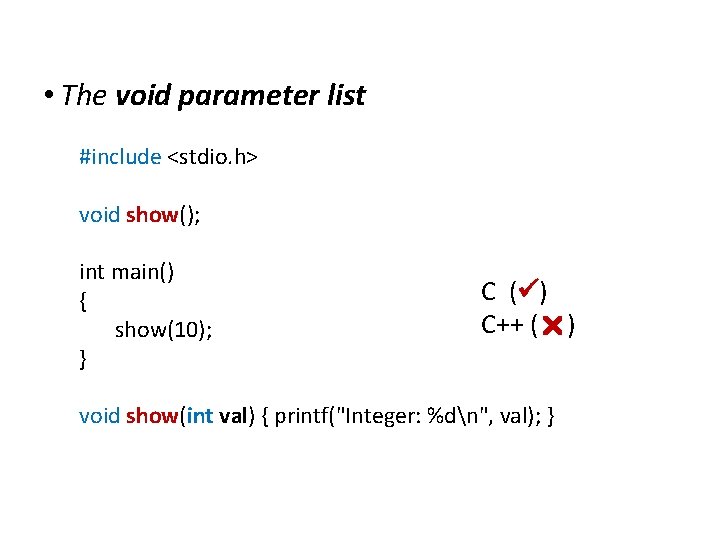
Differences between C and C++ • The void parameter list #include <stdio. h> void show(); int main() { show(10); } C ( ) C++ ( ) void show(int val) { printf("Integer: %dn", val); }
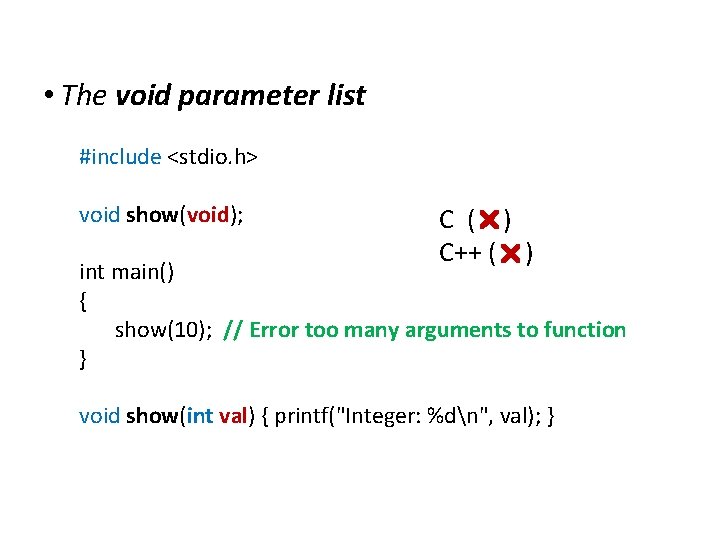
Differences between C and C++ • The void parameter list #include <stdio. h> void show(void); C ( ) C++ ( ) int main() { show(10); // Error too many arguments to function } void show(int val) { printf("Integer: %dn", val); }
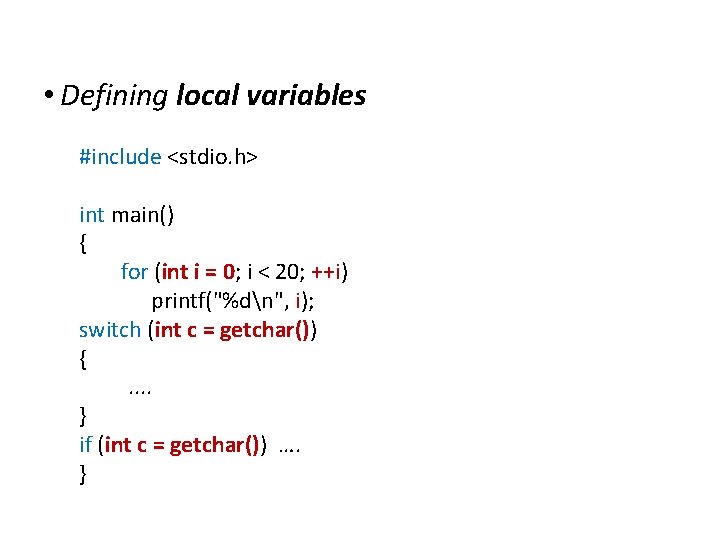
Differences between C and C++ • Defining local variables #include <stdio. h> int main() { for (int i = 0; i < 20; ++i) printf("%dn", i); switch (int c = getchar()) { . . } if (int c = getchar()) …. }
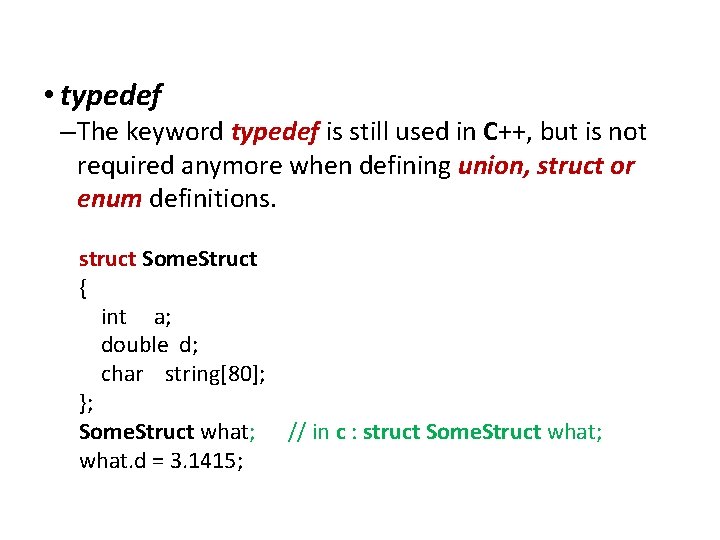
Differences between C and C++ • typedef – The keyword typedef is still used in C++, but is not required anymore when defining union, struct or enum definitions. struct Some. Struct { int a; double d; char string[80]; }; Some. Struct what; // in c : struct Some. Struct what; what. d = 3. 1415;
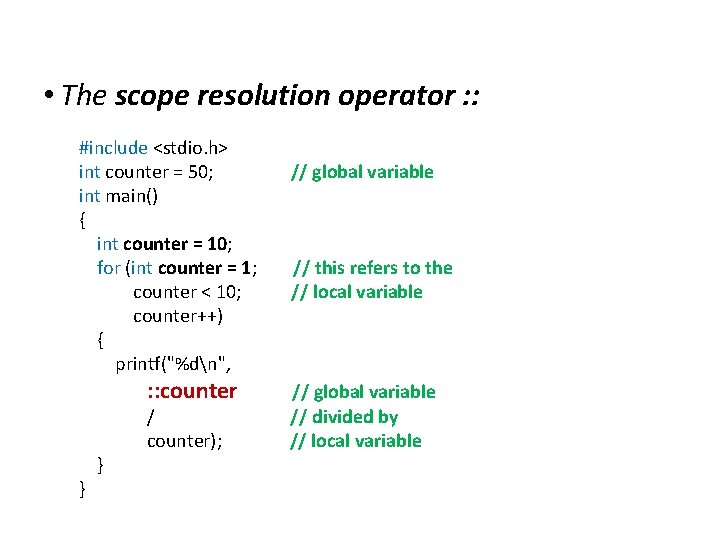
Extensions to C • The scope resolution operator : : #include <stdio. h> int counter = 50; // global variable int main() { int counter = 10; for (int counter = 1; // this refers to the counter < 10; // local variable counter++) { printf("%dn", : : counter // global variable // divided by counter); // local variable } }
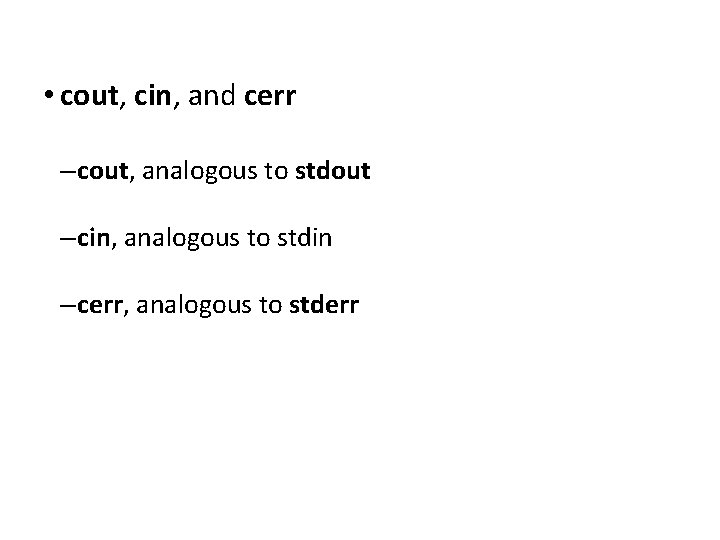
Extensions to C • cout, cin, and cerr – cout, analogous to stdout – cin, analogous to stdin – cerr, analogous to stderr
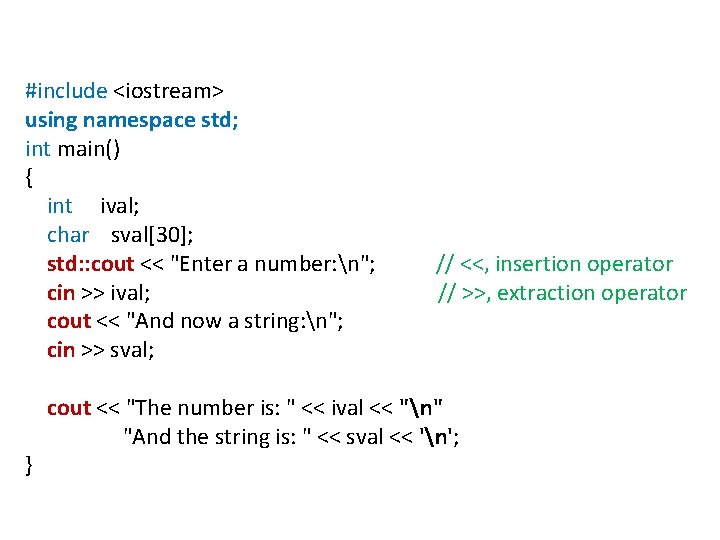
Extensions to C #include <iostream> using namespace std; int main() { int ival; char sval[30]; std: : cout << "Enter a number: n"; // <<, insertion operator cin >> ival; // >>, extraction operator cout << "And now a string: n"; cin >> sval; cout << "The number is: " << ival << "n" "And the string is: " << sval << 'n'; }
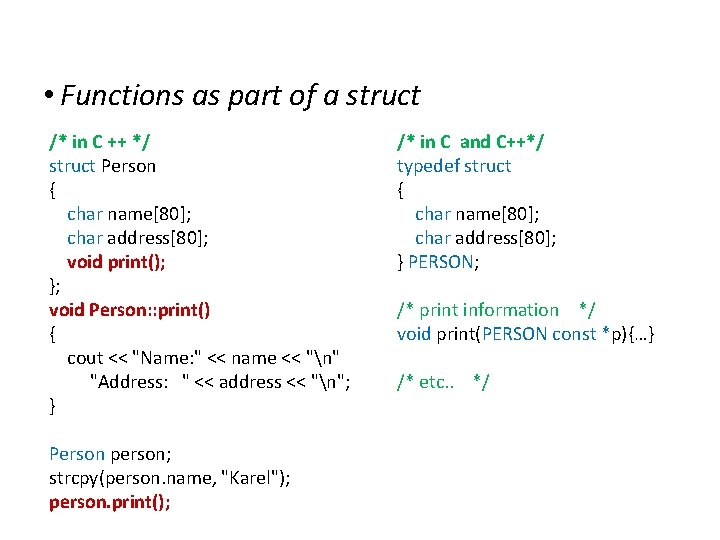
Extensions to C • Functions as part of a struct /* in C ++ */ struct Person { char name[80]; char address[80]; void print(); }; void Person: : print() { cout << "Name: " << name << "n" "Address: " << address << "n"; } Person person; strcpy(person. name, "Karel"); person. print(); /* in C and C++*/ typedef struct { char name[80]; char address[80]; } PERSON; /* print information */ void print(PERSON const *p){…} /* etc. . */
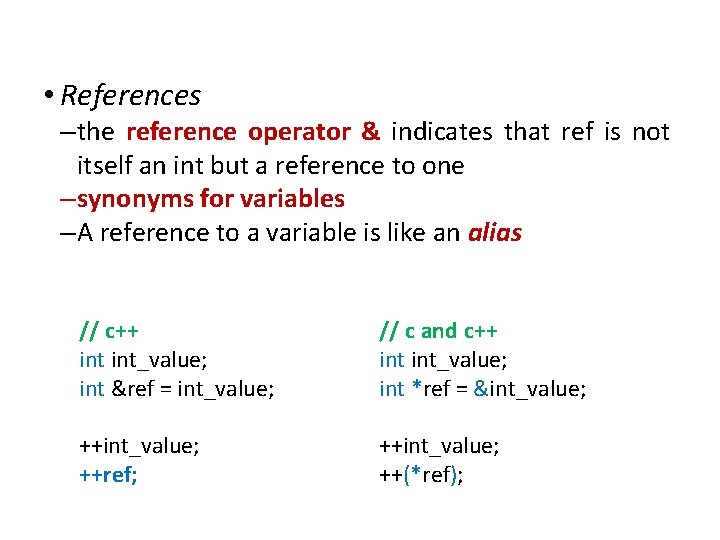
Extensions to C • References – the reference operator & indicates that ref is not itself an int but a reference to one – synonyms for variables – A reference to a variable is like an alias // c++ int_value; int &ref = int_value; // c and c++ int_value; int *ref = &int_value; ++ref; ++int_value; ++(*ref);
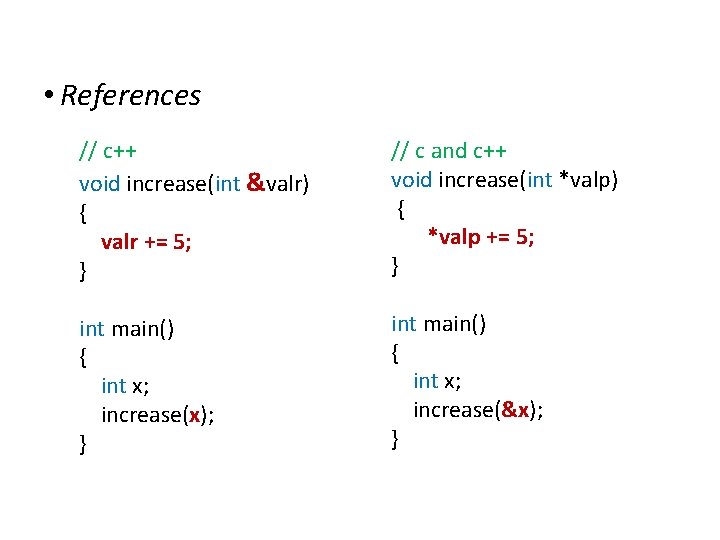
Extensions to C • References // c++ void increase(int &valr) { valr += 5; } // c and c++ void increase(int *valp) { *valp += 5; } int main() { int x; increase(x); } int main() { int x; increase(&x); }
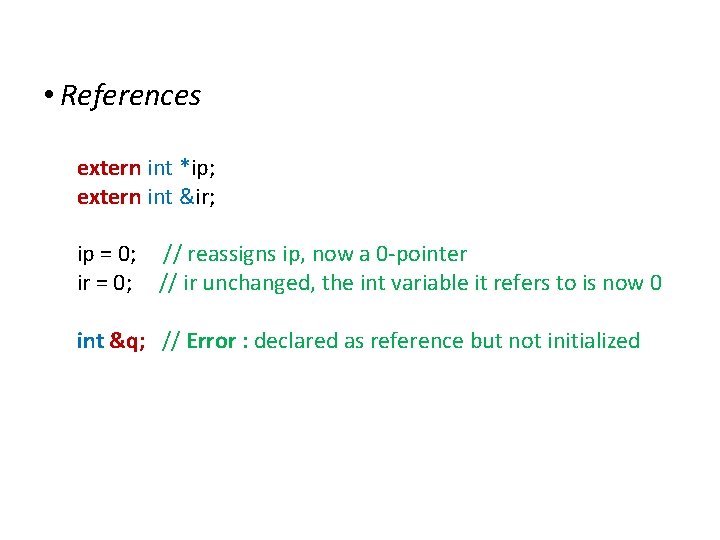
Extensions to C • References extern int *ip; extern int &ir; ip = 0; // reassigns ip, now a 0 -pointer ir = 0; // ir unchanged, the int variable it refers to is now 0 int &q; // Error : declared as reference but not initialized
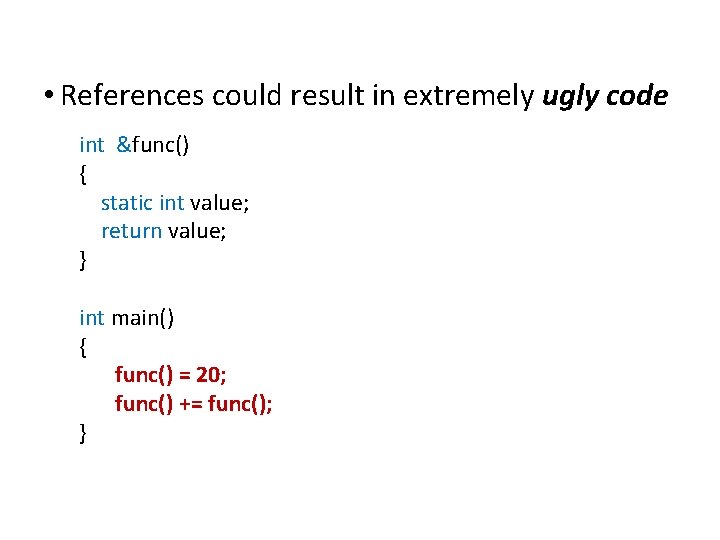
Extensions to C • References could result in extremely ugly code int &func() { static int value; return value; } int main() { func() = 20; func() += func(); }
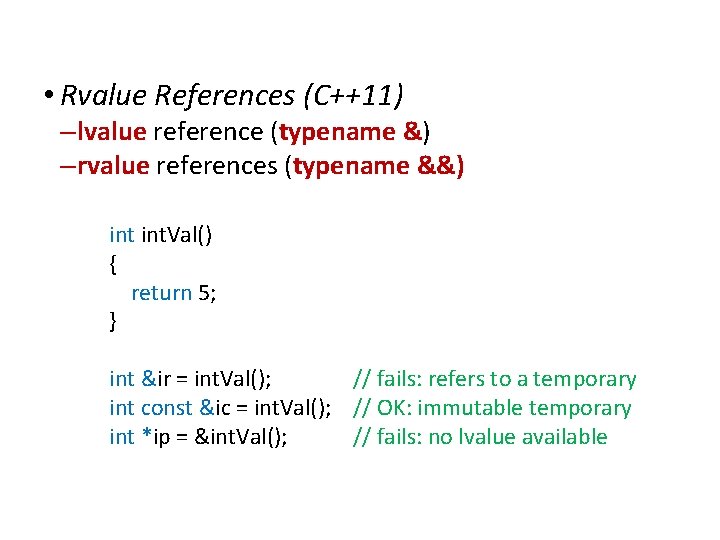
Extensions to C • Rvalue References (C++11) – lvalue reference (typename &) – rvalue references (typename &&) int. Val() { return 5; } int &ir = int. Val(); // fails: refers to a temporary int const &ic = int. Val(); // OK: immutable temporary int *ip = &int. Val(); // fails: no lvalue available
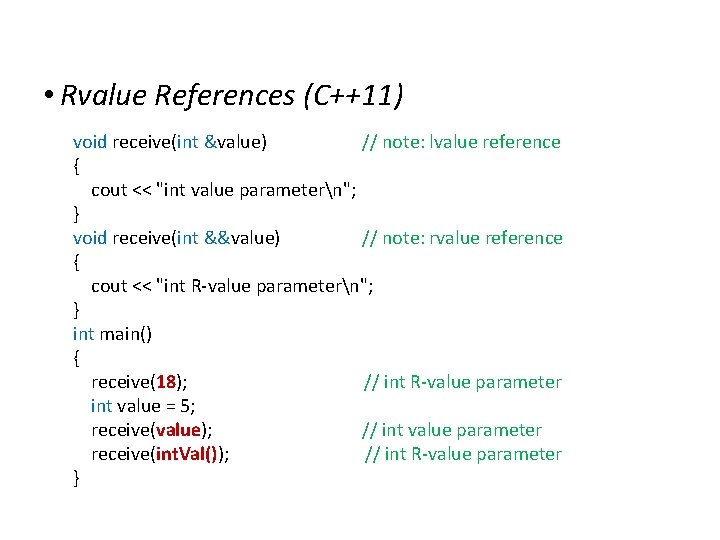
Extensions to C • Rvalue References (C++11) void receive(int &value) // note: lvalue reference { cout << "int value parametern"; } void receive(int &&value) // note: rvalue reference { cout << "int R-value parametern"; } int main() { receive(18); // int R-value parameter int value = 5; receive(value); // int value parameter receive(int. Val()); // int R-value parameter }
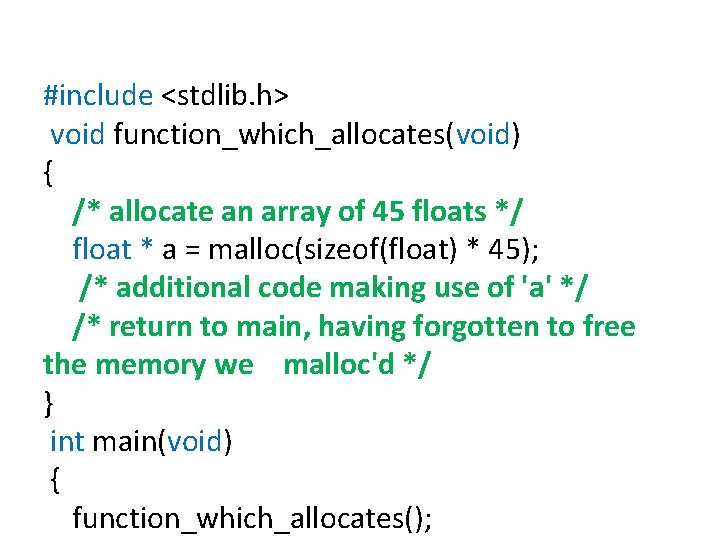
Memory leak #include <stdlib. h> void function_which_allocates(void) { /* allocate an array of 45 floats */ float * a = malloc(sizeof(float) * 45); /* additional code making use of 'a' */ /* return to main, having forgotten to free the memory we malloc'd */ } int main(void) { function_which_allocates();
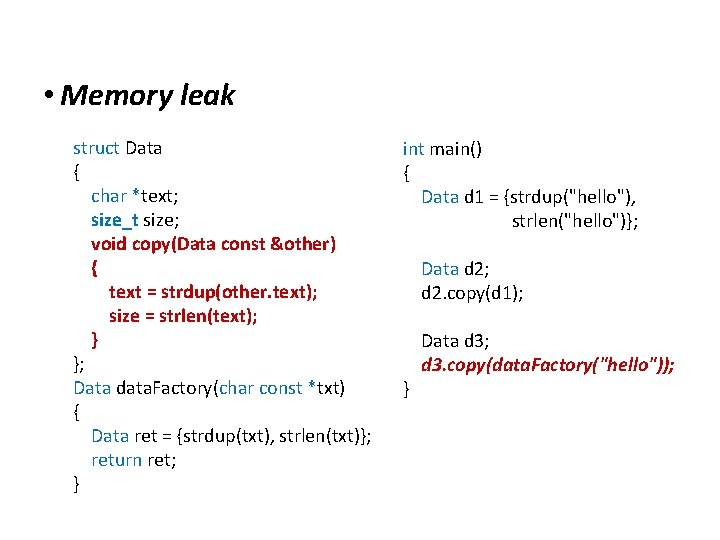
Extensions to C • Memory leak struct Data { char *text; size_t size; void copy(Data const &other) { text = strdup(other. text); size = strlen(text); } }; Data data. Factory(char const *txt) { Data ret = {strdup(txt), strlen(txt)}; return ret; } int main() { Data d 1 = {strdup("hello"), strlen("hello")}; Data d 2; d 2. copy(d 1); Data d 3; d 3. copy(data. Factory("hello")); }
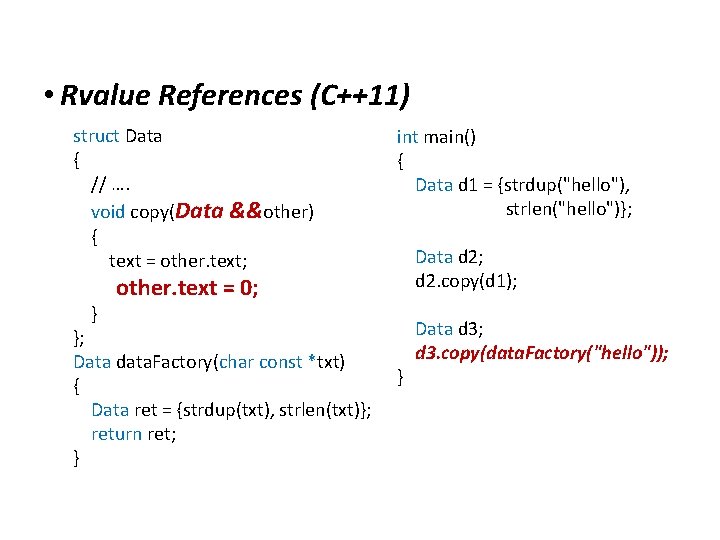
Extensions to C • Rvalue References (C++11) struct Data { // …. void copy(Data &&other) { text = other. text; other. text = 0; } }; Data data. Factory(char const *txt) { Data ret = {strdup(txt), strlen(txt)}; return ret; } int main() { Data d 1 = {strdup("hello"), strlen("hello")}; Data d 2; d 2. copy(d 1); Data d 3; d 3. copy(data. Factory("hello")); }
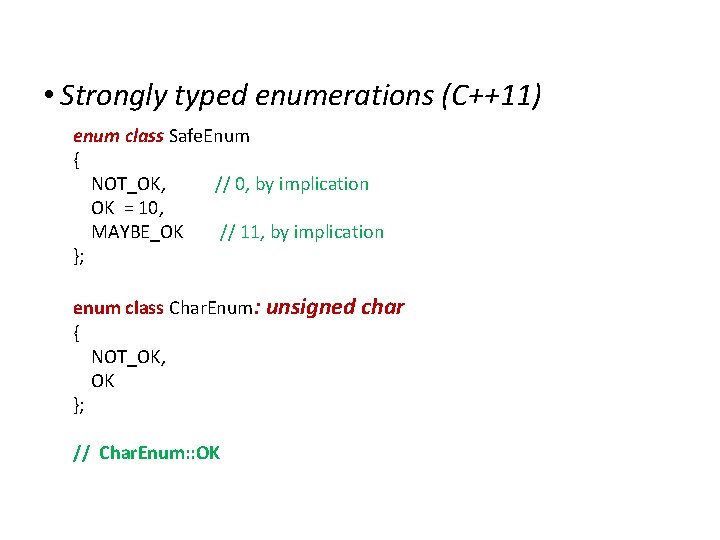
Extensions to C • Strongly typed enumerations (C++11) enum class Safe. Enum { NOT_OK, // 0, by implication OK = 10, MAYBE_OK // 11, by implication }; enum class Char. Enum: unsigned char { NOT_OK, OK }; // Char. Enum: : OK
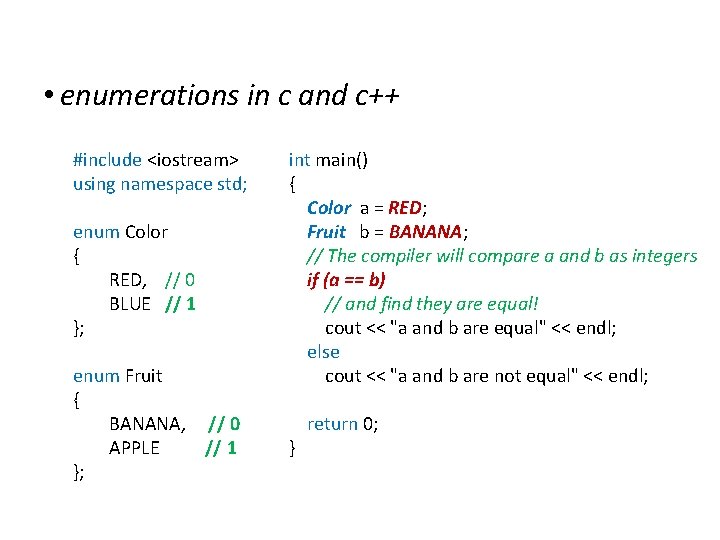
Extensions to C • enumerations in c and c++ #include <iostream> using namespace std; enum Color { RED, // 0 BLUE // 1 }; enum Fruit { BANANA, // 0 APPLE // 1 }; int main() { Color a = RED; Fruit b = BANANA; // The compiler will compare a and b as integers if (a == b) // and find they are equal! cout << "a and b are equal" << endl; else cout << "a and b are not equal" << endl; return 0; }
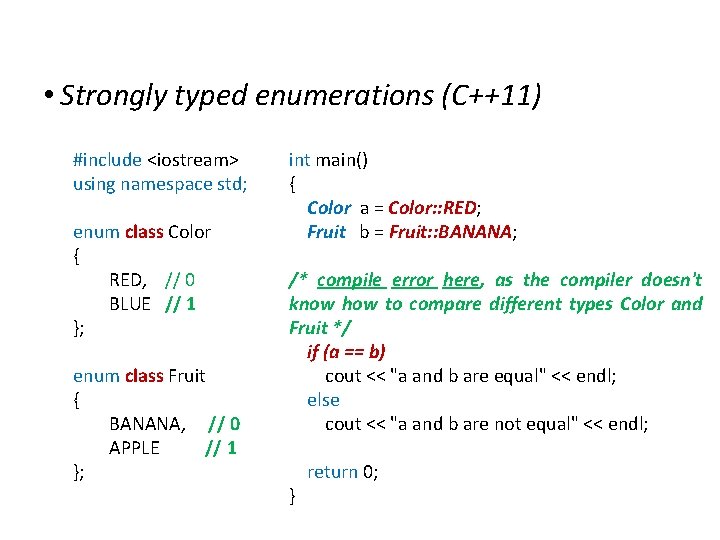
Extensions to C • Strongly typed enumerations (C++11) #include <iostream> using namespace std; enum class Color { RED, // 0 BLUE // 1 }; enum class Fruit { BANANA, // 0 APPLE // 1 }; int main() { Color a = Color: : RED; Fruit b = Fruit: : BANANA; /* compile error here, as the compiler doesn't know how to compare different types Color and Fruit */ if (a == b) cout << "a and b are equal" << endl; else cout << "a and b are not equal" << endl; return 0; }
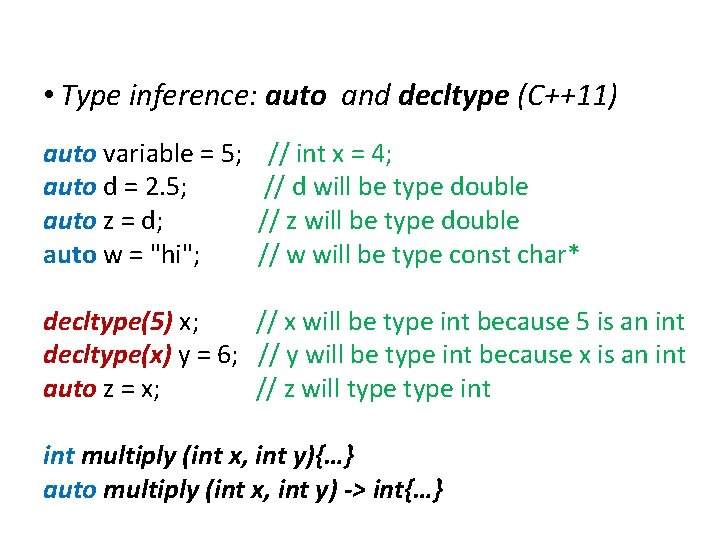
Extensions to C • Type inference: auto and decltype (C++11) auto variable = 5; // int x = 4; auto d = 2. 5; // d will be type double auto z = d; // z will be type double auto w = "hi"; // w will be type const char* decltype(5) x; // x will be type int because 5 is an int decltype(x) y = 6; // y will be type int because x is an int auto z = x; // z will type int multiply (int x, int y){…} auto multiply (int x, int y) -> int{…}
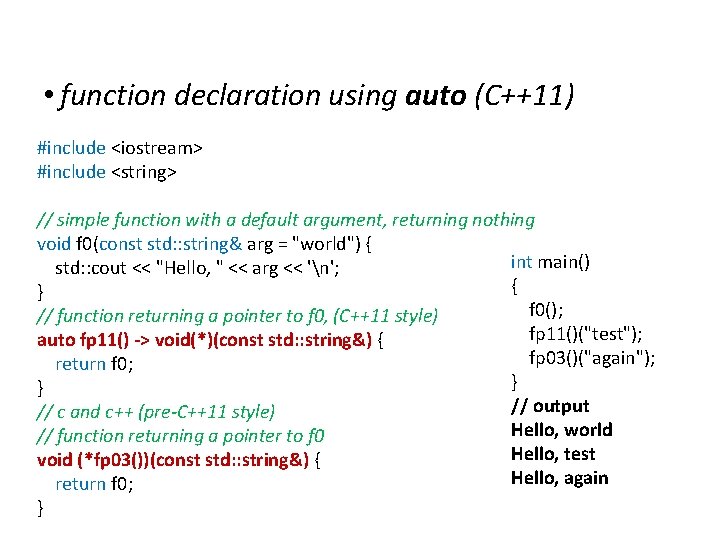
Extensions to C • function declaration using auto (C++11) #include <iostream> #include <string> // simple function with a default argument, returning nothing void f 0(const std: : string& arg = "world") { int main() std: : cout << "Hello, " << arg << 'n'; { } f 0(); // function returning a pointer to f 0, (C++11 style) fp 11()("test"); auto fp 11() -> void(*)(const std: : string&) { fp 03()("again"); return f 0; } } // output // c and c++ (pre-C++11 style) Hello, world // function returning a pointer to f 0 Hello, test void (*fp 03())(const std: : string&) { Hello, again return f 0; }
![Extensions to C Rangebased forloops C11 struct Big Struct double array100 int Extensions to C • Range-based for-loops (C++11) struct Big. Struct { double array[100]; int](https://slidetodoc.com/presentation_image_h/9338e8fb76f47ecbf126ab6c6b08158c/image-31.jpg)
Extensions to C • Range-based for-loops (C++11) struct Big. Struct { double array[100]; int last; }; // assume int array[30] for (auto &element: array) statement Big. Struct data[100]; // assume properly initialized elsewhere int count. Used() { int sum = 0; // const &: the elements aren't modified for (auto const &element: data) sum += element. last; return sum; }
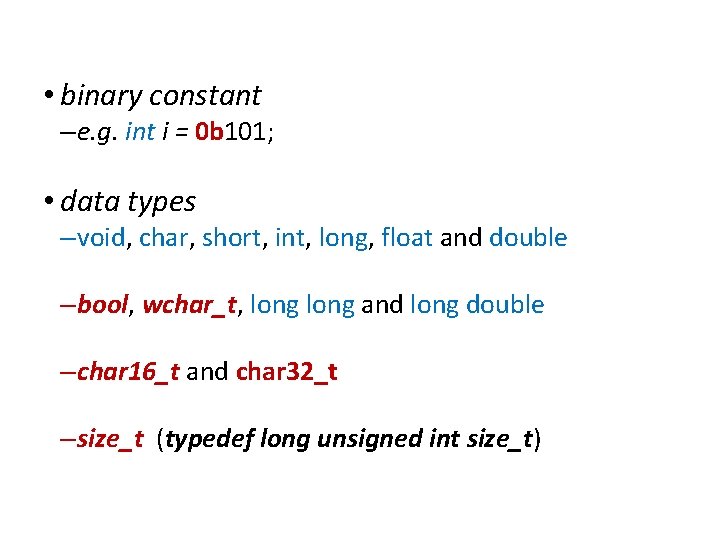
Extensions to C • binary constant – e. g. int i = 0 b 101; • data types – void, char, short, int, long, float and double – bool, wchar_t, long and long double – char 16_t and char 32_t – size_t (typedef long unsigned int size_t)
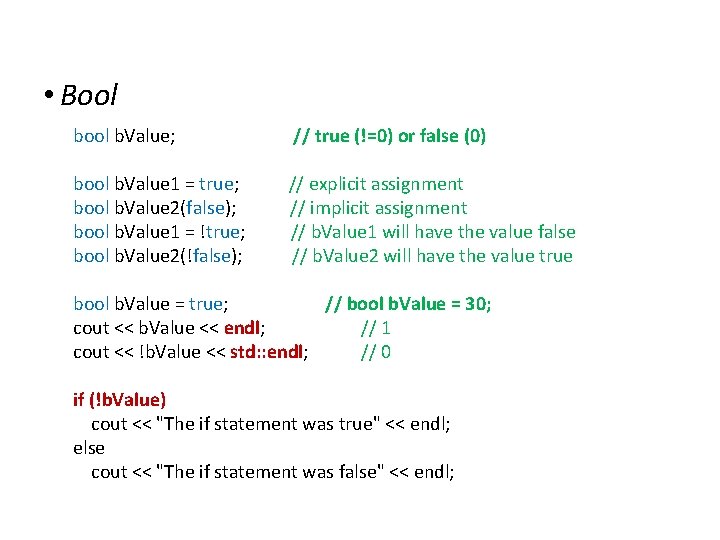
Extensions to C • Bool b. Value; // true (!=0) or false (0) bool b. Value 1 = true; // explicit assignment bool b. Value 2(false); // implicit assignment bool b. Value 1 = !true; // b. Value 1 will have the value false bool b. Value 2(!false); // b. Value 2 will have the value true bool b. Value = true; // bool b. Value = 30; cout << b. Value << endl; // 1 cout << !b. Value << std: : endl; // 0 if (!b. Value) cout << "The if statement was true" << endl; else cout << "The if statement was false" << endl;
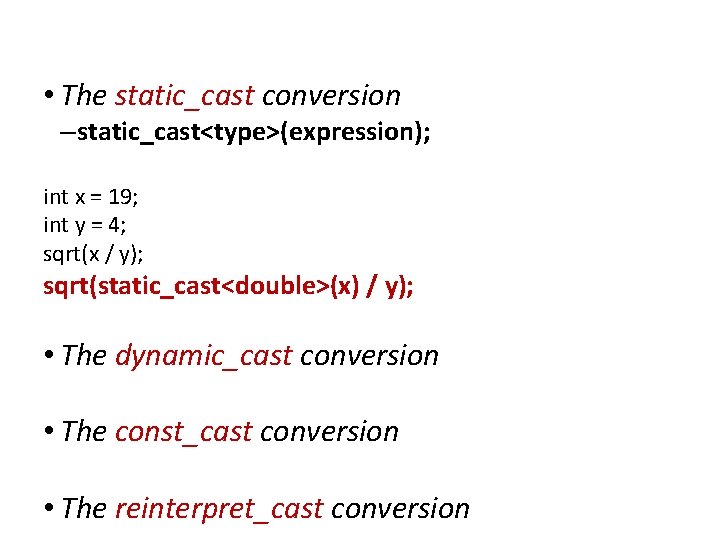
Extensions to C • The static_cast conversion – static_cast<type>(expression); int x = 19; int y = 4; sqrt(x / y); sqrt(static_cast<double>(x) / y); • The dynamic_cast conversion • The const_cast conversion • The reinterpret_cast conversion
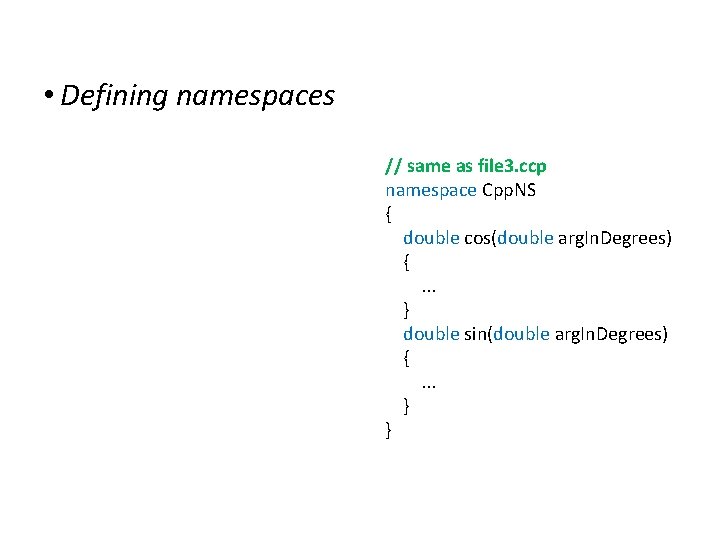
Namespaces • Defining namespaces // same as file 3. ccp namespace Cpp. NS { double cos(double arg. In. Degrees) { . . . } double sin(double arg. In. Degrees) { . . . } }
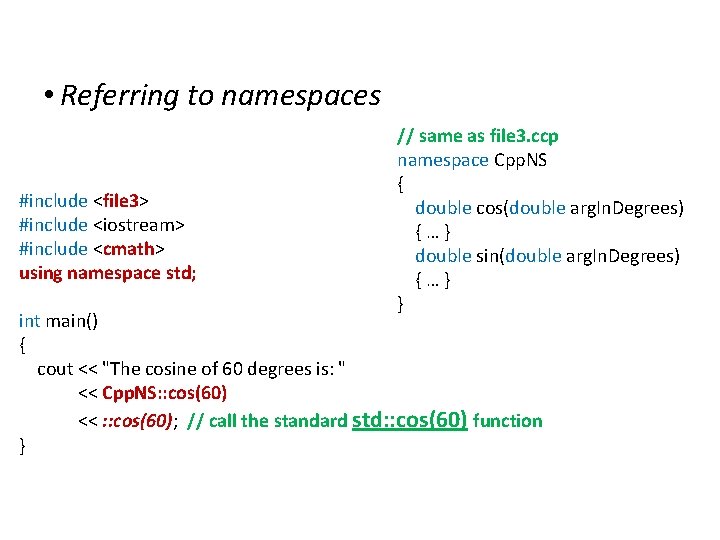
Namespaces • Referring to namespaces #include <file 3> #include <iostream> #include <cmath> using namespace std; // same as file 3. ccp namespace Cpp. NS { double cos(double arg. In. Degrees) { … } double sin(double arg. In. Degrees) { … } } int main() { cout << "The cosine of 60 degrees is: " << Cpp. NS: : cos(60) << : : cos(60); // call the standard std: : cos(60) function }
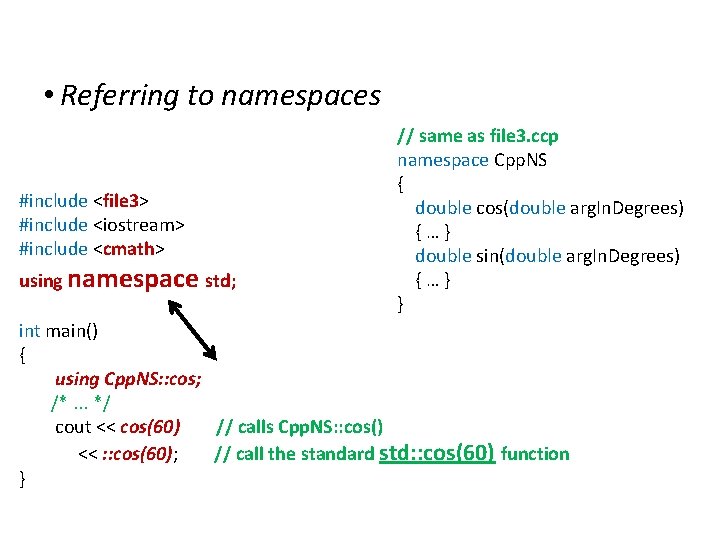
Namespaces • Referring to namespaces #include <file 3> #include <iostream> #include <cmath> using namespace std; // same as file 3. ccp namespace Cpp. NS { double cos(double arg. In. Degrees) { … } double sin(double arg. In. Degrees) { … } } int main() { using Cpp. NS: : cos; /*. . . */ cout << cos(60) // calls Cpp. NS: : cos() << : : cos(60); // call the standard std: : cos(60) function }
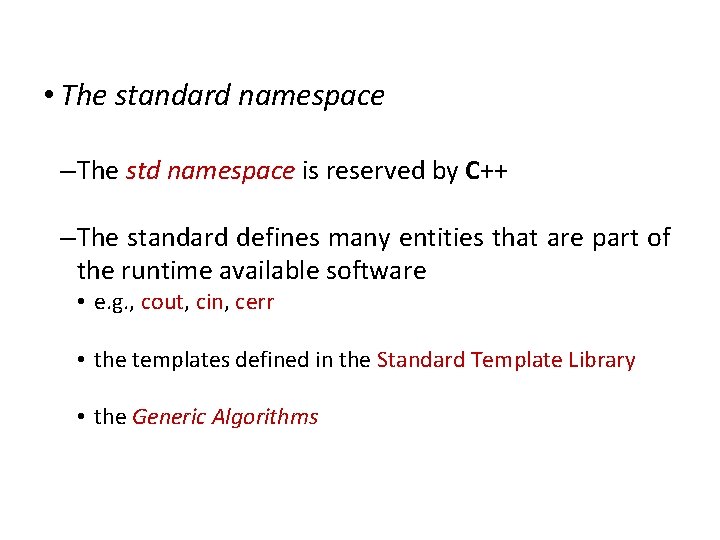
Namespaces • The standard namespace – The std namespace is reserved by C++ – The standard defines many entities that are part of the runtime available software • e. g. , cout, cin, cerr • the templates defined in the Standard Template Library • the Generic Algorithms
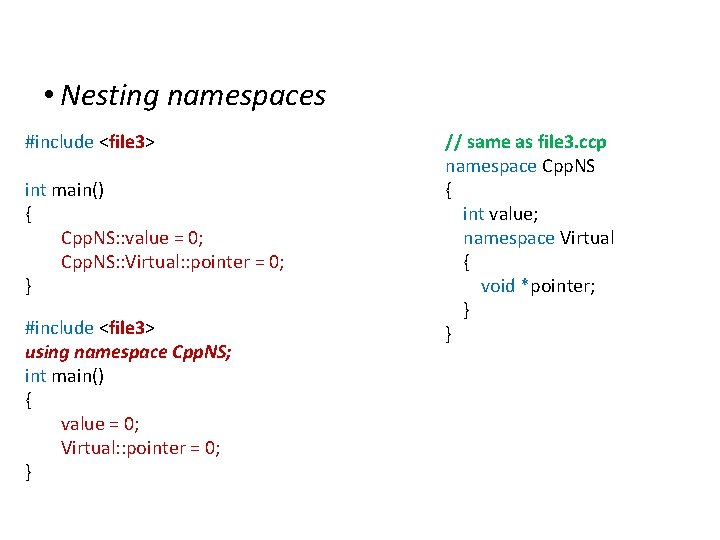
Namespaces • Nesting namespaces #include <file 3> int main() { Cpp. NS: : value = 0; Cpp. NS: : Virtual: : pointer = 0; } #include <file 3> using namespace Cpp. NS; int main() { value = 0; Virtual: : pointer = 0; } // same as file 3. ccp namespace Cpp. NS { int value; namespace Virtual { void *pointer; } }
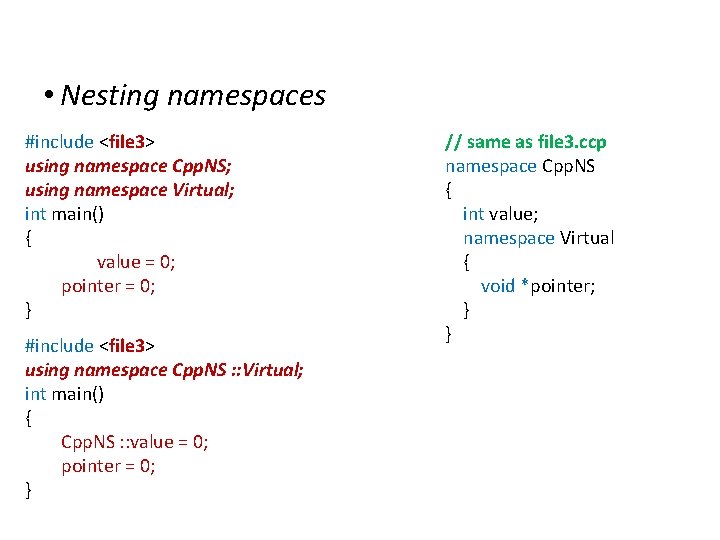
Namespaces • Nesting namespaces #include <file 3> using namespace Cpp. NS; using namespace Virtual; int main() { value = 0; pointer = 0; } #include <file 3> using namespace Cpp. NS : : Virtual; int main() { Cpp. NS : : value = 0; pointer = 0; } // same as file 3. ccp namespace Cpp. NS { int value; namespace Virtual { void *pointer; } }
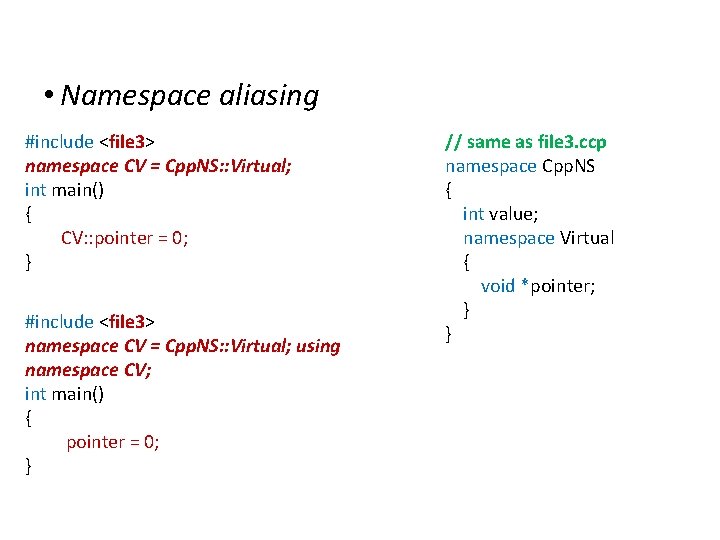
Namespaces • Namespace aliasing #include <file 3> namespace CV = Cpp. NS: : Virtual; int main() { CV: : pointer = 0; } #include <file 3> namespace CV = Cpp. NS: : Virtual; using namespace CV; int main() { pointer = 0; } // same as file 3. ccp namespace Cpp. NS { int value; namespace Virtual { void *pointer; } }
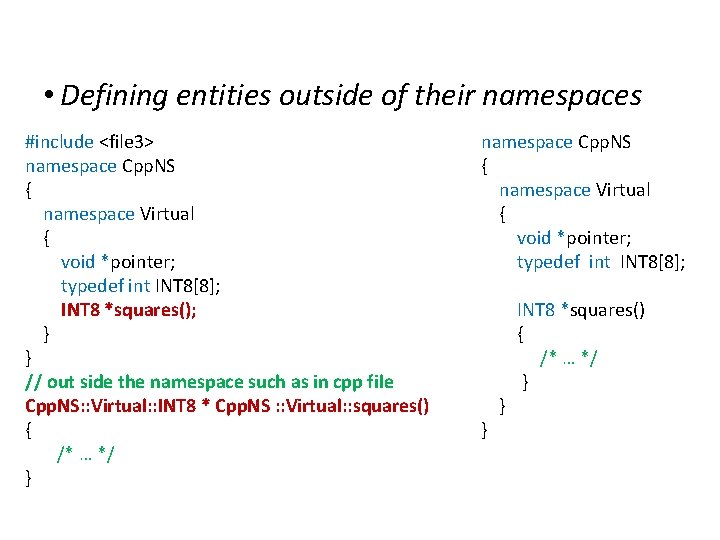
Namespaces • Defining entities outside of their namespaces #include <file 3> namespace Cpp. NS { namespace Virtual { void *pointer; typedef int INT 8[8]; INT 8 *squares(); } } // out side the namespace such as in cpp file Cpp. NS: : Virtual: : INT 8 * Cpp. NS : : Virtual: : squares() { /* … */ } namespace Cpp. NS { namespace Virtual { void *pointer; typedef int INT 8[8]; INT 8 *squares() { /* … */ } } }
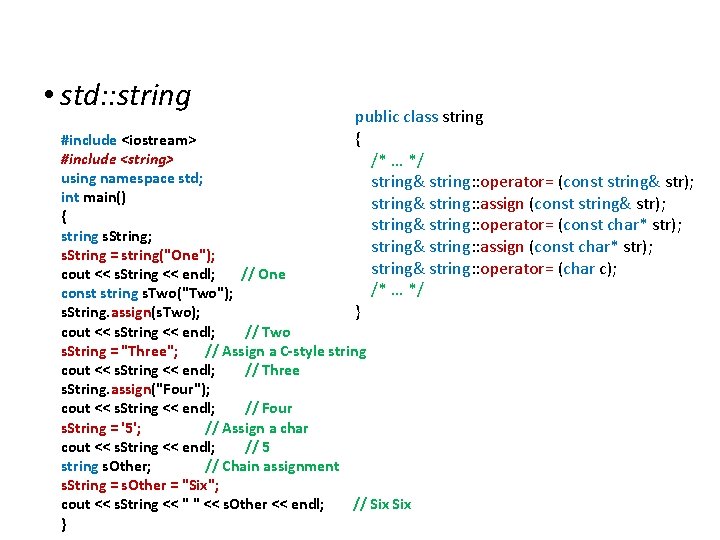
String • std: : string public class string { /* … */ string& string: : operator= (const string& str); string& string: : assign (const string& str); string& string: : operator= (const char* str); string& string: : assign (const char* str); string& string: : operator= (char c); /* … */ } #include <iostream> #include <string> using namespace std; int main() { string s. String; s. String = string("One"); cout << s. String << endl; // One const string s. Two("Two"); s. String. assign(s. Two); cout << s. String << endl; // Two s. String = "Three"; // Assign a C-style string cout << s. String << endl; // Three s. String. assign("Four"); cout << s. String << endl; // Four s. String = '5'; // Assign a char cout << s. String << endl; // 5 string s. Other; // Chain assignment s. String = s. Other = "Six"; cout << s. String << " " << s. Other << endl; // Six }
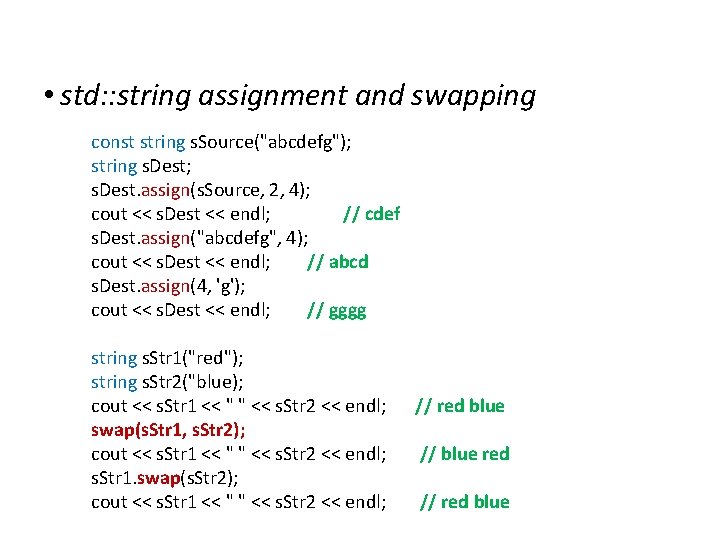
String • std: : string assignment and swapping const string s. Source("abcdefg"); string s. Dest; s. Dest. assign(s. Source, 2, 4); cout << s. Dest << endl; // cdef s. Dest. assign("abcdefg", 4); cout << s. Dest << endl; // abcd s. Dest. assign(4, 'g'); cout << s. Dest << endl; // gggg string s. Str 1("red"); string s. Str 2("blue); cout << s. Str 1 << " " << s. Str 2 << endl; swap(s. Str 1, s. Str 2); cout << s. Str 1 << " " << s. Str 2 << endl; s. Str 1. swap(s. Str 2); cout << s. Str 1 << " " << s. Str 2 << endl; // red blue // blue red // red blue
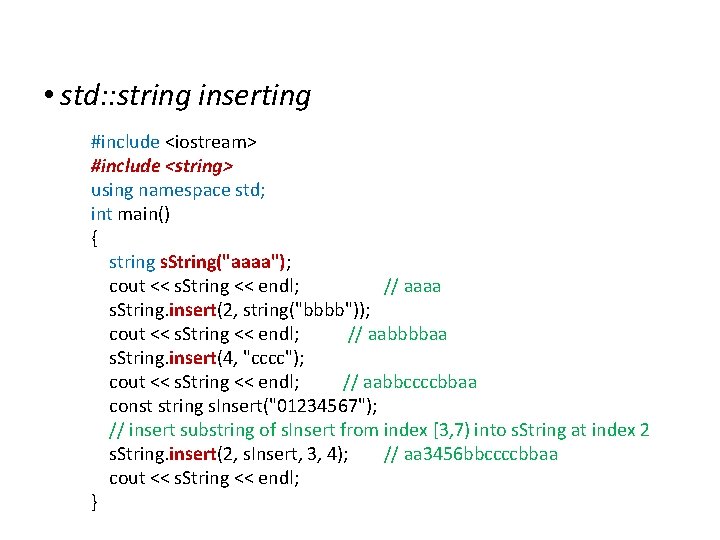
String • std: : string inserting #include <iostream> #include <string> using namespace std; int main() { string s. String("aaaa"); cout << s. String << endl; // aaaa s. String. insert(2, string("bbbb")); cout << s. String << endl; // aabbbbaa s. String. insert(4, "cccc"); cout << s. String << endl; // aabbccccbbaa const string s. Insert("01234567"); // insert substring of s. Insert from index [3, 7) into s. String at index 2 s. String. insert(2, s. Insert, 3, 4); // aa 3456 bbccccbbaa cout << s. String << endl; }
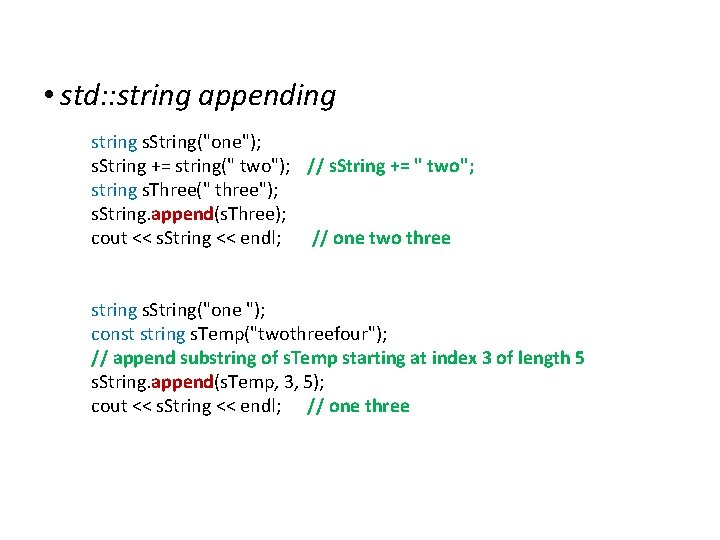
String • std: : string appending string s. String("one"); s. String += string(" two"); // s. String += " two"; string s. Three(" three"); s. String. append(s. Three); cout << s. String << endl; // one two three string s. String("one "); const string s. Temp("twothreefour"); // append substring of s. Temp starting at index 3 of length 5 s. String. append(s. Temp, 3, 5); cout << s. String << endl; // one three
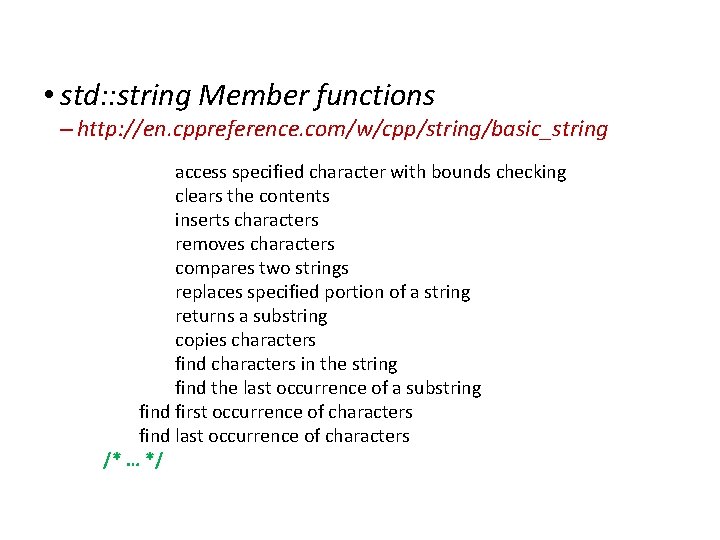
String • std: : string Member functions – http: //en. cppreference. com/w/cpp/string/basic_string access specified character with bounds checking clears the contents inserts characters removes characters compares two strings replaces specified portion of a string returns a substring copies characters find characters in the string find the last occurrence of a substring find first occurrence of characters find last occurrence of characters /* … */
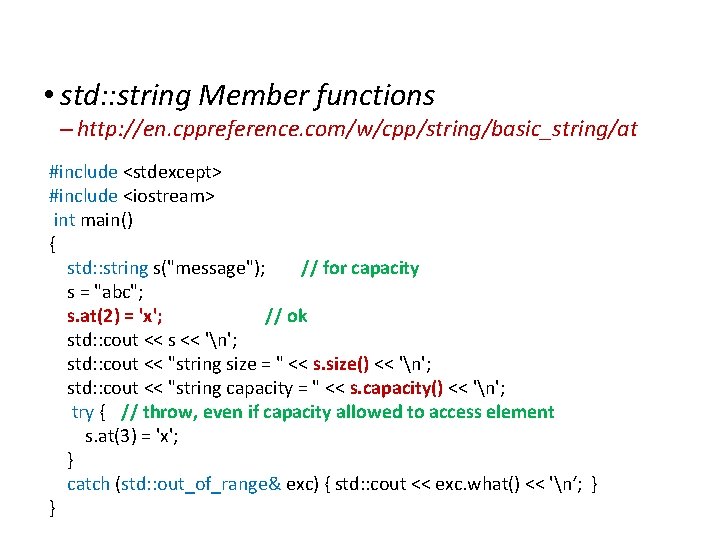
String • std: : string Member functions – http: //en. cppreference. com/w/cpp/string/basic_string/at #include <stdexcept> #include <iostream> int main() { std: : string s("message"); // for capacity abx s = "abc"; string size = 3 s. at(2) = 'x'; // ok string capacity = 7 std: : cout << s << 'n'; basic_string: : at std: : cout << "string size = " << s. size() << 'n'; std: : cout << "string capacity = " << s. capacity() << 'n'; try { // throw, even if capacity allowed to access element s. at(3) = 'x'; } catch (std: : out_of_range& exc) { std: : cout << exc. what() << 'n‘; } }
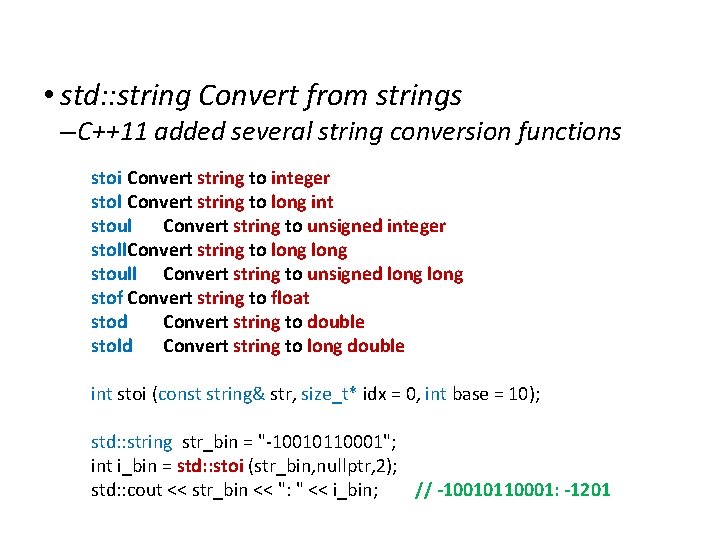
String • std: : string Convert from strings – C++11 added several string conversion functions stoi Convert string to integer stol Convert string to long int stoul Convert string to unsigned integer stoll. Convert string to long stoull Convert string to unsigned long stof Convert string to float stod Convert string to double stold Convert string to long double int stoi (const string& str, size_t* idx = 0, int base = 10); std: : string str_bin = "-10010110001"; int i_bin = std: : stoi (str_bin, nullptr, 2); std: : cout << str_bin << ": " << i_bin; // -10010110001: -1201
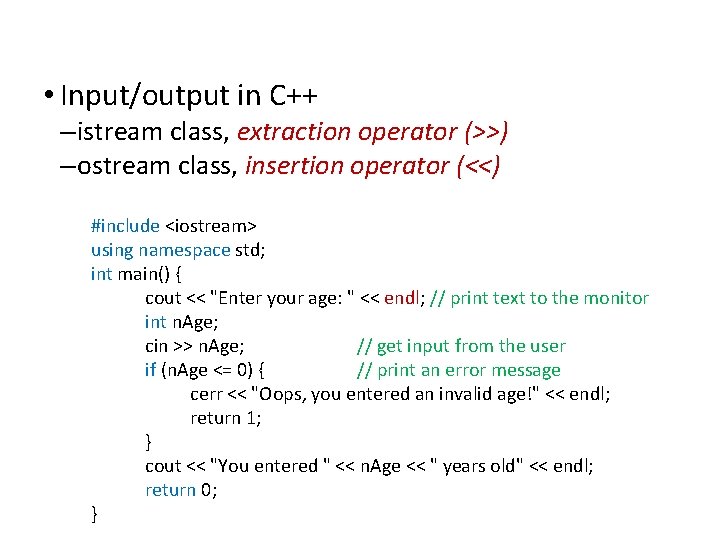
IOStreams • Input/output in C++ – istream class, extraction operator (>>) – ostream class, insertion operator (<<) #include <iostream> using namespace std; int main() { cout << "Enter your age: " << endl; // print text to the monitor int n. Age; cin >> n. Age; // get input from the user if (n. Age <= 0) { // print an error message cerr << "Oops, you entered an invalid age!" << endl; return 1; } cout << "You entered " << n. Age << " years old" << endl; return 0; }
![IOStreams extraction operator char buf10 cin buf what happens if IOStreams • extraction operator (>>) char buf[10]; cin >> buf; // what happens if](https://slidetodoc.com/presentation_image_h/9338e8fb76f47ecbf126ab6c6b08158c/image-51.jpg)
IOStreams • extraction operator (>>) char buf[10]; cin >> buf; // what happens if the user enters 18 characters? /* * C++ provides a manipulator known as setw (in the iomanip. h header) that can * be used to limit the number of characters read in from a stream. */ #include <iomanip. h> char buf[10]; cin >> setw(10) >> buf; /* Any remaining characters will be left in the stream until the next extraction */
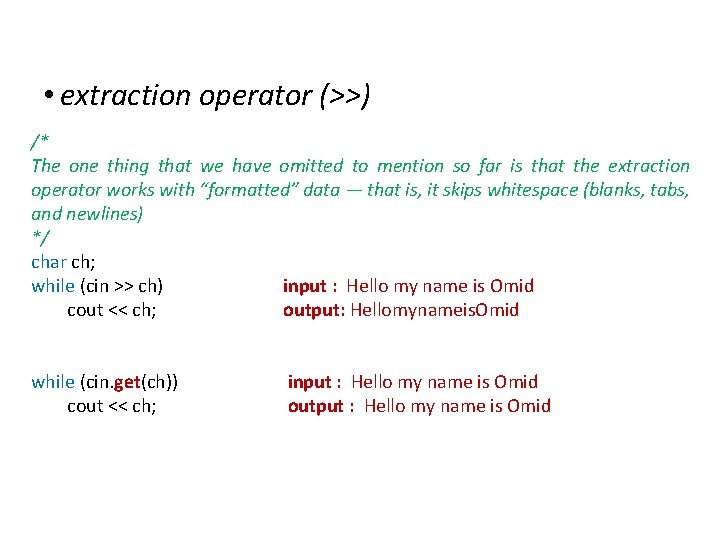
IOStreams • extraction operator (>>) /* The one thing that we have omitted to mention so far is that the extraction operator works with “formatted” data — that is, it skips whitespace (blanks, tabs, and newlines) */ char ch; while (cin >> ch) input : Hello my name is Omid cout << ch; output: Hellomynameis. Omid while (cin. get(ch)) cout << ch; input : Hello my name is Omid output : Hello my name is Omid
![IOStreams extraction operator char str Buf11 only read the first 10 IOStreams • extraction operator (>>) char str. Buf[11]; // only read the first 10](https://slidetodoc.com/presentation_image_h/9338e8fb76f47ecbf126ab6c6b08158c/image-53.jpg)
IOStreams • extraction operator (>>) char str. Buf[11]; // only read the first 10 characters cin. get(str. Buf, 11); input : Hello my name is Omid cout << str. Buf << endl; output : Hello my n /* One important thing to note about get() is that it does not read in a newline character! This can cause some unexpected results */ char str. Buf[11]; cin. get(str. Buf, 11); input : Hello! � cout << str. Buf << endl; output : Hello cin. get(str. Buf, 11); cout << str. Buf << endl; /* Consequently, getline() like get() but reads the newline as well */
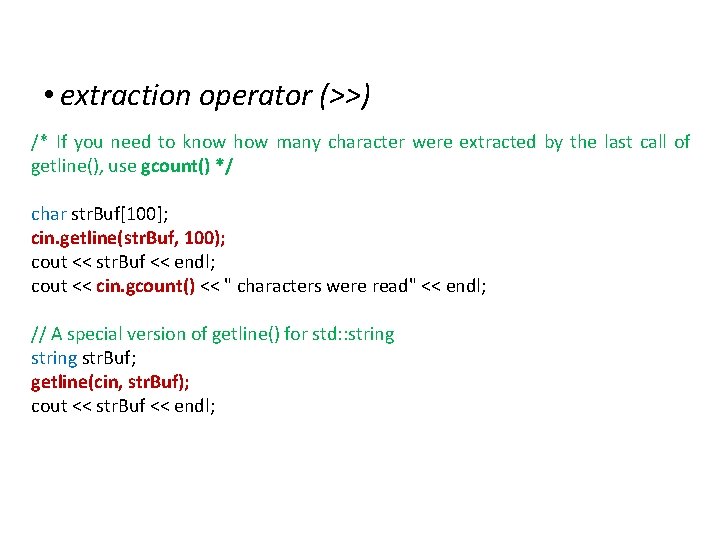
IOStreams • extraction operator (>>) /* If you need to know how many character were extracted by the last call of getline(), use gcount() */ char str. Buf[100]; cin. getline(str. Buf, 100); cout << str. Buf << endl; cout << cin. gcount() << " characters were read" << endl; // A special version of getline() for std: : string str. Buf; getline(cin, str. Buf); cout << str. Buf << endl;
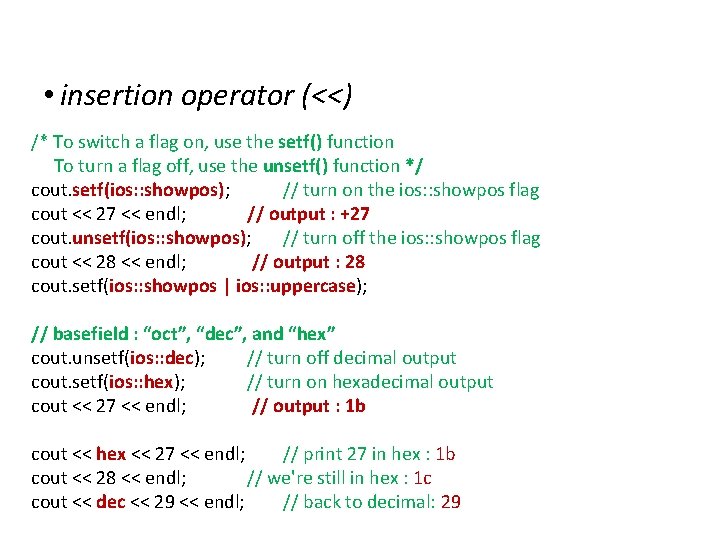
IOStreams • insertion operator (<<) /* To switch a flag on, use the setf() function To turn a flag off, use the unsetf() function */ cout. setf(ios: : showpos); // turn on the ios: : showpos flag cout << 27 << endl; // output : +27 cout. unsetf(ios: : showpos); // turn off the ios: : showpos flag cout << 28 << endl; // output : 28 cout. setf(ios: : showpos | ios: : uppercase); // basefield : “oct”, “dec”, and “hex” cout. unsetf(ios: : dec); // turn off decimal output cout. setf(ios: : hex); // turn on hexadecimal output cout << 27 << endl; // output : 1 b cout << hex << 27 << endl; // print 27 in hex : 1 b cout << 28 << endl; // we're still in hex : 1 c cout << dec << 29 << endl; // back to decimal: 29
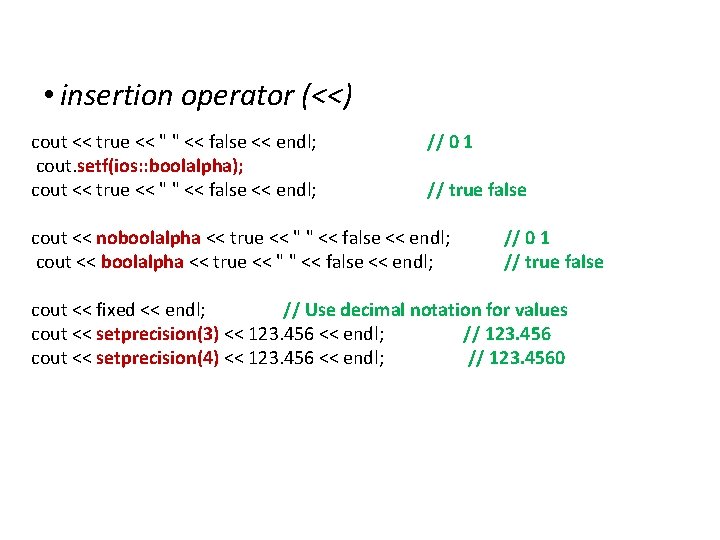
IOStreams • insertion operator (<<) cout << true << " " << false << endl; // 0 1 cout. setf(ios: : boolalpha); cout << true << " " << false << endl; // true false cout << noboolalpha << true << " " << false << endl; // 0 1 cout << boolalpha << true << " " << false << endl; // true false cout << fixed << endl; // Use decimal notation for values cout << setprecision(3) << 123. 456 << endl; // 123. 456 cout << setprecision(4) << 123. 456 << endl; // 123. 4560
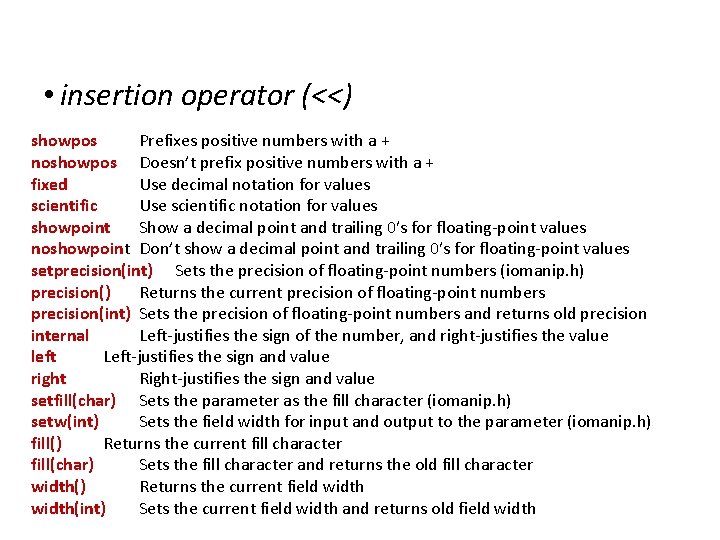
IOStreams • insertion operator (<<) showpos Prefixes positive numbers with a + noshowpos Doesn’t prefix positive numbers with a + fixed Use decimal notation for values scientific Use scientific notation for values showpoint Show a decimal point and trailing 0′s for floating-point values noshowpoint Don’t show a decimal point and trailing 0′s for floating-point values setprecision(int) Sets the precision of floating-point numbers (iomanip. h) precision() Returns the current precision of floating-point numbers precision(int) Sets the precision of floating-point numbers and returns old precision internal Left-justifies the sign of the number, and right-justifies the value left Left-justifies the sign and value right Right-justifies the sign and value setfill(char) Sets the parameter as the fill character (iomanip. h) setw(int) Sets the field width for input and output to the parameter (iomanip. h) fill() Returns the current fill character fill(char) Sets the fill character and returns the old fill character width() Returns the current field width(int) Sets the current field width and returns old field width
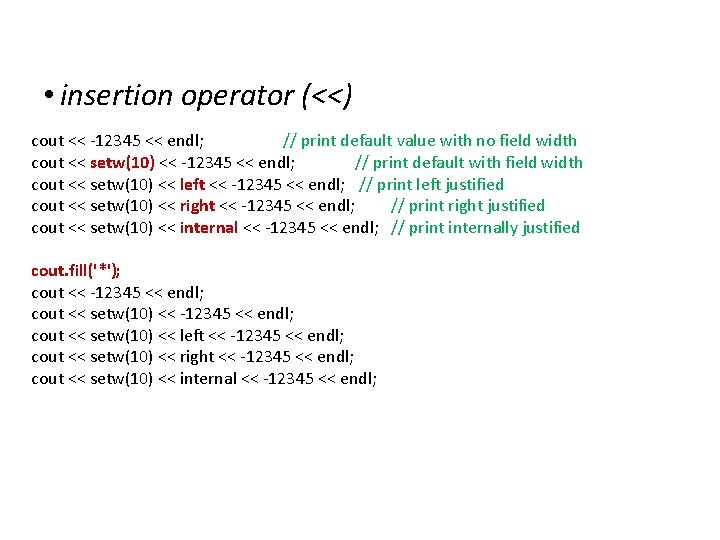
IOStreams • insertion operator (<<) cout << -12345 << endl; // print default value with no field width cout << setw(10) << -12345 << endl; // print default with field width cout << setw(10) << left << -12345 << endl; // print left justified cout << setw(10) << right << -12345 << endl; // print right justified cout << setw(10) << internal << -12345 << endl; // print internally justified cout. fill('*'); cout << -12345 << endl; cout << setw(10) << left << -12345 << endl; cout << setw(10) << right << -12345 << endl; cout << setw(10) << internal << -12345 << endl; -12345 - 12345 -12345 ****-12345****-12345 -****12345
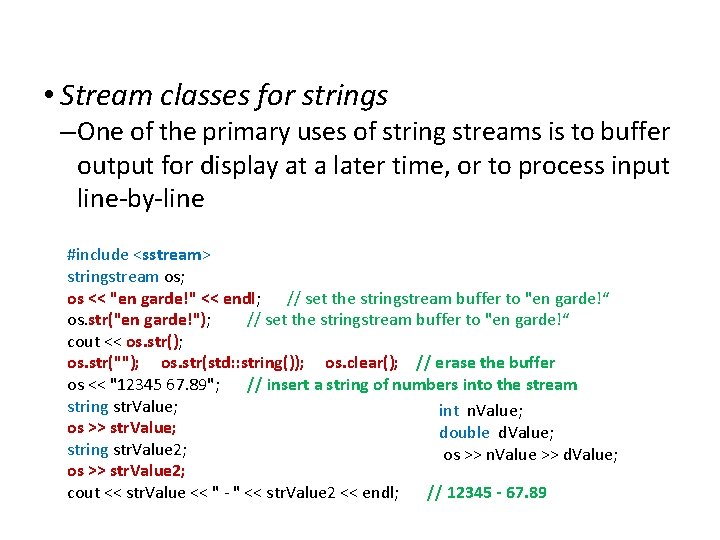
IOStreams • Stream classes for strings – One of the primary uses of string streams is to buffer output for display at a later time, or to process input line-by-line #include <sstream> stringstream os; os << "en garde!" << endl; // set the stringstream buffer to "en garde!“ os. str("en garde!"); // set the stringstream buffer to "en garde!“ cout << os. str(); os. str(""); os. str(std: : string()); os. clear(); // erase the buffer os << "12345 67. 89"; // insert a string of numbers into the stream string str. Value; int n. Value; os >> str. Value; double d. Value; string str. Value 2; os >> n. Value >> d. Value; os >> str. Value 2; cout << str. Value << " - " << str. Value 2 << endl; // 12345 - 67. 89
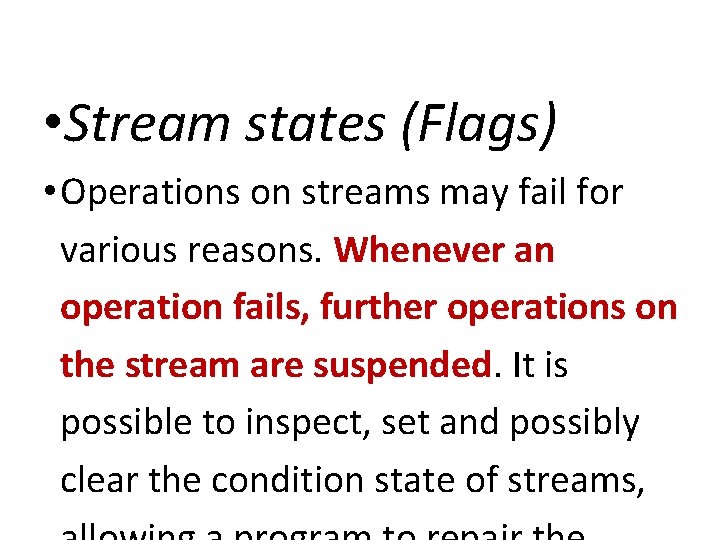
IOStreams • Stream states (Flags) • Operations on streams may fail for various reasons. Whenever an operation fails, further operations on the stream are suspended. It is possible to inspect, set and possibly clear the condition state of streams,
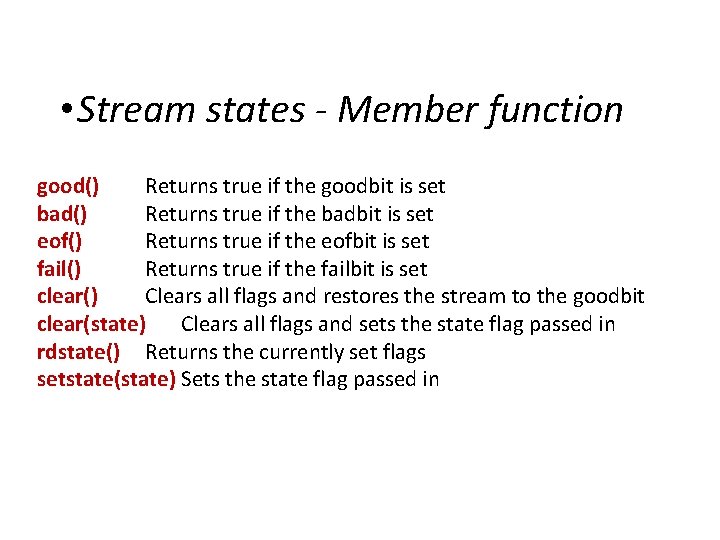
IOStreams • Stream states - Member function good() Returns true if the goodbit is set bad() Returns true if the badbit is set eof() Returns true if the eofbit is set fail() Returns true if the failbit is set clear() Clears all flags and restores the stream to the goodbit clear(state) Clears all flags and sets the state flag passed in rdstate() Returns the currently set flags setstate(state) Sets the state flag passed in
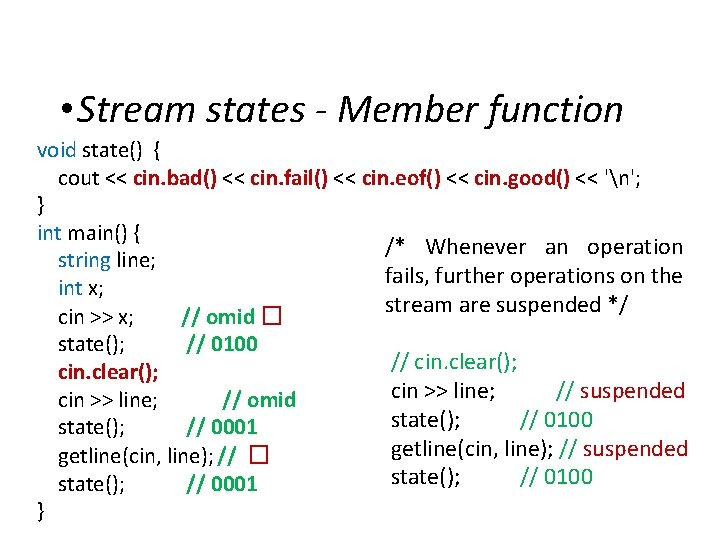
IOStreams • Stream states - Member function void state() { cout << cin. bad() << cin. fail() << cin. eof() << cin. good() << 'n'; } int main() { /* Whenever an operation string line; fails, further operations on the int x; stream are suspended */ cin >> x; // omid � state(); // 0100 // cin. clear(); cin >> line; // suspended cin >> line; // omid state(); // 0100 state(); // 0001 getline(cin, line); // suspended getline(cin, line); // � state(); // 0100 state(); // 0001 }
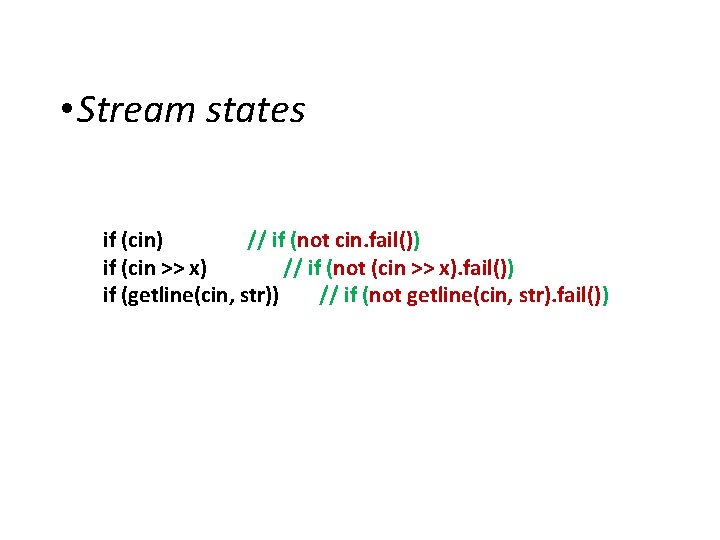
IOStreams • Stream states if (cin) // if (not cin. fail()) if (cin >> x) // if (not (cin >> x). fail()) if (getline(cin, str)) // if (not getline(cin, str). fail())
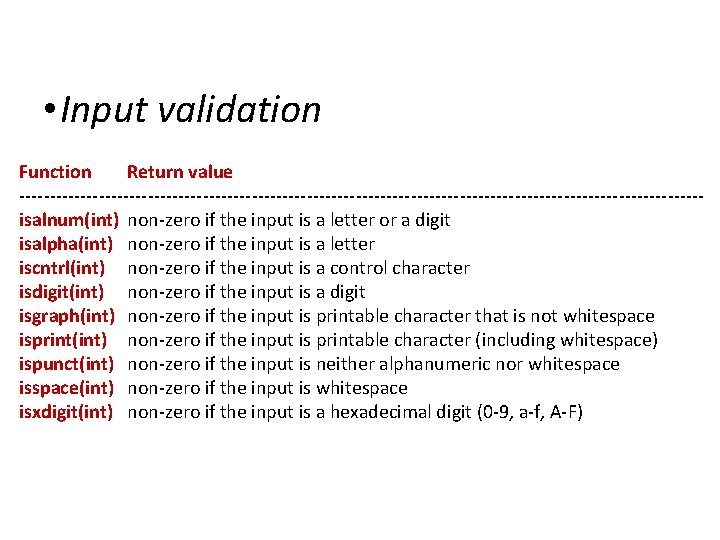
IOStreams • Input validation Function Return value --------------------------------------------------------isalnum(int) non-zero if the input is a letter or a digit isalpha(int) non-zero if the input is a letter iscntrl(int) non-zero if the input is a control character isdigit(int) non-zero if the input is a digit isgraph(int) non-zero if the input is printable character that is not whitespace isprint(int) non-zero if the input is printable character (including whitespace) ispunct(int) non-zero if the input is neither alphanumeric nor whitespace isspace(int) non-zero if the input is whitespace isxdigit(int) non-zero if the input is a hexadecimal digit (0 -9, a-f, A-F)
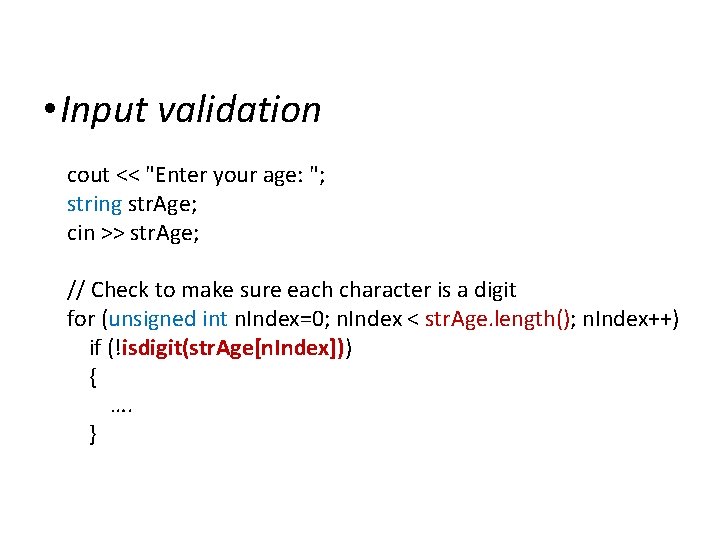
IOStreams • Input validation cout << "Enter your age: "; string str. Age; cin >> str. Age; // Check to make sure each character is a digit for (unsigned int n. Index=0; n. Index < str. Age. length(); n. Index++) if (!isdigit(str. Age[n. Index])) { …. }
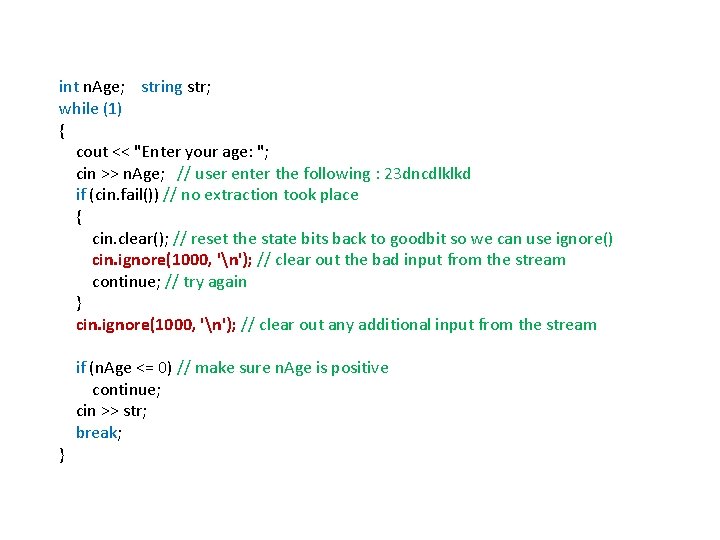
Your practice: run it int n. Age; string str; while (1) { cout << "Enter your age: "; cin >> n. Age; // user enter the following : 23 dncdlklkd if (cin. fail()) // no extraction took place { cin. clear(); // reset the state bits back to goodbit so we can use ignore() cin. ignore(1000, 'n'); // clear out the bad input from the stream continue; // try again } cin. ignore(1000, 'n'); // clear out any additional input from the stream if (n. Age <= 0) // make sure n. Age is positive continue; cin >> str; break; }
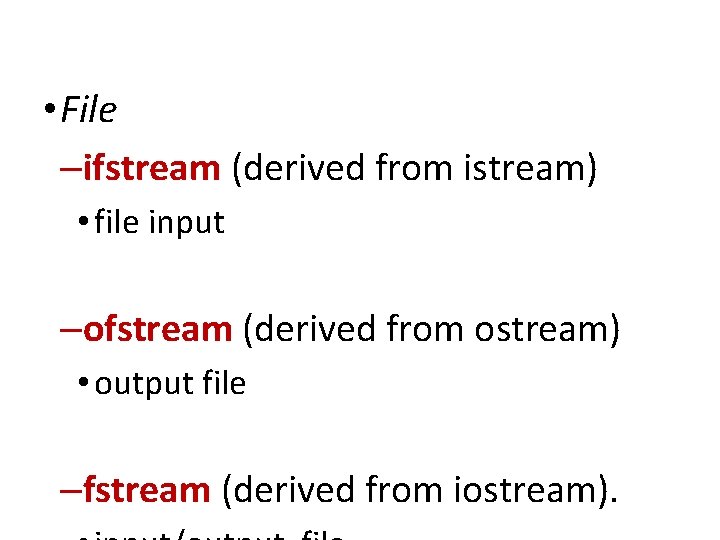
IOStreams • File –ifstream (derived from istream) • file input –ofstream (derived from ostream) • output file –fstream (derived from iostream).
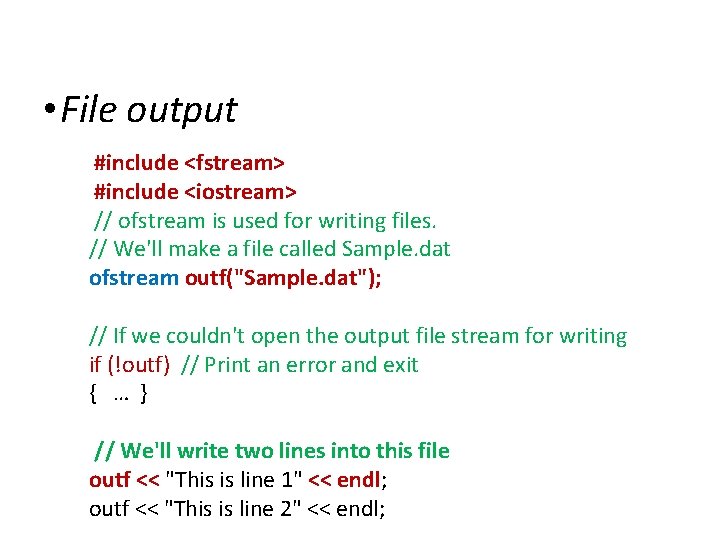
IOStreams • File output #include <fstream> #include <iostream> // ofstream is used for writing files. // We'll make a file called Sample. dat ofstream outf("Sample. dat"); // If we couldn't open the output file stream for writing if (!outf) // Print an error and exit { … } // We'll write two lines into this file outf << "This is line 1" << endl; outf << "This is line 2" << endl;
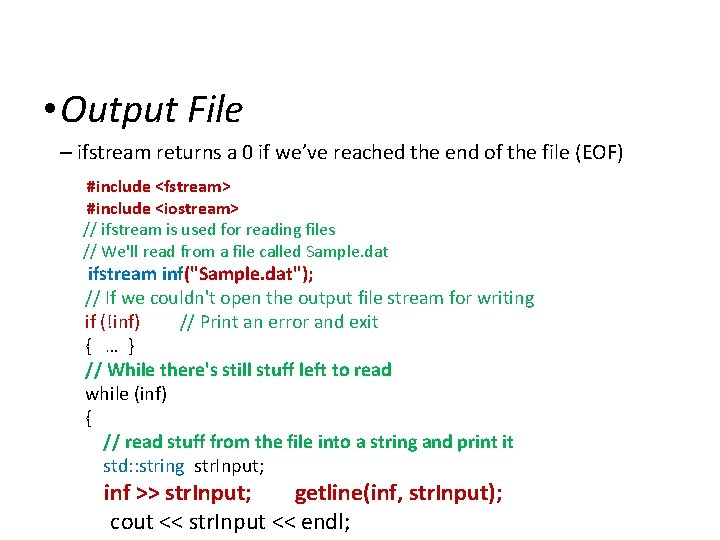
IOStreams • Output File – ifstream returns a 0 if we’ve reached the end of the file (EOF) #include <fstream> #include <iostream> // ifstream is used for reading files // We'll read from a file called Sample. dat ifstream inf("Sample. dat"); // If we couldn't open the output file stream for writing if (!inf) // Print an error and exit { … } // While there's still stuff left to read while (inf) { // read stuff from the file into a string and print it std: : string str. Input; inf >> str. Input; getline(inf, str. Input); cout << str. Input << endl;
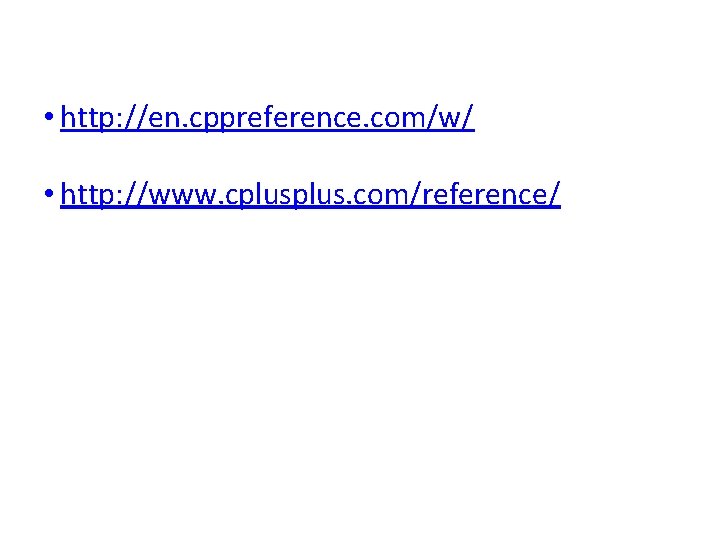
Reference • http: //en. cppreference. com/w/ • http: //www. cplus. com/reference/