Pascal CSE 4102 Prof Steven A Demurjian Computer
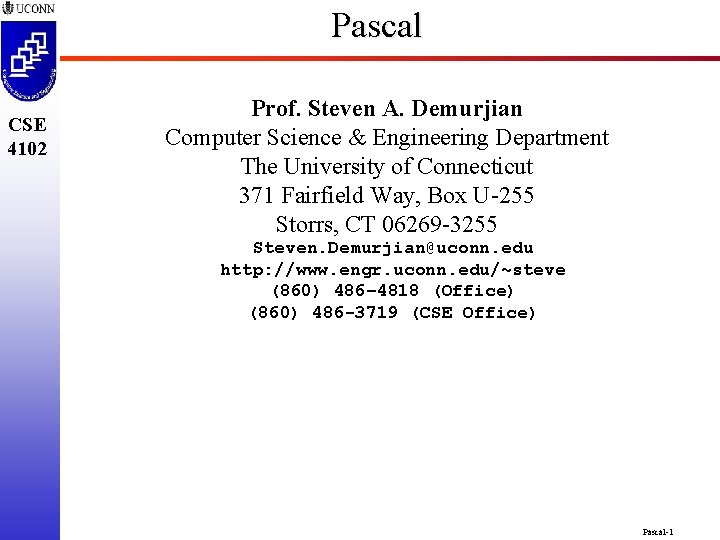
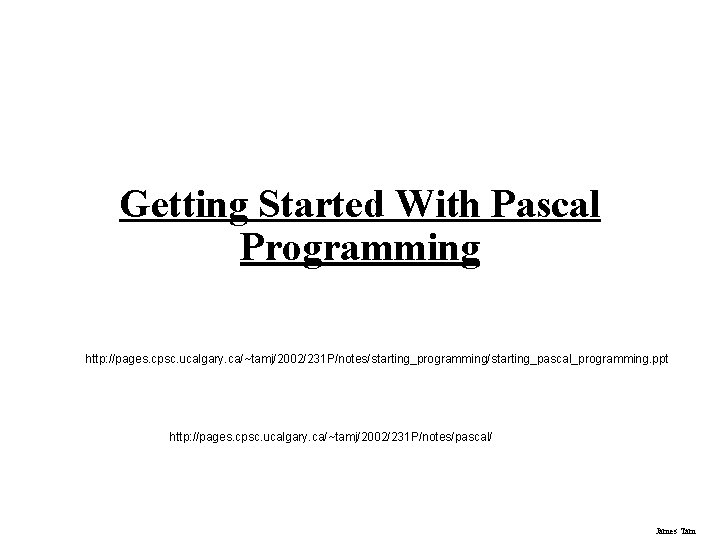
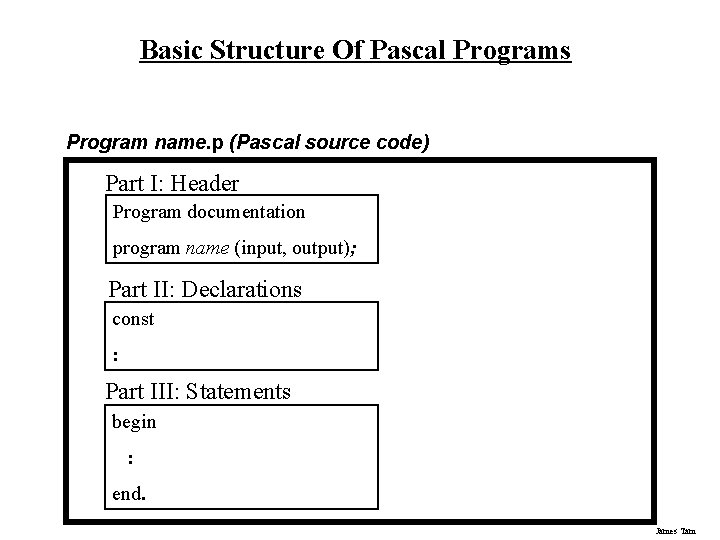
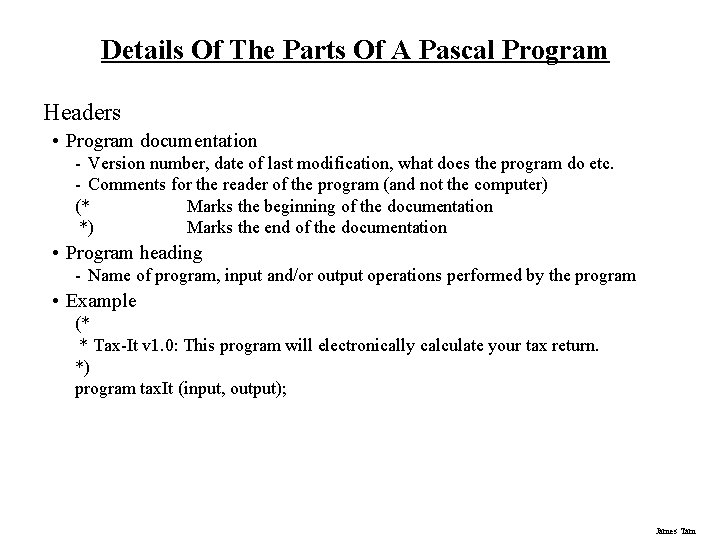
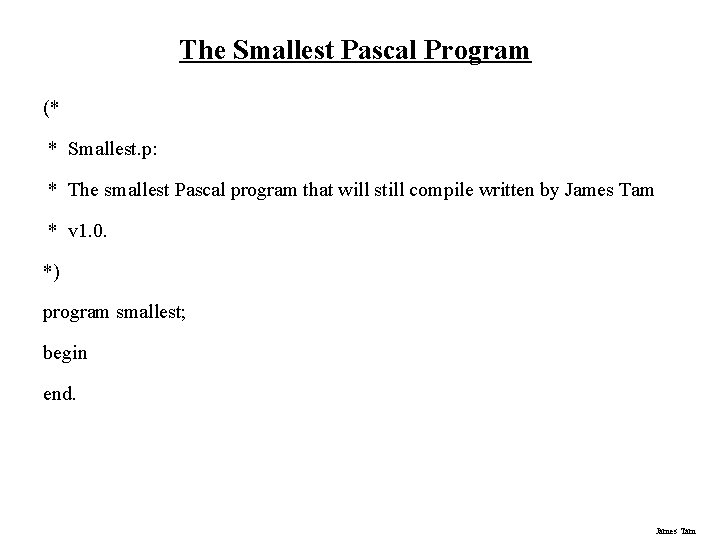
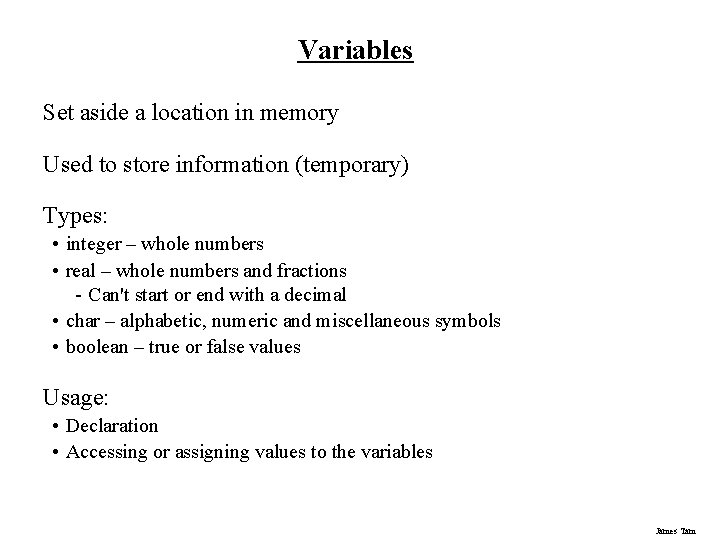
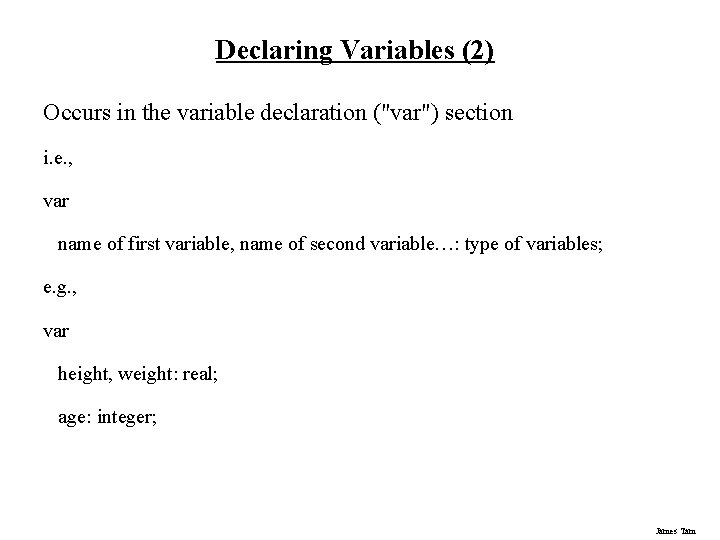
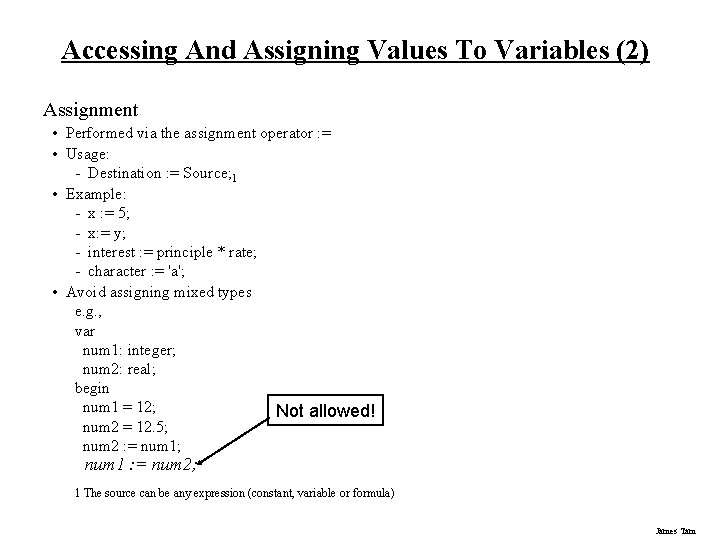
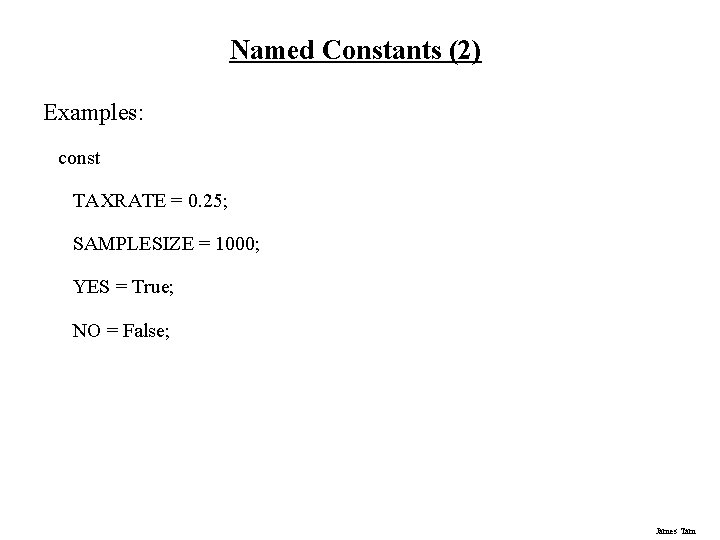
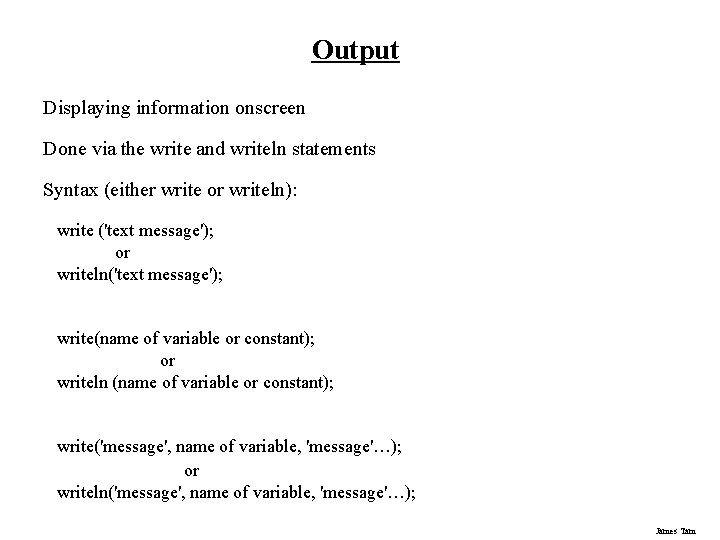
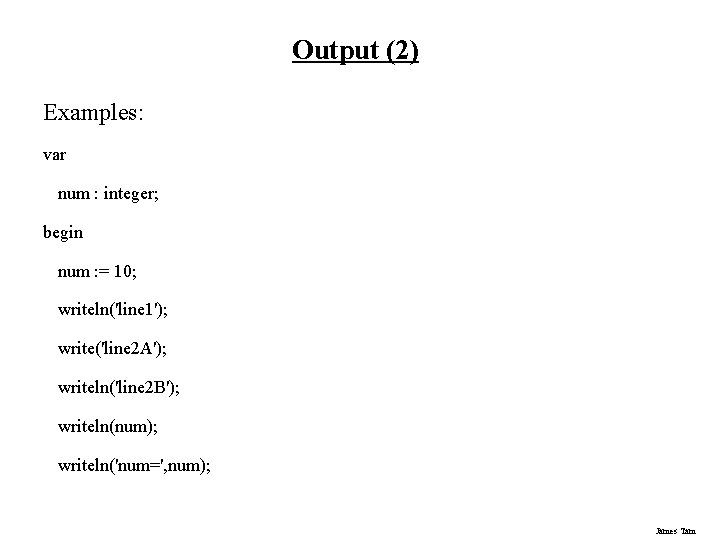
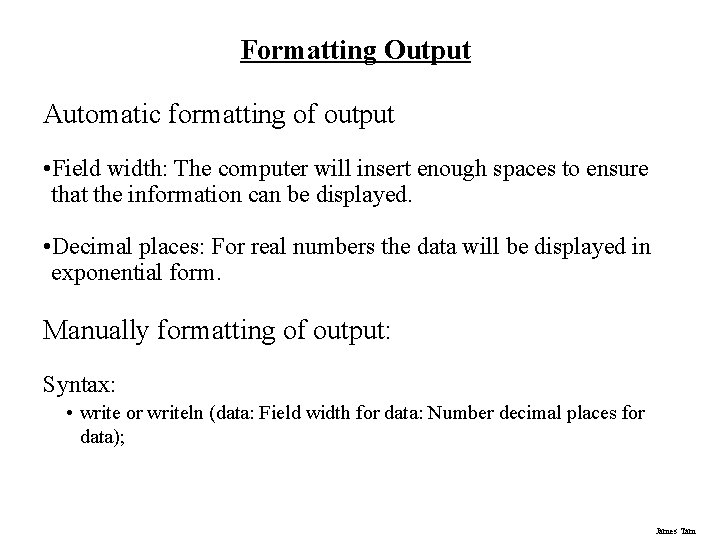
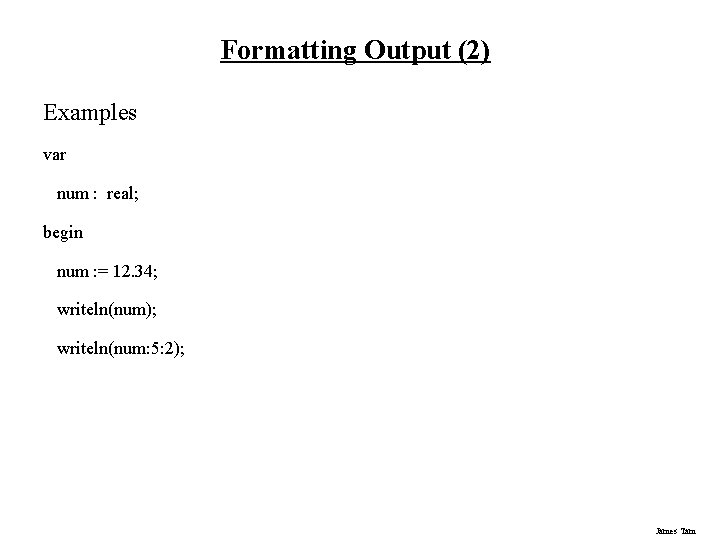
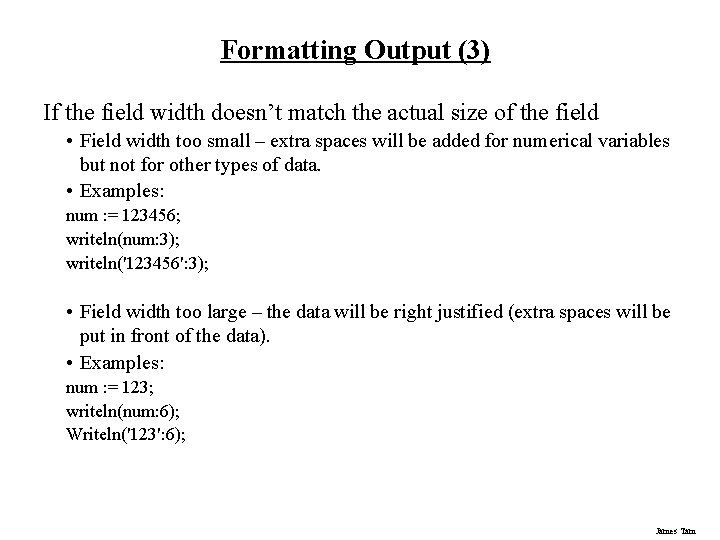
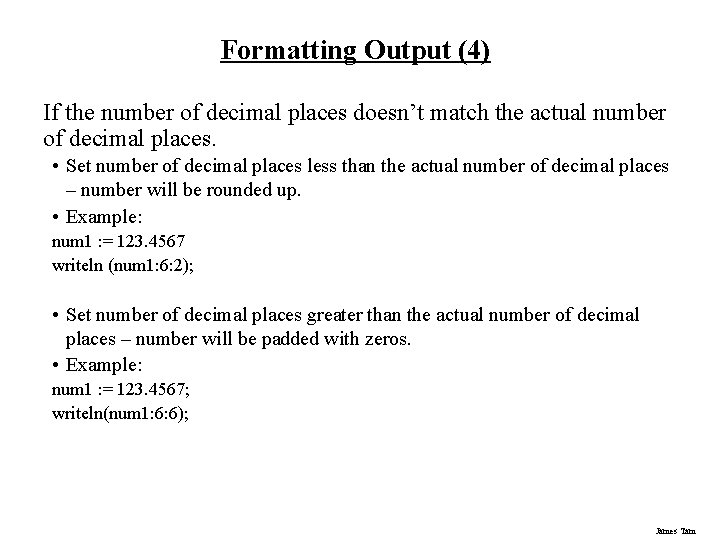
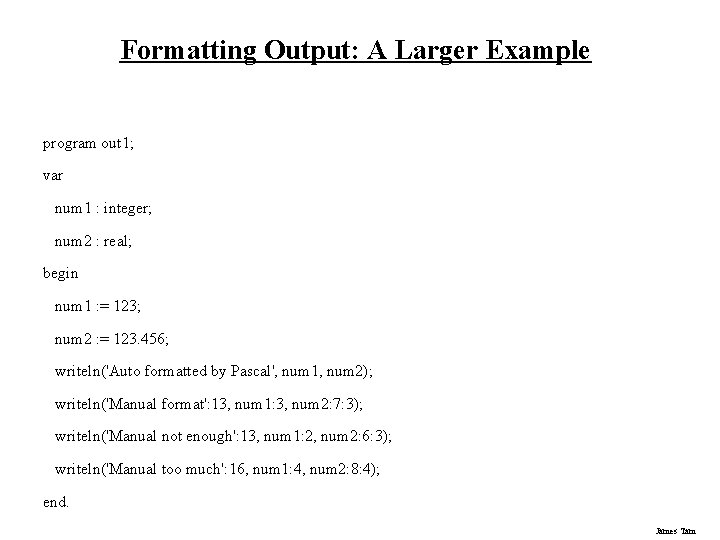
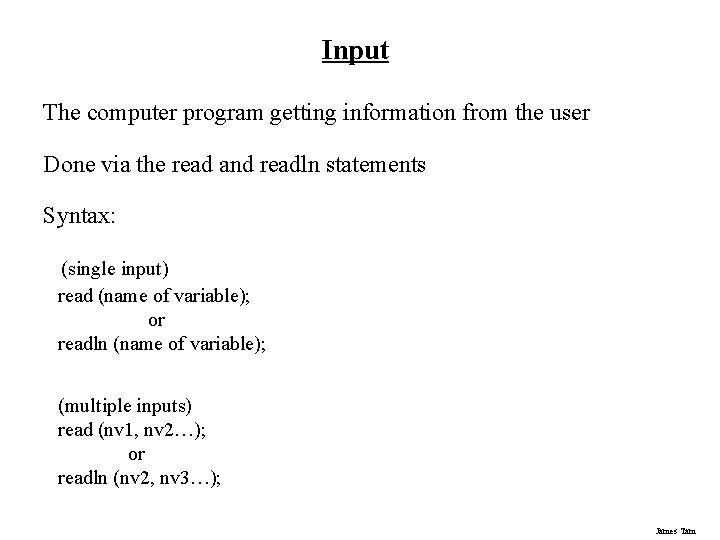
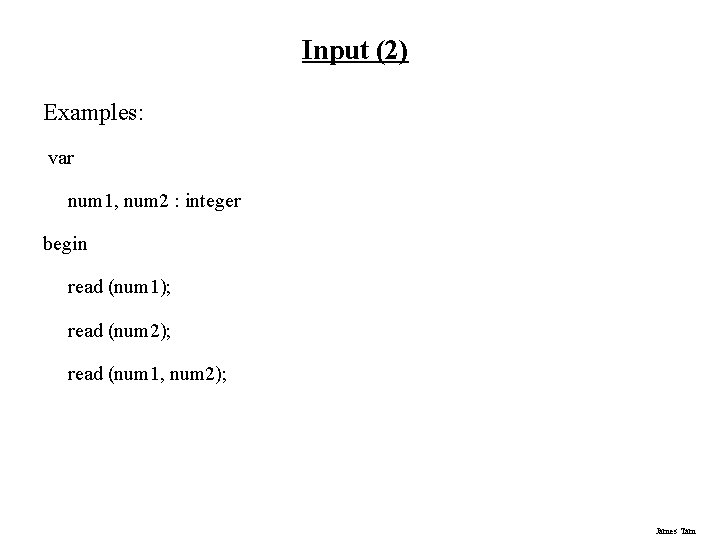
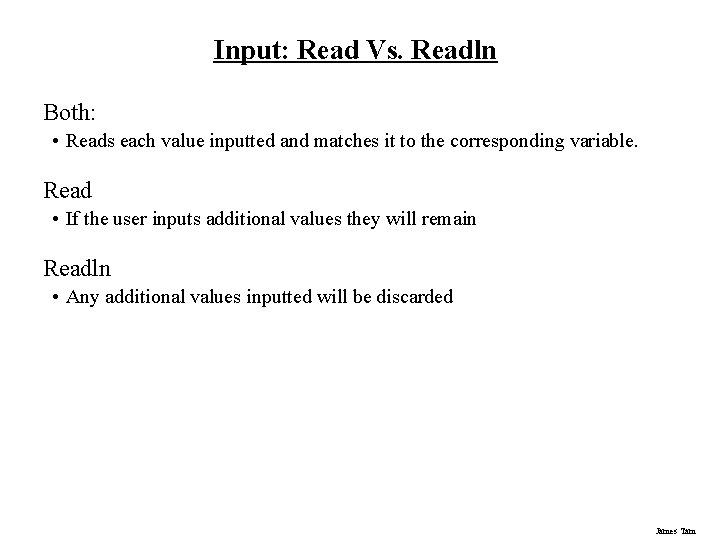
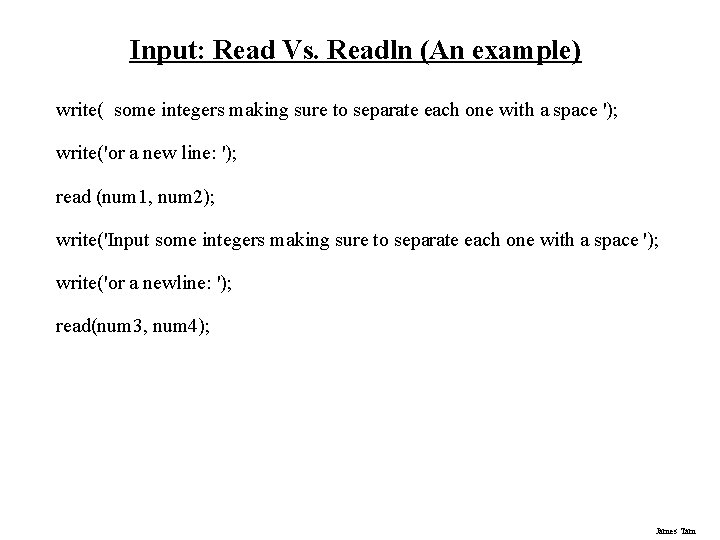
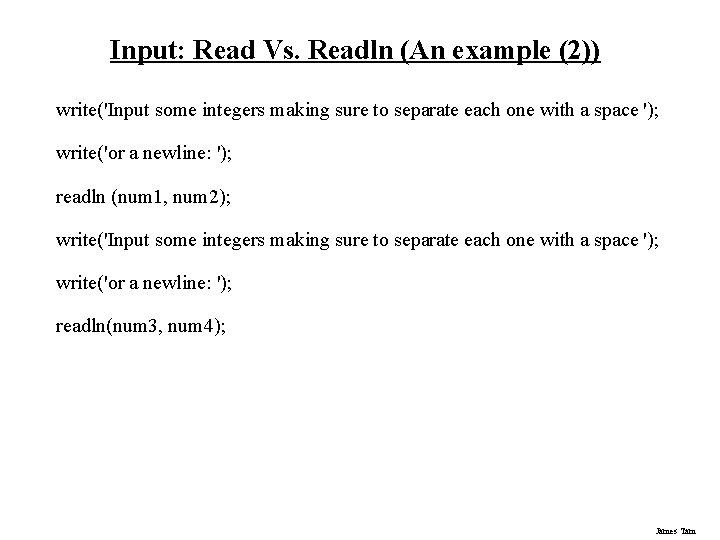
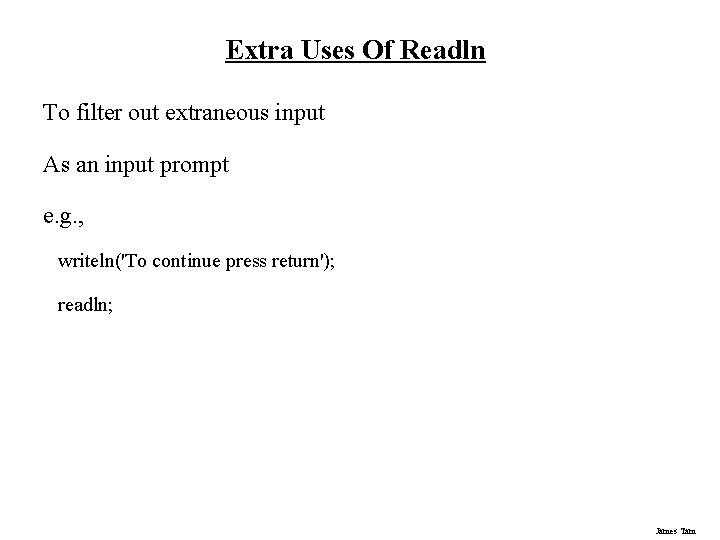
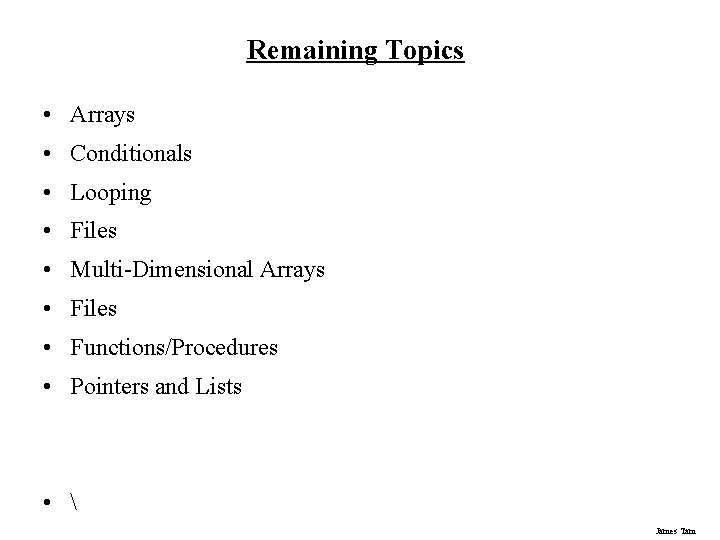
![Arrays const CLASSSIZE = 5; var class. Grades : array [1. . CLASSSIZE] of Arrays const CLASSSIZE = 5; var class. Grades : array [1. . CLASSSIZE] of](https://slidetodoc.com/presentation_image_h2/ed92b301b7f9d83e665b7d2596230111/image-24.jpg)
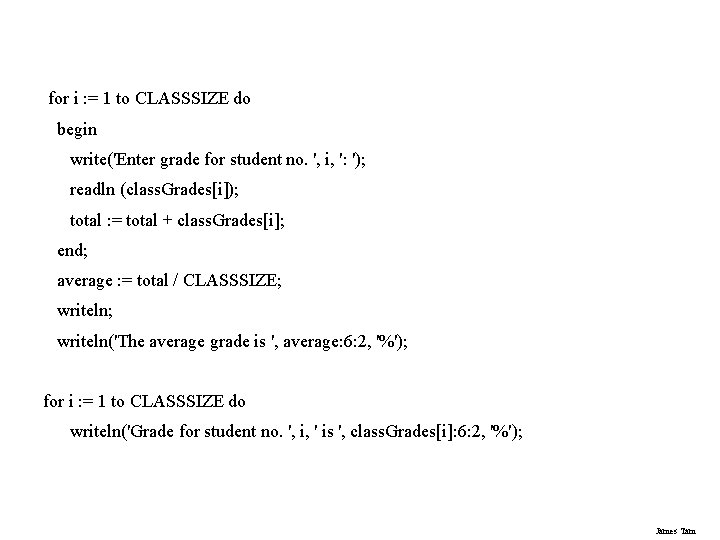
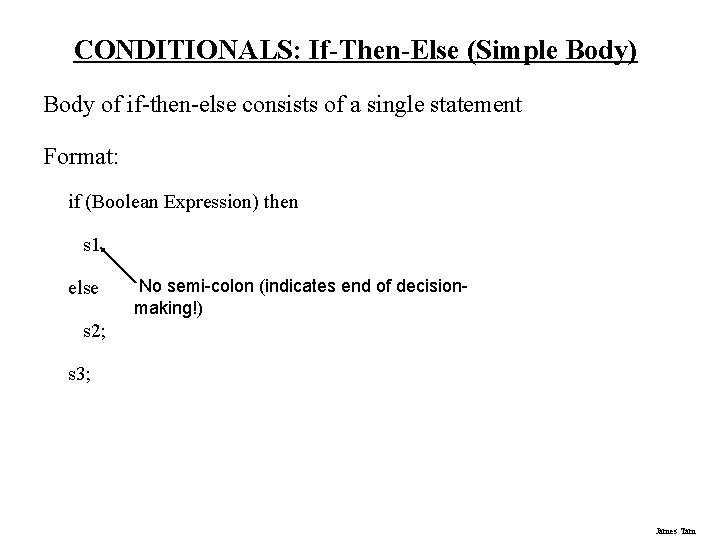
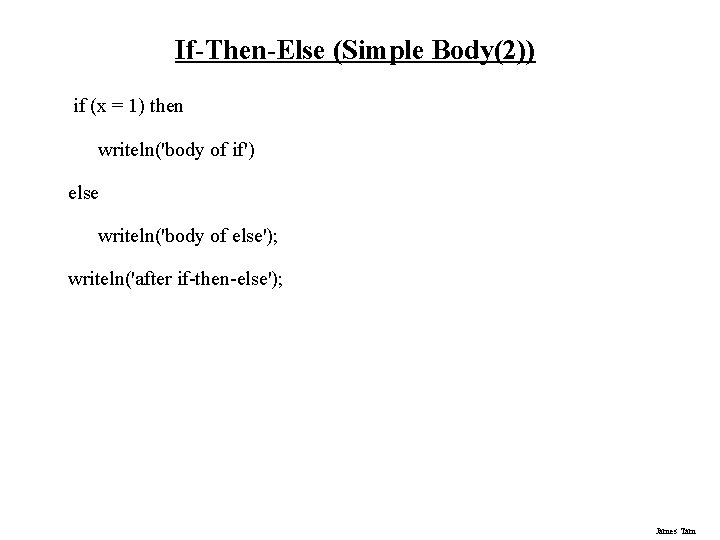
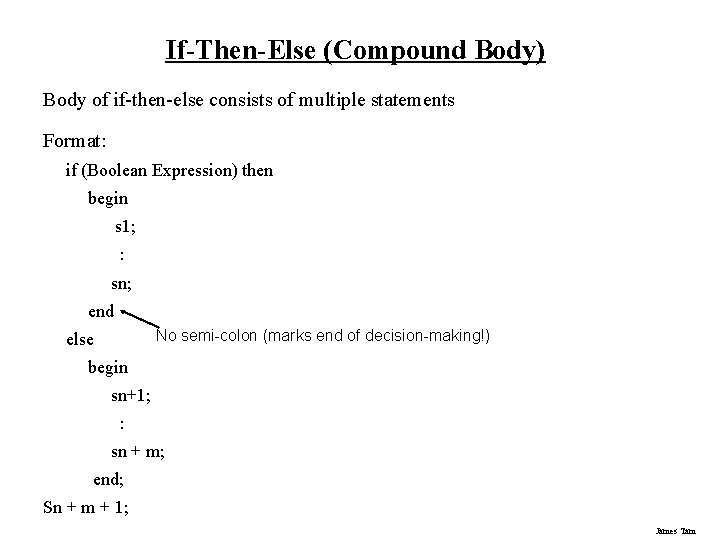
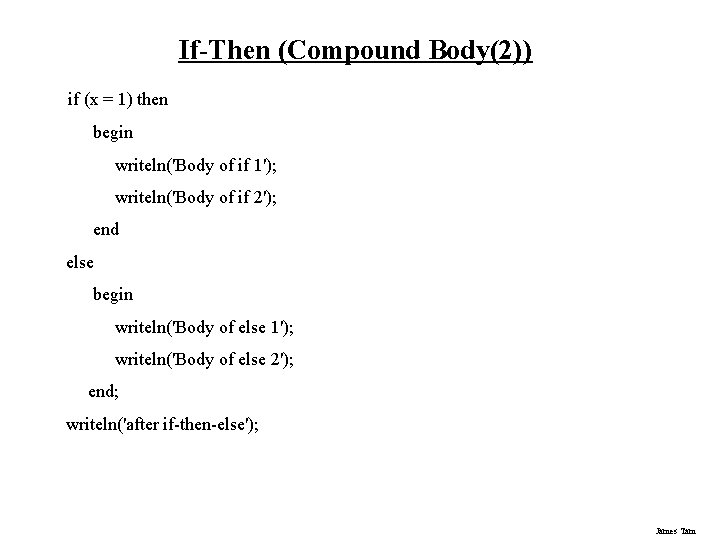
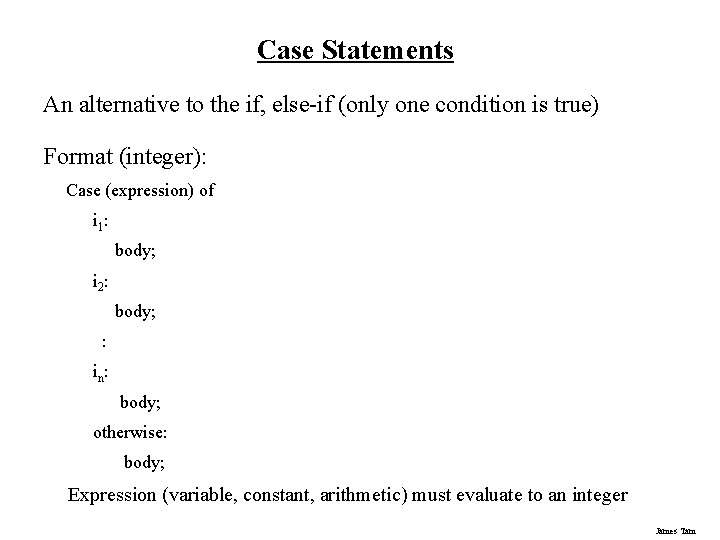
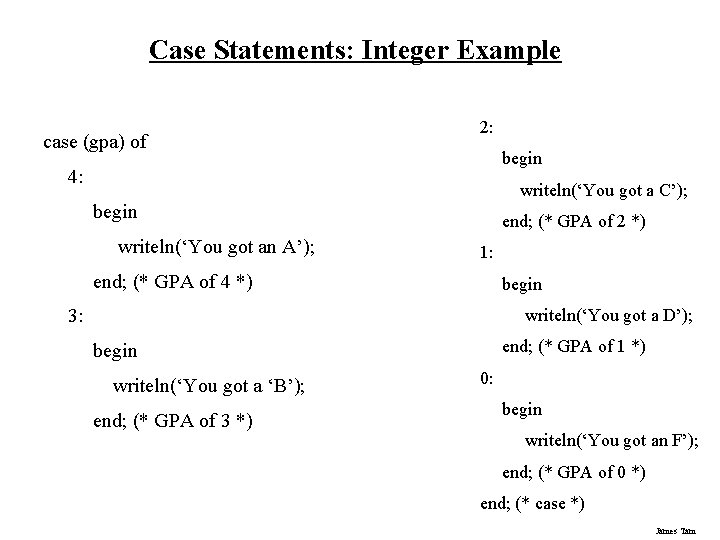
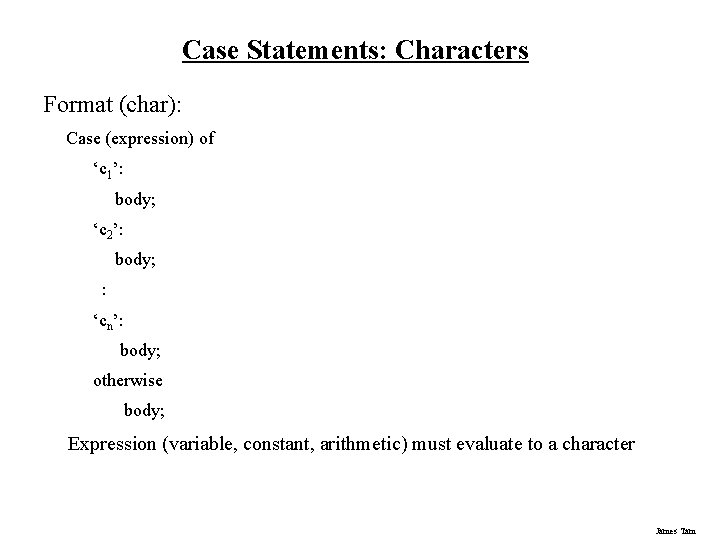
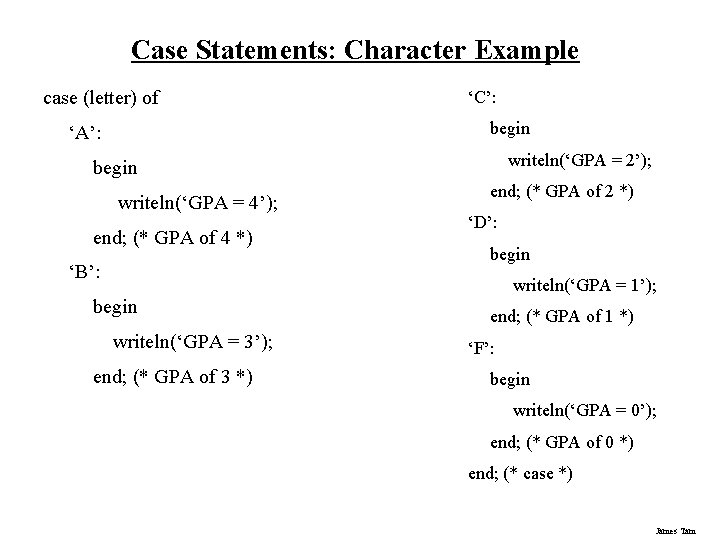
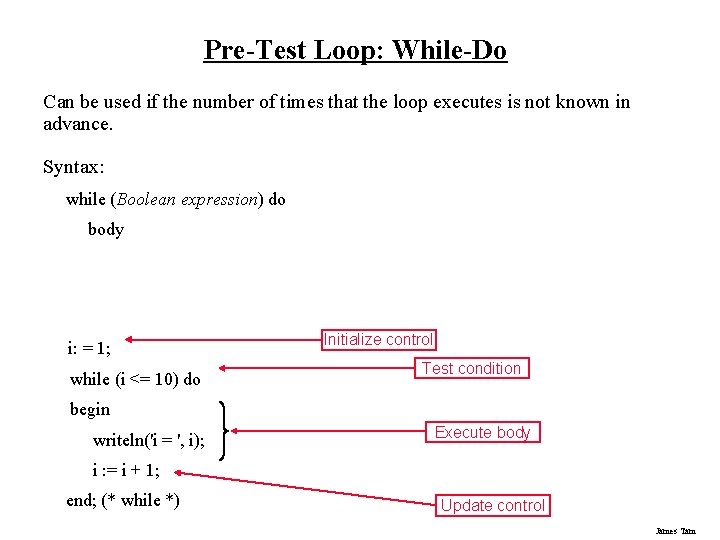
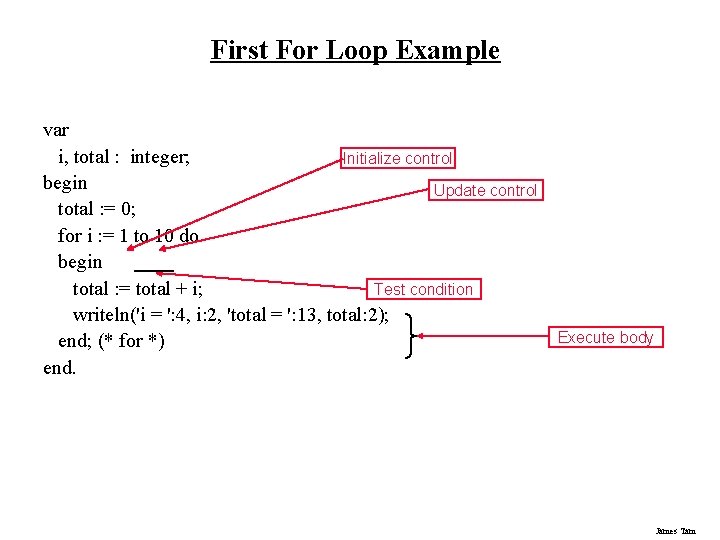
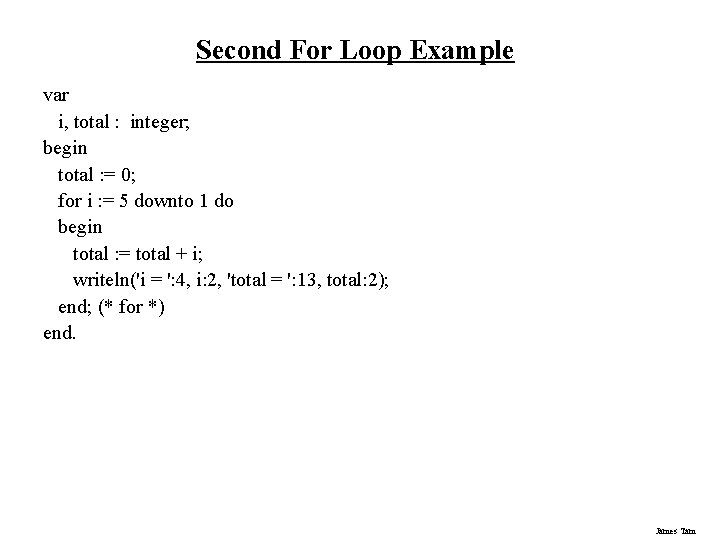
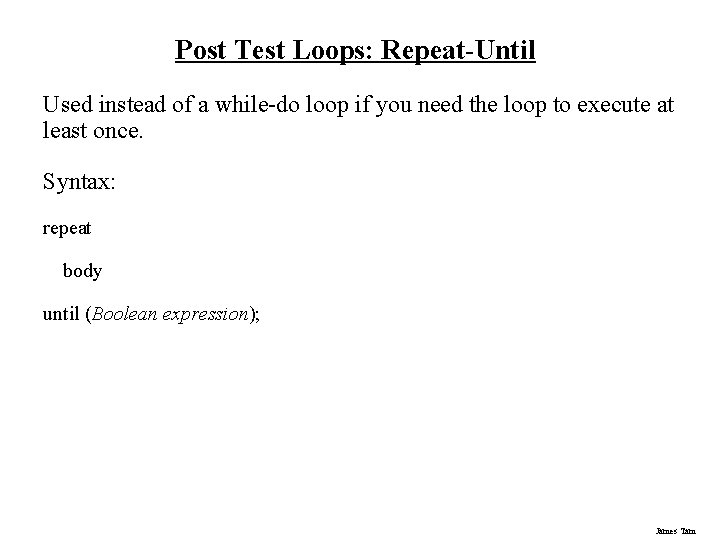
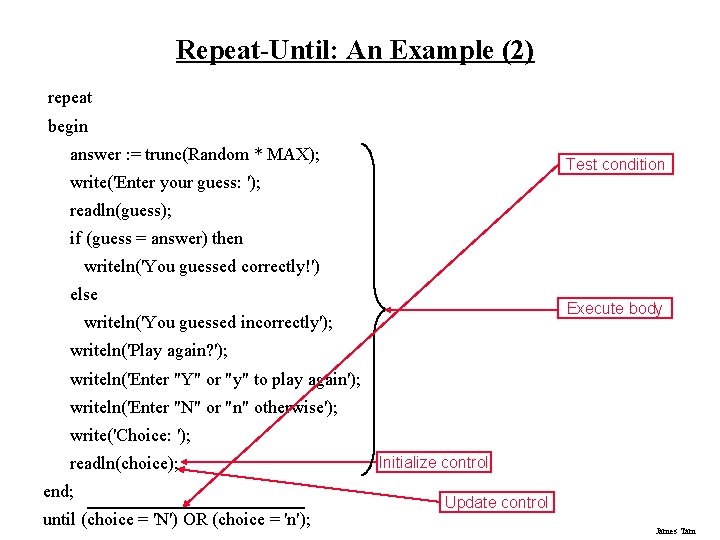
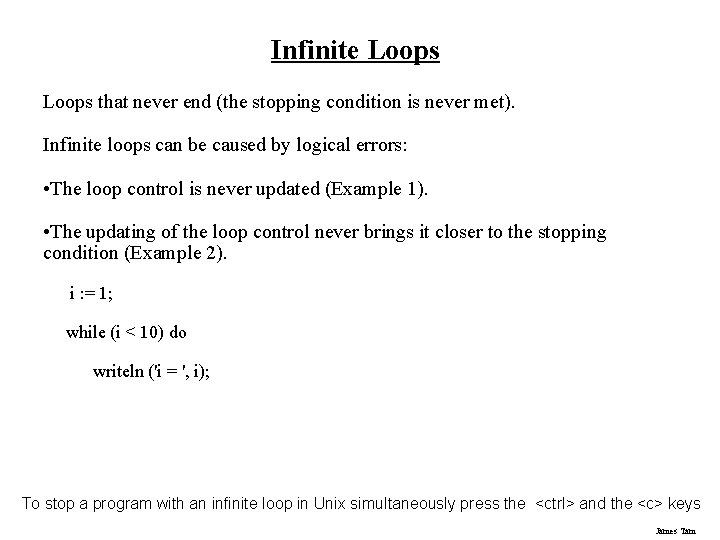
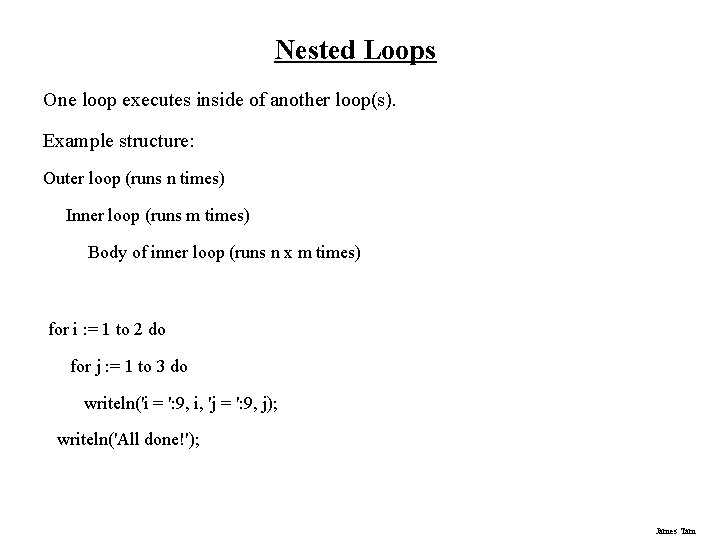
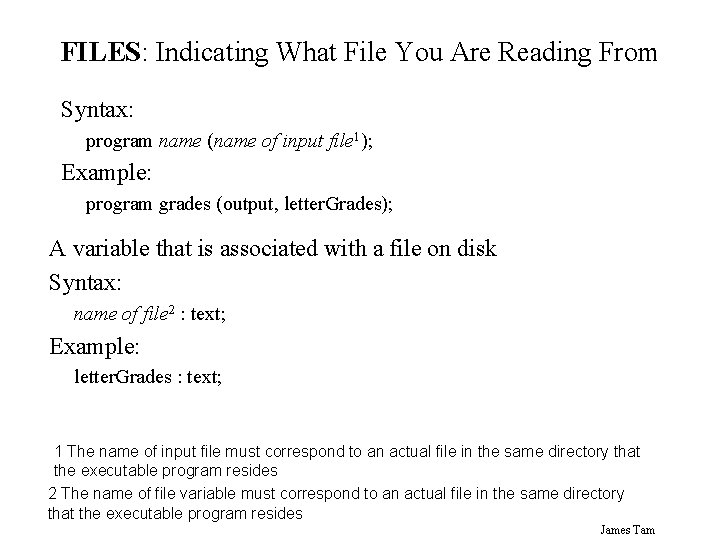
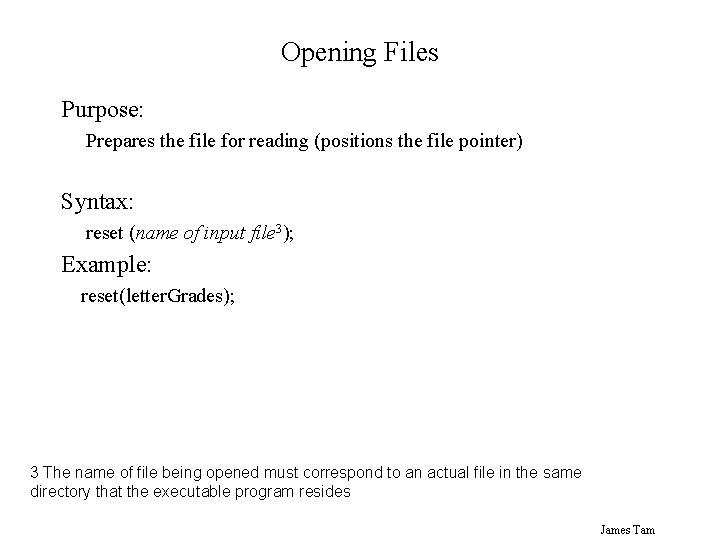
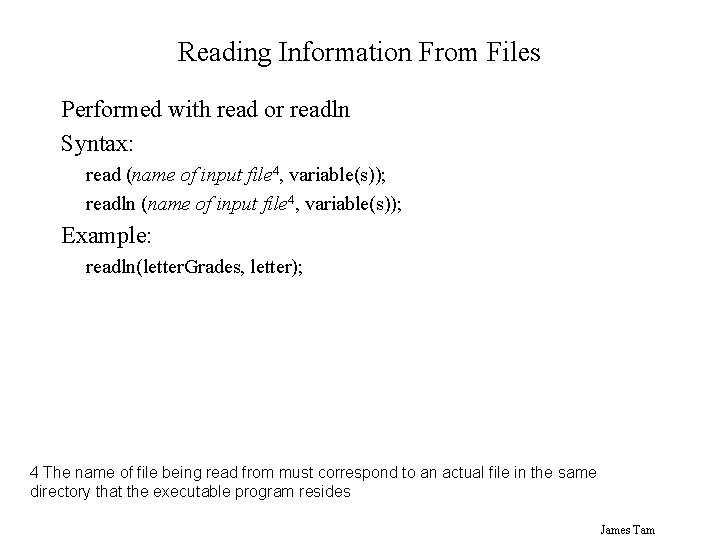
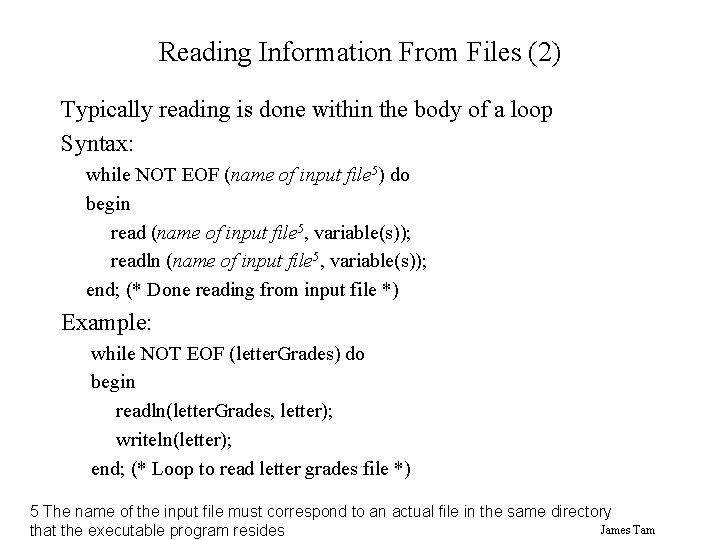
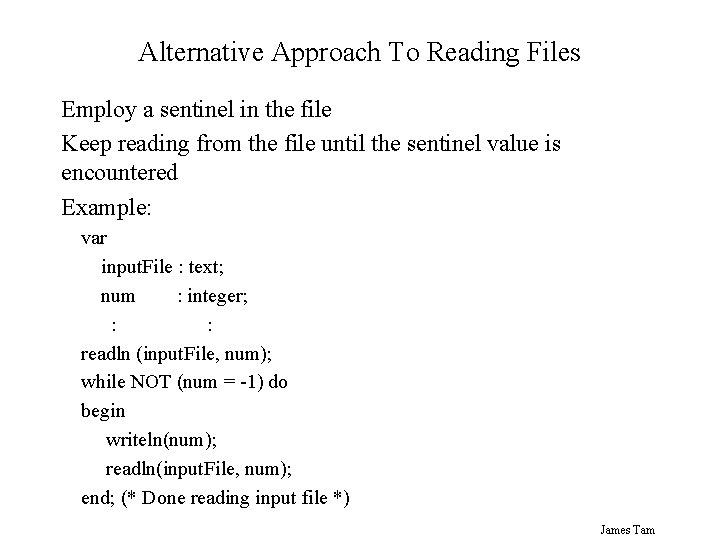
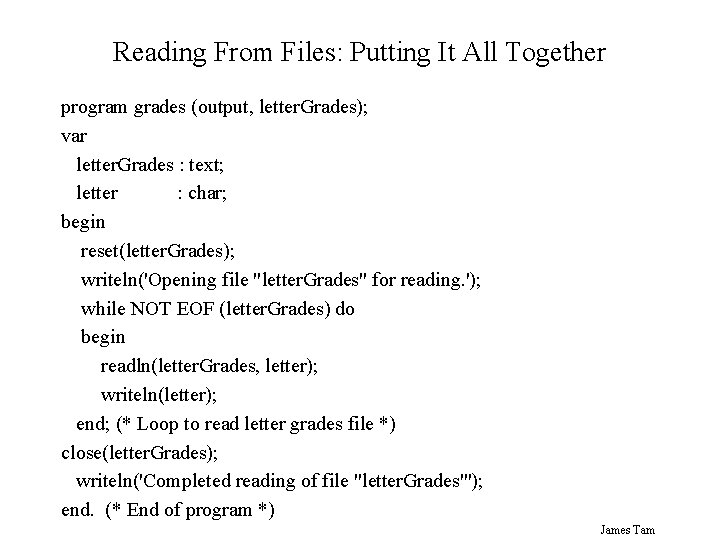
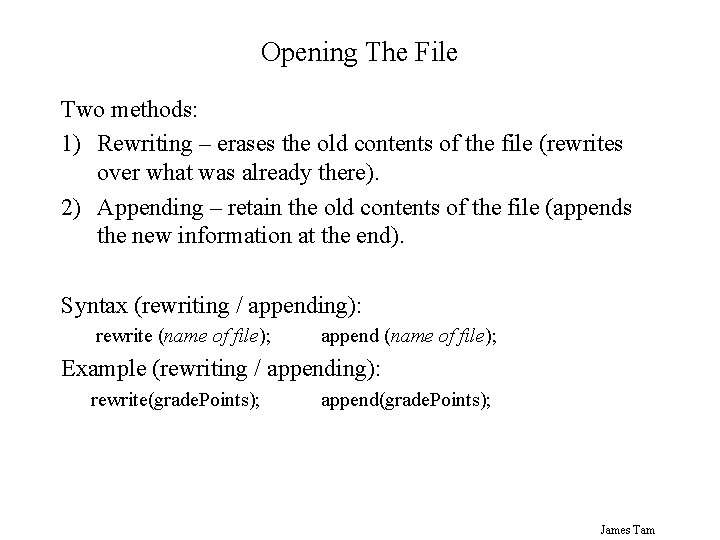
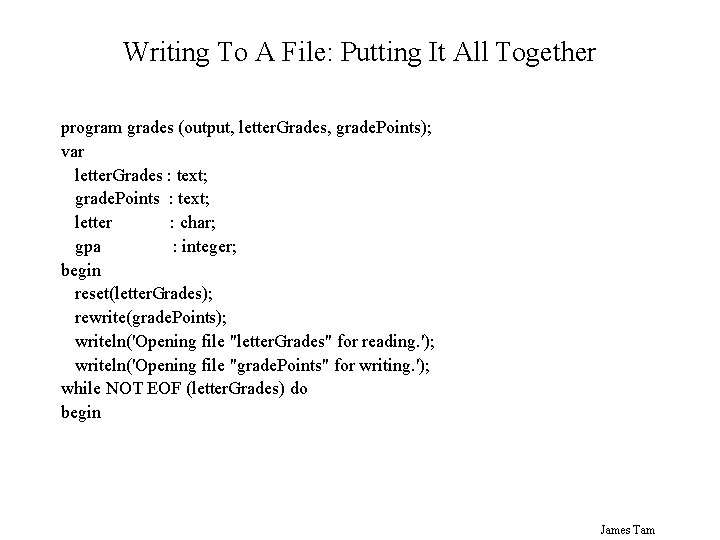
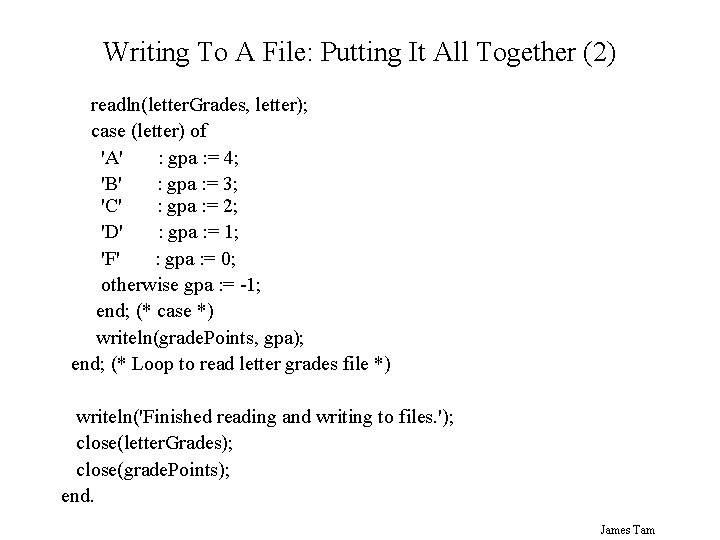
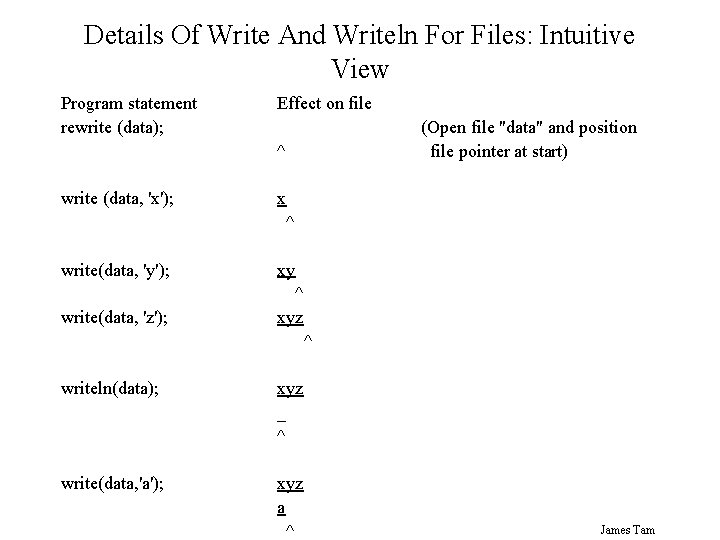
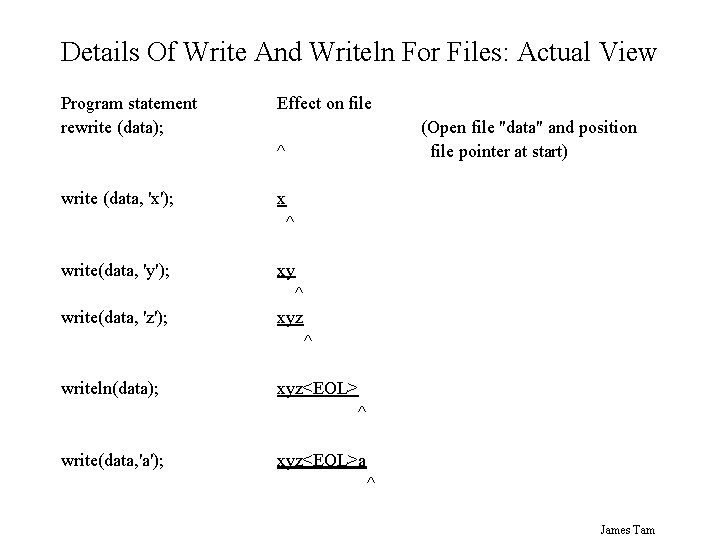
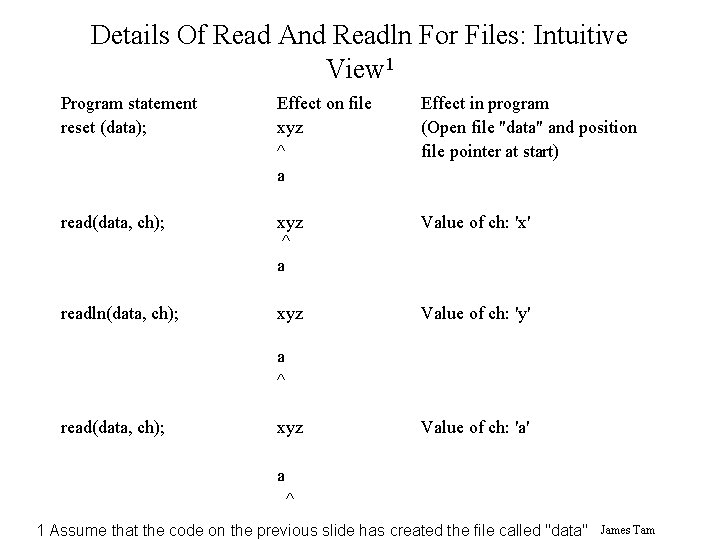
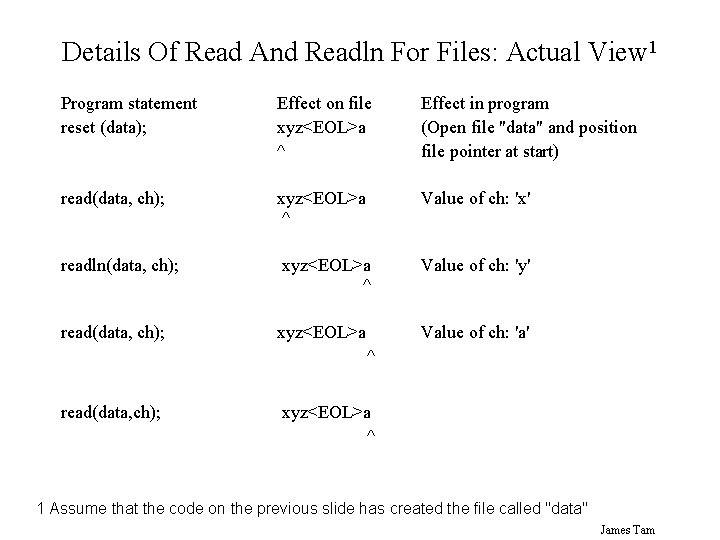
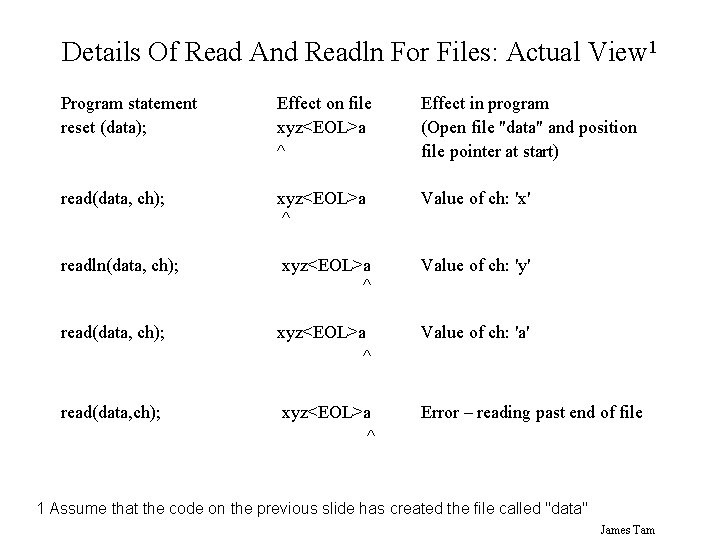
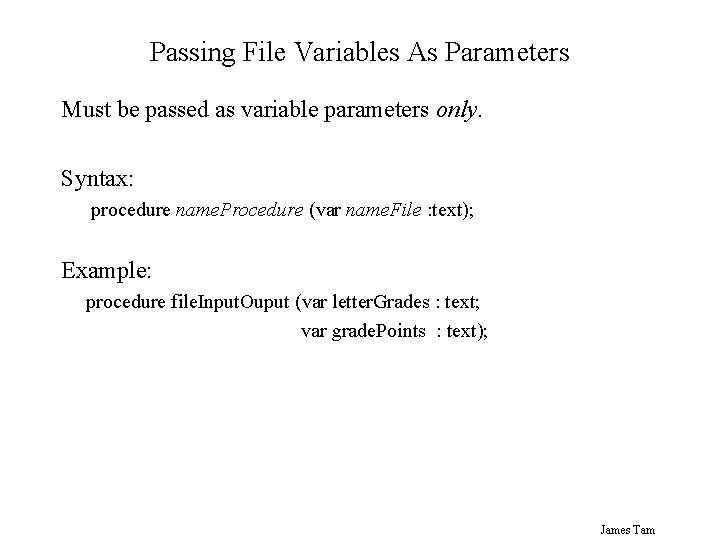
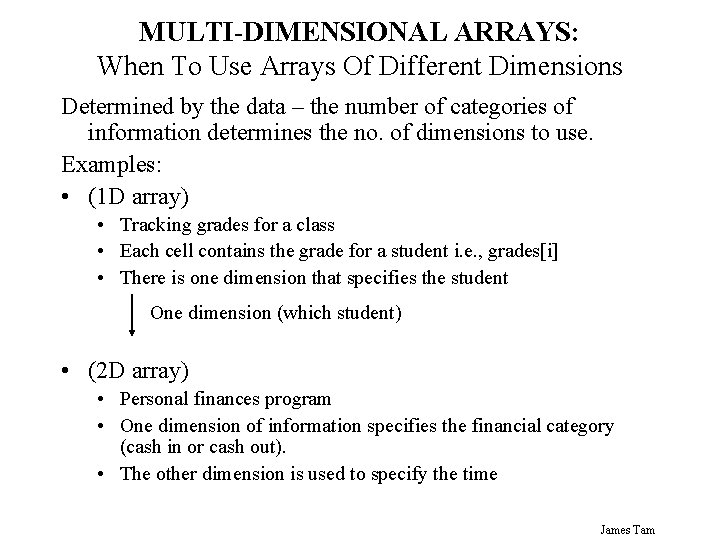
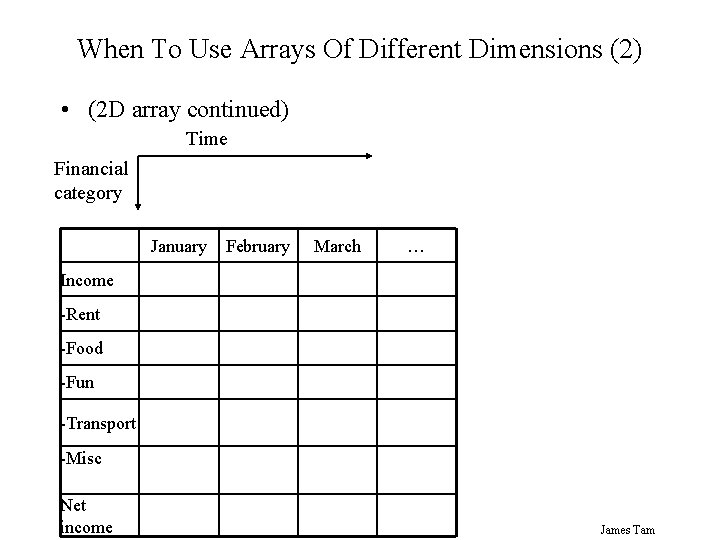
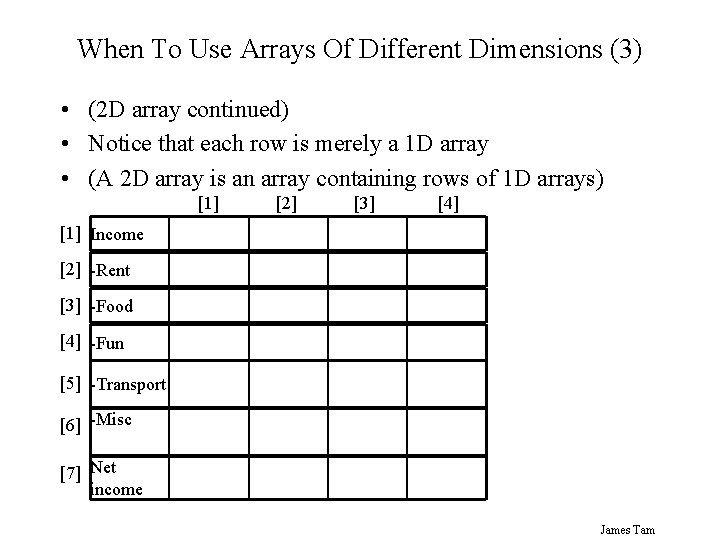
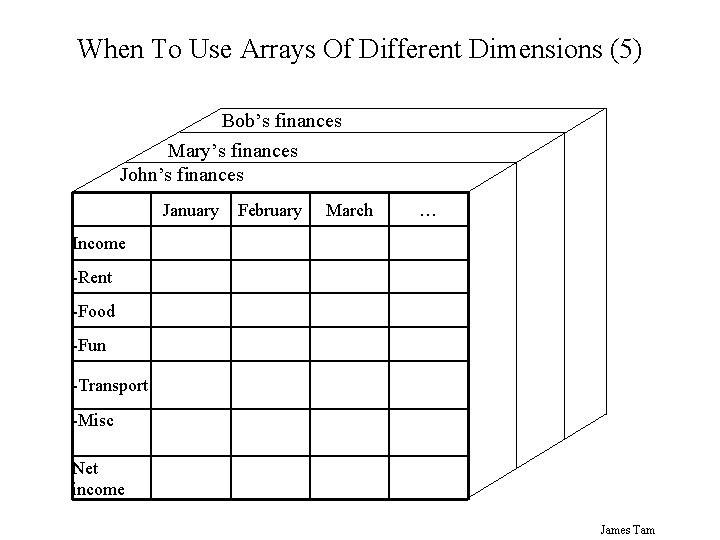
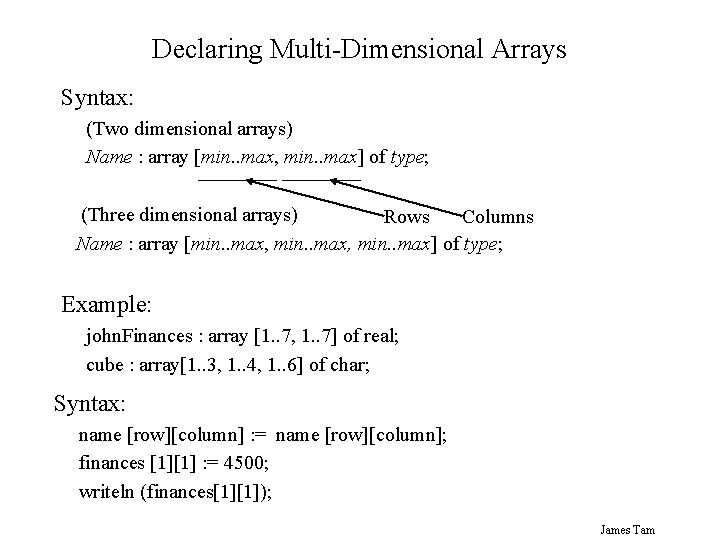
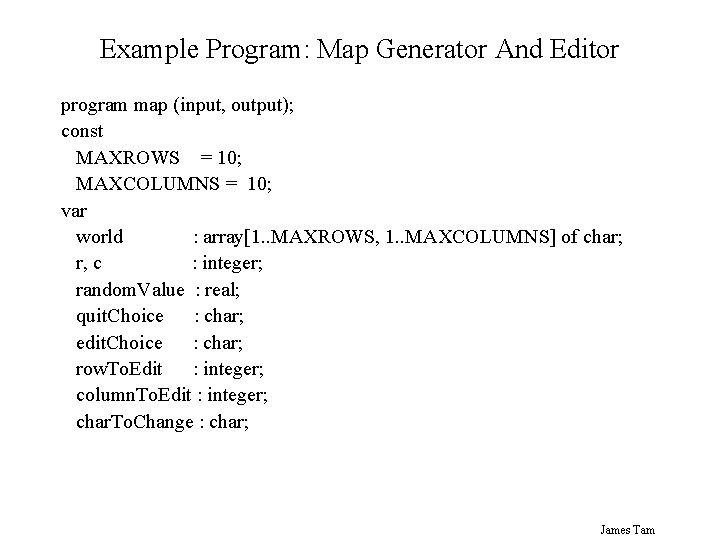
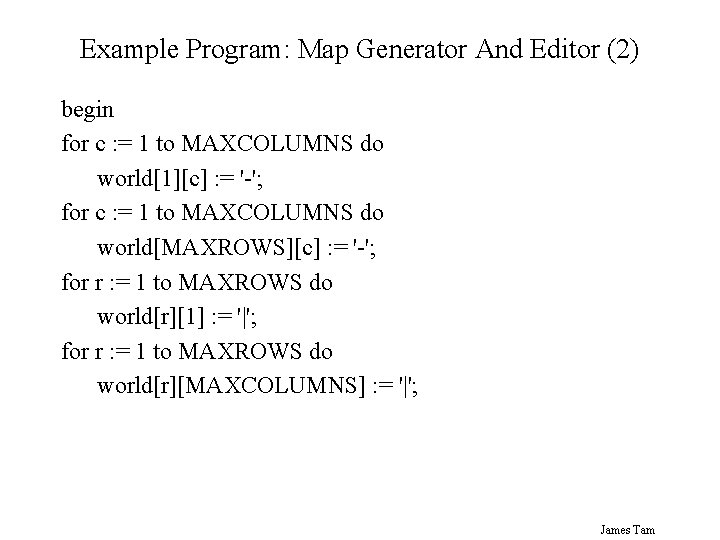
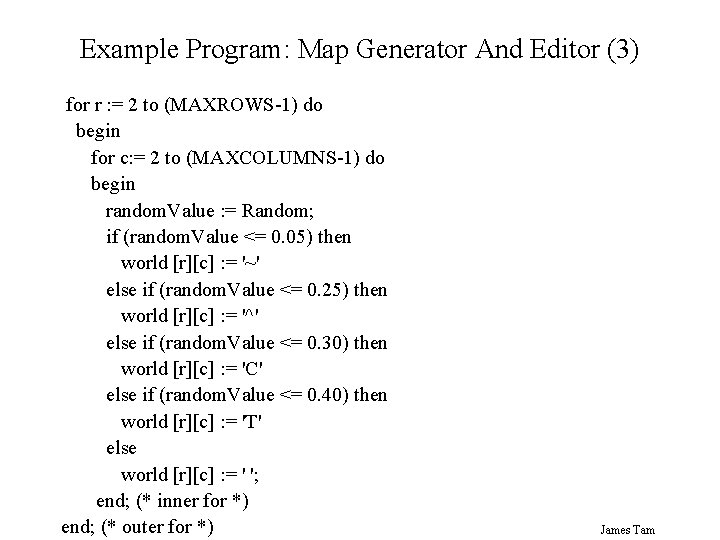
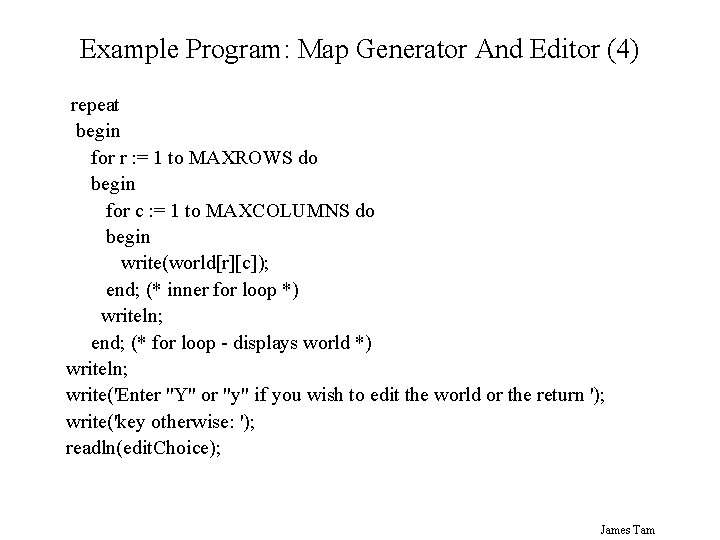
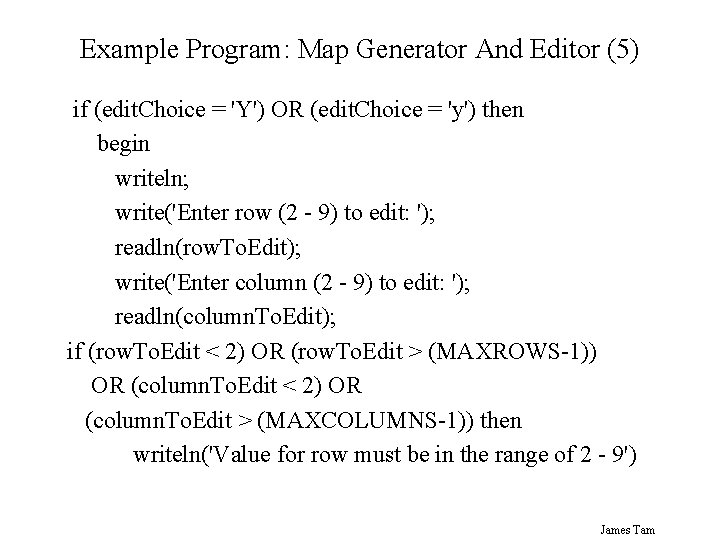
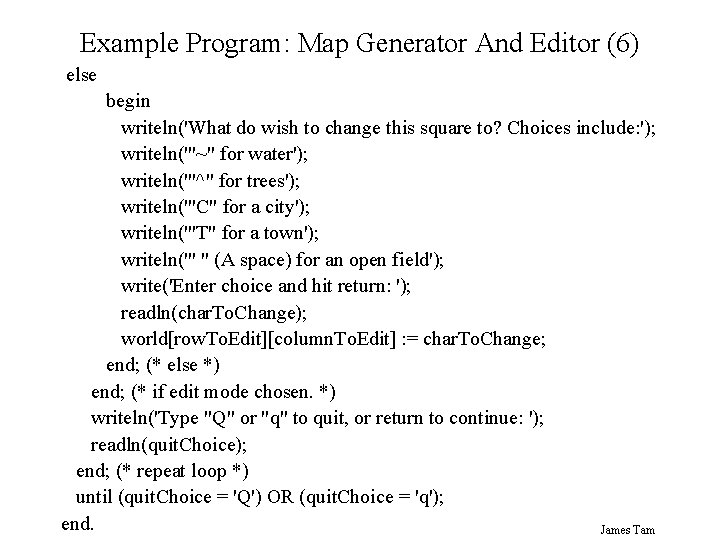
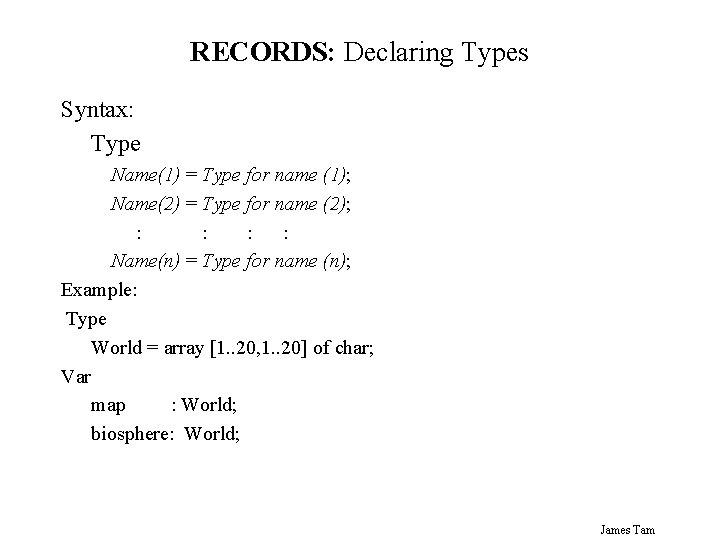
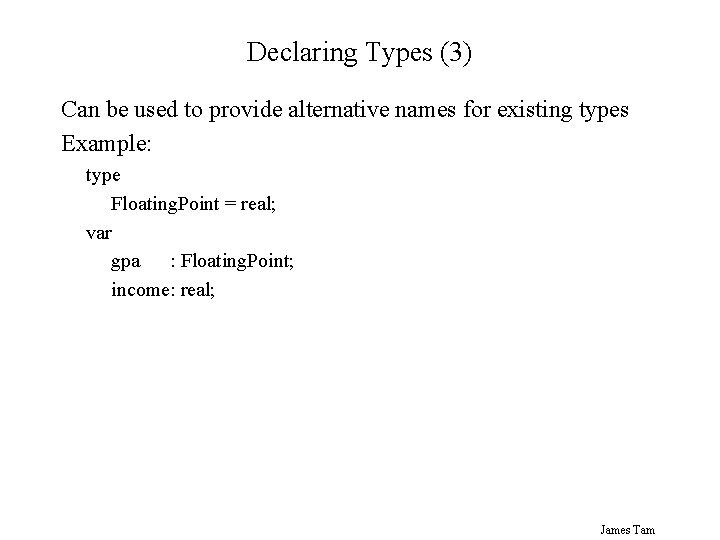
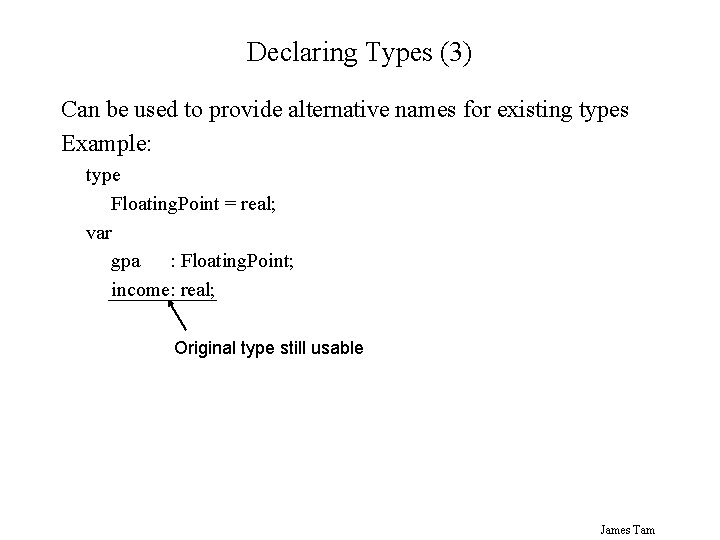
![Declaring Types (4) Example: Type world = array [1. . 20, 1. . 20] Declaring Types (4) Example: Type world = array [1. . 20, 1. . 20]](https://slidetodoc.com/presentation_image_h2/ed92b301b7f9d83e665b7d2596230111/image-70.jpg)
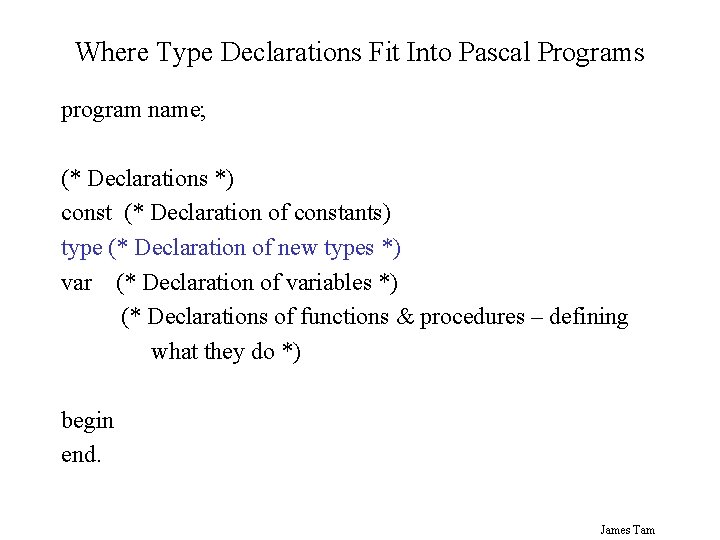
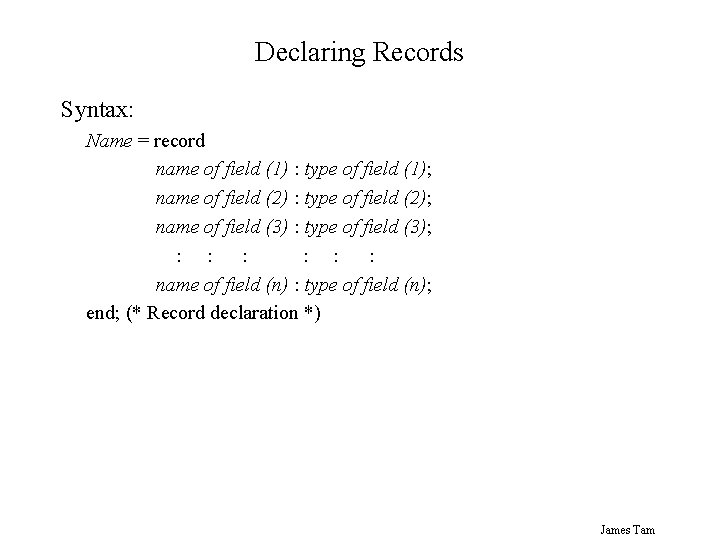
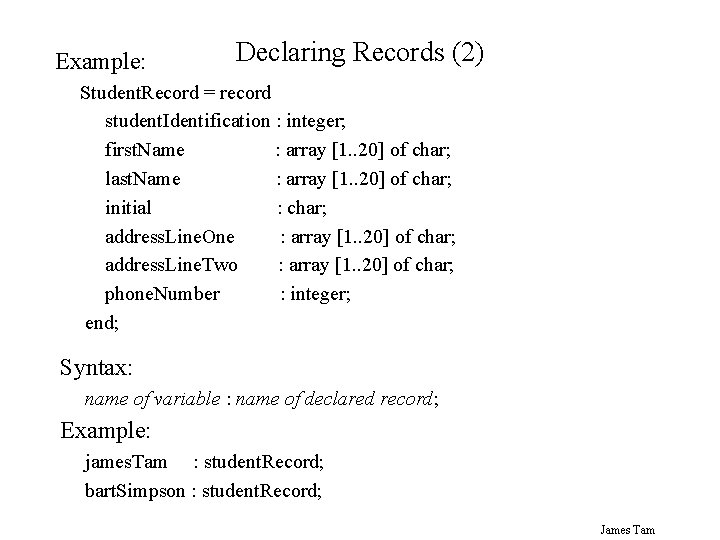
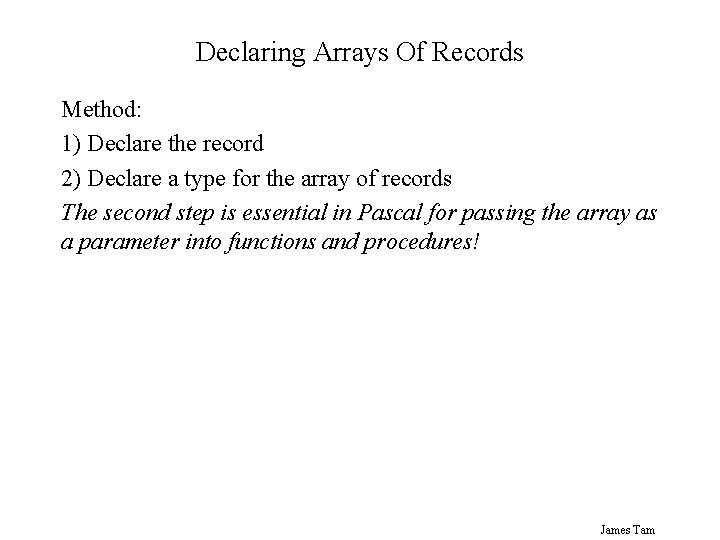
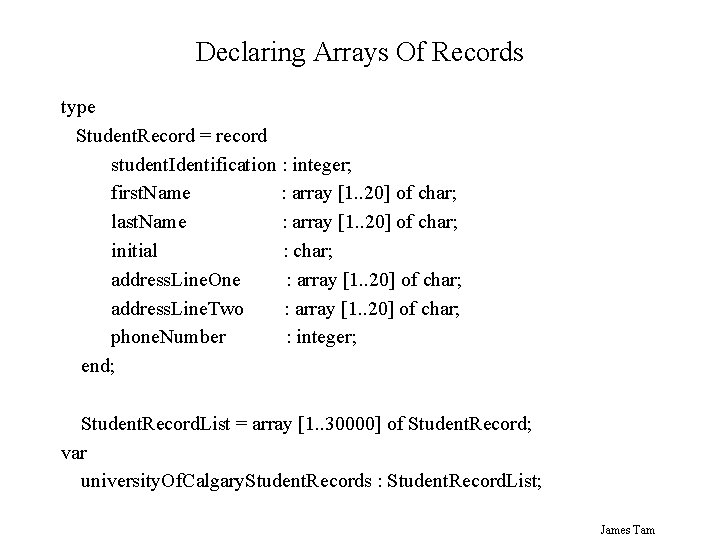
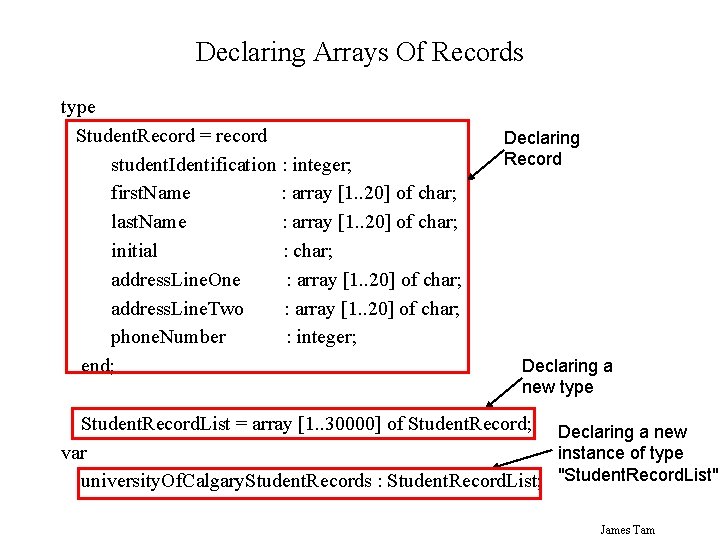
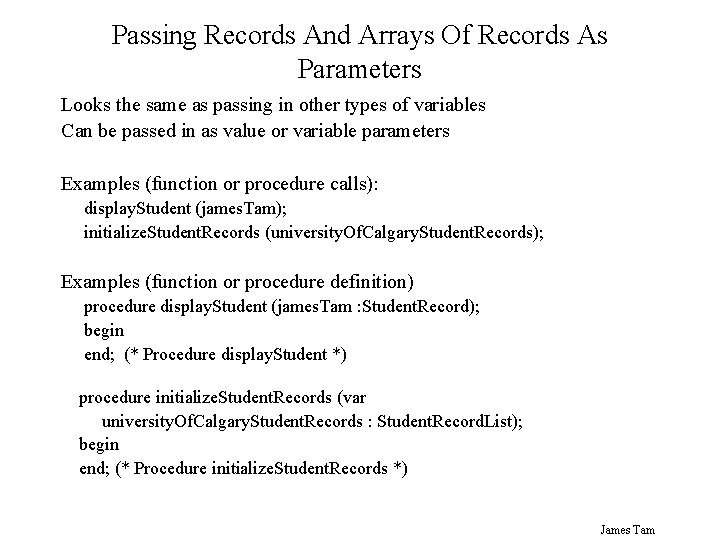
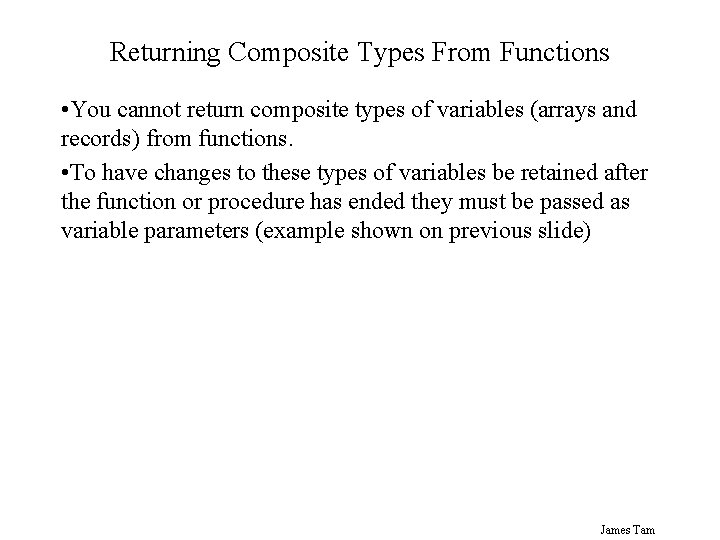
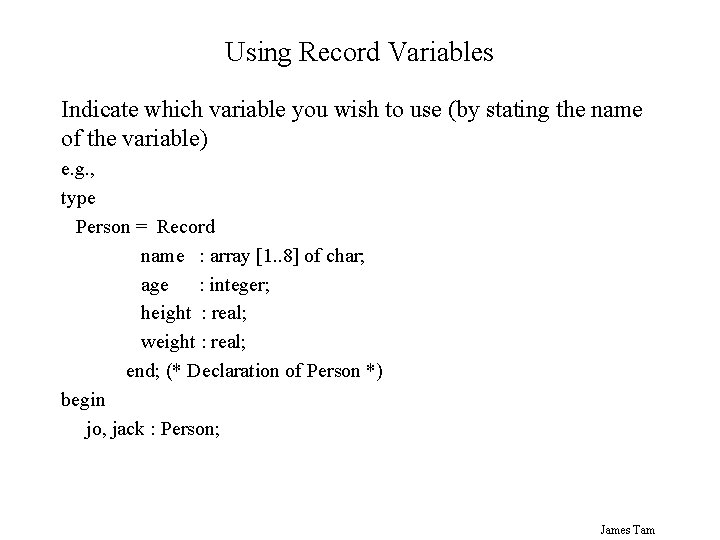
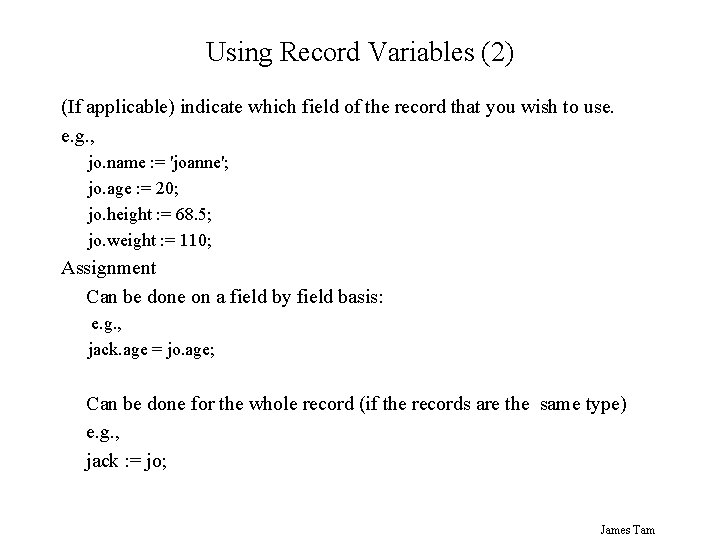
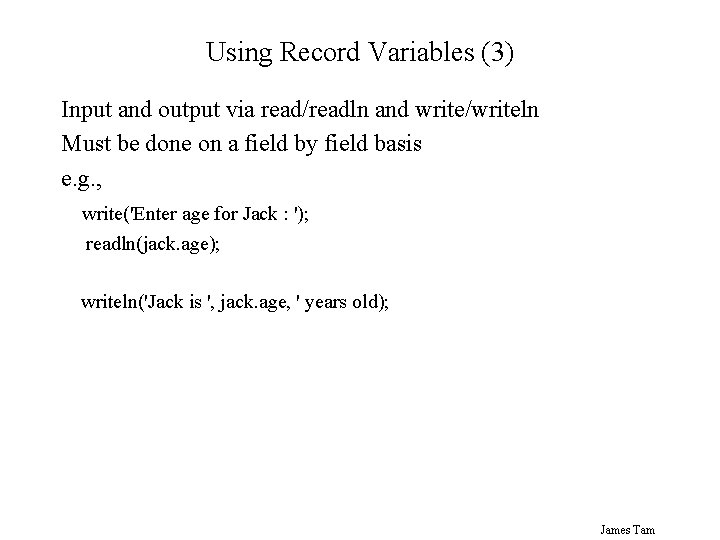
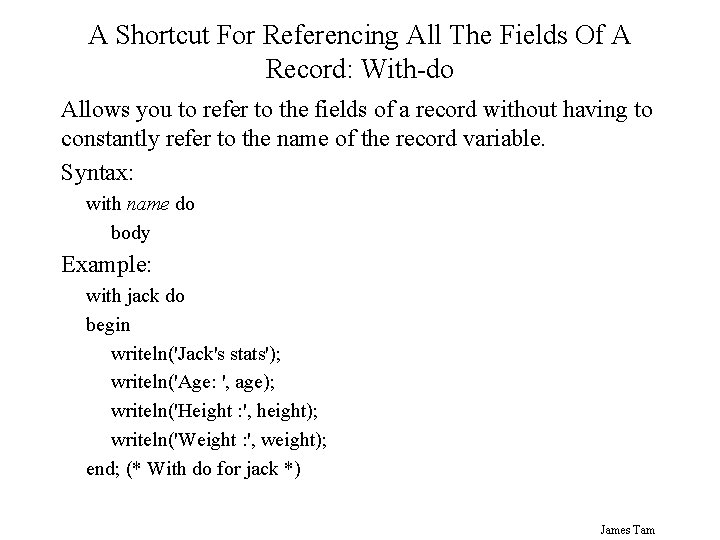
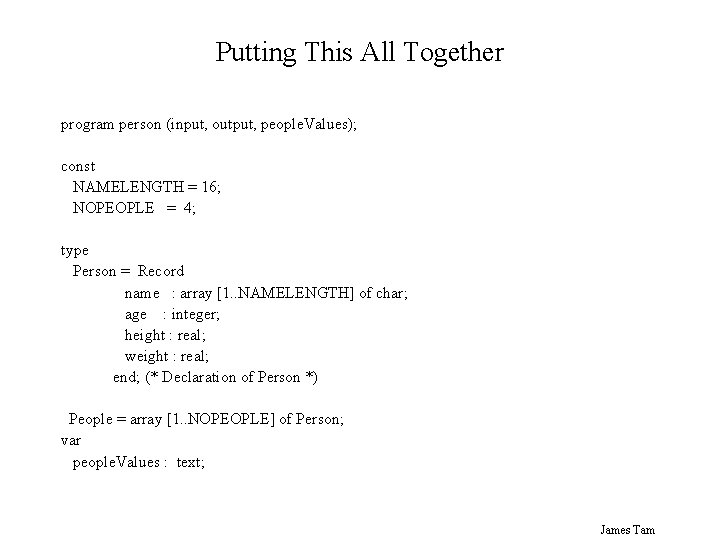
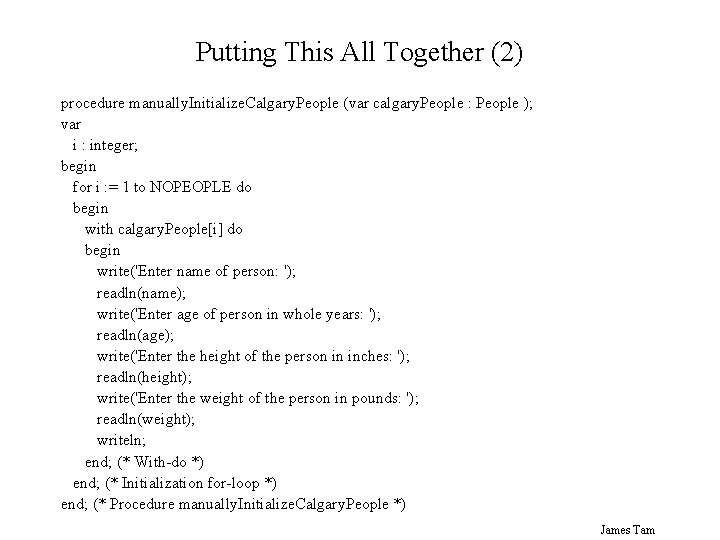
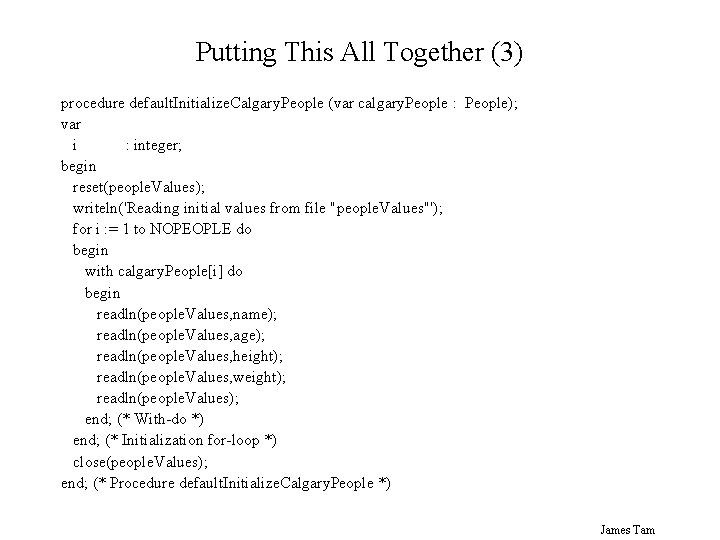
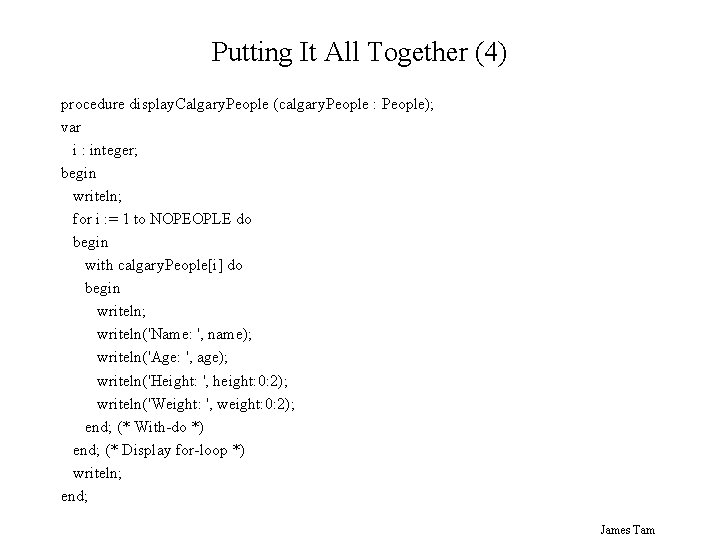
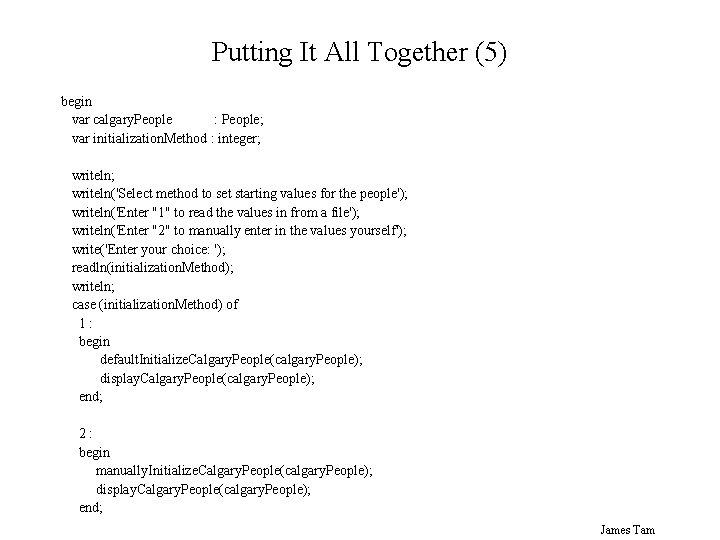
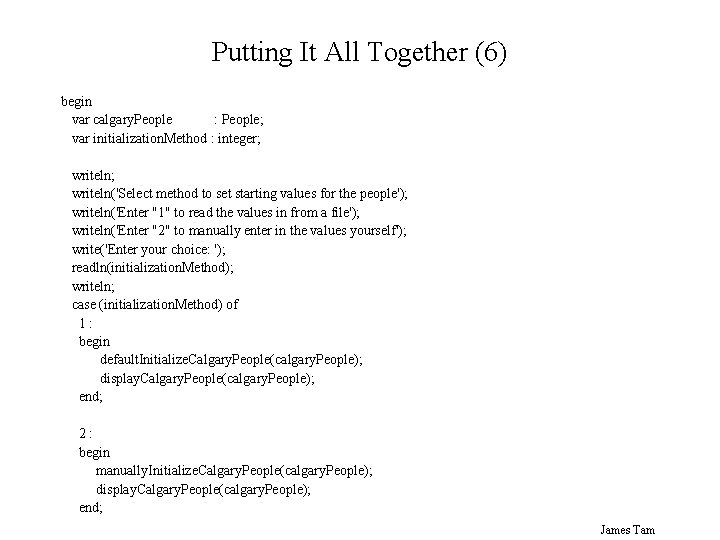
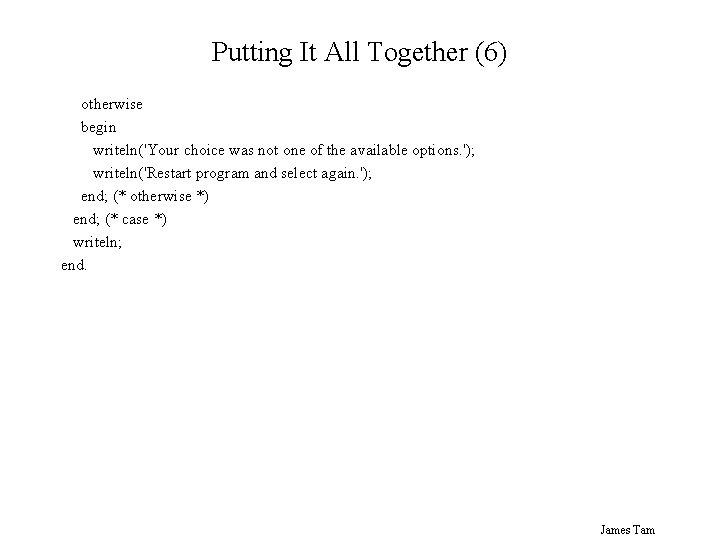
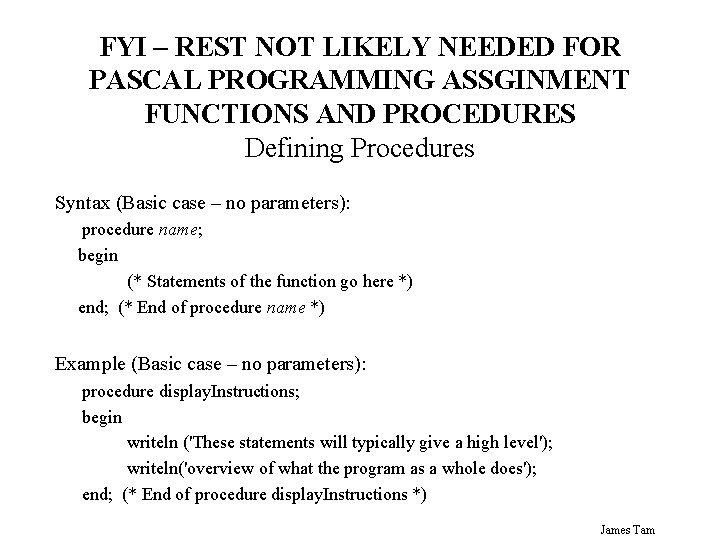
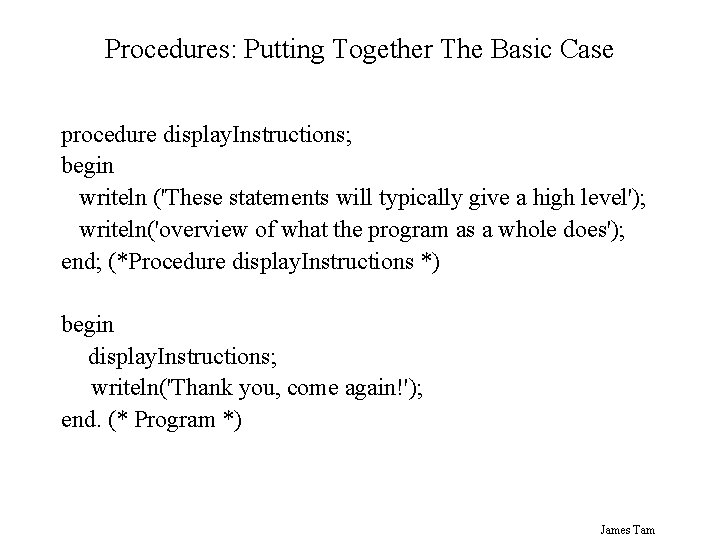
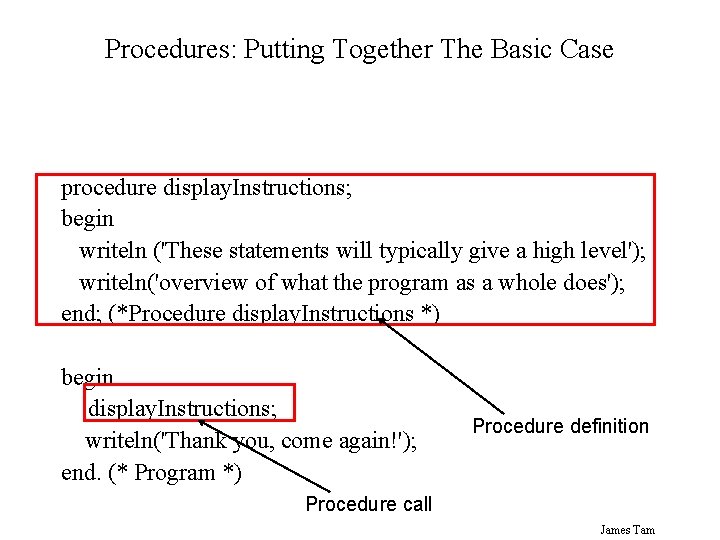
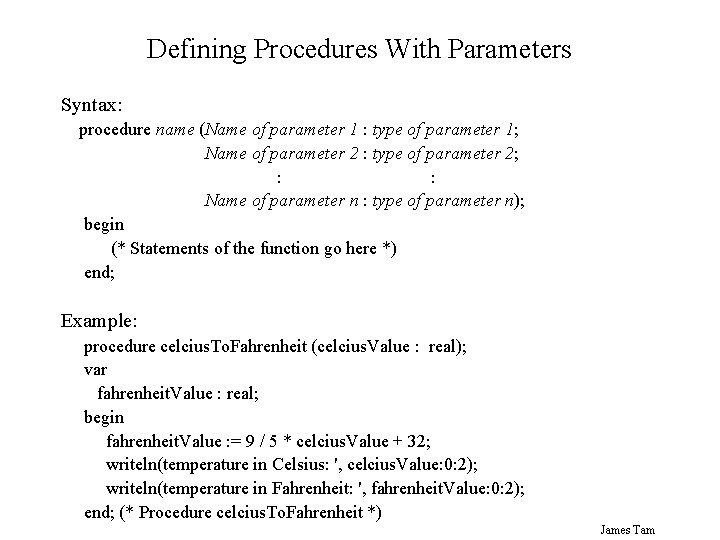
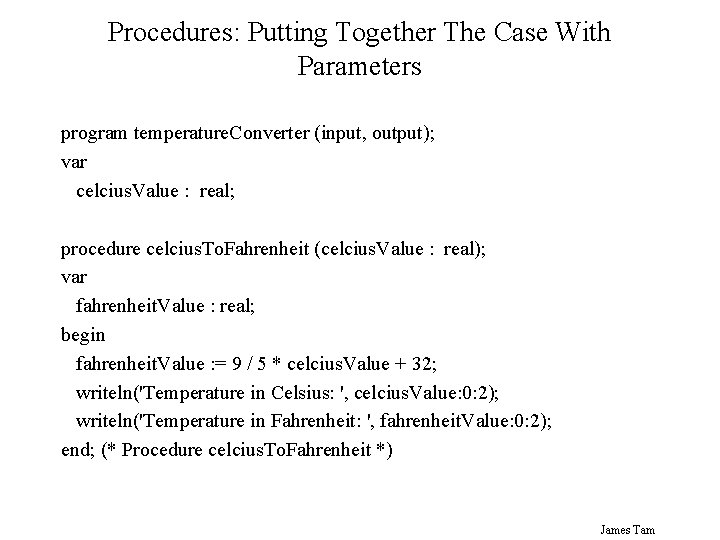
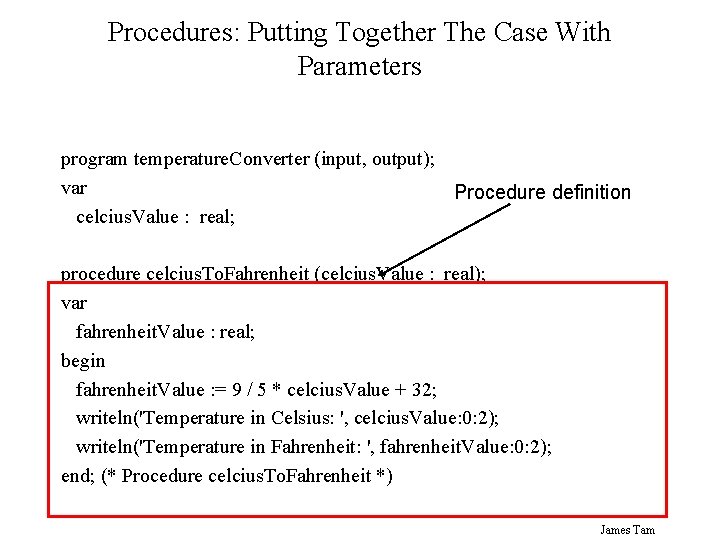
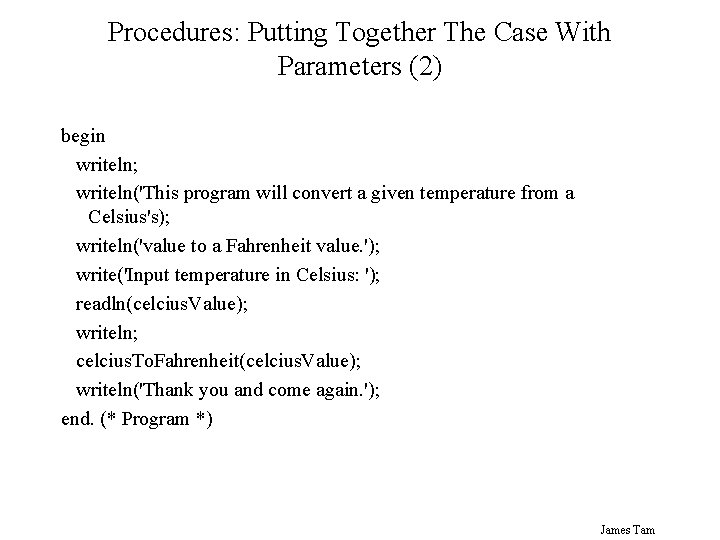
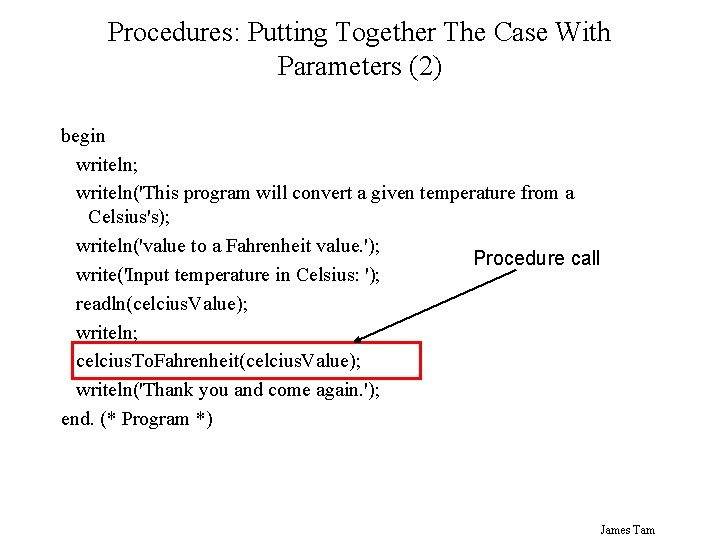
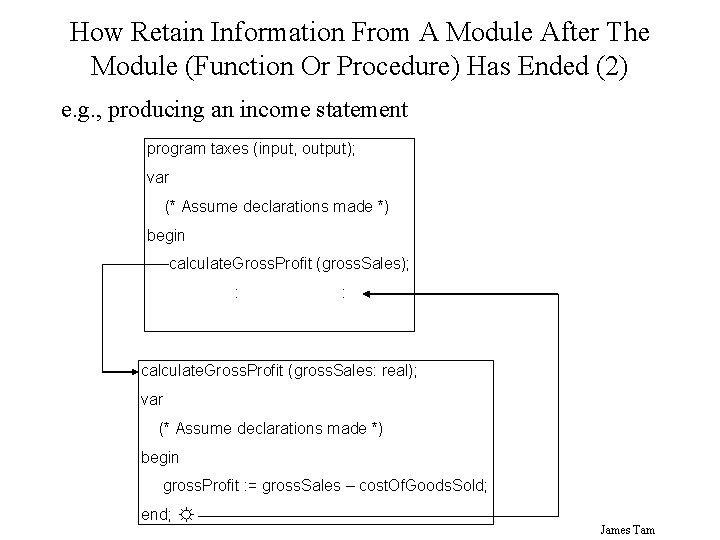
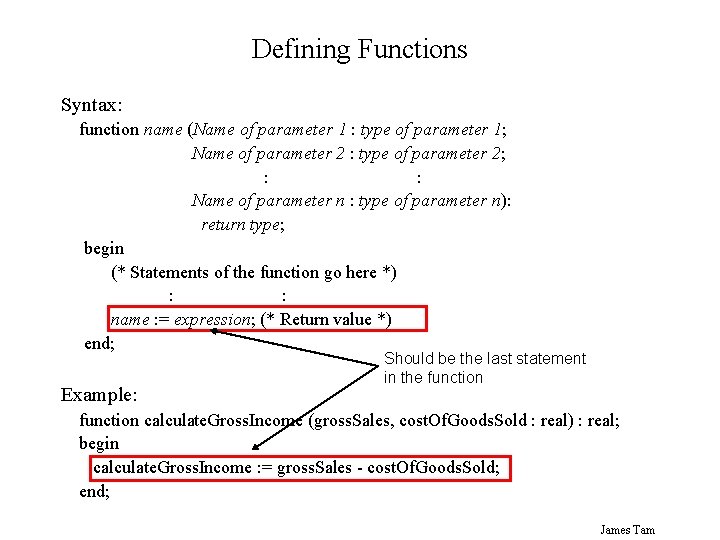
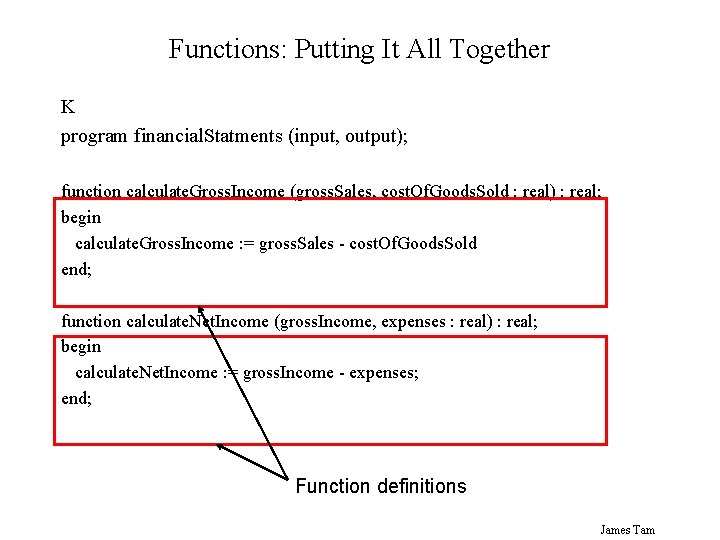
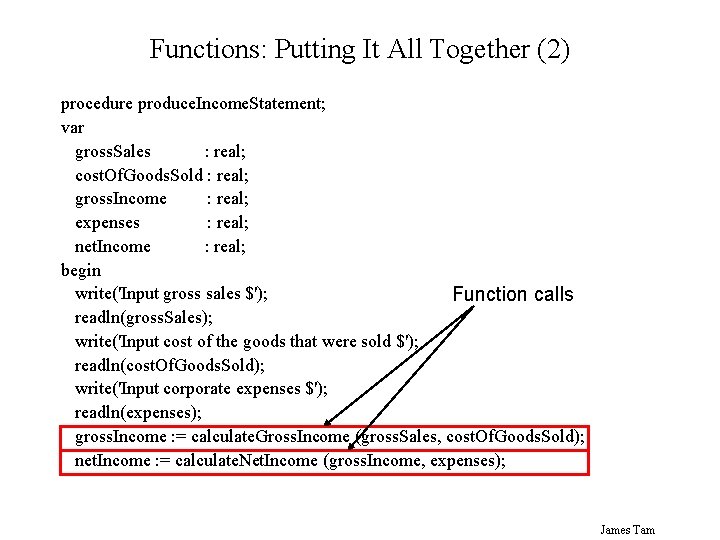
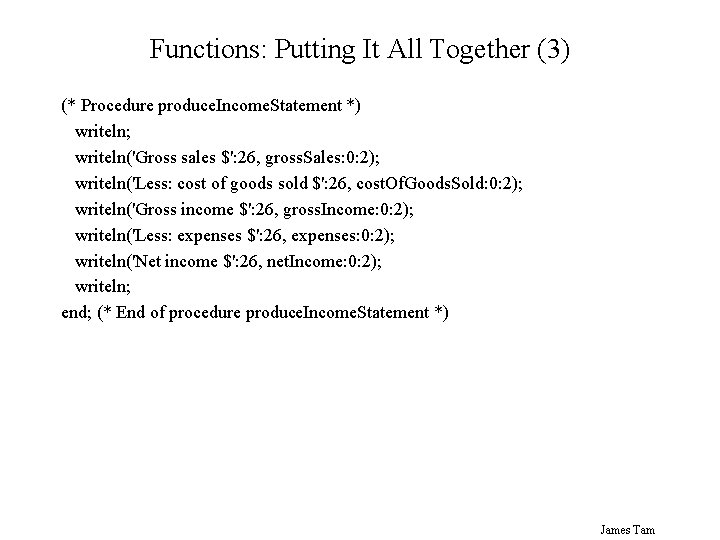
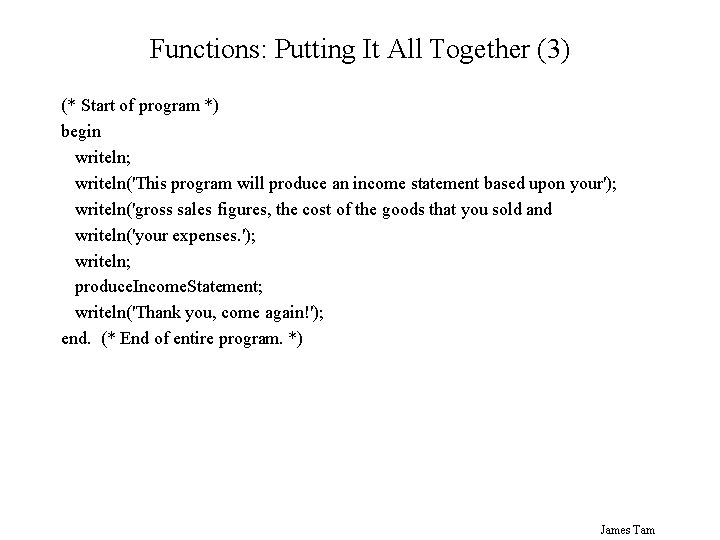
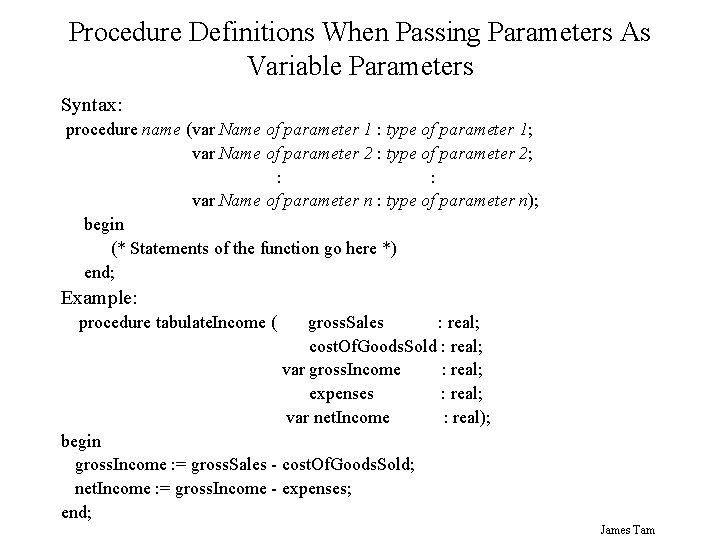
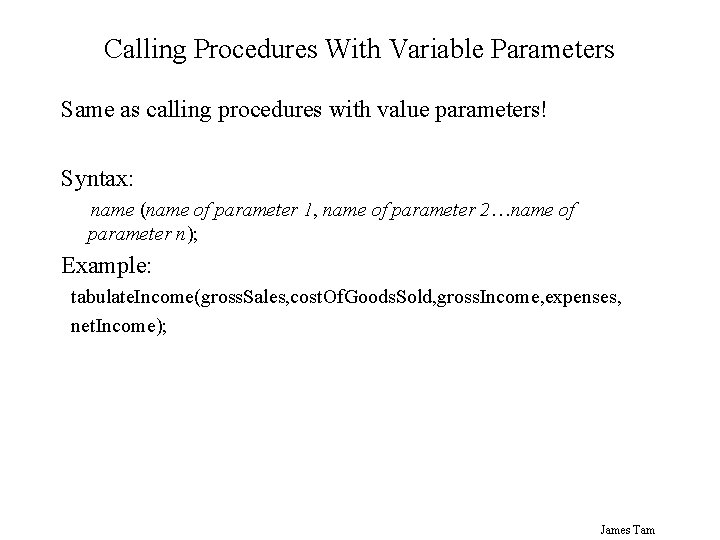
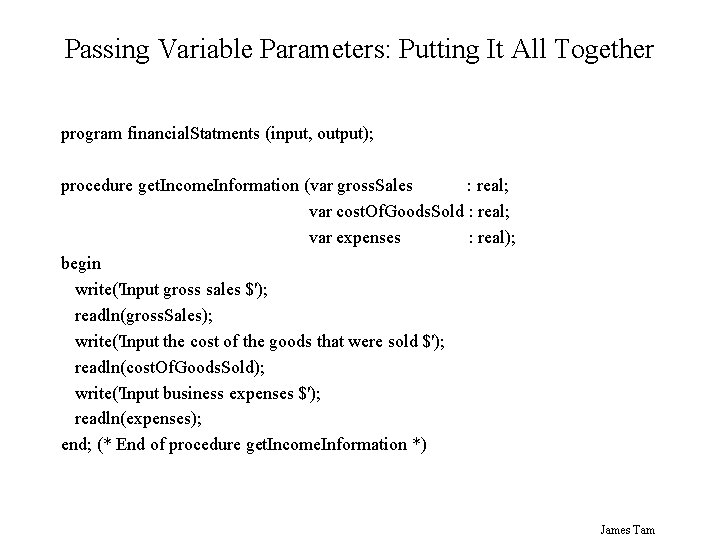
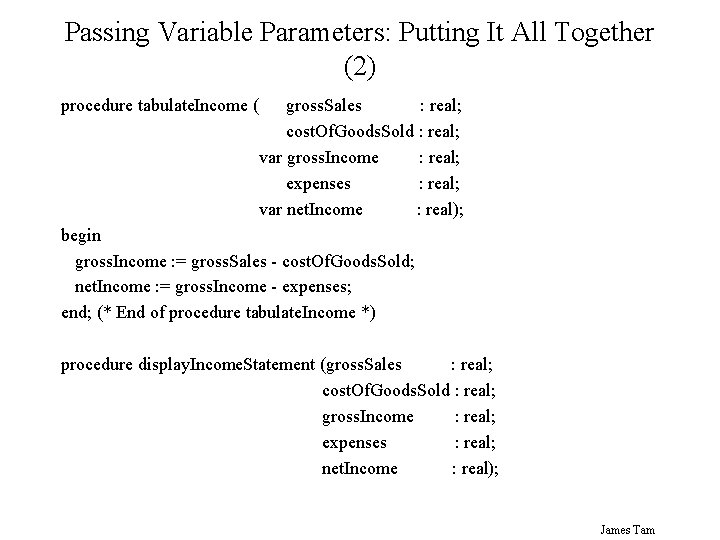
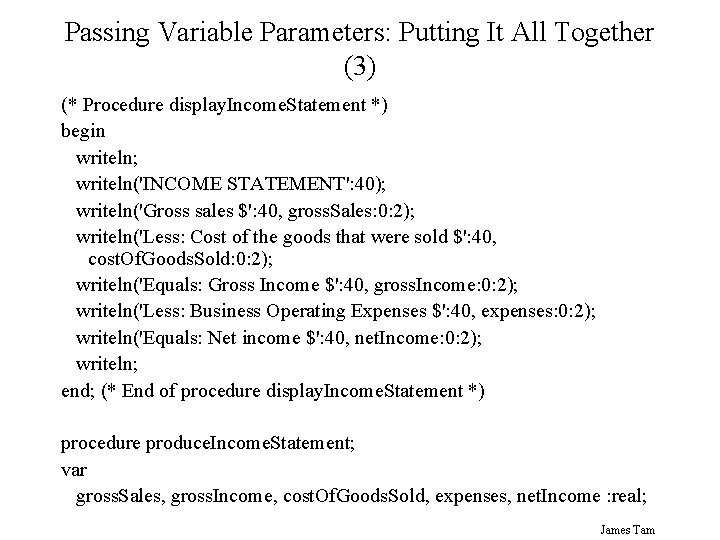
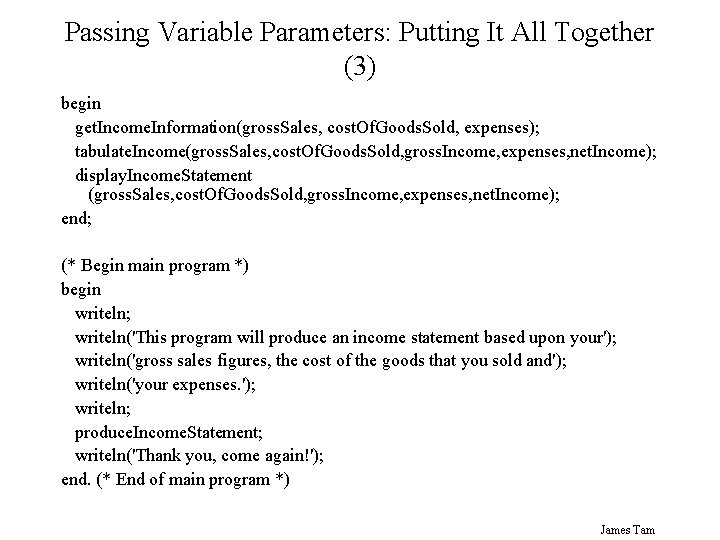
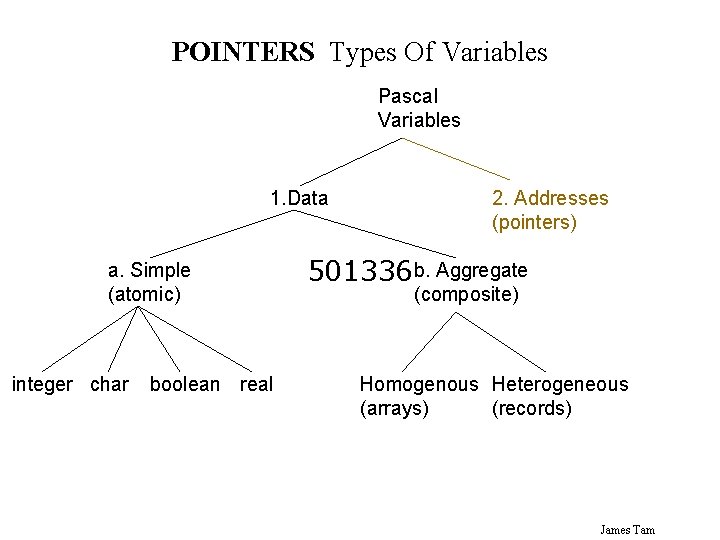
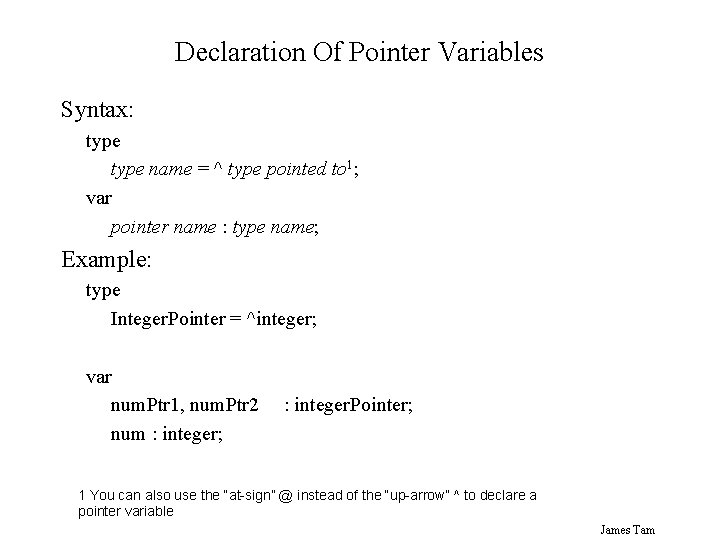
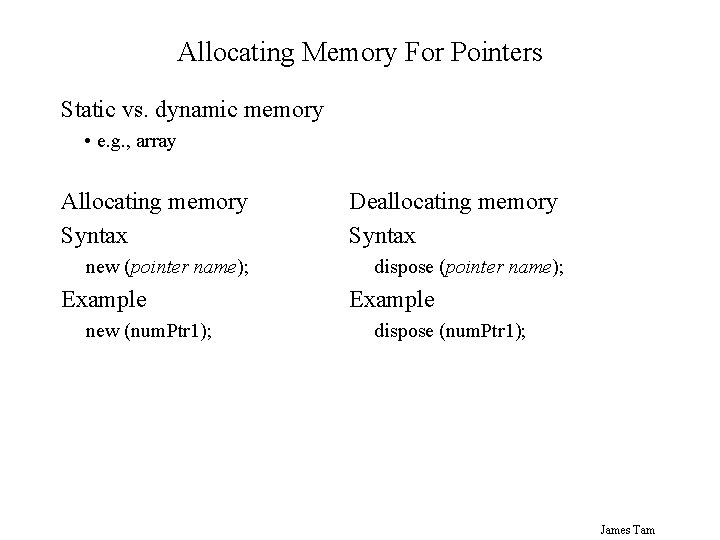
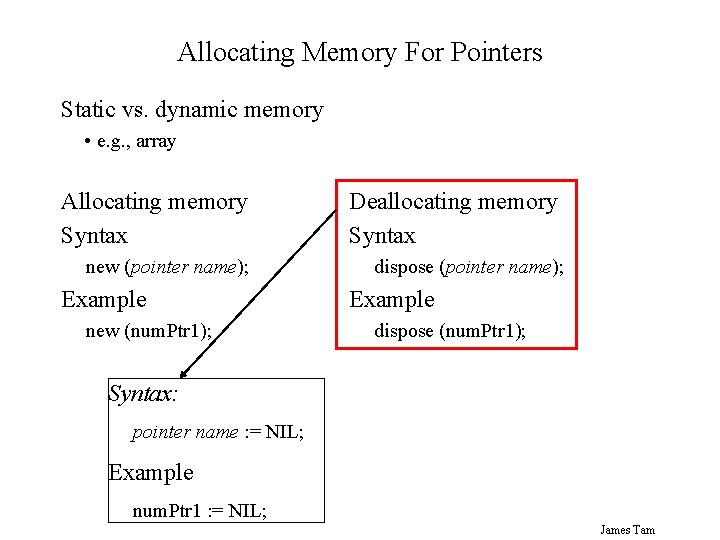
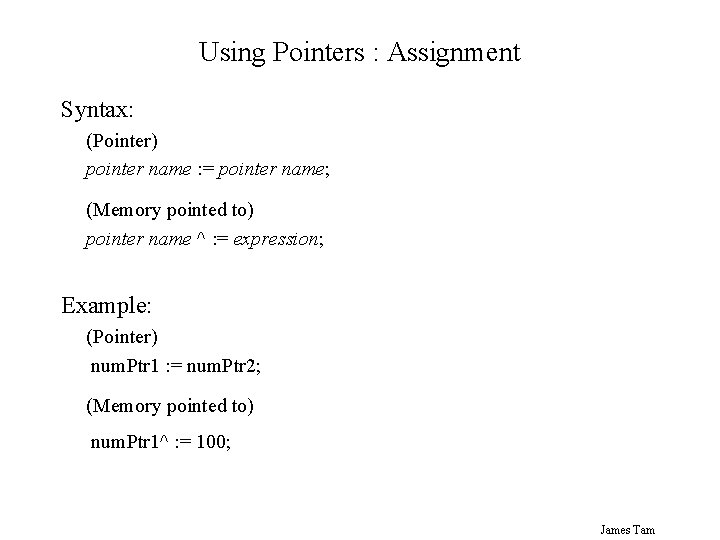
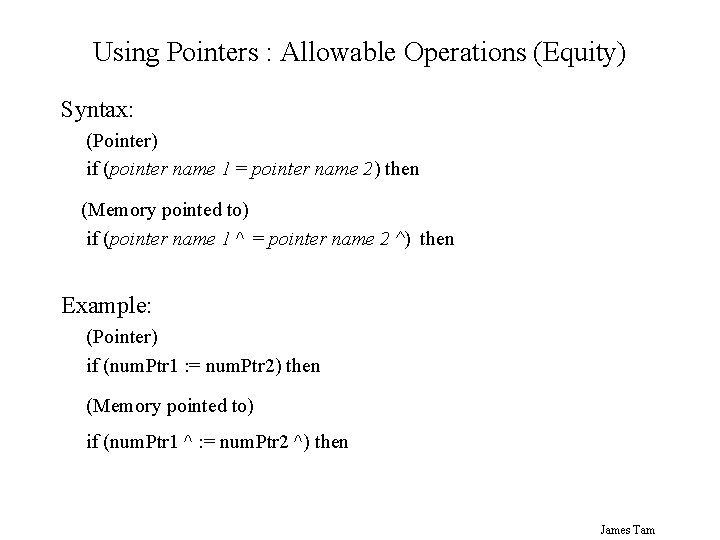
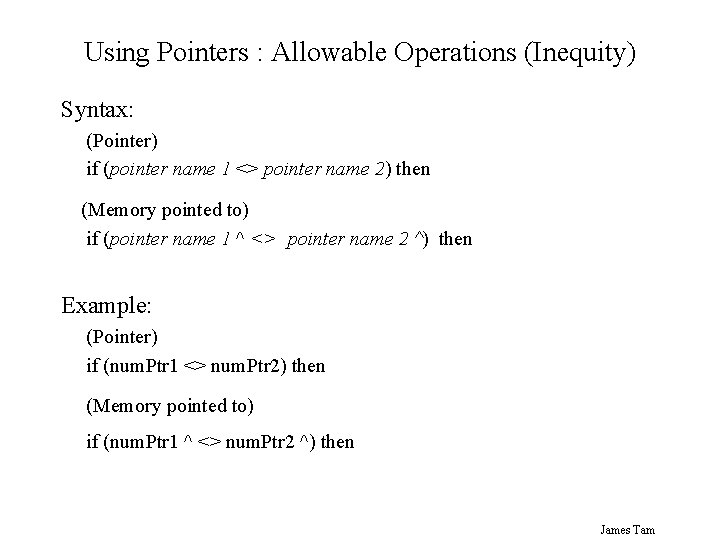
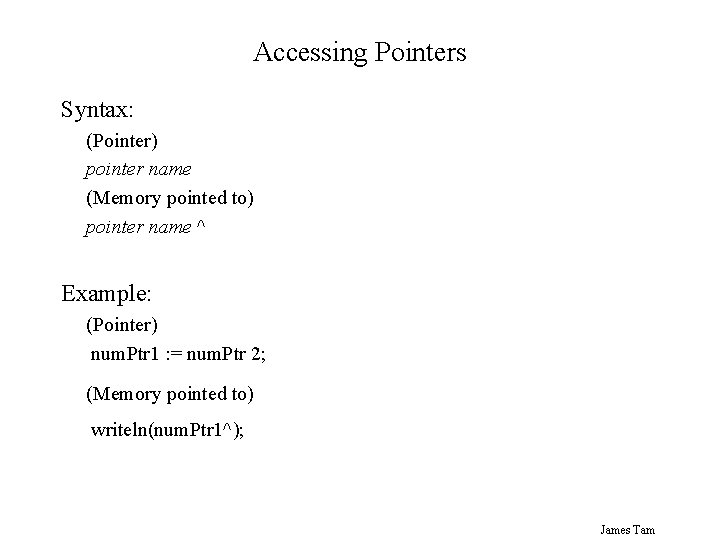
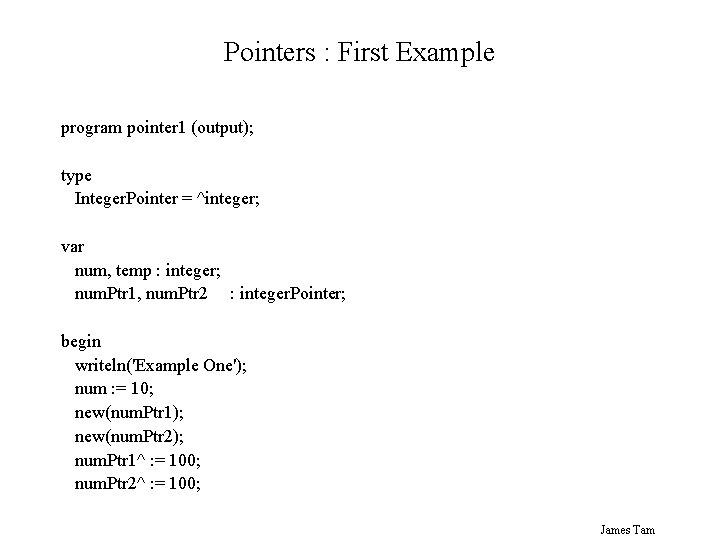
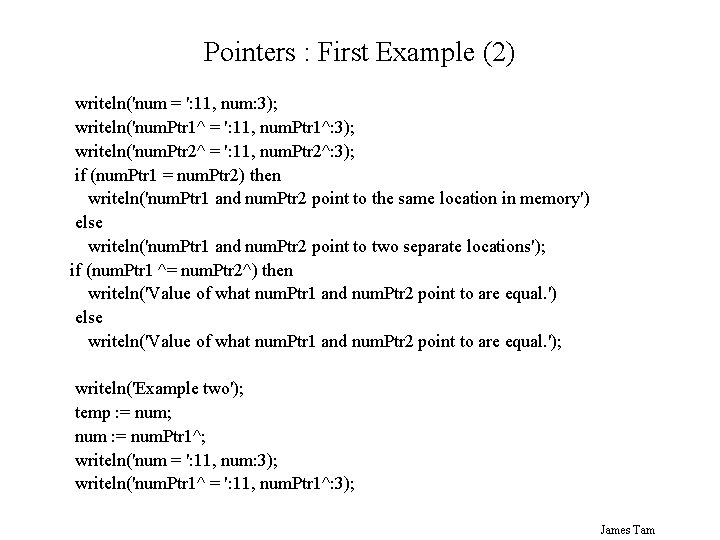
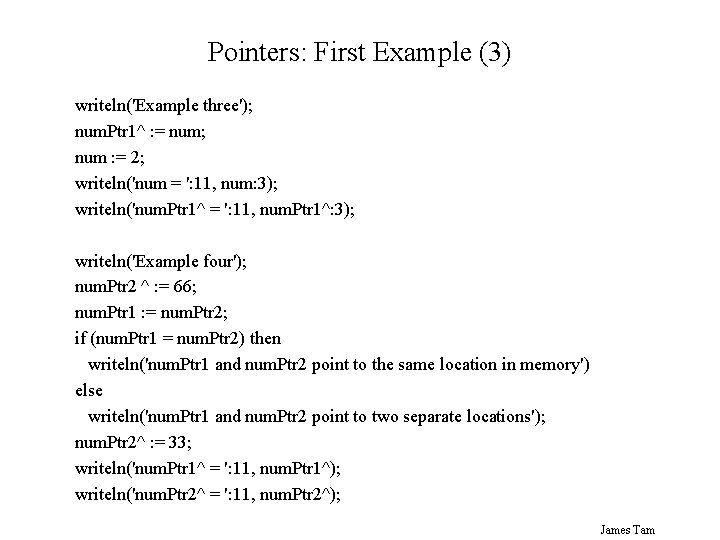
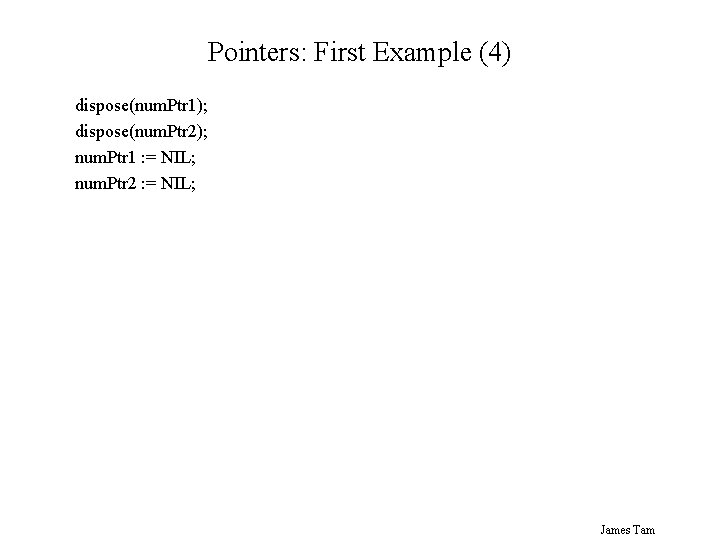
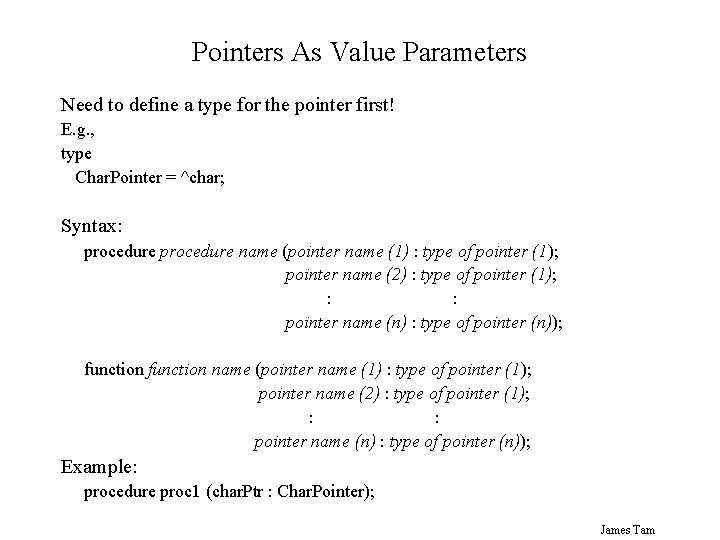
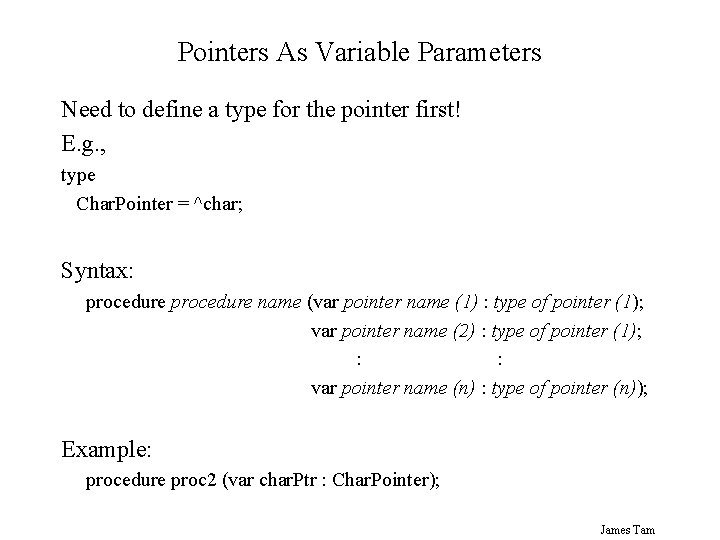
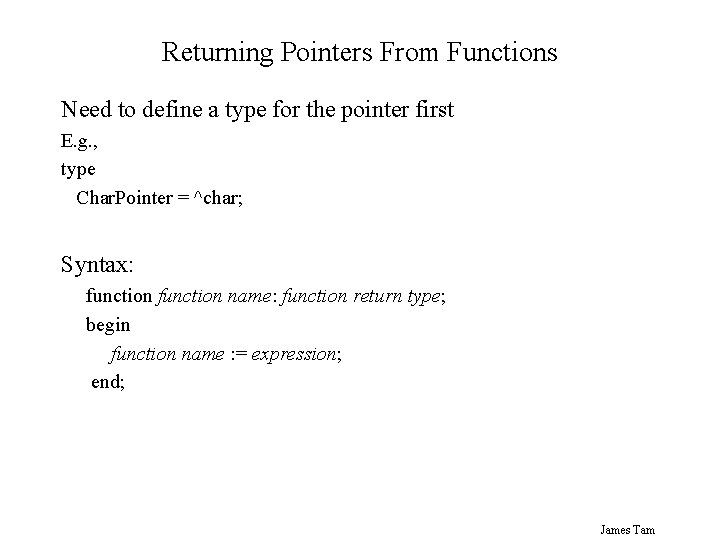
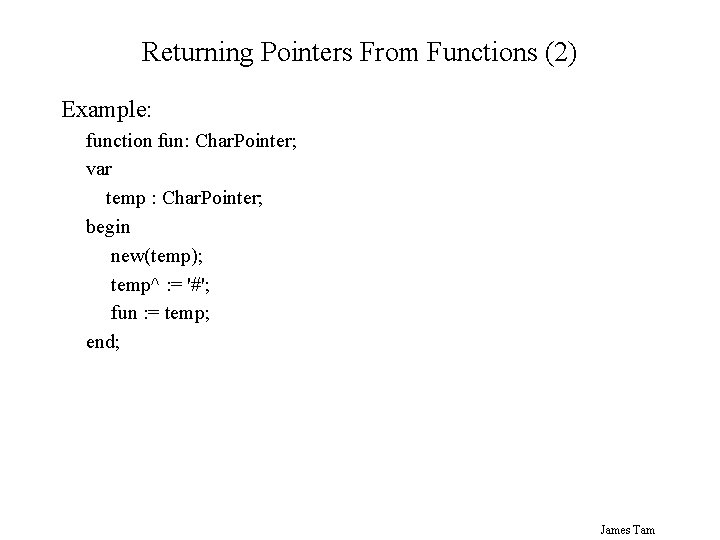
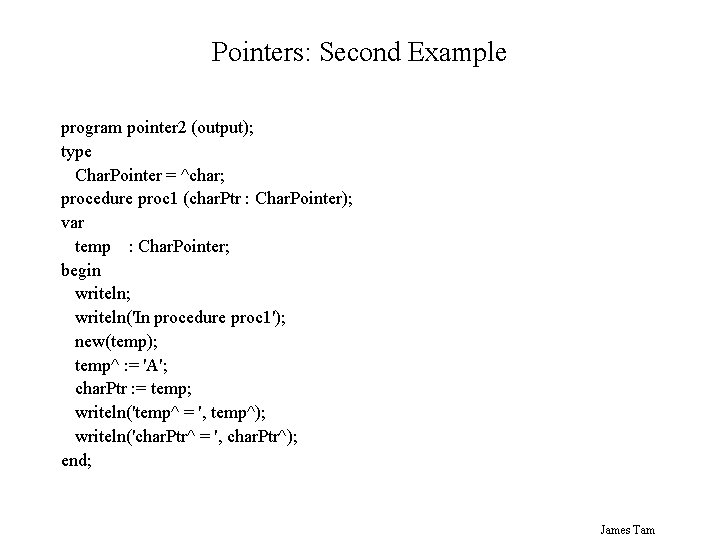
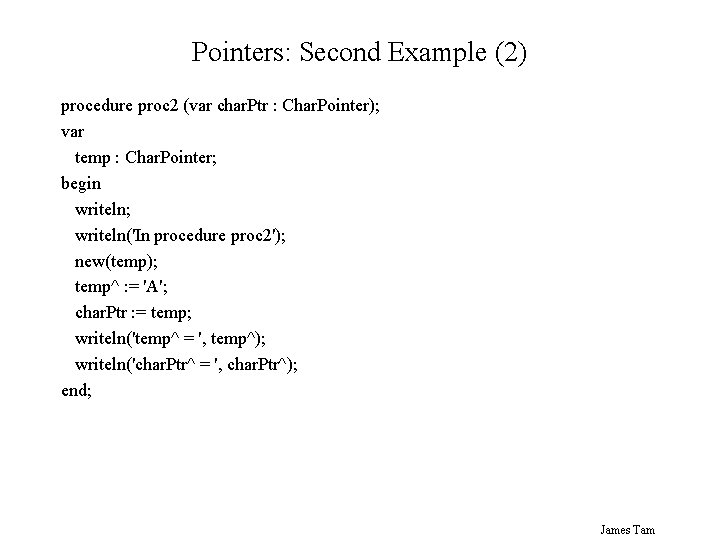
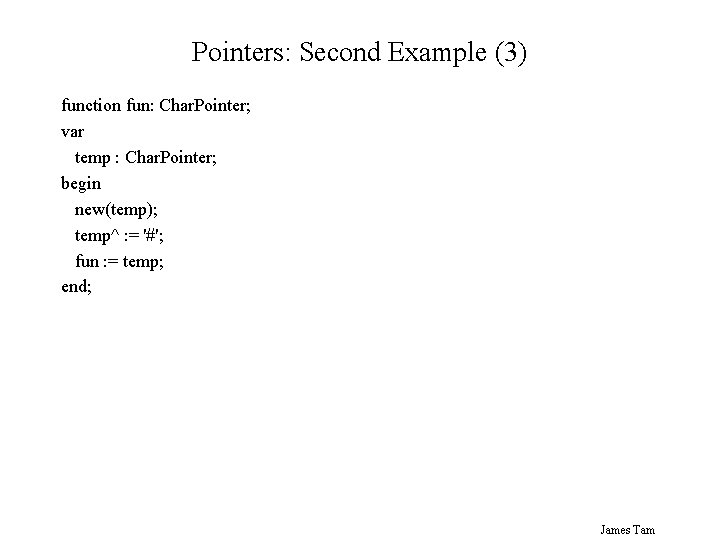
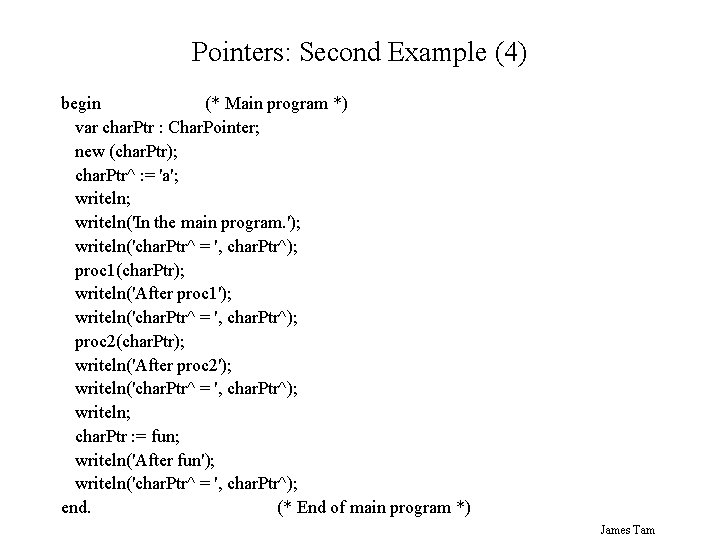
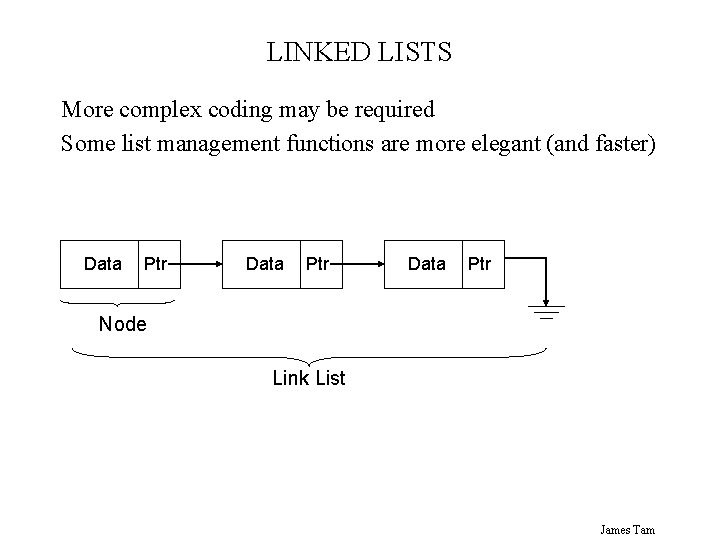
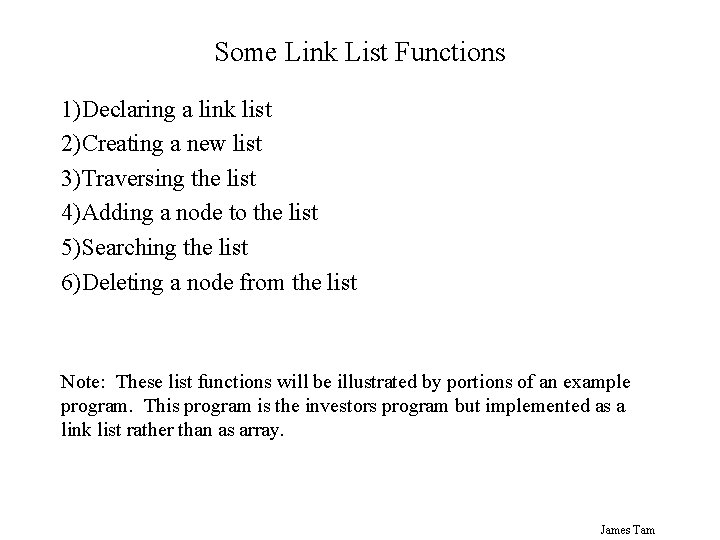
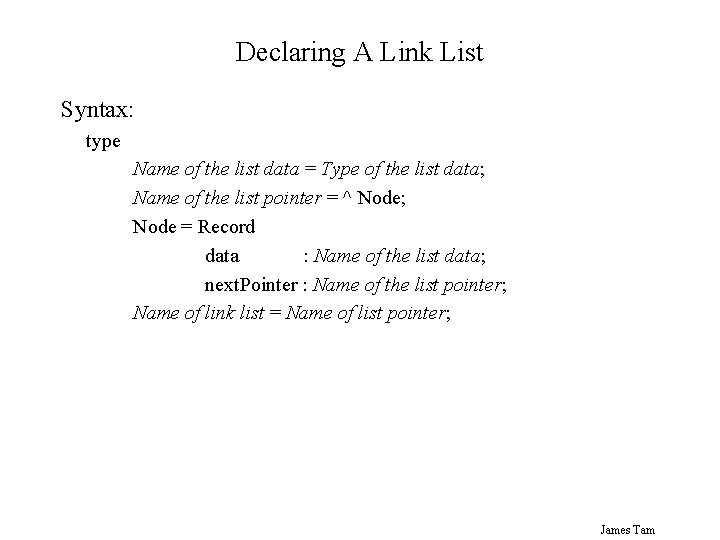
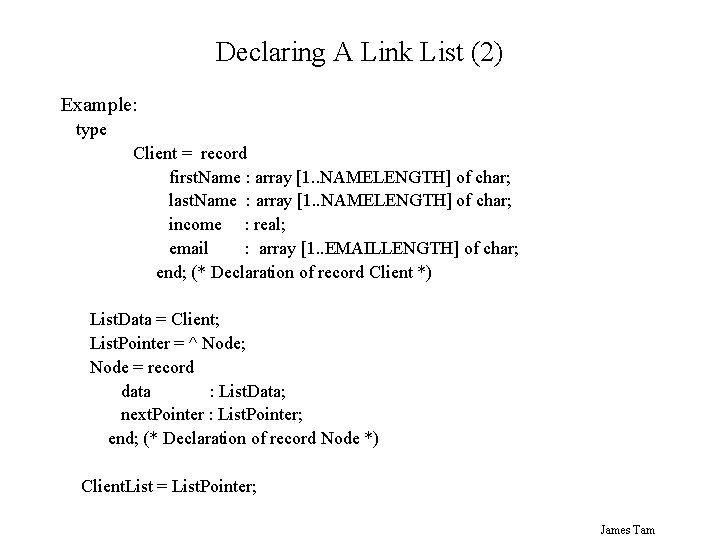
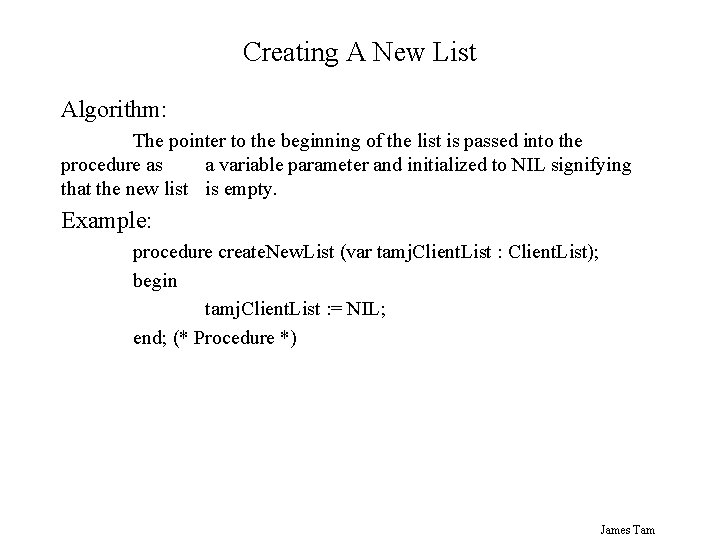
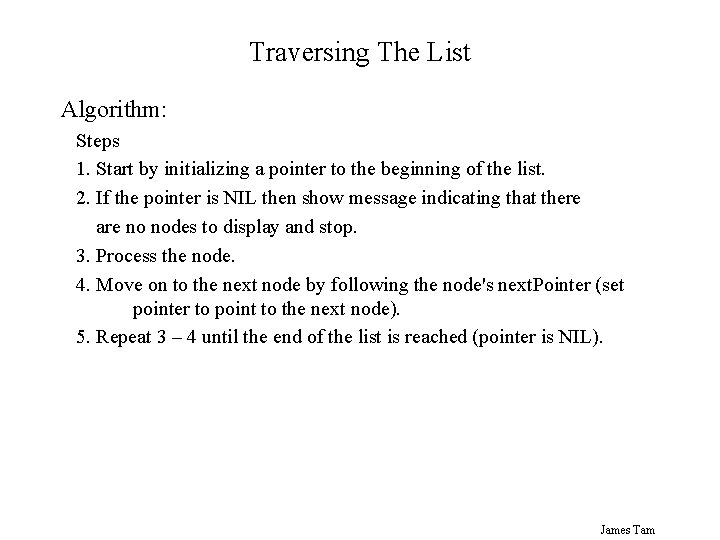
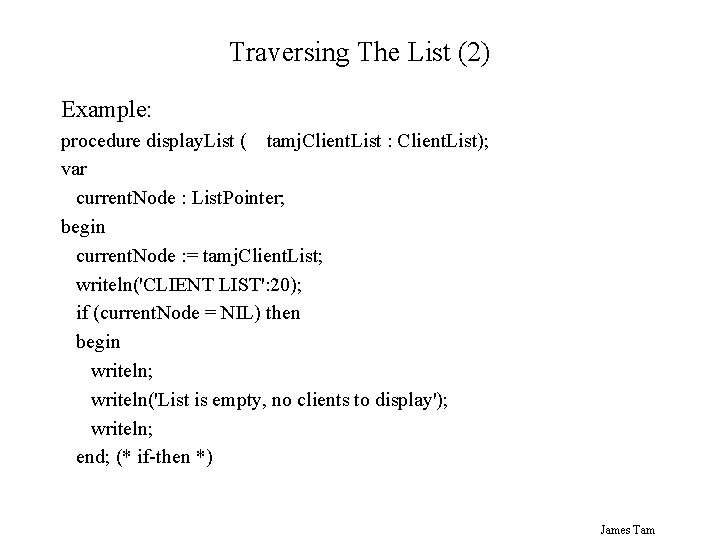
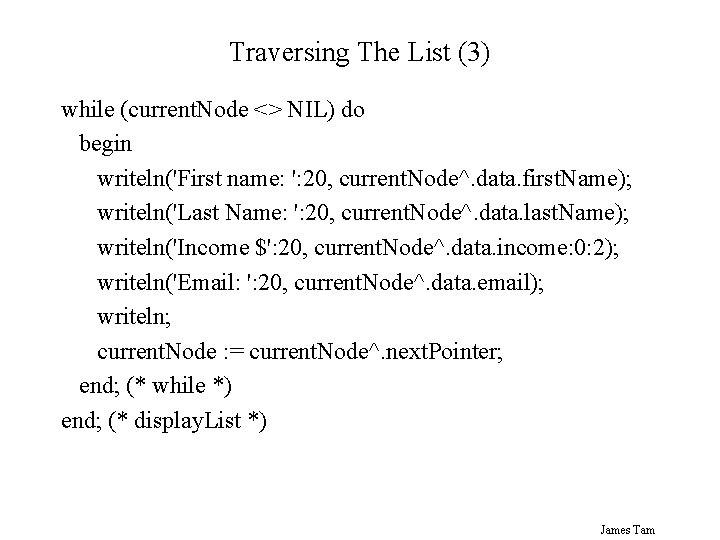
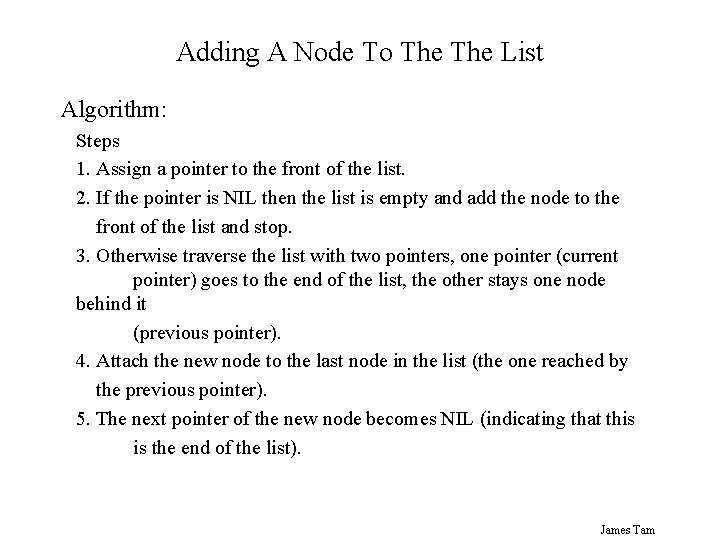
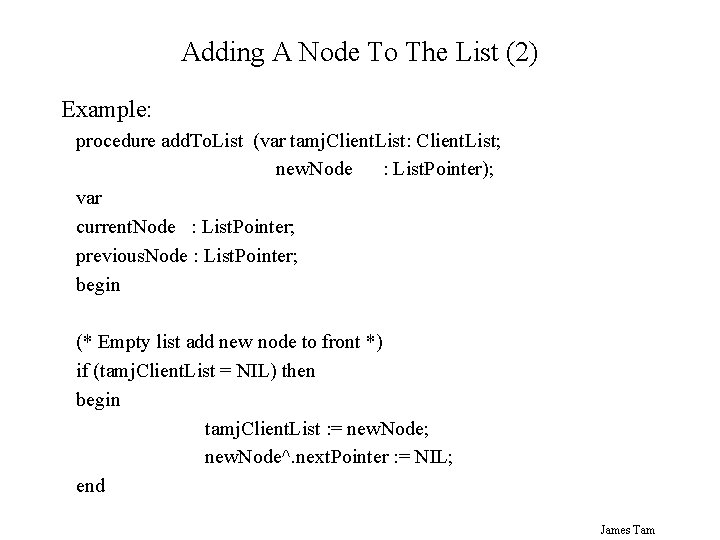
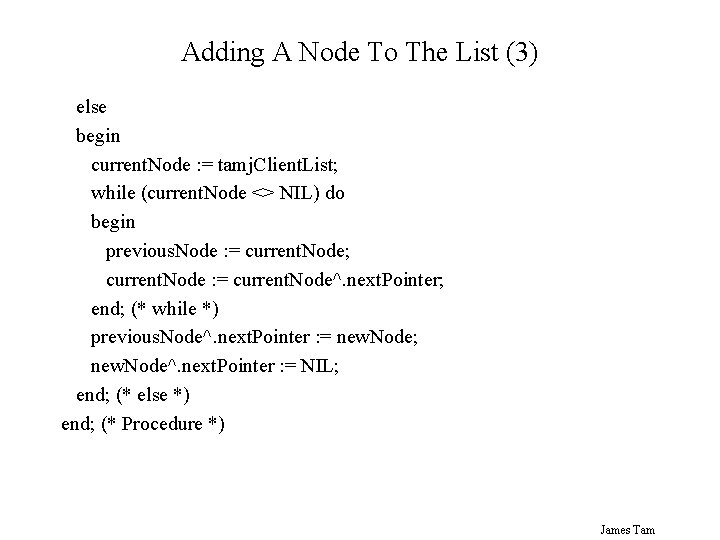
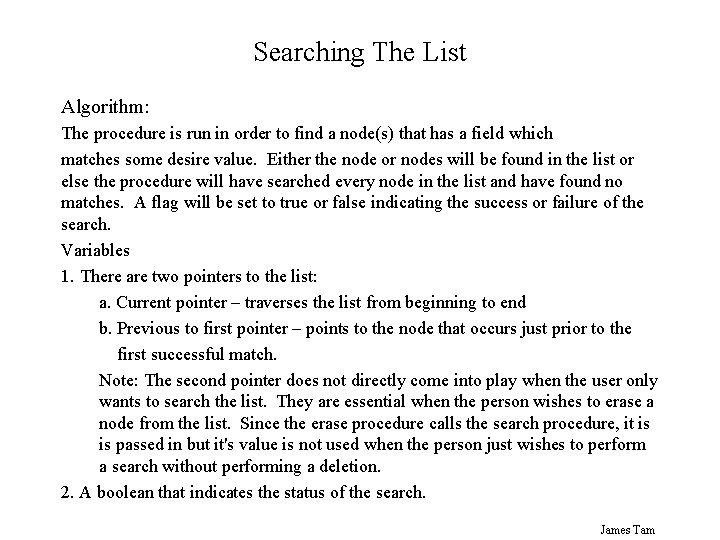
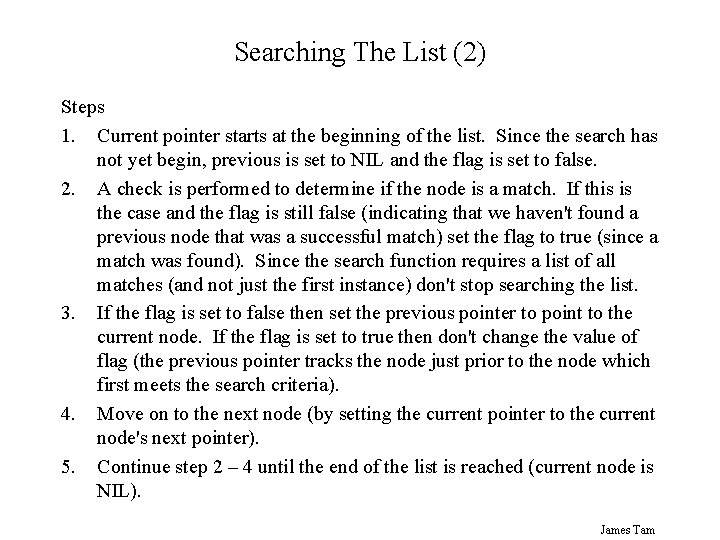
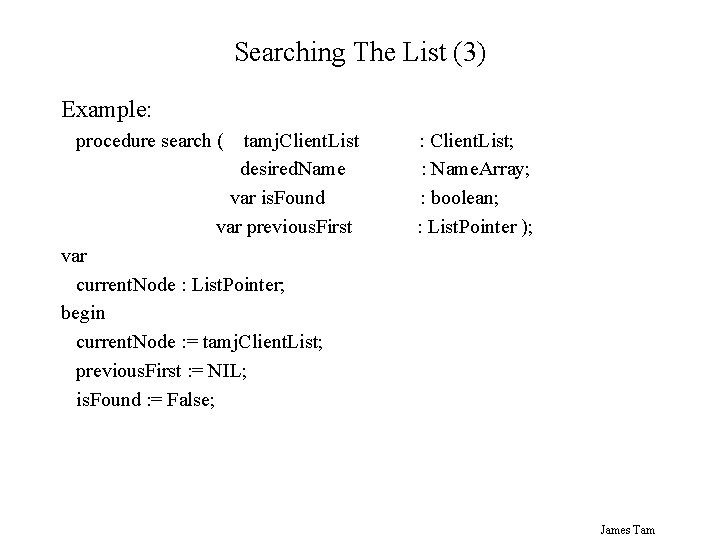
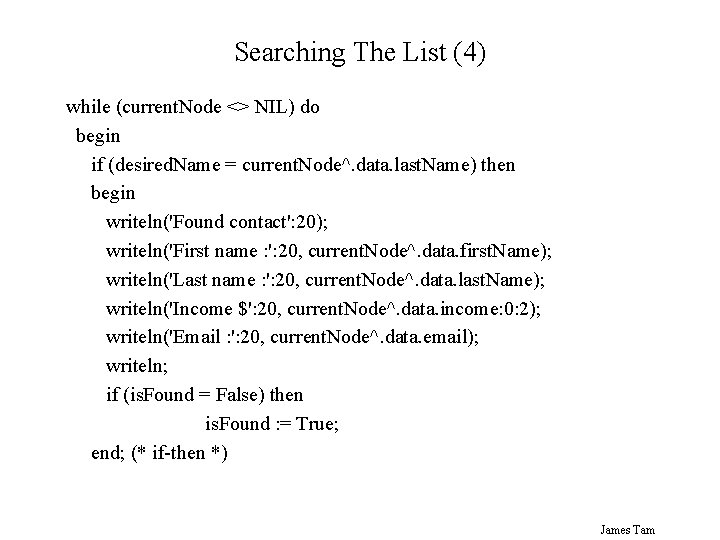
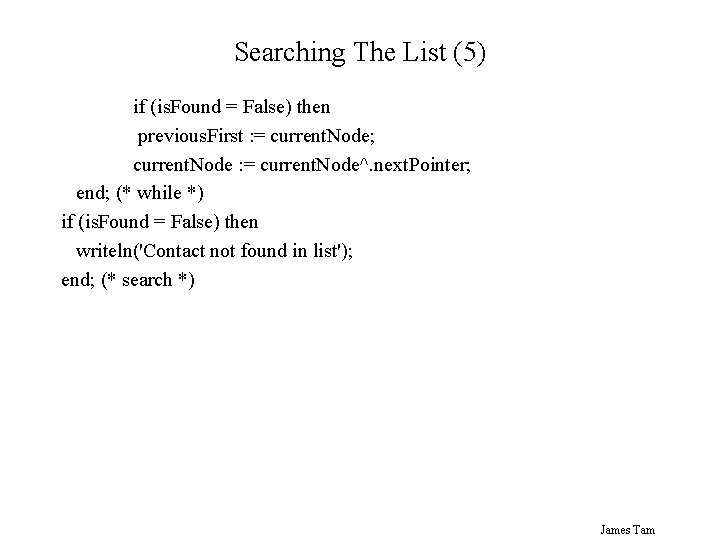
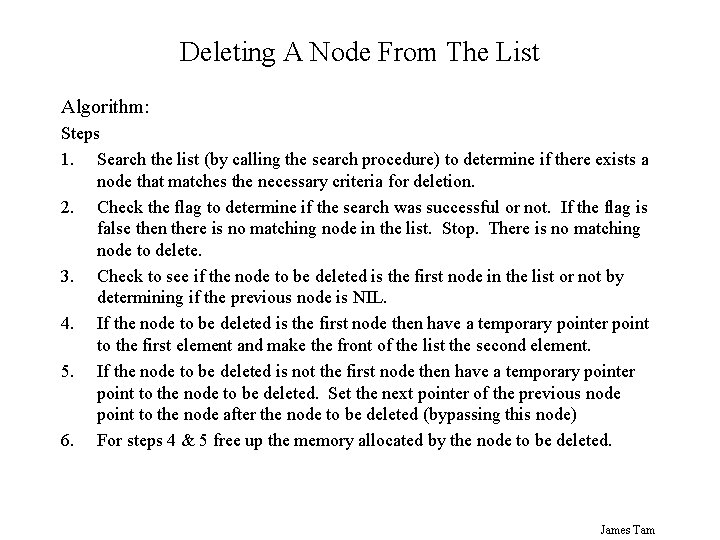
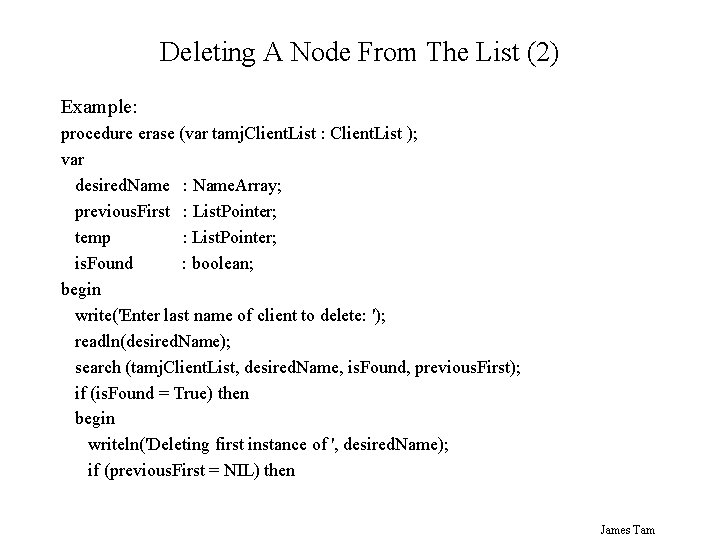
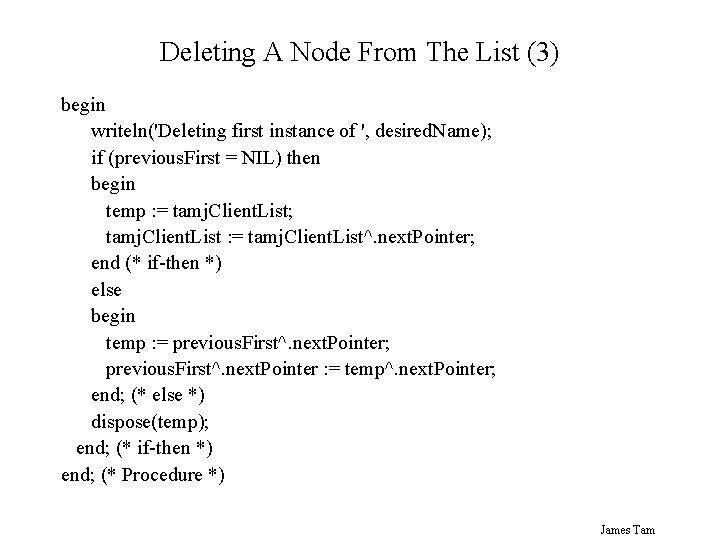
- Slides: 148
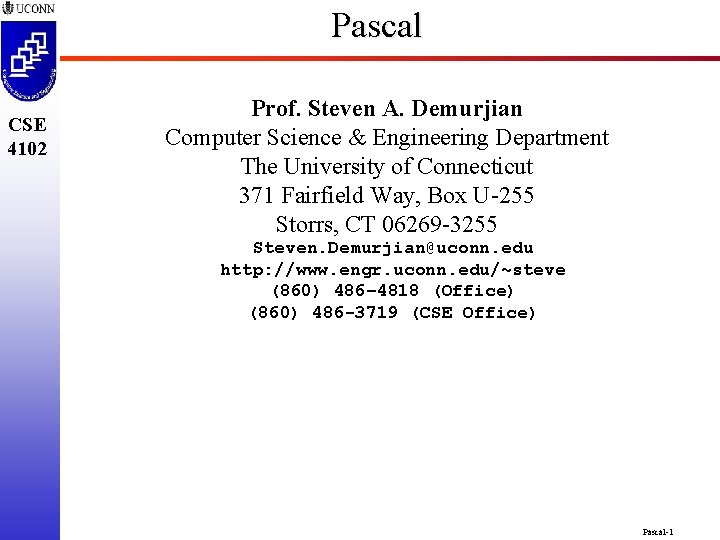
Pascal CSE 4102 Prof. Steven A. Demurjian Computer Science & Engineering Department The University of Connecticut 371 Fairfield Way, Box U-255 Storrs, CT 06269 -3255 Steven. Demurjian@uconn. edu http: //www. engr. uconn. edu/~steve (860) 486– 4818 (Office) (860) 486 -3719 (CSE Office) Pascal-1
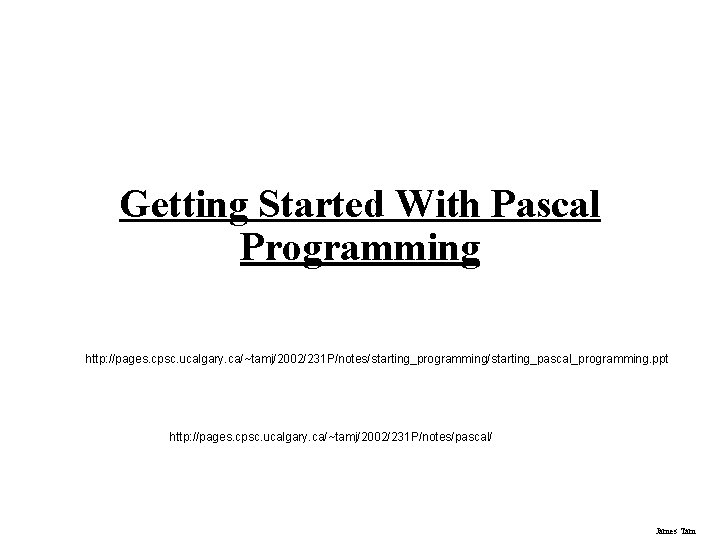
Getting Started With Pascal Programming http: //pages. cpsc. ucalgary. ca/~tamj/2002/231 P/notes/starting_programming/starting_pascal_programming. ppt http: //pages. cpsc. ucalgary. ca/~tamj/2002/231 P/notes/pascal/ James Tam
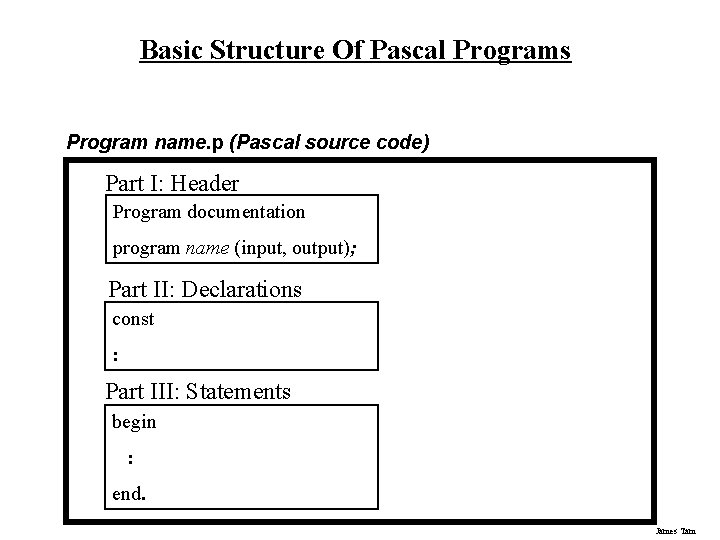
Basic Structure Of Pascal Programs Program name. p (Pascal source code) Part I: Header Program documentation program name (input, output); Part II: Declarations const : Part III: Statements begin : end. James Tam
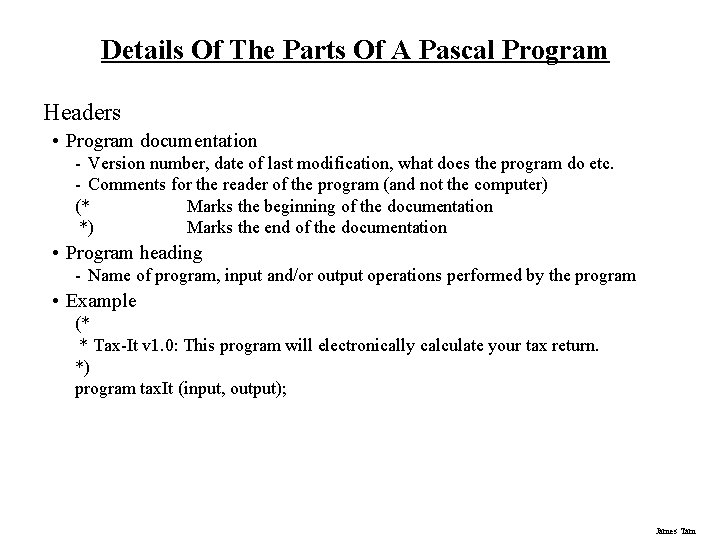
Details Of The Parts Of A Pascal Program Headers • Program documentation - Version number, date of last modification, what does the program do etc. - Comments for the reader of the program (and not the computer) (* Marks the beginning of the documentation *) Marks the end of the documentation • Program heading - Name of program, input and/or output operations performed by the program • Example (* * Tax-It v 1. 0: This program will electronically calculate your tax return. *) program tax. It (input, output); James Tam
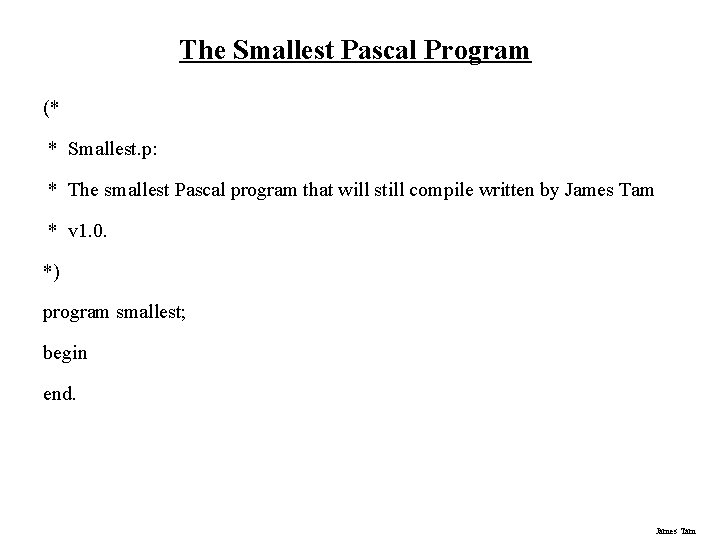
The Smallest Pascal Program (* * Smallest. p: * The smallest Pascal program that will still compile written by James Tam * v 1. 0. *) program smallest; begin end. James Tam
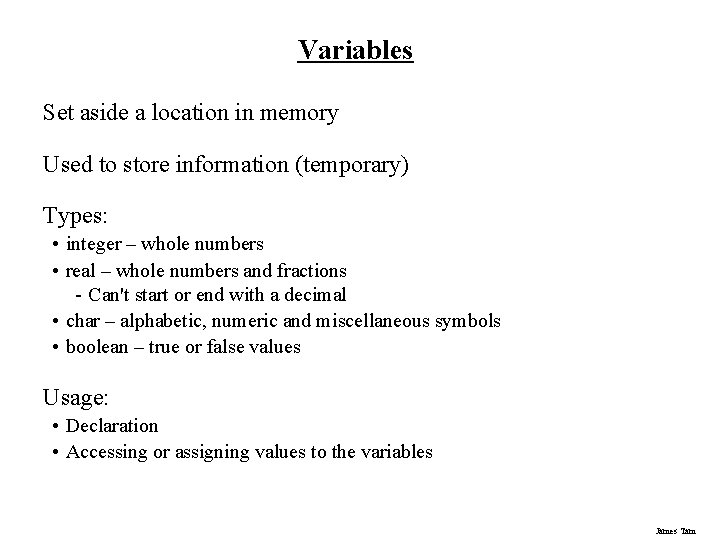
Variables Set aside a location in memory Used to store information (temporary) Types: • integer – whole numbers • real – whole numbers and fractions - Can't start or end with a decimal • char – alphabetic, numeric and miscellaneous symbols • boolean – true or false values Usage: • Declaration • Accessing or assigning values to the variables James Tam
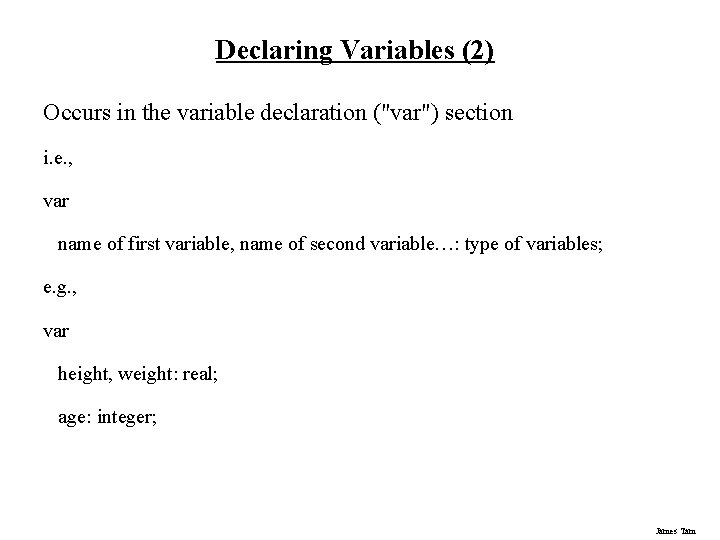
Declaring Variables (2) Occurs in the variable declaration ("var") section i. e. , var name of first variable, name of second variable…: type of variables; e. g. , var height, weight: real; age: integer; James Tam
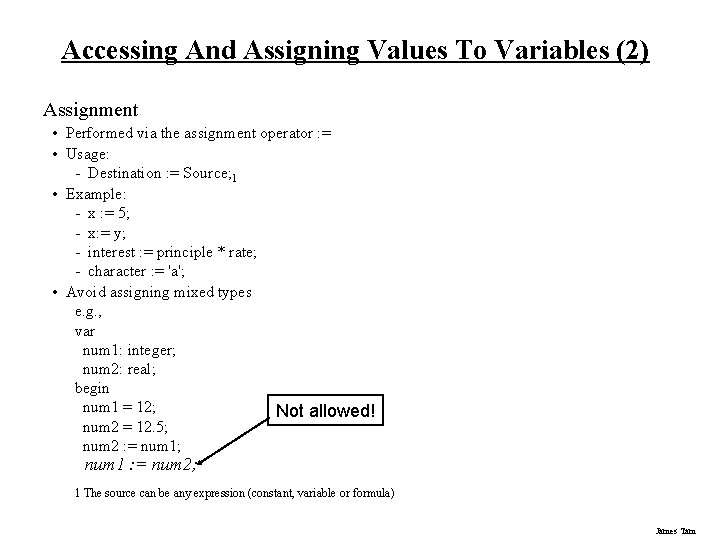
Accessing And Assigning Values To Variables (2) Assignment • Performed via the assignment operator : = • Usage: - Destination : = Source; 1 • Example: - x : = 5; - x: = y; - interest : = principle * rate; - character : = 'a'; • Avoid assigning mixed types e. g. , var num 1: integer; num 2: real; begin num 1 = 12; Not allowed! num 2 = 12. 5; num 2 : = num 1; num 1 : = num 2; 1 The source can be any expression (constant, variable or formula) James Tam
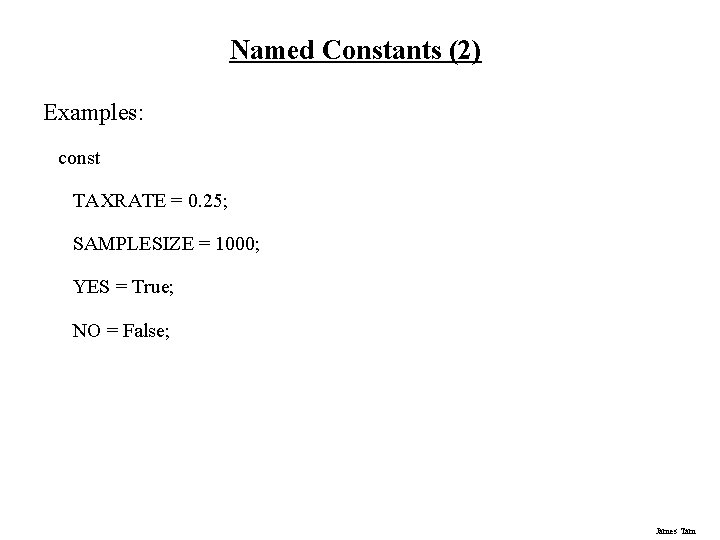
Named Constants (2) Examples: const TAXRATE = 0. 25; SAMPLESIZE = 1000; YES = True; NO = False; James Tam
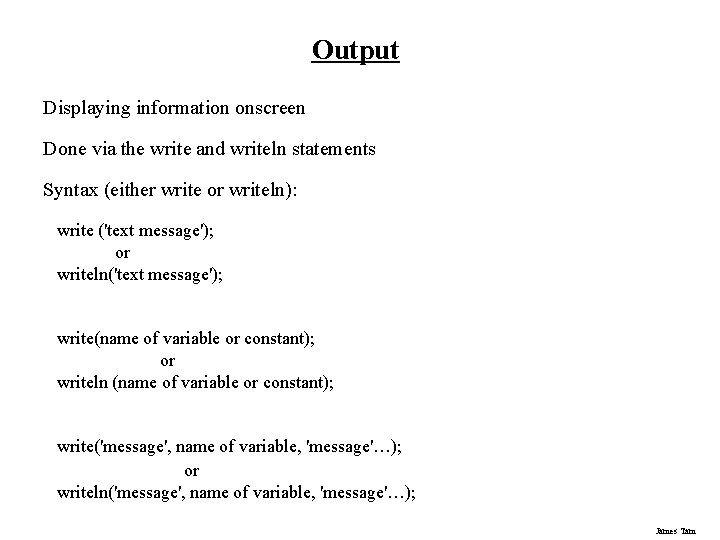
Output Displaying information onscreen Done via the write and writeln statements Syntax (either write or writeln): write ('text message'); or writeln('text message'); write(name of variable or constant); or writeln (name of variable or constant); write('message', name of variable, 'message'…); or writeln('message', name of variable, 'message'…); James Tam
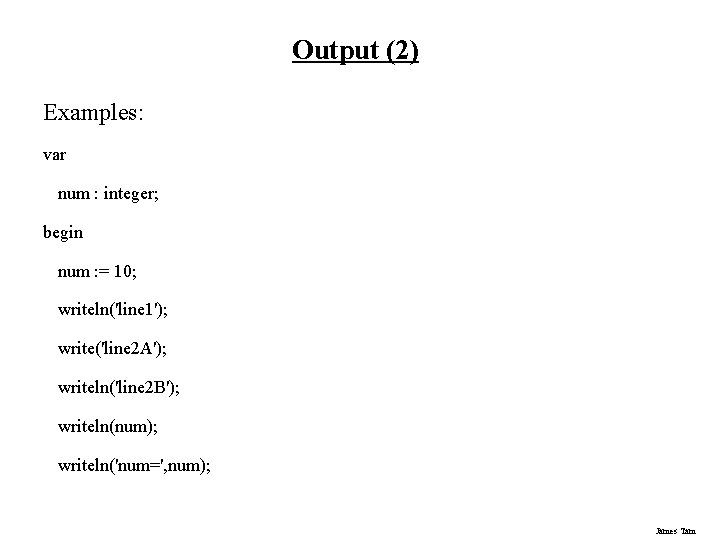
Output (2) Examples: var num : integer; begin num : = 10; writeln('line 1'); write('line 2 A'); writeln('line 2 B'); writeln(num); writeln('num=', num); James Tam
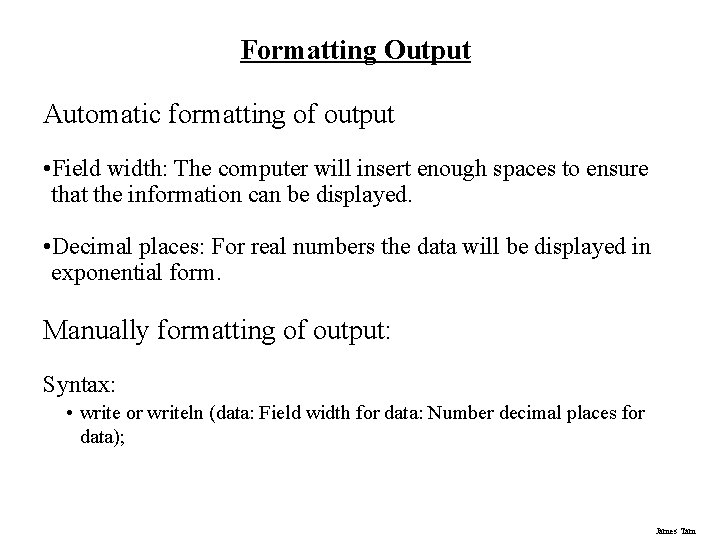
Formatting Output Automatic formatting of output • Field width: The computer will insert enough spaces to ensure that the information can be displayed. • Decimal places: For real numbers the data will be displayed in exponential form. Manually formatting of output: Syntax: • write or writeln (data: Field width for data: Number decimal places for data); James Tam
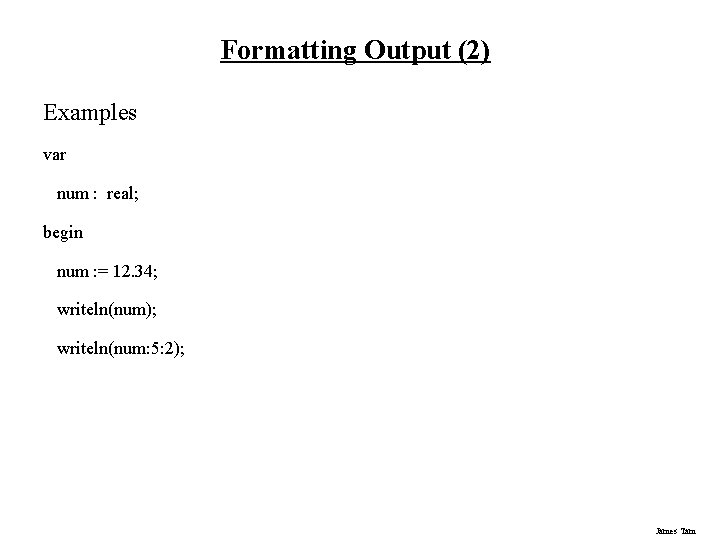
Formatting Output (2) Examples var num : real; begin num : = 12. 34; writeln(num); writeln(num: 5: 2); James Tam
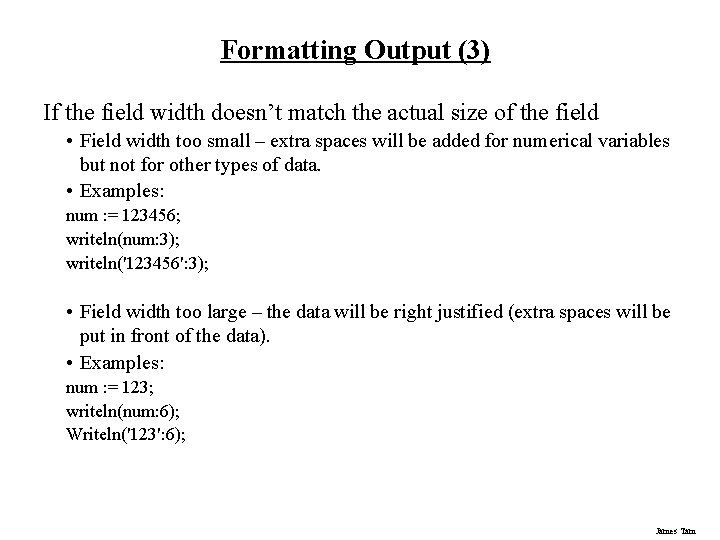
Formatting Output (3) If the field width doesn’t match the actual size of the field • Field width too small – extra spaces will be added for numerical variables but not for other types of data. • Examples: num : = 123456; writeln(num: 3); writeln('123456': 3); • Field width too large – the data will be right justified (extra spaces will be put in front of the data). • Examples: num : = 123; writeln(num: 6); Writeln('123': 6); James Tam
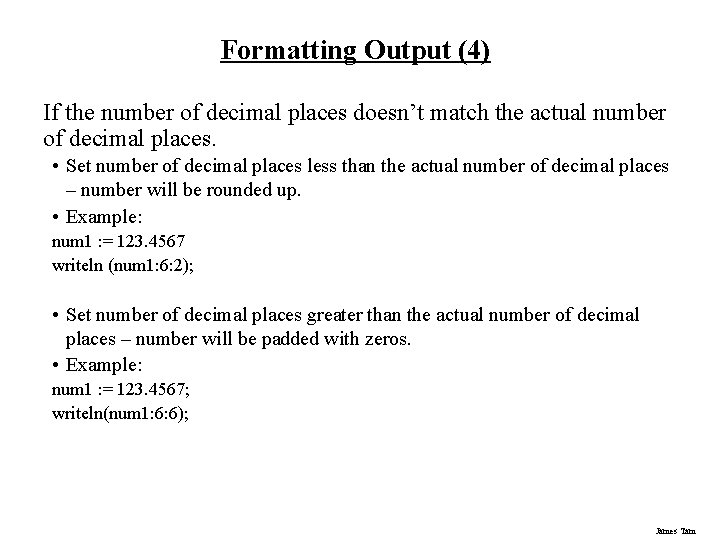
Formatting Output (4) If the number of decimal places doesn’t match the actual number of decimal places. • Set number of decimal places less than the actual number of decimal places – number will be rounded up. • Example: num 1 : = 123. 4567 writeln (num 1: 6: 2); • Set number of decimal places greater than the actual number of decimal places – number will be padded with zeros. • Example: num 1 : = 123. 4567; writeln(num 1: 6: 6); James Tam
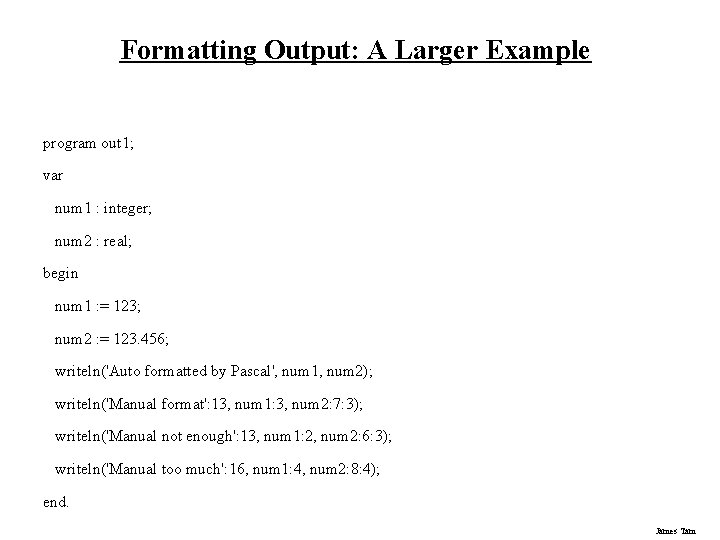
Formatting Output: A Larger Example program out 1; var num 1 : integer; num 2 : real; begin num 1 : = 123; num 2 : = 123. 456; writeln('Auto formatted by Pascal', num 1, num 2); writeln('Manual format': 13, num 1: 3, num 2: 7: 3); writeln('Manual not enough': 13, num 1: 2, num 2: 6: 3); writeln('Manual too much': 16, num 1: 4, num 2: 8: 4); end. James Tam
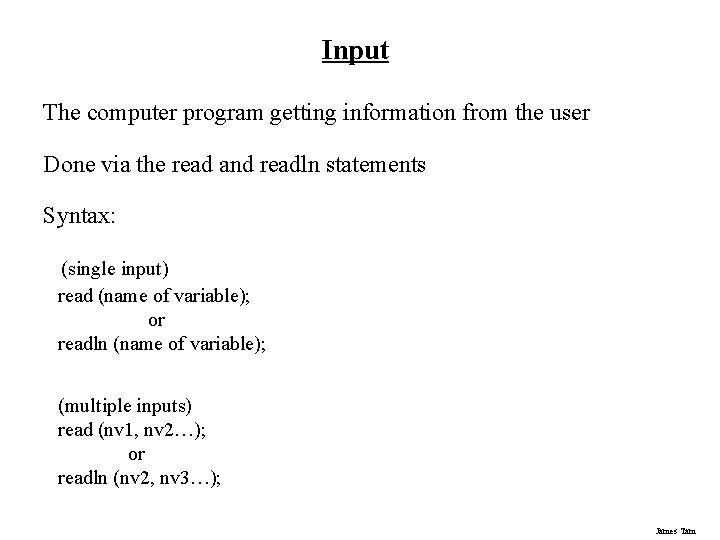
Input The computer program getting information from the user Done via the read and readln statements Syntax: (single input) read (name of variable); or readln (name of variable); (multiple inputs) read (nv 1, nv 2…); or readln (nv 2, nv 3…); James Tam
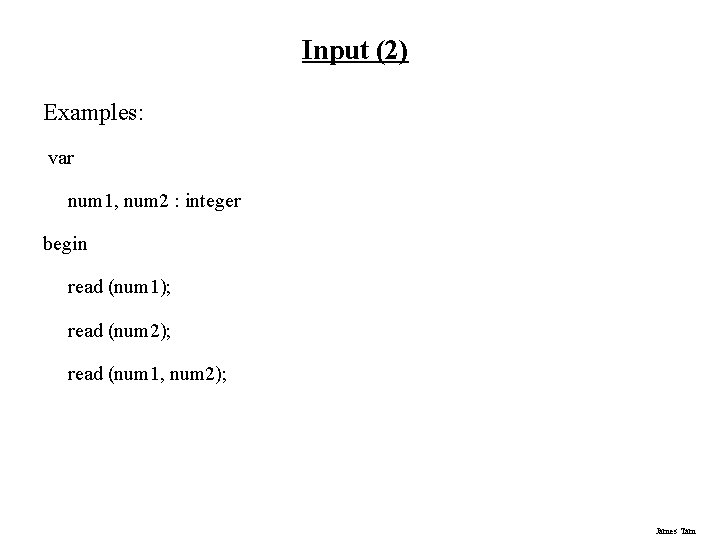
Input (2) Examples: var num 1, num 2 : integer begin read (num 1); read (num 2); read (num 1, num 2); James Tam
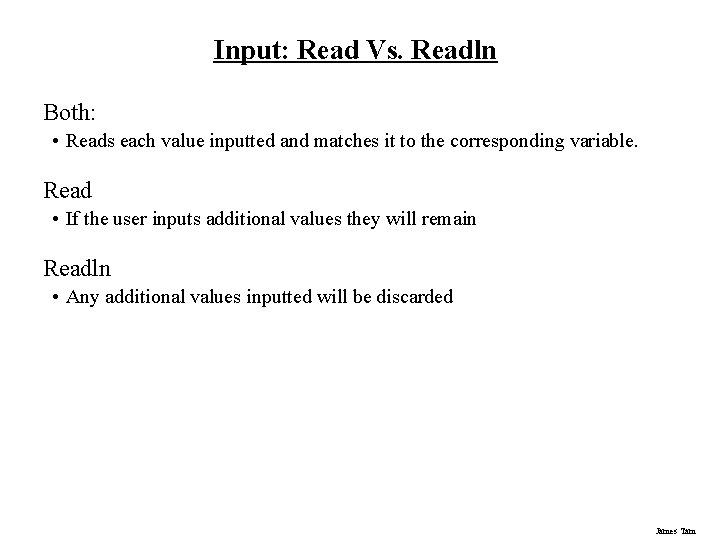
Input: Read Vs. Readln Both: • Reads each value inputted and matches it to the corresponding variable. Read • If the user inputs additional values they will remain Readln • Any additional values inputted will be discarded James Tam
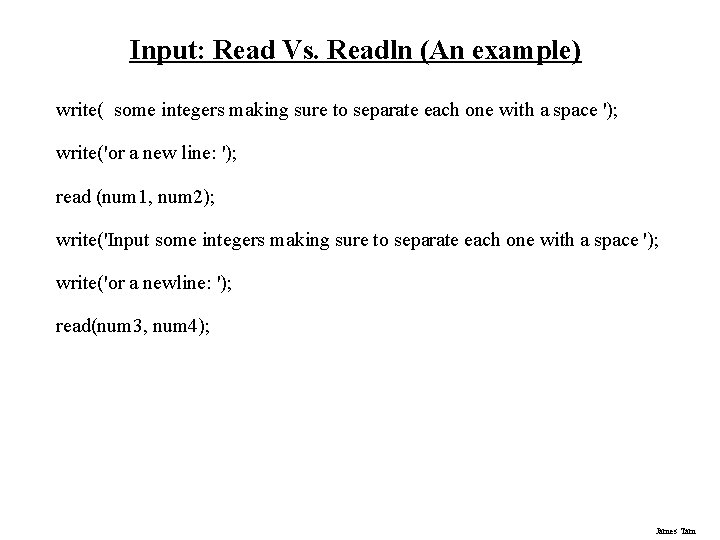
Input: Read Vs. Readln (An example) write( some integers making sure to separate each one with a space '); write('or a new line: '); read (num 1, num 2); write('Input some integers making sure to separate each one with a space '); write('or a newline: '); read(num 3, num 4); James Tam
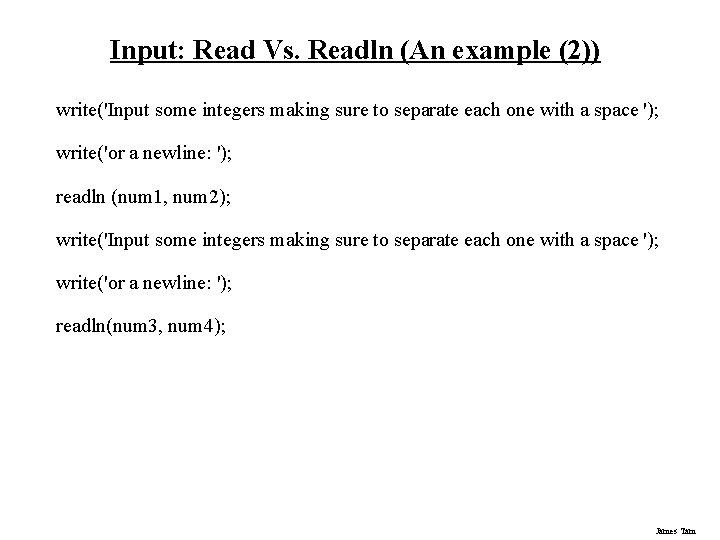
Input: Read Vs. Readln (An example (2)) write('Input some integers making sure to separate each one with a space '); write('or a newline: '); readln (num 1, num 2); write('Input some integers making sure to separate each one with a space '); write('or a newline: '); readln(num 3, num 4); James Tam
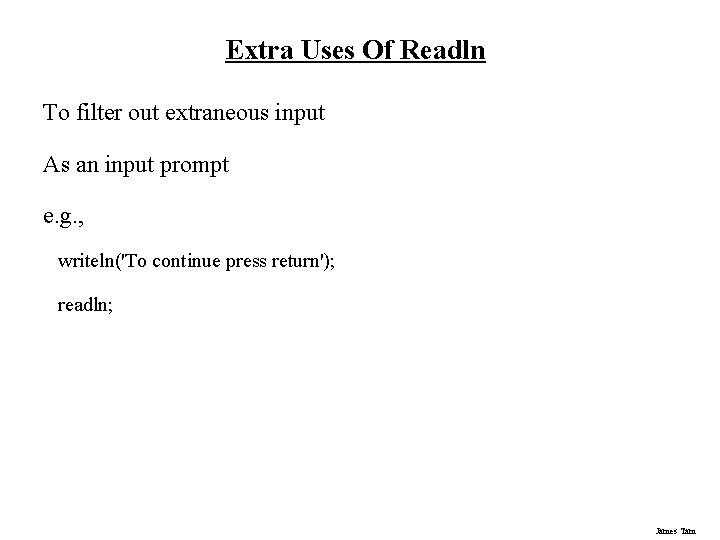
Extra Uses Of Readln To filter out extraneous input As an input prompt e. g. , writeln('To continue press return'); readln; James Tam
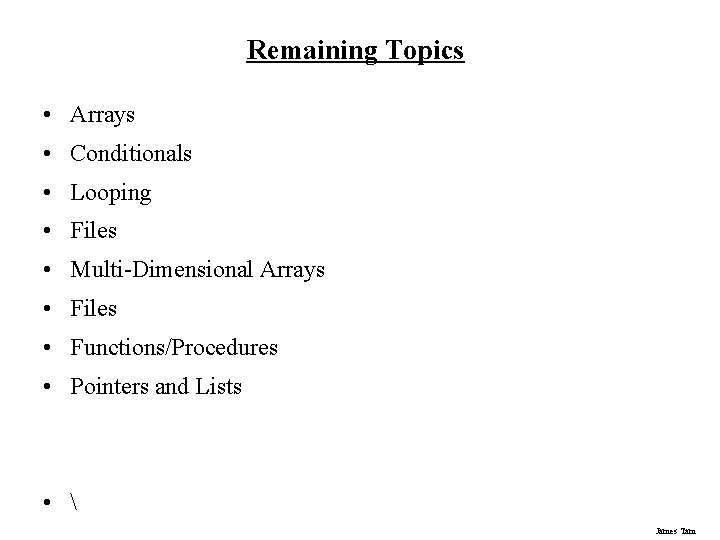
Remaining Topics • Arrays • Conditionals • Looping • Files • Multi-Dimensional Arrays • Files • Functions/Procedures • Pointers and Lists • James Tam
![Arrays const CLASSSIZE 5 var class Grades array 1 CLASSSIZE of Arrays const CLASSSIZE = 5; var class. Grades : array [1. . CLASSSIZE] of](https://slidetodoc.com/presentation_image_h2/ed92b301b7f9d83e665b7d2596230111/image-24.jpg)
Arrays const CLASSSIZE = 5; var class. Grades : array [1. . CLASSSIZE] of real; i : integer; total, average : real; begin total : = 0; James Tam
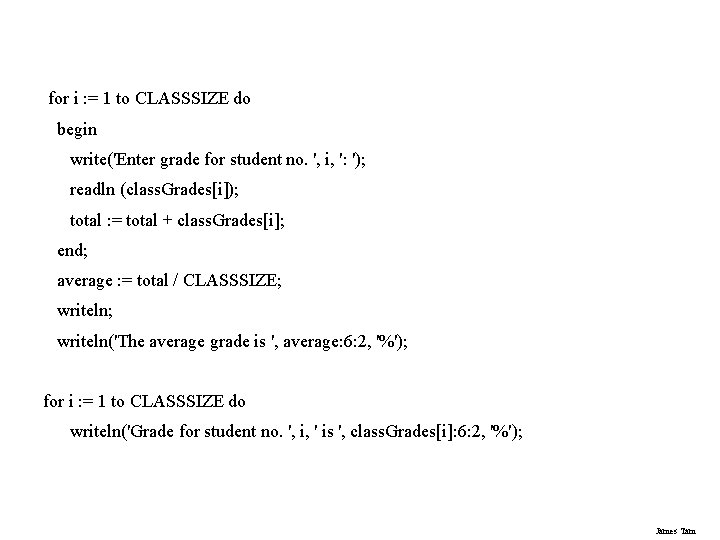
for i : = 1 to CLASSSIZE do begin write('Enter grade for student no. ', i, ': '); readln (class. Grades[i]); total : = total + class. Grades[i]; end; average : = total / CLASSSIZE; writeln('The average grade is ', average: 6: 2, '%'); for i : = 1 to CLASSSIZE do writeln('Grade for student no. ', i, ' is ', class. Grades[i]: 6: 2, '%'); James Tam
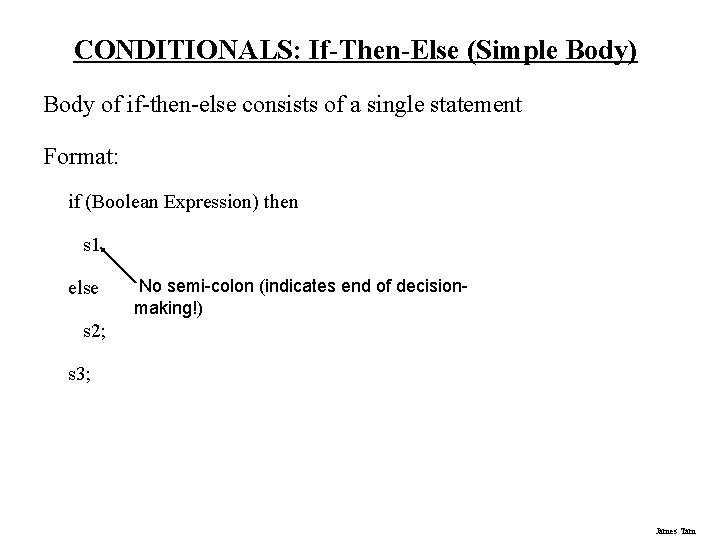
CONDITIONALS: If-Then-Else (Simple Body) Body of if-then-else consists of a single statement Format: if (Boolean Expression) then s 1 else No semi-colon (indicates end of decisionmaking!) s 2; s 3; James Tam
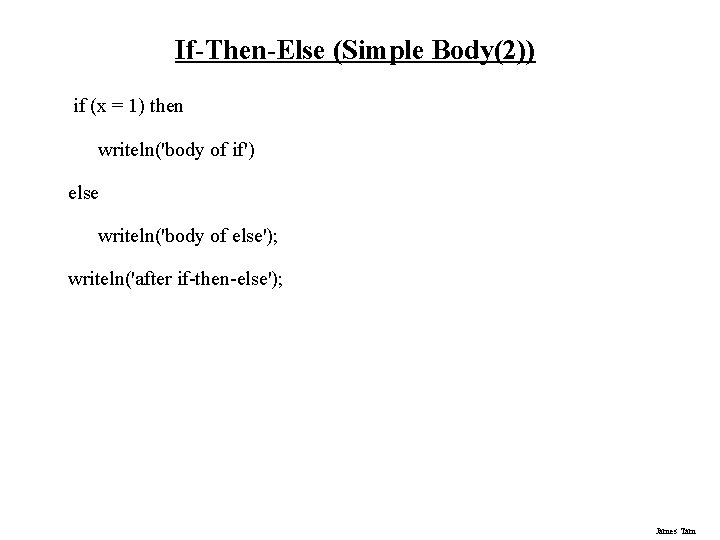
If-Then-Else (Simple Body(2)) if (x = 1) then writeln('body of if') else writeln('body of else'); writeln('after if-then-else'); James Tam
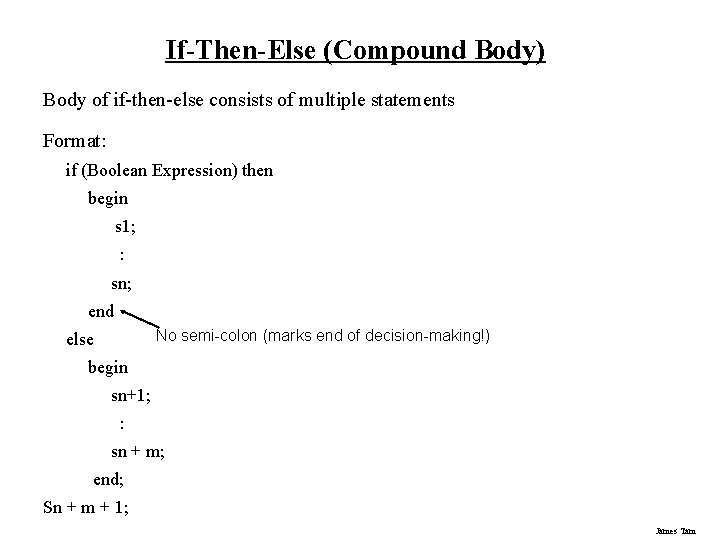
If-Then-Else (Compound Body) Body of if-then-else consists of multiple statements Format: if (Boolean Expression) then begin s 1; : sn; end No semi-colon (marks end of decision-making!) else begin sn+1; : sn + m; end; Sn + m + 1; James Tam
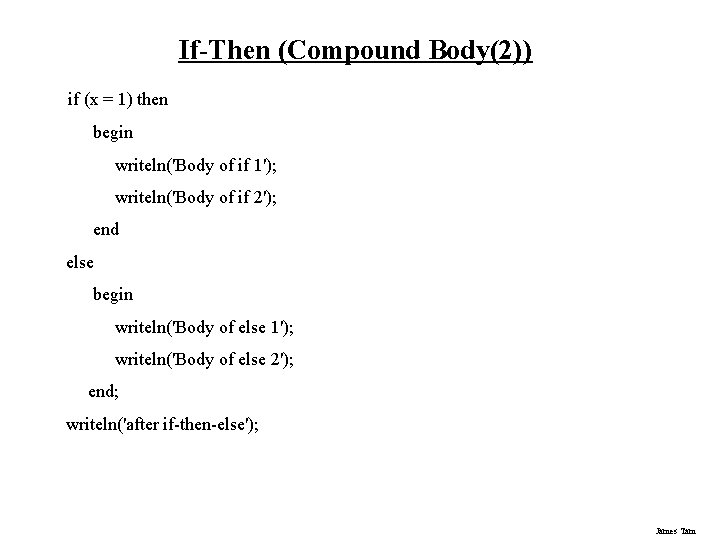
If-Then (Compound Body(2)) if (x = 1) then begin writeln('Body of if 1'); writeln('Body of if 2'); end else begin writeln('Body of else 1'); writeln('Body of else 2'); end; writeln('after if-then-else'); James Tam
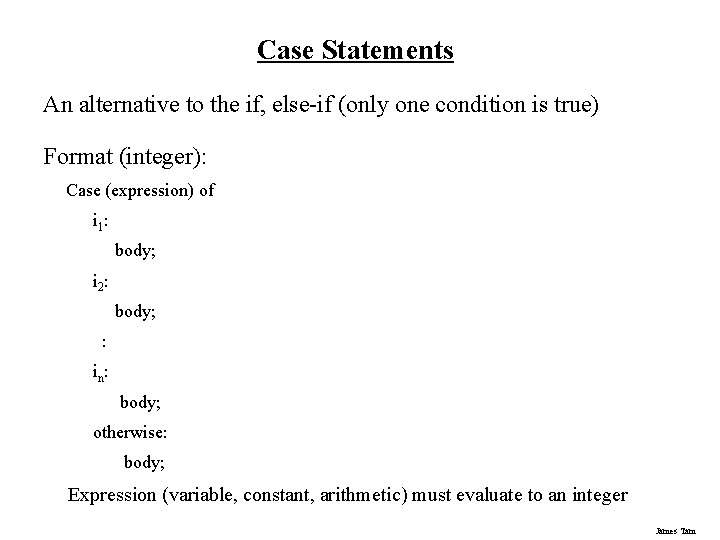
Case Statements An alternative to the if, else-if (only one condition is true) Format (integer): Case (expression) of i 1 : body; i 2 : body; : in : body; otherwise: body; Expression (variable, constant, arithmetic) must evaluate to an integer James Tam
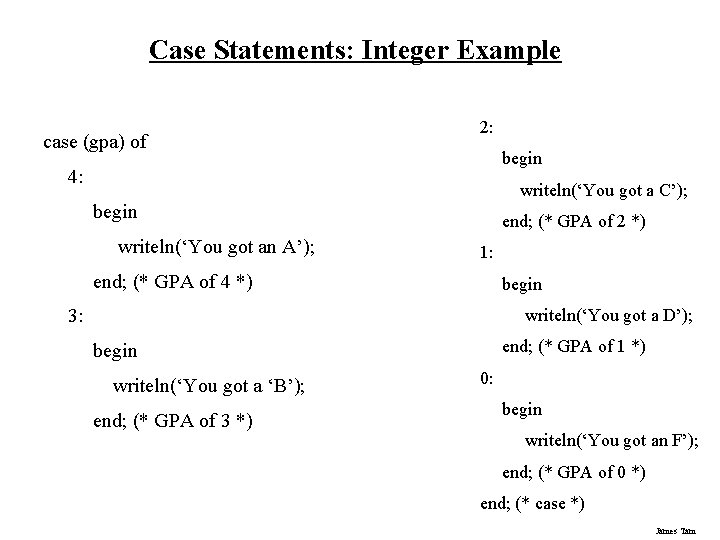
Case Statements: Integer Example case (gpa) of 2: begin 4: writeln(‘You got a C’); begin writeln(‘You got an A’); end; (* GPA of 2 *) 1: end; (* GPA of 4 *) begin 3: writeln(‘You got a D’); end; (* GPA of 1 *) begin writeln(‘You got a ‘B’); end; (* GPA of 3 *) 0: begin writeln(‘You got an F’); end; (* GPA of 0 *) end; (* case *) James Tam
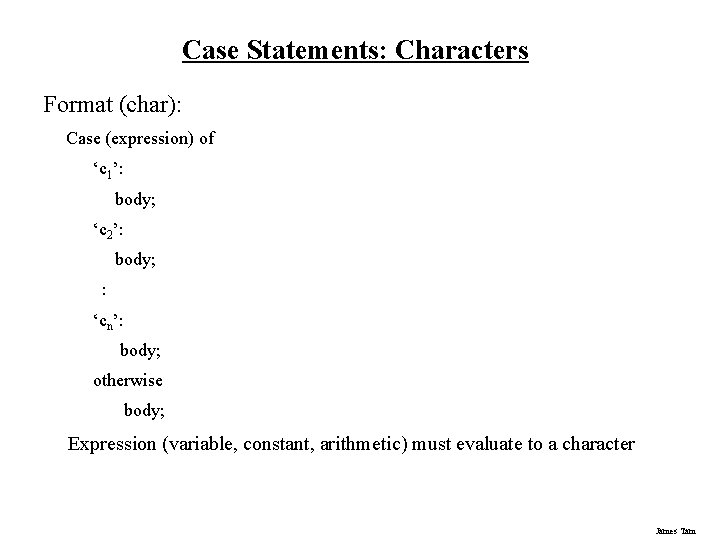
Case Statements: Characters Format (char): Case (expression) of ‘c 1’: body; ‘c 2’: body; : ‘cn’: body; otherwise body; Expression (variable, constant, arithmetic) must evaluate to a character James Tam
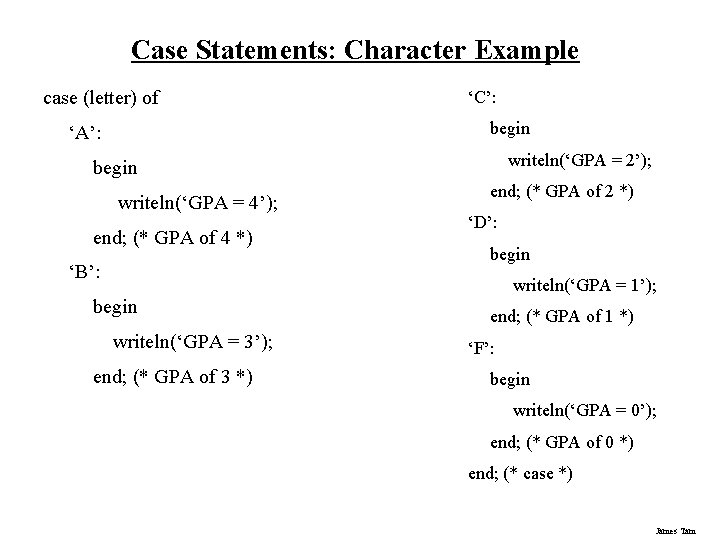
Case Statements: Character Example case (letter) of ‘C’: begin ‘A’: writeln(‘GPA = 2’); begin writeln(‘GPA = 4’); end; (* GPA of 4 *) ‘B’: end; (* GPA of 2 *) ‘D’: begin writeln(‘GPA = 1’); begin writeln(‘GPA = 3’); end; (* GPA of 3 *) end; (* GPA of 1 *) ‘F’: begin writeln(‘GPA = 0’); end; (* GPA of 0 *) end; (* case *) James Tam
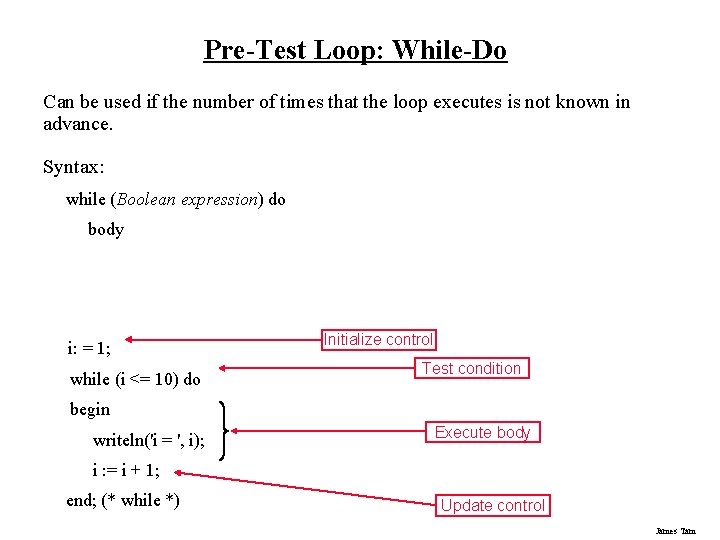
Pre-Test Loop: While-Do Can be used if the number of times that the loop executes is not known in advance. Syntax: while (Boolean expression) do body i: = 1; while (i <= 10) do Initialize control Test condition begin writeln('i = ', i); Execute body i : = i + 1; end; (* while *) Update control James Tam
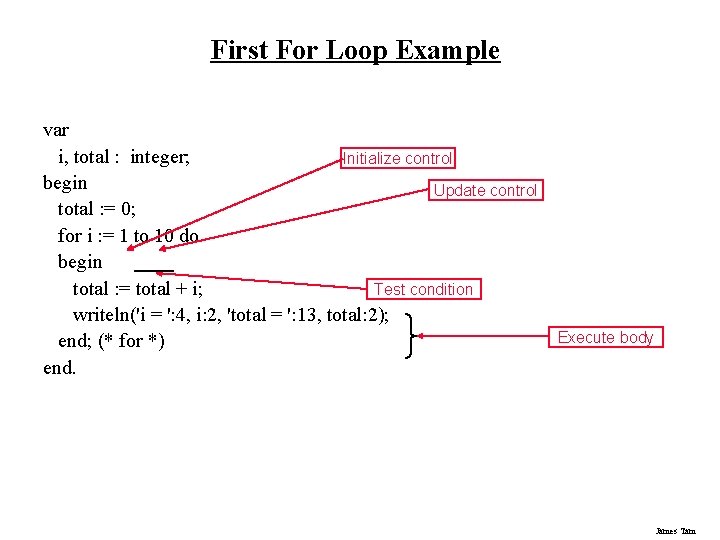
First For Loop Example var i, total : integer; Initialize control begin Update control total : = 0; for i : = 1 to 10 do begin Test condition total : = total + i; writeln('i = ': 4, i: 2, 'total = ': 13, total: 2); end; (* for *) end. Execute body James Tam
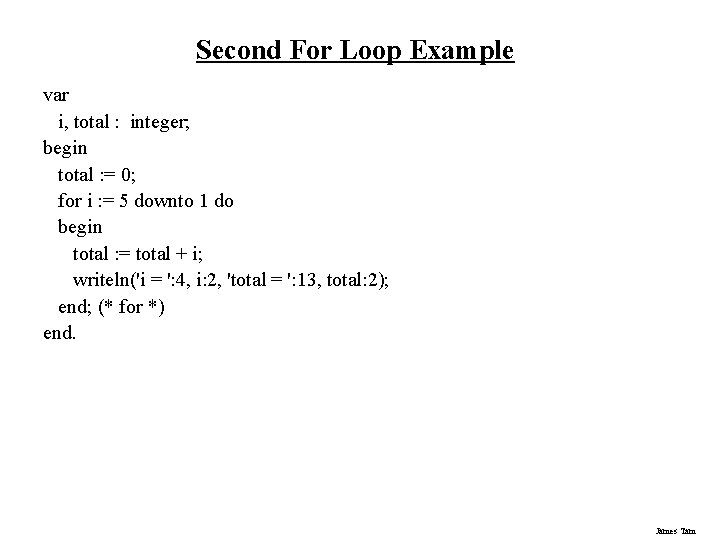
Second For Loop Example var i, total : integer; begin total : = 0; for i : = 5 downto 1 do begin total : = total + i; writeln('i = ': 4, i: 2, 'total = ': 13, total: 2); end; (* for *) end. James Tam
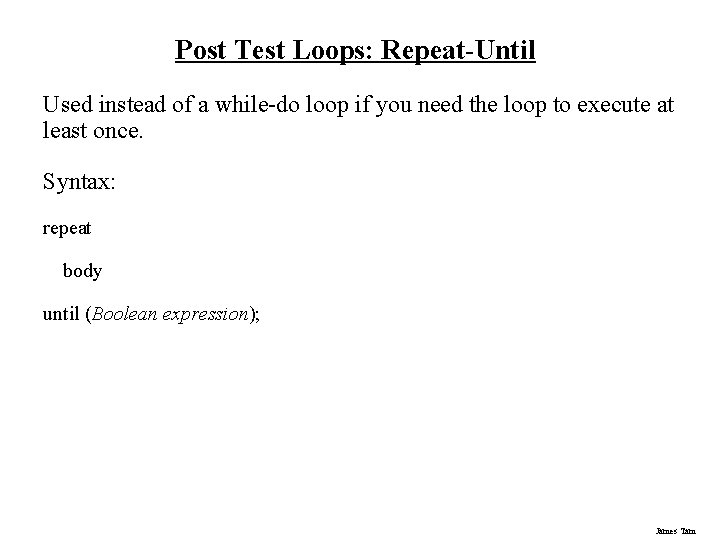
Post Test Loops: Repeat-Until Used instead of a while-do loop if you need the loop to execute at least once. Syntax: repeat body until (Boolean expression); James Tam
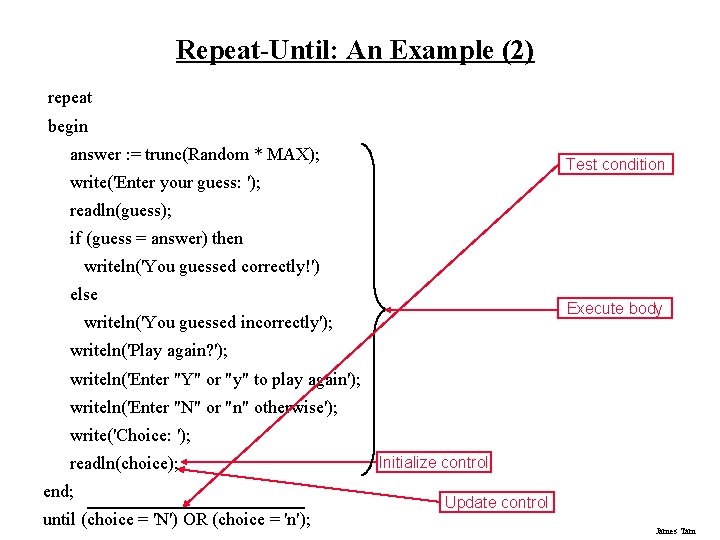
Repeat-Until: An Example (2) repeat begin answer : = trunc(Random * MAX); Test condition write('Enter your guess: '); readln(guess); if (guess = answer) then writeln('You guessed correctly!') else Execute body writeln('You guessed incorrectly'); writeln('Play again? '); writeln('Enter "Y" or "y" to play again'); writeln('Enter "N" or "n" otherwise'); write('Choice: '); readln(choice); end; until (choice = 'N') OR (choice = 'n'); Initialize control Update control James Tam
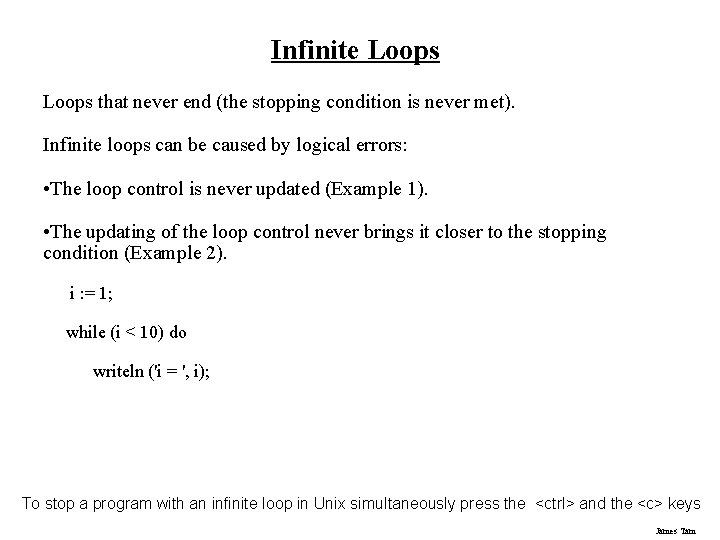
Infinite Loops that never end (the stopping condition is never met). Infinite loops can be caused by logical errors: • The loop control is never updated (Example 1). • The updating of the loop control never brings it closer to the stopping condition (Example 2). i : = 1; while (i < 10) do writeln ('i = ', i); To stop a program with an infinite loop in Unix simultaneously press the <ctrl> and the <c> keys James Tam
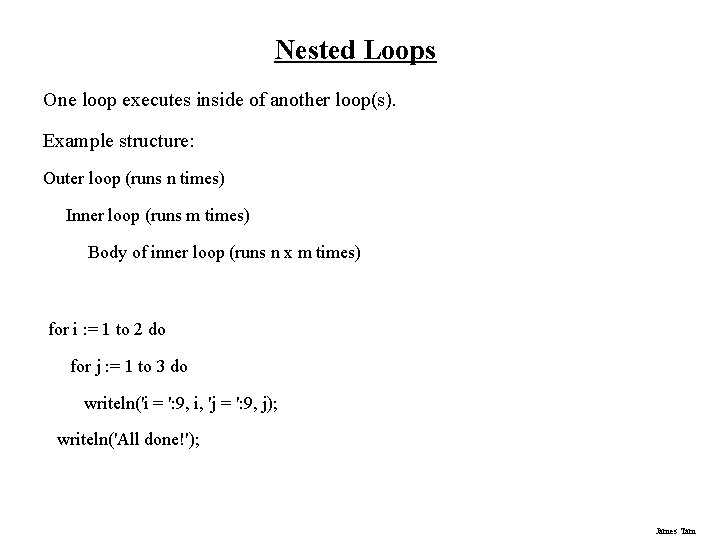
Nested Loops One loop executes inside of another loop(s). Example structure: Outer loop (runs n times) Inner loop (runs m times) Body of inner loop (runs n x m times) for i : = 1 to 2 do for j : = 1 to 3 do writeln('i = ': 9, i, 'j = ': 9, j); writeln('All done!'); James Tam
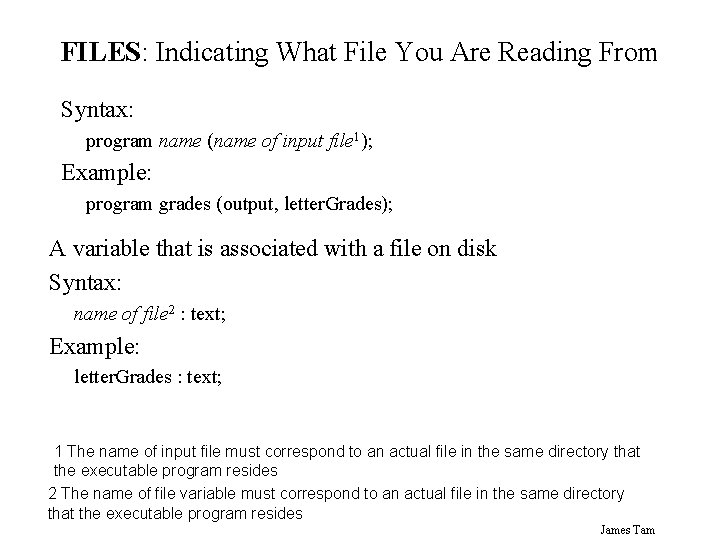
FILES: Indicating What File You Are Reading From Syntax: program name (name of input file 1); Example: program grades (output, letter. Grades); A variable that is associated with a file on disk Syntax: name of file 2 : text; Example: letter. Grades : text; 1 The name of input file must correspond to an actual file in the same directory that the executable program resides 2 The name of file variable must correspond to an actual file in the same directory that the executable program resides James Tam
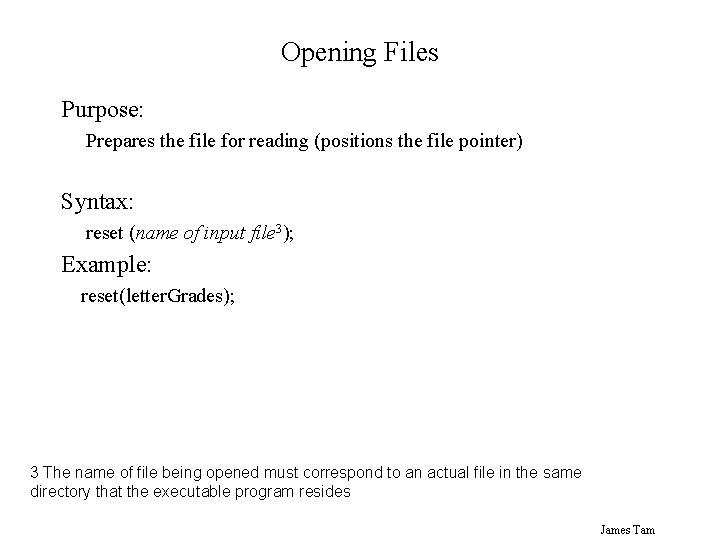
Opening Files Purpose: Prepares the file for reading (positions the file pointer) Syntax: reset (name of input file 3); Example: reset(letter. Grades); 3 The name of file being opened must correspond to an actual file in the same directory that the executable program resides James Tam
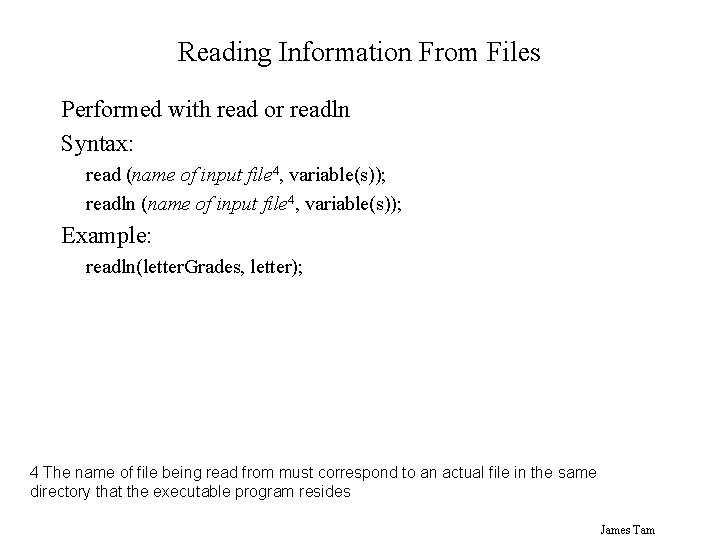
Reading Information From Files Performed with read or readln Syntax: read (name of input file 4, variable(s)); readln (name of input file 4, variable(s)); Example: readln(letter. Grades, letter); 4 The name of file being read from must correspond to an actual file in the same directory that the executable program resides James Tam
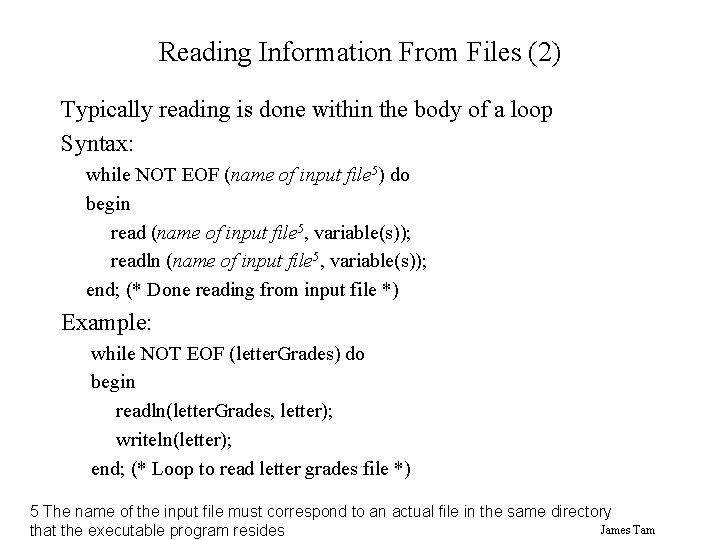
Reading Information From Files (2) Typically reading is done within the body of a loop Syntax: while NOT EOF (name of input file 5) do begin read (name of input file 5, variable(s)); readln (name of input file 5, variable(s)); end; (* Done reading from input file *) Example: while NOT EOF (letter. Grades) do begin readln(letter. Grades, letter); writeln(letter); end; (* Loop to read letter grades file *) 5 The name of the input file must correspond to an actual file in the same directory James Tam that the executable program resides
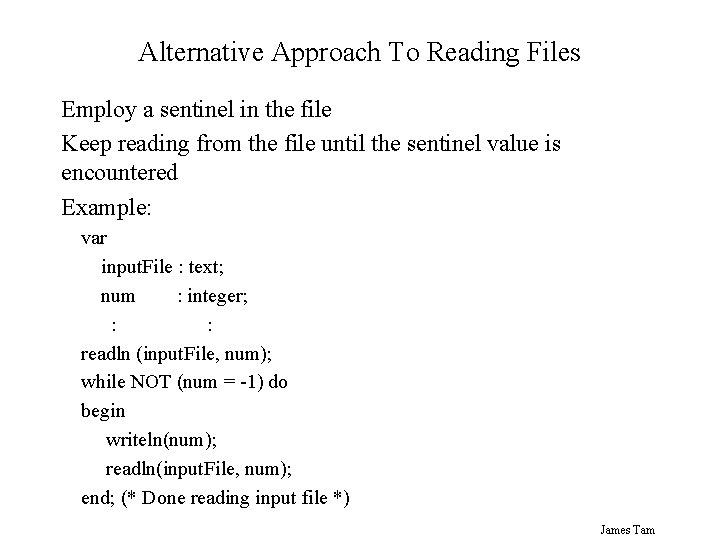
Alternative Approach To Reading Files Employ a sentinel in the file Keep reading from the file until the sentinel value is encountered Example: var input. File : text; num : integer; : : readln (input. File, num); while NOT (num = -1) do begin writeln(num); readln(input. File, num); end; (* Done reading input file *) James Tam
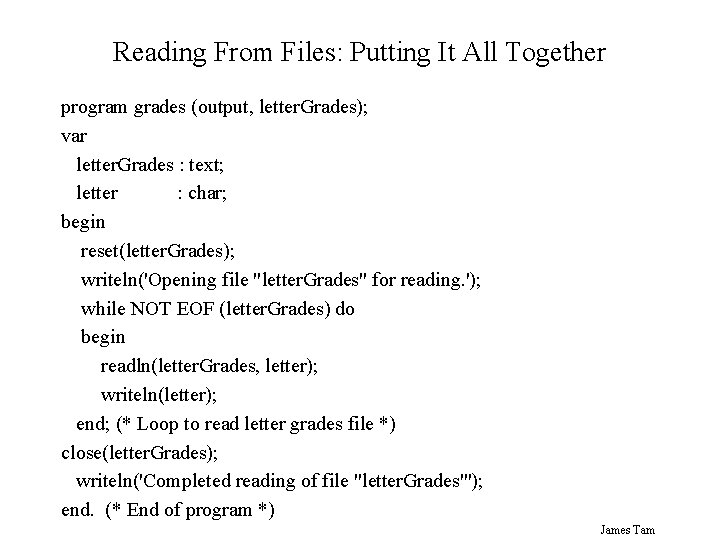
Reading From Files: Putting It All Together program grades (output, letter. Grades); var letter. Grades : text; letter : char; begin reset(letter. Grades); writeln('Opening file "letter. Grades" for reading. '); while NOT EOF (letter. Grades) do begin readln(letter. Grades, letter); writeln(letter); end; (* Loop to read letter grades file *) close(letter. Grades); writeln('Completed reading of file "letter. Grades"'); end. (* End of program *) James Tam
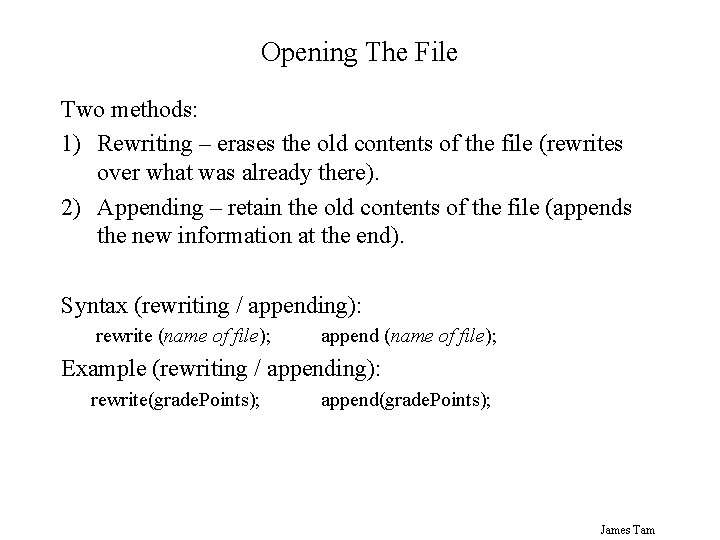
Opening The File Two methods: 1) Rewriting – erases the old contents of the file (rewrites over what was already there). 2) Appending – retain the old contents of the file (appends the new information at the end). Syntax (rewriting / appending): rewrite (name of file); append (name of file); Example (rewriting / appending): rewrite(grade. Points); append(grade. Points); James Tam
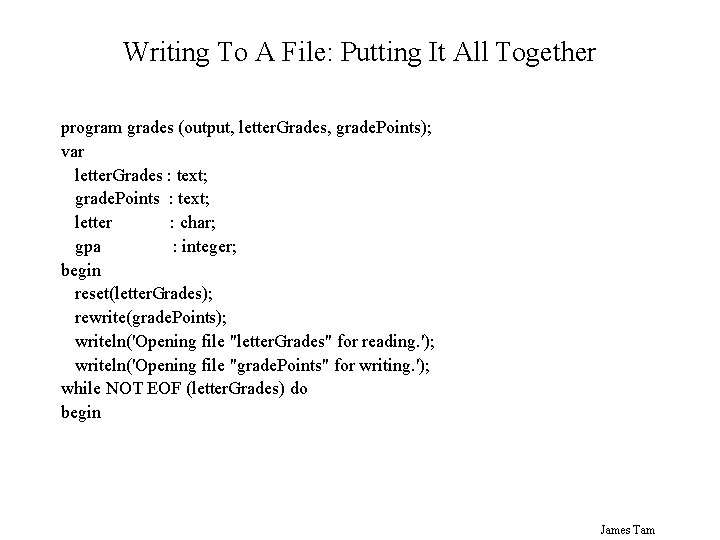
Writing To A File: Putting It All Together program grades (output, letter. Grades, grade. Points); var letter. Grades : text; grade. Points : text; letter : char; gpa : integer; begin reset(letter. Grades); rewrite(grade. Points); writeln('Opening file "letter. Grades" for reading. '); writeln('Opening file "grade. Points" for writing. '); while NOT EOF (letter. Grades) do begin James Tam
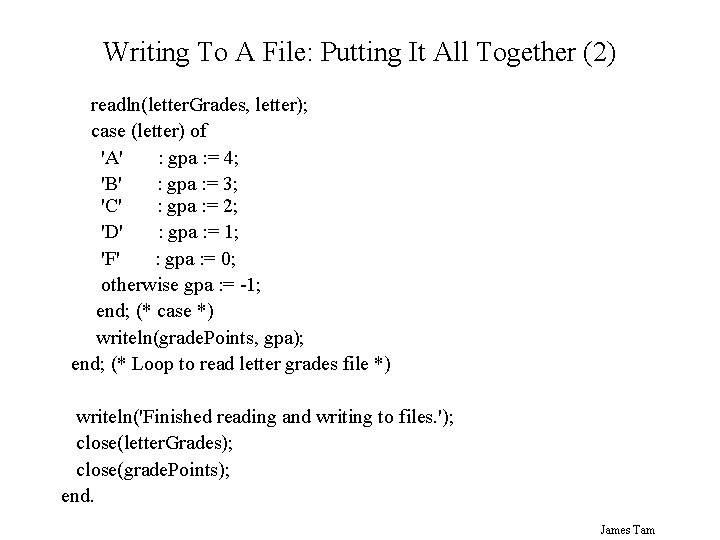
Writing To A File: Putting It All Together (2) readln(letter. Grades, letter); case (letter) of 'A' : gpa : = 4; 'B' : gpa : = 3; 'C' : gpa : = 2; 'D' : gpa : = 1; 'F' : gpa : = 0; otherwise gpa : = -1; end; (* case *) writeln(grade. Points, gpa); end; (* Loop to read letter grades file *) writeln('Finished reading and writing to files. '); close(letter. Grades); close(grade. Points); end. James Tam
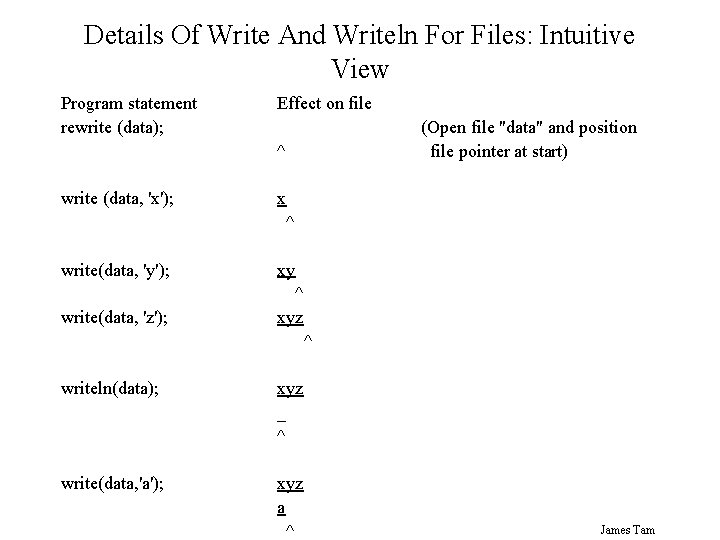
Details Of Write And Writeln For Files: Intuitive View Program statement rewrite (data); Effect on file (Open file "data" and position file pointer at start) ^ write (data, 'x'); x ^ write(data, 'y'); xy write(data, 'z'); ^ xyz ^ writeln(data); xyz _ ^ write(data, 'a'); xyz a ^ James Tam
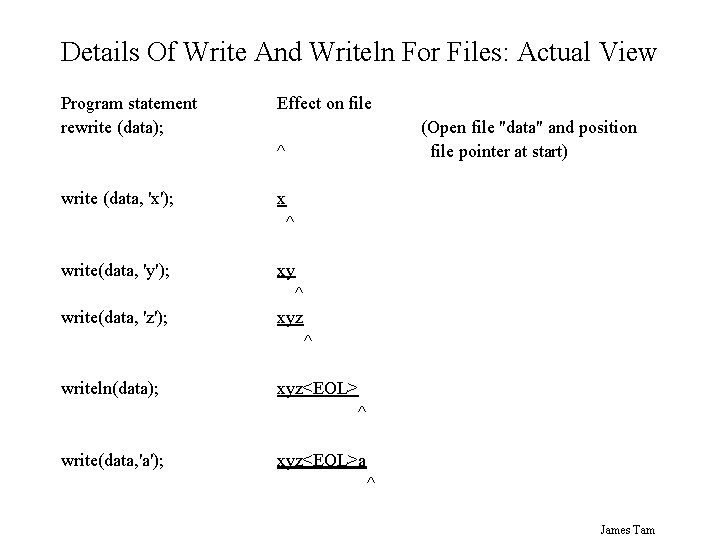
Details Of Write And Writeln For Files: Actual View Program statement rewrite (data); Effect on file (Open file "data" and position file pointer at start) ^ write (data, 'x'); x ^ write(data, 'y'); xy write(data, 'z'); ^ xyz ^ writeln(data); xyz<EOL> ^ write(data, 'a'); xyz<EOL>a ^ James Tam
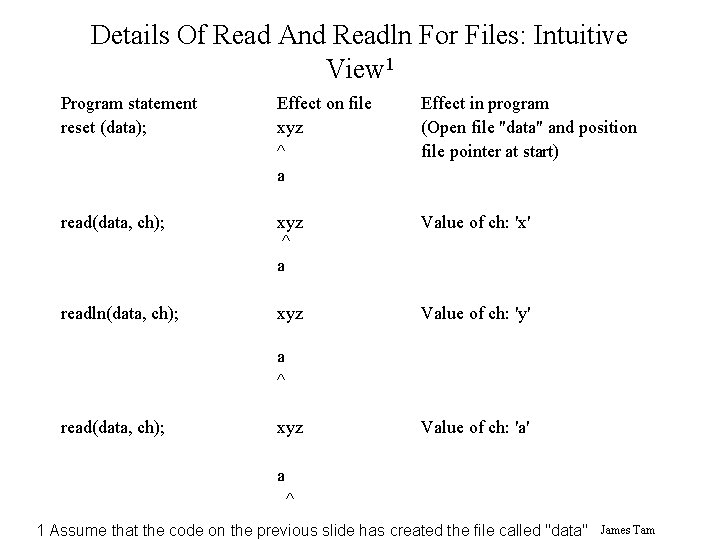
Details Of Read And Readln For Files: Intuitive View 1 Program statement reset (data); Effect on file xyz ^ a Effect in program (Open file "data" and position file pointer at start) read(data, ch); xyz ^ a Value of ch: 'x' readln(data, ch); xyz Value of ch: 'y' a ^ read(data, ch); xyz Value of ch: 'a' a ^ 1 Assume that the code on the previous slide has created the file called "data" James Tam
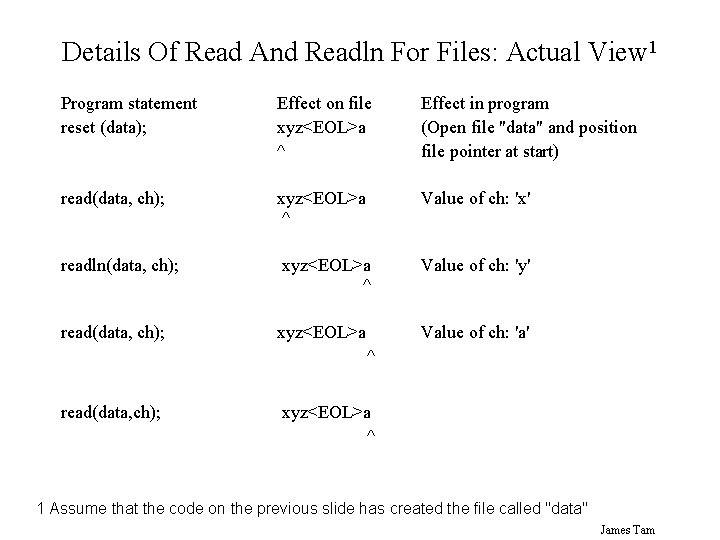
Details Of Read And Readln For Files: Actual View 1 Program statement reset (data); Effect on file xyz<EOL>a ^ Effect in program (Open file "data" and position file pointer at start) read(data, ch); xyz<EOL>a ^ Value of ch: 'x' readln(data, ch); xyz<EOL>a ^ Value of ch: 'y' read(data, ch); xyz<EOL>a Value of ch: 'a' ^ read(data, ch); xyz<EOL>a ^ 1 Assume that the code on the previous slide has created the file called "data" James Tam
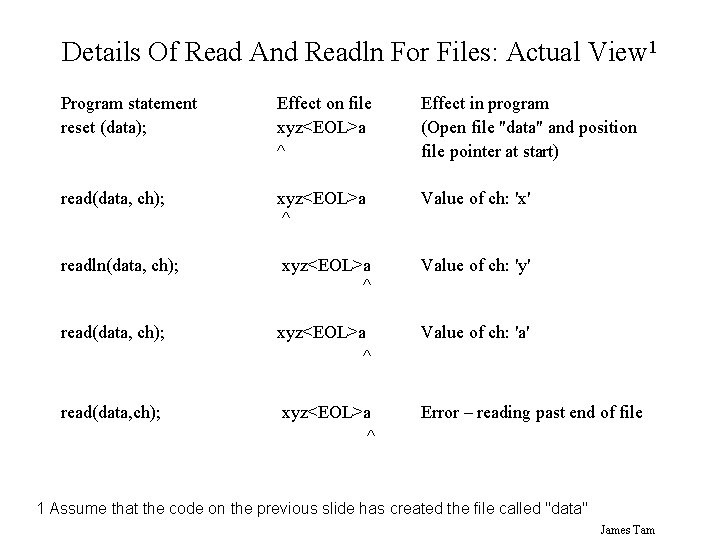
Details Of Read And Readln For Files: Actual View 1 Program statement reset (data); Effect on file xyz<EOL>a ^ Effect in program (Open file "data" and position file pointer at start) read(data, ch); xyz<EOL>a ^ Value of ch: 'x' readln(data, ch); xyz<EOL>a ^ Value of ch: 'y' read(data, ch); xyz<EOL>a ^ Value of ch: 'a' read(data, ch); xyz<EOL>a ^ Error – reading past end of file 1 Assume that the code on the previous slide has created the file called "data" James Tam
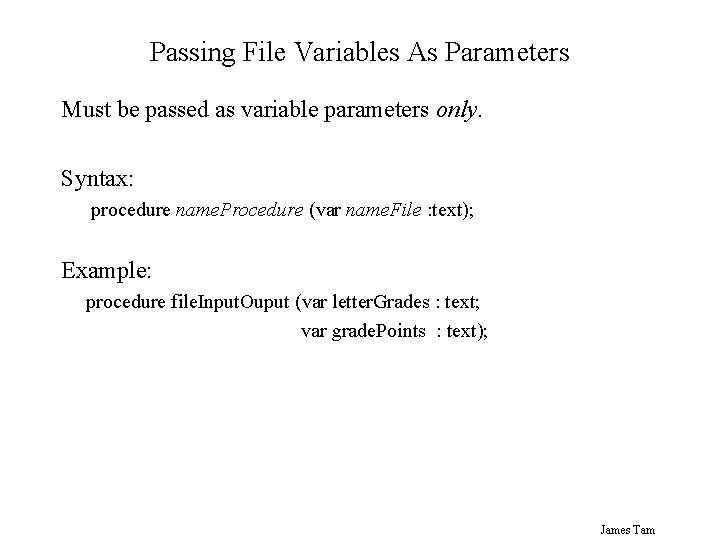
Passing File Variables As Parameters Must be passed as variable parameters only. Syntax: procedure name. Procedure (var name. File : text); Example: procedure file. Input. Ouput (var letter. Grades : text; var grade. Points : text); James Tam
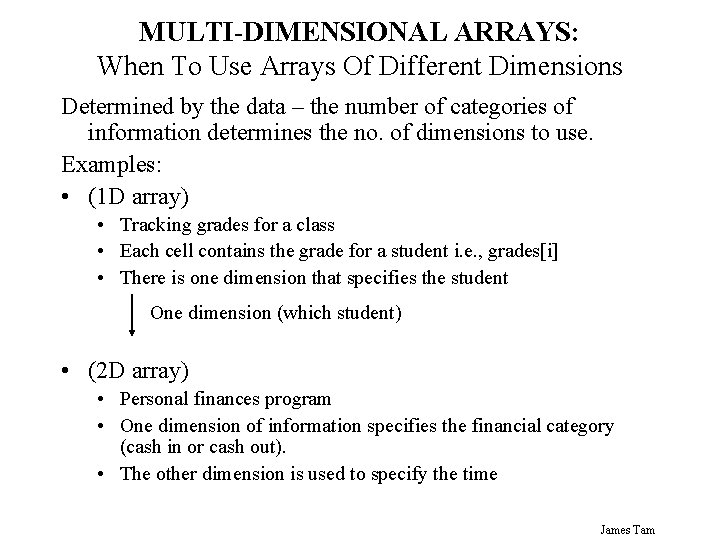
MULTI-DIMENSIONAL ARRAYS: When To Use Arrays Of Different Dimensions Determined by the data – the number of categories of information determines the no. of dimensions to use. Examples: • (1 D array) • Tracking grades for a class • Each cell contains the grade for a student i. e. , grades[i] • There is one dimension that specifies the student One dimension (which student) • (2 D array) • Personal finances program • One dimension of information specifies the financial category (cash in or cash out). • The other dimension is used to specify the time James Tam
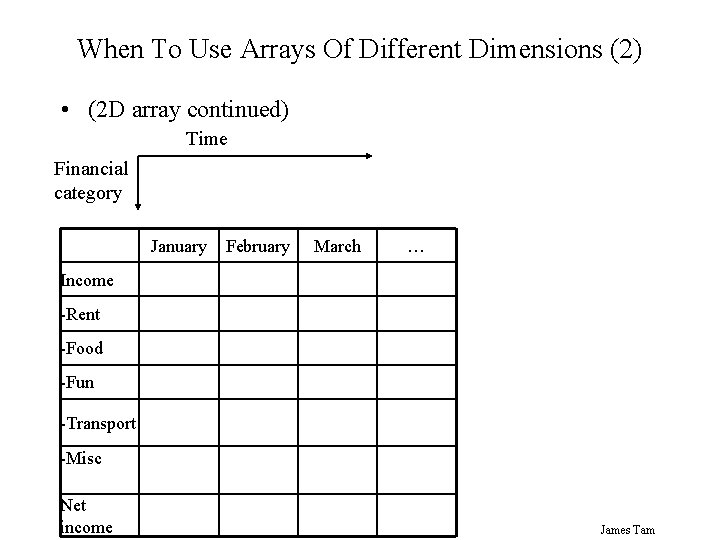
When To Use Arrays Of Different Dimensions (2) • (2 D array continued) Time Financial category January February March … Income -Rent -Food -Fun -Transport -Misc Net income James Tam
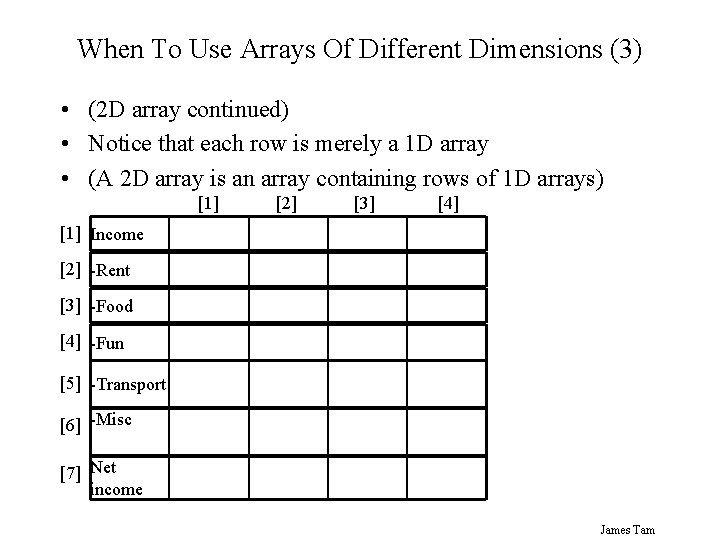
When To Use Arrays Of Different Dimensions (3) • (2 D array continued) • Notice that each row is merely a 1 D array • (A 2 D array is an array containing rows of 1 D arrays) [1] [2] [3] [4] [1] Income [2] -Rent [3] -Food [4] -Fun [5] -Transport [6] -Misc [7] Net income James Tam
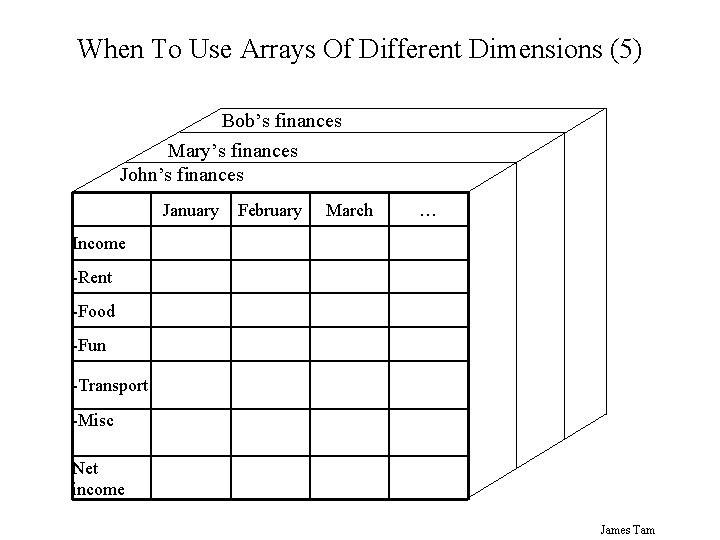
When To Use Arrays Of Different Dimensions (5) Bob’s finances Mary’s finances John’s finances January February March … Income -Rent -Food -Fun -Transport -Misc Net income James Tam
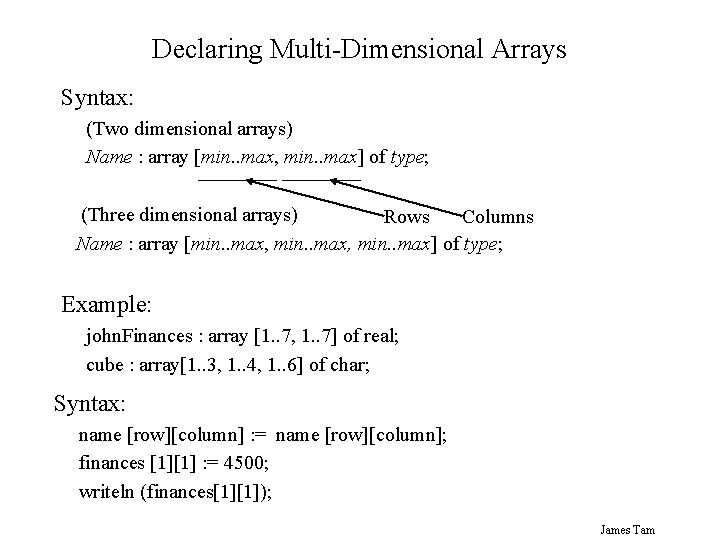
Declaring Multi-Dimensional Arrays Syntax: (Two dimensional arrays) Name : array [min. . max, min. . max] of type; (Three dimensional arrays) Rows Columns Name : array [min. . max, min. . max] of type; Example: john. Finances : array [1. . 7, 1. . 7] of real; cube : array[1. . 3, 1. . 4, 1. . 6] of char; Syntax: name [row][column] : = name [row][column]; finances [1][1] : = 4500; writeln (finances[1][1]); James Tam
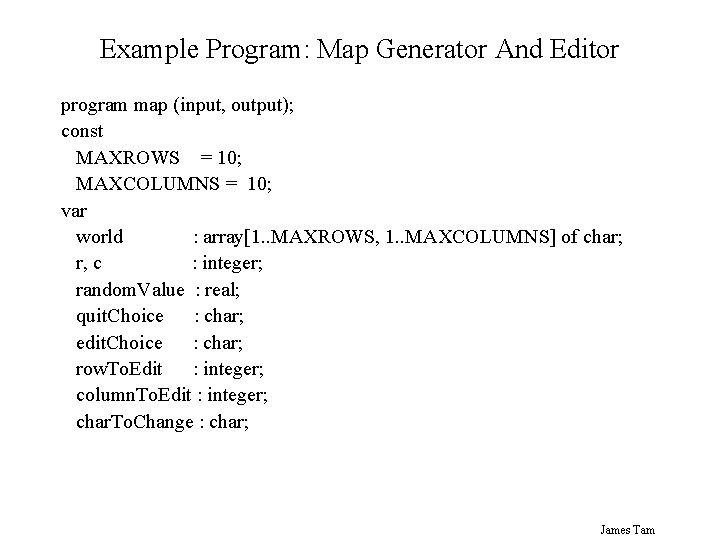
Example Program: Map Generator And Editor program map (input, output); const MAXROWS = 10; MAXCOLUMNS = 10; var world : array[1. . MAXROWS, 1. . MAXCOLUMNS] of char; r, c : integer; random. Value : real; quit. Choice : char; edit. Choice : char; row. To. Edit : integer; column. To. Edit : integer; char. To. Change : char; James Tam
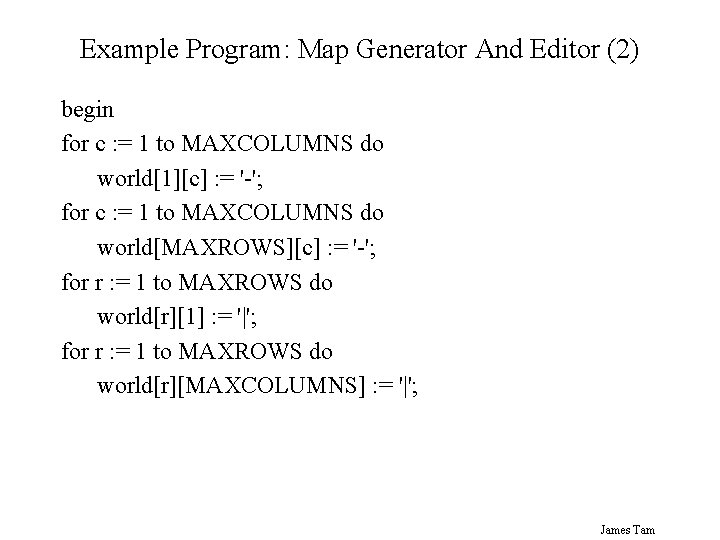
Example Program: Map Generator And Editor (2) begin for c : = 1 to MAXCOLUMNS do world[1][c] : = '-'; for c : = 1 to MAXCOLUMNS do world[MAXROWS][c] : = '-'; for r : = 1 to MAXROWS do world[r][1] : = '|'; for r : = 1 to MAXROWS do world[r][MAXCOLUMNS] : = '|'; James Tam
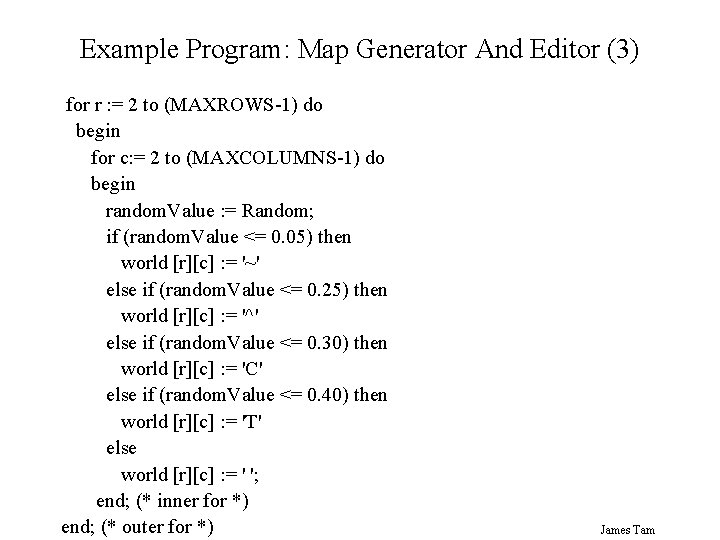
Example Program: Map Generator And Editor (3) for r : = 2 to (MAXROWS-1) do begin for c: = 2 to (MAXCOLUMNS-1) do begin random. Value : = Random; if (random. Value <= 0. 05) then world [r][c] : = '~' else if (random. Value <= 0. 25) then world [r][c] : = '^' else if (random. Value <= 0. 30) then world [r][c] : = 'C' else if (random. Value <= 0. 40) then world [r][c] : = 'T' else world [r][c] : = ' '; end; (* inner for *) end; (* outer for *) James Tam
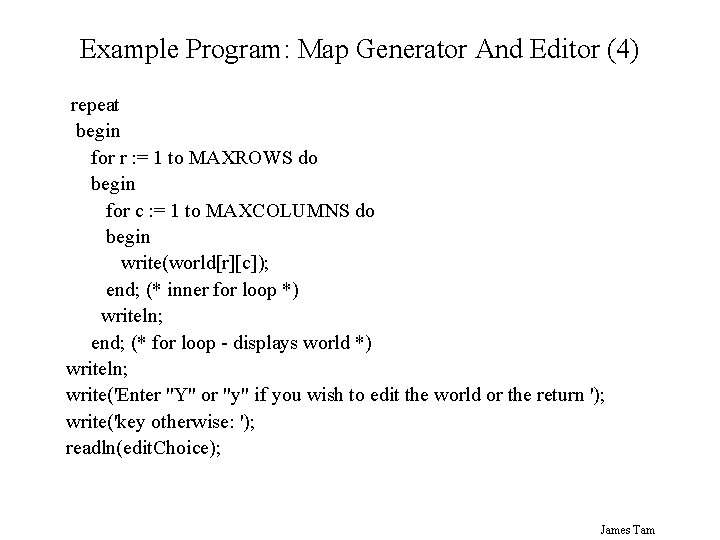
Example Program: Map Generator And Editor (4) repeat begin for r : = 1 to MAXROWS do begin for c : = 1 to MAXCOLUMNS do begin write(world[r][c]); end; (* inner for loop *) writeln; end; (* for loop - displays world *) writeln; write('Enter "Y" or "y" if you wish to edit the world or the return '); write('key otherwise: '); readln(edit. Choice); James Tam
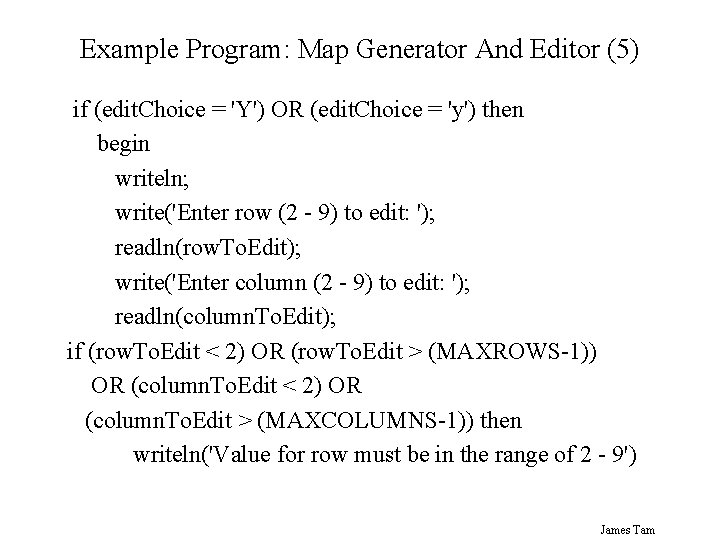
Example Program: Map Generator And Editor (5) if (edit. Choice = 'Y') OR (edit. Choice = 'y') then begin writeln; write('Enter row (2 - 9) to edit: '); readln(row. To. Edit); write('Enter column (2 - 9) to edit: '); readln(column. To. Edit); if (row. To. Edit < 2) OR (row. To. Edit > (MAXROWS-1)) OR (column. To. Edit < 2) OR (column. To. Edit > (MAXCOLUMNS-1)) then writeln('Value for row must be in the range of 2 - 9') James Tam
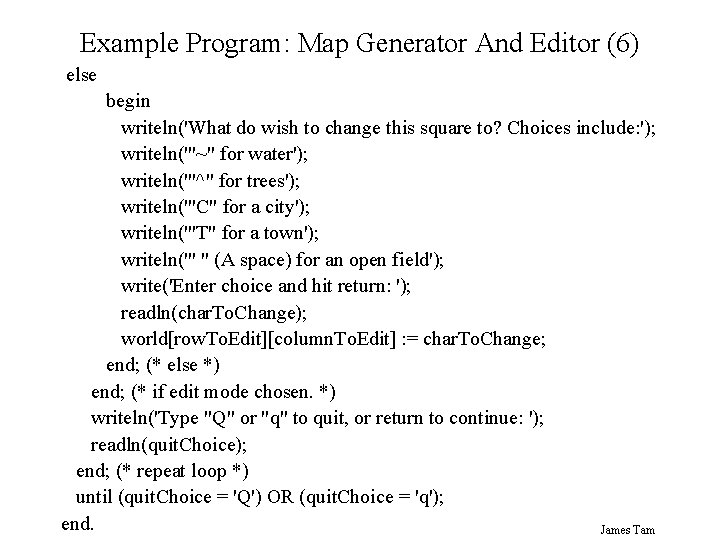
Example Program: Map Generator And Editor (6) else begin writeln('What do wish to change this square to? Choices include: '); writeln('"~" for water'); writeln('"^" for trees'); writeln('"C" for a city'); writeln('"T" for a town'); writeln('" " (A space) for an open field'); write('Enter choice and hit return: '); readln(char. To. Change); world[row. To. Edit][column. To. Edit] : = char. To. Change; end; (* else *) end; (* if edit mode chosen. *) writeln('Type "Q" or "q" to quit, or return to continue: '); readln(quit. Choice); end; (* repeat loop *) until (quit. Choice = 'Q') OR (quit. Choice = 'q'); end. James Tam
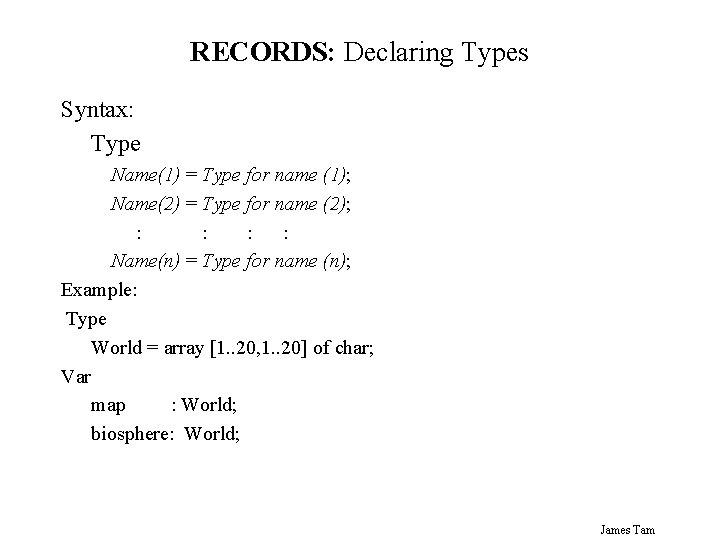
RECORDS: Declaring Types Syntax: Type Name(1) = Type for name (1); Name(2) = Type for name (2); : : Name(n) = Type for name (n); Example: Type World = array [1. . 20, 1. . 20] of char; Var map : World; biosphere: World; James Tam
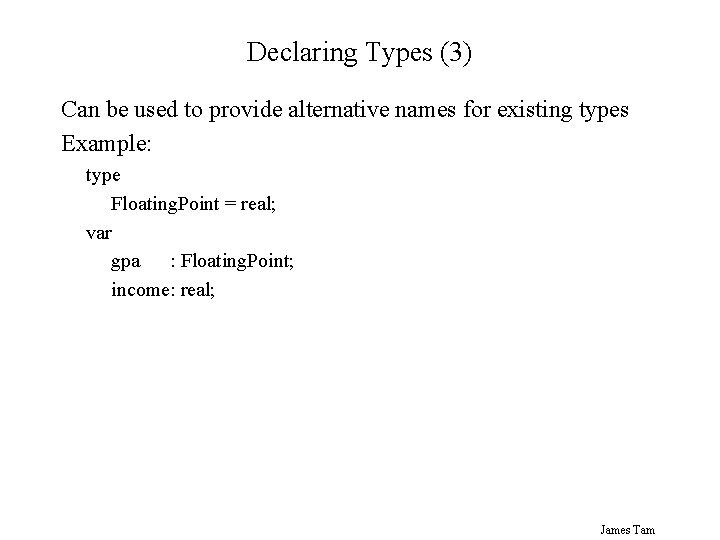
Declaring Types (3) Can be used to provide alternative names for existing types Example: type Floating. Point = real; var gpa : Floating. Point; income: real; James Tam
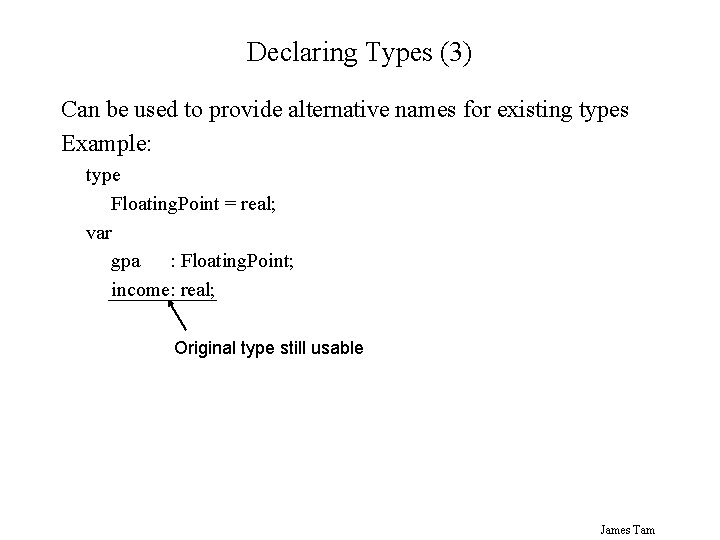
Declaring Types (3) Can be used to provide alternative names for existing types Example: type Floating. Point = real; var gpa : Floating. Point; income: real; Original type still usable James Tam
![Declaring Types 4 Example Type world array 1 20 1 20 Declaring Types (4) Example: Type world = array [1. . 20, 1. . 20]](https://slidetodoc.com/presentation_image_h2/ed92b301b7f9d83e665b7d2596230111/image-70.jpg)
Declaring Types (4) Example: Type world = array [1. . 20, 1. . 20] of char; Var Declaring the map : world; type - defining what the type biosphere: world; consists of Declaring variables of the new type James Tam
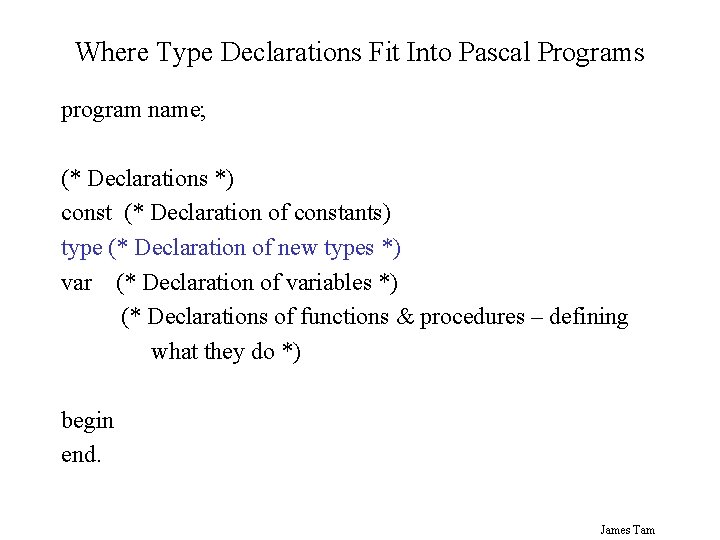
Where Type Declarations Fit Into Pascal Programs program name; (* Declarations *) const (* Declaration of constants) type (* Declaration of new types *) var (* Declaration of variables *) (* Declarations of functions & procedures – defining what they do *) begin end. James Tam
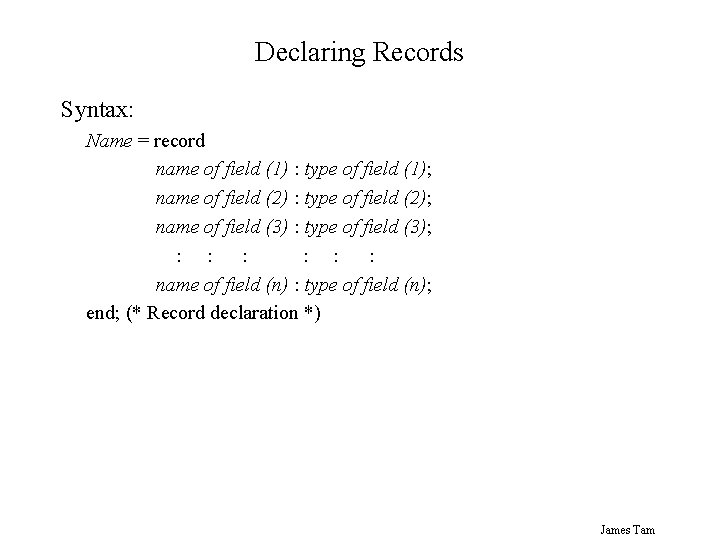
Declaring Records Syntax: Name = record name of field (1) : type of field (1); name of field (2) : type of field (2); name of field (3) : type of field (3); : : : name of field (n) : type of field (n); end; (* Record declaration *) James Tam
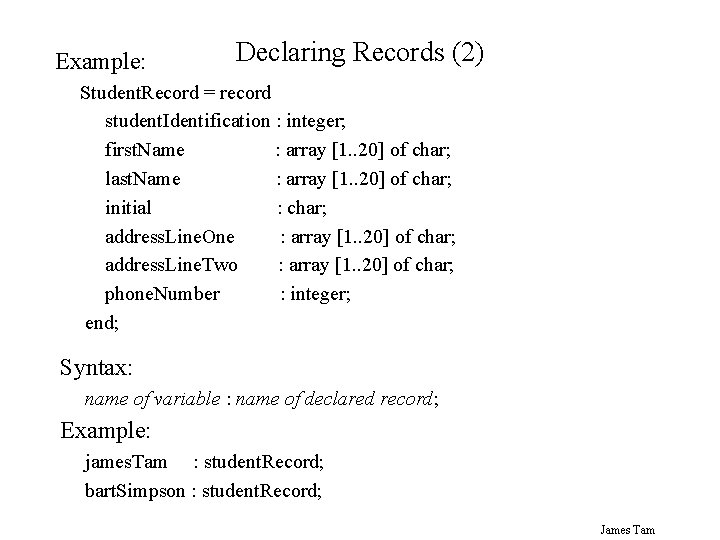
Example: Declaring Records (2) Student. Record = record student. Identification : integer; first. Name : array [1. . 20] of char; last. Name : array [1. . 20] of char; initial : char; address. Line. One : array [1. . 20] of char; address. Line. Two : array [1. . 20] of char; phone. Number : integer; end; Syntax: name of variable : name of declared record; Example: james. Tam : student. Record; bart. Simpson : student. Record; James Tam
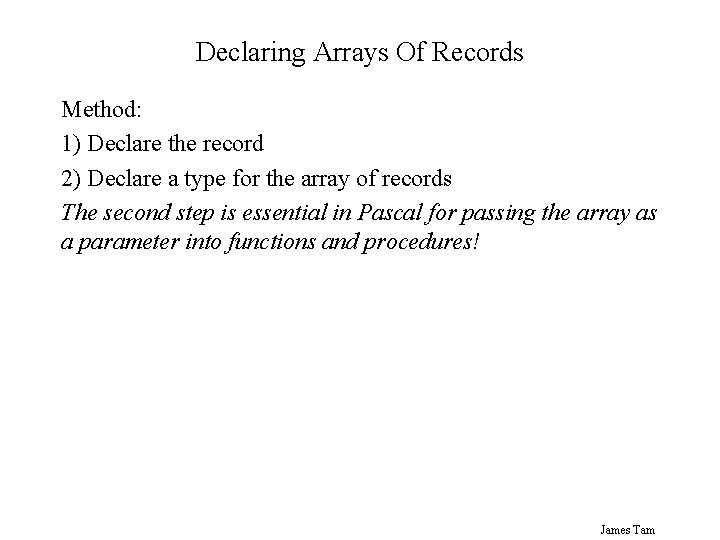
Declaring Arrays Of Records Method: 1) Declare the record 2) Declare a type for the array of records The second step is essential in Pascal for passing the array as a parameter into functions and procedures! James Tam
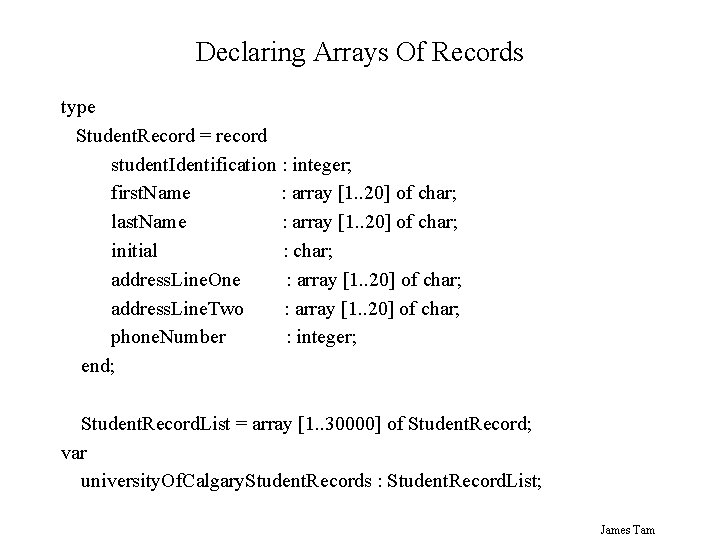
Declaring Arrays Of Records type Student. Record = record student. Identification : integer; first. Name : array [1. . 20] of char; last. Name : array [1. . 20] of char; initial : char; address. Line. One : array [1. . 20] of char; address. Line. Two : array [1. . 20] of char; phone. Number : integer; end; Student. Record. List = array [1. . 30000] of Student. Record; var university. Of. Calgary. Student. Records : Student. Record. List; James Tam
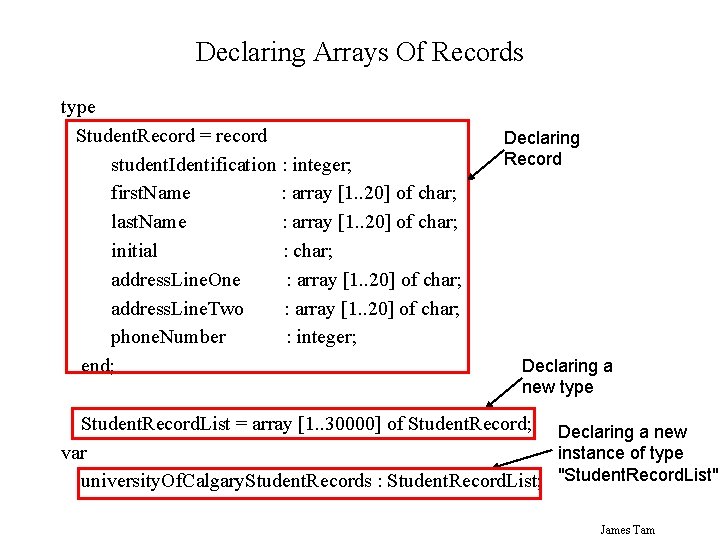
Declaring Arrays Of Records type Student. Record = record student. Identification : integer; first. Name : array [1. . 20] of char; last. Name : array [1. . 20] of char; initial : char; address. Line. One : array [1. . 20] of char; address. Line. Two : array [1. . 20] of char; phone. Number : integer; end; Declaring Record Declaring a new type Student. Record. List = array [1. . 30000] of Student. Record; Declaring a new var instance of type university. Of. Calgary. Student. Records : Student. Record. List; "Student. Record. List" James Tam
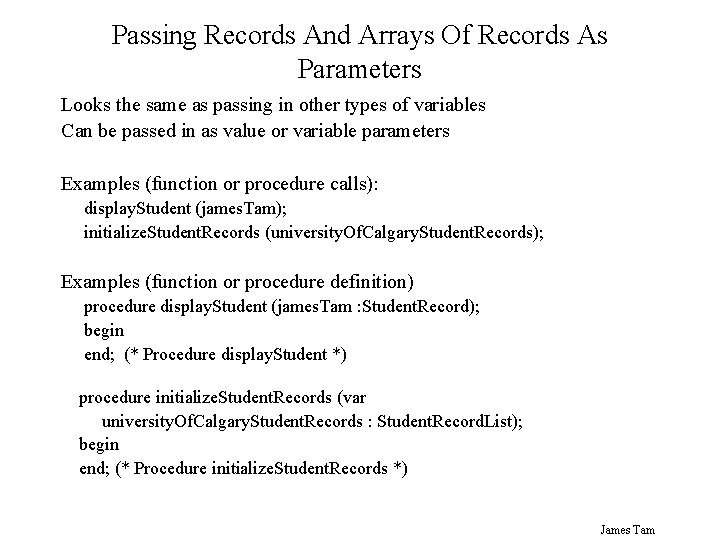
Passing Records And Arrays Of Records As Parameters Looks the same as passing in other types of variables Can be passed in as value or variable parameters Examples (function or procedure calls): display. Student (james. Tam); initialize. Student. Records (university. Of. Calgary. Student. Records); Examples (function or procedure definition) procedure display. Student (james. Tam : Student. Record); begin end; (* Procedure display. Student *) procedure initialize. Student. Records (var university. Of. Calgary. Student. Records : Student. Record. List); begin end; (* Procedure initialize. Student. Records *) James Tam
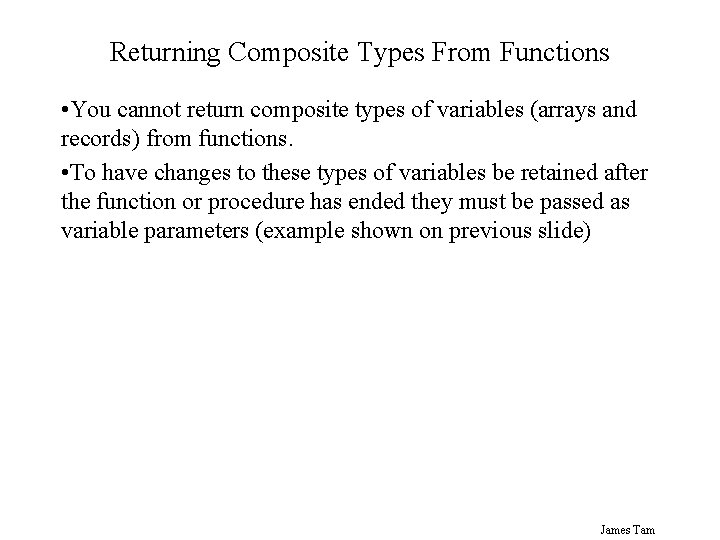
Returning Composite Types From Functions • You cannot return composite types of variables (arrays and records) from functions. • To have changes to these types of variables be retained after the function or procedure has ended they must be passed as variable parameters (example shown on previous slide) James Tam
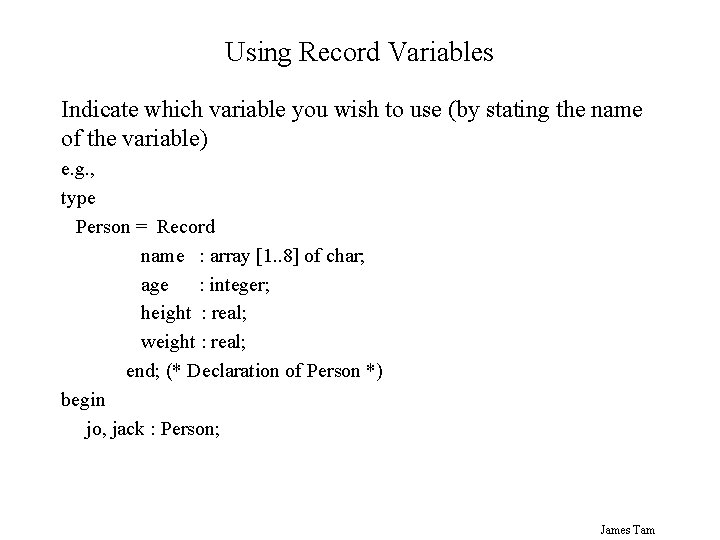
Using Record Variables Indicate which variable you wish to use (by stating the name of the variable) e. g. , type Person = Record name : array [1. . 8] of char; age : integer; height : real; weight : real; end; (* Declaration of Person *) begin jo, jack : Person; James Tam
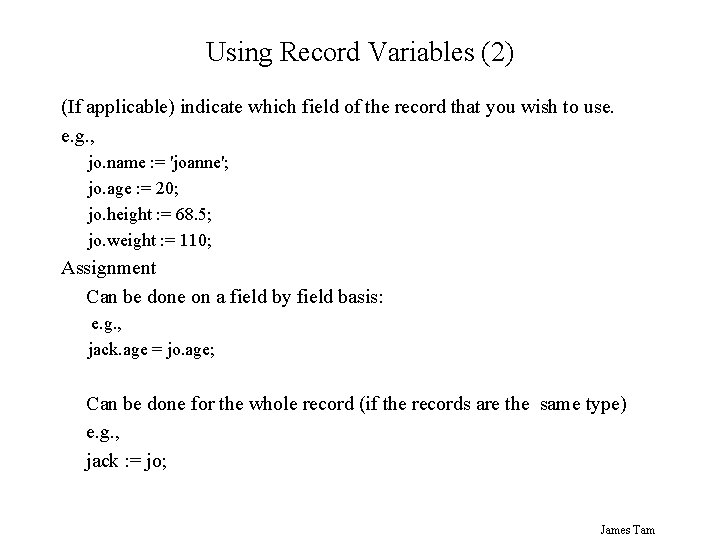
Using Record Variables (2) (If applicable) indicate which field of the record that you wish to use. e. g. , jo. name : = 'joanne'; jo. age : = 20; jo. height : = 68. 5; jo. weight : = 110; Assignment Can be done on a field by field basis: e. g. , jack. age = jo. age; Can be done for the whole record (if the records are the same type) e. g. , jack : = jo; James Tam
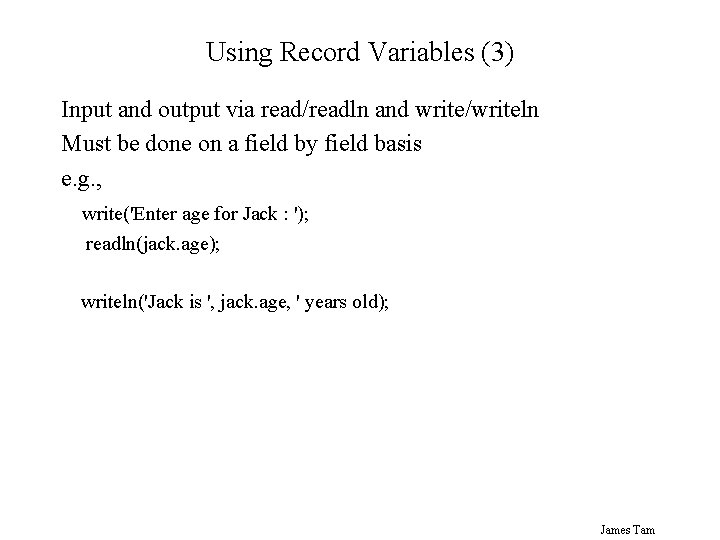
Using Record Variables (3) Input and output via read/readln and write/writeln Must be done on a field by field basis e. g. , write('Enter age for Jack : '); readln(jack. age); writeln('Jack is ', jack. age, ' years old); James Tam
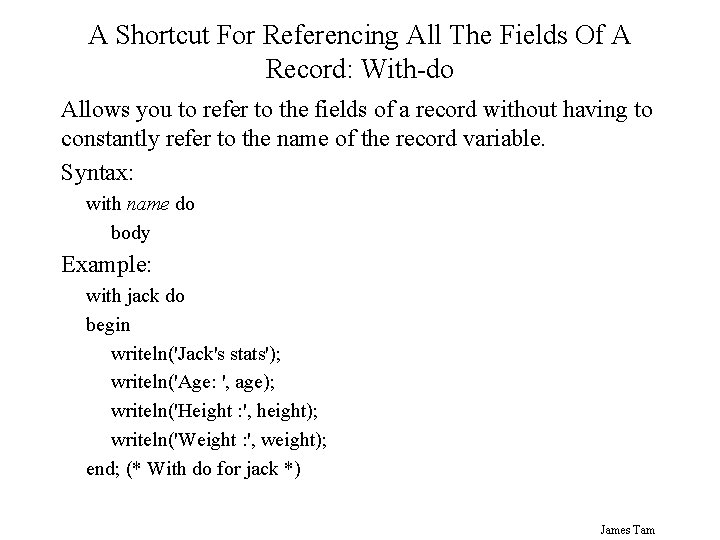
A Shortcut For Referencing All The Fields Of A Record: With-do Allows you to refer to the fields of a record without having to constantly refer to the name of the record variable. Syntax: with name do body Example: with jack do begin writeln('Jack's stats'); writeln('Age: ', age); writeln('Height : ', height); writeln('Weight : ', weight); end; (* With do for jack *) James Tam
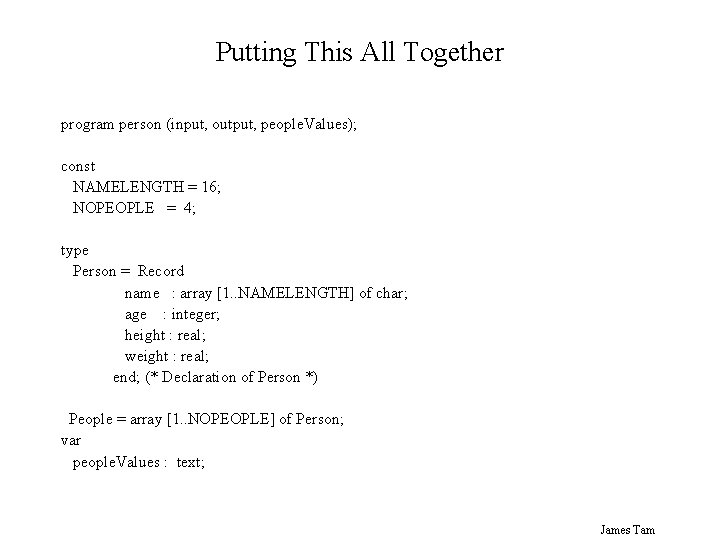
Putting This All Together program person (input, output, people. Values); const NAMELENGTH = 16; NOPEOPLE = 4; type Person = Record name : array [1. . NAMELENGTH] of char; age : integer; height : real; weight : real; end; (* Declaration of Person *) People = array [1. . NOPEOPLE] of Person; var people. Values : text; James Tam
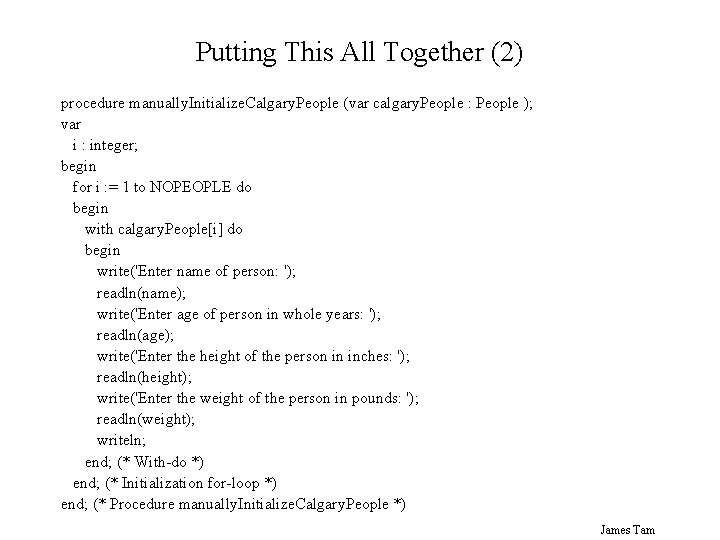
Putting This All Together (2) procedure manually. Initialize. Calgary. People (var calgary. People : People ); var i : integer; begin for i : = 1 to NOPEOPLE do begin with calgary. People[i] do begin write('Enter name of person: '); readln(name); write('Enter age of person in whole years: '); readln(age); write('Enter the height of the person in inches: '); readln(height); write('Enter the weight of the person in pounds: '); readln(weight); writeln; end; (* With-do *) end; (* Initialization for-loop *) end; (* Procedure manually. Initialize. Calgary. People *) James Tam
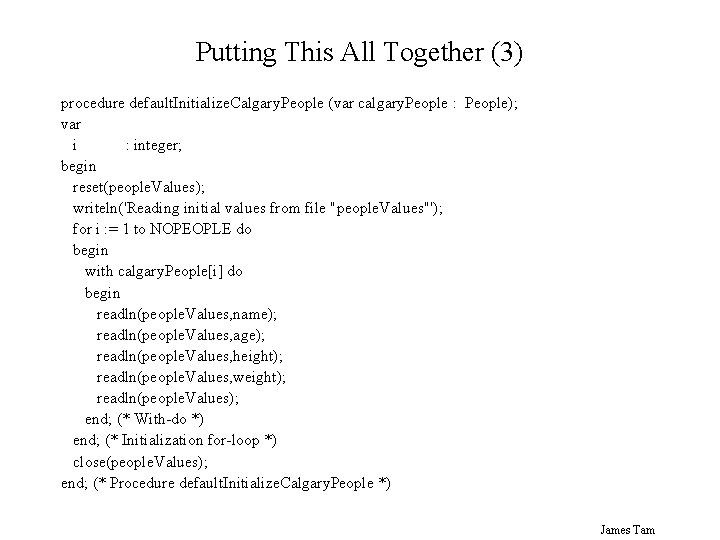
Putting This All Together (3) procedure default. Initialize. Calgary. People (var calgary. People : People); var i : integer; begin reset(people. Values); writeln('Reading initial values from file "people. Values"'); for i : = 1 to NOPEOPLE do begin with calgary. People[i] do begin readln(people. Values, name); readln(people. Values, age); readln(people. Values, height); readln(people. Values, weight); readln(people. Values); end; (* With-do *) end; (* Initialization for-loop *) close(people. Values); end; (* Procedure default. Initialize. Calgary. People *) James Tam
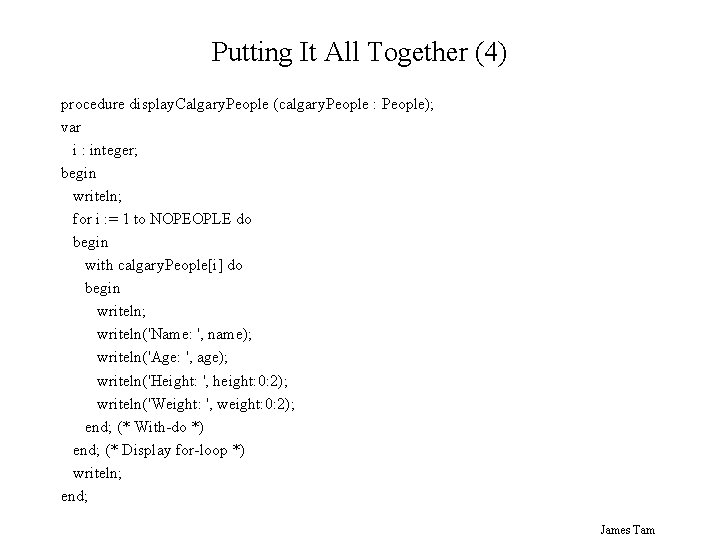
Putting It All Together (4) procedure display. Calgary. People (calgary. People : People); var i : integer; begin writeln; for i : = 1 to NOPEOPLE do begin with calgary. People[i] do begin writeln; writeln('Name: ', name); writeln('Age: ', age); writeln('Height: ', height: 0: 2); writeln('Weight: ', weight: 0: 2); end; (* With-do *) end; (* Display for-loop *) writeln; end; James Tam
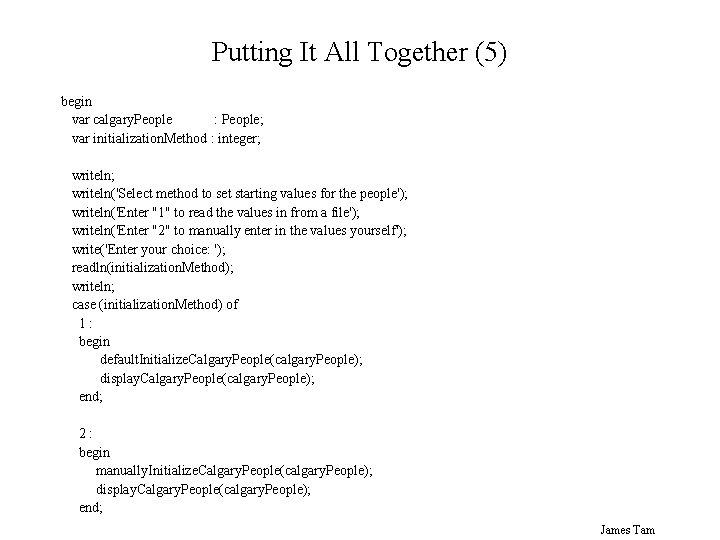
Putting It All Together (5) begin var calgary. People : People; var initialization. Method : integer; writeln('Select method to set starting values for the people'); writeln('Enter "1" to read the values in from a file'); writeln('Enter "2" to manually enter in the values yourself'); write('Enter your choice: '); readln(initialization. Method); writeln; case (initialization. Method) of 1: begin default. Initialize. Calgary. People(calgary. People); display. Calgary. People(calgary. People); end; 2: begin manually. Initialize. Calgary. People(calgary. People); display. Calgary. People(calgary. People); end; James Tam
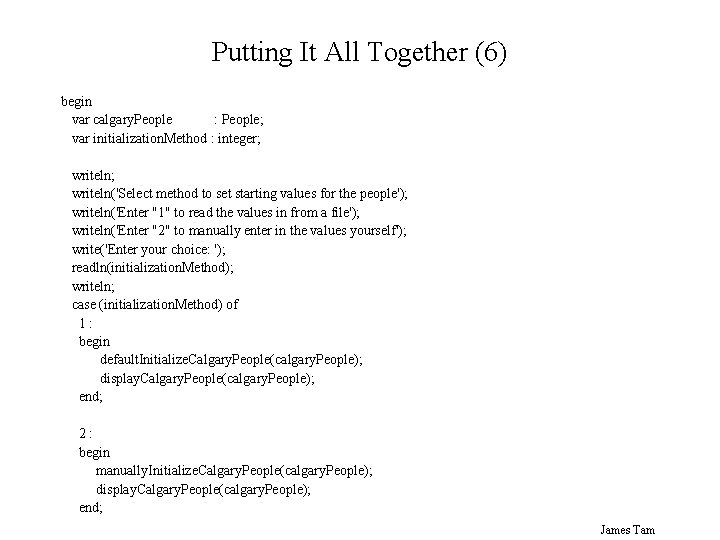
Putting It All Together (6) begin var calgary. People : People; var initialization. Method : integer; writeln('Select method to set starting values for the people'); writeln('Enter "1" to read the values in from a file'); writeln('Enter "2" to manually enter in the values yourself'); write('Enter your choice: '); readln(initialization. Method); writeln; case (initialization. Method) of 1: begin default. Initialize. Calgary. People(calgary. People); display. Calgary. People(calgary. People); end; 2: begin manually. Initialize. Calgary. People(calgary. People); display. Calgary. People(calgary. People); end; James Tam
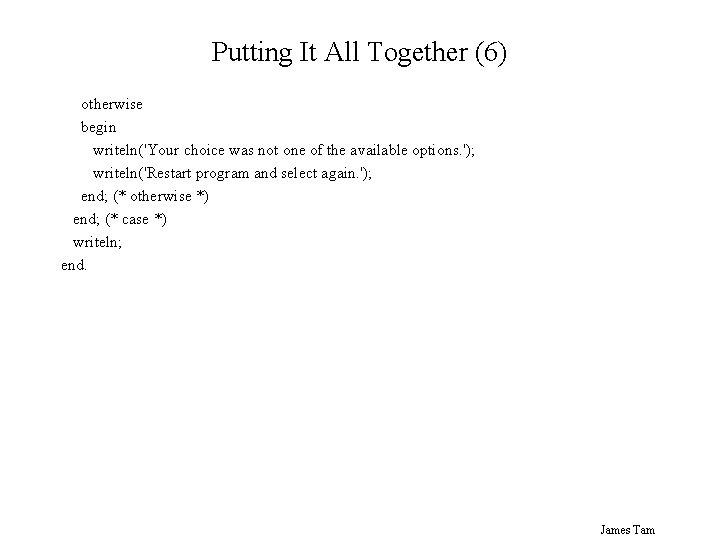
Putting It All Together (6) otherwise begin writeln('Your choice was not one of the available options. '); writeln('Restart program and select again. '); end; (* otherwise *) end; (* case *) writeln; end. James Tam
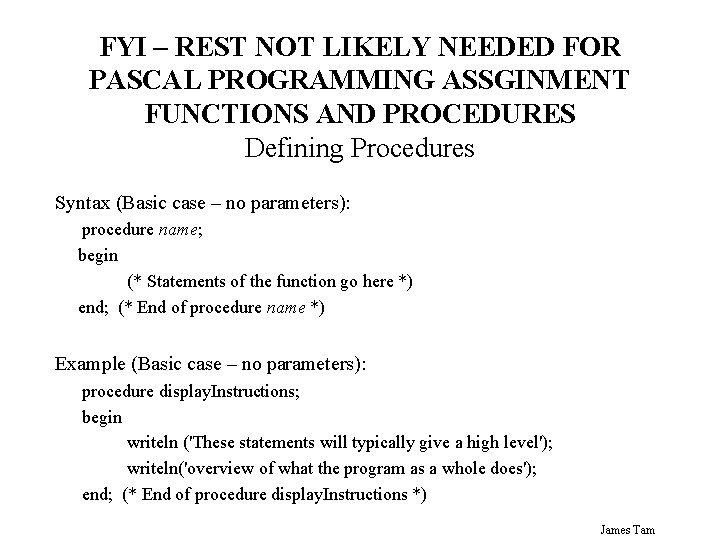
FYI – REST NOT LIKELY NEEDED FOR PASCAL PROGRAMMING ASSGINMENT FUNCTIONS AND PROCEDURES Defining Procedures Syntax (Basic case – no parameters): procedure name; begin (* Statements of the function go here *) end; (* End of procedure name *) Example (Basic case – no parameters): procedure display. Instructions; begin writeln ('These statements will typically give a high level'); writeln('overview of what the program as a whole does'); end; (* End of procedure display. Instructions *) James Tam
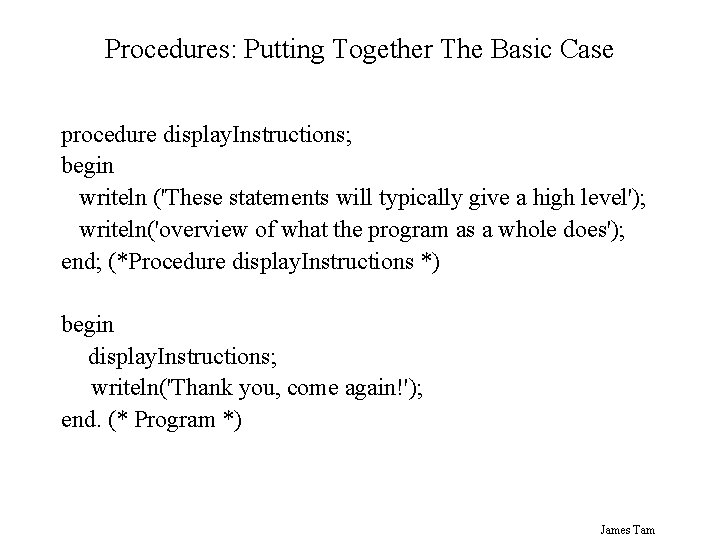
Procedures: Putting Together The Basic Case procedure display. Instructions; begin writeln ('These statements will typically give a high level'); writeln('overview of what the program as a whole does'); end; (*Procedure display. Instructions *) begin display. Instructions; writeln('Thank you, come again!'); end. (* Program *) James Tam
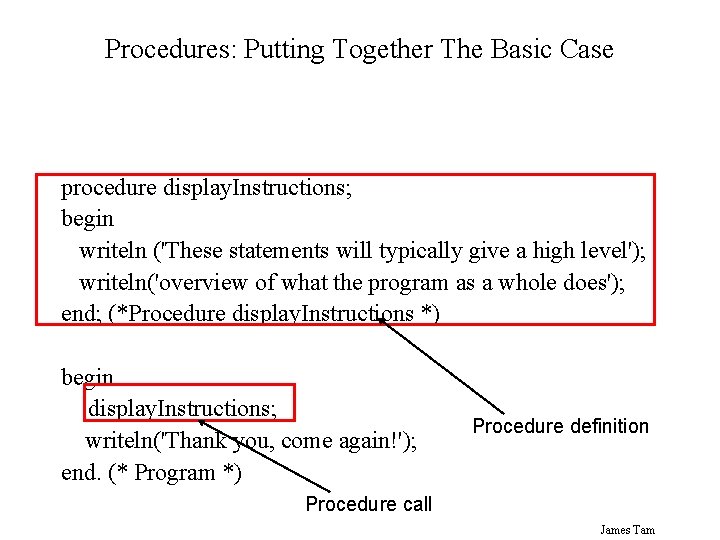
Procedures: Putting Together The Basic Case procedure display. Instructions; begin writeln ('These statements will typically give a high level'); writeln('overview of what the program as a whole does'); end; (*Procedure display. Instructions *) begin display. Instructions; writeln('Thank you, come again!'); end. (* Program *) Procedure definition Procedure call James Tam
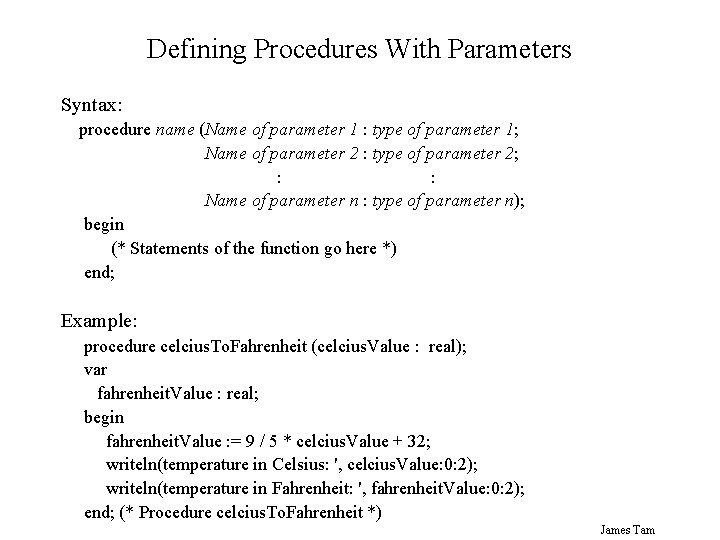
Defining Procedures With Parameters Syntax: procedure name (Name of parameter 1 : type of parameter 1; Name of parameter 2 : type of parameter 2; : : Name of parameter n : type of parameter n); begin (* Statements of the function go here *) end; Example: procedure celcius. To. Fahrenheit (celcius. Value : real); var fahrenheit. Value : real; begin fahrenheit. Value : = 9 / 5 * celcius. Value + 32; writeln(temperature in Celsius: ', celcius. Value: 0: 2); writeln(temperature in Fahrenheit: ', fahrenheit. Value: 0: 2); end; (* Procedure celcius. To. Fahrenheit *) James Tam
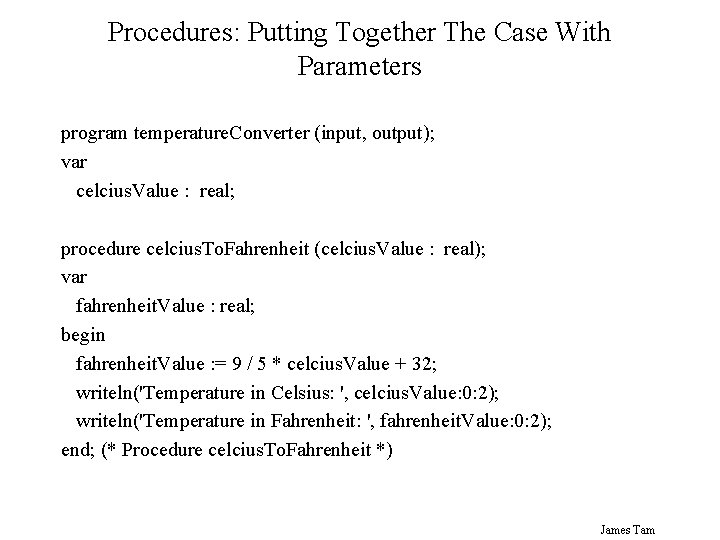
Procedures: Putting Together The Case With Parameters program temperature. Converter (input, output); var celcius. Value : real; procedure celcius. To. Fahrenheit (celcius. Value : real); var fahrenheit. Value : real; begin fahrenheit. Value : = 9 / 5 * celcius. Value + 32; writeln('Temperature in Celsius: ', celcius. Value: 0: 2); writeln('Temperature in Fahrenheit: ', fahrenheit. Value: 0: 2); end; (* Procedure celcius. To. Fahrenheit *) James Tam
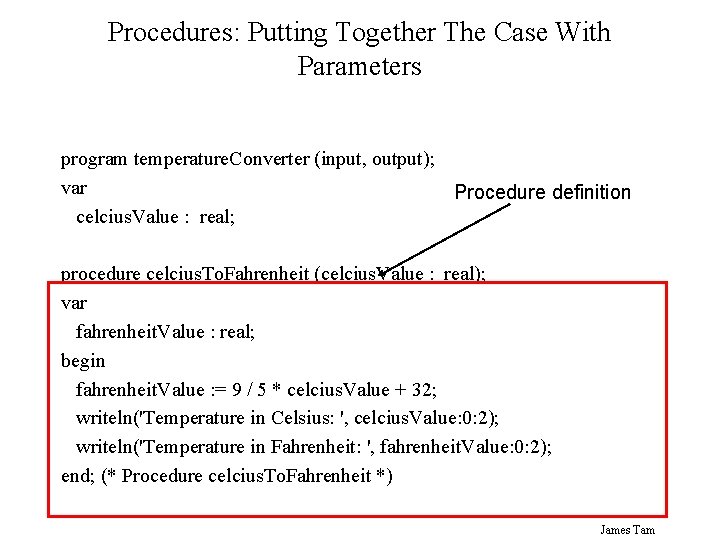
Procedures: Putting Together The Case With Parameters program temperature. Converter (input, output); var Procedure definition celcius. Value : real; procedure celcius. To. Fahrenheit (celcius. Value : real); var fahrenheit. Value : real; begin fahrenheit. Value : = 9 / 5 * celcius. Value + 32; writeln('Temperature in Celsius: ', celcius. Value: 0: 2); writeln('Temperature in Fahrenheit: ', fahrenheit. Value: 0: 2); end; (* Procedure celcius. To. Fahrenheit *) James Tam
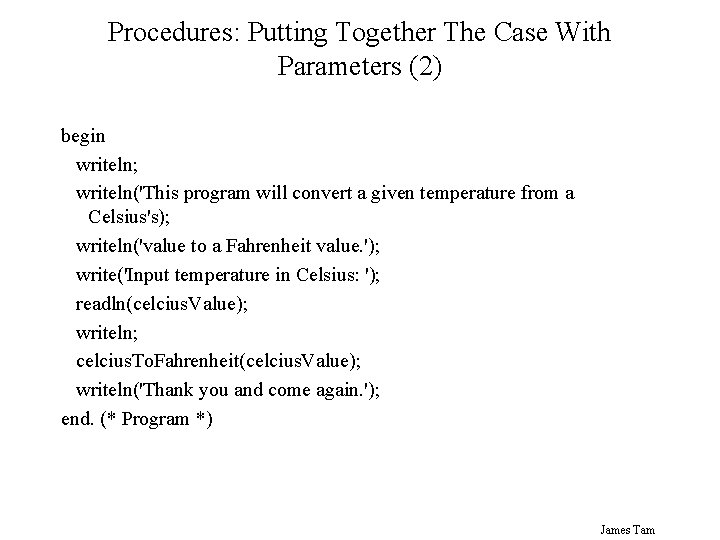
Procedures: Putting Together The Case With Parameters (2) begin writeln; writeln('This program will convert a given temperature from a Celsius's); writeln('value to a Fahrenheit value. '); write('Input temperature in Celsius: '); readln(celcius. Value); writeln; celcius. To. Fahrenheit(celcius. Value); writeln('Thank you and come again. '); end. (* Program *) James Tam
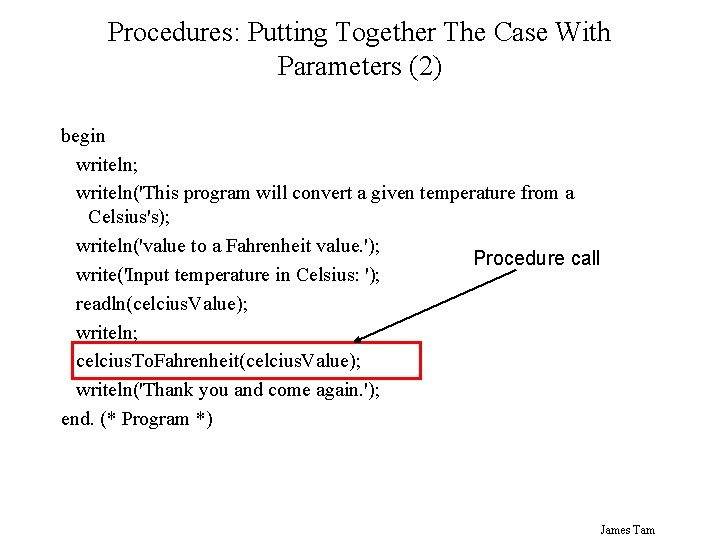
Procedures: Putting Together The Case With Parameters (2) begin writeln; writeln('This program will convert a given temperature from a Celsius's); writeln('value to a Fahrenheit value. '); Procedure call write('Input temperature in Celsius: '); readln(celcius. Value); writeln; celcius. To. Fahrenheit(celcius. Value); writeln('Thank you and come again. '); end. (* Program *) James Tam
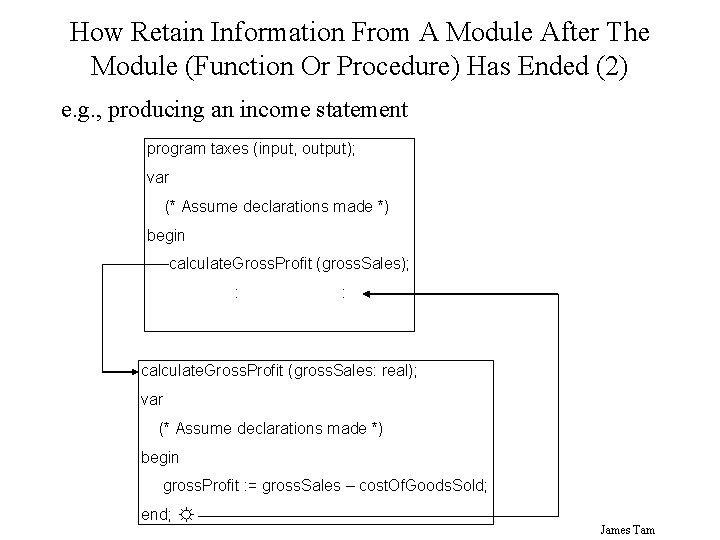
How Retain Information From A Module After The Module (Function Or Procedure) Has Ended (2) e. g. , producing an income statement program taxes (input, output); var (* Assume declarations made *) begin calculate. Gross. Profit (gross. Sales); : : calculate. Gross. Profit (gross. Sales: real); var (* Assume declarations made *) begin gross. Profit : = gross. Sales – cost. Of. Goods. Sold; end; ☼ James Tam
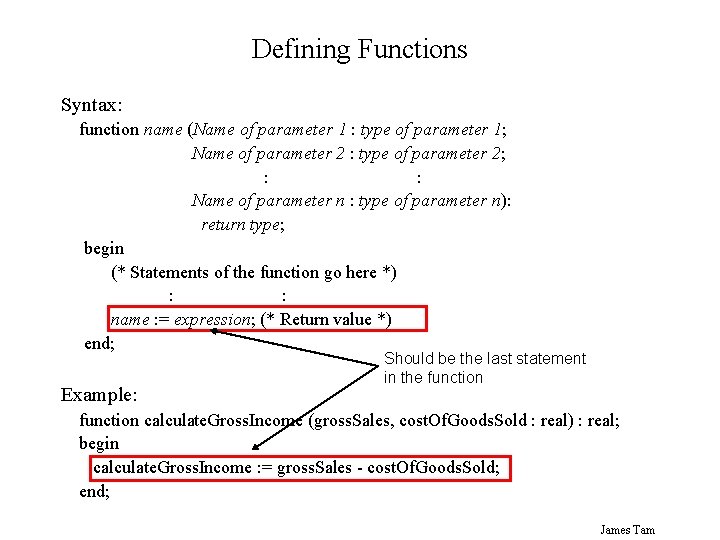
Defining Functions Syntax: function name (Name of parameter 1 : type of parameter 1; Name of parameter 2 : type of parameter 2; : : Name of parameter n : type of parameter n): return type; begin (* Statements of the function go here *) : : name : = expression; (* Return value *) end; Example: Should be the last statement in the function calculate. Gross. Income (gross. Sales, cost. Of. Goods. Sold : real) : real; begin calculate. Gross. Income : = gross. Sales - cost. Of. Goods. Sold; end; James Tam
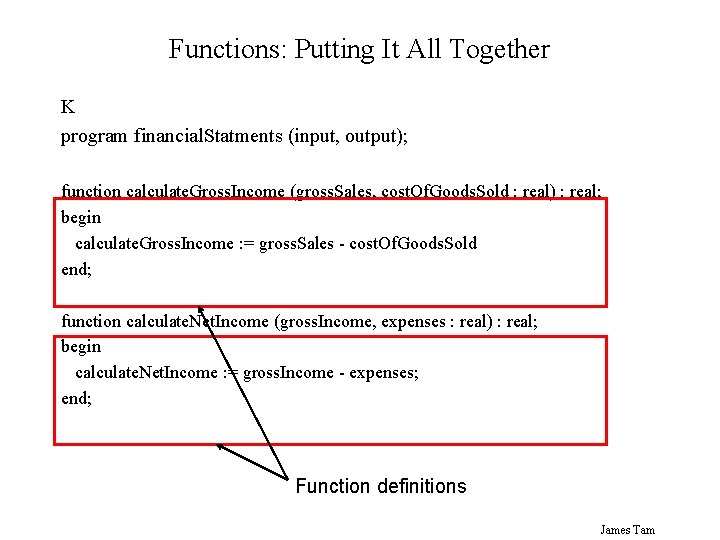
Functions: Putting It All Together K program financial. Statments (input, output); function calculate. Gross. Income (gross. Sales, cost. Of. Goods. Sold : real) : real; begin calculate. Gross. Income : = gross. Sales - cost. Of. Goods. Sold end; function calculate. Net. Income (gross. Income, expenses : real) : real; begin calculate. Net. Income : = gross. Income - expenses; end; Function definitions James Tam
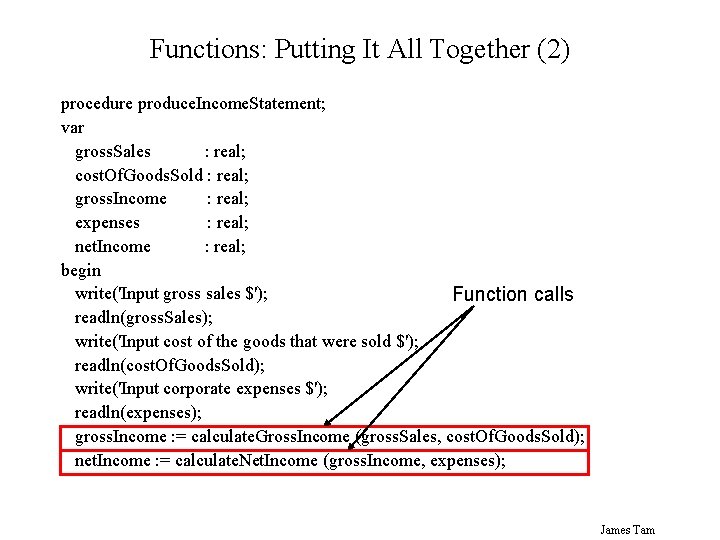
Functions: Putting It All Together (2) procedure produce. Income. Statement; var gross. Sales : real; cost. Of. Goods. Sold : real; gross. Income : real; expenses : real; net. Income : real; begin write('Input gross sales $'); Function calls readln(gross. Sales); write('Input cost of the goods that were sold $'); readln(cost. Of. Goods. Sold); write('Input corporate expenses $'); readln(expenses); gross. Income : = calculate. Gross. Income (gross. Sales, cost. Of. Goods. Sold); net. Income : = calculate. Net. Income (gross. Income, expenses); James Tam
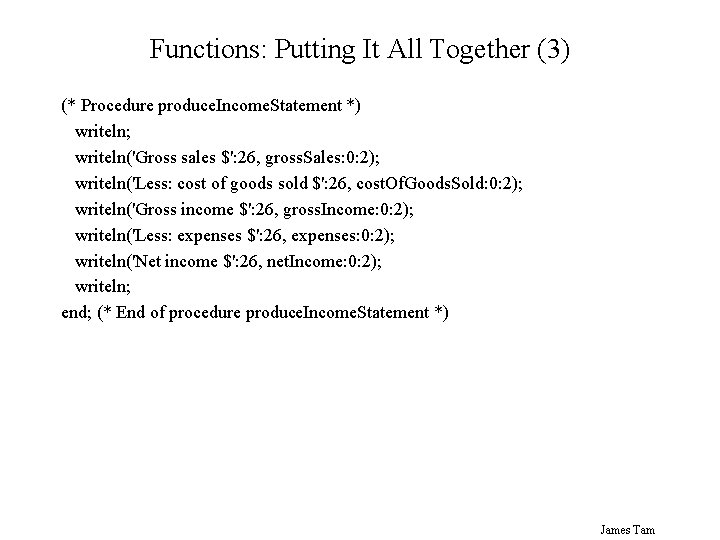
Functions: Putting It All Together (3) (* Procedure produce. Income. Statement *) writeln; writeln('Gross sales $': 26, gross. Sales: 0: 2); writeln('Less: cost of goods sold $': 26, cost. Of. Goods. Sold: 0: 2); writeln('Gross income $': 26, gross. Income: 0: 2); writeln('Less: expenses $': 26, expenses: 0: 2); writeln('Net income $': 26, net. Income: 0: 2); writeln; end; (* End of procedure produce. Income. Statement *) James Tam
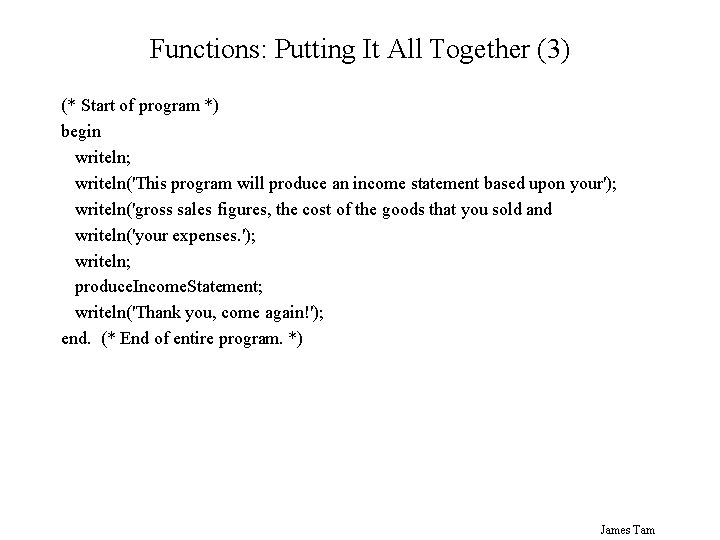
Functions: Putting It All Together (3) (* Start of program *) begin writeln; writeln('This program will produce an income statement based upon your'); writeln('gross sales figures, the cost of the goods that you sold and writeln('your expenses. '); writeln; produce. Income. Statement; writeln('Thank you, come again!'); end. (* End of entire program. *) James Tam
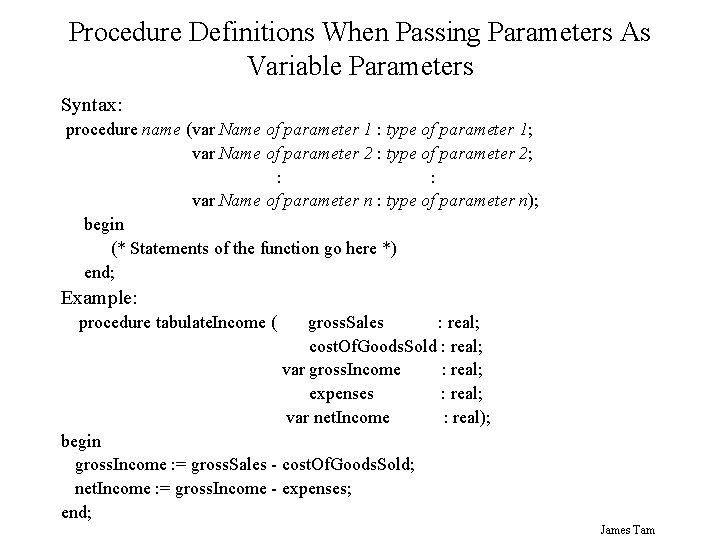
Procedure Definitions When Passing Parameters As Variable Parameters Syntax: procedure name (var Name of parameter 1 : type of parameter 1; var Name of parameter 2 : type of parameter 2; : : var Name of parameter n : type of parameter n); begin (* Statements of the function go here *) end; Example: procedure tabulate. Income ( gross. Sales : real; cost. Of. Goods. Sold : real; var gross. Income : real; expenses : real; var net. Income : real); begin gross. Income : = gross. Sales - cost. Of. Goods. Sold; net. Income : = gross. Income - expenses; end; James Tam
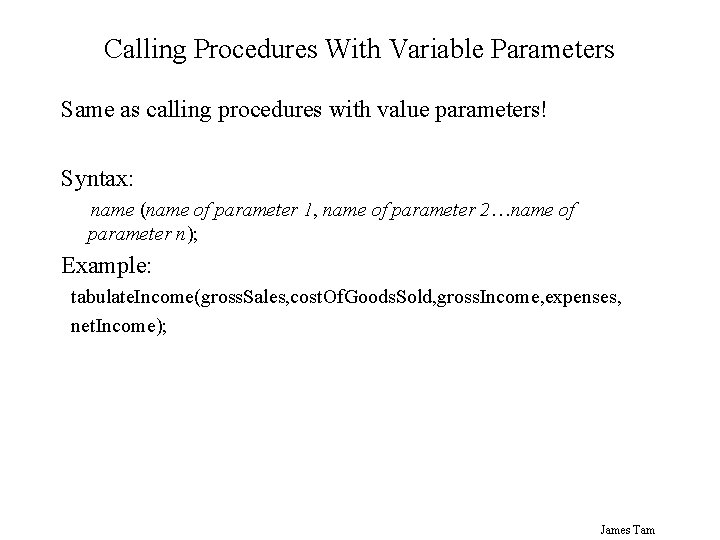
Calling Procedures With Variable Parameters Same as calling procedures with value parameters! Syntax: name (name of parameter 1, name of parameter 2…name of parameter n); Example: tabulate. Income(gross. Sales, cost. Of. Goods. Sold, gross. Income, expenses, net. Income); James Tam
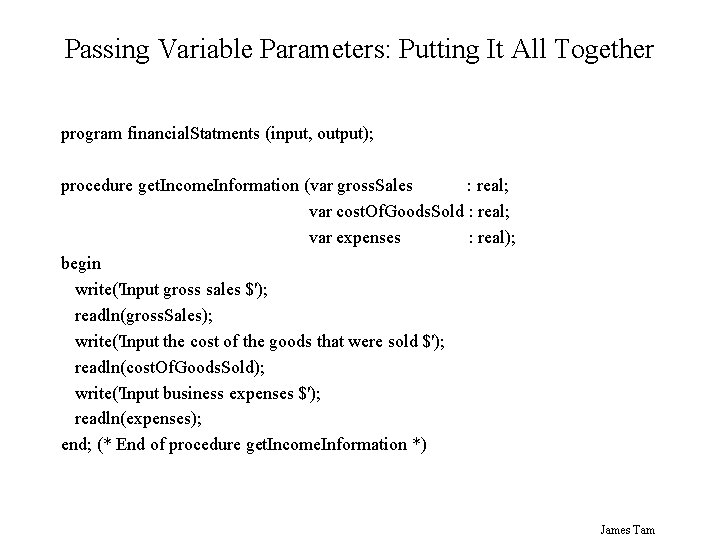
Passing Variable Parameters: Putting It All Together program financial. Statments (input, output); procedure get. Income. Information (var gross. Sales : real; var cost. Of. Goods. Sold : real; var expenses : real); begin write('Input gross sales $'); readln(gross. Sales); write('Input the cost of the goods that were sold $'); readln(cost. Of. Goods. Sold); write('Input business expenses $'); readln(expenses); end; (* End of procedure get. Income. Information *) James Tam
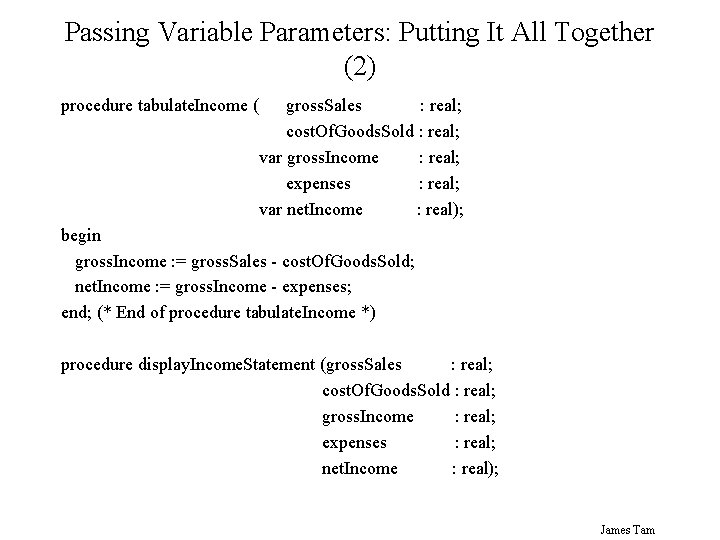
Passing Variable Parameters: Putting It All Together (2) procedure tabulate. Income ( gross. Sales : real; cost. Of. Goods. Sold : real; var gross. Income : real; expenses : real; var net. Income : real); begin gross. Income : = gross. Sales - cost. Of. Goods. Sold; net. Income : = gross. Income - expenses; end; (* End of procedure tabulate. Income *) procedure display. Income. Statement (gross. Sales : real; cost. Of. Goods. Sold : real; gross. Income : real; expenses : real; net. Income : real); James Tam
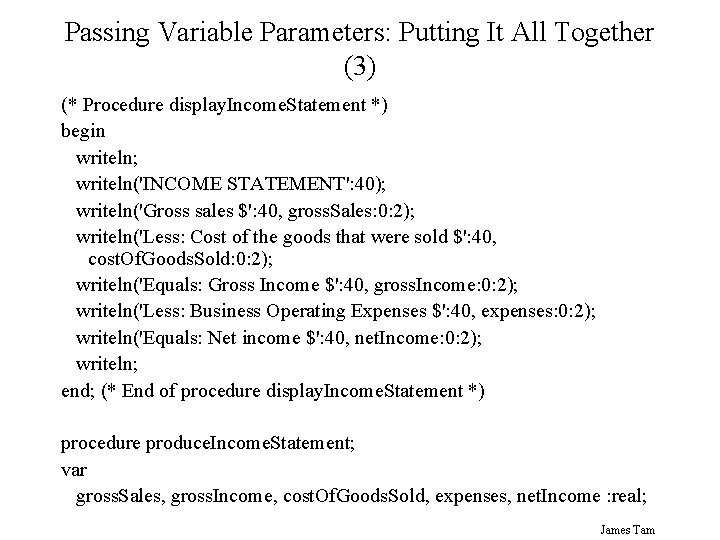
Passing Variable Parameters: Putting It All Together (3) (* Procedure display. Income. Statement *) begin writeln; writeln('INCOME STATEMENT': 40); writeln('Gross sales $': 40, gross. Sales: 0: 2); writeln('Less: Cost of the goods that were sold $': 40, cost. Of. Goods. Sold: 0: 2); writeln('Equals: Gross Income $': 40, gross. Income: 0: 2); writeln('Less: Business Operating Expenses $': 40, expenses: 0: 2); writeln('Equals: Net income $': 40, net. Income: 0: 2); writeln; end; (* End of procedure display. Income. Statement *) procedure produce. Income. Statement; var gross. Sales, gross. Income, cost. Of. Goods. Sold, expenses, net. Income : real; James Tam
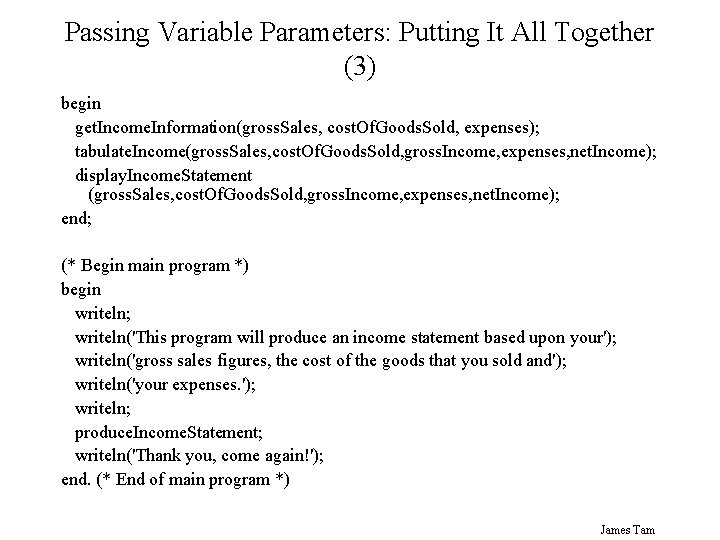
Passing Variable Parameters: Putting It All Together (3) begin get. Income. Information(gross. Sales, cost. Of. Goods. Sold, expenses); tabulate. Income(gross. Sales, cost. Of. Goods. Sold, gross. Income, expenses, net. Income); display. Income. Statement (gross. Sales, cost. Of. Goods. Sold, gross. Income, expenses, net. Income); end; (* Begin main program *) begin writeln; writeln('This program will produce an income statement based upon your'); writeln('gross sales figures, the cost of the goods that you sold and'); writeln('your expenses. '); writeln; produce. Income. Statement; writeln('Thank you, come again!'); end. (* End of main program *) James Tam
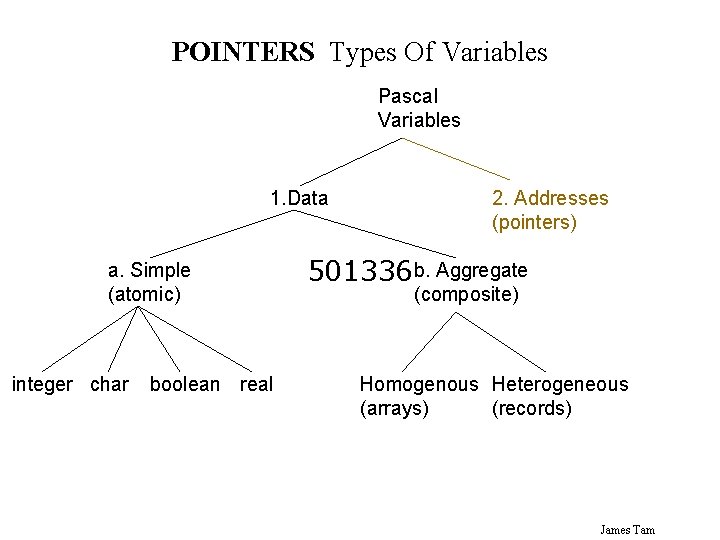
POINTERS Types Of Variables Pascal Variables 1. Data a. Simple (atomic) integer char boolean real 2. Addresses (pointers) 501336 b. Aggregate (composite) Homogenous Heterogeneous (arrays) (records) James Tam
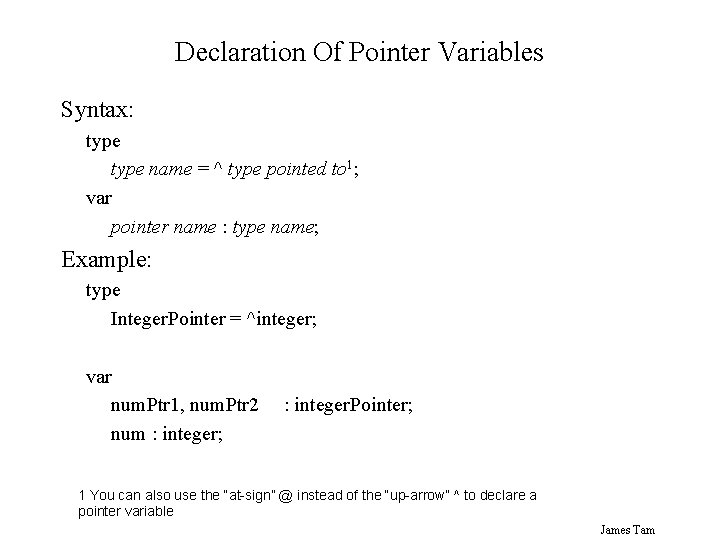
Declaration Of Pointer Variables Syntax: type name = ^ type pointed to 1; var pointer name : type name; Example: type Integer. Pointer = ^integer; var num. Ptr 1, num. Ptr 2 num : integer; : integer. Pointer; 1 You can also use the “at-sign” @ instead of the “up-arrow” ^ to declare a pointer variable James Tam
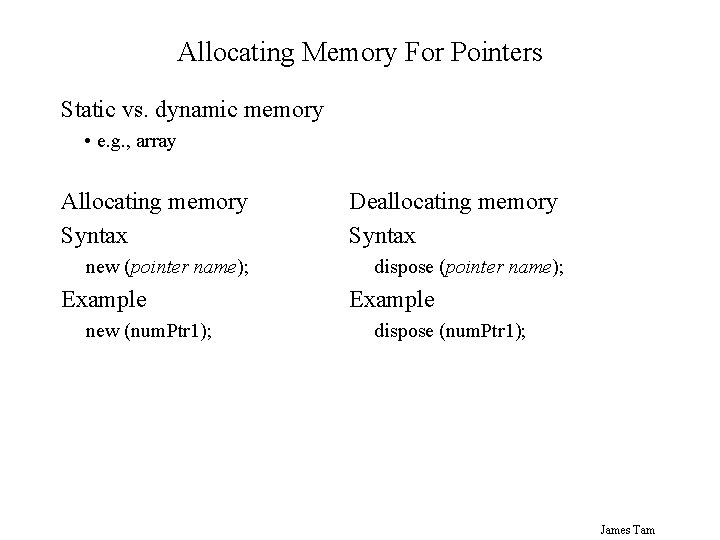
Allocating Memory For Pointers Static vs. dynamic memory • e. g. , array Allocating memory Syntax new (pointer name); Example new (num. Ptr 1); Deallocating memory Syntax dispose (pointer name); Example dispose (num. Ptr 1); James Tam
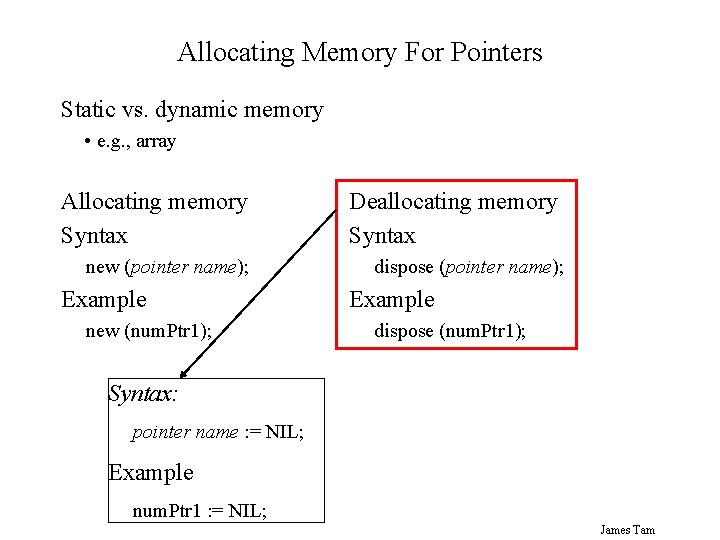
Allocating Memory For Pointers Static vs. dynamic memory • e. g. , array Allocating memory Syntax new (pointer name); Example new (num. Ptr 1); Deallocating memory Syntax dispose (pointer name); Example dispose (num. Ptr 1); Syntax: pointer name : = NIL; Example num. Ptr 1 : = NIL; James Tam
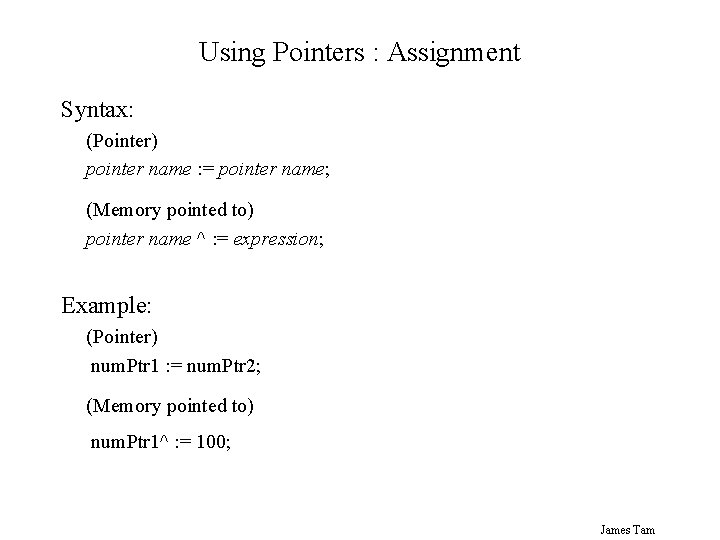
Using Pointers : Assignment Syntax: (Pointer) pointer name : = pointer name; (Memory pointed to) pointer name ^ : = expression; Example: (Pointer) num. Ptr 1 : = num. Ptr 2; (Memory pointed to) num. Ptr 1^ : = 100; James Tam
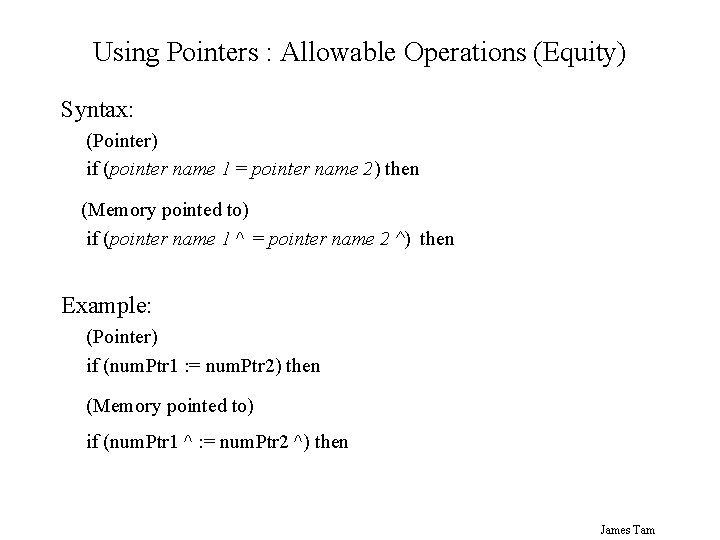
Using Pointers : Allowable Operations (Equity) Syntax: (Pointer) if (pointer name 1 = pointer name 2) then (Memory pointed to) if (pointer name 1 ^ = pointer name 2 ^) then Example: (Pointer) if (num. Ptr 1 : = num. Ptr 2) then (Memory pointed to) if (num. Ptr 1 ^ : = num. Ptr 2 ^) then James Tam
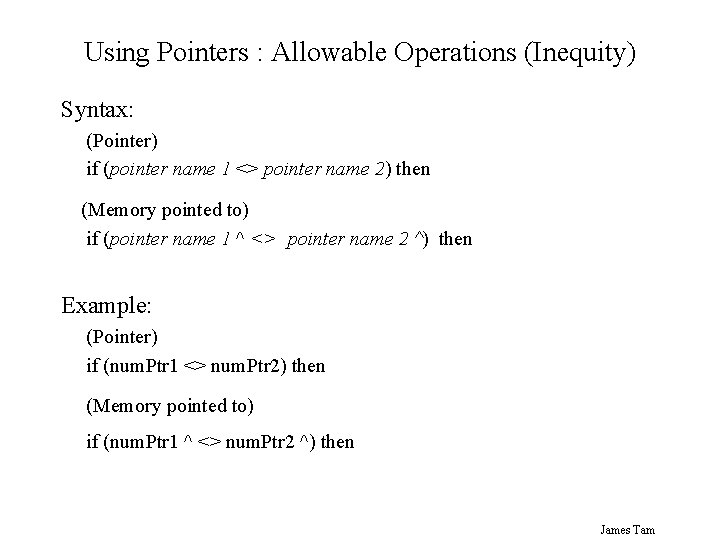
Using Pointers : Allowable Operations (Inequity) Syntax: (Pointer) if (pointer name 1 <> pointer name 2) then (Memory pointed to) if (pointer name 1 ^ <> pointer name 2 ^) then Example: (Pointer) if (num. Ptr 1 <> num. Ptr 2) then (Memory pointed to) if (num. Ptr 1 ^ <> num. Ptr 2 ^) then James Tam
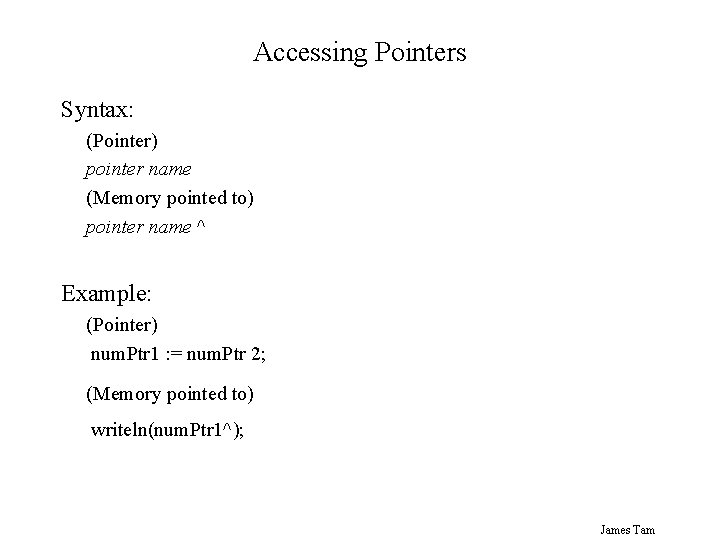
Accessing Pointers Syntax: (Pointer) pointer name (Memory pointed to) pointer name ^ Example: (Pointer) num. Ptr 1 : = num. Ptr 2; (Memory pointed to) writeln(num. Ptr 1^); James Tam
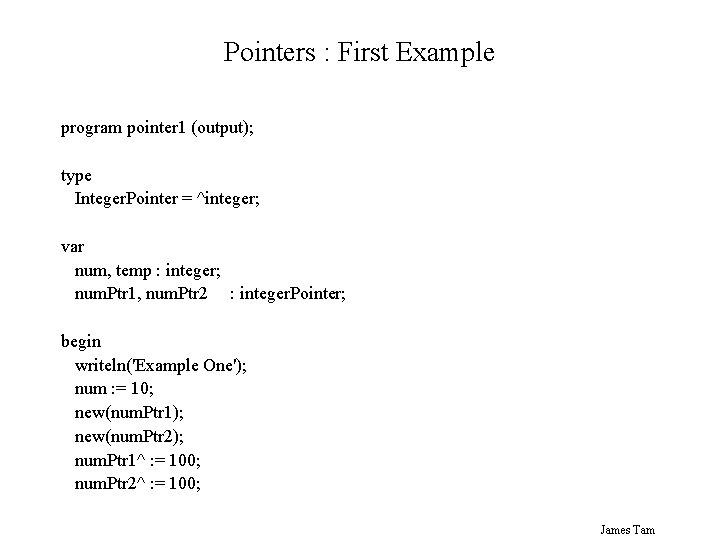
Pointers : First Example program pointer 1 (output); type Integer. Pointer = ^integer; var num, temp : integer; num. Ptr 1, num. Ptr 2 : integer. Pointer; begin writeln('Example One'); num : = 10; new(num. Ptr 1); new(num. Ptr 2); num. Ptr 1^ : = 100; num. Ptr 2^ : = 100; James Tam
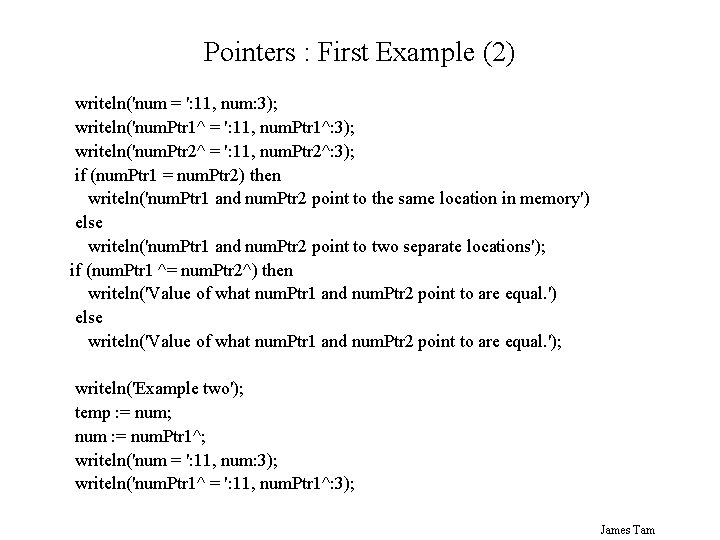
Pointers : First Example (2) writeln('num = ': 11, num: 3); writeln('num. Ptr 1^ = ': 11, num. Ptr 1^: 3); writeln('num. Ptr 2^ = ': 11, num. Ptr 2^: 3); if (num. Ptr 1 = num. Ptr 2) then writeln('num. Ptr 1 and num. Ptr 2 point to the same location in memory') else writeln('num. Ptr 1 and num. Ptr 2 point to two separate locations'); if (num. Ptr 1 ^= num. Ptr 2^) then writeln('Value of what num. Ptr 1 and num. Ptr 2 point to are equal. ') else writeln('Value of what num. Ptr 1 and num. Ptr 2 point to are equal. '); writeln('Example two'); temp : = num; num : = num. Ptr 1^; writeln('num = ': 11, num: 3); writeln('num. Ptr 1^ = ': 11, num. Ptr 1^: 3); James Tam
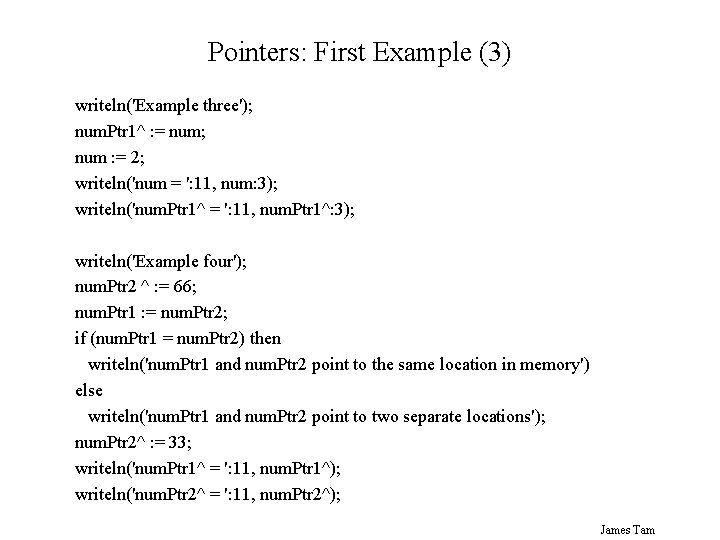
Pointers: First Example (3) writeln('Example three'); num. Ptr 1^ : = num; num : = 2; writeln('num = ': 11, num: 3); writeln('num. Ptr 1^ = ': 11, num. Ptr 1^: 3); writeln('Example four'); num. Ptr 2 ^ : = 66; num. Ptr 1 : = num. Ptr 2; if (num. Ptr 1 = num. Ptr 2) then writeln('num. Ptr 1 and num. Ptr 2 point to the same location in memory') else writeln('num. Ptr 1 and num. Ptr 2 point to two separate locations'); num. Ptr 2^ : = 33; writeln('num. Ptr 1^ = ': 11, num. Ptr 1^); writeln('num. Ptr 2^ = ': 11, num. Ptr 2^); James Tam
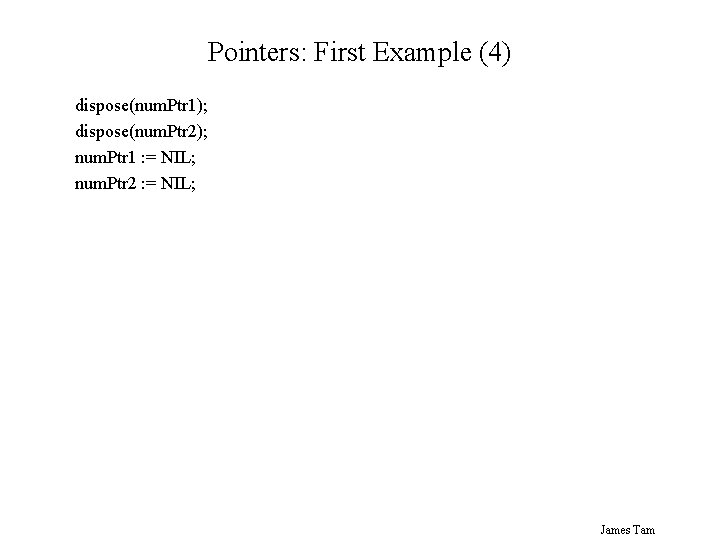
Pointers: First Example (4) dispose(num. Ptr 1); dispose(num. Ptr 2); num. Ptr 1 : = NIL; num. Ptr 2 : = NIL; James Tam
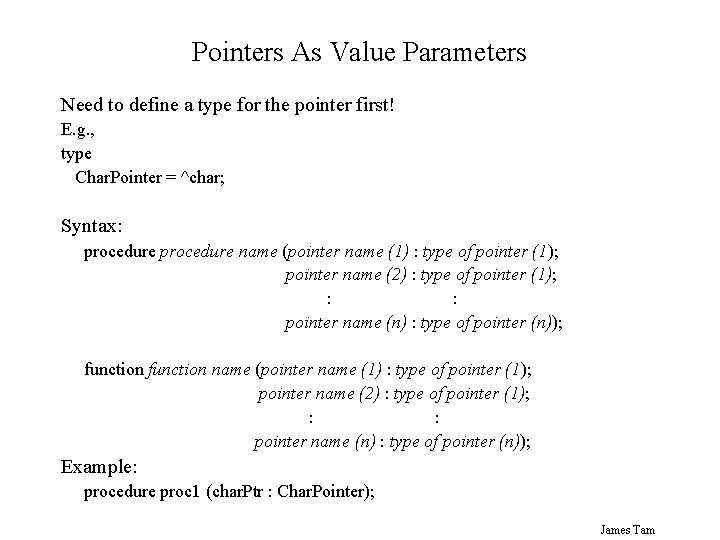
Pointers As Value Parameters Need to define a type for the pointer first! E. g. , type Char. Pointer = ^char; Syntax: procedure name (pointer name (1) : type of pointer (1); pointer name (2) : type of pointer (1); : : pointer name (n) : type of pointer (n)); function name (pointer name (1) : type of pointer (1); pointer name (2) : type of pointer (1); : : pointer name (n) : type of pointer (n)); Example: procedure proc 1 (char. Ptr : Char. Pointer); James Tam
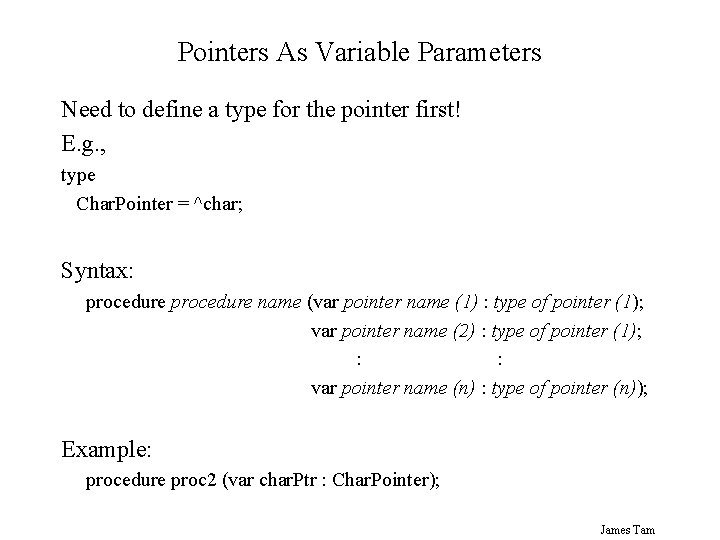
Pointers As Variable Parameters Need to define a type for the pointer first! E. g. , type Char. Pointer = ^char; Syntax: procedure name (var pointer name (1) : type of pointer (1); var pointer name (2) : type of pointer (1); : : var pointer name (n) : type of pointer (n)); Example: procedure proc 2 (var char. Ptr : Char. Pointer); James Tam
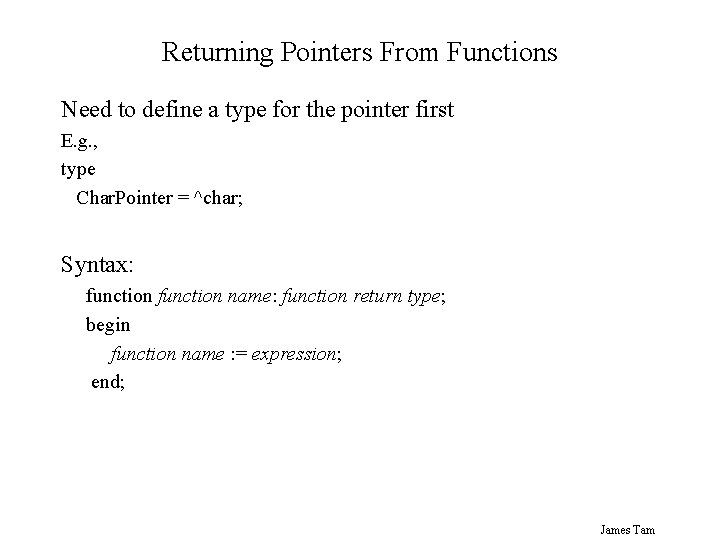
Returning Pointers From Functions Need to define a type for the pointer first E. g. , type Char. Pointer = ^char; Syntax: function name: function return type; begin function name : = expression; end; James Tam
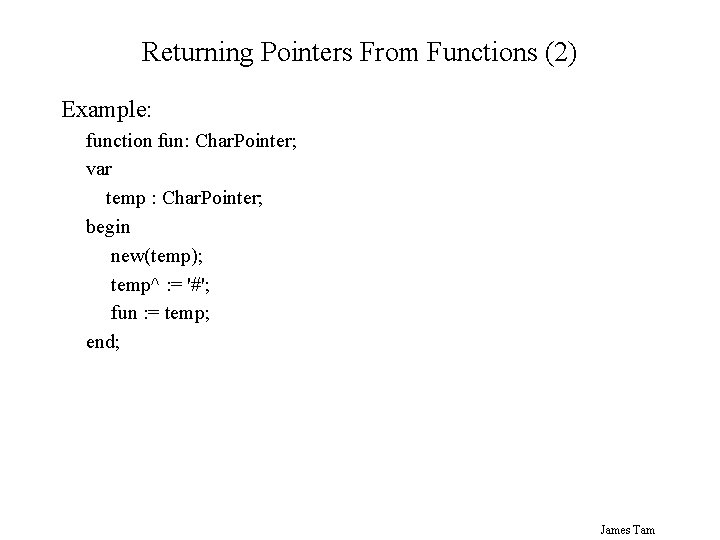
Returning Pointers From Functions (2) Example: function fun: Char. Pointer; var temp : Char. Pointer; begin new(temp); temp^ : = '#'; fun : = temp; end; James Tam
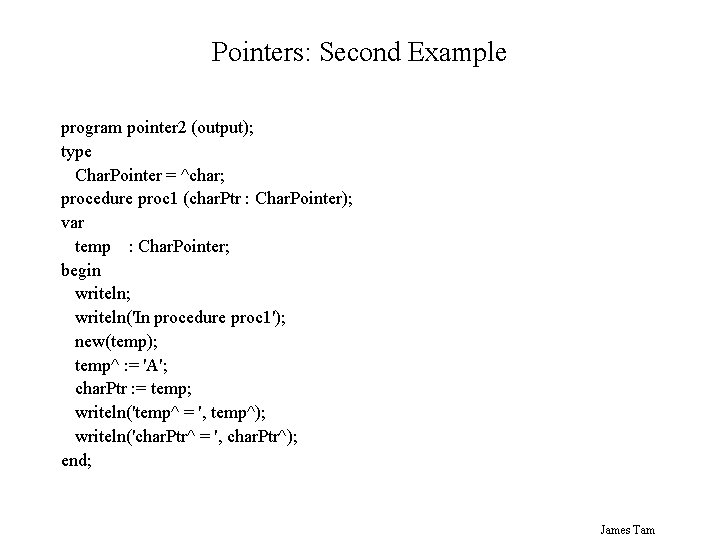
Pointers: Second Example program pointer 2 (output); type Char. Pointer = ^char; procedure proc 1 (char. Ptr : Char. Pointer); var temp : Char. Pointer; begin writeln; writeln('In procedure proc 1'); new(temp); temp^ : = 'A'; char. Ptr : = temp; writeln('temp^ = ', temp^); writeln('char. Ptr^ = ', char. Ptr^); end; James Tam
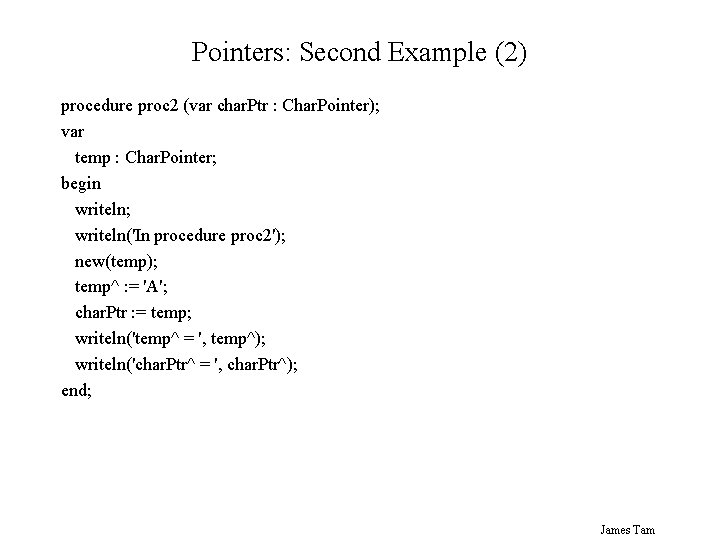
Pointers: Second Example (2) procedure proc 2 (var char. Ptr : Char. Pointer); var temp : Char. Pointer; begin writeln; writeln('In procedure proc 2'); new(temp); temp^ : = 'A'; char. Ptr : = temp; writeln('temp^ = ', temp^); writeln('char. Ptr^ = ', char. Ptr^); end; James Tam
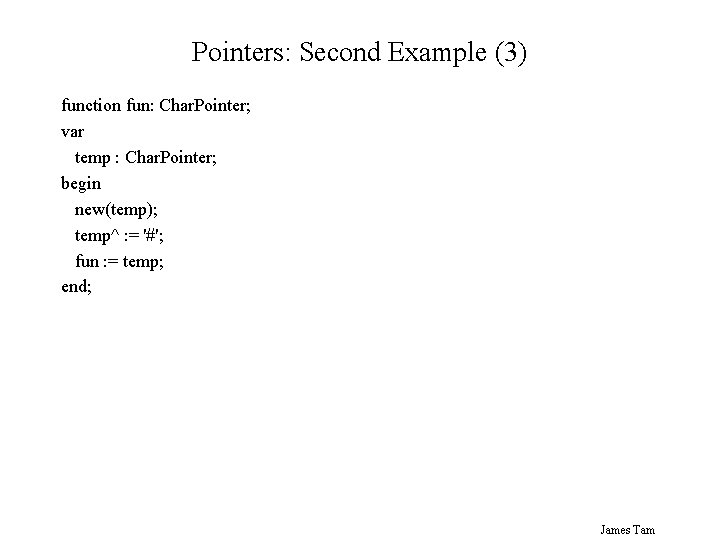
Pointers: Second Example (3) function fun: Char. Pointer; var temp : Char. Pointer; begin new(temp); temp^ : = '#'; fun : = temp; end; James Tam
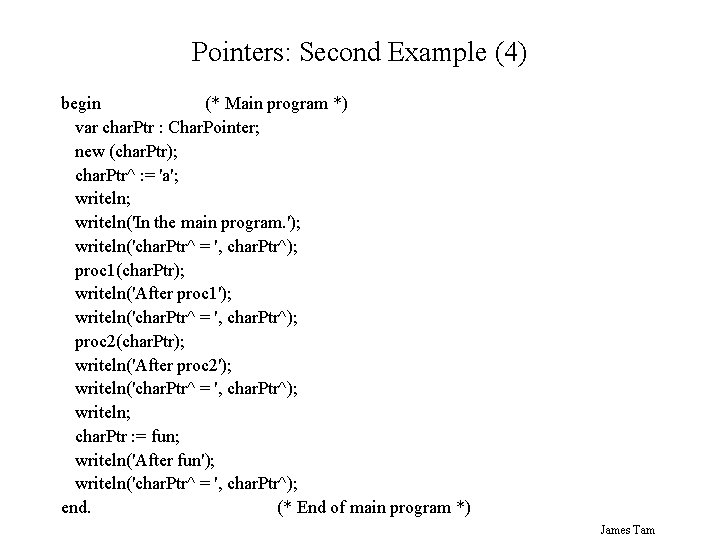
Pointers: Second Example (4) begin (* Main program *) var char. Ptr : Char. Pointer; new (char. Ptr); char. Ptr^ : = 'a'; writeln('In the main program. '); writeln('char. Ptr^ = ', char. Ptr^); proc 1(char. Ptr); writeln('After proc 1'); writeln('char. Ptr^ = ', char. Ptr^); proc 2(char. Ptr); writeln('After proc 2'); writeln('char. Ptr^ = ', char. Ptr^); writeln; char. Ptr : = fun; writeln('After fun'); writeln('char. Ptr^ = ', char. Ptr^); end. (* End of main program *) James Tam
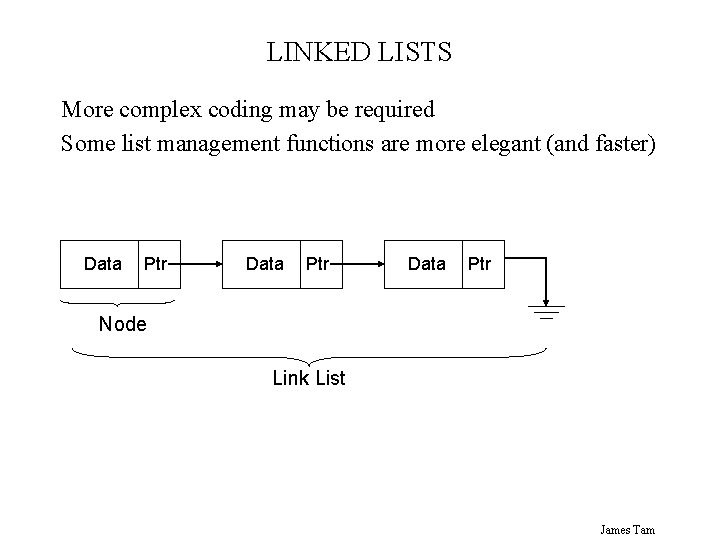
LINKED LISTS More complex coding may be required Some list management functions are more elegant (and faster) Data Ptr Node Link List James Tam
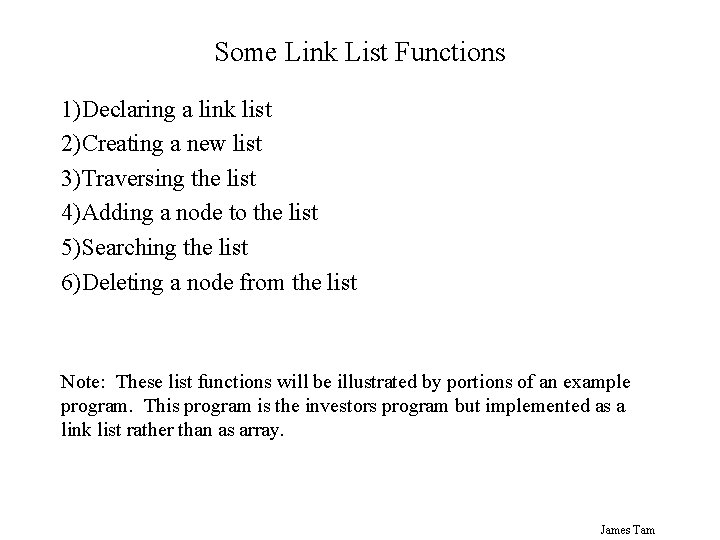
Some Link List Functions 1)Declaring a link list 2)Creating a new list 3)Traversing the list 4)Adding a node to the list 5)Searching the list 6)Deleting a node from the list Note: These list functions will be illustrated by portions of an example program. This program is the investors program but implemented as a link list rather than as array. James Tam
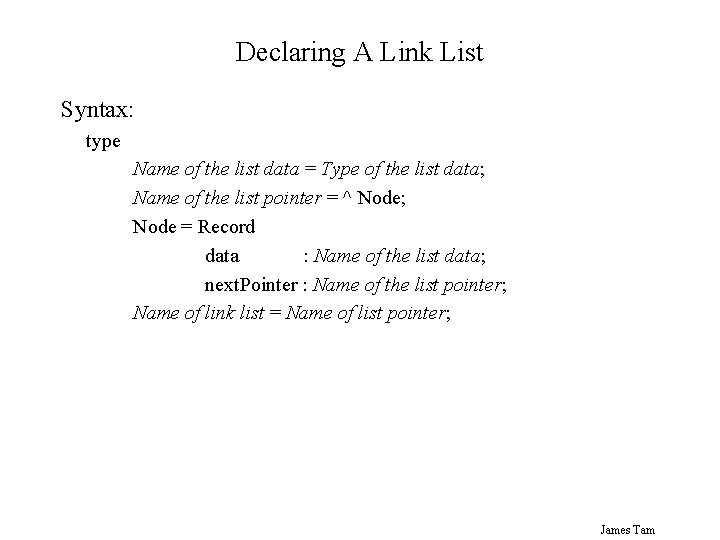
Declaring A Link List Syntax: type Name of the list data = Type of the list data; Name of the list pointer = ^ Node; Node = Record data : Name of the list data; next. Pointer : Name of the list pointer; Name of link list = Name of list pointer; James Tam
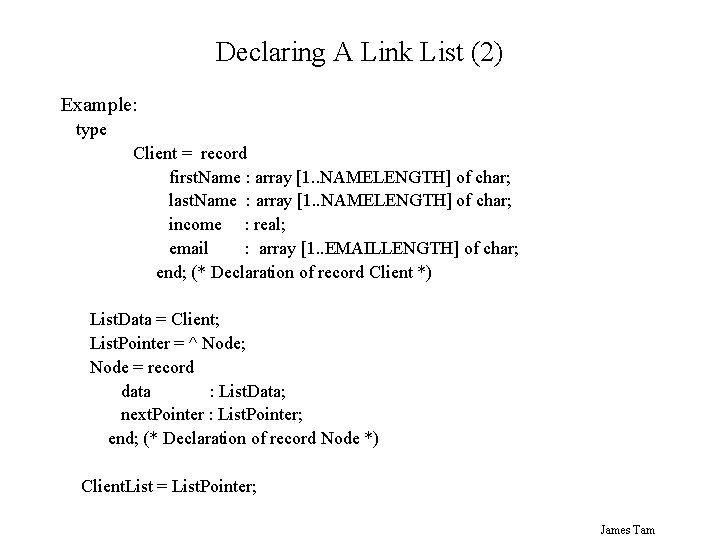
Declaring A Link List (2) Example: type Client = record first. Name : array [1. . NAMELENGTH] of char; last. Name : array [1. . NAMELENGTH] of char; income : real; email : array [1. . EMAILLENGTH] of char; end; (* Declaration of record Client *) List. Data = Client; List. Pointer = ^ Node; Node = record data : List. Data; next. Pointer : List. Pointer; end; (* Declaration of record Node *) Client. List = List. Pointer; James Tam
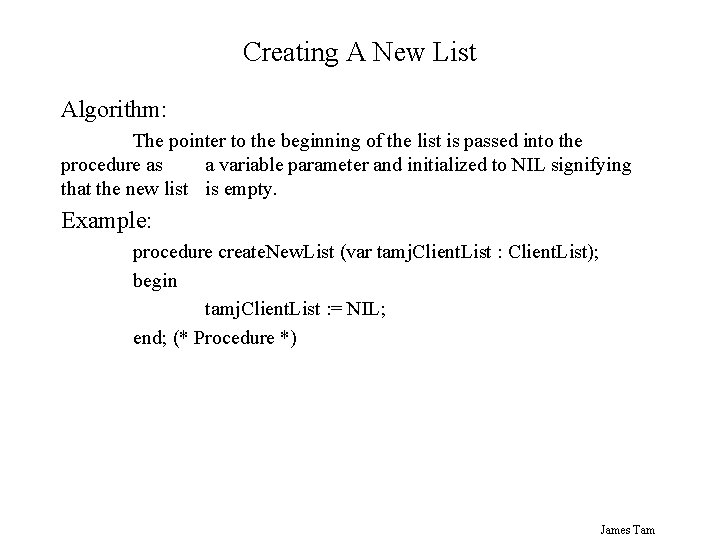
Creating A New List Algorithm: The pointer to the beginning of the list is passed into the procedure as a variable parameter and initialized to NIL signifying that the new list is empty. Example: procedure create. New. List (var tamj. Client. List : Client. List); begin tamj. Client. List : = NIL; end; (* Procedure *) James Tam
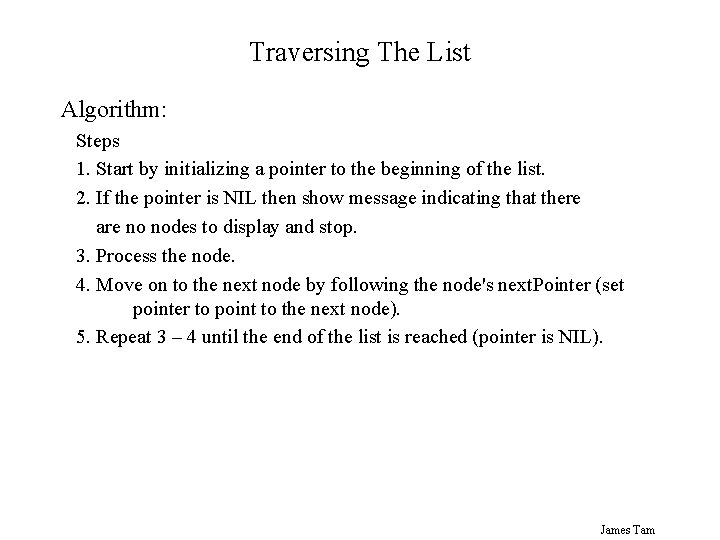
Traversing The List Algorithm: Steps 1. Start by initializing a pointer to the beginning of the list. 2. If the pointer is NIL then show message indicating that there are no nodes to display and stop. 3. Process the node. 4. Move on to the next node by following the node's next. Pointer (set pointer to point to the next node). 5. Repeat 3 – 4 until the end of the list is reached (pointer is NIL). James Tam
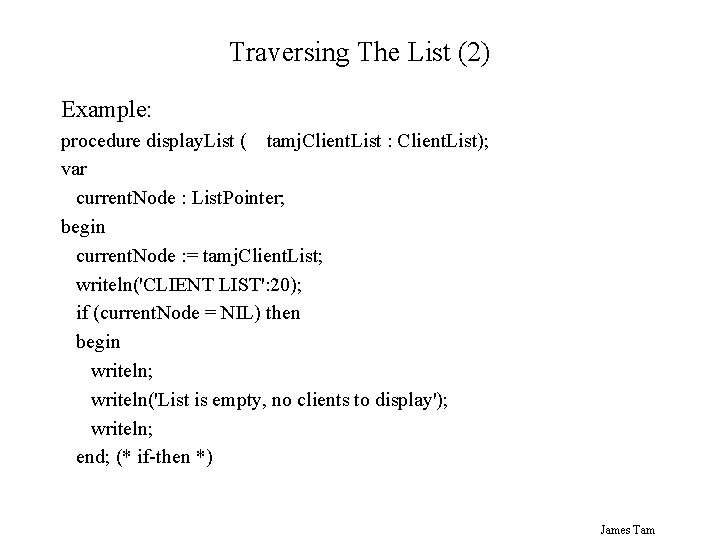
Traversing The List (2) Example: procedure display. List ( tamj. Client. List : Client. List); var current. Node : List. Pointer; begin current. Node : = tamj. Client. List; writeln('CLIENT LIST': 20); if (current. Node = NIL) then begin writeln; writeln('List is empty, no clients to display'); writeln; end; (* if-then *) James Tam
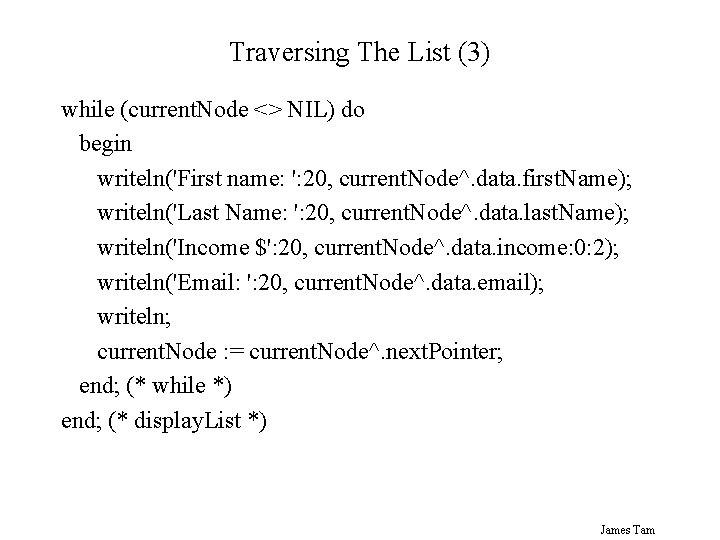
Traversing The List (3) while (current. Node <> NIL) do begin writeln('First name: ': 20, current. Node^. data. first. Name); writeln('Last Name: ': 20, current. Node^. data. last. Name); writeln('Income $': 20, current. Node^. data. income: 0: 2); writeln('Email: ': 20, current. Node^. data. email); writeln; current. Node : = current. Node^. next. Pointer; end; (* while *) end; (* display. List *) James Tam
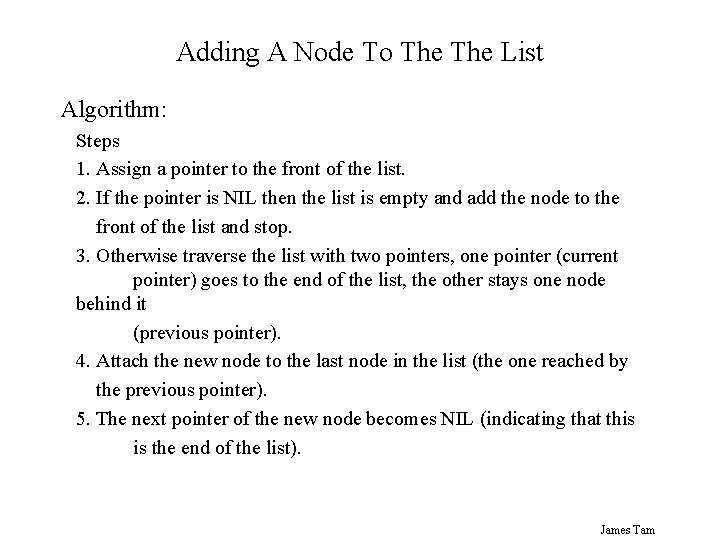
Adding A Node To The List Algorithm: Steps 1. Assign a pointer to the front of the list. 2. If the pointer is NIL then the list is empty and add the node to the front of the list and stop. 3. Otherwise traverse the list with two pointers, one pointer (current pointer) goes to the end of the list, the other stays one node behind it (previous pointer). 4. Attach the new node to the last node in the list (the one reached by the previous pointer). 5. The next pointer of the new node becomes NIL (indicating that this is the end of the list). James Tam
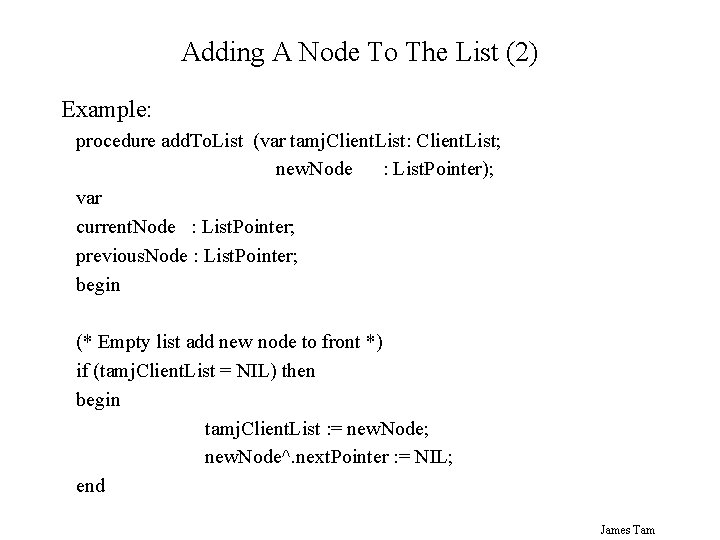
Adding A Node To The List (2) Example: procedure add. To. List (var tamj. Client. List: Client. List; new. Node : List. Pointer); var current. Node : List. Pointer; previous. Node : List. Pointer; begin (* Empty list add new node to front *) if (tamj. Client. List = NIL) then begin tamj. Client. List : = new. Node; new. Node^. next. Pointer : = NIL; end James Tam
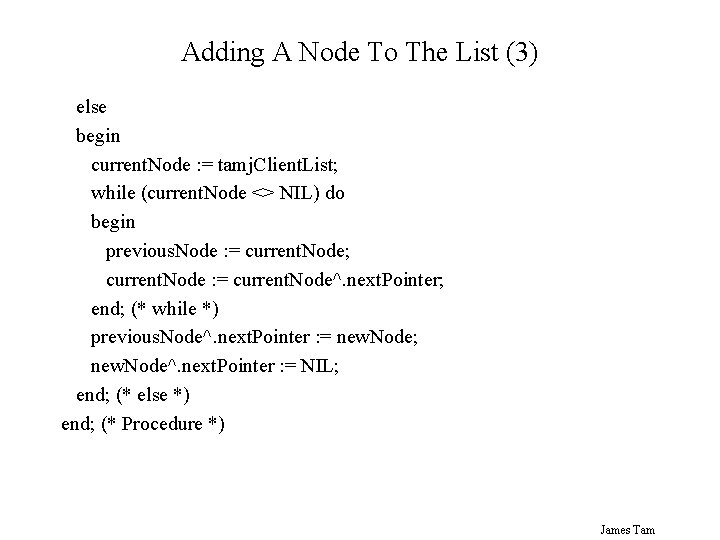
Adding A Node To The List (3) else begin current. Node : = tamj. Client. List; while (current. Node <> NIL) do begin previous. Node : = current. Node; current. Node : = current. Node^. next. Pointer; end; (* while *) previous. Node^. next. Pointer : = new. Node; new. Node^. next. Pointer : = NIL; end; (* else *) end; (* Procedure *) James Tam
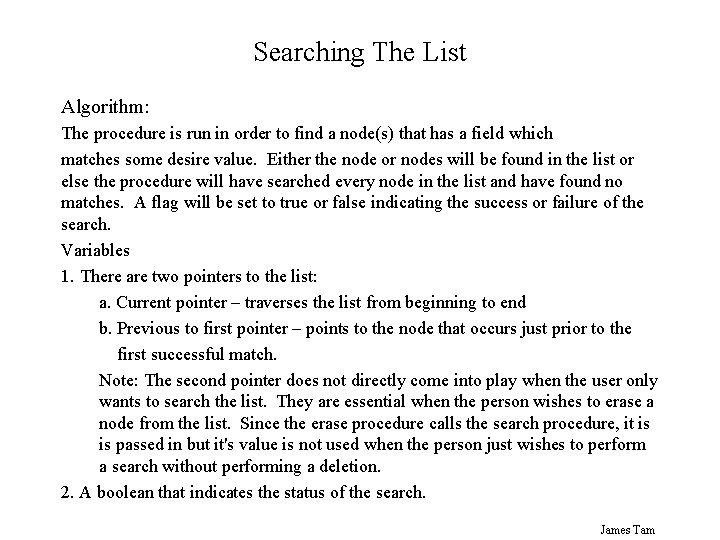
Searching The List Algorithm: The procedure is run in order to find a node(s) that has a field which matches some desire value. Either the node or nodes will be found in the list or else the procedure will have searched every node in the list and have found no matches. A flag will be set to true or false indicating the success or failure of the search. Variables 1. There are two pointers to the list: a. Current pointer – traverses the list from beginning to end b. Previous to first pointer – points to the node that occurs just prior to the first successful match. Note: The second pointer does not directly come into play when the user only wants to search the list. They are essential when the person wishes to erase a node from the list. Since the erase procedure calls the search procedure, it is is passed in but it's value is not used when the person just wishes to perform a search without performing a deletion. 2. A boolean that indicates the status of the search. James Tam
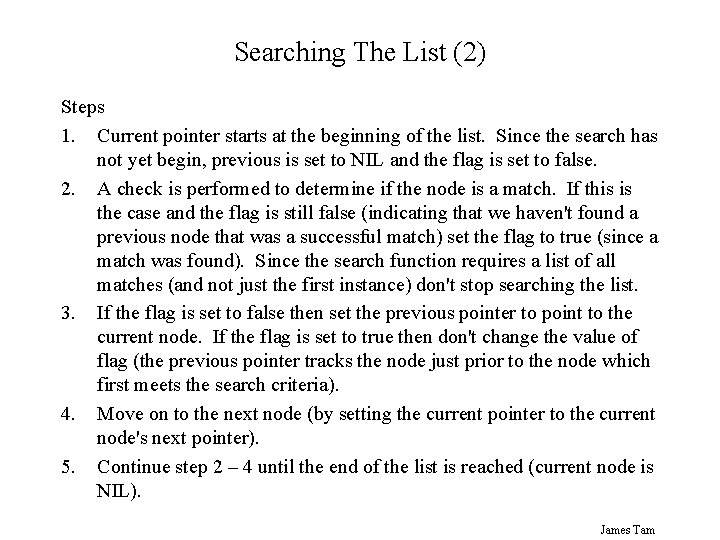
Searching The List (2) Steps 1. Current pointer starts at the beginning of the list. Since the search has not yet begin, previous is set to NIL and the flag is set to false. 2. A check is performed to determine if the node is a match. If this is the case and the flag is still false (indicating that we haven't found a previous node that was a successful match) set the flag to true (since a match was found). Since the search function requires a list of all matches (and not just the first instance) don't stop searching the list. 3. If the flag is set to false then set the previous pointer to point to the current node. If the flag is set to true then don't change the value of flag (the previous pointer tracks the node just prior to the node which first meets the search criteria). 4. Move on to the next node (by setting the current pointer to the current node's next pointer). 5. Continue step 2 – 4 until the end of the list is reached (current node is NIL). James Tam
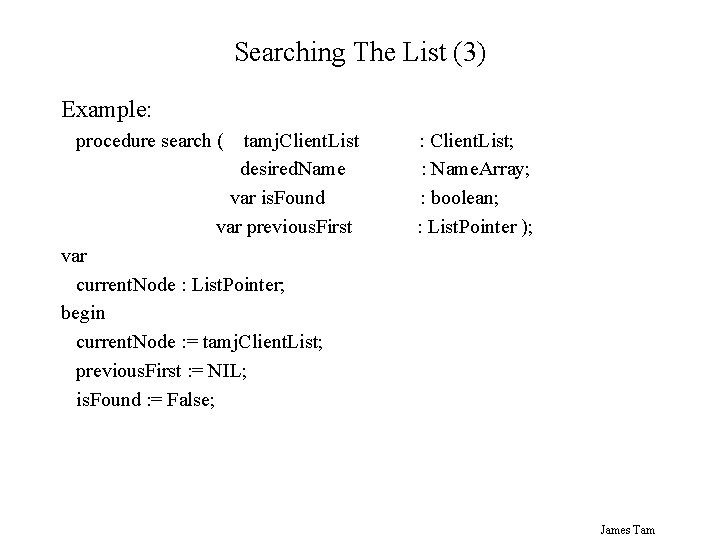
Searching The List (3) Example: procedure search ( tamj. Client. List desired. Name var is. Found var previous. First : Client. List; : Name. Array; : boolean; : List. Pointer ); var current. Node : List. Pointer; begin current. Node : = tamj. Client. List; previous. First : = NIL; is. Found : = False; James Tam
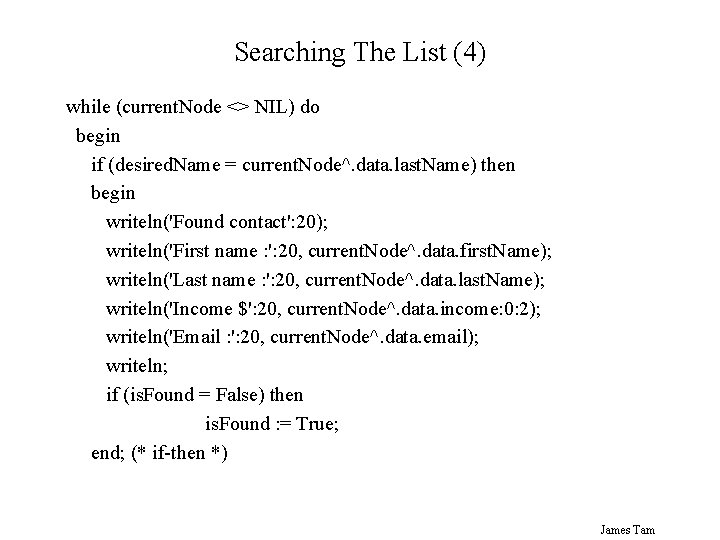
Searching The List (4) while (current. Node <> NIL) do begin if (desired. Name = current. Node^. data. last. Name) then begin writeln('Found contact': 20); writeln('First name : ': 20, current. Node^. data. first. Name); writeln('Last name : ': 20, current. Node^. data. last. Name); writeln('Income $': 20, current. Node^. data. income: 0: 2); writeln('Email : ': 20, current. Node^. data. email); writeln; if (is. Found = False) then is. Found : = True; end; (* if-then *) James Tam
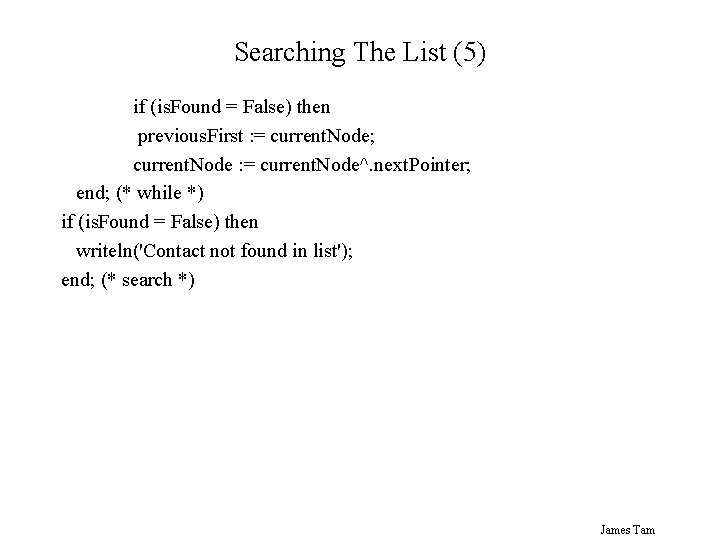
Searching The List (5) if (is. Found = False) then previous. First : = current. Node; current. Node : = current. Node^. next. Pointer; end; (* while *) if (is. Found = False) then writeln('Contact not found in list'); end; (* search *) James Tam
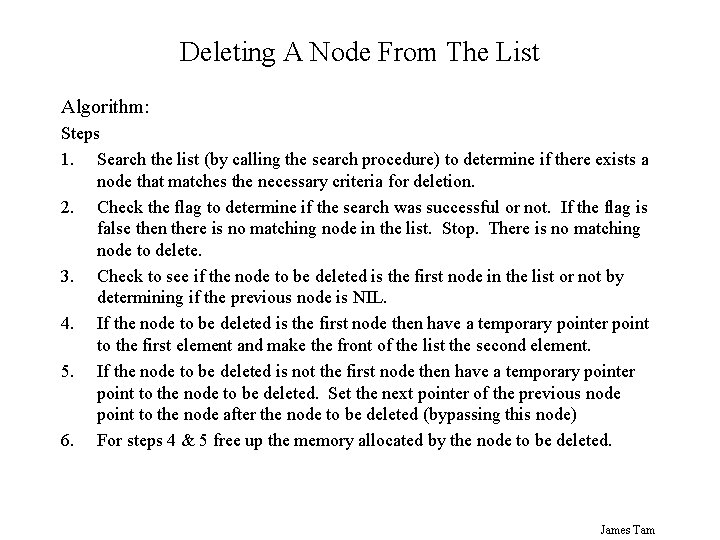
Deleting A Node From The List Algorithm: Steps 1. Search the list (by calling the search procedure) to determine if there exists a node that matches the necessary criteria for deletion. 2. Check the flag to determine if the search was successful or not. If the flag is false then there is no matching node in the list. Stop. There is no matching node to delete. 3. Check to see if the node to be deleted is the first node in the list or not by determining if the previous node is NIL. 4. If the node to be deleted is the first node then have a temporary pointer point to the first element and make the front of the list the second element. 5. If the node to be deleted is not the first node then have a temporary pointer point to the node to be deleted. Set the next pointer of the previous node point to the node after the node to be deleted (bypassing this node) 6. For steps 4 & 5 free up the memory allocated by the node to be deleted. James Tam
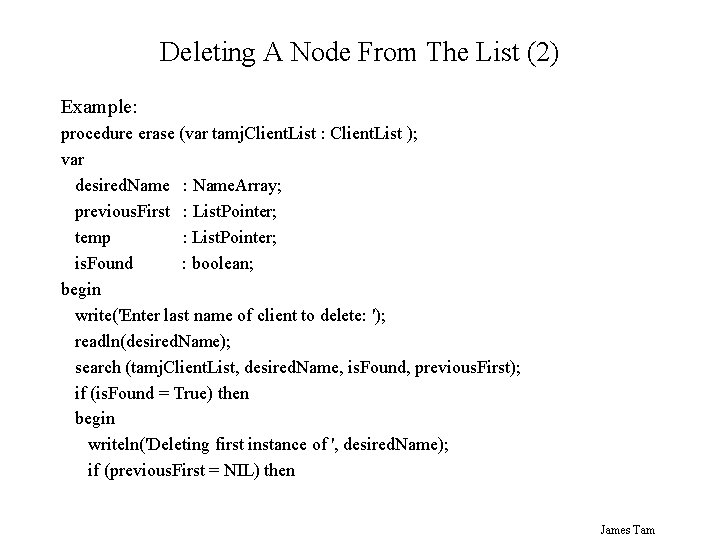
Deleting A Node From The List (2) Example: procedure erase (var tamj. Client. List : Client. List ); var desired. Name : Name. Array; previous. First : List. Pointer; temp : List. Pointer; is. Found : boolean; begin write('Enter last name of client to delete: '); readln(desired. Name); search (tamj. Client. List, desired. Name, is. Found, previous. First); if (is. Found = True) then begin writeln('Deleting first instance of ', desired. Name); if (previous. First = NIL) then James Tam
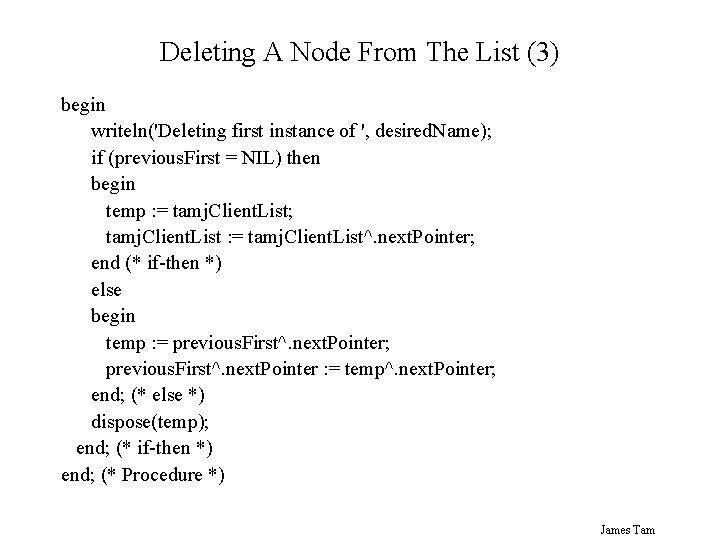
Deleting A Node From The List (3) begin writeln('Deleting first instance of ', desired. Name); if (previous. First = NIL) then begin temp : = tamj. Client. List; tamj. Client. List : = tamj. Client. List^. next. Pointer; end (* if-then *) else begin temp : = previous. First^. next. Pointer; previous. First^. next. Pointer : = temp^. next. Pointer; end; (* else *) dispose(temp); end; (* if-then *) end; (* Procedure *) James Tam
Cse pif
Cse 4102
Cse 4102
Cs 4102 uva
Ns4102
Pastor steven khoury
Careerready101
Titleist slides
Steven mair westminster
Carol lynn benson
Satisfaction
Steven levy wired
Steven benini
Unland irreversible witness
Steven johnson menular atau tidak
Dr steven kahn
Steven kyle cornell
Steven walchek
Steven boyce md
Personal na layunin ng misyon sa buhay
Resectoscopie
Quem fundou a apple
Steven hustinx
Exurbanisation definition
Steven briggs ucsd
Harry williams lmhc
Steven spewak
Steven unikewicz
Steven unikewicz
Elixir roadmap
Steven pinker spracherwerbstheorie
Steven berkoff fact file
Ethel porras uf
Steven low caltech
Steven stanko
Asic
Steven goldin md
Ritchie valens joseph steven valenzuela
Woodlane surgery ruislip
Lonnie trumbull
Steven tamm
Steven berkowitz md
Richard solit
Steven cunnane
Steven tam md
Seacoast water utility
Dr steven goldin
Dr steven kahn
Steven rudick md
Steven fang
Steven paul jobs biography
Bryan abramowitz md
Steven wallace ucla
Steven pemberton css
Steven fang
Steven johnson everything bad is good for you
Steven reiss motivation
Jason dearden
Dr steven goldin
Dr steven kahn
Customer service dialogue
Maktteorier
Dr steven williams
Steven warren md
Aggrevated assult
Steven anlage
Steven 90 day fiancé autistic
Horizon house steven holl
Steven smolders
Paul steven conroy
Steven kelk
Steven pollock physics
Dr gerhardt dallas
Supa forensics
Steven conolly
Steven neftel
Touring mobilis verkeersinfo
Steven miles md
Steven proto
Steven butts
Steven pariente
Apa wire
Dr steven lucas
Nikiforos nikiforou
Steven younan
Steven lade
Steven rochford
Steve jobs dislessia
Steven vidaurri kansas city
Dr steven bloomfield
Steven teske
Steven lim arbitrator
Steven osman
Choice and partnership approach website
Citazionismo postmoderno
Aeren lpo
Streetcar named desire character analysis
Steven de costa
Steven garfin md
Steve snow riot games
Relatos de monstruos steven zorn
Pinsp definition
Steven penney
Steven irving
Dr steven miles
Steven lundberg
Steven hulshoff tu delft
Steven fitzpatrick
Steven frank valadez
Dr steven huang
A circular fountain with a 5-m radius
Steven klooster
Rit mechanical engineering curriculum
Steven hotze
Steven janke
In his course steven roberts uses original films with
Steven lukes magt
Steven rumbuc
Gapdmp
Steven colgan
Steven bolling md
Steven e brown
Dr joshua snodgrass
Steven whang
Steven jacoby
Los 4 pasos básicos de la salsa
Steven lassiter
Steven rudich
Steven teoh
Steven blyth
Dr steven harris lyme
Steven douglas slides
Steven blumgart
Colonel by ib program
W. steven barnett
Asian hacks
Steven sopko
Define components of computer
What is computer organization
Monitor printer speaker and projector are blank devices
Structure of computers
Computer architecture and computer organization difference
Basic computer organization and design
Register in computer organization
Wg to pascal
Blaise pascal university
Pascal hammel paul brousse
Pengertian bahasa pemrograman pascal
Principio di pascal