DATA STRUCTURE TRIE What Is TRIE Trie Is
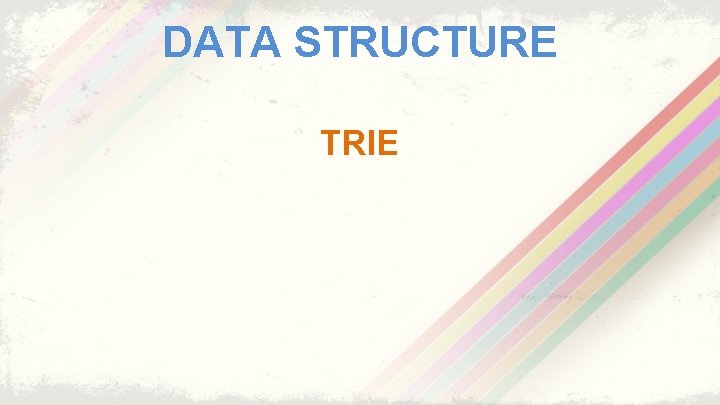
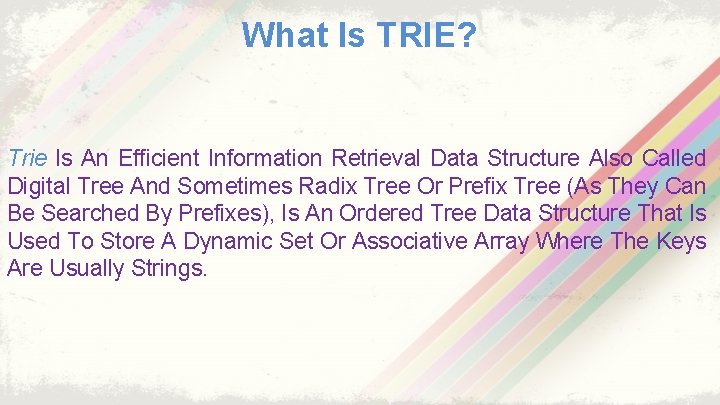
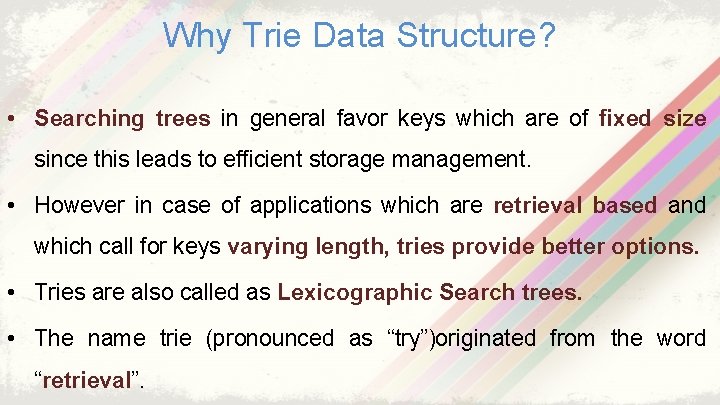
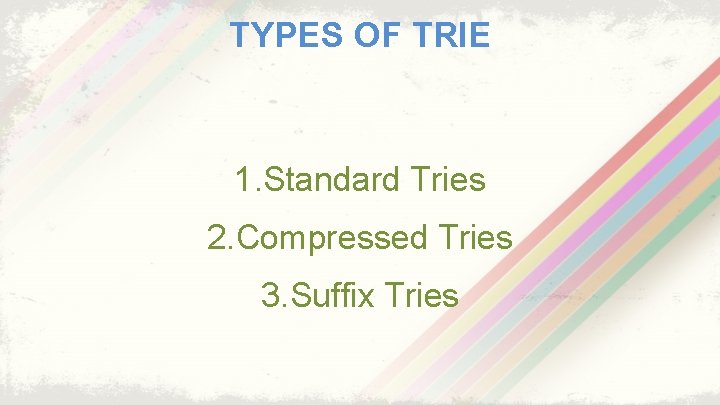
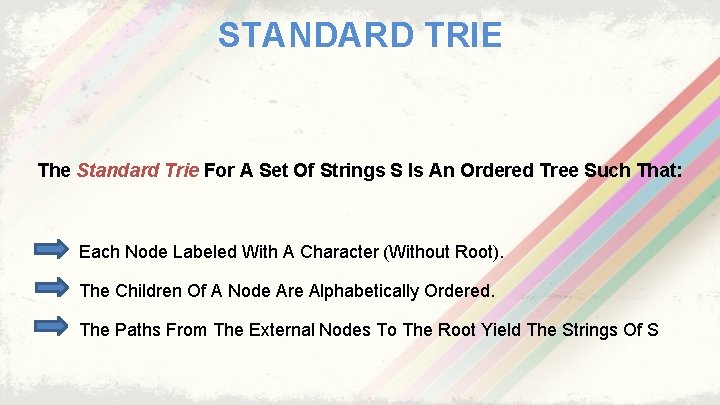
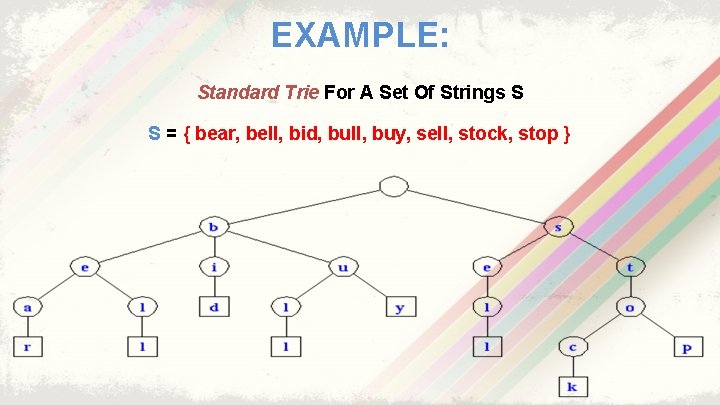
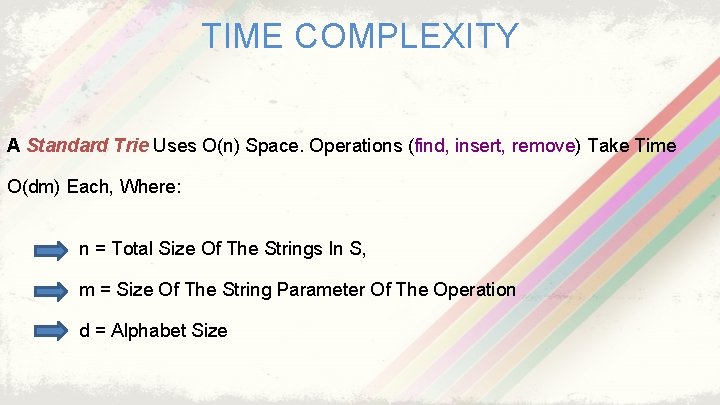
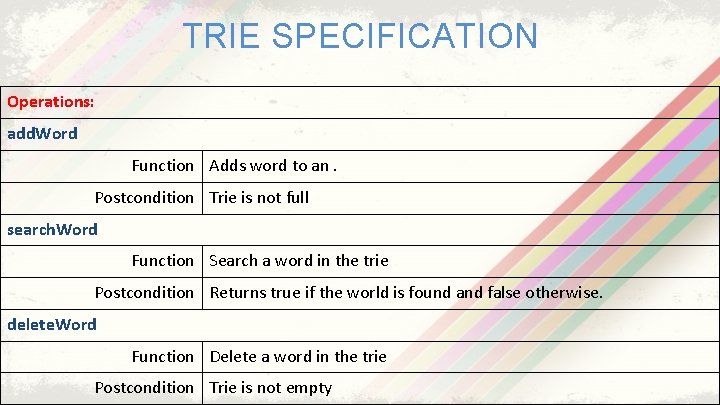
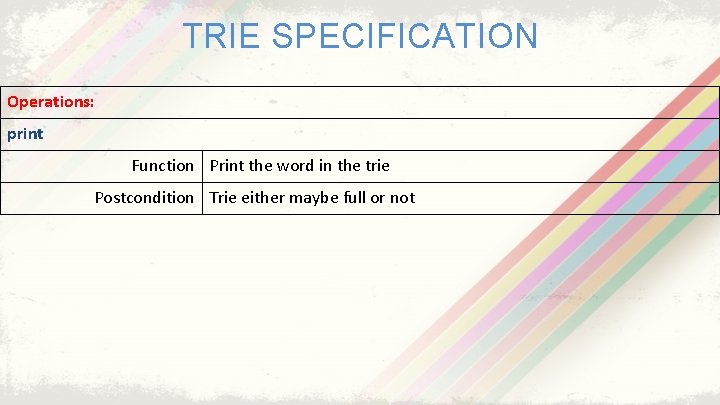
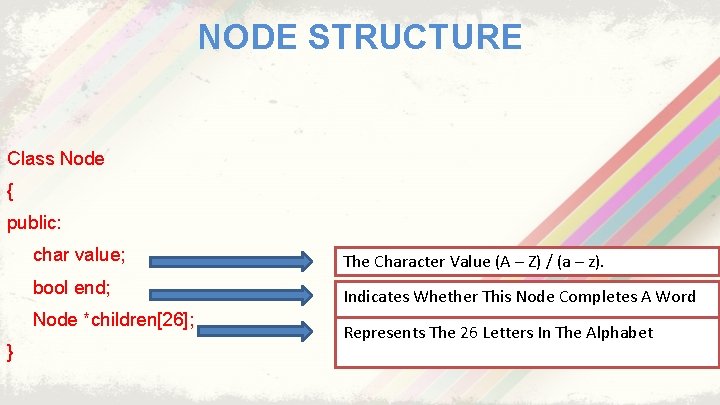
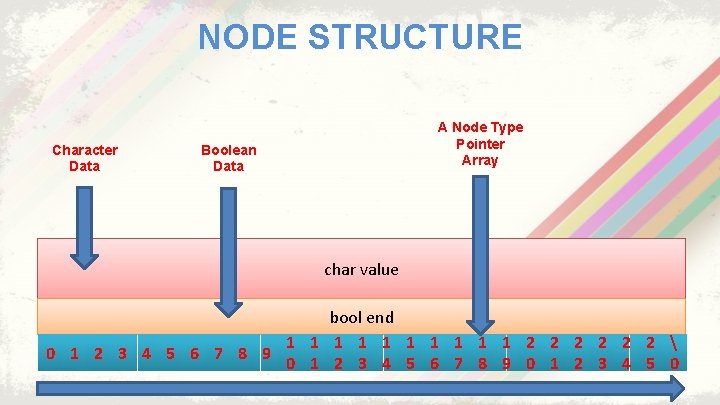
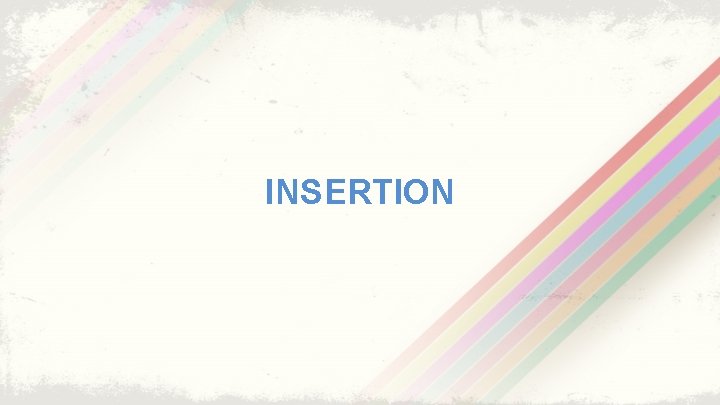
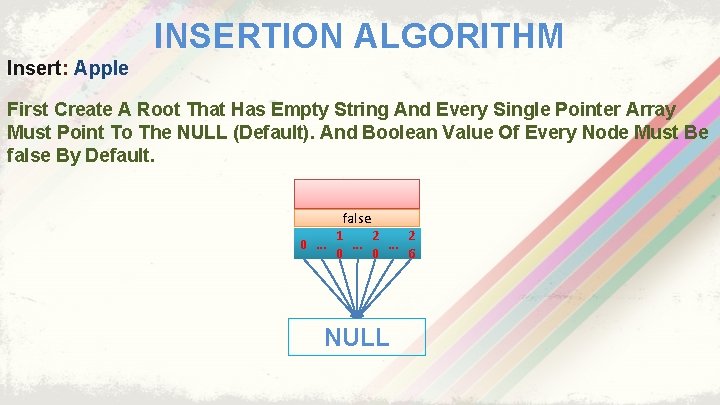
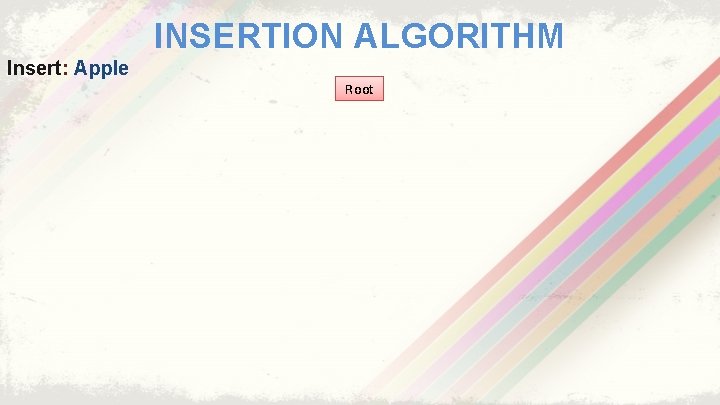
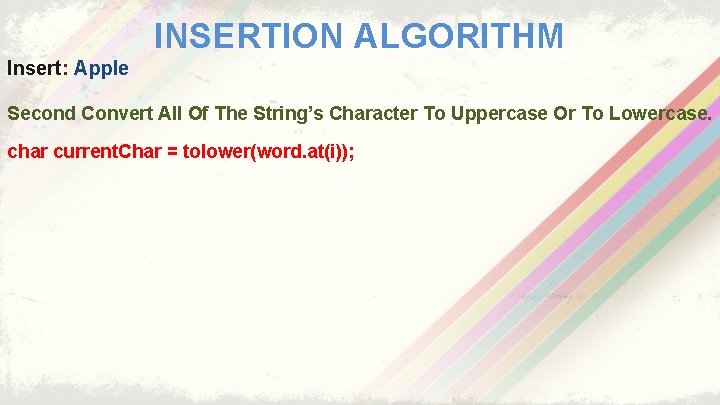
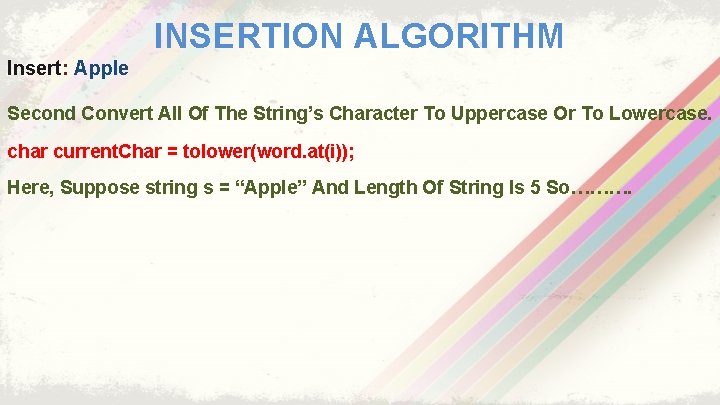
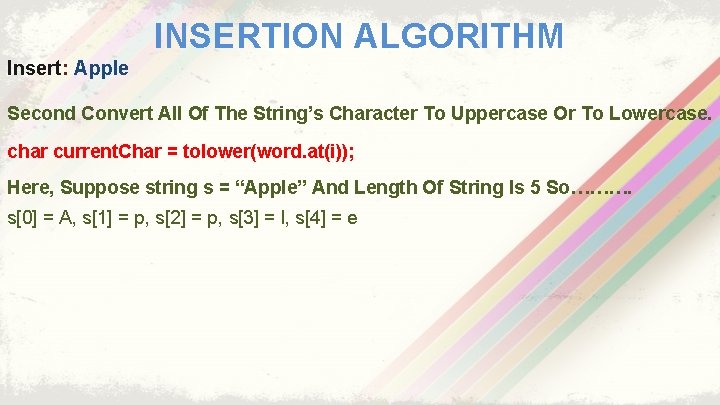
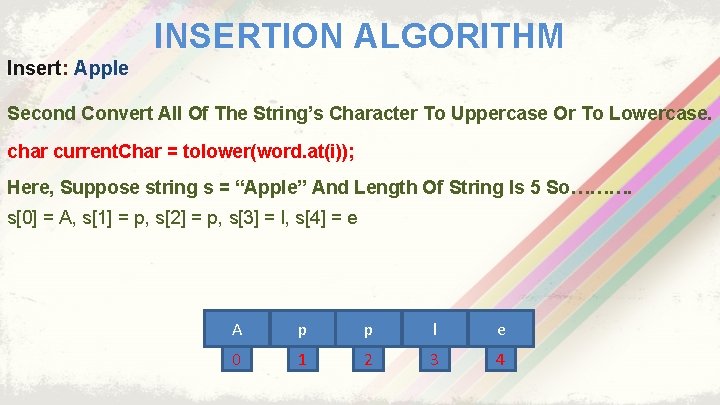
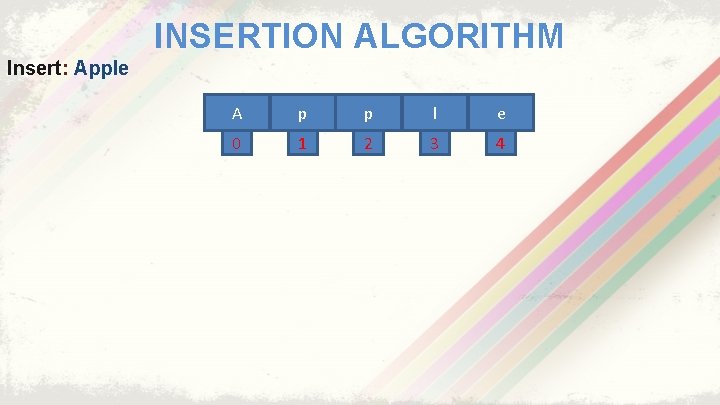
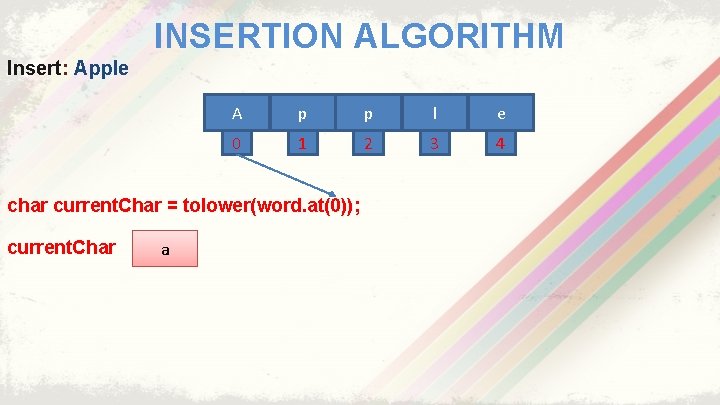
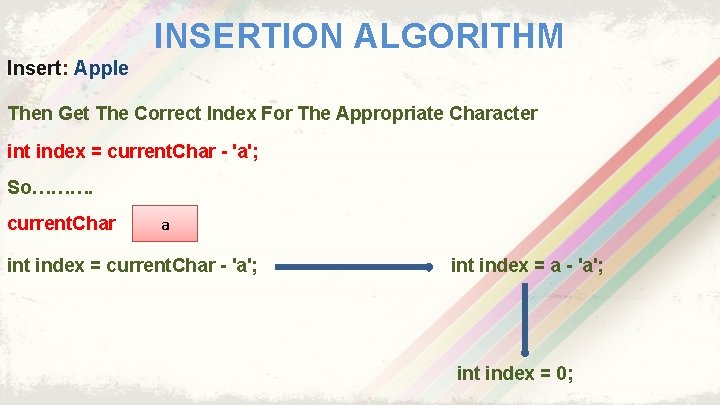
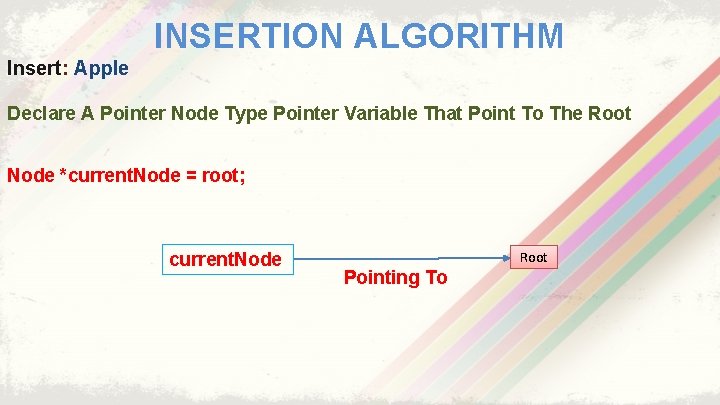
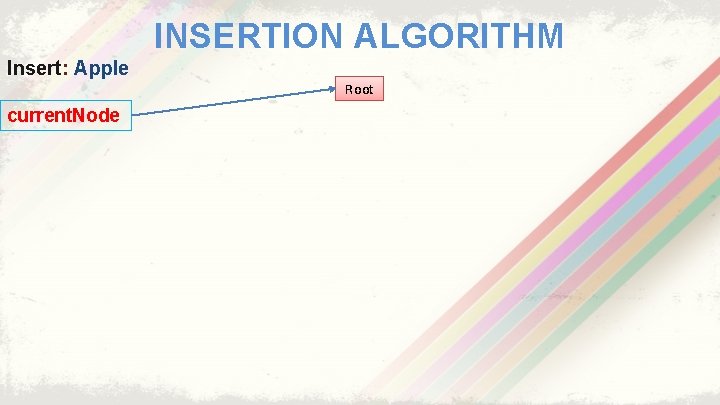
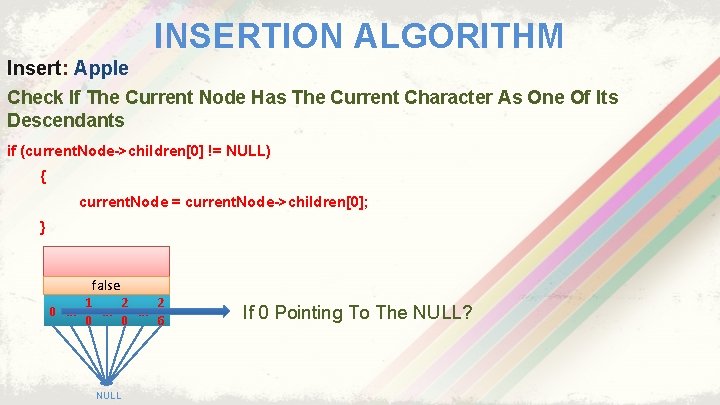
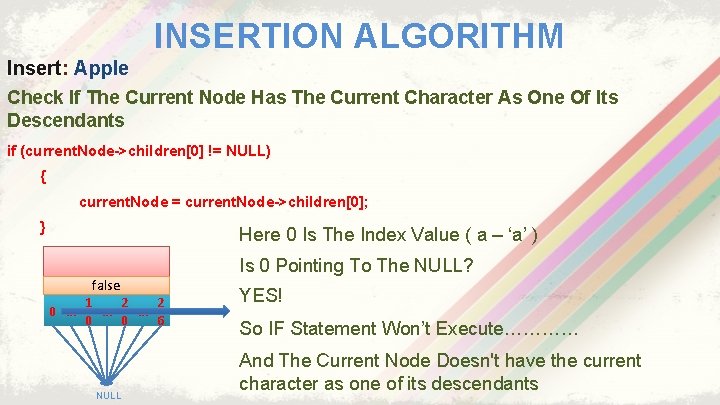
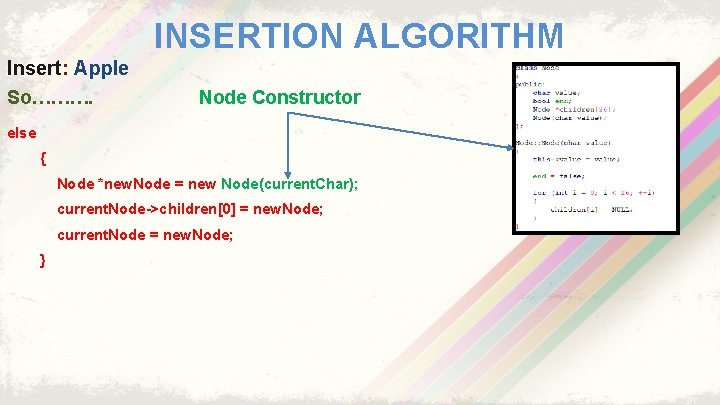
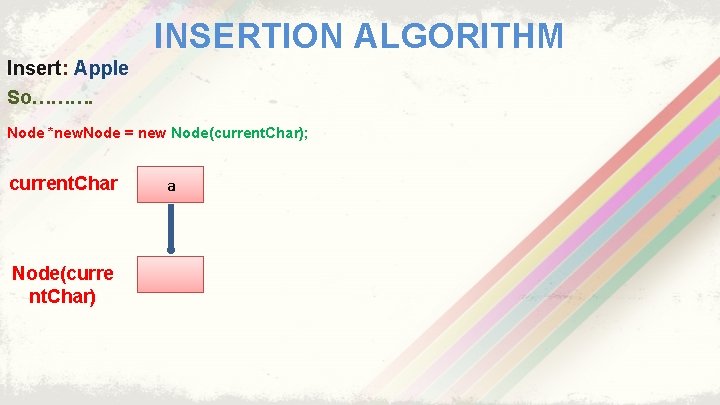
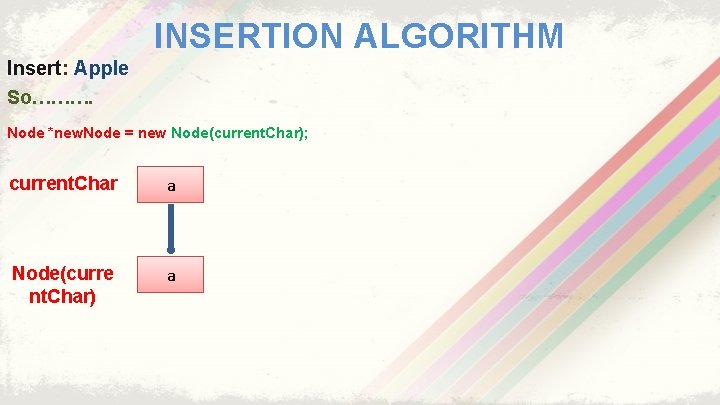
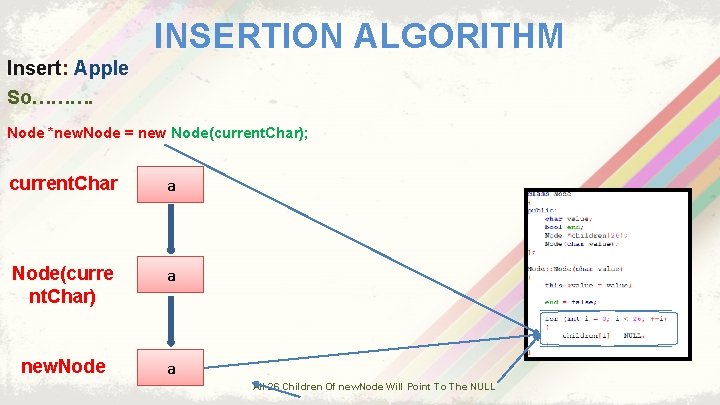
![INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node a current. INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node a current.](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-30.jpg)
![INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node current. Node INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node current. Node](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-31.jpg)
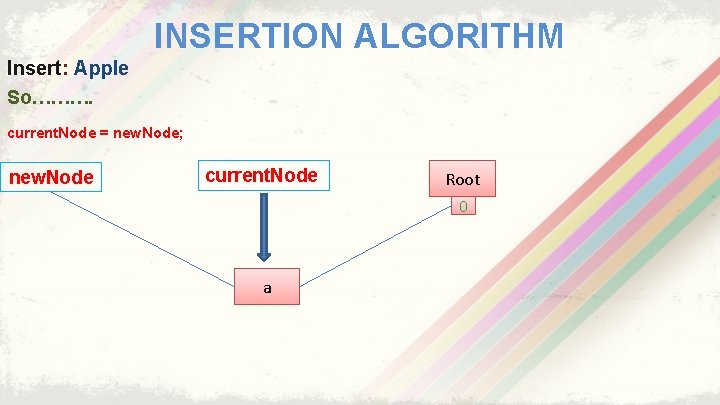
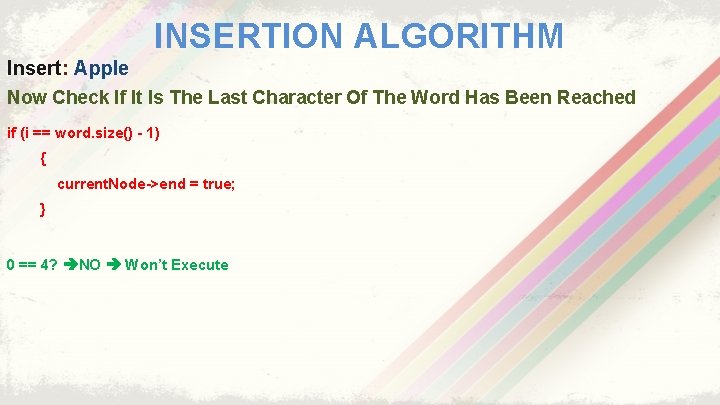
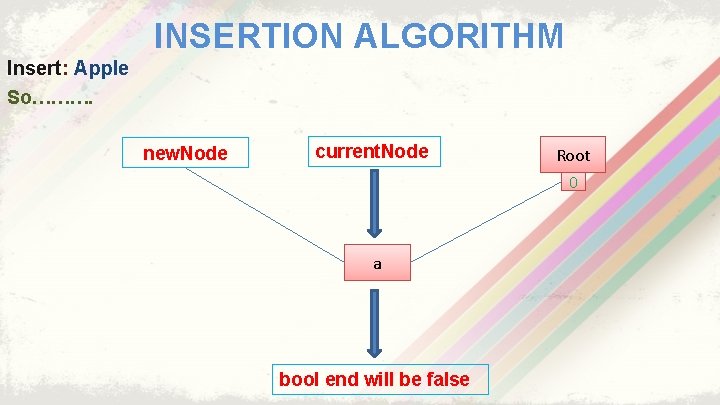
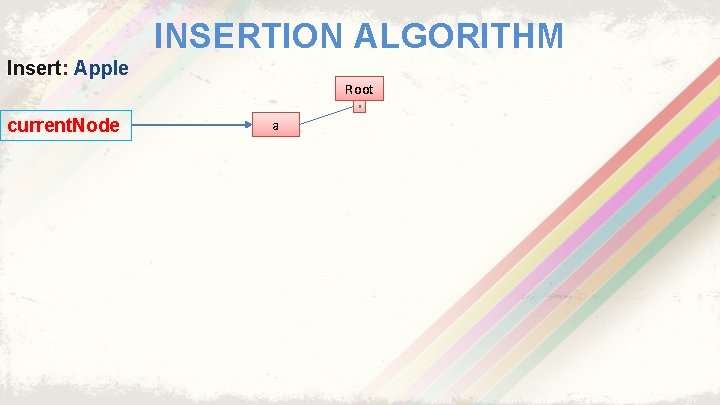
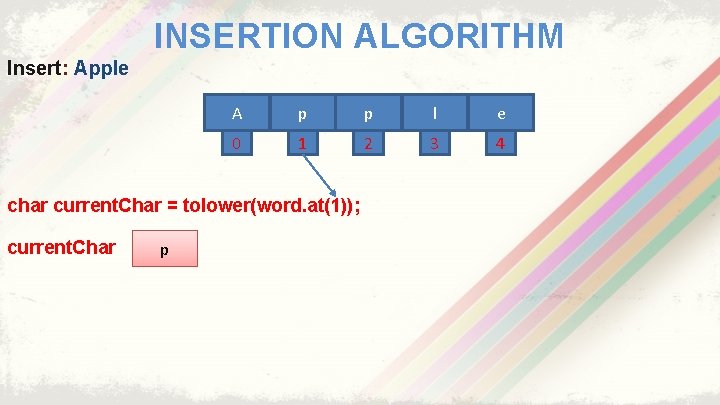
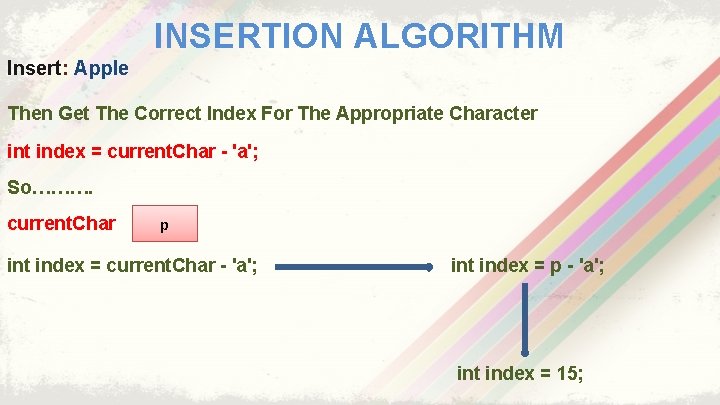
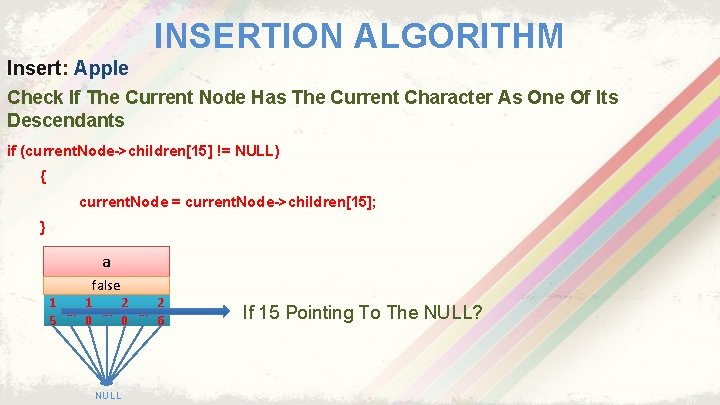
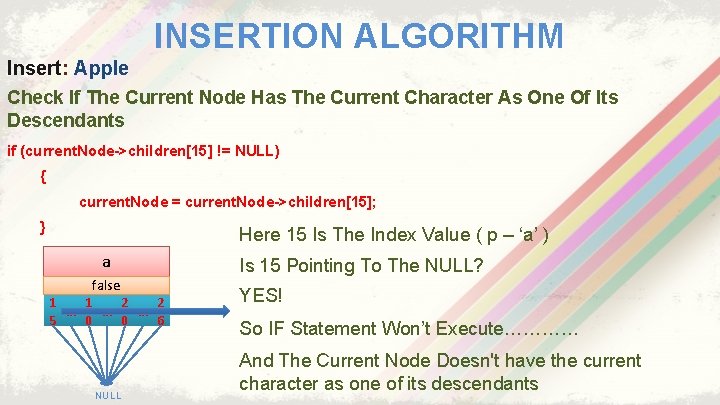
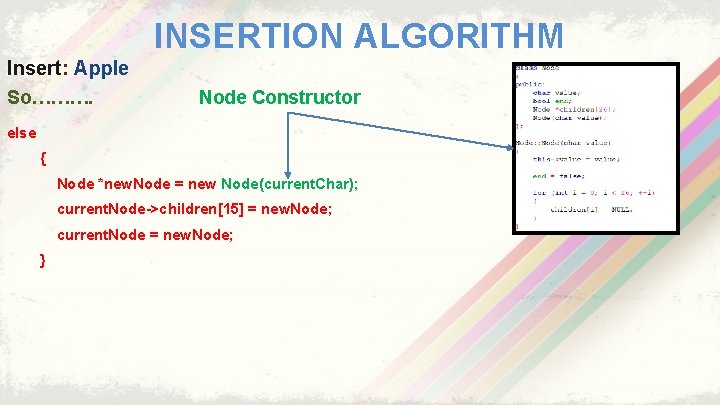
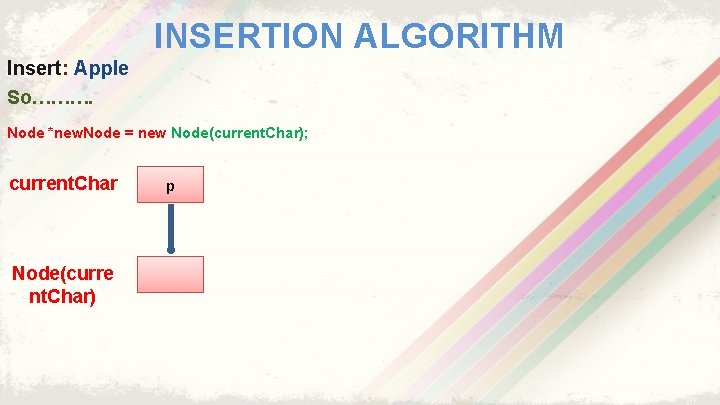
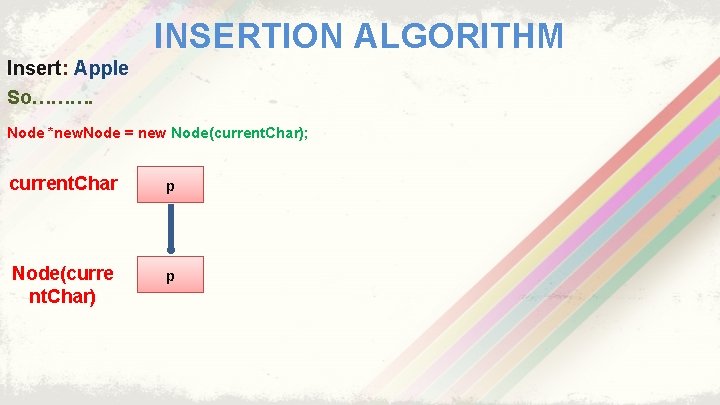
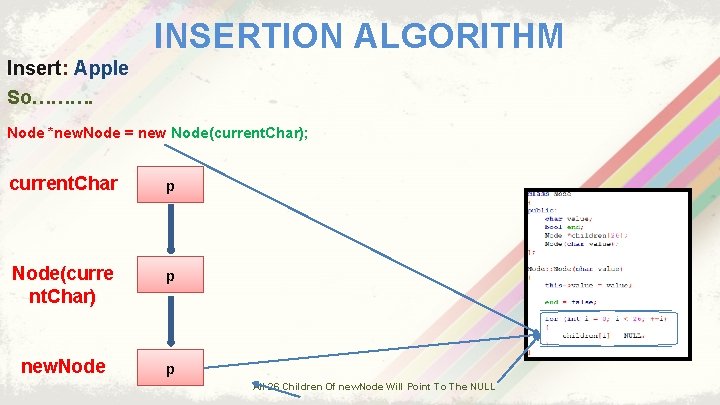
![INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node p current. INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node p current.](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-44.jpg)
![INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node current. Node INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node current. Node](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-45.jpg)
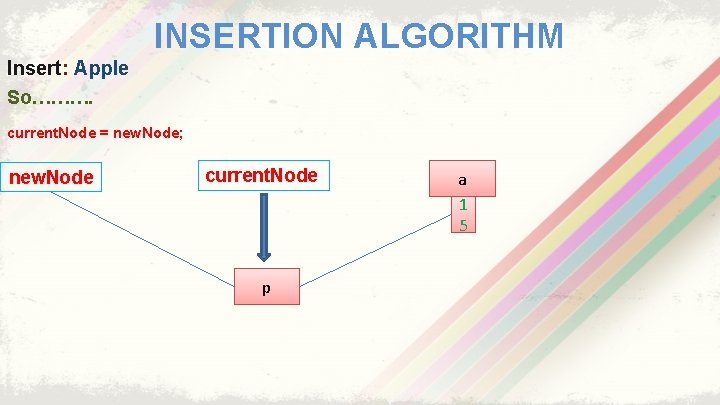
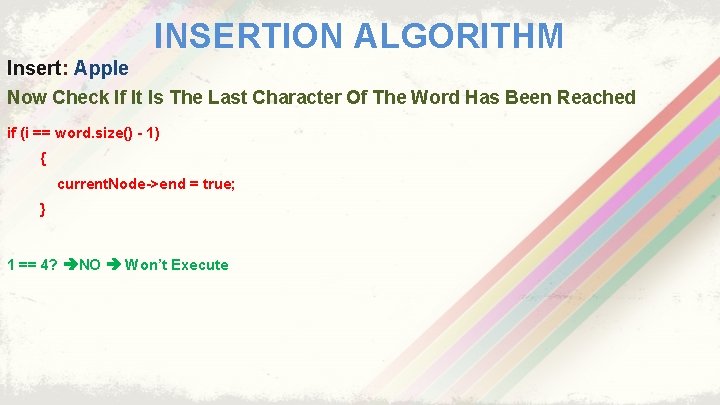
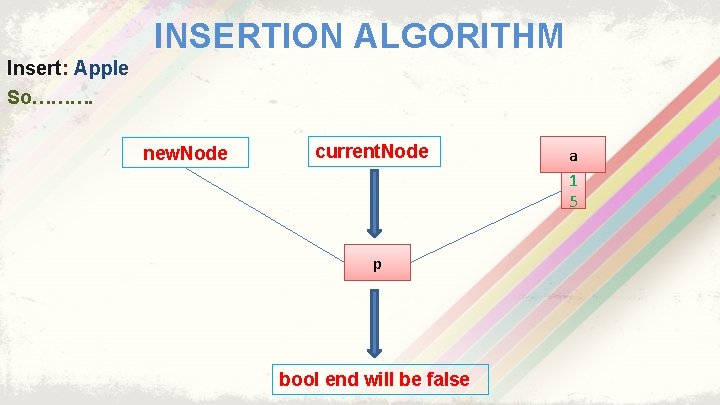
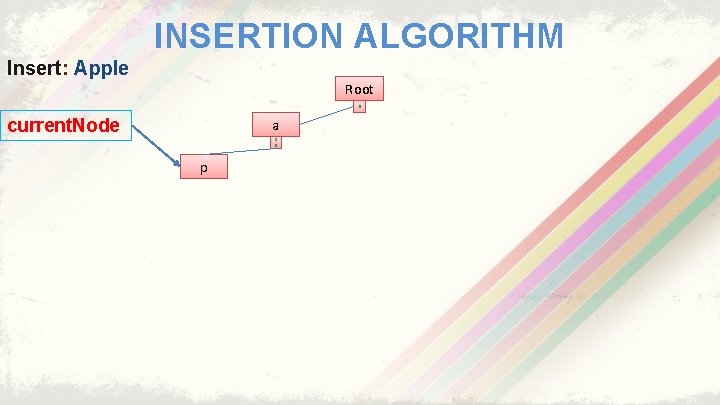
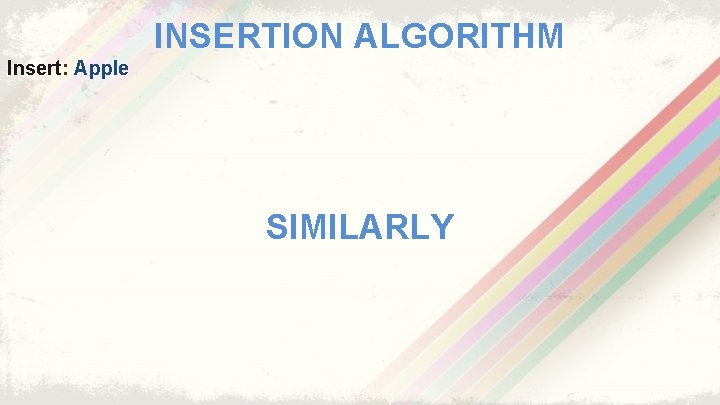
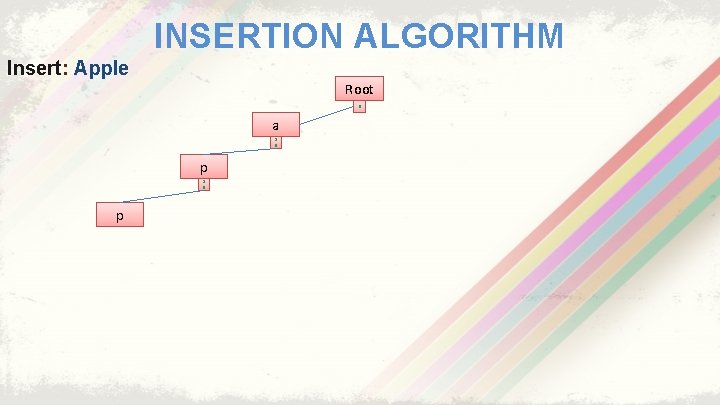
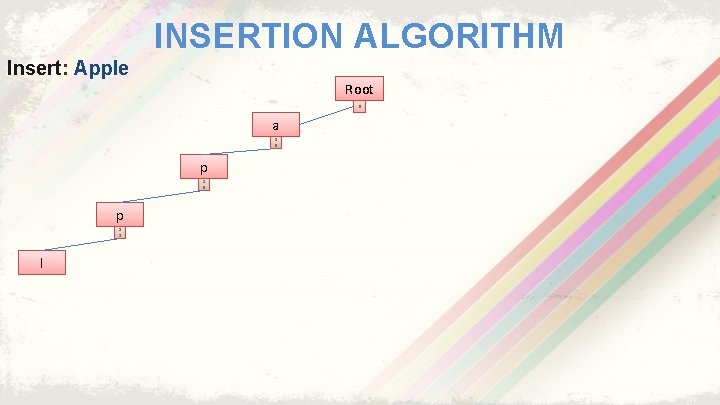
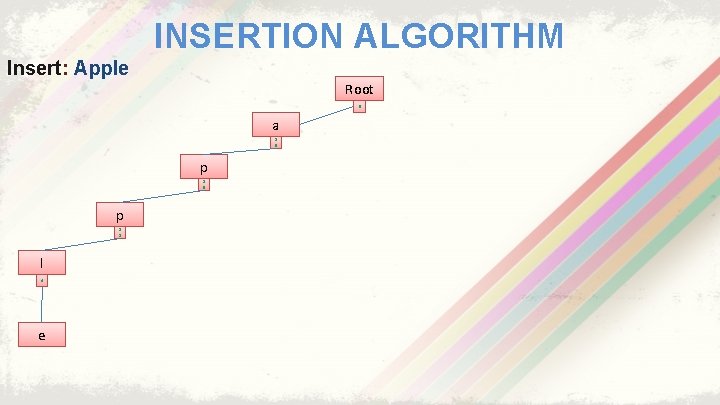
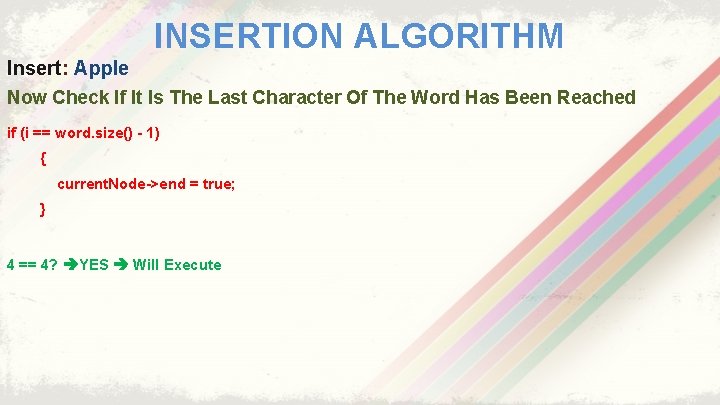
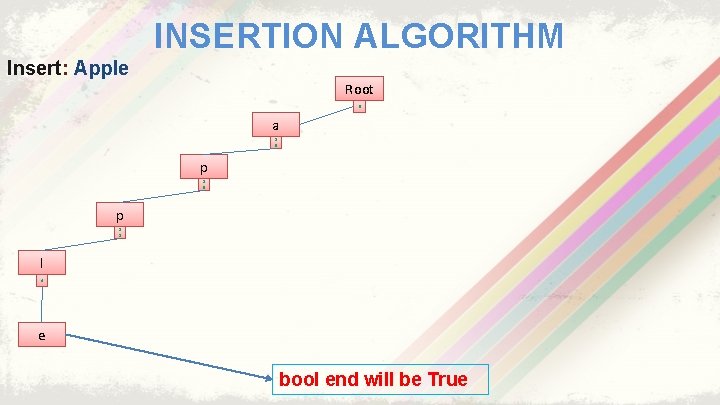
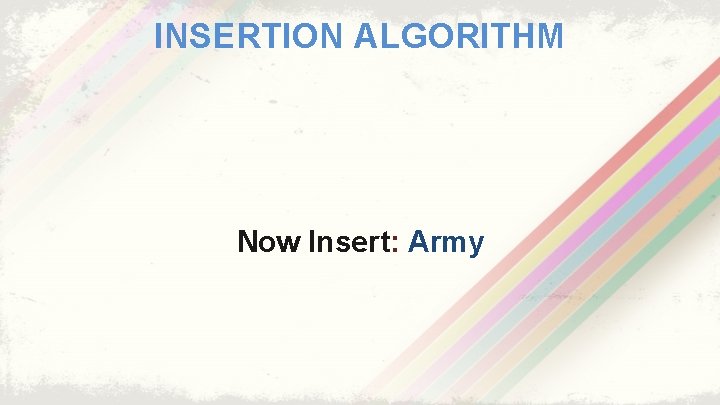
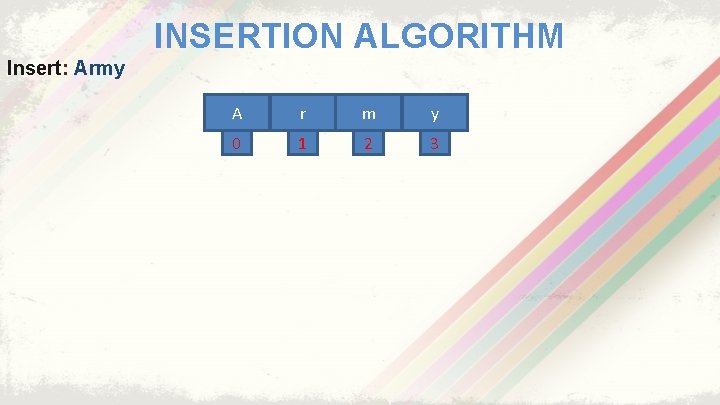
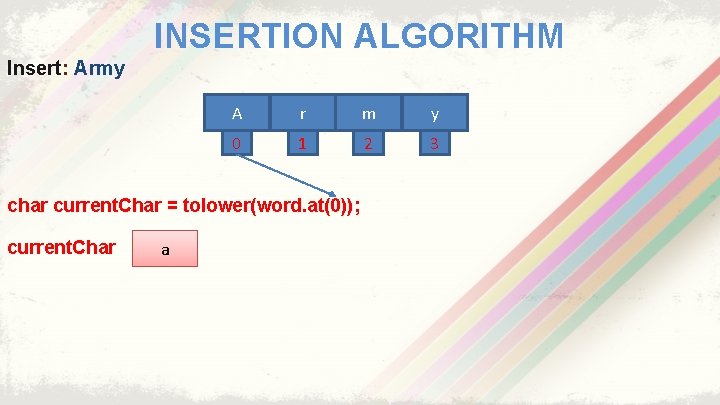
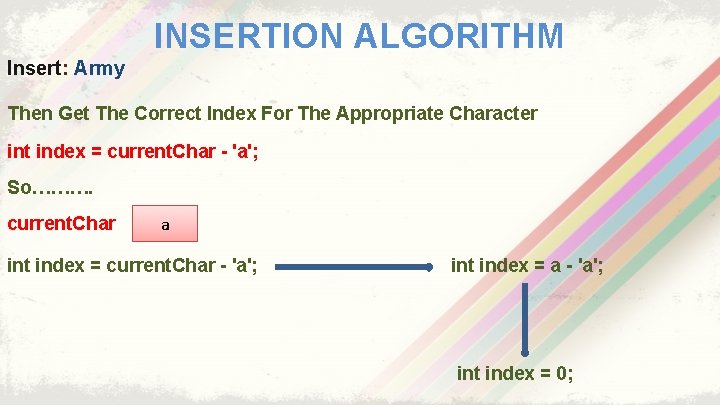
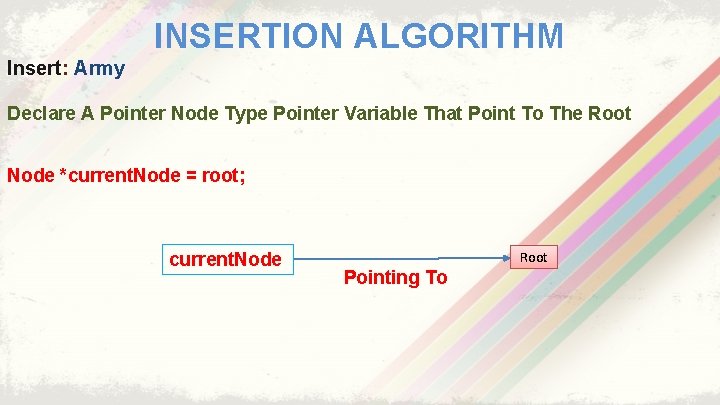
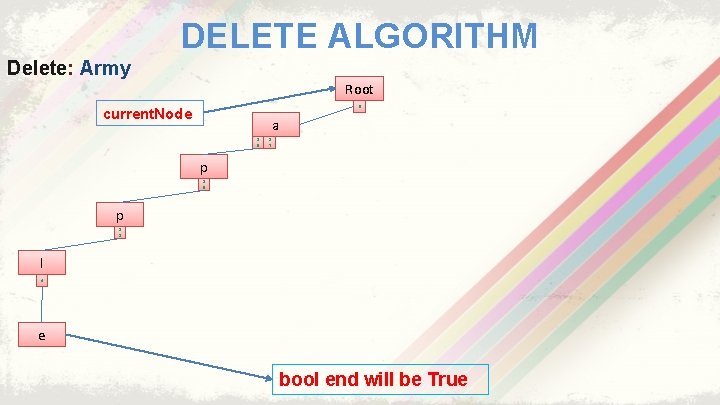
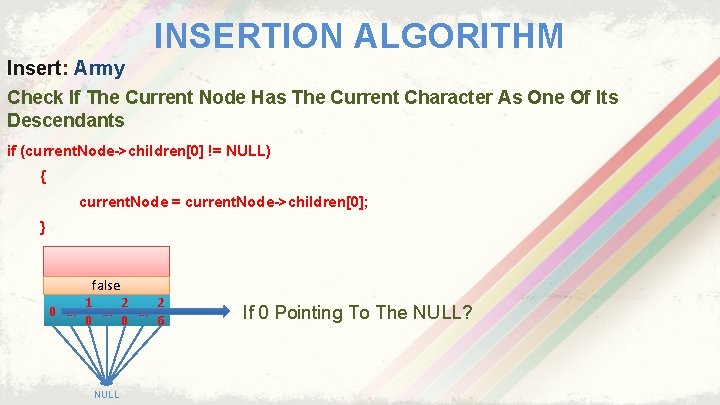
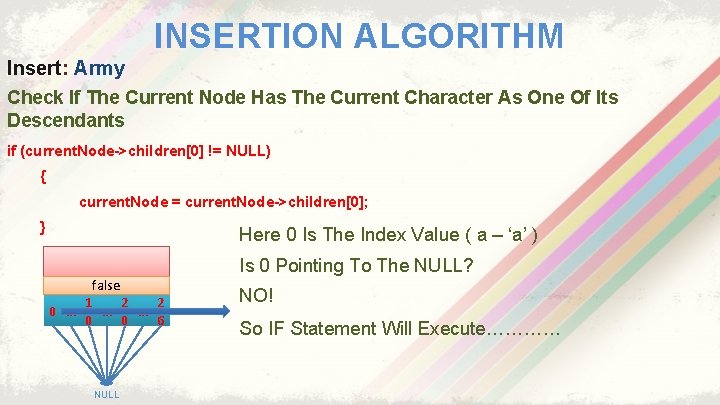
![INSERTION ALGORITHM Insert: Army So………. current. Node = current. Node->children[0]; INSERTION ALGORITHM Insert: Army So………. current. Node = current. Node->children[0];](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-64.jpg)
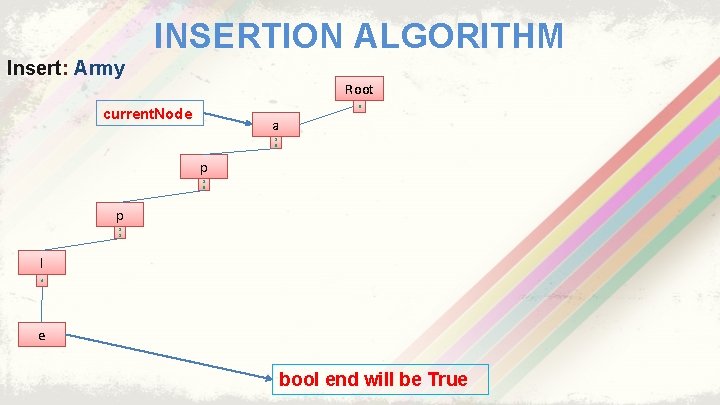
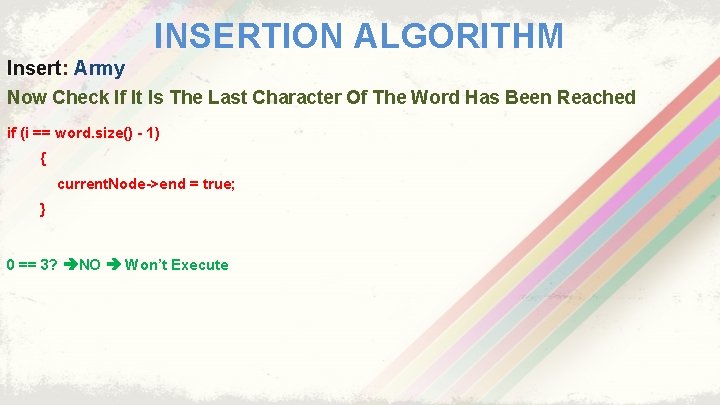
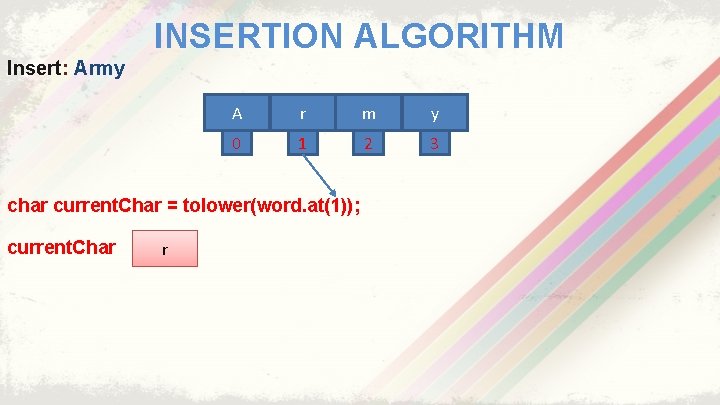
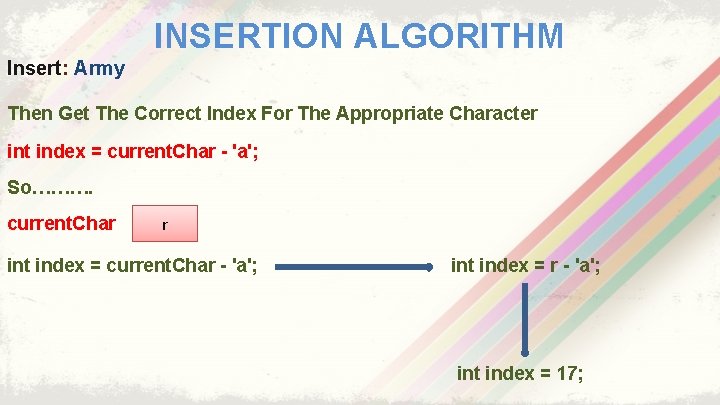
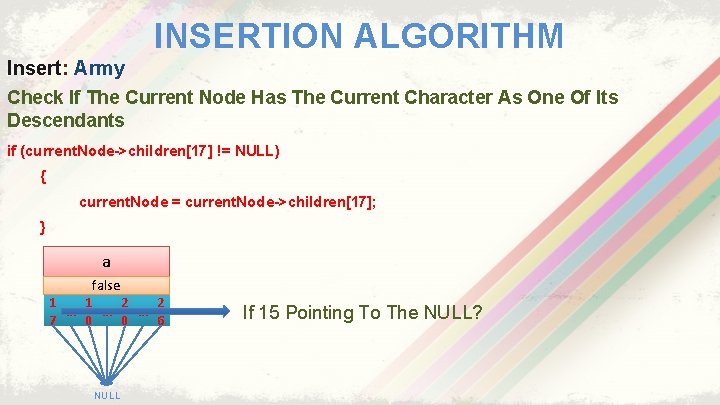
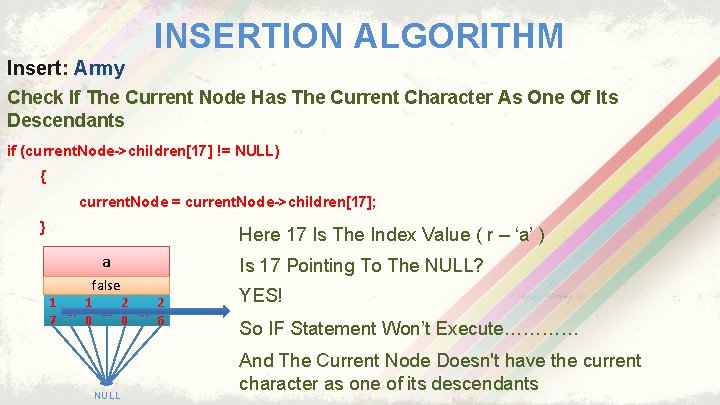
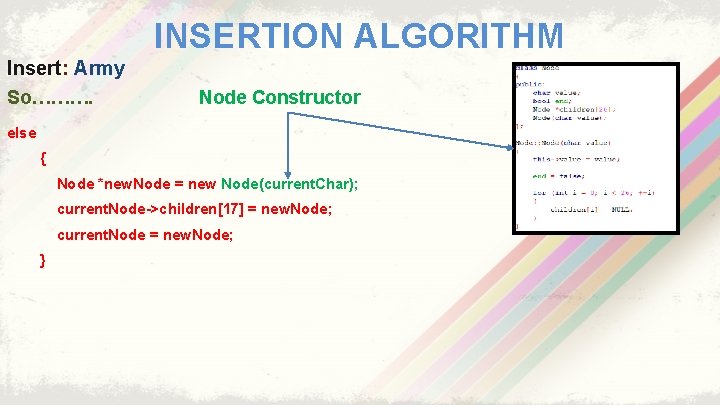
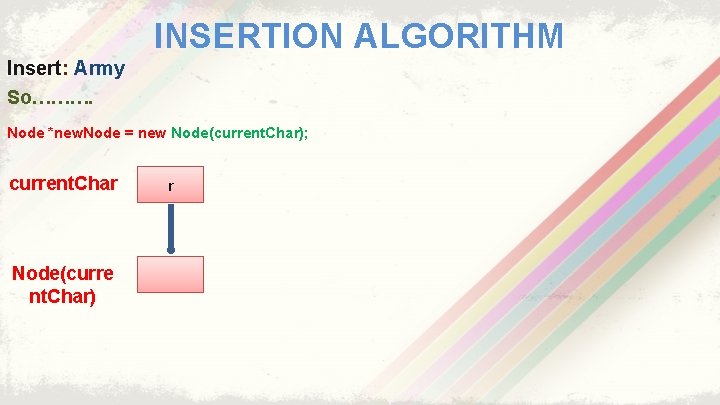
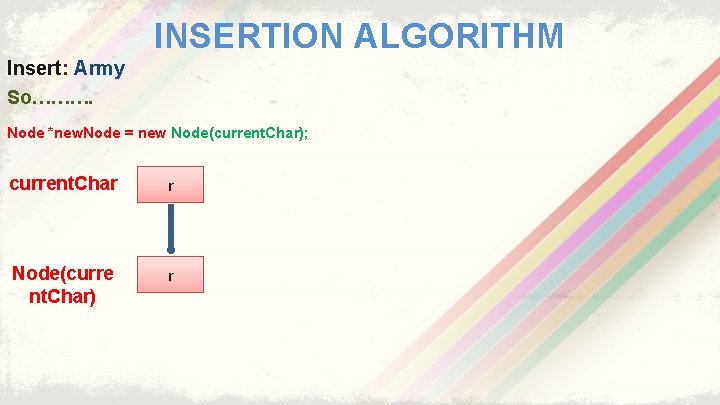
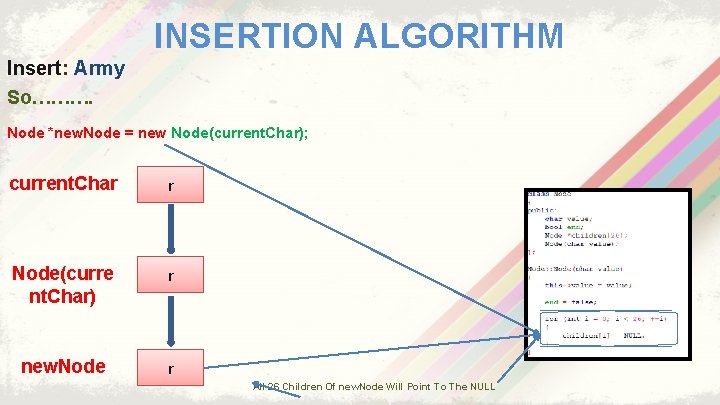
![INSERTION ALGORITHM Insert: Army So………. current. Node->children[17] = new. Node; INSERTION ALGORITHM Insert: Army So………. current. Node->children[17] = new. Node;](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-75.jpg)
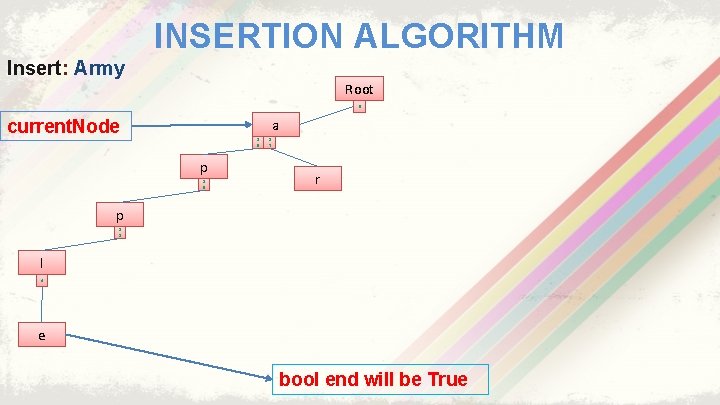
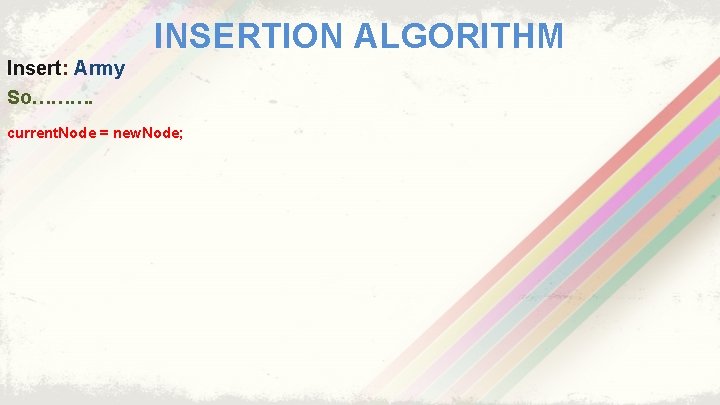
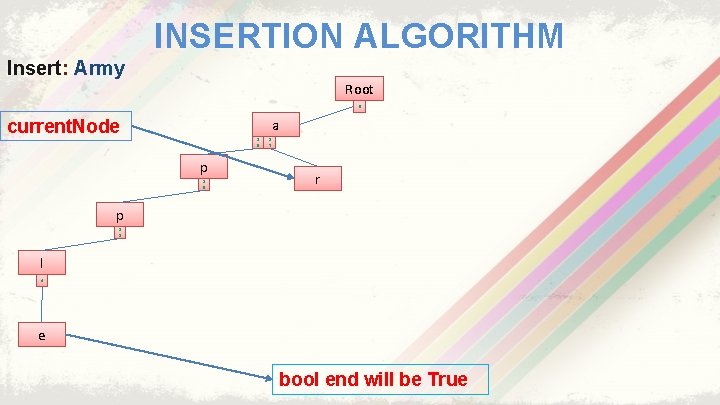
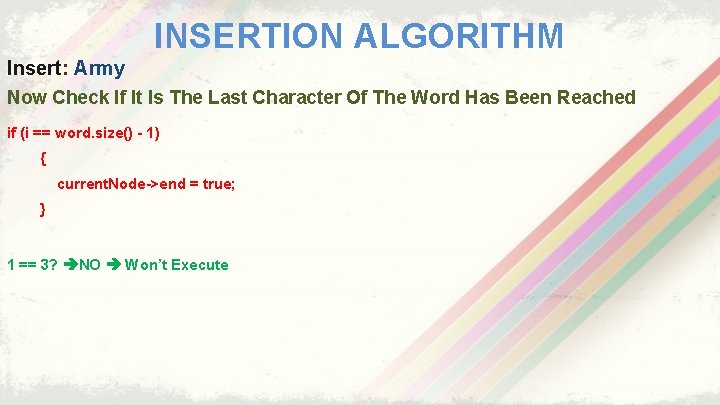
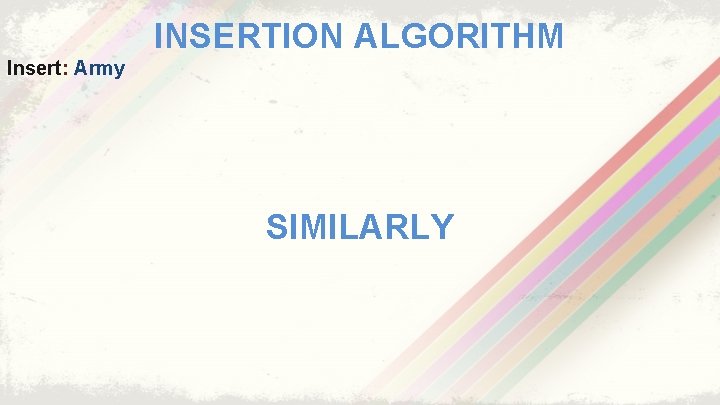
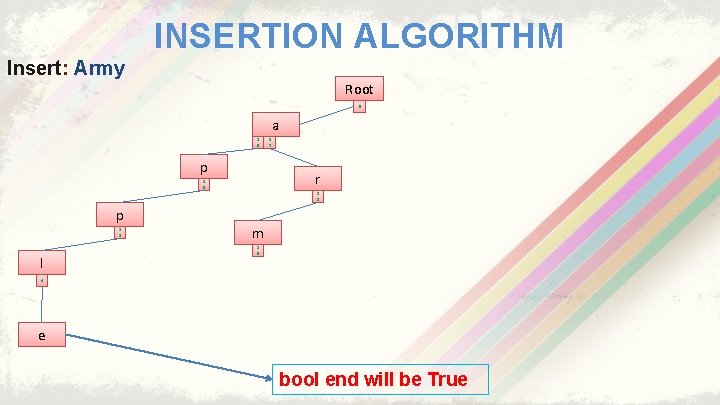
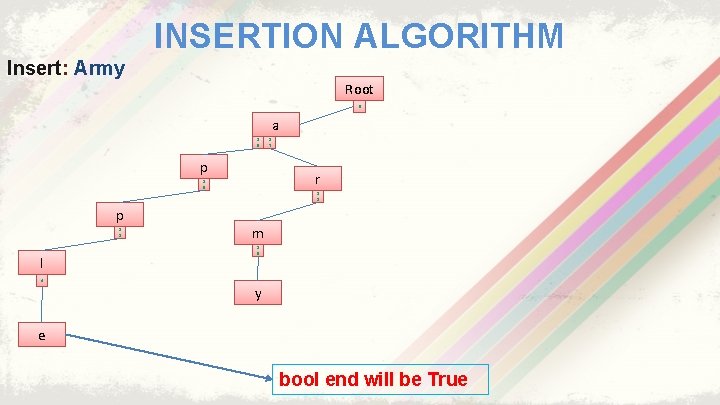
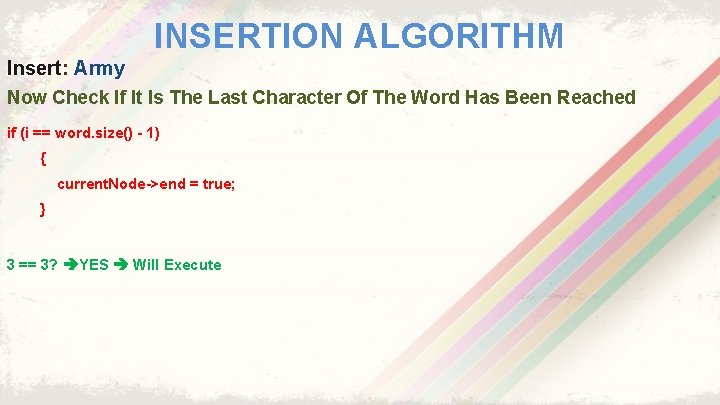
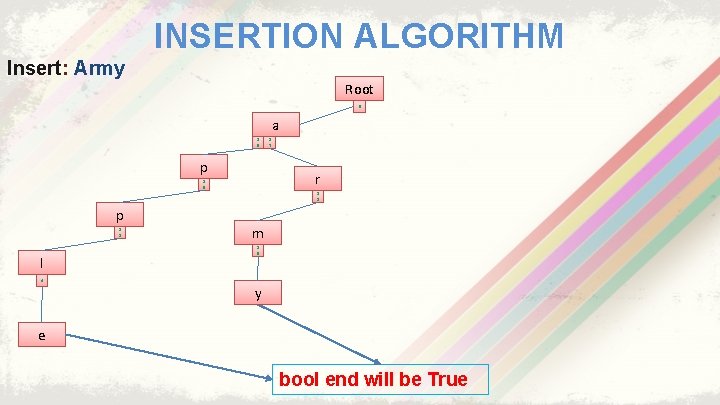
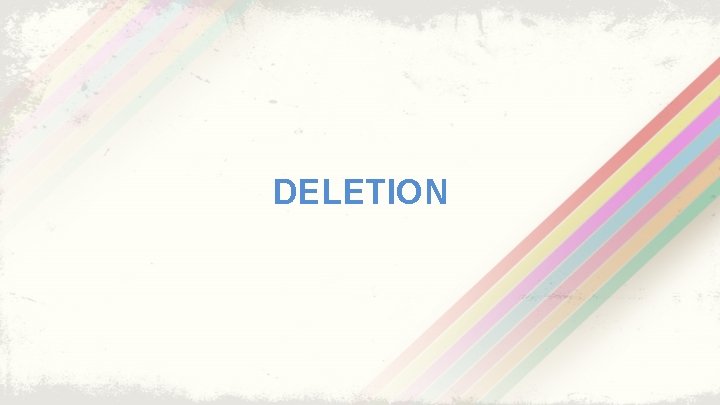
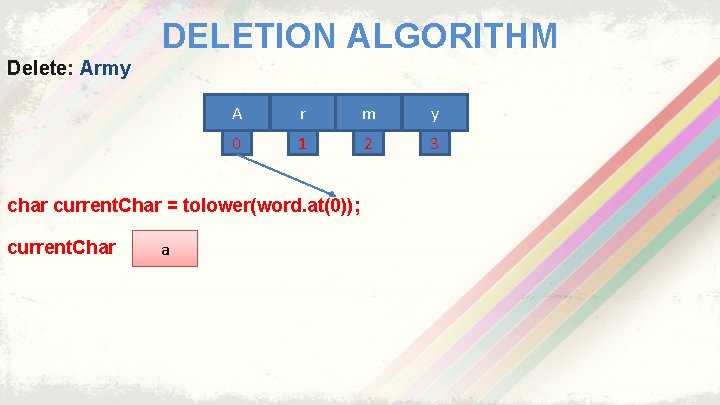
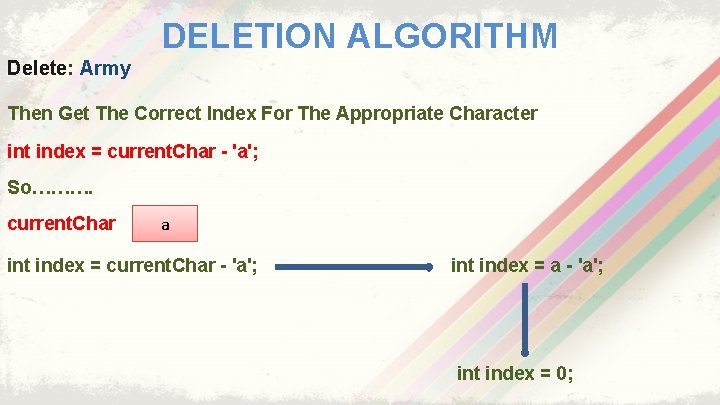
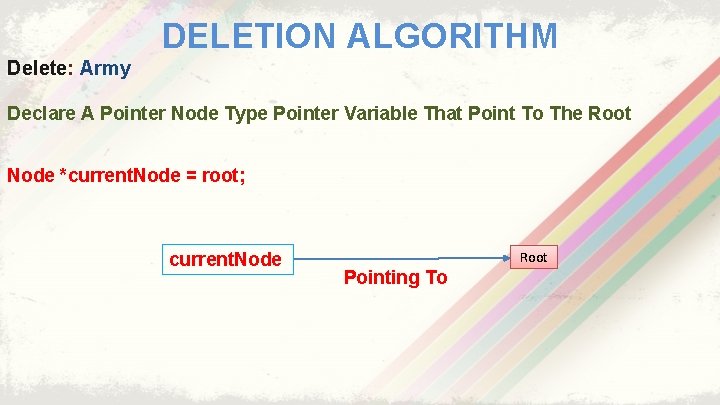
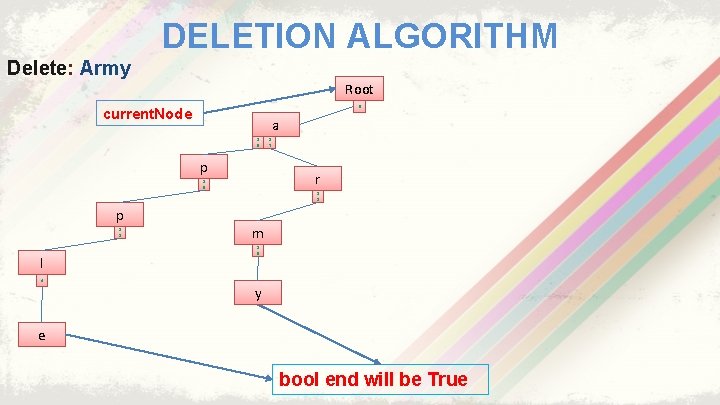
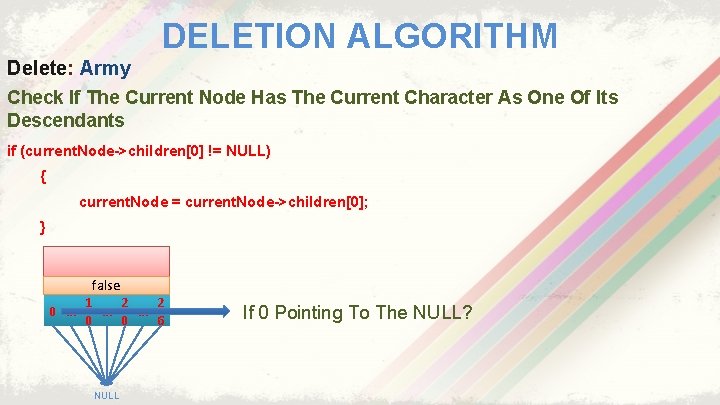
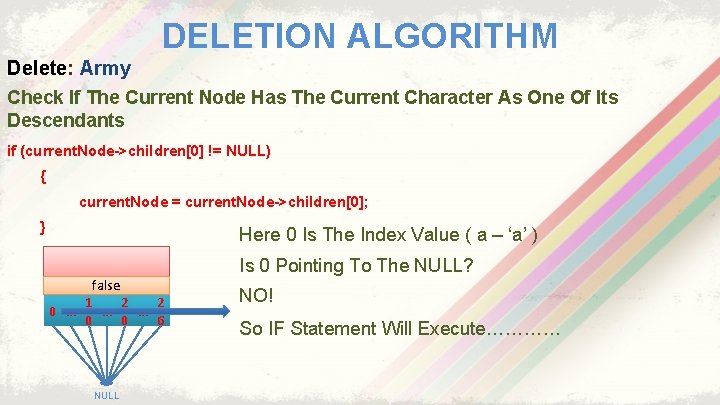
![DELETION ALGORITHM Delete: Army So………. current. Node = current. Node->children[0]; DELETION ALGORITHM Delete: Army So………. current. Node = current. Node->children[0];](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-92.jpg)
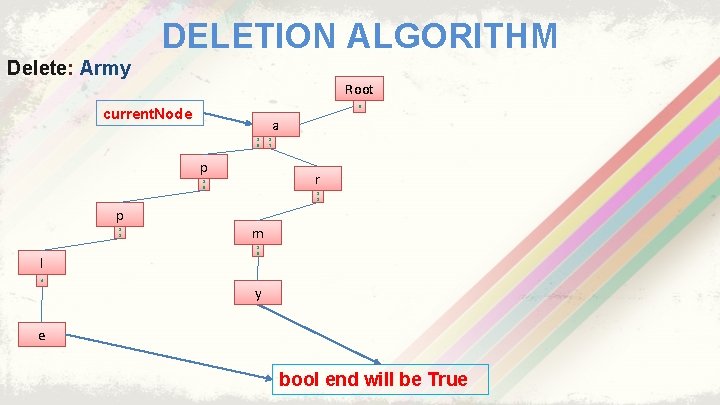
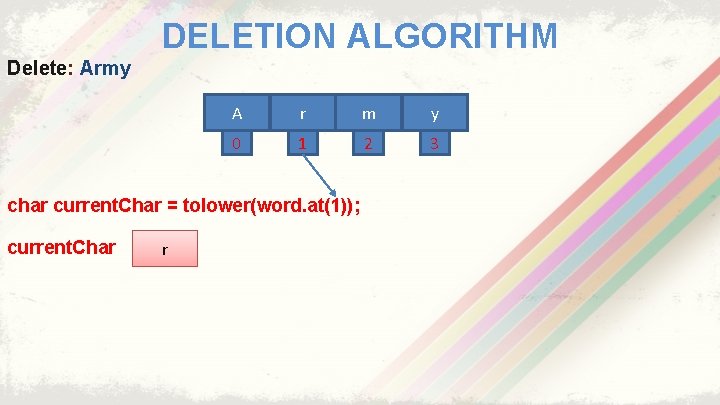
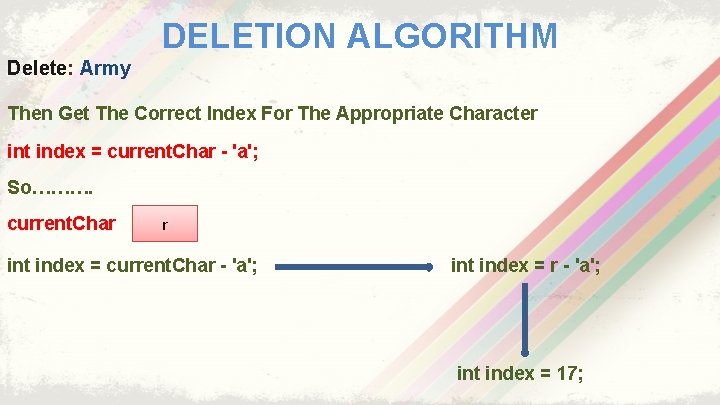
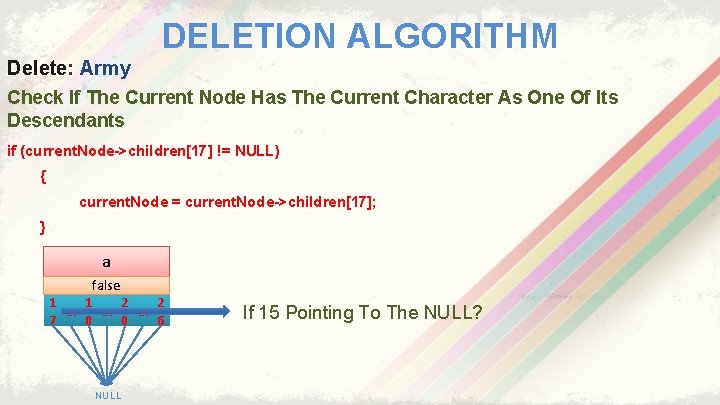
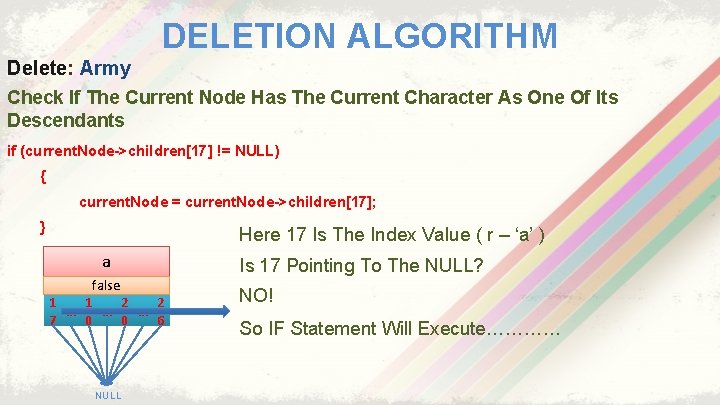
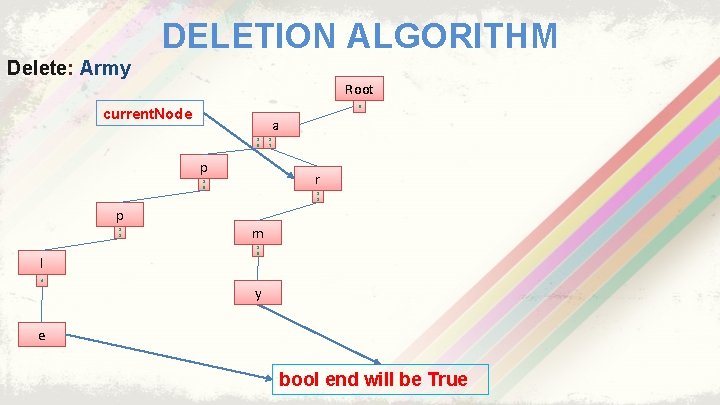
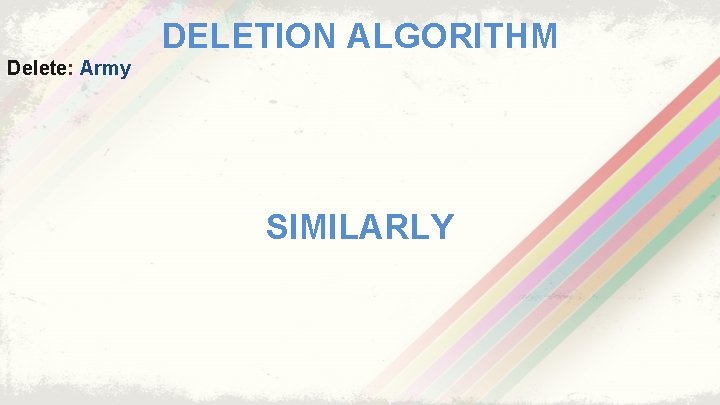
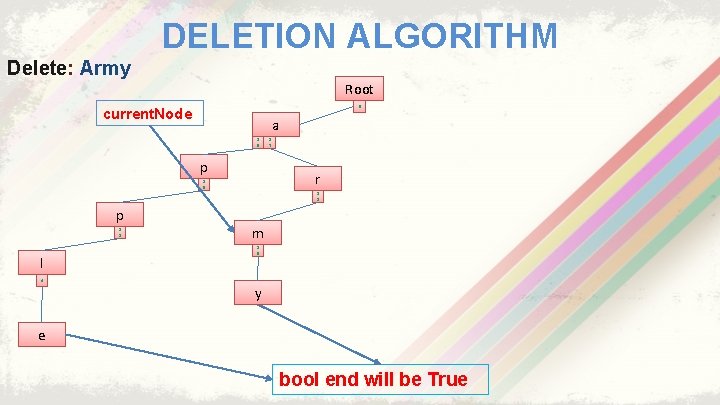
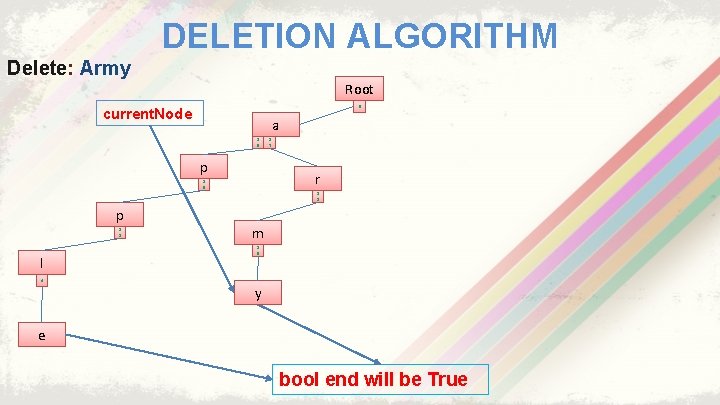
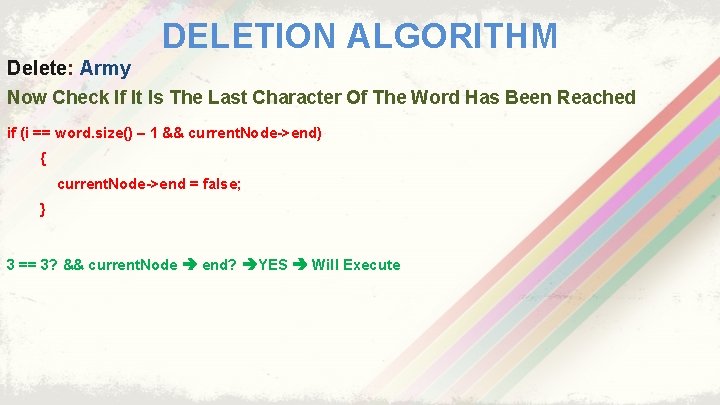
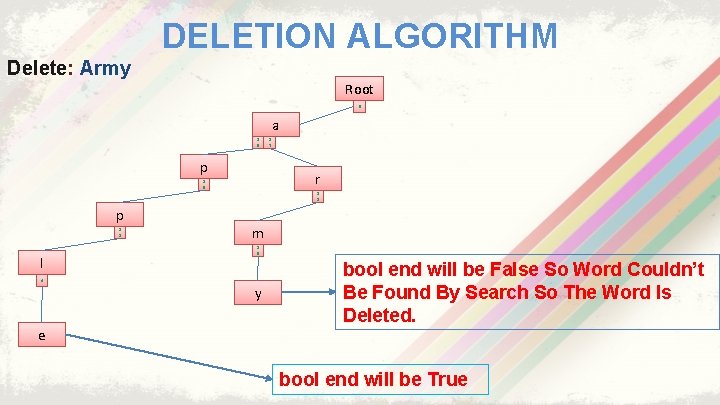
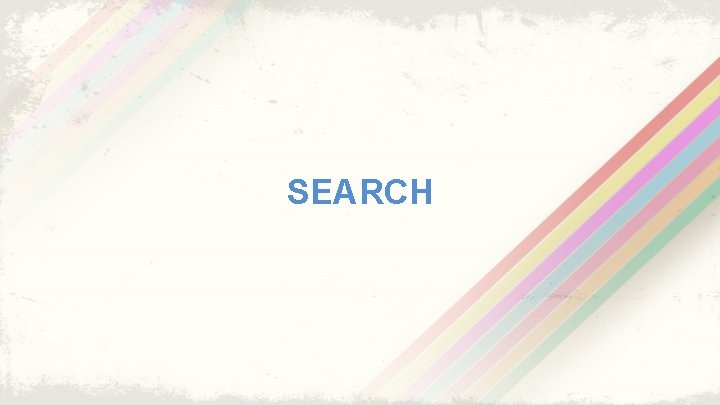
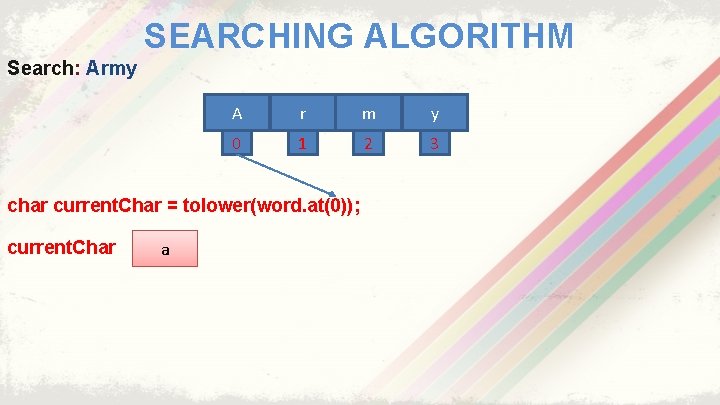
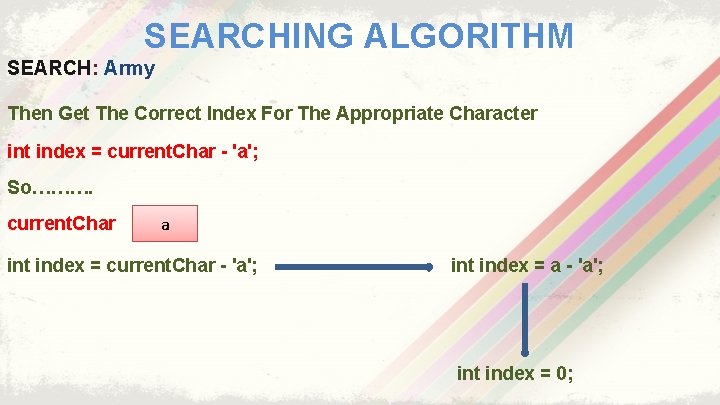
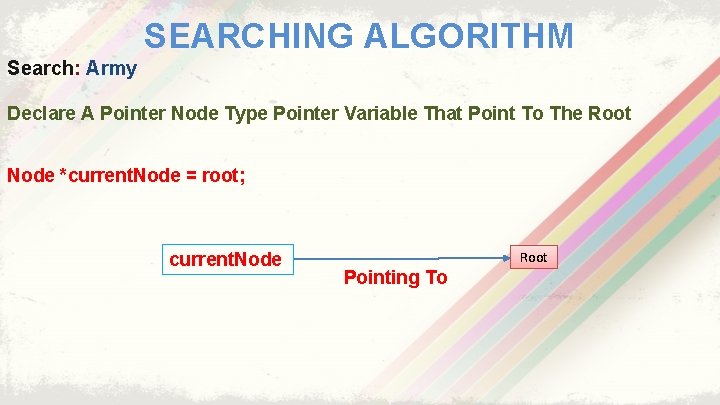
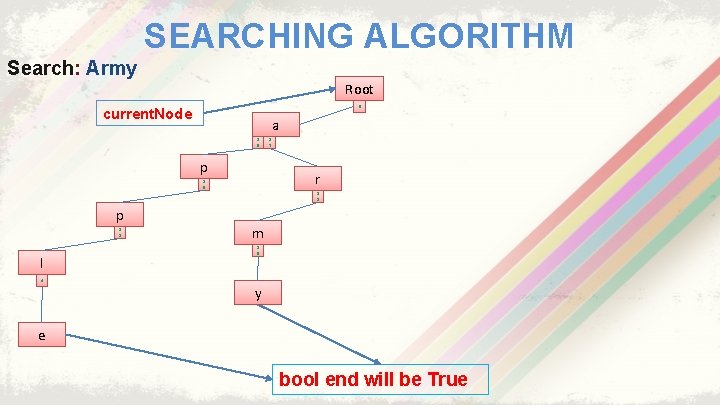
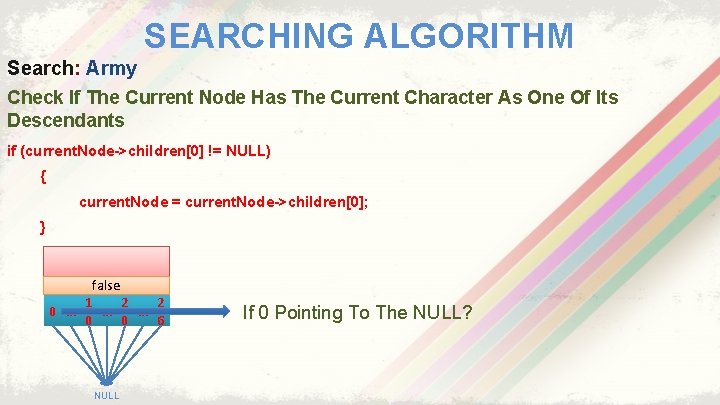
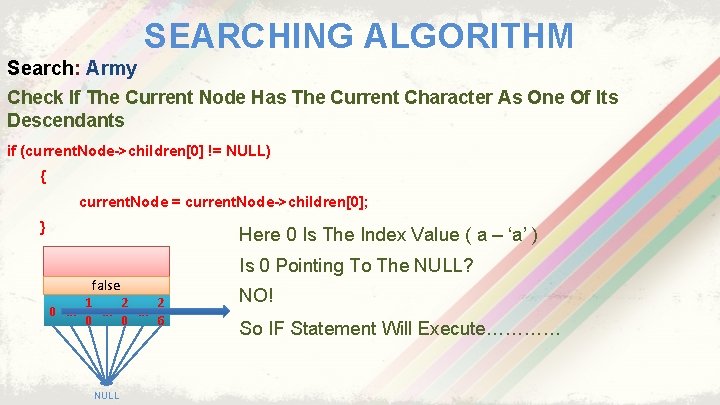
![SEARCHING ALGORITHM Search: Army So………. current. Node = current. Node->children[0]; SEARCHING ALGORITHM Search: Army So………. current. Node = current. Node->children[0];](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-111.jpg)
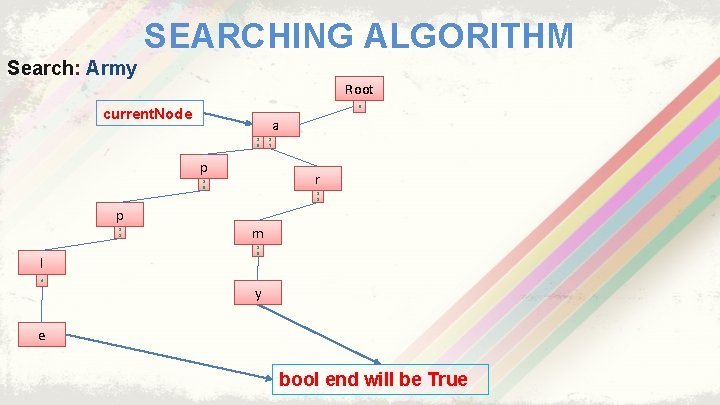
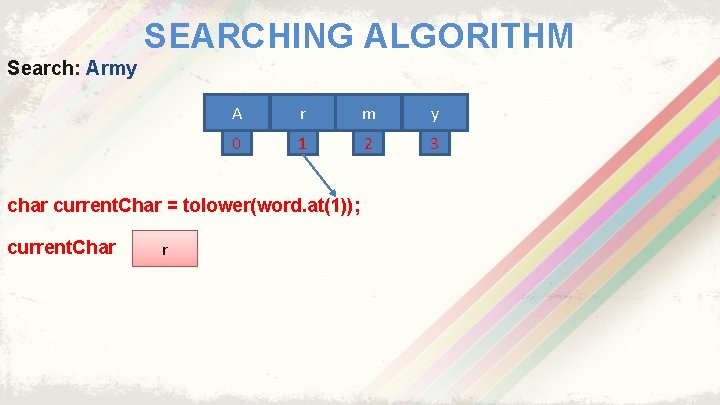
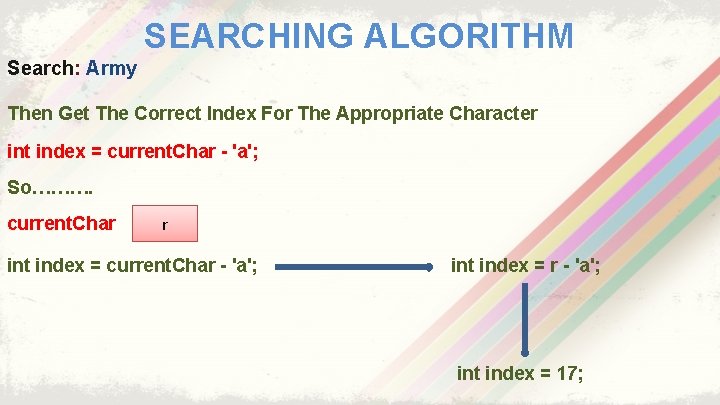
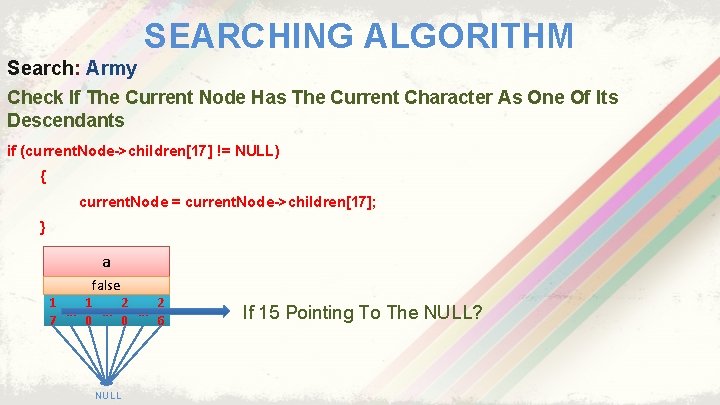
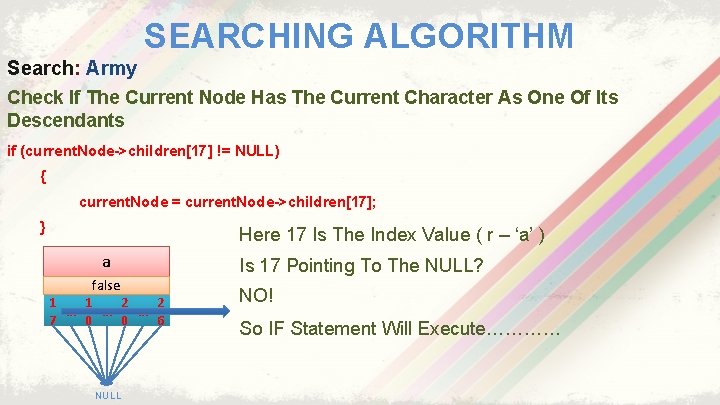
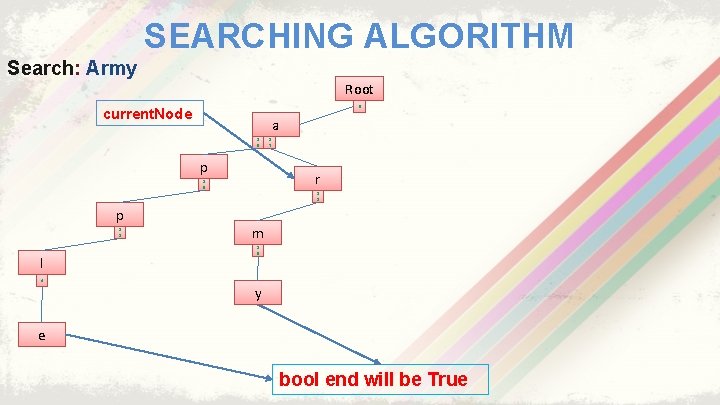
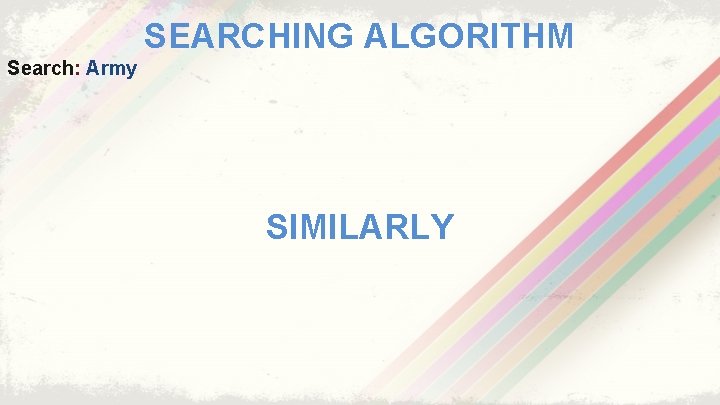
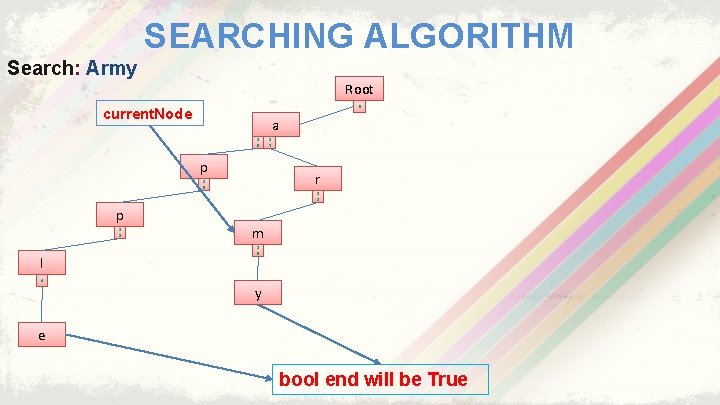
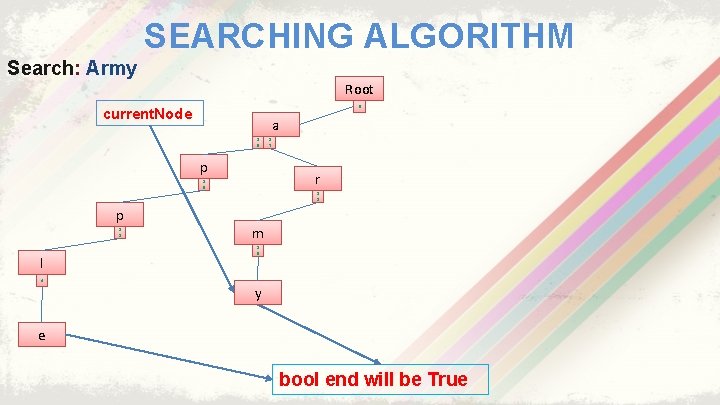
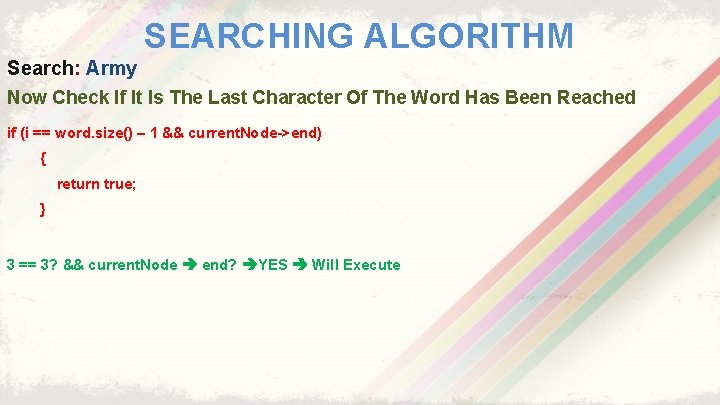
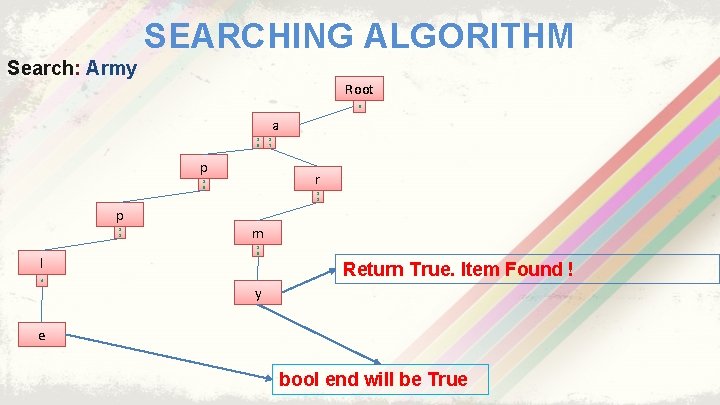
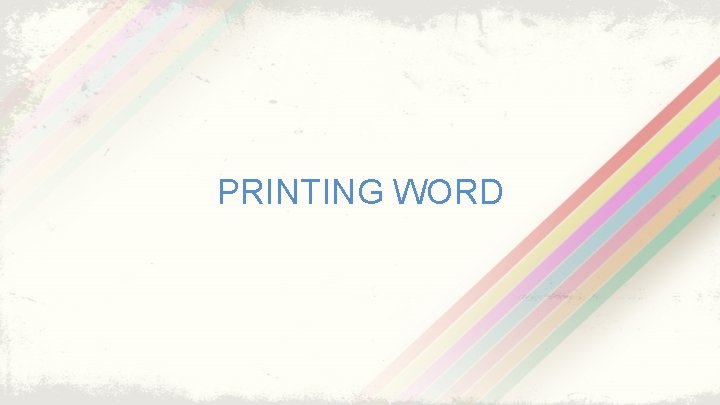
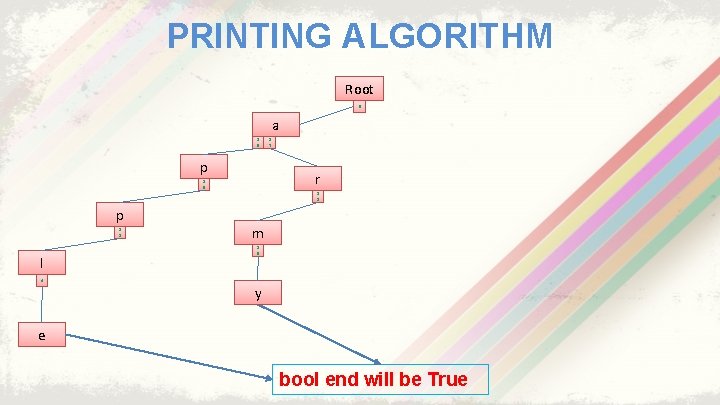
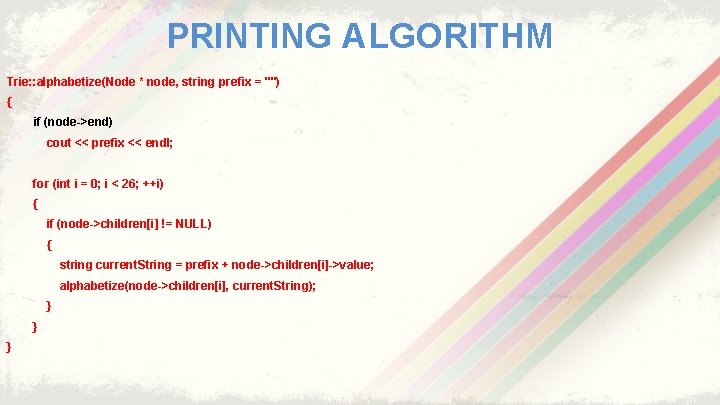
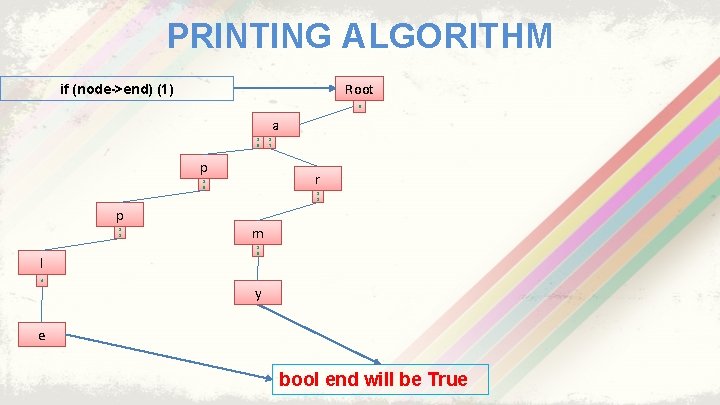
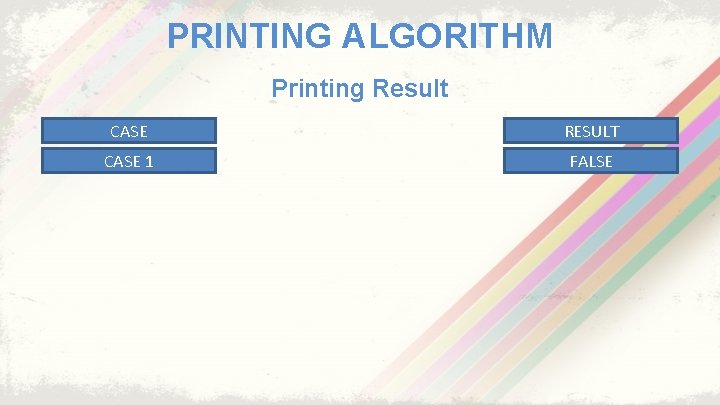
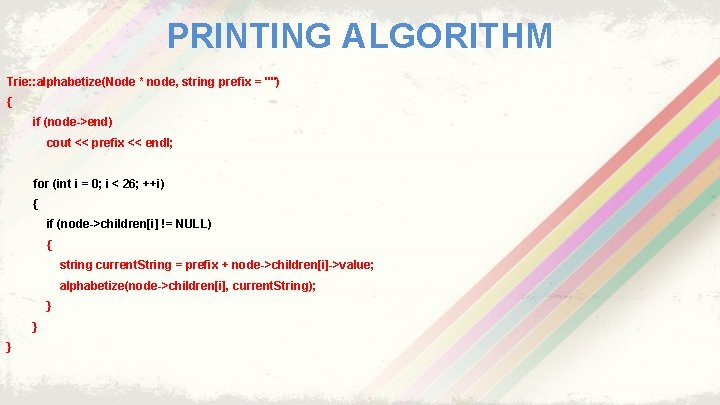
![PRINTING ALGORITHM Root if (node->children[0] != NULL) (2) 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[0] != NULL) (2) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-129.jpg)
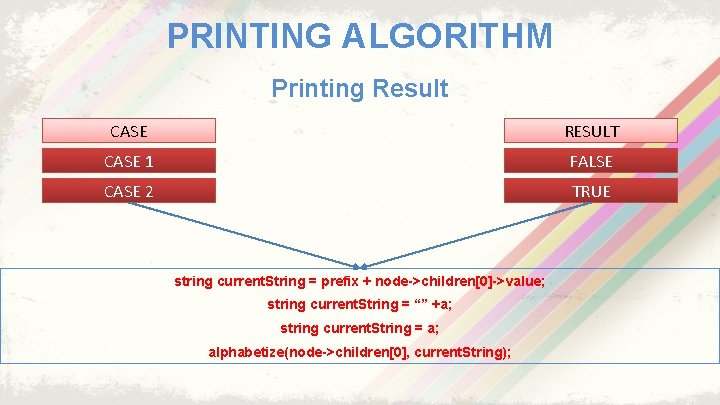
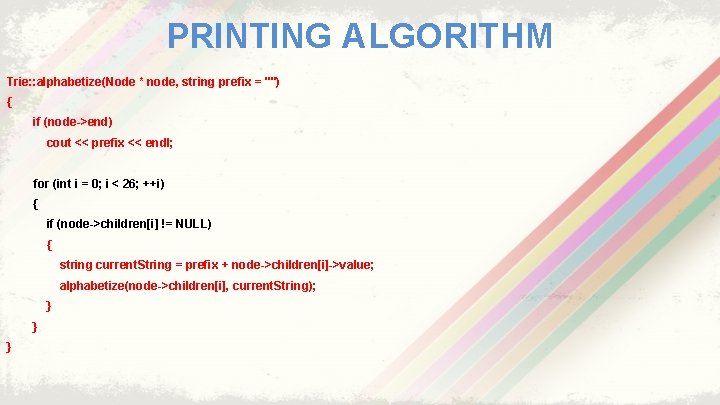
![PRINTING ALGORITHM Root if (node->children[1] != NULL) (3) 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[1] != NULL) (3) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-132.jpg)
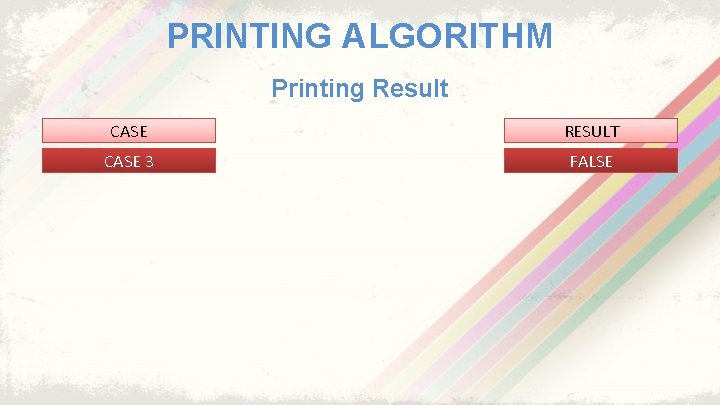
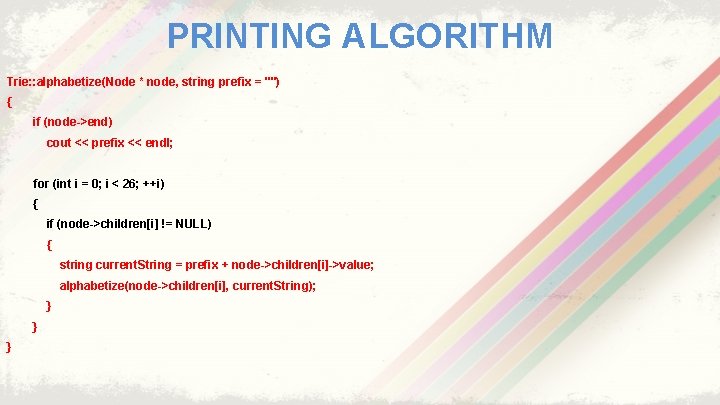
![PRINTING ALGORITHM Root if (node->children[2] != NULL) (4) 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[2] != NULL) (4) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-135.jpg)
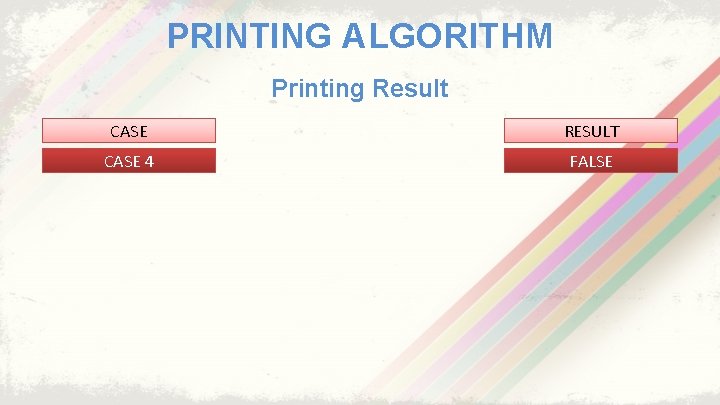
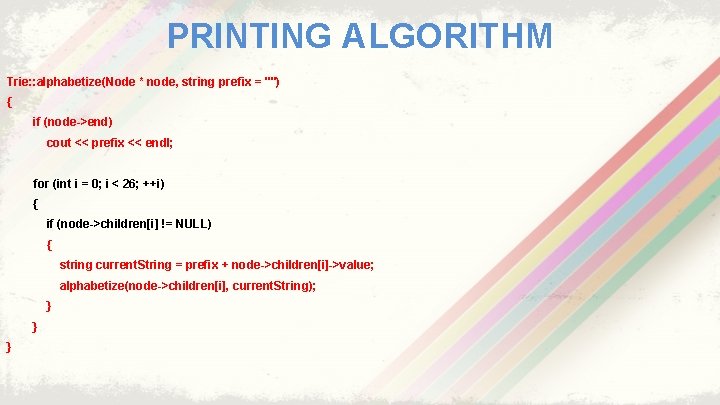
![PRINTING ALGORITHM Root if (node->children[15] != NULL) (17) 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[15] != NULL) (17) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-138.jpg)
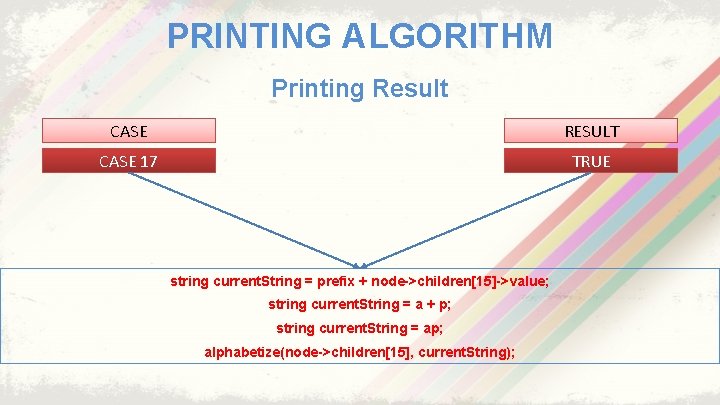
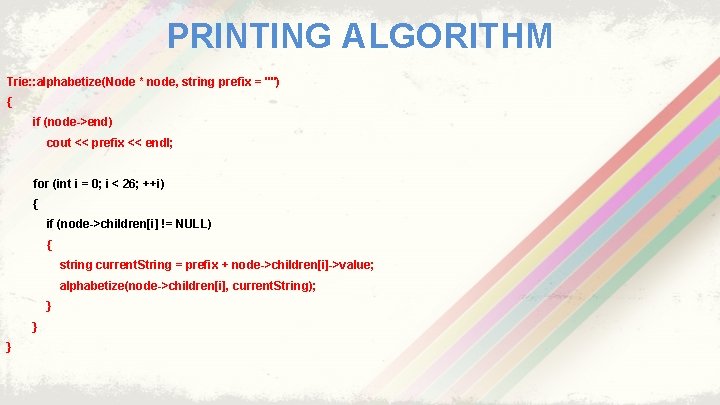
![PRINTING ALGORITHM Root if (node->children[15] != NULL) (18) 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[15] != NULL) (18) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-141.jpg)
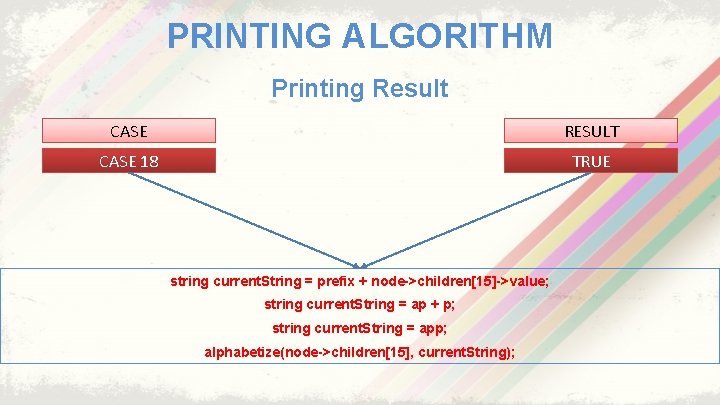
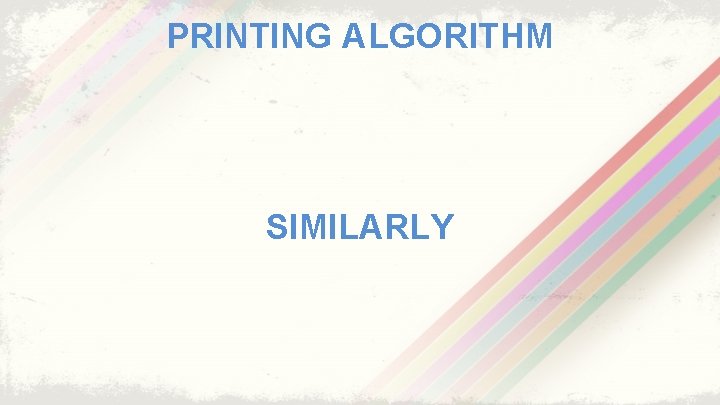
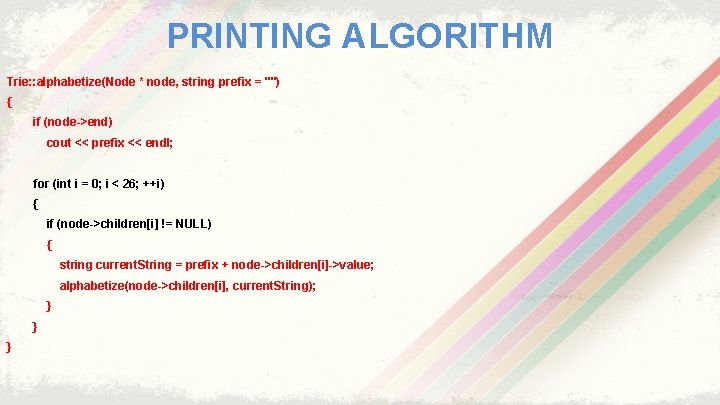
![PRINTING ALGORITHM Root if (node->children[4] != NULL) (19) 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[4] != NULL) (19) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-145.jpg)
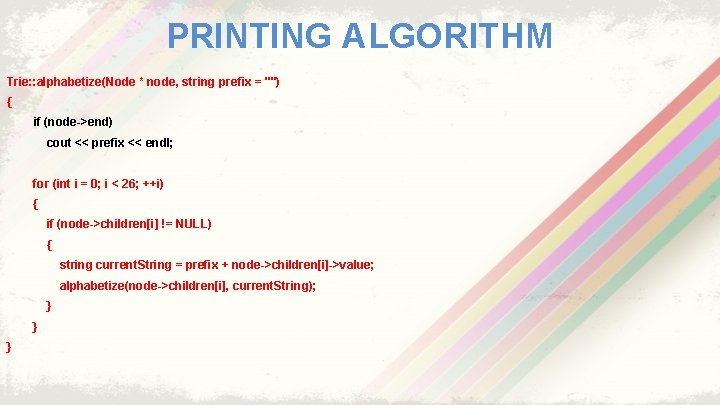
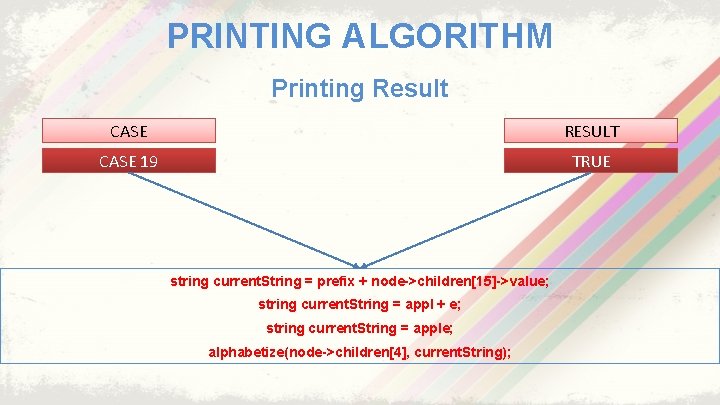
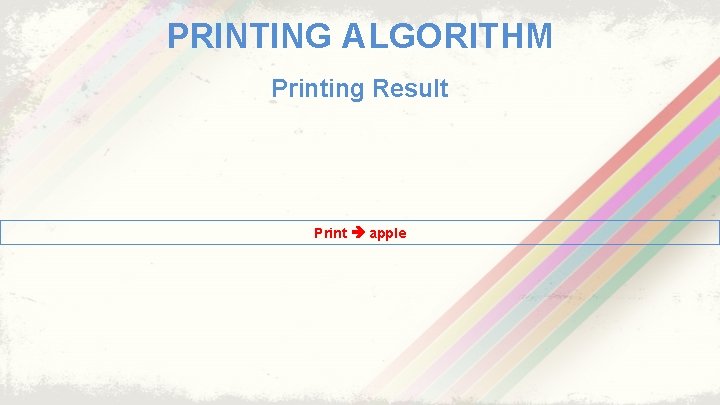
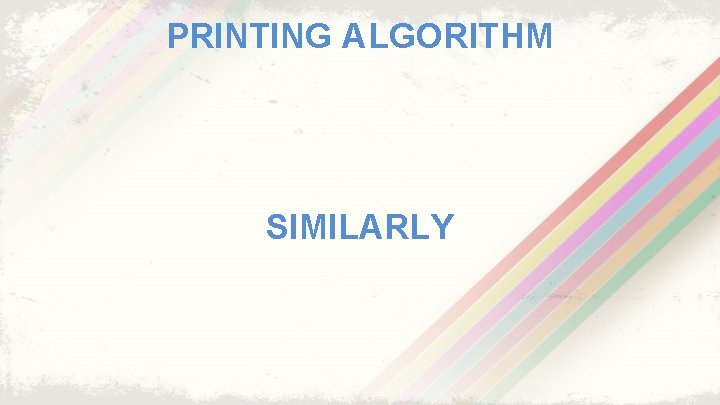
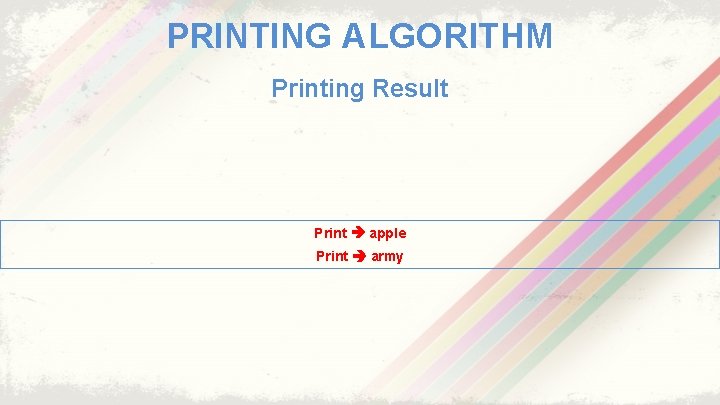
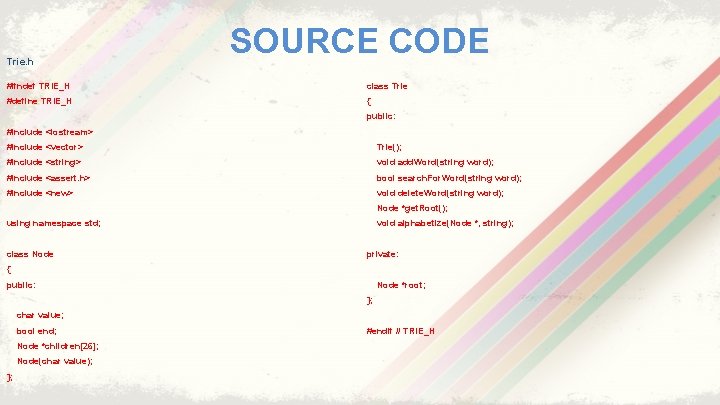
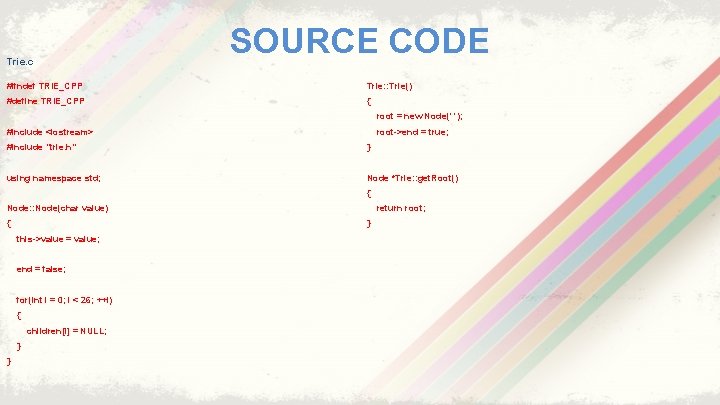
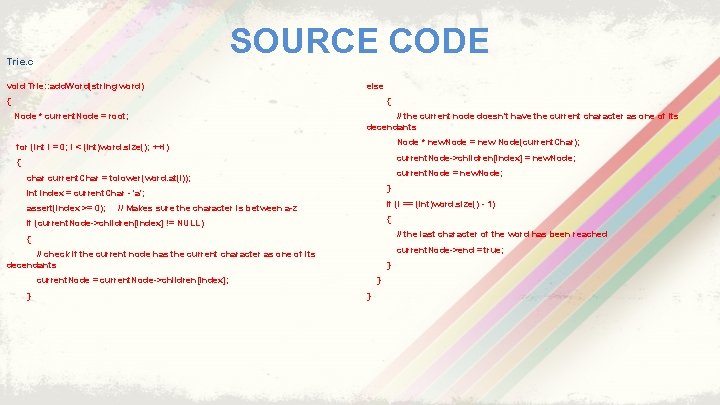
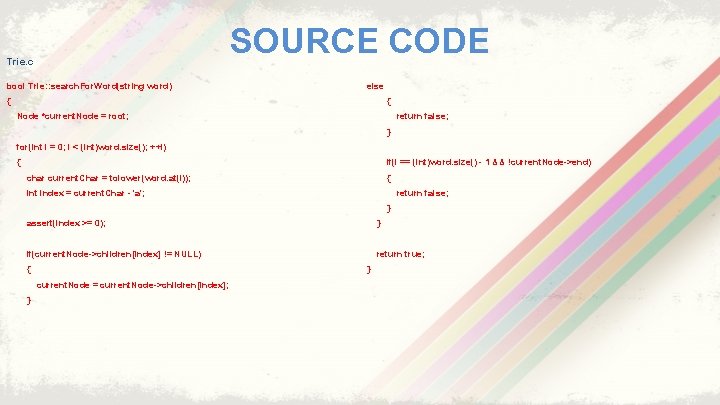
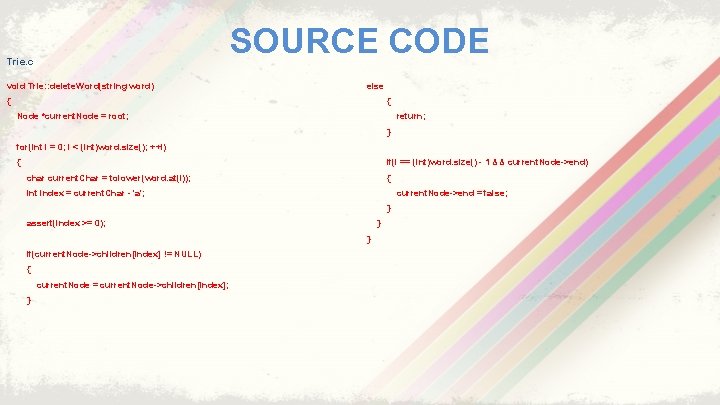
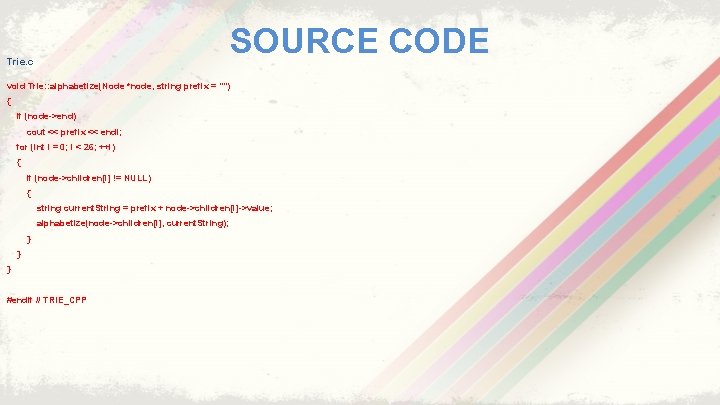
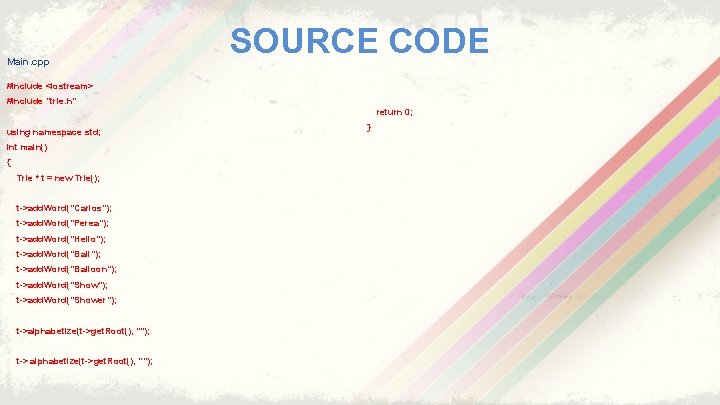
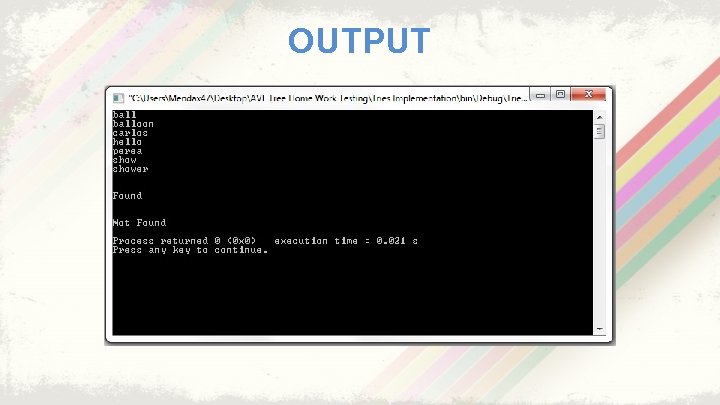
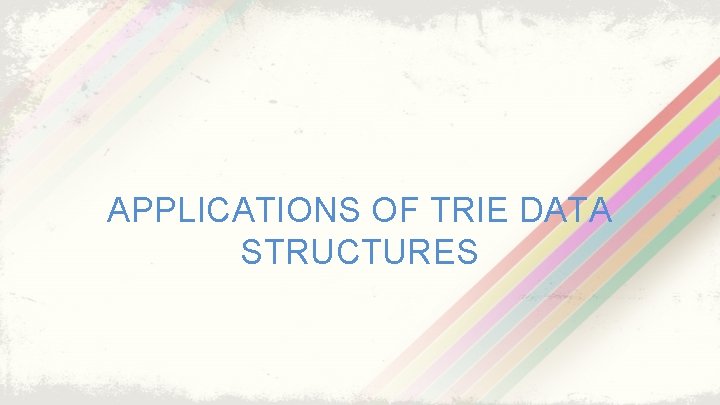
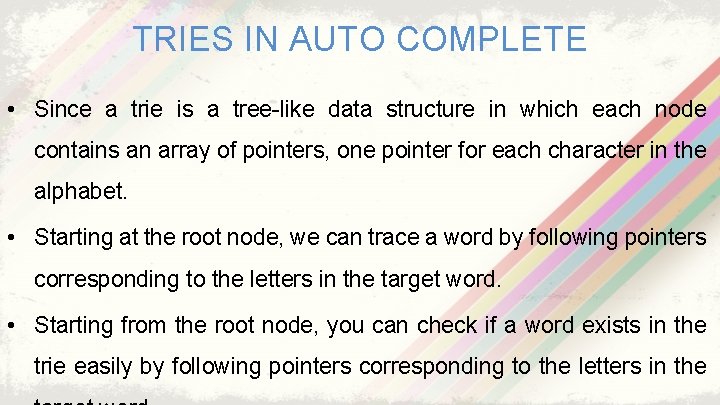
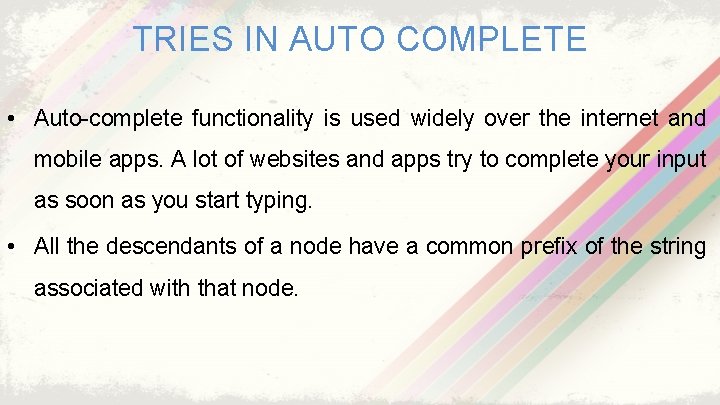
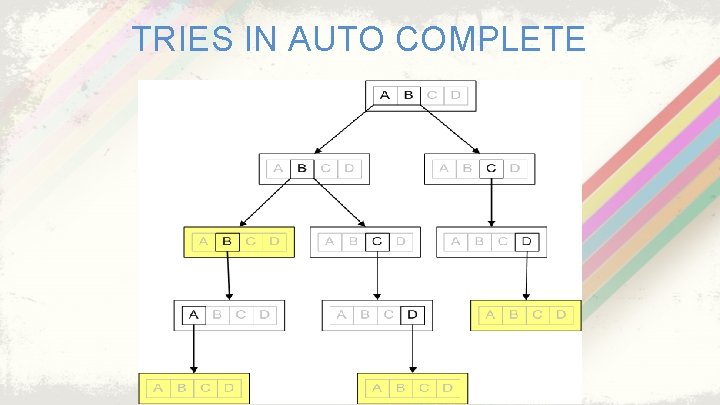
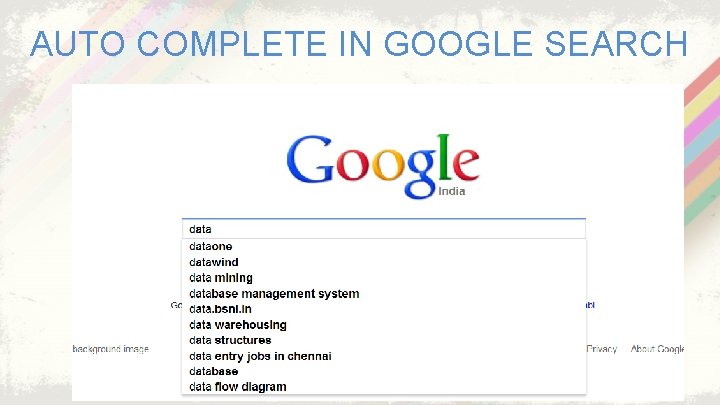
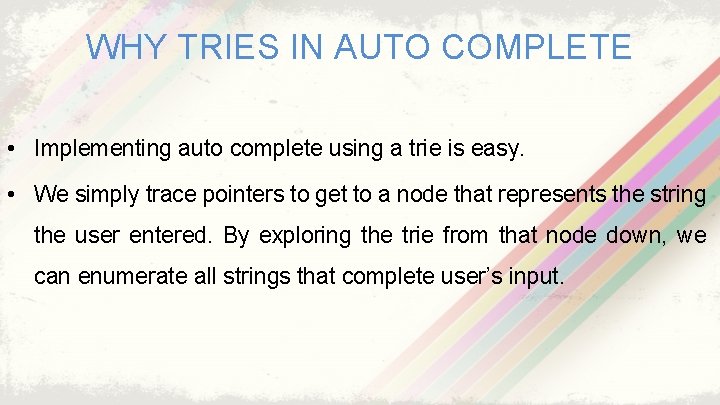
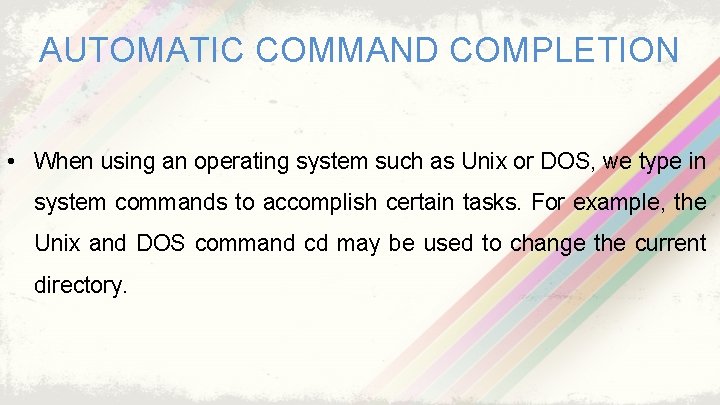
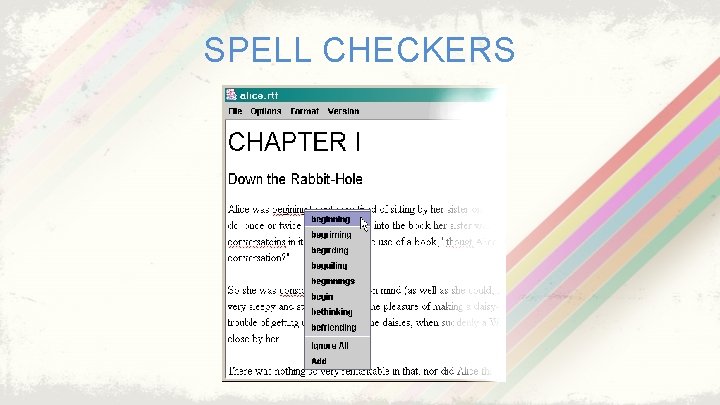
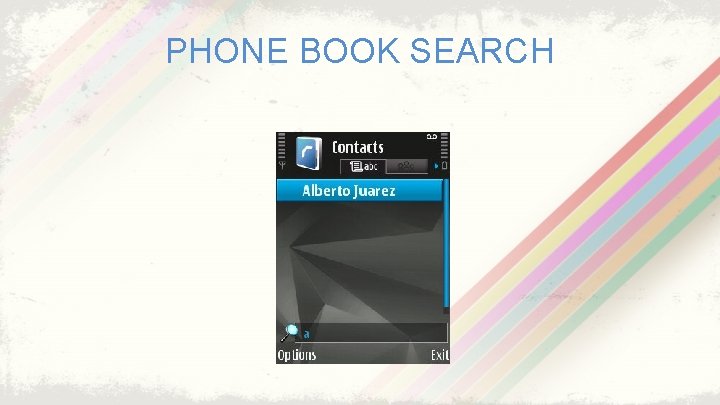
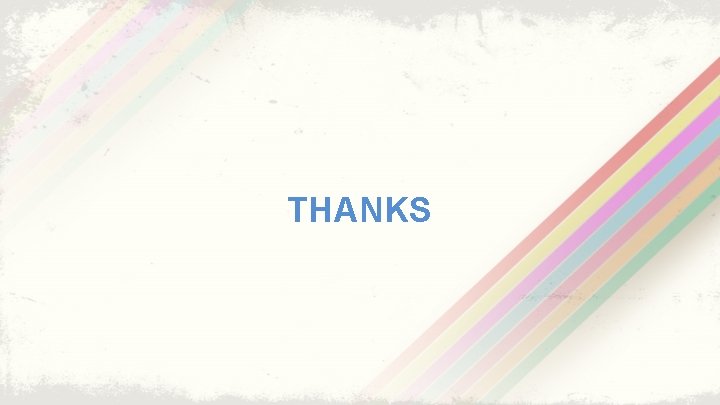
- Slides: 168
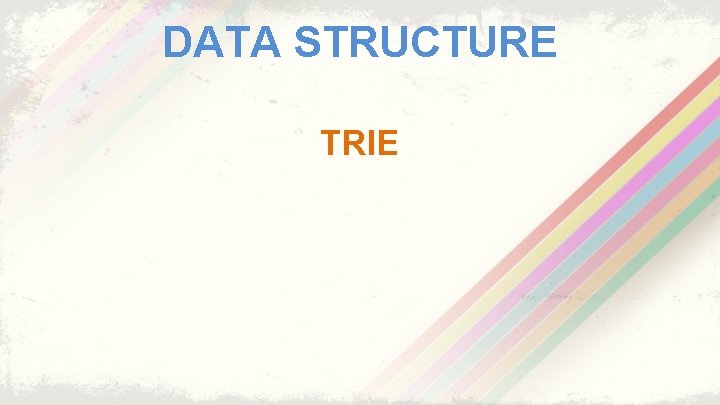
DATA STRUCTURE TRIE
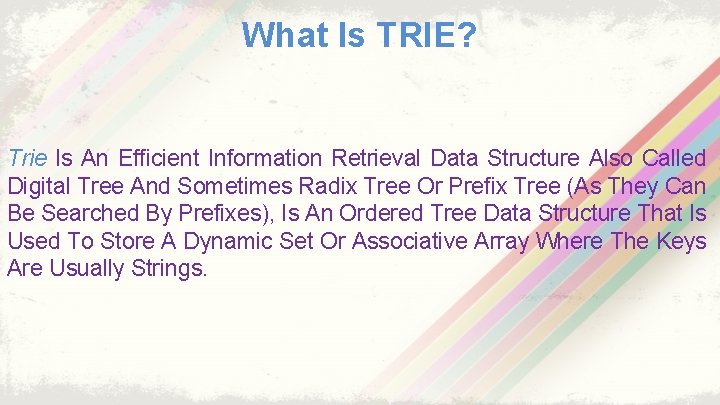
What Is TRIE? Trie Is An Efficient Information Retrieval Data Structure Also Called Digital Tree And Sometimes Radix Tree Or Prefix Tree (As They Can Be Searched By Prefixes), Is An Ordered Tree Data Structure That Is Used To Store A Dynamic Set Or Associative Array Where The Keys Are Usually Strings.
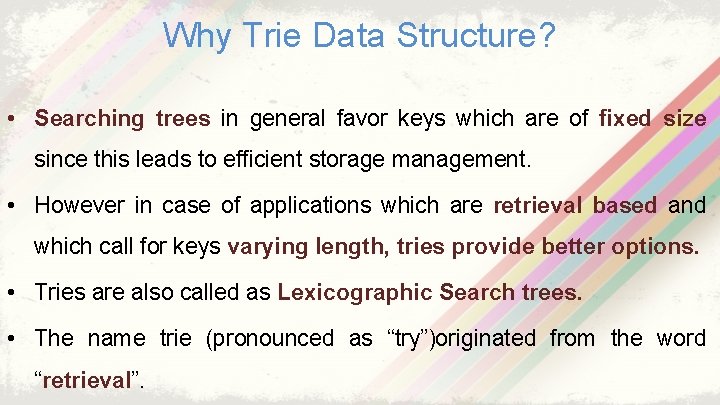
Why Trie Data Structure? • Searching trees in general favor keys which are of fixed size since this leads to efficient storage management. • However in case of applications which are retrieval based and which call for keys varying length, tries provide better options. • Tries are also called as Lexicographic Search trees. • The name trie (pronounced as “try”)originated from the word “retrieval”.
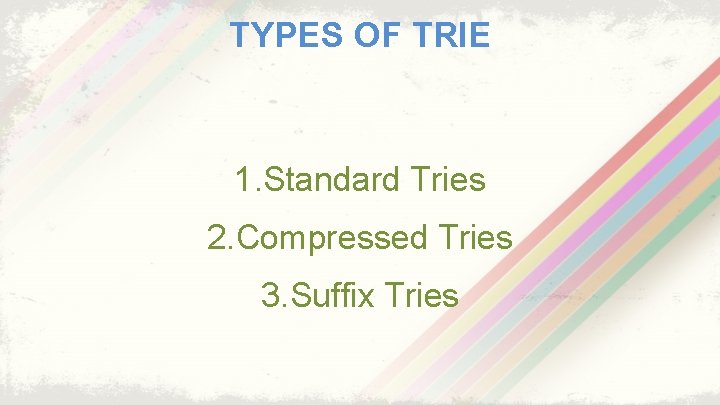
TYPES OF TRIE 1. Standard Tries 2. Compressed Tries 3. Suffix Tries
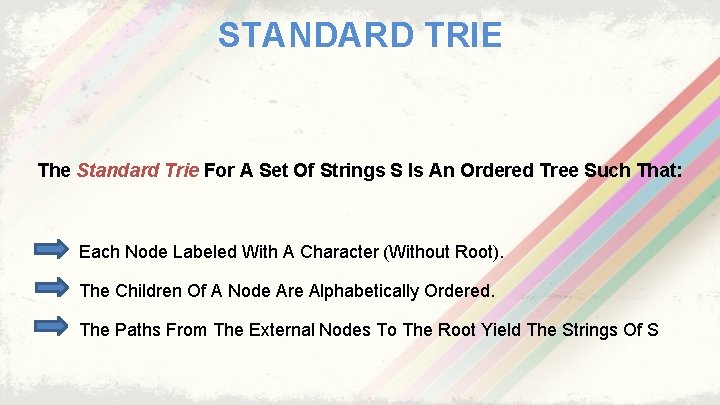
STANDARD TRIE The Standard Trie For A Set Of Strings S Is An Ordered Tree Such That: Each Node Labeled With A Character (Without Root). The Children Of A Node Are Alphabetically Ordered. The Paths From The External Nodes To The Root Yield The Strings Of S
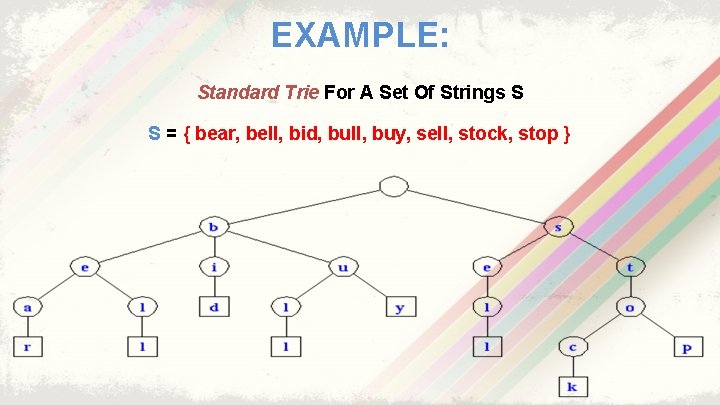
EXAMPLE: Standard Trie For A Set Of Strings S S = { bear, bell, bid, bull, buy, sell, stock, stop }
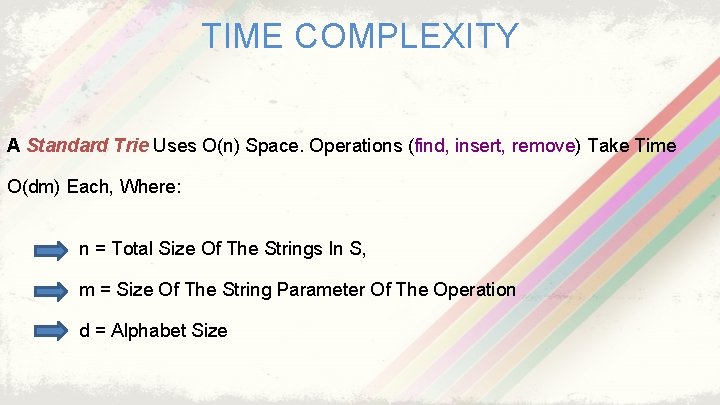
TIME COMPLEXITY A Standard Trie Uses O(n) Space. Operations (find, insert, remove) Take Time O(dm) Each, Where: n = Total Size Of The Strings In S, m = Size Of The String Parameter Of The Operation d = Alphabet Size
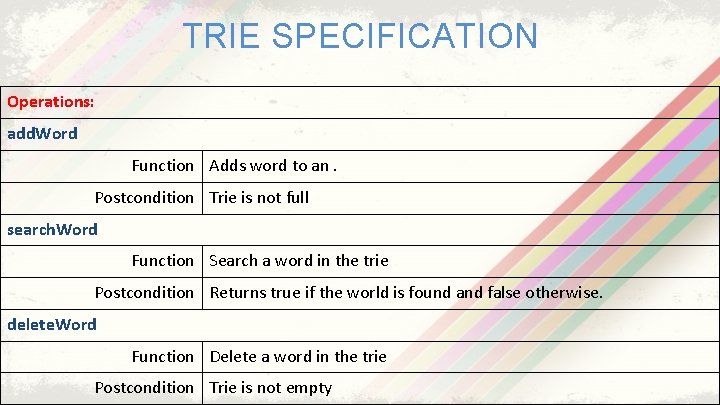
TRIE SPECIFICATION Operations: add. Word Function Adds word to an. Postcondition Trie is not full search. Word Function Search a word in the trie Postcondition Returns true if the world is found and false otherwise. delete. Word Function Delete a word in the trie Postcondition Trie is not empty
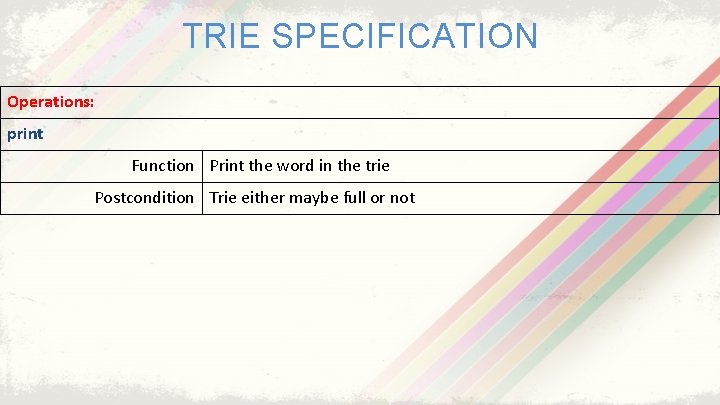
TRIE SPECIFICATION Operations: print Function Print the word in the trie Postcondition Trie either maybe full or not
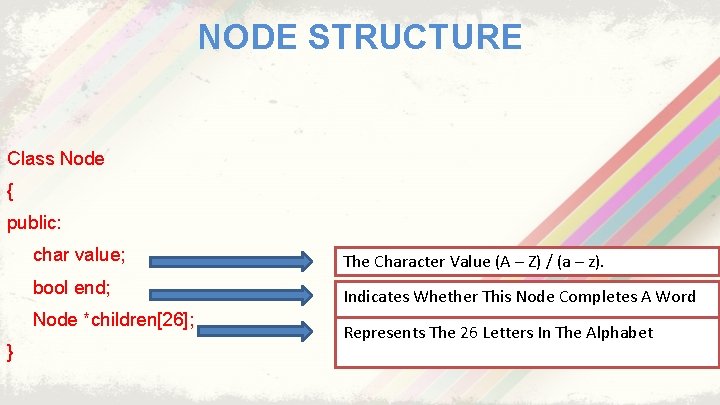
NODE STRUCTURE Class Node { public: char value; The Character Value (A – Z) / (a – z). bool end; Indicates Whether This Node Completes A Word Node *children[26]; } Represents The 26 Letters In The Alphabet
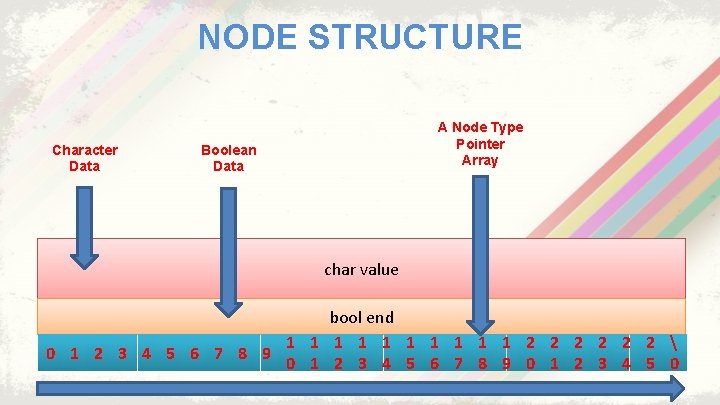
NODE STRUCTURE Character Data A Node Type Pointer Array Boolean Data char value bool end 1 1 1 1 1 2 2 2 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 0
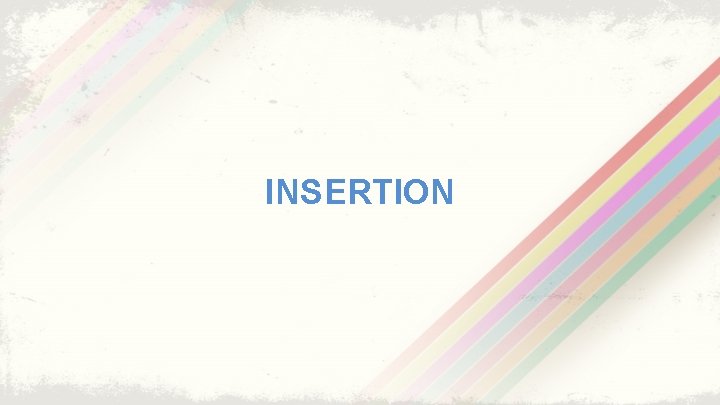
INSERTION
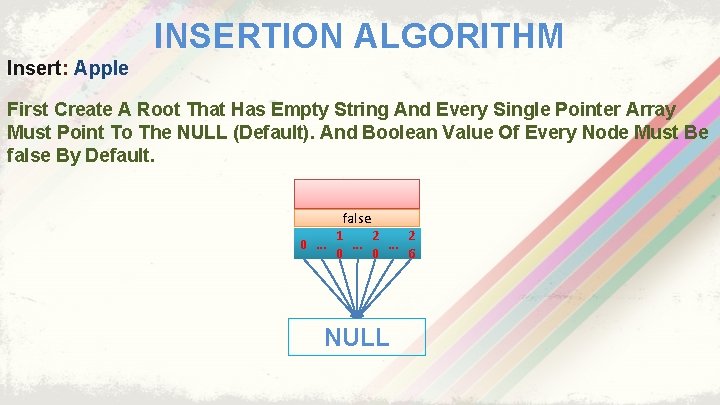
INSERTION ALGORITHM Insert: Apple First Create A Root That Has Empty String And Every Single Pointer Array Must Point To The NULL (Default). And Boolean Value Of Every Node Must Be false By Default. false 1 2 2 0 … … … 0 0 6 NULL
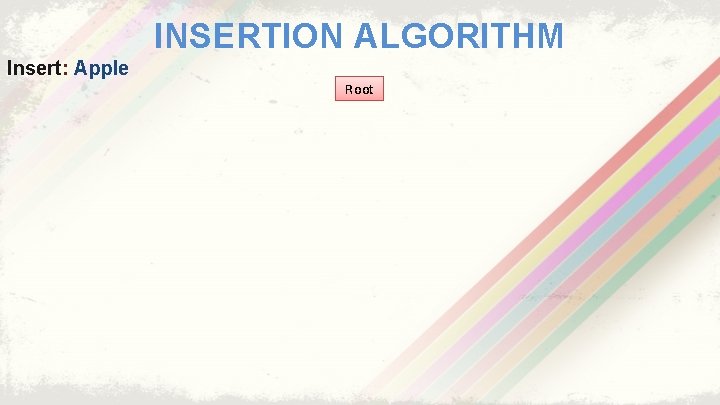
INSERTION ALGORITHM Insert: Apple Root
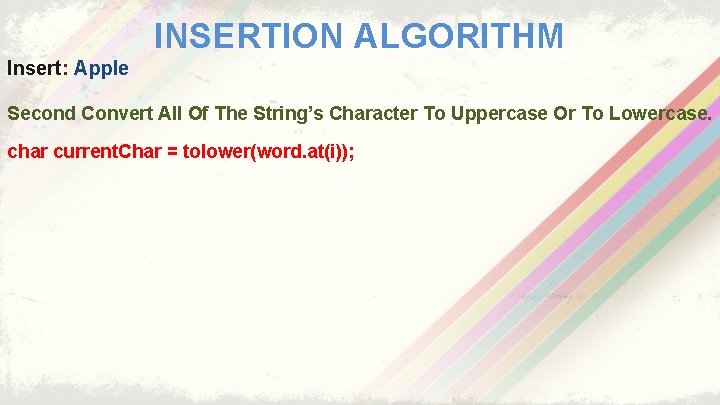
INSERTION ALGORITHM Insert: Apple Second Convert All Of The String’s Character To Uppercase Or To Lowercase. char current. Char = tolower(word. at(i));
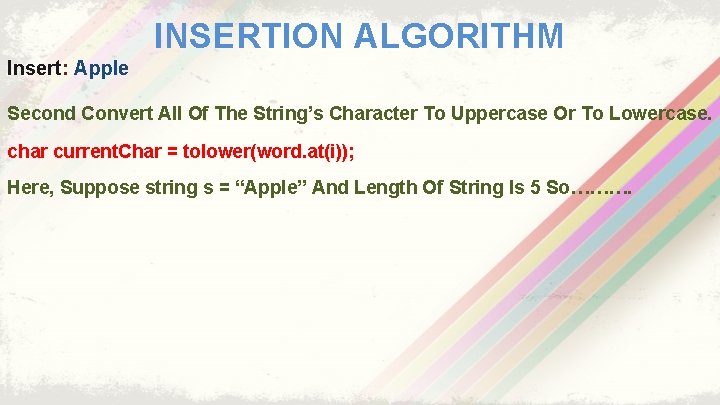
INSERTION ALGORITHM Insert: Apple Second Convert All Of The String’s Character To Uppercase Or To Lowercase. char current. Char = tolower(word. at(i)); Here, Suppose string s = “Apple” And Length Of String Is 5 So……….
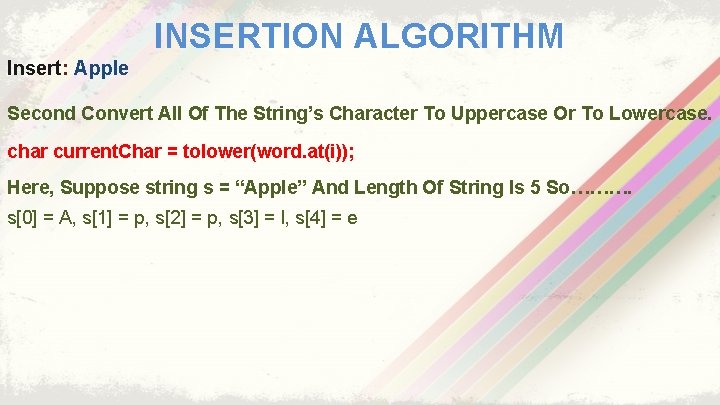
INSERTION ALGORITHM Insert: Apple Second Convert All Of The String’s Character To Uppercase Or To Lowercase. char current. Char = tolower(word. at(i)); Here, Suppose string s = “Apple” And Length Of String Is 5 So………. s[0] = A, s[1] = p, s[2] = p, s[3] = l, s[4] = e
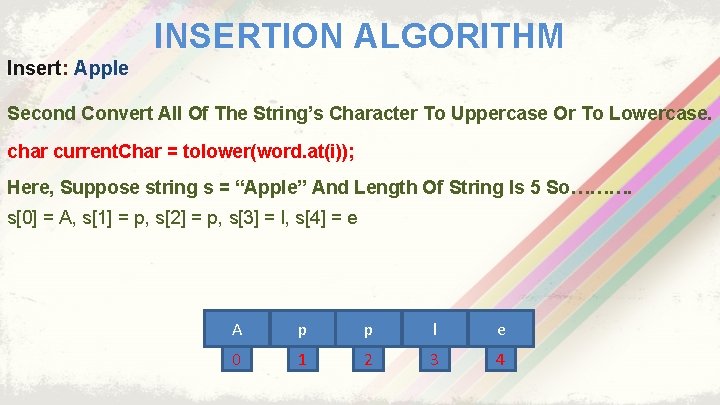
INSERTION ALGORITHM Insert: Apple Second Convert All Of The String’s Character To Uppercase Or To Lowercase. char current. Char = tolower(word. at(i)); Here, Suppose string s = “Apple” And Length Of String Is 5 So………. s[0] = A, s[1] = p, s[2] = p, s[3] = l, s[4] = e A p p l e 0 1 2 3 4
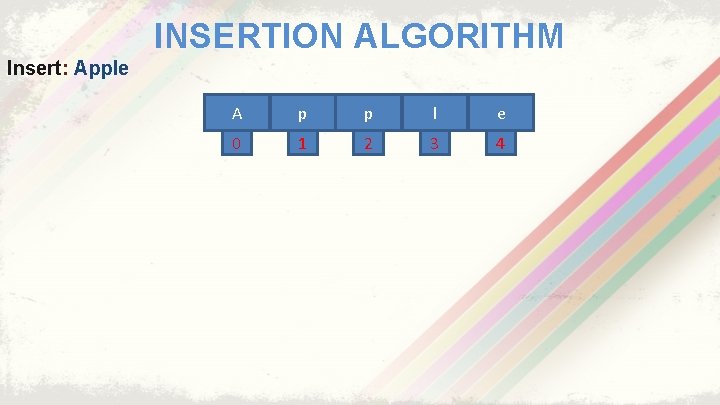
INSERTION ALGORITHM Insert: Apple A p p l e 0 1 2 3 4
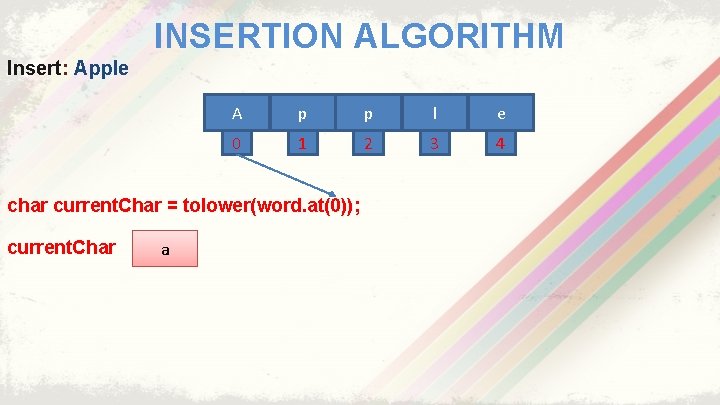
INSERTION ALGORITHM Insert: Apple A p p l e 0 1 2 3 4 char current. Char = tolower(word. at(0)); current. Char a
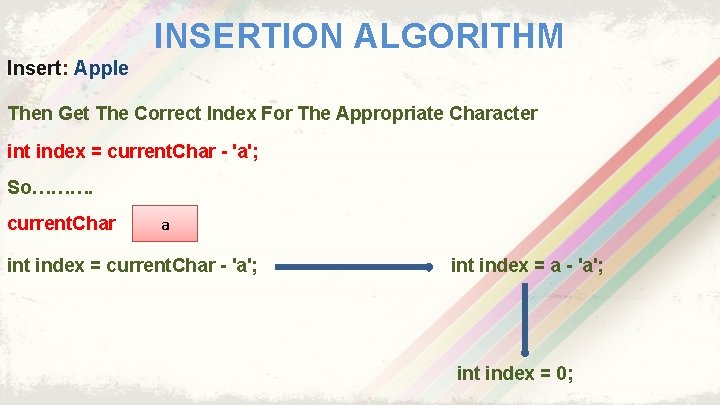
INSERTION ALGORITHM Insert: Apple Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char a int index = current. Char - 'a'; int index = a - 'a'; int index = 0;
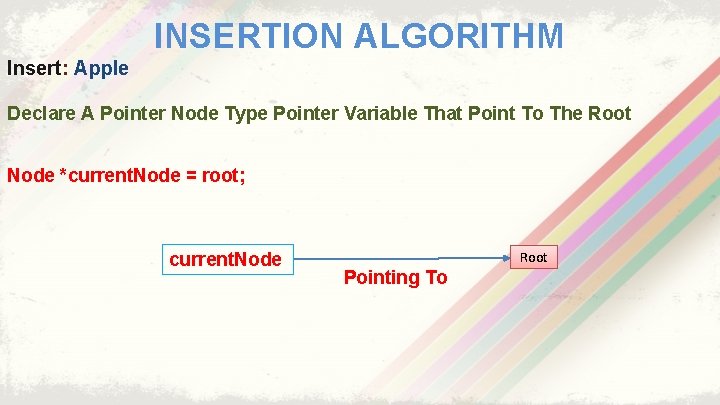
INSERTION ALGORITHM Insert: Apple Declare A Pointer Node Type Pointer Variable That Point To The Root Node *current. Node = root; current. Node Root Pointing To
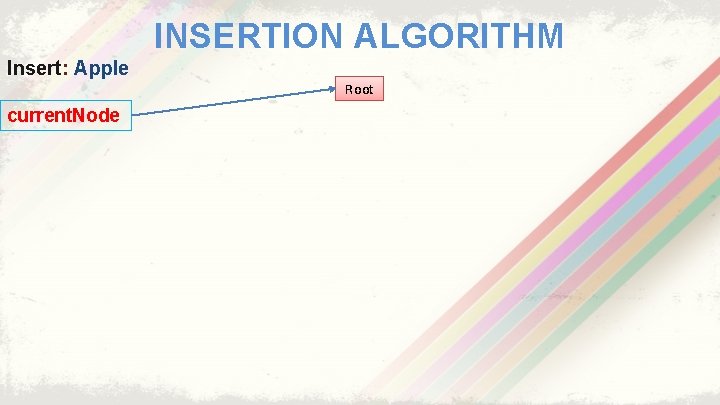
INSERTION ALGORITHM Insert: Apple current. Node Root
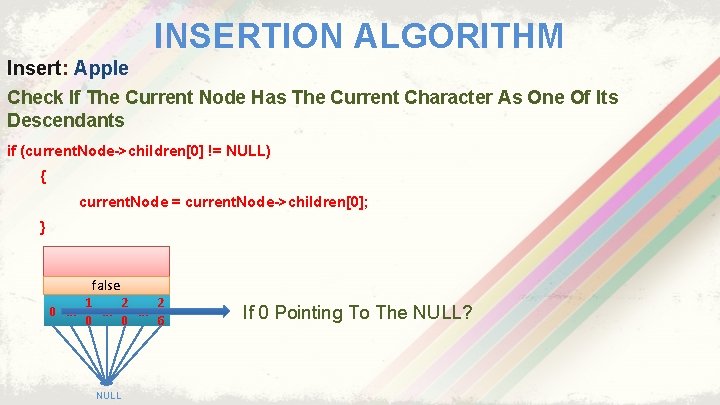
INSERTION ALGORITHM Insert: Apple Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } false 1 2 2 0 … … … 0 0 6 NULL If 0 Pointing To The NULL?
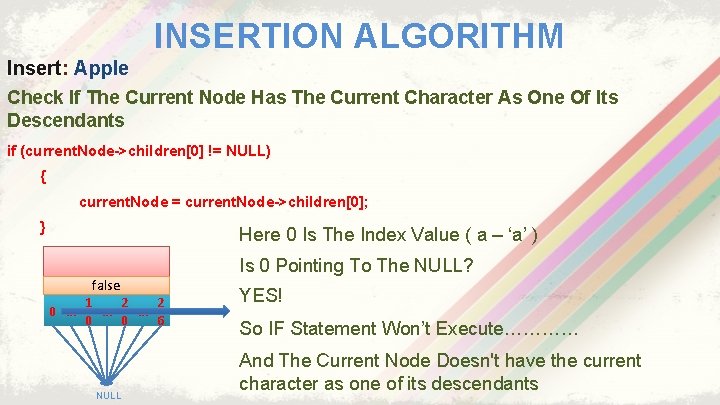
INSERTION ALGORITHM Insert: Apple Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } Here 0 Is The Index Value ( a – ‘a’ ) false 1 2 2 0 … … … 0 0 6 NULL Is 0 Pointing To The NULL? YES! So IF Statement Won’t Execute………… And The Current Node Doesn't have the current character as one of its descendants
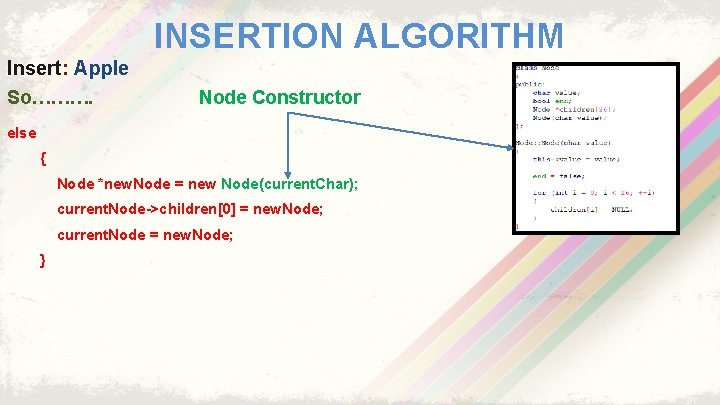
INSERTION ALGORITHM Insert: Apple So………. Node Constructor else { Node *new. Node = new Node(current. Char); current. Node->children[0] = new. Node; current. Node = new. Node; }
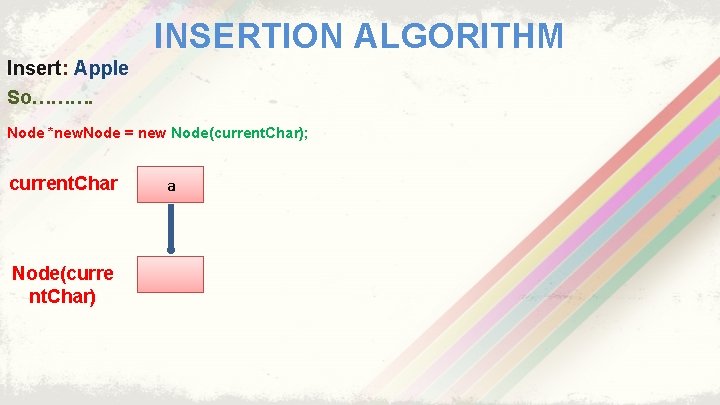
INSERTION ALGORITHM Insert: Apple So………. Node *new. Node = new Node(current. Char); current. Char Node(curre nt. Char) a
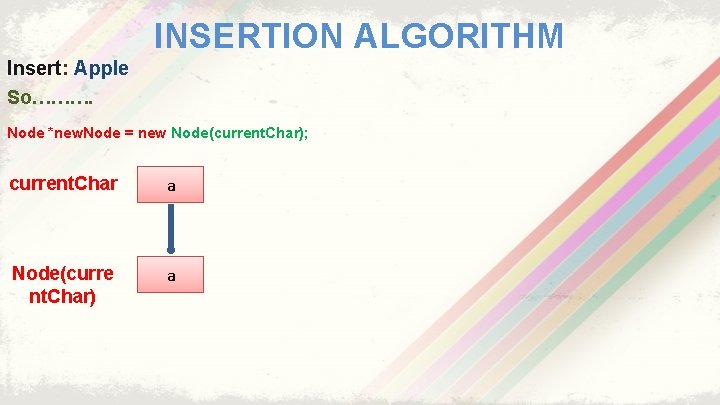
INSERTION ALGORITHM Insert: Apple So………. Node *new. Node = new Node(current. Char); current. Char a Node(curre nt. Char) a
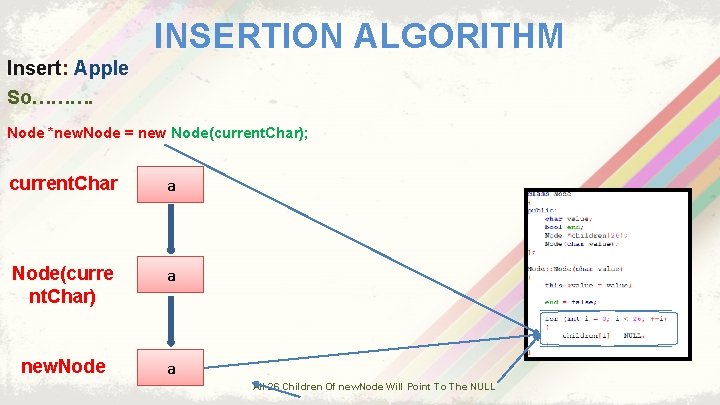
INSERTION ALGORITHM Insert: Apple So………. Node *new. Node = new Node(current. Char); current. Char a Node(curre nt. Char) a new. Node a All 26 Children Of new. Node Will Point To The NULL
![INSERTION ALGORITHM Insert Apple So current Nodechildren0 new Node new Node a current INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node a current.](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-30.jpg)
INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node a current. Node Root 0
![INSERTION ALGORITHM Insert Apple So current Nodechildren0 new Node new Node current Node INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node current. Node](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-31.jpg)
INSERTION ALGORITHM Insert: Apple So………. current. Node->children[0] = new. Node; new. Node current. Node Root 0 a
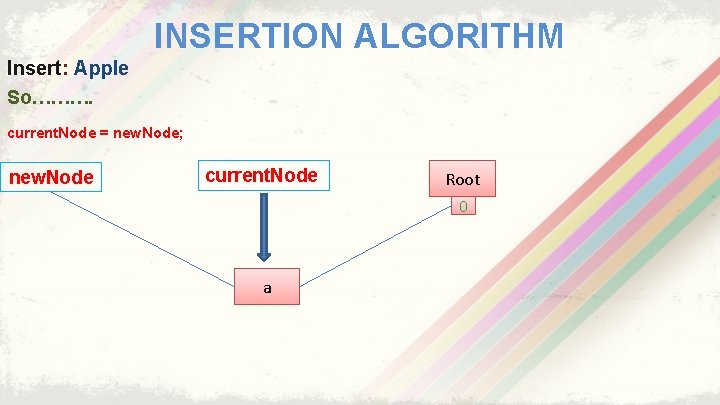
INSERTION ALGORITHM Insert: Apple So………. current. Node = new. Node; new. Node current. Node Root 0 a
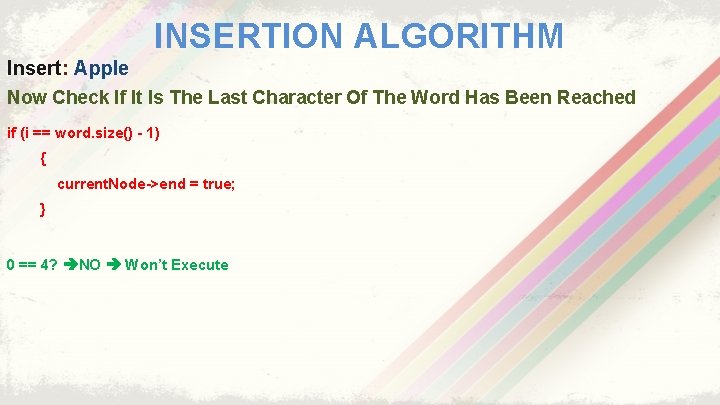
INSERTION ALGORITHM Insert: Apple Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() - 1) { current. Node->end = true; } 0 == 4? NO Won’t Execute
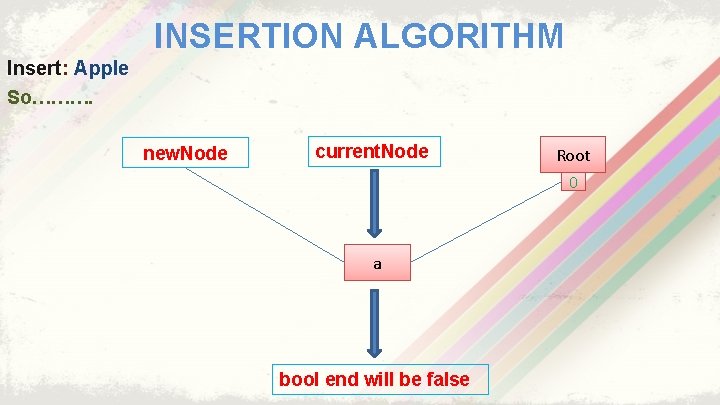
INSERTION ALGORITHM Insert: Apple So………. new. Node current. Node Root 0 a bool end will be false
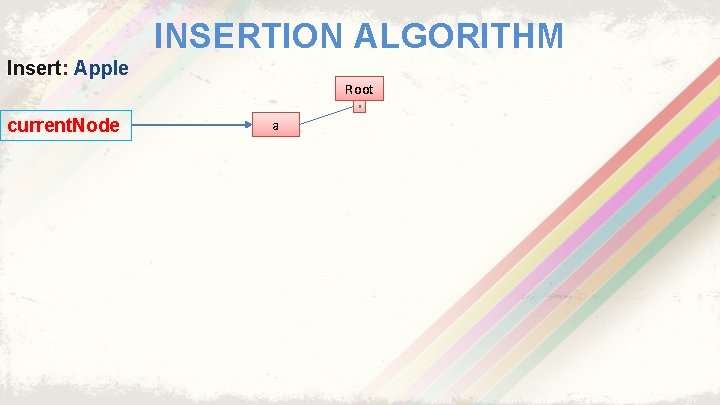
INSERTION ALGORITHM Insert: Apple Root 0 current. Node a
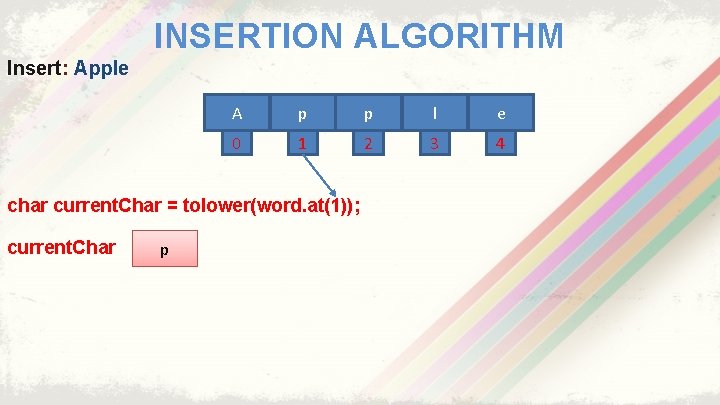
INSERTION ALGORITHM Insert: Apple A p p l e 0 1 2 3 4 char current. Char = tolower(word. at(1)); current. Char p
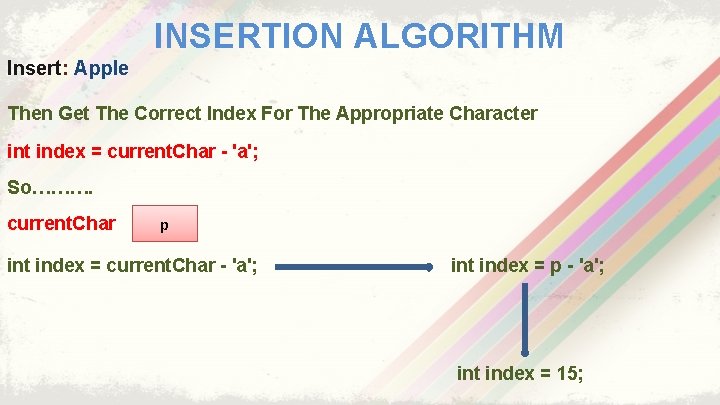
INSERTION ALGORITHM Insert: Apple Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char p int index = current. Char - 'a'; int index = p - 'a'; int index = 15;
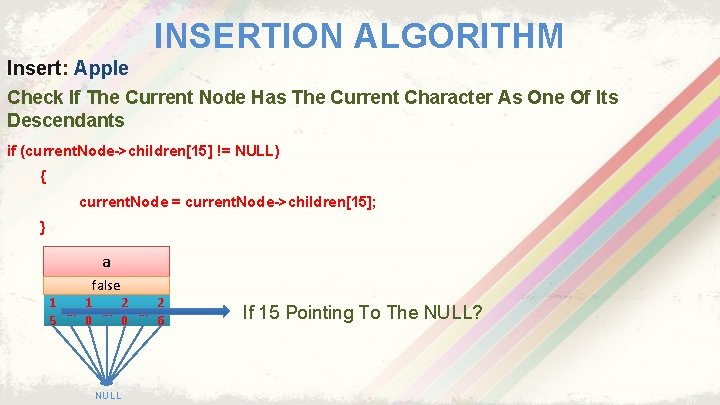
INSERTION ALGORITHM Insert: Apple Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[15] != NULL) { current. Node = current. Node->children[15]; } a false 1 2 2 1 … … … 0 0 6 5 NULL If 15 Pointing To The NULL?
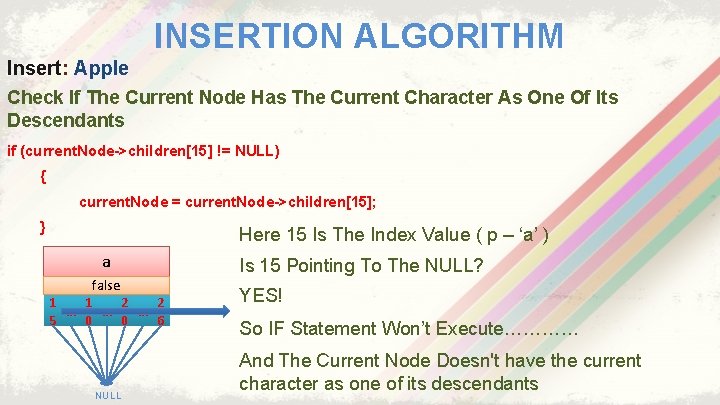
INSERTION ALGORITHM Insert: Apple Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[15] != NULL) { current. Node = current. Node->children[15]; } Here 15 Is The Index Value ( p – ‘a’ ) a false 1 2 2 1 … … … 0 0 6 5 NULL Is 15 Pointing To The NULL? YES! So IF Statement Won’t Execute………… And The Current Node Doesn't have the current character as one of its descendants
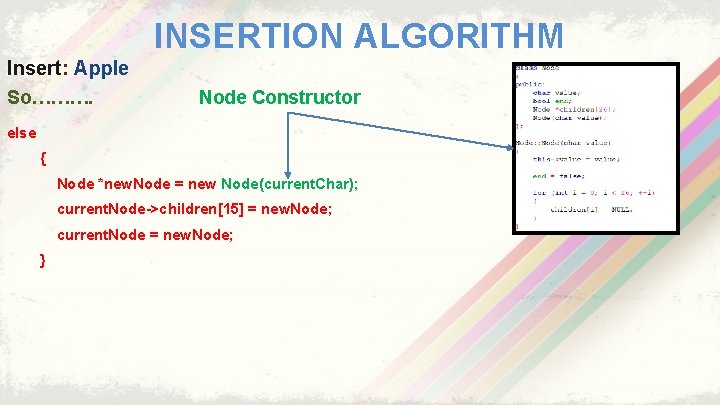
INSERTION ALGORITHM Insert: Apple So………. Node Constructor else { Node *new. Node = new Node(current. Char); current. Node->children[15] = new. Node; current. Node = new. Node; }
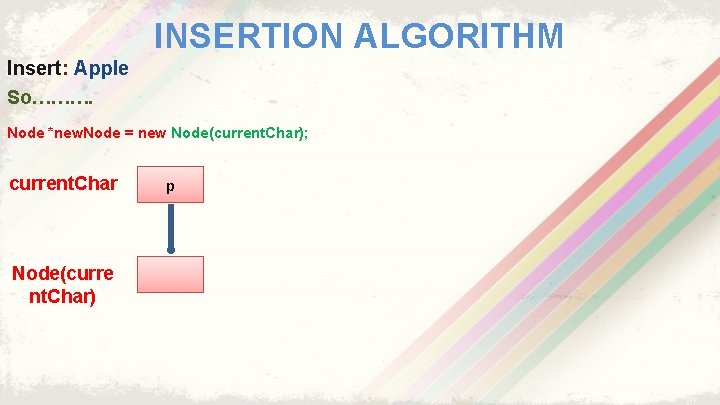
INSERTION ALGORITHM Insert: Apple So………. Node *new. Node = new Node(current. Char); current. Char Node(curre nt. Char) p
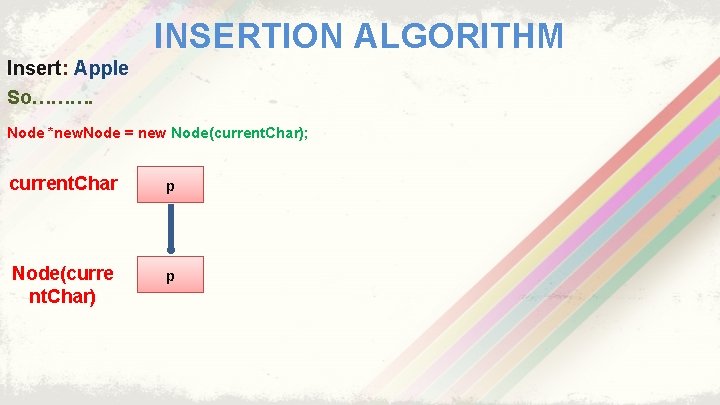
INSERTION ALGORITHM Insert: Apple So………. Node *new. Node = new Node(current. Char); current. Char p Node(curre nt. Char) p
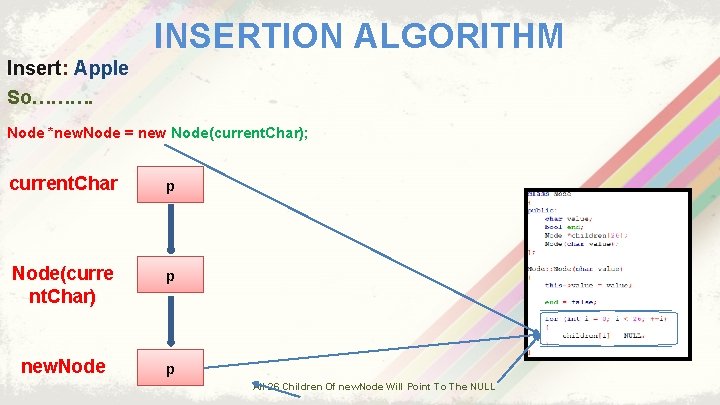
INSERTION ALGORITHM Insert: Apple So………. Node *new. Node = new Node(current. Char); current. Char p Node(curre nt. Char) p new. Node p All 26 Children Of new. Node Will Point To The NULL
![INSERTION ALGORITHM Insert Apple So current Nodechildren15 new Node new Node p current INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node p current.](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-44.jpg)
INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node p current. Node a 1 5
![INSERTION ALGORITHM Insert Apple So current Nodechildren15 new Node new Node current Node INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node current. Node](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-45.jpg)
INSERTION ALGORITHM Insert: Apple So………. current. Node->children[15] = new. Node; new. Node current. Node p a 1 5
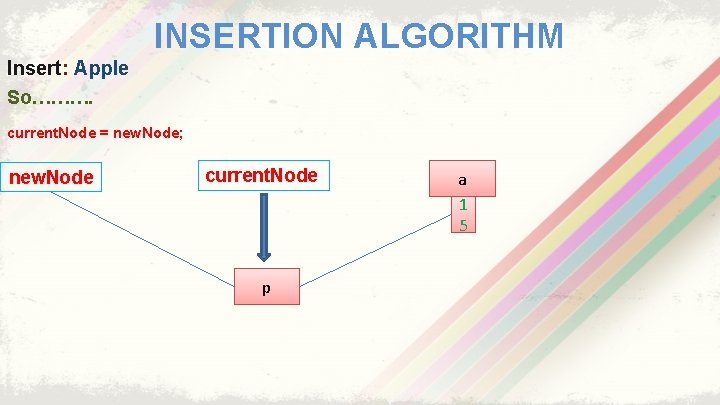
INSERTION ALGORITHM Insert: Apple So………. current. Node = new. Node; new. Node current. Node p a 1 5
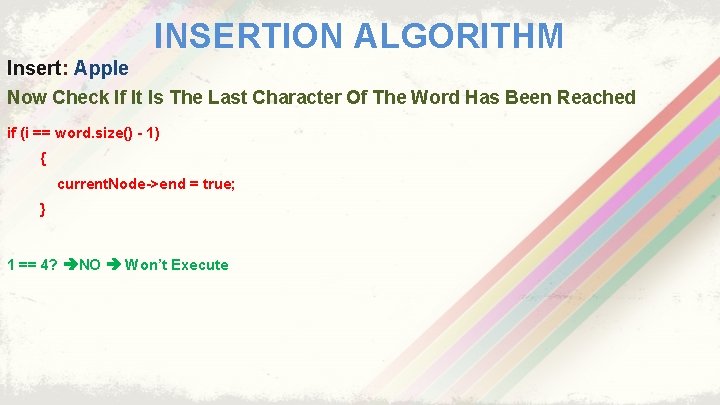
INSERTION ALGORITHM Insert: Apple Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() - 1) { current. Node->end = true; } 1 == 4? NO Won’t Execute
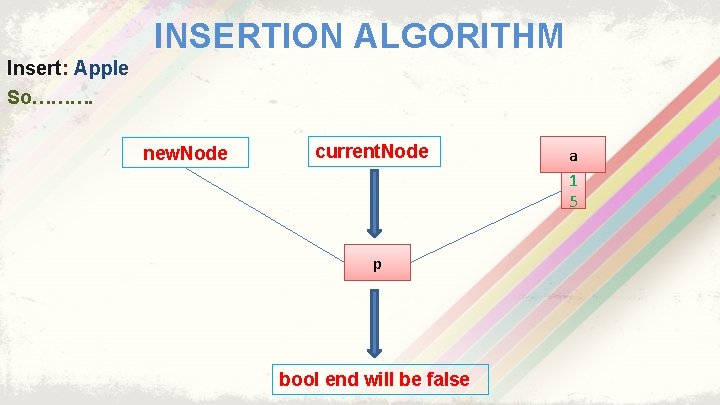
INSERTION ALGORITHM Insert: Apple So………. new. Node current. Node p bool end will be false a 1 5
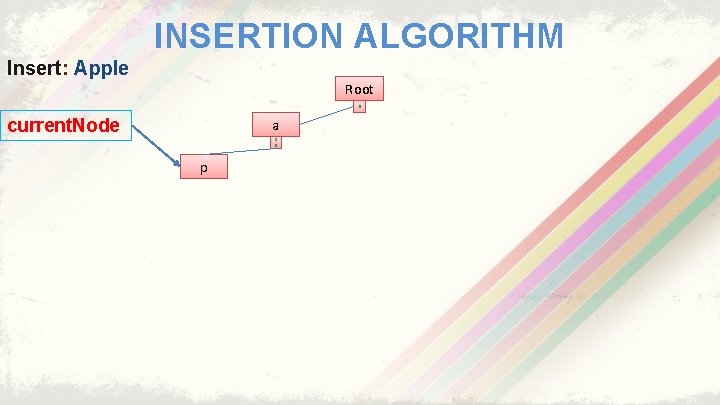
INSERTION ALGORITHM Insert: Apple Root 0 current. Node a 1 5 p
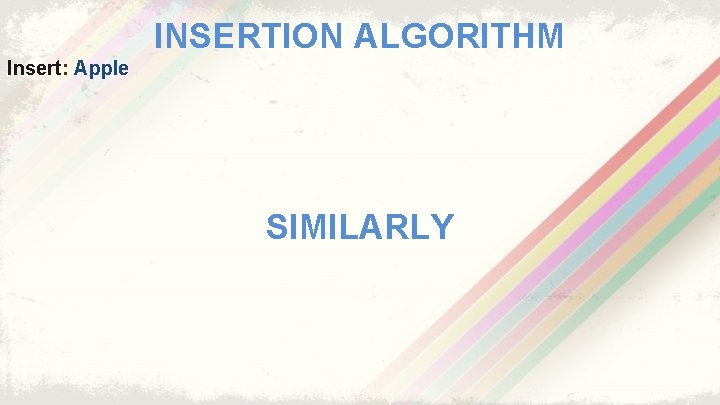
INSERTION ALGORITHM Insert: Apple SIMILARLY
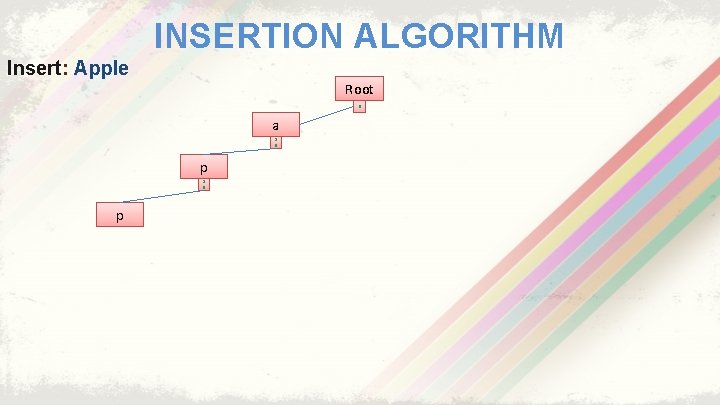
INSERTION ALGORITHM Insert: Apple Root 0 a 1 5 p
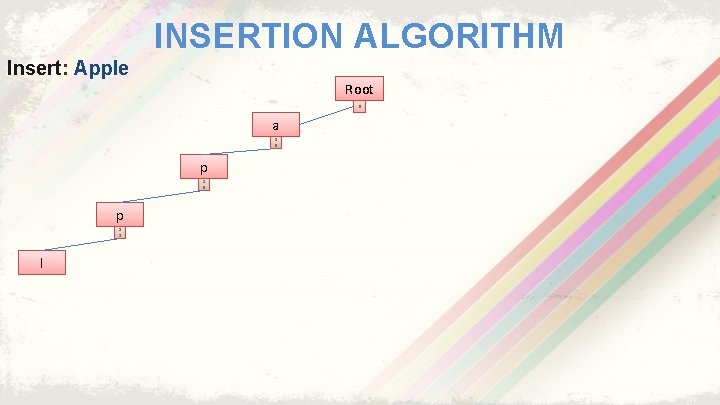
INSERTION ALGORITHM Insert: Apple Root 0 a 1 5 p 1 1 l
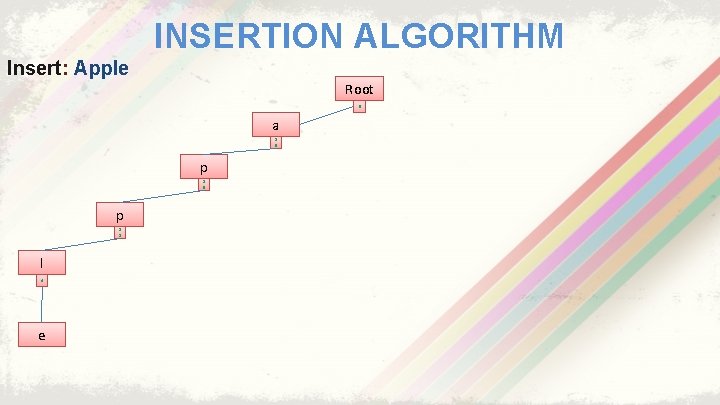
INSERTION ALGORITHM Insert: Apple Root 0 a 1 5 p 1 1 l 4 e
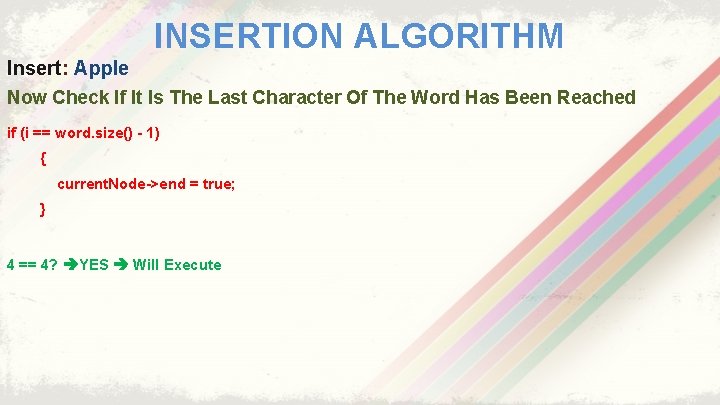
INSERTION ALGORITHM Insert: Apple Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() - 1) { current. Node->end = true; } 4 == 4? YES Will Execute
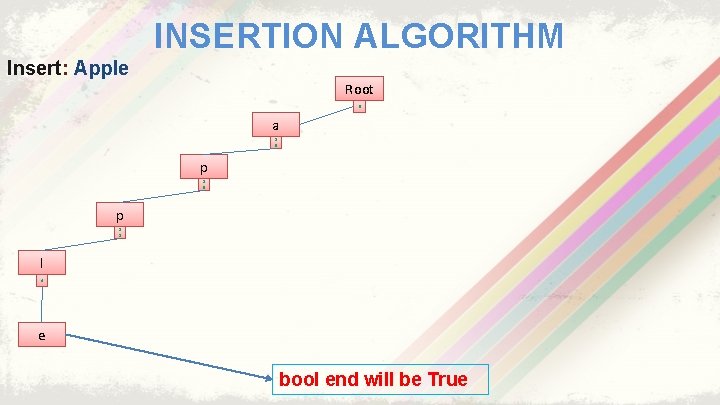
INSERTION ALGORITHM Insert: Apple Root 0 a 1 5 p 1 1 l 4 e bool end will be True
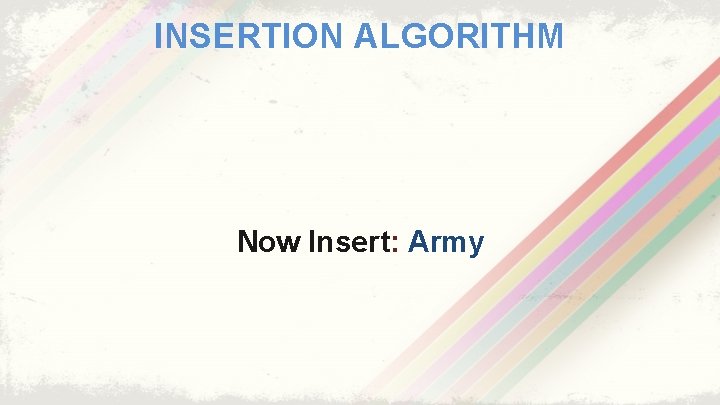
INSERTION ALGORITHM Now Insert: Army
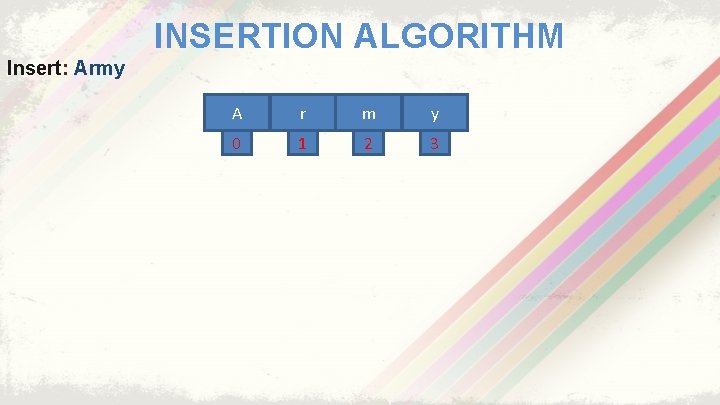
INSERTION ALGORITHM Insert: Army A r m y 0 1 2 3
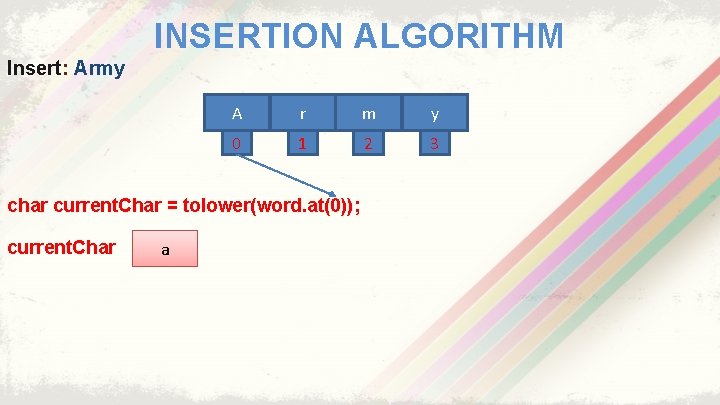
INSERTION ALGORITHM Insert: Army A r m y 0 1 2 3 char current. Char = tolower(word. at(0)); current. Char a
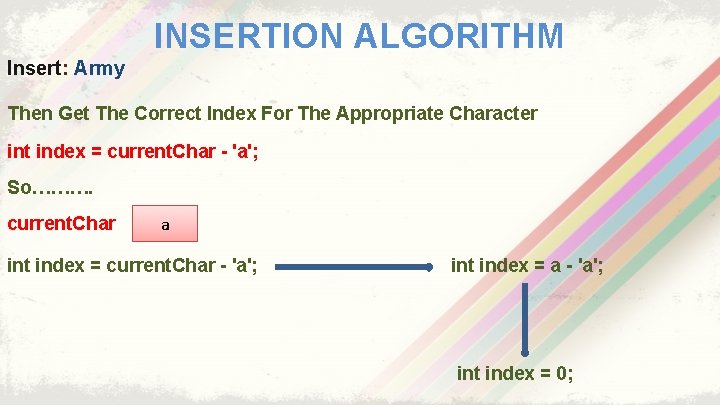
INSERTION ALGORITHM Insert: Army Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char a int index = current. Char - 'a'; int index = a - 'a'; int index = 0;
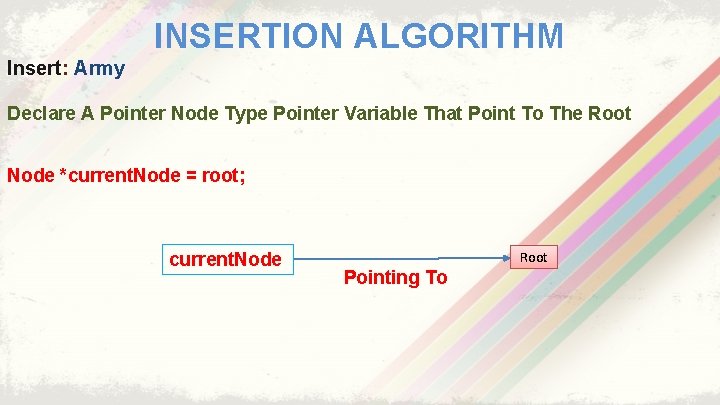
INSERTION ALGORITHM Insert: Army Declare A Pointer Node Type Pointer Variable That Point To The Root Node *current. Node = root; current. Node Root Pointing To
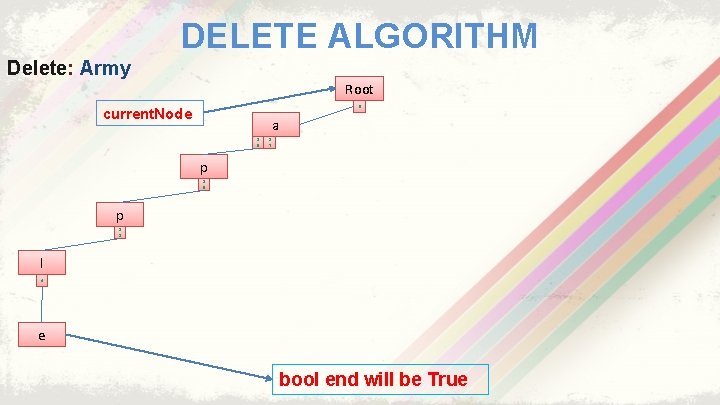
DELETE ALGORITHM Delete: Army Root 0 current. Node a 1 5 1 7 p 1 5 p 1 1 l 4 e bool end will be True
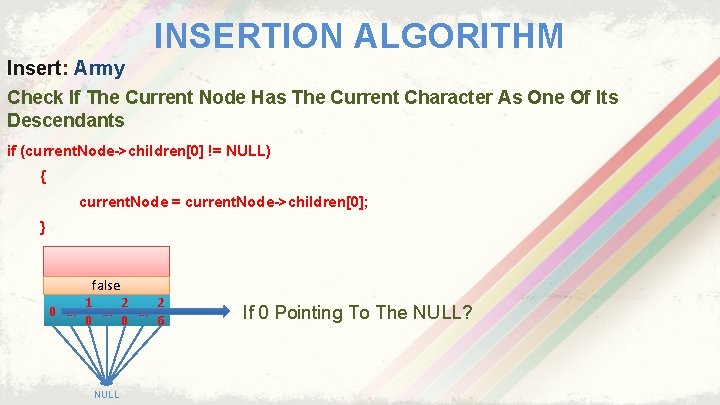
INSERTION ALGORITHM Insert: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } false 1 2 2 0 … … … 0 0 6 NULL If 0 Pointing To The NULL?
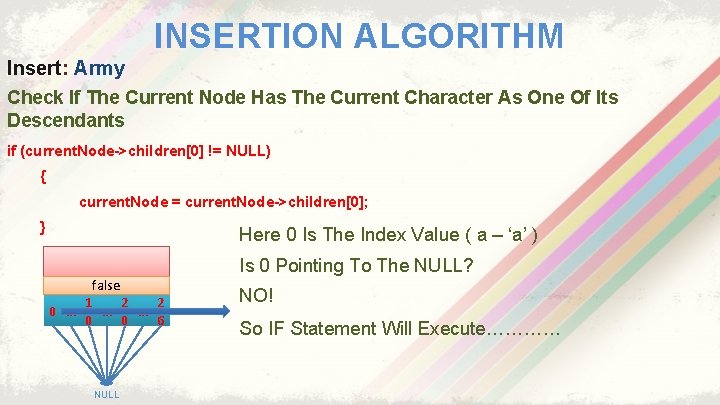
INSERTION ALGORITHM Insert: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } Here 0 Is The Index Value ( a – ‘a’ ) false 1 2 2 0 … … … 0 0 6 NULL Is 0 Pointing To The NULL? NO! So IF Statement Will Execute…………
![INSERTION ALGORITHM Insert Army So current Node current Nodechildren0 INSERTION ALGORITHM Insert: Army So………. current. Node = current. Node->children[0];](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-64.jpg)
INSERTION ALGORITHM Insert: Army So………. current. Node = current. Node->children[0];
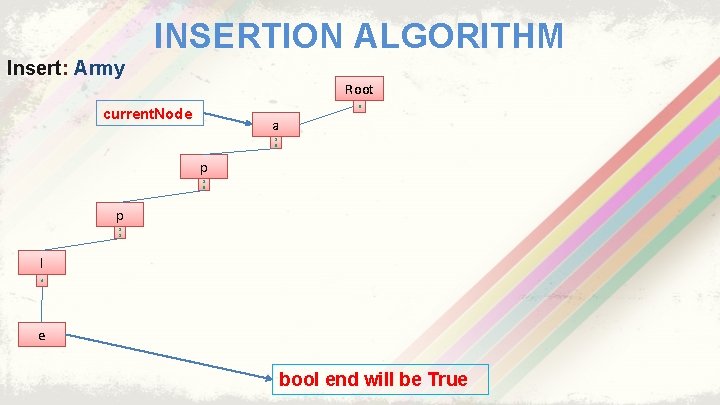
INSERTION ALGORITHM Insert: Army Root 0 current. Node a 1 5 p 1 1 l 4 e bool end will be True
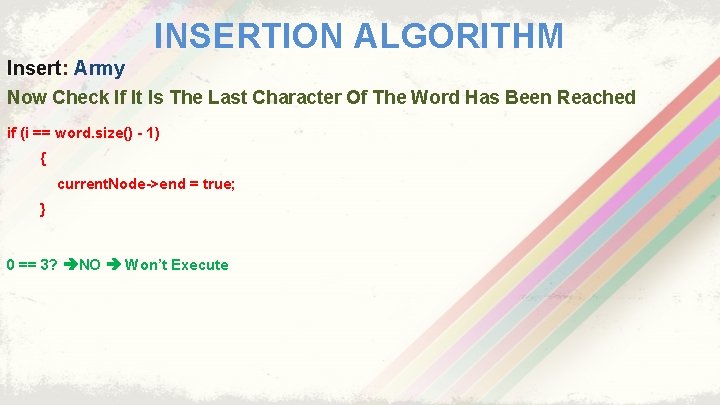
INSERTION ALGORITHM Insert: Army Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() - 1) { current. Node->end = true; } 0 == 3? NO Won’t Execute
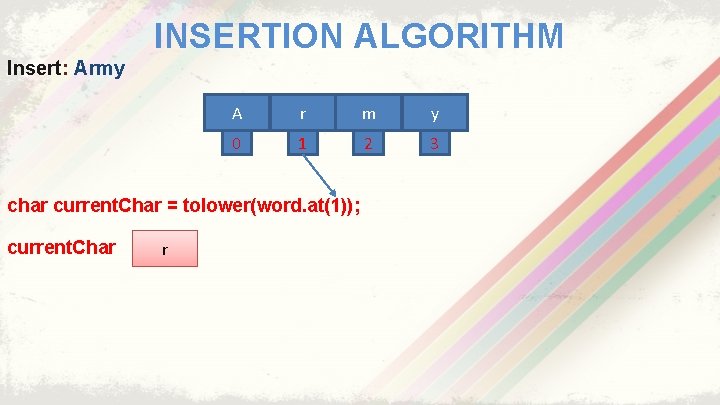
INSERTION ALGORITHM Insert: Army A r m y 0 1 2 3 char current. Char = tolower(word. at(1)); current. Char r
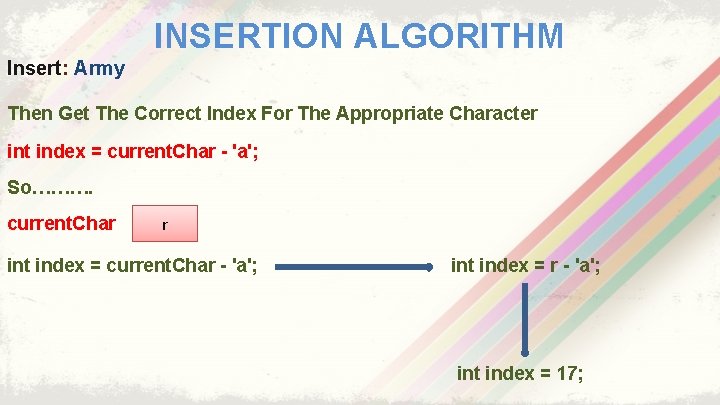
INSERTION ALGORITHM Insert: Army Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char r int index = current. Char - 'a'; int index = 17;
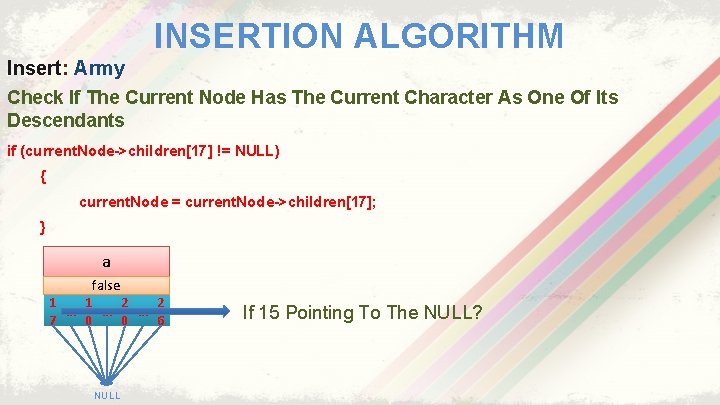
INSERTION ALGORITHM Insert: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[17] != NULL) { current. Node = current. Node->children[17]; } a false 1 2 2 1 … … … 0 0 6 7 NULL If 15 Pointing To The NULL?
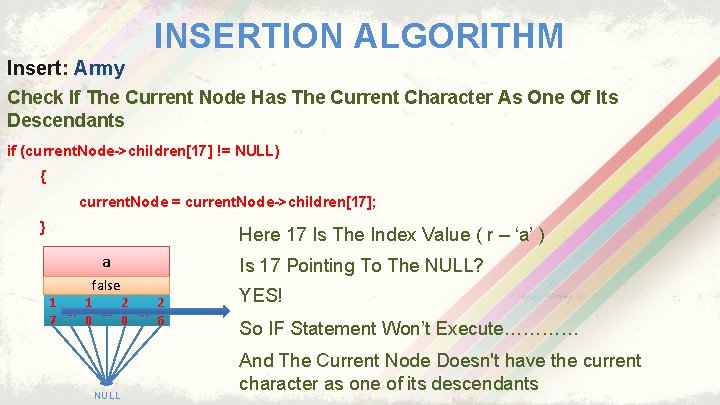
INSERTION ALGORITHM Insert: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[17] != NULL) { current. Node = current. Node->children[17]; } Here 17 Is The Index Value ( r – ‘a’ ) a false 1 2 2 1 … … … 0 0 6 7 NULL Is 17 Pointing To The NULL? YES! So IF Statement Won’t Execute………… And The Current Node Doesn't have the current character as one of its descendants
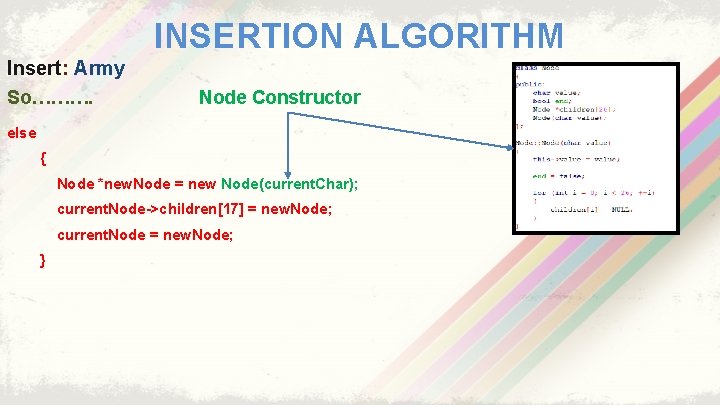
INSERTION ALGORITHM Insert: Army So………. Node Constructor else { Node *new. Node = new Node(current. Char); current. Node->children[17] = new. Node; current. Node = new. Node; }
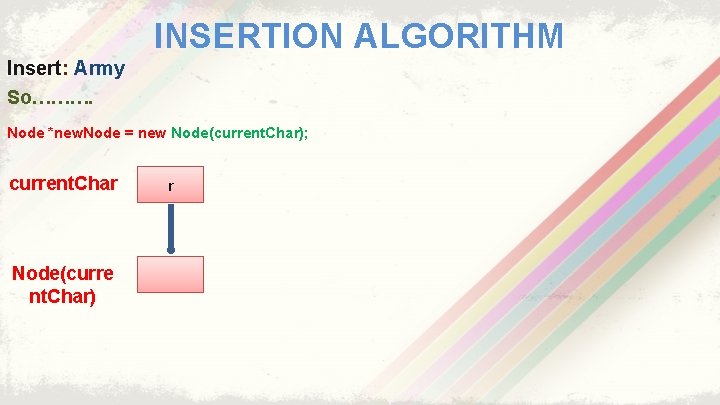
INSERTION ALGORITHM Insert: Army So………. Node *new. Node = new Node(current. Char); current. Char Node(curre nt. Char) r
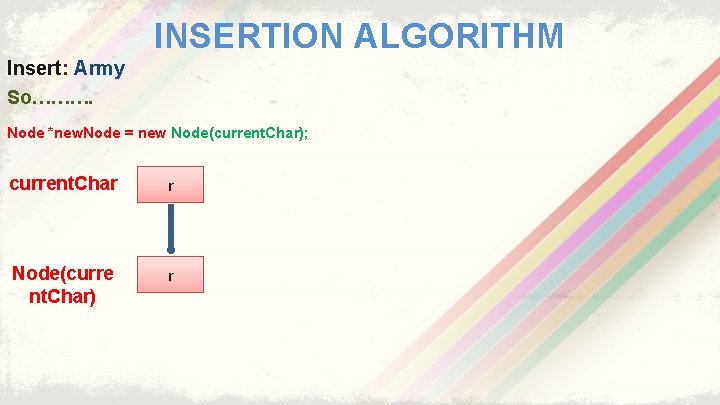
INSERTION ALGORITHM Insert: Army So………. Node *new. Node = new Node(current. Char); current. Char r Node(curre nt. Char) r
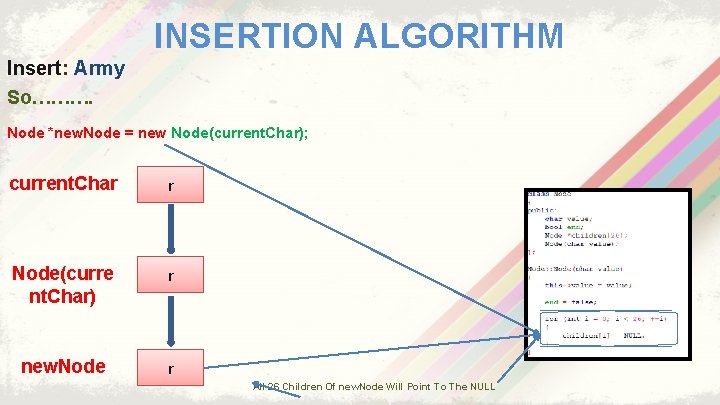
INSERTION ALGORITHM Insert: Army So………. Node *new. Node = new Node(current. Char); current. Char r Node(curre nt. Char) r new. Node r All 26 Children Of new. Node Will Point To The NULL
![INSERTION ALGORITHM Insert Army So current Nodechildren17 new Node INSERTION ALGORITHM Insert: Army So………. current. Node->children[17] = new. Node;](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-75.jpg)
INSERTION ALGORITHM Insert: Army So………. current. Node->children[17] = new. Node;
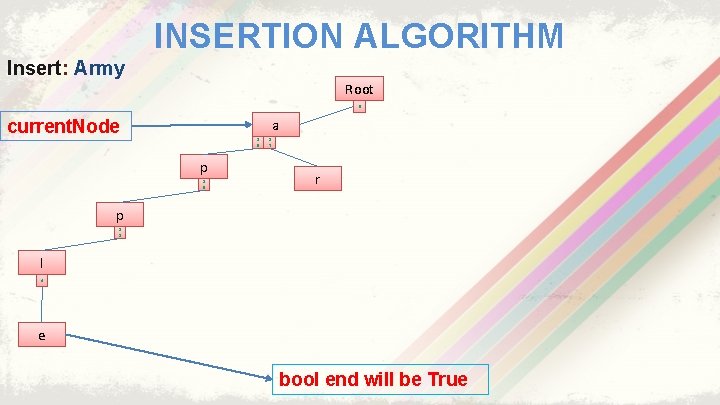
INSERTION ALGORITHM Insert: Army Root 0 a current. Node 1 5 p 1 5 1 7 r p 1 1 l 4 e bool end will be True
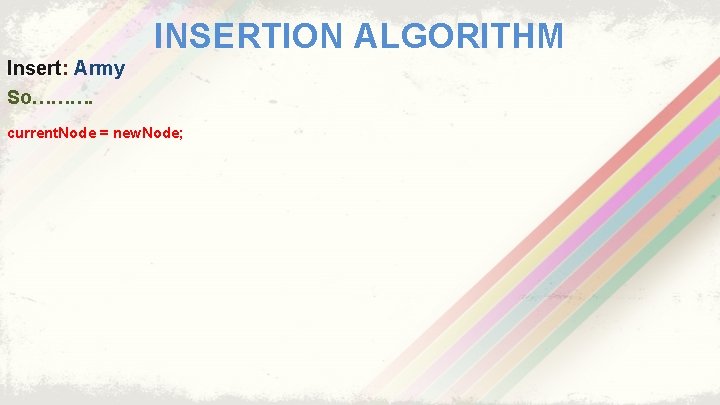
INSERTION ALGORITHM Insert: Army So………. current. Node = new. Node;
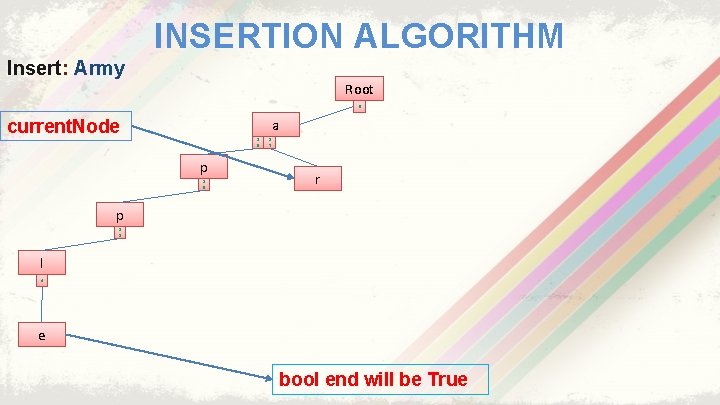
INSERTION ALGORITHM Insert: Army Root 0 a current. Node 1 5 p 1 5 1 7 r p 1 1 l 4 e bool end will be True
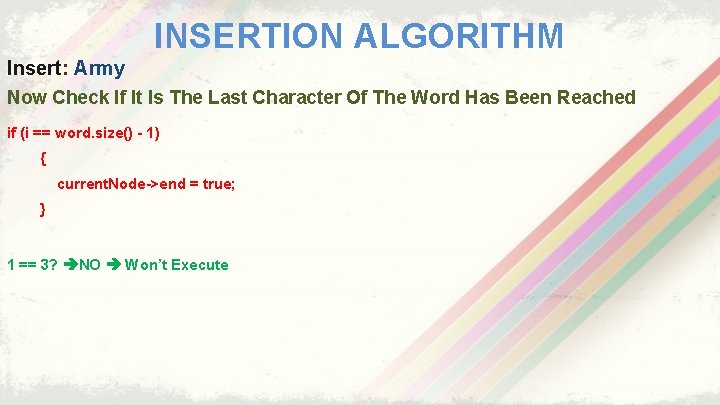
INSERTION ALGORITHM Insert: Army Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() - 1) { current. Node->end = true; } 1 == 3? NO Won’t Execute
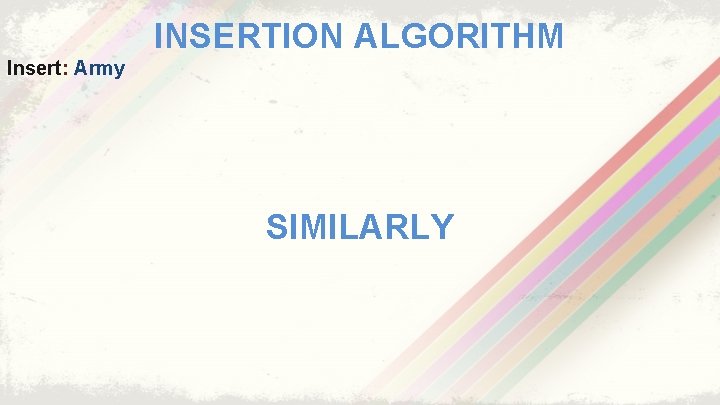
INSERTION ALGORITHM Insert: Army SIMILARLY
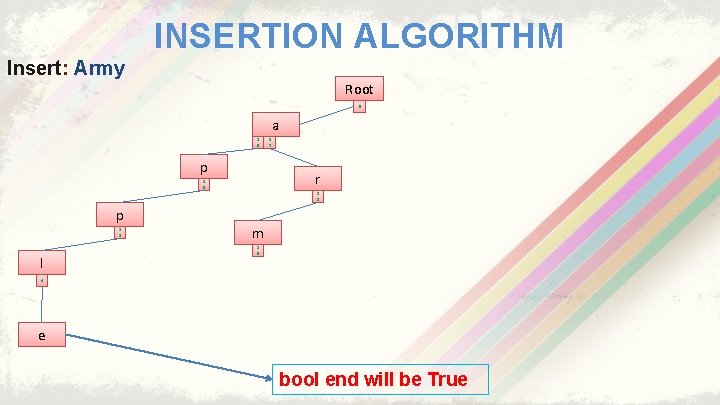
INSERTION ALGORITHM Insert: Army Root 0 a 1 5 p r 1 5 p 1 1 l 1 7 1 2 m 1 5 4 e bool end will be True
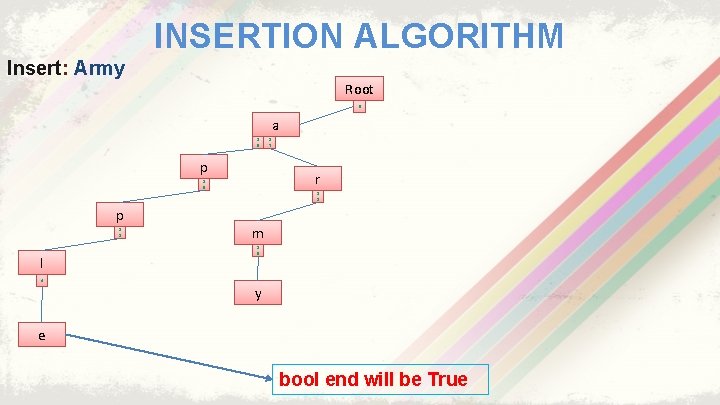
INSERTION ALGORITHM Insert: Army Root 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
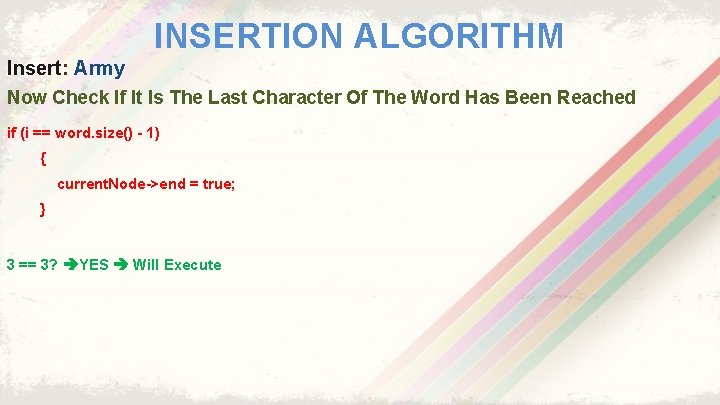
INSERTION ALGORITHM Insert: Army Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() - 1) { current. Node->end = true; } 3 == 3? YES Will Execute
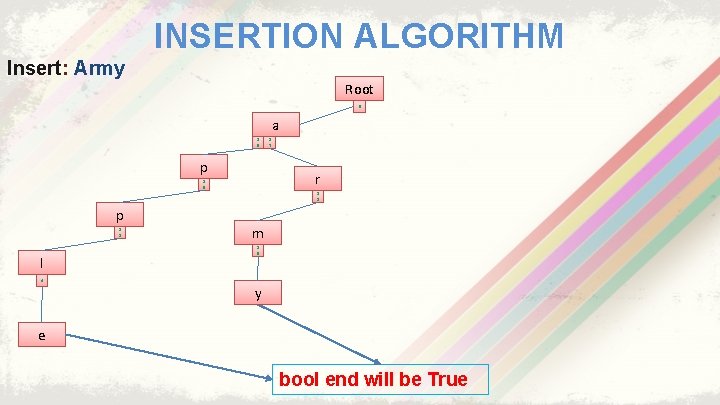
INSERTION ALGORITHM Insert: Army Root 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
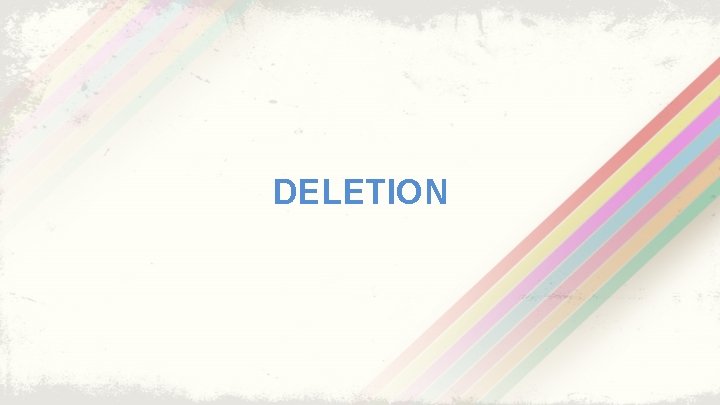
DELETION
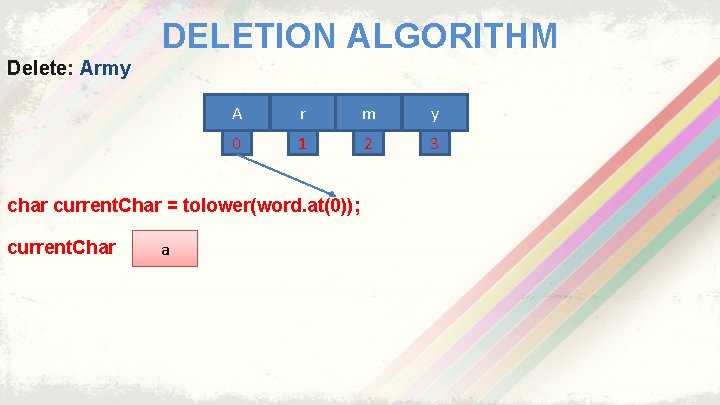
DELETION ALGORITHM Delete: Army A r m y 0 1 2 3 char current. Char = tolower(word. at(0)); current. Char a
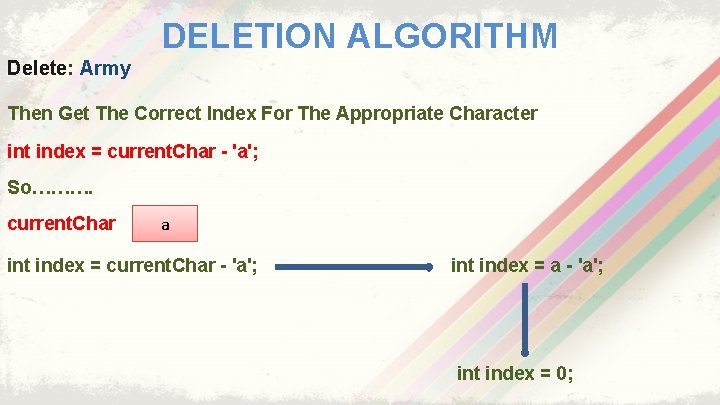
DELETION ALGORITHM Delete: Army Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char a int index = current. Char - 'a'; int index = a - 'a'; int index = 0;
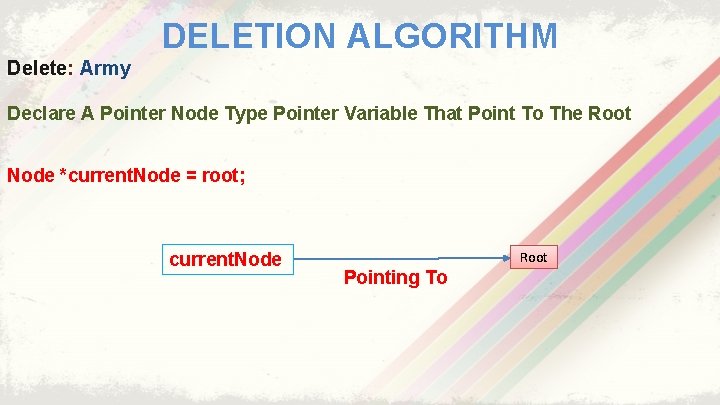
DELETION ALGORITHM Delete: Army Declare A Pointer Node Type Pointer Variable That Point To The Root Node *current. Node = root; current. Node Root Pointing To
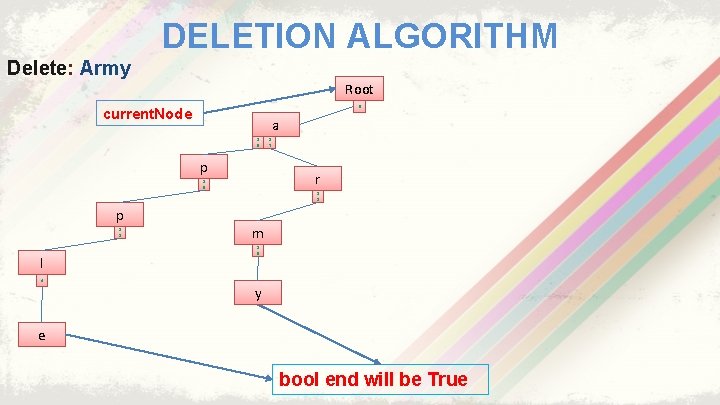
DELETION ALGORITHM Delete: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
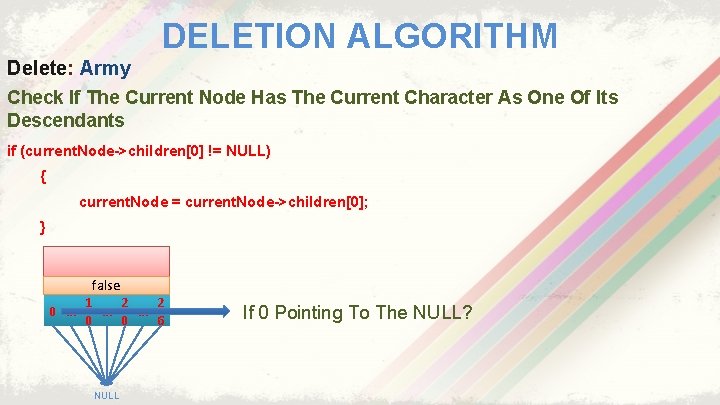
DELETION ALGORITHM Delete: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } false 1 2 2 0 … … … 0 0 6 NULL If 0 Pointing To The NULL?
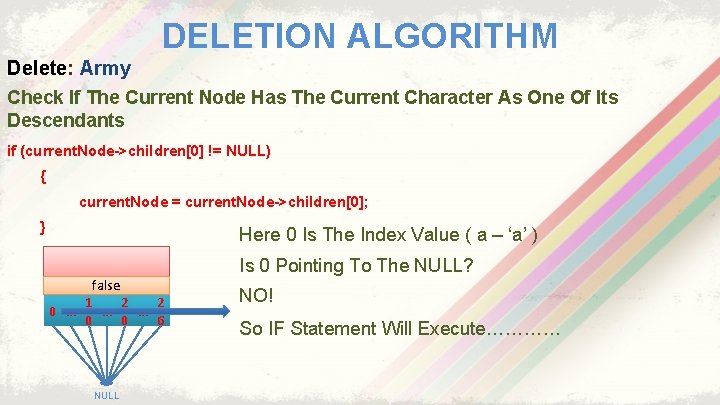
DELETION ALGORITHM Delete: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } Here 0 Is The Index Value ( a – ‘a’ ) false 1 2 2 0 … … … 0 0 6 NULL Is 0 Pointing To The NULL? NO! So IF Statement Will Execute…………
![DELETION ALGORITHM Delete Army So current Node current Nodechildren0 DELETION ALGORITHM Delete: Army So………. current. Node = current. Node->children[0];](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-92.jpg)
DELETION ALGORITHM Delete: Army So………. current. Node = current. Node->children[0];
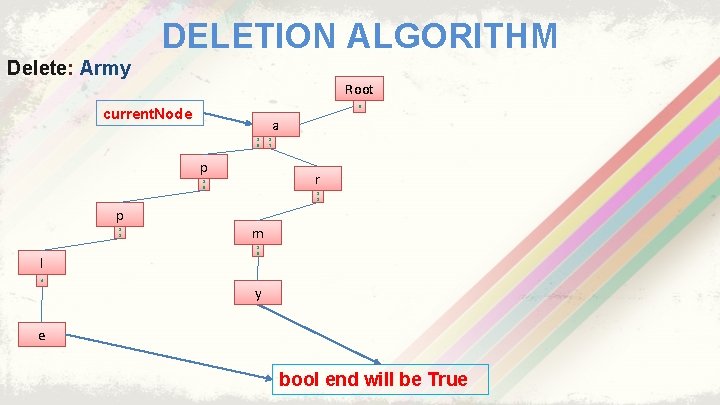
DELETION ALGORITHM Delete: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
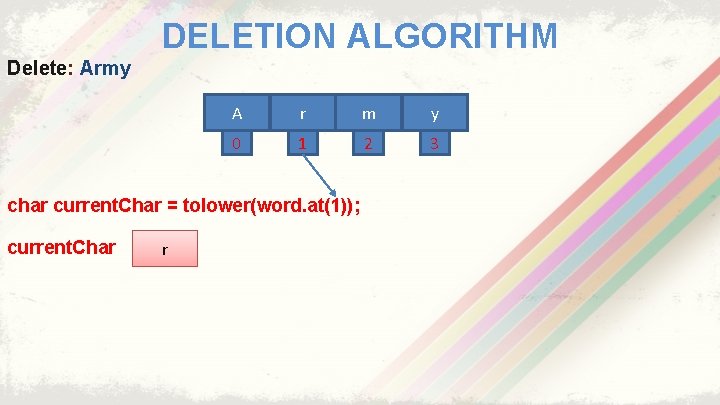
DELETION ALGORITHM Delete: Army A r m y 0 1 2 3 char current. Char = tolower(word. at(1)); current. Char r
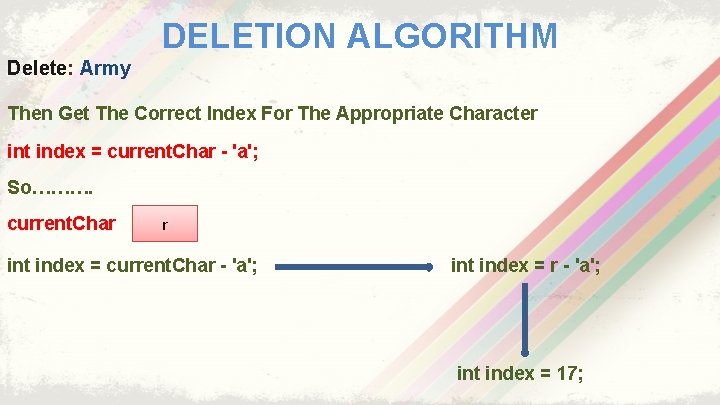
DELETION ALGORITHM Delete: Army Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char r int index = current. Char - 'a'; int index = 17;
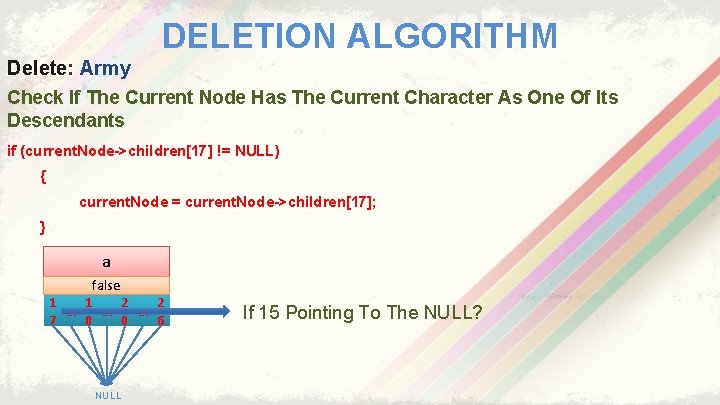
DELETION ALGORITHM Delete: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[17] != NULL) { current. Node = current. Node->children[17]; } a false 1 2 2 1 … … … 0 0 6 7 NULL If 15 Pointing To The NULL?
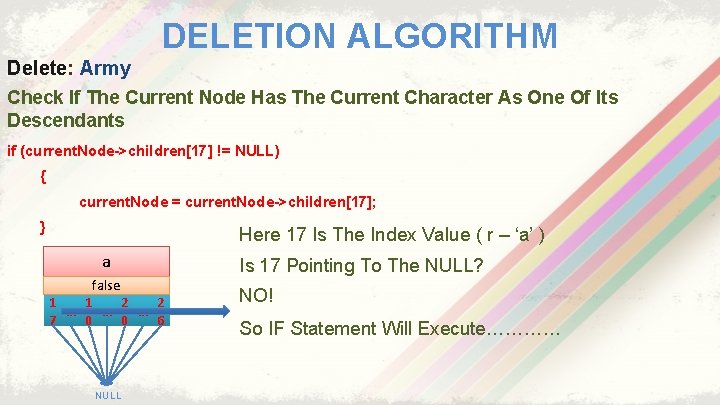
DELETION ALGORITHM Delete: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[17] != NULL) { current. Node = current. Node->children[17]; } Here 17 Is The Index Value ( r – ‘a’ ) a false 1 2 2 1 … … … 0 0 6 7 NULL Is 17 Pointing To The NULL? NO! So IF Statement Will Execute…………
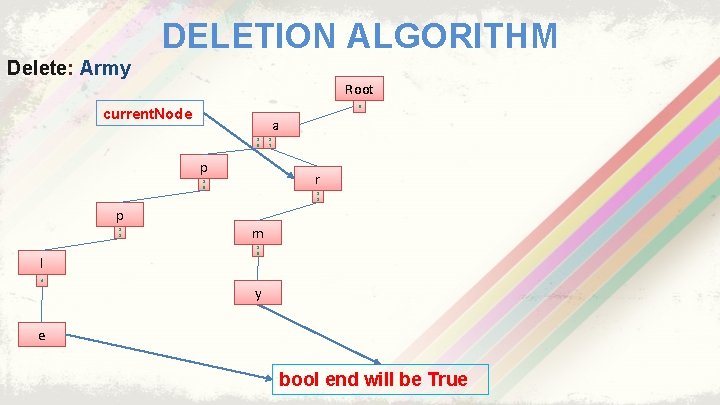
DELETION ALGORITHM Delete: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
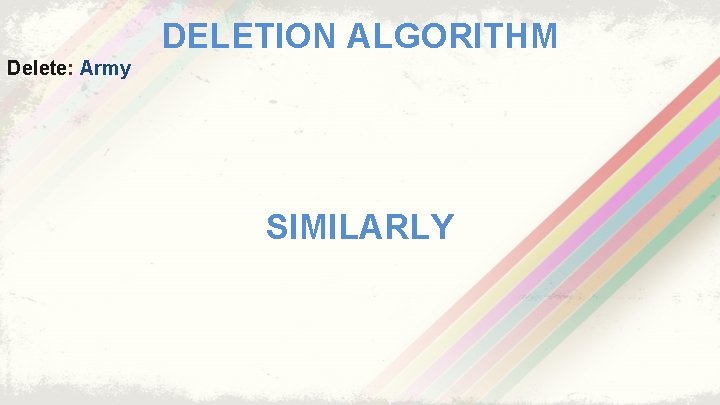
DELETION ALGORITHM Delete: Army SIMILARLY
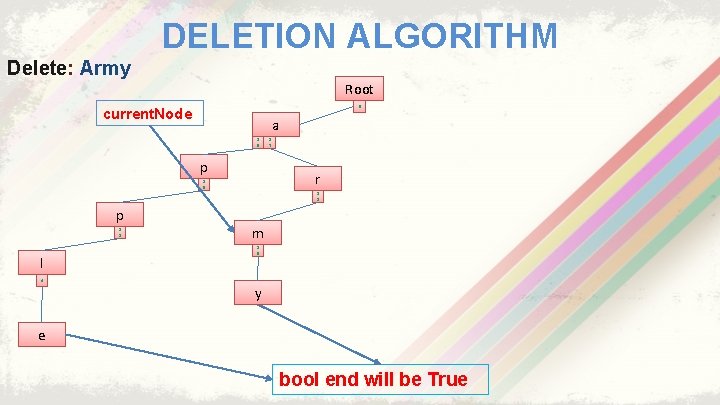
DELETION ALGORITHM Delete: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
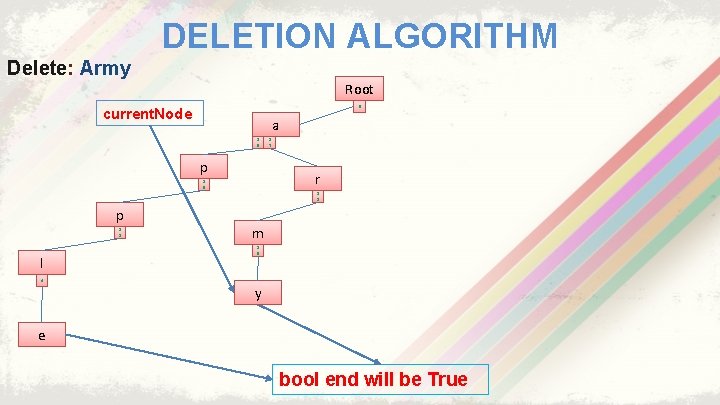
DELETION ALGORITHM Delete: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
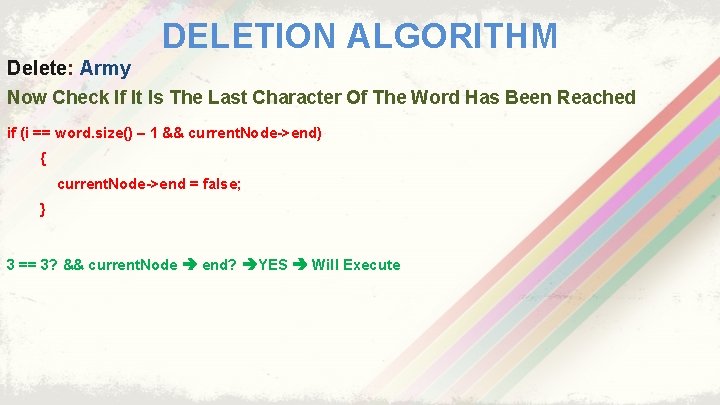
DELETION ALGORITHM Delete: Army Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() – 1 && current. Node->end) { current. Node->end = false; } 3 == 3? && current. Node end? YES Will Execute
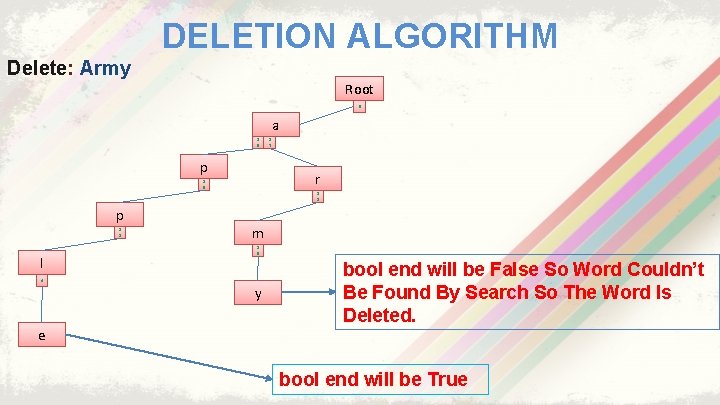
DELETION ALGORITHM Delete: Army Root 0 a 1 5 p r 1 5 p 1 1 l 4 e 1 7 1 2 m 1 5 y bool end will be False So Word Couldn’t Be Found By Search So The Word Is Deleted. bool end will be True
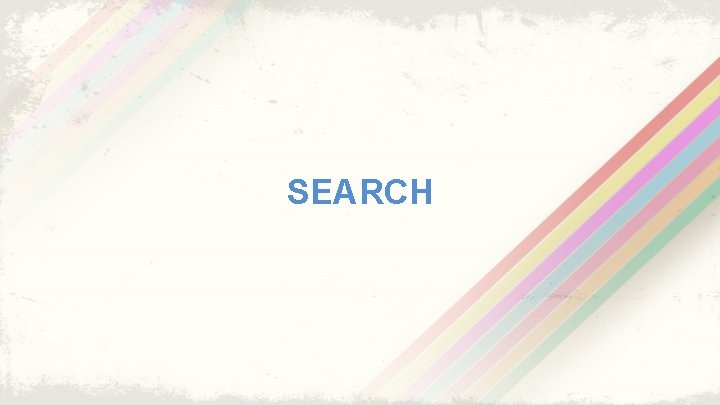
SEARCH
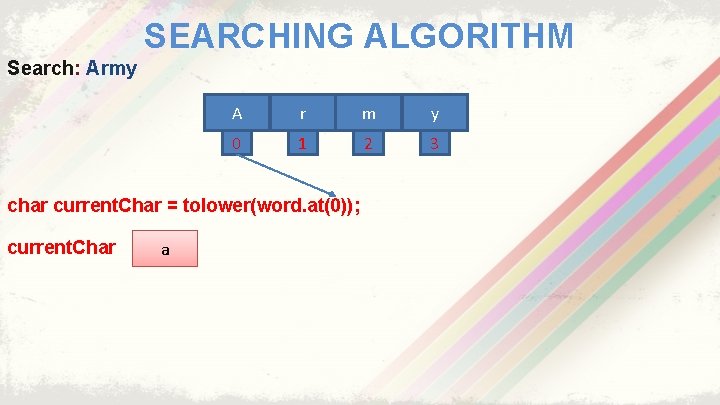
SEARCHING ALGORITHM Search: Army A r m y 0 1 2 3 char current. Char = tolower(word. at(0)); current. Char a
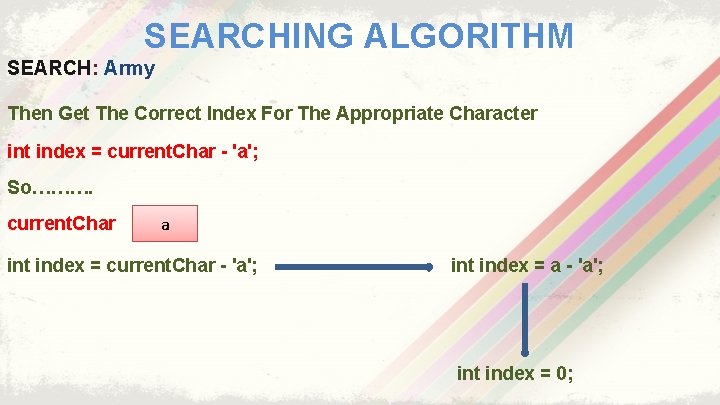
SEARCHING ALGORITHM SEARCH: Army Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char a int index = current. Char - 'a'; int index = a - 'a'; int index = 0;
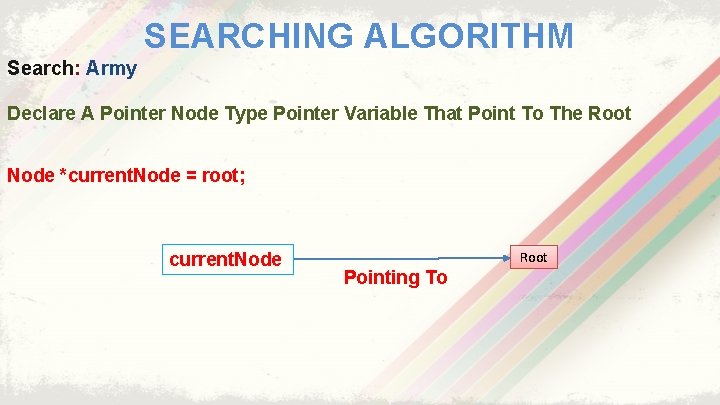
SEARCHING ALGORITHM Search: Army Declare A Pointer Node Type Pointer Variable That Point To The Root Node *current. Node = root; current. Node Root Pointing To
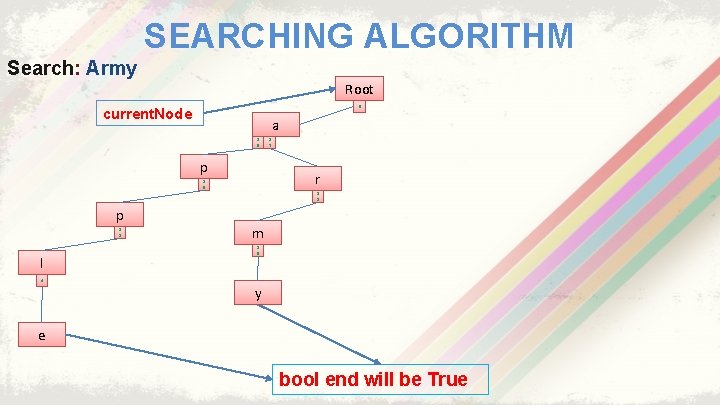
SEARCHING ALGORITHM Search: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
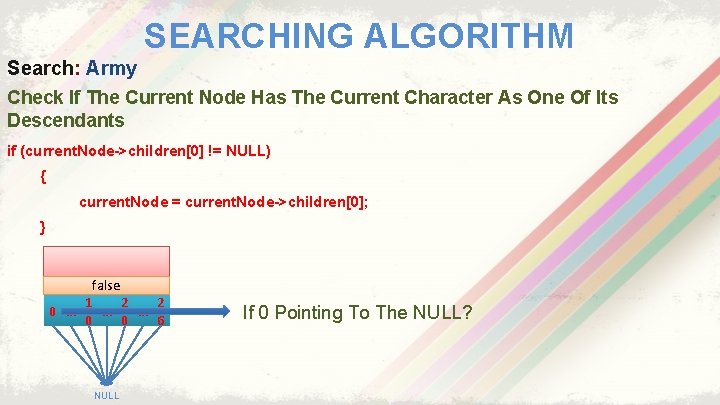
SEARCHING ALGORITHM Search: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } false 1 2 2 0 … … … 0 0 6 NULL If 0 Pointing To The NULL?
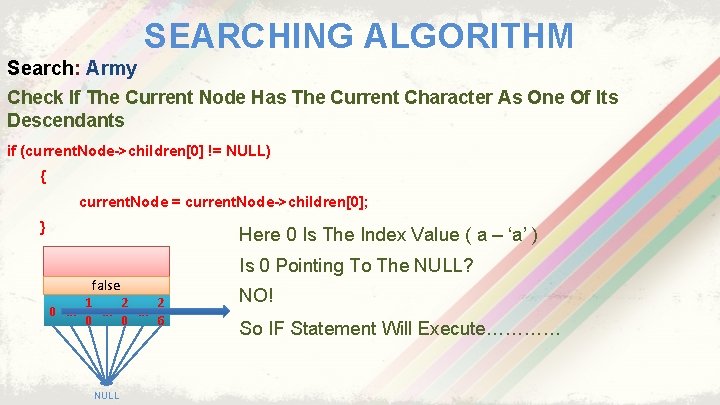
SEARCHING ALGORITHM Search: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[0] != NULL) { current. Node = current. Node->children[0]; } Here 0 Is The Index Value ( a – ‘a’ ) false 1 2 2 0 … … … 0 0 6 NULL Is 0 Pointing To The NULL? NO! So IF Statement Will Execute…………
![SEARCHING ALGORITHM Search Army So current Node current Nodechildren0 SEARCHING ALGORITHM Search: Army So………. current. Node = current. Node->children[0];](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-111.jpg)
SEARCHING ALGORITHM Search: Army So………. current. Node = current. Node->children[0];
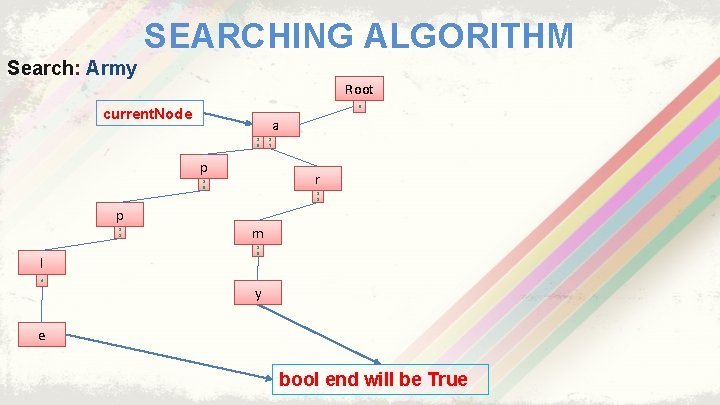
SEARCHING ALGORITHM Search: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
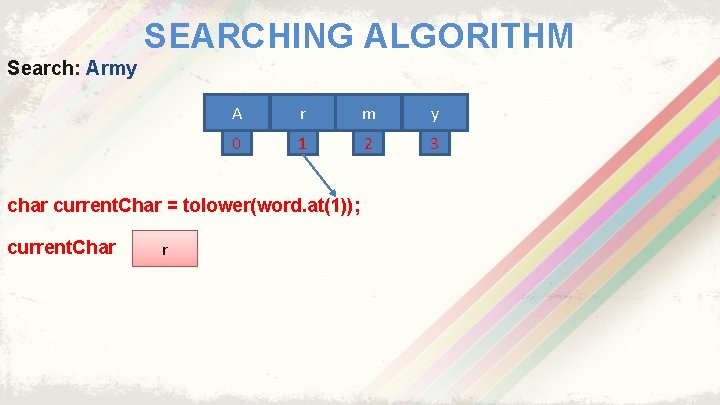
SEARCHING ALGORITHM Search: Army A r m y 0 1 2 3 char current. Char = tolower(word. at(1)); current. Char r
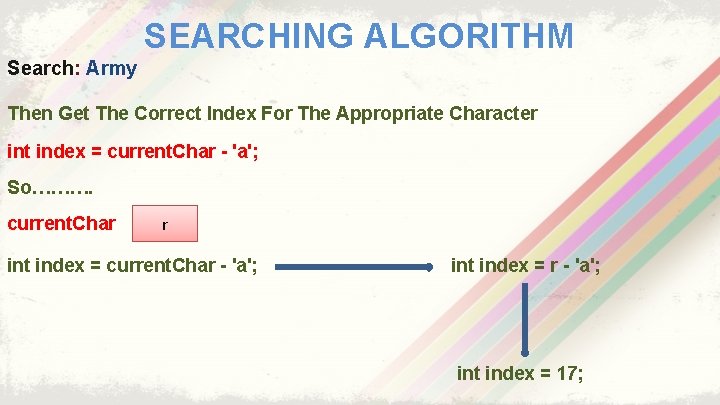
SEARCHING ALGORITHM Search: Army Then Get The Correct Index For The Appropriate Character int index = current. Char - 'a'; So………. current. Char r int index = current. Char - 'a'; int index = 17;
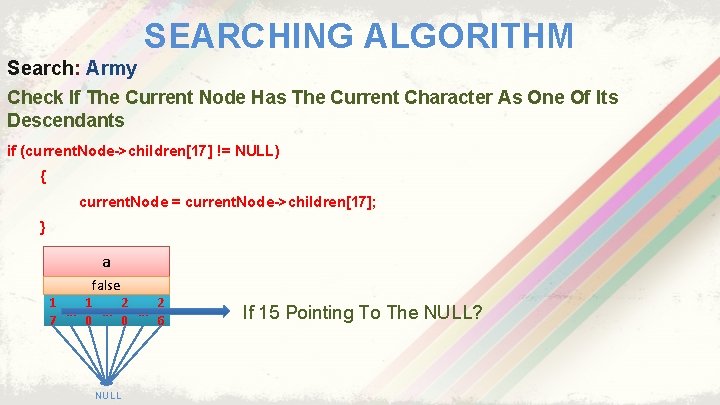
SEARCHING ALGORITHM Search: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[17] != NULL) { current. Node = current. Node->children[17]; } a false 1 2 2 1 … … … 0 0 6 7 NULL If 15 Pointing To The NULL?
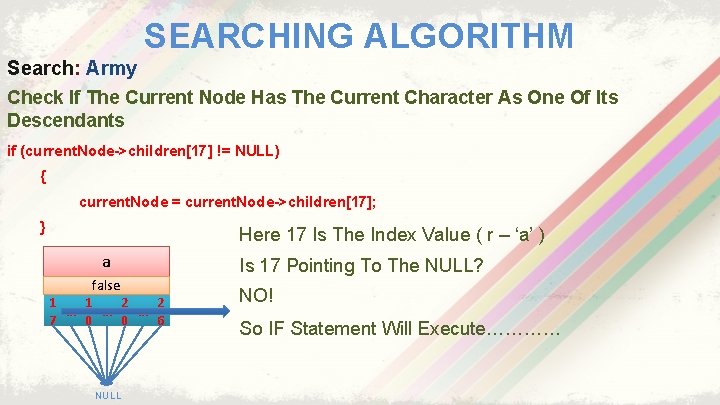
SEARCHING ALGORITHM Search: Army Check If The Current Node Has The Current Character As One Of Its Descendants if (current. Node->children[17] != NULL) { current. Node = current. Node->children[17]; } Here 17 Is The Index Value ( r – ‘a’ ) a false 1 2 2 1 … … … 0 0 6 7 NULL Is 17 Pointing To The NULL? NO! So IF Statement Will Execute…………
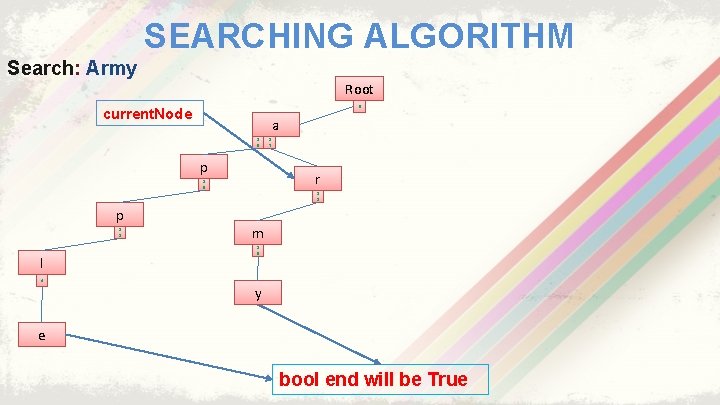
SEARCHING ALGORITHM Search: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
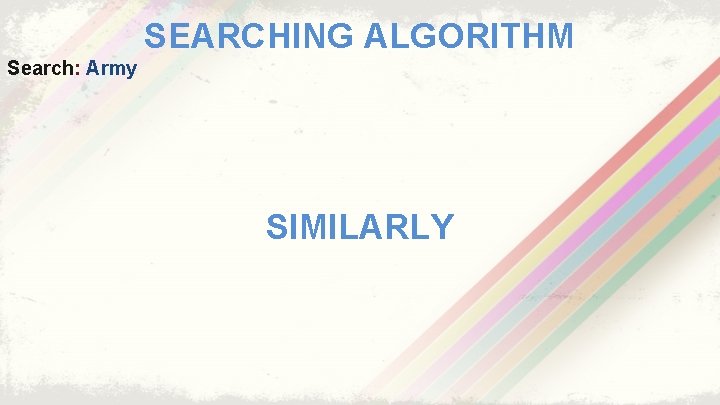
SEARCHING ALGORITHM Search: Army SIMILARLY
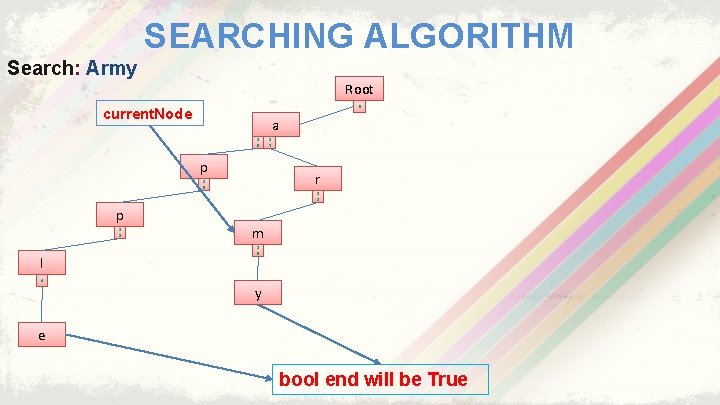
SEARCHING ALGORITHM Search: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
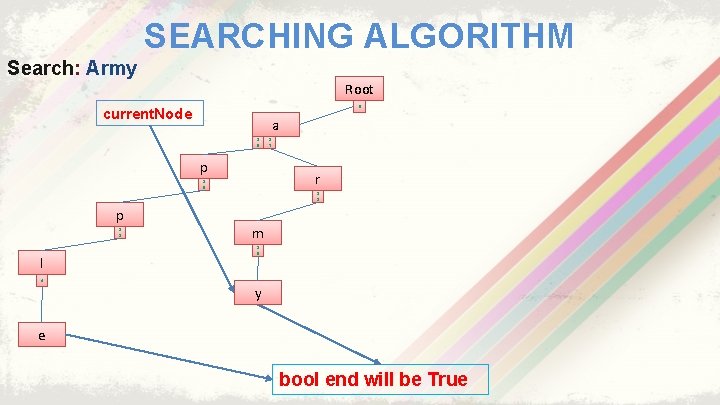
SEARCHING ALGORITHM Search: Army Root 0 current. Node a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
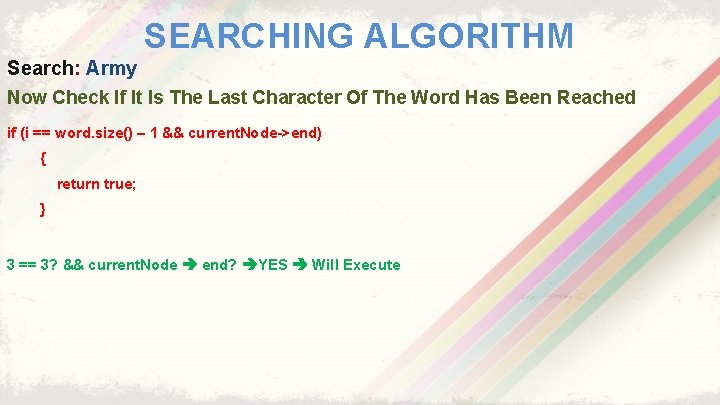
SEARCHING ALGORITHM Search: Army Now Check If It Is The Last Character Of The Word Has Been Reached if (i == word. size() – 1 && current. Node->end) { return true; } 3 == 3? && current. Node end? YES Will Execute
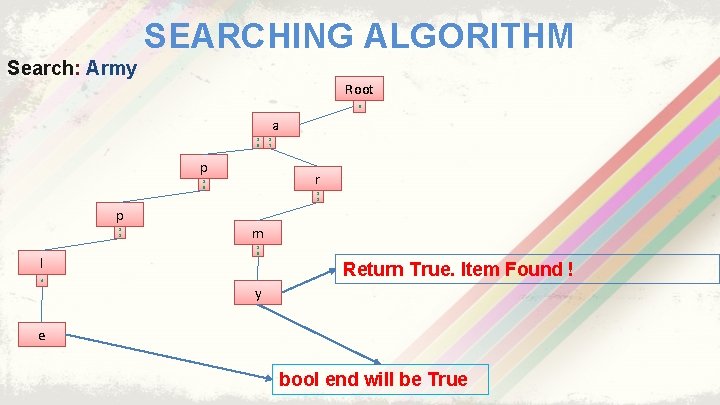
SEARCHING ALGORITHM Search: Army Root 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 Return True. Item Found ! y e bool end will be True
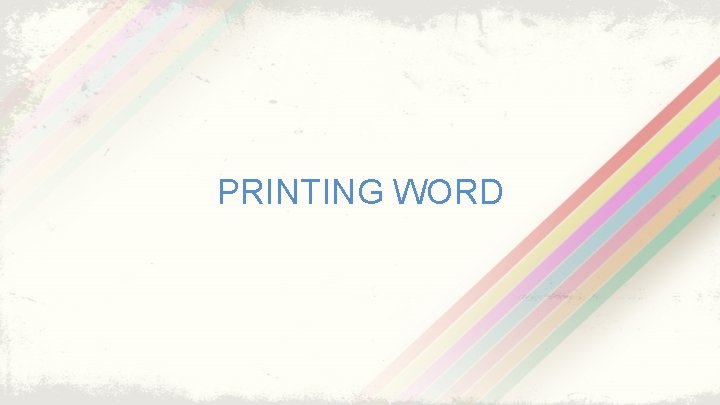
PRINTING WORD
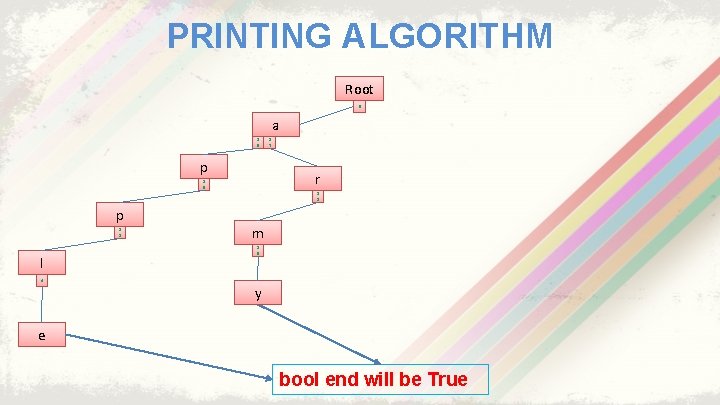
PRINTING ALGORITHM Root 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
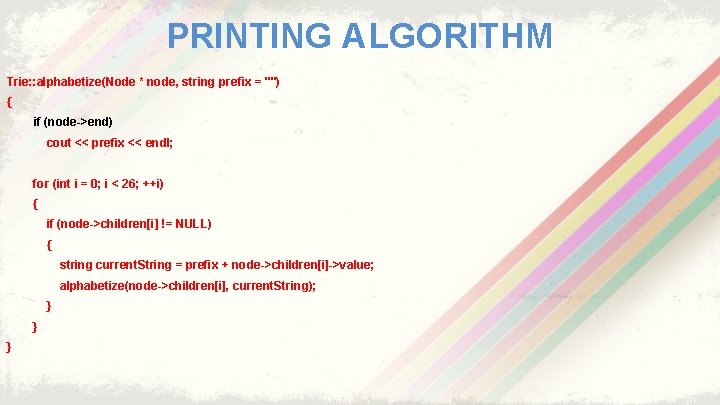
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
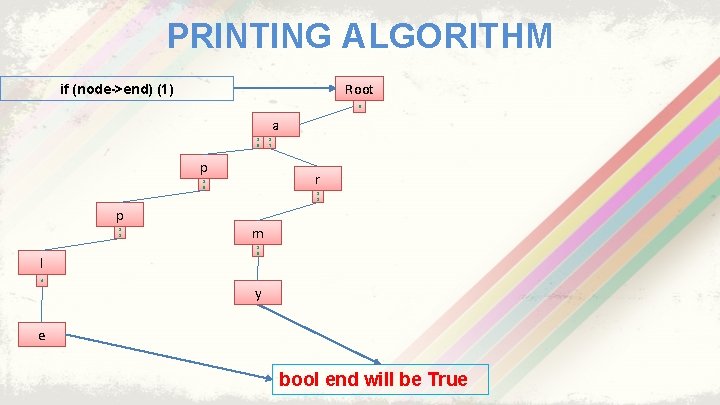
PRINTING ALGORITHM Root if (node->end) (1) 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
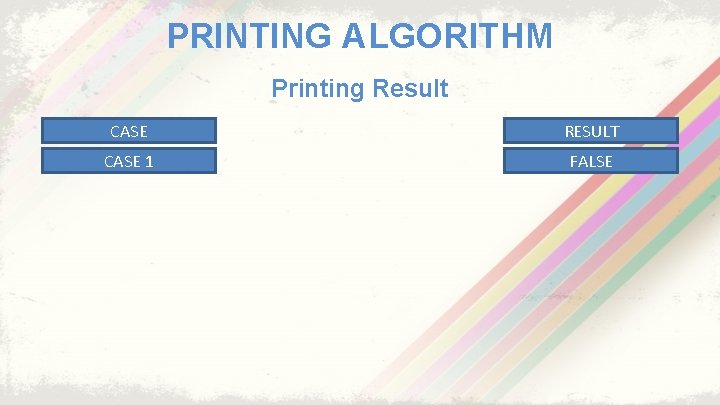
PRINTING ALGORITHM Printing Result CASE RESULT CASE 1 FALSE
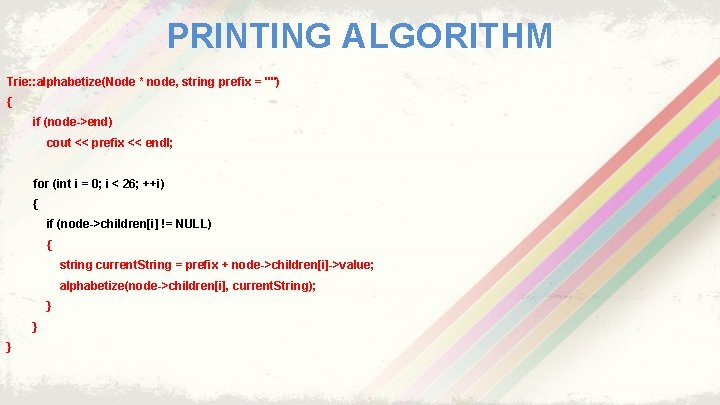
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
![PRINTING ALGORITHM Root if nodechildren0 NULL 2 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[0] != NULL) (2) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-129.jpg)
PRINTING ALGORITHM Root if (node->children[0] != NULL) (2) 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
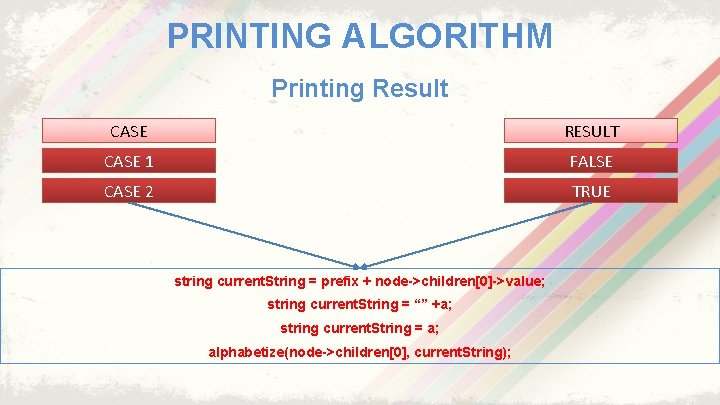
PRINTING ALGORITHM Printing Result CASE RESULT CASE 1 FALSE CASE 2 TRUE string current. String = prefix + node->children[0]->value; string current. String = “” +a; string current. String = a; alphabetize(node->children[0], current. String);
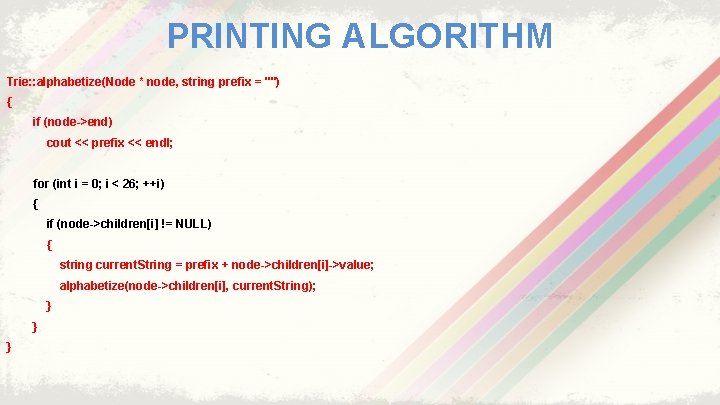
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
![PRINTING ALGORITHM Root if nodechildren1 NULL 3 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[1] != NULL) (3) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-132.jpg)
PRINTING ALGORITHM Root if (node->children[1] != NULL) (3) 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
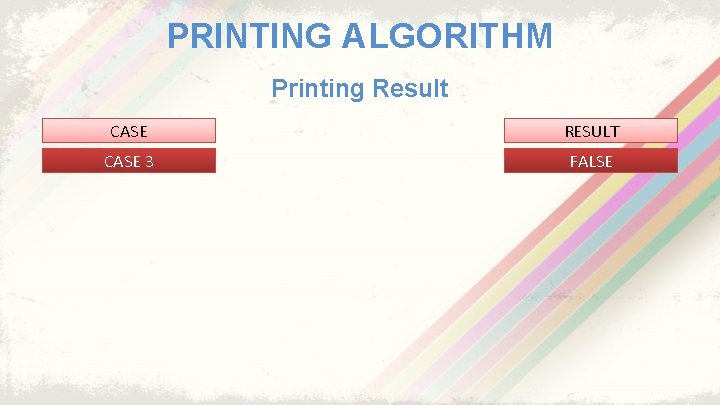
PRINTING ALGORITHM Printing Result CASE RESULT CASE 3 FALSE
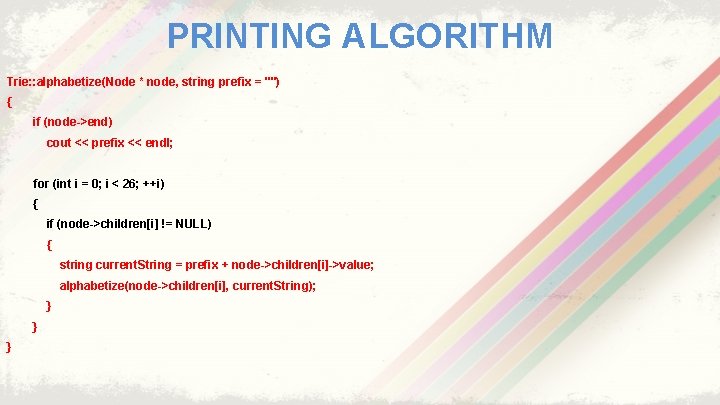
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
![PRINTING ALGORITHM Root if nodechildren2 NULL 4 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[2] != NULL) (4) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-135.jpg)
PRINTING ALGORITHM Root if (node->children[2] != NULL) (4) 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
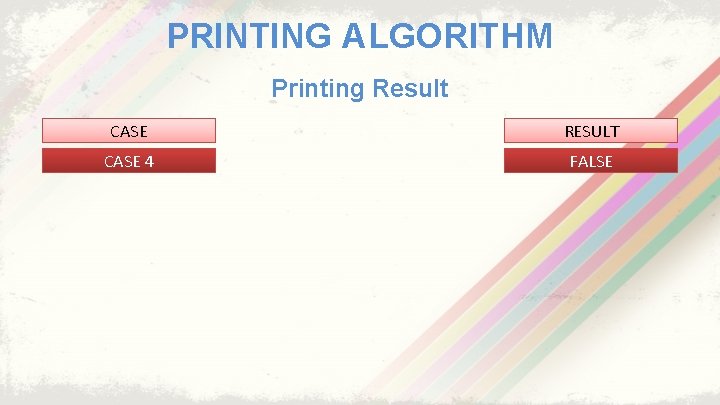
PRINTING ALGORITHM Printing Result CASE RESULT CASE 4 FALSE
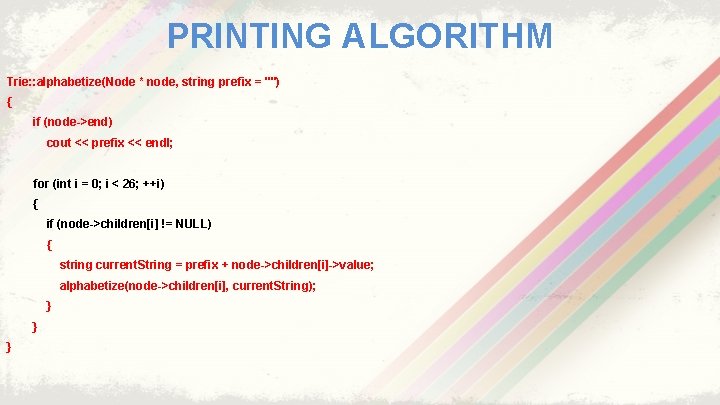
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
![PRINTING ALGORITHM Root if nodechildren15 NULL 17 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[15] != NULL) (17) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-138.jpg)
PRINTING ALGORITHM Root if (node->children[15] != NULL) (17) 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
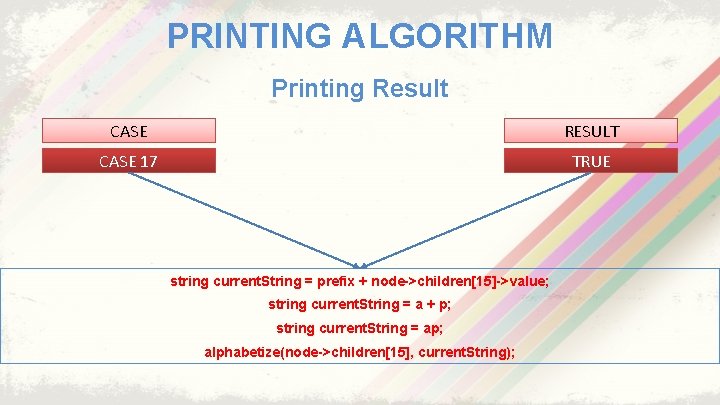
PRINTING ALGORITHM Printing Result CASE RESULT CASE 17 TRUE string current. String = prefix + node->children[15]->value; string current. String = a + p; string current. String = ap; alphabetize(node->children[15], current. String);
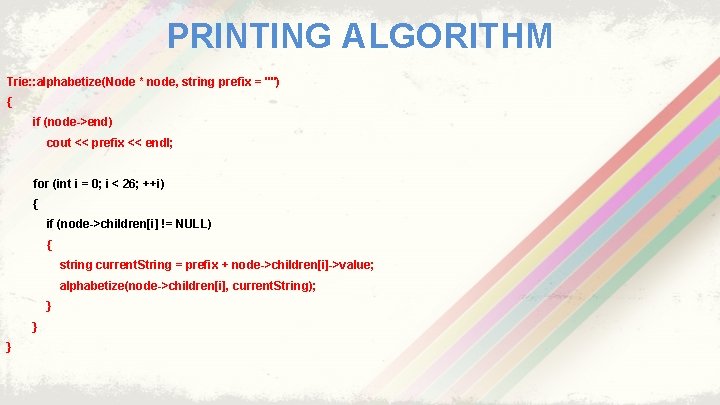
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
![PRINTING ALGORITHM Root if nodechildren15 NULL 18 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[15] != NULL) (18) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-141.jpg)
PRINTING ALGORITHM Root if (node->children[15] != NULL) (18) 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
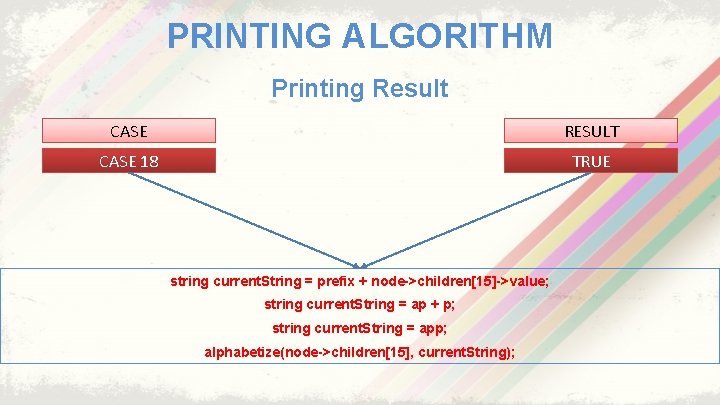
PRINTING ALGORITHM Printing Result CASE RESULT CASE 18 TRUE string current. String = prefix + node->children[15]->value; string current. String = ap + p; string current. String = app; alphabetize(node->children[15], current. String);
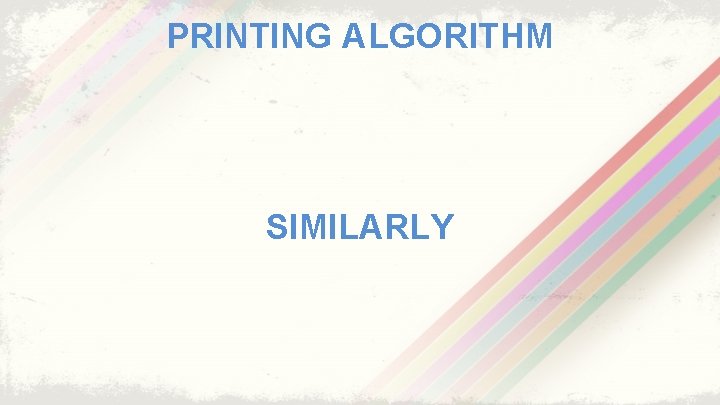
PRINTING ALGORITHM SIMILARLY
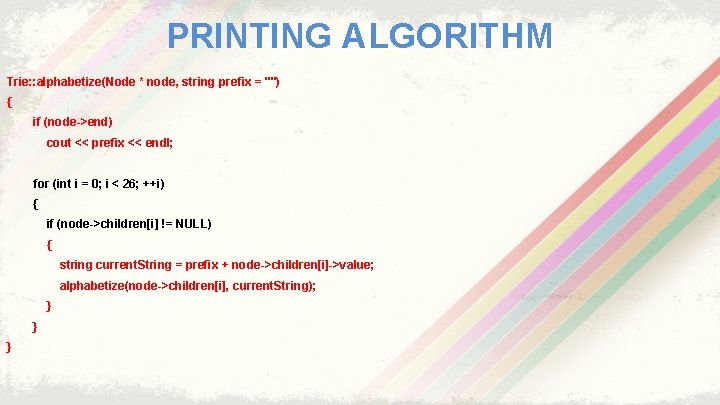
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
![PRINTING ALGORITHM Root if nodechildren4 NULL 19 0 a 1 5 p r PRINTING ALGORITHM Root if (node->children[4] != NULL) (19) 0 a 1 5 p r](https://slidetodoc.com/presentation_image_h2/d3be6cde35146b0f3f5a9fad63a2c91a/image-145.jpg)
PRINTING ALGORITHM Root if (node->children[4] != NULL) (19) 0 a 1 5 p r 1 5 p 1 1 l 4 1 7 1 2 m 1 5 y e bool end will be True
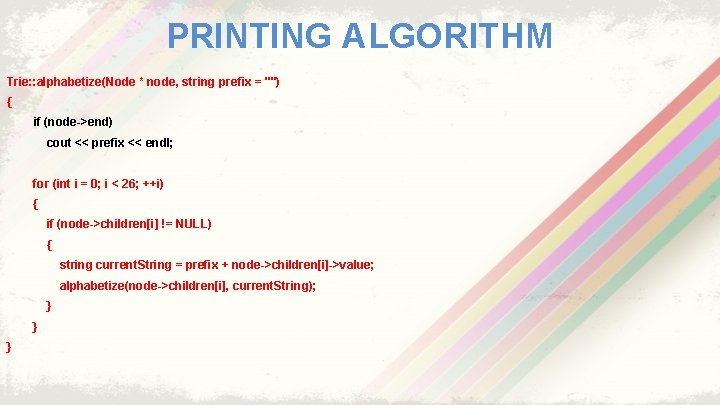
PRINTING ALGORITHM Trie: : alphabetize(Node * node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } }
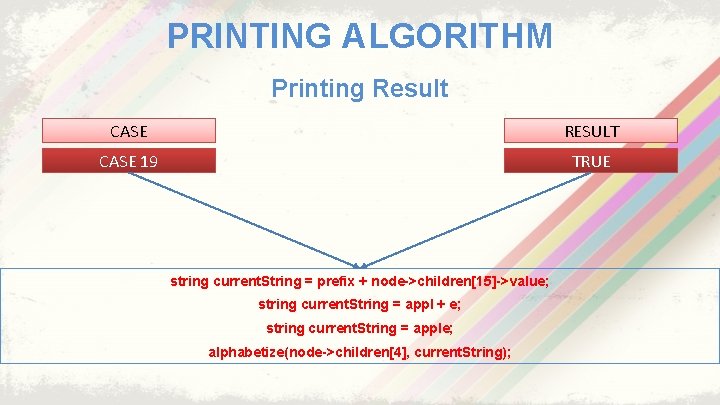
PRINTING ALGORITHM Printing Result CASE RESULT CASE 19 TRUE string current. String = prefix + node->children[15]->value; string current. String = appl + e; string current. String = apple; alphabetize(node->children[4], current. String);
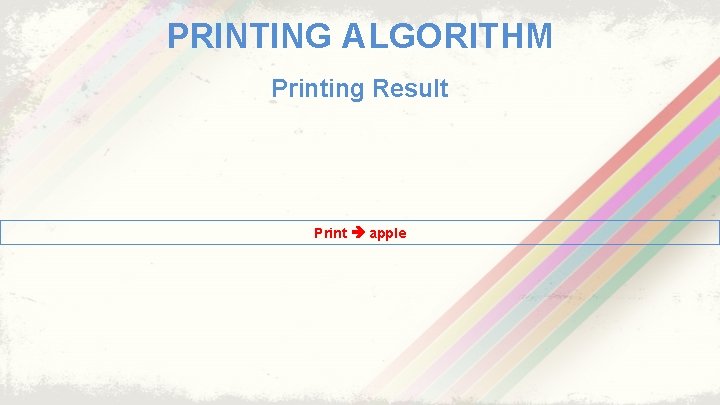
PRINTING ALGORITHM Printing Result Print apple
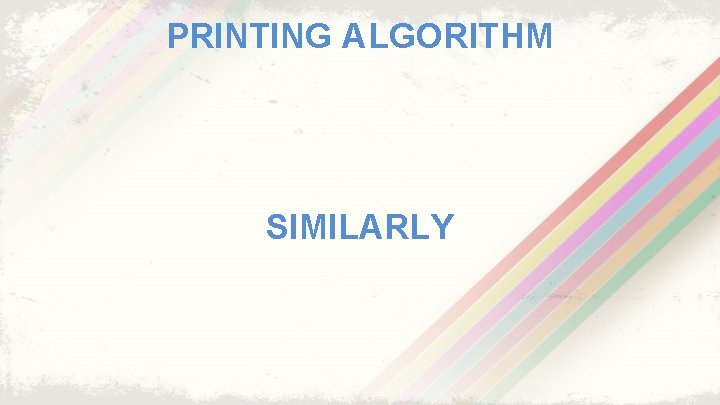
PRINTING ALGORITHM SIMILARLY
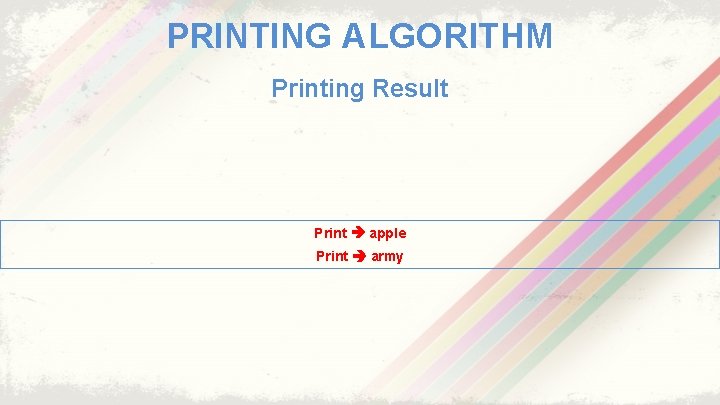
PRINTING ALGORITHM Printing Result Print apple Print army
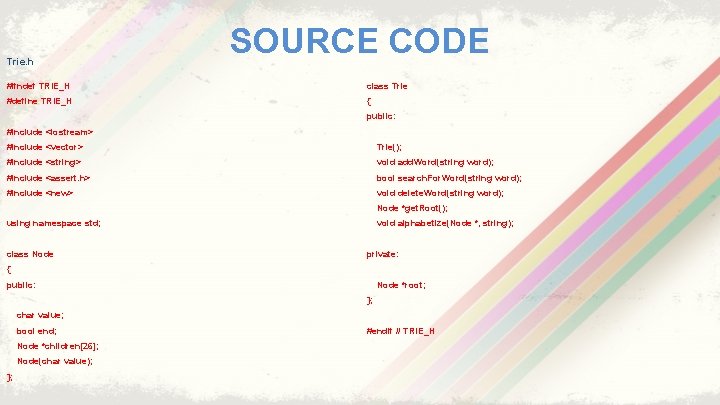
Trie. h SOURCE CODE #ifndef TRIE_H class Trie #define TRIE_H { public: #include <iostream> #include <vector> Trie(); #include <string> void add. Word(string word); #include <assert. h> bool search. For. Word(string word); #include <new> void delete. Word(string word); Node *get. Root(); using namespace std; class Node void alphabetize(Node *, string); private: { public: Node *root; }; char value; bool end; Node *children[26]; Node(char value); }; #endif // TRIE_H
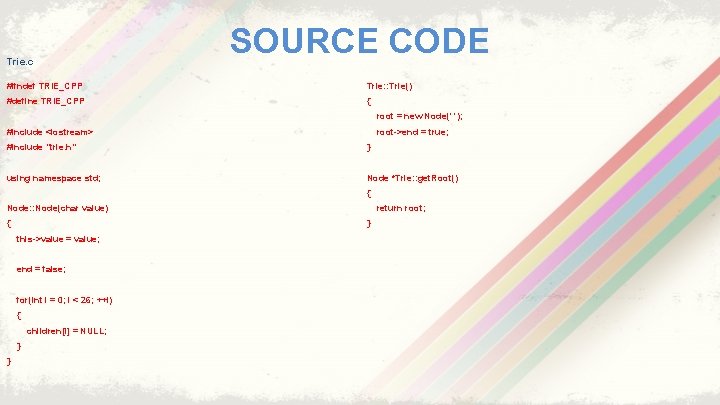
Trie. c SOURCE CODE #ifndef TRIE_CPP Trie: : Trie() #define TRIE_CPP { root = new Node(' '); #include <iostream> root->end = true; #include "trie. h" } using namespace std; Node *Trie: : get. Root() { Node: : Node(char value) { } this->value = value; end = false; for(int i = 0; i < 26; ++i) { children[i] = NULL; } } return root;
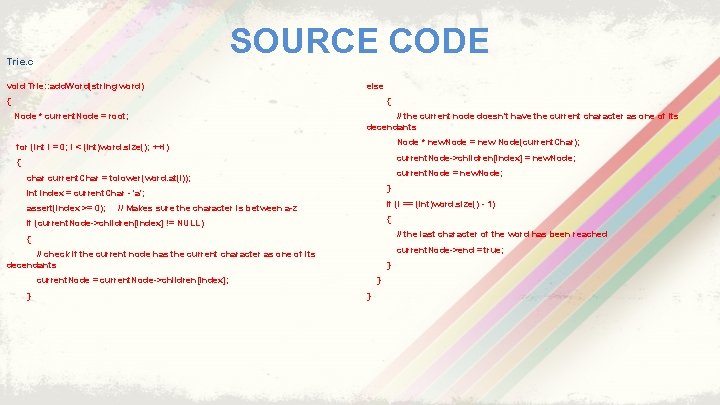
SOURCE CODE Trie. c void Trie: : add. Word(string word) else { { Node * current. Node = root; // the current node doesn't have the current character as one of its decendants Node * new. Node = new Node(current. Char); for (int i = 0; i < (int)word. size(); ++i) current. Node->children[index] = new. Node; { current. Node = new. Node; char current. Char = tolower(word. at(i)); } int index = current. Char - 'a'; assert(index >= 0); if (i == (int)word. size() - 1) // Makes sure the character is between a-z { if (current. Node->children[index] != NULL) // the last character of the word has been reached { current. Node->end = true; // check if the current node has the current character as one of its decendants } current. Node = current. Node->children[index]; } } }
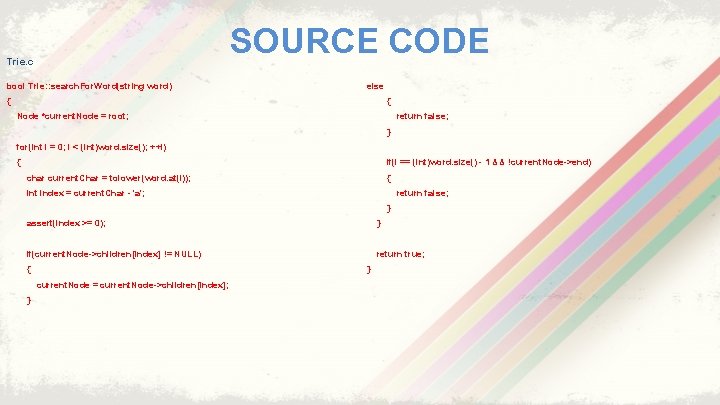
SOURCE CODE Trie. c bool Trie: : search. For. Word(string word) else { { return false; Node *current. Node = root; } for(int i = 0; i < (int)word. size(); ++i) if(i == (int)word. size() - 1 && !current. Node->end) { { char current. Char = tolower(word. at(i)); return false; int index = current. Char - 'a'; } assert(index >= 0); } if(current. Node->children[index] != NULL) return true; } { current. Node = current. Node->children[index]; }
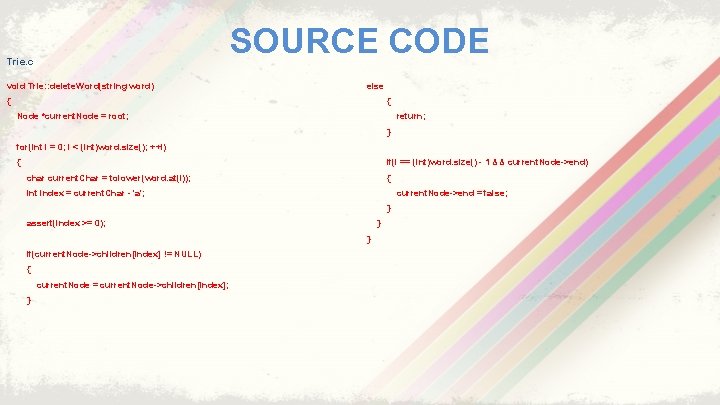
SOURCE CODE Trie. c void Trie: : delete. Word(string word) else { { return; Node *current. Node = root; } for(int i = 0; i < (int)word. size(); ++i) if(i == (int)word. size() - 1 && current. Node->end) { { char current. Char = tolower(word. at(i)); current. Node->end = false; int index = current. Char - 'a'; } } assert(index >= 0); } if(current. Node->children[index] != NULL) { current. Node = current. Node->children[index]; }
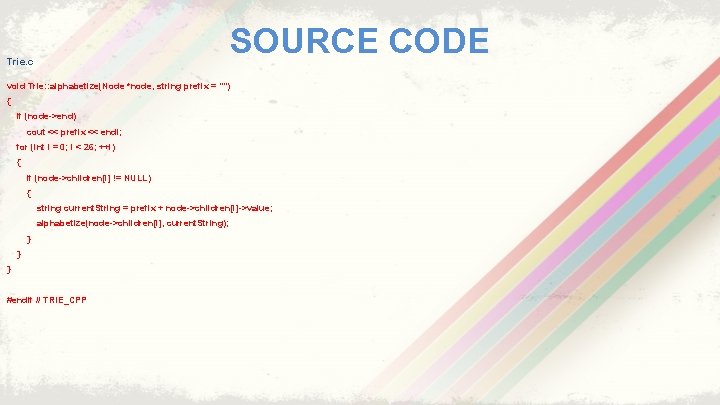
SOURCE CODE Trie. c void Trie: : alphabetize(Node *node, string prefix = "") { if (node->end) cout << prefix << endl; for (int i = 0; i < 26; ++i) { if (node->children[i] != NULL) { string current. String = prefix + node->children[i]->value; alphabetize(node->children[i], current. String); } } } #endif // TRIE_CPP
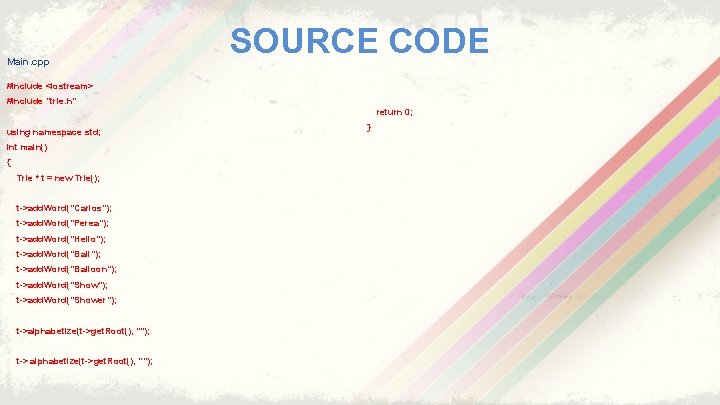
Main. cpp SOURCE CODE #include <iostream> #include “trie. h” return 0; using namespace std; int main() { Trie * t = new Trie(); t->add. Word("Carlos"); t->add. Word("Perea"); t->add. Word("Hello"); t->add. Word("Balloon"); t->add. Word("Shower"); t->alphabetize(t->get. Root(), ""); t-> alphabetize(t->get. Root(), ""); }
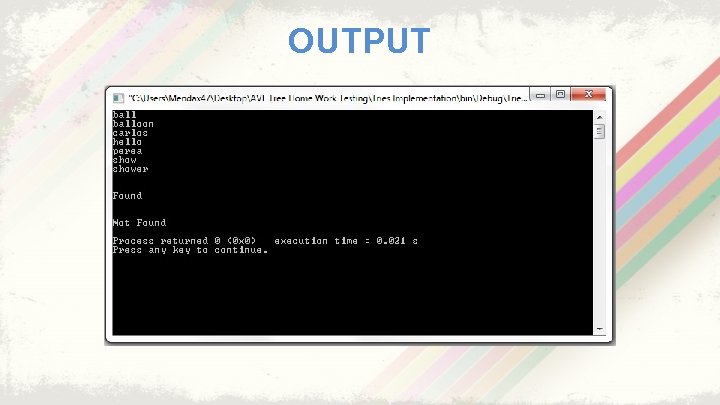
OUTPUT
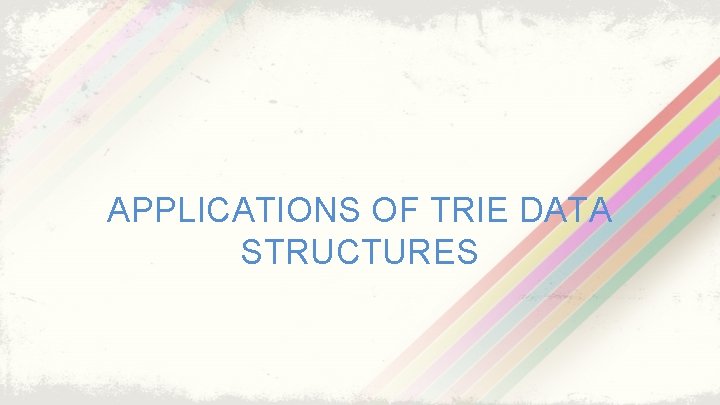
APPLICATIONS OF TRIE DATA STRUCTURES
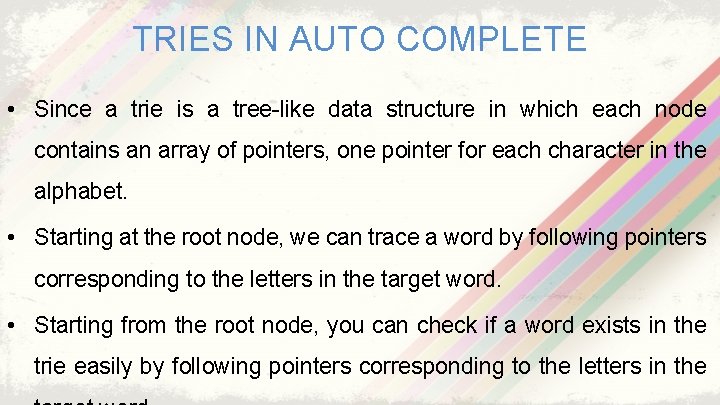
TRIES IN AUTO COMPLETE • Since a trie is a tree-like data structure in which each node contains an array of pointers, one pointer for each character in the alphabet. • Starting at the root node, we can trace a word by following pointers corresponding to the letters in the target word. • Starting from the root node, you can check if a word exists in the trie easily by following pointers corresponding to the letters in the
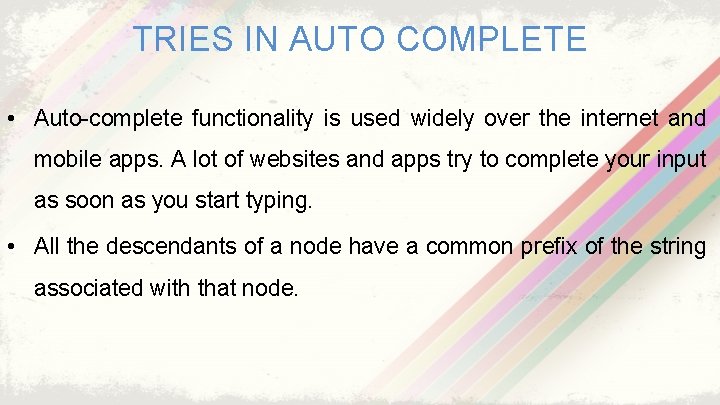
TRIES IN AUTO COMPLETE • Auto-complete functionality is used widely over the internet and mobile apps. A lot of websites and apps try to complete your input as soon as you start typing. • All the descendants of a node have a common prefix of the string associated with that node.
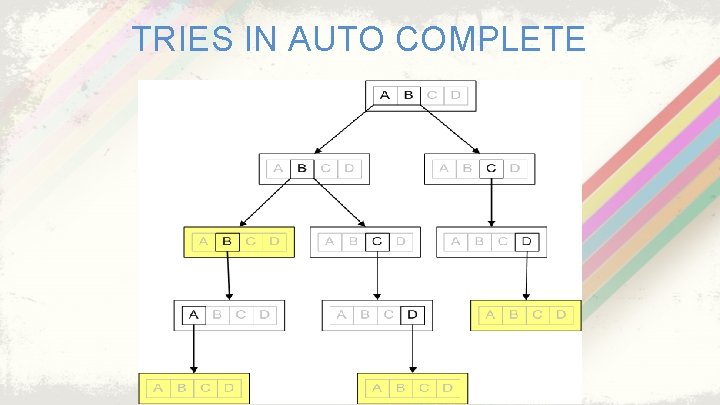
TRIES IN AUTO COMPLETE
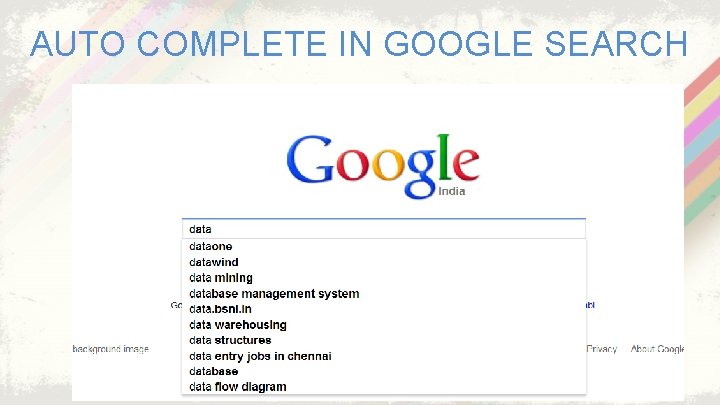
AUTO COMPLETE IN GOOGLE SEARCH
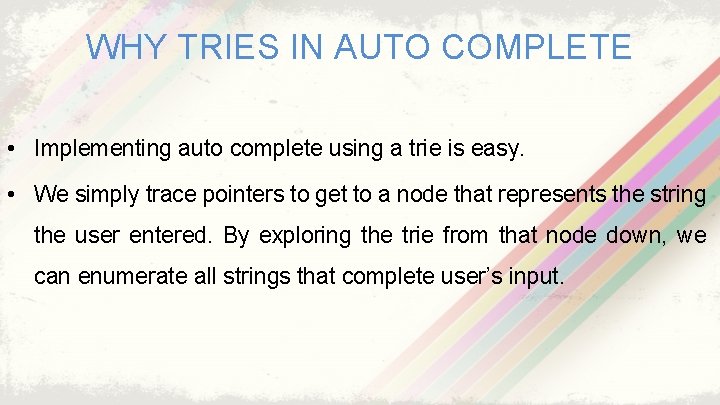
WHY TRIES IN AUTO COMPLETE • Implementing auto complete using a trie is easy. • We simply trace pointers to get to a node that represents the string the user entered. By exploring the trie from that node down, we can enumerate all strings that complete user’s input.
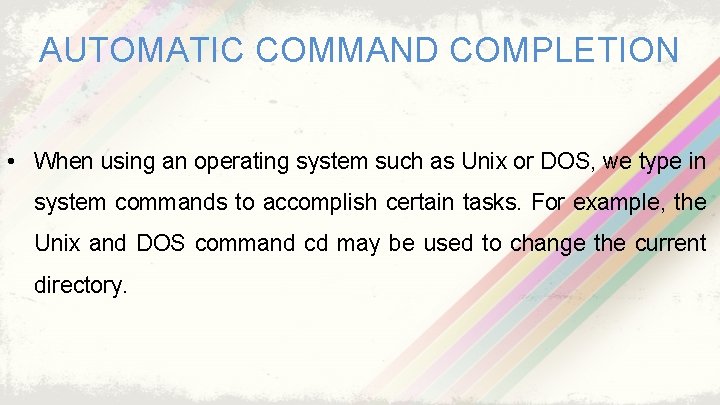
AUTOMATIC COMMAND COMPLETION • When using an operating system such as Unix or DOS, we type in system commands to accomplish certain tasks. For example, the Unix and DOS command cd may be used to change the current directory.
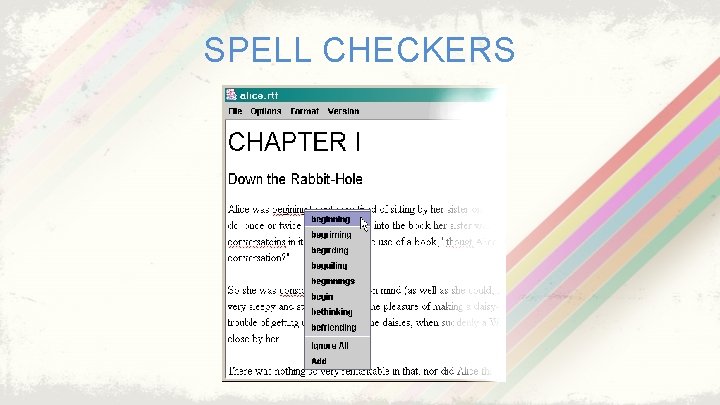
SPELL CHECKERS
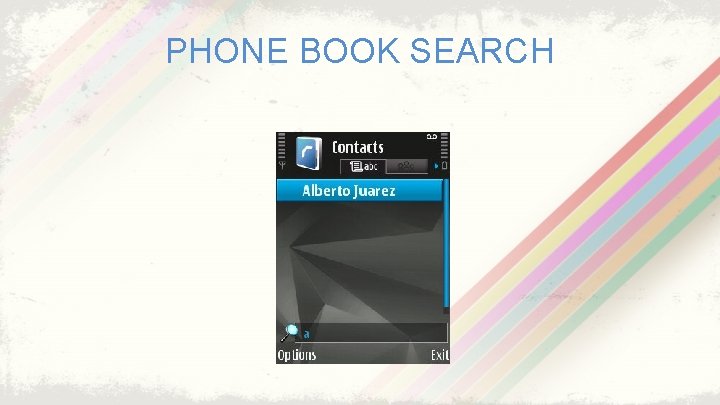
PHONE BOOK SEARCH
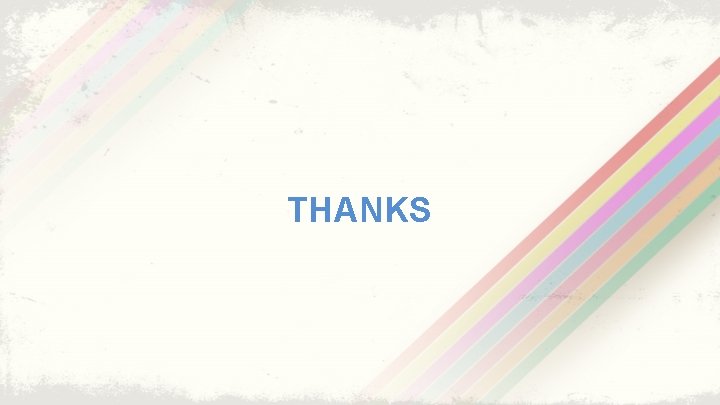
THANKS
Static and dynamic queue in data structure
R way trie
Compressed trie
Binary trie
Go go gophers huffman coding
Trie performance
Patricia trie
Hash trie
Boyer moore
Multiway trie
Record adalah
Elementary data organization
Physical state of covalent compounds
Union myunion structure my structure integer m float n
Ionic covalent metallic
@prof._jane_blendes:https://semawur.com/xujhhf16p
Transformational grammar
Subject-dqrnghtp
Giant molecular structure vs simple molecular structure
Deep and surface structure in linguistics
What is linear data structure
Sequential organization
Linear and nonlinear data structure
Diff between primitive and nonprimitive
Linear and nonlinear data structure
Bfs tree traversal
Topological sort
What is threaded binary tree
Struktur data queue
Data structure metrics
Graph traversal in data structure
Sparse matrix in data structure
Polynomial addition using linked list
Sparse matrix in data structure
Josephus problem solution in c using array
Linux kernel map data structure
Output restricted deque
What is data structure in gis
What is gis data structure
Full binary tree in data structure
Stack data structure exercises
Forest in data structure
Knuth's boundary tags
Dynamic memory allocation in data structure
Document object model
Python inverted index
Non primitive data structure
Data structure operations
Forest in data structure
Algorithm complexity
Disjoint set adt
Data structure examples
Singly linked list in data structure
Dynamic memory allocation in data structure
Bag data structure
Traversing linear array algorithm
Jenis jenis queue
Abstract data types
Rubik's cube solver 3x3
Siblings in tree data structure
Shortest paths and transitive closure in data structure
Polynomial representation using generalized linked list
Kodes
Overloading in data structure
Column major wise formula
Data structure definition
Linked list java
Union data structure
Data structure for text editor
Calendar data structure
Static vs dynamic website
Pseudocode
Derived data types in c
Hndit.com
Data structure problems
Dijkstra's algorithm table
Data structure server
Smart open
Deaps in data structure
Graph data structure in c
Tree data structure problems
Transform flow and transaction flow
Data structure picture
Data commentary meaning
Winged-edge data structure
Binary tree in data structure
Relational data structure
Queue data structure
Logical data structure
Mid-square hashing
Greedy method in data structure
Dynamic structures
Tree traversal in data structure
Data structure visualization tool
Timeline data structure
Recv winsock
Bag data structure
What are the advantages of arrays?
Representation of linear array in data structure
Min-max heap
Avl data structure
Structured tools
First in first out
Recall data structure
Queue in data structure
Classic data structures by debasis samanta ppt
Parallel arrays examples
Graph data structure python
Postfix expression
Double hash table
Augmented data structure examples
Aggregate data structure
Permuterm index of x*y*z
Ephemeral data structure
Ephemeral data structure
Dynamic data structure java
Linux kernel map data structure
Deap data structure
Fundamentals of data structure in c
Non linear data structure
Usable vs useful
Subjective data vs objective data
Components of gis
Tentukan simpangan baku dari data 2 3 4 5 6
Contoh data primer
Spatial data and attribute data
Data reliability and validity
Prinsip dasar analisis kualitas lingkungan
Snapshot standby database license
Categorical data vs numerical data
Respiration meaning in bengali
Continuous data vs discrete
Data reduction in data mining
What is kdd process in data mining
Contoh data warehouse dan data mart
Building blocks of data warehouse
Task abstraction example
Data cleaning problems and current approaches
Data integration in data preprocessing
Data on the inside vs data on the outside
What is missing data in data mining
Data reduction in data mining
Data reduction in data mining
Data reduction in data mining
Contoh data mart
Raster vs vector data
Siklus manajemen data kesehatan
Define data collection method
Data cube technology in data mining
Data reduction in data mining
Data preparation and basic data analysis
Qualitative and quantitative data analysis
Vector vs raster data
How to find standard deviation of grouped data
How to convert unstructured data into structured data
Variance formula for ungrouped data
Spatial data vs non spatial data
Syndicated panel survey measure the
The terms external secondary data and syndicated
Contoh peta jabatan dinas
Materi operasi perangkat lunak pengolah data
Definisi kamus data
Difference between operational and informational data
Javachive
Data warehouses generalize and consolidate data in
Data warehouse dan data mining
Data mining dan data warehouse
Data quality and data cleaning an overview
Data acquisition and data analysis