kernel data structures outline linked list queues maps
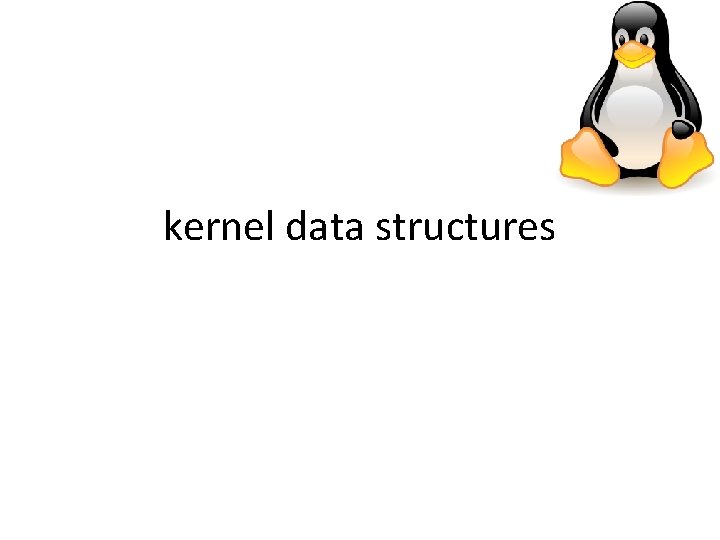
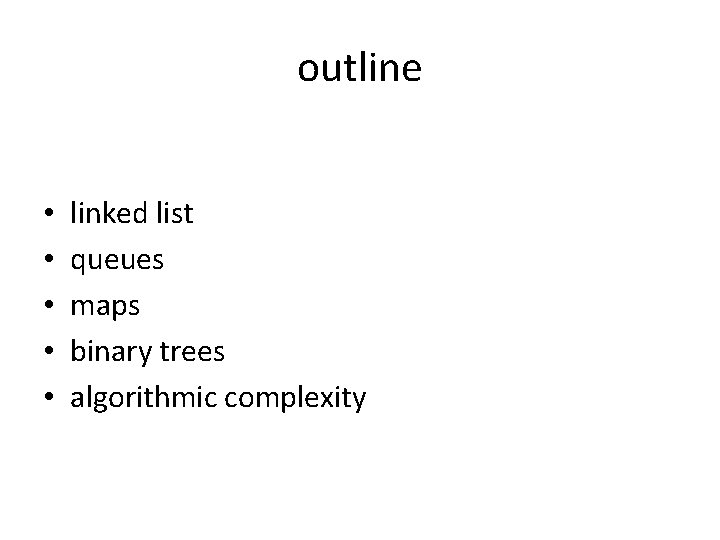
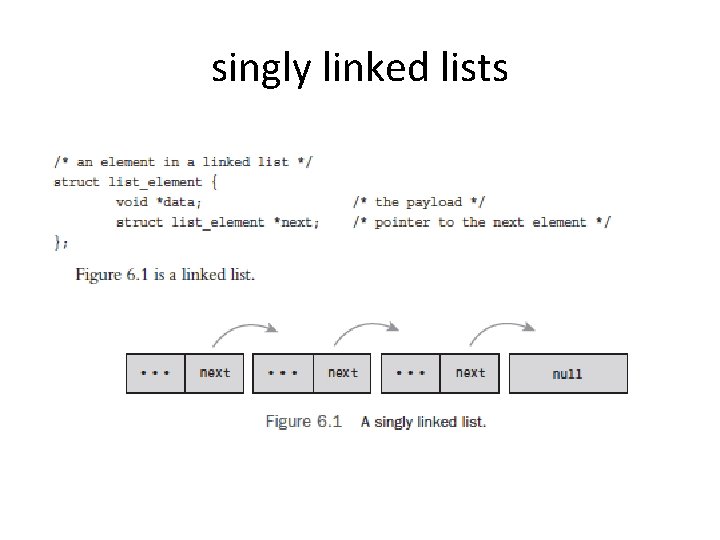
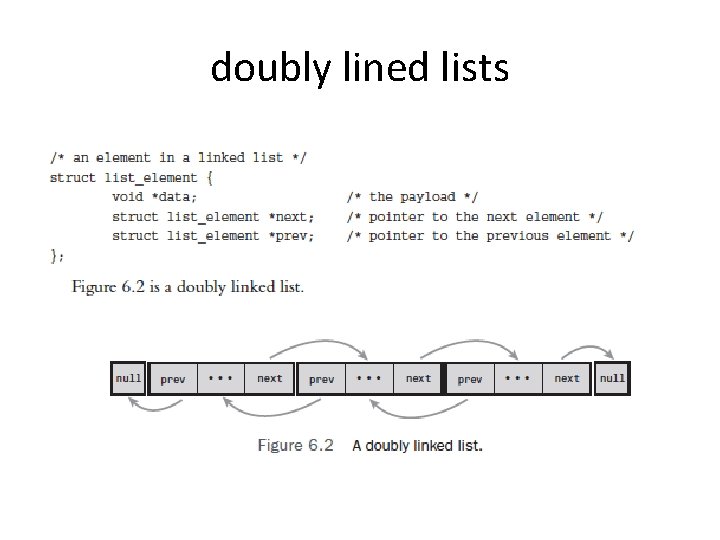
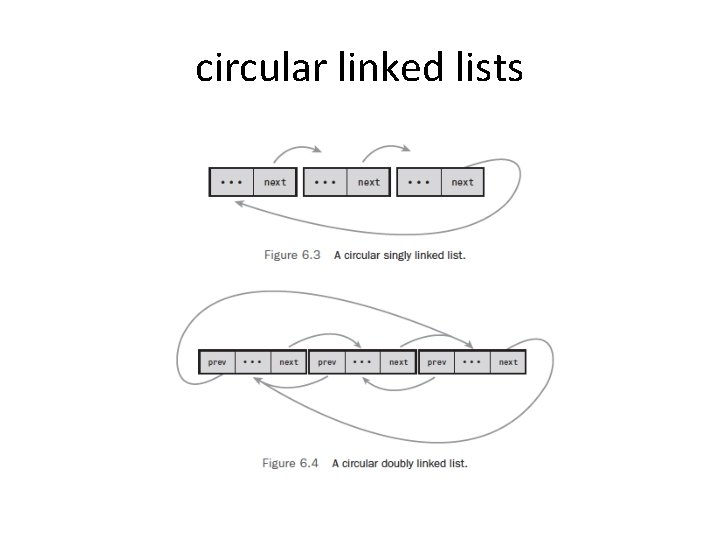
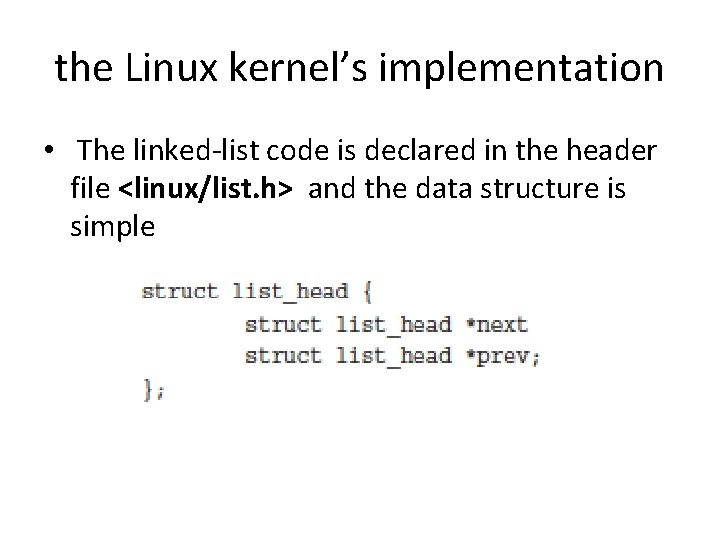
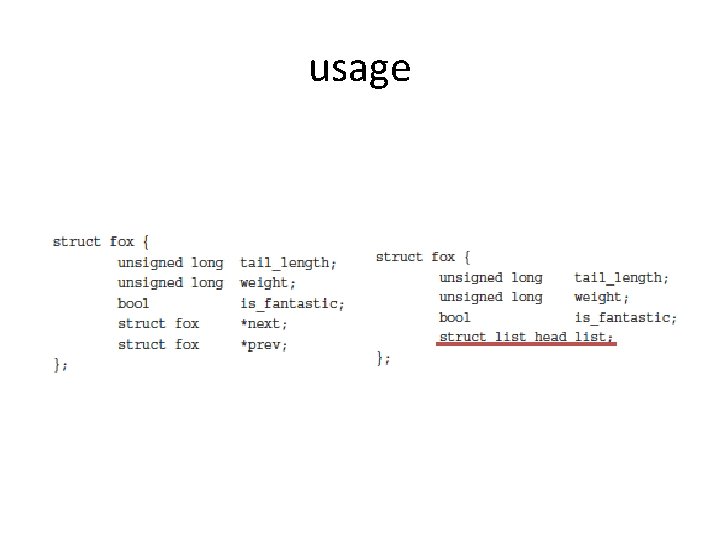
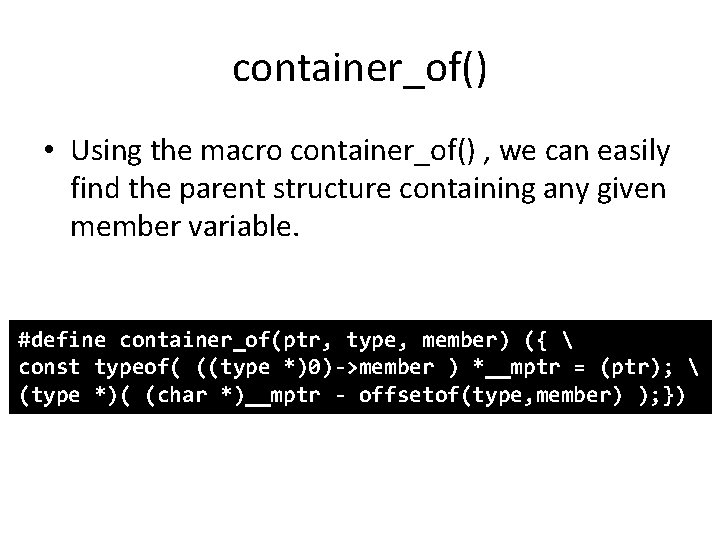
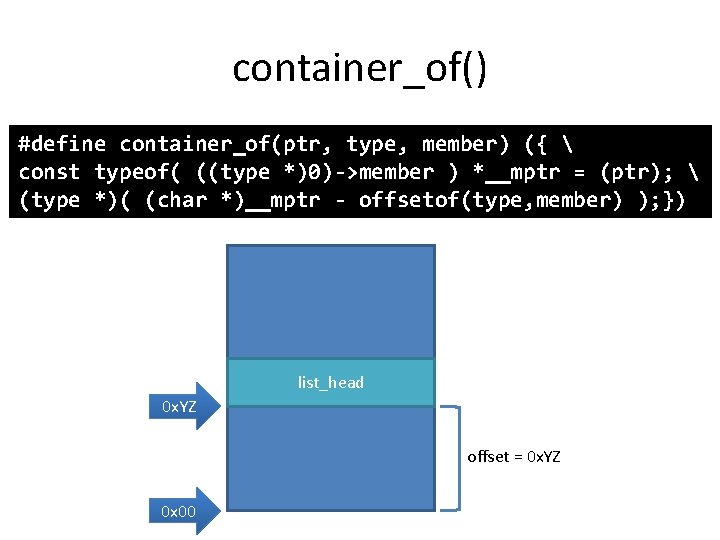
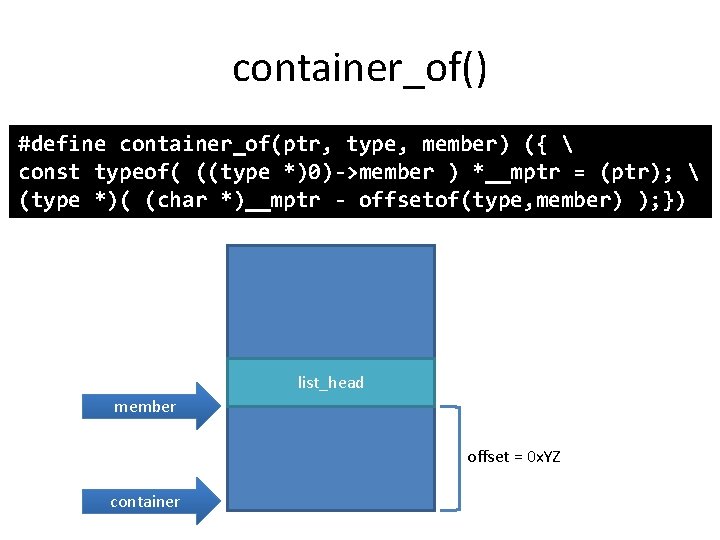
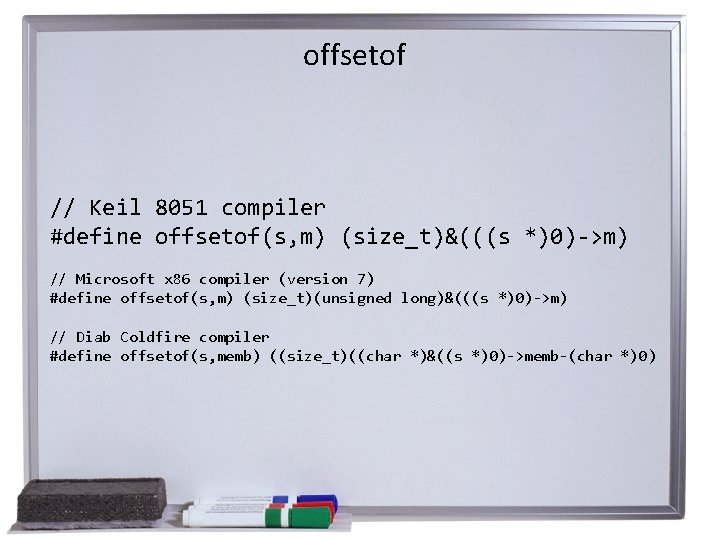
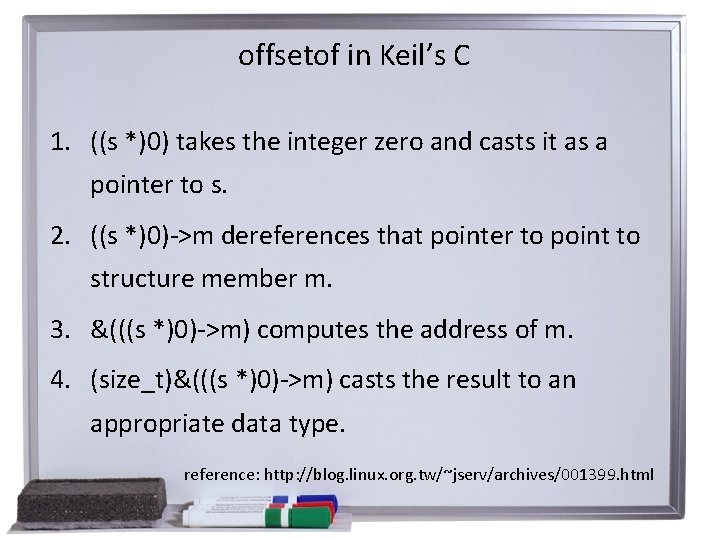
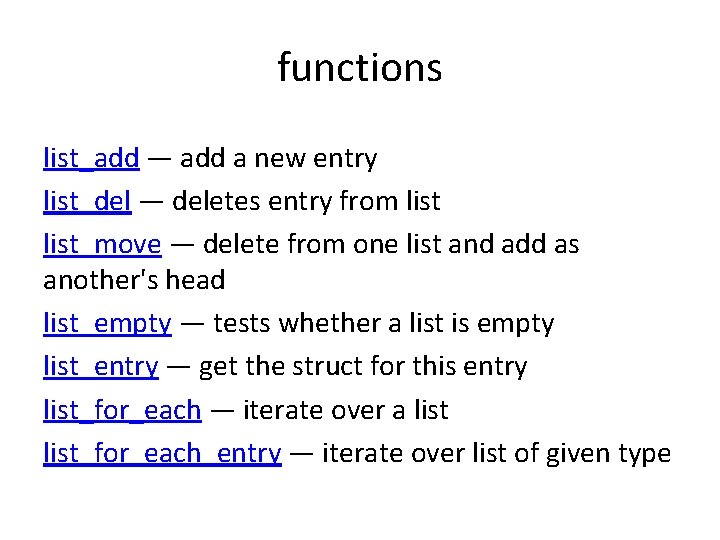
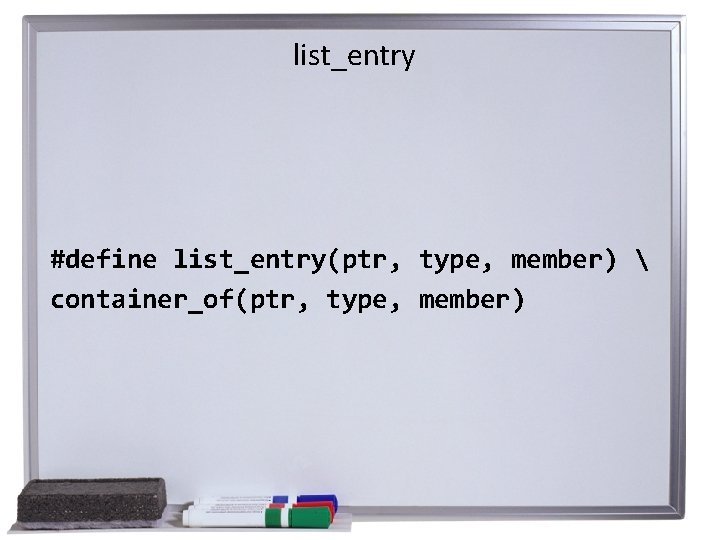
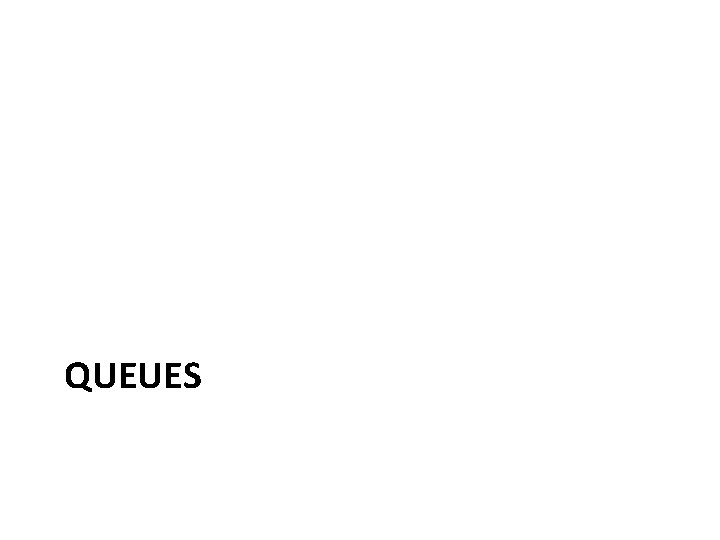
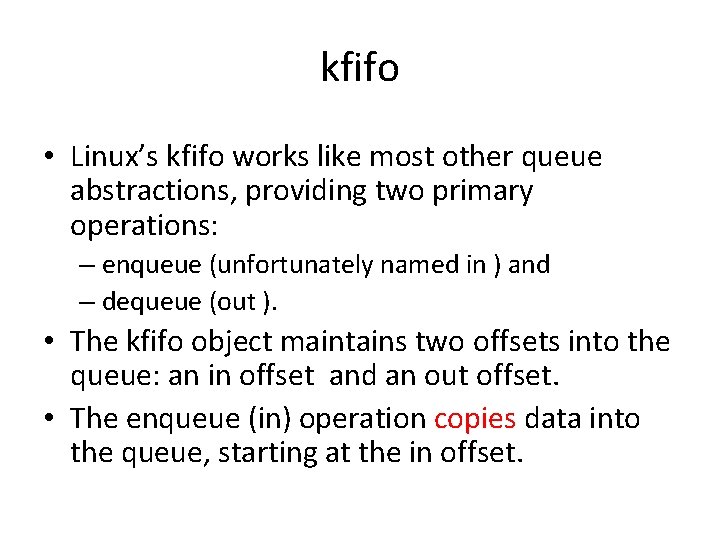
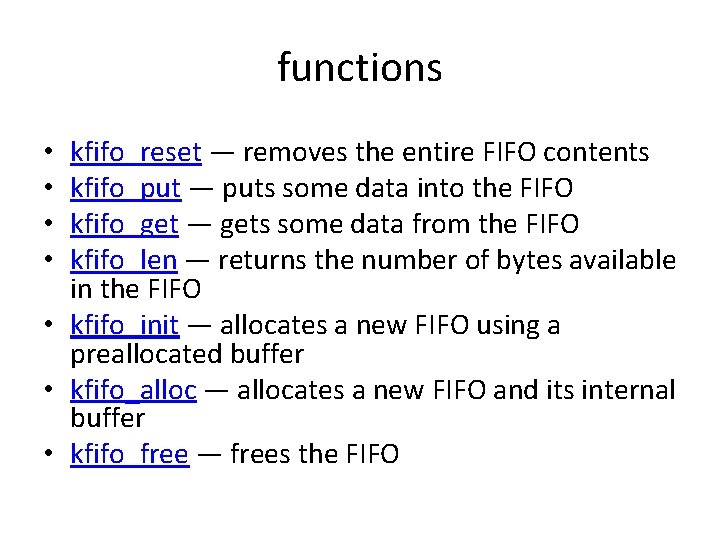
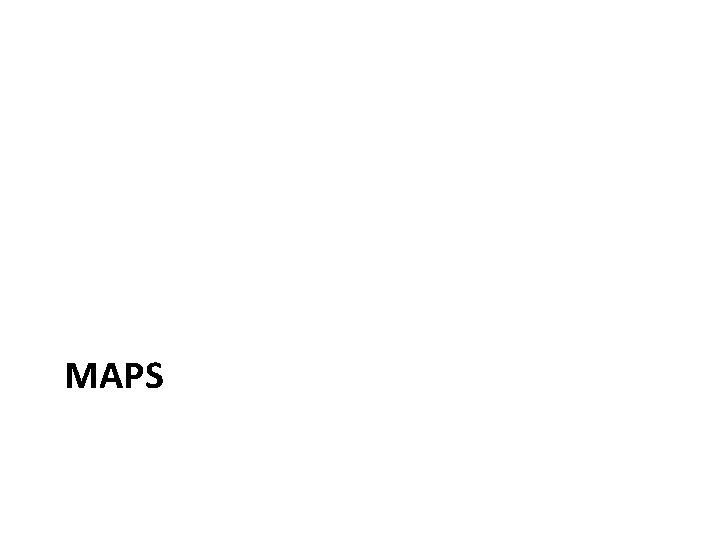
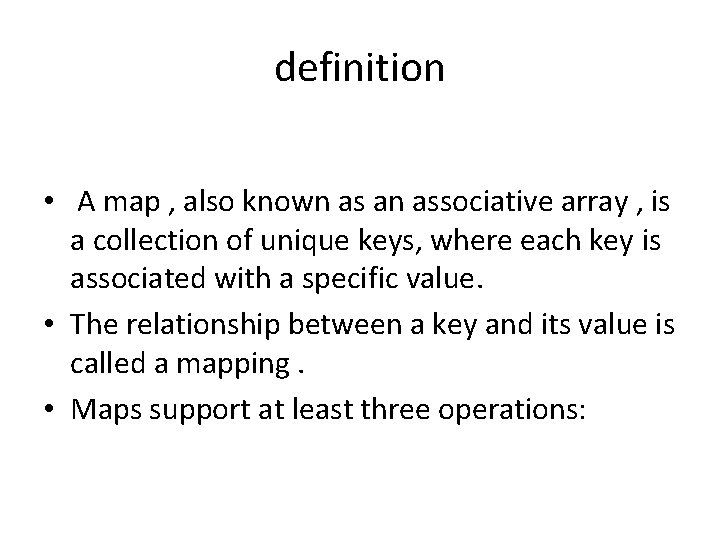
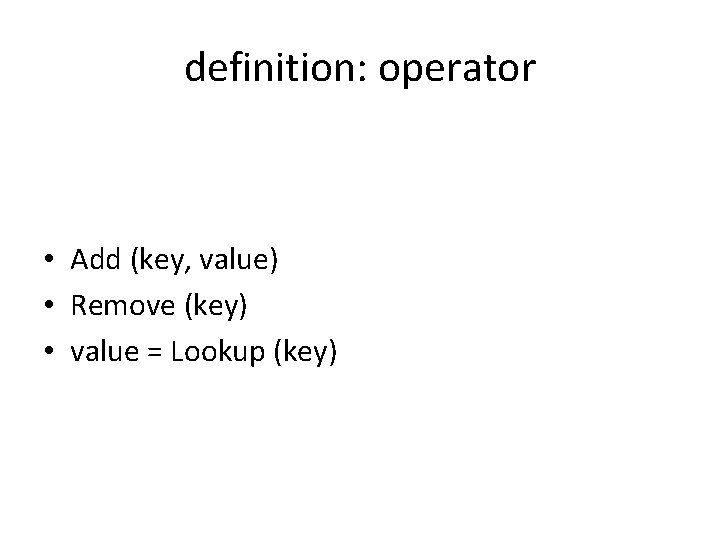
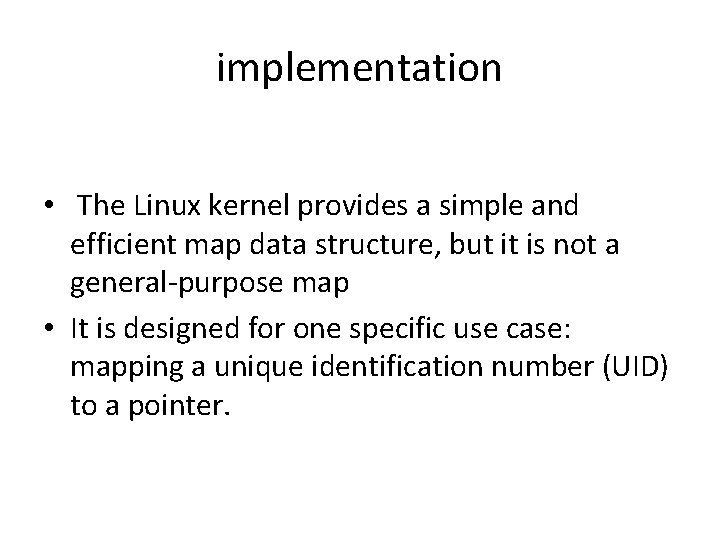
- Slides: 21
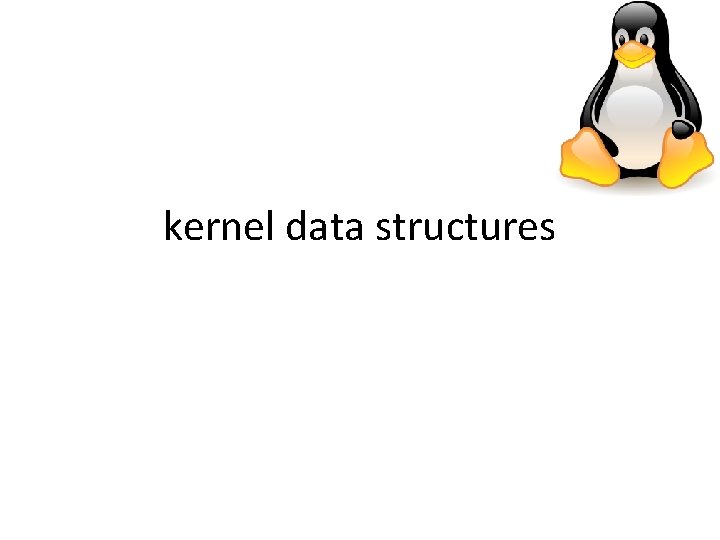
kernel data structures
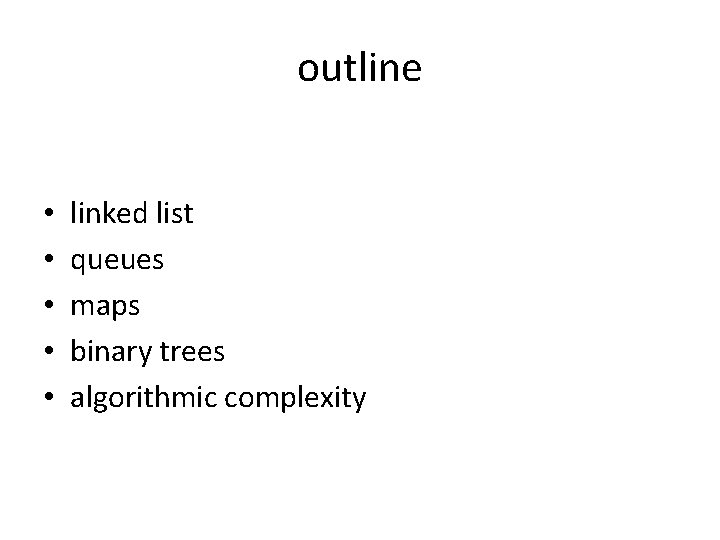
outline • • • linked list queues maps binary trees algorithmic complexity
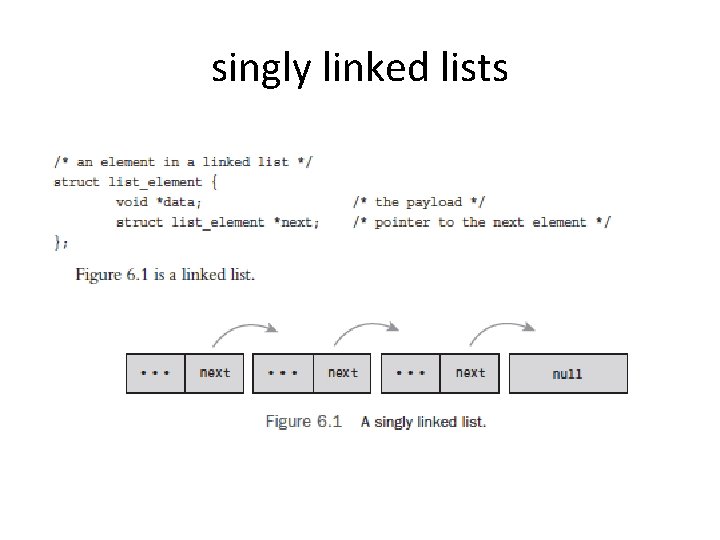
singly linked lists
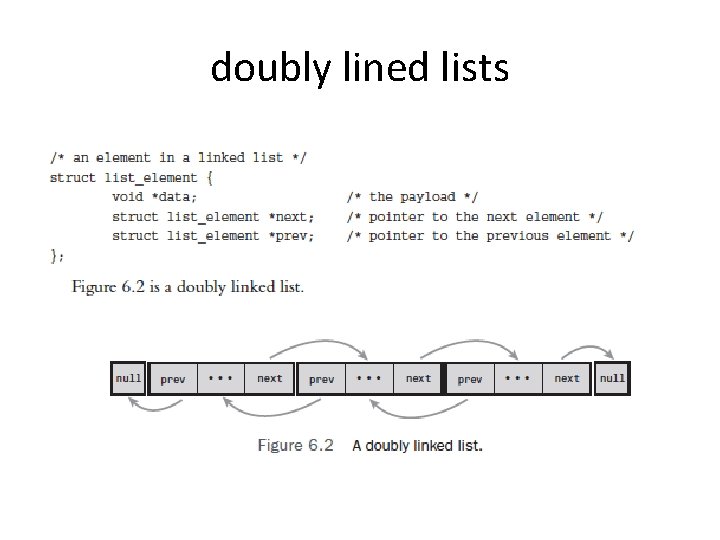
doubly lined lists
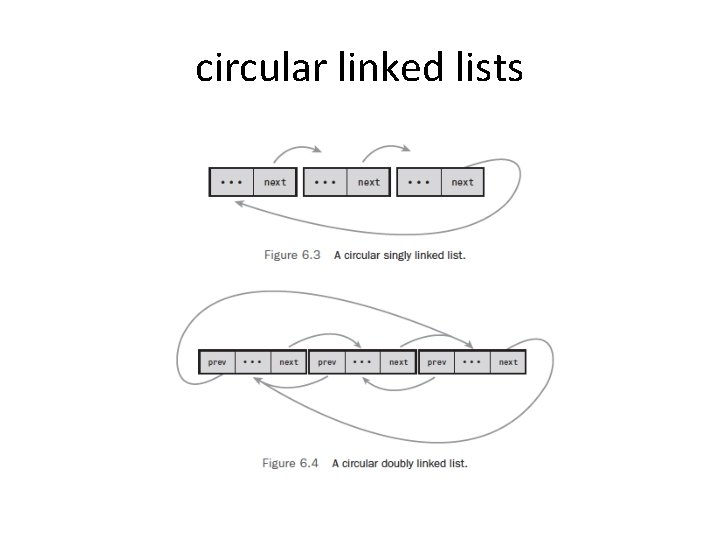
circular linked lists
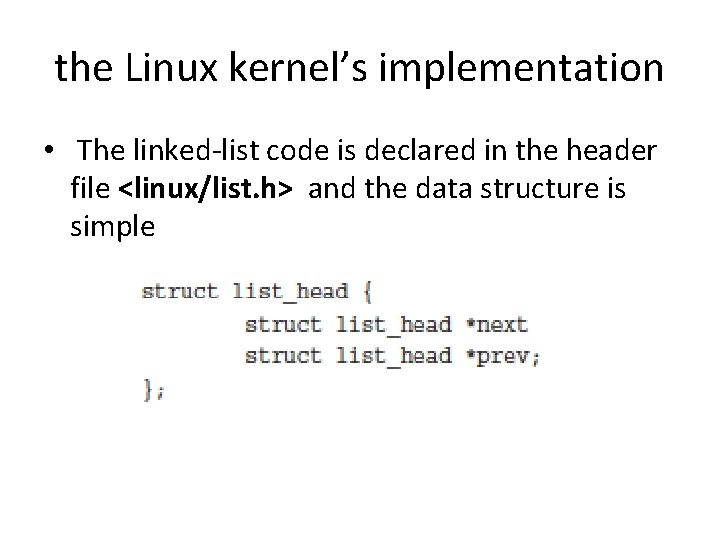
the Linux kernel’s implementation • The linked-list code is declared in the header file <linux/list. h> and the data structure is simple
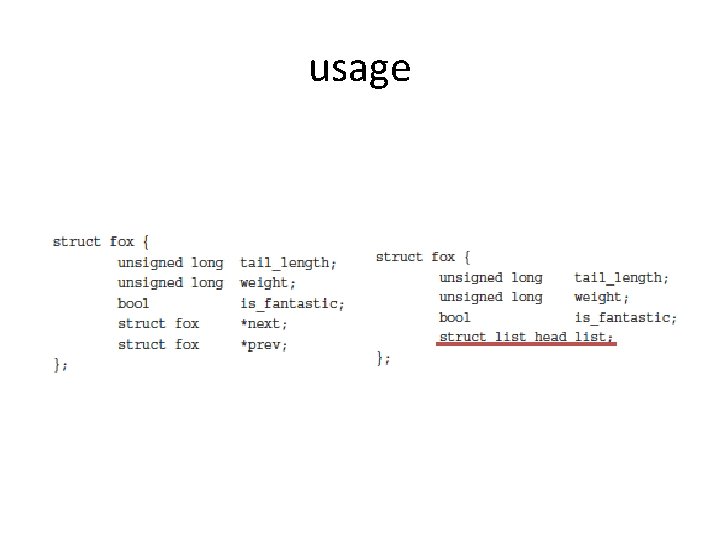
usage
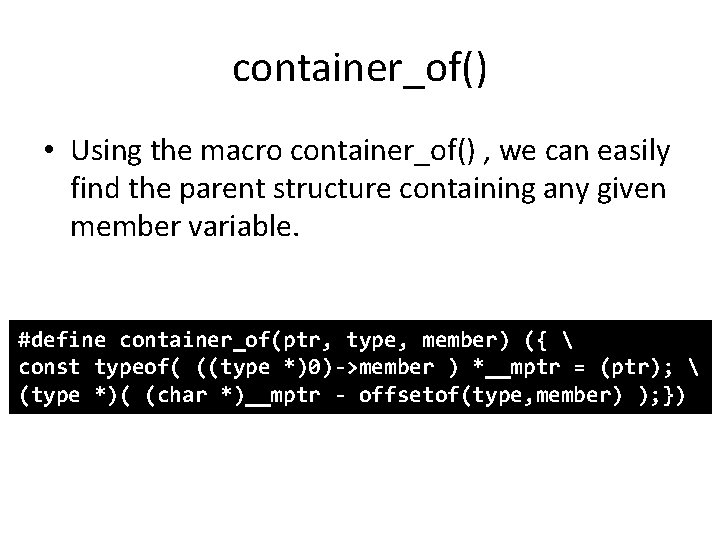
container_of() • Using the macro container_of() , we can easily find the parent structure containing any given member variable. #define container_of(ptr, type, member) ({ const typeof( ((type *)0)->member ) *__mptr = (ptr); (type *)( (char *)__mptr - offsetof(type, member) ); })
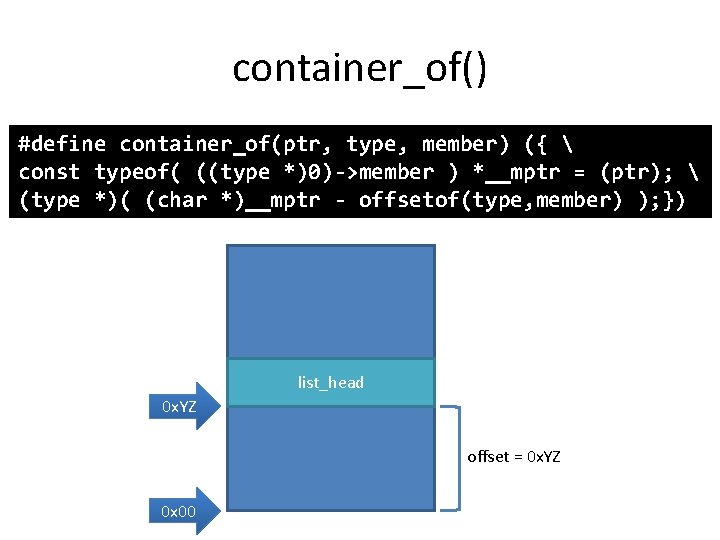
container_of() #define container_of(ptr, type, member) ({ const typeof( ((type *)0)->member ) *__mptr = (ptr); (type *)( (char *)__mptr - offsetof(type, member) ); }) list_head 0 x. YZ offset = 0 x. YZ 0 x 00
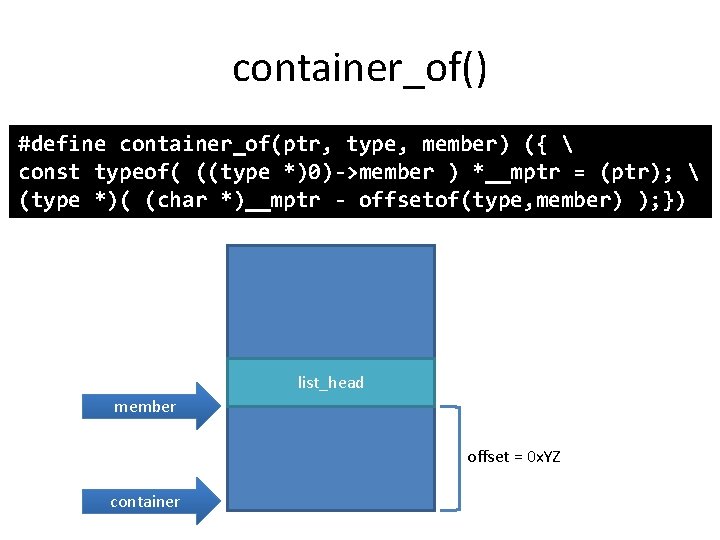
container_of() #define container_of(ptr, type, member) ({ const typeof( ((type *)0)->member ) *__mptr = (ptr); (type *)( (char *)__mptr - offsetof(type, member) ); }) list_head member offset = 0 x. YZ container
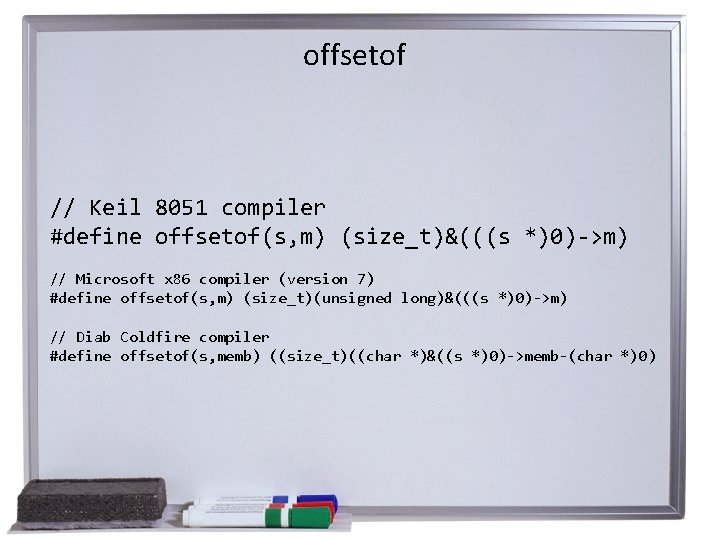
offsetof // Keil 8051 compiler #define offsetof(s, m) (size_t)&(((s *)0)->m) // Microsoft x 86 compiler (version 7) #define offsetof(s, m) (size_t)(unsigned long)&(((s *)0)->m) // Diab Coldfire compiler #define offsetof(s, memb) ((size_t)((char *)&((s *)0)->memb-(char *)0)
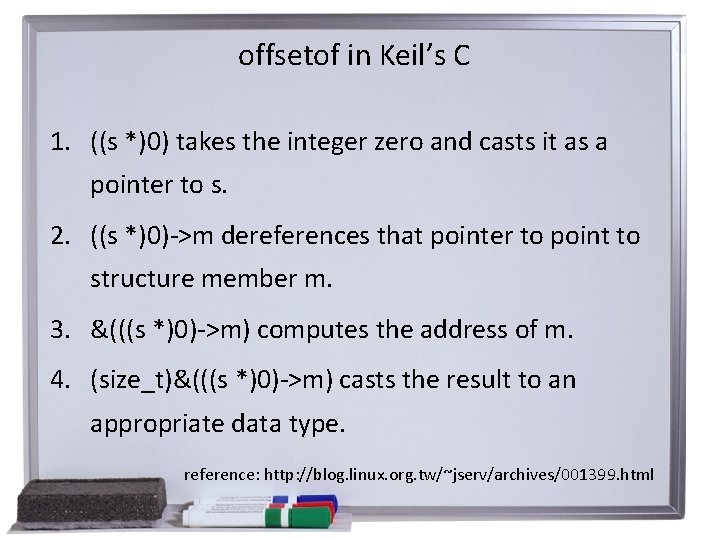
offsetof in Keil’s C 1. ((s *)0) takes the integer zero and casts it as a pointer to s. 2. ((s *)0)->m dereferences that pointer to point to structure member m. 3. &(((s *)0)->m) computes the address of m. 4. (size_t)&(((s *)0)->m) casts the result to an appropriate data type. reference: http: //blog. linux. org. tw/~jserv/archives/001399. html
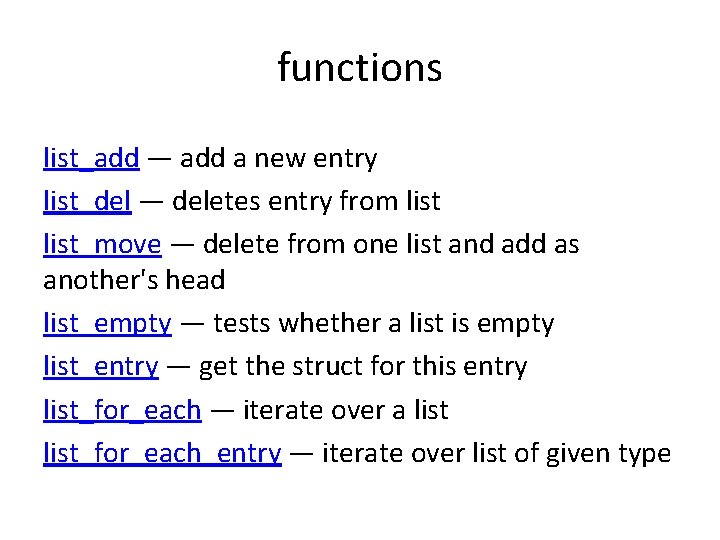
functions list_add — add a new entry list_del — deletes entry from list_move — delete from one list and add as another's head list_empty — tests whether a list is empty list_entry — get the struct for this entry list_for_each — iterate over a list_for_each_entry — iterate over list of given type
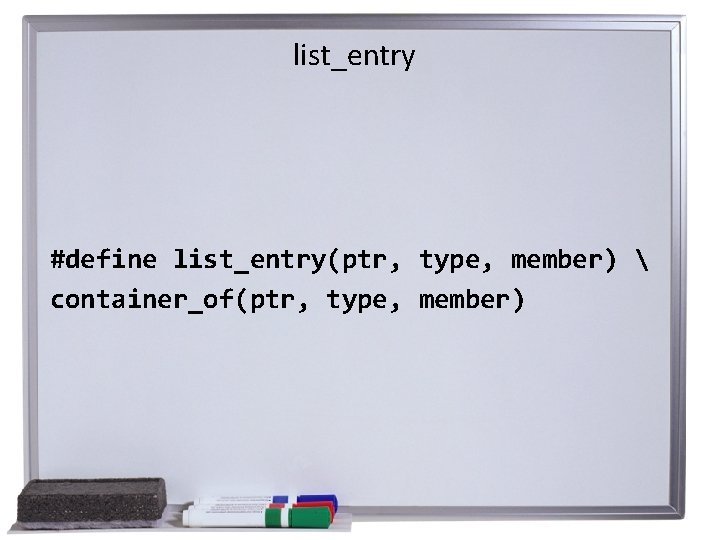
list_entry #define list_entry(ptr, type, member) container_of(ptr, type, member)
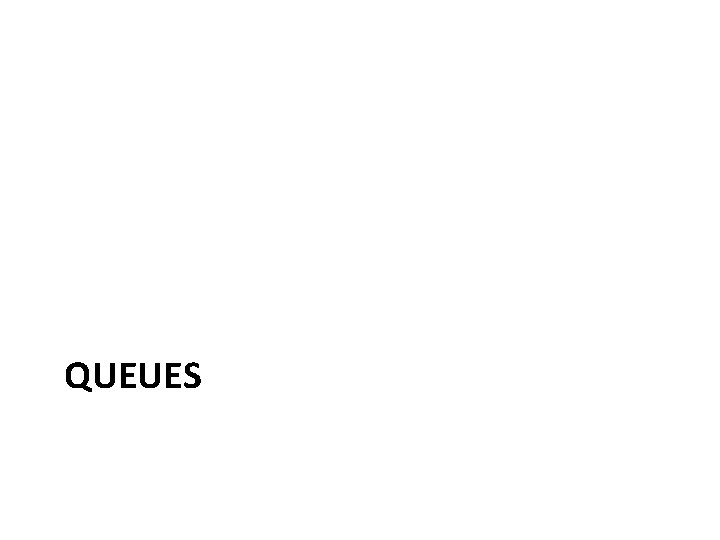
QUEUES
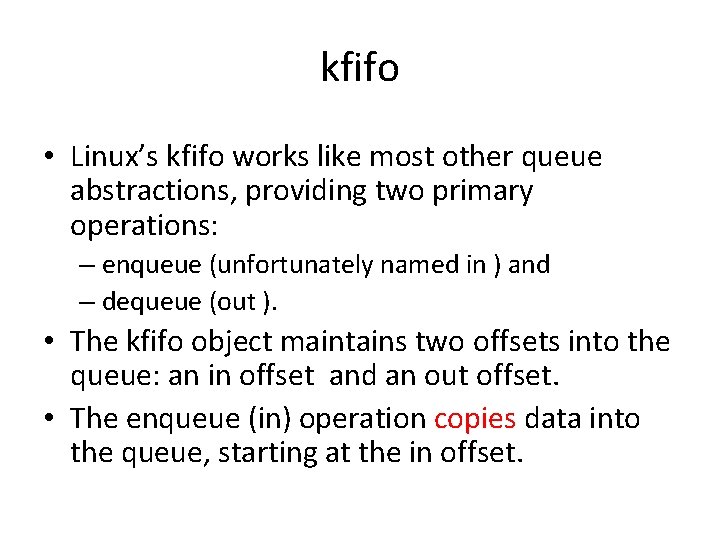
kfifo • Linux’s kfifo works like most other queue abstractions, providing two primary operations: – enqueue (unfortunately named in ) and – dequeue (out ). • The kfifo object maintains two offsets into the queue: an in offset and an out offset. • The enqueue (in) operation copies data into the queue, starting at the in offset.
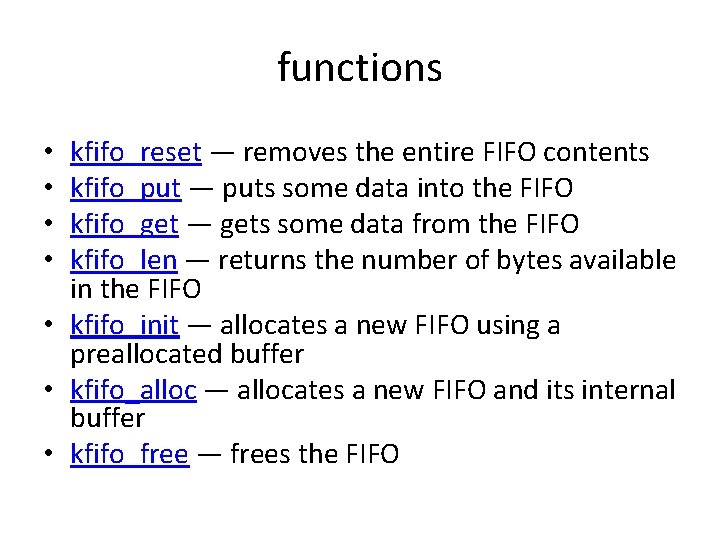
functions kfifo_reset — removes the entire FIFO contents kfifo_put — puts some data into the FIFO kfifo_get — gets some data from the FIFO kfifo_len — returns the number of bytes available in the FIFO • kfifo_init — allocates a new FIFO using a preallocated buffer • kfifo_alloc — allocates a new FIFO and its internal buffer • kfifo_free — frees the FIFO • •
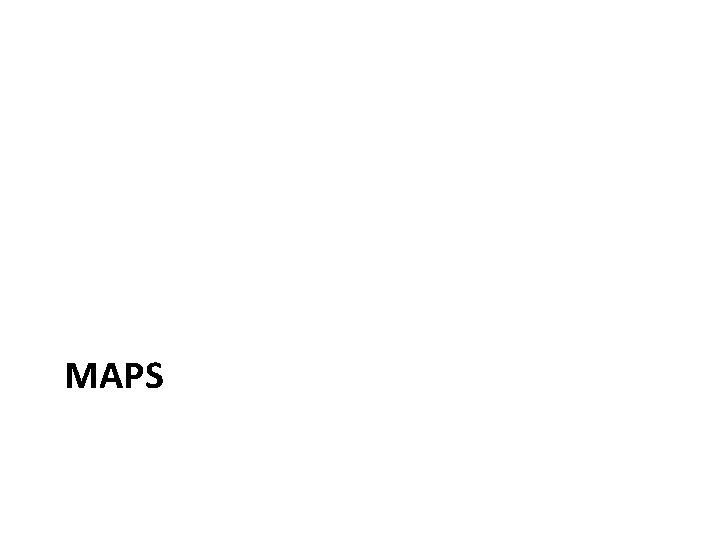
MAPS
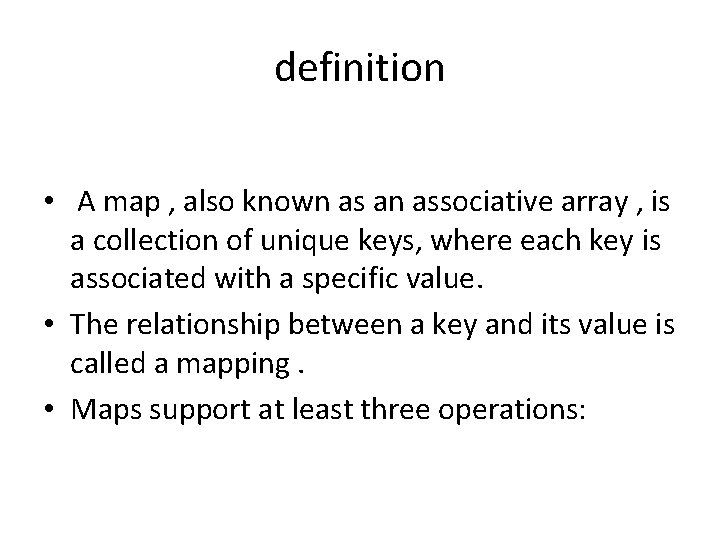
definition • A map , also known as an associative array , is a collection of unique keys, where each key is associated with a specific value. • The relationship between a key and its value is called a mapping. • Maps support at least three operations:
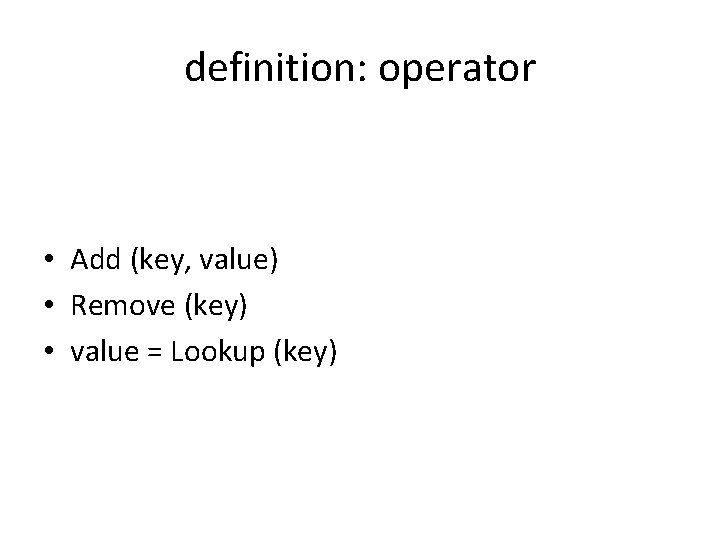
definition: operator • Add (key, value) • Remove (key) • value = Lookup (key)
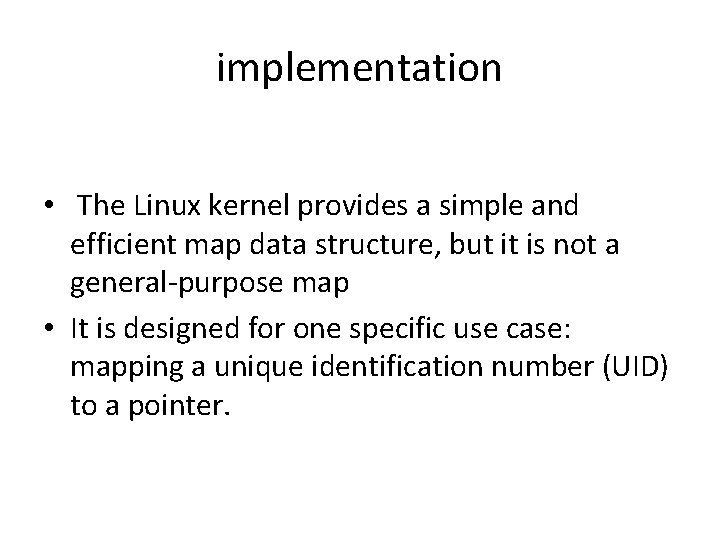
implementation • The Linux kernel provides a simple and efficient map data structure, but it is not a general-purpose map • It is designed for one specific use case: mapping a unique identification number (UID) to a pointer.
Singly vs doubly linked list
Singly vs doubly linked list
List adalah
Linux kernel data structures
Jenis struktur data
Java data structures
Polynomial addition in data structure using linked list
How to add polynomials using linked list
Maps reittihaku
Stacks and queues in python
Priority queues: quiz
Java stacks and queues
Queue representation
Java stack exercises
Ipcs unix
Adaptable priority queue
Pipes in rtos
Applications of priority queues
Queue head tail
Aganj
Java stacks and queues
Analogous structures