Graphs C Data Structure Runtimes Data Structure Insert
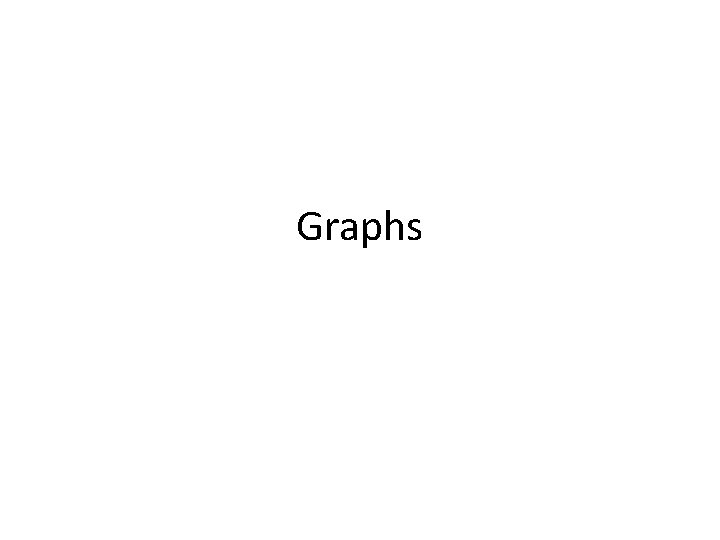
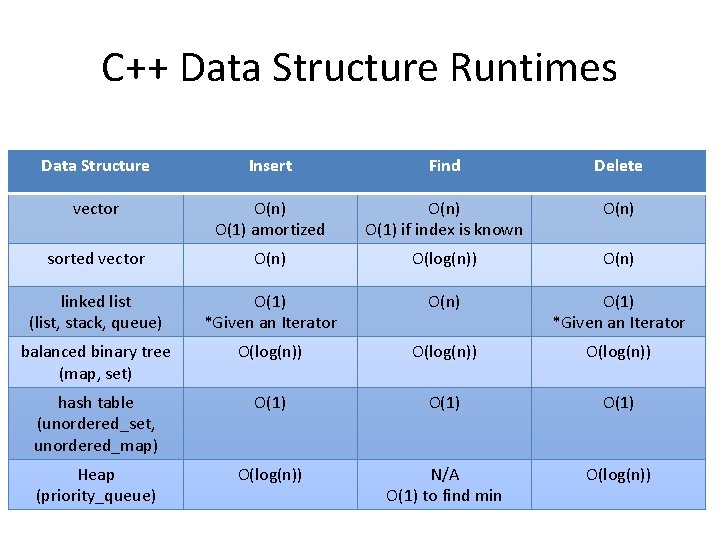
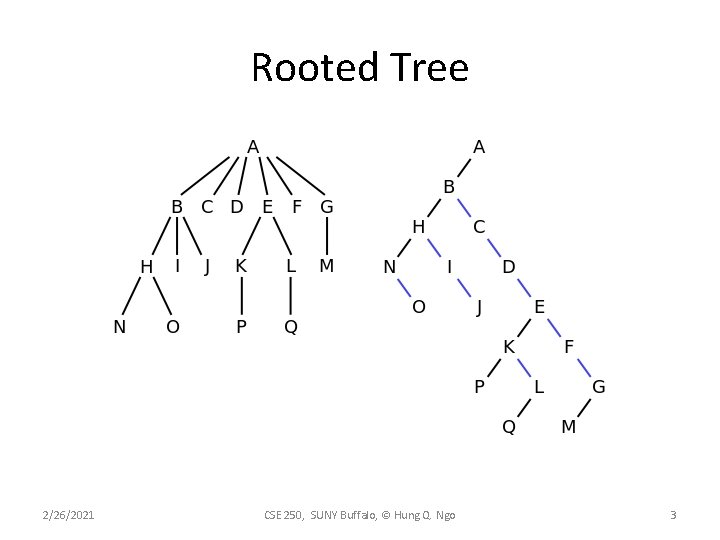
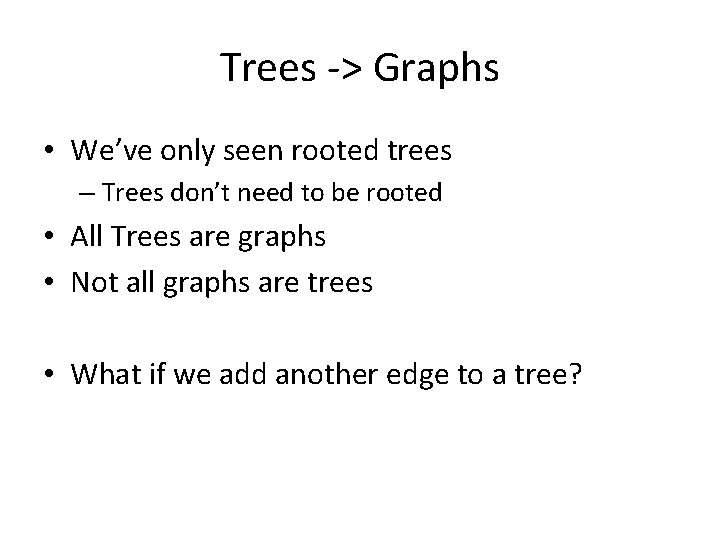
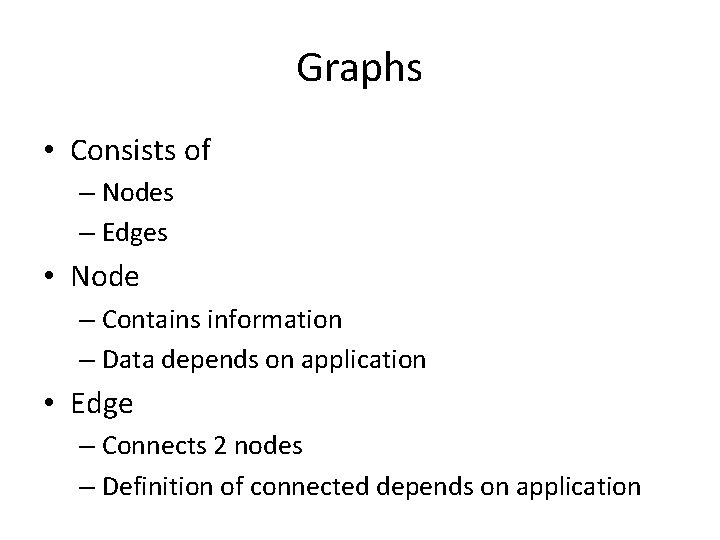
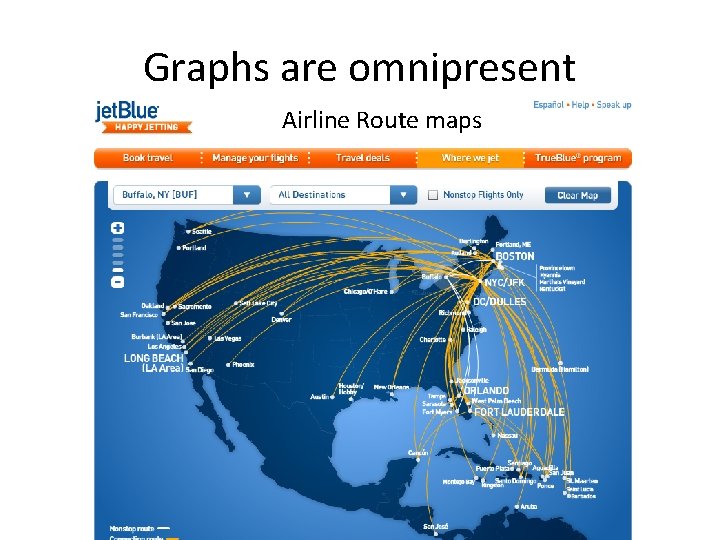
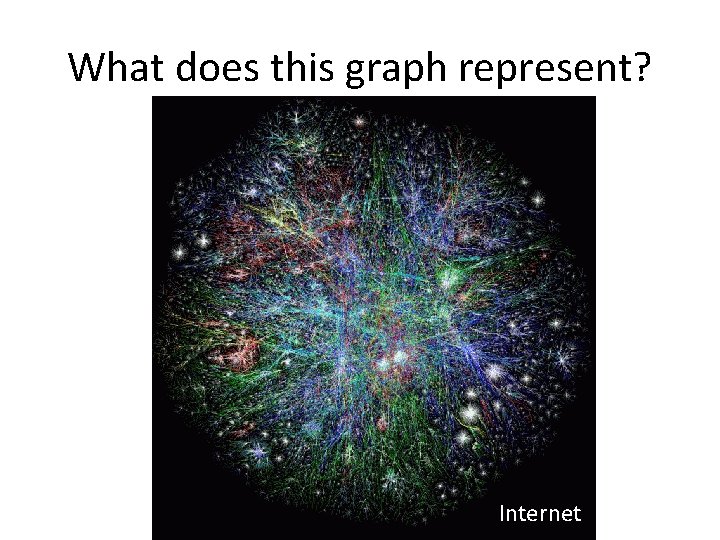
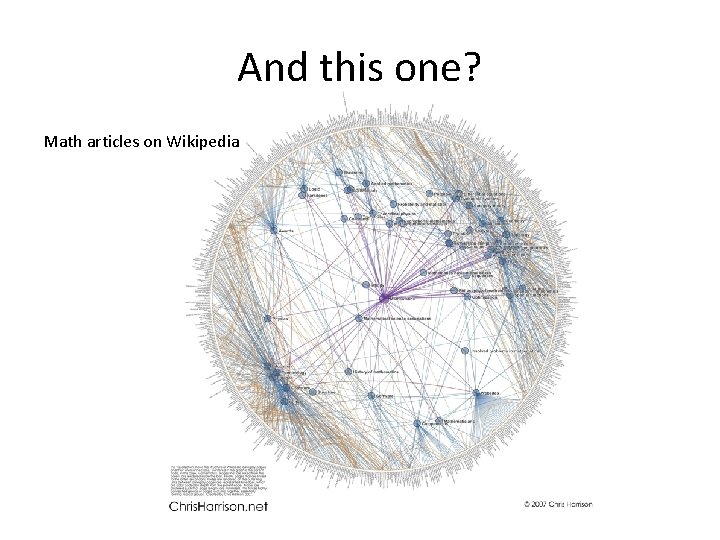
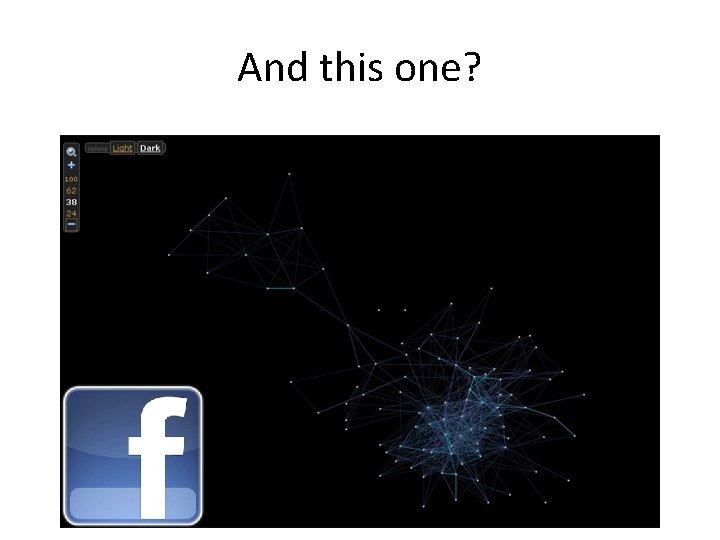
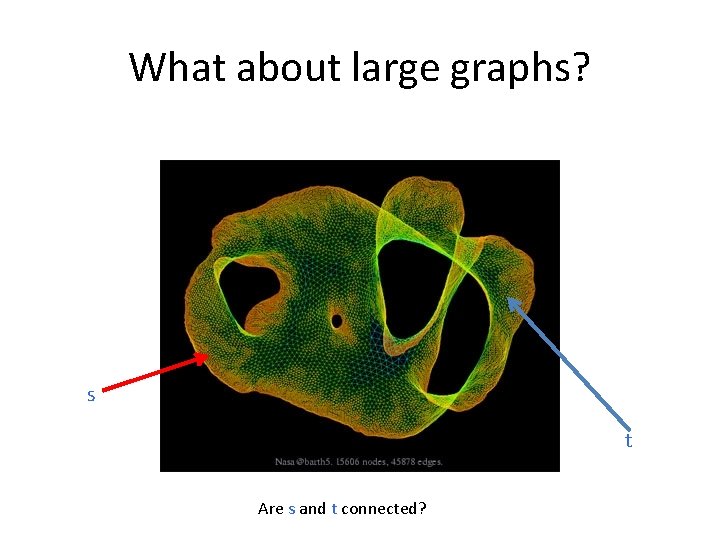
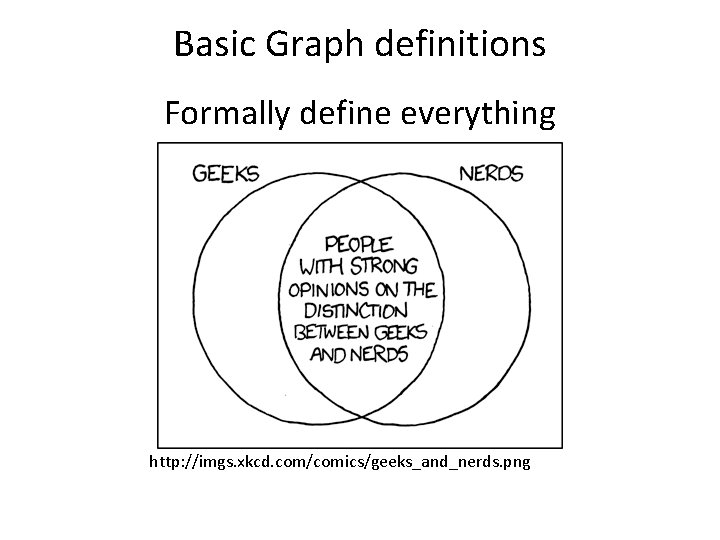
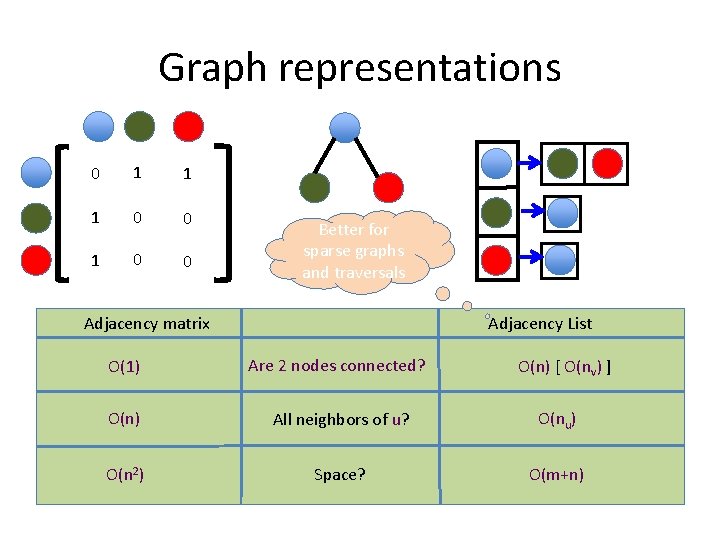
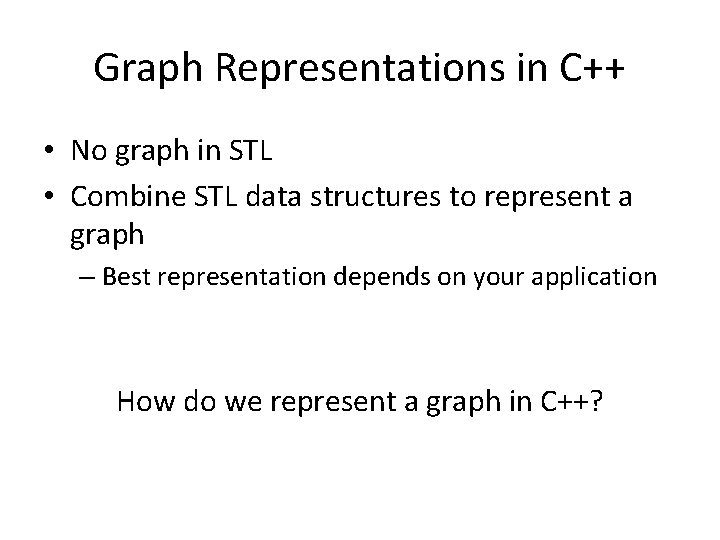
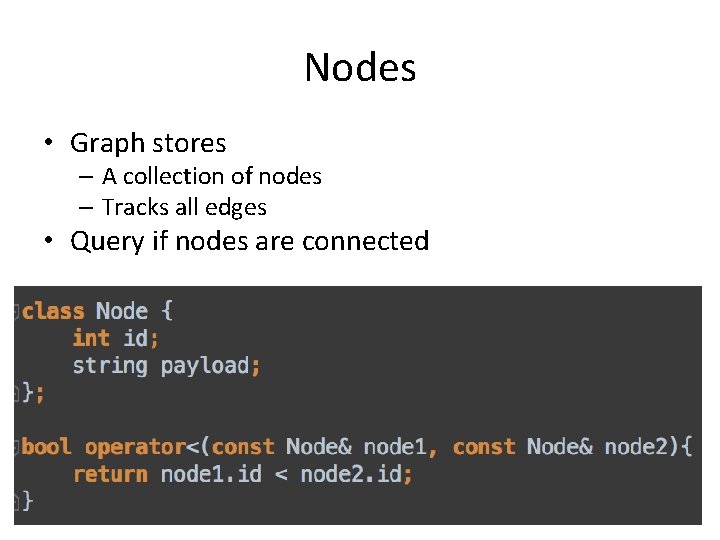
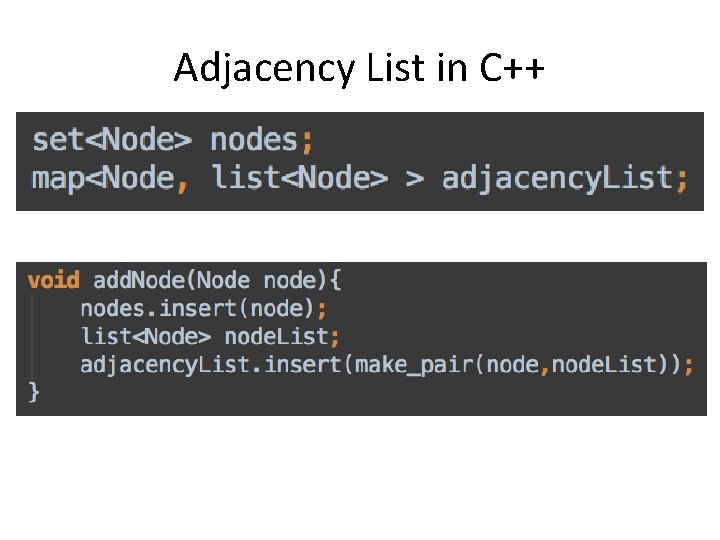
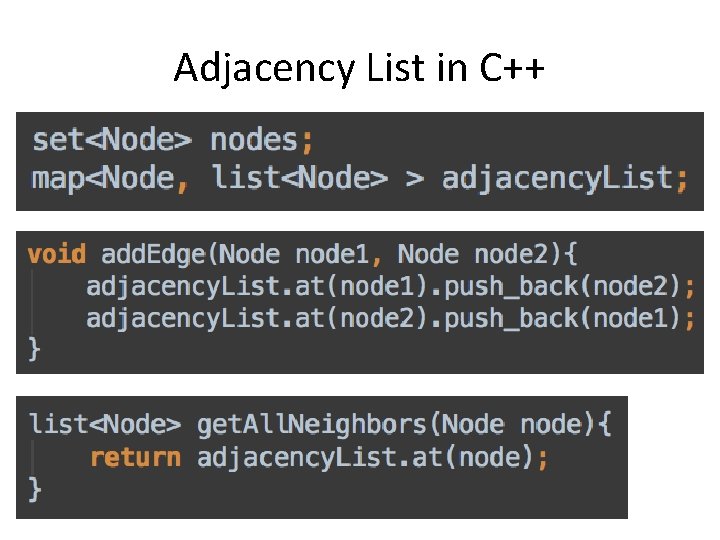
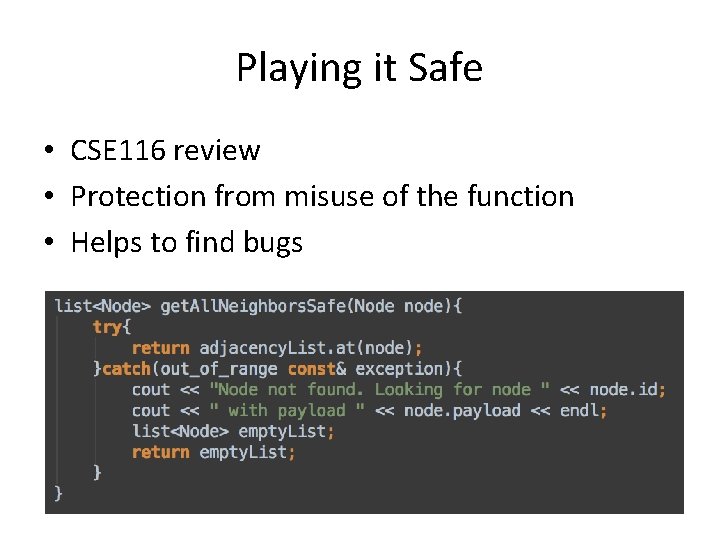
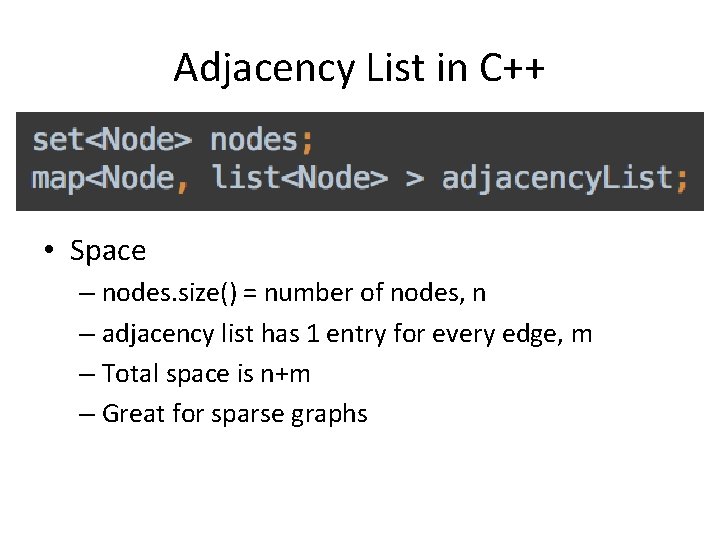
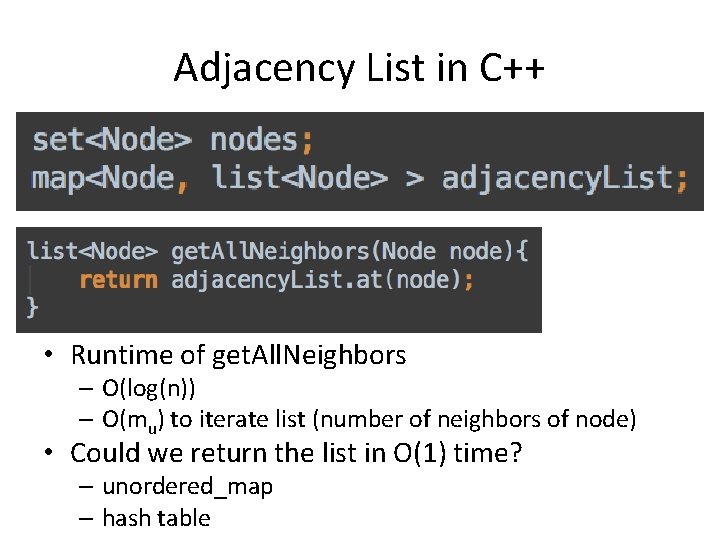
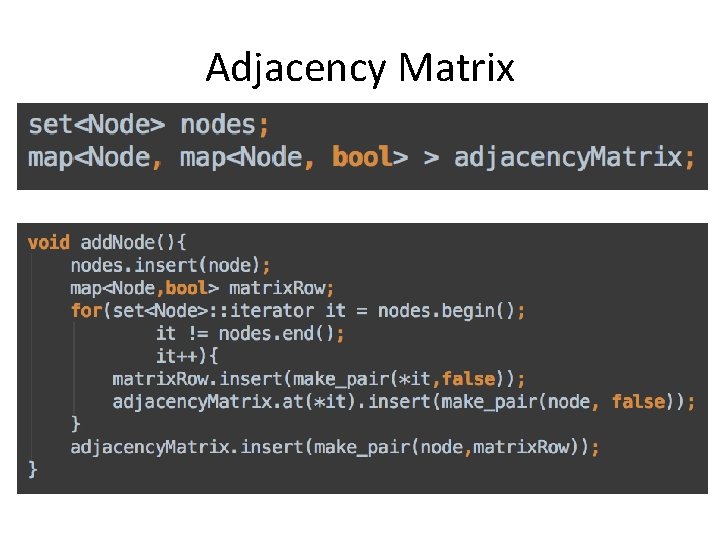
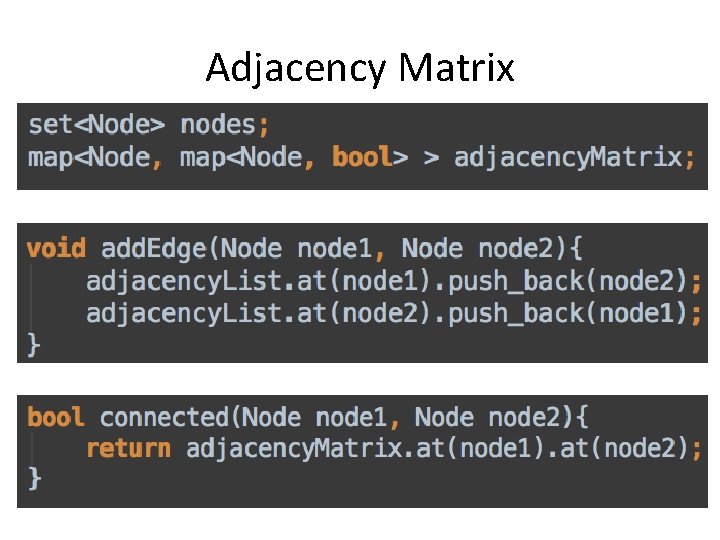
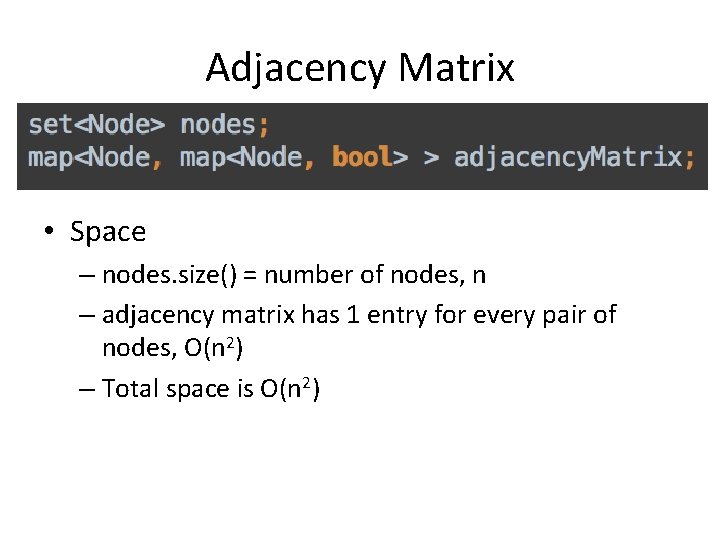
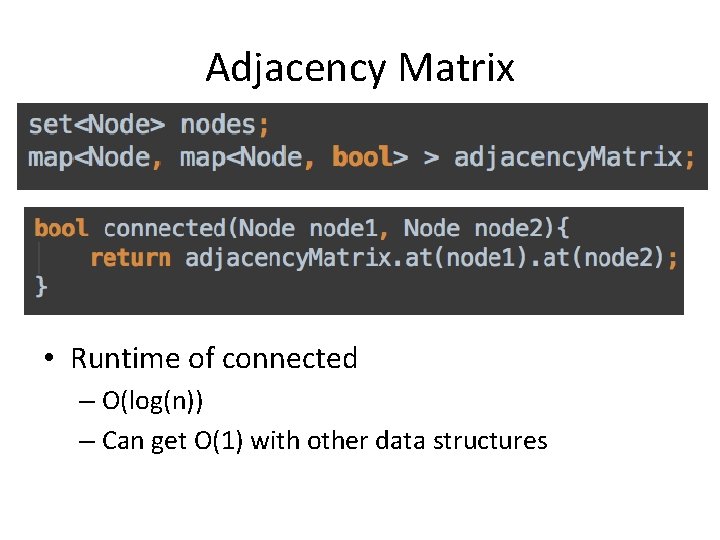
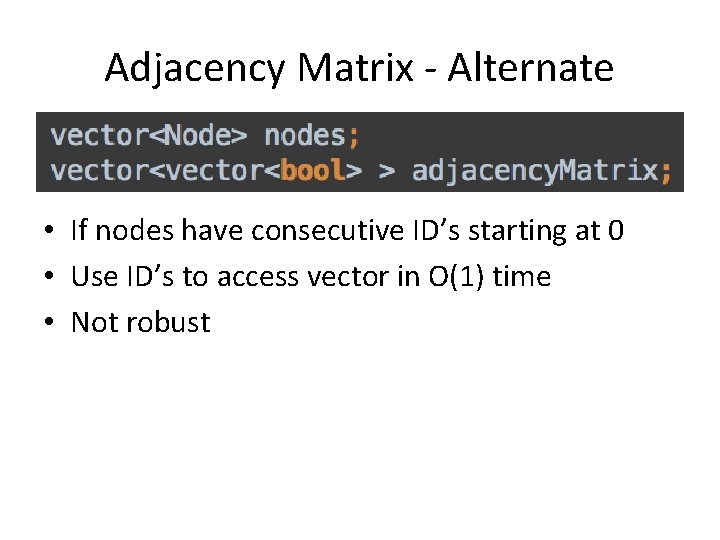
- Slides: 24
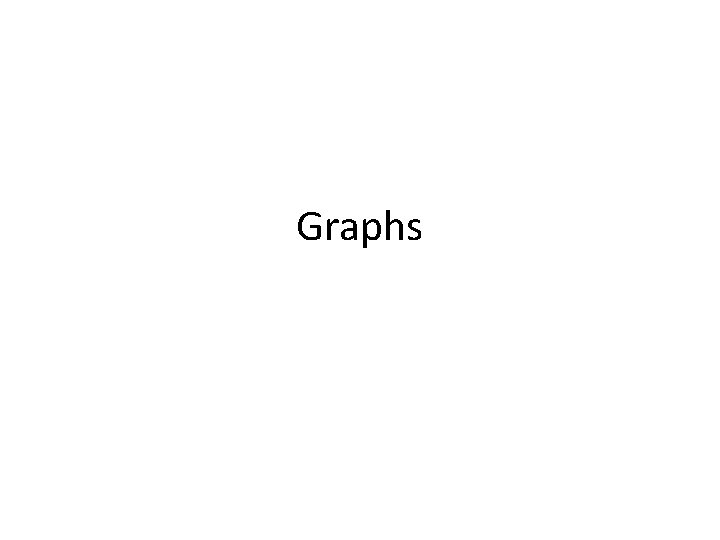
Graphs
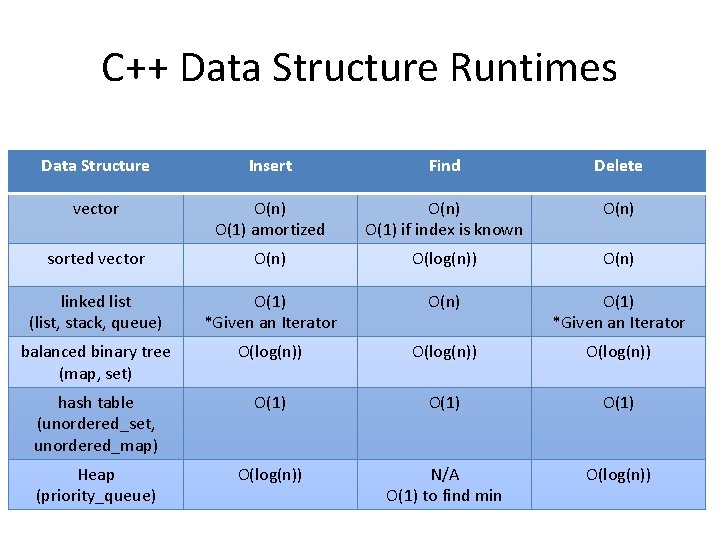
C++ Data Structure Runtimes Data Structure Insert Find Delete vector O(n) O(1) amortized O(n) O(1) if index is known O(n) sorted vector O(n) O(log(n)) O(n) linked list (list, stack, queue) O(1) *Given an Iterator O(n) O(1) *Given an Iterator balanced binary tree (map, set) O(log(n)) hash table (unordered_set, unordered_map) O(1) Heap (priority_queue) O(log(n)) N/A O(1) to find min O(log(n))
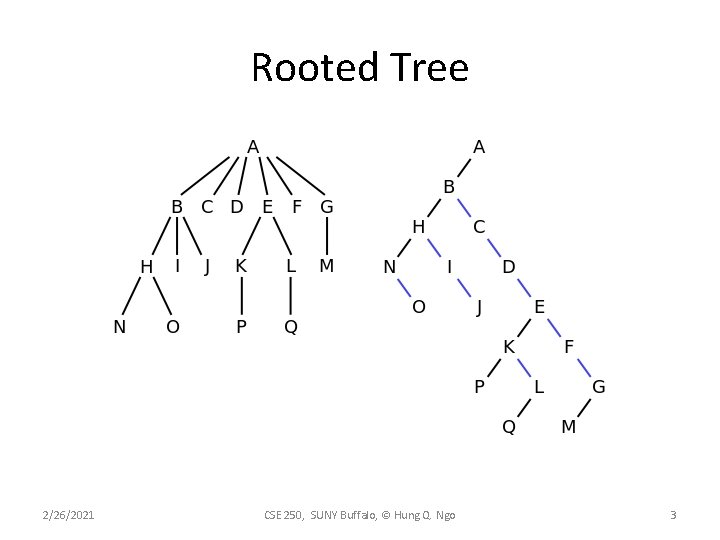
Rooted Tree 2/26/2021 CSE 250, SUNY Buffalo, © Hung Q. Ngo 3
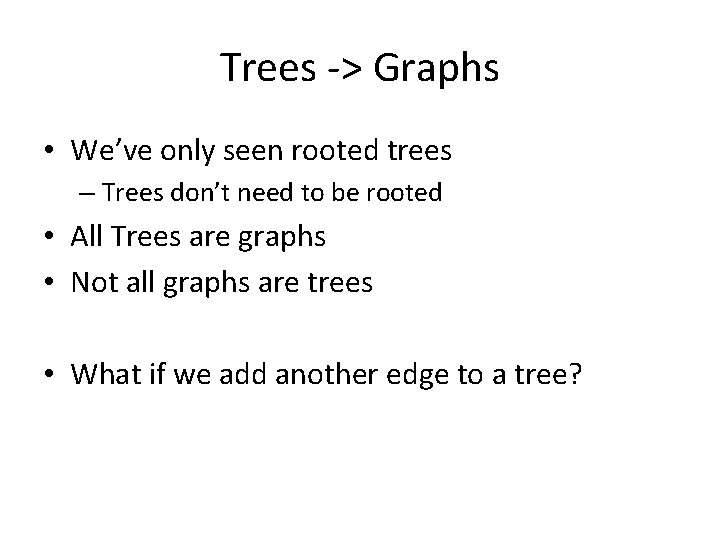
Trees -> Graphs • We’ve only seen rooted trees – Trees don’t need to be rooted • All Trees are graphs • Not all graphs are trees • What if we add another edge to a tree?
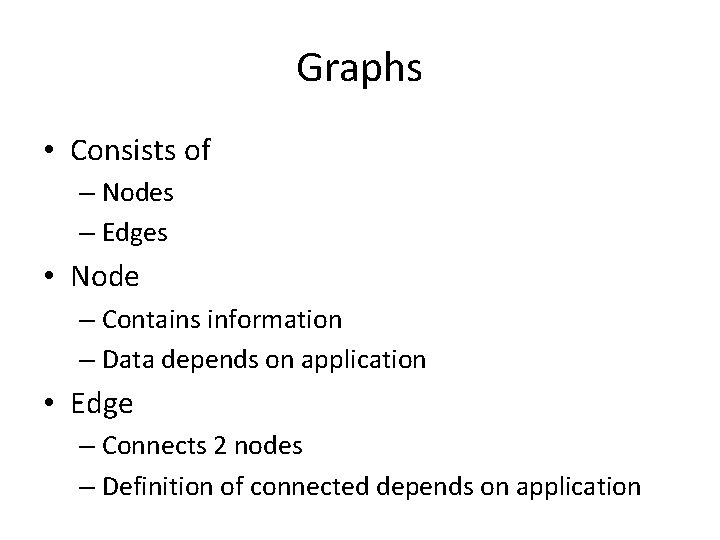
Graphs • Consists of – Nodes – Edges • Node – Contains information – Data depends on application • Edge – Connects 2 nodes – Definition of connected depends on application
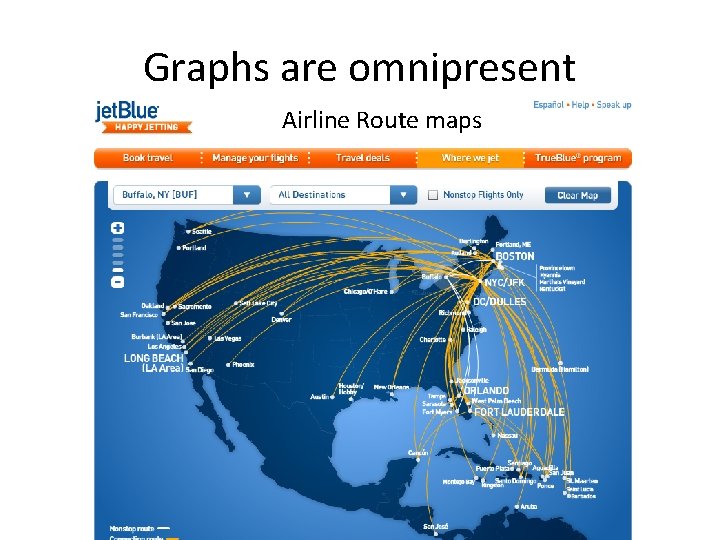
Graphs are omnipresent Airline Route maps
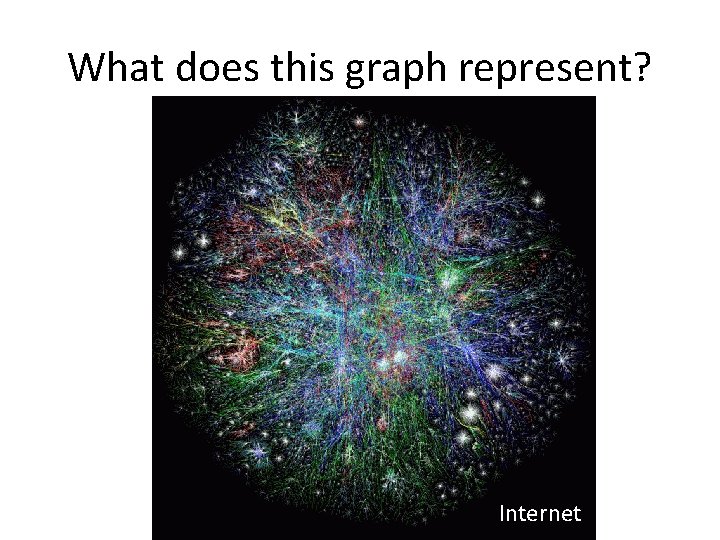
What does this graph represent? Internet
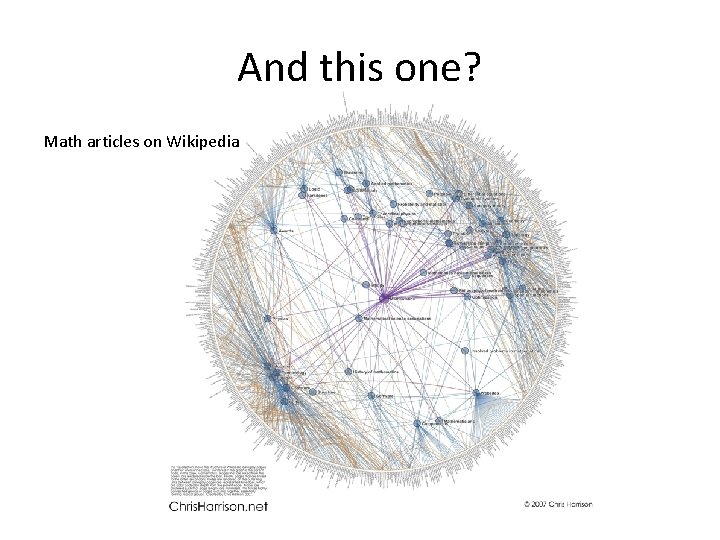
And this one? Math articles on Wikipedia
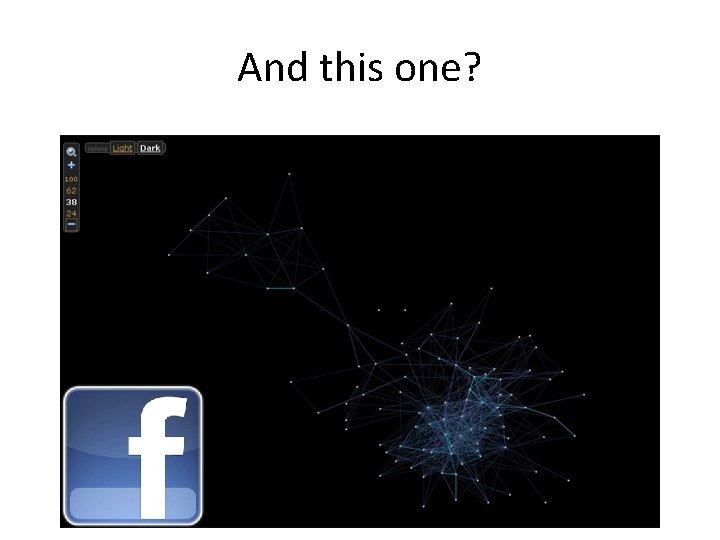
And this one?
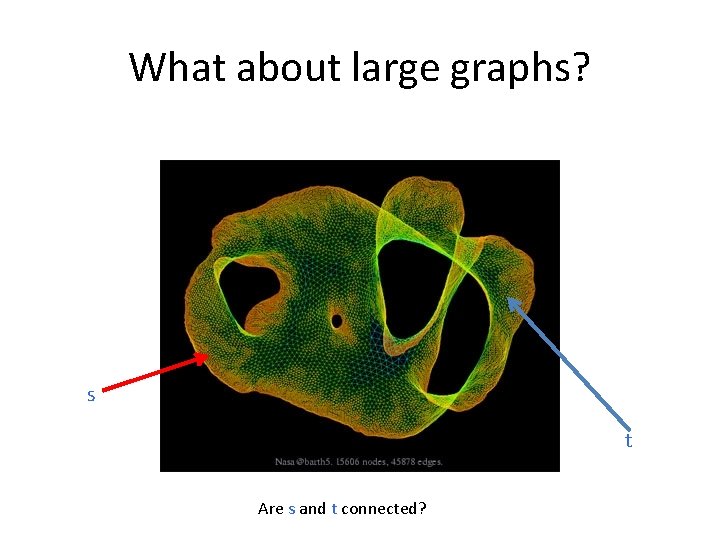
What about large graphs? s t Are s and t connected?
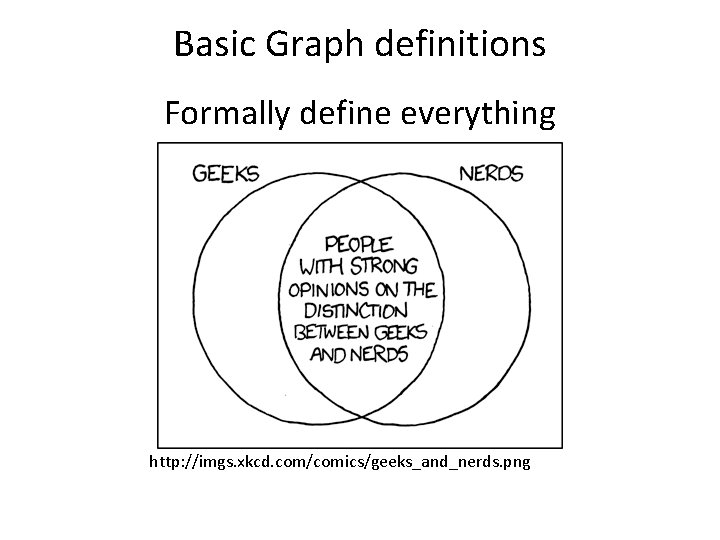
Basic Graph definitions Formally define everything http: //imgs. xkcd. com/comics/geeks_and_nerds. png
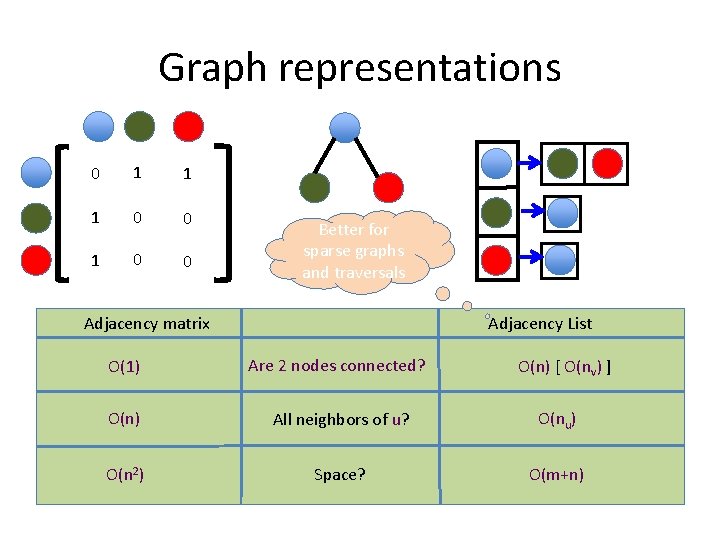
Graph representations 0 1 1 1 0 0 Better for sparse graphs and traversals Adjacency matrix Adjacency List O(1) Are 2 nodes connected? O(n) All neighbors of u? O(nu) O(n 2) Space? O(m+n) O(n) [ O(nv) ]
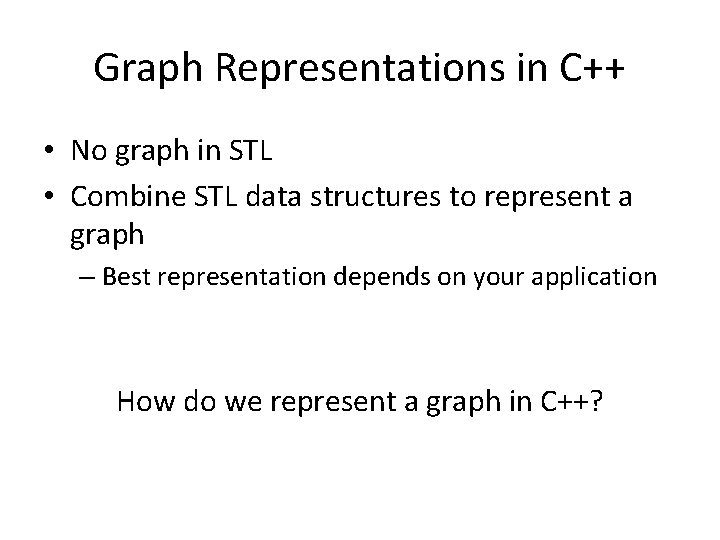
Graph Representations in C++ • No graph in STL • Combine STL data structures to represent a graph – Best representation depends on your application How do we represent a graph in C++?
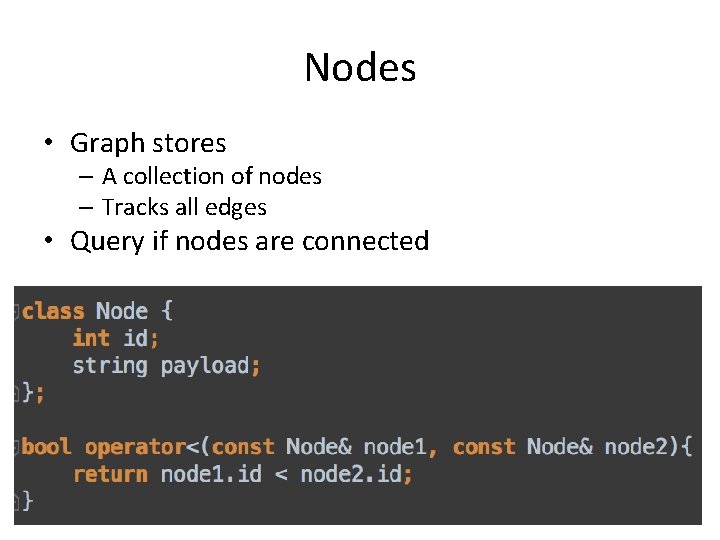
Nodes • Graph stores – A collection of nodes – Tracks all edges • Query if nodes are connected
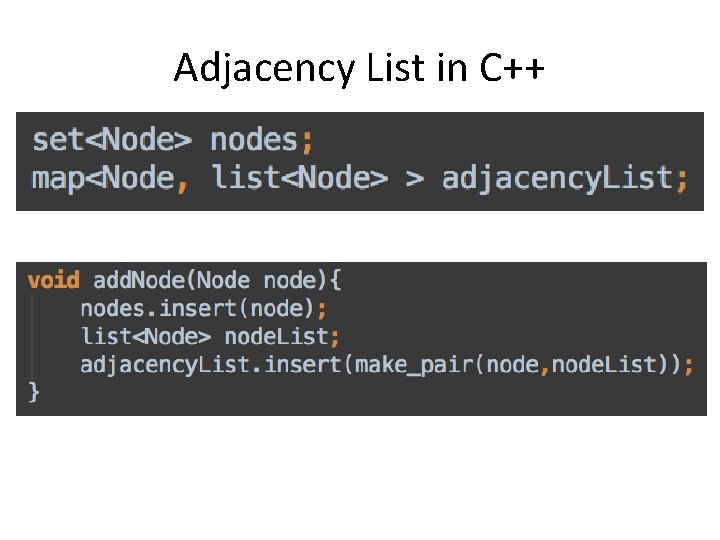
Adjacency List in C++
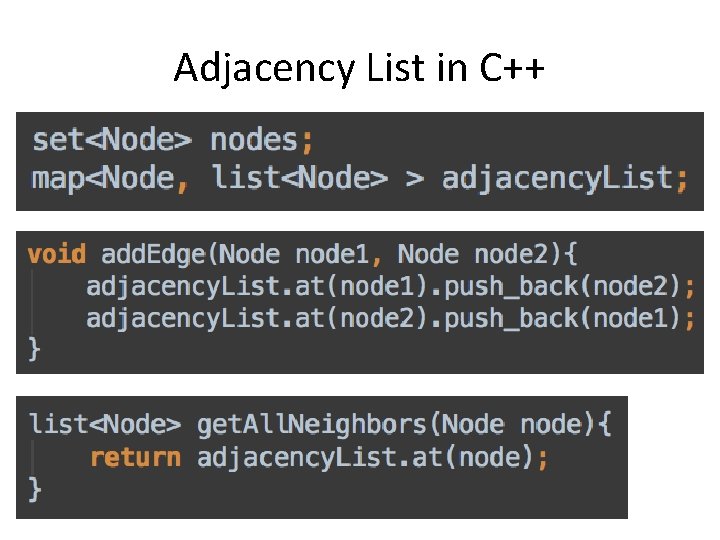
Adjacency List in C++
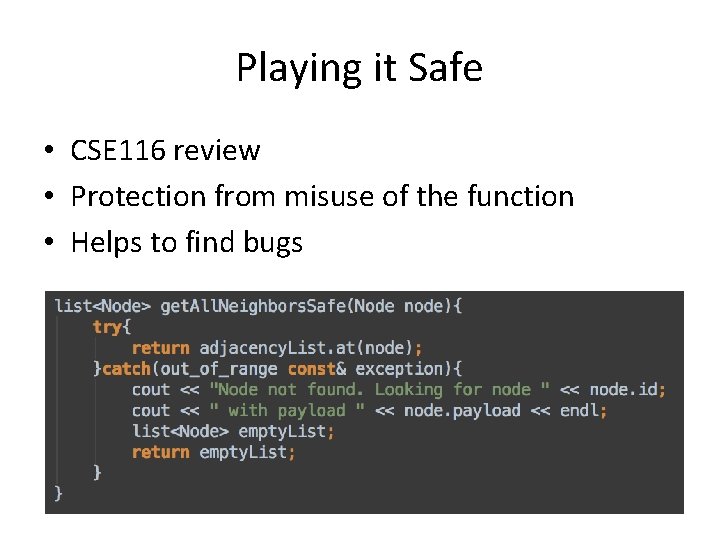
Playing it Safe • CSE 116 review • Protection from misuse of the function • Helps to find bugs
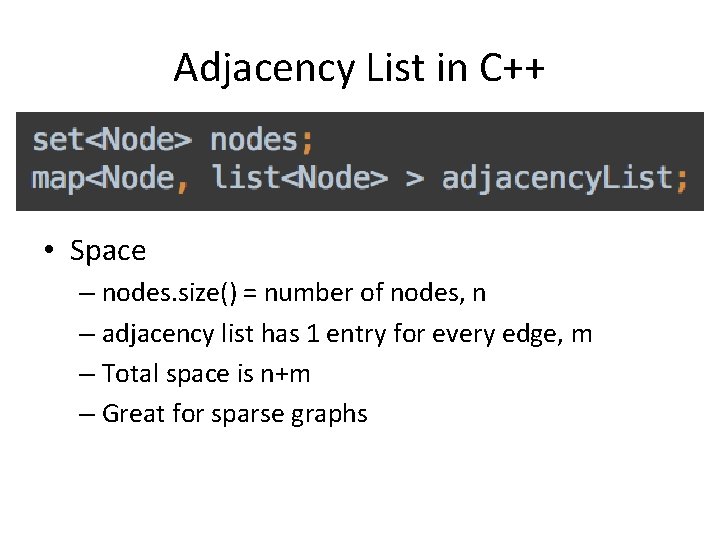
Adjacency List in C++ • Space – nodes. size() = number of nodes, n – adjacency list has 1 entry for every edge, m – Total space is n+m – Great for sparse graphs
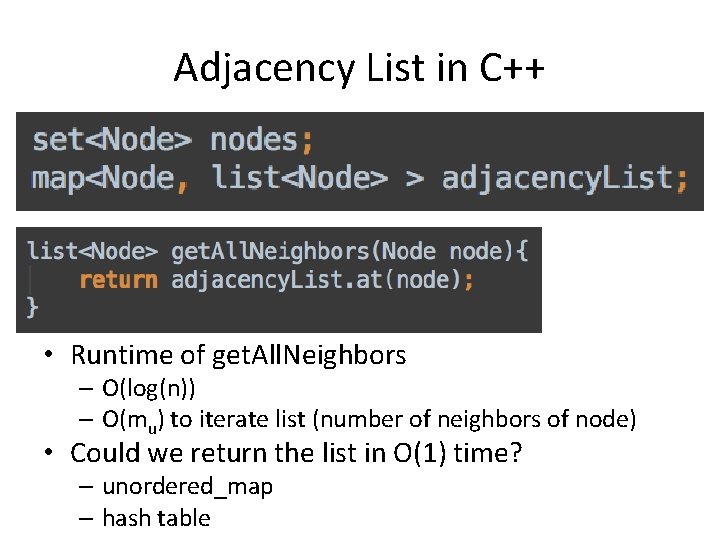
Adjacency List in C++ • Runtime of get. All. Neighbors – O(log(n)) – O(mu) to iterate list (number of neighbors of node) • Could we return the list in O(1) time? – unordered_map – hash table
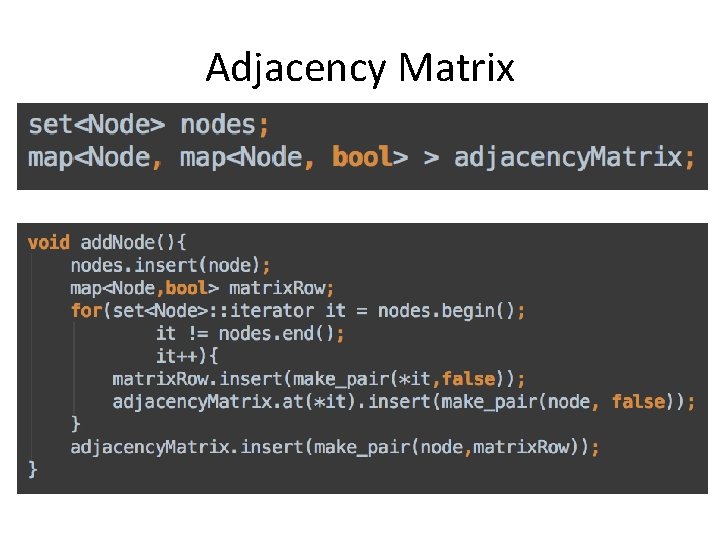
Adjacency Matrix
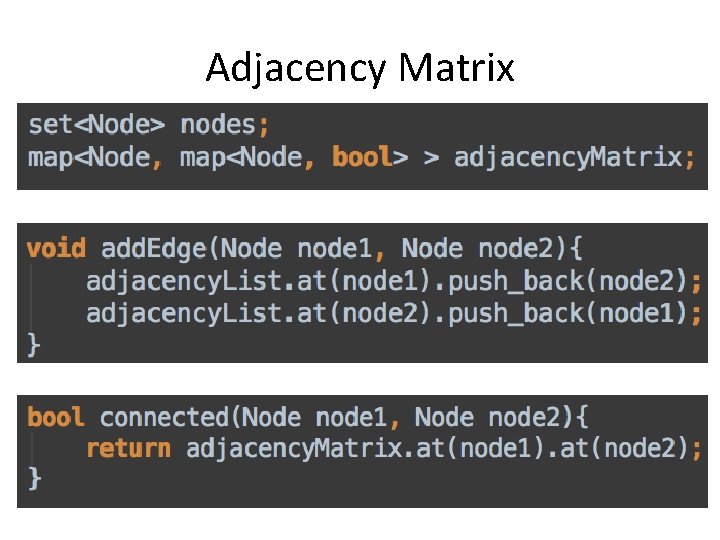
Adjacency Matrix
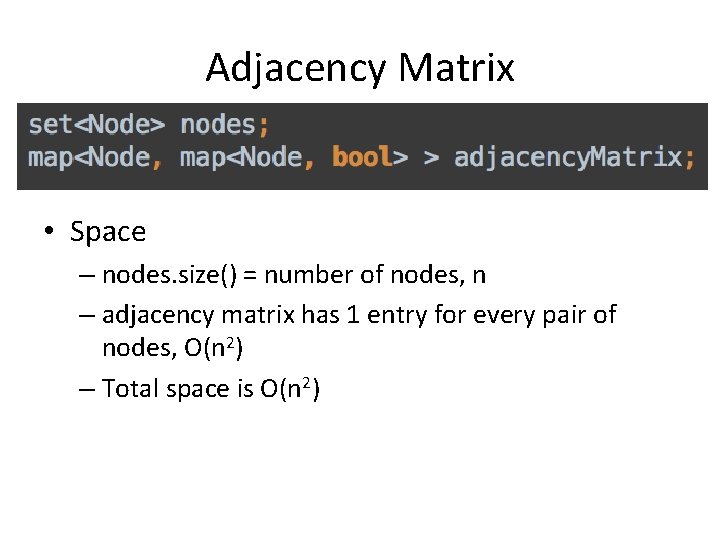
Adjacency Matrix • Space – nodes. size() = number of nodes, n – adjacency matrix has 1 entry for every pair of nodes, O(n 2) – Total space is O(n 2)
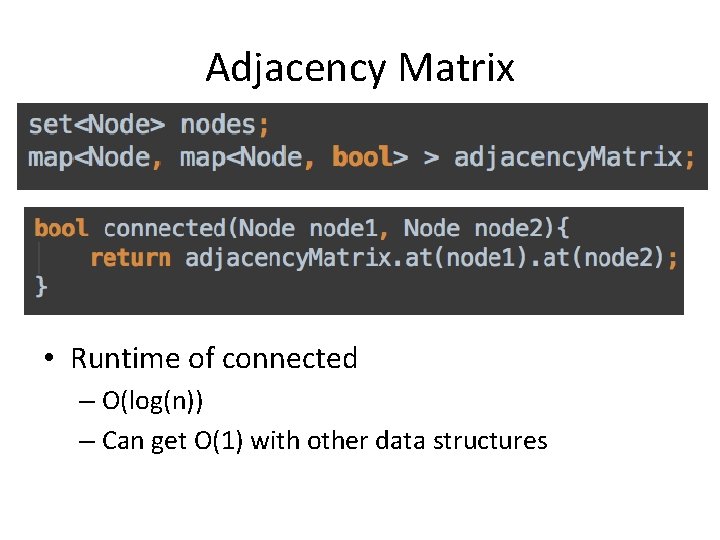
Adjacency Matrix • Runtime of connected – O(log(n)) – Can get O(1) with other data structures
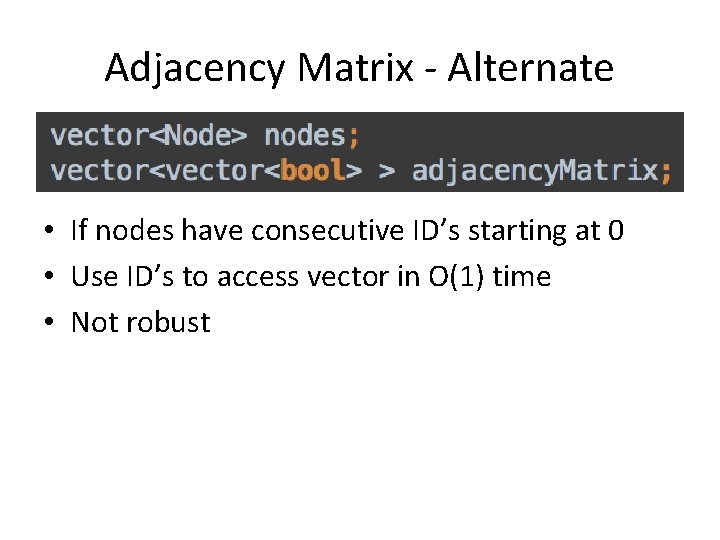
Adjacency Matrix - Alternate • If nodes have consecutive ID’s starting at 0 • Use ID’s to access vector in O(1) time • Not robust
Data structure runtimes
Software implementation of state graph
Speed and velocity
Graphs that enlighten and graphs that deceive
End behavior chart
Queue is a static data structure
Number of terms example
Structure in graphs of polynomial functions
Interpreting graphs science
Uniform bell-shaped skewed-right skewed-left
Scatter plot bivariate data
Displaying quantitative data
Data tables and graphs
Quote weaving examples
Void insert
Imipenem, cilastatin, and relebactam package insert
Actions of biceps brachii
ınsert tuşu
What is insert shot
Insert element in priority queue
Periodic table insert
Processing page
Name of logo
Horizontal
Atra flex coupling insert