Data Structures Lakshmish Ramaswamy Example Text editor software
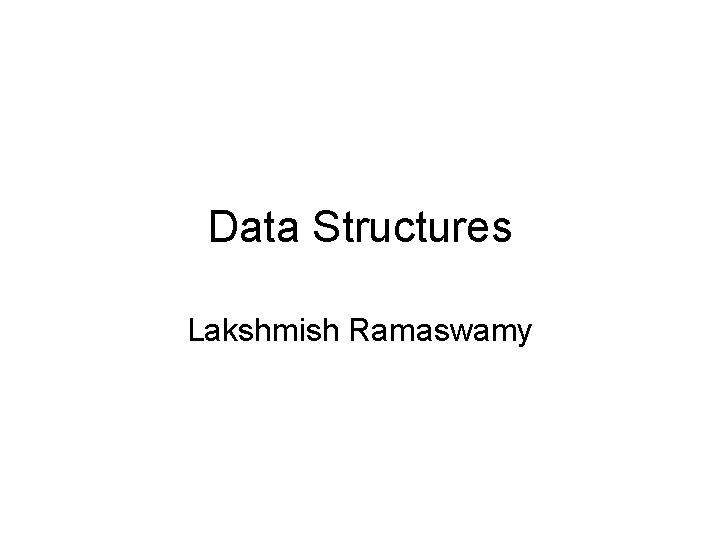
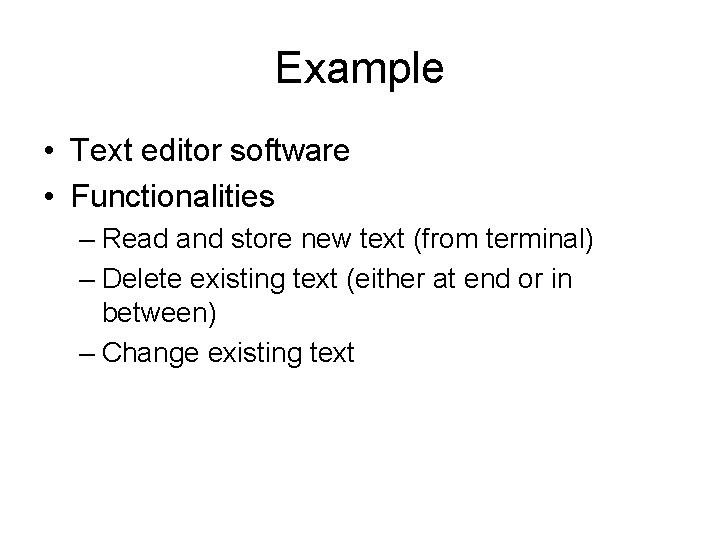
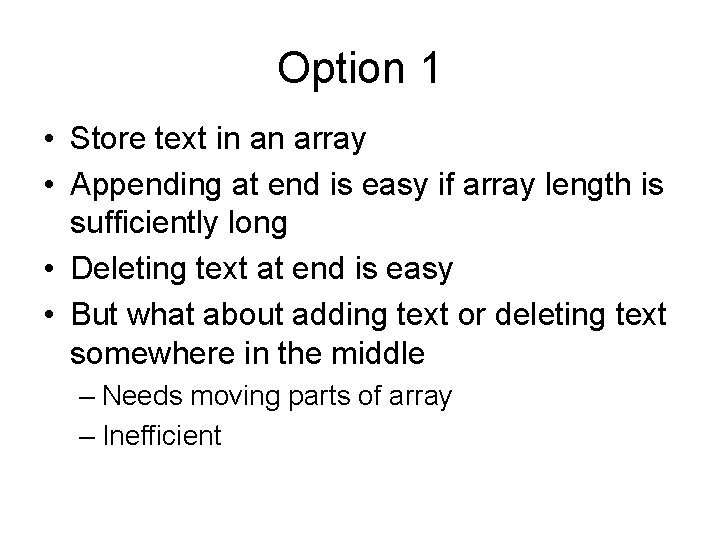
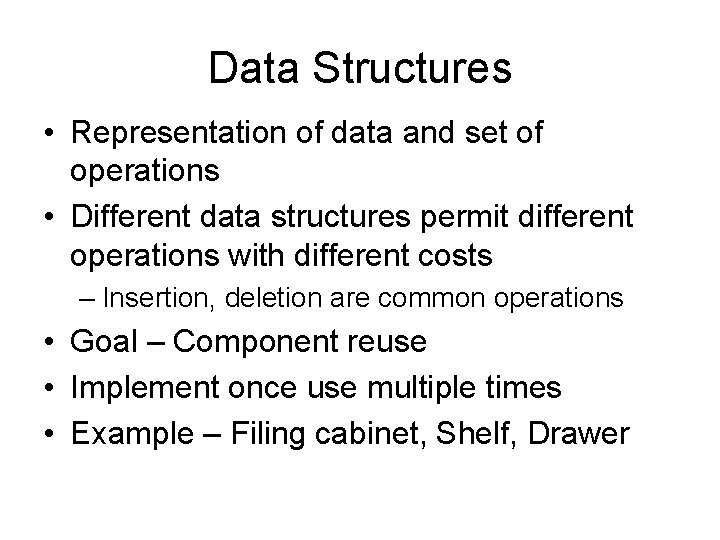
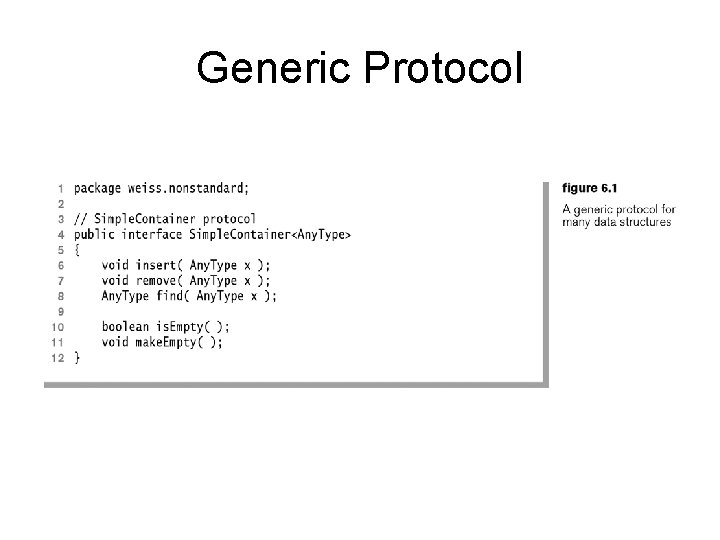
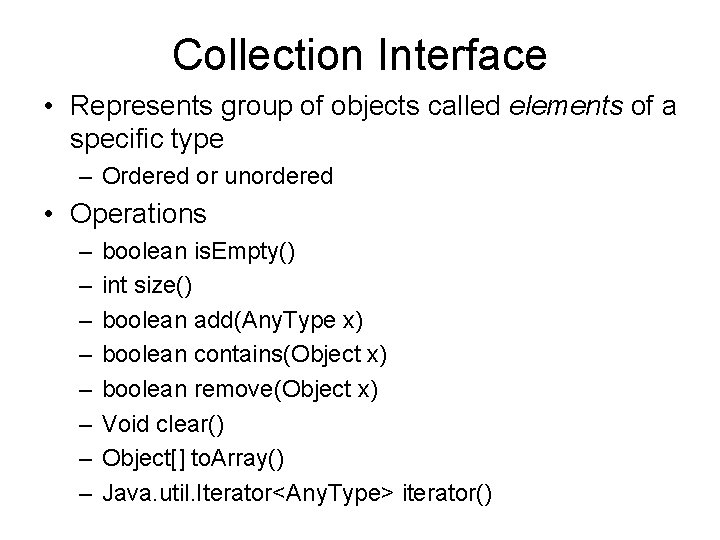
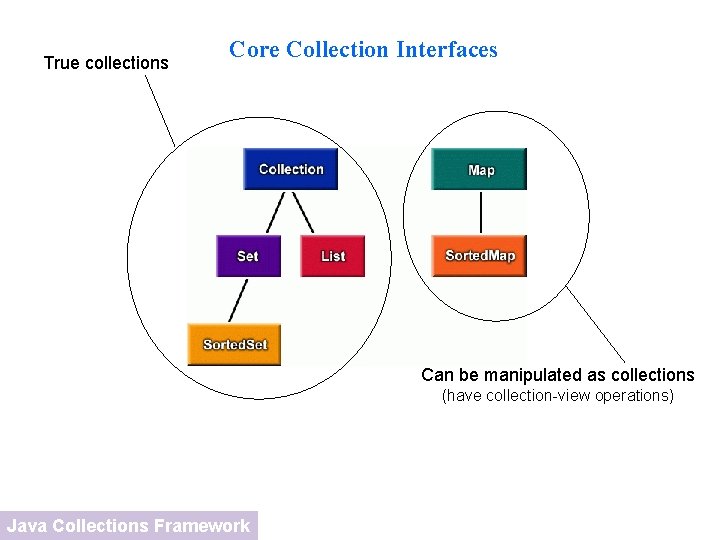
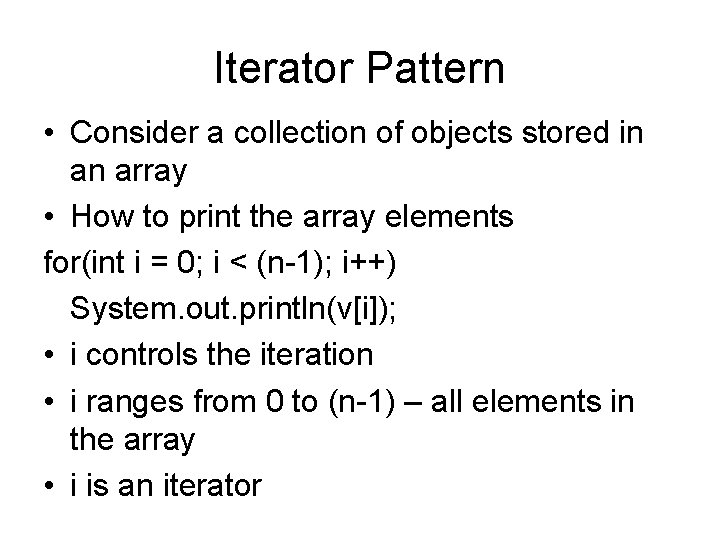
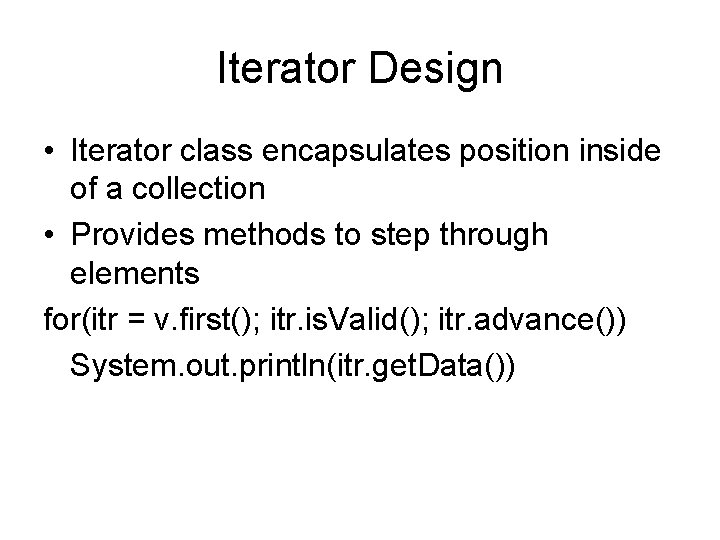
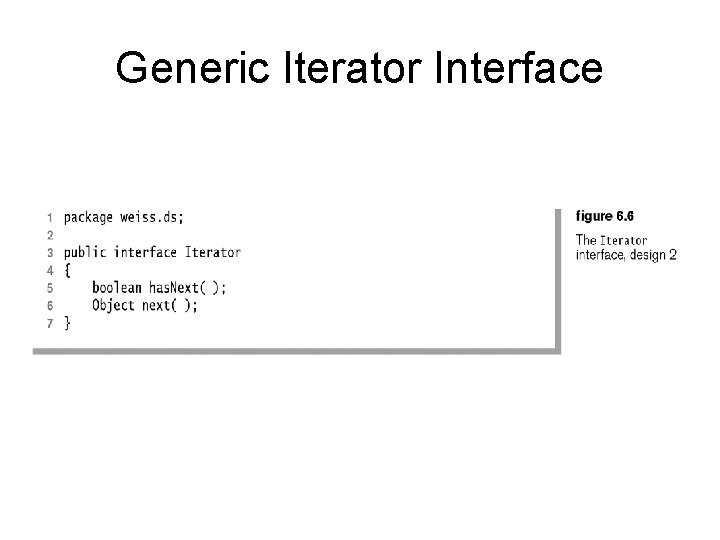
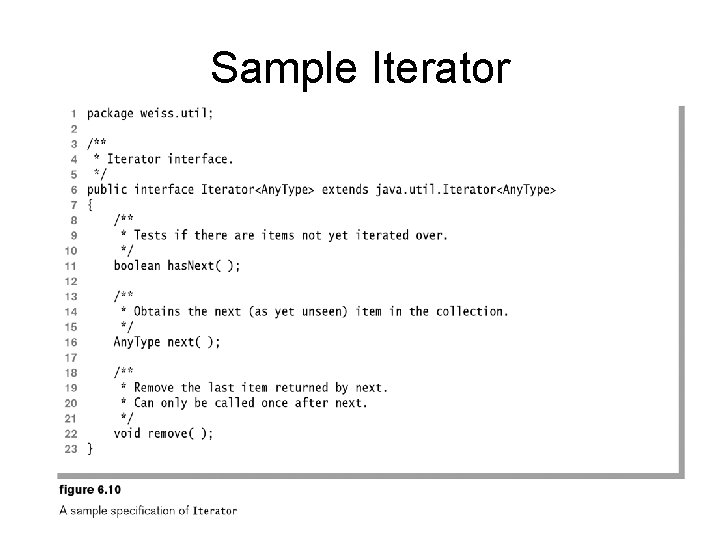
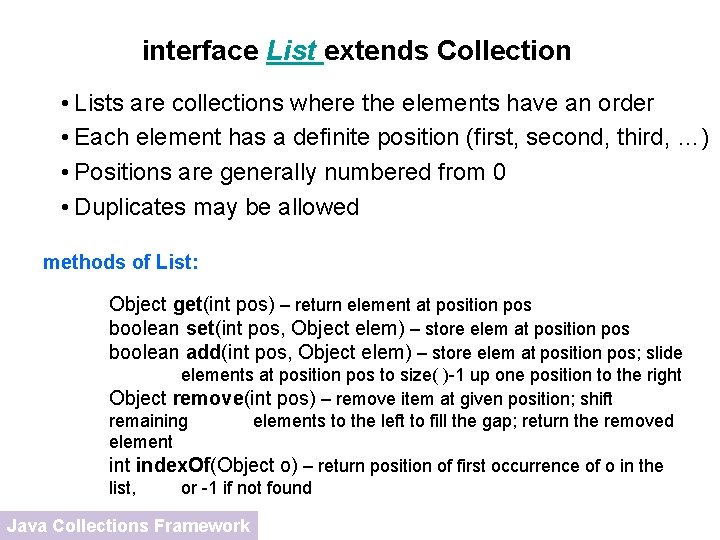
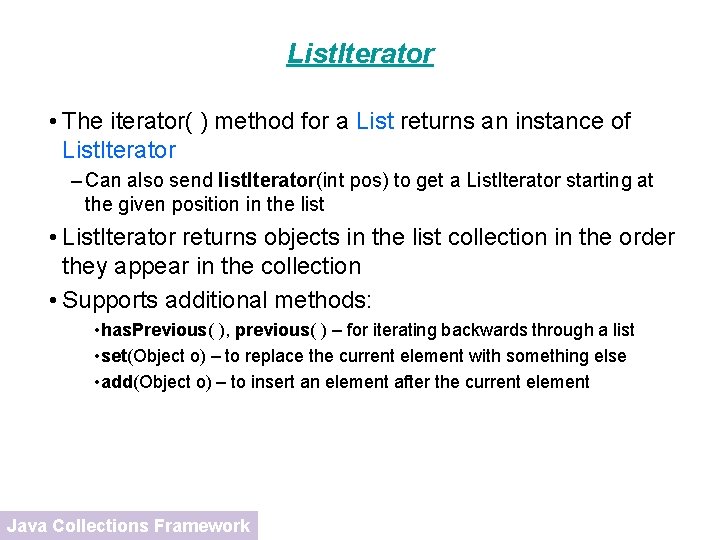
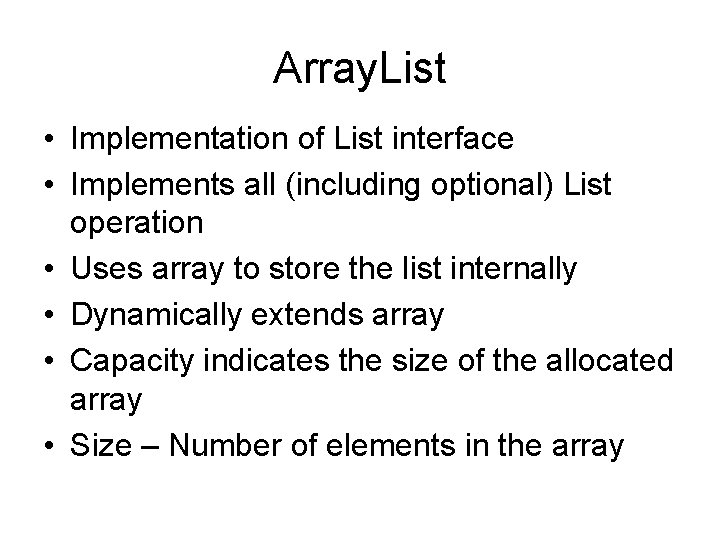
![Implementation public class Array. List implements List{ private object[] elements; private int capacity; private Implementation public class Array. List implements List{ private object[] elements; private int capacity; private](https://slidetodoc.com/presentation_image_h2/3eb1917577390cb56cea0bcc96b5a12e/image-15.jpg)
![Constructors public Array. List(){ capacity = 10; size = 0; elements = new object[capacity]; Constructors public Array. List(){ capacity = 10; size = 0; elements = new object[capacity];](https://slidetodoc.com/presentation_image_h2/3eb1917577390cb56cea0bcc96b5a12e/image-16.jpg)
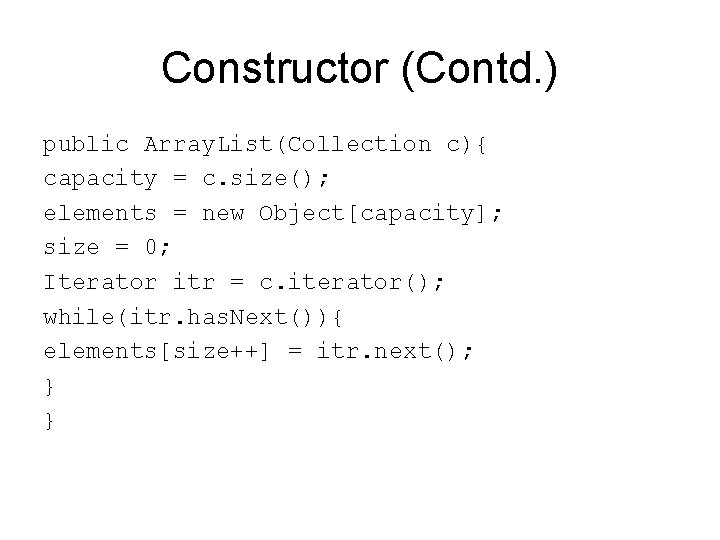
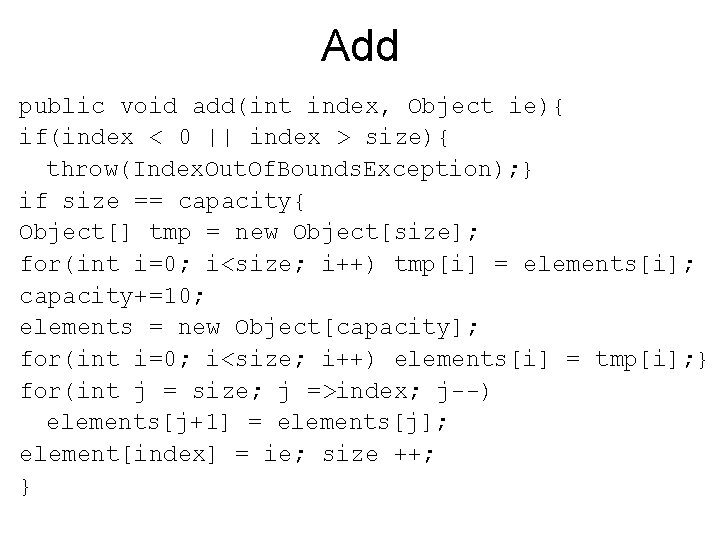
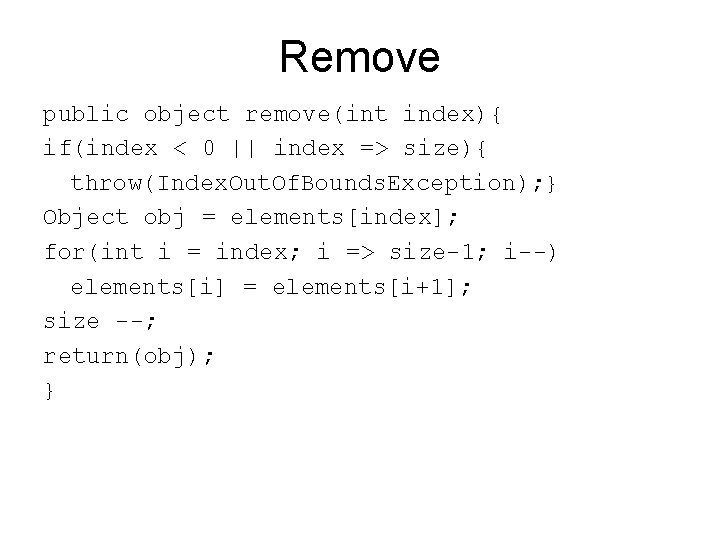
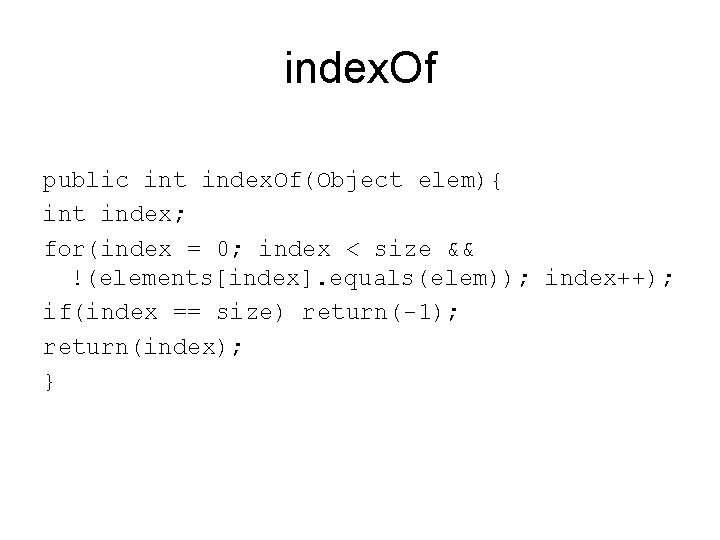
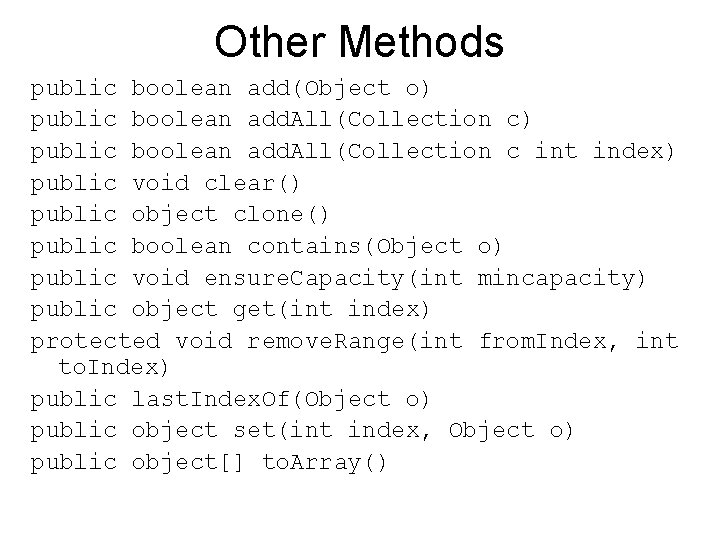
- Slides: 21
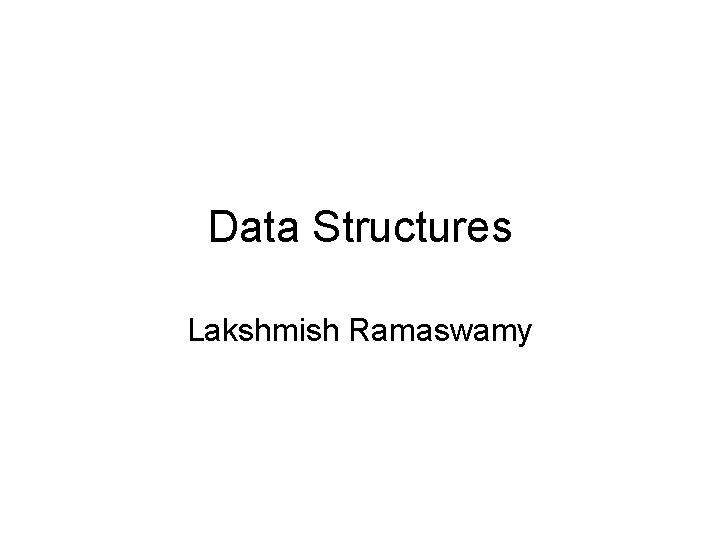
Data Structures Lakshmish Ramaswamy
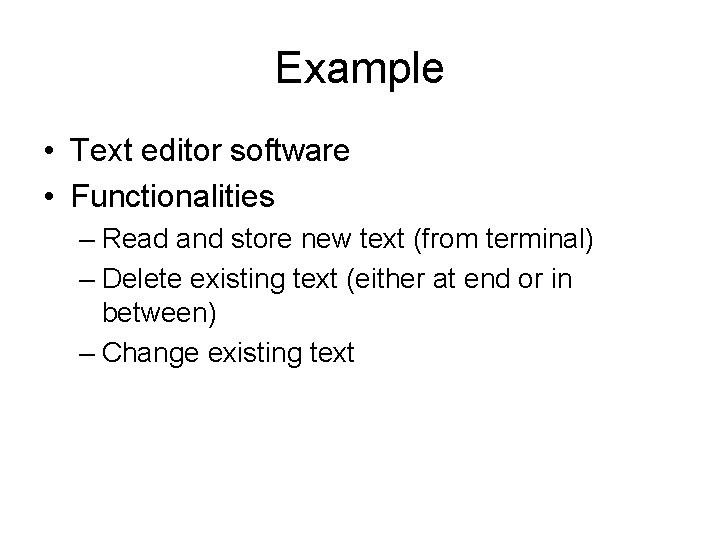
Example • Text editor software • Functionalities – Read and store new text (from terminal) – Delete existing text (either at end or in between) – Change existing text
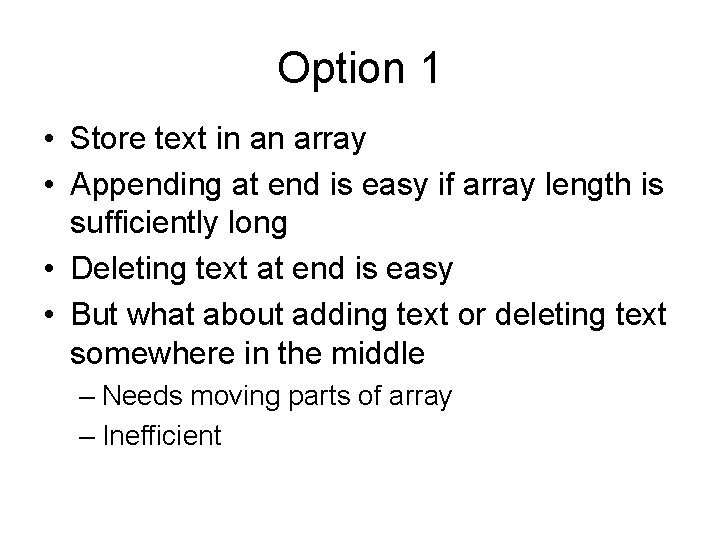
Option 1 • Store text in an array • Appending at end is easy if array length is sufficiently long • Deleting text at end is easy • But what about adding text or deleting text somewhere in the middle – Needs moving parts of array – Inefficient
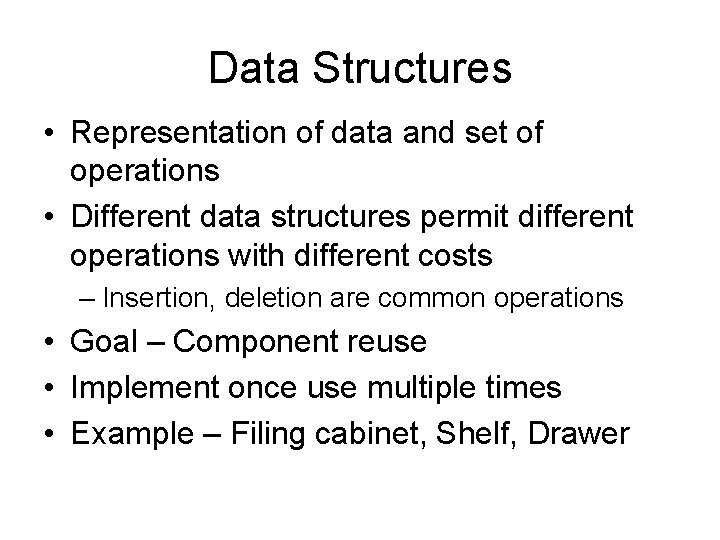
Data Structures • Representation of data and set of operations • Different data structures permit different operations with different costs – Insertion, deletion are common operations • Goal – Component reuse • Implement once use multiple times • Example – Filing cabinet, Shelf, Drawer
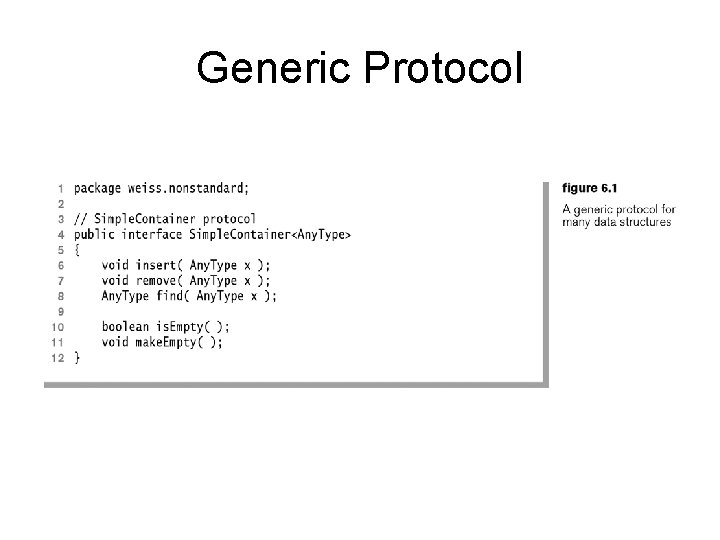
Generic Protocol
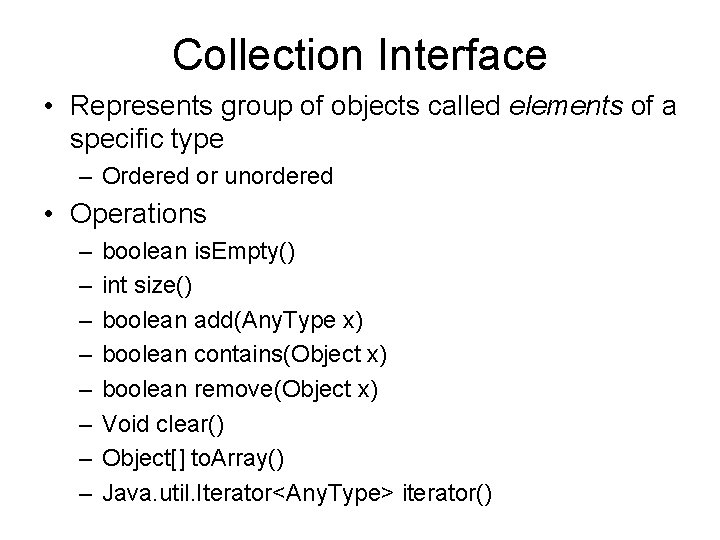
Collection Interface • Represents group of objects called elements of a specific type – Ordered or unordered • Operations – – – – boolean is. Empty() int size() boolean add(Any. Type x) boolean contains(Object x) boolean remove(Object x) Void clear() Object[] to. Array() Java. util. Iterator<Any. Type> iterator()
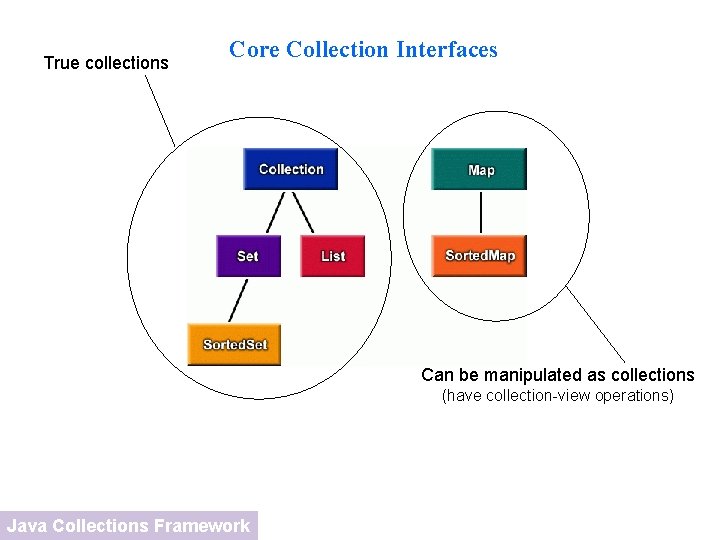
True collections Core Collection Interfaces Can be manipulated as collections (have collection-view operations) Java Collections Framework
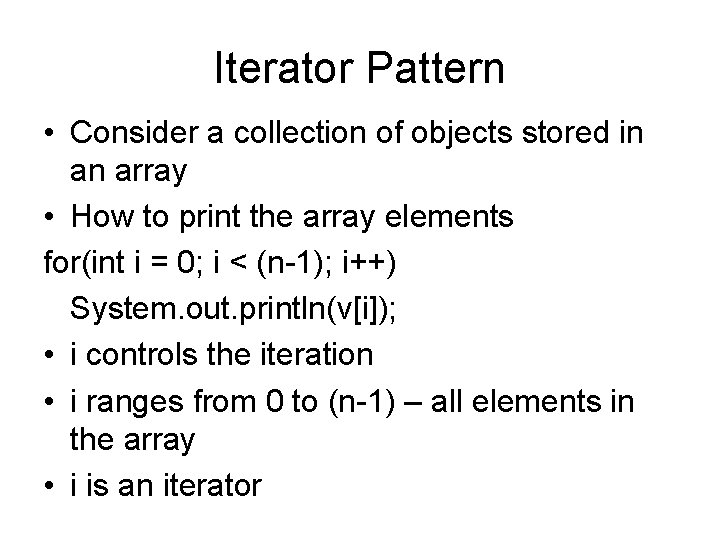
Iterator Pattern • Consider a collection of objects stored in an array • How to print the array elements for(int i = 0; i < (n-1); i++) System. out. println(v[i]); • i controls the iteration • i ranges from 0 to (n-1) – all elements in the array • i is an iterator
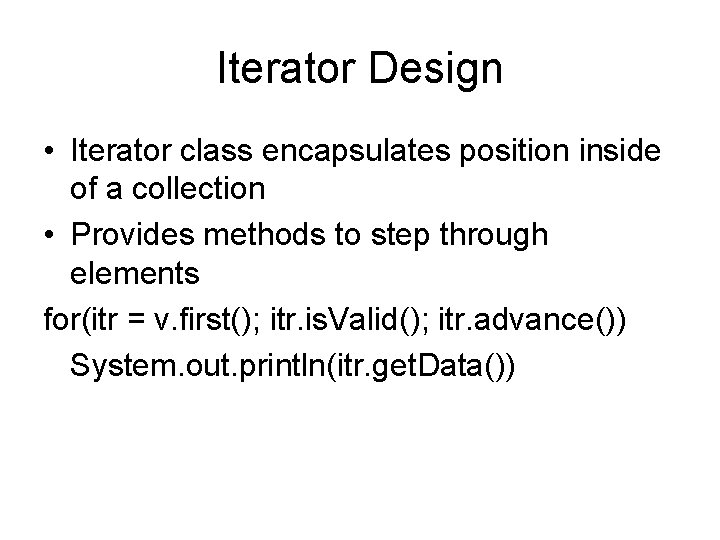
Iterator Design • Iterator class encapsulates position inside of a collection • Provides methods to step through elements for(itr = v. first(); itr. is. Valid(); itr. advance()) System. out. println(itr. get. Data())
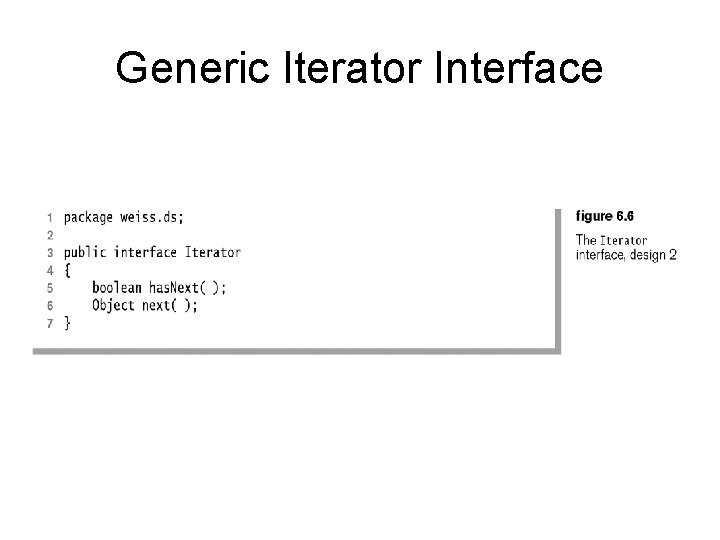
Generic Iterator Interface
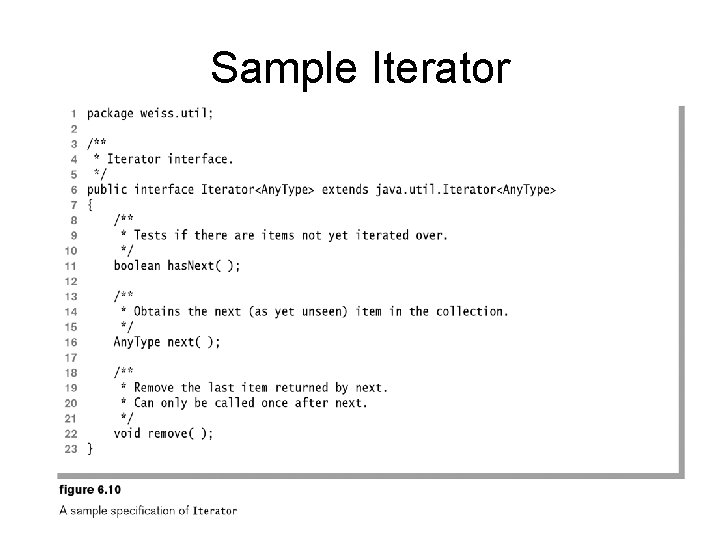
Sample Iterator
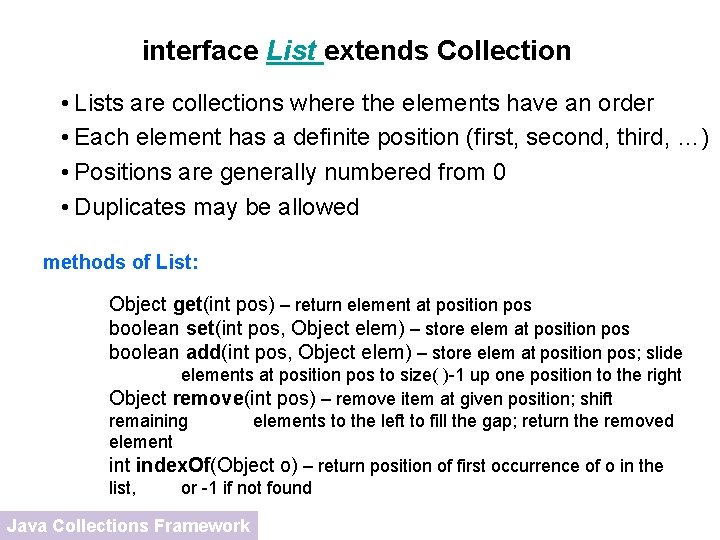
interface List extends Collection • Lists are collections where the elements have an order • Each element has a definite position (first, second, third, …) • Positions are generally numbered from 0 • Duplicates may be allowed methods of List: Object get(int pos) – return element at position pos boolean set(int pos, Object elem) – store elem at position pos boolean add(int pos, Object elem) – store elem at position pos; slide elements at position pos to size( )-1 up one position to the right Object remove(int pos) – remove item at given position; shift remaining elements to the left to fill the gap; return the removed element index. Of(Object o) – return position of first occurrence of o in the list, or -1 if not found Java Collections Framework
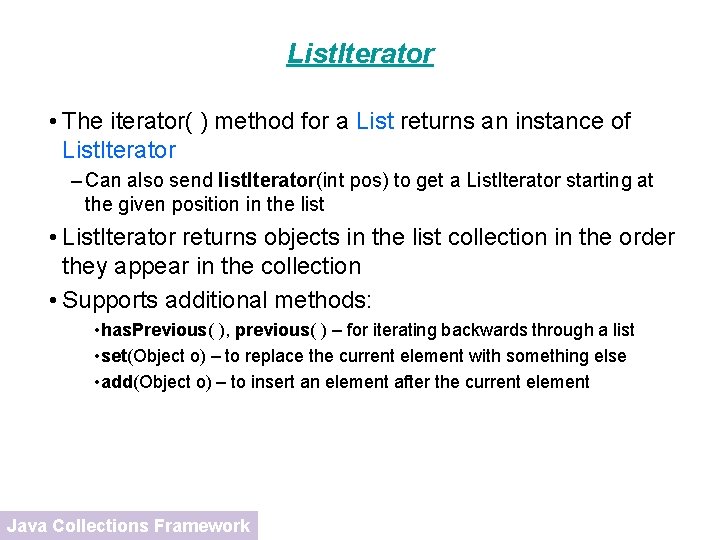
List. Iterator • The iterator( ) method for a List returns an instance of List. Iterator – Can also send list. Iterator(int pos) to get a List. Iterator starting at the given position in the list • List. Iterator returns objects in the list collection in the order they appear in the collection • Supports additional methods: • has. Previous( ), previous( ) – for iterating backwards through a list • set(Object o) – to replace the current element with something else • add(Object o) – to insert an element after the current element Java Collections Framework
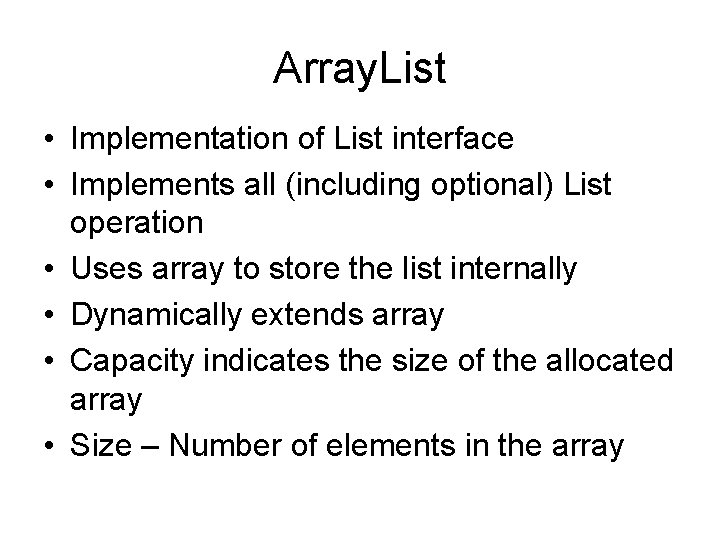
Array. List • Implementation of List interface • Implements all (including optional) List operation • Uses array to store the list internally • Dynamically extends array • Capacity indicates the size of the allocated array • Size – Number of elements in the array
![Implementation public class Array List implements List private object elements private int capacity private Implementation public class Array. List implements List{ private object[] elements; private int capacity; private](https://slidetodoc.com/presentation_image_h2/3eb1917577390cb56cea0bcc96b5a12e/image-15.jpg)
Implementation public class Array. List implements List{ private object[] elements; private int capacity; private int size; }
![Constructors public Array List capacity 10 size 0 elements new objectcapacity Constructors public Array. List(){ capacity = 10; size = 0; elements = new object[capacity];](https://slidetodoc.com/presentation_image_h2/3eb1917577390cb56cea0bcc96b5a12e/image-16.jpg)
Constructors public Array. List(){ capacity = 10; size = 0; elements = new object[capacity]; } public Array. List(int initialcapacity){ capacity = initialcapacity; size = 0; elements = new object[capacity]; }
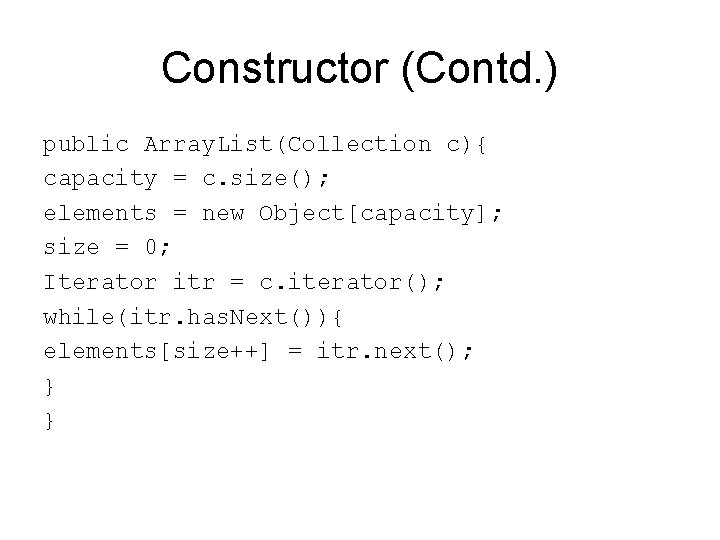
Constructor (Contd. ) public Array. List(Collection c){ capacity = c. size(); elements = new Object[capacity]; size = 0; Iterator itr = c. iterator(); while(itr. has. Next()){ elements[size++] = itr. next(); } }
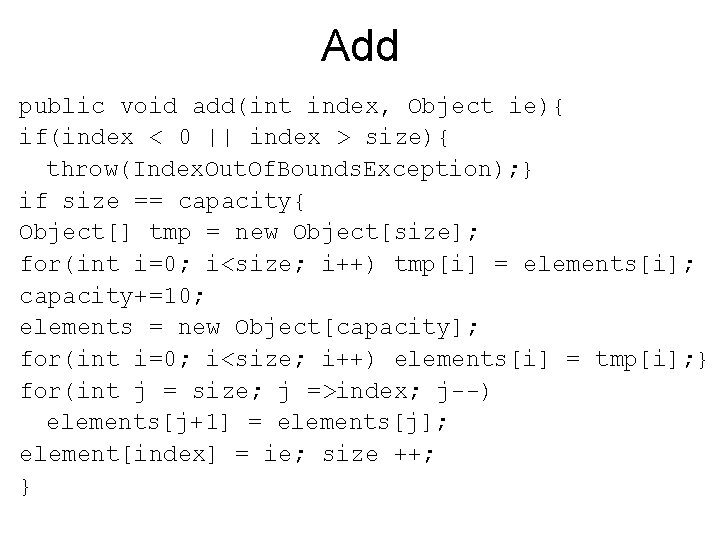
Add public void add(int index, Object ie){ if(index < 0 || index > size){ throw(Index. Out. Of. Bounds. Exception); } if size == capacity{ Object[] tmp = new Object[size]; for(int i=0; i<size; i++) tmp[i] = elements[i]; capacity+=10; elements = new Object[capacity]; for(int i=0; i<size; i++) elements[i] = tmp[i]; } for(int j = size; j =>index; j--) elements[j+1] = elements[j]; element[index] = ie; size ++; }
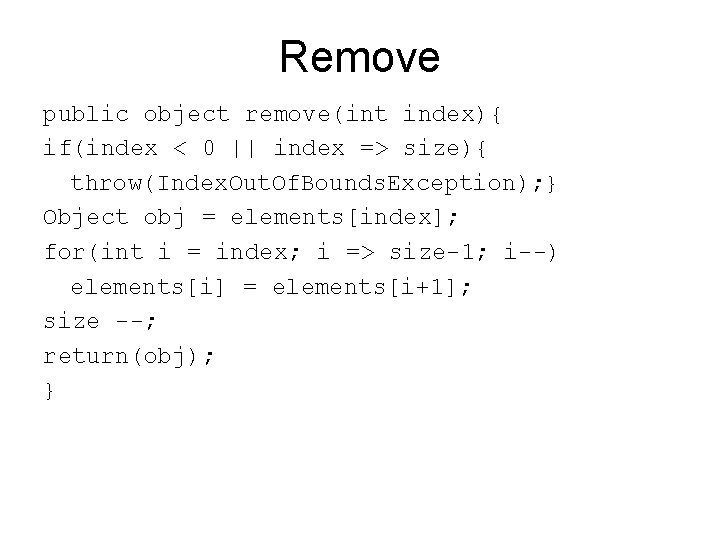
Remove public object remove(int index){ if(index < 0 || index => size){ throw(Index. Out. Of. Bounds. Exception); } Object obj = elements[index]; for(int i = index; i => size-1; i--) elements[i] = elements[i+1]; size --; return(obj); }
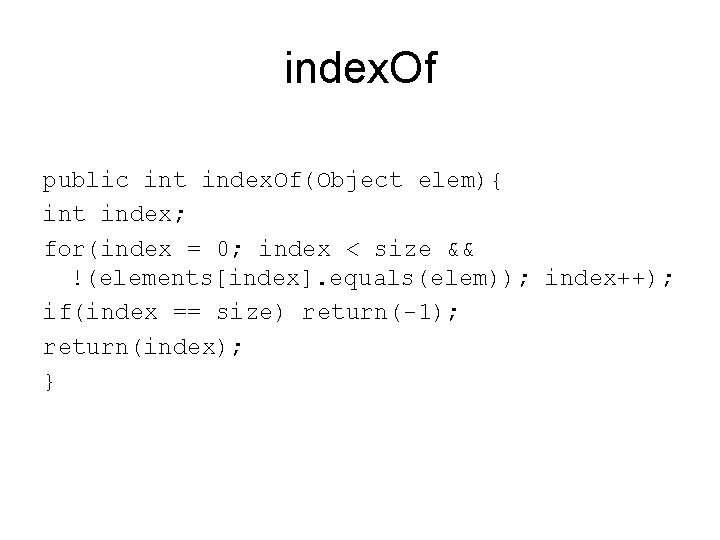
index. Of public int index. Of(Object elem){ int index; for(index = 0; index < size && !(elements[index]. equals(elem)); index++); if(index == size) return(-1); return(index); }
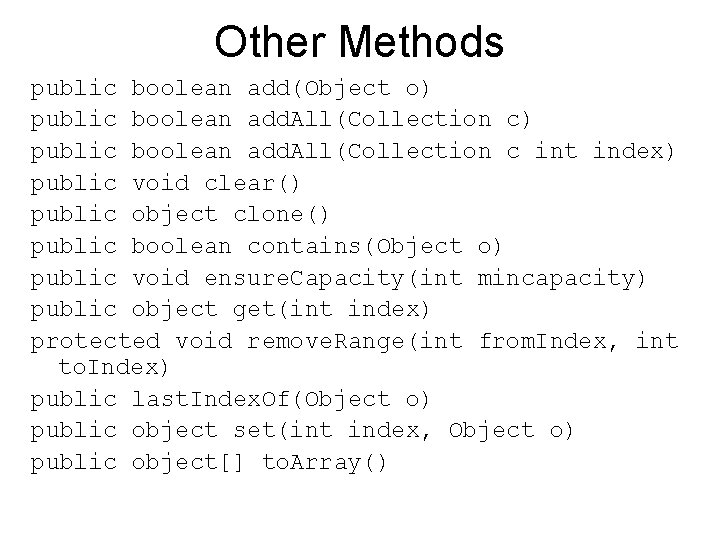
Other Methods public boolean add(Object o) public boolean add. All(Collection c int index) public void clear() public object clone() public boolean contains(Object o) public void ensure. Capacity(int mincapacity) public object get(int index) protected void remove. Range(int from. Index, int to. Index) public last. Index. Of(Object o) public object set(int index, Object o) public object[] to. Array()
Lakshmish ramaswamy
Nodes in blender
Making connections images
Data structure for text editor
Suresh sundaresan
Empno ename sal a822 ramaswamy
Prema ramaswamy md
Text features
Homologous structures example
Sitecore rich text editor custom button
Classical text editor
Fungsi fasilitas text editor untuk dos adalah
In lexi document editor for undoable user operation
Usna summer seminar
Simple text editor softuni
Linkage loader and linkage editor
Spss syntax editor
Stata infix
Hec-ras deck roadway data editor
Aoifesnotes
Transitions text structure
Identify the text type