Introduction Of Queue Introduction n n A queue
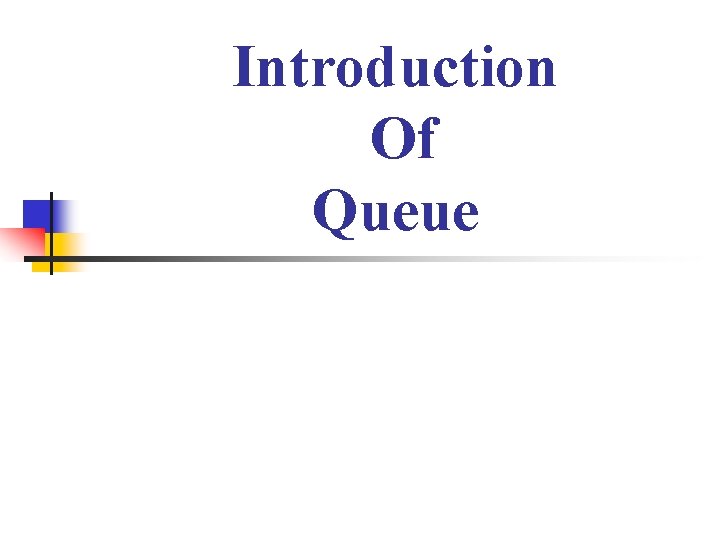
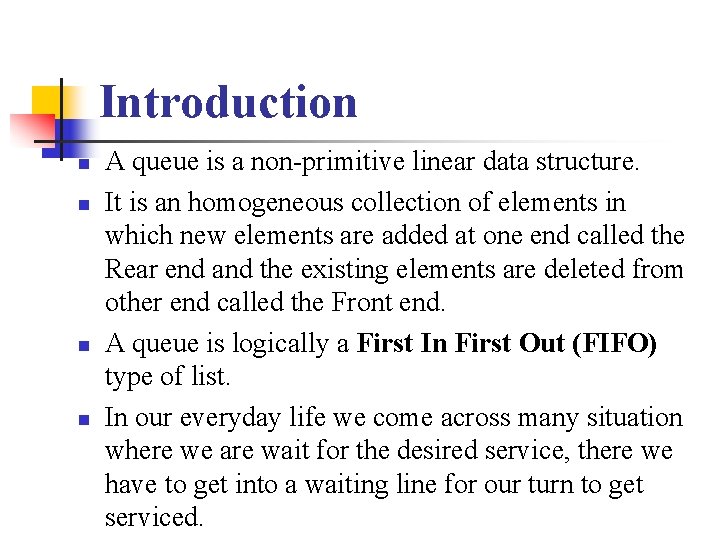
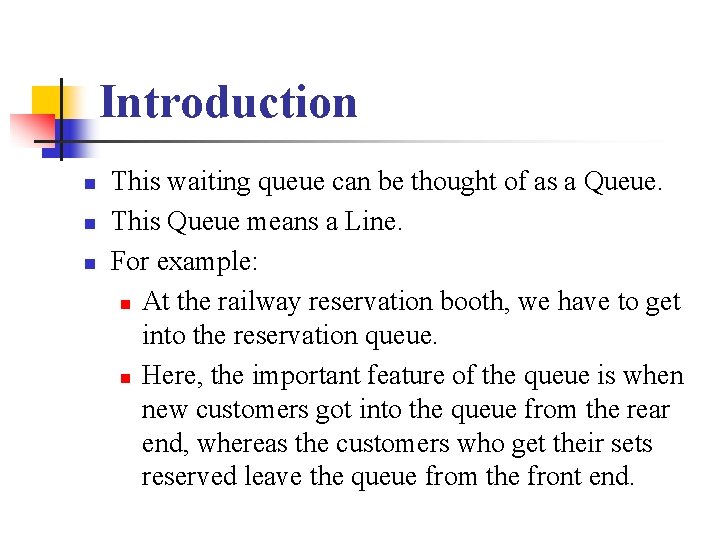
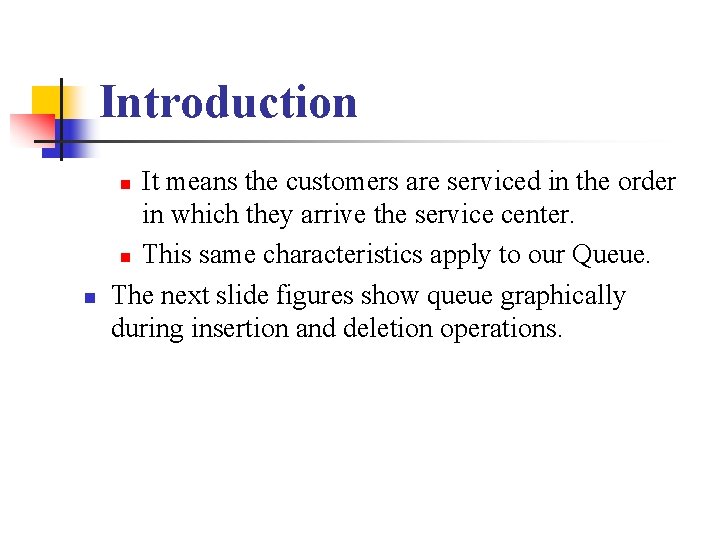
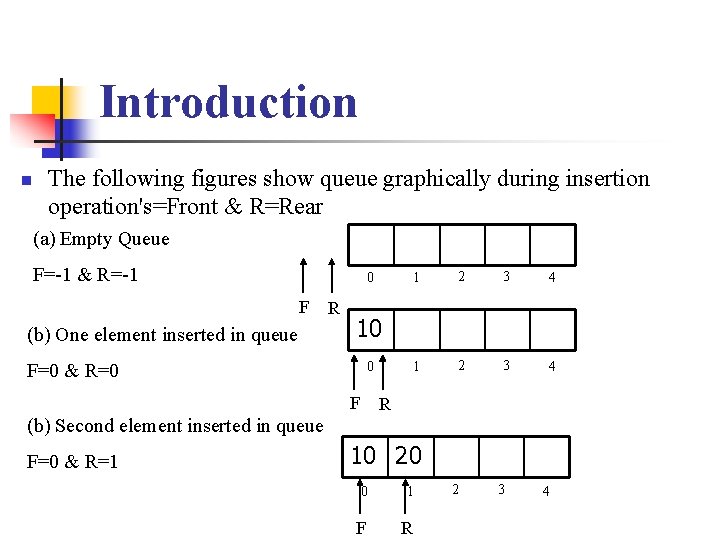
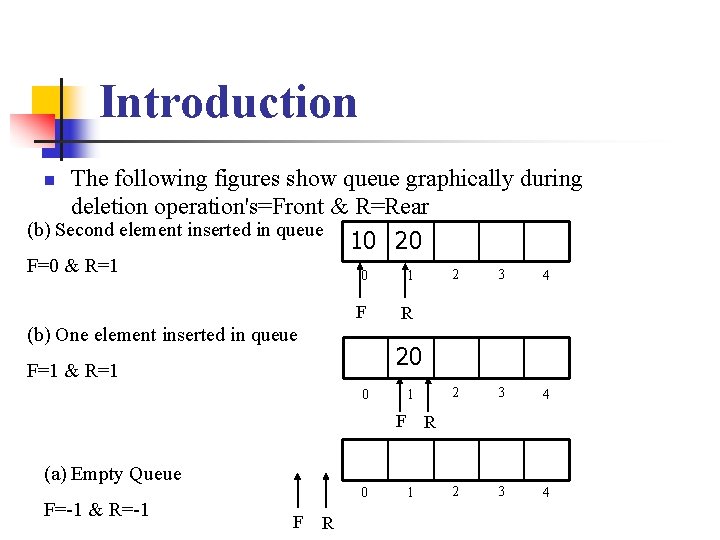
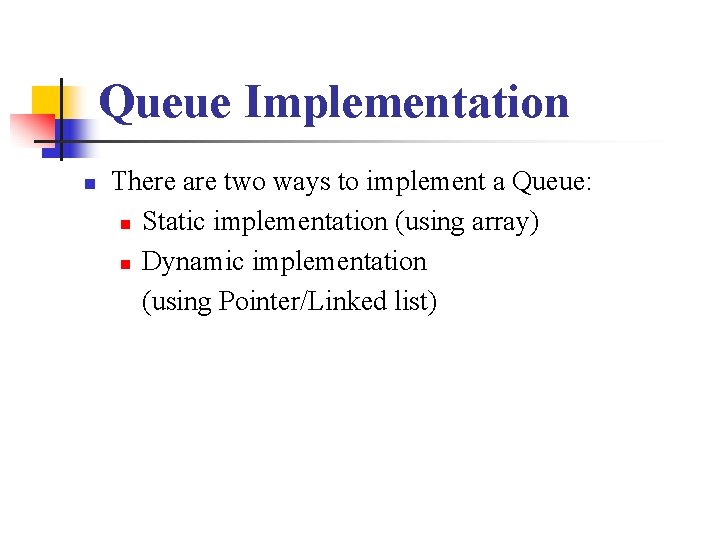
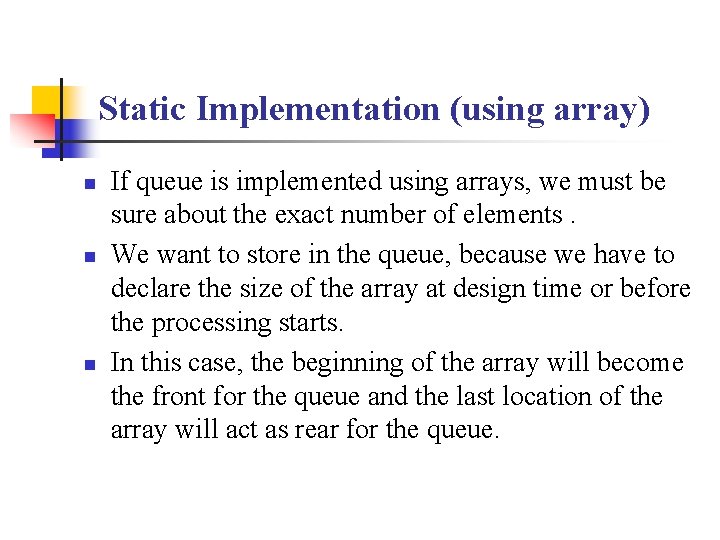
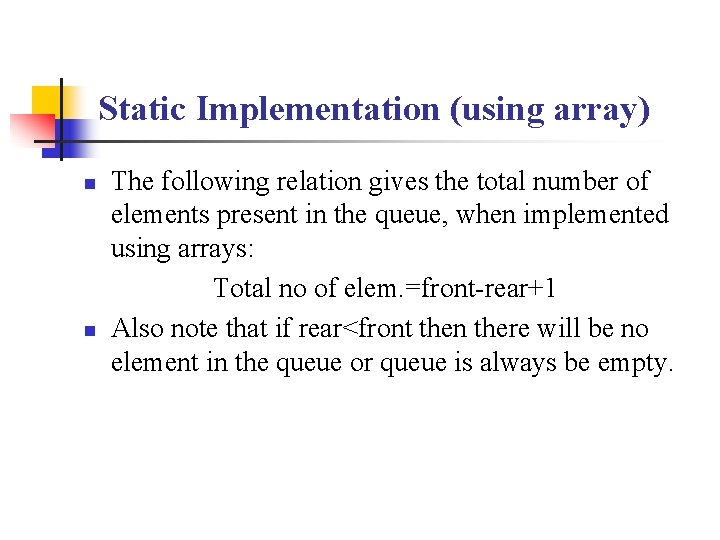
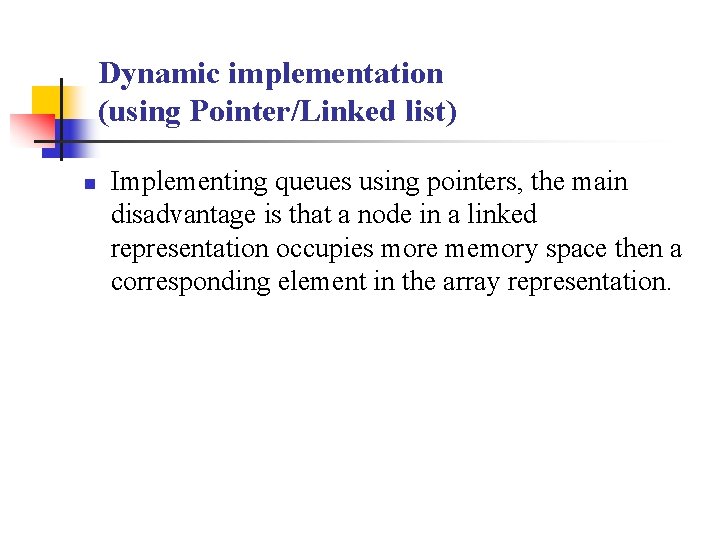
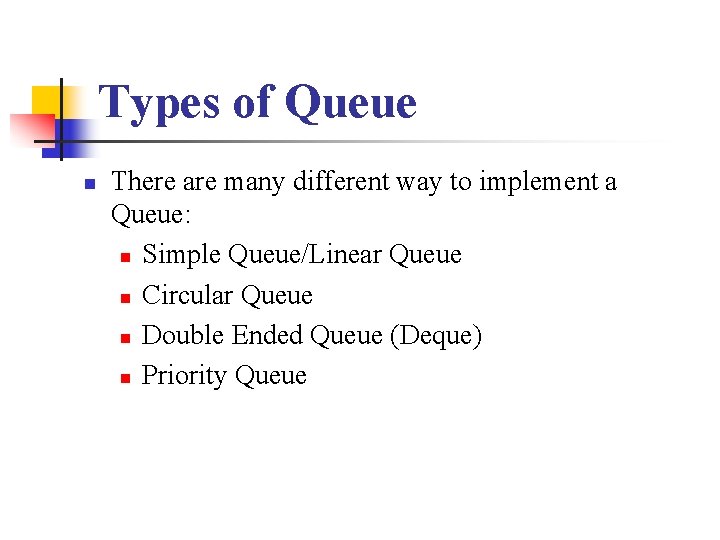
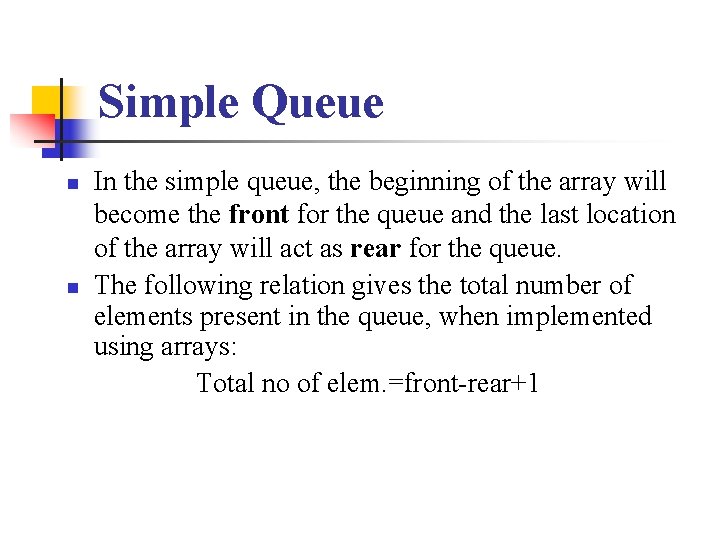
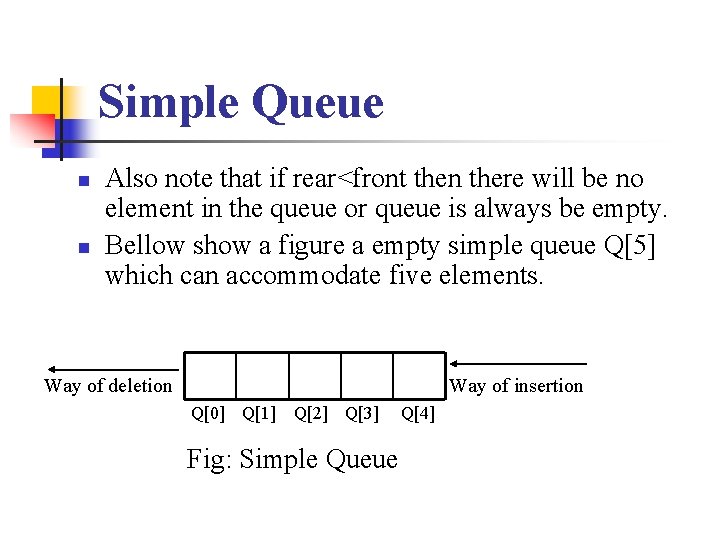
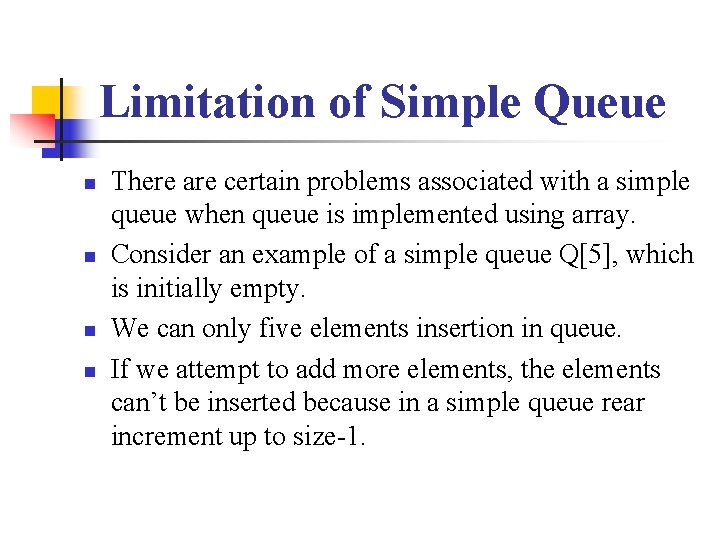
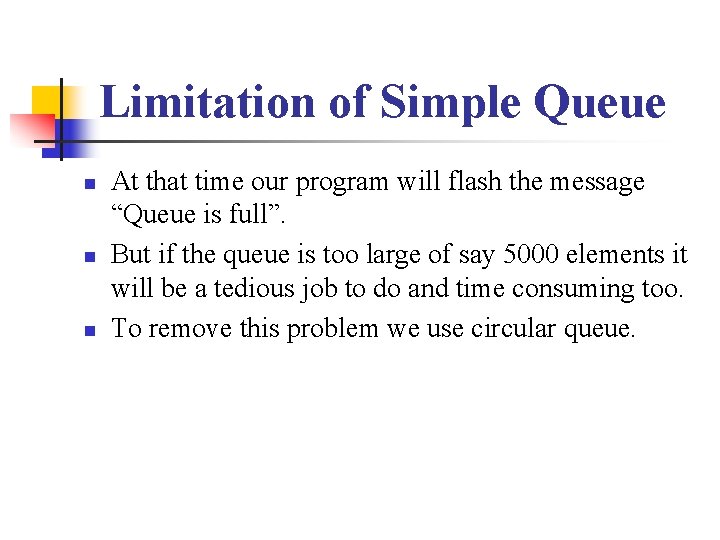
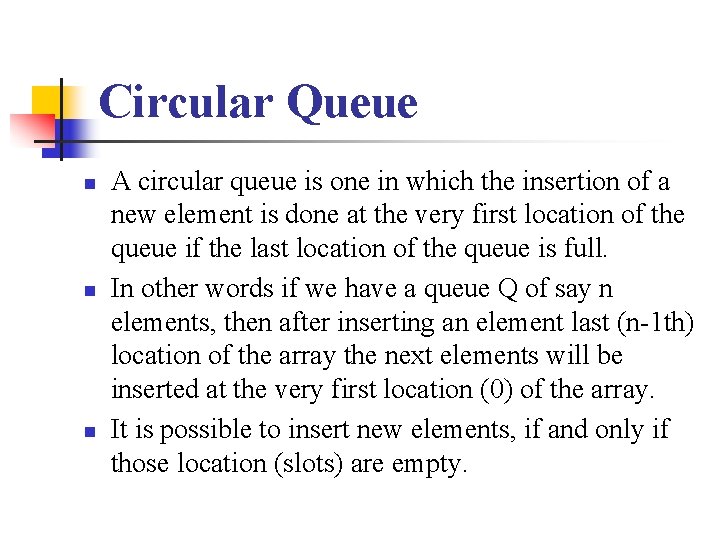
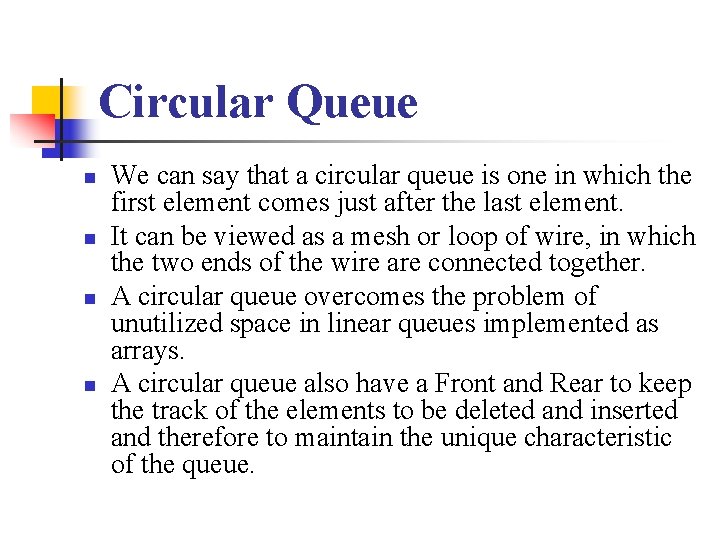
![Circular Queue n Bellow show a figure a empty circular queue Q[5] which can Circular Queue n Bellow show a figure a empty circular queue Q[5] which can](https://slidetodoc.com/presentation_image/aa02b301bdd47827eafeddd1c8a50721/image-18.jpg)
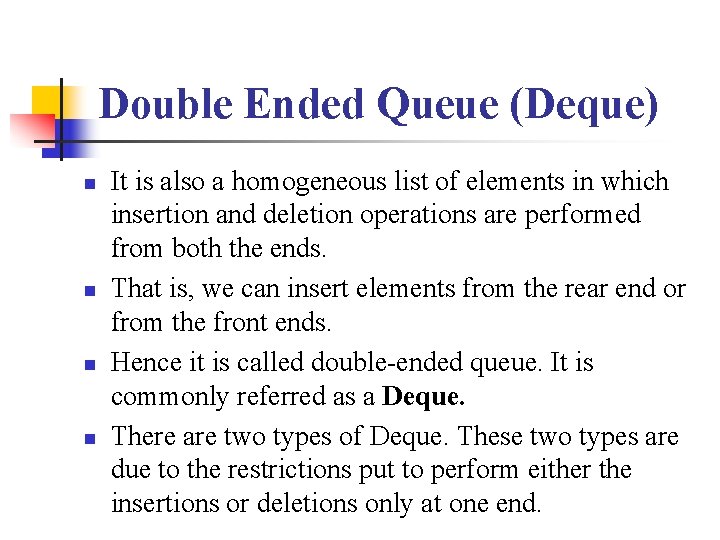
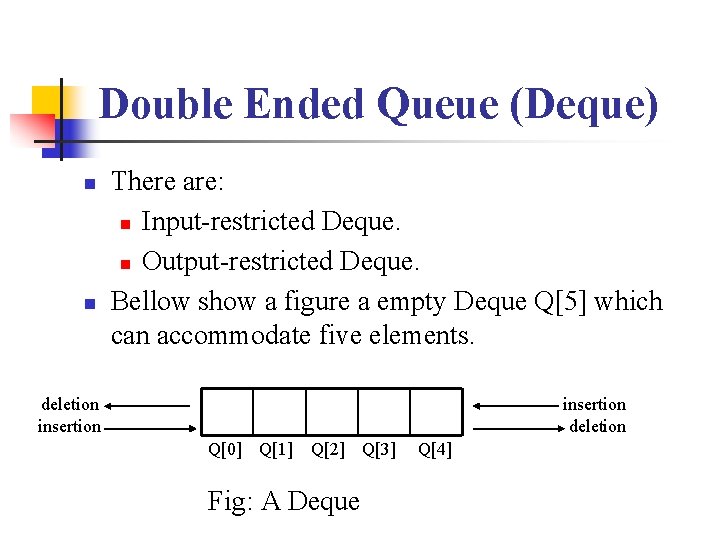
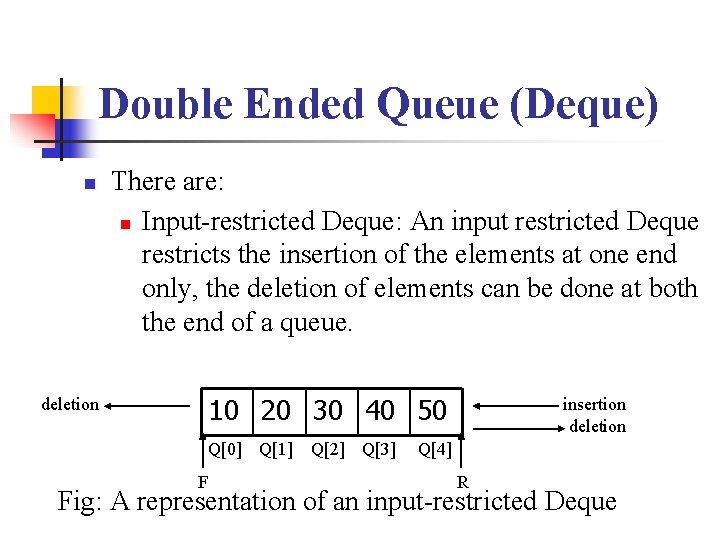
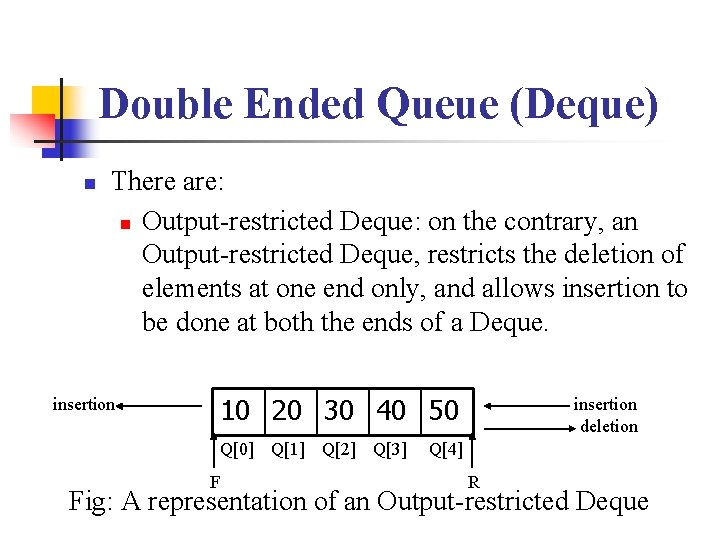
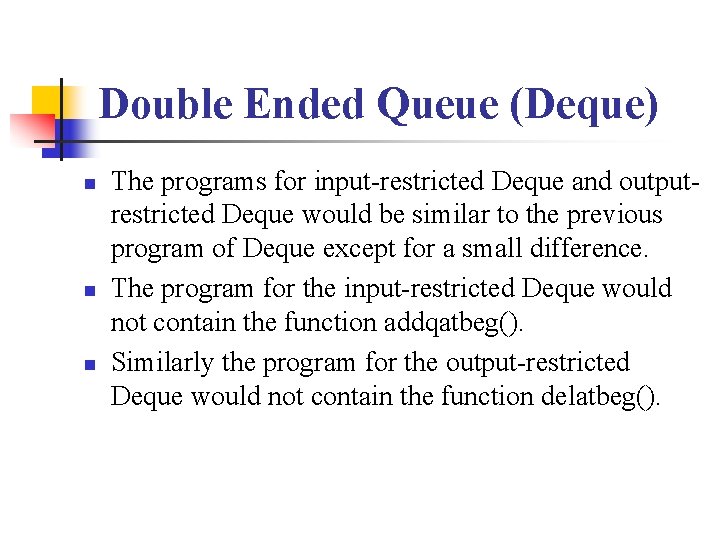
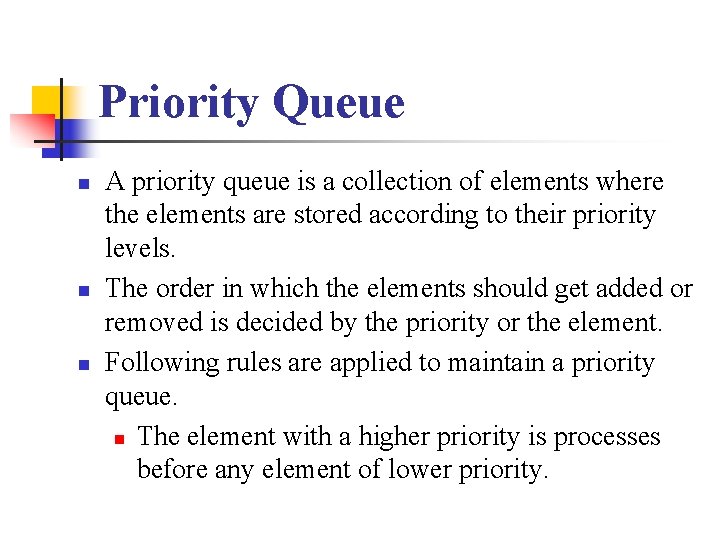
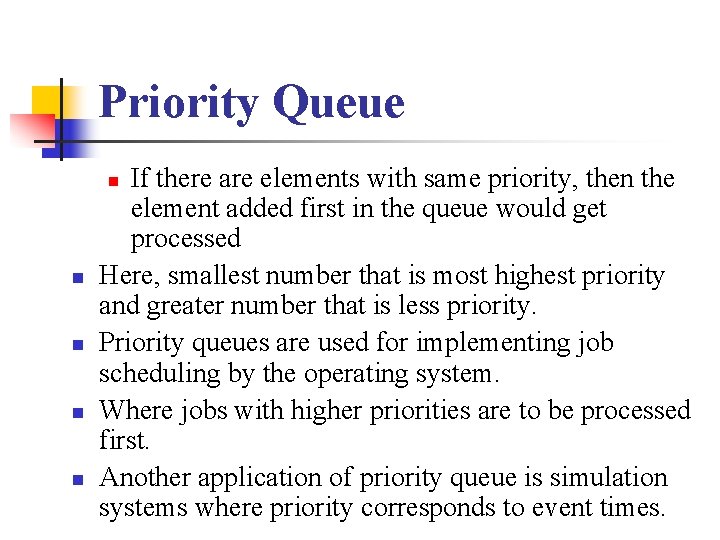
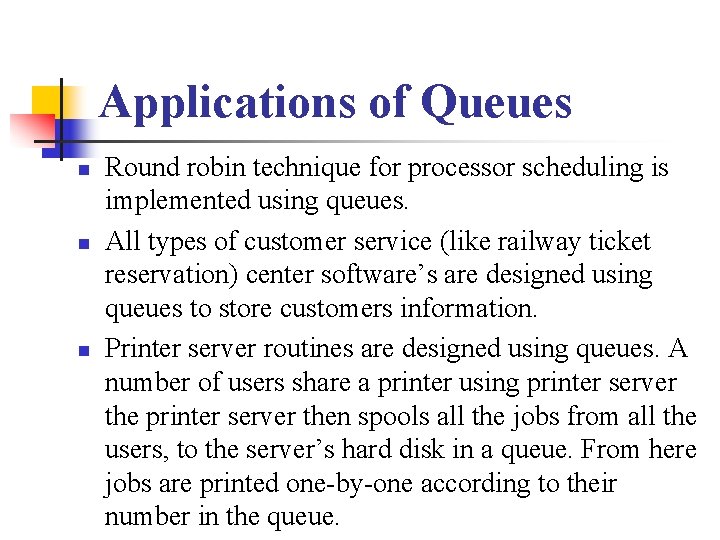
- Slides: 26
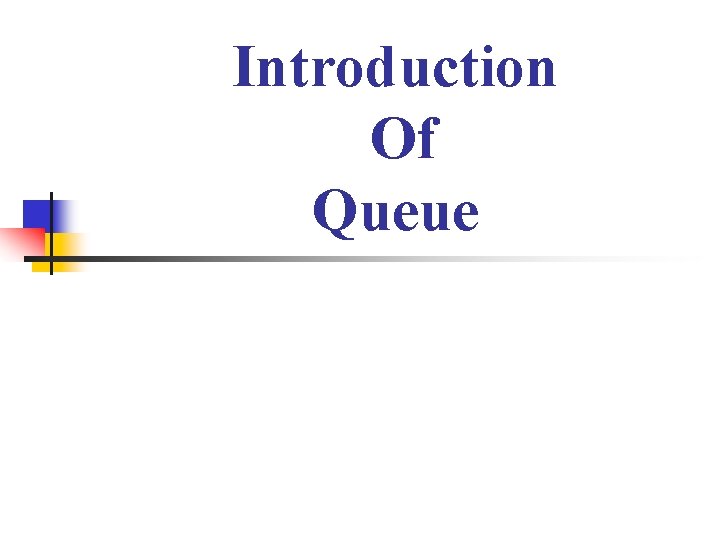
Introduction Of Queue
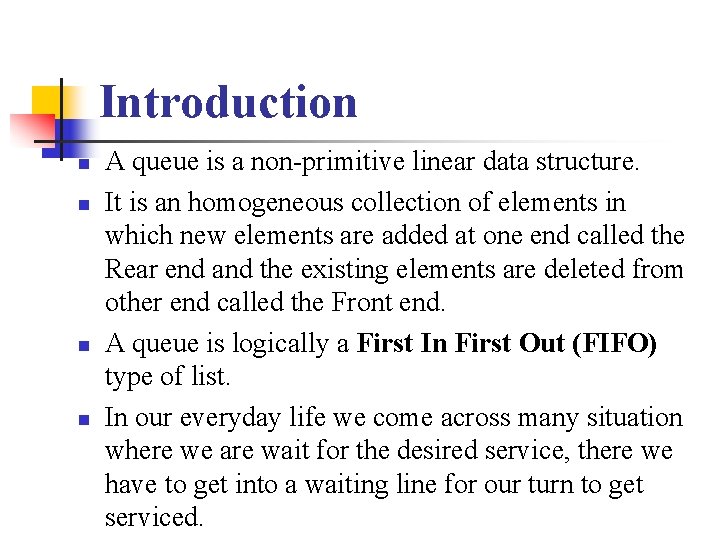
Introduction n n A queue is a non-primitive linear data structure. It is an homogeneous collection of elements in which new elements are added at one end called the Rear end and the existing elements are deleted from other end called the Front end. A queue is logically a First In First Out (FIFO) type of list. In our everyday life we come across many situation where we are wait for the desired service, there we have to get into a waiting line for our turn to get serviced.
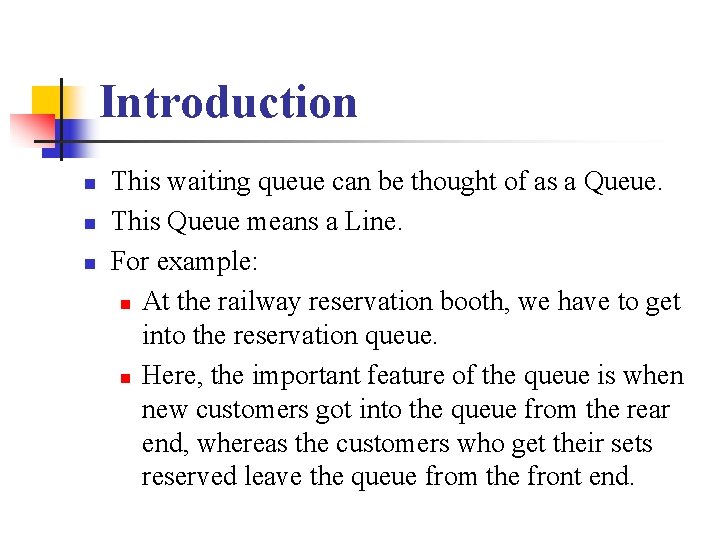
Introduction n This waiting queue can be thought of as a Queue. This Queue means a Line. For example: n At the railway reservation booth, we have to get into the reservation queue. n Here, the important feature of the queue is when new customers got into the queue from the rear end, whereas the customers who get their sets reserved leave the queue from the front end.
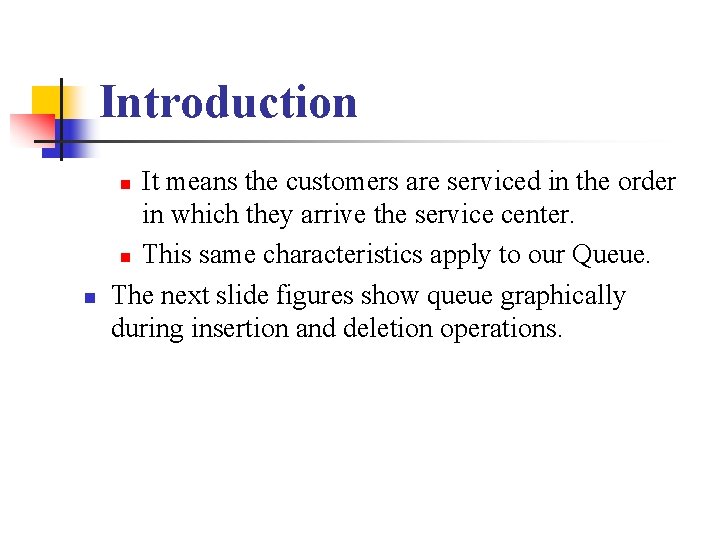
Introduction It means the customers are serviced in the order in which they arrive the service center. n This same characteristics apply to our Queue. The next slide figures show queue graphically during insertion and deletion operations. n n
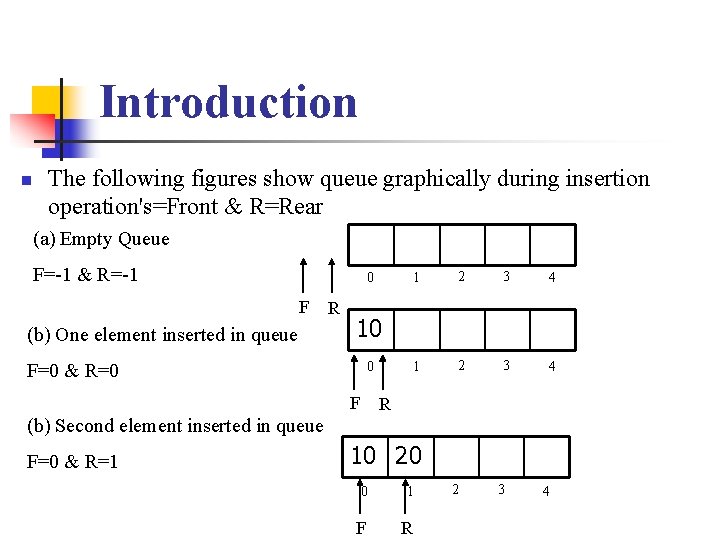
Introduction n The following figures show queue graphically during insertion operation's=Front & R=Rear (a) Empty Queue F=-1 & R=-1 0 F (b) One element inserted in queue R 1 2 3 4 10 0 F=0 & R=0 F R (b) Second element inserted in queue F=0 & R=1 10 20 0 1 F R 2 3 4
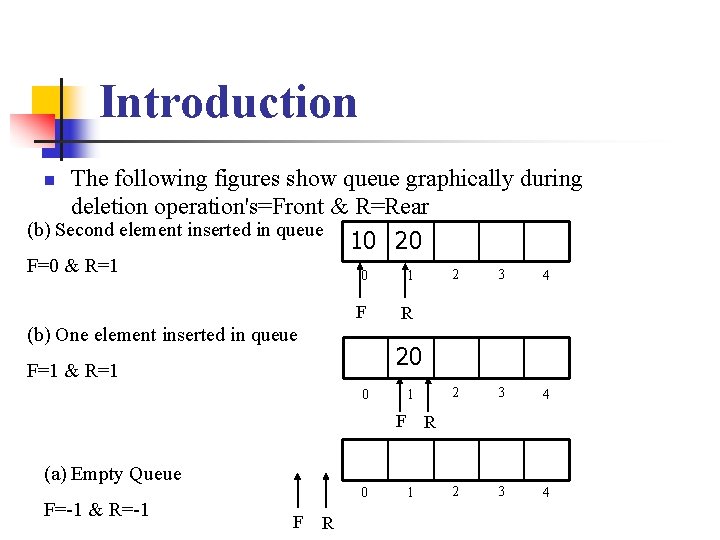
Introduction The following figures show queue graphically during deletion operation's=Front & R=Rear (b) Second element inserted in queue 10 20 n F=0 & R=1 0 1 F R (b) One element inserted in queue 0 1 F 4 2 3 4 R (a) Empty Queue 0 F 3 20 F=1 & R=1 F=-1 & R=-1 2 R 1
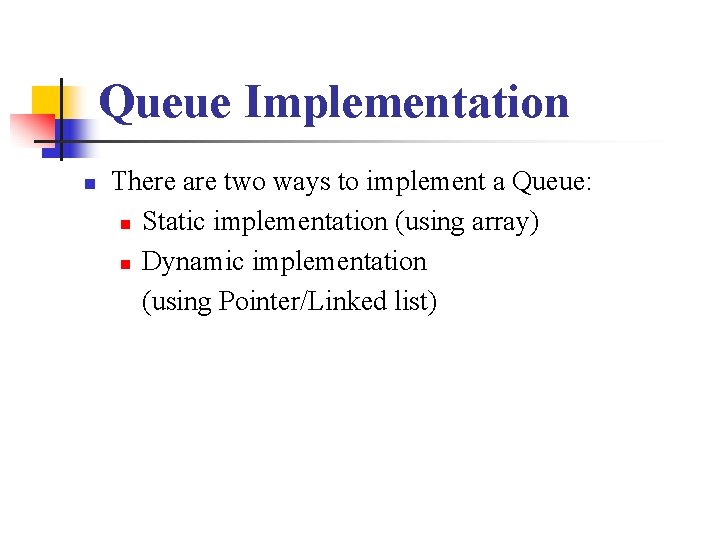
Queue Implementation n There are two ways to implement a Queue: n Static implementation (using array) n Dynamic implementation (using Pointer/Linked list)
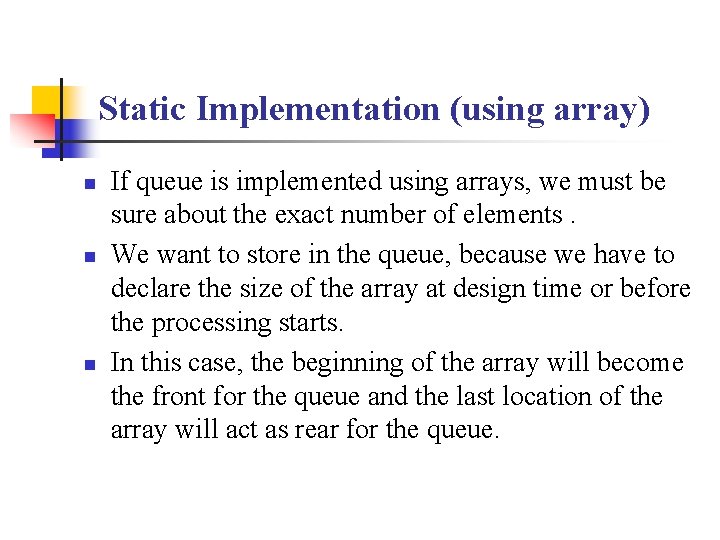
Static Implementation (using array) n n n If queue is implemented using arrays, we must be sure about the exact number of elements. We want to store in the queue, because we have to declare the size of the array at design time or before the processing starts. In this case, the beginning of the array will become the front for the queue and the last location of the array will act as rear for the queue.
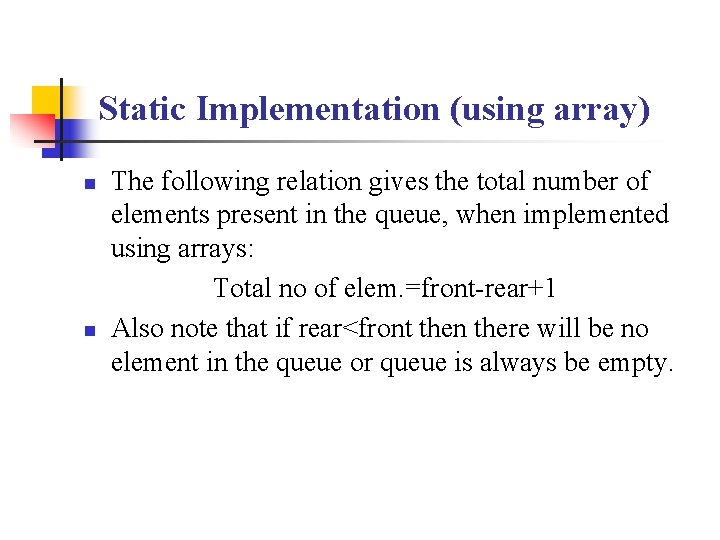
Static Implementation (using array) n n The following relation gives the total number of elements present in the queue, when implemented using arrays: Total no of elem. =front-rear+1 Also note that if rear<front then there will be no element in the queue or queue is always be empty.
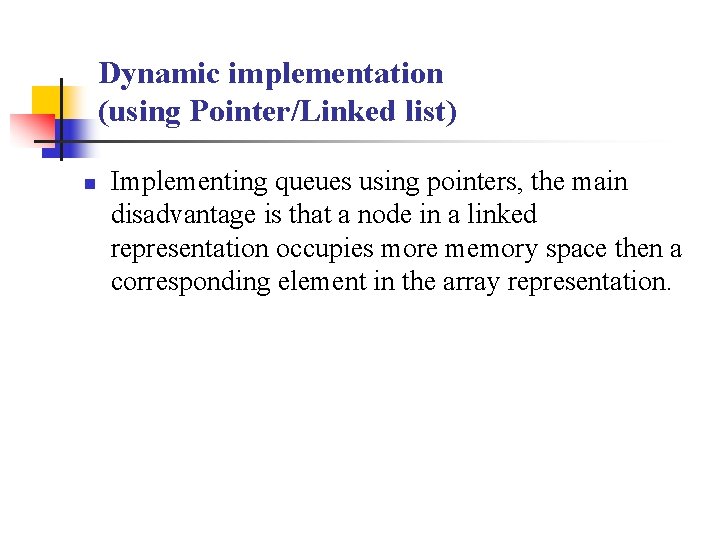
Dynamic implementation (using Pointer/Linked list) n Implementing queues using pointers, the main disadvantage is that a node in a linked representation occupies more memory space then a corresponding element in the array representation.
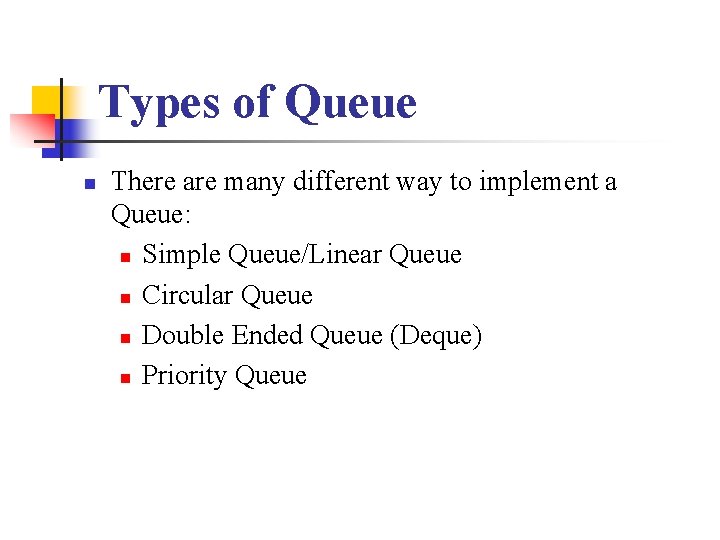
Types of Queue n There are many different way to implement a Queue: n Simple Queue/Linear Queue n Circular Queue n Double Ended Queue (Deque) n Priority Queue
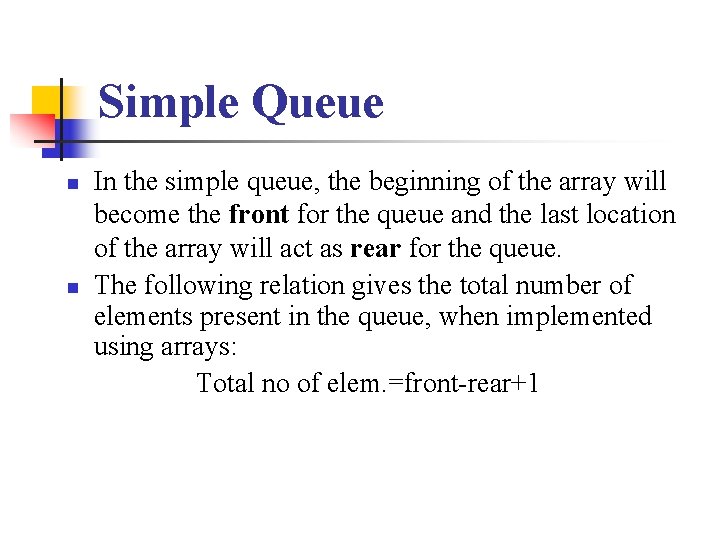
Simple Queue n n In the simple queue, the beginning of the array will become the front for the queue and the last location of the array will act as rear for the queue. The following relation gives the total number of elements present in the queue, when implemented using arrays: Total no of elem. =front-rear+1
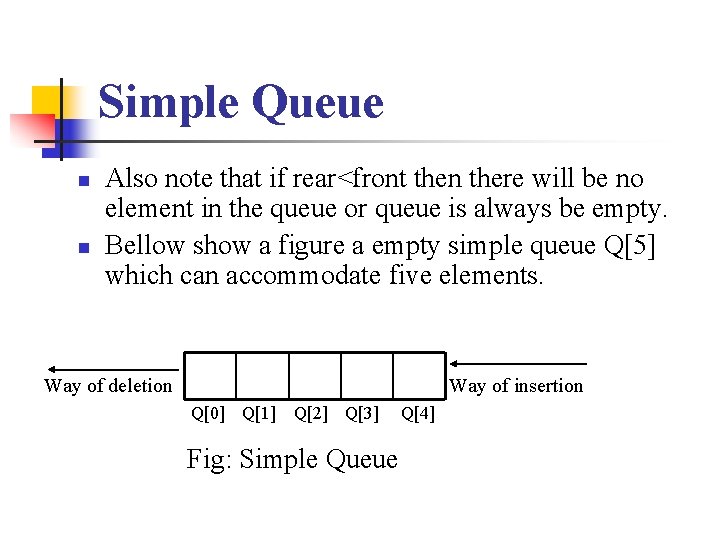
Simple Queue n n Also note that if rear<front then there will be no element in the queue or queue is always be empty. Bellow show a figure a empty simple queue Q[5] which can accommodate five elements. Way of deletion Way of insertion Q[0] Q[1] Q[2] Q[3] Fig: Simple Queue Q[4]
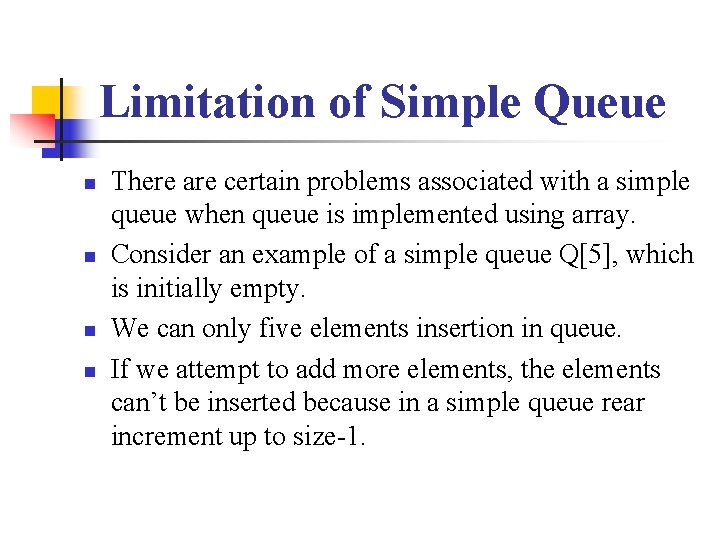
Limitation of Simple Queue n n There are certain problems associated with a simple queue when queue is implemented using array. Consider an example of a simple queue Q[5], which is initially empty. We can only five elements insertion in queue. If we attempt to add more elements, the elements can’t be inserted because in a simple queue rear increment up to size-1.
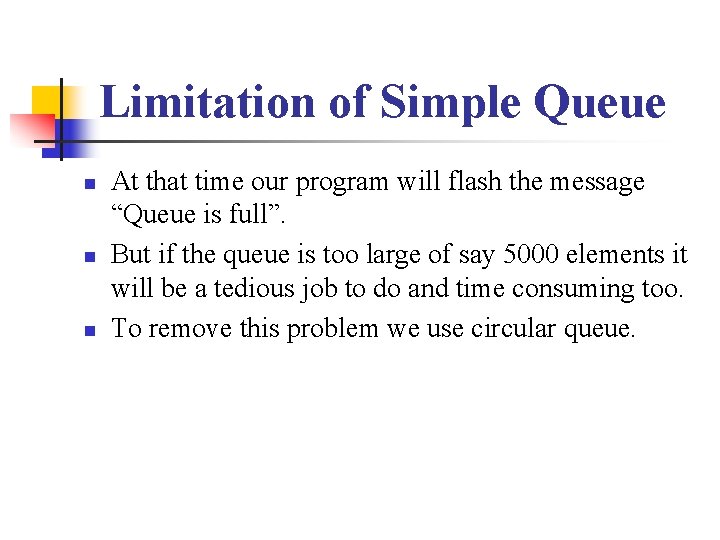
Limitation of Simple Queue n n n At that time our program will flash the message “Queue is full”. But if the queue is too large of say 5000 elements it will be a tedious job to do and time consuming too. To remove this problem we use circular queue.
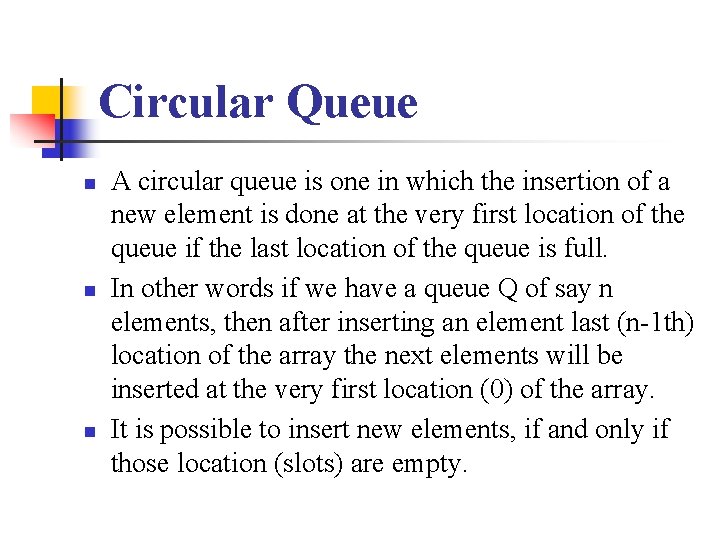
Circular Queue n n n A circular queue is one in which the insertion of a new element is done at the very first location of the queue if the last location of the queue is full. In other words if we have a queue Q of say n elements, then after inserting an element last (n-1 th) location of the array the next elements will be inserted at the very first location (0) of the array. It is possible to insert new elements, if and only if those location (slots) are empty.
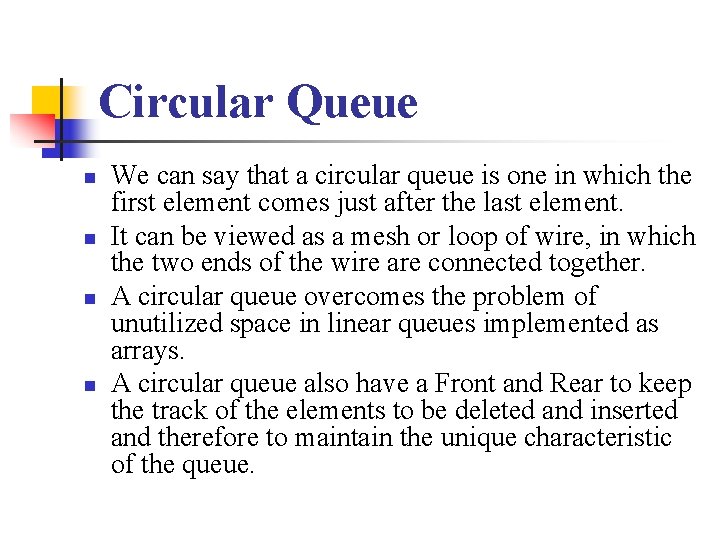
Circular Queue n n We can say that a circular queue is one in which the first element comes just after the last element. It can be viewed as a mesh or loop of wire, in which the two ends of the wire are connected together. A circular queue overcomes the problem of unutilized space in linear queues implemented as arrays. A circular queue also have a Front and Rear to keep the track of the elements to be deleted and inserted and therefore to maintain the unique characteristic of the queue.
![Circular Queue n Bellow show a figure a empty circular queue Q5 which can Circular Queue n Bellow show a figure a empty circular queue Q[5] which can](https://slidetodoc.com/presentation_image/aa02b301bdd47827eafeddd1c8a50721/image-18.jpg)
Circular Queue n Bellow show a figure a empty circular queue Q[5] which can accommodate five elements. Q[0] Q[4] Q[1] ` Q[2] Q[3] Fig: Circular Queue
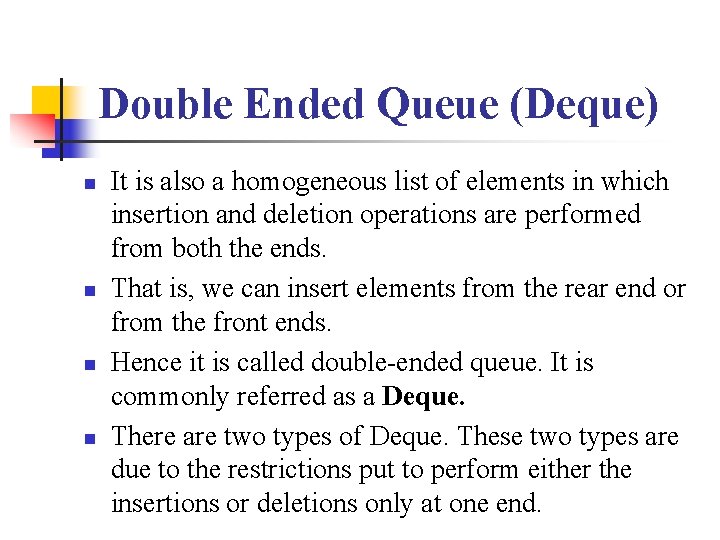
Double Ended Queue (Deque) n n It is also a homogeneous list of elements in which insertion and deletion operations are performed from both the ends. That is, we can insert elements from the rear end or from the front ends. Hence it is called double-ended queue. It is commonly referred as a Deque. There are two types of Deque. These two types are due to the restrictions put to perform either the insertions or deletions only at one end.
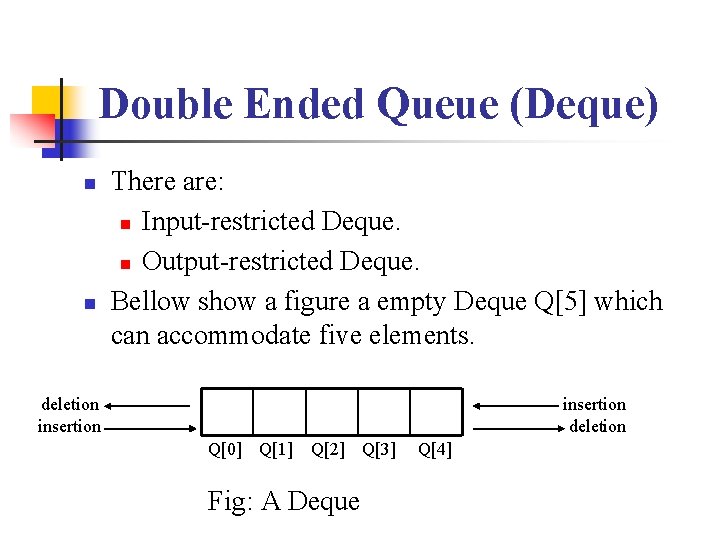
Double Ended Queue (Deque) n n There are: n Input-restricted Deque. n Output-restricted Deque. Bellow show a figure a empty Deque Q[5] which can accommodate five elements. deletion insertion deletion Q[0] Q[1] Q[2] Q[3] Fig: A Deque Q[4]
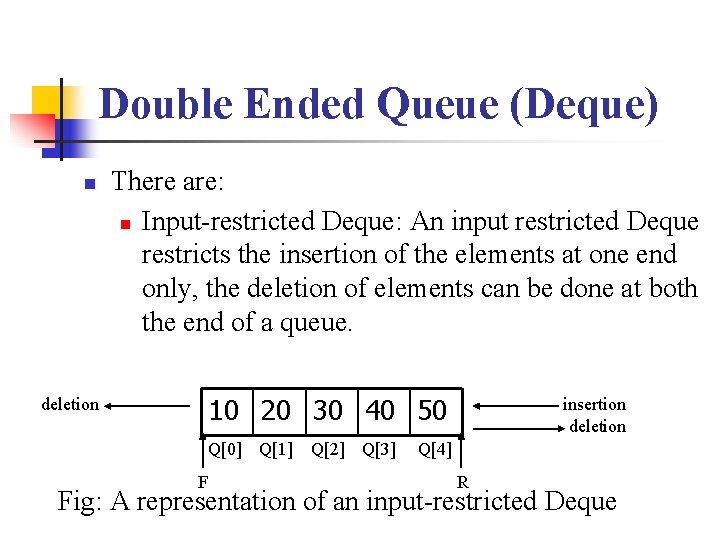
Double Ended Queue (Deque) n deletion There are: n Input-restricted Deque: An input restricted Deque restricts the insertion of the elements at one end only, the deletion of elements can be done at both the end of a queue. 10 20 30 40 50 Q[0] Q[1] Q[2] Q[3] F insertion deletion Q[4] R Fig: A representation of an input-restricted Deque
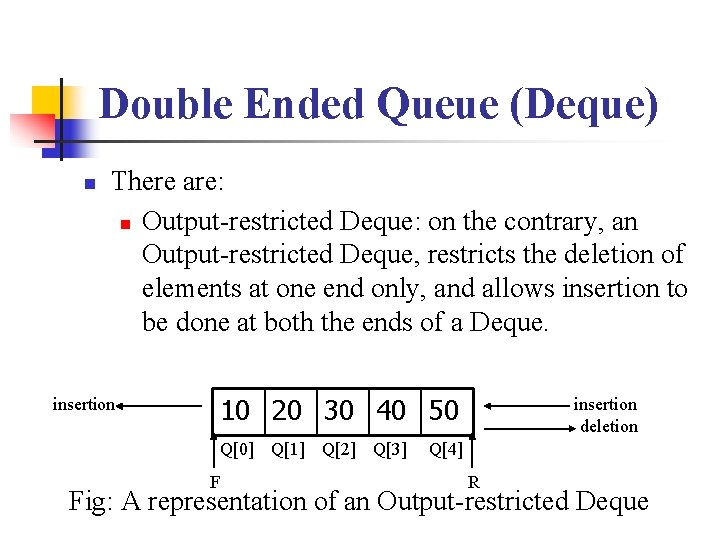
Double Ended Queue (Deque) n There are: n Output-restricted Deque: on the contrary, an Output-restricted Deque, restricts the deletion of elements at one end only, and allows insertion to be done at both the ends of a Deque. insertion 10 20 30 40 50 Q[0] Q[1] Q[2] Q[3] F insertion deletion Q[4] R Fig: A representation of an Output-restricted Deque
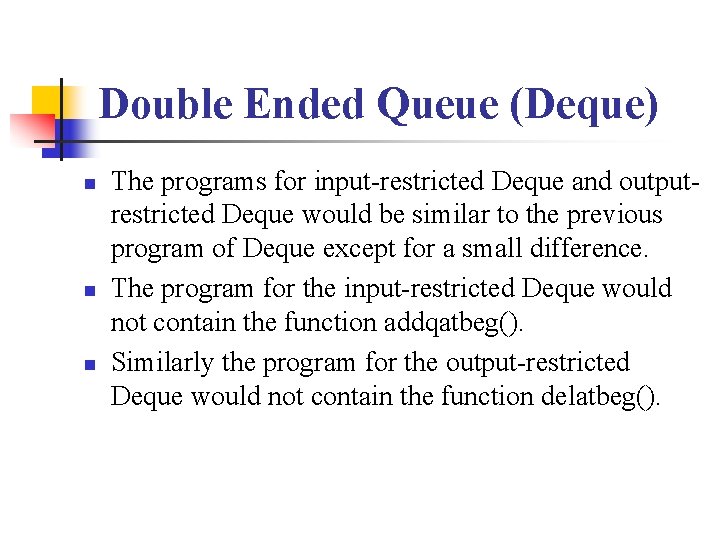
Double Ended Queue (Deque) n n n The programs for input-restricted Deque and outputrestricted Deque would be similar to the previous program of Deque except for a small difference. The program for the input-restricted Deque would not contain the function addqatbeg(). Similarly the program for the output-restricted Deque would not contain the function delatbeg().
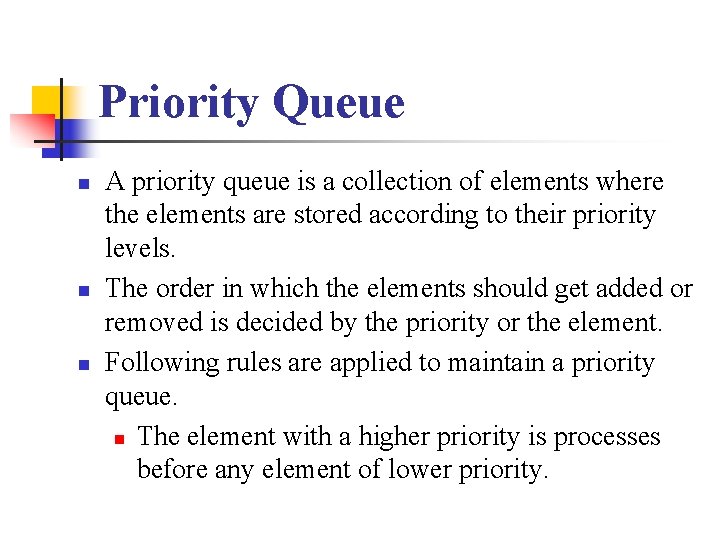
Priority Queue n n n A priority queue is a collection of elements where the elements are stored according to their priority levels. The order in which the elements should get added or removed is decided by the priority or the element. Following rules are applied to maintain a priority queue. n The element with a higher priority is processes before any element of lower priority.
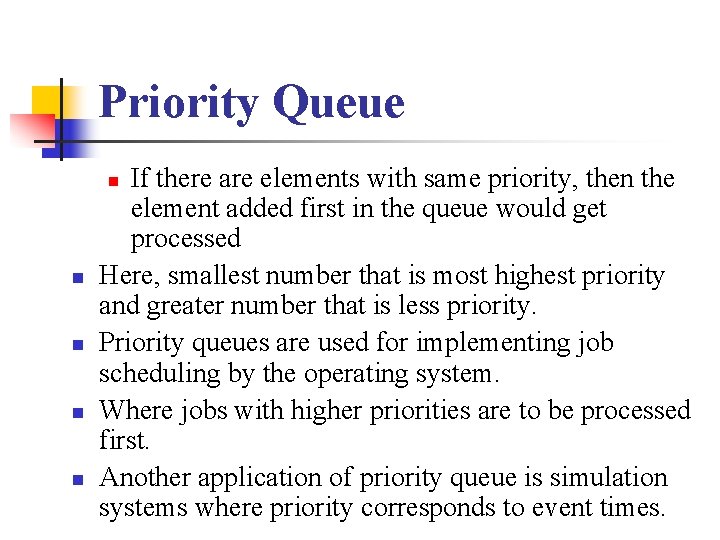
Priority Queue If there are elements with same priority, then the element added first in the queue would get processed Here, smallest number that is most highest priority and greater number that is less priority. Priority queues are used for implementing job scheduling by the operating system. Where jobs with higher priorities are to be processed first. Another application of priority queue is simulation systems where priority corresponds to event times. n n n
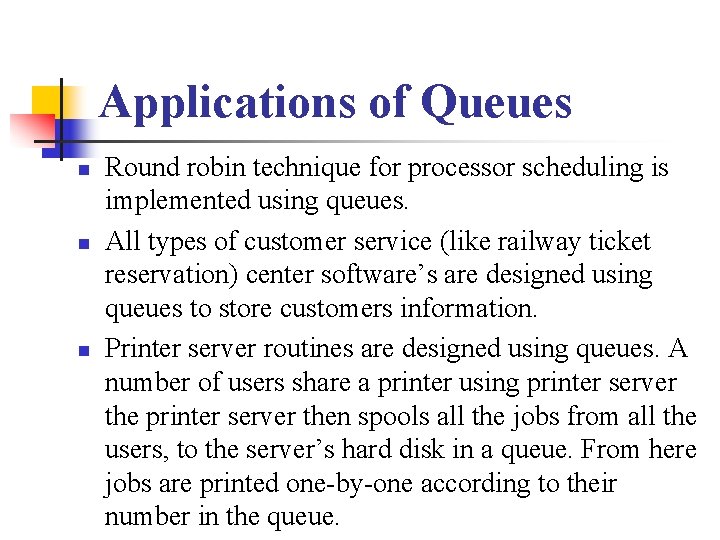
Applications of Queues n n n Round robin technique for processor scheduling is implemented using queues. All types of customer service (like railway ticket reservation) center software’s are designed using queues to store customers information. Printer server routines are designed using queues. A number of users share a printer using printer server then spools all the jobs from all the users, to the server’s hard disk in a queue. From here jobs are printed one-by-one according to their number in the queue.