Operator Overloading CS 308 Data Structures What is
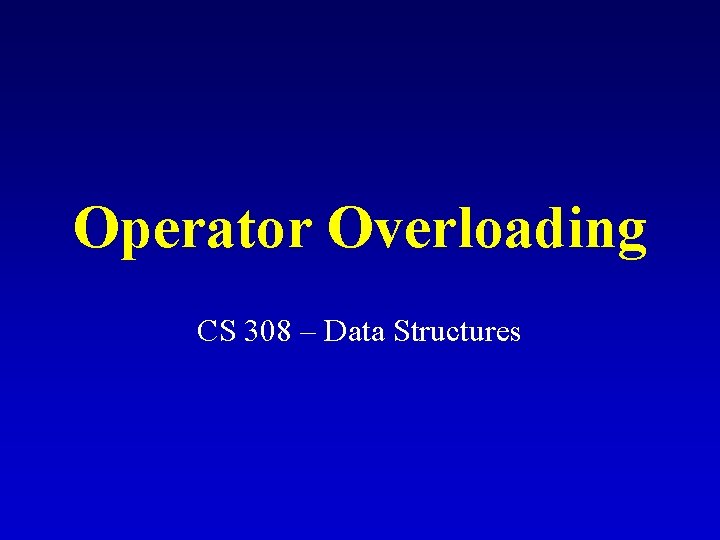
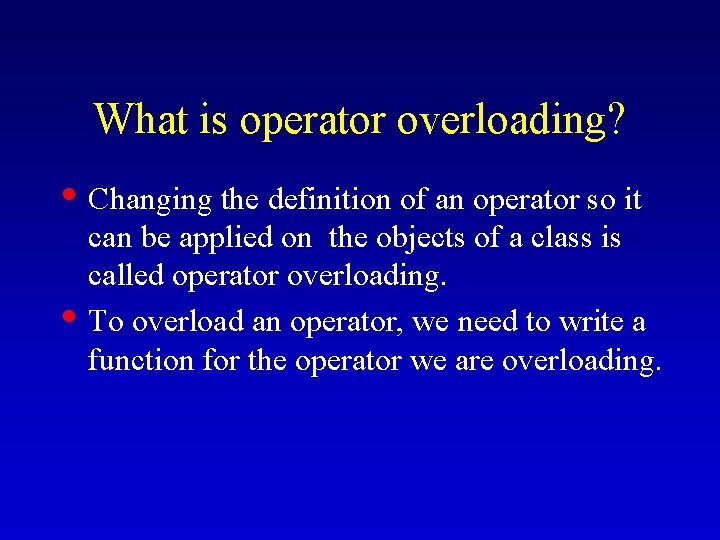
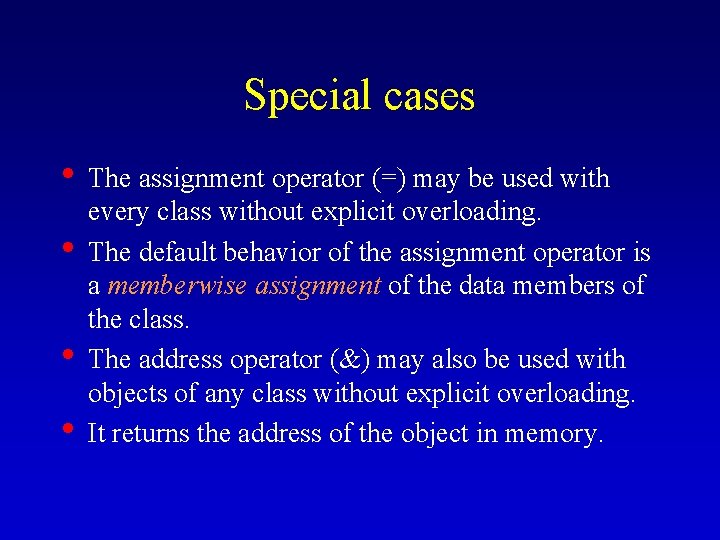
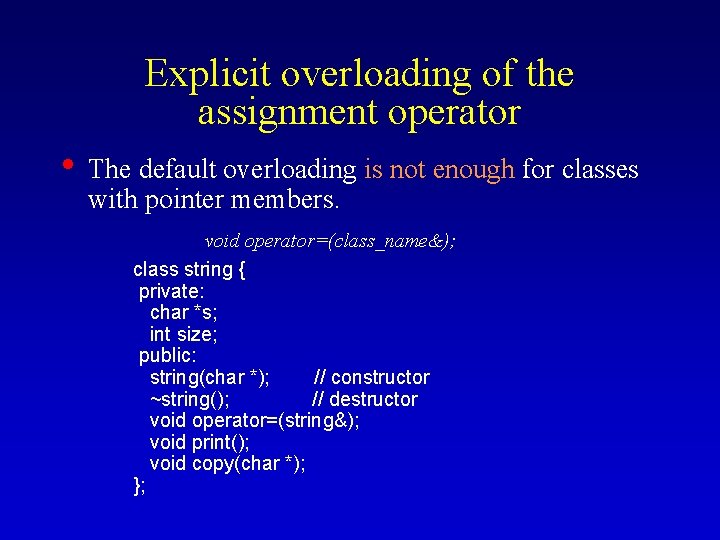
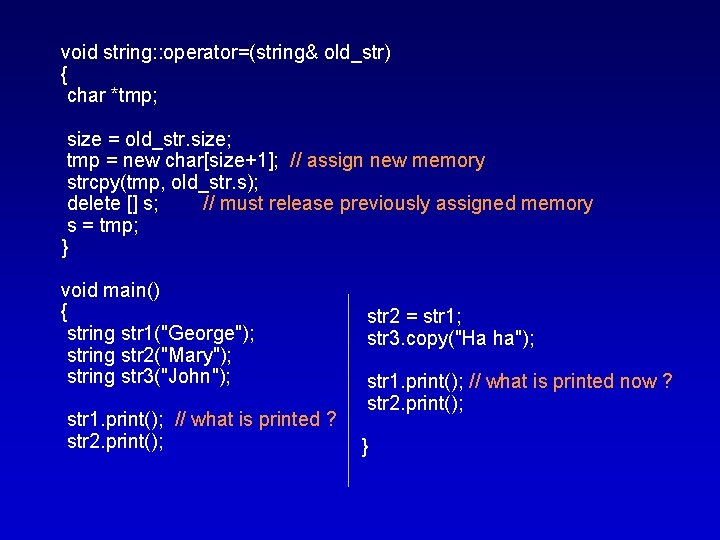
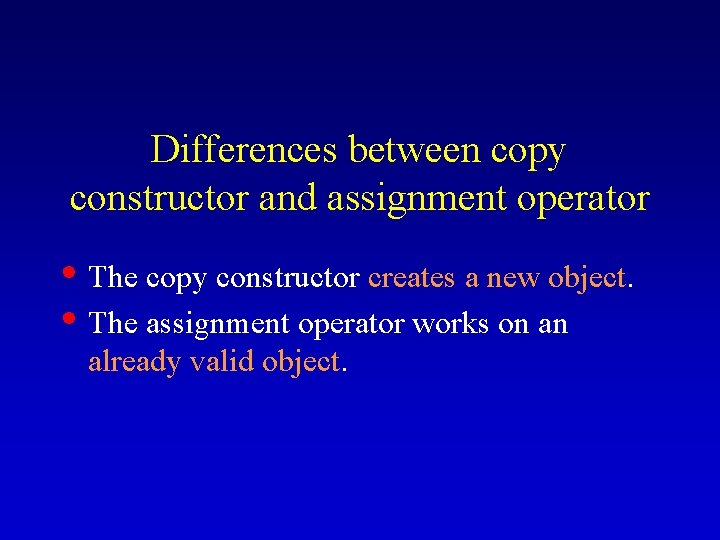
![Another example: overloading the [] operator class Array { private: int num. Elems; int Another example: overloading the [] operator class Array { private: int num. Elems; int](https://slidetodoc.com/presentation_image/356645950b8f13176c9e4c505bd4824e/image-7.jpg)
 { if ((index < 0) || (index >= num. int& Array: : operator[](int index) { if ((index < 0) || (index >= num.](https://slidetodoc.com/presentation_image/356645950b8f13176c9e4c505bd4824e/image-8.jpg)
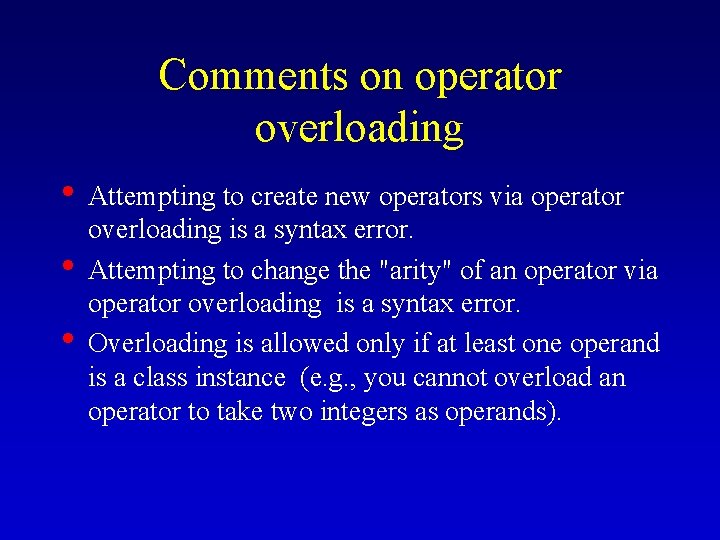
- Slides: 9
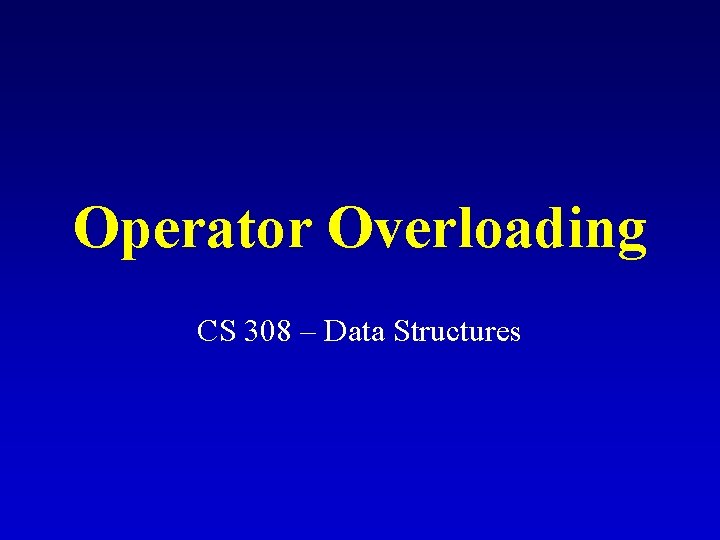
Operator Overloading CS 308 – Data Structures
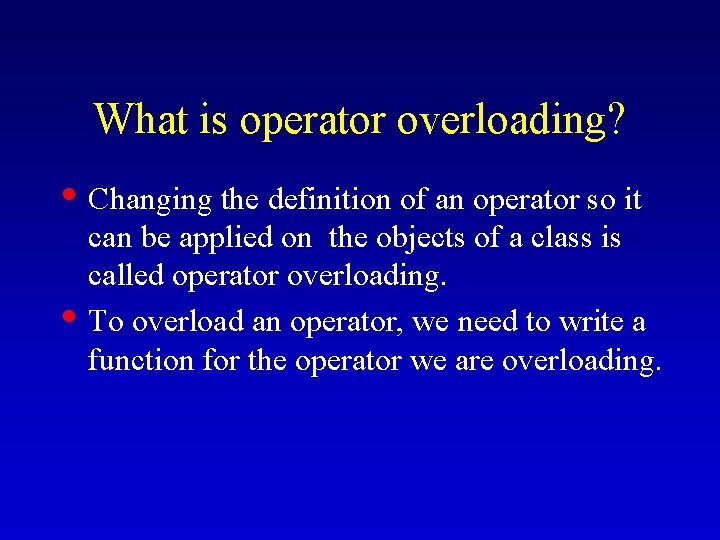
What is operator overloading? • Changing the definition of an operator so it • can be applied on the objects of a class is called operator overloading. To overload an operator, we need to write a function for the operator we are overloading.
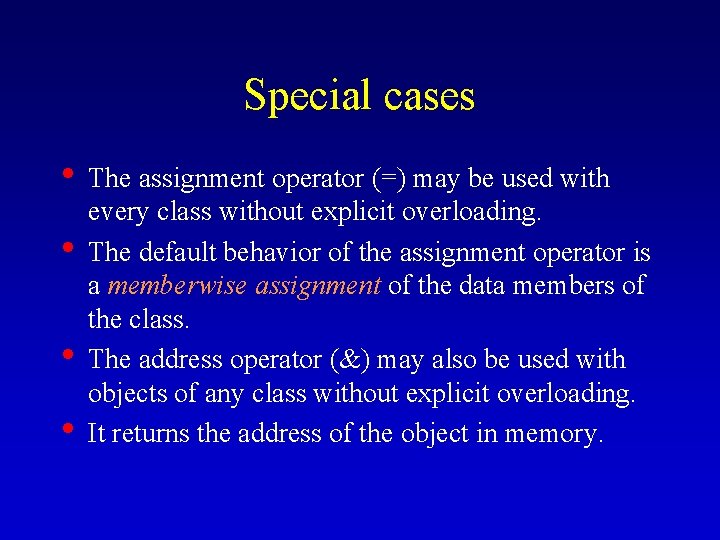
Special cases • The assignment operator (=) may be used with • • • every class without explicit overloading. The default behavior of the assignment operator is a memberwise assignment of the data members of the class. The address operator (&) may also be used with objects of any class without explicit overloading. It returns the address of the object in memory.
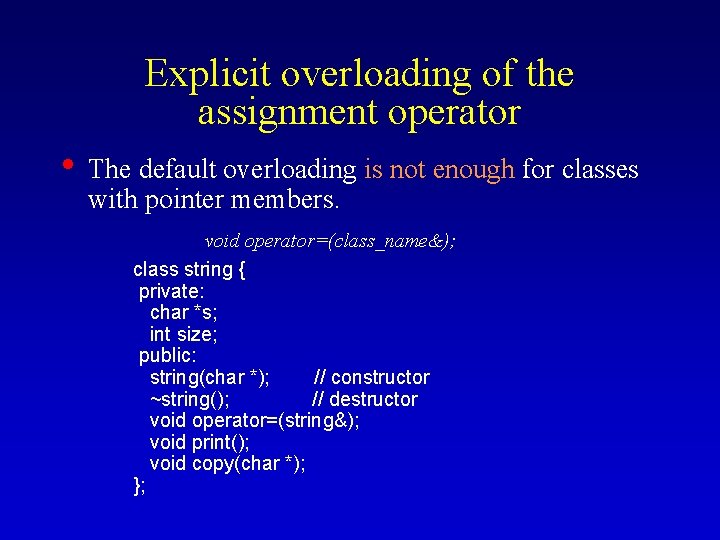
Explicit overloading of the assignment operator • The default overloading is not enough for classes with pointer members. void operator=(class_name&); class string { private: char *s; int size; public: string(char *); // constructor ~string(); // destructor void operator=(string&); void print(); void copy(char *); };
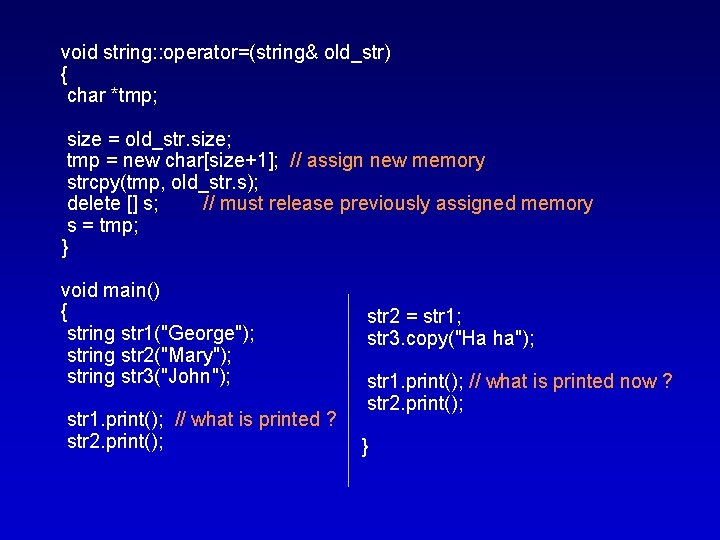
void string: : operator=(string& old_str) { char *tmp; size = old_str. size; tmp = new char[size+1]; // assign new memory strcpy(tmp, old_str. s); delete [] s; // must release previously assigned memory s = tmp; } void main() { str 2 = str 1; string str 1("George"); str 3. copy("Ha ha"); string str 2("Mary"); string str 3("John"); str 1. print(); // what is printed now ? str 2. print(); str 1. print(); // what is printed ? str 2. print(); }
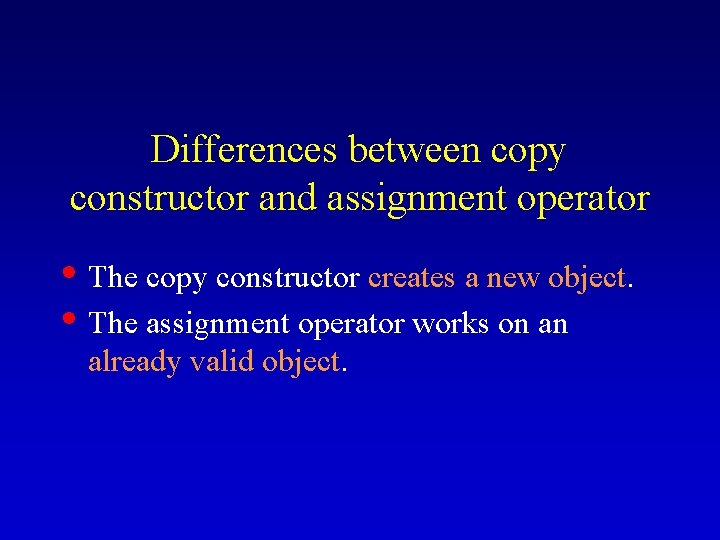
Differences between copy constructor and assignment operator • The copy constructor creates a new object. • The assignment operator works on an already valid object.
![Another example overloading the operator class Array private int num Elems int Another example: overloading the [] operator class Array { private: int num. Elems; int](https://slidetodoc.com/presentation_image/356645950b8f13176c9e4c505bd4824e/image-7.jpg)
Another example: overloading the [] operator class Array { private: int num. Elems; int *arr; public: Array(int); // constructor ~Array(); // destructor int& operator[](int); }; Array: : Array(int n) { num. Elems = n; arr = new int[n]; } Array: : ~Array() { delete [] arr; }
 { if ((index < 0) || (index >= num.](https://slidetodoc.com/presentation_image/356645950b8f13176c9e4c505bd4824e/image-8.jpg)
int& Array: : operator[](int index) { if ((index < 0) || (index >= num. Elems)) { cout << "Out of bounds error !!" << endl; exit(0); // error: invalid index !! } else return(arr[index]); } void main() { int i; Array A(10); for(i=0; i<=10; i++) // i=10: error !! A[i] = i; }
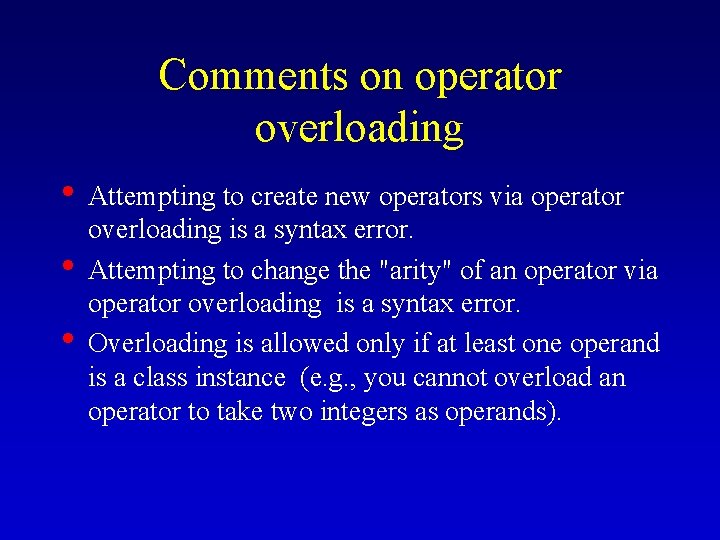
Comments on operator overloading • Attempting to create new operators via operator • • overloading is a syntax error. Attempting to change the "arity" of an operator via operator overloading is a syntax error. Overloading is allowed only if at least one operand is a class instance (e. g. , you cannot overload an operator to take two integers as operands).