Operator Overloading Operator Overloading allows a programmer to
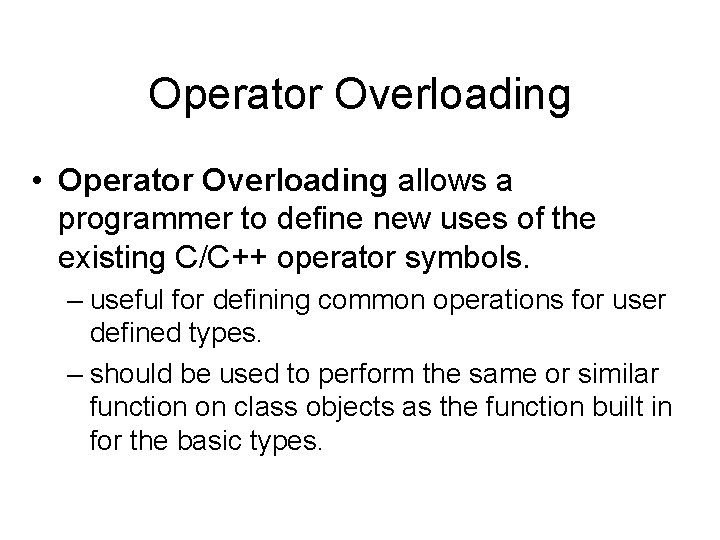
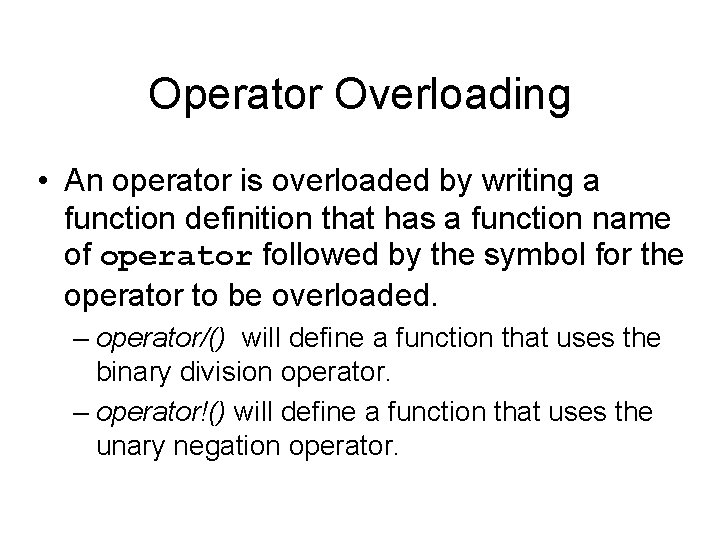
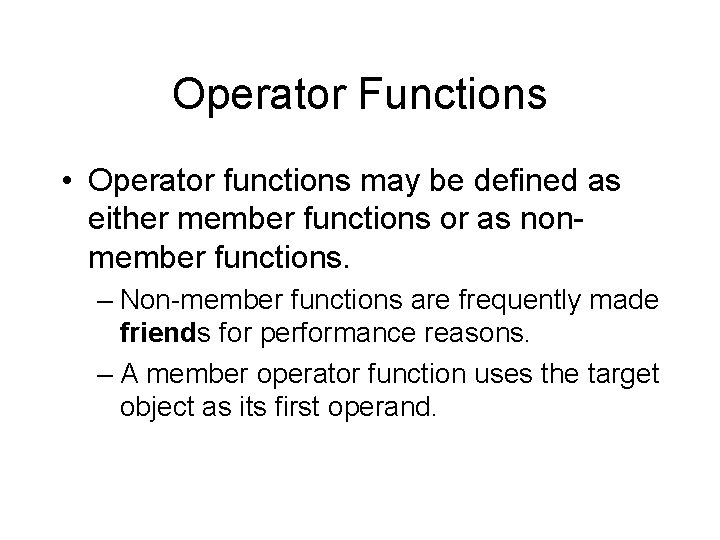
![Operator Functions • The operator overloading functions for overloading (), [], -> or the Operator Functions • The operator overloading functions for overloading (), [], -> or the](https://slidetodoc.com/presentation_image/dc525d20d0645698814c9fc1744780bb/image-4.jpg)
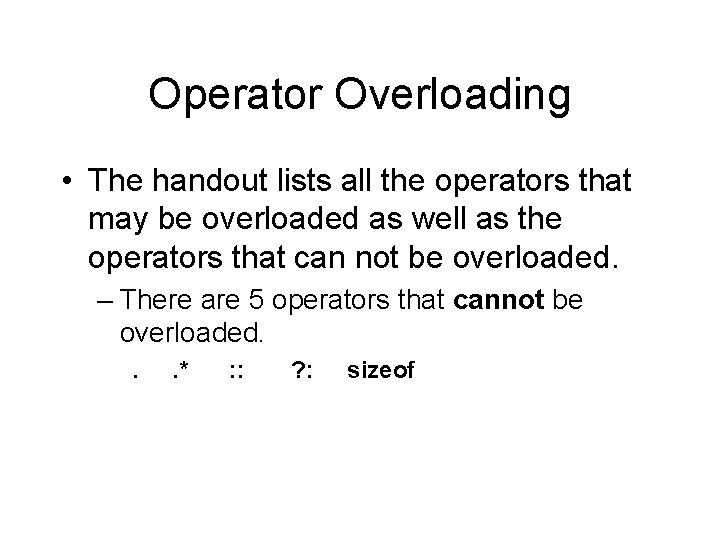
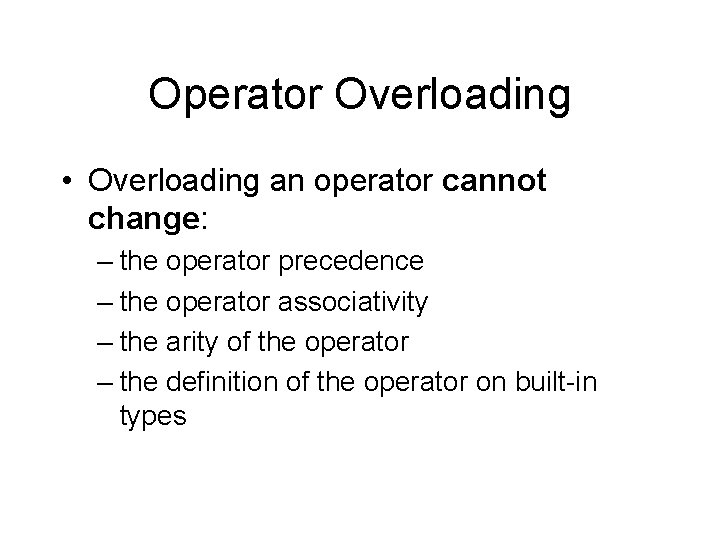
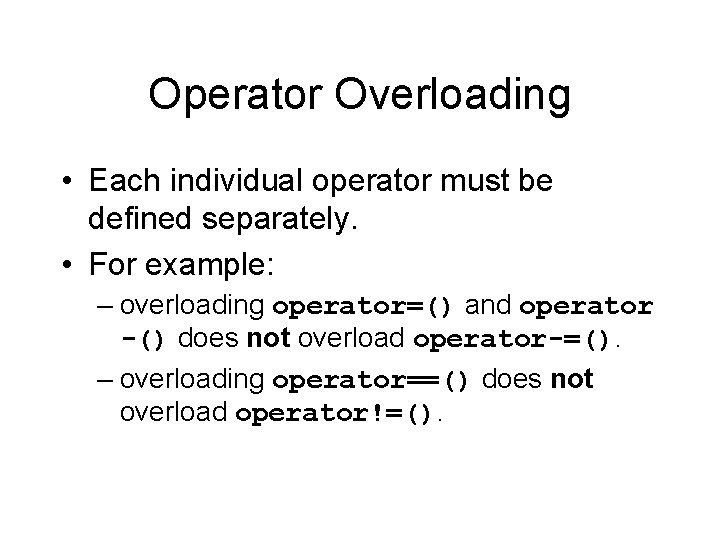
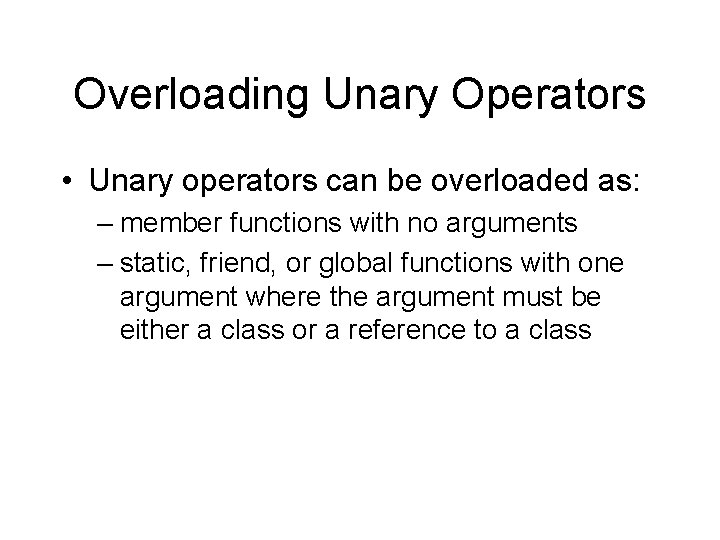
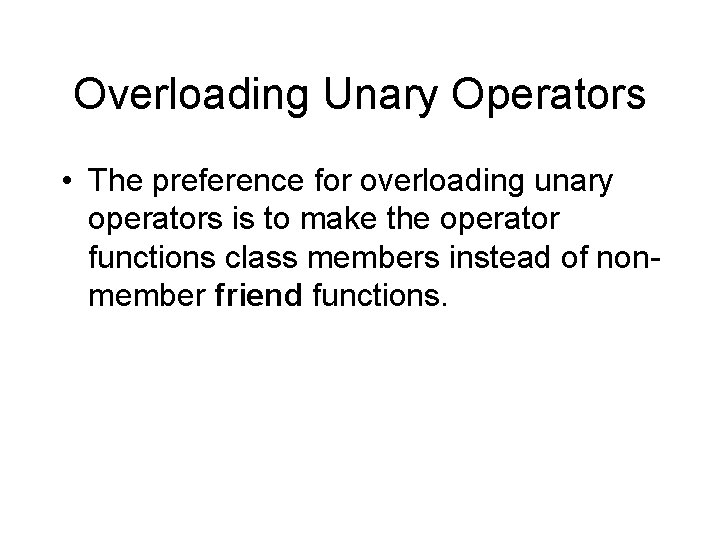
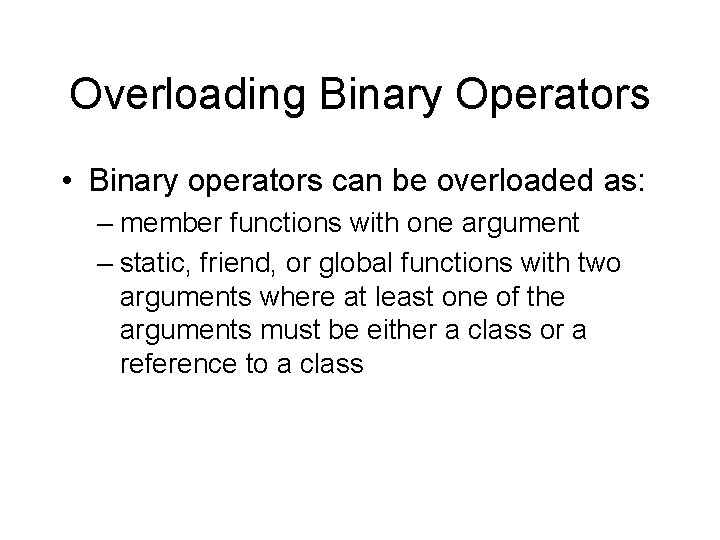
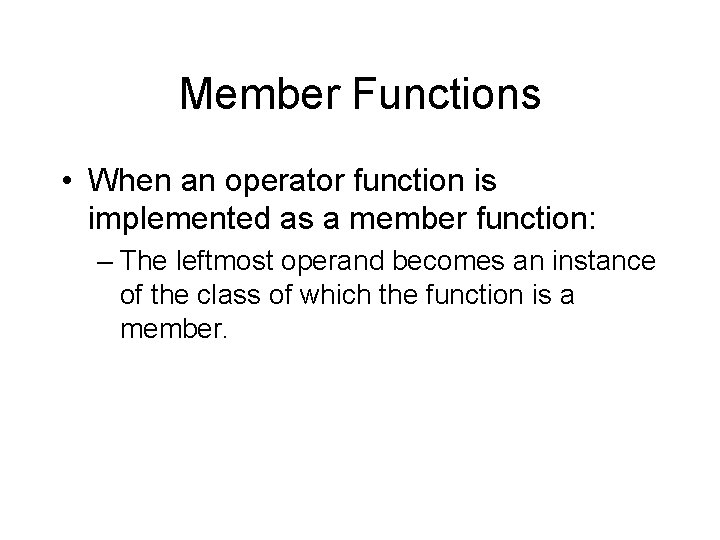
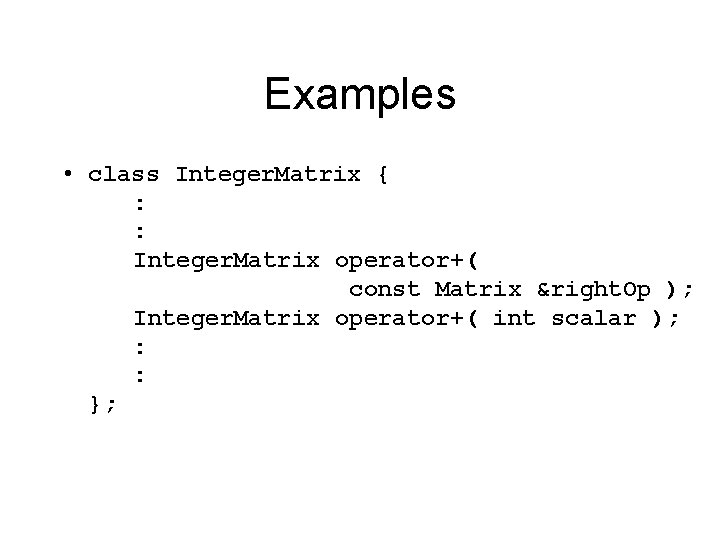
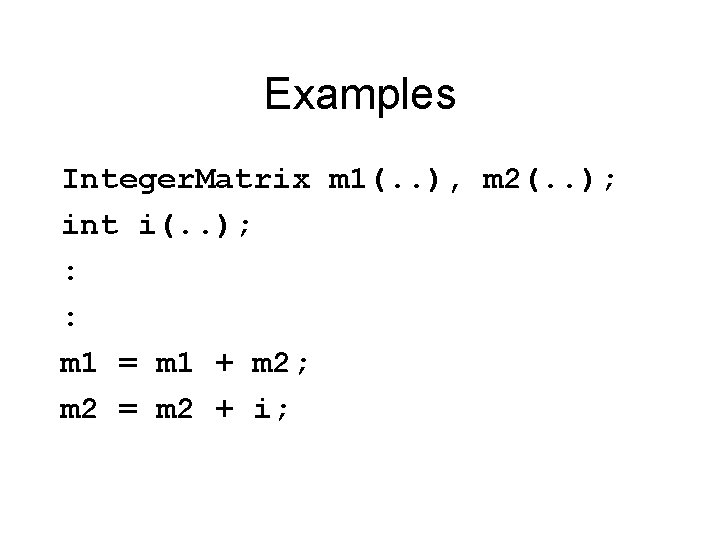
; : Examples • class String. Array { : : String &operator[]( int index ); :](https://slidetodoc.com/presentation_image/dc525d20d0645698814c9fc1744780bb/image-14.jpg)
. . – To use: String. Examples • String &String: : operator[]( int index ). . – To use: String.](https://slidetodoc.com/presentation_image/dc525d20d0645698814c9fc1744780bb/image-15.jpg)
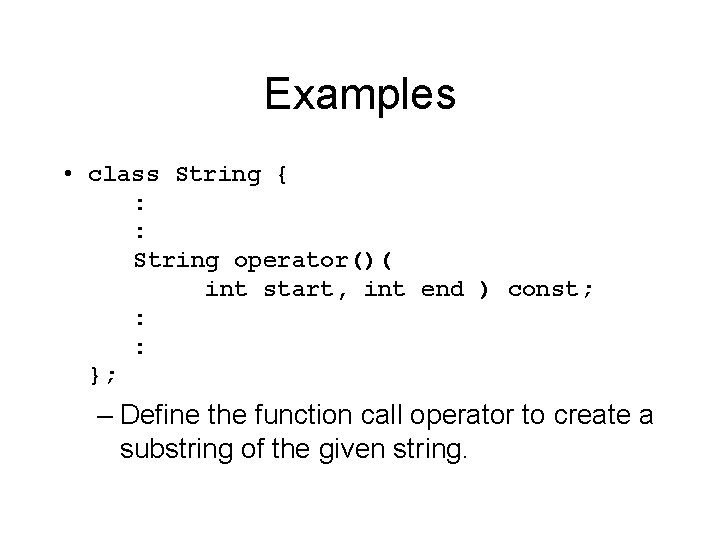
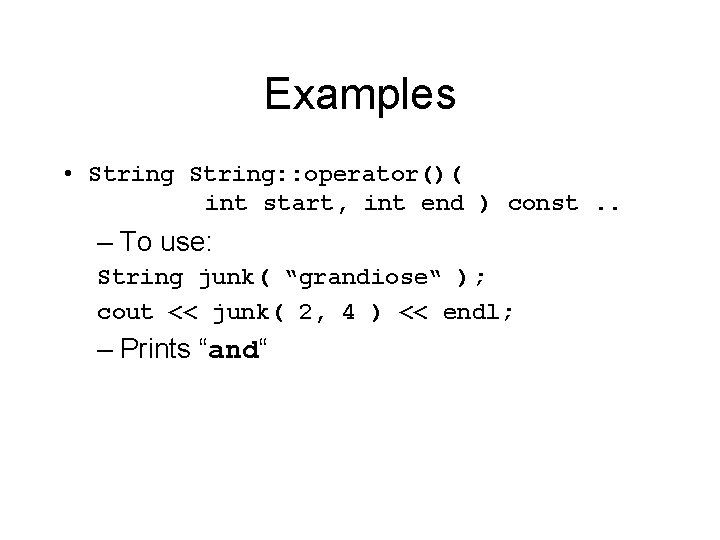
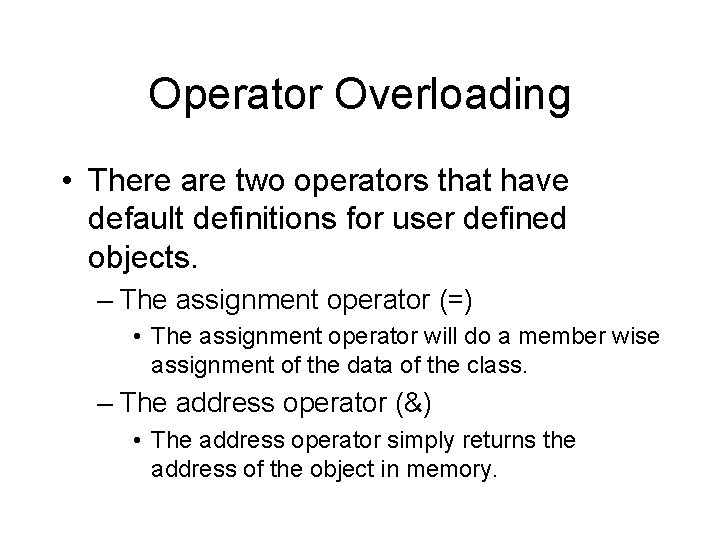
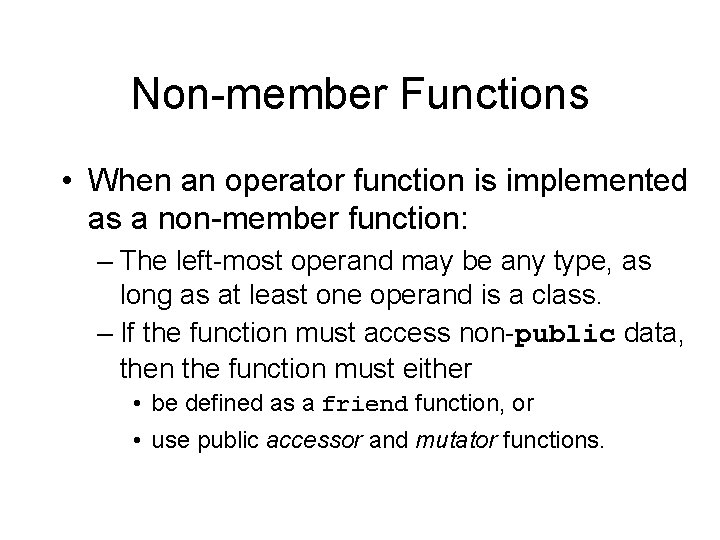
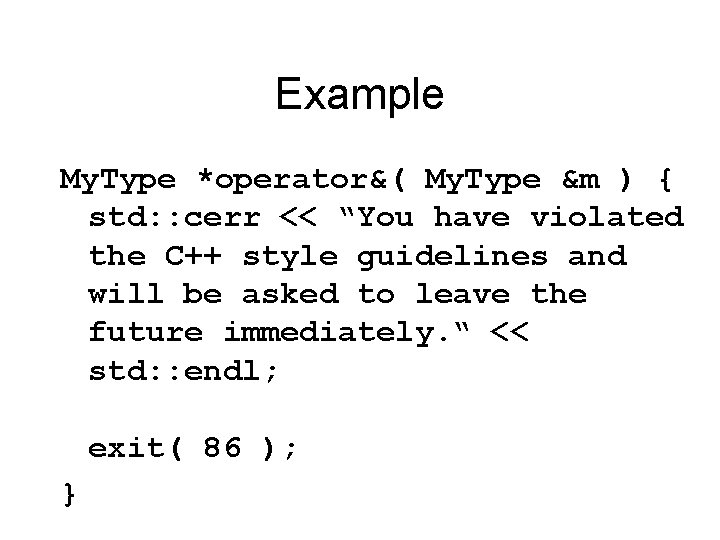
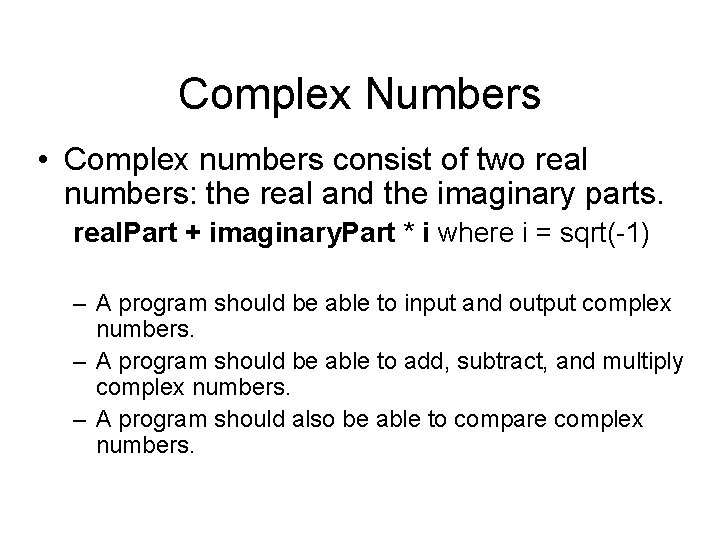
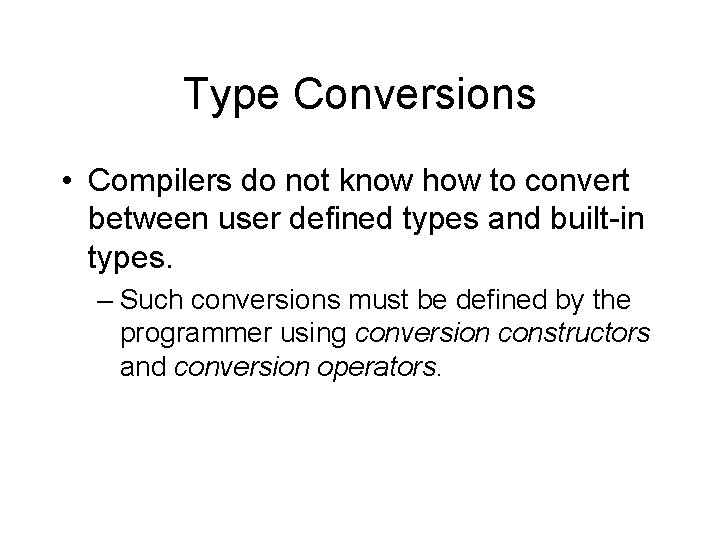
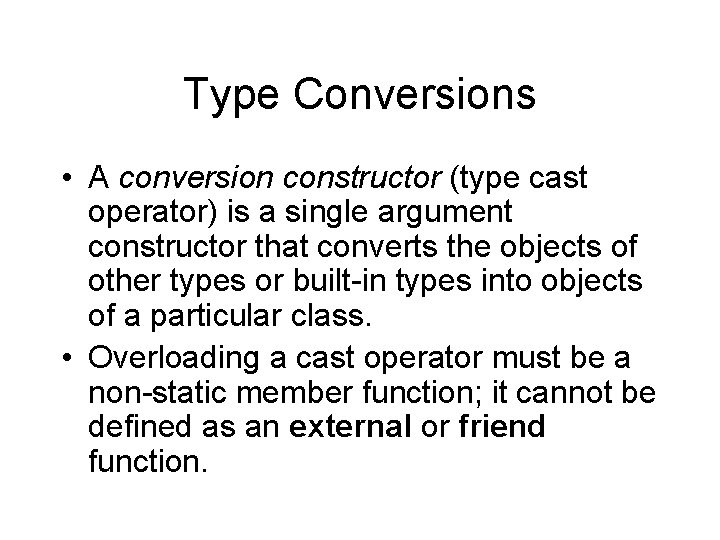
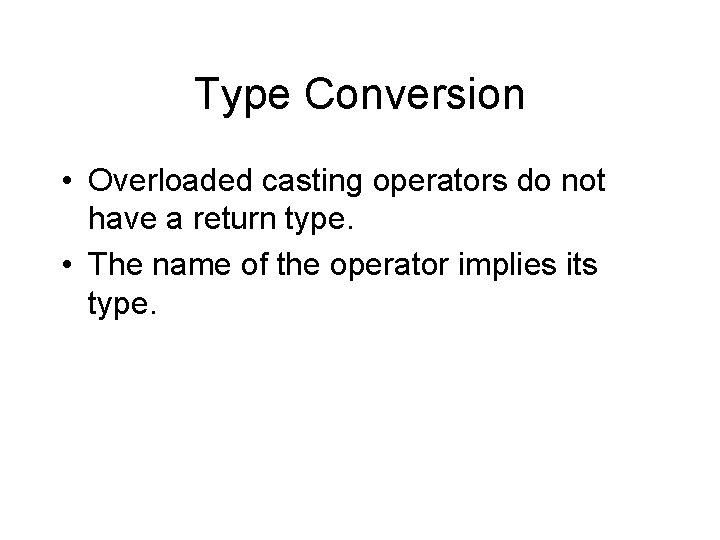
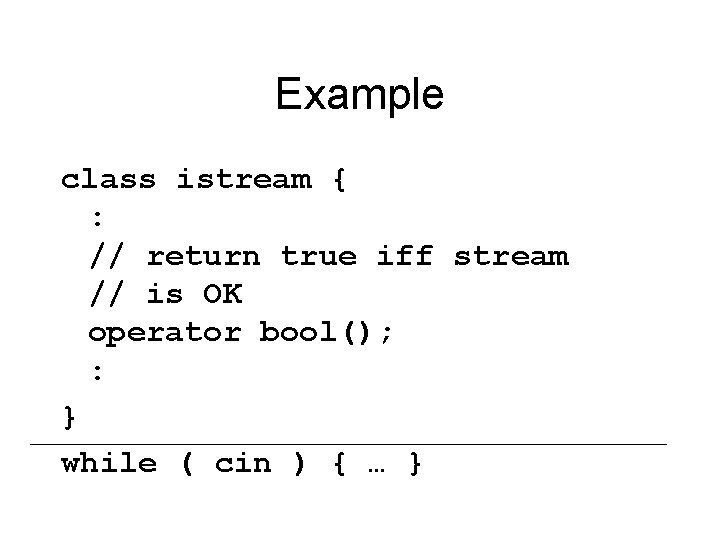
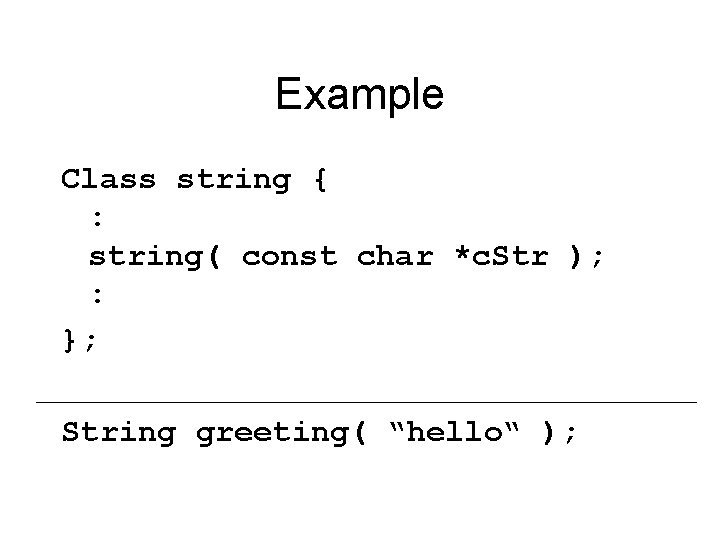
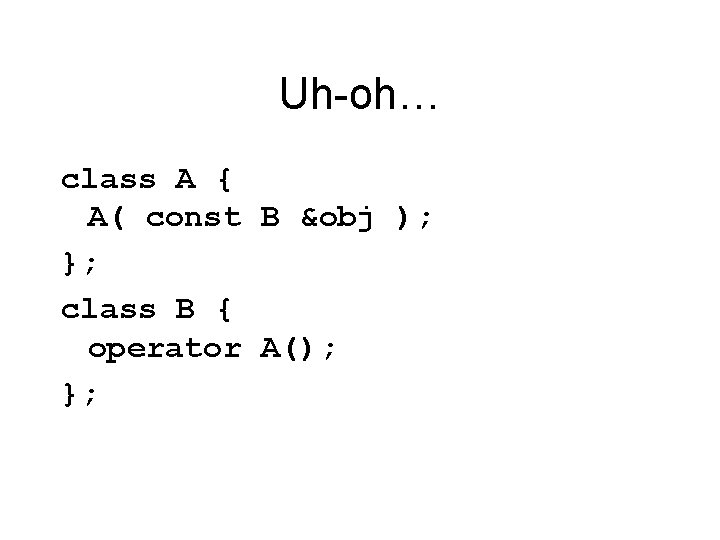
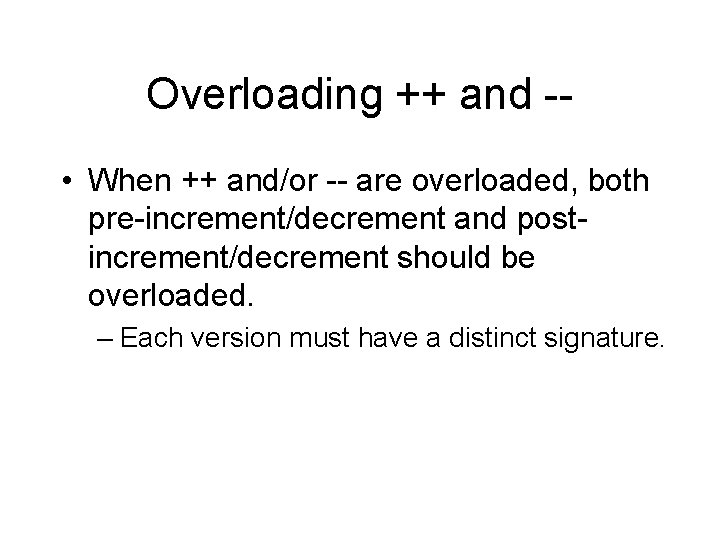
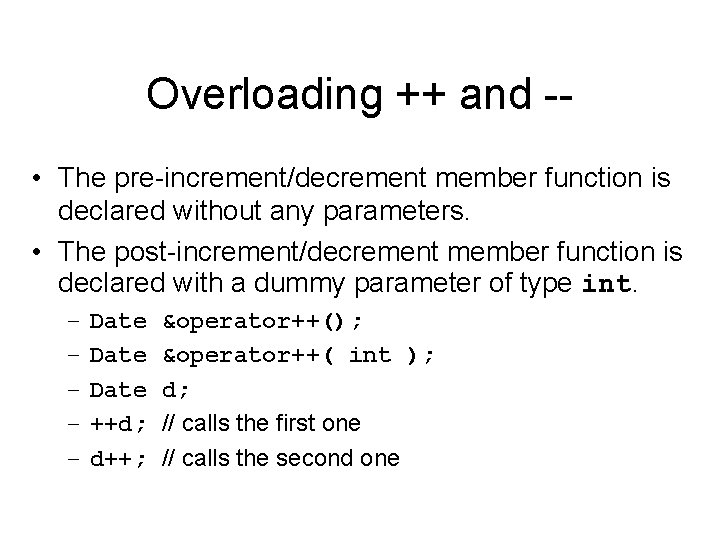
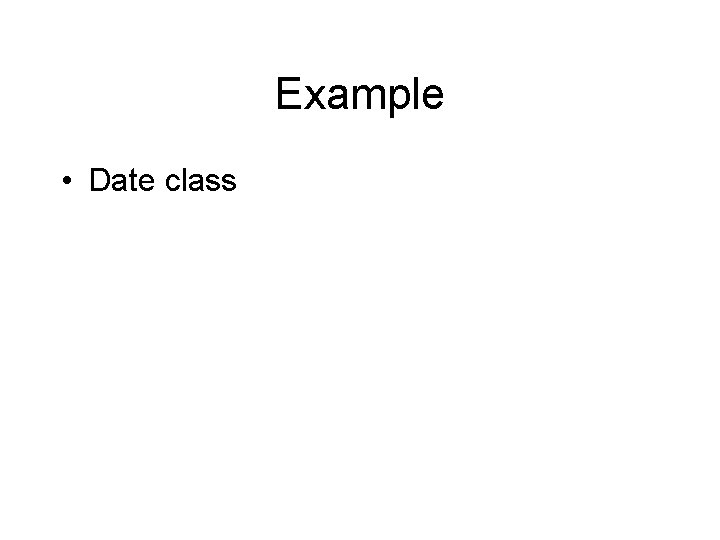
- Slides: 30
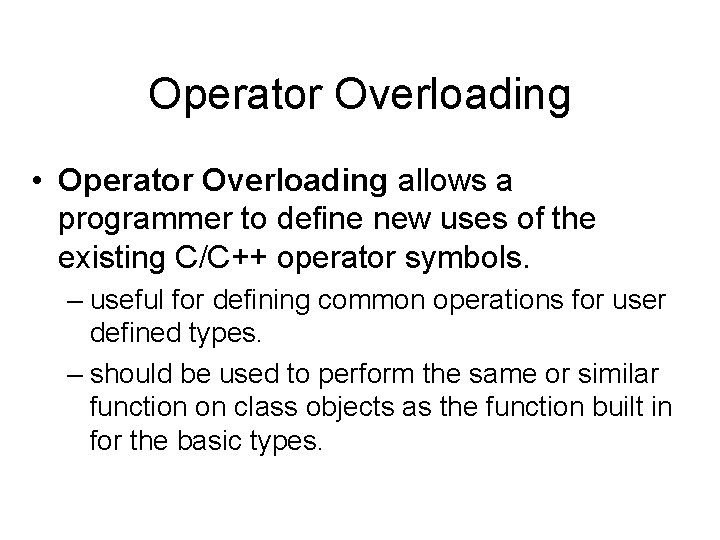
Operator Overloading • Operator Overloading allows a programmer to define new uses of the existing C/C++ operator symbols. – useful for defining common operations for user defined types. – should be used to perform the same or similar function on class objects as the function built in for the basic types.
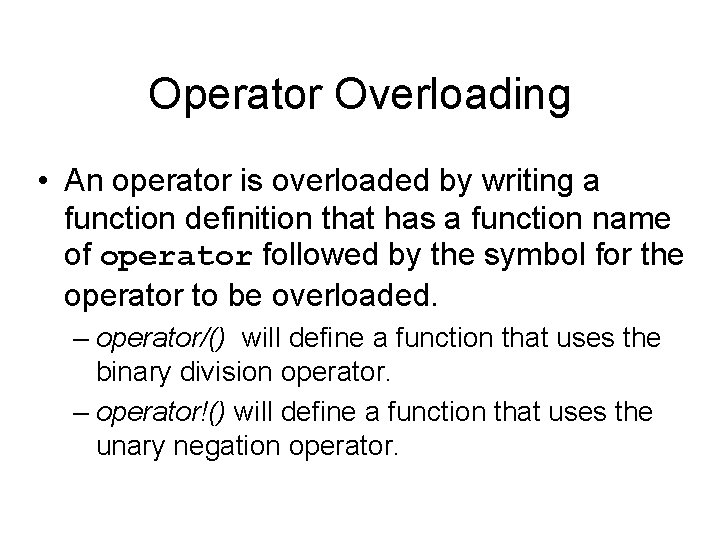
Operator Overloading • An operator is overloaded by writing a function definition that has a function name of operator followed by the symbol for the operator to be overloaded. – operator/() will define a function that uses the binary division operator. – operator!() will define a function that uses the unary negation operator.
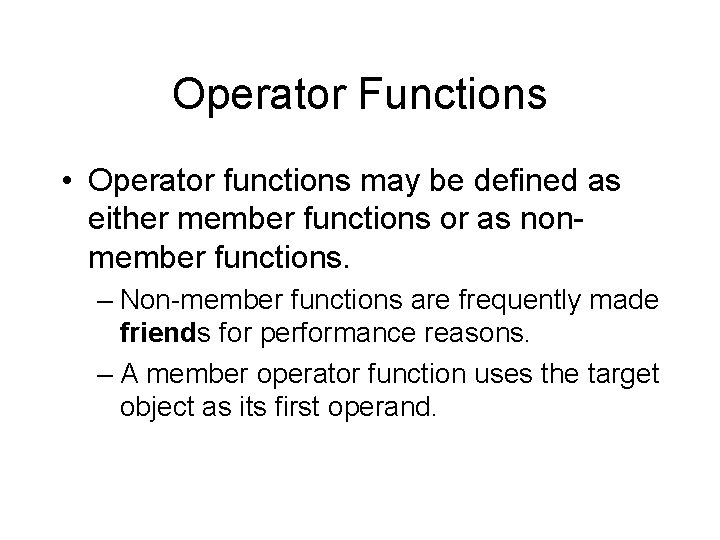
Operator Functions • Operator functions may be defined as either member functions or as nonmember functions. – Non-member functions are frequently made friends for performance reasons. – A member operator function uses the target object as its first operand.
![Operator Functions The operator overloading functions for overloading or the Operator Functions • The operator overloading functions for overloading (), [], -> or the](https://slidetodoc.com/presentation_image/dc525d20d0645698814c9fc1744780bb/image-4.jpg)
Operator Functions • The operator overloading functions for overloading (), [], -> or the assignment operators must be declared as class member functions. • All other operators may be declared as non-member functions.
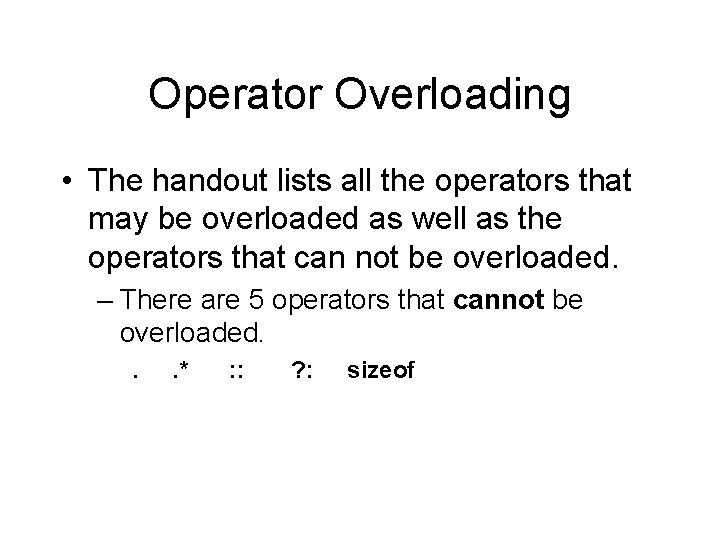
Operator Overloading • The handout lists all the operators that may be overloaded as well as the operators that can not be overloaded. – There are 5 operators that cannot be overloaded. . . * : : ? : sizeof
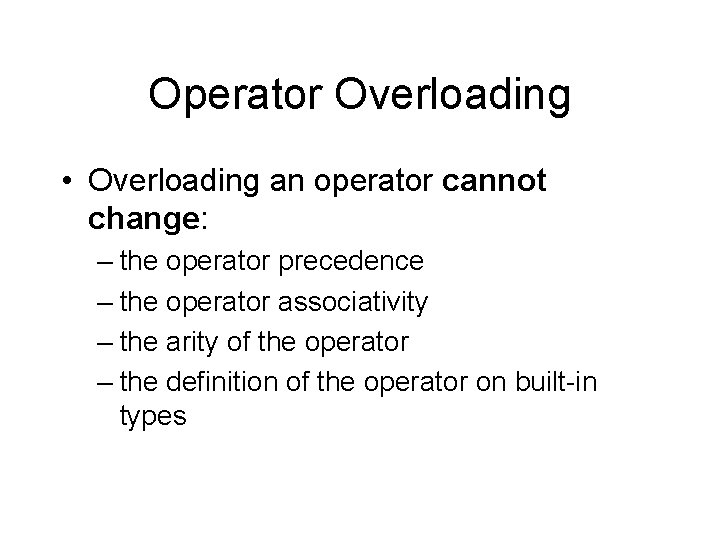
Operator Overloading • Overloading an operator cannot change: – the operator precedence – the operator associativity – the arity of the operator – the definition of the operator on built-in types
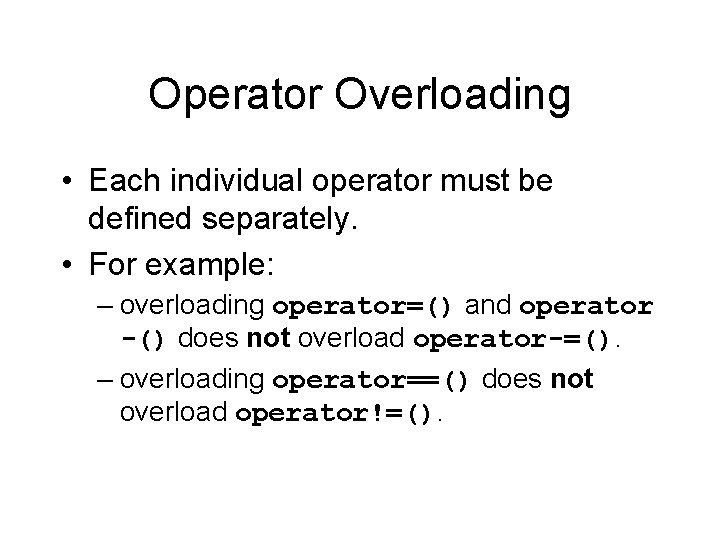
Operator Overloading • Each individual operator must be defined separately. • For example: – overloading operator=() and operator -() does not overload operator-=(). – overloading operator==() does not overload operator!=().
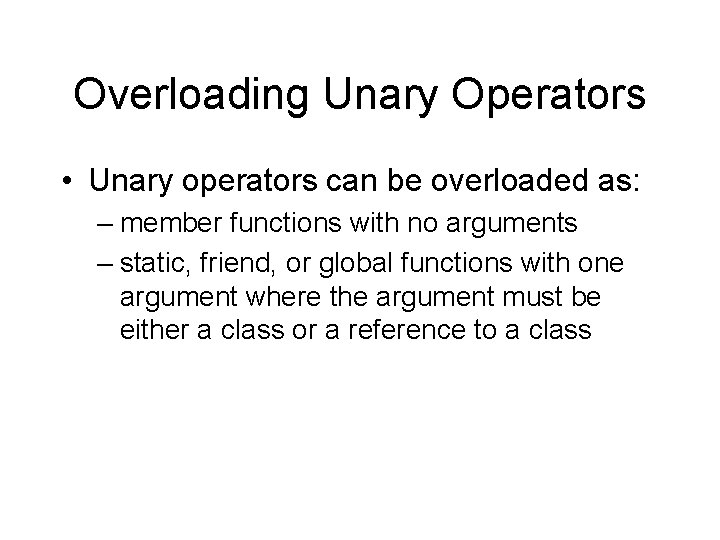
Overloading Unary Operators • Unary operators can be overloaded as: – member functions with no arguments – static, friend, or global functions with one argument where the argument must be either a class or a reference to a class
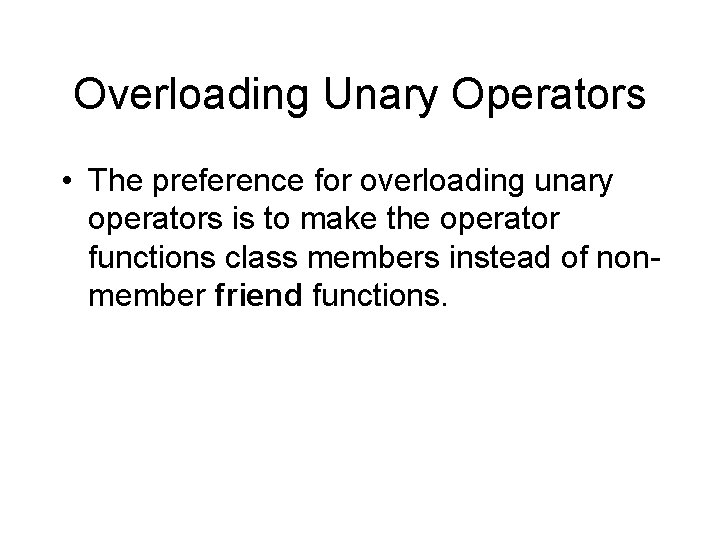
Overloading Unary Operators • The preference for overloading unary operators is to make the operator functions class members instead of nonmember friend functions.
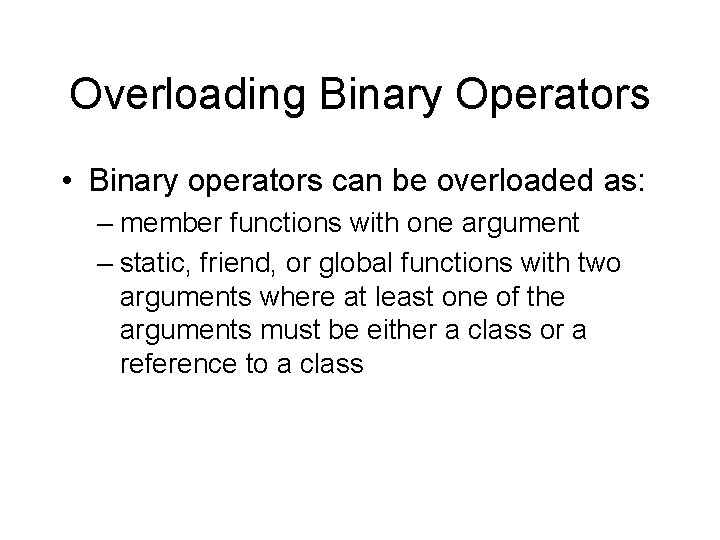
Overloading Binary Operators • Binary operators can be overloaded as: – member functions with one argument – static, friend, or global functions with two arguments where at least one of the arguments must be either a class or a reference to a class
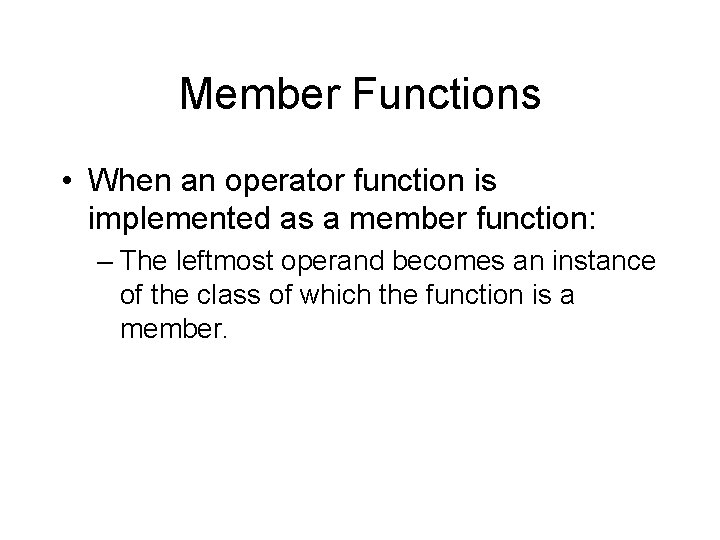
Member Functions • When an operator function is implemented as a member function: – The leftmost operand becomes an instance of the class of which the function is a member.
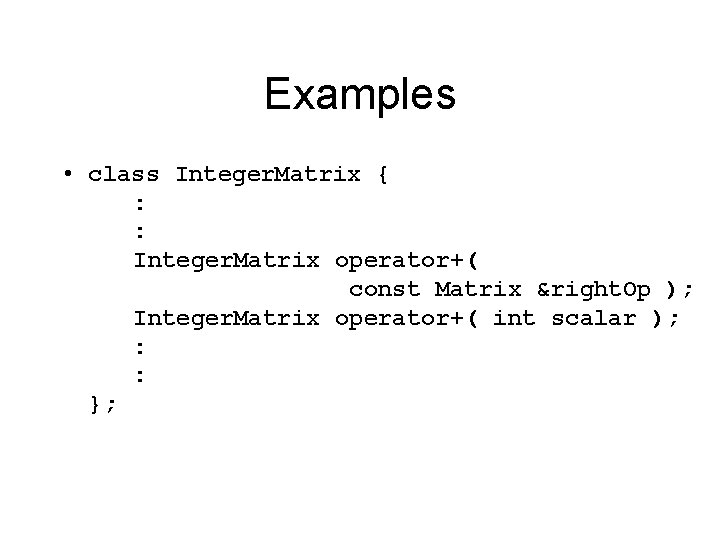
Examples • class Integer. Matrix { : : Integer. Matrix operator+( const Matrix &right. Op ); Integer. Matrix operator+( int scalar ); : : };
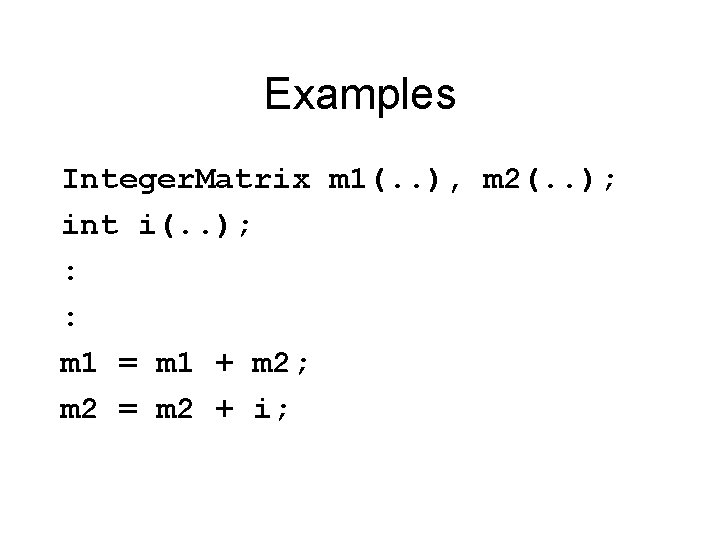
Examples Integer. Matrix m 1(. . ), m 2(. . ); int i(. . ); : : m 1 = m 1 + m 2; m 2 = m 2 + i;
; :](https://slidetodoc.com/presentation_image/dc525d20d0645698814c9fc1744780bb/image-14.jpg)
Examples • class String. Array { : : String &operator[]( int index ); : : }; – Define the indexing operator to work on String. Arrays indexed with integers.
. . – To use: String.](https://slidetodoc.com/presentation_image/dc525d20d0645698814c9fc1744780bb/image-15.jpg)
Examples • String &String: : operator[]( int index ). . – To use: String. Array stones(…); cout << “ 5 th element = “ << stones[4]; int i( 0 ); stones[ i ] = “air“;
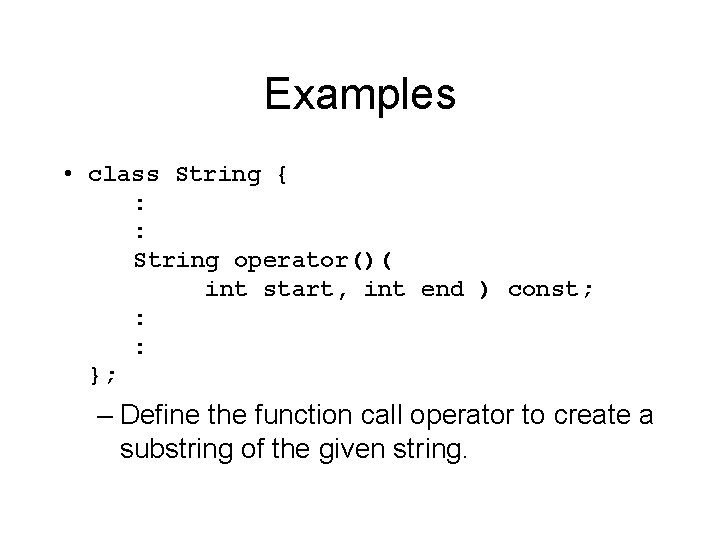
Examples • class String { : : String operator()( int start, int end ) const; : : }; – Define the function call operator to create a substring of the given string.
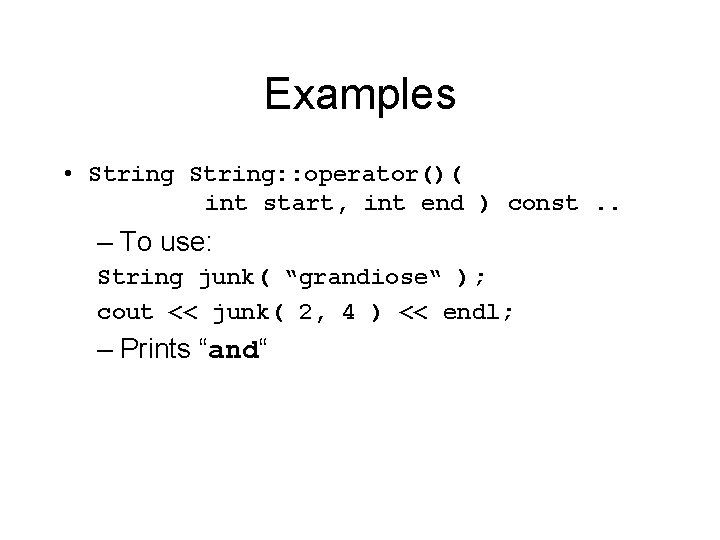
Examples • String: : operator()( int start, int end ) const. . – To use: String junk( “grandiose“ ); cout << junk( 2, 4 ) << endl; – Prints “and“
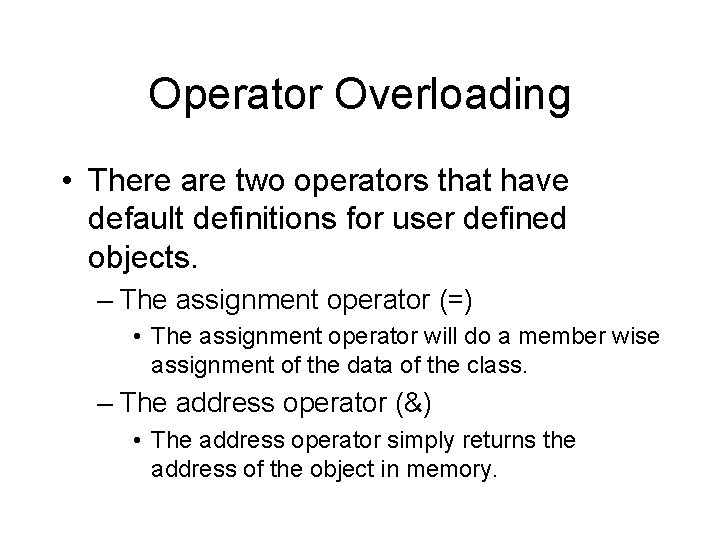
Operator Overloading • There are two operators that have default definitions for user defined objects. – The assignment operator (=) • The assignment operator will do a member wise assignment of the data of the class. – The address operator (&) • The address operator simply returns the address of the object in memory.
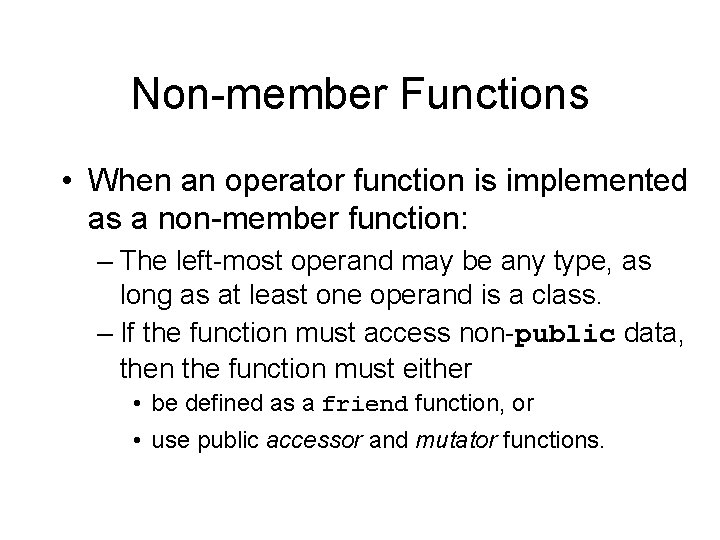
Non-member Functions • When an operator function is implemented as a non-member function: – The left-most operand may be any type, as long as at least one operand is a class. – If the function must access non-public data, then the function must either • be defined as a friend function, or • use public accessor and mutator functions.
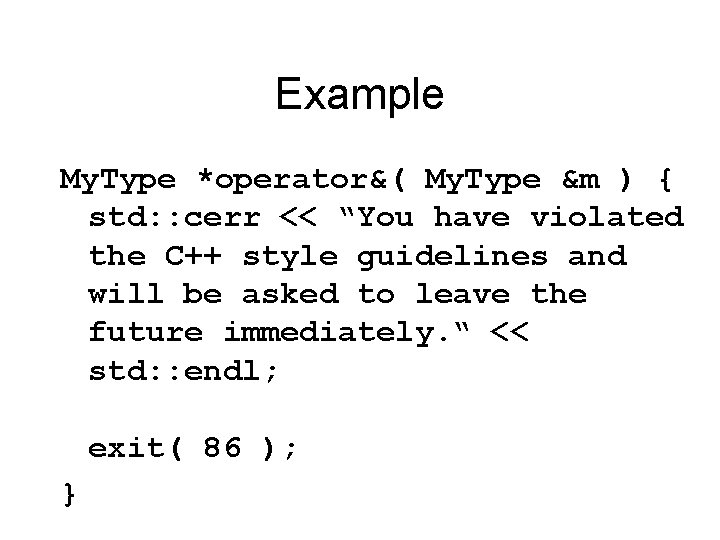
Example My. Type *operator&( My. Type &m ) { std: : cerr << “You have violated the C++ style guidelines and will be asked to leave the future immediately. “ << std: : endl; exit( 86 ); }
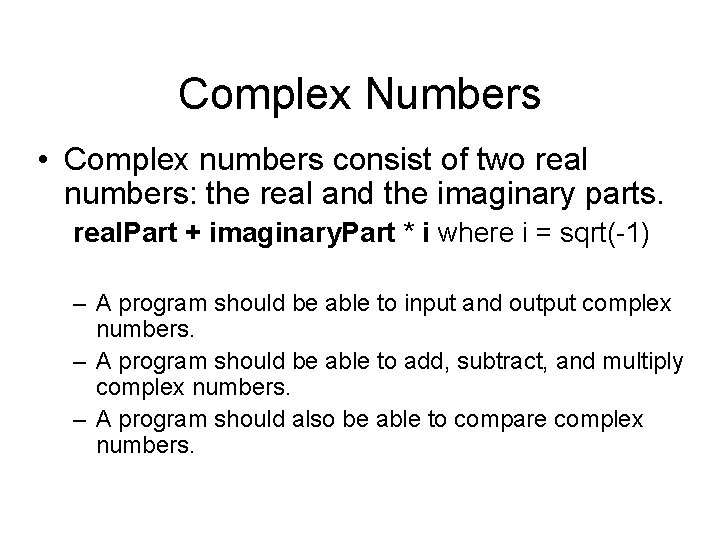
Complex Numbers • Complex numbers consist of two real numbers: the real and the imaginary parts. real. Part + imaginary. Part * i where i = sqrt(-1) – A program should be able to input and output complex numbers. – A program should be able to add, subtract, and multiply complex numbers. – A program should also be able to compare complex numbers.
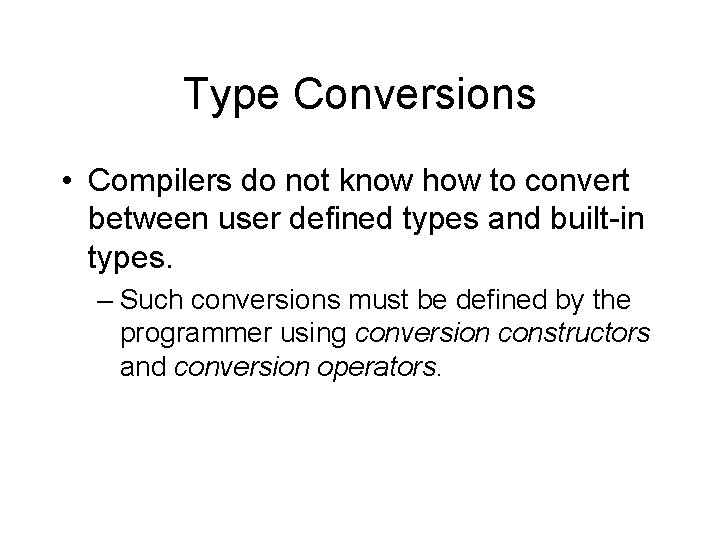
Type Conversions • Compilers do not know how to convert between user defined types and built-in types. – Such conversions must be defined by the programmer using conversion constructors and conversion operators.
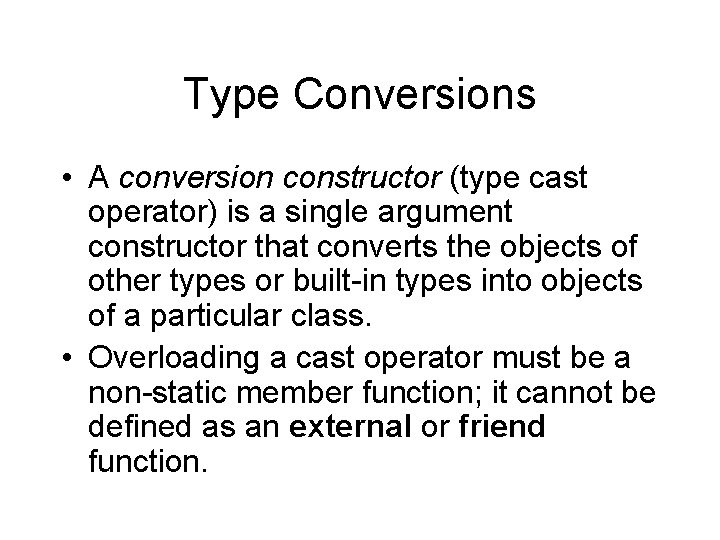
Type Conversions • A conversion constructor (type cast operator) is a single argument constructor that converts the objects of other types or built-in types into objects of a particular class. • Overloading a cast operator must be a non-static member function; it cannot be defined as an external or friend function.
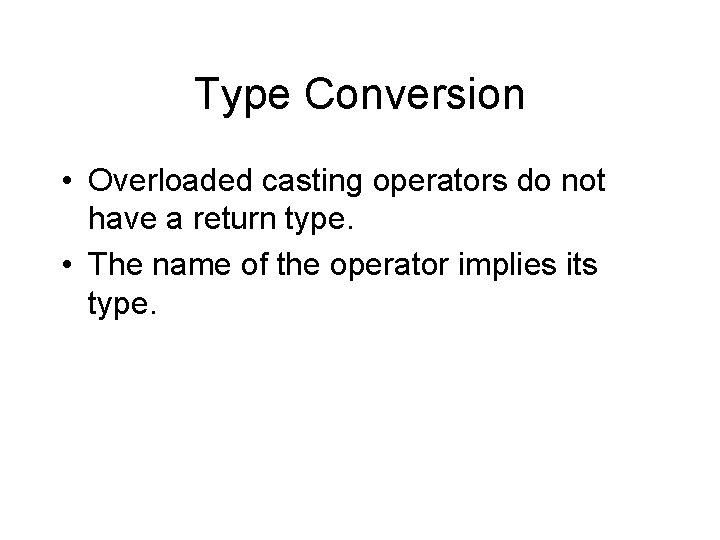
Type Conversion • Overloaded casting operators do not have a return type. • The name of the operator implies its type.
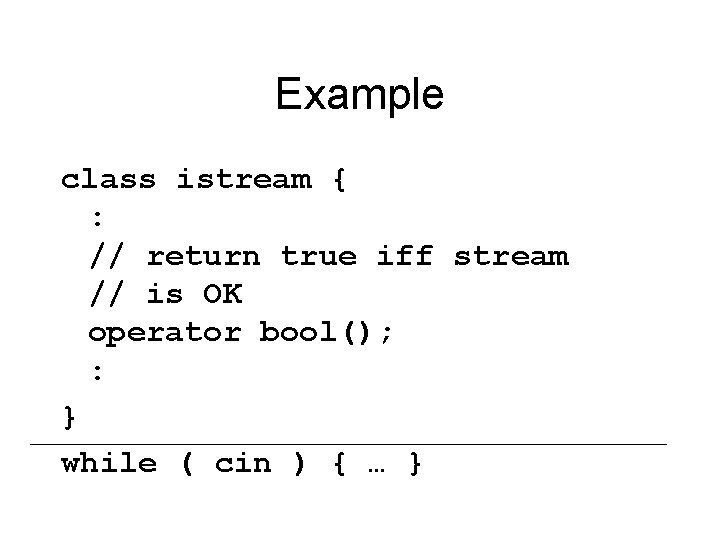
Example class istream { : // return true iff stream // is OK operator bool(); : } while ( cin ) { … }
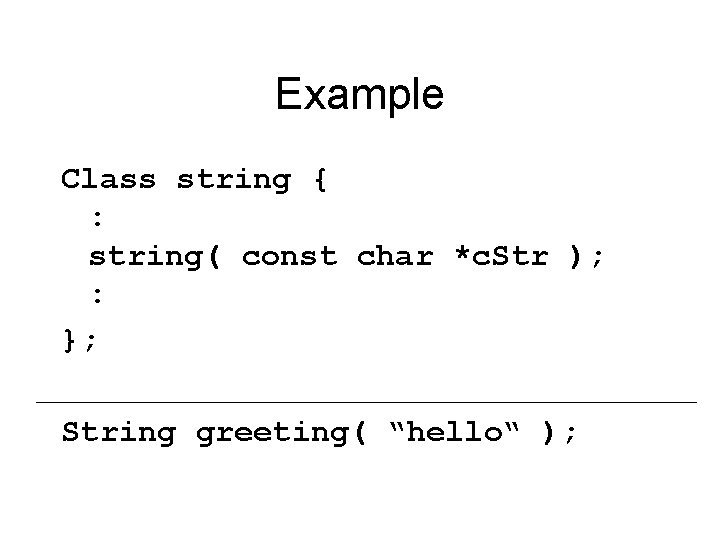
Example Class string { : string( const char *c. Str ); : }; String greeting( “hello“ );
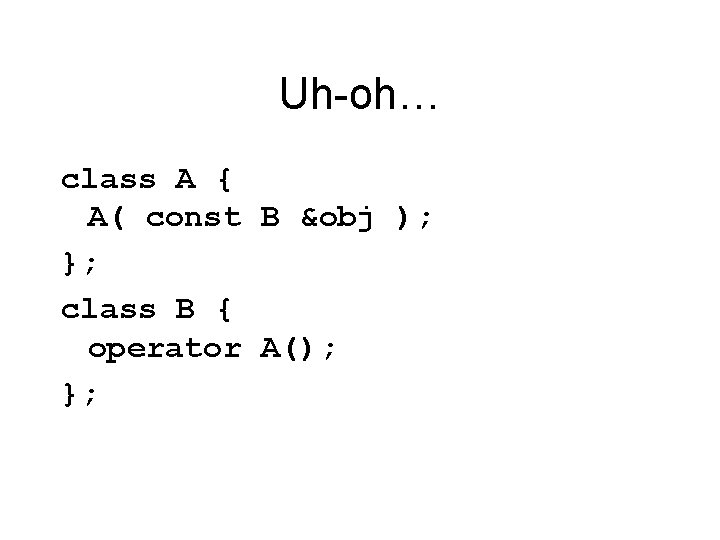
Uh-oh… class A { A( const B &obj ); }; class B { operator A(); };
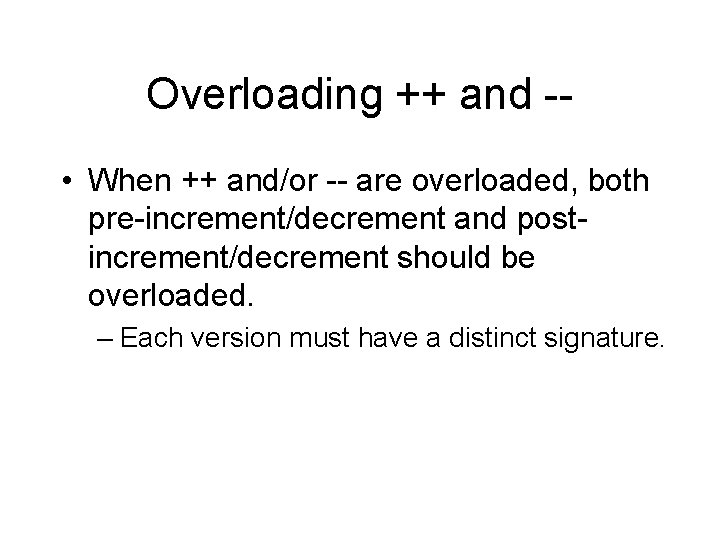
Overloading ++ and - • When ++ and/or -- are overloaded, both pre-increment/decrement and postincrement/decrement should be overloaded. – Each version must have a distinct signature.
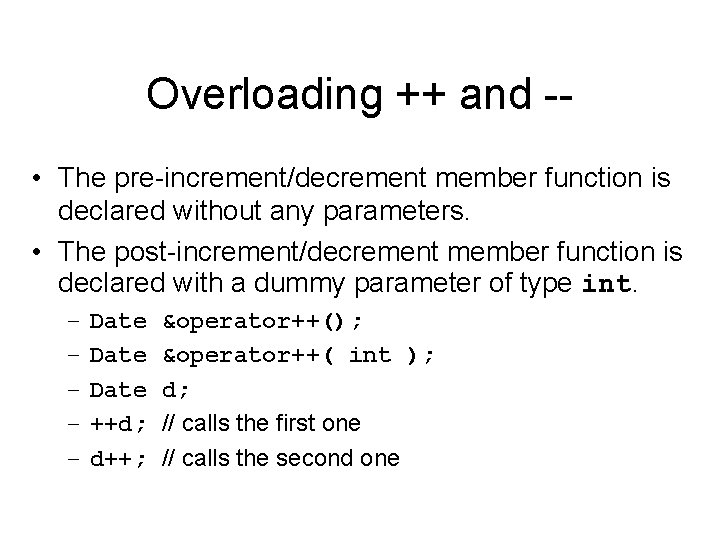
Overloading ++ and - • The pre-increment/decrement member function is declared without any parameters. • The post-increment/decrement member function is declared with a dummy parameter of type int. – – – Date ++d; d++; &operator++(); &operator++( int ); d; // calls the first one // calls the second one
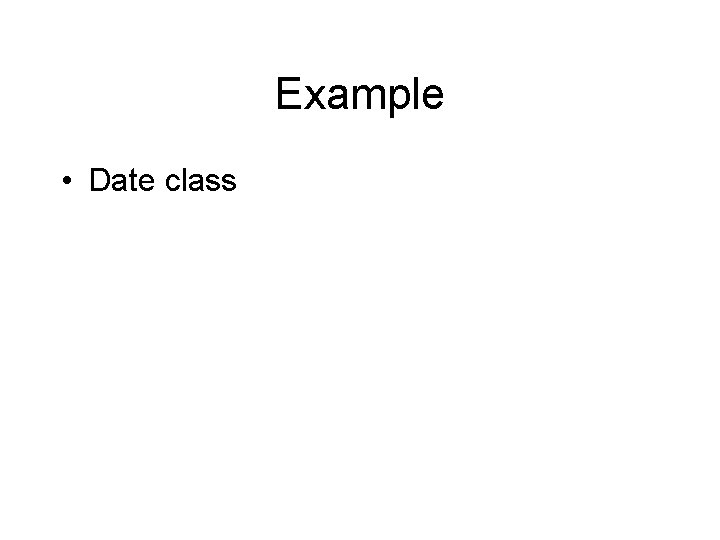
Example • Date class
Pitfalls of operator overloading in c++
Delete operator overloading in c++
Cps235
Binary operator overloading using friend function
Unary operator overloading
Compound assignment operators in c with example
Overload parenthesis operator c++
Operators overloaded for string objects
Overload stream insertion operator c++ template
Cps235
Berbagai macam operator beserta prioritas operator
Binary and unary operators
Pada tipe data boolean berlaku operator-operator
Pada tipe data boolean berlaku operator-operator
Enigma programmer
A programmer can choose to ignore an unchecked exception.
Computer programer job description
Therac 25 programmer
Etika programmer
Cure programmer
Competent programmer hypothesis
Iproxcard programmer
Etika programmer
Seasoned programmer
Hardware programmer
Computer programmer
Intel fpga download cable
Mercedes smart key programming
My future profession programmer
Programmer excuses
Computer programmer bls