CHAPTER 4 CS 3370 C Expressions Operators Unary
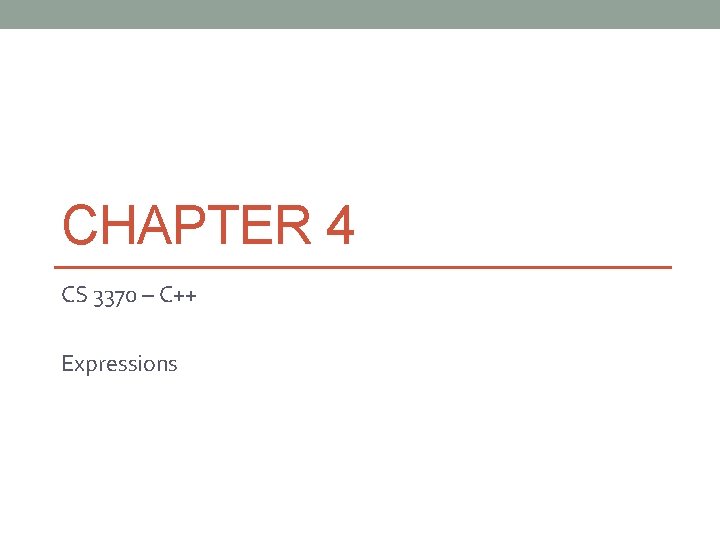
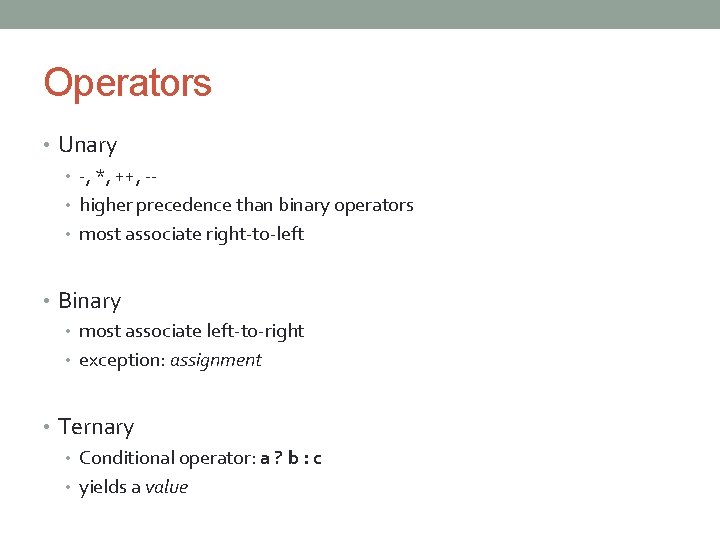
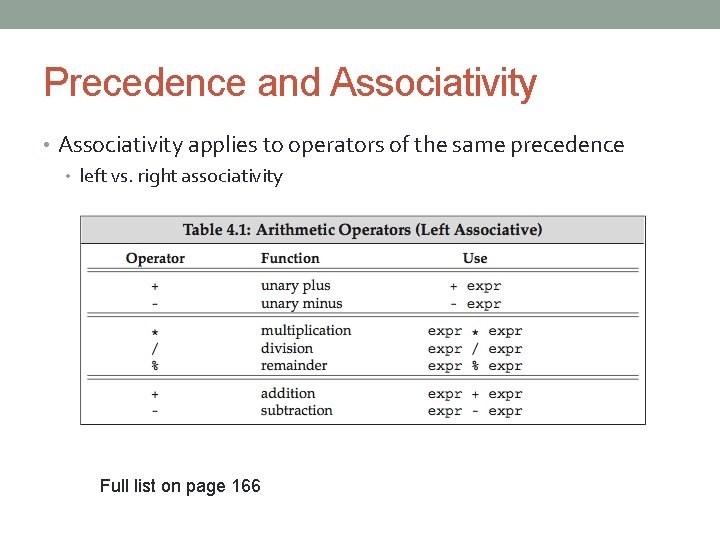
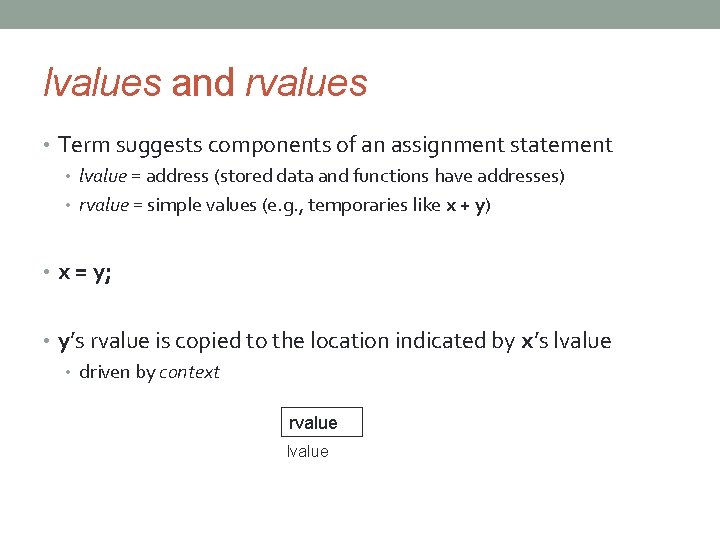
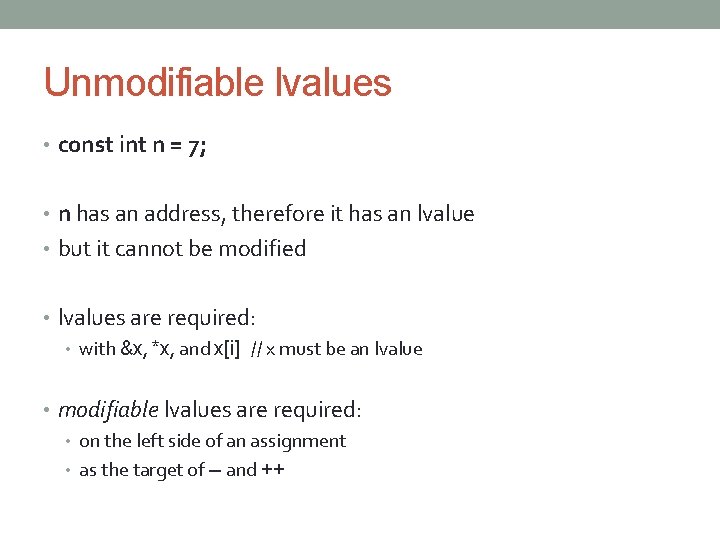
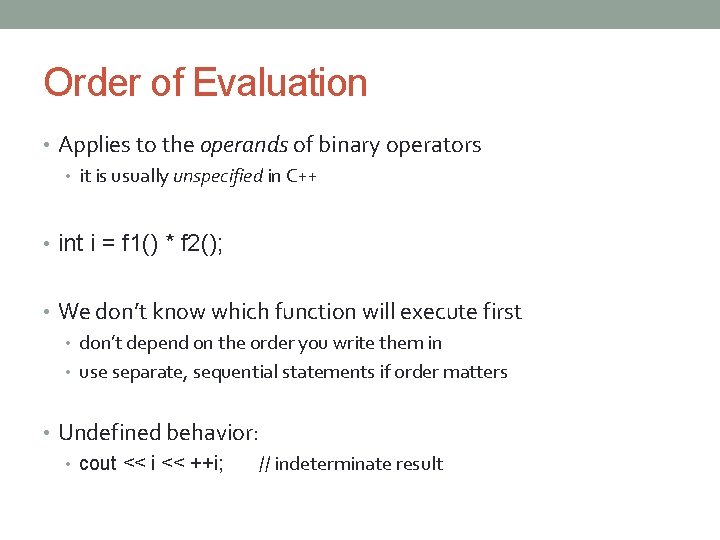
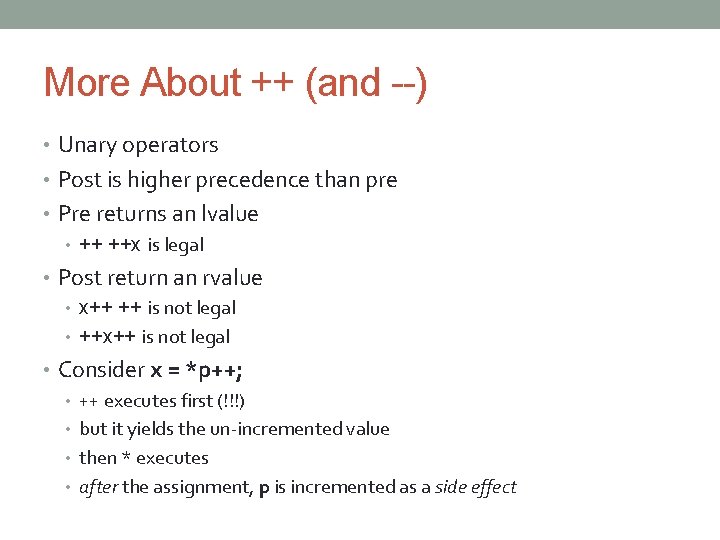
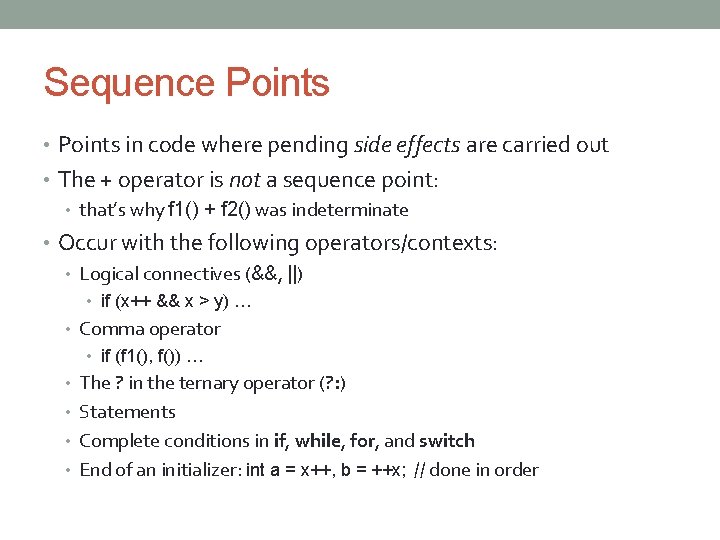
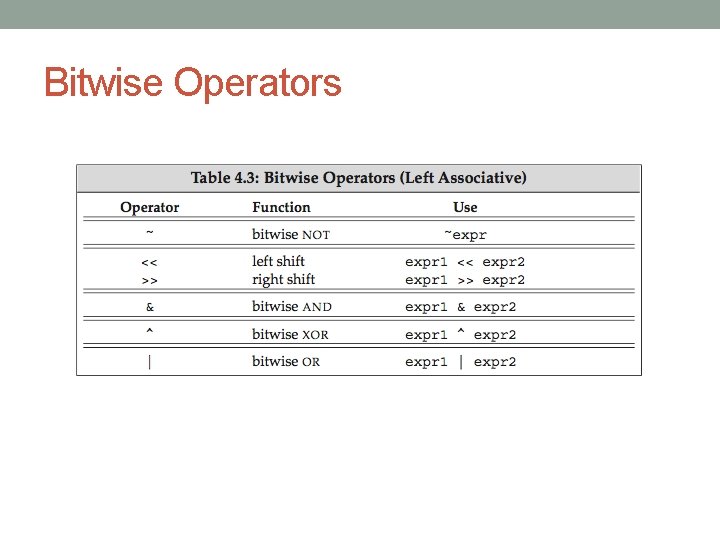
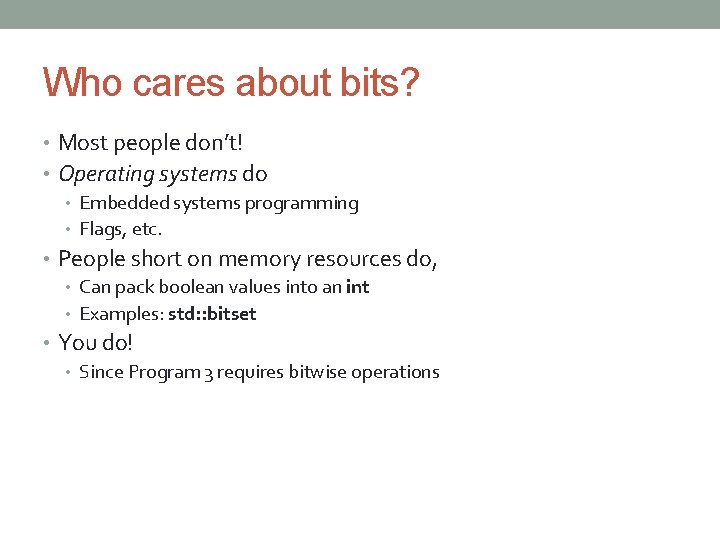
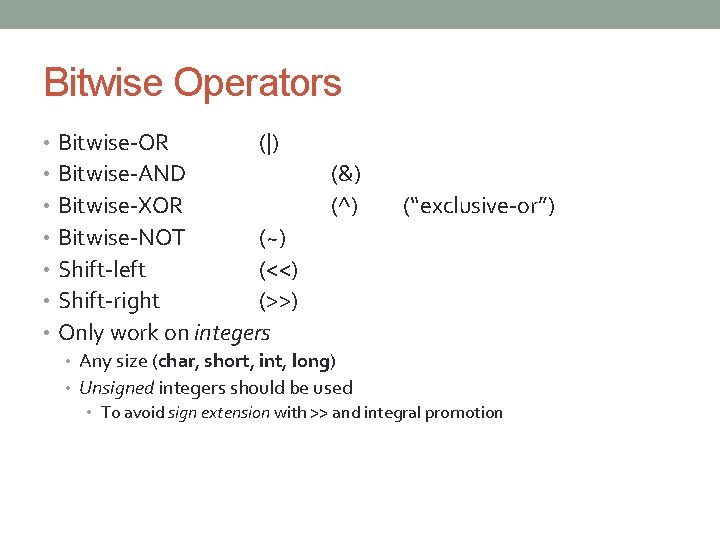
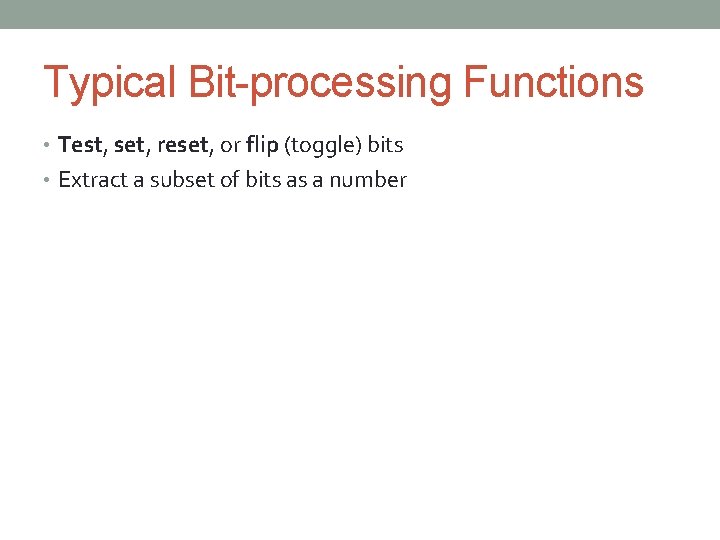
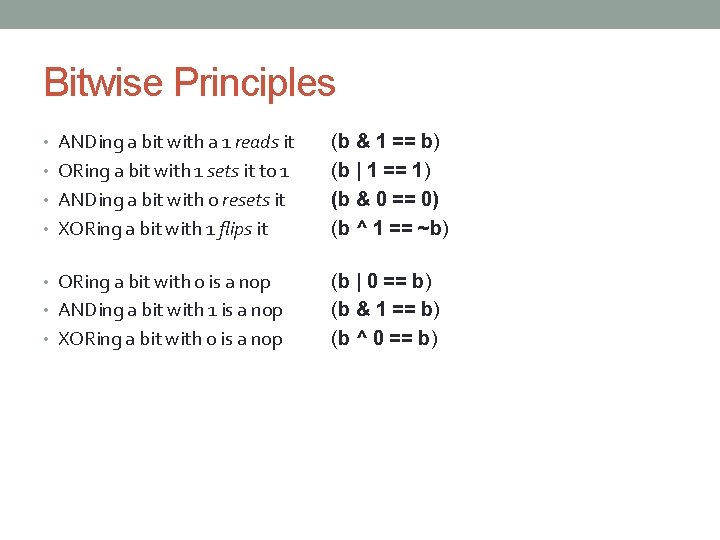
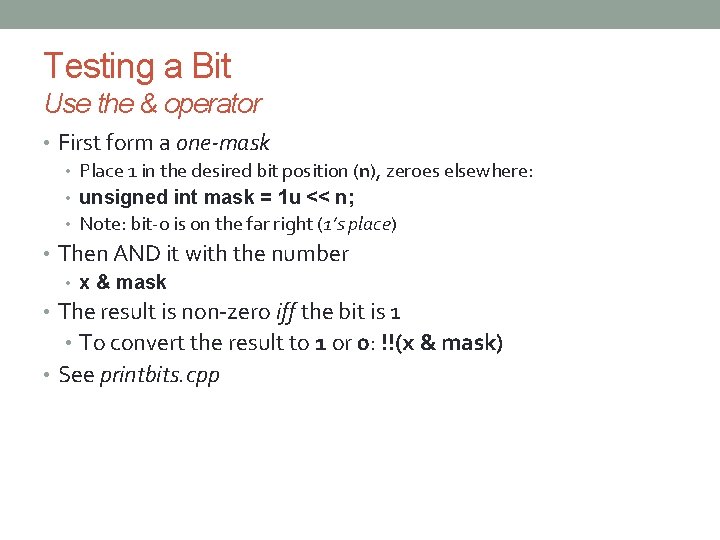
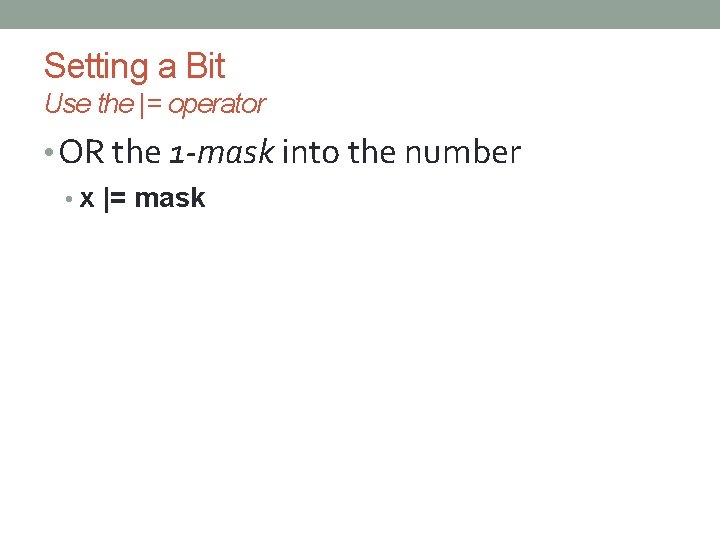
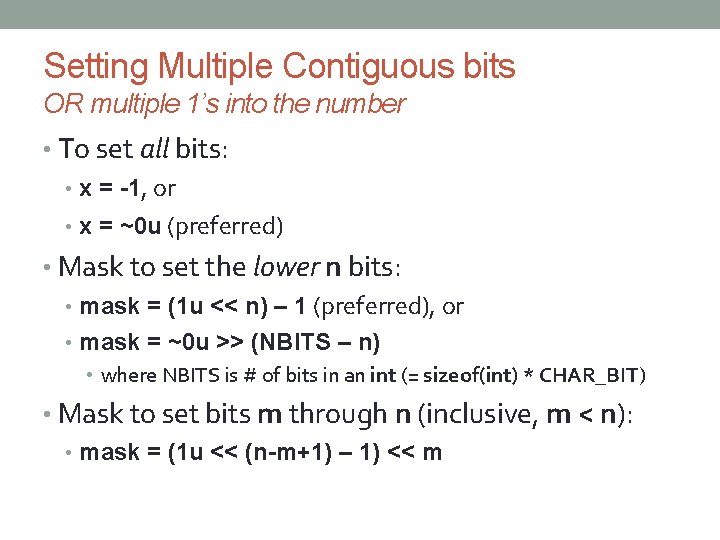
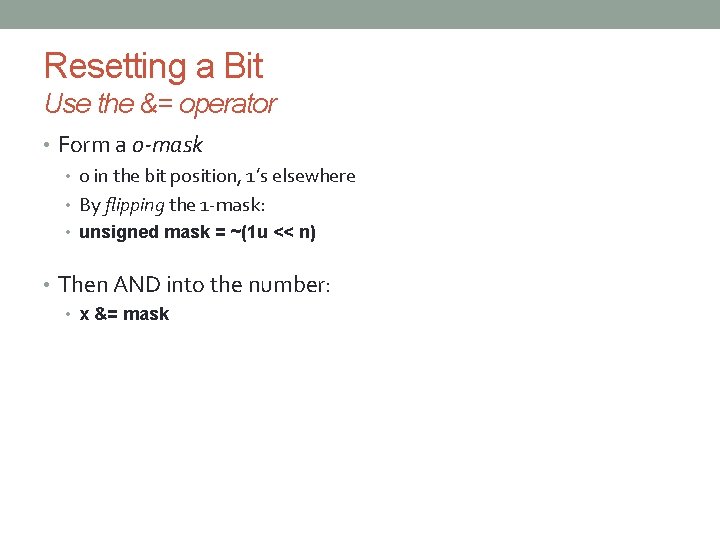
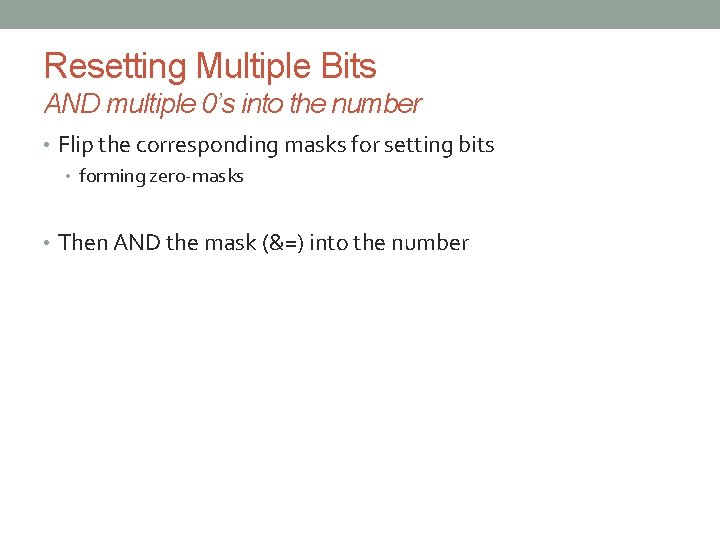
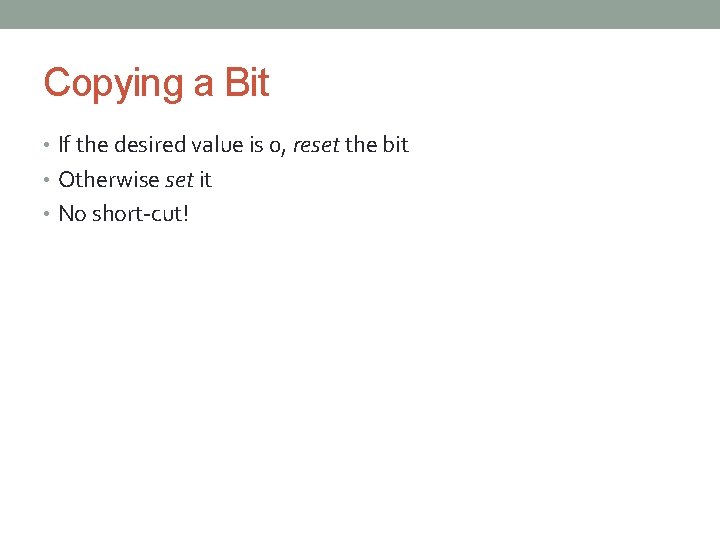
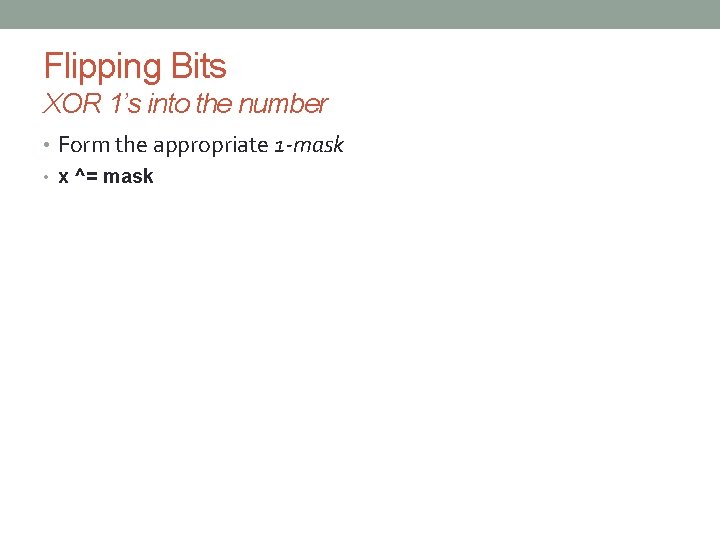
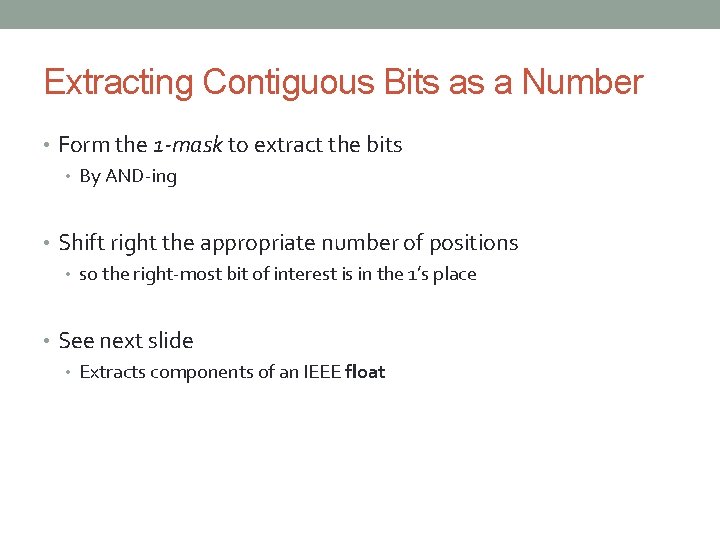
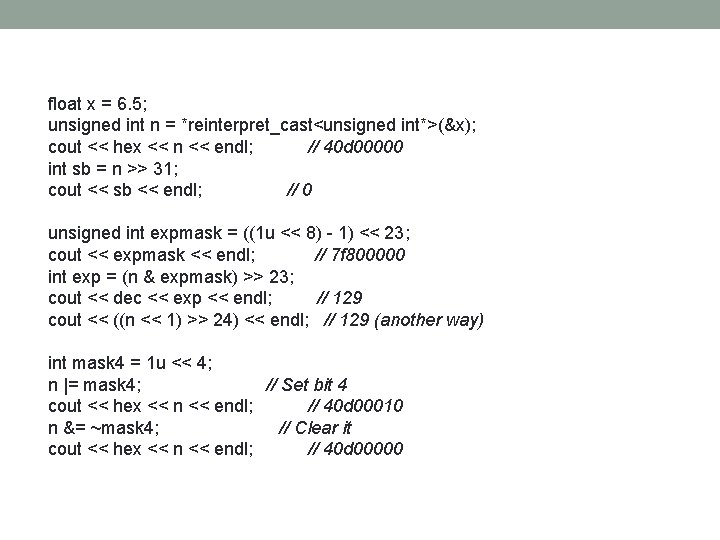
- Slides: 22
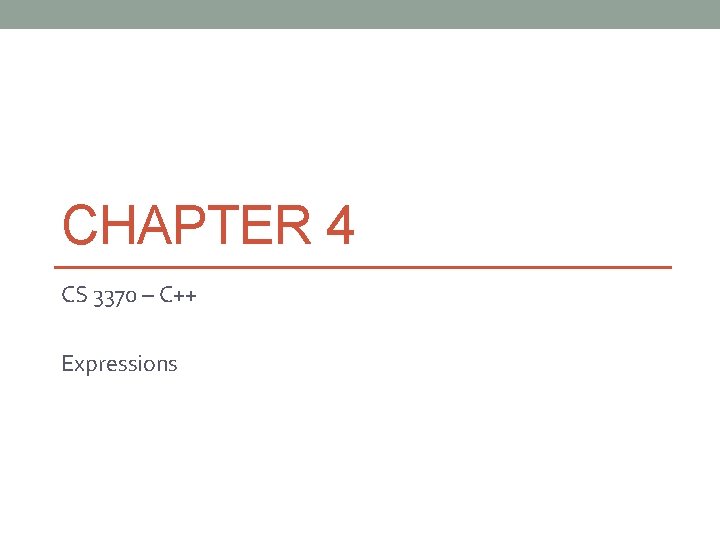
CHAPTER 4 CS 3370 – C++ Expressions
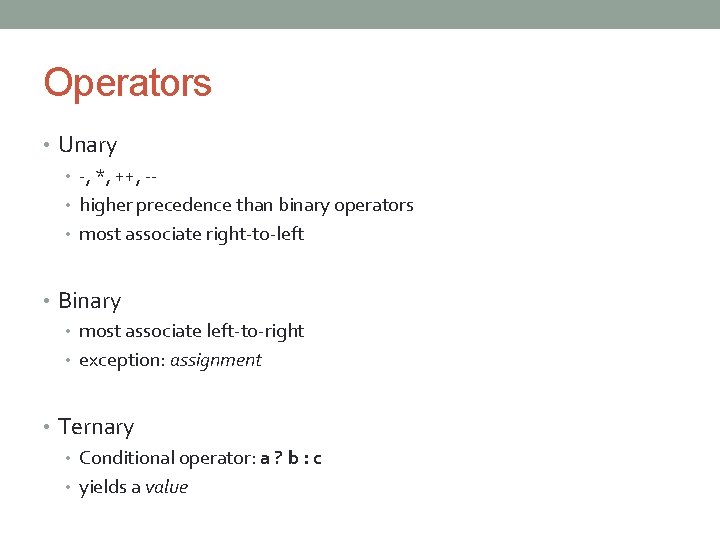
Operators • Unary • -, *, ++, - • higher precedence than binary operators • most associate right-to-left • Binary • most associate left-to-right • exception: assignment • Ternary • Conditional operator: a ? b : c • yields a value
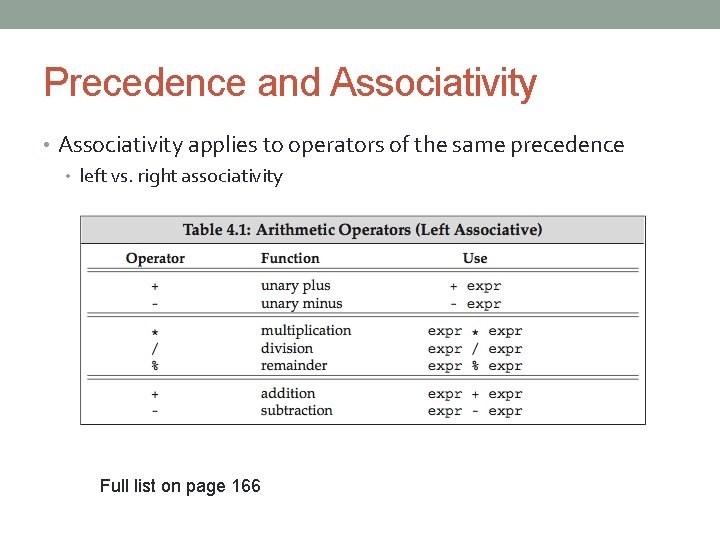
Precedence and Associativity • Associativity applies to operators of the same precedence • left vs. right associativity Full list on page 166
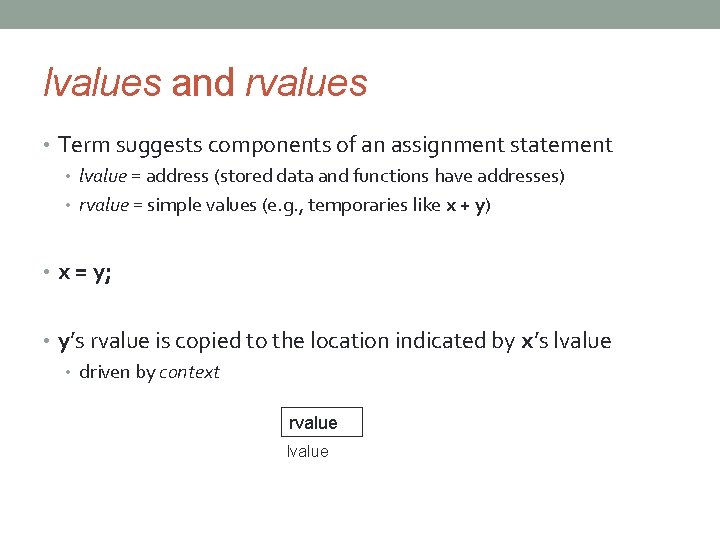
lvalues and rvalues • Term suggests components of an assignment statement • lvalue = address (stored data and functions have addresses) • rvalue = simple values (e. g. , temporaries like x + y) • x = y; • y’s rvalue is copied to the location indicated by x’s lvalue • driven by context rvalue lvalue
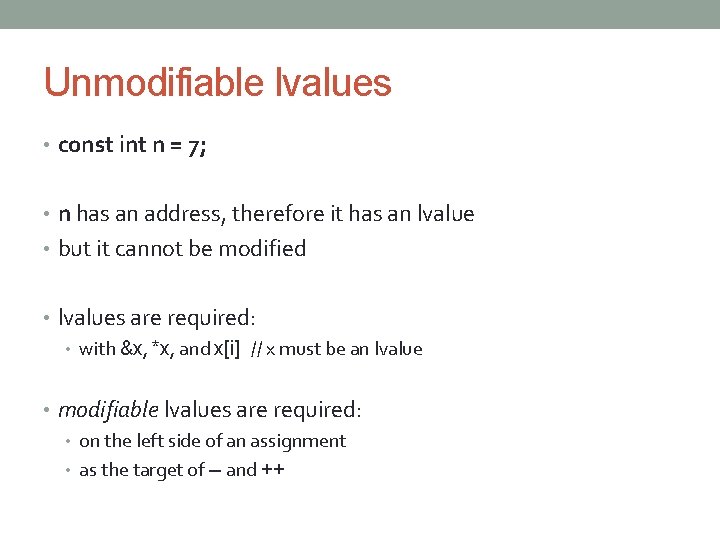
Unmodifiable lvalues • const int n = 7; • n has an address, therefore it has an lvalue • but it cannot be modified • lvalues are required: • with &x, *x, and x[i] // x must be an lvalue • modifiable lvalues are required: • on the left side of an assignment • as the target of -- and ++
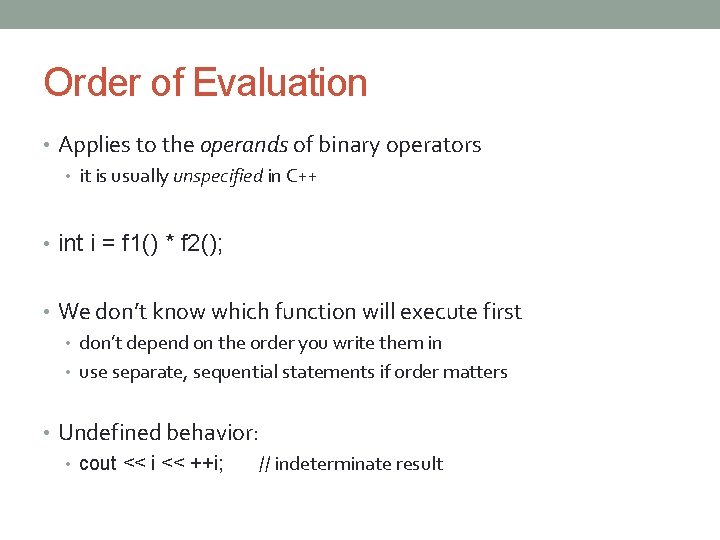
Order of Evaluation • Applies to the operands of binary operators • it is usually unspecified in C++ • int i = f 1() * f 2(); • We don’t know which function will execute first • don’t depend on the order you write them in • use separate, sequential statements if order matters • Undefined behavior: • cout << i << ++i; // indeterminate result
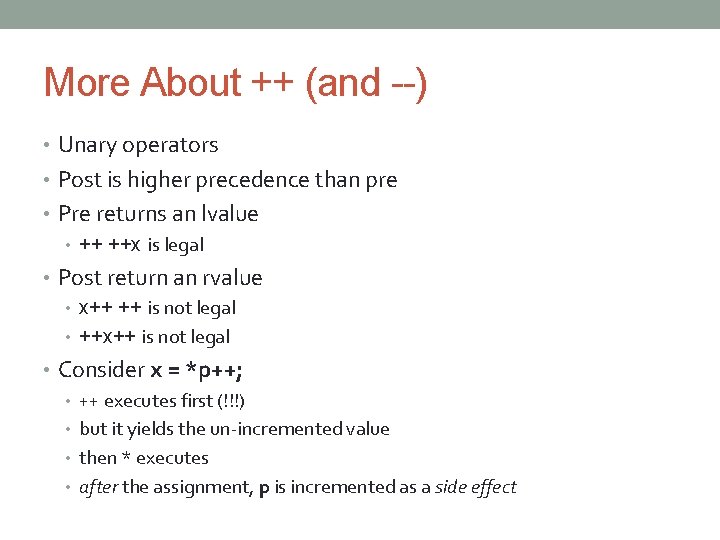
More About ++ (and --) • Unary operators • Post is higher precedence than pre • Pre returns an lvalue • ++ ++x is legal • Post return an rvalue • x++ ++ is not legal • ++x++ is not legal • Consider x = *p++; • ++ executes first (!!!) • but it yields the un-incremented value • then * executes • after the assignment, p is incremented as a side effect
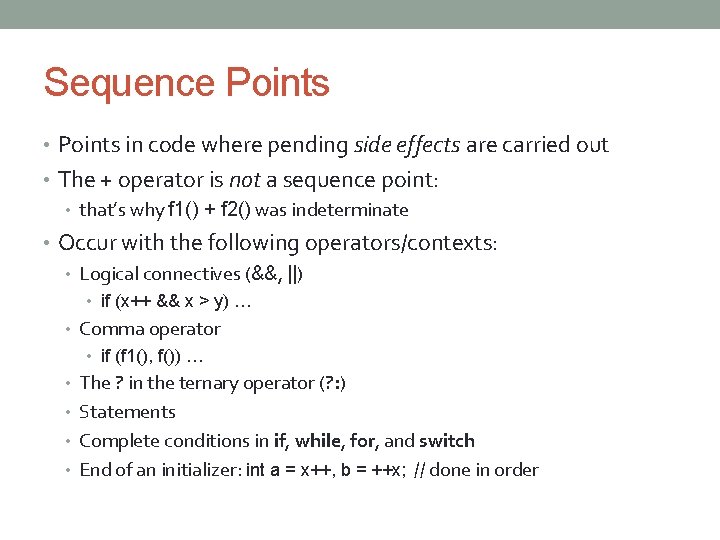
Sequence Points • Points in code where pending side effects are carried out • The + operator is not a sequence point: • that’s why f 1() + f 2() was indeterminate • Occur with the following operators/contexts: • Logical connectives (&&, ||) • if (x++ && x > y) … • Comma operator • if (f 1(), f()) … • The ? in the ternary operator (? : ) • Statements • Complete conditions in if, while, for, and switch • End of an initializer: int a = x++, b = ++x; // done in order
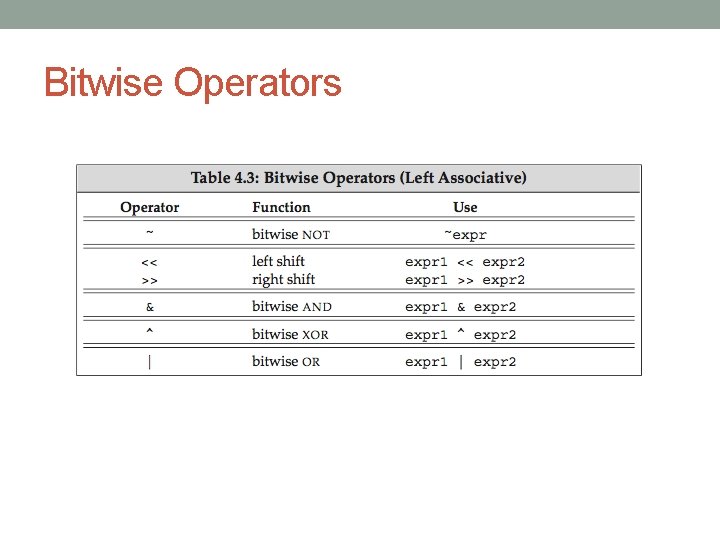
Bitwise Operators
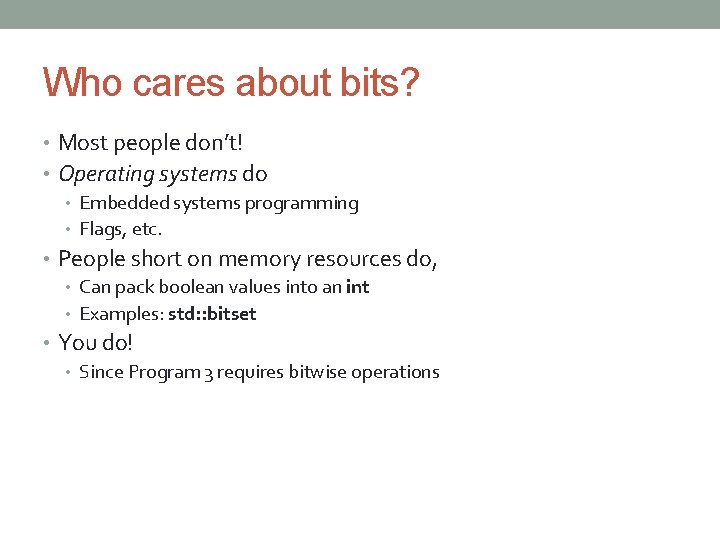
Who cares about bits? • Most people don’t! • Operating systems do • Embedded systems programming • Flags, etc. • People short on memory resources do, • Can pack boolean values into an int • Examples: std: : bitset • You do! • Since Program 3 requires bitwise operations
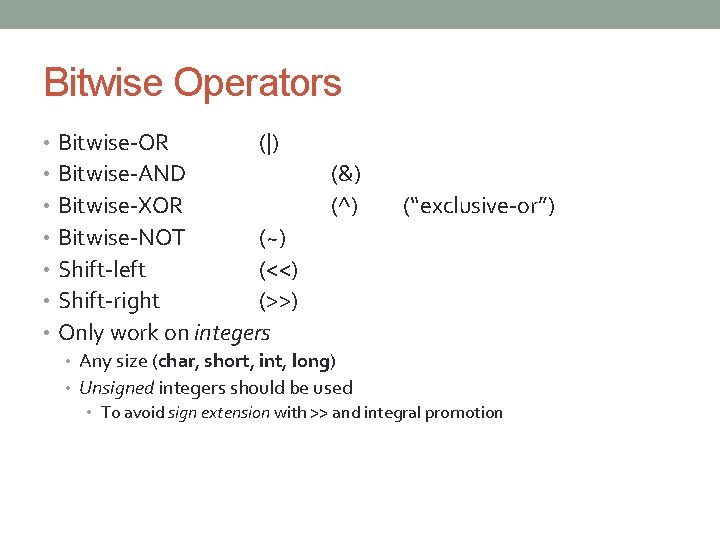
Bitwise Operators • Bitwise-OR (|) • Bitwise-AND • Bitwise-XOR (&) (^) (“exclusive-or”) • Bitwise-NOT (~) • Shift-left (<<) • Shift-right (>>) • Only work on integers • Any size (char, short, int, long) • Unsigned integers should be used • To avoid sign extension with >> and integral promotion
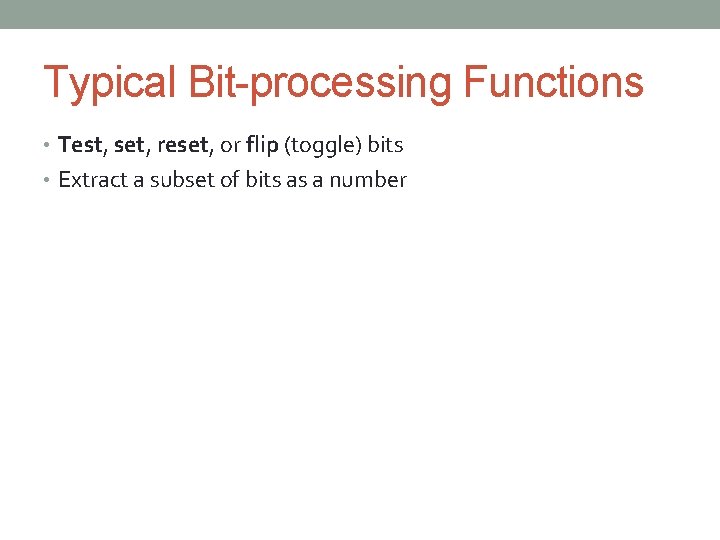
Typical Bit-processing Functions • Test, set, reset, or flip (toggle) bits • Extract a subset of bits as a number
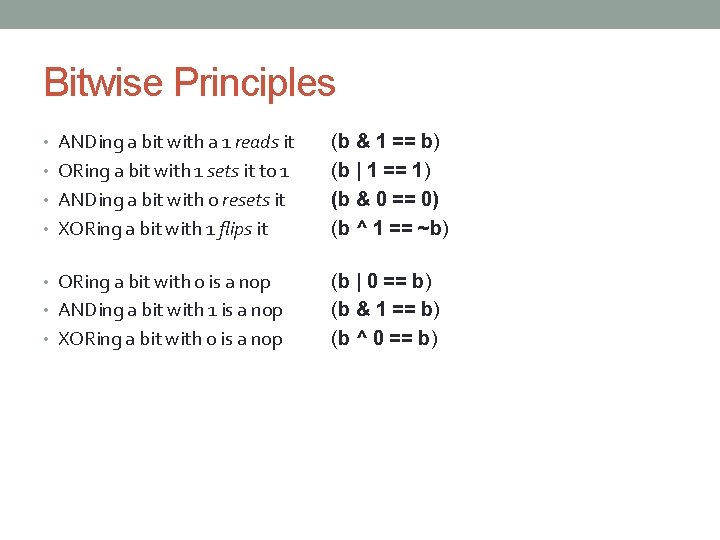
Bitwise Principles • ANDing a bit with a 1 reads it • ORing a bit with 1 sets it to 1 • ANDing a bit with 0 resets it • XORing a bit with 1 flips it • ORing a bit with 0 is a nop • ANDing a bit with 1 is a nop • XORing a bit with 0 is a nop (b & 1 == b) (b | 1 == 1) (b & 0 == 0) (b ^ 1 == ~b) (b | 0 == b) (b & 1 == b) (b ^ 0 == b)
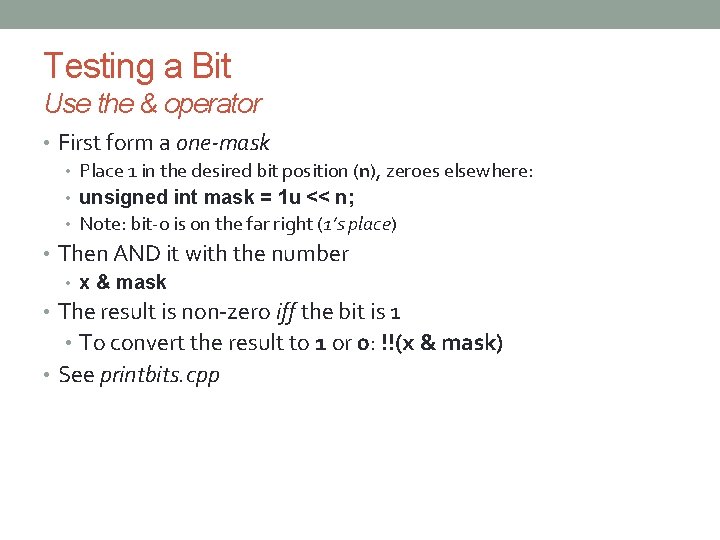
Testing a Bit Use the & operator • First form a one-mask • Place 1 in the desired bit position (n), zeroes elsewhere: • unsigned int mask = 1 u << n; • Note: bit-0 is on the far right (1’s place) • Then AND it with the number • x & mask • The result is non-zero iff the bit is 1 • To convert the result to 1 or 0: !!(x & mask) • See printbits. cpp
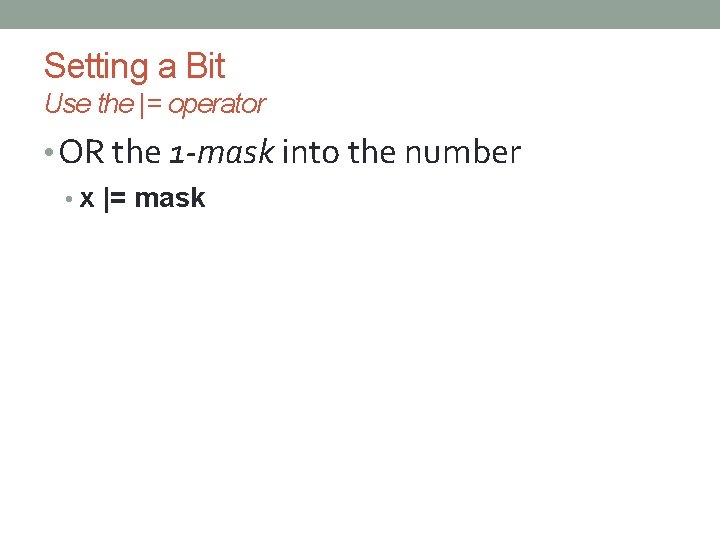
Setting a Bit Use the |= operator • OR the 1 -mask into the number • x |= mask
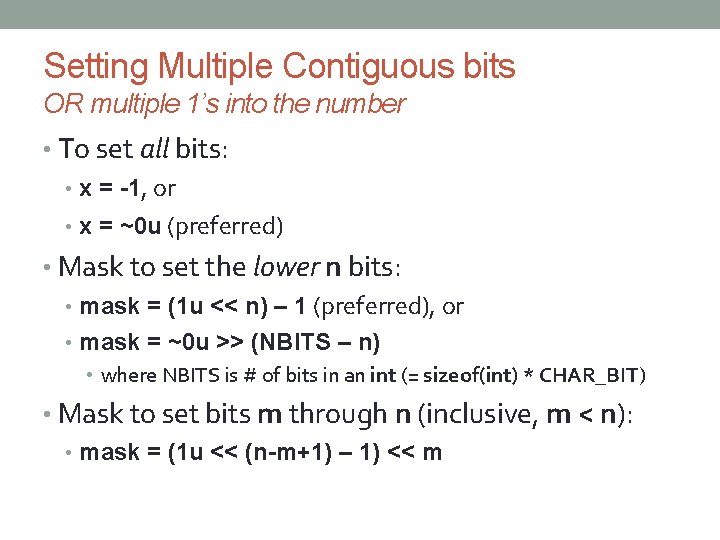
Setting Multiple Contiguous bits OR multiple 1’s into the number • To set all bits: • x = -1, or • x = ~0 u (preferred) • Mask to set the lower n bits: • mask = (1 u << n) – 1 (preferred), or • mask = ~0 u >> (NBITS – n) • where NBITS is # of bits in an int (= sizeof(int) * CHAR_BIT) • Mask to set bits m through n (inclusive, m < n): • mask = (1 u << (n-m+1) – 1) << m
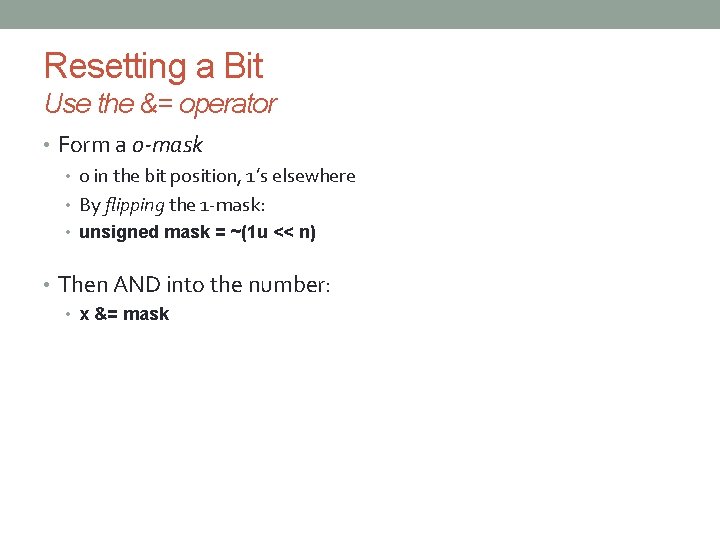
Resetting a Bit Use the &= operator • Form a 0 -mask • 0 in the bit position, 1’s elsewhere • By flipping the 1 -mask: • unsigned mask = ~(1 u << n) • Then AND into the number: • x &= mask
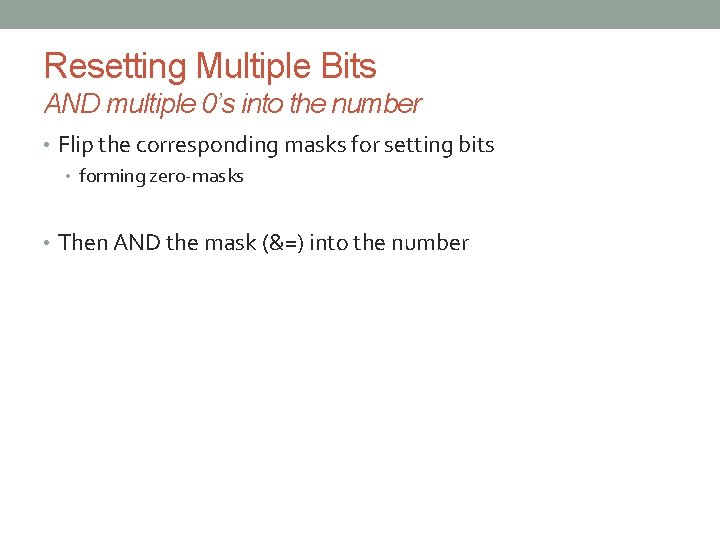
Resetting Multiple Bits AND multiple 0’s into the number • Flip the corresponding masks for setting bits • forming zero-masks • Then AND the mask (&=) into the number
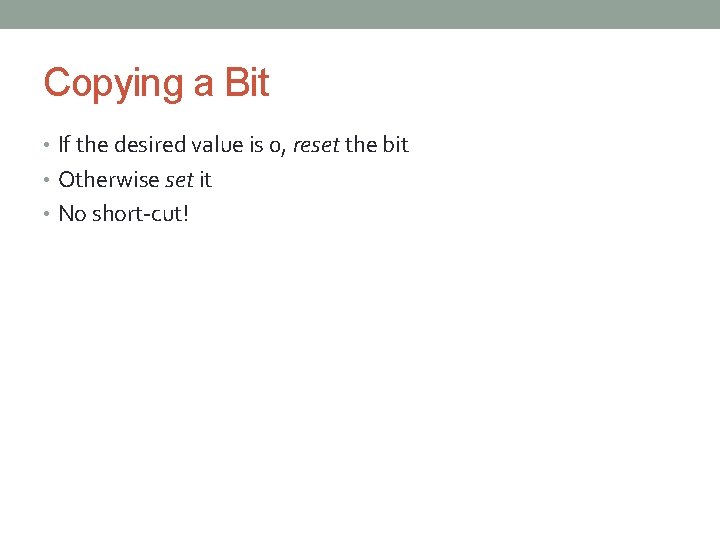
Copying a Bit • If the desired value is 0, reset the bit • Otherwise set it • No short-cut!
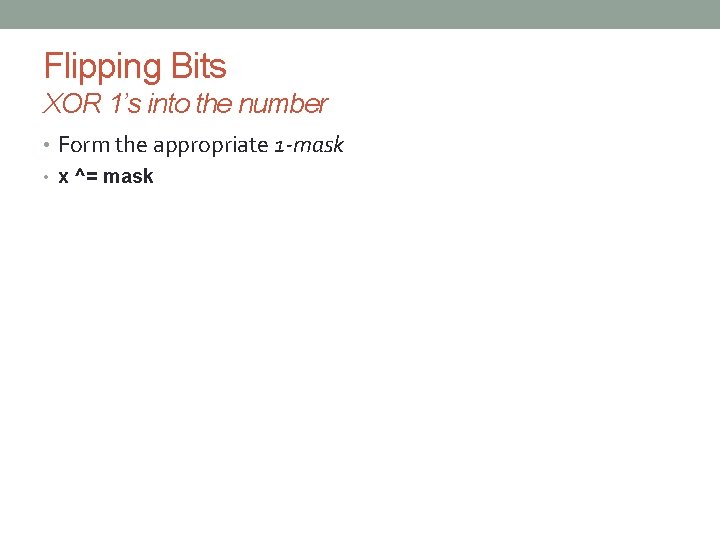
Flipping Bits XOR 1’s into the number • Form the appropriate 1 -mask • x ^= mask
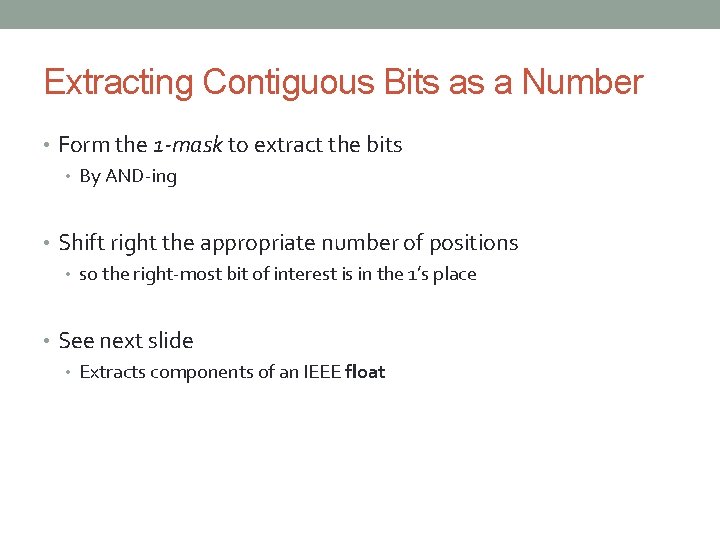
Extracting Contiguous Bits as a Number • Form the 1 -mask to extract the bits • By AND-ing • Shift right the appropriate number of positions • so the right-most bit of interest is in the 1’s place • See next slide • Extracts components of an IEEE float
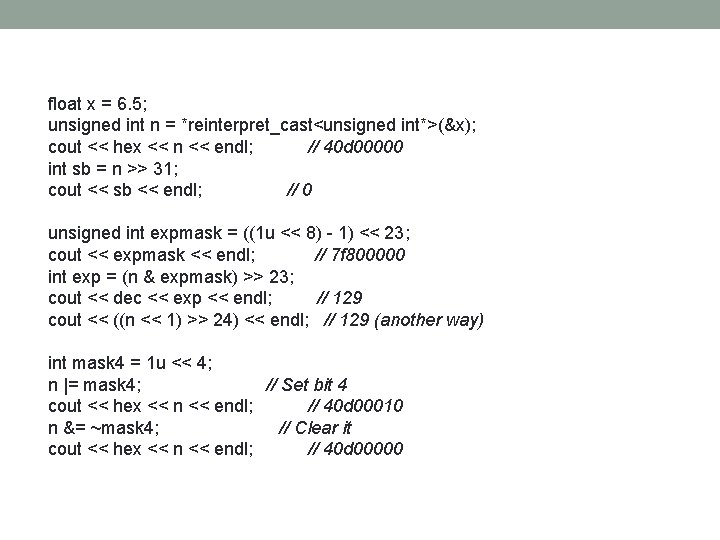
float x = 6. 5; unsigned int n = *reinterpret_cast<unsigned int*>(&x); cout << hex << n << endl; // 40 d 00000 int sb = n >> 31; cout << sb << endl; // 0 unsigned int expmask = ((1 u << 8) - 1) << 23; cout << expmask << endl; // 7 f 800000 int exp = (n & expmask) >> 23; cout << dec << exp << endl; // 129 cout << ((n << 1) >> 24) << endl; // 129 (another way) int mask 4 = 1 u << 4; n |= mask 4; // Set bit 4 cout << hex << n << endl; // 40 d 00010 n &= ~mask 4; // Clear it cout << hex << n << endl; // 40 d 00000