Function and Operator Overloading Overloading Review of function
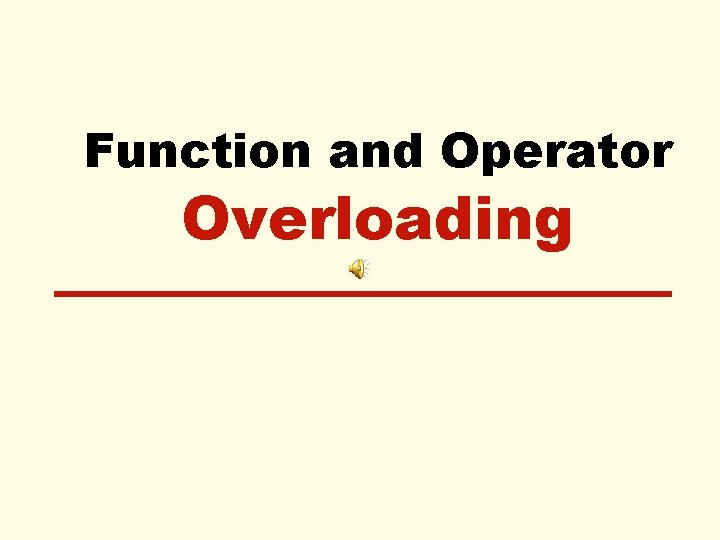
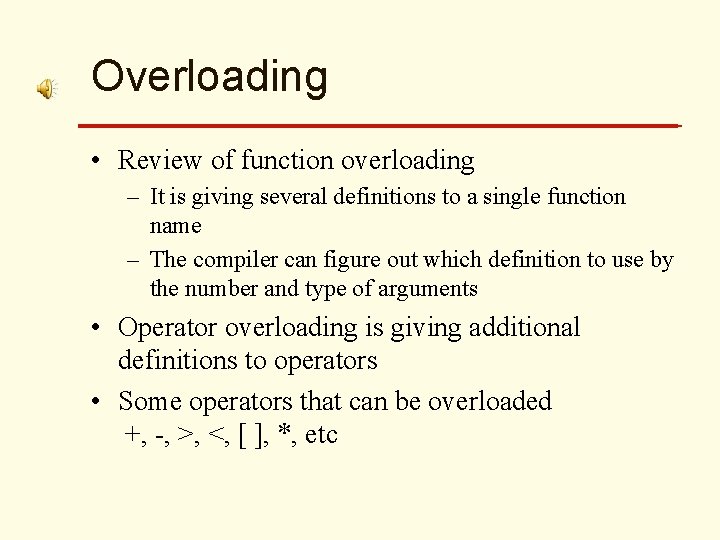
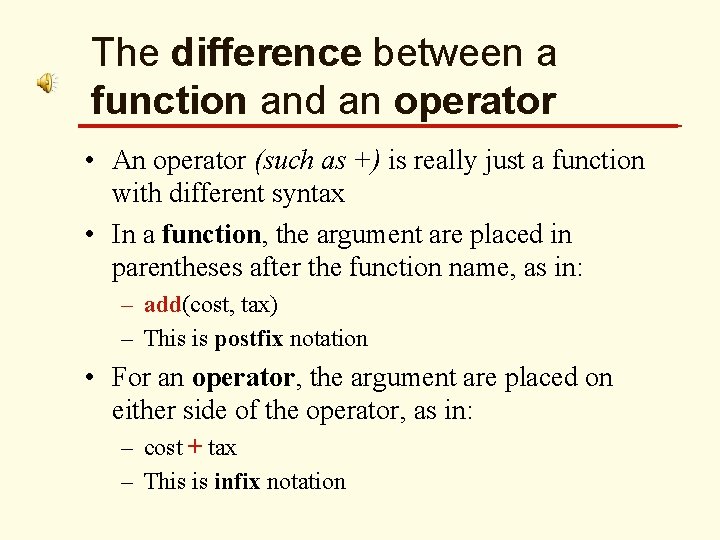
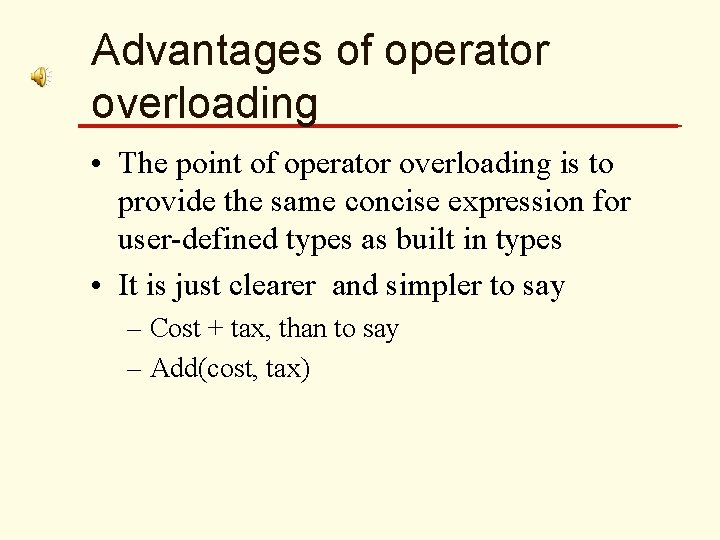
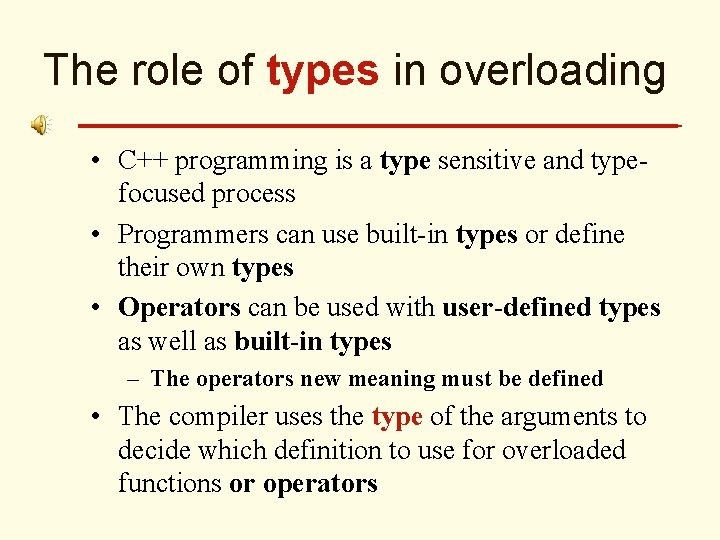
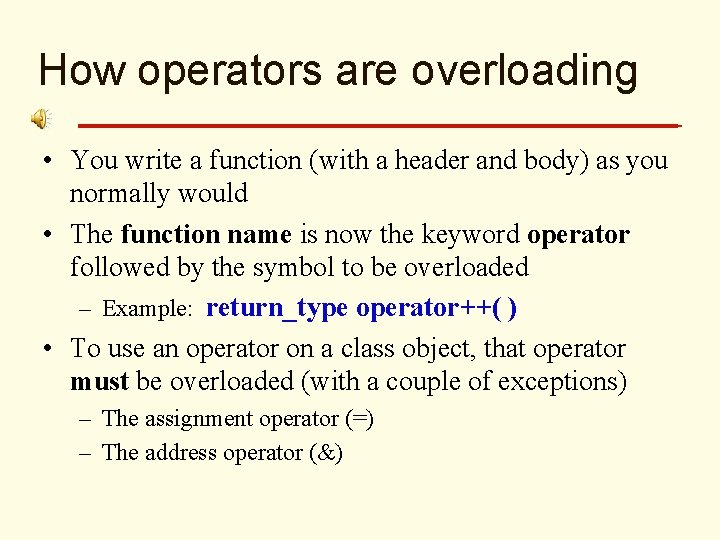
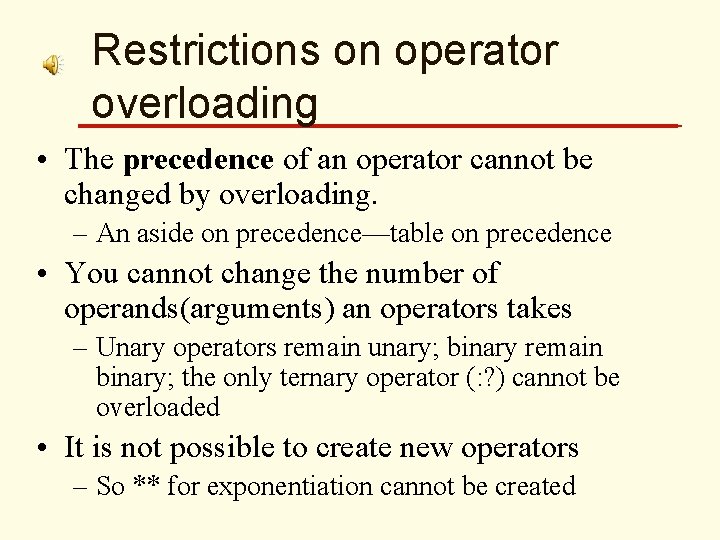
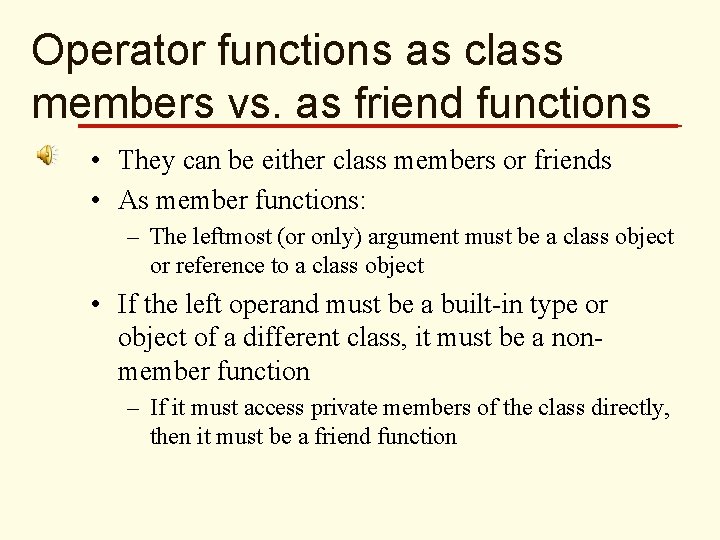
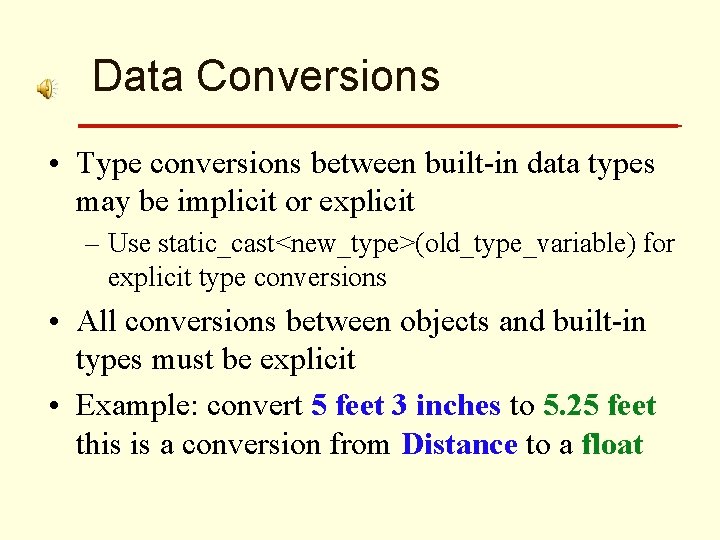
- Slides: 9
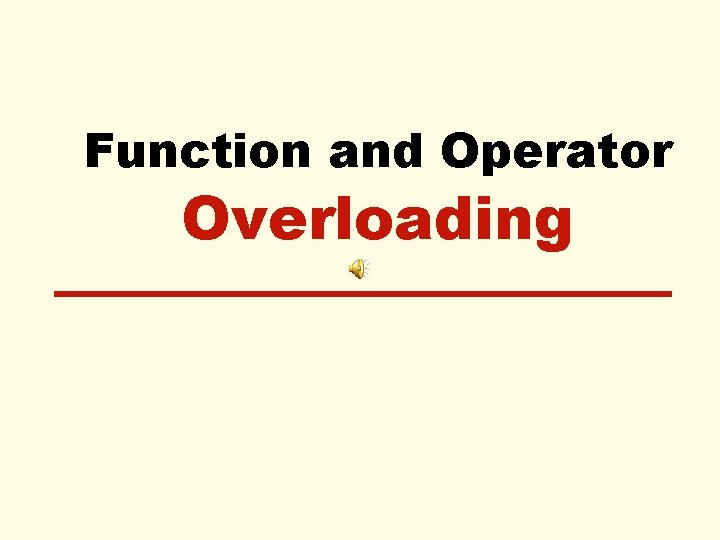
Function and Operator Overloading
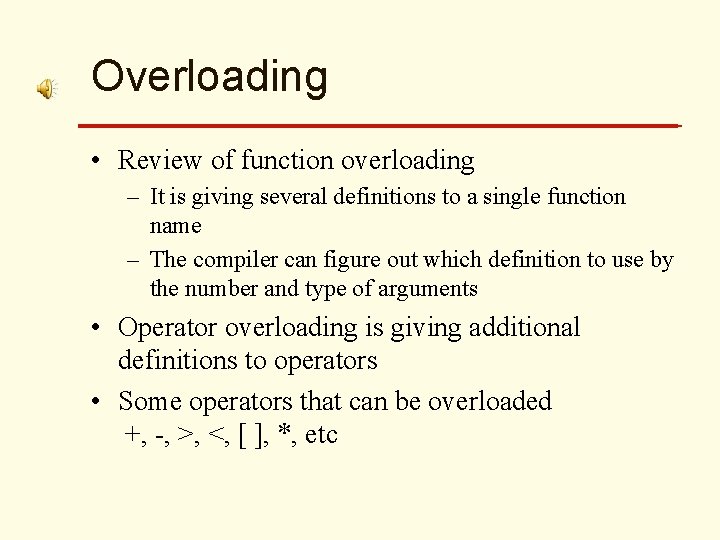
Overloading • Review of function overloading – It is giving several definitions to a single function name – The compiler can figure out which definition to use by the number and type of arguments • Operator overloading is giving additional definitions to operators • Some operators that can be overloaded +, -, >, <, [ ], *, etc
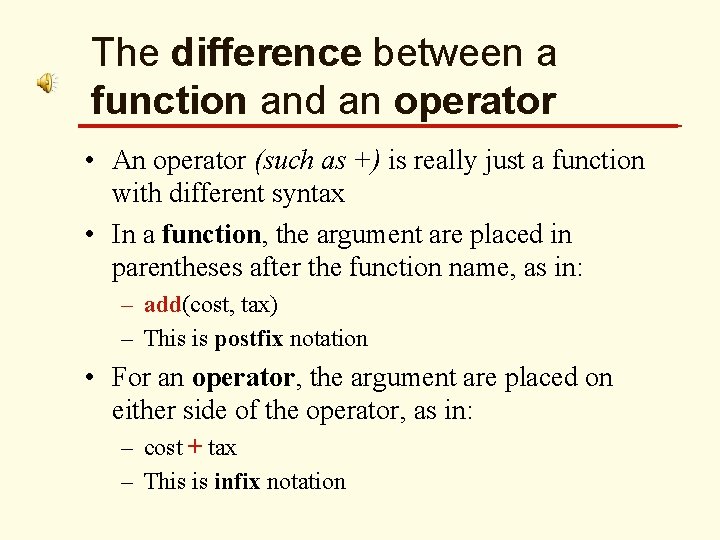
The difference between a function and an operator • An operator (such as +) is really just a function with different syntax • In a function, the argument are placed in parentheses after the function name, as in: – add(cost, tax) – This is postfix notation • For an operator, the argument are placed on either side of the operator, as in: – cost + tax – This is infix notation
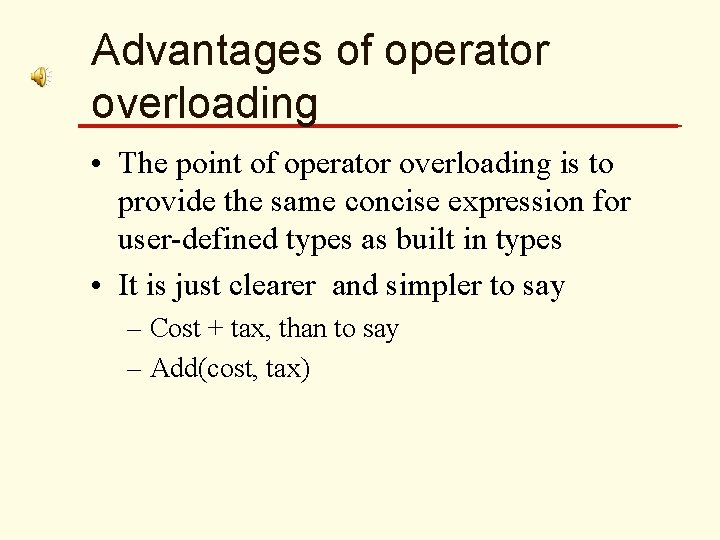
Advantages of operator overloading • The point of operator overloading is to provide the same concise expression for user-defined types as built in types • It is just clearer and simpler to say – Cost + tax, than to say – Add(cost, tax)
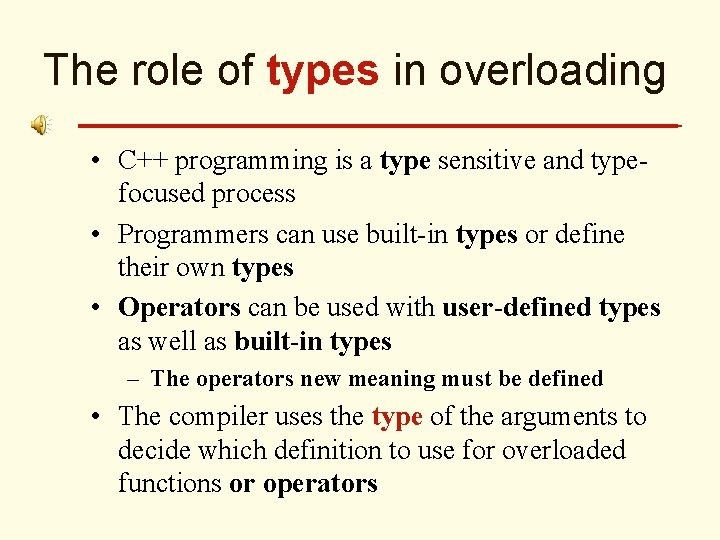
The role of types in overloading • C++ programming is a type sensitive and typefocused process • Programmers can use built-in types or define their own types • Operators can be used with user-defined types as well as built-in types – The operators new meaning must be defined • The compiler uses the type of the arguments to decide which definition to use for overloaded functions or operators
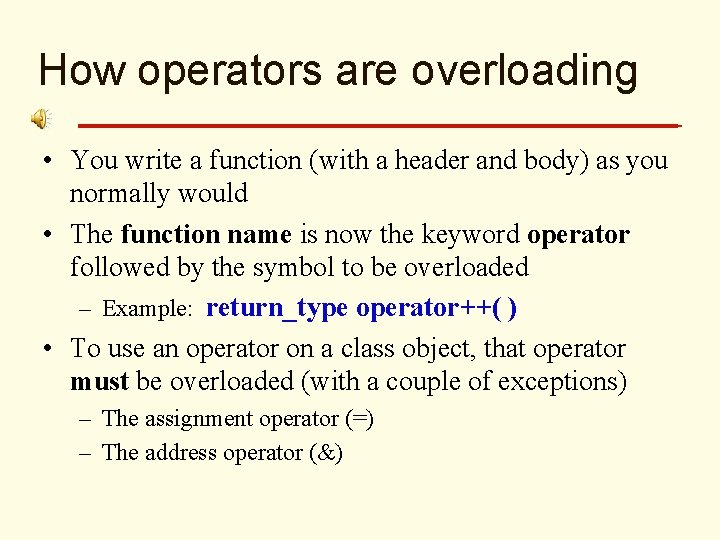
How operators are overloading • You write a function (with a header and body) as you normally would • The function name is now the keyword operator followed by the symbol to be overloaded – Example: return_type operator++( ) • To use an operator on a class object, that operator must be overloaded (with a couple of exceptions) – The assignment operator (=) – The address operator (&)
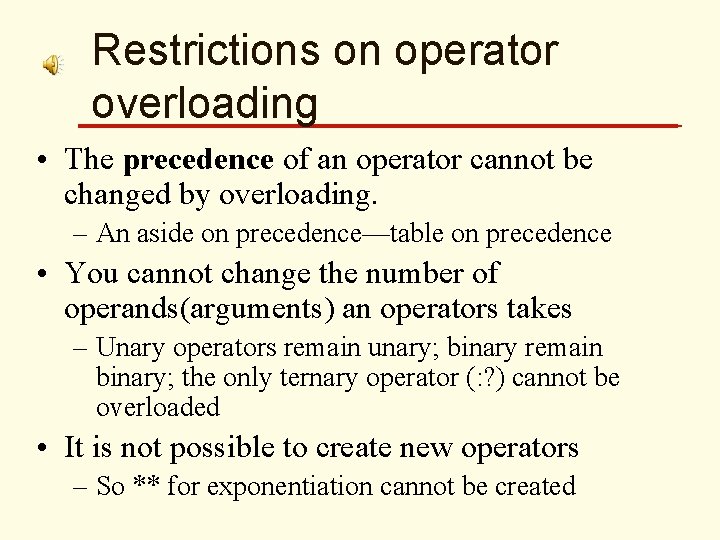
Restrictions on operator overloading • The precedence of an operator cannot be changed by overloading. – An aside on precedence—table on precedence • You cannot change the number of operands(arguments) an operators takes – Unary operators remain unary; binary remain binary; the only ternary operator (: ? ) cannot be overloaded • It is not possible to create new operators – So ** for exponentiation cannot be created
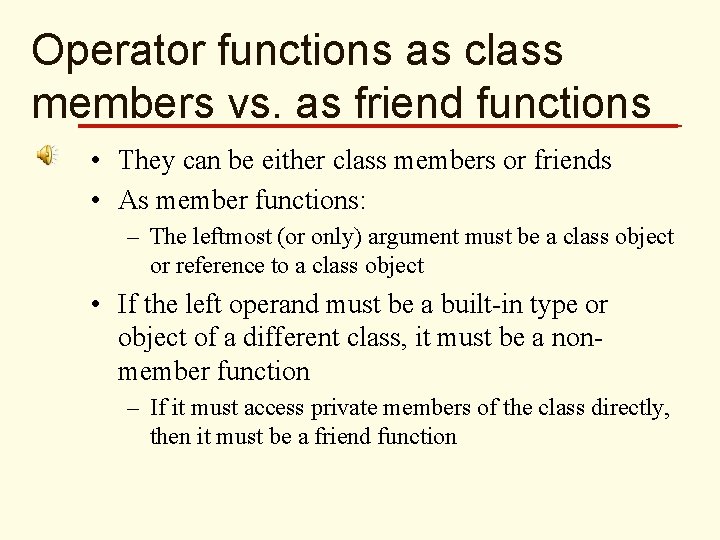
Operator functions as class members vs. as friend functions • They can be either class members or friends • As member functions: – The leftmost (or only) argument must be a class object or reference to a class object • If the left operand must be a built-in type or object of a different class, it must be a nonmember function – If it must access private members of the class directly, then it must be a friend function
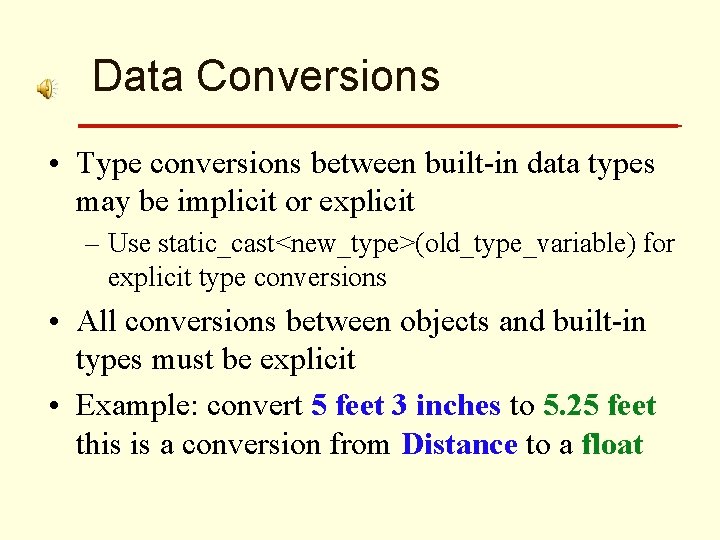
Data Conversions • Type conversions between built-in data types may be implicit or explicit – Use static_cast<new_type>(old_type_variable) for explicit type conversions • All conversions between objects and built-in types must be explicit • Example: convert 5 feet 3 inches to 5. 25 feet this is a conversion from Distance to a float