Compound Assignment Operators in C Simple assignment operator
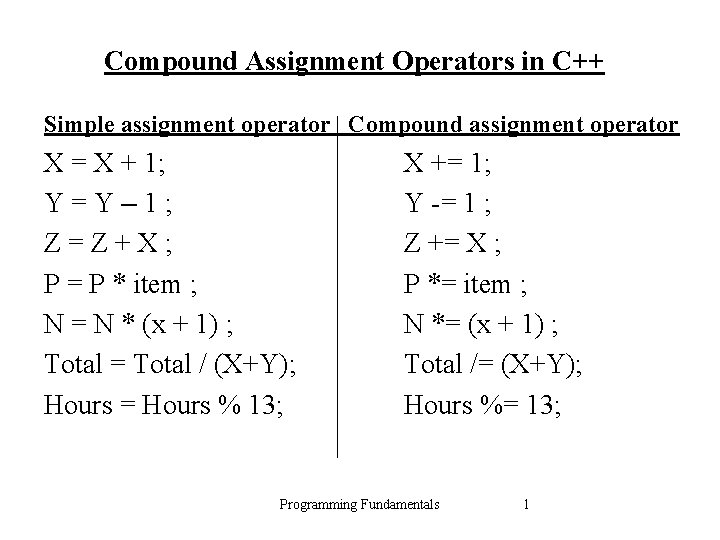
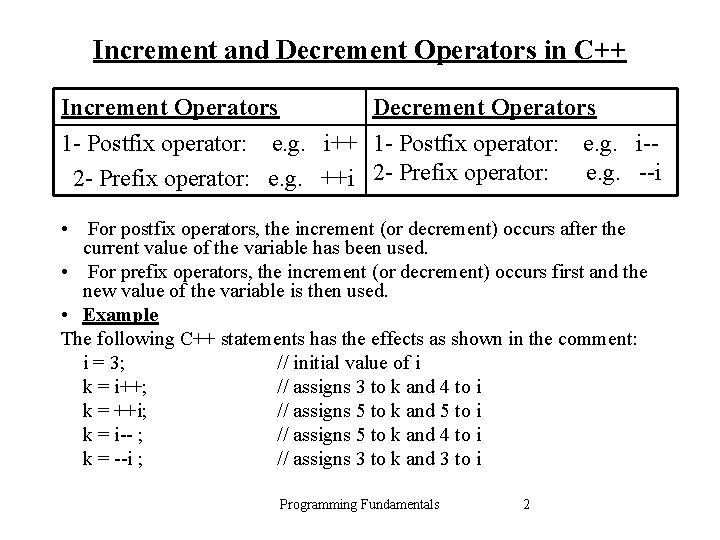
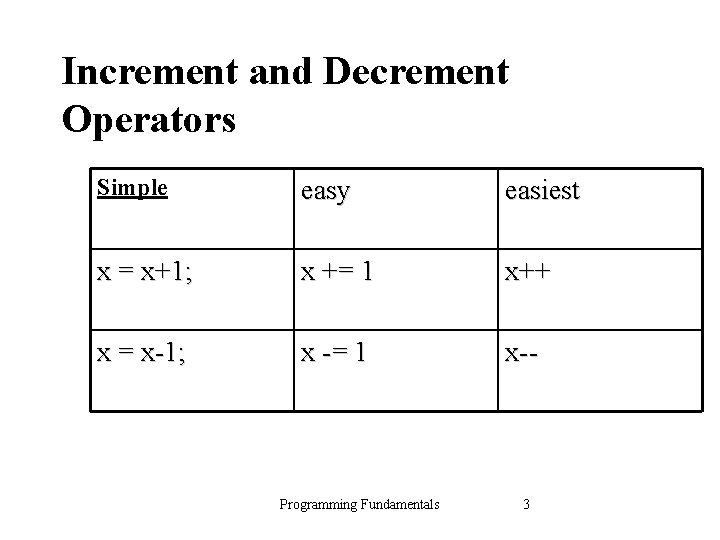
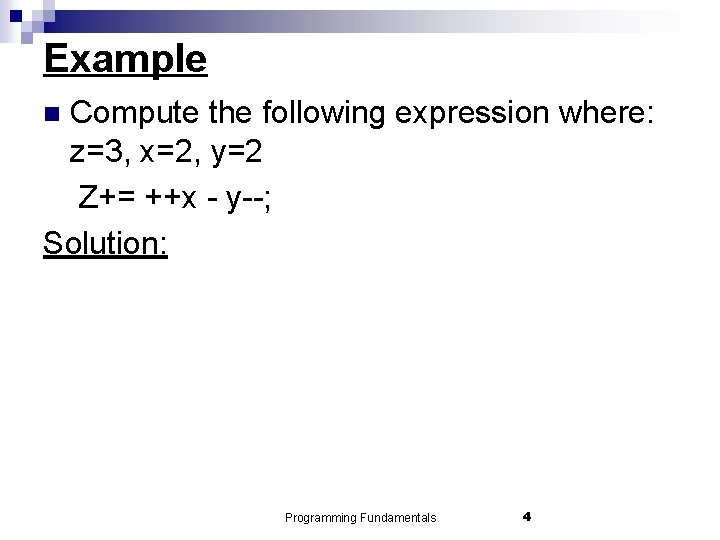
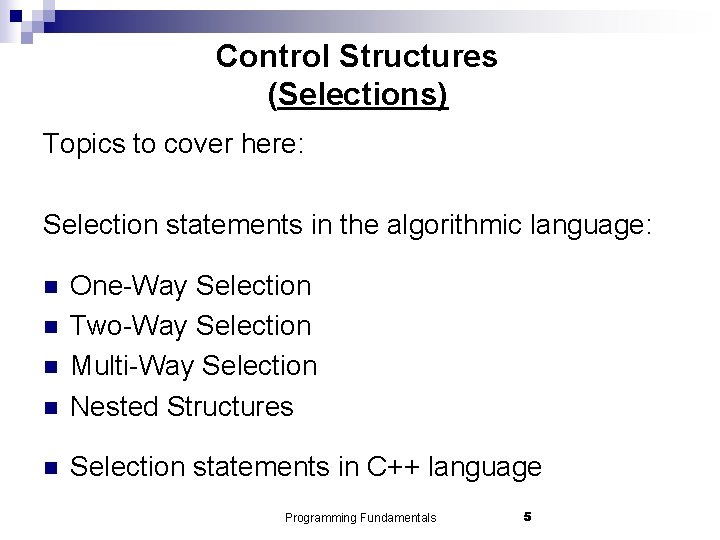
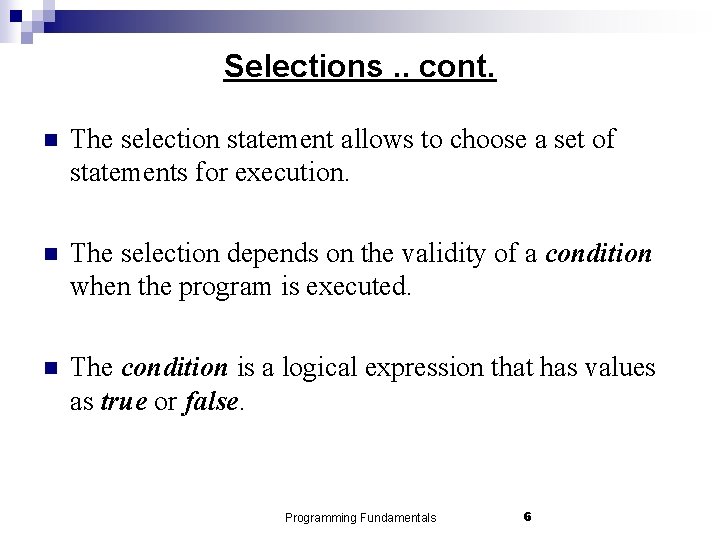
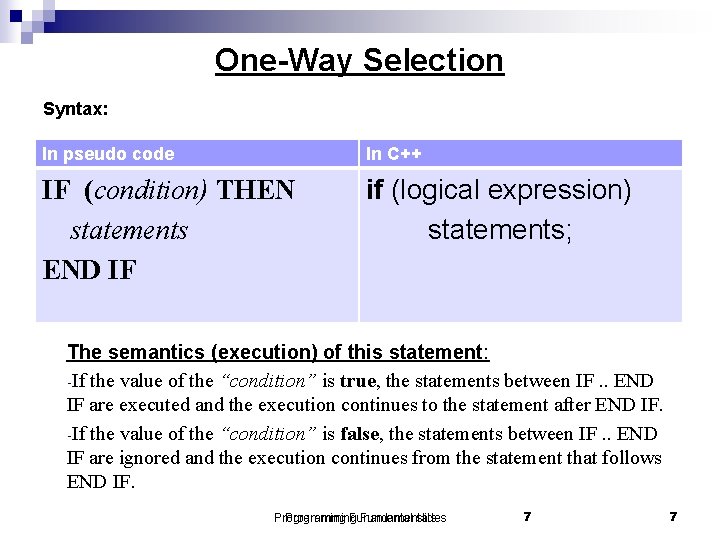
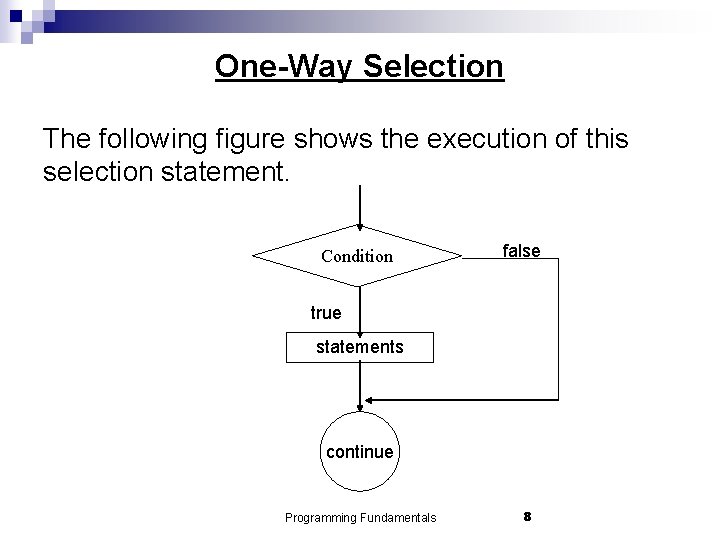
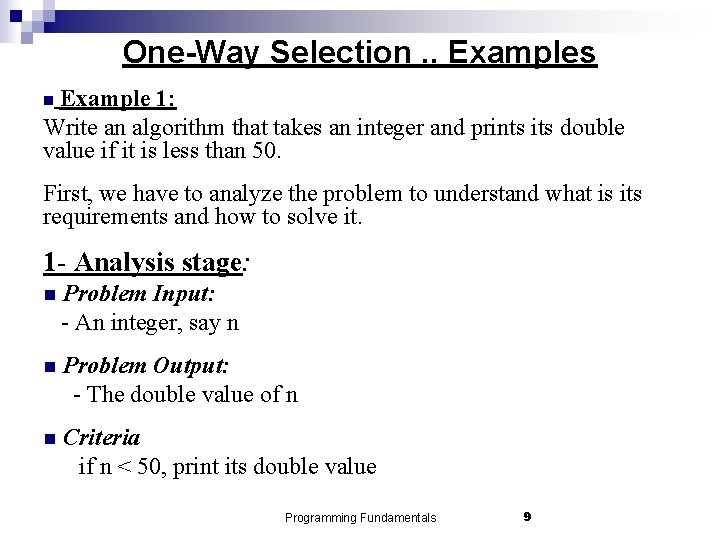
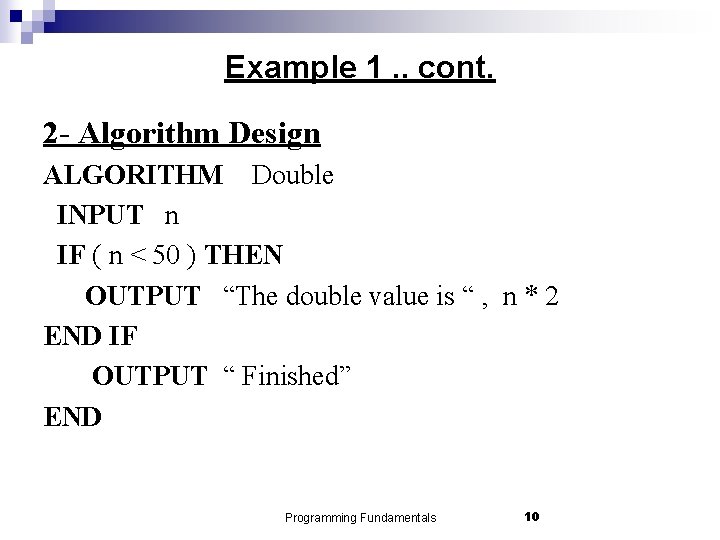
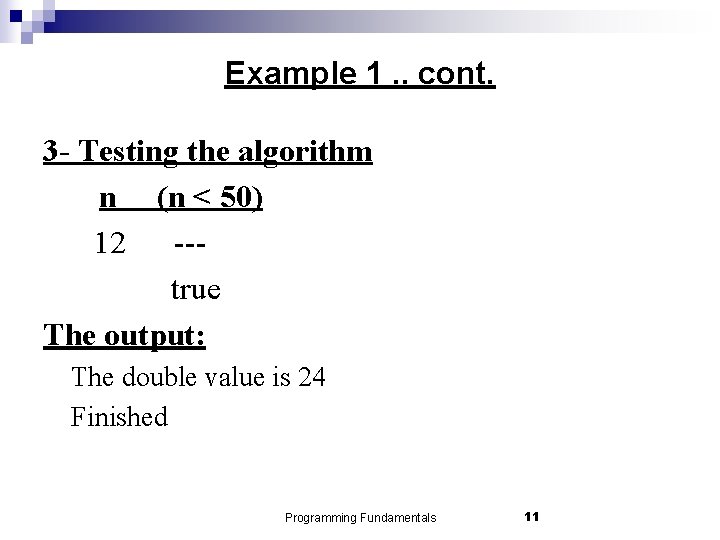
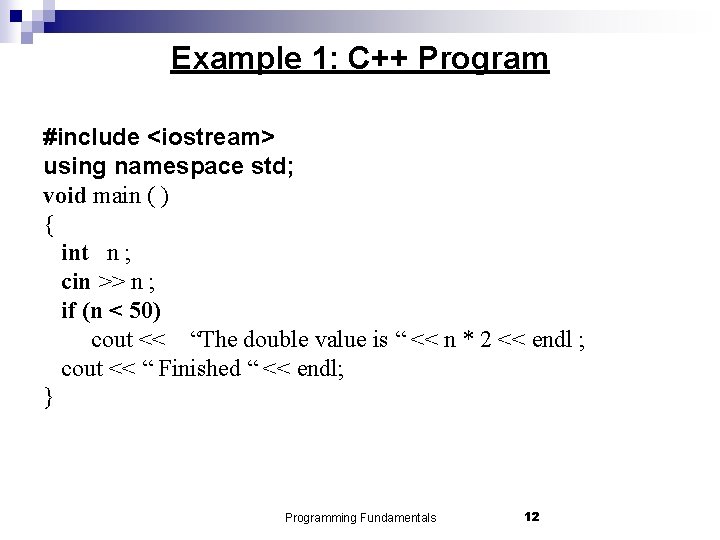
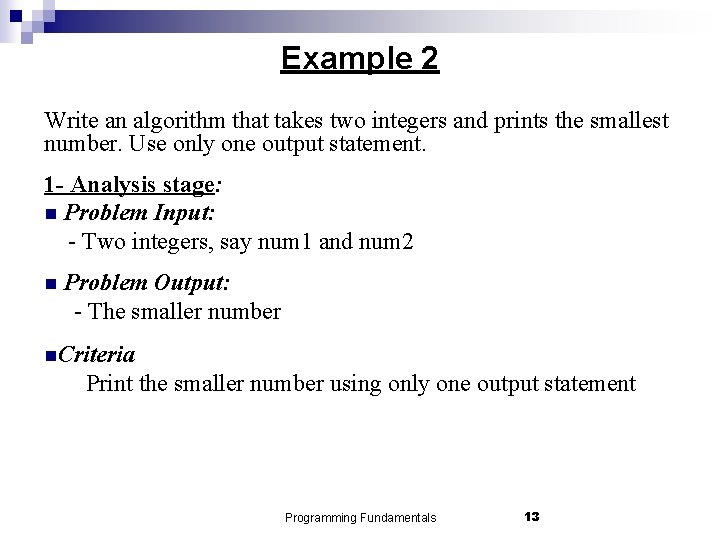
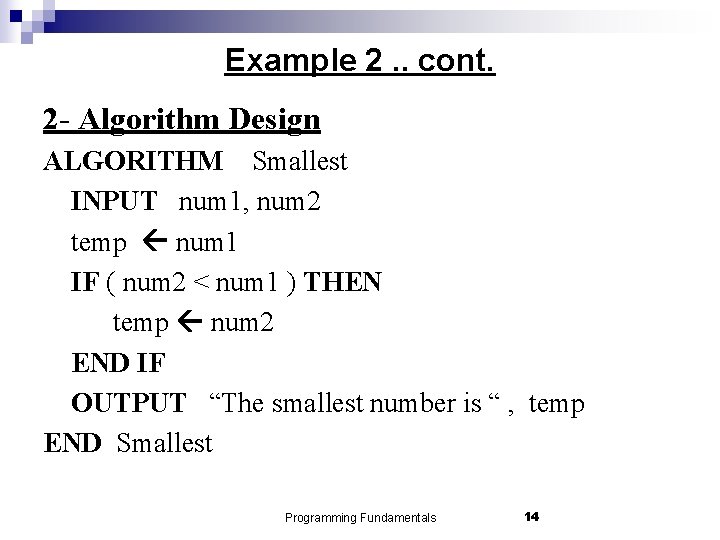
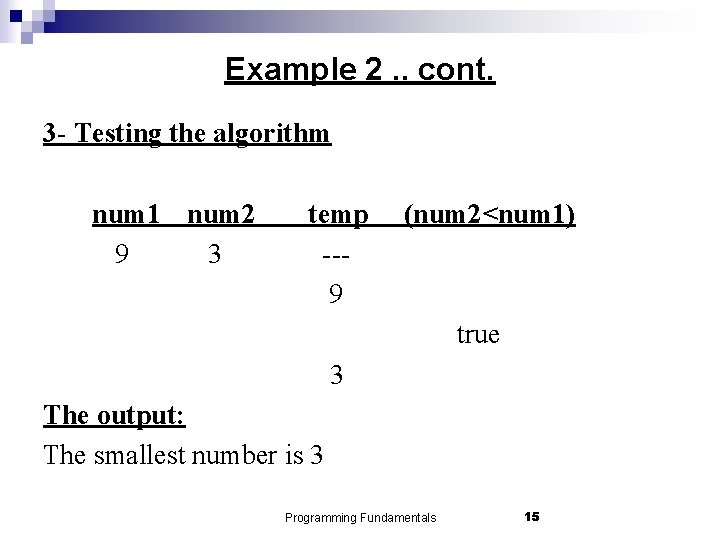
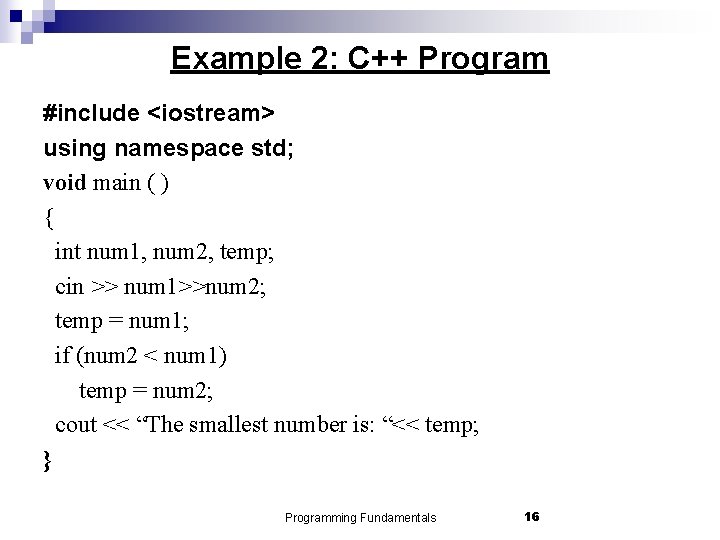
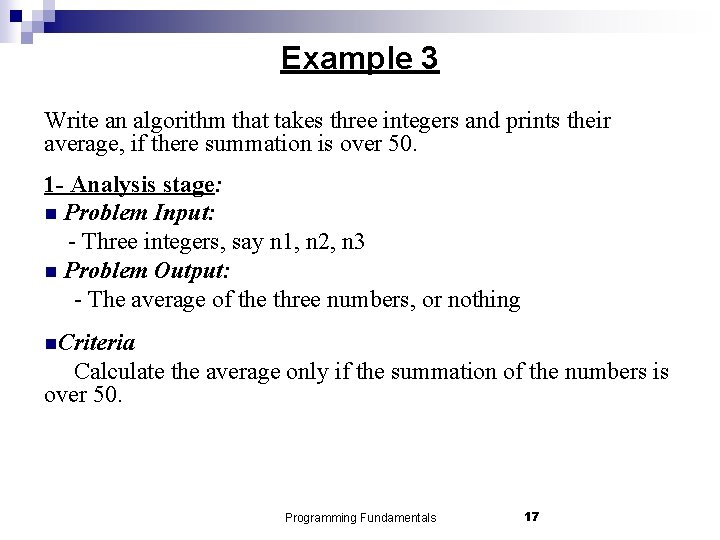
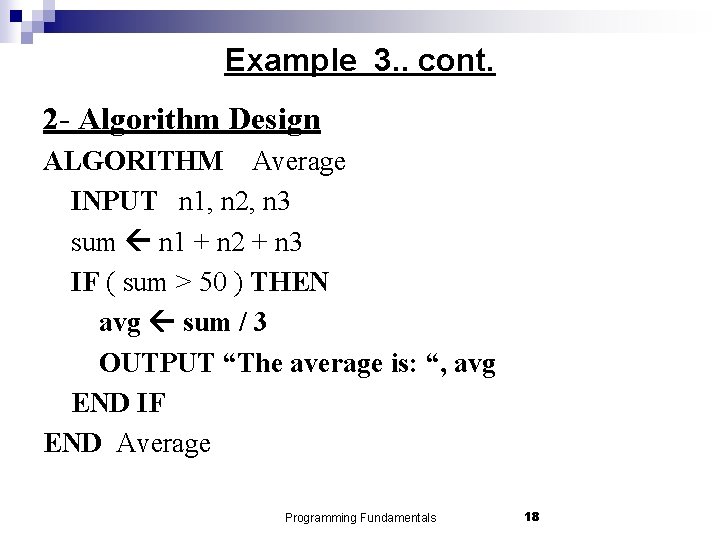
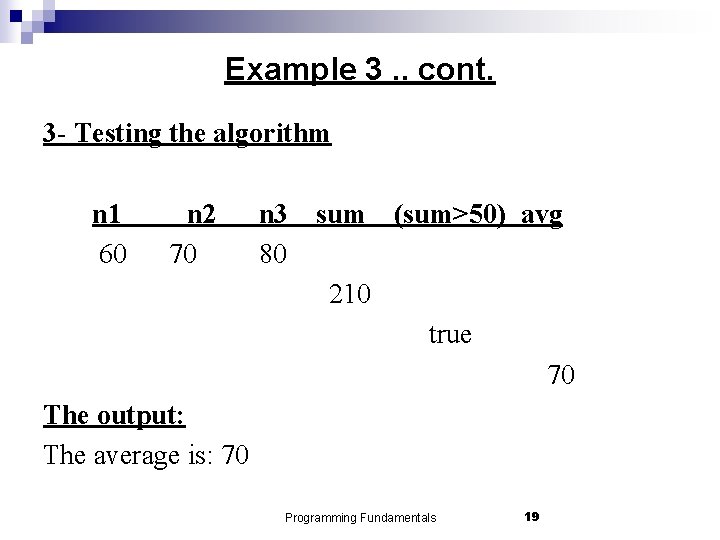
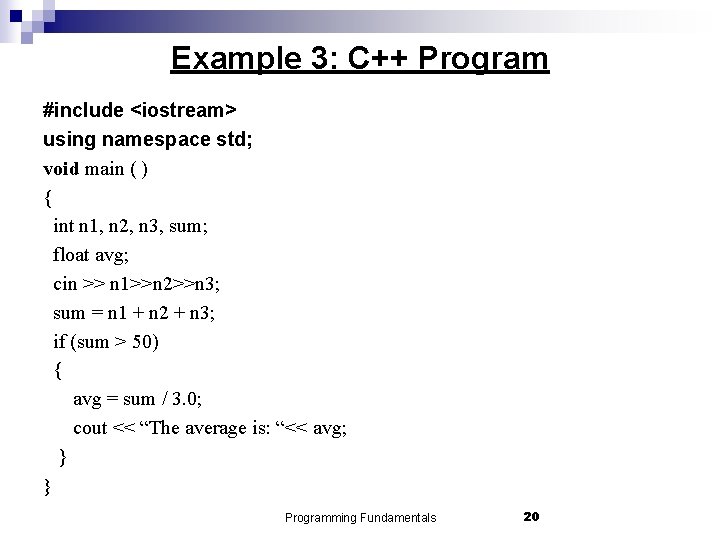
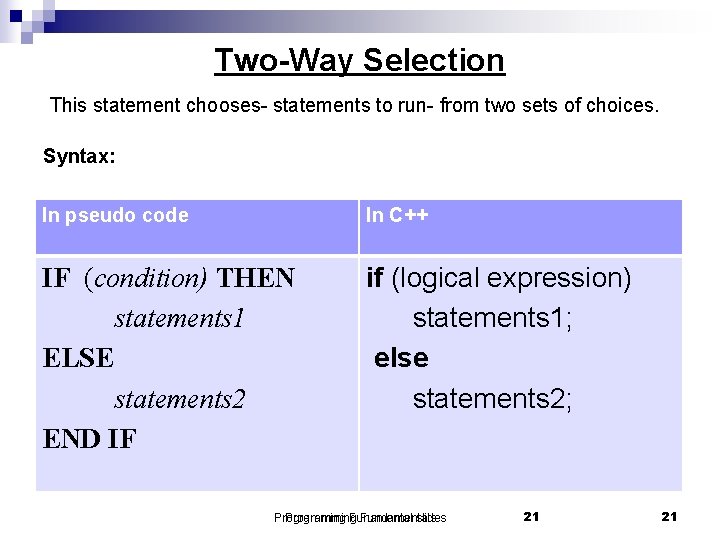
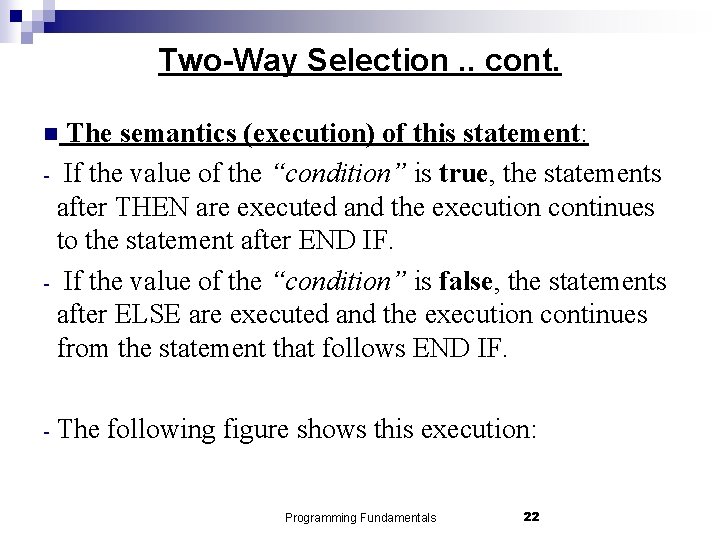
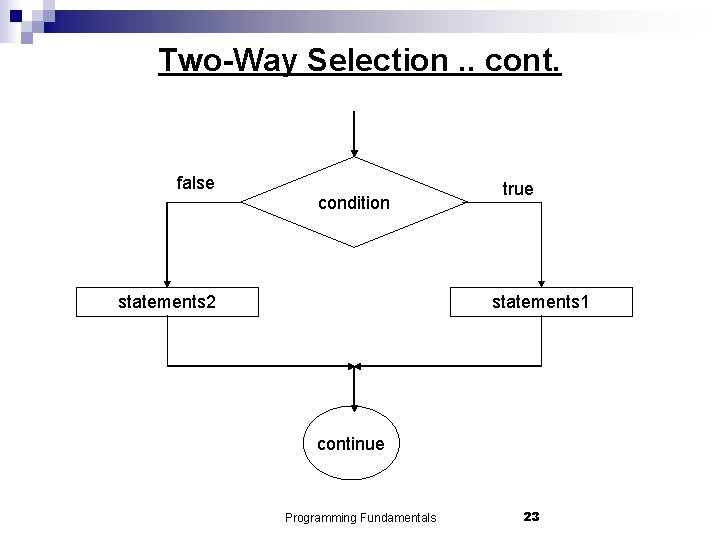
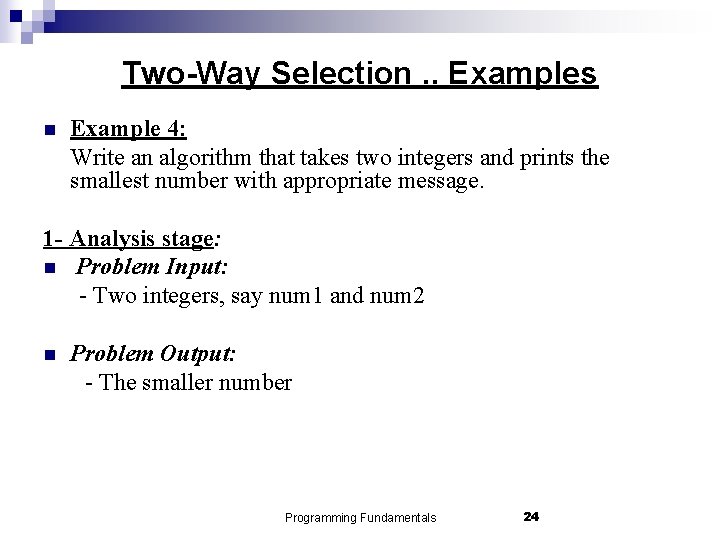
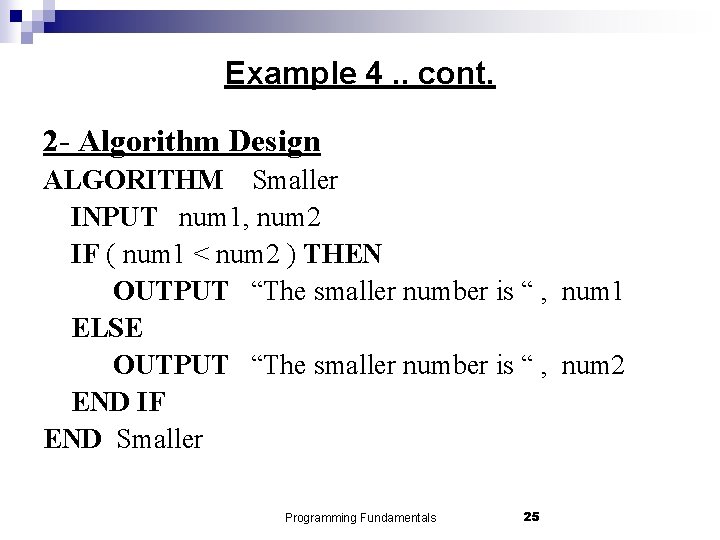
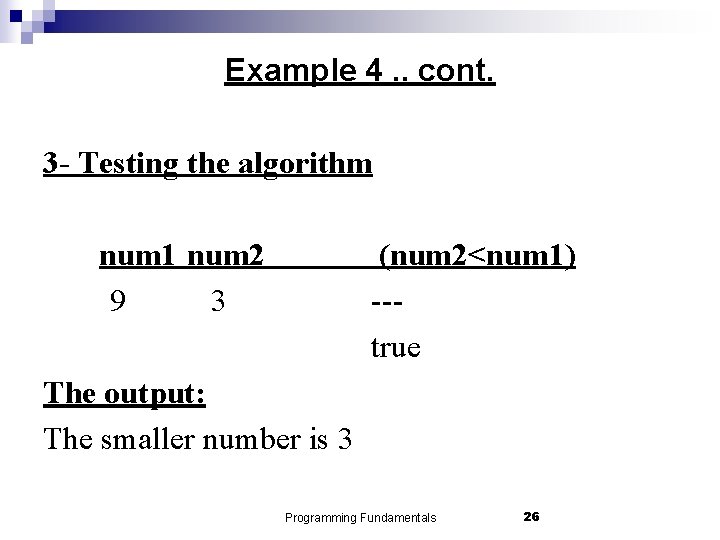
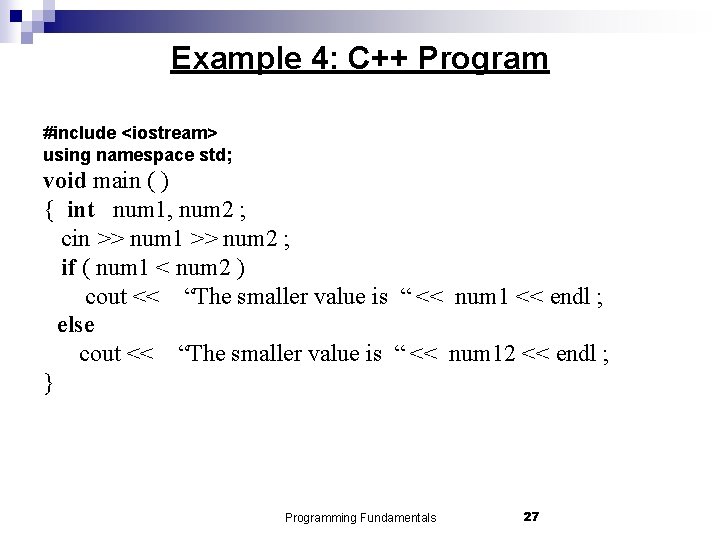
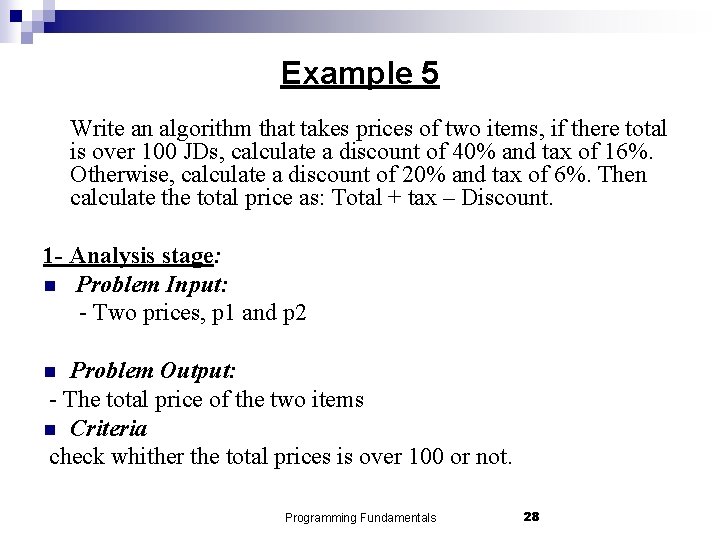
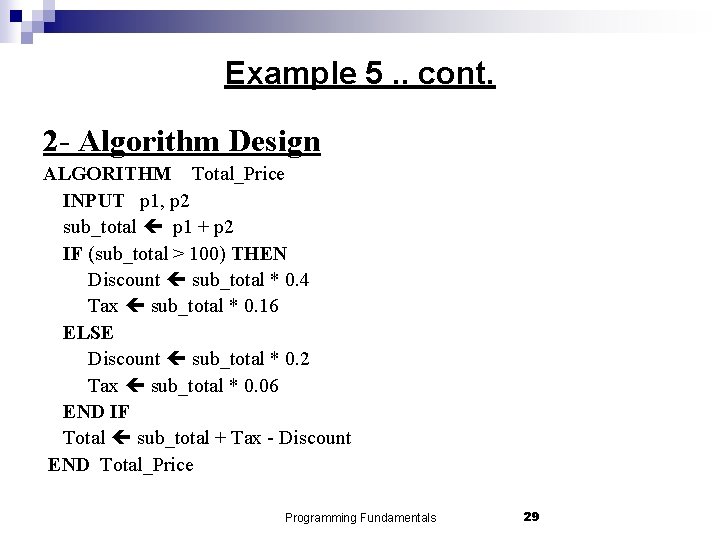
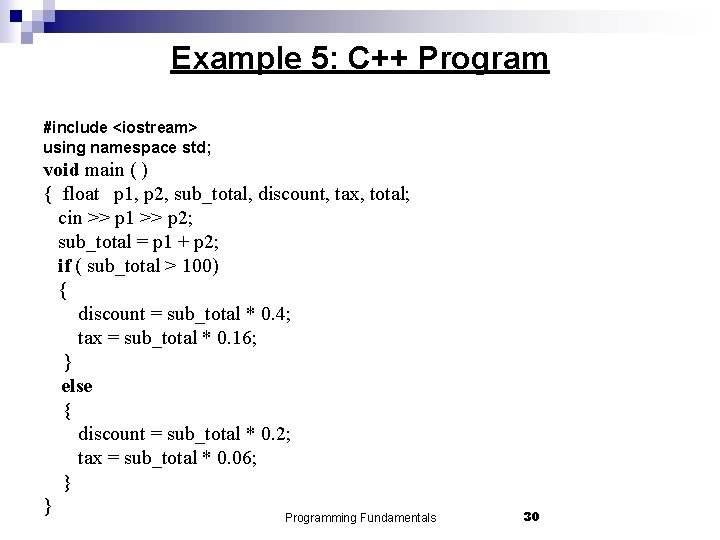
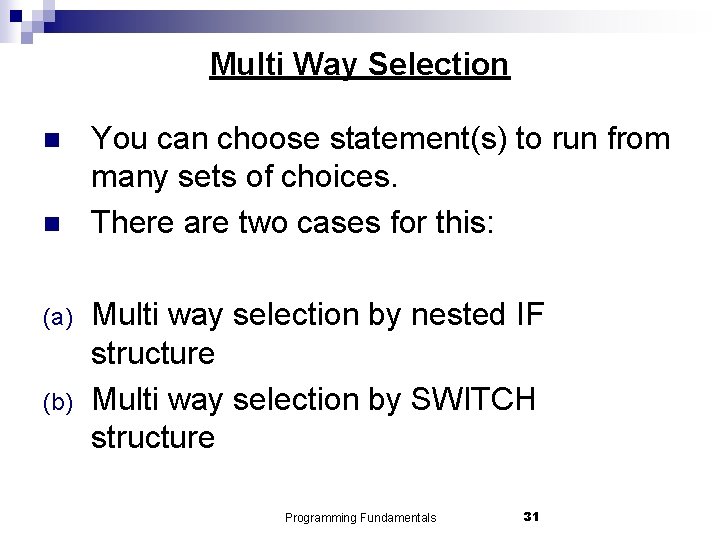
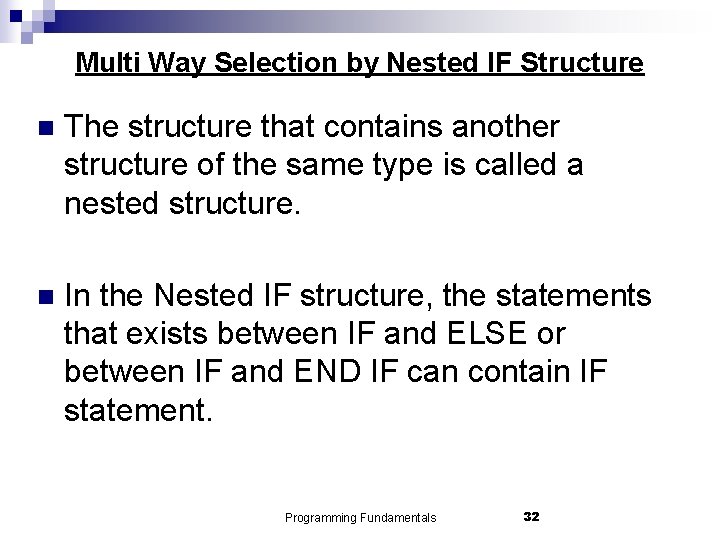
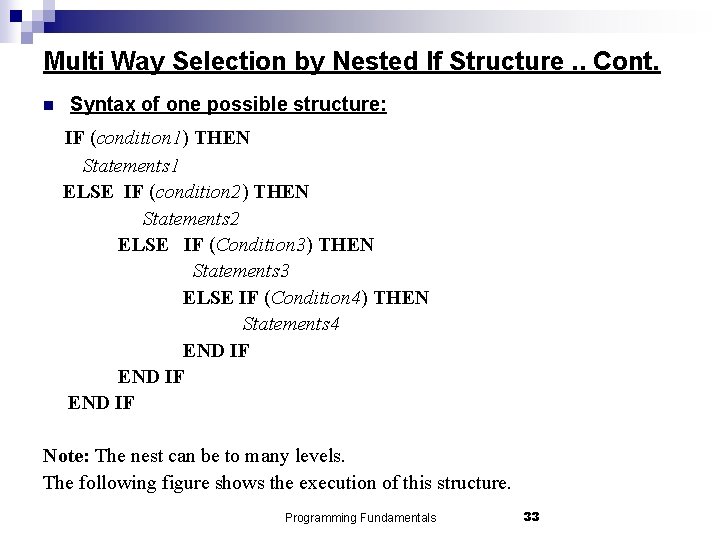
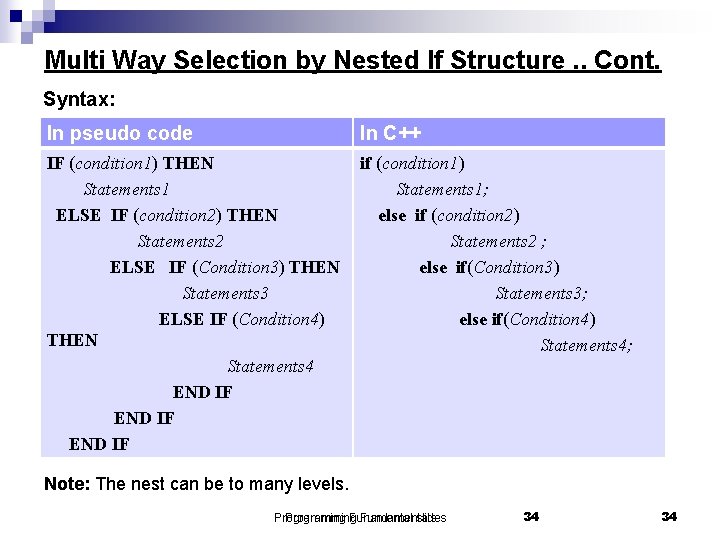
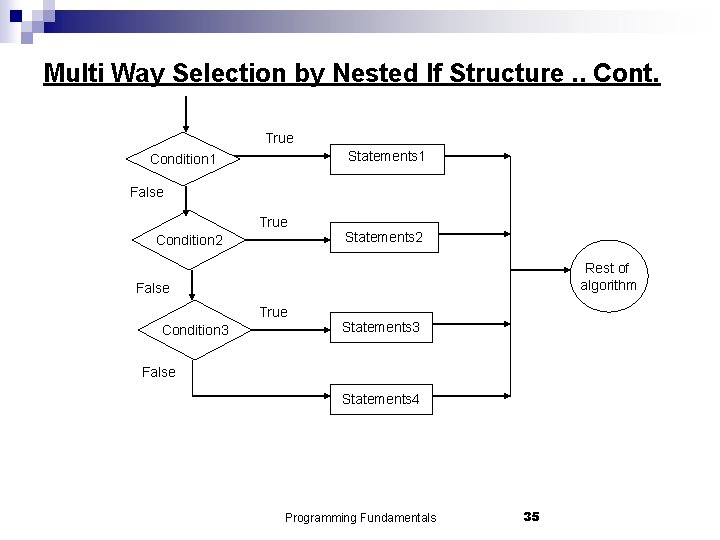
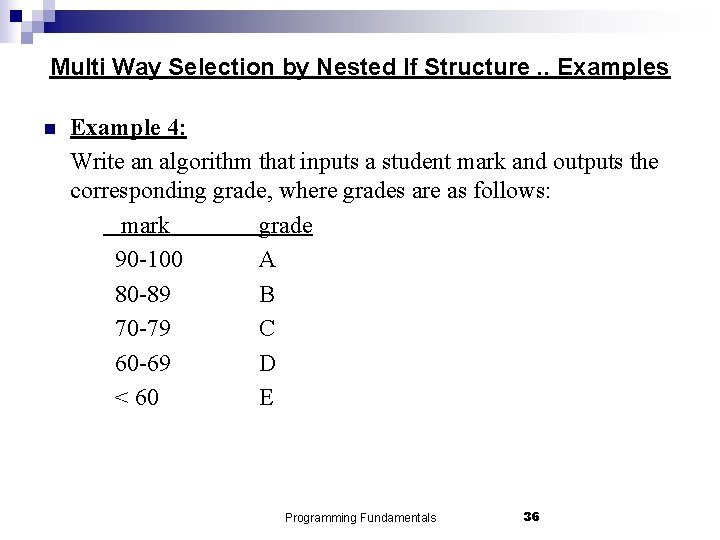
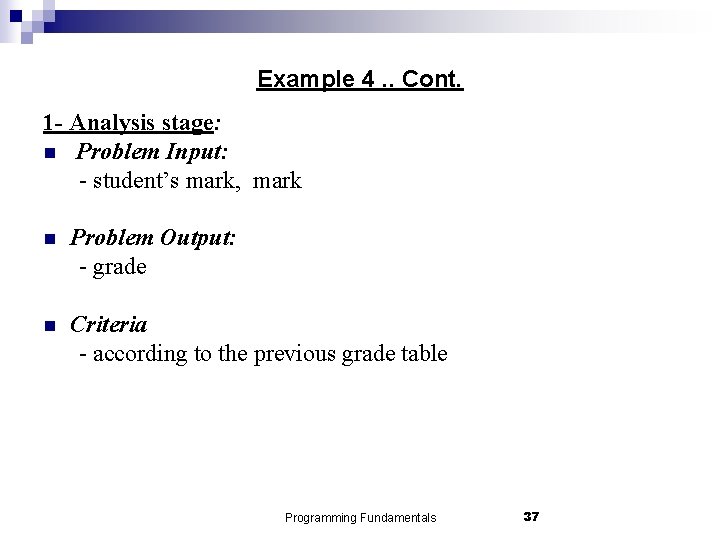
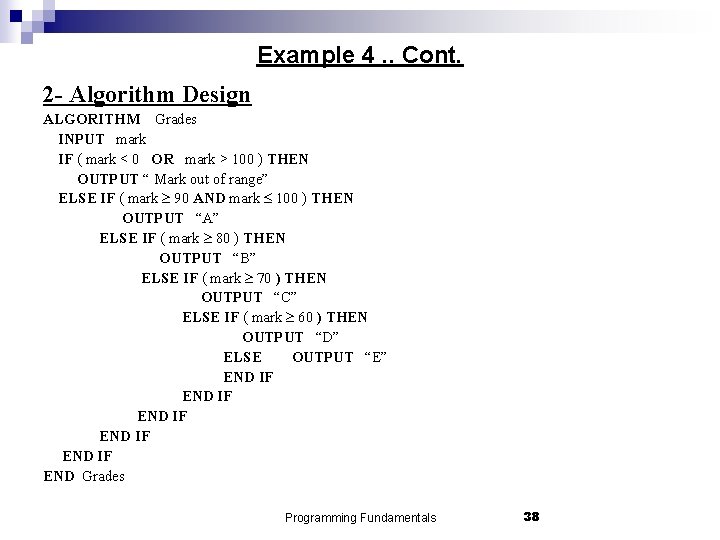
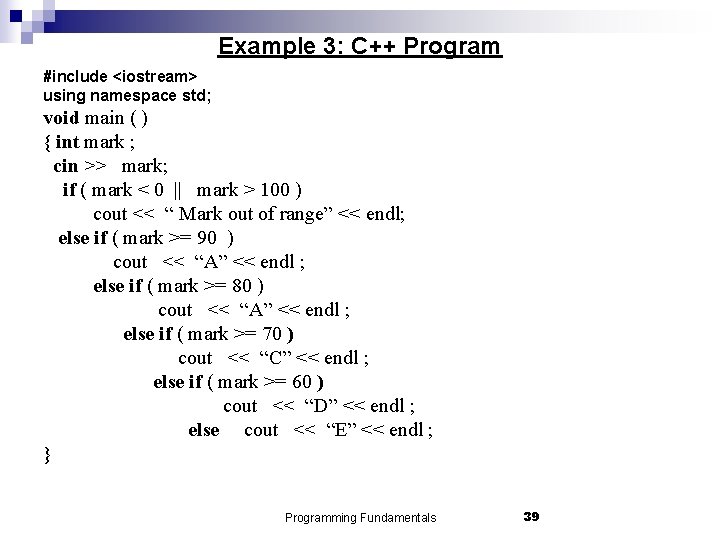
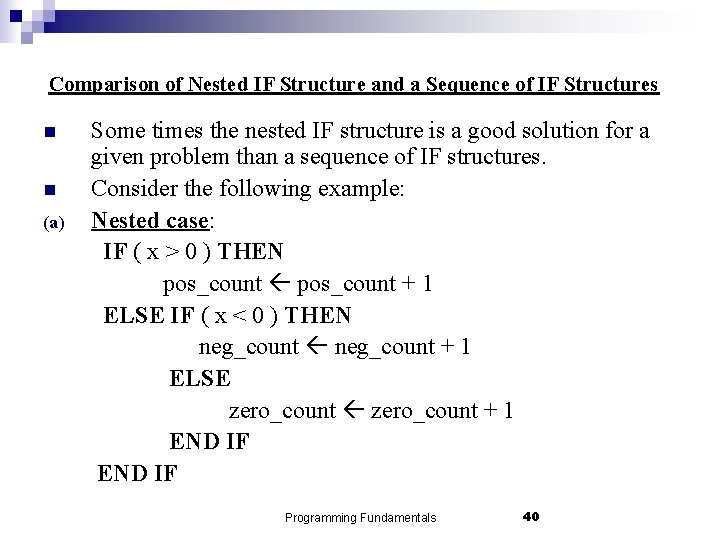
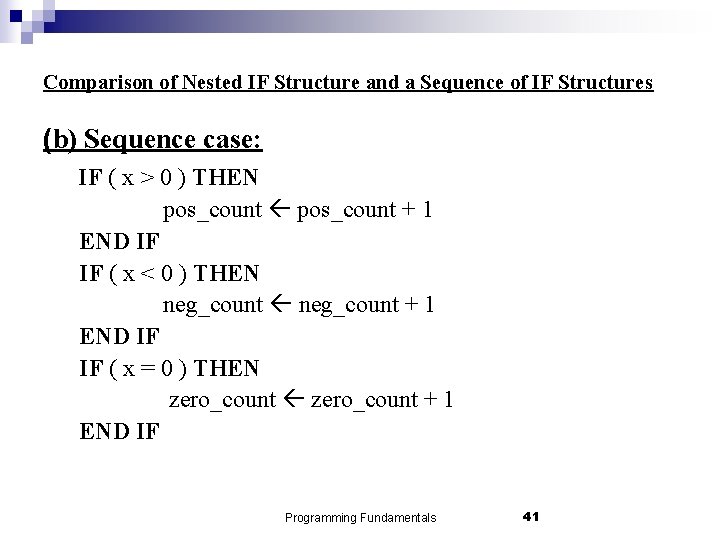
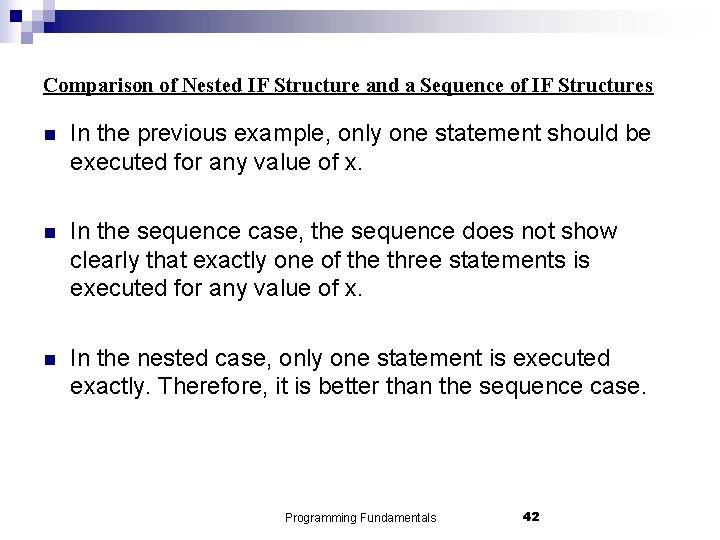
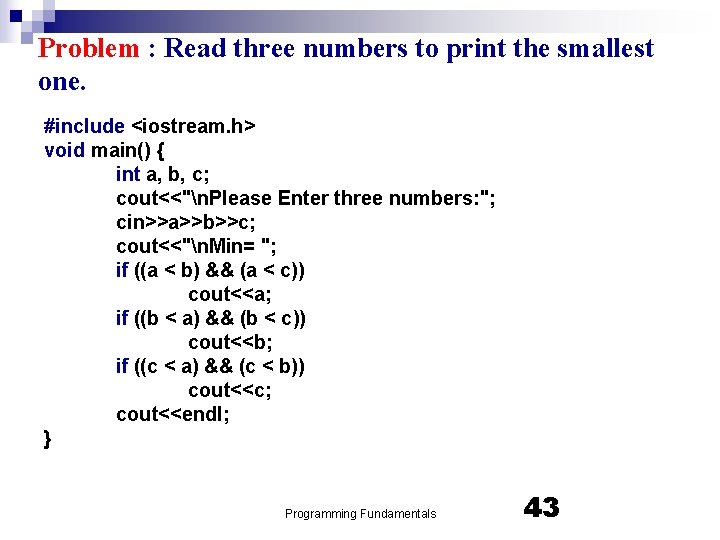
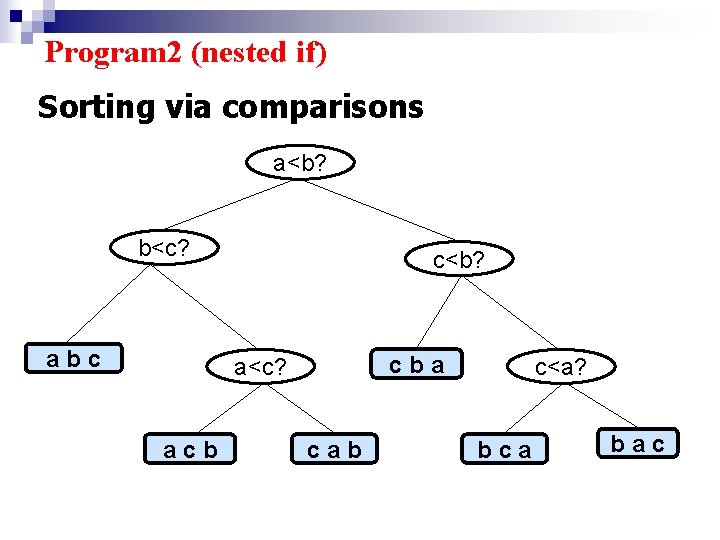
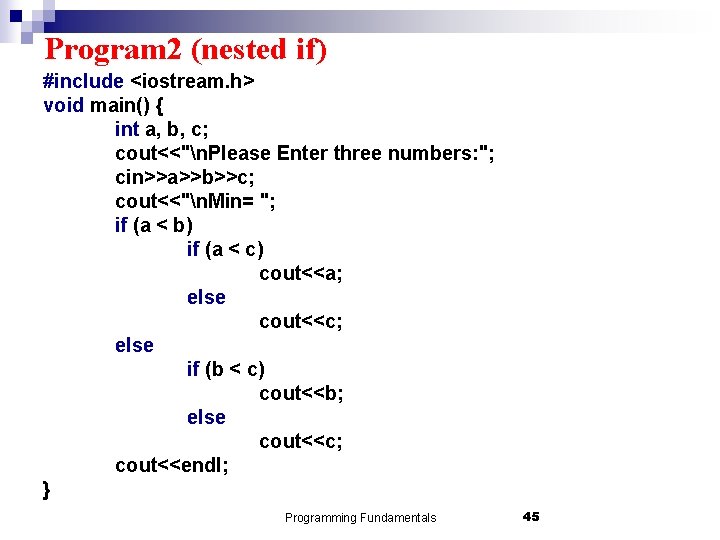
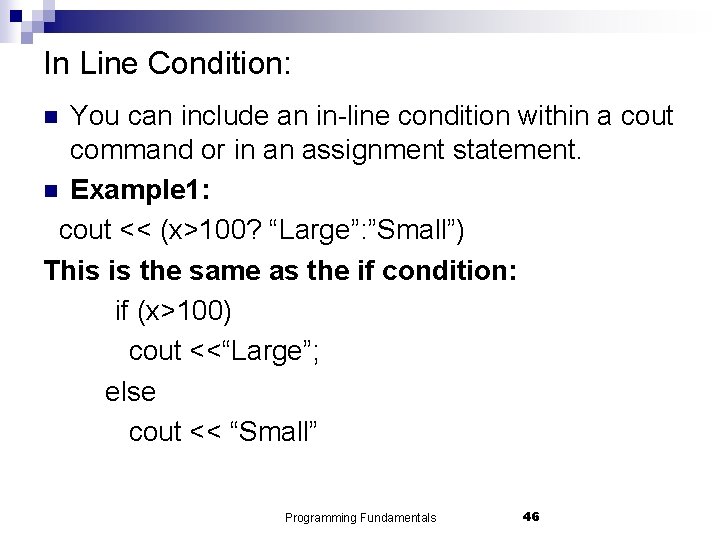
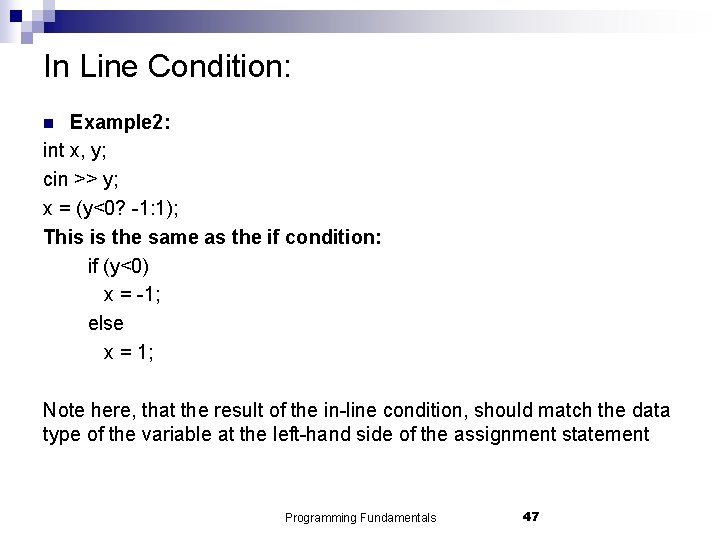
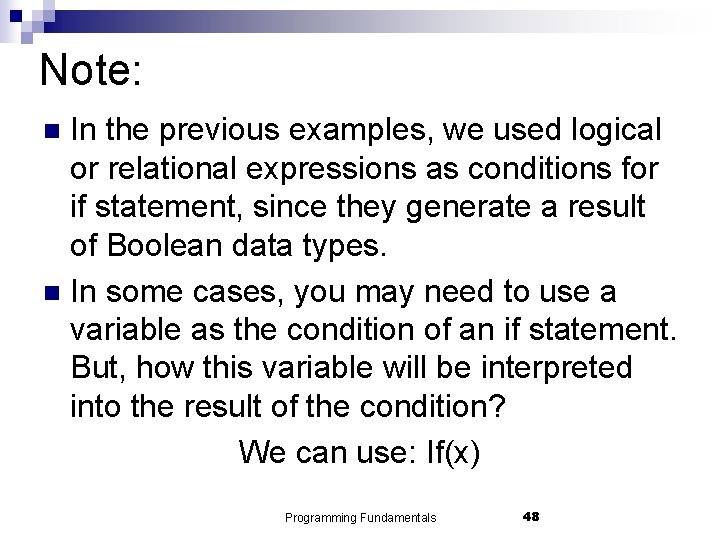
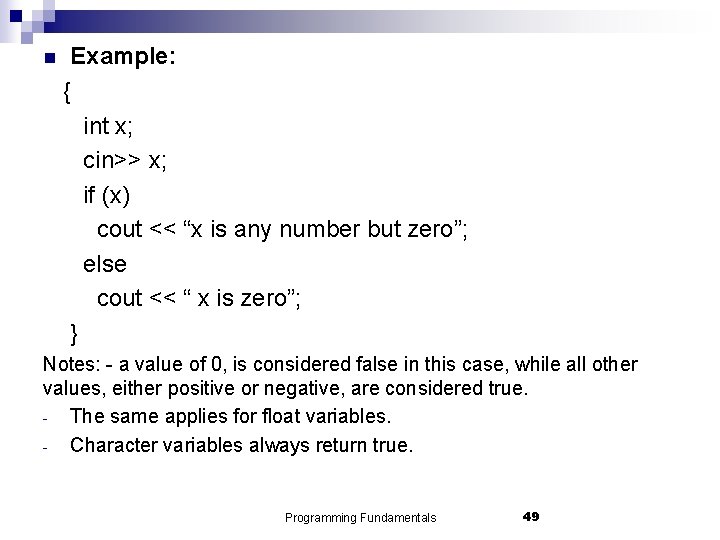
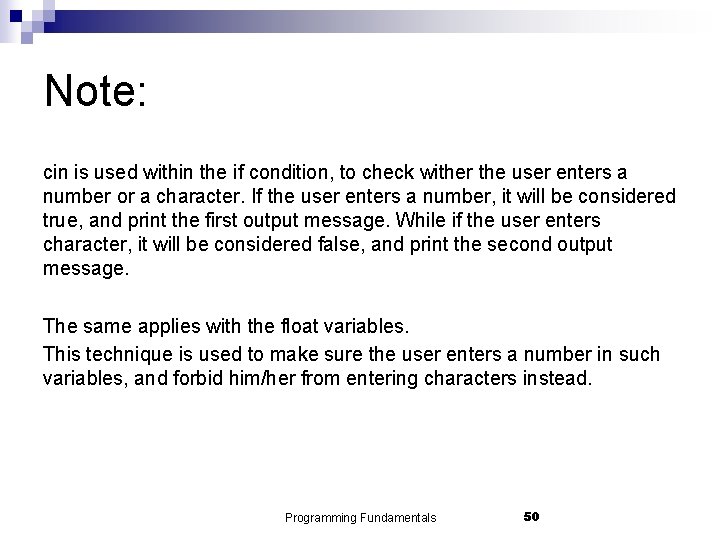
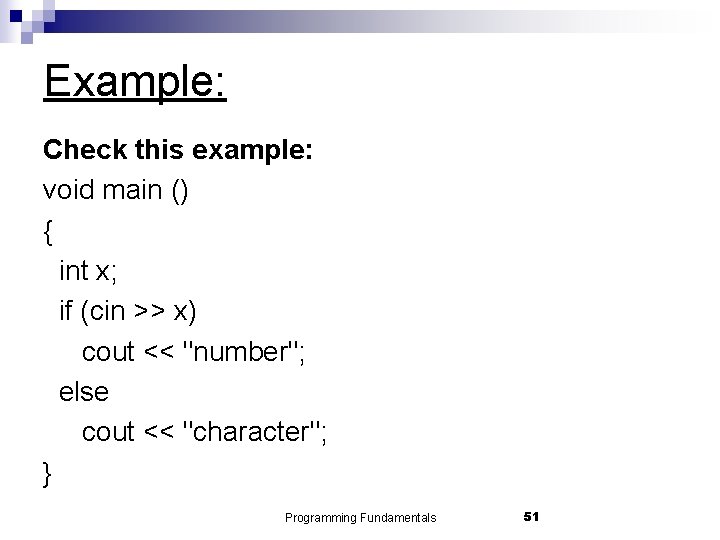
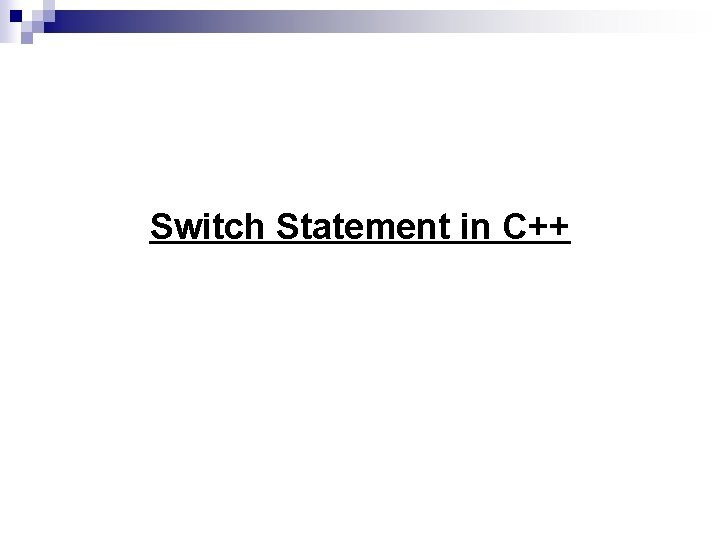
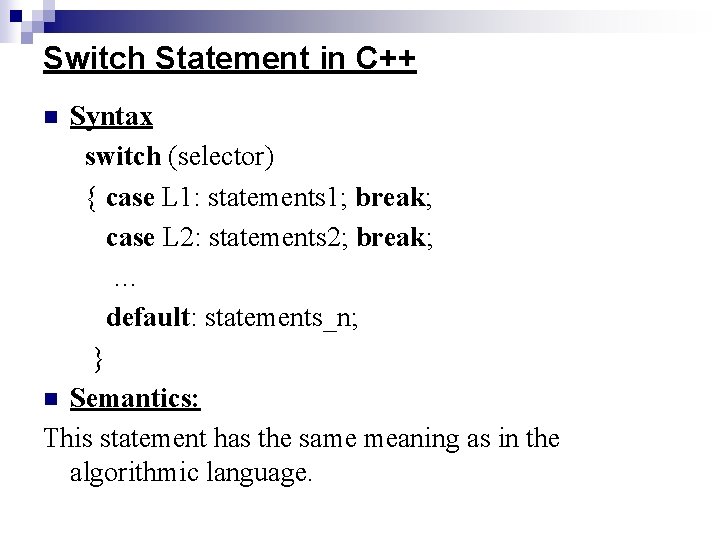
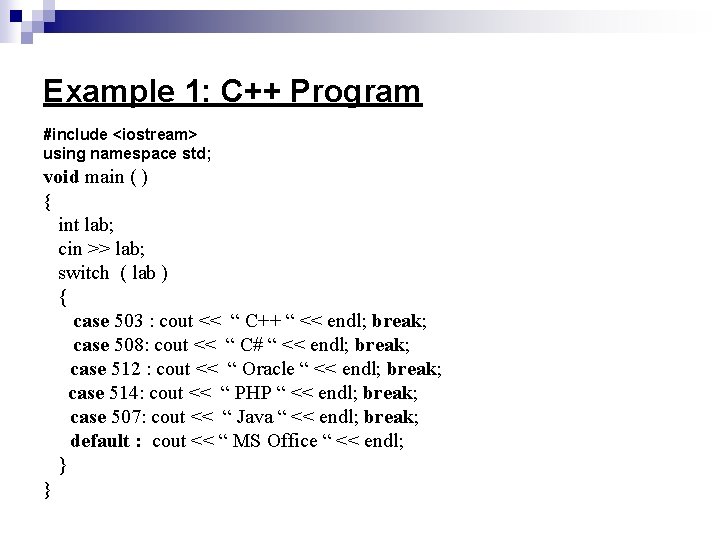
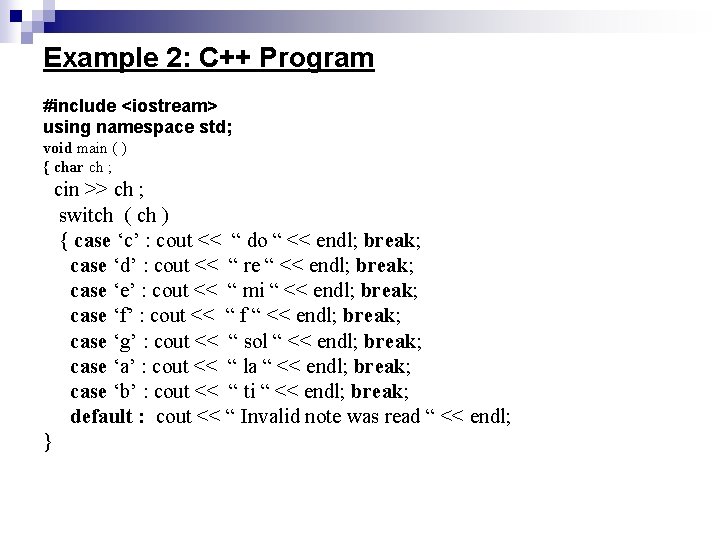
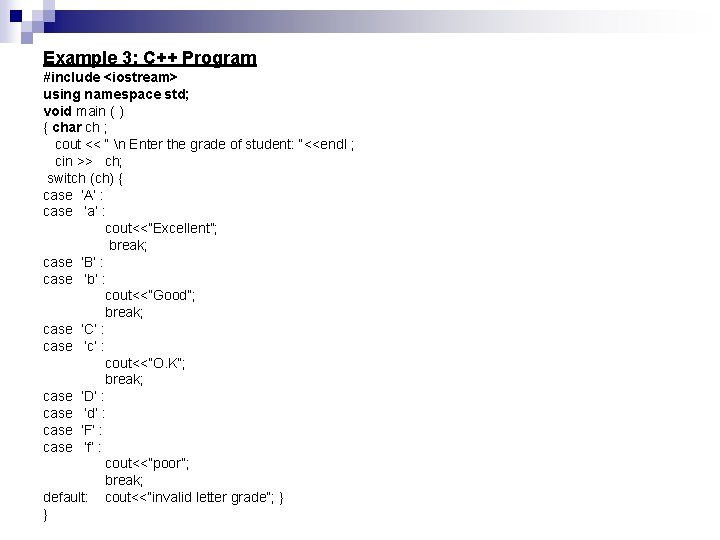
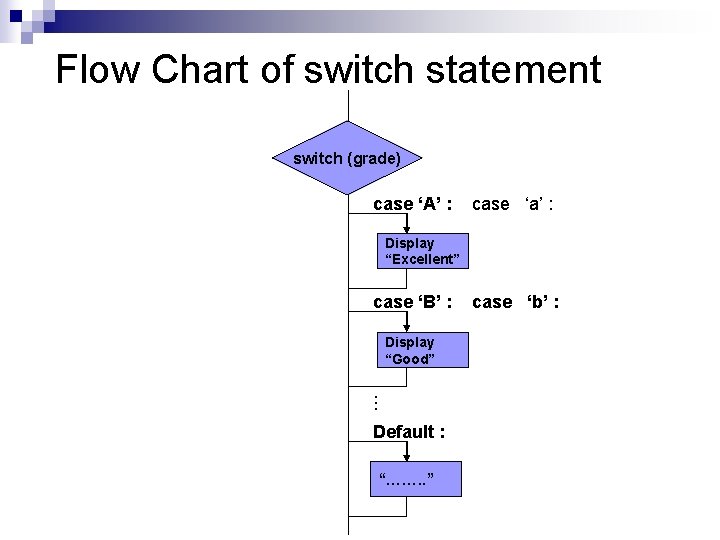
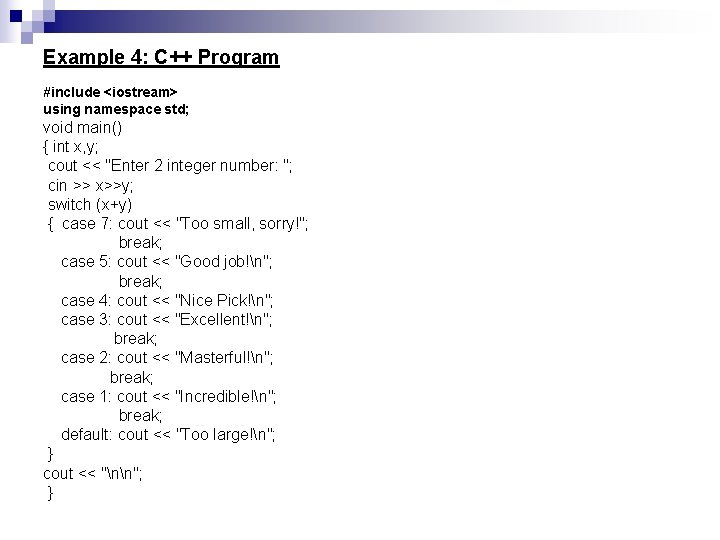
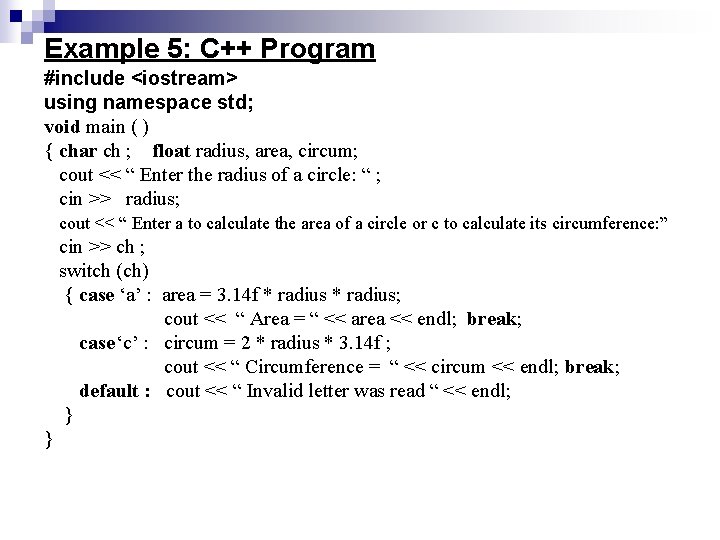
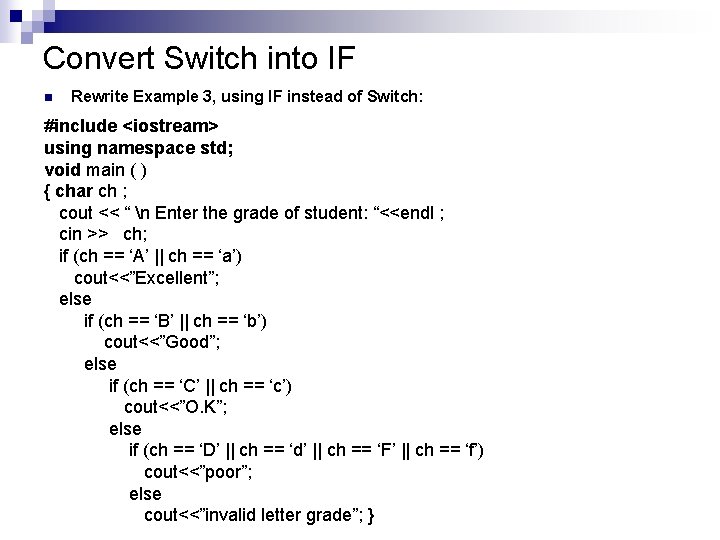
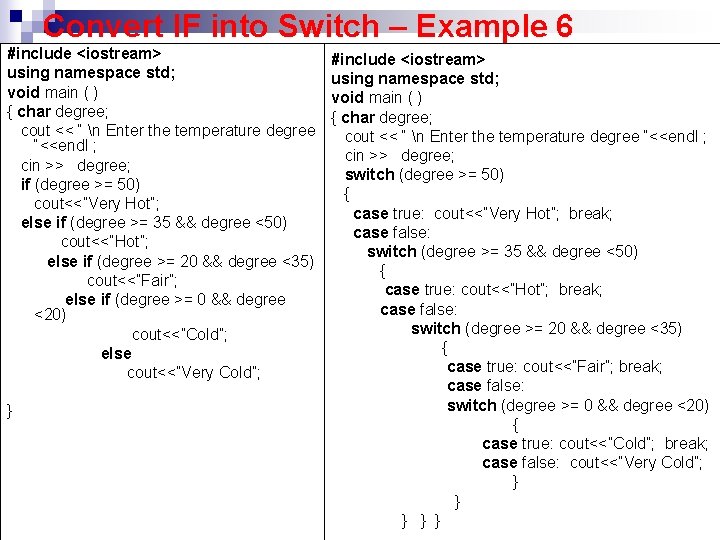
- Slides: 61
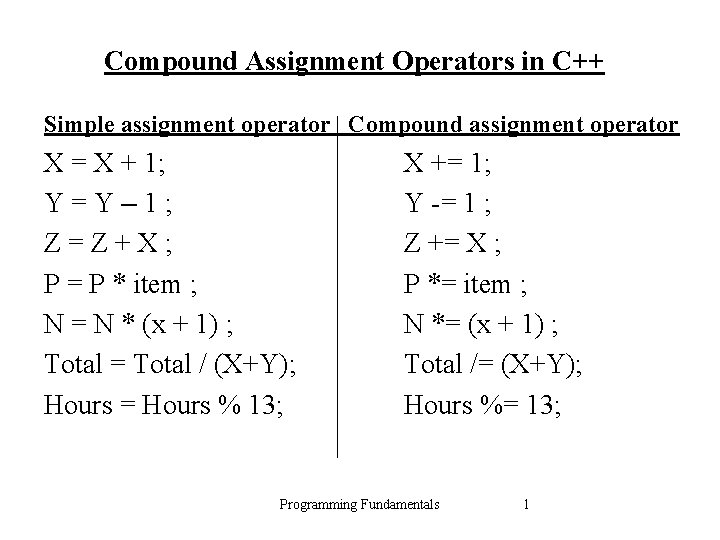
Compound Assignment Operators in C++ Simple assignment operator Compound assignment operator X = X + 1; Y=Y– 1; Z=Z+X; P = P * item ; N = N * (x + 1) ; Total = Total / (X+Y); Hours = Hours % 13; X += 1; Y -= 1 ; Z += X ; P *= item ; N *= (x + 1) ; Total /= (X+Y); Hours %= 13; Programming Fundamentals 1
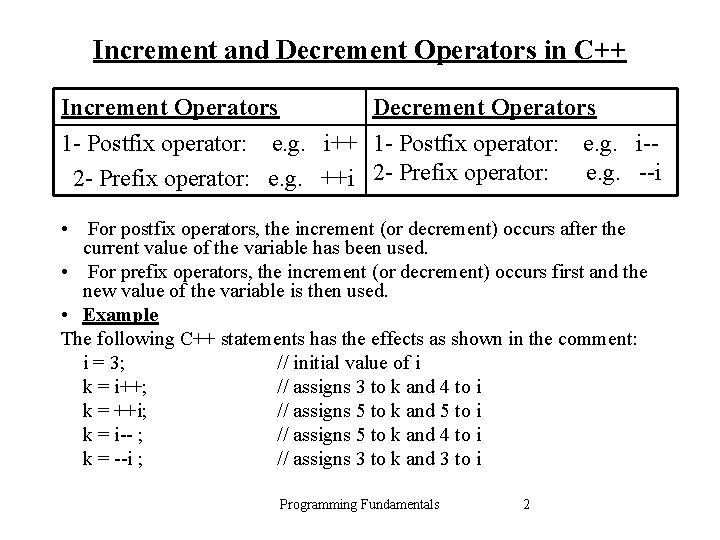
Increment and Decrement Operators in C++ Increment Operators Decrement Operators 1 - Postfix operator: e. g. i++ 1 - Postfix operator: e. g. i-e. g. --i 2 - Prefix operator: e. g. ++i 2 - Prefix operator: • For postfix operators, the increment (or decrement) occurs after the current value of the variable has been used. • For prefix operators, the increment (or decrement) occurs first and the new value of the variable is then used. • Example The following C++ statements has the effects as shown in the comment: i = 3; // initial value of i k = i++; // assigns 3 to k and 4 to i k = ++i; // assigns 5 to k and 5 to i k = i-- ; // assigns 5 to k and 4 to i k = --i ; // assigns 3 to k and 3 to i Programming Fundamentals 2
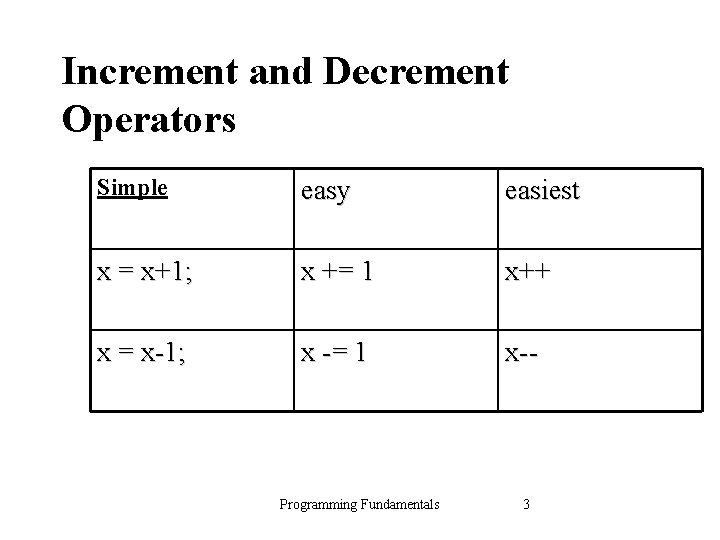
Increment and Decrement Operators Simple easy easiest x = x+1; x += 1 x++ x = x-1; x -= 1 x-- Programming Fundamentals 3
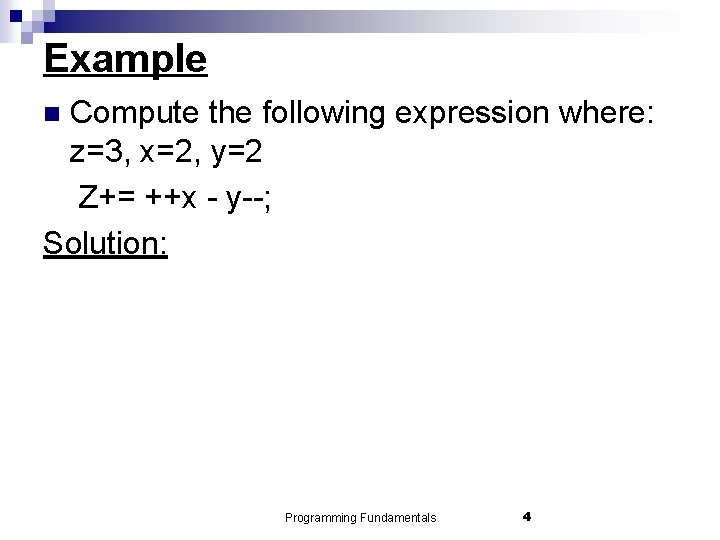
Example Compute the following expression where: z=3, x=2, y=2 Z+= ++x - y--; Solution: n Programming Fundamentals 4
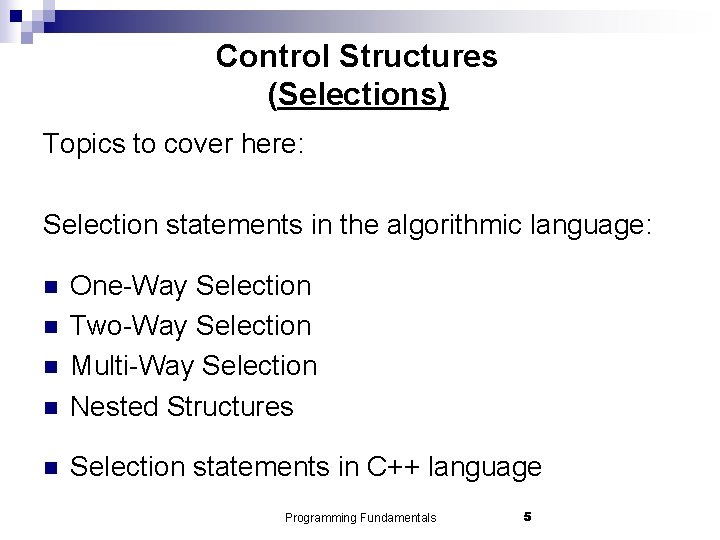
Control Structures (Selections) Topics to cover here: Selection statements in the algorithmic language: n One-Way Selection Two-Way Selection Multi-Way Selection Nested Structures n Selection statements in C++ language n n n Programming Fundamentals 5
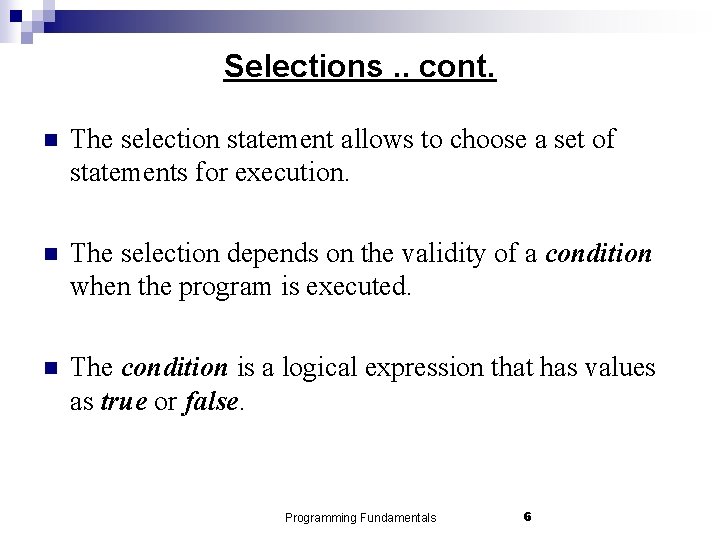
Selections. . cont. n The selection statement allows to choose a set of statements for execution. n The selection depends on the validity of a condition when the program is executed. n The condition is a logical expression that has values as true or false. Programming Fundamentals 6
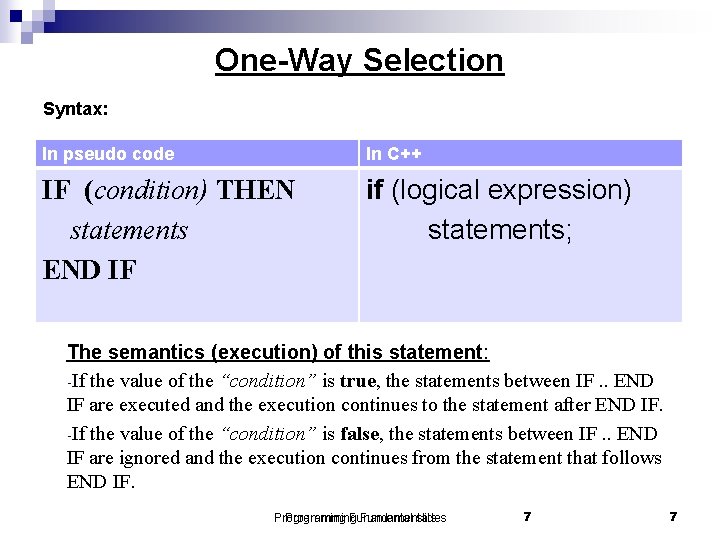
One-Way Selection Syntax: In pseudo code In C++ IF (condition) THEN statements END IF if (logical expression) statements; The semantics (execution) of this statement: -If the value of the “condition” is true, the statements between IF. . END IF are executed and the execution continues to the statement after END IF. -If the value of the “condition” is false, the statements between IF. . END IF are ignored and the execution continues from the statement that follows END IF. Programming Funamental Fundamentals slides 7 7
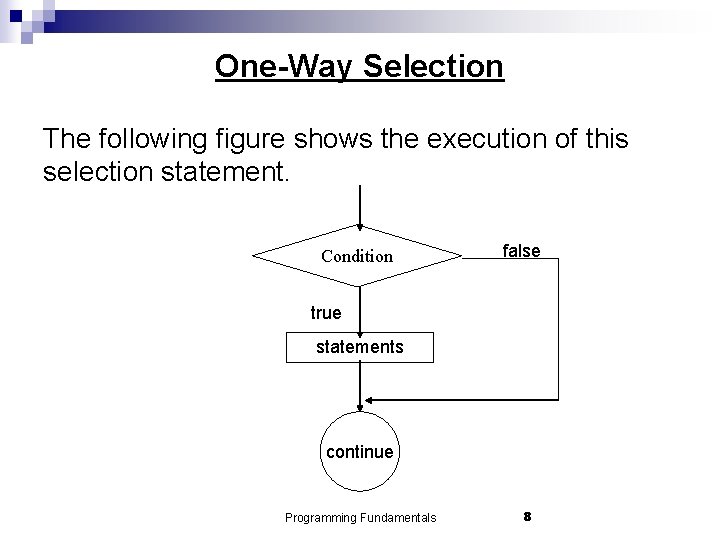
One-Way Selection The following figure shows the execution of this selection statement. Condition false true statements continue Programming Fundamentals 8
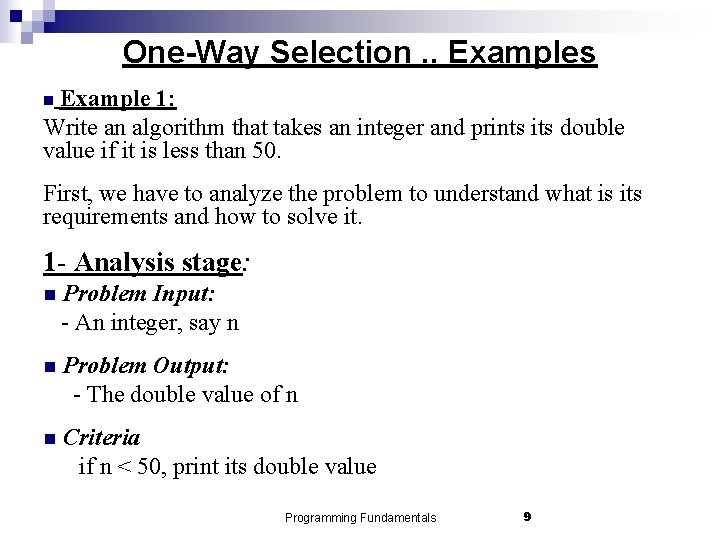
One-Way Selection. . Examples Example 1: Write an algorithm that takes an integer and prints its double value if it is less than 50. n First, we have to analyze the problem to understand what is its requirements and how to solve it. 1 - Analysis stage: n Problem Input: - An integer, say n n Problem Output: - The double value of n n Criteria if n < 50, print its double value Programming Fundamentals 9
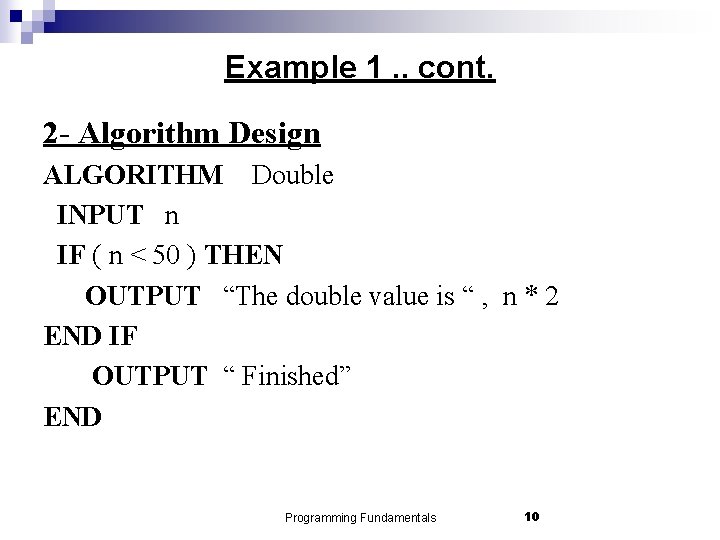
Example 1. . cont. 2 - Algorithm Design ALGORITHM Double INPUT n IF ( n < 50 ) THEN OUTPUT “The double value is “ , n * 2 END IF OUTPUT “ Finished” END Programming Fundamentals 10
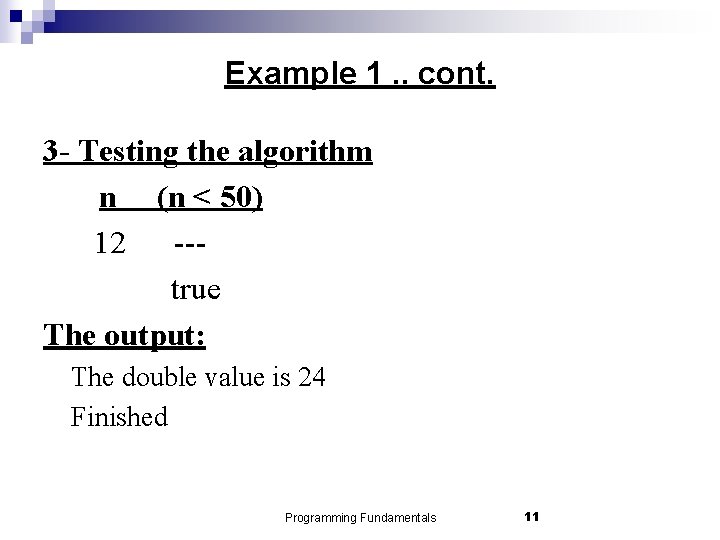
Example 1. . cont. 3 - Testing the algorithm n (n < 50) 12 --true The output: The double value is 24 Finished Programming Fundamentals 11
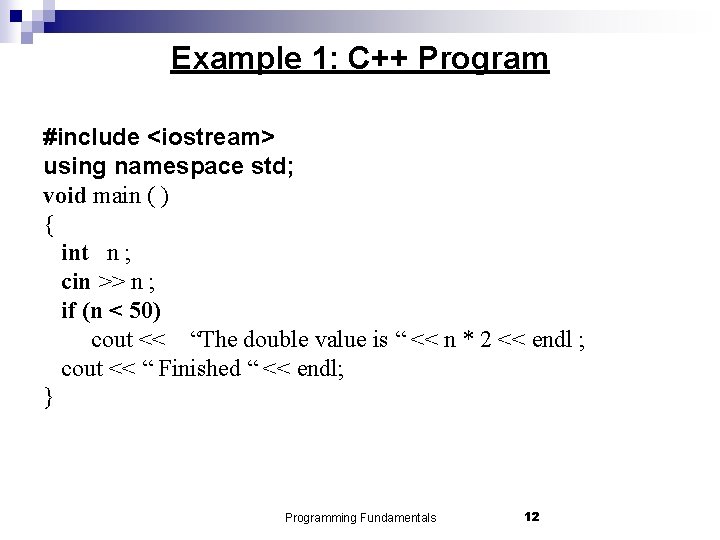
Example 1: C++ Program #include <iostream> using namespace std; void main ( ) { int n ; cin >> n ; if (n < 50) cout << “The double value is “ << n * 2 << endl ; cout << “ Finished “ << endl; } Programming Fundamentals 12
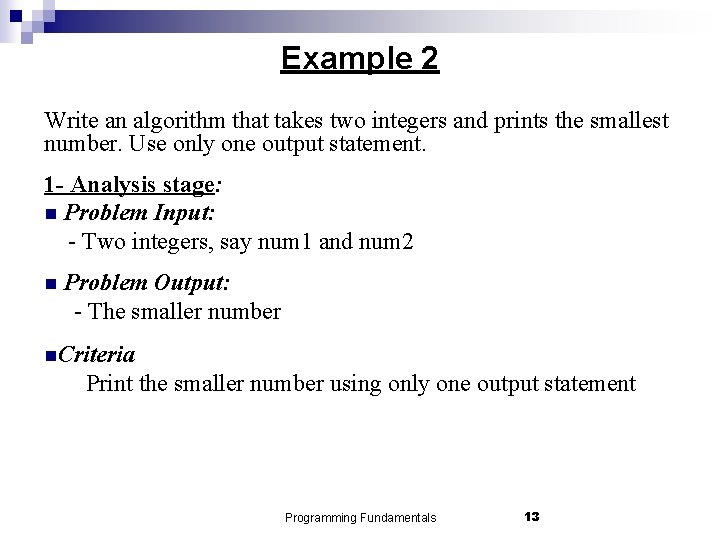
Example 2 Write an algorithm that takes two integers and prints the smallest number. Use only one output statement. 1 - Analysis stage: n Problem Input: - Two integers, say num 1 and num 2 n Problem Output: - The smaller number n. Criteria Print the smaller number using only one output statement Programming Fundamentals 13
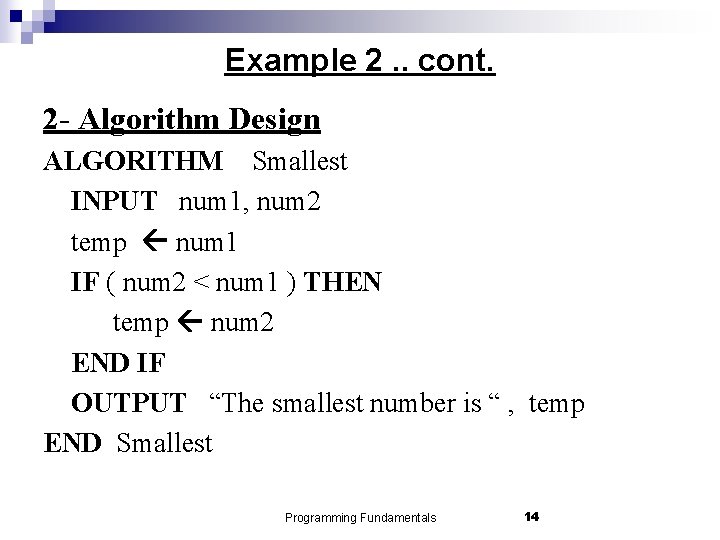
Example 2. . cont. 2 - Algorithm Design ALGORITHM Smallest INPUT num 1, num 2 temp num 1 IF ( num 2 < num 1 ) THEN temp num 2 END IF OUTPUT “The smallest number is “ , temp END Smallest Programming Fundamentals 14
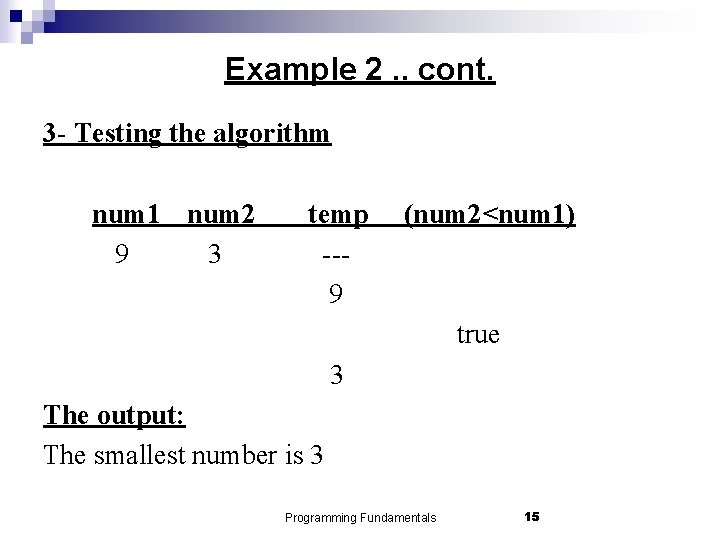
Example 2. . cont. 3 - Testing the algorithm num 1 num 2 9 3 temp --9 (num 2<num 1) true 3 The output: The smallest number is 3 Programming Fundamentals 15
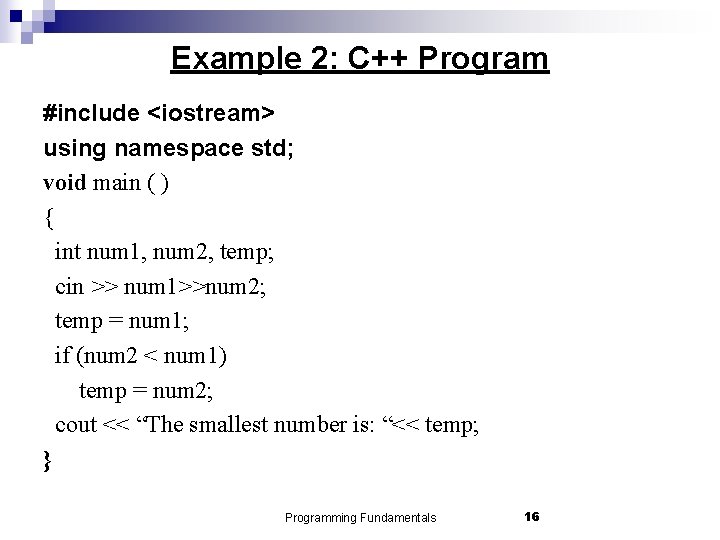
Example 2: C++ Program #include <iostream> using namespace std; void main ( ) { int num 1, num 2, temp; cin >> num 1>>num 2; temp = num 1; if (num 2 < num 1) temp = num 2; cout << “The smallest number is: “<< temp; } Programming Fundamentals 16
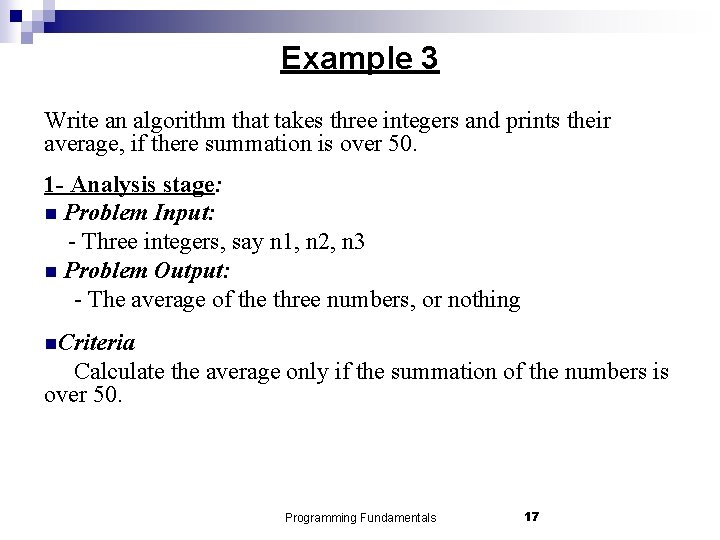
Example 3 Write an algorithm that takes three integers and prints their average, if there summation is over 50. 1 - Analysis stage: n Problem Input: - Three integers, say n 1, n 2, n 3 n Problem Output: - The average of the three numbers, or nothing n. Criteria Calculate the average only if the summation of the numbers is over 50. Programming Fundamentals 17
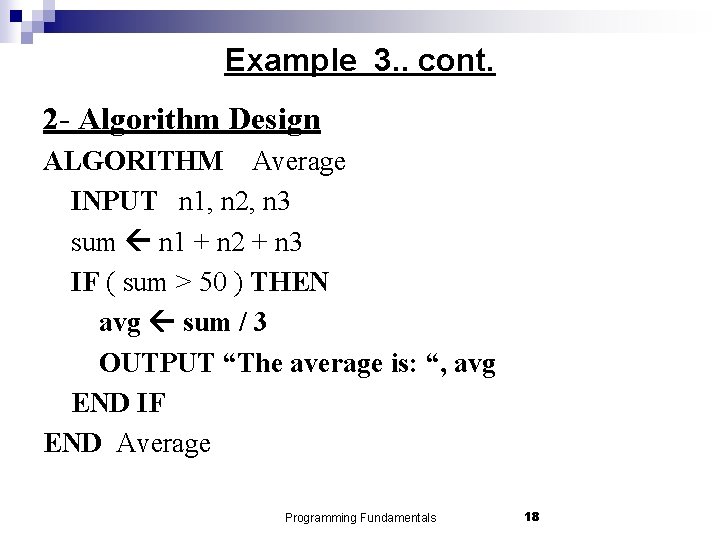
Example 3. . cont. 2 - Algorithm Design ALGORITHM Average INPUT n 1, n 2, n 3 sum n 1 + n 2 + n 3 IF ( sum > 50 ) THEN avg sum / 3 OUTPUT “The average is: “, avg END IF END Average Programming Fundamentals 18
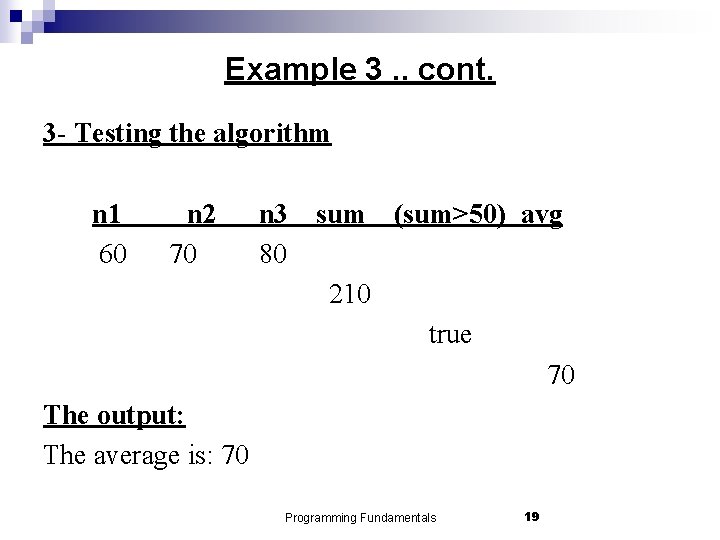
Example 3. . cont. 3 - Testing the algorithm n 1 60 n 2 70 n 3 80 sum (sum>50) avg 210 true 70 The output: The average is: 70 Programming Fundamentals 19
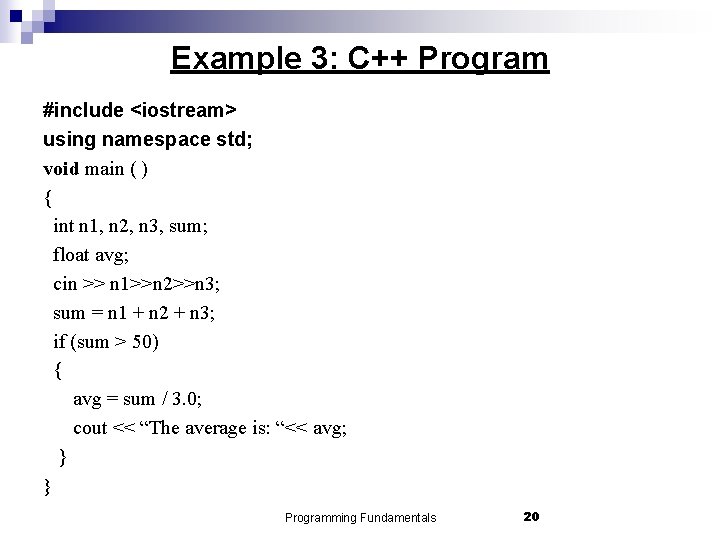
Example 3: C++ Program #include <iostream> using namespace std; void main ( ) { int n 1, n 2, n 3, sum; float avg; cin >> n 1>>n 2>>n 3; sum = n 1 + n 2 + n 3; if (sum > 50) { avg = sum / 3. 0; cout << “The average is: “<< avg; } } Programming Fundamentals 20
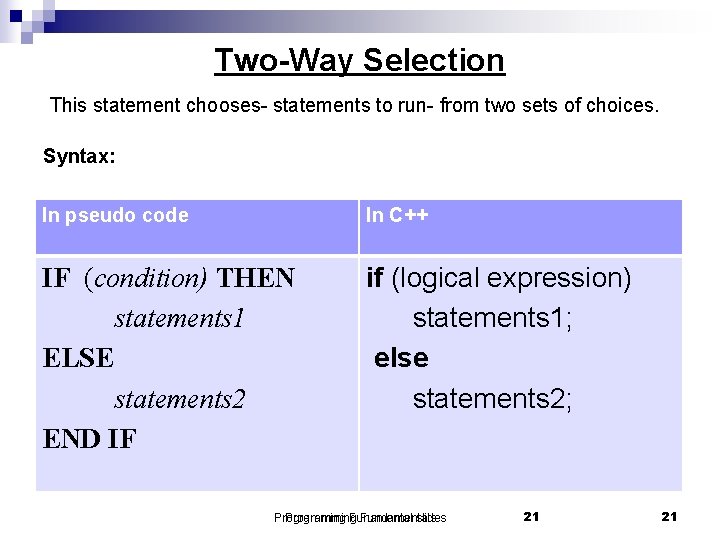
Two-Way Selection This statement chooses- statements to run- from two sets of choices. Syntax: In pseudo code In C++ IF (condition) THEN statements 1 ELSE statements 2 END IF if (logical expression) statements 1; else statements 2; Programming Funamental Fundamentals slides 21 21
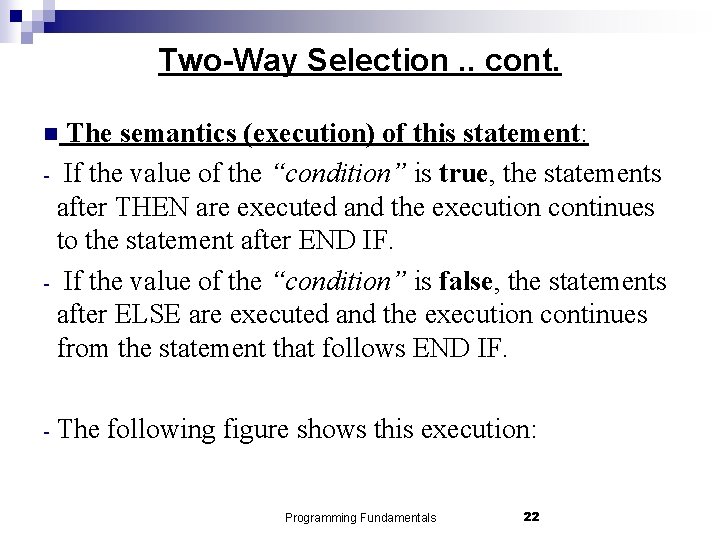
Two-Way Selection. . cont. The semantics (execution) of this statement: - If the value of the “condition” is true, the statements after THEN are executed and the execution continues to the statement after END IF. - If the value of the “condition” is false, the statements after ELSE are executed and the execution continues from the statement that follows END IF. n - The following figure shows this execution: Programming Fundamentals 22
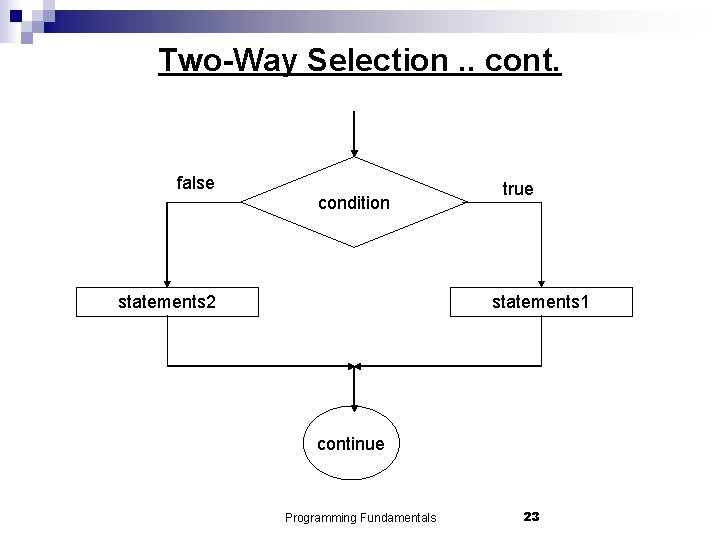
Two-Way Selection. . cont. false condition statements 2 true statements 1 continue Programming Fundamentals 23
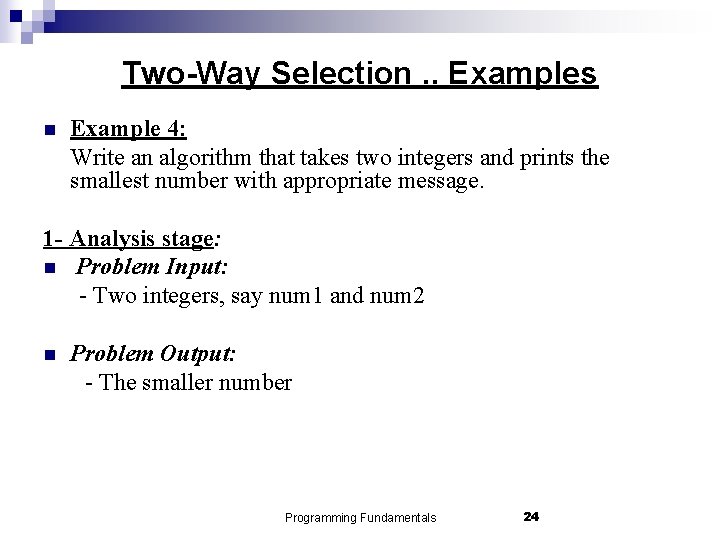
Two-Way Selection. . Examples n Example 4: Write an algorithm that takes two integers and prints the smallest number with appropriate message. 1 - Analysis stage: n Problem Input: - Two integers, say num 1 and num 2 n Problem Output: - The smaller number Programming Fundamentals 24
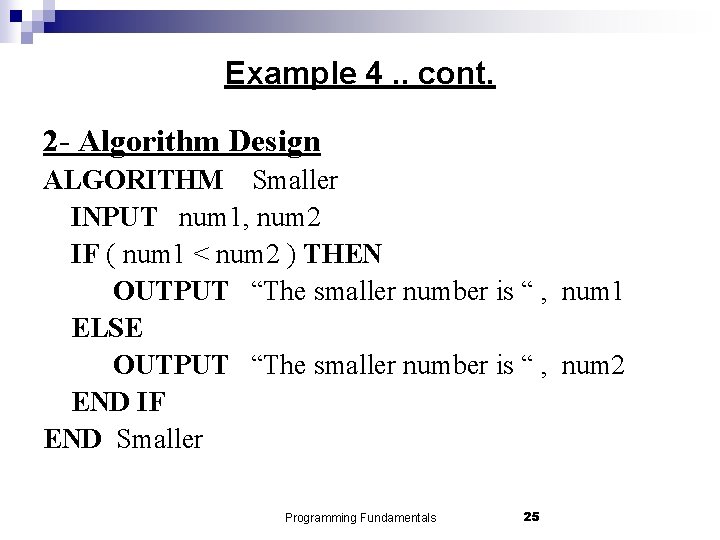
Example 4. . cont. 2 - Algorithm Design ALGORITHM Smaller INPUT num 1, num 2 IF ( num 1 < num 2 ) THEN OUTPUT “The smaller number is “ , num 1 ELSE OUTPUT “The smaller number is “ , num 2 END IF END Smaller Programming Fundamentals 25
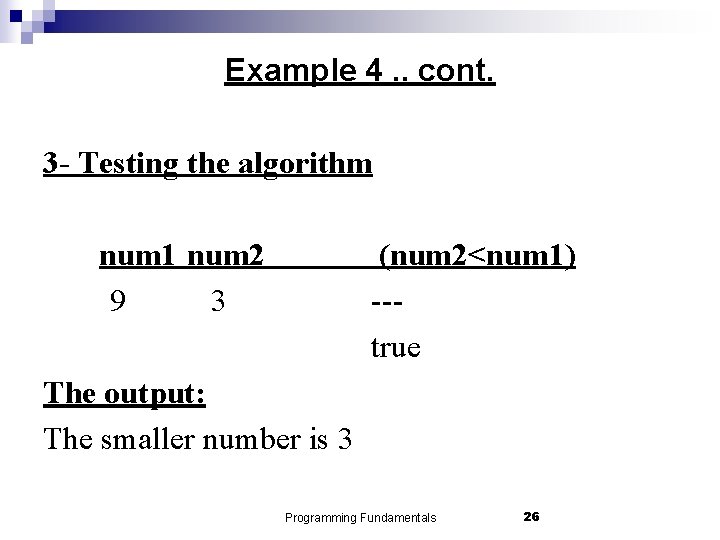
Example 4. . cont. 3 - Testing the algorithm num 1 num 2 9 3 (num 2<num 1) --true The output: The smaller number is 3 Programming Fundamentals 26
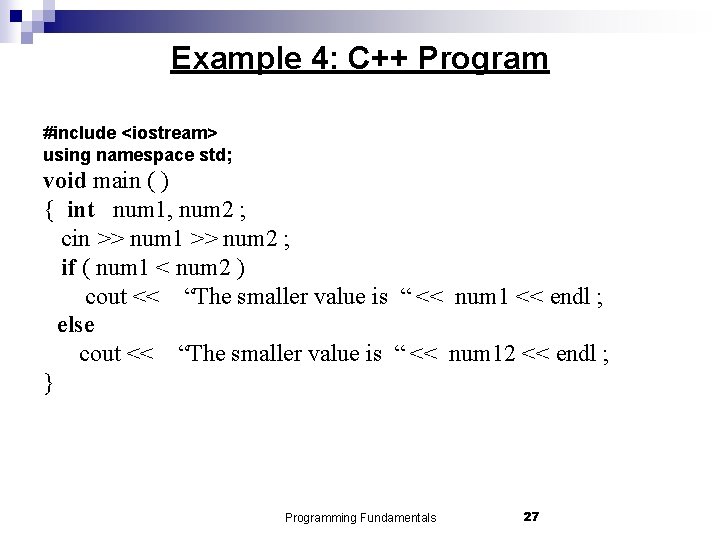
Example 4: C++ Program #include <iostream> using namespace std; void main ( ) { int num 1, num 2 ; cin >> num 1 >> num 2 ; if ( num 1 < num 2 ) cout << “The smaller value is “ << num 1 << endl ; else cout << “The smaller value is “ << num 12 << endl ; } Programming Fundamentals 27
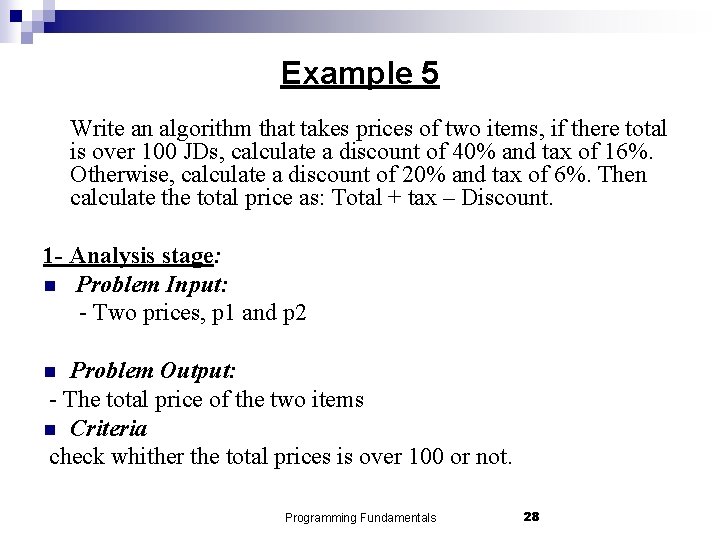
Example 5 Write an algorithm that takes prices of two items, if there total is over 100 JDs, calculate a discount of 40% and tax of 16%. Otherwise, calculate a discount of 20% and tax of 6%. Then calculate the total price as: Total + tax – Discount. 1 - Analysis stage: n Problem Input: - Two prices, p 1 and p 2 Problem Output: - The total price of the two items n Criteria check whither the total prices is over 100 or not. n Programming Fundamentals 28
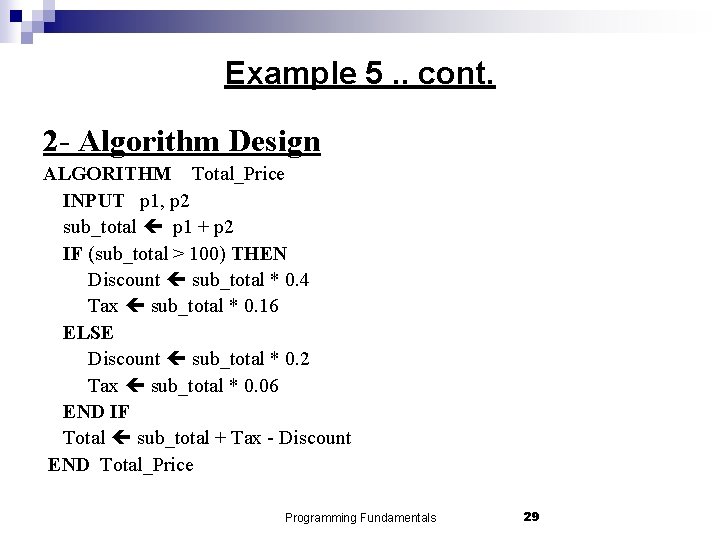
Example 5. . cont. 2 - Algorithm Design ALGORITHM Total_Price INPUT p 1, p 2 sub_total p 1 + p 2 IF (sub_total > 100) THEN Discount sub_total * 0. 4 Tax sub_total * 0. 16 ELSE Discount sub_total * 0. 2 Tax sub_total * 0. 06 END IF Total sub_total + Tax - Discount END Total_Price Programming Fundamentals 29
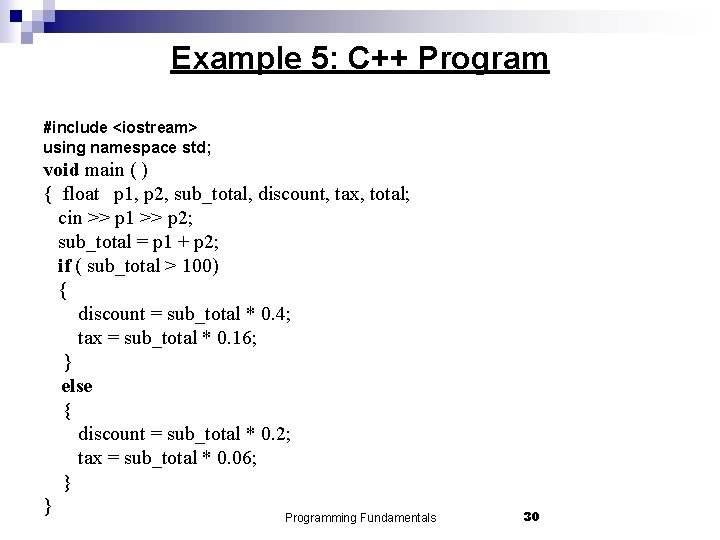
Example 5: C++ Program #include <iostream> using namespace std; void main ( ) { float p 1, p 2, sub_total, discount, tax, total; cin >> p 1 >> p 2; sub_total = p 1 + p 2; if ( sub_total > 100) { discount = sub_total * 0. 4; tax = sub_total * 0. 16; } else { discount = sub_total * 0. 2; tax = sub_total * 0. 06; } } Programming Fundamentals 30
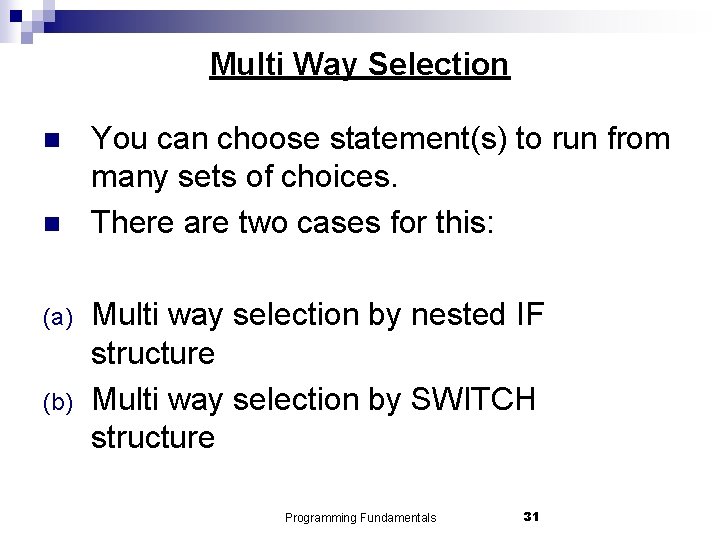
Multi Way Selection n n (a) (b) You can choose statement(s) to run from many sets of choices. There are two cases for this: Multi way selection by nested IF structure Multi way selection by SWITCH structure Programming Fundamentals 31
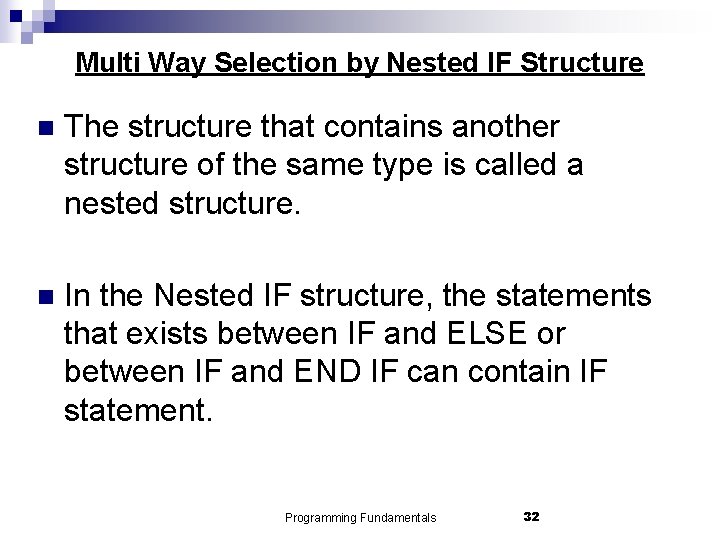
Multi Way Selection by Nested IF Structure n The structure that contains another structure of the same type is called a nested structure. n In the Nested IF structure, the statements that exists between IF and ELSE or between IF and END IF can contain IF statement. Programming Fundamentals 32
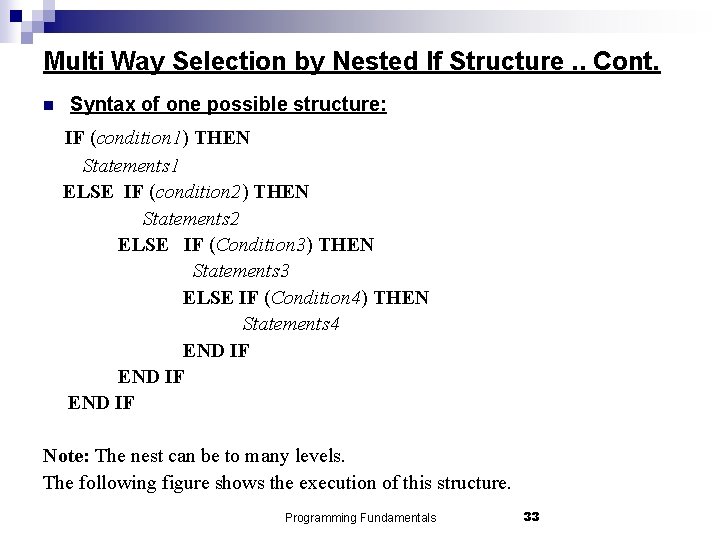
Multi Way Selection by Nested If Structure. . Cont. n Syntax of one possible structure: IF (condition 1) THEN Statements 1 ELSE IF (condition 2) THEN Statements 2 ELSE IF (Condition 3) THEN Statements 3 ELSE IF (Condition 4) THEN Statements 4 END IF Note: The nest can be to many levels. The following figure shows the execution of this structure. Programming Fundamentals 33
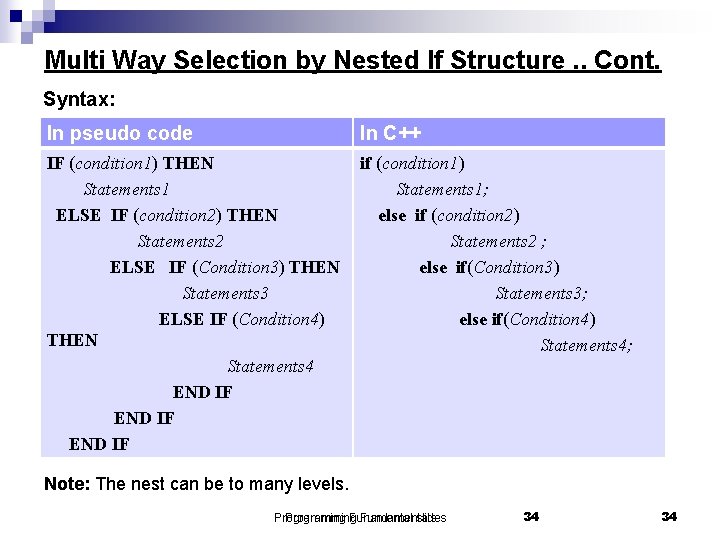
Multi Way Selection by Nested If Structure. . Cont. Syntax: In pseudo code In C++ IF (condition 1) THEN Statements 1 ELSE IF (condition 2) THEN Statements 2 ELSE IF (Condition 3) THEN Statements 3 ELSE IF (Condition 4) THEN Statements 4 END IF if (condition 1) Statements 1; else if (condition 2) Statements 2 ; else if(Condition 3) Statements 3; else if(Condition 4) Statements 4; Note: The nest can be to many levels. Programming Funamental Fundamentals slides 34 34
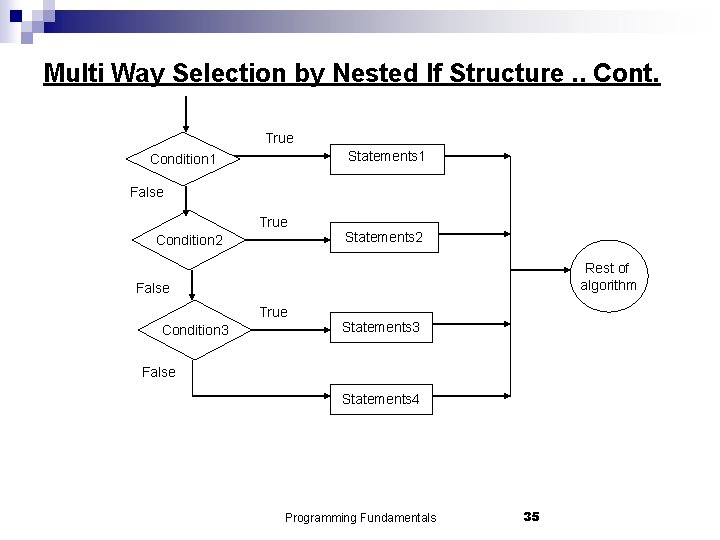
Multi Way Selection by Nested If Structure. . Cont. True Statements 1 Condition 1 False True Condition 2 Statements 2 Rest of algorithm False True Condition 3 Statements 3 False Statements 4 Programming Fundamentals 35
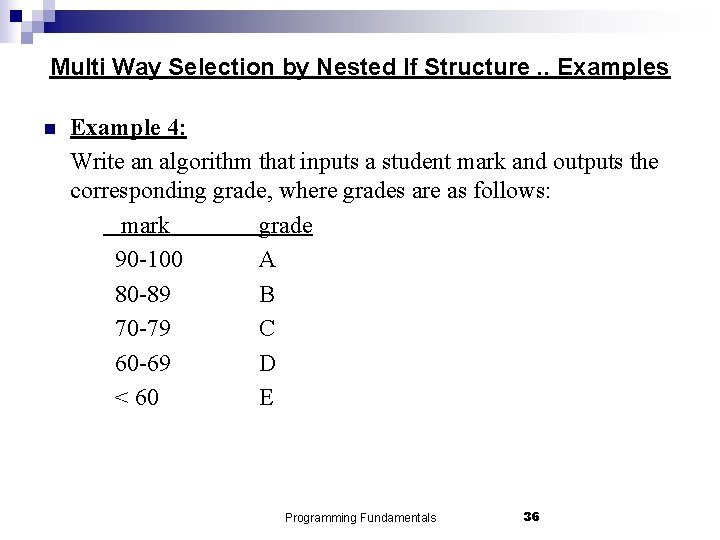
Multi Way Selection by Nested If Structure. . Examples n Example 4: Write an algorithm that inputs a student mark and outputs the corresponding grade, where grades are as follows: mark grade 90 -100 A 80 -89 B 70 -79 C 60 -69 D < 60 E Programming Fundamentals 36
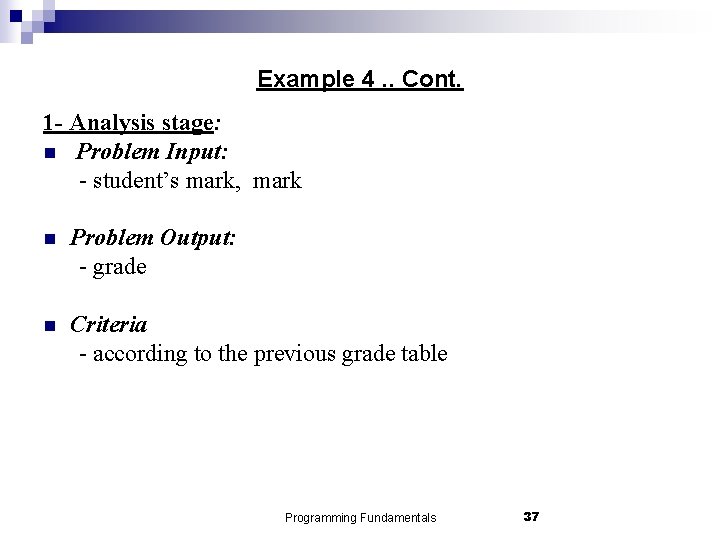
Example 4. . Cont. 1 - Analysis stage: n Problem Input: - student’s mark, mark n Problem Output: - grade n Criteria - according to the previous grade table Programming Fundamentals 37
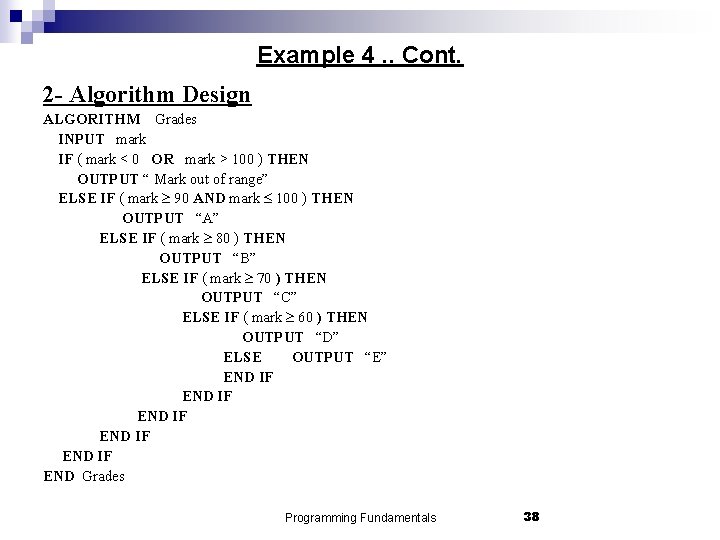
Example 4. . Cont. 2 - Algorithm Design ALGORITHM Grades INPUT mark IF ( mark < 0 OR mark > 100 ) THEN OUTPUT “ Mark out of range” ELSE IF ( mark 90 AND mark 100 ) THEN OUTPUT “A” ELSE IF ( mark 80 ) THEN OUTPUT “B” ELSE IF ( mark 70 ) THEN OUTPUT “C” ELSE IF ( mark 60 ) THEN OUTPUT “D” ELSE OUTPUT “E” END IF END IF END Grades Programming Fundamentals 38
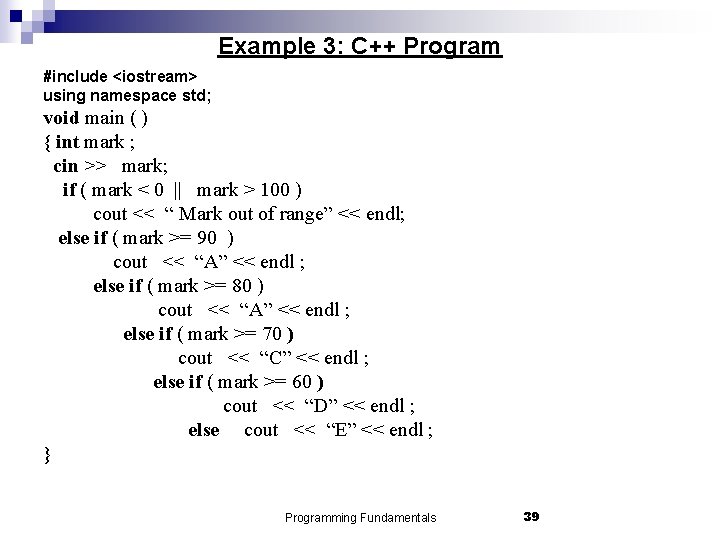
Example 3: C++ Program #include <iostream> using namespace std; void main ( ) { int mark ; cin >> mark; if ( mark < 0 || mark > 100 ) cout << “ Mark out of range” << endl; else if ( mark >= 90 ) cout << “A” << endl ; else if ( mark >= 80 ) cout << “A” << endl ; else if ( mark >= 70 ) cout << “C” << endl ; else if ( mark >= 60 ) cout << “D” << endl ; else cout << “E” << endl ; } Programming Fundamentals 39
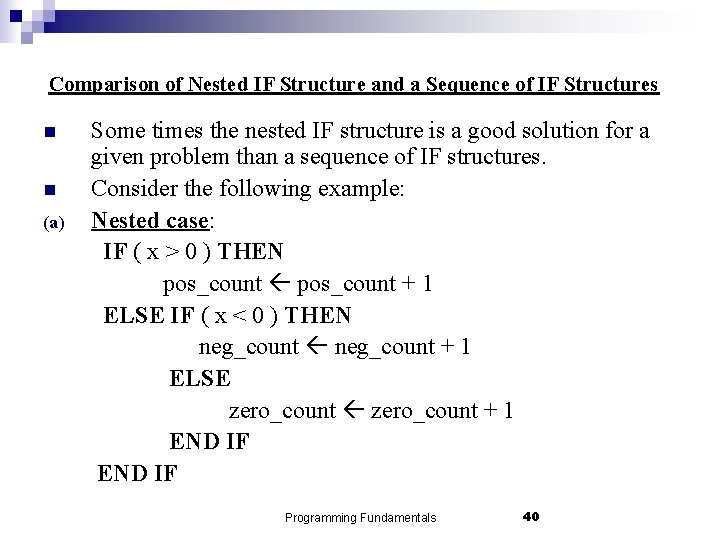
Comparison of Nested IF Structure and a Sequence of IF Structures n n (a) Some times the nested IF structure is a good solution for a given problem than a sequence of IF structures. Consider the following example: Nested case: IF ( x > 0 ) THEN pos_count + 1 ELSE IF ( x < 0 ) THEN neg_count + 1 ELSE zero_count + 1 END IF Programming Fundamentals 40
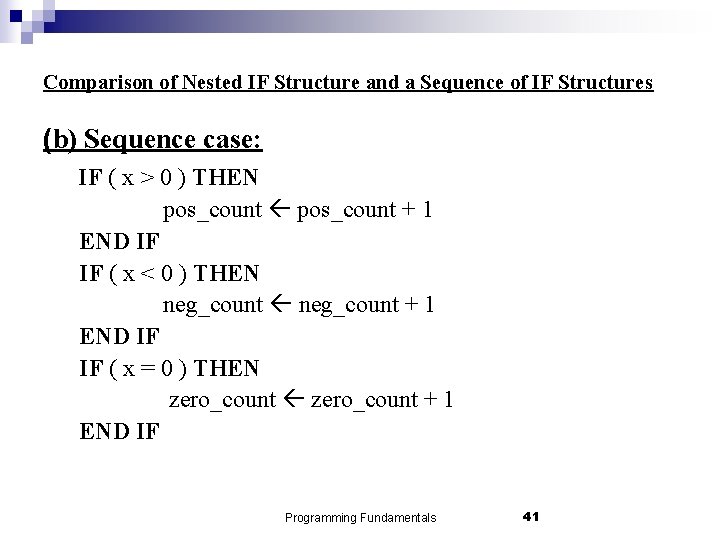
Comparison of Nested IF Structure and a Sequence of IF Structures (b) Sequence case: IF ( x > 0 ) THEN pos_count + 1 END IF IF ( x < 0 ) THEN neg_count + 1 END IF IF ( x = 0 ) THEN zero_count + 1 END IF Programming Fundamentals 41
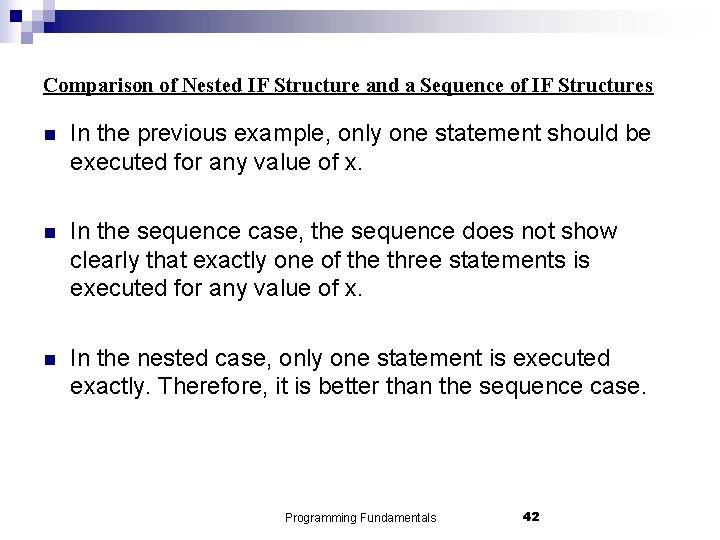
Comparison of Nested IF Structure and a Sequence of IF Structures n In the previous example, only one statement should be executed for any value of x. n In the sequence case, the sequence does not show clearly that exactly one of the three statements is executed for any value of x. n In the nested case, only one statement is executed exactly. Therefore, it is better than the sequence case. Programming Fundamentals 42
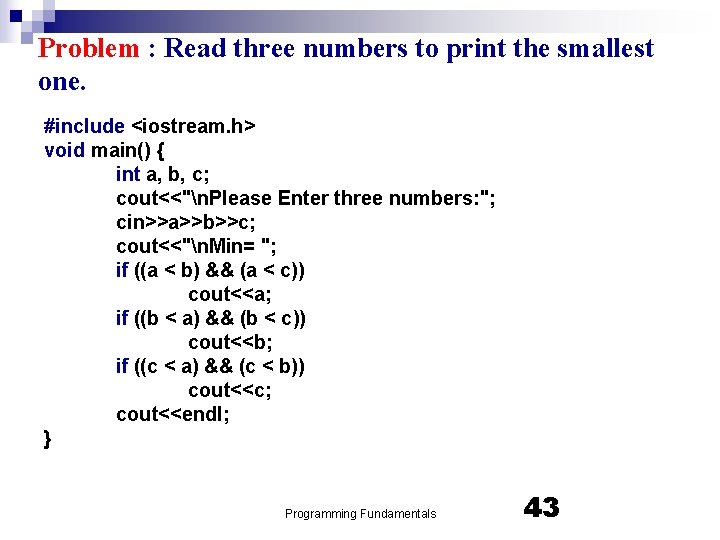
Problem : Read three numbers to print the smallest one. #include <iostream. h> void main() { int a, b, c; cout<<"n. Please Enter three numbers: "; cin>>a>>b>>c; cout<<"n. Min= "; if ((a < b) && (a < c)) cout<<a; if ((b < a) && (b < c)) cout<<b; if ((c < a) && (c < b)) cout<<c; cout<<endl; } Programming Fundamentals 43
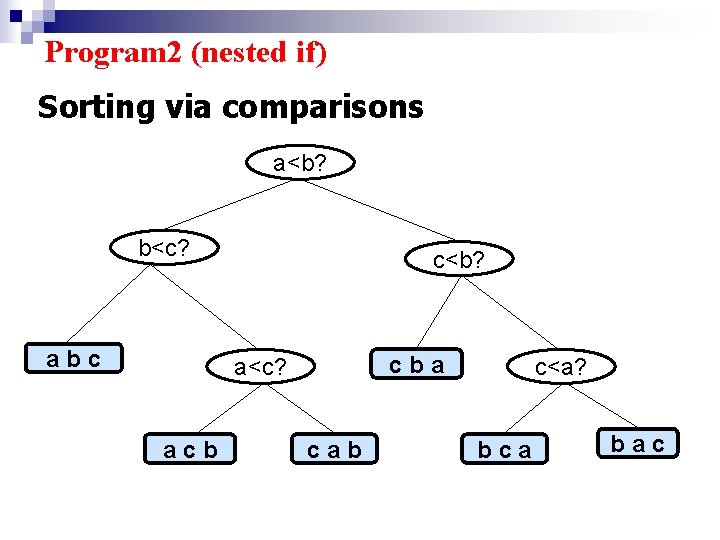
Program 2 (nested if) Sorting via comparisons a<b? b<c? abc c<b? cba a<c? acb cab c<a? bca bac
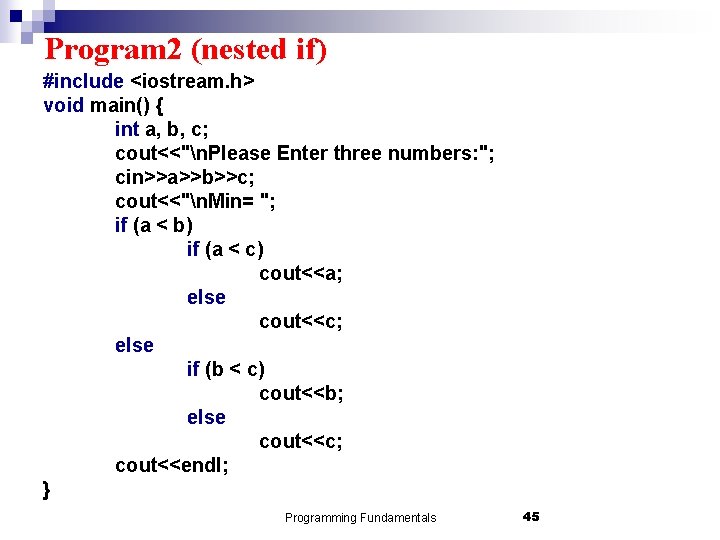
Program 2 (nested if) #include <iostream. h> void main() { int a, b, c; cout<<"n. Please Enter three numbers: "; cin>>a>>b>>c; cout<<"n. Min= "; if (a < b) if (a < c) cout<<a; else cout<<c; else if (b < c) cout<<b; else cout<<c; cout<<endl; } Programming Fundamentals 45
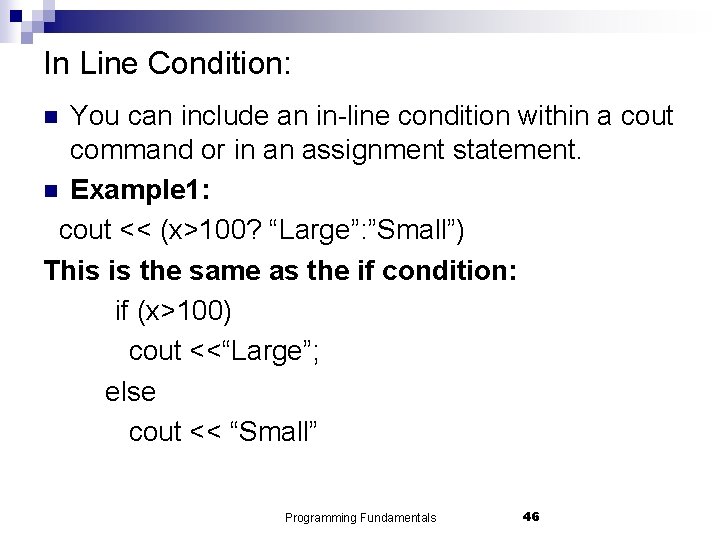
In Line Condition: You can include an in-line condition within a cout command or in an assignment statement. n Example 1: cout << (x>100? “Large”: ”Small”) This is the same as the if condition: if (x>100) cout <<“Large”; else cout << “Small” n Programming Fundamentals 46
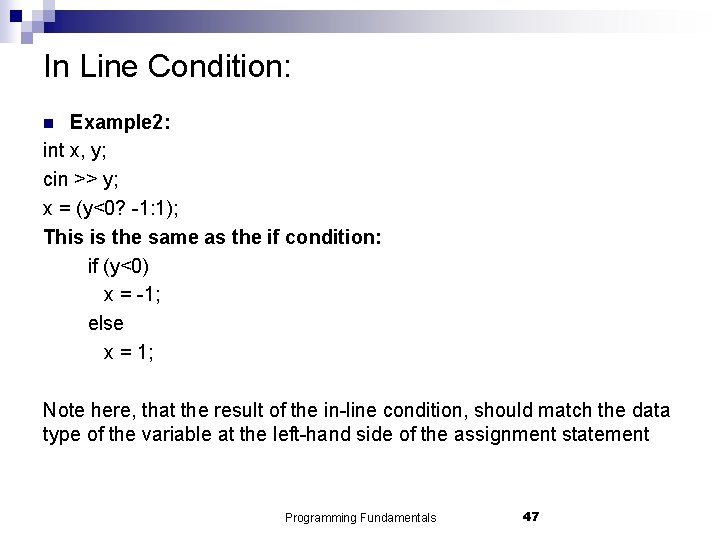
In Line Condition: Example 2: int x, y; cin >> y; x = (y<0? -1: 1); This is the same as the if condition: if (y<0) x = -1; else x = 1; n Note here, that the result of the in-line condition, should match the data type of the variable at the left-hand side of the assignment statement Programming Fundamentals 47
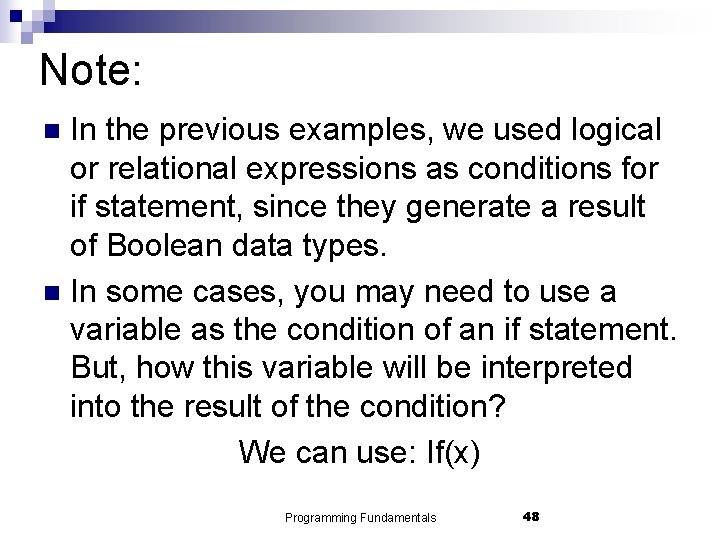
Note: In the previous examples, we used logical or relational expressions as conditions for if statement, since they generate a result of Boolean data types. n In some cases, you may need to use a variable as the condition of an if statement. But, how this variable will be interpreted into the result of the condition? We can use: If(x) n Programming Fundamentals 48
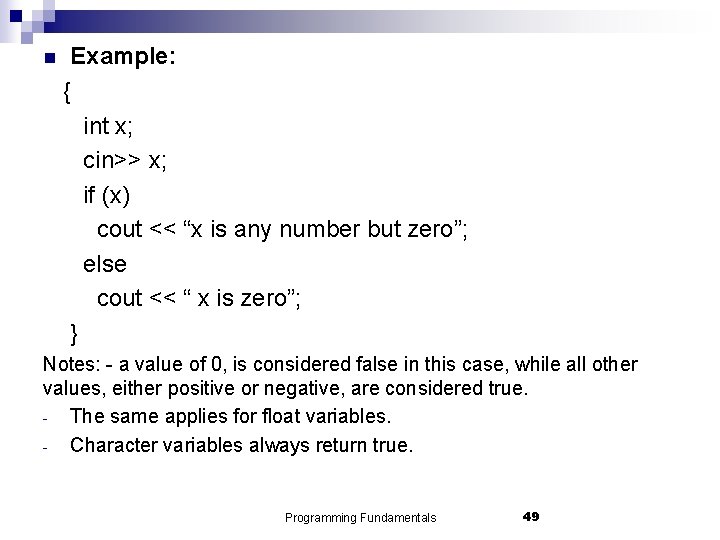
n Example: { int x; cin>> x; if (x) cout << “x is any number but zero”; else cout << “ x is zero”; } Notes: - a value of 0, is considered false in this case, while all other values, either positive or negative, are considered true. - The same applies for float variables. - Character variables always return true. Programming Fundamentals 49
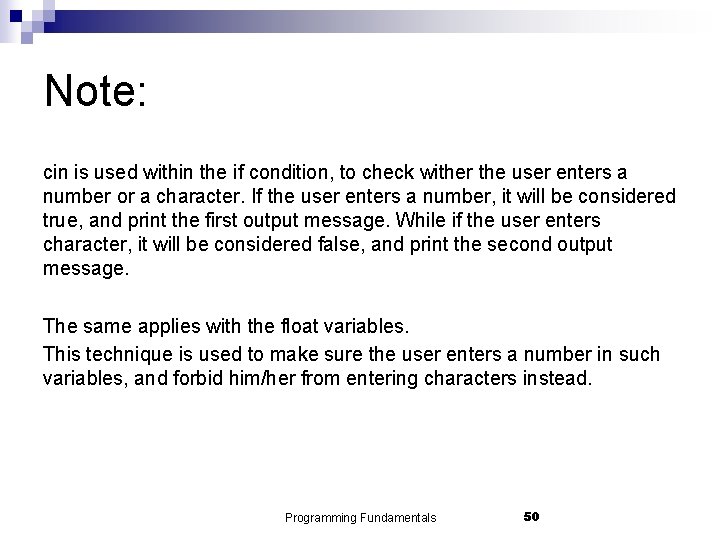
Note: cin is used within the if condition, to check wither the user enters a number or a character. If the user enters a number, it will be considered true, and print the first output message. While if the user enters character, it will be considered false, and print the second output message. The same applies with the float variables. This technique is used to make sure the user enters a number in such variables, and forbid him/her from entering characters instead. Programming Fundamentals 50
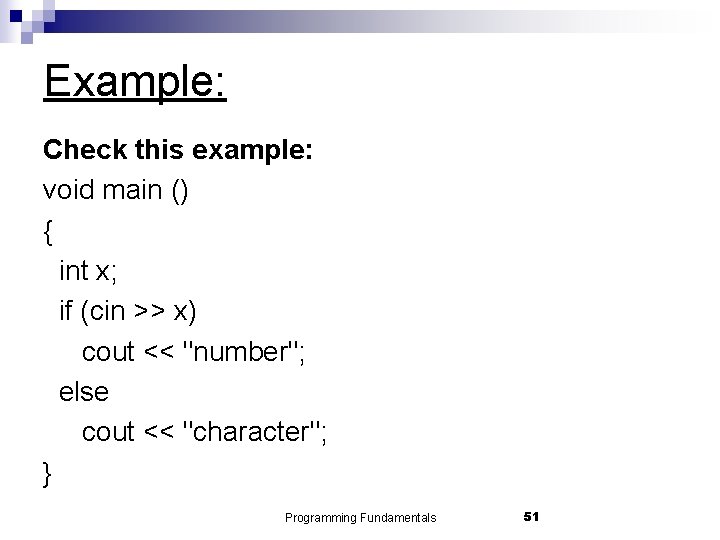
Example: Check this example: void main () { int x; if (cin >> x) cout << "number"; else cout << "character"; } Programming Fundamentals 51
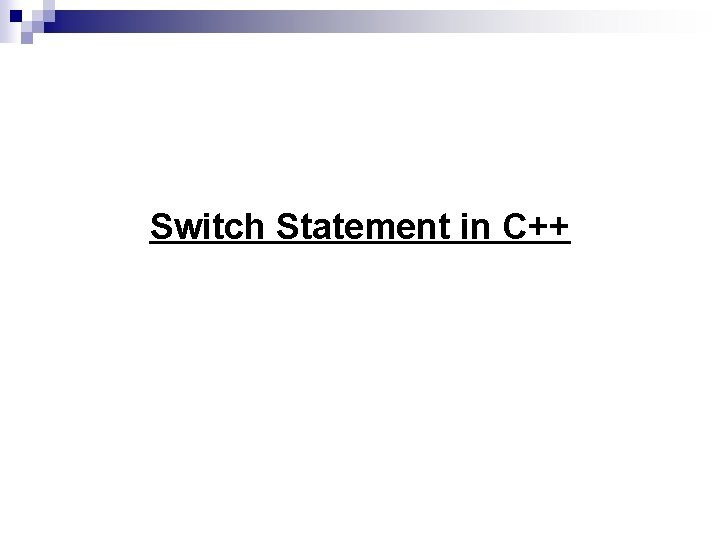
Switch Statement in C++
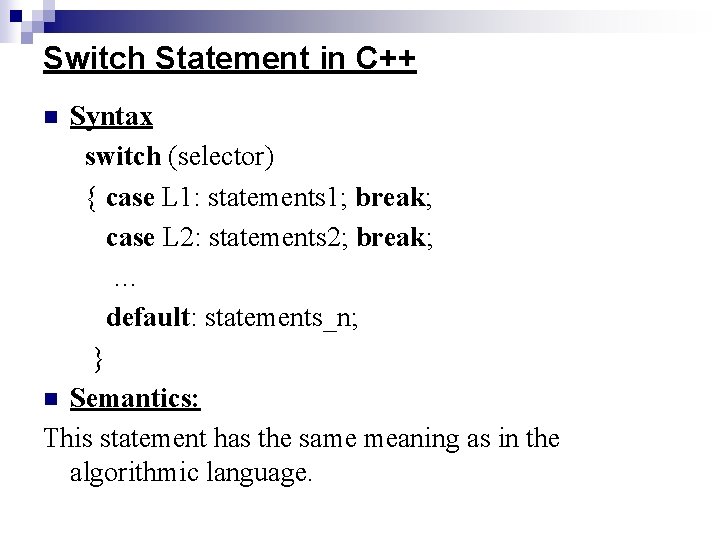
Switch Statement in C++ Syntax switch (selector) { case L 1: statements 1; break; case L 2: statements 2; break; … default: statements_n; } n Semantics: This statement has the same meaning as in the algorithmic language. n
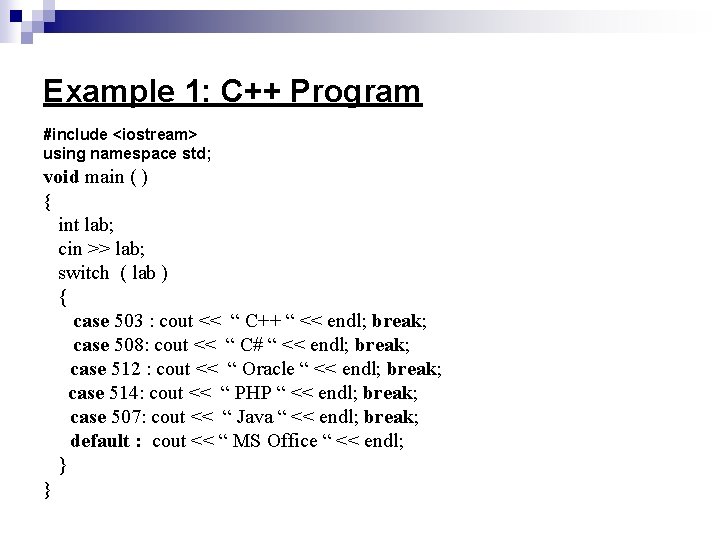
Example 1: C++ Program #include <iostream> using namespace std; void main ( ) { int lab; cin >> lab; switch ( lab ) { case 503 : cout << “ C++ “ << endl; break; case 508: cout << “ C# “ << endl; break; case 512 : cout << “ Oracle “ << endl; break; case 514: cout << “ PHP “ << endl; break; case 507: cout << “ Java “ << endl; break; default : cout << “ MS Office “ << endl; } }
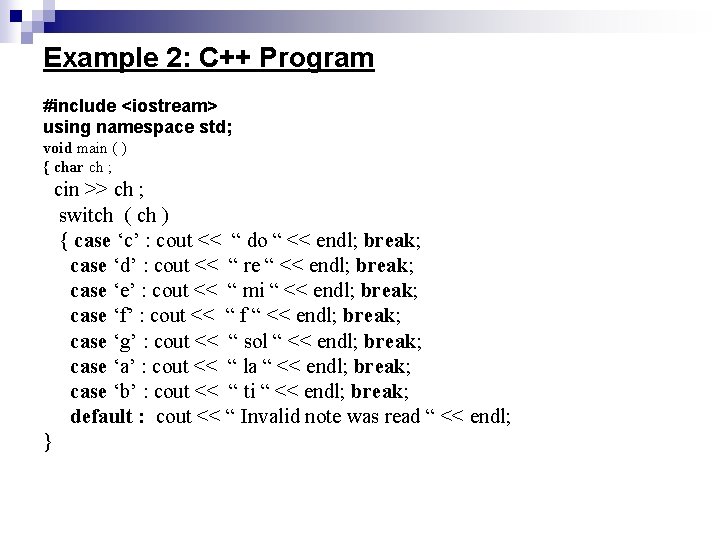
Example 2: C++ Program #include <iostream> using namespace std; void main ( ) { char ch ; cin >> ch ; switch ( ch ) { case ‘c’ : cout << “ do “ << endl; break; case ‘d’ : cout << “ re “ << endl; break; case ‘e’ : cout << “ mi “ << endl; break; case ‘f’ : cout << “ f “ << endl; break; case ‘g’ : cout << “ sol “ << endl; break; case ‘a’ : cout << “ la “ << endl; break; case ‘b’ : cout << “ ti “ << endl; break; default : cout << “ Invalid note was read “ << endl; }
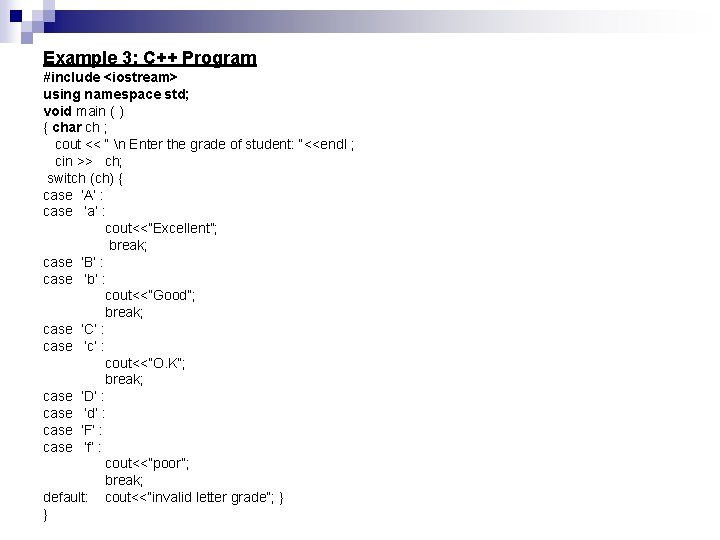
Example 3: C++ Program #include <iostream> using namespace std; void main ( ) { char ch ; cout << “ n Enter the grade of student: “<<endl ; cin >> ch; switch (ch) { case ‘A’ : case ‘a’ : cout<<”Excellent”; break; case ‘B’ : case ‘b’ : cout<<”Good”; break; case ‘C’ : case ‘c’ : cout<<”O. K”; break; case ‘D’ : case ‘d’ : case ‘F’ : case ‘f’ : cout<<”poor”; break; default: cout<<”invalid letter grade”; } }
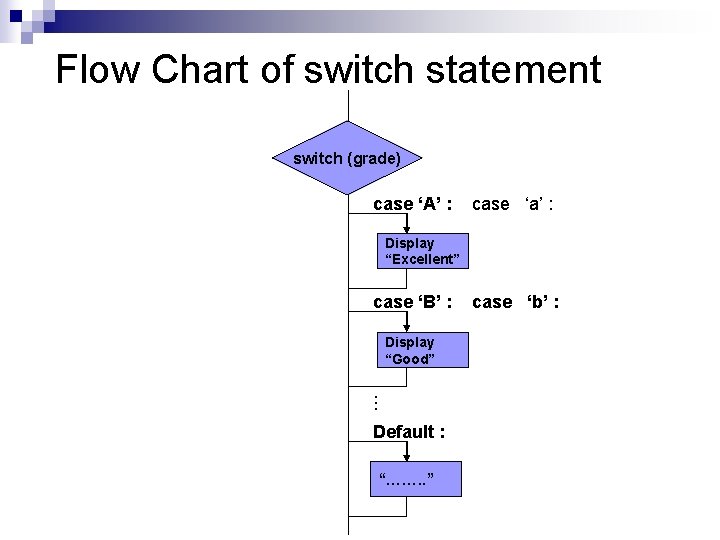
Flow Chart of switch statement switch (grade) case ‘A’ : case ‘a’ : Display “Excellent” case ‘B’ : Display “Good” … Default : “……. . ” case ‘b’ :
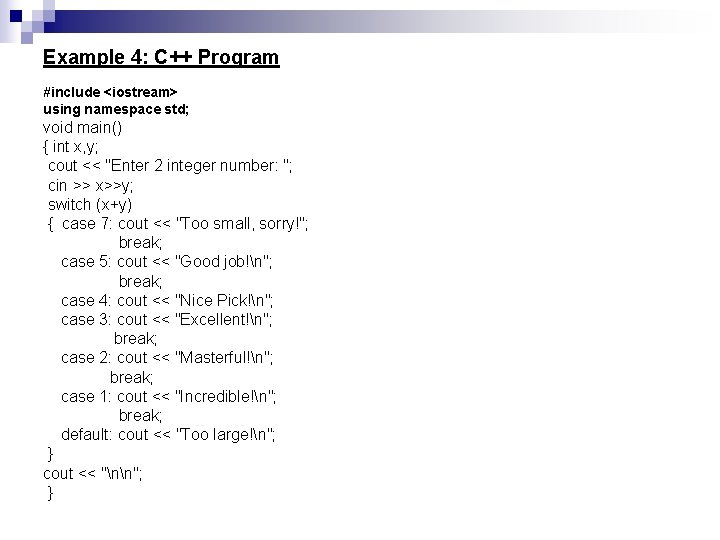
Example 4: C++ Program #include <iostream> using namespace std; void main() { int x, y; cout << "Enter 2 integer number: "; cin >> x>>y; switch (x+y) { case 7: cout << "Too small, sorry!"; break; case 5: cout << "Good job!n"; break; case 4: cout << "Nice Pick!n"; case 3: cout << "Excellent!n"; break; case 2: cout << "Masterful!n"; break; case 1: cout << "Incredible!n"; break; default: cout << "Too large!n"; } cout << "nn"; }
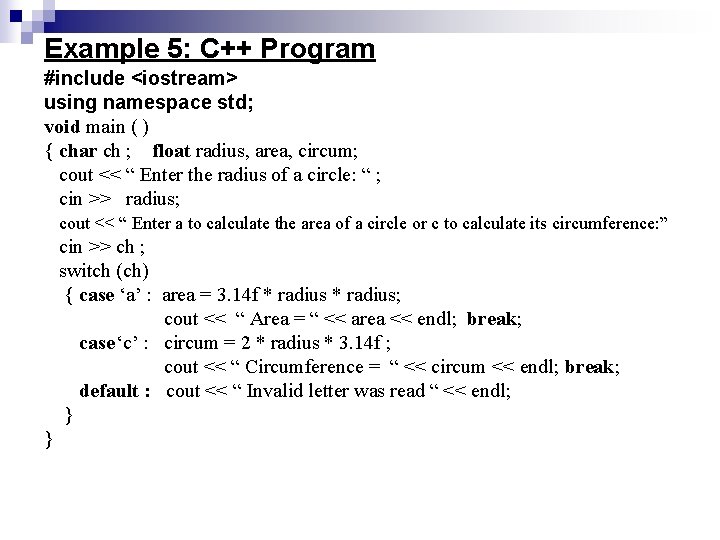
Example 5: C++ Program #include <iostream> using namespace std; void main ( ) { char ch ; float radius, area, circum; cout << “ Enter the radius of a circle: “ ; cin >> radius; cout << “ Enter a to calculate the area of a circle or c to calculate its circumference: ” cin >> ch ; switch (ch) { case ‘a’ : area = 3. 14 f * radius; cout << “ Area = “ << area << endl; break; case‘c’ : circum = 2 * radius * 3. 14 f ; cout << “ Circumference = “ << circum << endl; break; default : cout << “ Invalid letter was read “ << endl; } }
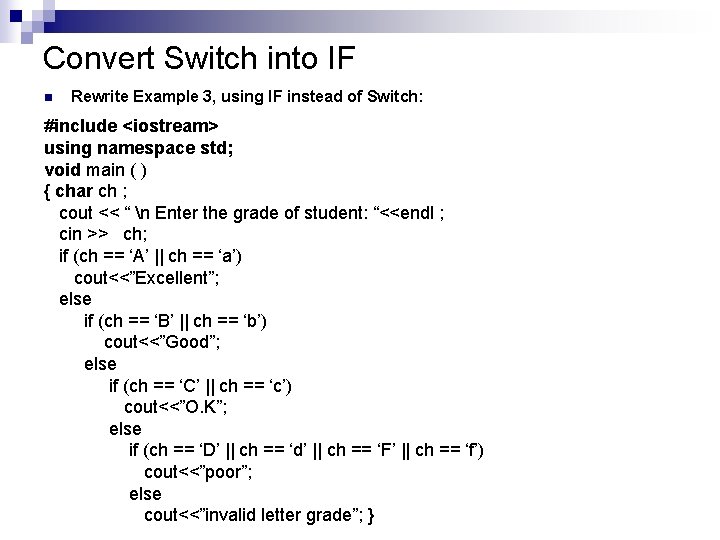
Convert Switch into IF n Rewrite Example 3, using IF instead of Switch: #include <iostream> using namespace std; void main ( ) { char ch ; cout << “ n Enter the grade of student: “<<endl ; cin >> ch; if (ch == ‘A’ || ch == ‘a’) cout<<”Excellent”; else if (ch == ‘B’ || ch == ‘b’) cout<<”Good”; else if (ch == ‘C’ || ch == ‘c’) cout<<”O. K”; else if (ch == ‘D’ || ch == ‘d’ || ch == ‘F’ || ch == ‘f’) cout<<”poor”; else cout<<”invalid letter grade”; }
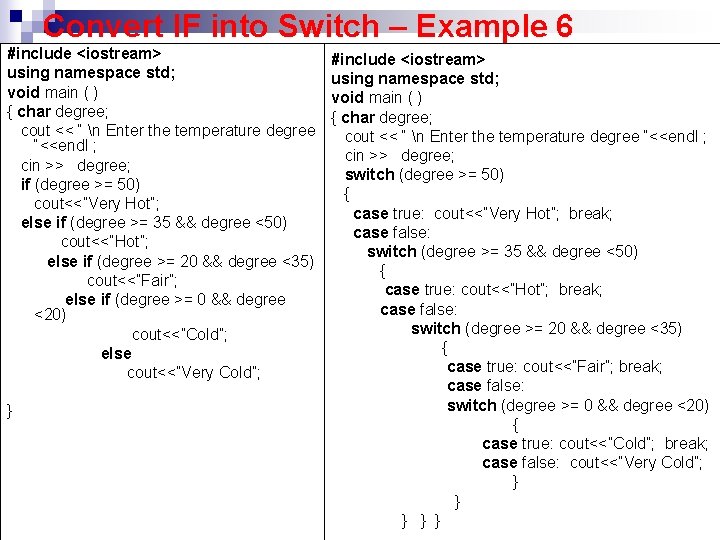
Convert IF into Switch – Example 6 #include <iostream> using namespace std; void main ( ) { char degree; cout << “ n Enter the temperature degree “<<endl ; cin >> degree; if (degree >= 50) cout<<”Very Hot”; else if (degree >= 35 && degree <50) cout<<”Hot”; else if (degree >= 20 && degree <35) cout<<”Fair”; else if (degree >= 0 && degree <20) cout<<”Cold”; else cout<<”Very Cold”; } #include <iostream> using namespace std; void main ( ) { char degree; cout << “ n Enter the temperature degree “<<endl ; cin >> degree; switch (degree >= 50) { case true: cout<<”Very Hot”; break; case false: switch (degree >= 35 && degree <50) { case true: cout<<”Hot”; break; case false: switch (degree >= 20 && degree <35) { case true: cout<<”Fair”; break; case false: switch (degree >= 0 && degree <20) { case true: cout<<”Cold”; break; case false: cout<<”Very Cold”; } } }