Constructor overloading Destructor Function Overloading 1 Constructor overloading
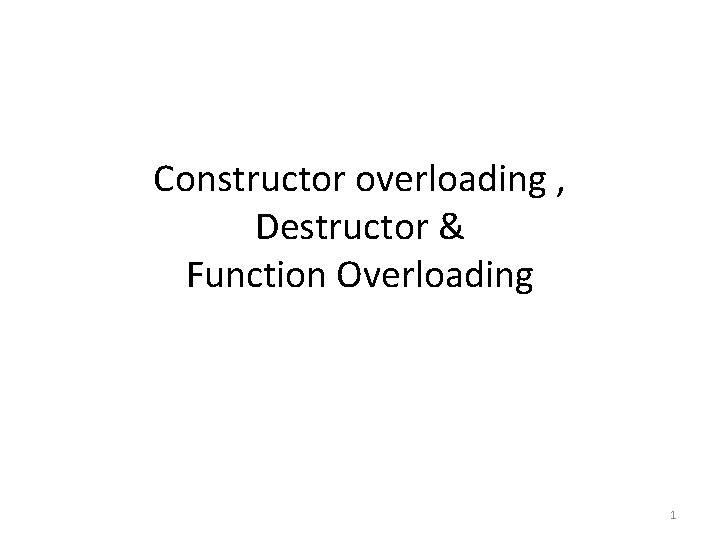
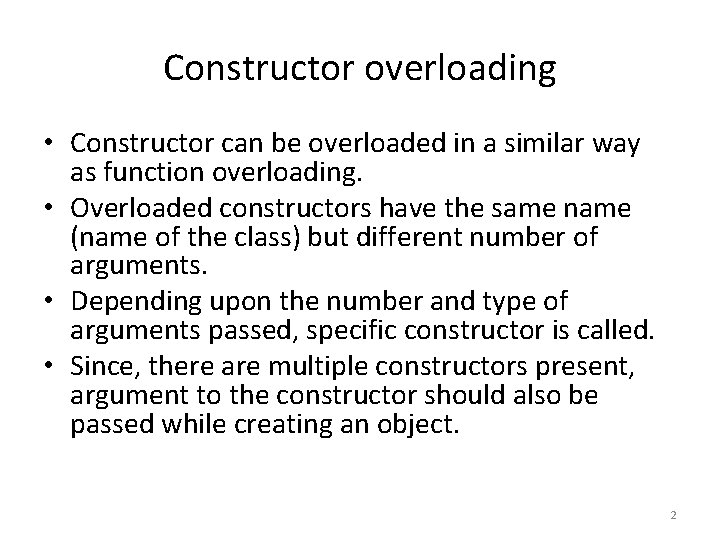
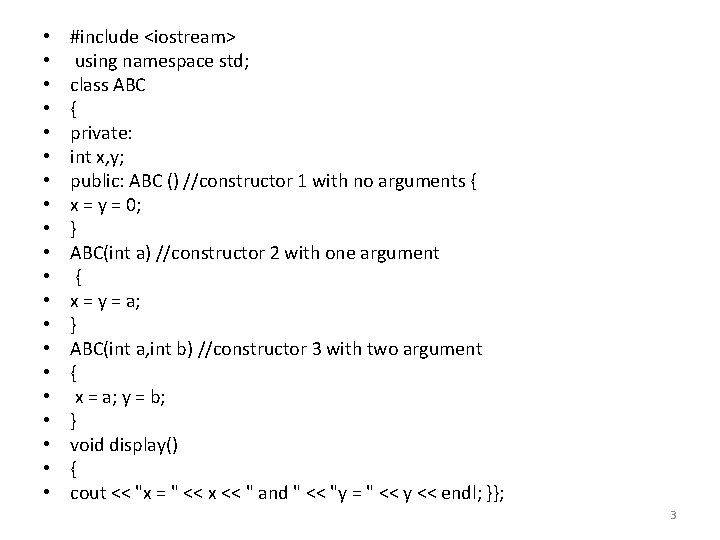
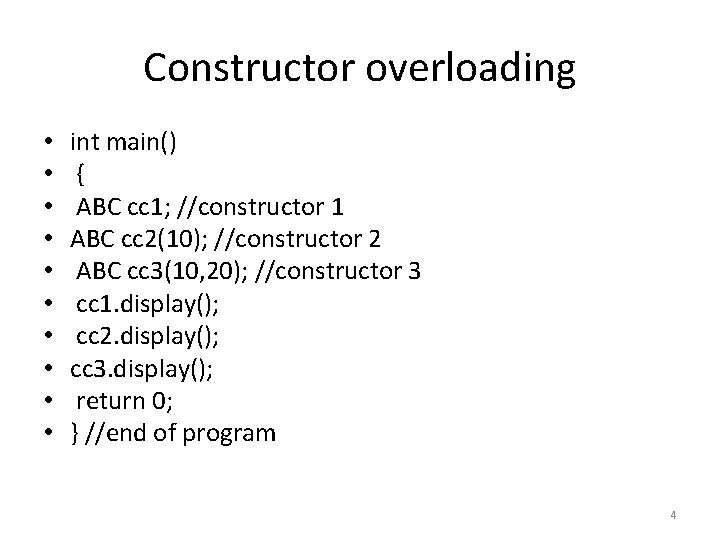
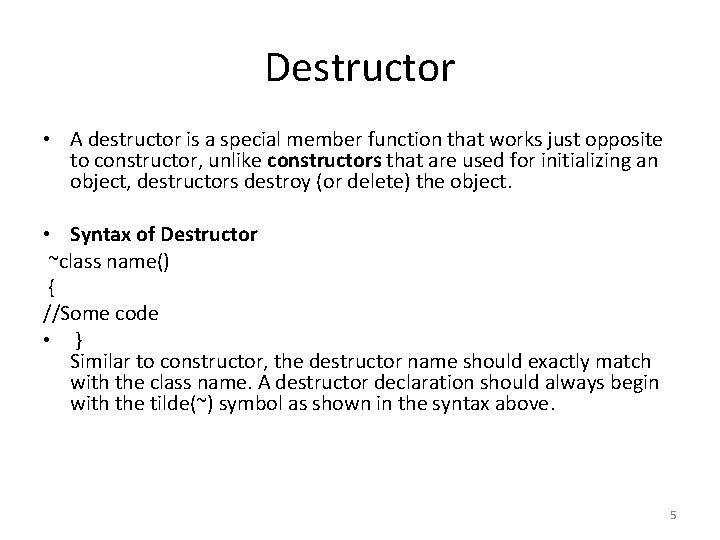
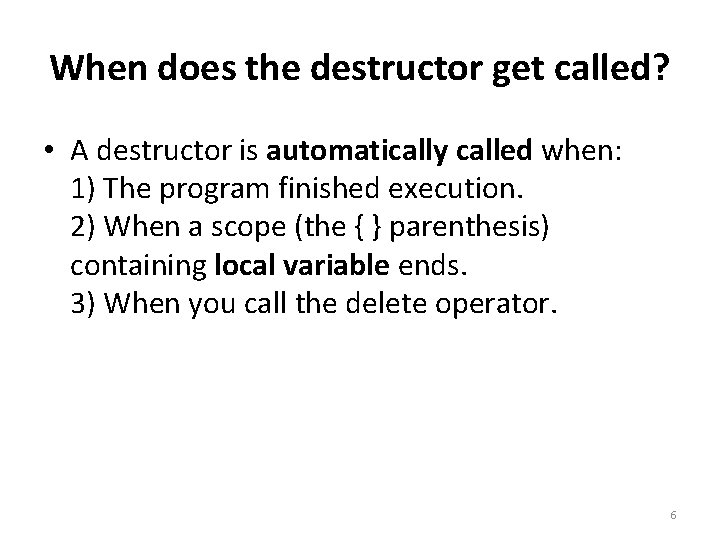
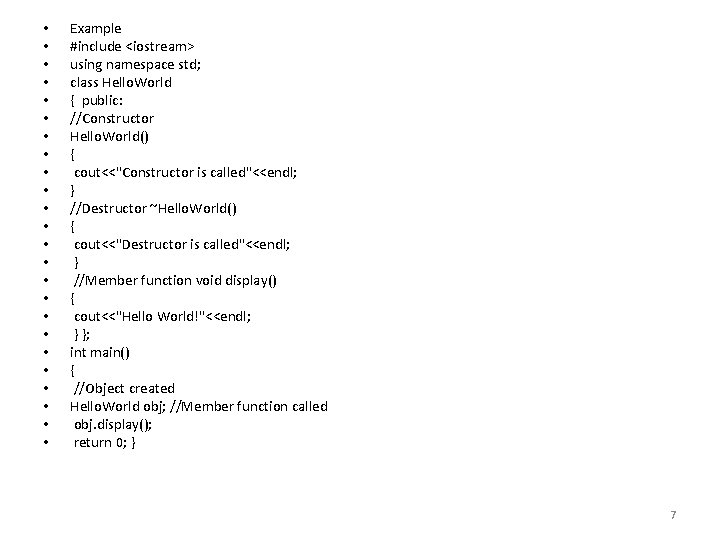
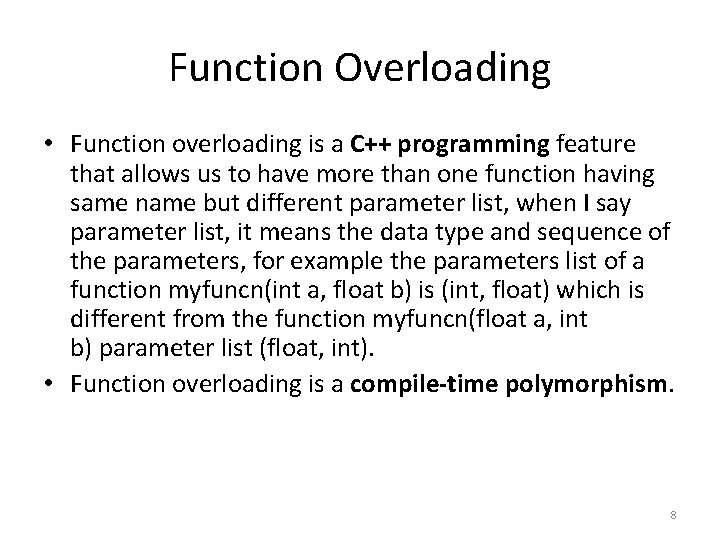
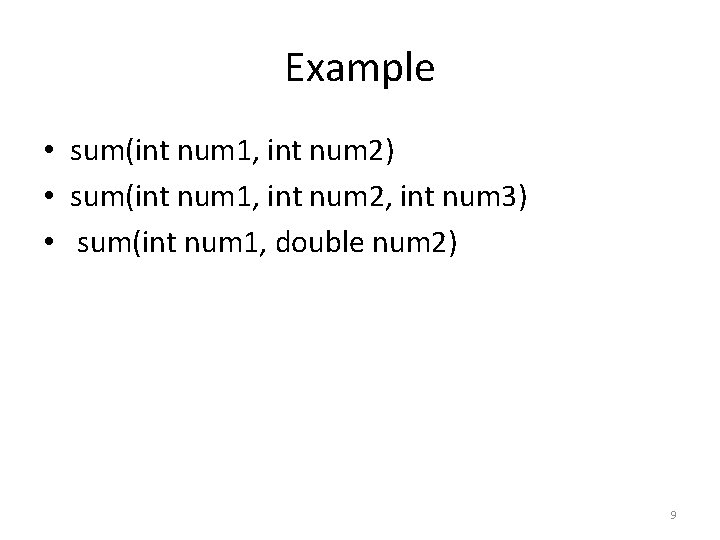
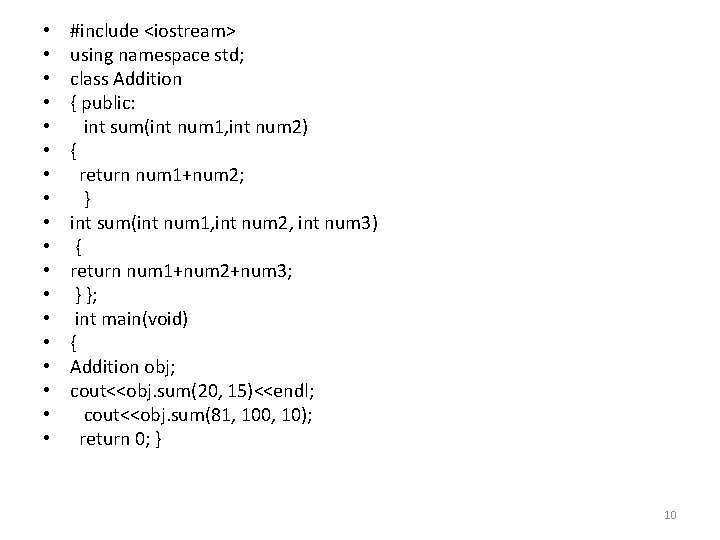
- Slides: 10
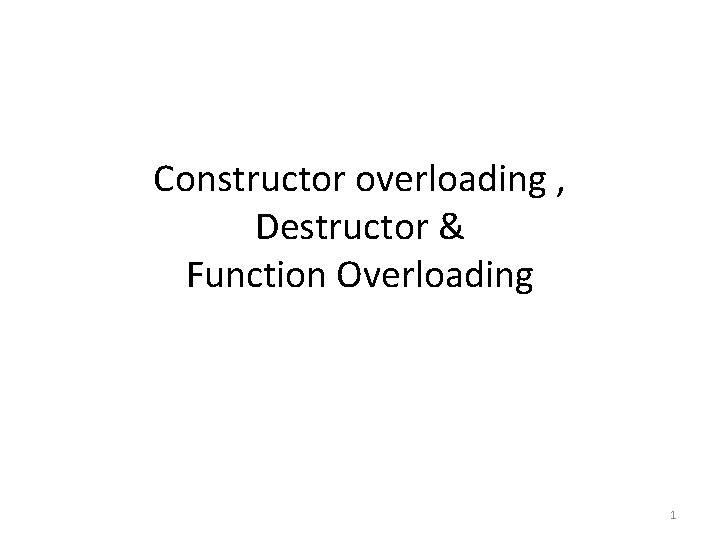
Constructor overloading , Destructor & Function Overloading 1
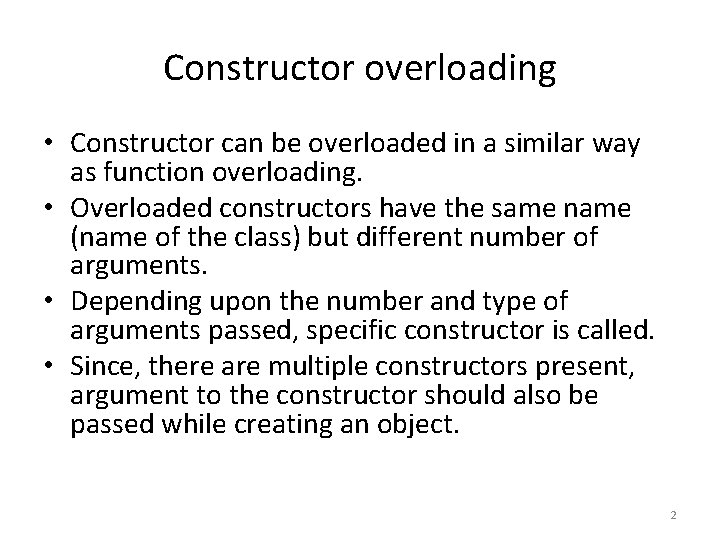
Constructor overloading • Constructor can be overloaded in a similar way as function overloading. • Overloaded constructors have the same name (name of the class) but different number of arguments. • Depending upon the number and type of arguments passed, specific constructor is called. • Since, there are multiple constructors present, argument to the constructor should also be passed while creating an object. 2
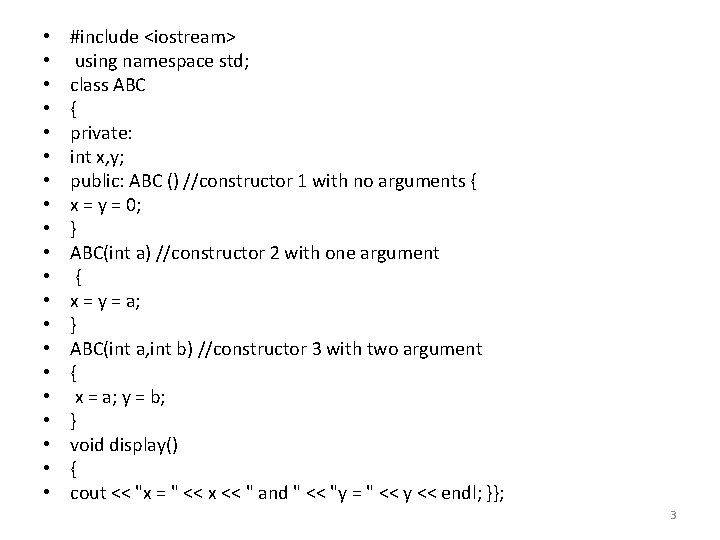
• • • • • #include <iostream> using namespace std; class ABC { private: int x, y; public: ABC () //constructor 1 with no arguments { x = y = 0; } ABC(int a) //constructor 2 with one argument { x = y = a; } ABC(int a, int b) //constructor 3 with two argument { x = a; y = b; } void display() { cout << "x = " << x << " and " << "y = " << y << endl; }}; 3
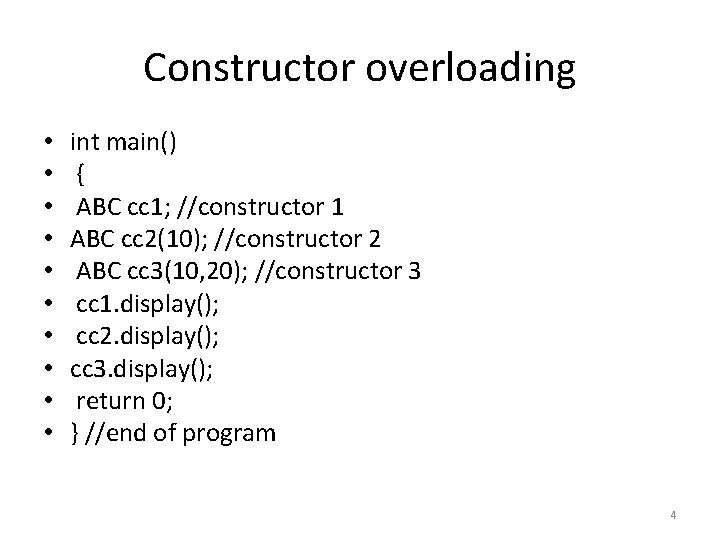
Constructor overloading • • • int main() { ABC cc 1; //constructor 1 ABC cc 2(10); //constructor 2 ABC cc 3(10, 20); //constructor 3 cc 1. display(); cc 2. display(); cc 3. display(); return 0; } //end of program 4
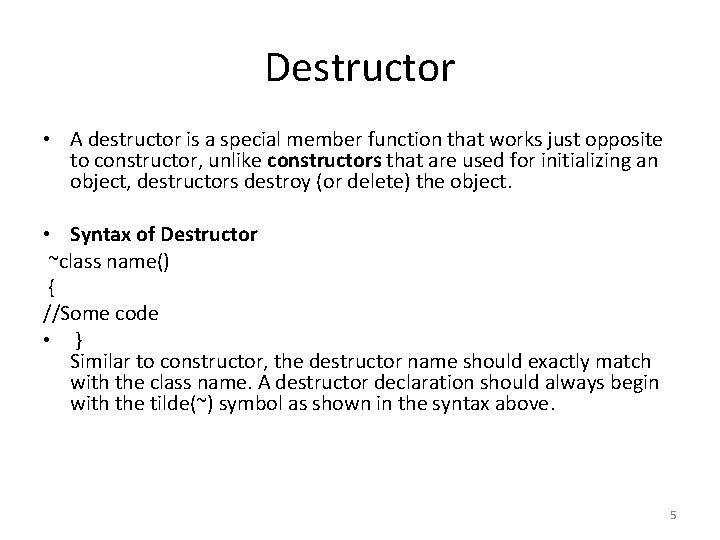
Destructor • A destructor is a special member function that works just opposite to constructor, unlike constructors that are used for initializing an object, destructors destroy (or delete) the object. • Syntax of Destructor ~class name() { //Some code • } Similar to constructor, the destructor name should exactly match with the class name. A destructor declaration should always begin with the tilde(~) symbol as shown in the syntax above. 5
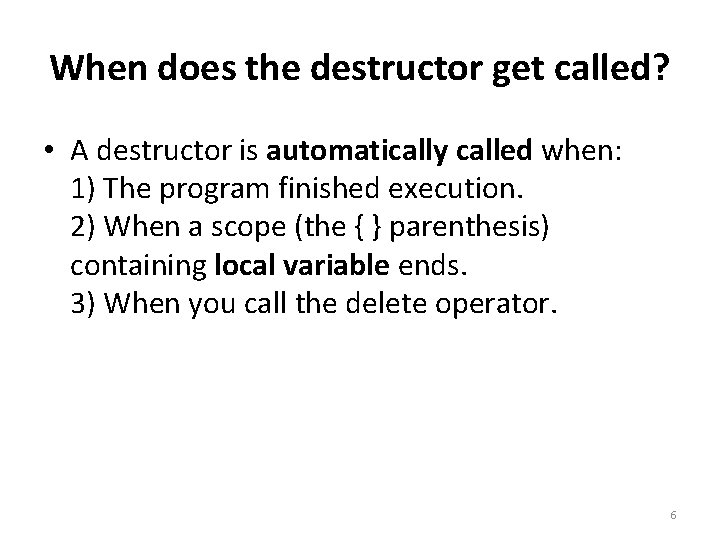
When does the destructor get called? • A destructor is automatically called when: 1) The program finished execution. 2) When a scope (the { } parenthesis) containing local variable ends. 3) When you call the delete operator. 6
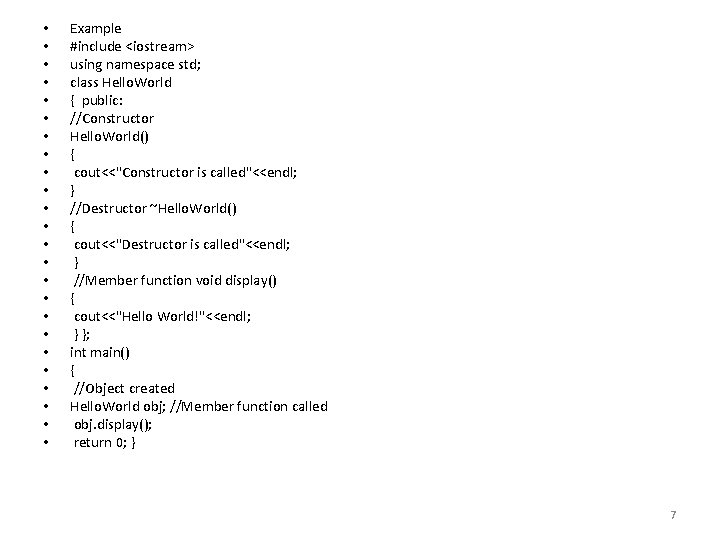
• • • • • • Example #include <iostream> using namespace std; class Hello. World { public: //Constructor Hello. World() { cout<<"Constructor is called"<<endl; } //Destructor ~Hello. World() { cout<<"Destructor is called"<<endl; } //Member function void display() { cout<<"Hello World!"<<endl; } }; int main() { //Object created Hello. World obj; //Member function called obj. display(); return 0; } 7
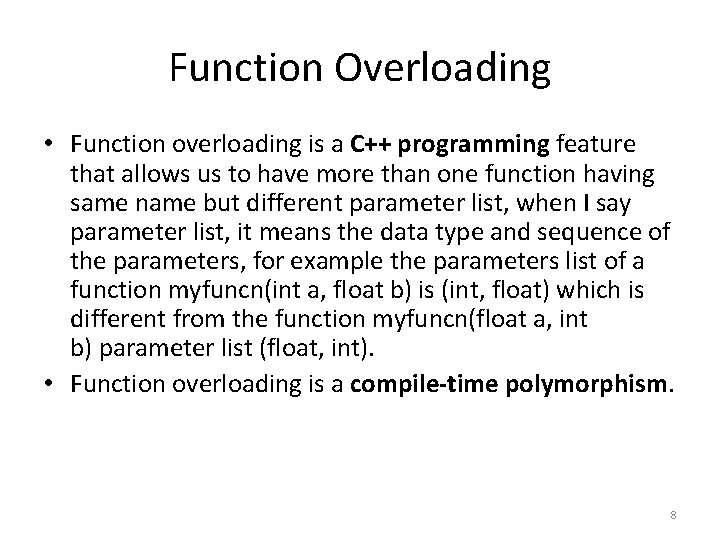
Function Overloading • Function overloading is a C++ programming feature that allows us to have more than one function having same name but different parameter list, when I say parameter list, it means the data type and sequence of the parameters, for example the parameters list of a function myfuncn(int a, float b) is (int, float) which is different from the function myfuncn(float a, int b) parameter list (float, int). • Function overloading is a compile-time polymorphism. 8
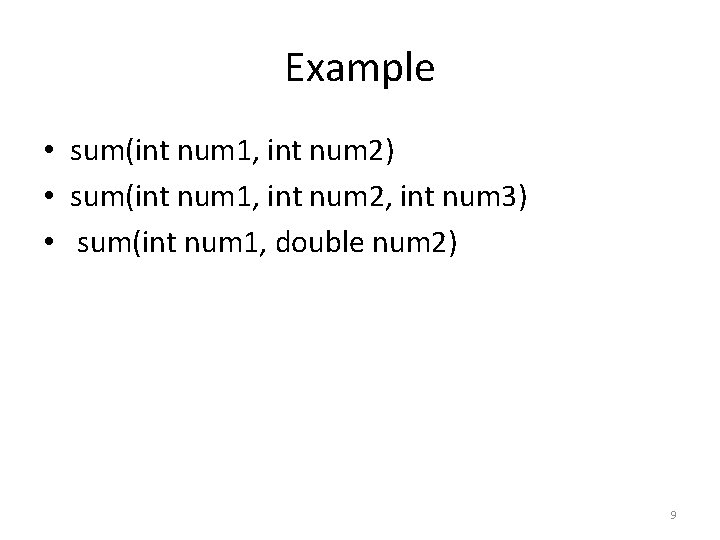
Example • sum(int num 1, int num 2) • sum(int num 1, int num 2, int num 3) • sum(int num 1, double num 2) 9
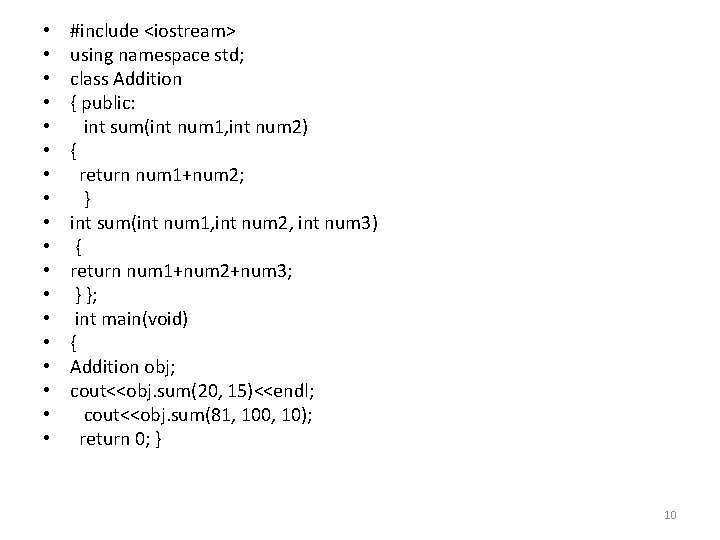
• • • • • #include <iostream> using namespace std; class Addition { public: int sum(int num 1, int num 2) { return num 1+num 2; } int sum(int num 1, int num 2, int num 3) { return num 1+num 2+num 3; } }; int main(void) { Addition obj; cout<<obj. sum(20, 15)<<endl; cout<<obj. sum(81, 100, 10); return 0; } 10
Constructor
What is a virtual function c++
Malik jahan khan
Aspidiotus destructor
Melolontinae
C# struct vs class
Has virtual functions and accessible non-virtual destructor
Destructor blas de lezo
How is function overloading different from template class
Binary operator overloading using friend function
Define function overloading