Classes Constructors and Overloading Overloading Constructor In Rectangle
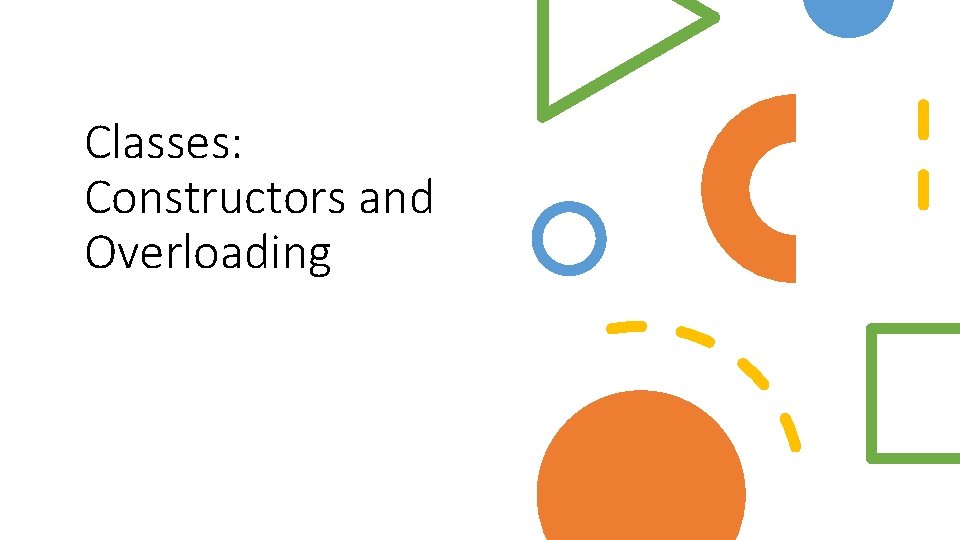
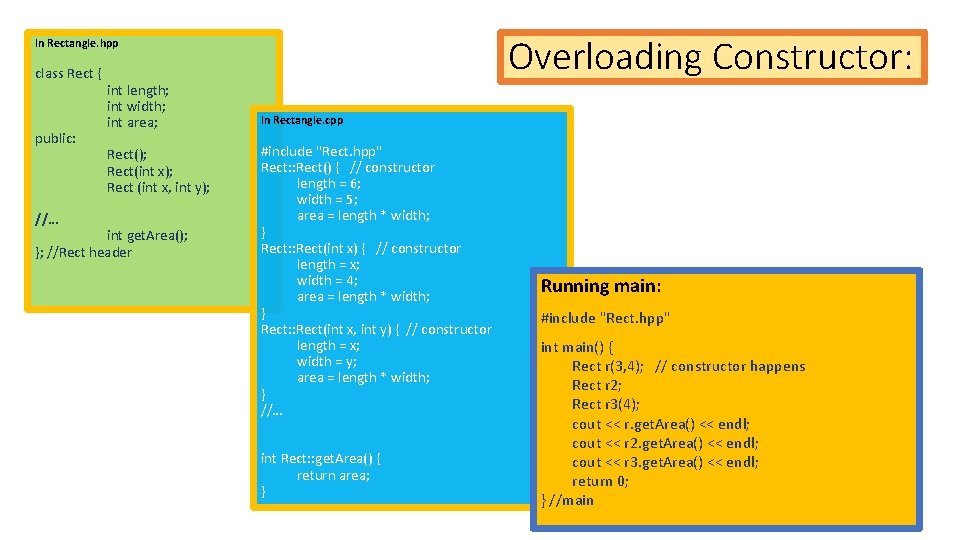
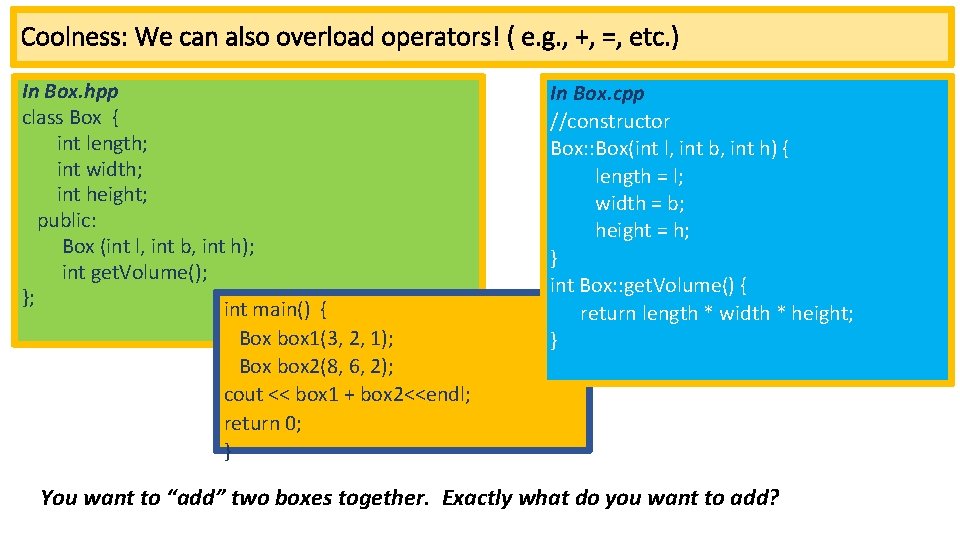
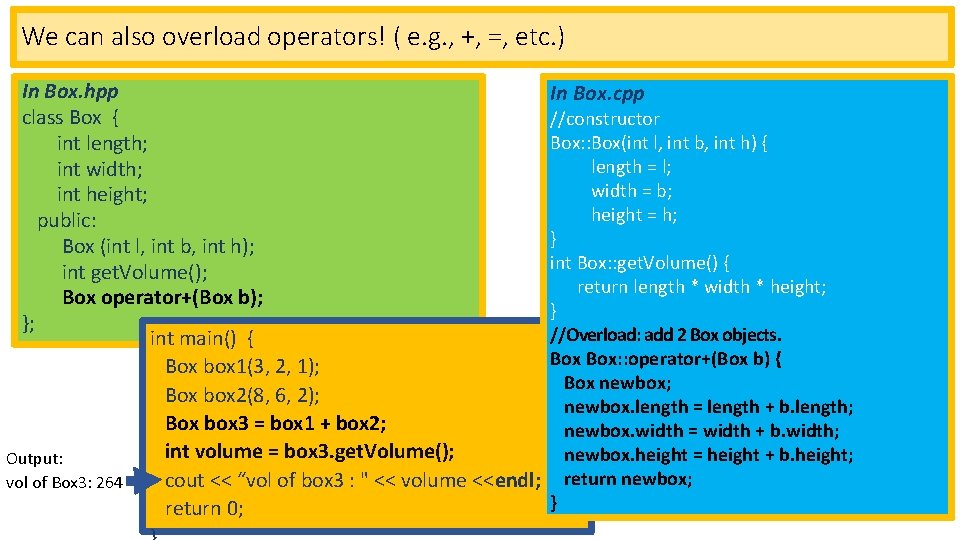
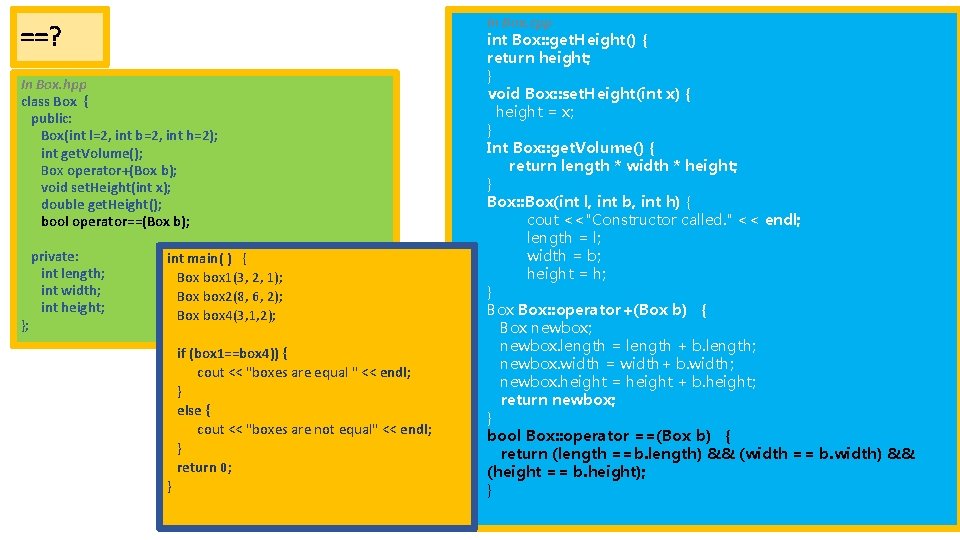
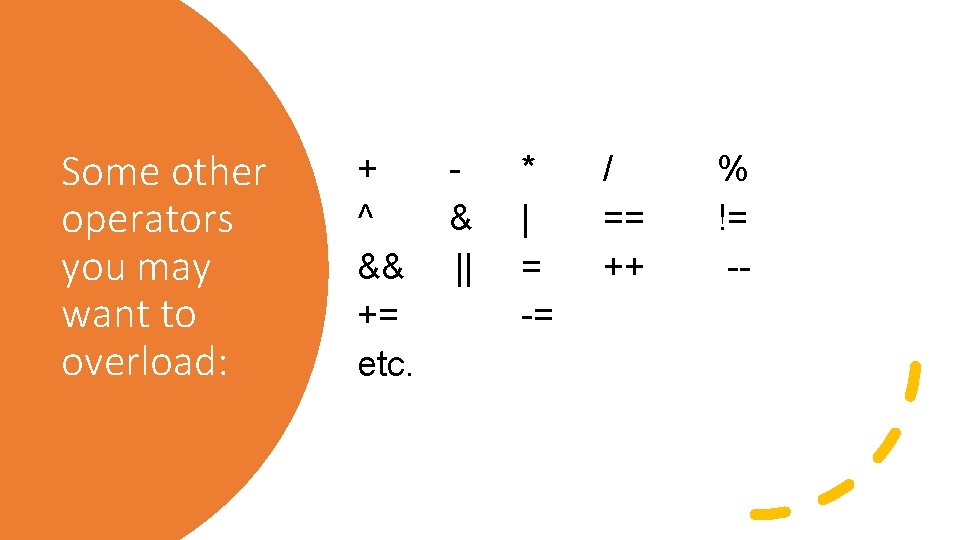
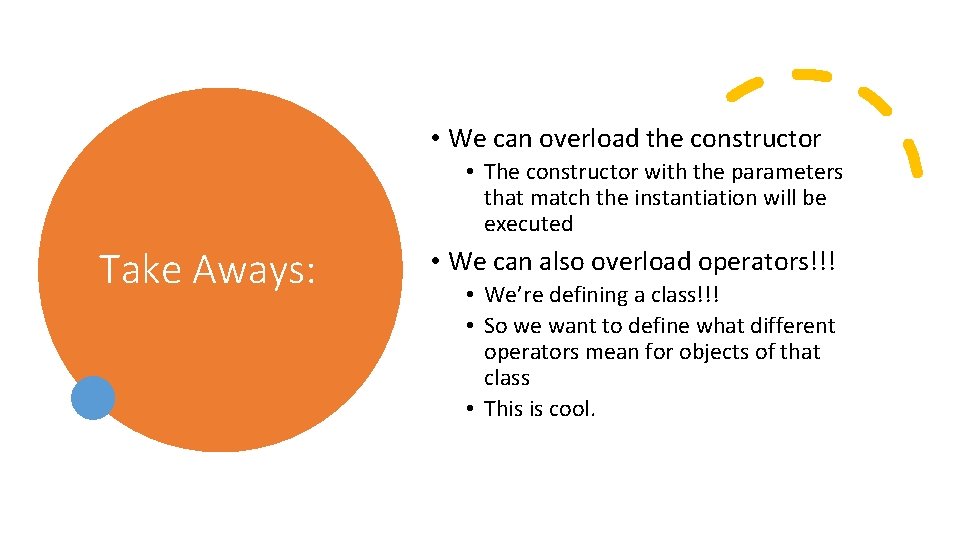
- Slides: 7
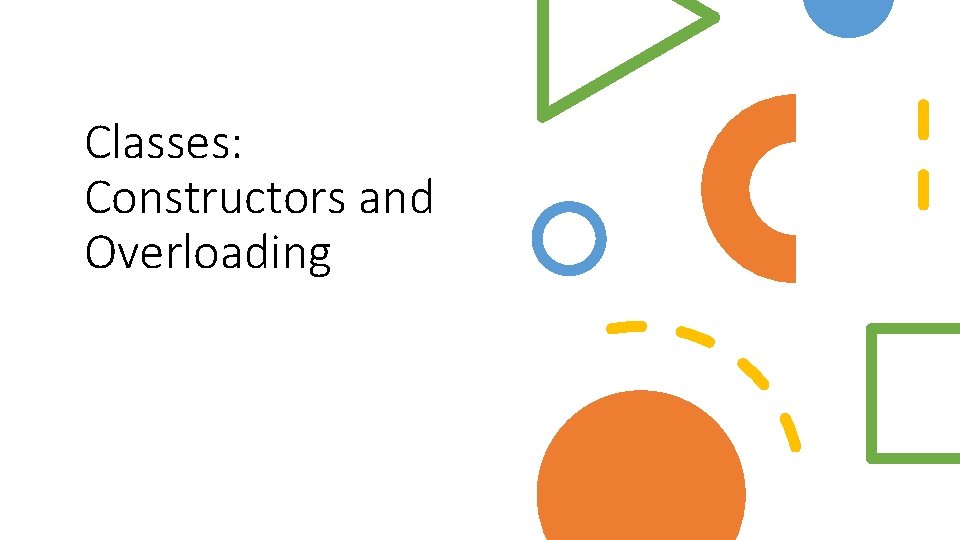
Classes: Constructors and Overloading
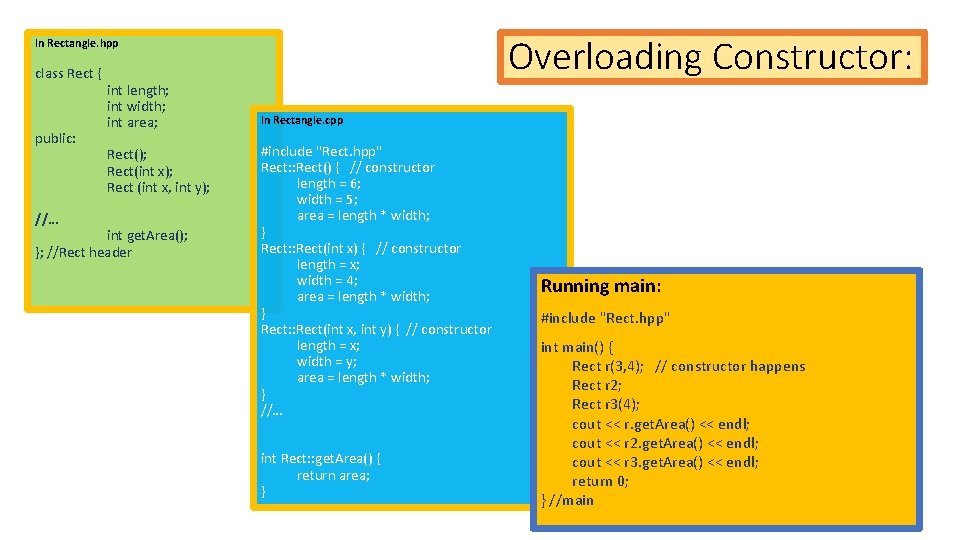
Overloading Constructor: In Rectangle. hpp class Rect { public: //… int length; int width; int area; Rect(); Rect(int x); Rect (int x, int y); int get. Area(); }; //Rect header In Rectangle. cpp #include "Rect. hpp" Rect: : Rect() { // constructor length = 6; width = 5; area = length * width; } Rect: : Rect(int x) { // constructor length = x; width = 4; area = length * width; } Rect: : Rect(int x, int y) { // constructor length = x; width = y; area = length * width; } //… int Rect: : get. Area() { return area; } Running main: #include "Rect. hpp" int main() { Rect r(3, 4); // constructor happens Rect r 2; Rect r 3(4); cout << r. get. Area() << endl; cout << r 2. get. Area() << endl; cout << r 3. get. Area() << endl; return 0; } //main
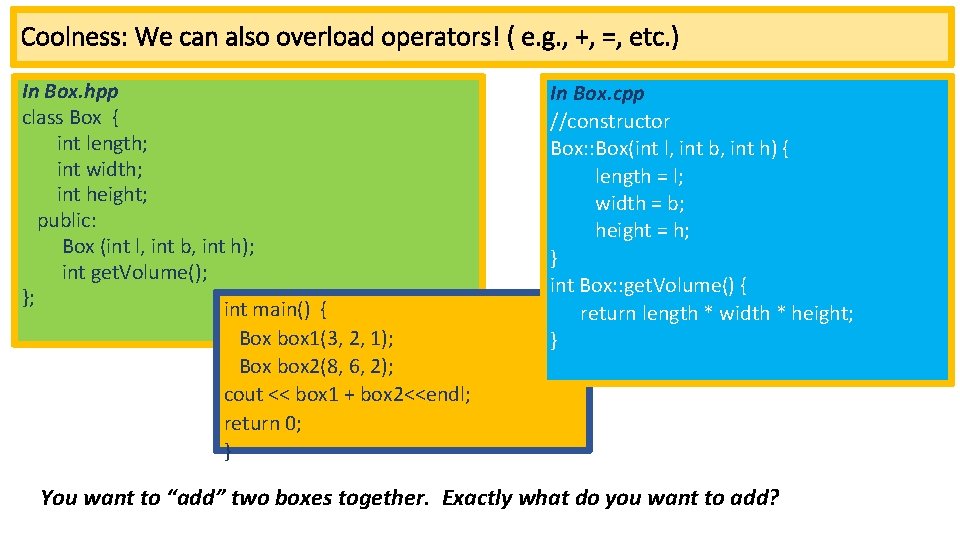
Coolness: We can also overload operators! ( e. g. , +, =, etc. ) In Box. hpp class Box { int length; int width; int height; public: Box (int l, int b, int h); int get. Volume(); }; int main() { Box box 1(3, 2, 1); Box box 2(8, 6, 2); cout << box 1 + box 2<<endl; return 0; } In Box. cpp //constructor Box: : Box(int l, int b, int h) { length = l; width = b; height = h; } int Box: : get. Volume() { return length * width * height; } You want to “add” two boxes together. Exactly what do you want to add?
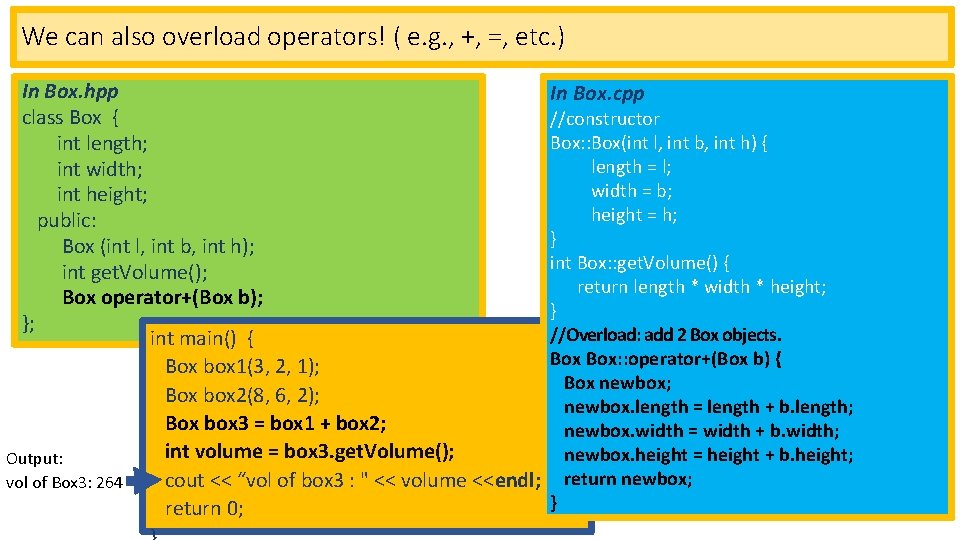
We can also overload operators! ( e. g. , +, =, etc. ) In Box. hpp class Box { int length; int width; int height; public: Box (int l, int b, int h); int get. Volume(); Box operator+(Box b); }; int main() { Box box 1(3, 2, 1); Box box 2(8, 6, 2); Box box 3 = box 1 + box 2; int volume = box 3. get. Volume(); Output: cout << “vol of box 3 : " << volume <<endl; vol of Box 3: 264 return 0; In Box. cpp //constructor Box: : Box(int l, int b, int h) { length = l; width = b; height = h; } int Box: : get. Volume() { return length * width * height; } //Overload: add 2 Box objects. Box: : operator+(Box b) { Box newbox; newbox. length = length + b. length; newbox. width = width + b. width; newbox. height = height + b. height; return newbox; }
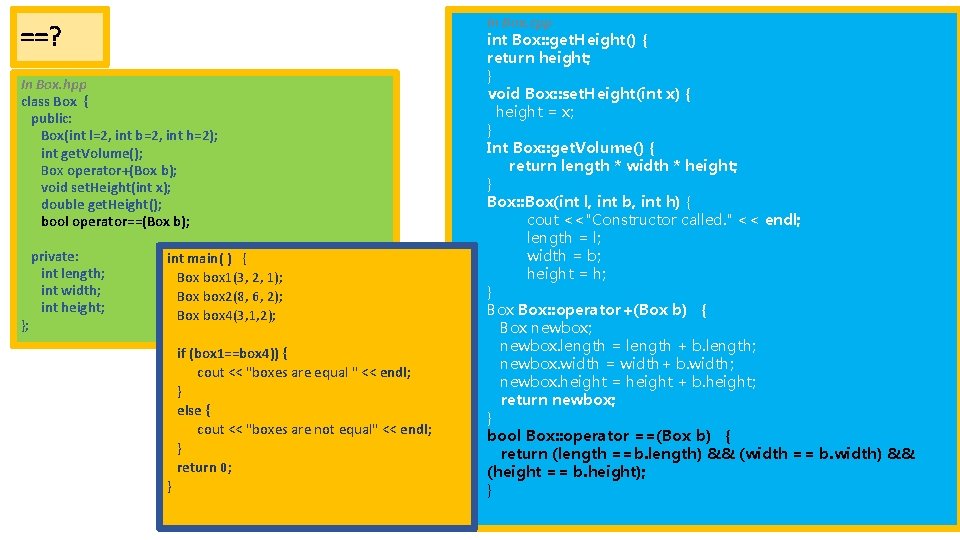
==? In Box. hpp class Box { public: Box(int l=2, int b=2, int h=2); int get. Volume(); Box operator+(Box b); void set. Height(int x); double get. Height(); bool operator==(Box b); }; private: int length; int width; int height; int main( ) { Box box 1(3, 2, 1); Box box 2(8, 6, 2); Box box 4(3, 1, 2); if (box 1==box 4)) { cout << "boxes are equal " << endl; } else { cout << "boxes are not equal" << endl; } return 0; } In Box. cpp int Box: : get. Height() { return height; } void Box: : set. Height(int x) { height = x; } Int Box: : get. Volume() { return length * width * height; } Box: : Box(int l, int b, int h) { cout <<"Constructor called. " << endl; length = l; width = b; height = h; } Box: : operator+(Box b) { Box newbox; newbox. length = length + b. length; newbox. width = width+ b. width; newbox. height = height + b. height; return newbox; } bool Box: : operator ==(Box b) { return (length ==b. length) && (width == b. width) && (height == b. height); }
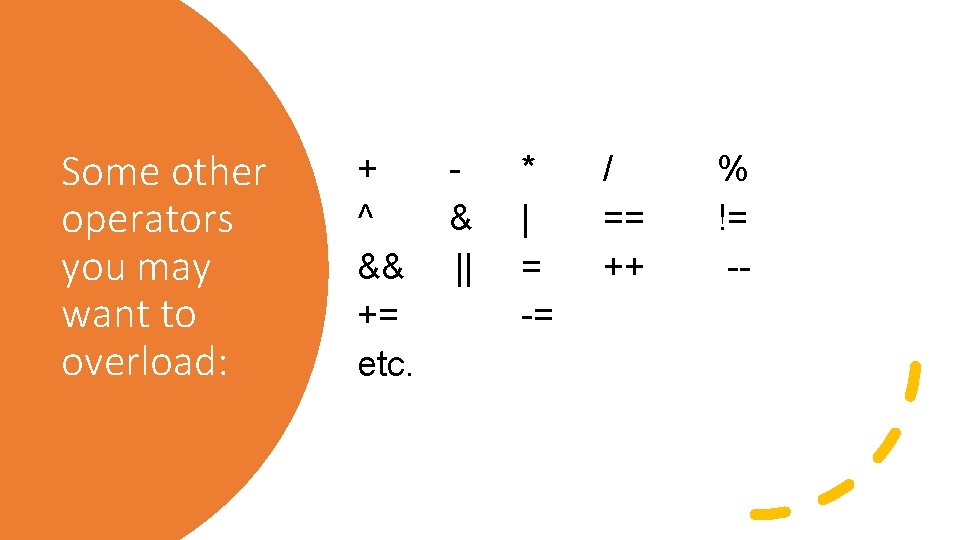
Some other operators you may want to overload: + ^ & && || += etc. * | = -= / == ++ % != --
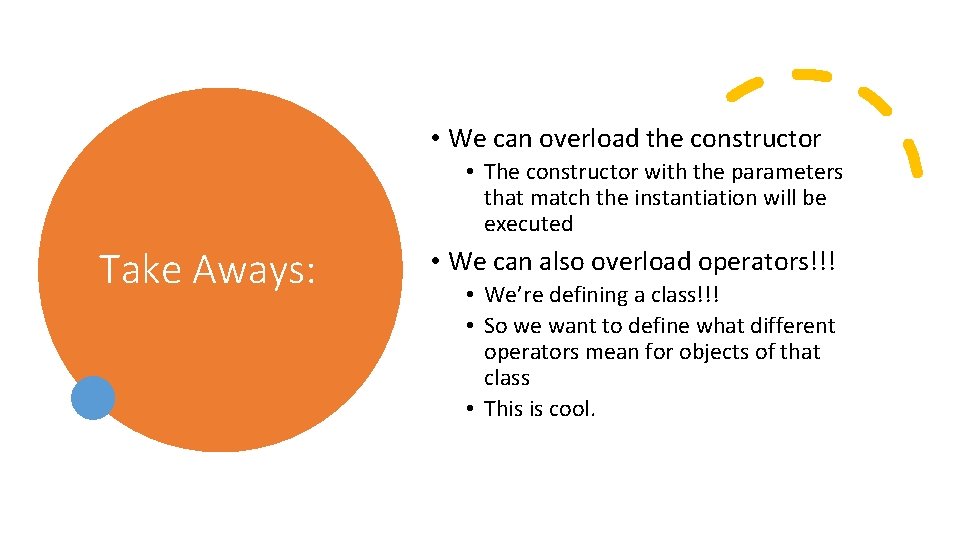
• We can overload the constructor • The constructor with the parameters that match the instantiation will be executed Take Aways: • We can also overload operators!!! • We’re defining a class!!! • So we want to define what different operators mean for objects of that class • This is cool.