Chapter No 3 Constructor Destructor Constructor Constructor is
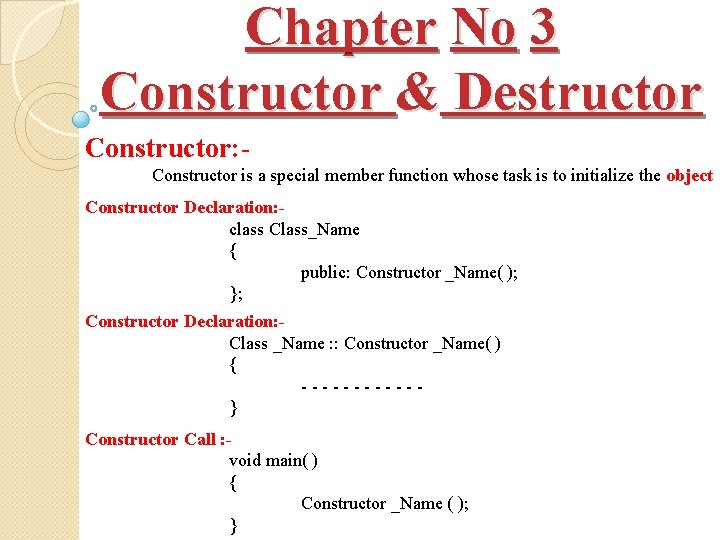
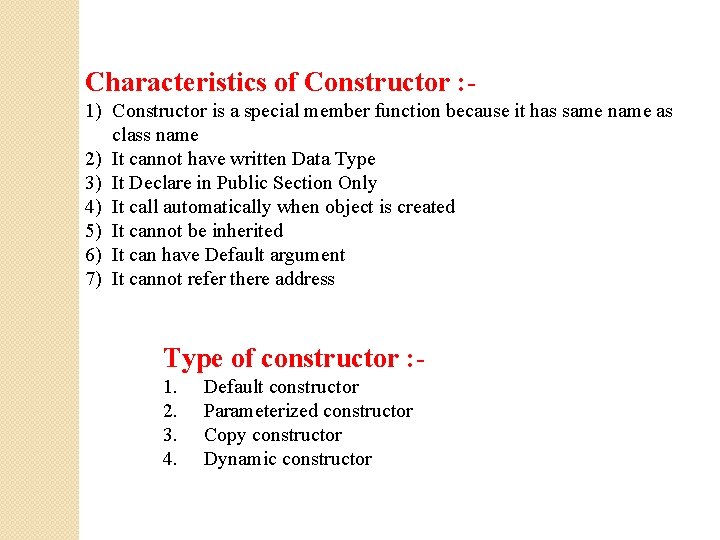
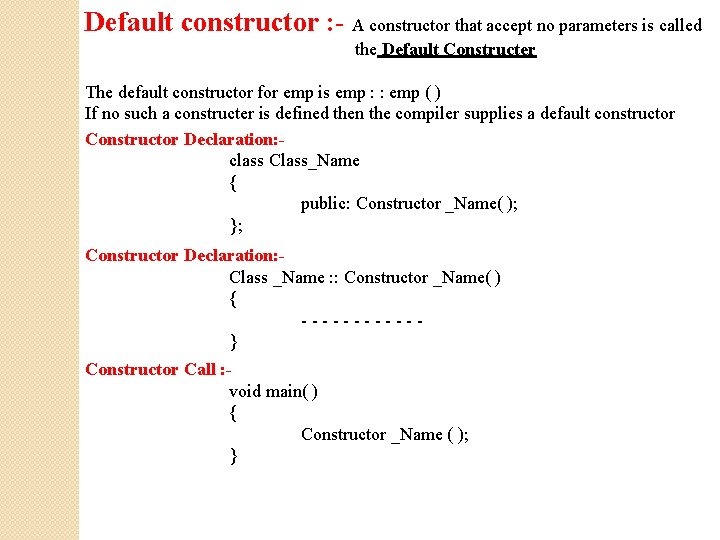
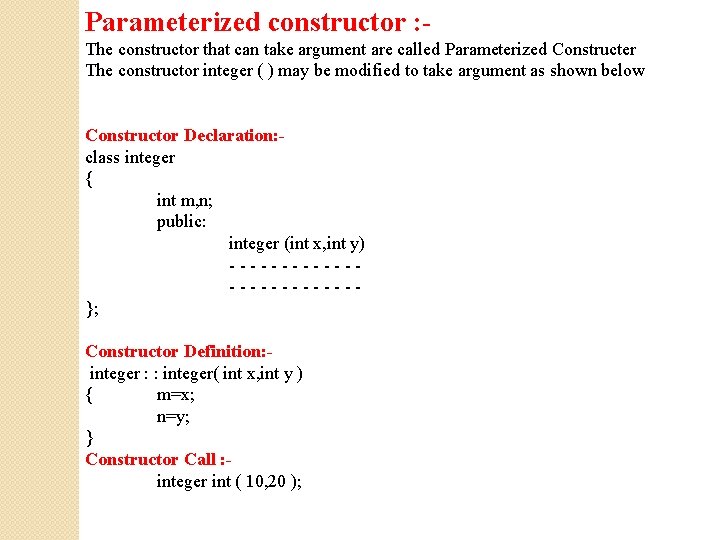
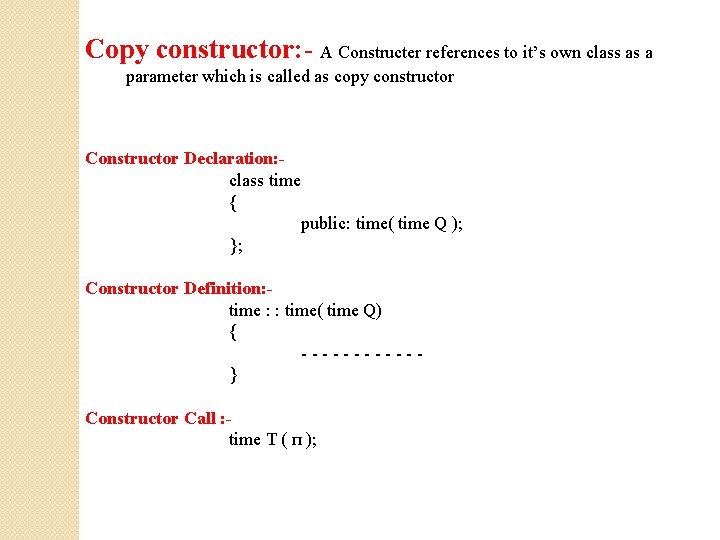
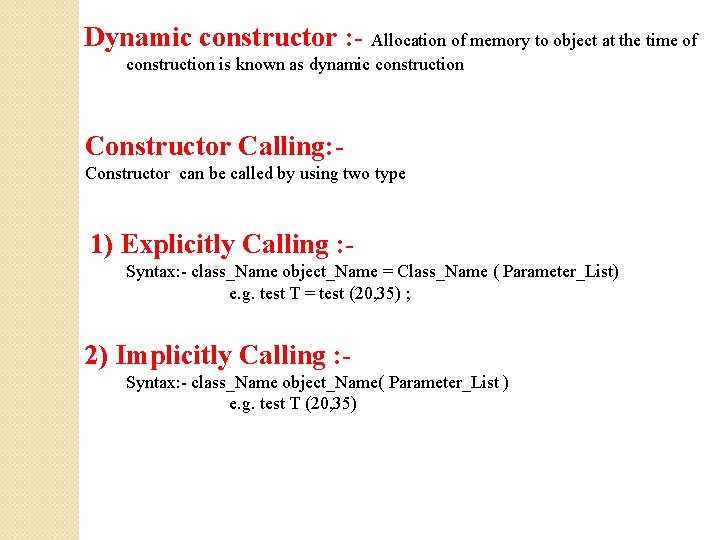
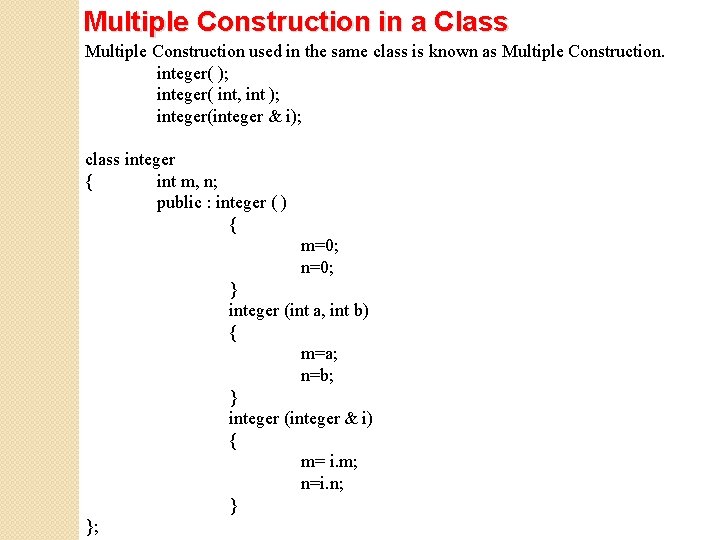
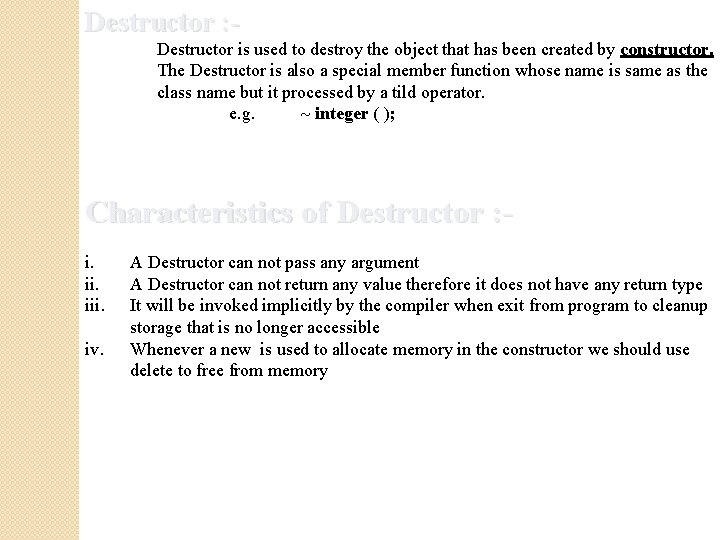
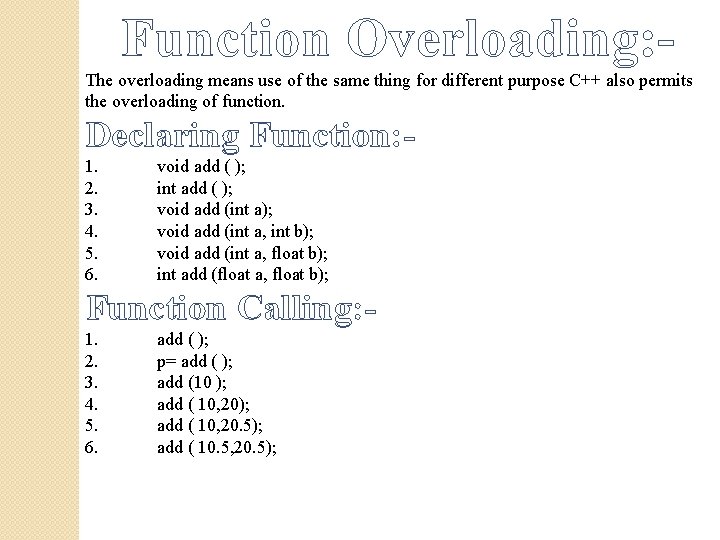
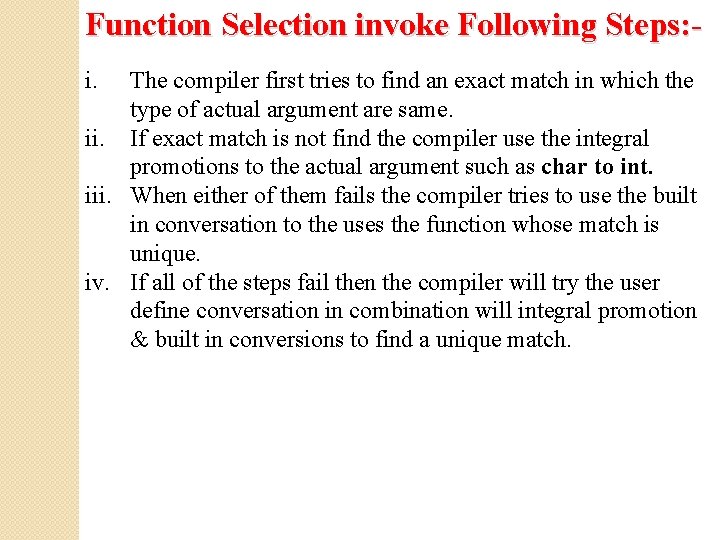
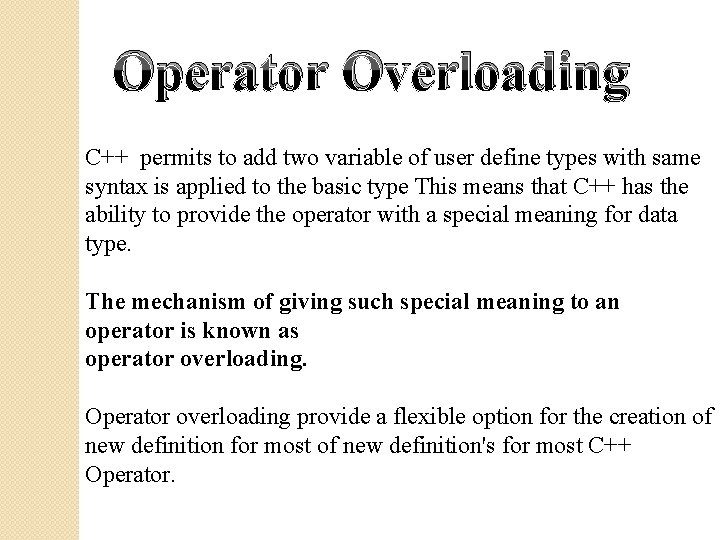
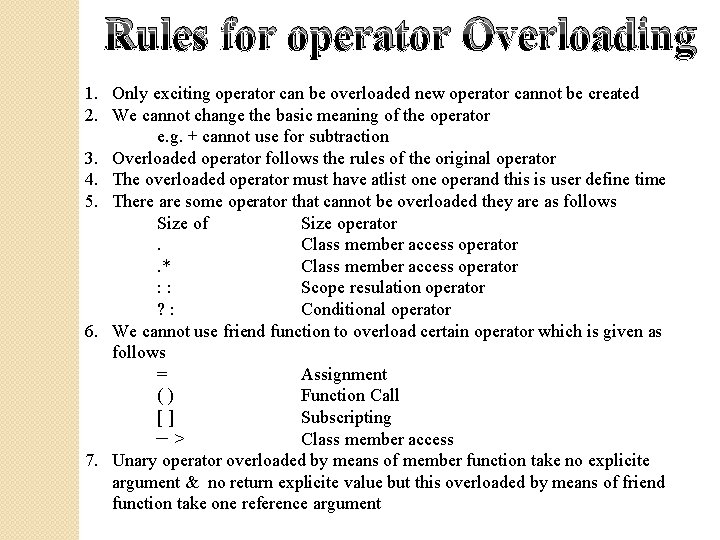
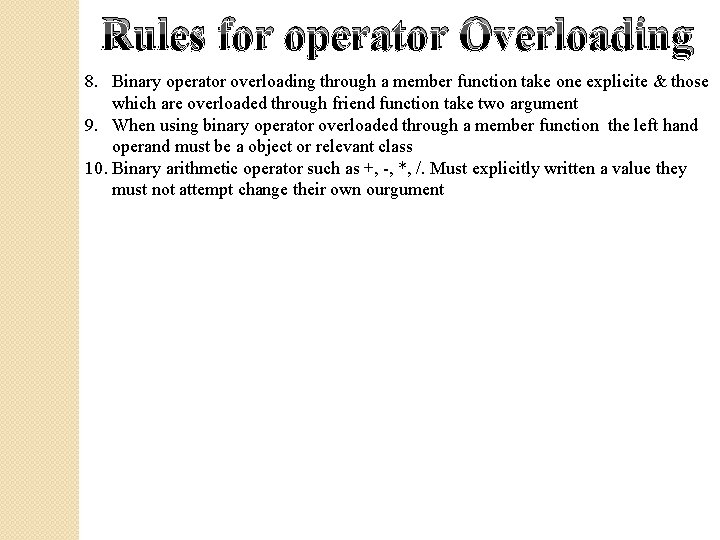
- Slides: 13
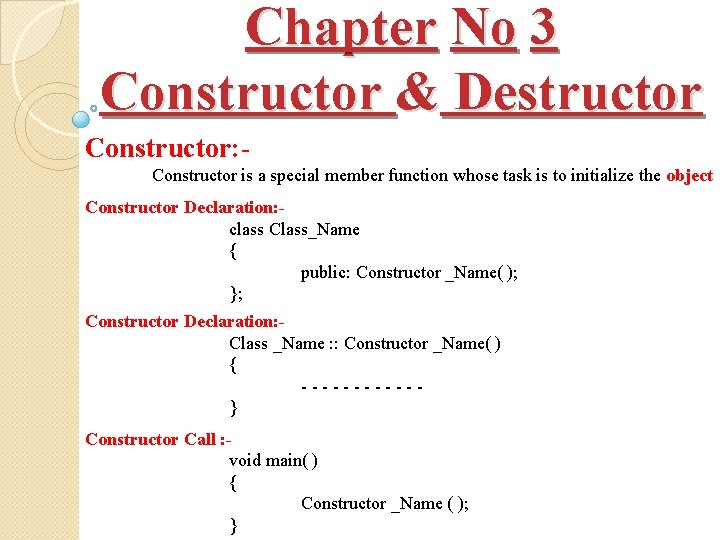
Chapter No 3 Constructor & Destructor Constructor: Constructor is a special member function whose task is to initialize the object Constructor Declaration: class Class_Name { public: Constructor _Name( ); }; Constructor Declaration: Class _Name : : Constructor _Name( ) { ------} Constructor Call : void main( ) { Constructor _Name ( ); }
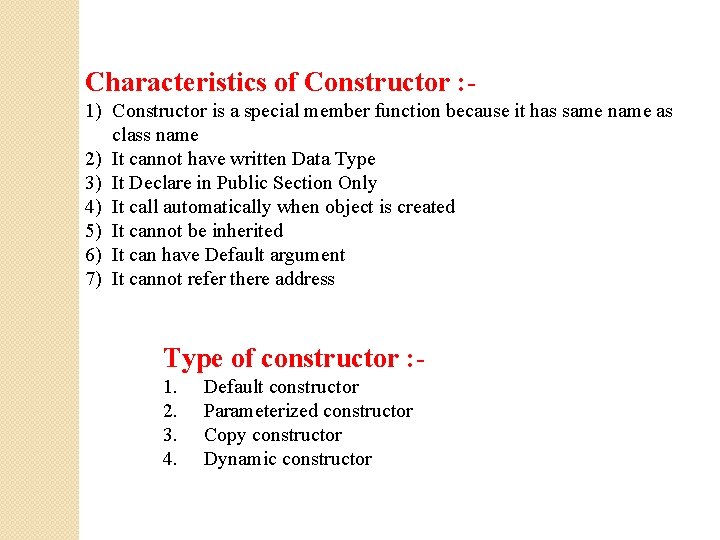
Characteristics of Constructor : 1) Constructor is a special member function because it has same name as class name 2) It cannot have written Data Type 3) It Declare in Public Section Only 4) It call automatically when object is created 5) It cannot be inherited 6) It can have Default argument 7) It cannot refer there address Type of constructor : 1. 2. 3. 4. Default constructor Parameterized constructor Copy constructor Dynamic constructor
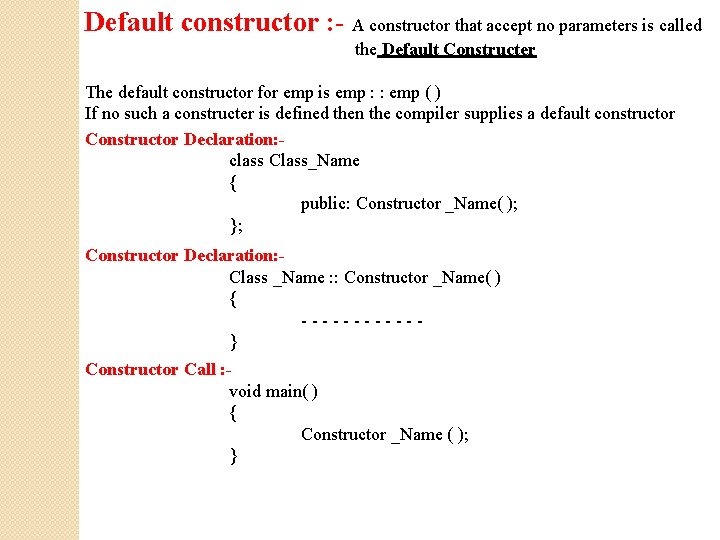
Default constructor : - A constructor that accept no parameters is called the Default Constructer The default constructor for emp is emp : : emp ( ) If no such a constructer is defined then the compiler supplies a default constructor Constructor Declaration: class Class_Name { public: Constructor _Name( ); }; Constructor Declaration: Class _Name : : Constructor _Name( ) { ------} Constructor Call : void main( ) { Constructor _Name ( ); }
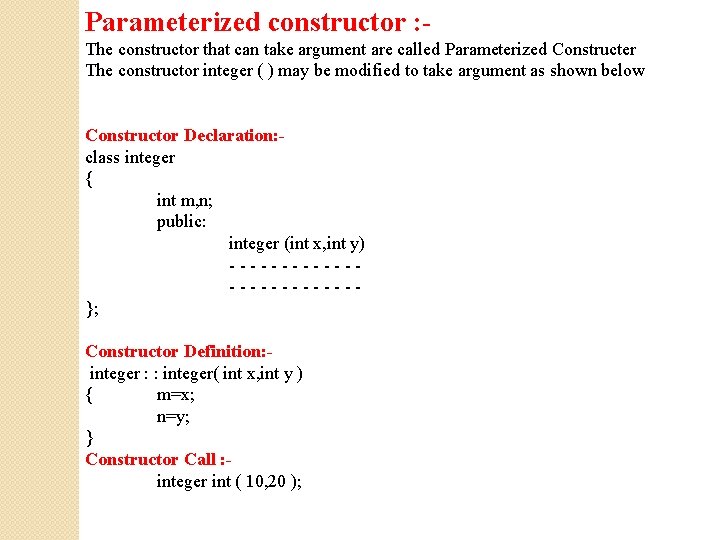
Parameterized constructor : The constructor that can take argument are called Parameterized Constructer The constructor integer ( ) may be modified to take argument as shown below Constructor Declaration: class integer { int m, n; public: integer (int x, int y) ------------}; Constructor Definition: integer : : integer( int x, int y ) { m=x; n=y; } Constructor Call : integer int ( 10, 20 );
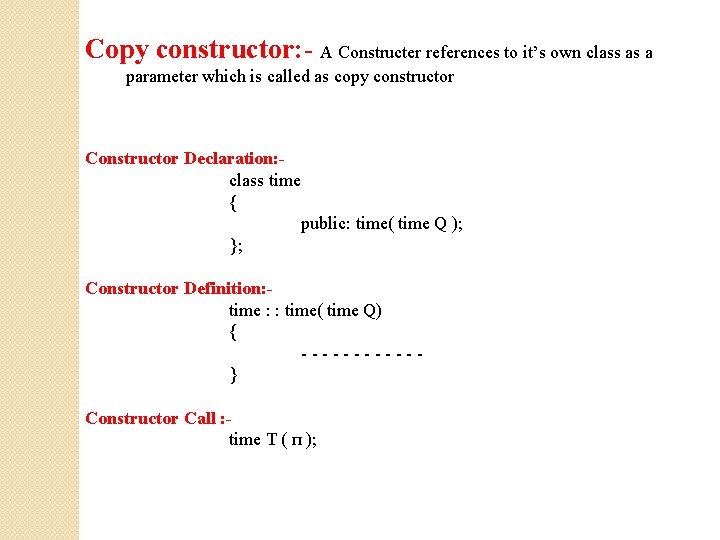
Copy constructor: - A Constructer references to it’s own class as a parameter which is called as copy constructor Constructor Declaration: class time { public: time( time Q ); }; Constructor Definition: time : : time( time Q) { ------} Constructor Call : time T ( п );
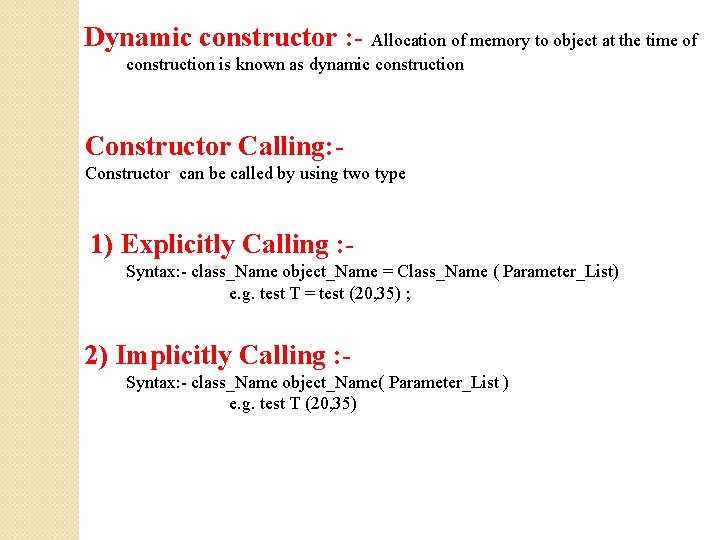
Dynamic constructor : - Allocation of memory to object at the time of construction is known as dynamic construction Constructor Calling: Constructor can be called by using two type 1) Explicitly Calling : Syntax: - class_Name object_Name = Class_Name ( Parameter_List) e. g. test T = test (20, 35) ; 2) Implicitly Calling : Syntax: - class_Name object_Name( Parameter_List ) e. g. test T (20, 35)
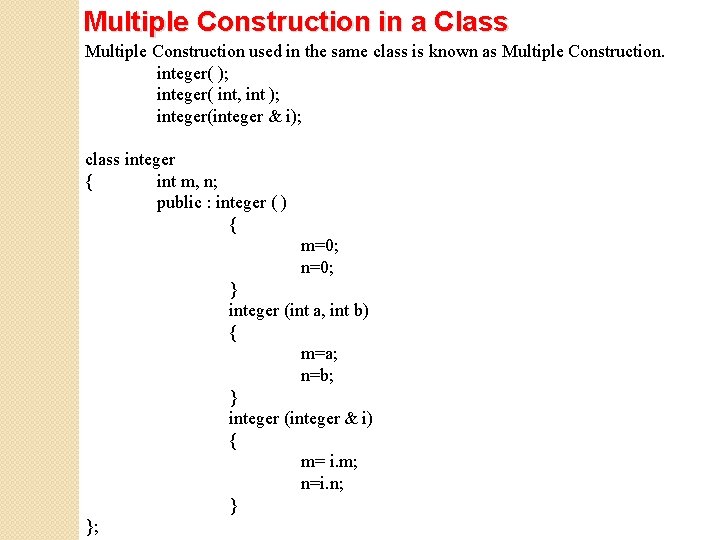
Multiple Construction in a Class Multiple Construction used in the same class is known as Multiple Construction. integer( ); integer( int, int ); integer(integer & i); class integer { int m, n; public : integer ( ) { m=0; n=0; } integer (int a, int b) { m=a; n=b; } integer (integer & i) { m= i. m; n=i. n; } };
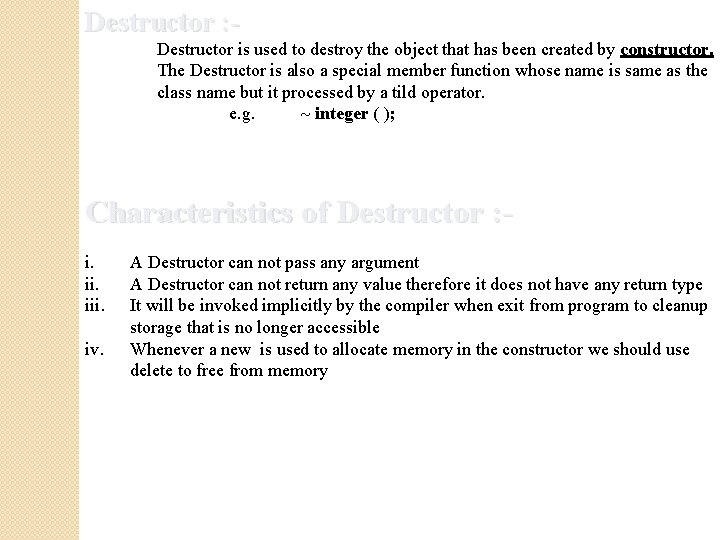
Destructor : Destructor is used to destroy the object that has been created by constructor. The Destructor is also a special member function whose name is same as the class name but it processed by a tild operator. e. g. ~ integer ( ); Characteristics of Destructor : i. iii. iv. A Destructor can not pass any argument A Destructor can not return any value therefore it does not have any return type It will be invoked implicitly by the compiler when exit from program to cleanup storage that is no longer accessible Whenever a new is used to allocate memory in the constructor we should use delete to free from memory
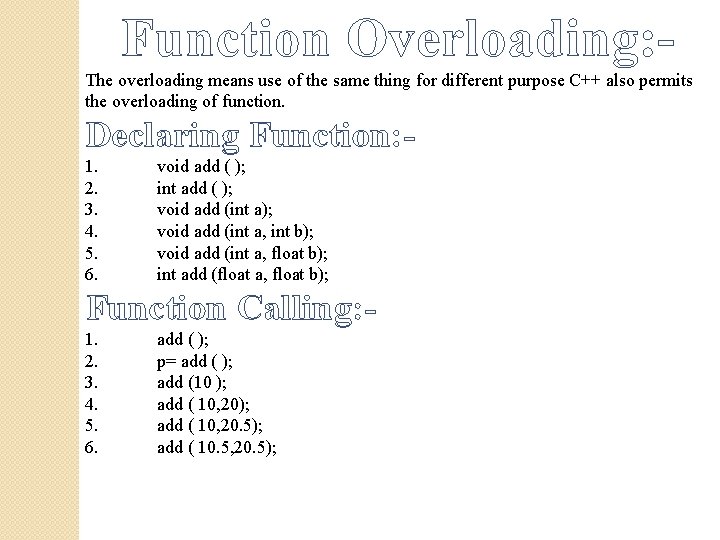
Function Overloading: The overloading means use of the same thing for different purpose C++ also permits the overloading of function. Declaring Function: 1. 2. 3. 4. 5. 6. void add ( ); int add ( ); void add (int a, int b); void add (int a, float b); int add (float a, float b); Function Calling: 1. 2. 3. 4. 5. 6. add ( ); p= add ( ); add (10 ); add ( 10, 20. 5); add ( 10. 5, 20. 5);
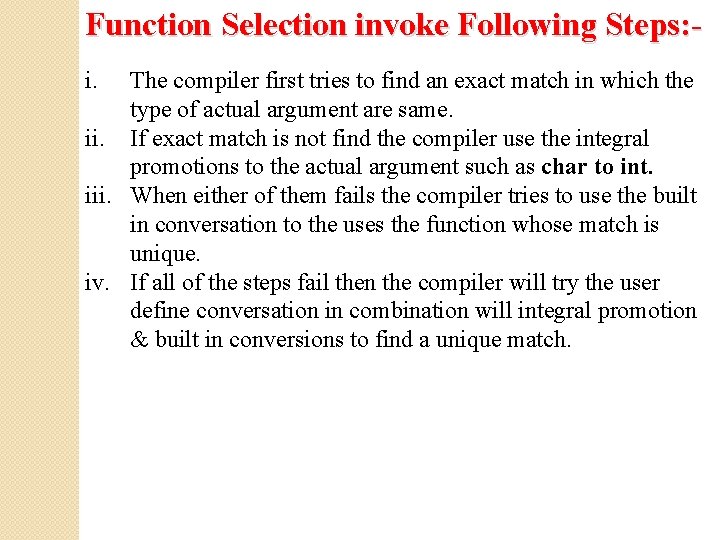
Function Selection invoke Following Steps: i. The compiler first tries to find an exact match in which the type of actual argument are same. ii. If exact match is not find the compiler use the integral promotions to the actual argument such as char to int. iii. When either of them fails the compiler tries to use the built in conversation to the uses the function whose match is unique. iv. If all of the steps fail then the compiler will try the user define conversation in combination will integral promotion & built in conversions to find a unique match.
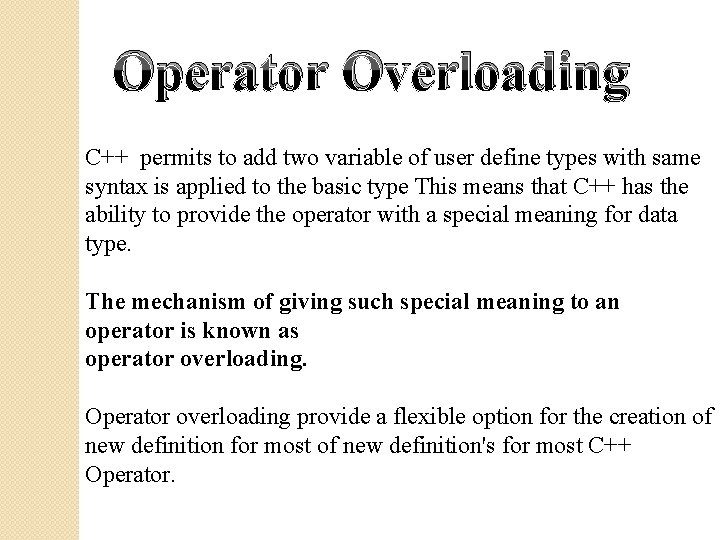
Operator Overloading C++ permits to add two variable of user define types with same syntax is applied to the basic type This means that C++ has the ability to provide the operator with a special meaning for data type. The mechanism of giving such special meaning to an operator is known as operator overloading. Operator overloading provide a flexible option for the creation of new definition for most of new definition's for most C++ Operator.
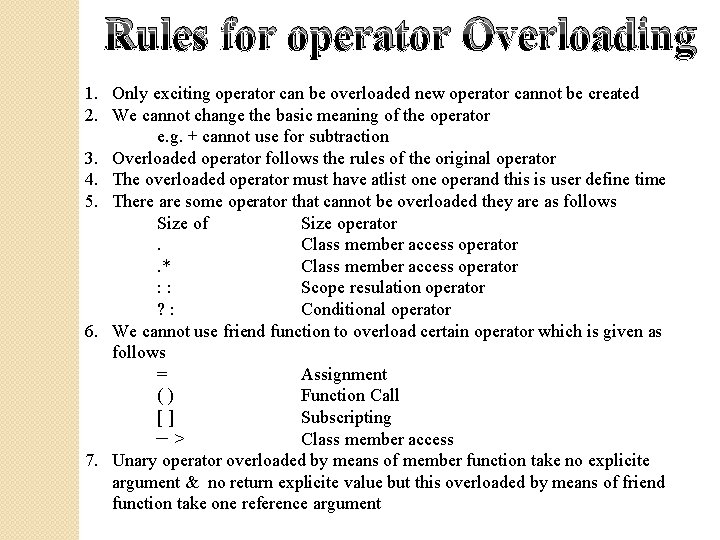
Rules for operator Overloading 1. Only exciting operator can be overloaded new operator cannot be created 2. We cannot change the basic meaning of the operator e. g. + cannot use for subtraction 3. Overloaded operator follows the rules of the original operator 4. The overloaded operator must have atlist one operand this is user define time 5. There are some operator that cannot be overloaded they are as follows Size of Size operator. Class member access operator. * Class member access operator : : Scope resulation operator ? : Conditional operator 6. We cannot use friend function to overload certain operator which is given as follows = Assignment () Function Call [] Subscripting > Class member access 7. Unary operator overloaded by means of member function take no explicite argument & no return explicite value but this overloaded by means of friend function take one reference argument
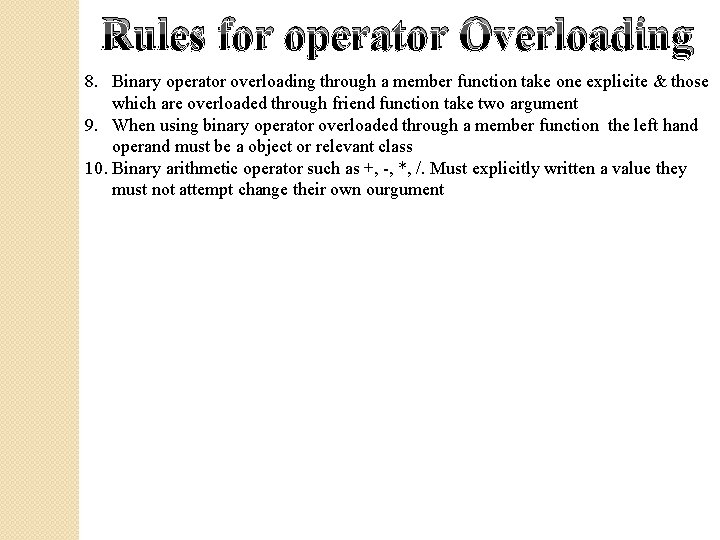
Rules for operator Overloading 8. Binary operator overloading through a member function take one explicite & those which are overloaded through friend function take two argument 9. When using binary operator overloaded through a member function the left hand operand must be a object or relevant class 10. Binary arithmetic operator such as +, -, *, /. Must explicitly written a value they must not attempt change their own ourgument
What are virtual functions in c++
Khan el destructor
Aspidiotus destructor
Peardak
C# struct destructor
Has virtual functions and accessible non-virtual destructor
Destructor blas de lezo
Marcus biel
Difference between default and parameterized constructor
Shallow copy constructor
Deep copy constructor c++
Characteristics of constructor
Characteristics of constructor
Types of constructor in c++