SESSION IV DESTRUCTOR What is a destructor It
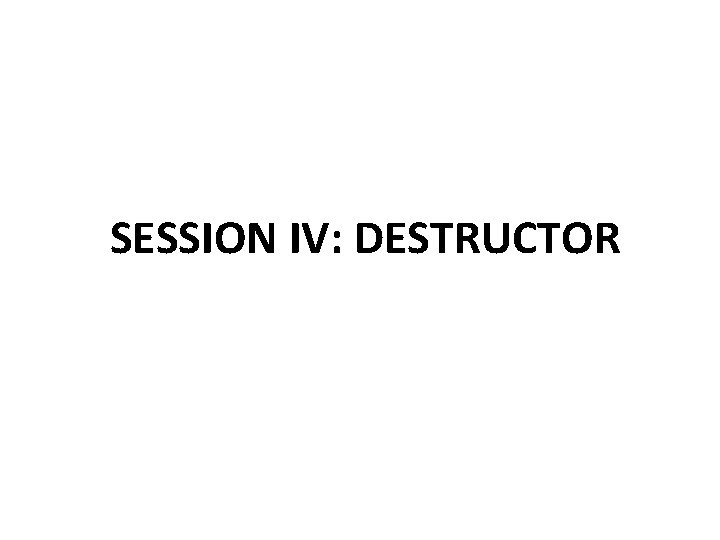
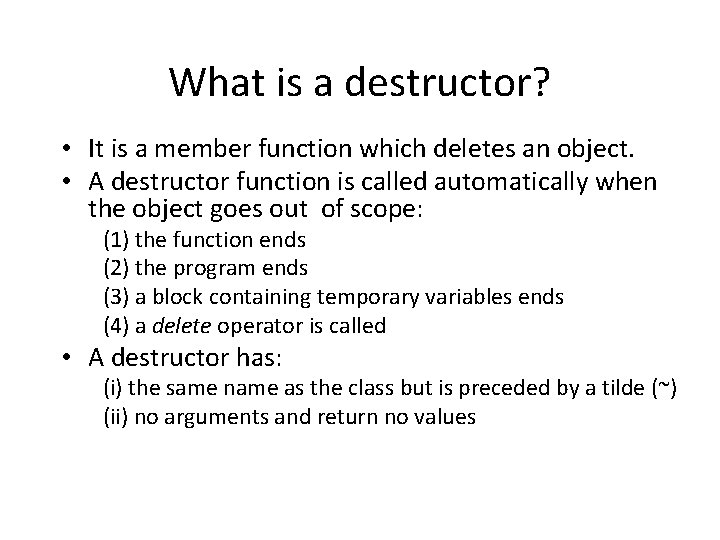
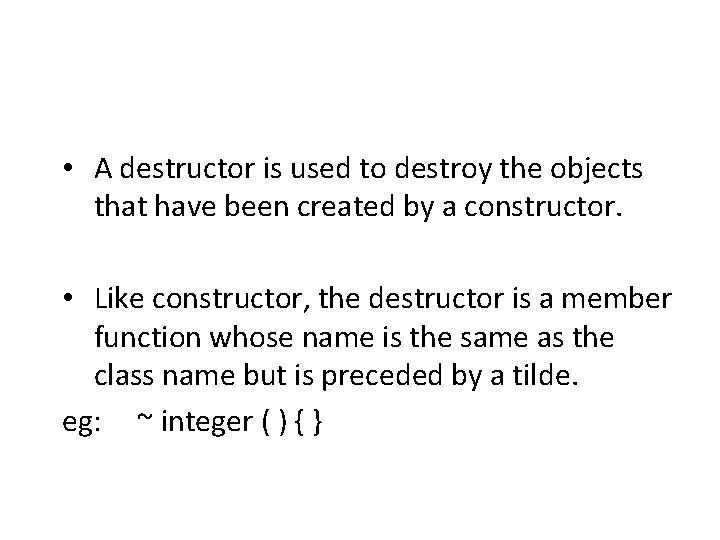
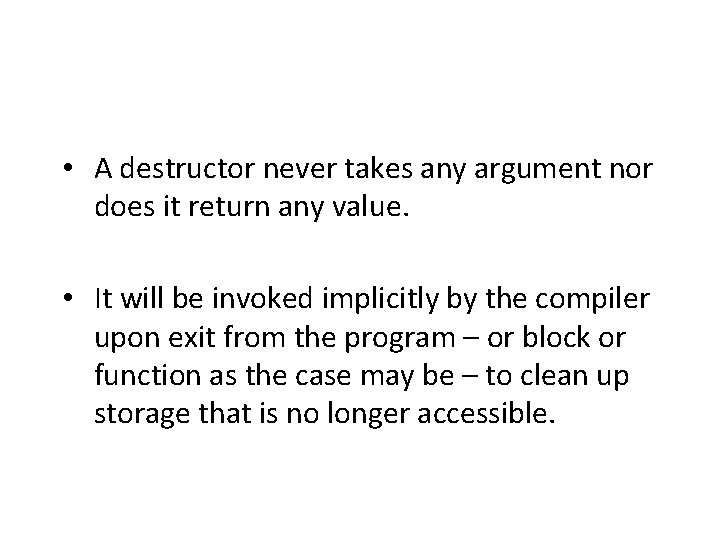
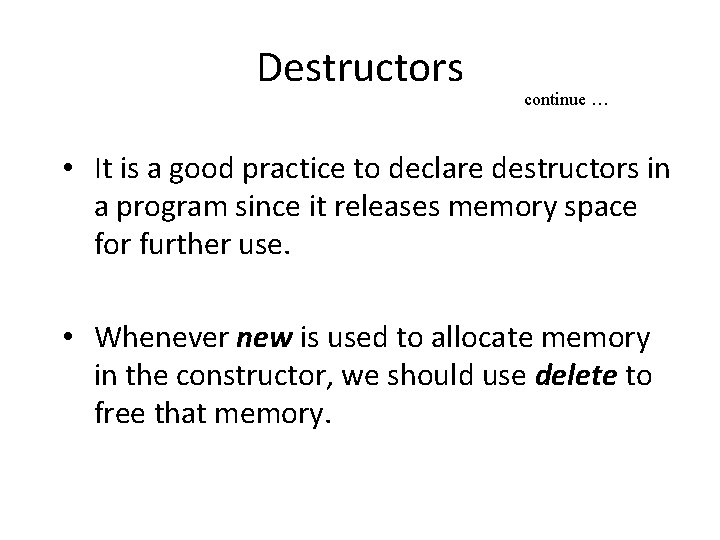
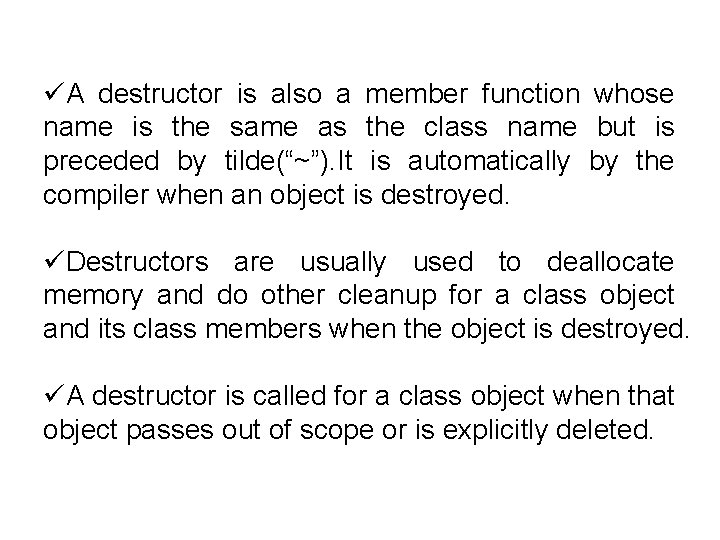
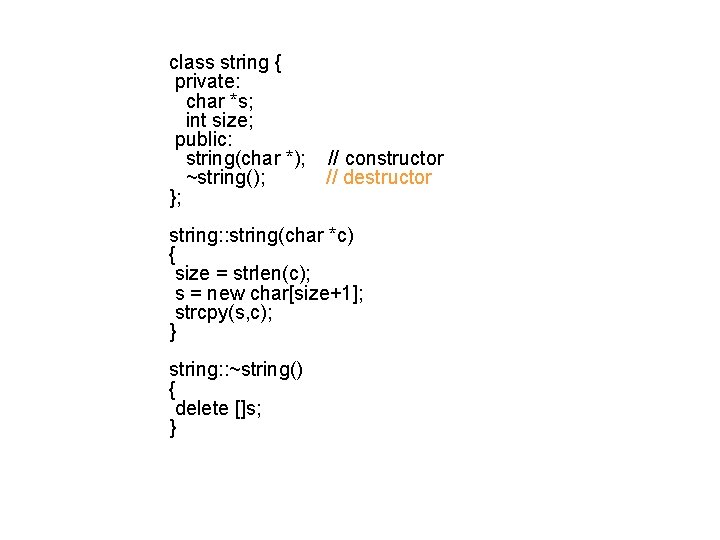
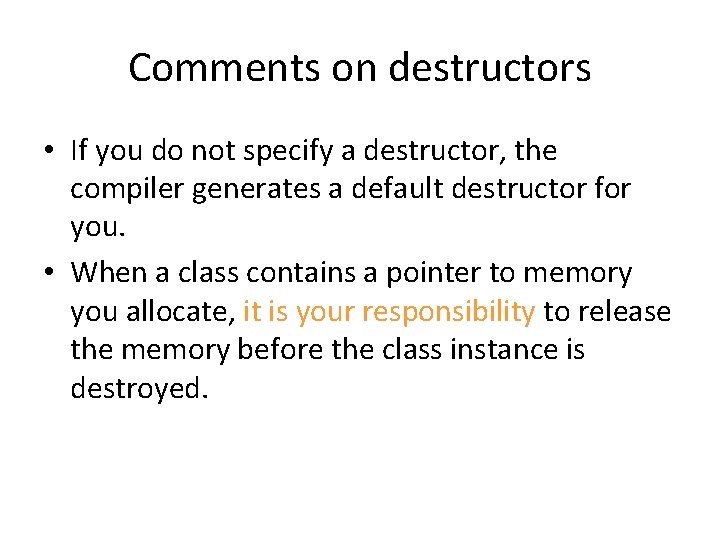
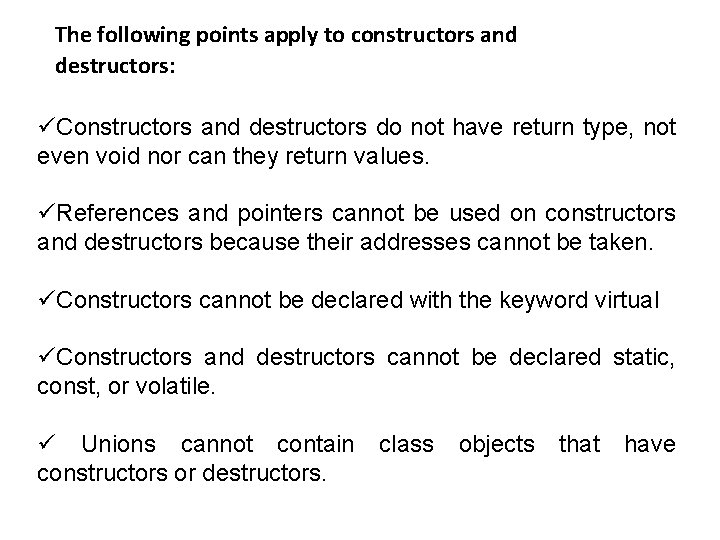
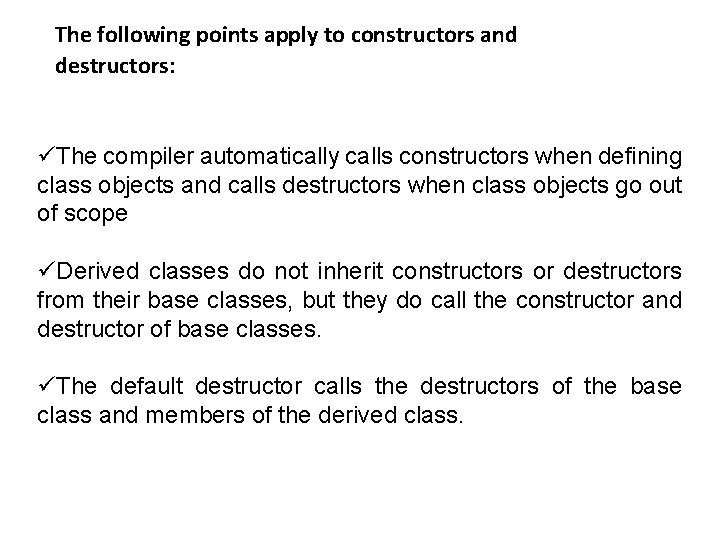
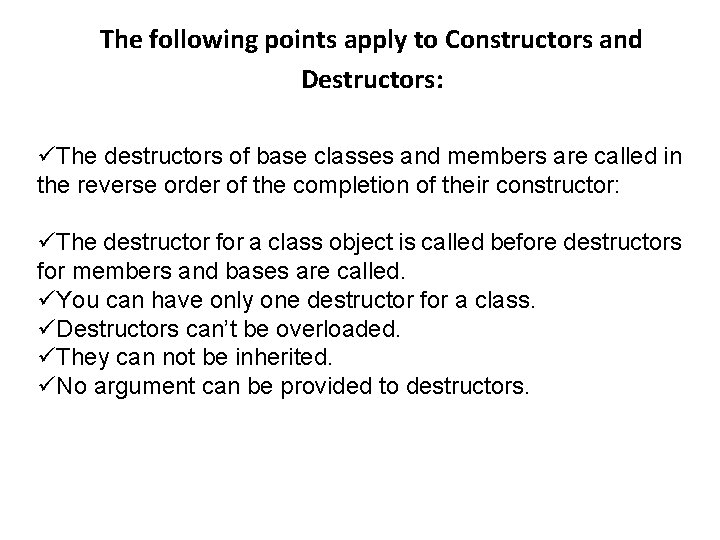
- Slides: 11
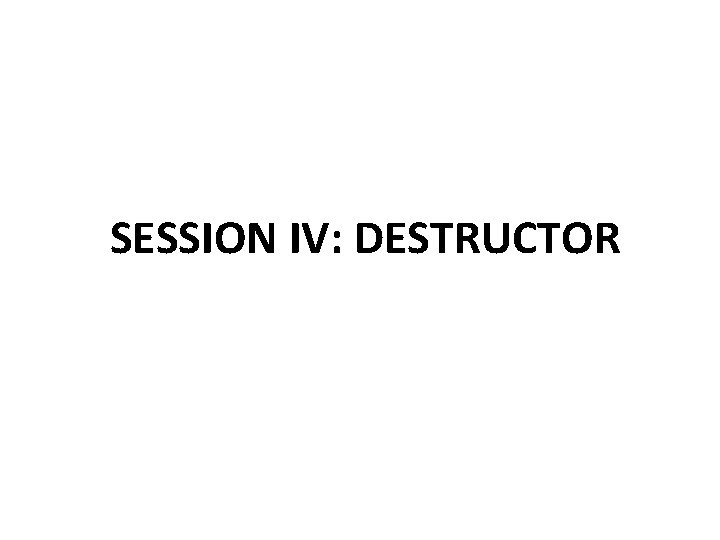
SESSION IV: DESTRUCTOR
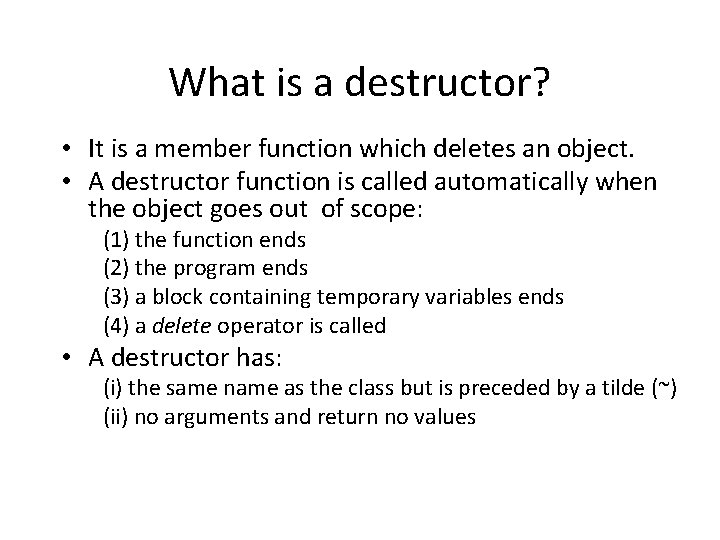
What is a destructor? • It is a member function which deletes an object. • A destructor function is called automatically when the object goes out of scope: (1) the function ends (2) the program ends (3) a block containing temporary variables ends (4) a delete operator is called • A destructor has: (i) the same name as the class but is preceded by a tilde (~) (ii) no arguments and return no values
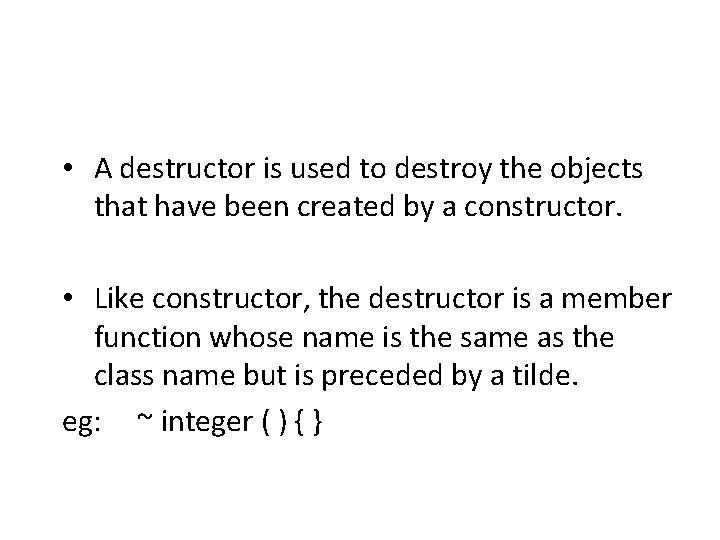
• A destructor is used to destroy the objects that have been created by a constructor. • Like constructor, the destructor is a member function whose name is the same as the class name but is preceded by a tilde. eg: ~ integer ( ) { }
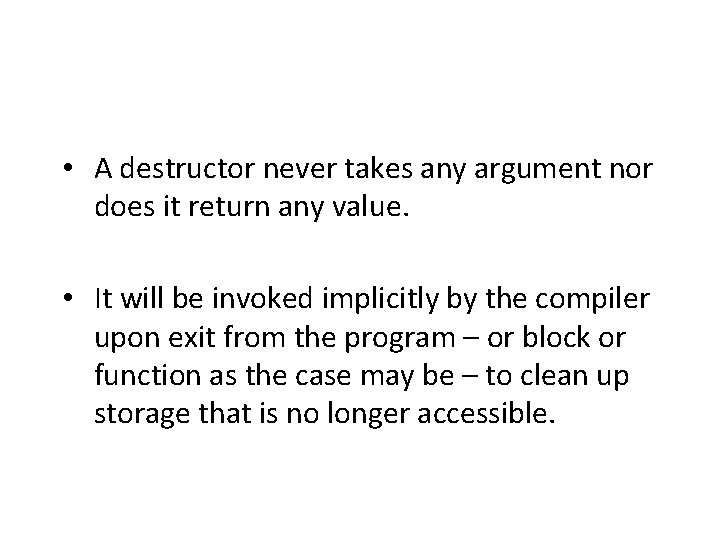
• A destructor never takes any argument nor does it return any value. • It will be invoked implicitly by the compiler upon exit from the program – or block or function as the case may be – to clean up storage that is no longer accessible.
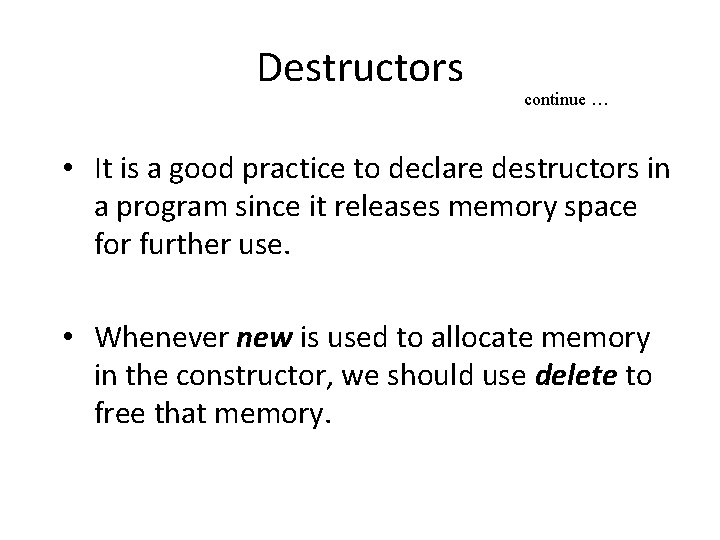
Destructors continue … • It is a good practice to declare destructors in a program since it releases memory space for further use. • Whenever new is used to allocate memory in the constructor, we should use delete to free that memory.
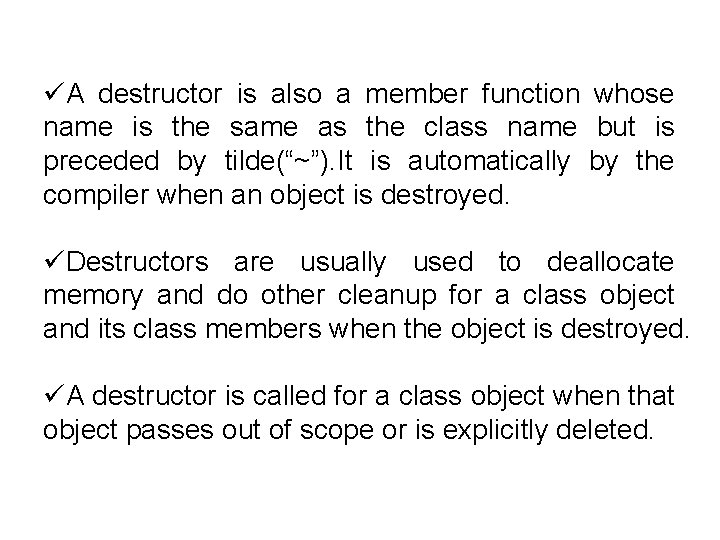
üA destructor is also a member function whose name is the same as the class name but is preceded by tilde(“~”). It is automatically by the compiler when an object is destroyed. üDestructors are usually used to deallocate memory and do other cleanup for a class object and its class members when the object is destroyed. üA destructor is called for a class object when that object passes out of scope or is explicitly deleted.
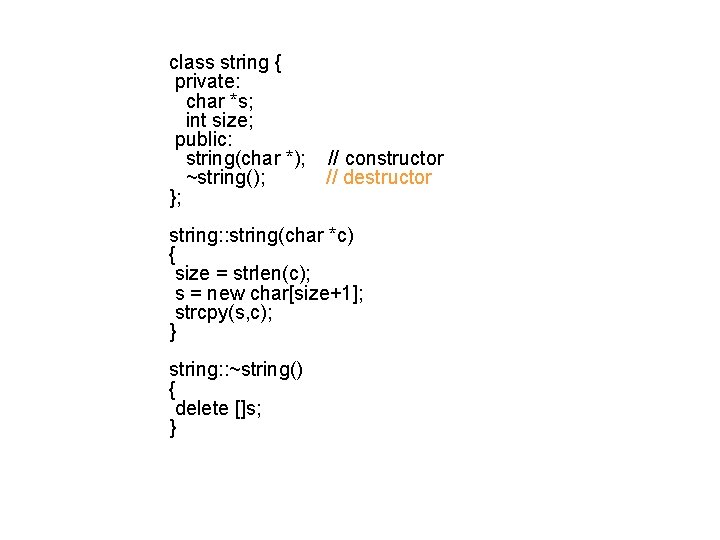
class string { private: char *s; int size; public: string(char *); ~string(); }; // constructor // destructor string: : string(char *c) { size = strlen(c); s = new char[size+1]; strcpy(s, c); } string: : ~string() { delete []s; }
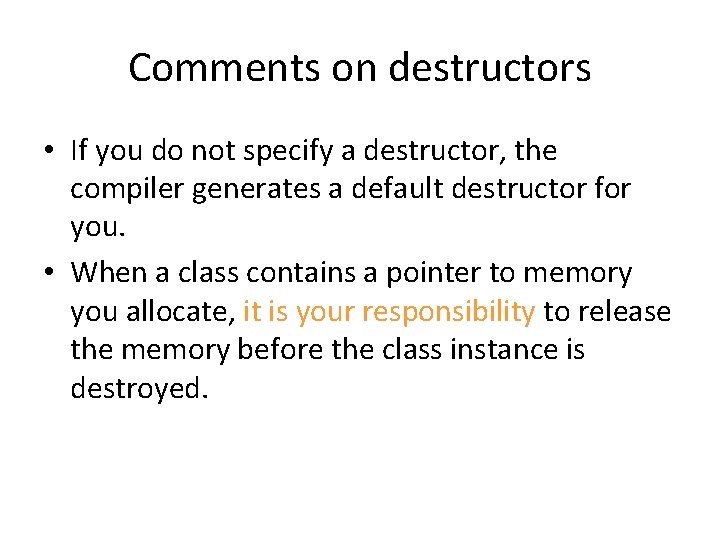
Comments on destructors • If you do not specify a destructor, the compiler generates a default destructor for you. • When a class contains a pointer to memory you allocate, it is your responsibility to release the memory before the class instance is destroyed.
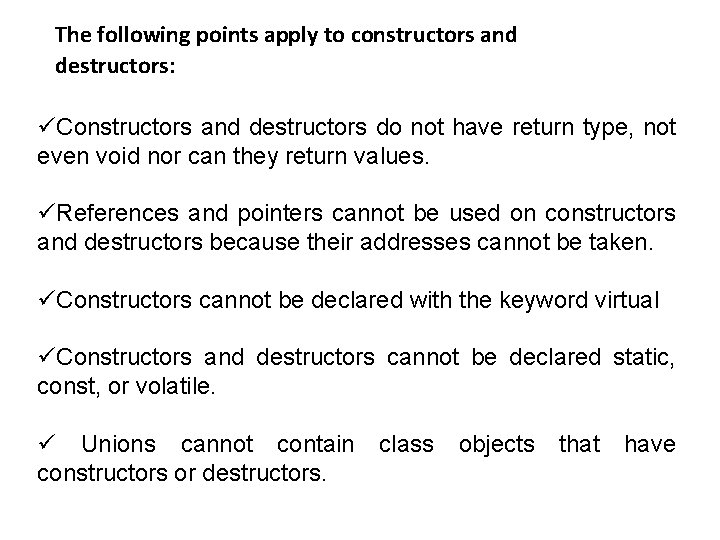
The following points apply to constructors and destructors: üConstructors and destructors do not have return type, not even void nor can they return values. üReferences and pointers cannot be used on constructors and destructors because their addresses cannot be taken. üConstructors cannot be declared with the keyword virtual üConstructors and destructors cannot be declared static, const, or volatile. ü Unions cannot contain constructors or destructors. class objects that have
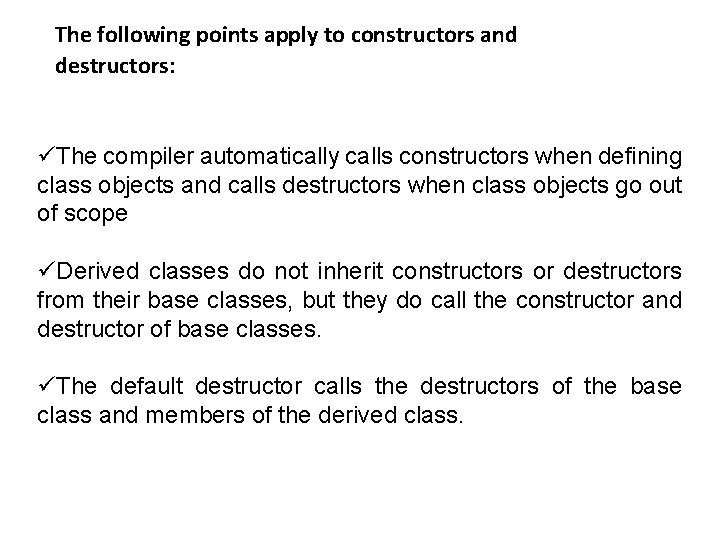
The following points apply to constructors and destructors: üThe compiler automatically calls constructors when defining class objects and calls destructors when class objects go out of scope üDerived classes do not inherit constructors or destructors from their base classes, but they do call the constructor and destructor of base classes. üThe default destructor calls the destructors of the base class and members of the derived class.
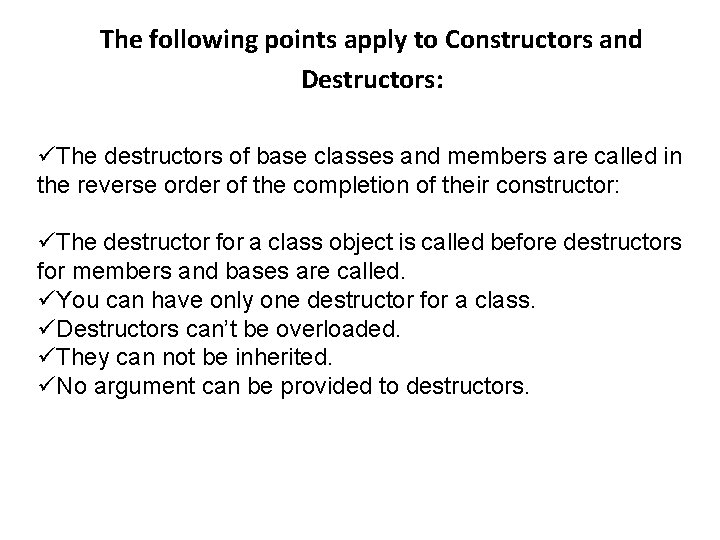
The following points apply to Constructors and Destructors: üThe destructors of base classes and members are called in the reverse order of the completion of their constructor: üThe destructor for a class object is called before destructors for members and bases are called. üYou can have only one destructor for a class. üDestructors can’t be overloaded. üThey can not be inherited. üNo argument can be provided to destructors.